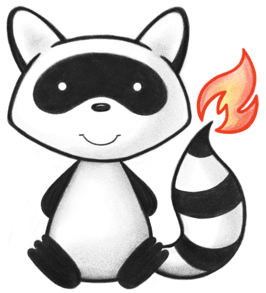
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum ParticipantTypeEnum { 009 010 /** 011 * Display: <b>Translator</b><br> 012 * Code Value: <b>translator</b> 013 * 014 * A translator who is facilitating communication with the patient during the encounter. 015 */ 016 TRANSLATOR("translator", "http://hl7.org/fhir/participant-type"), 017 018 /** 019 * Display: <b>Emergency</b><br> 020 * Code Value: <b>emergency</b> 021 * 022 * A person to be contacted in case of an emergency during the encounter. 023 */ 024 EMERGENCY("emergency", "http://hl7.org/fhir/participant-type"), 025 026 /** 027 * Code Value: <b>SPRF</b> 028 */ 029 SPRF("SPRF", "http://hl7.org/fhir/v3/ParticipationType"), 030 031 /** 032 * Code Value: <b>PPRF</b> 033 */ 034 PPRF("PPRF", "http://hl7.org/fhir/v3/ParticipationType"), 035 036 /** 037 * Code Value: <b>PART</b> 038 */ 039 PART("PART", "http://hl7.org/fhir/v3/ParticipationType"), 040 041 ; 042 043 /** 044 * Identifier for this Value Set: 045 * 046 */ 047 public static final String VALUESET_IDENTIFIER = ""; 048 049 /** 050 * Name for this Value Set: 051 * ParticipantType 052 */ 053 public static final String VALUESET_NAME = "ParticipantType"; 054 055 private static Map<String, ParticipantTypeEnum> CODE_TO_ENUM = new HashMap<String, ParticipantTypeEnum>(); 056 private static Map<String, Map<String, ParticipantTypeEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, ParticipantTypeEnum>>(); 057 058 private final String myCode; 059 private final String mySystem; 060 061 static { 062 for (ParticipantTypeEnum next : ParticipantTypeEnum.values()) { 063 CODE_TO_ENUM.put(next.getCode(), next); 064 065 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 066 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, ParticipantTypeEnum>()); 067 } 068 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 069 } 070 } 071 072 /** 073 * Returns the code associated with this enumerated value 074 */ 075 public String getCode() { 076 return myCode; 077 } 078 079 /** 080 * Returns the code system associated with this enumerated value 081 */ 082 public String getSystem() { 083 return mySystem; 084 } 085 086 /** 087 * Returns the enumerated value associated with this code 088 */ 089 public static ParticipantTypeEnum forCode(String theCode) { 090 ParticipantTypeEnum retVal = CODE_TO_ENUM.get(theCode); 091 return retVal; 092 } 093 094 /** 095 * Converts codes to their respective enumerated values 096 */ 097 public static final IValueSetEnumBinder<ParticipantTypeEnum> VALUESET_BINDER = new IValueSetEnumBinder<ParticipantTypeEnum>() { 098 @Override 099 public String toCodeString(ParticipantTypeEnum theEnum) { 100 return theEnum.getCode(); 101 } 102 103 @Override 104 public String toSystemString(ParticipantTypeEnum theEnum) { 105 return theEnum.getSystem(); 106 } 107 108 @Override 109 public ParticipantTypeEnum fromCodeString(String theCodeString) { 110 return CODE_TO_ENUM.get(theCodeString); 111 } 112 113 @Override 114 public ParticipantTypeEnum fromCodeString(String theCodeString, String theSystemString) { 115 Map<String, ParticipantTypeEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 116 if (map == null) { 117 return null; 118 } 119 return map.get(theCodeString); 120 } 121 122 }; 123 124 /** 125 * Constructor 126 */ 127 ParticipantTypeEnum(String theCode, String theSystem) { 128 myCode = theCode; 129 mySystem = theSystem; 130 } 131 132 133}