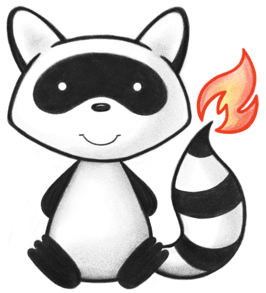
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum CommunicationRequestStatusEnum { 009 010 /** 011 * Display: <b>Proposed</b><br> 012 * Code Value: <b>proposed</b> 013 * 014 * The request has been proposed. 015 */ 016 PROPOSED("proposed", "http://hl7.org/fhir/communication-request-status"), 017 018 /** 019 * Display: <b>Planned</b><br> 020 * Code Value: <b>planned</b> 021 * 022 * The request has been planned. 023 */ 024 PLANNED("planned", "http://hl7.org/fhir/communication-request-status"), 025 026 /** 027 * Display: <b>Requested</b><br> 028 * Code Value: <b>requested</b> 029 * 030 * The request has been placed. 031 */ 032 REQUESTED("requested", "http://hl7.org/fhir/communication-request-status"), 033 034 /** 035 * Display: <b>Received</b><br> 036 * Code Value: <b>received</b> 037 * 038 * The receiving system has received the request but not yet decided whether it will be performed. 039 */ 040 RECEIVED("received", "http://hl7.org/fhir/communication-request-status"), 041 042 /** 043 * Display: <b>Accepted</b><br> 044 * Code Value: <b>accepted</b> 045 * 046 * The receiving system has accepted the order, but work has not yet commenced. 047 */ 048 ACCEPTED("accepted", "http://hl7.org/fhir/communication-request-status"), 049 050 /** 051 * Display: <b>In Progress</b><br> 052 * Code Value: <b>in-progress</b> 053 * 054 * The work to fulfill the order is happening. 055 */ 056 IN_PROGRESS("in-progress", "http://hl7.org/fhir/communication-request-status"), 057 058 /** 059 * Display: <b>Completed</b><br> 060 * Code Value: <b>completed</b> 061 * 062 * The work has been complete, the report(s) released, and no further work is planned. 063 */ 064 COMPLETED("completed", "http://hl7.org/fhir/communication-request-status"), 065 066 /** 067 * Display: <b>Suspended</b><br> 068 * Code Value: <b>suspended</b> 069 * 070 * The request has been held by originating system/user request. 071 */ 072 SUSPENDED("suspended", "http://hl7.org/fhir/communication-request-status"), 073 074 /** 075 * Display: <b>Rejected</b><br> 076 * Code Value: <b>rejected</b> 077 * 078 * The receiving system has declined to fulfill the request 079 */ 080 REJECTED("rejected", "http://hl7.org/fhir/communication-request-status"), 081 082 /** 083 * Display: <b>Failed</b><br> 084 * Code Value: <b>failed</b> 085 * 086 * The communication was attempted, but due to some procedural error, it could not be completed. 087 */ 088 FAILED("failed", "http://hl7.org/fhir/communication-request-status"), 089 090 ; 091 092 /** 093 * Identifier for this Value Set: 094 * 095 */ 096 public static final String VALUESET_IDENTIFIER = ""; 097 098 /** 099 * Name for this Value Set: 100 * CommunicationRequestStatus 101 */ 102 public static final String VALUESET_NAME = "CommunicationRequestStatus"; 103 104 private static Map<String, CommunicationRequestStatusEnum> CODE_TO_ENUM = new HashMap<String, CommunicationRequestStatusEnum>(); 105 private static Map<String, Map<String, CommunicationRequestStatusEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, CommunicationRequestStatusEnum>>(); 106 107 private final String myCode; 108 private final String mySystem; 109 110 static { 111 for (CommunicationRequestStatusEnum next : CommunicationRequestStatusEnum.values()) { 112 CODE_TO_ENUM.put(next.getCode(), next); 113 114 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 115 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, CommunicationRequestStatusEnum>()); 116 } 117 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 118 } 119 } 120 121 /** 122 * Returns the code associated with this enumerated value 123 */ 124 public String getCode() { 125 return myCode; 126 } 127 128 /** 129 * Returns the code system associated with this enumerated value 130 */ 131 public String getSystem() { 132 return mySystem; 133 } 134 135 /** 136 * Returns the enumerated value associated with this code 137 */ 138 public static CommunicationRequestStatusEnum forCode(String theCode) { 139 CommunicationRequestStatusEnum retVal = CODE_TO_ENUM.get(theCode); 140 return retVal; 141 } 142 143 /** 144 * Converts codes to their respective enumerated values 145 */ 146 public static final IValueSetEnumBinder<CommunicationRequestStatusEnum> VALUESET_BINDER = new IValueSetEnumBinder<CommunicationRequestStatusEnum>() { 147 @Override 148 public String toCodeString(CommunicationRequestStatusEnum theEnum) { 149 return theEnum.getCode(); 150 } 151 152 @Override 153 public String toSystemString(CommunicationRequestStatusEnum theEnum) { 154 return theEnum.getSystem(); 155 } 156 157 @Override 158 public CommunicationRequestStatusEnum fromCodeString(String theCodeString) { 159 return CODE_TO_ENUM.get(theCodeString); 160 } 161 162 @Override 163 public CommunicationRequestStatusEnum fromCodeString(String theCodeString, String theSystemString) { 164 Map<String, CommunicationRequestStatusEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 165 if (map == null) { 166 return null; 167 } 168 return map.get(theCodeString); 169 } 170 171 }; 172 173 /** 174 * Constructor 175 */ 176 CommunicationRequestStatusEnum(String theCode, String theSystem) { 177 myCode = theCode; 178 mySystem = theSystem; 179 } 180 181 182}