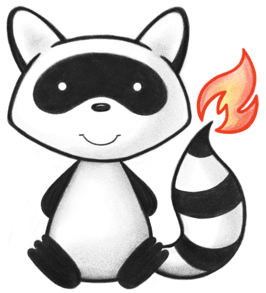
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum EncounterClassEnum { 009 010 /** 011 * Display: <b>Inpatient</b><br> 012 * Code Value: <b>inpatient</b> 013 * 014 * An encounter during which the patient is hospitalized and stays overnight. 015 */ 016 INPATIENT("inpatient", "http://hl7.org/fhir/encounter-class"), 017 018 /** 019 * Display: <b>Outpatient</b><br> 020 * Code Value: <b>outpatient</b> 021 * 022 * An encounter during which the patient is not hospitalized overnight. 023 */ 024 OUTPATIENT("outpatient", "http://hl7.org/fhir/encounter-class"), 025 026 /** 027 * Display: <b>Ambulatory</b><br> 028 * Code Value: <b>ambulatory</b> 029 * 030 * An encounter where the patient visits the practitioner in his/her office, e.g. a G.P. visit. 031 */ 032 AMBULATORY("ambulatory", "http://hl7.org/fhir/encounter-class"), 033 034 /** 035 * Display: <b>Emergency</b><br> 036 * Code Value: <b>emergency</b> 037 * 038 * An encounter in the Emergency Care Department. 039 */ 040 EMERGENCY("emergency", "http://hl7.org/fhir/encounter-class"), 041 042 /** 043 * Display: <b>Home</b><br> 044 * Code Value: <b>home</b> 045 * 046 * An encounter where the practitioner visits the patient at his/her home. 047 */ 048 HOME("home", "http://hl7.org/fhir/encounter-class"), 049 050 /** 051 * Display: <b>Field</b><br> 052 * Code Value: <b>field</b> 053 * 054 * An encounter taking place outside the regular environment for giving care. 055 */ 056 FIELD("field", "http://hl7.org/fhir/encounter-class"), 057 058 /** 059 * Display: <b>Daytime</b><br> 060 * Code Value: <b>daytime</b> 061 * 062 * An encounter where the patient needs more prolonged treatment or investigations than outpatients, but who do not need to stay in the hospital overnight. 063 */ 064 DAYTIME("daytime", "http://hl7.org/fhir/encounter-class"), 065 066 /** 067 * Display: <b>Virtual</b><br> 068 * Code Value: <b>virtual</b> 069 * 070 * An encounter that takes place where the patient and practitioner do not physically meet but use electronic means for contact. 071 */ 072 VIRTUAL("virtual", "http://hl7.org/fhir/encounter-class"), 073 074 /** 075 * Display: <b>Other</b><br> 076 * Code Value: <b>other</b> 077 * 078 * Any other encounter type that is not described by one of the other values. Where this is used it is expected that an implementer will include an extension value to define what the actual other type is. 079 */ 080 OTHER("other", "http://hl7.org/fhir/encounter-class"), 081 082 ; 083 084 /** 085 * Identifier for this Value Set: 086 * 087 */ 088 public static final String VALUESET_IDENTIFIER = ""; 089 090 /** 091 * Name for this Value Set: 092 * EncounterClass 093 */ 094 public static final String VALUESET_NAME = "EncounterClass"; 095 096 private static Map<String, EncounterClassEnum> CODE_TO_ENUM = new HashMap<String, EncounterClassEnum>(); 097 private static Map<String, Map<String, EncounterClassEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, EncounterClassEnum>>(); 098 099 private final String myCode; 100 private final String mySystem; 101 102 static { 103 for (EncounterClassEnum next : EncounterClassEnum.values()) { 104 CODE_TO_ENUM.put(next.getCode(), next); 105 106 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 107 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, EncounterClassEnum>()); 108 } 109 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 110 } 111 } 112 113 /** 114 * Returns the code associated with this enumerated value 115 */ 116 public String getCode() { 117 return myCode; 118 } 119 120 /** 121 * Returns the code system associated with this enumerated value 122 */ 123 public String getSystem() { 124 return mySystem; 125 } 126 127 /** 128 * Returns the enumerated value associated with this code 129 */ 130 public static EncounterClassEnum forCode(String theCode) { 131 EncounterClassEnum retVal = CODE_TO_ENUM.get(theCode); 132 return retVal; 133 } 134 135 /** 136 * Converts codes to their respective enumerated values 137 */ 138 public static final IValueSetEnumBinder<EncounterClassEnum> VALUESET_BINDER = new IValueSetEnumBinder<EncounterClassEnum>() { 139 @Override 140 public String toCodeString(EncounterClassEnum theEnum) { 141 return theEnum.getCode(); 142 } 143 144 @Override 145 public String toSystemString(EncounterClassEnum theEnum) { 146 return theEnum.getSystem(); 147 } 148 149 @Override 150 public EncounterClassEnum fromCodeString(String theCodeString) { 151 return CODE_TO_ENUM.get(theCodeString); 152 } 153 154 @Override 155 public EncounterClassEnum fromCodeString(String theCodeString, String theSystemString) { 156 Map<String, EncounterClassEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 157 if (map == null) { 158 return null; 159 } 160 return map.get(theCodeString); 161 } 162 163 }; 164 165 /** 166 * Constructor 167 */ 168 EncounterClassEnum(String theCode, String theSystem) { 169 myCode = theCode; 170 mySystem = theSystem; 171 } 172 173 174}