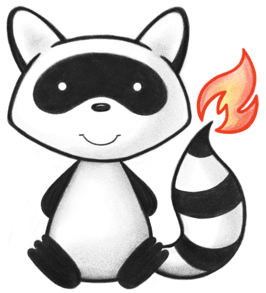
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum DiagnosticOrderStatusEnum { 009 010 /** 011 * Display: <b>Proposed</b><br> 012 * Code Value: <b>proposed</b> 013 * 014 * The request has been proposed. 015 */ 016 PROPOSED("proposed", "http://hl7.org/fhir/diagnostic-order-status"), 017 018 /** 019 * Display: <b>Draft</b><br> 020 * Code Value: <b>draft</b> 021 * 022 * The request is in preliminary form prior to being sent. 023 */ 024 DRAFT("draft", "http://hl7.org/fhir/diagnostic-order-status"), 025 026 /** 027 * Display: <b>Planned</b><br> 028 * Code Value: <b>planned</b> 029 * 030 * The request has been planned. 031 */ 032 PLANNED("planned", "http://hl7.org/fhir/diagnostic-order-status"), 033 034 /** 035 * Display: <b>Requested</b><br> 036 * Code Value: <b>requested</b> 037 * 038 * The request has been placed. 039 */ 040 REQUESTED("requested", "http://hl7.org/fhir/diagnostic-order-status"), 041 042 /** 043 * Display: <b>Received</b><br> 044 * Code Value: <b>received</b> 045 * 046 * The receiving system has received the order, but not yet decided whether it will be performed. 047 */ 048 RECEIVED("received", "http://hl7.org/fhir/diagnostic-order-status"), 049 050 /** 051 * Display: <b>Accepted</b><br> 052 * Code Value: <b>accepted</b> 053 * 054 * The receiving system has accepted the order, but work has not yet commenced. 055 */ 056 ACCEPTED("accepted", "http://hl7.org/fhir/diagnostic-order-status"), 057 058 /** 059 * Display: <b>In-Progress</b><br> 060 * Code Value: <b>in-progress</b> 061 * 062 * The work to fulfill the order is happening. 063 */ 064 IN_PROGRESS("in-progress", "http://hl7.org/fhir/diagnostic-order-status"), 065 066 /** 067 * Display: <b>Review</b><br> 068 * Code Value: <b>review</b> 069 * 070 * The work is complete, and the outcomes are being reviewed for approval. 071 */ 072 REVIEW("review", "http://hl7.org/fhir/diagnostic-order-status"), 073 074 /** 075 * Display: <b>Completed</b><br> 076 * Code Value: <b>completed</b> 077 * 078 * The work has been completed, the report(s) released, and no further work is planned. 079 */ 080 COMPLETED("completed", "http://hl7.org/fhir/diagnostic-order-status"), 081 082 /** 083 * Display: <b>Cancelled</b><br> 084 * Code Value: <b>cancelled</b> 085 * 086 * The request has been withdrawn. 087 */ 088 CANCELLED("cancelled", "http://hl7.org/fhir/diagnostic-order-status"), 089 090 /** 091 * Display: <b>Suspended</b><br> 092 * Code Value: <b>suspended</b> 093 * 094 * The request has been held by originating system/user request. 095 */ 096 SUSPENDED("suspended", "http://hl7.org/fhir/diagnostic-order-status"), 097 098 /** 099 * Display: <b>Rejected</b><br> 100 * Code Value: <b>rejected</b> 101 * 102 * The receiving system has declined to fulfill the request. 103 */ 104 REJECTED("rejected", "http://hl7.org/fhir/diagnostic-order-status"), 105 106 /** 107 * Display: <b>Failed</b><br> 108 * Code Value: <b>failed</b> 109 * 110 * The diagnostic investigation was attempted, but due to some procedural error, it could not be completed. 111 */ 112 FAILED("failed", "http://hl7.org/fhir/diagnostic-order-status"), 113 114 ; 115 116 /** 117 * Identifier for this Value Set: 118 * 119 */ 120 public static final String VALUESET_IDENTIFIER = ""; 121 122 /** 123 * Name for this Value Set: 124 * DiagnosticOrderStatus 125 */ 126 public static final String VALUESET_NAME = "DiagnosticOrderStatus"; 127 128 private static Map<String, DiagnosticOrderStatusEnum> CODE_TO_ENUM = new HashMap<String, DiagnosticOrderStatusEnum>(); 129 private static Map<String, Map<String, DiagnosticOrderStatusEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, DiagnosticOrderStatusEnum>>(); 130 131 private final String myCode; 132 private final String mySystem; 133 134 static { 135 for (DiagnosticOrderStatusEnum next : DiagnosticOrderStatusEnum.values()) { 136 CODE_TO_ENUM.put(next.getCode(), next); 137 138 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 139 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, DiagnosticOrderStatusEnum>()); 140 } 141 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 142 } 143 } 144 145 /** 146 * Returns the code associated with this enumerated value 147 */ 148 public String getCode() { 149 return myCode; 150 } 151 152 /** 153 * Returns the code system associated with this enumerated value 154 */ 155 public String getSystem() { 156 return mySystem; 157 } 158 159 /** 160 * Returns the enumerated value associated with this code 161 */ 162 public static DiagnosticOrderStatusEnum forCode(String theCode) { 163 DiagnosticOrderStatusEnum retVal = CODE_TO_ENUM.get(theCode); 164 return retVal; 165 } 166 167 /** 168 * Converts codes to their respective enumerated values 169 */ 170 public static final IValueSetEnumBinder<DiagnosticOrderStatusEnum> VALUESET_BINDER = new IValueSetEnumBinder<DiagnosticOrderStatusEnum>() { 171 @Override 172 public String toCodeString(DiagnosticOrderStatusEnum theEnum) { 173 return theEnum.getCode(); 174 } 175 176 @Override 177 public String toSystemString(DiagnosticOrderStatusEnum theEnum) { 178 return theEnum.getSystem(); 179 } 180 181 @Override 182 public DiagnosticOrderStatusEnum fromCodeString(String theCodeString) { 183 return CODE_TO_ENUM.get(theCodeString); 184 } 185 186 @Override 187 public DiagnosticOrderStatusEnum fromCodeString(String theCodeString, String theSystemString) { 188 Map<String, DiagnosticOrderStatusEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 189 if (map == null) { 190 return null; 191 } 192 return map.get(theCodeString); 193 } 194 195 }; 196 197 /** 198 * Constructor 199 */ 200 DiagnosticOrderStatusEnum(String theCode, String theSystem) { 201 myCode = theCode; 202 mySystem = theSystem; 203 } 204 205 206}