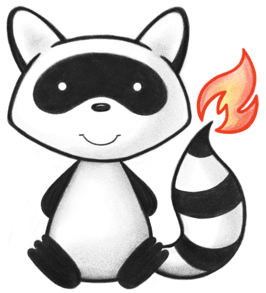
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum KOStitleEnum { 009 010 /** 011 * Code Value: <b>113000</b> 012 */ 013 _113000("113000", "http://nema.org/dicom/dicm"), 014 015 /** 016 * Code Value: <b>113001</b> 017 */ 018 _113001("113001", "http://nema.org/dicom/dicm"), 019 020 /** 021 * Code Value: <b>113002</b> 022 */ 023 _113002("113002", "http://nema.org/dicom/dicm"), 024 025 /** 026 * Code Value: <b>113003</b> 027 */ 028 _113003("113003", "http://nema.org/dicom/dicm"), 029 030 /** 031 * Code Value: <b>113004</b> 032 */ 033 _113004("113004", "http://nema.org/dicom/dicm"), 034 035 /** 036 * Code Value: <b>113005</b> 037 */ 038 _113005("113005", "http://nema.org/dicom/dicm"), 039 040 /** 041 * Code Value: <b>113006</b> 042 */ 043 _113006("113006", "http://nema.org/dicom/dicm"), 044 045 /** 046 * Code Value: <b>113007</b> 047 */ 048 _113007("113007", "http://nema.org/dicom/dicm"), 049 050 /** 051 * Code Value: <b>113008</b> 052 */ 053 _113008("113008", "http://nema.org/dicom/dicm"), 054 055 /** 056 * Code Value: <b>113009</b> 057 */ 058 _113009("113009", "http://nema.org/dicom/dicm"), 059 060 /** 061 * Code Value: <b>113010</b> 062 */ 063 _113010("113010", "http://nema.org/dicom/dicm"), 064 065 /** 066 * Code Value: <b>113013</b> 067 */ 068 _113013("113013", "http://nema.org/dicom/dicm"), 069 070 /** 071 * Code Value: <b>113018</b> 072 */ 073 _113018("113018", "http://nema.org/dicom/dicm"), 074 075 /** 076 * Code Value: <b>113020</b> 077 */ 078 _113020("113020", "http://nema.org/dicom/dicm"), 079 080 /** 081 * Code Value: <b>113021</b> 082 */ 083 _113021("113021", "http://nema.org/dicom/dicm"), 084 085 /** 086 * Code Value: <b>113030</b> 087 */ 088 _113030("113030", "http://nema.org/dicom/dicm"), 089 090 /** 091 * Code Value: <b>113031</b> 092 */ 093 _113031("113031", "http://nema.org/dicom/dicm"), 094 095 /** 096 * Code Value: <b>113032</b> 097 */ 098 _113032("113032", "http://nema.org/dicom/dicm"), 099 100 /** 101 * Code Value: <b>113033</b> 102 */ 103 _113033("113033", "http://nema.org/dicom/dicm"), 104 105 /** 106 * Code Value: <b>113034</b> 107 */ 108 _113034("113034", "http://nema.org/dicom/dicm"), 109 110 /** 111 * Code Value: <b>113035</b> 112 */ 113 _113035("113035", "http://nema.org/dicom/dicm"), 114 115 /** 116 * Code Value: <b>113036</b> 117 */ 118 _113036("113036", "http://nema.org/dicom/dicm"), 119 120 /** 121 * Code Value: <b>113037</b> 122 */ 123 _113037("113037", "http://nema.org/dicom/dicm"), 124 125 /** 126 * Code Value: <b>113038</b> 127 */ 128 _113038("113038", "http://nema.org/dicom/dicm"), 129 130 /** 131 * Code Value: <b>113039</b> 132 */ 133 _113039("113039", "http://nema.org/dicom/dicm"), 134 135 ; 136 137 /** 138 * Identifier for this Value Set: 139 * 140 */ 141 public static final String VALUESET_IDENTIFIER = ""; 142 143 /** 144 * Name for this Value Set: 145 * KOStitle 146 */ 147 public static final String VALUESET_NAME = "KOStitle"; 148 149 private static Map<String, KOStitleEnum> CODE_TO_ENUM = new HashMap<String, KOStitleEnum>(); 150 private static Map<String, Map<String, KOStitleEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, KOStitleEnum>>(); 151 152 private final String myCode; 153 private final String mySystem; 154 155 static { 156 for (KOStitleEnum next : KOStitleEnum.values()) { 157 CODE_TO_ENUM.put(next.getCode(), next); 158 159 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 160 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, KOStitleEnum>()); 161 } 162 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 163 } 164 } 165 166 /** 167 * Returns the code associated with this enumerated value 168 */ 169 public String getCode() { 170 return myCode; 171 } 172 173 /** 174 * Returns the code system associated with this enumerated value 175 */ 176 public String getSystem() { 177 return mySystem; 178 } 179 180 /** 181 * Returns the enumerated value associated with this code 182 */ 183 public static KOStitleEnum forCode(String theCode) { 184 KOStitleEnum retVal = CODE_TO_ENUM.get(theCode); 185 return retVal; 186 } 187 188 /** 189 * Converts codes to their respective enumerated values 190 */ 191 public static final IValueSetEnumBinder<KOStitleEnum> VALUESET_BINDER = new IValueSetEnumBinder<KOStitleEnum>() { 192 @Override 193 public String toCodeString(KOStitleEnum theEnum) { 194 return theEnum.getCode(); 195 } 196 197 @Override 198 public String toSystemString(KOStitleEnum theEnum) { 199 return theEnum.getSystem(); 200 } 201 202 @Override 203 public KOStitleEnum fromCodeString(String theCodeString) { 204 return CODE_TO_ENUM.get(theCodeString); 205 } 206 207 @Override 208 public KOStitleEnum fromCodeString(String theCodeString, String theSystemString) { 209 Map<String, KOStitleEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 210 if (map == null) { 211 return null; 212 } 213 return map.get(theCodeString); 214 } 215 216 }; 217 218 /** 219 * Constructor 220 */ 221 KOStitleEnum(String theCode, String theSystem) { 222 myCode = theCode; 223 mySystem = theSystem; 224 } 225 226 227}