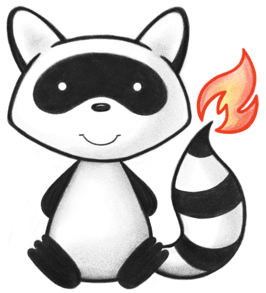
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum ProcedureStatusEnum { 009 010 /** 011 * Display: <b>In Progress</b><br> 012 * Code Value: <b>in-progress</b> 013 * 014 * The procedure is still occurring. 015 */ 016 IN_PROGRESS("in-progress", "http://hl7.org/fhir/procedure-status"), 017 018 /** 019 * Display: <b>Aboted</b><br> 020 * Code Value: <b>aborted</b> 021 * 022 * The procedure was terminated without completing successfully. 023 */ 024 ABOTED("aborted", "http://hl7.org/fhir/procedure-status"), 025 026 /** 027 * Display: <b>Completed</b><br> 028 * Code Value: <b>completed</b> 029 * 030 * All actions involved in the procedure have taken place. 031 */ 032 COMPLETED("completed", "http://hl7.org/fhir/procedure-status"), 033 034 /** 035 * Display: <b>Entered in Error</b><br> 036 * Code Value: <b>entered-in-error</b> 037 * 038 * The statement was entered in error and Is not valid. 039 */ 040 ENTERED_IN_ERROR("entered-in-error", "http://hl7.org/fhir/procedure-status"), 041 042 ; 043 044 /** 045 * Identifier for this Value Set: 046 * 047 */ 048 public static final String VALUESET_IDENTIFIER = ""; 049 050 /** 051 * Name for this Value Set: 052 * ProcedureStatus 053 */ 054 public static final String VALUESET_NAME = "ProcedureStatus"; 055 056 private static Map<String, ProcedureStatusEnum> CODE_TO_ENUM = new HashMap<String, ProcedureStatusEnum>(); 057 private static Map<String, Map<String, ProcedureStatusEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, ProcedureStatusEnum>>(); 058 059 private final String myCode; 060 private final String mySystem; 061 062 static { 063 for (ProcedureStatusEnum next : ProcedureStatusEnum.values()) { 064 CODE_TO_ENUM.put(next.getCode(), next); 065 066 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 067 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, ProcedureStatusEnum>()); 068 } 069 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 070 } 071 } 072 073 /** 074 * Returns the code associated with this enumerated value 075 */ 076 public String getCode() { 077 return myCode; 078 } 079 080 /** 081 * Returns the code system associated with this enumerated value 082 */ 083 public String getSystem() { 084 return mySystem; 085 } 086 087 /** 088 * Returns the enumerated value associated with this code 089 */ 090 public static ProcedureStatusEnum forCode(String theCode) { 091 ProcedureStatusEnum retVal = CODE_TO_ENUM.get(theCode); 092 return retVal; 093 } 094 095 /** 096 * Converts codes to their respective enumerated values 097 */ 098 public static final IValueSetEnumBinder<ProcedureStatusEnum> VALUESET_BINDER = new IValueSetEnumBinder<ProcedureStatusEnum>() { 099 @Override 100 public String toCodeString(ProcedureStatusEnum theEnum) { 101 return theEnum.getCode(); 102 } 103 104 @Override 105 public String toSystemString(ProcedureStatusEnum theEnum) { 106 return theEnum.getSystem(); 107 } 108 109 @Override 110 public ProcedureStatusEnum fromCodeString(String theCodeString) { 111 return CODE_TO_ENUM.get(theCodeString); 112 } 113 114 @Override 115 public ProcedureStatusEnum fromCodeString(String theCodeString, String theSystemString) { 116 Map<String, ProcedureStatusEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 117 if (map == null) { 118 return null; 119 } 120 return map.get(theCodeString); 121 } 122 123 }; 124 125 /** 126 * Constructor 127 */ 128 ProcedureStatusEnum(String theCode, String theSystem) { 129 myCode = theCode; 130 mySystem = theSystem; 131 } 132 133 134}