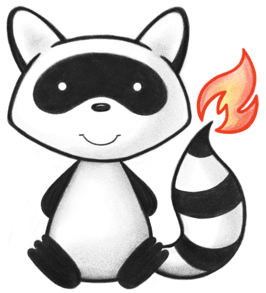
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum InstanceAvailabilityEnum { 009 010 /** 011 * Code Value: <b>ONLINE</b> 012 */ 013 ONLINE("ONLINE", "http://nema.org/dicom/dicm"), 014 015 /** 016 * Code Value: <b>OFFLINE</b> 017 */ 018 OFFLINE("OFFLINE", "http://nema.org/dicom/dicm"), 019 020 /** 021 * Code Value: <b>NEARLINE</b> 022 */ 023 NEARLINE("NEARLINE", "http://nema.org/dicom/dicm"), 024 025 /** 026 * Code Value: <b>UNAVAILABLE</b> 027 */ 028 UNAVAILABLE("UNAVAILABLE", "http://nema.org/dicom/dicm"), 029 030 ; 031 032 /** 033 * Identifier for this Value Set: 034 * 035 */ 036 public static final String VALUESET_IDENTIFIER = ""; 037 038 /** 039 * Name for this Value Set: 040 * InstanceAvailability 041 */ 042 public static final String VALUESET_NAME = "InstanceAvailability"; 043 044 private static Map<String, InstanceAvailabilityEnum> CODE_TO_ENUM = new HashMap<String, InstanceAvailabilityEnum>(); 045 private static Map<String, Map<String, InstanceAvailabilityEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, InstanceAvailabilityEnum>>(); 046 047 private final String myCode; 048 private final String mySystem; 049 050 static { 051 for (InstanceAvailabilityEnum next : InstanceAvailabilityEnum.values()) { 052 CODE_TO_ENUM.put(next.getCode(), next); 053 054 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 055 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, InstanceAvailabilityEnum>()); 056 } 057 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 058 } 059 } 060 061 /** 062 * Returns the code associated with this enumerated value 063 */ 064 public String getCode() { 065 return myCode; 066 } 067 068 /** 069 * Returns the code system associated with this enumerated value 070 */ 071 public String getSystem() { 072 return mySystem; 073 } 074 075 /** 076 * Returns the enumerated value associated with this code 077 */ 078 public static InstanceAvailabilityEnum forCode(String theCode) { 079 InstanceAvailabilityEnum retVal = CODE_TO_ENUM.get(theCode); 080 return retVal; 081 } 082 083 /** 084 * Converts codes to their respective enumerated values 085 */ 086 public static final IValueSetEnumBinder<InstanceAvailabilityEnum> VALUESET_BINDER = new IValueSetEnumBinder<InstanceAvailabilityEnum>() { 087 @Override 088 public String toCodeString(InstanceAvailabilityEnum theEnum) { 089 return theEnum.getCode(); 090 } 091 092 @Override 093 public String toSystemString(InstanceAvailabilityEnum theEnum) { 094 return theEnum.getSystem(); 095 } 096 097 @Override 098 public InstanceAvailabilityEnum fromCodeString(String theCodeString) { 099 return CODE_TO_ENUM.get(theCodeString); 100 } 101 102 @Override 103 public InstanceAvailabilityEnum fromCodeString(String theCodeString, String theSystemString) { 104 Map<String, InstanceAvailabilityEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 105 if (map == null) { 106 return null; 107 } 108 return map.get(theCodeString); 109 } 110 111 }; 112 113 /** 114 * Constructor 115 */ 116 InstanceAvailabilityEnum(String theCode, String theSystem) { 117 myCode = theCode; 118 mySystem = theSystem; 119 } 120 121 122}