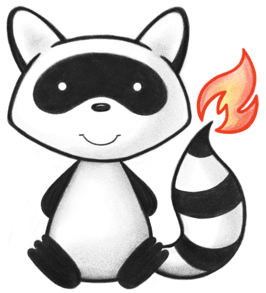
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum OrderStatusEnum { 009 010 /** 011 * Display: <b>Pending</b><br> 012 * Code Value: <b>pending</b> 013 * 014 * The order is known, but no processing has occurred at this time 015 */ 016 PENDING("pending", "http://hl7.org/fhir/order-status"), 017 018 /** 019 * Display: <b>Review</b><br> 020 * Code Value: <b>review</b> 021 * 022 * The order is undergoing initial processing to determine whether it will be accepted (usually this involves human review) 023 */ 024 REVIEW("review", "http://hl7.org/fhir/order-status"), 025 026 /** 027 * Display: <b>Rejected</b><br> 028 * Code Value: <b>rejected</b> 029 * 030 * The order was rejected because of a workflow/business logic reason 031 */ 032 REJECTED("rejected", "http://hl7.org/fhir/order-status"), 033 034 /** 035 * Display: <b>Error</b><br> 036 * Code Value: <b>error</b> 037 * 038 * The order was unable to be processed because of a technical error (i.e. unexpected error) 039 */ 040 ERROR("error", "http://hl7.org/fhir/order-status"), 041 042 /** 043 * Display: <b>Accepted</b><br> 044 * Code Value: <b>accepted</b> 045 * 046 * The order has been accepted, and work is in progress. 047 */ 048 ACCEPTED("accepted", "http://hl7.org/fhir/order-status"), 049 050 /** 051 * Display: <b>Cancelled</b><br> 052 * Code Value: <b>cancelled</b> 053 * 054 * Processing the order was halted at the initiators request. 055 */ 056 CANCELLED("cancelled", "http://hl7.org/fhir/order-status"), 057 058 /** 059 * Display: <b>Replaced</b><br> 060 * Code Value: <b>replaced</b> 061 * 062 * The order has been cancelled and replaced by another. 063 */ 064 REPLACED("replaced", "http://hl7.org/fhir/order-status"), 065 066 /** 067 * Display: <b>Aborted</b><br> 068 * Code Value: <b>aborted</b> 069 * 070 * Processing the order was stopped because of some workflow/business logic reason. 071 */ 072 ABORTED("aborted", "http://hl7.org/fhir/order-status"), 073 074 /** 075 * Display: <b>Completed</b><br> 076 * Code Value: <b>completed</b> 077 * 078 * The order has been completed. 079 */ 080 COMPLETED("completed", "http://hl7.org/fhir/order-status"), 081 082 ; 083 084 /** 085 * Identifier for this Value Set: 086 * 087 */ 088 public static final String VALUESET_IDENTIFIER = ""; 089 090 /** 091 * Name for this Value Set: 092 * OrderStatus 093 */ 094 public static final String VALUESET_NAME = "OrderStatus"; 095 096 private static Map<String, OrderStatusEnum> CODE_TO_ENUM = new HashMap<String, OrderStatusEnum>(); 097 private static Map<String, Map<String, OrderStatusEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, OrderStatusEnum>>(); 098 099 private final String myCode; 100 private final String mySystem; 101 102 static { 103 for (OrderStatusEnum next : OrderStatusEnum.values()) { 104 CODE_TO_ENUM.put(next.getCode(), next); 105 106 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 107 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, OrderStatusEnum>()); 108 } 109 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 110 } 111 } 112 113 /** 114 * Returns the code associated with this enumerated value 115 */ 116 public String getCode() { 117 return myCode; 118 } 119 120 /** 121 * Returns the code system associated with this enumerated value 122 */ 123 public String getSystem() { 124 return mySystem; 125 } 126 127 /** 128 * Returns the enumerated value associated with this code 129 */ 130 public static OrderStatusEnum forCode(String theCode) { 131 OrderStatusEnum retVal = CODE_TO_ENUM.get(theCode); 132 return retVal; 133 } 134 135 /** 136 * Converts codes to their respective enumerated values 137 */ 138 public static final IValueSetEnumBinder<OrderStatusEnum> VALUESET_BINDER = new IValueSetEnumBinder<OrderStatusEnum>() { 139 @Override 140 public String toCodeString(OrderStatusEnum theEnum) { 141 return theEnum.getCode(); 142 } 143 144 @Override 145 public String toSystemString(OrderStatusEnum theEnum) { 146 return theEnum.getSystem(); 147 } 148 149 @Override 150 public OrderStatusEnum fromCodeString(String theCodeString) { 151 return CODE_TO_ENUM.get(theCodeString); 152 } 153 154 @Override 155 public OrderStatusEnum fromCodeString(String theCodeString, String theSystemString) { 156 Map<String, OrderStatusEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 157 if (map == null) { 158 return null; 159 } 160 return map.get(theCodeString); 161 } 162 163 }; 164 165 /** 166 * Constructor 167 */ 168 OrderStatusEnum(String theCode, String theSystem) { 169 myCode = theCode; 170 mySystem = theSystem; 171 } 172 173 174}