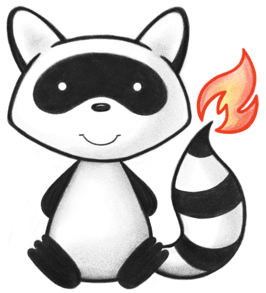
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum EventTimingEnum { 009 010 /** 011 * Code Value: <b>HS</b> 012 */ 013 HS("HS", "http://hl7.org/fhir/v3/TimingEvent"), 014 015 /** 016 * Code Value: <b>WAKE</b> 017 */ 018 WAKE("WAKE", "http://hl7.org/fhir/v3/TimingEvent"), 019 020 /** 021 * Code Value: <b>C</b> 022 */ 023 C("C", "http://hl7.org/fhir/v3/TimingEvent"), 024 025 /** 026 * Code Value: <b>CM</b> 027 */ 028 CM("CM", "http://hl7.org/fhir/v3/TimingEvent"), 029 030 /** 031 * Code Value: <b>CD</b> 032 */ 033 CD("CD", "http://hl7.org/fhir/v3/TimingEvent"), 034 035 /** 036 * Code Value: <b>CV</b> 037 */ 038 CV("CV", "http://hl7.org/fhir/v3/TimingEvent"), 039 040 /** 041 * Code Value: <b>AC</b> 042 */ 043 AC("AC", "http://hl7.org/fhir/v3/TimingEvent"), 044 045 /** 046 * Code Value: <b>ACM</b> 047 */ 048 ACM("ACM", "http://hl7.org/fhir/v3/TimingEvent"), 049 050 /** 051 * Code Value: <b>ACD</b> 052 */ 053 ACD("ACD", "http://hl7.org/fhir/v3/TimingEvent"), 054 055 /** 056 * Code Value: <b>ACV</b> 057 */ 058 ACV("ACV", "http://hl7.org/fhir/v3/TimingEvent"), 059 060 /** 061 * Code Value: <b>PC</b> 062 */ 063 PC("PC", "http://hl7.org/fhir/v3/TimingEvent"), 064 065 /** 066 * Code Value: <b>PCM</b> 067 */ 068 PCM("PCM", "http://hl7.org/fhir/v3/TimingEvent"), 069 070 /** 071 * Code Value: <b>PCD</b> 072 */ 073 PCD("PCD", "http://hl7.org/fhir/v3/TimingEvent"), 074 075 /** 076 * Code Value: <b>PCV</b> 077 */ 078 PCV("PCV", "http://hl7.org/fhir/v3/TimingEvent"), 079 080 ; 081 082 /** 083 * Identifier for this Value Set: 084 * 085 */ 086 public static final String VALUESET_IDENTIFIER = ""; 087 088 /** 089 * Name for this Value Set: 090 * EventTiming 091 */ 092 public static final String VALUESET_NAME = "EventTiming"; 093 094 private static Map<String, EventTimingEnum> CODE_TO_ENUM = new HashMap<String, EventTimingEnum>(); 095 private static Map<String, Map<String, EventTimingEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, EventTimingEnum>>(); 096 097 private final String myCode; 098 private final String mySystem; 099 100 static { 101 for (EventTimingEnum next : EventTimingEnum.values()) { 102 CODE_TO_ENUM.put(next.getCode(), next); 103 104 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 105 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, EventTimingEnum>()); 106 } 107 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 108 } 109 } 110 111 /** 112 * Returns the code associated with this enumerated value 113 */ 114 public String getCode() { 115 return myCode; 116 } 117 118 /** 119 * Returns the code system associated with this enumerated value 120 */ 121 public String getSystem() { 122 return mySystem; 123 } 124 125 /** 126 * Returns the enumerated value associated with this code 127 */ 128 public static EventTimingEnum forCode(String theCode) { 129 EventTimingEnum retVal = CODE_TO_ENUM.get(theCode); 130 return retVal; 131 } 132 133 /** 134 * Converts codes to their respective enumerated values 135 */ 136 public static final IValueSetEnumBinder<EventTimingEnum> VALUESET_BINDER = new IValueSetEnumBinder<EventTimingEnum>() { 137 @Override 138 public String toCodeString(EventTimingEnum theEnum) { 139 return theEnum.getCode(); 140 } 141 142 @Override 143 public String toSystemString(EventTimingEnum theEnum) { 144 return theEnum.getSystem(); 145 } 146 147 @Override 148 public EventTimingEnum fromCodeString(String theCodeString) { 149 return CODE_TO_ENUM.get(theCodeString); 150 } 151 152 @Override 153 public EventTimingEnum fromCodeString(String theCodeString, String theSystemString) { 154 Map<String, EventTimingEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 155 if (map == null) { 156 return null; 157 } 158 return map.get(theCodeString); 159 } 160 161 }; 162 163 /** 164 * Constructor 165 */ 166 EventTimingEnum(String theCode, String theSystem) { 167 myCode = theCode; 168 mySystem = theSystem; 169 } 170 171 172}