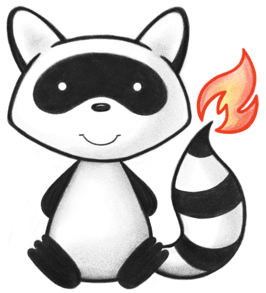
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum ListOrderCodesEnum { 009 010 /** 011 * Display: <b>Sorted by User</b><br> 012 * Code Value: <b>user</b> 013 * 014 * The list was sorted by a user. The criteria the user used are not specified. 015 */ 016 SORTED_BY_USER("user", "http://hl7.org/fhir/list-order"), 017 018 /** 019 * Display: <b>Sorted by System</b><br> 020 * Code Value: <b>system</b> 021 * 022 * The list was sorted by the system. The criteria the user used are not specified; define additional codes to specify a particular order (or use other defined codes). 023 */ 024 SORTED_BY_SYSTEM("system", "http://hl7.org/fhir/list-order"), 025 026 /** 027 * Display: <b>Sorted by Event Date</b><br> 028 * Code Value: <b>event-date</b> 029 * 030 * The list is sorted by the data of the event. This can be used when the list has items which are dates with past or future events. 031 */ 032 SORTED_BY_EVENT_DATE("event-date", "http://hl7.org/fhir/list-order"), 033 034 /** 035 * Display: <b>Sorted by Item Date</b><br> 036 * Code Value: <b>entry-date</b> 037 * 038 * The list is sorted by the date the item was added to the list. Note that the date added to the list is not explicit in the list itself. 039 */ 040 SORTED_BY_ITEM_DATE("entry-date", "http://hl7.org/fhir/list-order"), 041 042 /** 043 * Display: <b>Sorted by Priority</b><br> 044 * Code Value: <b>priority</b> 045 * 046 * The list is sorted by priority. The exact method in which priority has been determined is not specified. 047 */ 048 SORTED_BY_PRIORITY("priority", "http://hl7.org/fhir/list-order"), 049 050 /** 051 * Display: <b>Sorted Alphabetically</b><br> 052 * Code Value: <b>alphabetic</b> 053 * 054 * The list is sorted alphabetically by an unspecified property of the items in the list. 055 */ 056 SORTED_ALPHABETICALLY("alphabetic", "http://hl7.org/fhir/list-order"), 057 058 /** 059 * Display: <b>Sorted by Category</b><br> 060 * Code Value: <b>category</b> 061 * 062 * The list is sorted categorically by an unspecified property of the items in the list. 063 */ 064 SORTED_BY_CATEGORY("category", "http://hl7.org/fhir/list-order"), 065 066 /** 067 * Display: <b>Sorted by Patient</b><br> 068 * Code Value: <b>patient</b> 069 * 070 * The list is sorted by patient, with items for each patient grouped together. 071 */ 072 SORTED_BY_PATIENT("patient", "http://hl7.org/fhir/list-order"), 073 074 ; 075 076 /** 077 * Identifier for this Value Set: 078 * 079 */ 080 public static final String VALUESET_IDENTIFIER = ""; 081 082 /** 083 * Name for this Value Set: 084 * List Order Codes 085 */ 086 public static final String VALUESET_NAME = "List Order Codes"; 087 088 private static Map<String, ListOrderCodesEnum> CODE_TO_ENUM = new HashMap<String, ListOrderCodesEnum>(); 089 private static Map<String, Map<String, ListOrderCodesEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, ListOrderCodesEnum>>(); 090 091 private final String myCode; 092 private final String mySystem; 093 094 static { 095 for (ListOrderCodesEnum next : ListOrderCodesEnum.values()) { 096 CODE_TO_ENUM.put(next.getCode(), next); 097 098 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 099 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, ListOrderCodesEnum>()); 100 } 101 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 102 } 103 } 104 105 /** 106 * Returns the code associated with this enumerated value 107 */ 108 public String getCode() { 109 return myCode; 110 } 111 112 /** 113 * Returns the code system associated with this enumerated value 114 */ 115 public String getSystem() { 116 return mySystem; 117 } 118 119 /** 120 * Returns the enumerated value associated with this code 121 */ 122 public static ListOrderCodesEnum forCode(String theCode) { 123 ListOrderCodesEnum retVal = CODE_TO_ENUM.get(theCode); 124 return retVal; 125 } 126 127 /** 128 * Converts codes to their respective enumerated values 129 */ 130 public static final IValueSetEnumBinder<ListOrderCodesEnum> VALUESET_BINDER = new IValueSetEnumBinder<ListOrderCodesEnum>() { 131 @Override 132 public String toCodeString(ListOrderCodesEnum theEnum) { 133 return theEnum.getCode(); 134 } 135 136 @Override 137 public String toSystemString(ListOrderCodesEnum theEnum) { 138 return theEnum.getSystem(); 139 } 140 141 @Override 142 public ListOrderCodesEnum fromCodeString(String theCodeString) { 143 return CODE_TO_ENUM.get(theCodeString); 144 } 145 146 @Override 147 public ListOrderCodesEnum fromCodeString(String theCodeString, String theSystemString) { 148 Map<String, ListOrderCodesEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 149 if (map == null) { 150 return null; 151 } 152 return map.get(theCodeString); 153 } 154 155 }; 156 157 /** 158 * Constructor 159 */ 160 ListOrderCodesEnum(String theCode, String theSystem) { 161 myCode = theCode; 162 mySystem = theSystem; 163 } 164 165 166}