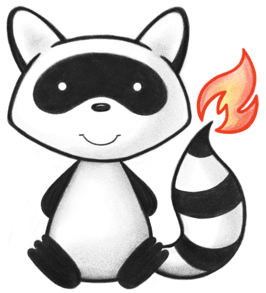
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum DeviceMetricColorEnum { 009 010 /** 011 * Display: <b>Color Black</b><br> 012 * Code Value: <b>black</b> 013 * 014 * Color for representation - black. 015 */ 016 COLOR_BLACK("black", "http://hl7.org/fhir/metric-color"), 017 018 /** 019 * Display: <b>Color Red</b><br> 020 * Code Value: <b>red</b> 021 * 022 * Color for representation - red. 023 */ 024 COLOR_RED("red", "http://hl7.org/fhir/metric-color"), 025 026 /** 027 * Display: <b>Color Green</b><br> 028 * Code Value: <b>green</b> 029 * 030 * Color for representation - green. 031 */ 032 COLOR_GREEN("green", "http://hl7.org/fhir/metric-color"), 033 034 /** 035 * Display: <b>Color Yellow</b><br> 036 * Code Value: <b>yellow</b> 037 * 038 * Color for representation - yellow. 039 */ 040 COLOR_YELLOW("yellow", "http://hl7.org/fhir/metric-color"), 041 042 /** 043 * Display: <b>Color Blue</b><br> 044 * Code Value: <b>blue</b> 045 * 046 * Color for representation - blue. 047 */ 048 COLOR_BLUE("blue", "http://hl7.org/fhir/metric-color"), 049 050 /** 051 * Display: <b>Color Magenta</b><br> 052 * Code Value: <b>magenta</b> 053 * 054 * Color for representation - magenta. 055 */ 056 COLOR_MAGENTA("magenta", "http://hl7.org/fhir/metric-color"), 057 058 /** 059 * Display: <b>Color Cyan</b><br> 060 * Code Value: <b>cyan</b> 061 * 062 * Color for representation - cyan. 063 */ 064 COLOR_CYAN("cyan", "http://hl7.org/fhir/metric-color"), 065 066 /** 067 * Display: <b>Color White</b><br> 068 * Code Value: <b>white</b> 069 * 070 * Color for representation - white. 071 */ 072 COLOR_WHITE("white", "http://hl7.org/fhir/metric-color"), 073 074 ; 075 076 /** 077 * Identifier for this Value Set: 078 * 079 */ 080 public static final String VALUESET_IDENTIFIER = ""; 081 082 /** 083 * Name for this Value Set: 084 * DeviceMetricColor 085 */ 086 public static final String VALUESET_NAME = "DeviceMetricColor"; 087 088 private static Map<String, DeviceMetricColorEnum> CODE_TO_ENUM = new HashMap<String, DeviceMetricColorEnum>(); 089 private static Map<String, Map<String, DeviceMetricColorEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, DeviceMetricColorEnum>>(); 090 091 private final String myCode; 092 private final String mySystem; 093 094 static { 095 for (DeviceMetricColorEnum next : DeviceMetricColorEnum.values()) { 096 CODE_TO_ENUM.put(next.getCode(), next); 097 098 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 099 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, DeviceMetricColorEnum>()); 100 } 101 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 102 } 103 } 104 105 /** 106 * Returns the code associated with this enumerated value 107 */ 108 public String getCode() { 109 return myCode; 110 } 111 112 /** 113 * Returns the code system associated with this enumerated value 114 */ 115 public String getSystem() { 116 return mySystem; 117 } 118 119 /** 120 * Returns the enumerated value associated with this code 121 */ 122 public static DeviceMetricColorEnum forCode(String theCode) { 123 DeviceMetricColorEnum retVal = CODE_TO_ENUM.get(theCode); 124 return retVal; 125 } 126 127 /** 128 * Converts codes to their respective enumerated values 129 */ 130 public static final IValueSetEnumBinder<DeviceMetricColorEnum> VALUESET_BINDER = new IValueSetEnumBinder<DeviceMetricColorEnum>() { 131 @Override 132 public String toCodeString(DeviceMetricColorEnum theEnum) { 133 return theEnum.getCode(); 134 } 135 136 @Override 137 public String toSystemString(DeviceMetricColorEnum theEnum) { 138 return theEnum.getSystem(); 139 } 140 141 @Override 142 public DeviceMetricColorEnum fromCodeString(String theCodeString) { 143 return CODE_TO_ENUM.get(theCodeString); 144 } 145 146 @Override 147 public DeviceMetricColorEnum fromCodeString(String theCodeString, String theSystemString) { 148 Map<String, DeviceMetricColorEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 149 if (map == null) { 150 return null; 151 } 152 return map.get(theCodeString); 153 } 154 155 }; 156 157 /** 158 * Constructor 159 */ 160 DeviceMetricColorEnum(String theCode, String theSystem) { 161 myCode = theCode; 162 mySystem = theSystem; 163 } 164 165 166}