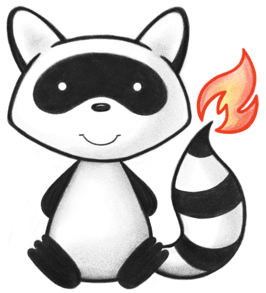
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum MeasmntPrincipleEnum { 009 010 /** 011 * Display: <b>MSP Other</b><br> 012 * Code Value: <b>other</b> 013 * 014 * Measurement principle isn't in the list. 015 */ 016 MSP_OTHER("other", "http://hl7.org/fhir/measurement-principle"), 017 018 /** 019 * Display: <b>MSP Chemical</b><br> 020 * Code Value: <b>chemical</b> 021 * 022 * Measurement is done using the chemical principle. 023 */ 024 MSP_CHEMICAL("chemical", "http://hl7.org/fhir/measurement-principle"), 025 026 /** 027 * Display: <b>MSP Electrical</b><br> 028 * Code Value: <b>electrical</b> 029 * 030 * Measurement is done using the electrical principle. 031 */ 032 MSP_ELECTRICAL("electrical", "http://hl7.org/fhir/measurement-principle"), 033 034 /** 035 * Display: <b>MSP Impedance</b><br> 036 * Code Value: <b>impedance</b> 037 * 038 * Measurement is done using the impedance principle. 039 */ 040 MSP_IMPEDANCE("impedance", "http://hl7.org/fhir/measurement-principle"), 041 042 /** 043 * Display: <b>MSP Nuclear</b><br> 044 * Code Value: <b>nuclear</b> 045 * 046 * Measurement is done using the nuclear principle. 047 */ 048 MSP_NUCLEAR("nuclear", "http://hl7.org/fhir/measurement-principle"), 049 050 /** 051 * Display: <b>MSP Optical</b><br> 052 * Code Value: <b>optical</b> 053 * 054 * Measurement is done using the optical principle. 055 */ 056 MSP_OPTICAL("optical", "http://hl7.org/fhir/measurement-principle"), 057 058 /** 059 * Display: <b>MSP Thermal</b><br> 060 * Code Value: <b>thermal</b> 061 * 062 * Measurement is done using the thermal principle. 063 */ 064 MSP_THERMAL("thermal", "http://hl7.org/fhir/measurement-principle"), 065 066 /** 067 * Display: <b>MSP Biological</b><br> 068 * Code Value: <b>biological</b> 069 * 070 * Measurement is done using the biological principle. 071 */ 072 MSP_BIOLOGICAL("biological", "http://hl7.org/fhir/measurement-principle"), 073 074 /** 075 * Display: <b>MSP Mechanical</b><br> 076 * Code Value: <b>mechanical</b> 077 * 078 * Measurement is done using the mechanical principle. 079 */ 080 MSP_MECHANICAL("mechanical", "http://hl7.org/fhir/measurement-principle"), 081 082 /** 083 * Display: <b>MSP Acoustical</b><br> 084 * Code Value: <b>acoustical</b> 085 * 086 * Measurement is done using the acoustical principle. 087 */ 088 MSP_ACOUSTICAL("acoustical", "http://hl7.org/fhir/measurement-principle"), 089 090 /** 091 * Display: <b>MSP Manual</b><br> 092 * Code Value: <b>manual</b> 093 * 094 * Measurement is done using the manual principle. 095 */ 096 MSP_MANUAL("manual", "http://hl7.org/fhir/measurement-principle"), 097 098 ; 099 100 /** 101 * Identifier for this Value Set: 102 * 103 */ 104 public static final String VALUESET_IDENTIFIER = ""; 105 106 /** 107 * Name for this Value Set: 108 * Measmnt-Principle 109 */ 110 public static final String VALUESET_NAME = "Measmnt-Principle"; 111 112 private static Map<String, MeasmntPrincipleEnum> CODE_TO_ENUM = new HashMap<String, MeasmntPrincipleEnum>(); 113 private static Map<String, Map<String, MeasmntPrincipleEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, MeasmntPrincipleEnum>>(); 114 115 private final String myCode; 116 private final String mySystem; 117 118 static { 119 for (MeasmntPrincipleEnum next : MeasmntPrincipleEnum.values()) { 120 CODE_TO_ENUM.put(next.getCode(), next); 121 122 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 123 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, MeasmntPrincipleEnum>()); 124 } 125 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 126 } 127 } 128 129 /** 130 * Returns the code associated with this enumerated value 131 */ 132 public String getCode() { 133 return myCode; 134 } 135 136 /** 137 * Returns the code system associated with this enumerated value 138 */ 139 public String getSystem() { 140 return mySystem; 141 } 142 143 /** 144 * Returns the enumerated value associated with this code 145 */ 146 public static MeasmntPrincipleEnum forCode(String theCode) { 147 MeasmntPrincipleEnum retVal = CODE_TO_ENUM.get(theCode); 148 return retVal; 149 } 150 151 /** 152 * Converts codes to their respective enumerated values 153 */ 154 public static final IValueSetEnumBinder<MeasmntPrincipleEnum> VALUESET_BINDER = new IValueSetEnumBinder<MeasmntPrincipleEnum>() { 155 @Override 156 public String toCodeString(MeasmntPrincipleEnum theEnum) { 157 return theEnum.getCode(); 158 } 159 160 @Override 161 public String toSystemString(MeasmntPrincipleEnum theEnum) { 162 return theEnum.getSystem(); 163 } 164 165 @Override 166 public MeasmntPrincipleEnum fromCodeString(String theCodeString) { 167 return CODE_TO_ENUM.get(theCodeString); 168 } 169 170 @Override 171 public MeasmntPrincipleEnum fromCodeString(String theCodeString, String theSystemString) { 172 Map<String, MeasmntPrincipleEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 173 if (map == null) { 174 return null; 175 } 176 return map.get(theCodeString); 177 } 178 179 }; 180 181 /** 182 * Constructor 183 */ 184 MeasmntPrincipleEnum(String theCode, String theSystem) { 185 myCode = theCode; 186 mySystem = theSystem; 187 } 188 189 190}