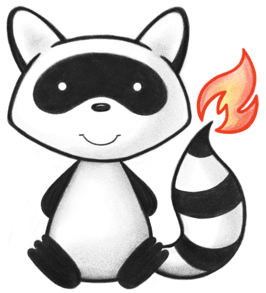
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum IssueTypeEnum { 009 010 /** 011 * Display: <b>Invalid Content</b><br> 012 * Code Value: <b>invalid</b> 013 * 014 * Content invalid against the specification or a profile. 015 */ 016 INVALID_CONTENT("invalid", "http://hl7.org/fhir/issue-type"), 017 018 /** 019 * Display: <b>Structural Issue</b><br> 020 * Code Value: <b>structure</b> 021 * 022 * A structural issue in the content such as wrong namespace, or unable to parse the content completely, or invalid json syntax. 023 */ 024 STRUCTURAL_ISSUE("structure", "http://hl7.org/fhir/issue-type"), 025 026 /** 027 * Display: <b>Required element missing</b><br> 028 * Code Value: <b>required</b> 029 * 030 * A required element is missing. 031 */ 032 REQUIRED_ELEMENT_MISSING("required", "http://hl7.org/fhir/issue-type"), 033 034 /** 035 * Display: <b>Element value invalid</b><br> 036 * Code Value: <b>value</b> 037 * 038 * An element value is invalid. 039 */ 040 ELEMENT_VALUE_INVALID("value", "http://hl7.org/fhir/issue-type"), 041 042 /** 043 * Display: <b>Validation rule failed</b><br> 044 * Code Value: <b>invariant</b> 045 * 046 * A content validation rule failed - e.g. a schematron rule. 047 */ 048 VALIDATION_RULE_FAILED("invariant", "http://hl7.org/fhir/issue-type"), 049 050 /** 051 * Display: <b>Security Problem</b><br> 052 * Code Value: <b>security</b> 053 * 054 * An authentication/authorization/permissions issue of some kind. 055 */ 056 SECURITY_PROBLEM("security", "http://hl7.org/fhir/issue-type"), 057 058 /** 059 * Display: <b>Login Required</b><br> 060 * Code Value: <b>login</b> 061 * 062 * The client needs to initiate an authentication process. 063 */ 064 LOGIN_REQUIRED("login", "http://hl7.org/fhir/issue-type"), 065 066 /** 067 * Display: <b>Unknown User</b><br> 068 * Code Value: <b>unknown</b> 069 * 070 * The user or system was not able to be authenticated (either there is no process, or the proferred token is unacceptable). 071 */ 072 UNKNOWN_USER("unknown", "http://hl7.org/fhir/issue-type"), 073 074 /** 075 * Display: <b>Session Expired</b><br> 076 * Code Value: <b>expired</b> 077 * 078 * User session expired; a login may be required. 079 */ 080 SESSION_EXPIRED("expired", "http://hl7.org/fhir/issue-type"), 081 082 /** 083 * Display: <b>Forbidden</b><br> 084 * Code Value: <b>forbidden</b> 085 * 086 * The user does not have the rights to perform this action. 087 */ 088 FORBIDDEN("forbidden", "http://hl7.org/fhir/issue-type"), 089 090 /** 091 * Display: <b>Information Suppressed</b><br> 092 * Code Value: <b>suppressed</b> 093 * 094 * Some information was not or may not have been returned due to business rules, consent or privacy rules, or access permission constraints. This information may be accessible through alternate processes. 095 */ 096 INFORMATION__SUPPRESSED("suppressed", "http://hl7.org/fhir/issue-type"), 097 098 /** 099 * Display: <b>Processing Failure</b><br> 100 * Code Value: <b>processing</b> 101 * 102 * Processing issues. These are expected to be final e.g. there is no point resubmitting the same content unchanged. 103 */ 104 PROCESSING_FAILURE("processing", "http://hl7.org/fhir/issue-type"), 105 106 /** 107 * Display: <b>Content not supported</b><br> 108 * Code Value: <b>not-supported</b> 109 * 110 * The resource or profile is not supported. 111 */ 112 CONTENT_NOT_SUPPORTED("not-supported", "http://hl7.org/fhir/issue-type"), 113 114 /** 115 * Display: <b>Duplicate</b><br> 116 * Code Value: <b>duplicate</b> 117 * 118 * An attempt was made to create a duplicate record. 