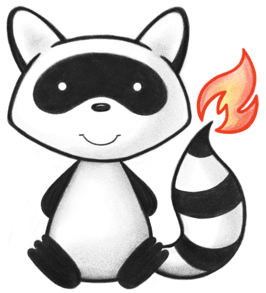
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum ConceptMapEquivalenceEnum { 009 010 /** 011 * Display: <b>Equivalent</b><br> 012 * Code Value: <b>equivalent</b> 013 * 014 * The definitions of the concepts mean the same thing (including when structural implications of meaning are considered) (i.e. extensionally identical). 015 */ 016 EQUIVALENT("equivalent", "http://hl7.org/fhir/concept-map-equivalence"), 017 018 /** 019 * Display: <b>Equal</b><br> 020 * Code Value: <b>equal</b> 021 * 022 * The definitions of the concepts are exactly the same (i.e. only grammatical differences) and structural implications of meaning are identical or irrelevant (i.e. intentionally identical). 023 */ 024 EQUAL("equal", "http://hl7.org/fhir/concept-map-equivalence"), 025 026 /** 027 * Display: <b>Wider</b><br> 028 * Code Value: <b>wider</b> 029 * 030 * The target mapping is wider in meaning than the source concept. 031 */ 032 WIDER("wider", "http://hl7.org/fhir/concept-map-equivalence"), 033 034 /** 035 * Display: <b>Subsumes</b><br> 036 * Code Value: <b>subsumes</b> 037 * 038 * The target mapping subsumes the meaning of the source concept (e.g. the source is-a target). 039 */ 040 SUBSUMES("subsumes", "http://hl7.org/fhir/concept-map-equivalence"), 041 042 /** 043 * Display: <b>Narrower</b><br> 044 * Code Value: <b>narrower</b> 045 * 046 * The target mapping is narrower in meaning that the source concept. The sense in which the mapping is narrower SHALL be described in the comments in this case, and applications should be careful when attempting to use these mappings operationally. 047 */ 048 NARROWER("narrower", "http://hl7.org/fhir/concept-map-equivalence"), 049 050 /** 051 * Display: <b>Specializes</b><br> 052 * Code Value: <b>specializes</b> 053 * 054 * The target mapping specializes the meaning of the source concept (e.g. the target is-a source). 055 */ 056 SPECIALIZES("specializes", "http://hl7.org/fhir/concept-map-equivalence"), 057 058 /** 059 * Display: <b>Inexact</b><br> 060 * Code Value: <b>inexact</b> 061 * 062 * The target mapping overlaps with the source concept, but both source and target cover additional meaning, or the definitions are imprecise and it is uncertain whether they have the same boundaries to their meaning. The sense in which the mapping is narrower SHALL be described in the comments in this case, and applications should be careful when attempting to use these mappings operationally. 063 */ 064 INEXACT("inexact", "http://hl7.org/fhir/concept-map-equivalence"), 065 066 /** 067 * Display: <b>Unmatched</b><br> 068 * Code Value: <b>unmatched</b> 069 * 070 * There is no match for this concept in the destination concept system. 071 */ 072 UNMATCHED("unmatched", "http://hl7.org/fhir/concept-map-equivalence"), 073 074 /** 075 * Display: <b>Disjoint</b><br> 076 * Code Value: <b>disjoint</b> 077 * 078 * This is an explicit assertion that there is no mapping between the source and target concept. 079 */ 080 DISJOINT("disjoint", "http://hl7.org/fhir/concept-map-equivalence"), 081 082 ; 083 084 /** 085 * Identifier for this Value Set: 086 * 087 */ 088 public static final String VALUESET_IDENTIFIER = ""; 089 090 /** 091 * Name for this Value Set: 092 * ConceptMapEquivalence 093 */ 094 public static final String VALUESET_NAME = "ConceptMapEquivalence"; 095 096 private static Map<String, ConceptMapEquivalenceEnum> CODE_TO_ENUM = new HashMap<String, ConceptMapEquivalenceEnum>(); 097 private static Map<String, Map<String, ConceptMapEquivalenceEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, ConceptMapEquivalenceEnum>>(); 098 099 private final String myCode; 100 private final String mySystem; 101 102 static { 103 for (ConceptMapEquivalenceEnum next : ConceptMapEquivalenceEnum.values()) { 104 CODE_TO_ENUM.put(next.getCode(), next); 105 106 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 107 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, ConceptMapEquivalenceEnum>()); 108 } 109 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 110 } 111 } 112 113 /** 114 * Returns the code associated with this enumerated value 115 */ 116 public String getCode() { 117 return myCode; 118 } 119 120 /** 121 * Returns the code system associated with this enumerated value 122 */ 123 public String getSystem() { 124 return mySystem; 125 } 126 127 /** 128 * Returns the enumerated value associated with this code 129 */ 130 public static ConceptMapEquivalenceEnum forCode(String theCode) { 131 ConceptMapEquivalenceEnum retVal = CODE_TO_ENUM.get(theCode); 132 return retVal; 133 } 134 135 /** 136 * Converts codes to their respective enumerated values 137 */ 138 public static final IValueSetEnumBinder<ConceptMapEquivalenceEnum> VALUESET_BINDER = new IValueSetEnumBinder<ConceptMapEquivalenceEnum>() { 139 @Override 140 public String toCodeString(ConceptMapEquivalenceEnum theEnum) { 141 return theEnum.getCode(); 142 } 143 144 @Override 145 public String toSystemString(ConceptMapEquivalenceEnum theEnum) { 146 return theEnum.getSystem(); 147 } 148 149 @Override 150 public ConceptMapEquivalenceEnum fromCodeString(String theCodeString) { 151 return CODE_TO_ENUM.get(theCodeString); 152 } 153 154 @Override 155 public ConceptMapEquivalenceEnum fromCodeString(String theCodeString, String theSystemString) { 156 Map<String, ConceptMapEquivalenceEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 157 if (map == null) { 158 return null; 159 } 160 return map.get(theCodeString); 161 } 162 163 }; 164 165 /** 166 * Constructor 167 */ 168 ConceptMapEquivalenceEnum(String theCode, String theSystem) { 169 myCode = theCode; 170 mySystem = theSystem; 171 } 172 173 174}