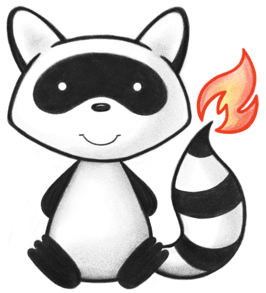
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum LocationTypeEnum { 009 010 /** 011 * Display: <b>Building</b><br> 012 * Code Value: <b>bu</b> 013 * 014 * Any Building or structure. This may contain rooms, corridors, wings, etc. It may not have walls, or a roof, but is considered a defined/allocated space. 015 */ 016 BUILDING("bu", "http://hl7.org/fhir/location-physical-type"), 017 018 /** 019 * Display: <b>Wing</b><br> 020 * Code Value: <b>wi</b> 021 * 022 * A Wing within a Building, this often contains levels, rooms and corridors. 023 */ 024 WING("wi", "http://hl7.org/fhir/location-physical-type"), 025 026 /** 027 * Display: <b>Level</b><br> 028 * Code Value: <b>lvl</b> 029 * 030 * A Level in a multi-level Building/Structure. 031 */ 032 LEVEL("lvl", "http://hl7.org/fhir/location-physical-type"), 033 034 /** 035 * Display: <b>Corridor</b><br> 036 * Code Value: <b>co</b> 037 * 038 * Any corridor within a Building, that is not within. 039 */ 040 CORRIDOR("co", "http://hl7.org/fhir/location-physical-type"), 041 042 /** 043 * Display: <b>Room</b><br> 044 * Code Value: <b>ro</b> 045 * 046 * A space that is allocated as a room, it may have walls/roof etc., but does not require these. 047 */ 048 ROOM("ro", "http://hl7.org/fhir/location-physical-type"), 049 050 /** 051 * Display: <b>Bed</b><br> 052 * Code Value: <b>bd</b> 053 * 054 * A space that is allocated for sleeping/laying on. 055 */ 056 BED("bd", "http://hl7.org/fhir/location-physical-type"), 057 058 /** 059 * Display: <b>Vehicle</b><br> 060 * Code Value: <b>ve</b> 061 * 062 * A means of transportation. 063 */ 064 VEHICLE("ve", "http://hl7.org/fhir/location-physical-type"), 065 066 /** 067 * Display: <b>House</b><br> 068 * Code Value: <b>ho</b> 069 * 070 * A residential dwelling. Usually used to reference a location that a person/patient may reside. 071 */ 072 HOUSE("ho", "http://hl7.org/fhir/location-physical-type"), 073 074 /** 075 * Display: <b>Cabinet</b><br> 076 * Code Value: <b>ca</b> 077 * 078 * A container that can store goods, equipment, medications or other items. 079 */ 080 CABINET("ca", "http://hl7.org/fhir/location-physical-type"), 081 082 /** 083 * Display: <b>Road</b><br> 084 * Code Value: <b>rd</b> 085 * 086 * A defined path to travel between 2 points that has a known name. 087 */ 088 ROAD("rd", "http://hl7.org/fhir/location-physical-type"), 089 090 /** 091 * Display: <b>Jurisdiction</b><br> 092 * Code Value: <b>jdn</b> 093 * 094 * A wide scope that covers a conceptual domain, such as a Nation (Country wide community or Federal Government - e.g. Ministry of Health), Province or State (community or Government), Business (throughout the enterprise), Nation with a business scope of an agency (e.g. CDC, FDA etc.) or a Business segment (UK Pharmacy). 095 */ 096 JURISDICTION("jdn", "http://hl7.org/fhir/location-physical-type"), 097 098 /** 099 * Display: <b>Area</b><br> 100 * Code Value: <b>area</b> 101 * 102 * A defined boundary, such as a state, region, country, county 103 */ 104 AREA("area", "http://hl7.org/fhir/location-physical-type"), 105 106 ; 107 108 /** 109 * Identifier for this Value Set: 110 * 111 */ 112 public static final String VALUESET_IDENTIFIER = ""; 113 114 /** 115 * Name for this Value Set: 116 * LocationType 117 */ 118 public static final String VALUESET_NAME = "LocationType"; 119 120 private static Map<String, LocationTypeEnum> CODE_TO_ENUM = new HashMap<String, LocationTypeEnum>(); 121 private static Map<String, Map<String, LocationTypeEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, LocationTypeEnum>>(); 122 123 private final String myCode; 124 private final String mySystem; 125 126 static { 127 for (LocationTypeEnum next : LocationTypeEnum.values()) { 128 CODE_TO_ENUM.put(next.getCode(), next); 129 130 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 131 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, LocationTypeEnum>()); 132 } 133 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 134 } 135 } 136 137 /** 138 * Returns the code associated with this enumerated value 139 */ 140 public String getCode() { 141 return myCode; 142 } 143 144 /** 145 * Returns the code system associated with this enumerated value 146 */ 147 public String getSystem() { 148 return mySystem; 149 } 150 151 /** 152 * Returns the enumerated value associated with this code 153 */ 154 public static LocationTypeEnum forCode(String theCode) { 155 LocationTypeEnum retVal = CODE_TO_ENUM.get(theCode); 156 return retVal; 157 } 158 159 /** 160 * Converts codes to their respective enumerated values 161 */ 162 public static final IValueSetEnumBinder<LocationTypeEnum> VALUESET_BINDER = new IValueSetEnumBinder<LocationTypeEnum>() { 163 @Override 164 public String toCodeString(LocationTypeEnum theEnum) { 165 return theEnum.getCode(); 166 } 167 168 @Override 169 public String toSystemString(LocationTypeEnum theEnum) { 170 return theEnum.getSystem(); 171 } 172 173 @Override 174 public LocationTypeEnum fromCodeString(String theCodeString) { 175 return CODE_TO_ENUM.get(theCodeString); 176 } 177 178 @Override 179 public LocationTypeEnum fromCodeString(String theCodeString, String theSystemString) { 180 Map<String, LocationTypeEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 181 if (map == null) { 182 return null; 183 } 184 return map.get(theCodeString); 185 } 186 187 }; 188 189 /** 190 * Constructor 191 */ 192 LocationTypeEnum(String theCode, String theSystem) { 193 myCode = theCode; 194 mySystem = theSystem; 195 } 196 197 198}