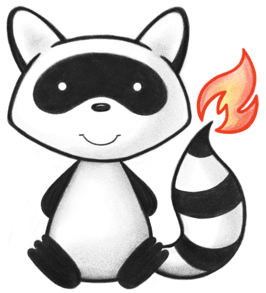
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum EpisodeOfCareStatusEnum { 009 010 /** 011 * Display: <b>Planned</b><br> 012 * Code Value: <b>planned</b> 013 * 014 * This episode of care is planned to start at the date specified in the period.start. During this status an organization may perform assessments to determine if they are eligible to receive services, or be organizing to make resources available to provide care services. 015 */ 016 PLANNED("planned", "http://hl7.org/fhir/episode-of-care-status"), 017 018 /** 019 * Display: <b>Waitlist</b><br> 020 * Code Value: <b>waitlist</b> 021 * 022 * This episode has been placed on a waitlist, pending the episode being made active (or cancelled). 023 */ 024 WAITLIST("waitlist", "http://hl7.org/fhir/episode-of-care-status"), 025 026 /** 027 * Display: <b>Active</b><br> 028 * Code Value: <b>active</b> 029 * 030 * This episode of care is current. 031 */ 032 ACTIVE("active", "http://hl7.org/fhir/episode-of-care-status"), 033 034 /** 035 * Display: <b>On Hold</b><br> 036 * Code Value: <b>onhold</b> 037 * 038 * This episode of care is on hold, the organization has limited responsibility for the patient (such as while on respite). 039 */ 040 ON_HOLD("onhold", "http://hl7.org/fhir/episode-of-care-status"), 041 042 /** 043 * Display: <b>Finished</b><br> 044 * Code Value: <b>finished</b> 045 * 046 * This episode of care is finished at the organization is not expecting to be providing care to the patient. Can also be known as "closed", "completed" or other similar terms. 047 */ 048 FINISHED("finished", "http://hl7.org/fhir/episode-of-care-status"), 049 050 /** 051 * Display: <b>Cancelled</b><br> 052 * Code Value: <b>cancelled</b> 053 * 054 * The episode of care was cancelled, or withdrawn from service, often selected during the planned stage as the patient may have gone elsewhere, or the circumstances have changed and the organization is unable to provide the care. It indicates that services terminated outside the planned/expected workflow. 055 */ 056 CANCELLED("cancelled", "http://hl7.org/fhir/episode-of-care-status"), 057 058 ; 059 060 /** 061 * Identifier for this Value Set: 062 * 063 */ 064 public static final String VALUESET_IDENTIFIER = ""; 065 066 /** 067 * Name for this Value Set: 068 * EpisodeOfCareStatus 069 */ 070 public static final String VALUESET_NAME = "EpisodeOfCareStatus"; 071 072 private static Map<String, EpisodeOfCareStatusEnum> CODE_TO_ENUM = new HashMap<String, EpisodeOfCareStatusEnum>(); 073 private static Map<String, Map<String, EpisodeOfCareStatusEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, EpisodeOfCareStatusEnum>>(); 074 075 private final String myCode; 076 private final String mySystem; 077 078 static { 079 for (EpisodeOfCareStatusEnum next : EpisodeOfCareStatusEnum.values()) { 080 CODE_TO_ENUM.put(next.getCode(), next); 081 082 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 083 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, EpisodeOfCareStatusEnum>()); 084 } 085 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 086 } 087 } 088 089 /** 090 * Returns the code associated with this enumerated value 091 */ 092 public String getCode() { 093 return myCode; 094 } 095 096 /** 097 * Returns the code system associated with this enumerated value 098 */ 099 public String getSystem() { 100 return mySystem; 101 } 102 103 /** 104 * Returns the enumerated value associated with this code 105 */ 106 public static EpisodeOfCareStatusEnum forCode(String theCode) { 107 EpisodeOfCareStatusEnum retVal = CODE_TO_ENUM.get(theCode); 108 return retVal; 109 } 110 111 /** 112 * Converts codes to their respective enumerated values 113 */ 114 public static final IValueSetEnumBinder<EpisodeOfCareStatusEnum> VALUESET_BINDER = new IValueSetEnumBinder<EpisodeOfCareStatusEnum>() { 115 @Override 116 public String toCodeString(EpisodeOfCareStatusEnum theEnum) { 117 return theEnum.getCode(); 118 } 119 120 @Override 121 public String toSystemString(EpisodeOfCareStatusEnum theEnum) { 122 return theEnum.getSystem(); 123 } 124 125 @Override 126 public EpisodeOfCareStatusEnum fromCodeString(String theCodeString) { 127 return CODE_TO_ENUM.get(theCodeString); 128 } 129 130 @Override 131 public EpisodeOfCareStatusEnum fromCodeString(String theCodeString, String theSystemString) { 132 Map<String, EpisodeOfCareStatusEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 133 if (map == null) { 134 return null; 135 } 136 return map.get(theCodeString); 137 } 138 139 }; 140 141 /** 142 * Constructor 143 */ 144 EpisodeOfCareStatusEnum(String theCode, String theSystem) { 145 myCode = theCode; 146 mySystem = theSystem; 147 } 148 149 150}