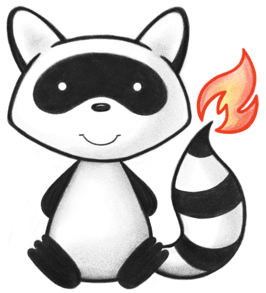
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum GuideResourcePurposeEnum { 009 010 /** 011 * Display: <b>Example</b><br> 012 * Code Value: <b>example</b> 013 * 014 * The resource is intended as an example. 015 */ 016 EXAMPLE("example", "http://hl7.org/fhir/guide-resource-purpose"), 017 018 /** 019 * Display: <b>Terminology</b><br> 020 * Code Value: <b>terminology</b> 021 * 022 * The resource defines a value set or concept map used in the implementation guide. 023 */ 024 TERMINOLOGY("terminology", "http://hl7.org/fhir/guide-resource-purpose"), 025 026 /** 027 * Display: <b>Profile</b><br> 028 * Code Value: <b>profile</b> 029 * 030 * The resource defines a profile (StructureDefinition) that is used in the implementation guide. 031 */ 032 PROFILE("profile", "http://hl7.org/fhir/guide-resource-purpose"), 033 034 /** 035 * Display: <b>Extension</b><br> 036 * Code Value: <b>extension</b> 037 * 038 * The resource defines an extension (StructureDefinition) that is used in the implementation guide. 039 */ 040 EXTENSION("extension", "http://hl7.org/fhir/guide-resource-purpose"), 041 042 /** 043 * Display: <b>Dictionary</b><br> 044 * Code Value: <b>dictionary</b> 045 * 046 * The resource contains a dictionary that is part of the implementation guide. 047 */ 048 DICTIONARY("dictionary", "http://hl7.org/fhir/guide-resource-purpose"), 049 050 /** 051 * Display: <b>Logical Model</b><br> 052 * Code Value: <b>logical</b> 053 * 054 * The resource defines a logical model (in a StructureDefinition) that is used in the implementation guide. 055 */ 056 LOGICAL_MODEL("logical", "http://hl7.org/fhir/guide-resource-purpose"), 057 058 ; 059 060 /** 061 * Identifier for this Value Set: 062 * 063 */ 064 public static final String VALUESET_IDENTIFIER = ""; 065 066 /** 067 * Name for this Value Set: 068 * GuideResourcePurpose 069 */ 070 public static final String VALUESET_NAME = "GuideResourcePurpose"; 071 072 private static Map<String, GuideResourcePurposeEnum> CODE_TO_ENUM = new HashMap<String, GuideResourcePurposeEnum>(); 073 private static Map<String, Map<String, GuideResourcePurposeEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, GuideResourcePurposeEnum>>(); 074 075 private final String myCode; 076 private final String mySystem; 077 078 static { 079 for (GuideResourcePurposeEnum next : GuideResourcePurposeEnum.values()) { 080 CODE_TO_ENUM.put(next.getCode(), next); 081 082 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 083 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, GuideResourcePurposeEnum>()); 084 } 085 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 086 } 087 } 088 089 /** 090 * Returns the code associated with this enumerated value 091 */ 092 public String getCode() { 093 return myCode; 094 } 095 096 /** 097 * Returns the code system associated with this enumerated value 098 */ 099 public String getSystem() { 100 return mySystem; 101 } 102 103 /** 104 * Returns the enumerated value associated with this code 105 */ 106 public static GuideResourcePurposeEnum forCode(String theCode) { 107 GuideResourcePurposeEnum retVal = CODE_TO_ENUM.get(theCode); 108 return retVal; 109 } 110 111 /** 112 * Converts codes to their respective enumerated values 113 */ 114 public static final IValueSetEnumBinder<GuideResourcePurposeEnum> VALUESET_BINDER = new IValueSetEnumBinder<GuideResourcePurposeEnum>() { 115 @Override 116 public String toCodeString(GuideResourcePurposeEnum theEnum) { 117 return theEnum.getCode(); 118 } 119 120 @Override 121 public String toSystemString(GuideResourcePurposeEnum theEnum) { 122 return theEnum.getSystem(); 123 } 124 125 @Override 126 public GuideResourcePurposeEnum fromCodeString(String theCodeString) { 127 return CODE_TO_ENUM.get(theCodeString); 128 } 129 130 @Override 131 public GuideResourcePurposeEnum fromCodeString(String theCodeString, String theSystemString) { 132 Map<String, GuideResourcePurposeEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 133 if (map == null) { 134 return null; 135 } 136 return map.get(theCodeString); 137 } 138 139 }; 140 141 /** 142 * Constructor 143 */ 144 GuideResourcePurposeEnum(String theCode, String theSystem) { 145 myCode = theCode; 146 mySystem = theSystem; 147 } 148 149 150}