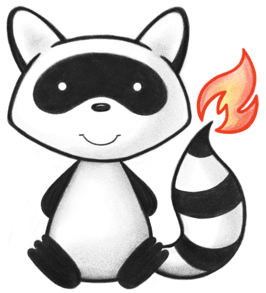
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum ContactPointUseEnum { 009 010 /** 011 * Display: <b>Home</b><br> 012 * Code Value: <b>home</b> 013 * 014 * A communication contact point at a home; attempted contacts for business purposes might intrude privacy and chances are one will contact family or other household members instead of the person one wishes to call. Typically used with urgent cases, or if no other contacts are available. 015 */ 016 HOME("home", "http://hl7.org/fhir/contact-point-use"), 017 018 /** 019 * Display: <b>Work</b><br> 020 * Code Value: <b>work</b> 021 * 022 * An office contact point. First choice for business related contacts during business hours. 023 */ 024 WORK("work", "http://hl7.org/fhir/contact-point-use"), 025 026 /** 027 * Display: <b>Temp</b><br> 028 * Code Value: <b>temp</b> 029 * 030 * A temporary contact point. The period can provide more detailed information. 031 */ 032 TEMP("temp", "http://hl7.org/fhir/contact-point-use"), 033 034 /** 035 * Display: <b>Old</b><br> 036 * Code Value: <b>old</b> 037 * 038 * This contact point is no longer in use (or was never correct, but retained for records). 039 */ 040 OLD("old", "http://hl7.org/fhir/contact-point-use"), 041 042 /** 043 * Display: <b>Mobile</b><br> 044 * Code Value: <b>mobile</b> 045 * 046 * A telecommunication device that moves and stays with its owner. May have characteristics of all other use codes, suitable for urgent matters, not the first choice for routine business. 047 */ 048 MOBILE("mobile", "http://hl7.org/fhir/contact-point-use"), 049 050 ; 051 052 /** 053 * Identifier for this Value Set: 054 * 055 */ 056 public static final String VALUESET_IDENTIFIER = ""; 057 058 /** 059 * Name for this Value Set: 060 * ContactPointUse 061 */ 062 public static final String VALUESET_NAME = "ContactPointUse"; 063 064 private static Map<String, ContactPointUseEnum> CODE_TO_ENUM = new HashMap<String, ContactPointUseEnum>(); 065 private static Map<String, Map<String, ContactPointUseEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, ContactPointUseEnum>>(); 066 067 private final String myCode; 068 private final String mySystem; 069 070 static { 071 for (ContactPointUseEnum next : ContactPointUseEnum.values()) { 072 CODE_TO_ENUM.put(next.getCode(), next); 073 074 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 075 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, ContactPointUseEnum>()); 076 } 077 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 078 } 079 } 080 081 /** 082 * Returns the code associated with this enumerated value 083 */ 084 public String getCode() { 085 return myCode; 086 } 087 088 /** 089 * Returns the code system associated with this enumerated value 090 */ 091 public String getSystem() { 092 return mySystem; 093 } 094 095 /** 096 * Returns the enumerated value associated with this code 097 */ 098 public static ContactPointUseEnum forCode(String theCode) { 099 ContactPointUseEnum retVal = CODE_TO_ENUM.get(theCode); 100 return retVal; 101 } 102 103 /** 104 * Converts codes to their respective enumerated values 105 */ 106 public static final IValueSetEnumBinder<ContactPointUseEnum> VALUESET_BINDER = new IValueSetEnumBinder<ContactPointUseEnum>() { 107 @Override 108 public String toCodeString(ContactPointUseEnum theEnum) { 109 return theEnum.getCode(); 110 } 111 112 @Override 113 public String toSystemString(ContactPointUseEnum theEnum) { 114 return theEnum.getSystem(); 115 } 116 117 @Override 118 public ContactPointUseEnum fromCodeString(String theCodeString) { 119 return CODE_TO_ENUM.get(theCodeString); 120 } 121 122 @Override 123 public ContactPointUseEnum fromCodeString(String theCodeString, String theSystemString) { 124 Map<String, ContactPointUseEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 125 if (map == null) { 126 return null; 127 } 128 return map.get(theCodeString); 129 } 130 131 }; 132 133 /** 134 * Constructor 135 */ 136 ContactPointUseEnum(String theCode, String theSystem) { 137 myCode = theCode; 138 mySystem = theSystem; 139 } 140 141 142}