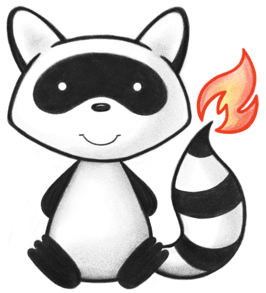
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum ObservationStatusEnum { 009 010 /** 011 * Display: <b>Registered</b><br> 012 * Code Value: <b>registered</b> 013 * 014 * The existence of the observation is registered, but there is no result yet available. 015 */ 016 REGISTERED("registered", "http://hl7.org/fhir/observation-status"), 017 018 /** 019 * Display: <b>Preliminary</b><br> 020 * Code Value: <b>preliminary</b> 021 * 022 * This is an initial or interim observation: data may be incomplete or unverified. 023 */ 024 PRELIMINARY("preliminary", "http://hl7.org/fhir/observation-status"), 025 026 /** 027 * Display: <b>Final</b><br> 028 * Code Value: <b>final</b> 029 * 030 * The observation is complete and verified by an authorized person. 031 */ 032 FINAL("final", "http://hl7.org/fhir/observation-status"), 033 034 /** 035 * Display: <b>Amended</b><br> 036 * Code Value: <b>amended</b> 037 * 038 * The observation has been modified subsequent to being Final, and is complete and verified by an authorized person. 039 */ 040 AMENDED("amended", "http://hl7.org/fhir/observation-status"), 041 042 /** 043 * Display: <b>cancelled</b><br> 044 * Code Value: <b>cancelled</b> 045 * 046 * The observation is unavailable because the measurement was not started or not completed (also sometimes called "aborted"). 047 */ 048 CANCELLED("cancelled", "http://hl7.org/fhir/observation-status"), 049 050 /** 051 * Display: <b>Entered in Error</b><br> 052 * Code Value: <b>entered-in-error</b> 053 * 054 * The observation has been withdrawn following previous final release. 055 */ 056 ENTERED_IN_ERROR("entered-in-error", "http://hl7.org/fhir/observation-status"), 057 058 /** 059 * Display: <b>Unknown Status</b><br> 060 * Code Value: <b>unknown</b> 061 * 062 * The observation status is unknown. Note that "unknown" is a value of last resort and every attempt should be made to provide a meaningful value other than "unknown". 063 */ 064 UNKNOWN_STATUS("unknown", "http://hl7.org/fhir/observation-status"), 065 066 ; 067 068 /** 069 * Identifier for this Value Set: 070 * 071 */ 072 public static final String VALUESET_IDENTIFIER = ""; 073 074 /** 075 * Name for this Value Set: 076 * ObservationStatus 077 */ 078 public static final String VALUESET_NAME = "ObservationStatus"; 079 080 private static Map<String, ObservationStatusEnum> CODE_TO_ENUM = new HashMap<String, ObservationStatusEnum>(); 081 private static Map<String, Map<String, ObservationStatusEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, ObservationStatusEnum>>(); 082 083 private final String myCode; 084 private final String mySystem; 085 086 static { 087 for (ObservationStatusEnum next : ObservationStatusEnum.values()) { 088 CODE_TO_ENUM.put(next.getCode(), next); 089 090 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 091 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, ObservationStatusEnum>()); 092 } 093 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 094 } 095 } 096 097 /** 098 * Returns the code associated with this enumerated value 099 */ 100 public String getCode() { 101 return myCode; 102 } 103 104 /** 105 * Returns the code system associated with this enumerated value 106 */ 107 public String getSystem() { 108 return mySystem; 109 } 110 111 /** 112 * Returns the enumerated value associated with this code 113 */ 114 public static ObservationStatusEnum forCode(String theCode) { 115 ObservationStatusEnum retVal = CODE_TO_ENUM.get(theCode); 116 return retVal; 117 } 118 119 /** 120 * Converts codes to their respective enumerated values 121 */ 122 public static final IValueSetEnumBinder<ObservationStatusEnum> VALUESET_BINDER = new IValueSetEnumBinder<ObservationStatusEnum>() { 123 @Override 124 public String toCodeString(ObservationStatusEnum theEnum) { 125 return theEnum.getCode(); 126 } 127 128 @Override 129 public String toSystemString(ObservationStatusEnum theEnum) { 130 return theEnum.getSystem(); 131 } 132 133 @Override 134 public ObservationStatusEnum fromCodeString(String theCodeString) { 135 return CODE_TO_ENUM.get(theCodeString); 136 } 137 138 @Override 139 public ObservationStatusEnum fromCodeString(String theCodeString, String theSystemString) { 140 Map<String, ObservationStatusEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 141 if (map == null) { 142 return null; 143 } 144 return map.get(theCodeString); 145 } 146 147 }; 148 149 /** 150 * Constructor 151 */ 152 ObservationStatusEnum(String theCode, String theSystem) { 153 myCode = theCode; 154 mySystem = theSystem; 155 } 156 157 158}