119 */ 120 DUPLICATE("duplicate", "http://hl7.org/fhir/issue-type"), 121 122 /** 123 * Display: <b>Not Found</b><br> 124 * Code Value: <b>not-found</b> 125 * 126 * The reference provided was not found. In a pure RESTful environment, this would be an HTTP 404 error, but this code may be used where the content is not found further into the application architecture. 127 */ 128 NOT_FOUND("not-found", "http://hl7.org/fhir/issue-type"), 129 130 /** 131 * Display: <b>Content Too Long</b><br> 132 * Code Value: <b>too-long</b> 133 * 134 * Provided content is too long (typically, this is a denial of service protection type of error). 135 */ 136 CONTENT_TOO_LONG("too-long", "http://hl7.org/fhir/issue-type"), 137 138 /** 139 * Display: <b>Invalid Code</b><br> 140 * Code Value: <b>code-invalid</b> 141 * 142 * The code or system could not be understood, or it was not valid in the context of a particular ValueSet.code. 143 */ 144 INVALID_CODE("code-invalid", "http://hl7.org/fhir/issue-type"), 145 146 /** 147 * Display: <b>Unacceptable Extension</b><br> 148 * Code Value: <b>extension</b> 149 * 150 * An extension was found that was not acceptable, could not be resolved, or a modifierExtension was not recognized. 151 */ 152 UNACCEPTABLE_EXTENSION("extension", "http://hl7.org/fhir/issue-type"), 153 154 /** 155 * Display: <b>Operation Too Costly</b><br> 156 * Code Value: <b>too-costly</b> 157 * 158 * The operation was stopped to protect server resources; e.g. a request for a value set expansion on all of SNOMED CT. 159 */ 160 OPERATION_TOO_COSTLY("too-costly", "http://hl7.org/fhir/issue-type"), 161 162 /** 163 * Display: <b>Business Rule Violation</b><br> 164 * Code Value: <b>business-rule</b> 165 * 166 * The content/operation failed to pass some business rule, and so could not proceed. 167 */ 168 BUSINESS_RULE_VIOLATION("business-rule", "http://hl7.org/fhir/issue-type"), 169 170 /** 171 * Display: <b>Edit Version Conflict</b><br> 172 * Code Value: <b>conflict</b> 173 * 174 * Content could not be accepted because of an edit conflict (i.e. version aware updates) (In a pure RESTful environment, this would be an HTTP 404 error, but this code may be used where the conflict is discovered further into the application architecture.) 175 */ 176 EDIT_VERSION_CONFLICT("conflict", "http://hl7.org/fhir/issue-type"), 177 178 /** 179 * Display: <b>Incomplete Results</b><br> 180 * Code Value: <b>incomplete</b> 181 * 182 * Not all data sources typically accessed could be reached, or responded in time, so the returned information may not be complete. 183 */ 184 INCOMPLETE_RESULTS("incomplete", "http://hl7.org/fhir/issue-type"), 185 186 /** 187 * Display: <b>Transient Issue</b><br> 188 * Code Value: <b>transient</b> 189 * 190 * Transient processing issues. The system receiving the error may be able to resubmit the same content once an underlying issue is resolved. 191 */ 192 TRANSIENT_ISSUE("transient", "http://hl7.org/fhir/issue-type"), 193 194 /** 195 * Display: <b>Lock Error</b><br> 196 * Code Value: <b>lock-error</b> 197 * 198 * A resource/record locking failure (usually in an underlying database). 199 */ 200 LOCK_ERROR("lock-error", "http://hl7.org/fhir/issue-type"), 201 202 /** 203 * Display: <b>No Store Available</b><br> 204 * Code Value: <b>no-store</b> 205 * 206 * The persistent store is unavailable; e.g. the database is down for maintenance or similar action. 