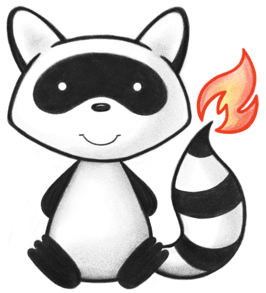
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum BindingStrengthEnum { 009 010 /** 011 * Display: <b>Required</b><br> 012 * Code Value: <b>required</b> 013 * 014 * To be conformant, instances of this element SHALL include a code from the specified value set. 015 */ 016 REQUIRED("required", "http://hl7.org/fhir/binding-strength"), 017 018 /** 019 * Display: <b>Extensible</b><br> 020 * Code Value: <b>extensible</b> 021 * 022 * To be conformant, instances of this element SHALL include a code from the specified value set if any of the codes within the value set can apply to the concept being communicated. If the valueset does not cover the concept (based on human review), alternate codings (or, data type allowing, text) may be included instead. 023 */ 024 EXTENSIBLE("extensible", "http://hl7.org/fhir/binding-strength"), 025 026 /** 027 * Display: <b>Preferred</b><br> 028 * Code Value: <b>preferred</b> 029 * 030 * Instances are encouraged to draw from the specified codes for interoperability purposes but are not required to do so to be considered conformant. 031 */ 032 PREFERRED("preferred", "http://hl7.org/fhir/binding-strength"), 033 034 /** 035 * Display: <b>Example</b><br> 036 * Code Value: <b>example</b> 037 * 038 * Instances are not expected or even encouraged to draw from the specified value set. The value set merely provides examples of the types of concepts intended to be included. 039 */ 040 EXAMPLE("example", "http://hl7.org/fhir/binding-strength"), 041 042 ; 043 044 /** 045 * Identifier for this Value Set: 046 * 047 */ 048 public static final String VALUESET_IDENTIFIER = ""; 049 050 /** 051 * Name for this Value Set: 052 * BindingStrength 053 */ 054 public static final String VALUESET_NAME = "BindingStrength"; 055 056 private static Map<String, BindingStrengthEnum> CODE_TO_ENUM = new HashMap<String, BindingStrengthEnum>(); 057 private static Map<String, Map<String, BindingStrengthEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, BindingStrengthEnum>>(); 058 059 private final String myCode; 060 private final String mySystem; 061 062 static { 063 for (BindingStrengthEnum next : BindingStrengthEnum.values()) { 064 CODE_TO_ENUM.put(next.getCode(), next); 065 066 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 067 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, BindingStrengthEnum>()); 068 } 069 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 070 } 071 } 072 073 /** 074 * Returns the code associated with this enumerated value 075 */ 076 public String getCode() { 077 return myCode; 078 } 079 080 /** 081 * Returns the code system associated with this enumerated value 082 */ 083 public String getSystem() { 084 return mySystem; 085 } 086 087 /** 088 * Returns the enumerated value associated with this code 089 */ 090 public static BindingStrengthEnum forCode(String theCode) { 091 BindingStrengthEnum retVal = CODE_TO_ENUM.get(theCode); 092 return retVal; 093 } 094 095 /** 096 * Converts codes to their respective enumerated values 097 */ 098 public static final IValueSetEnumBinder<BindingStrengthEnum> VALUESET_BINDER = new IValueSetEnumBinder<BindingStrengthEnum>() { 099 @Override 100 public String toCodeString(BindingStrengthEnum theEnum) { 101 return theEnum.getCode(); 102 } 103 104 @Override 105 public String toSystemString(BindingStrengthEnum theEnum) { 106 return theEnum.getSystem(); 107 } 108 109 @Override 110 public BindingStrengthEnum fromCodeString(String theCodeString) { 111 return CODE_TO_ENUM.get(theCodeString); 112 } 113 114 @Override 115 public BindingStrengthEnum fromCodeString(String theCodeString, String theSystemString) { 116 Map<String, BindingStrengthEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 117 if (map == null) { 118 return null; 119 } 120 return map.get(theCodeString); 121 } 122 123 }; 124 125 /** 126 * Constructor 127 */ 128 BindingStrengthEnum(String theCode, String theSystem) { 129 myCode = theCode; 130 mySystem = theSystem; 131 } 132 133 134}