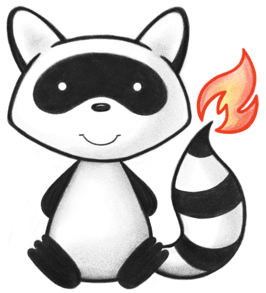
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum MaritalStatusCodesEnum { 009 010 /** 011 * Display: <b>Unmarried</b><br> 012 * Code Value: <b>U</b> 013 * 014 * The person is not presently married. The marital history is not known or stated. 015 */ 016 UNMARRIED("U", "http://hl7.org/fhir/marital-status"), 017 018 /** 019 * Code Value: <b>A</b> 020 */ 021 A("A", "http://hl7.org/fhir/v3/MaritalStatus"), 022 023 /** 024 * Code Value: <b>D</b> 025 */ 026 D("D", "http://hl7.org/fhir/v3/MaritalStatus"), 027 028 /** 029 * Code Value: <b>I</b> 030 */ 031 I("I", "http://hl7.org/fhir/v3/MaritalStatus"), 032 033 /** 034 * Code Value: <b>L</b> 035 */ 036 L("L", "http://hl7.org/fhir/v3/MaritalStatus"), 037 038 /** 039 * Code Value: <b>M</b> 040 */ 041 M("M", "http://hl7.org/fhir/v3/MaritalStatus"), 042 043 /** 044 * Code Value: <b>P</b> 045 */ 046 P("P", "http://hl7.org/fhir/v3/MaritalStatus"), 047 048 /** 049 * Code Value: <b>S</b> 050 */ 051 S("S", "http://hl7.org/fhir/v3/MaritalStatus"), 052 053 /** 054 * Code Value: <b>T</b> 055 */ 056 T("T", "http://hl7.org/fhir/v3/MaritalStatus"), 057 058 /** 059 * Code Value: <b>W</b> 060 */ 061 W("W", "http://hl7.org/fhir/v3/MaritalStatus"), 062 063 /** 064 * Code Value: <b>UNK</b> 065 */ 066 UNK("UNK", "http://hl7.org/fhir/v3/NullFlavor"), 067 068 ; 069 070 /** 071 * Identifier for this Value Set: 072 * 073 */ 074 public static final String VALUESET_IDENTIFIER = ""; 075 076 /** 077 * Name for this Value Set: 078 * Marital Status Codes 079 */ 080 public static final String VALUESET_NAME = "Marital Status Codes"; 081 082 private static Map<String, MaritalStatusCodesEnum> CODE_TO_ENUM = new HashMap<String, MaritalStatusCodesEnum>(); 083 private static Map<String, Map<String, MaritalStatusCodesEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, MaritalStatusCodesEnum>>(); 084 085 private final String myCode; 086 private final String mySystem; 087 088 static { 089 for (MaritalStatusCodesEnum next : MaritalStatusCodesEnum.values()) { 090 CODE_TO_ENUM.put(next.getCode(), next); 091 092 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 093 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, MaritalStatusCodesEnum>()); 094 } 095 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 096 } 097 } 098 099 /** 100 * Returns the code associated with this enumerated value 101 */ 102 public String getCode() { 103 return myCode; 104 } 105 106 /** 107 * Returns the code system associated with this enumerated value 108 */ 109 public String getSystem() { 110 return mySystem; 111 } 112 113 /** 114 * Returns the enumerated value associated with this code 115 */ 116 public static MaritalStatusCodesEnum forCode(String theCode) { 117 MaritalStatusCodesEnum retVal = CODE_TO_ENUM.get(theCode); 118 return retVal; 119 } 120 121 /** 122 * Converts codes to their respective enumerated values 123 */ 124 public static final IValueSetEnumBinder<MaritalStatusCodesEnum> VALUESET_BINDER = new IValueSetEnumBinder<MaritalStatusCodesEnum>() { 125 @Override 126 public String toCodeString(MaritalStatusCodesEnum theEnum) { 127 return theEnum.getCode(); 128 } 129 130 @Override 131 public String toSystemString(MaritalStatusCodesEnum theEnum) { 132 return theEnum.getSystem(); 133 } 134 135 @Override 136 public MaritalStatusCodesEnum fromCodeString(String theCodeString) { 137 return CODE_TO_ENUM.get(theCodeString); 138 } 139 140 @Override 141 public MaritalStatusCodesEnum fromCodeString(String theCodeString, String theSystemString) { 142 Map<String, MaritalStatusCodesEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 143 if (map == null) { 144 return null; 145 } 146 return map.get(theCodeString); 147 } 148 149 }; 150 151 /** 152 * Constructor 153 */ 154 MaritalStatusCodesEnum(String theCode, String theSystem) { 155 myCode = theCode; 156 mySystem = theSystem; 157 } 158 159 160}