207 */ 208 NO_STORE_AVAILABLE("no-store", "http://hl7.org/fhir/issue-type"), 209 210 /** 211 * Display: <b>Exception</b><br> 212 * Code Value: <b>exception</b> 213 * 214 * An unexpected internal error has occurred. 215 */ 216 EXCEPTION("exception", "http://hl7.org/fhir/issue-type"), 217 218 /** 219 * Display: <b>Timeout</b><br> 220 * Code Value: <b>timeout</b> 221 * 222 * An internal timeout has occurred. 223 */ 224 TIMEOUT("timeout", "http://hl7.org/fhir/issue-type"), 225 226 /** 227 * Display: <b>Throttled</b><br> 228 * Code Value: <b>throttled</b> 229 * 230 * The system is not prepared to handle this request due to load management. 231 */ 232 THROTTLED("throttled", "http://hl7.org/fhir/issue-type"), 233 234 /** 235 * Display: <b>Informational Note</b><br> 236 * Code Value: <b>informational</b> 237 * 238 * A message unrelated to the processing success of the completed operation (examples of the latter include things like reminders of password expiry, system maintenance times, etc.). 239 */ 240 INFORMATIONAL_NOTE("informational", "http://hl7.org/fhir/issue-type"), 241 242 ; 243 244 /** 245 * Identifier for this Value Set: 246 * 247 */ 248 public static final String VALUESET_IDENTIFIER = ""; 249 250 /** 251 * Name for this Value Set: 252 * IssueType 253 */ 254 public static final String VALUESET_NAME = "IssueType"; 255 256 private static Map<String, IssueTypeEnum> CODE_TO_ENUM = new HashMap<String, IssueTypeEnum>(); 257 private static Map<String, Map<String, IssueTypeEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, IssueTypeEnum>>(); 258 259 private final String myCode; 260 private final String mySystem; 261 262 static { 263 for (IssueTypeEnum next : IssueTypeEnum.values()) { 264 CODE_TO_ENUM.put(next.getCode(), next); 265 266 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 267 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, IssueTypeEnum>()); 268 } 269 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 270 } 271 } 272 273 /** 274 * Returns the code associated with this enumerated value 275 */ 276 public String getCode() { 277 return myCode; 278 } 279 280 /** 281 * Returns the code system associated with this enumerated value 282 */ 283 public String getSystem() { 284 return mySystem; 285 } 286 287 /** 288 * Returns the enumerated value associated with this code 289 */ 290 public static IssueTypeEnum forCode(String theCode) { 291 IssueTypeEnum retVal = CODE_TO_ENUM.get(theCode); 292 return retVal; 293 } 294 295 /** 296 * Converts codes to their respective enumerated values 297 */ 298 public static final IValueSetEnumBinder<IssueTypeEnum> VALUESET_BINDER = new IValueSetEnumBinder<IssueTypeEnum>() { 299 @Override 300 public String toCodeString(IssueTypeEnum theEnum) { 301 return theEnum.getCode(); 302 } 303 304 @Override 305 public String toSystemString(IssueTypeEnum theEnum) { 306 return theEnum.getSystem(); 307 } 308 309 @Override 310 public IssueTypeEnum fromCodeString(String theCodeString) { 311 return CODE_TO_ENUM.get(theCodeString); 312 } 313 314 @Override 315 public IssueTypeEnum fromCodeString(String theCodeString, String theSystemString) { 316 Map<String, IssueTypeEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 317 if (map == null) { 318 return null; 319 } 320 return map.get(theCodeString); 321 } 322 323 }; 324 325 /** 326 * Constructor 327 */ 328 IssueTypeEnum(String theCode, String theSystem) { 329 myCode = theCode; 330 mySystem = theSystem; 331 } 332 333 334}