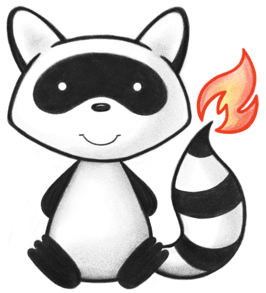
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum NameUseEnum { 009 010 /** 011 * Display: <b>Usual</b><br> 012 * Code Value: <b>usual</b> 013 * 014 * Known as/conventional/the one you normally use 015 */ 016 USUAL("usual", "http://hl7.org/fhir/name-use"), 017 018 /** 019 * Display: <b>Official</b><br> 020 * Code Value: <b>official</b> 021 * 022 * The formal name as registered in an official (government) registry, but which name might not be commonly used. May be called "legal name". 023 */ 024 OFFICIAL("official", "http://hl7.org/fhir/name-use"), 025 026 /** 027 * Display: <b>Temp</b><br> 028 * Code Value: <b>temp</b> 029 * 030 * A temporary name. Name.period can provide more detailed information. This may also be used for temporary names assigned at birth or in emergency situations. 031 */ 032 TEMP("temp", "http://hl7.org/fhir/name-use"), 033 034 /** 035 * Display: <b>Nickname</b><br> 036 * Code Value: <b>nickname</b> 037 * 038 * A name that is used to address the person in an informal manner, but is not part of their formal or usual name 039 */ 040 NICKNAME("nickname", "http://hl7.org/fhir/name-use"), 041 042 /** 043 * Display: <b>Anonymous</b><br> 044 * Code Value: <b>anonymous</b> 045 * 046 * Anonymous assigned name, alias, or pseudonym (used to protect a person's identity for privacy reasons) 047 */ 048 ANONYMOUS("anonymous", "http://hl7.org/fhir/name-use"), 049 050 /** 051 * Display: <b>Old</b><br> 052 * Code Value: <b>old</b> 053 * 054 * This name is no longer in use (or was never correct, but retained for records) 055 */ 056 OLD("old", "http://hl7.org/fhir/name-use"), 057 058 /** 059 * Display: <b>Maiden</b><br> 060 * Code Value: <b>maiden</b> 061 * 062 * A name used prior to marriage. Marriage naming customs vary greatly around the world. This name use is for use by applications that collect and store "maiden" names. Though the concept of maiden name is often gender specific, the use of this term is not gender specific. The use of this term does not imply any particular history for a person's name, nor should the maiden name be determined algorithmically. 063 */ 064 MAIDEN("maiden", "http://hl7.org/fhir/name-use"), 065 066 ; 067 068 /** 069 * Identifier for this Value Set: 070 * 071 */ 072 public static final String VALUESET_IDENTIFIER = ""; 073 074 /** 075 * Name for this Value Set: 076 * NameUse 077 */ 078 public static final String VALUESET_NAME = "NameUse"; 079 080 private static Map<String, NameUseEnum> CODE_TO_ENUM = new HashMap<String, NameUseEnum>(); 081 private static Map<String, Map<String, NameUseEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, NameUseEnum>>(); 082 083 private final String myCode; 084 private final String mySystem; 085 086 static { 087 for (NameUseEnum next : NameUseEnum.values()) { 088 CODE_TO_ENUM.put(next.getCode(), next); 089 090 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 091 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, NameUseEnum>()); 092 } 093 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 094 } 095 } 096 097 /** 098 * Returns the code associated with this enumerated value 099 */ 100 public String getCode() { 101 return myCode; 102 } 103 104 /** 105 * Returns the code system associated with this enumerated value 106 */ 107 public String getSystem() { 108 return mySystem; 109 } 110 111 /** 112 * Returns the enumerated value associated with this code 113 */ 114 public static NameUseEnum forCode(String theCode) { 115 NameUseEnum retVal = CODE_TO_ENUM.get(theCode); 116 return retVal; 117 } 118 119 /** 120 * Converts codes to their respective enumerated values 121 */ 122 public static final IValueSetEnumBinder<NameUseEnum> VALUESET_BINDER = new IValueSetEnumBinder<NameUseEnum>() { 123 @Override 124 public String toCodeString(NameUseEnum theEnum) { 125 return theEnum.getCode(); 126 } 127 128 @Override 129 public String toSystemString(NameUseEnum theEnum) { 130 return theEnum.getSystem(); 131 } 132 133 @Override 134 public NameUseEnum fromCodeString(String theCodeString) { 135 return CODE_TO_ENUM.get(theCodeString); 136 } 137 138 @Override 139 public NameUseEnum fromCodeString(String theCodeString, String theSystemString) { 140 Map<String, NameUseEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 141 if (map == null) { 142 return null; 143 } 144 return map.get(theCodeString); 145 } 146 147 }; 148 149 /** 150 * Constructor 151 */ 152 NameUseEnum(String theCode, String theSystem) { 153 myCode = theCode; 154 mySystem = theSystem; 155 } 156 157 158}