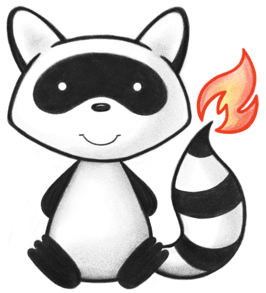
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum IdentifierTypeCodesEnum { 009 010 /** 011 * Display: <b>Universal Device Identifier</b><br> 012 * Code Value: <b>UDI</b> 013 * 014 * A identifier assigned to a device using the Universal Device Identifier framework as defined by FDA (http://www.fda.gov/MedicalDevices/DeviceRegulationandGuidance/UniqueDeviceIdentification/). 015 */ 016 UNIVERSAL_DEVICE_IDENTIFIER("UDI", "http://hl7.org/fhir/identifier-type"), 017 018 /** 019 * Display: <b>Serial Number</b><br> 020 * Code Value: <b>SNO</b> 021 * 022 * An identifier affixed to an item by the manufacturer when it is first made, where each item has a different identifier. 023 */ 024 SERIAL_NUMBER("SNO", "http://hl7.org/fhir/identifier-type"), 025 026 /** 027 * Display: <b>Social Beneficiary Identifier</b><br> 028 * Code Value: <b>SB</b> 029 * 030 * An identifier issued by a governmental organization to an individual for the purpose of the receipt of social services and benefits. 031 */ 032 SOCIAL_BENEFICIARY_IDENTIFIER("SB", "http://hl7.org/fhir/identifier-type"), 033 034 /** 035 * Display: <b>Placer Identifier</b><br> 036 * Code Value: <b>PLAC</b> 037 * 038 * The identifier associated with the person or service that requests or places an order. 039 */ 040 PLACER_IDENTIFIER("PLAC", "http://hl7.org/fhir/identifier-type"), 041 042 /** 043 * Display: <b>Filler Identifier</b><br> 044 * Code Value: <b>FILL</b> 045 * 046 * The Identifier associated with the person, or service, who produces the observations or fulfills the order requested by the requestor. 047 */ 048 FILLER_IDENTIFIER("FILL", "http://hl7.org/fhir/identifier-type"), 049 050 /** 051 * Code Value: <b>DL</b> 052 */ 053 DL("DL", "http://hl7.org/fhir/v2/0203"), 054 055 /** 056 * Code Value: <b>PPN</b> 057 */ 058 PPN("PPN", "http://hl7.org/fhir/v2/0203"), 059 060 /** 061 * Code Value: <b>BRN</b> 062 */ 063 BRN("BRN", "http://hl7.org/fhir/v2/0203"), 064 065 /** 066 * Code Value: <b>MR</b> 067 */ 068 MR("MR", "http://hl7.org/fhir/v2/0203"), 069 070 /** 071 * Code Value: <b>MCN</b> 072 */ 073 MCN("MCN", "http://hl7.org/fhir/v2/0203"), 074 075 /** 076 * Code Value: <b>EN</b> 077 */ 078 EN("EN", "http://hl7.org/fhir/v2/0203"), 079 080 /** 081 * Code Value: <b>TAX</b> 082 */ 083 TAX("TAX", "http://hl7.org/fhir/v2/0203"), 084 085 /** 086 * Code Value: <b>NIIP</b> 087 */ 088 NIIP("NIIP", "http://hl7.org/fhir/v2/0203"), 089 090 /** 091 * Code Value: <b>PRN</b> 092 */ 093 PRN("PRN", "http://hl7.org/fhir/v2/0203"), 094 095 /** 096 * Code Value: <b>MD</b> 097 */ 098 MD("MD", "http://hl7.org/fhir/v2/0203"), 099 100 /** 101 * Code Value: <b>DR</b> 102 */ 103 DR("DR", "http://hl7.org/fhir/v2/0203"), 104 105 ; 106 107 /** 108 * Identifier for this Value Set: 109 * 110 */ 111 public static final String VALUESET_IDENTIFIER = ""; 112 113 /** 114 * Name for this Value Set: 115 * Identifier Type Codes 116 */ 117 public static final String VALUESET_NAME = "Identifier Type Codes"; 118 119 private static Map<String, IdentifierTypeCodesEnum> CODE_TO_ENUM = new HashMap<String, IdentifierTypeCodesEnum>(); 120 private static Map<String, Map<String, IdentifierTypeCodesEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, IdentifierTypeCodesEnum>>(); 121 122 private final String myCode; 123 private final String mySystem; 124 125 static { 126 for (IdentifierTypeCodesEnum next : IdentifierTypeCodesEnum.values()) { 127 CODE_TO_ENUM.put(next.getCode(), next); 128 129 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 130 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, IdentifierTypeCodesEnum>()); 131 } 132 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 133 } 134 } 135 136 /** 137 * Returns the code associated with this enumerated value 138 */ 139 public String getCode() { 140 return myCode; 141 } 142 143 /** 144 * Returns the code system associated with this enumerated value 145 */ 146 public String getSystem() { 147 return mySystem; 148 } 149 150 /** 151 * Returns the enumerated value associated with this code 152 */ 153 public static IdentifierTypeCodesEnum forCode(String theCode) { 154 IdentifierTypeCodesEnum retVal = CODE_TO_ENUM.get(theCode); 155 return retVal; 156 } 157 158 /** 159 * Converts codes to their respective enumerated values 160 */ 161 public static final IValueSetEnumBinder<IdentifierTypeCodesEnum> VALUESET_BINDER = new IValueSetEnumBinder<IdentifierTypeCodesEnum>() { 162 @Override 163 public String toCodeString(IdentifierTypeCodesEnum theEnum) { 164 return theEnum.getCode(); 165 } 166 167 @Override 168 public String toSystemString(IdentifierTypeCodesEnum theEnum) { 169 return theEnum.getSystem(); 170 } 171 172 @Override 173 public IdentifierTypeCodesEnum fromCodeString(String theCodeString) { 174 return CODE_TO_ENUM.get(theCodeString); 175 } 176 177 @Override 178 public IdentifierTypeCodesEnum fromCodeString(String theCodeString, String theSystemString) { 179 Map<String, IdentifierTypeCodesEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 180 if (map == null) { 181 return null; 182 } 183 return map.get(theCodeString); 184 } 185 186 }; 187 188 /** 189 * Constructor 190 */ 191 IdentifierTypeCodesEnum(String theCode, String theSystem) { 192 myCode = theCode; 193 mySystem = theSystem; 194 } 195 196 197}