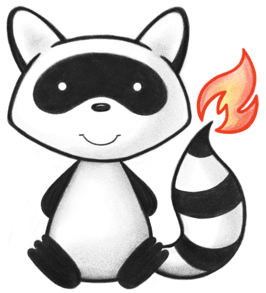
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum ContactPointSystemEnum { 009 010 /** 011 * Display: <b>Phone</b><br> 012 * Code Value: <b>phone</b> 013 * 014 * The value is a telephone number used for voice calls. Use of full international numbers starting with + is recommended to enable automatic dialing support but not required. 015 */ 016 PHONE("phone", "http://hl7.org/fhir/contact-point-system"), 017 018 /** 019 * Display: <b>Fax</b><br> 020 * Code Value: <b>fax</b> 021 * 022 * The value is a fax machine. Use of full international numbers starting with + is recommended to enable automatic dialing support but not required. 023 */ 024 FAX("fax", "http://hl7.org/fhir/contact-point-system"), 025 026 /** 027 * Display: <b>Email</b><br> 028 * Code Value: <b>email</b> 029 * 030 * The value is an email address. 031 */ 032 EMAIL("email", "http://hl7.org/fhir/contact-point-system"), 033 034 /** 035 * Display: <b>Pager</b><br> 036 * Code Value: <b>pager</b> 037 * 038 * The value is a pager number. These may be local pager numbers that are only usable on a particular pager system. 039 */ 040 PAGER("pager", "http://hl7.org/fhir/contact-point-system"), 041 042 /** 043 * Display: <b>URL</b><br> 044 * Code Value: <b>other</b> 045 * 046 * A contact that is not a phone, fax, or email address. The format of the value SHOULD be a URL. This is intended for various personal contacts including blogs, Twitter, Facebook, etc. Do not use for email addresses. If this is not a URL, then it will require human interpretation. 047 */ 048 URL("other", "http://hl7.org/fhir/contact-point-system"), 049 050 ; 051 052 /** 053 * Identifier for this Value Set: 054 * 055 */ 056 public static final String VALUESET_IDENTIFIER = ""; 057 058 /** 059 * Name for this Value Set: 060 * ContactPointSystem 061 */ 062 public static final String VALUESET_NAME = "ContactPointSystem"; 063 064 private static Map<String, ContactPointSystemEnum> CODE_TO_ENUM = new HashMap<String, ContactPointSystemEnum>(); 065 private static Map<String, Map<String, ContactPointSystemEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, ContactPointSystemEnum>>(); 066 067 private final String myCode; 068 private final String mySystem; 069 070 static { 071 for (ContactPointSystemEnum next : ContactPointSystemEnum.values()) { 072 CODE_TO_ENUM.put(next.getCode(), next); 073 074 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 075 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, ContactPointSystemEnum>()); 076 } 077 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 078 } 079 } 080 081 /** 082 * Returns the code associated with this enumerated value 083 */ 084 public String getCode() { 085 return myCode; 086 } 087 088 /** 089 * Returns the code system associated with this enumerated value 090 */ 091 public String getSystem() { 092 return mySystem; 093 } 094 095 /** 096 * Returns the enumerated value associated with this code 097 */ 098 public static ContactPointSystemEnum forCode(String theCode) { 099 ContactPointSystemEnum retVal = CODE_TO_ENUM.get(theCode); 100 return retVal; 101 } 102 103 /** 104 * Converts codes to their respective enumerated values 105 */ 106 public static final IValueSetEnumBinder<ContactPointSystemEnum> VALUESET_BINDER = new IValueSetEnumBinder<ContactPointSystemEnum>() { 107 @Override 108 public String toCodeString(ContactPointSystemEnum theEnum) { 109 return theEnum.getCode(); 110 } 111 112 @Override 113 public String toSystemString(ContactPointSystemEnum theEnum) { 114 return theEnum.getSystem(); 115 } 116 117 @Override 118 public ContactPointSystemEnum fromCodeString(String theCodeString) { 119 return CODE_TO_ENUM.get(theCodeString); 120 } 121 122 @Override 123 public ContactPointSystemEnum fromCodeString(String theCodeString, String theSystemString) { 124 Map<String, ContactPointSystemEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 125 if (map == null) { 126 return null; 127 } 128 return map.get(theCodeString); 129 } 130 131 }; 132 133 /** 134 * Constructor 135 */ 136 ContactPointSystemEnum(String theCode, String theSystem) { 137 myCode = theCode; 138 mySystem = theSystem; 139 } 140 141 142}