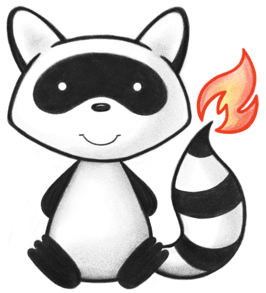
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum CarePlanRelationshipEnum { 009 010 /** 011 * Display: <b>Includes</b><br> 012 * Code Value: <b>includes</b> 013 * 014 * The referenced plan is considered to be part of this plan. 015 */ 016 INCLUDES("includes", "http://hl7.org/fhir/care-plan-relationship"), 017 018 /** 019 * Display: <b>Replaces</b><br> 020 * Code Value: <b>replaces</b> 021 * 022 * This plan takes the places of the referenced plan. 023 */ 024 REPLACES("replaces", "http://hl7.org/fhir/care-plan-relationship"), 025 026 /** 027 * Display: <b>Fulfills</b><br> 028 * Code Value: <b>fulfills</b> 029 * 030 * This plan provides details about how to perform activities defined at a higher level by the referenced plan. 031 */ 032 FULFILLS("fulfills", "http://hl7.org/fhir/care-plan-relationship"), 033 034 ; 035 036 /** 037 * Identifier for this Value Set: 038 * 039 */ 040 public static final String VALUESET_IDENTIFIER = ""; 041 042 /** 043 * Name for this Value Set: 044 * CarePlanRelationship 045 */ 046 public static final String VALUESET_NAME = "CarePlanRelationship"; 047 048 private static Map<String, CarePlanRelationshipEnum> CODE_TO_ENUM = new HashMap<String, CarePlanRelationshipEnum>(); 049 private static Map<String, Map<String, CarePlanRelationshipEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, CarePlanRelationshipEnum>>(); 050 051 private final String myCode; 052 private final String mySystem; 053 054 static { 055 for (CarePlanRelationshipEnum next : CarePlanRelationshipEnum.values()) { 056 CODE_TO_ENUM.put(next.getCode(), next); 057 058 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 059 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, CarePlanRelationshipEnum>()); 060 } 061 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 062 } 063 } 064 065 /** 066 * Returns the code associated with this enumerated value 067 */ 068 public String getCode() { 069 return myCode; 070 } 071 072 /** 073 * Returns the code system associated with this enumerated value 074 */ 075 public String getSystem() { 076 return mySystem; 077 } 078 079 /** 080 * Returns the enumerated value associated with this code 081 */ 082 public static CarePlanRelationshipEnum forCode(String theCode) { 083 CarePlanRelationshipEnum retVal = CODE_TO_ENUM.get(theCode); 084 return retVal; 085 } 086 087 /** 088 * Converts codes to their respective enumerated values 089 */ 090 public static final IValueSetEnumBinder<CarePlanRelationshipEnum> VALUESET_BINDER = new IValueSetEnumBinder<CarePlanRelationshipEnum>() { 091 @Override 092 public String toCodeString(CarePlanRelationshipEnum theEnum) { 093 return theEnum.getCode(); 094 } 095 096 @Override 097 public String toSystemString(CarePlanRelationshipEnum theEnum) { 098 return theEnum.getSystem(); 099 } 100 101 @Override 102 public CarePlanRelationshipEnum fromCodeString(String theCodeString) { 103 return CODE_TO_ENUM.get(theCodeString); 104 } 105 106 @Override 107 public CarePlanRelationshipEnum fromCodeString(String theCodeString, String theSystemString) { 108 Map<String, CarePlanRelationshipEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 109 if (map == null) { 110 return null; 111 } 112 return map.get(theCodeString); 113 } 114 115 }; 116 117 /** 118 * Constructor 119 */ 120 CarePlanRelationshipEnum(String theCode, String theSystem) { 121 myCode = theCode; 122 mySystem = theSystem; 123 } 124 125 126}