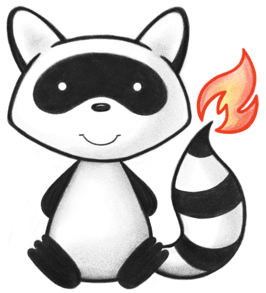
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum PropertyRepresentationEnum { 009 010 /** 011 * Display: <b>XML Attribute</b><br> 012 * Code Value: <b>xmlAttr</b> 013 * 014 * In XML, this property is represented as an attribute not an element. 015 */ 016 XML_ATTRIBUTE("xmlAttr", "http://hl7.org/fhir/property-representation"), 017 018 ; 019 020 /** 021 * Identifier for this Value Set: 022 * 023 */ 024 public static final String VALUESET_IDENTIFIER = ""; 025 026 /** 027 * Name for this Value Set: 028 * PropertyRepresentation 029 */ 030 public static final String VALUESET_NAME = "PropertyRepresentation"; 031 032 private static Map<String, PropertyRepresentationEnum> CODE_TO_ENUM = new HashMap<String, PropertyRepresentationEnum>(); 033 private static Map<String, Map<String, PropertyRepresentationEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, PropertyRepresentationEnum>>(); 034 035 private final String myCode; 036 private final String mySystem; 037 038 static { 039 for (PropertyRepresentationEnum next : PropertyRepresentationEnum.values()) { 040 CODE_TO_ENUM.put(next.getCode(), next); 041 042 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 043 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, PropertyRepresentationEnum>()); 044 } 045 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 046 } 047 } 048 049 /** 050 * Returns the code associated with this enumerated value 051 */ 052 public String getCode() { 053 return myCode; 054 } 055 056 /** 057 * Returns the code system associated with this enumerated value 058 */ 059 public String getSystem() { 060 return mySystem; 061 } 062 063 /** 064 * Returns the enumerated value associated with this code 065 */ 066 public static PropertyRepresentationEnum forCode(String theCode) { 067 PropertyRepresentationEnum retVal = CODE_TO_ENUM.get(theCode); 068 return retVal; 069 } 070 071 /** 072 * Converts codes to their respective enumerated values 073 */ 074 public static final IValueSetEnumBinder<PropertyRepresentationEnum> VALUESET_BINDER = new IValueSetEnumBinder<PropertyRepresentationEnum>() { 075 @Override 076 public String toCodeString(PropertyRepresentationEnum theEnum) { 077 return theEnum.getCode(); 078 } 079 080 @Override 081 public String toSystemString(PropertyRepresentationEnum theEnum) { 082 return theEnum.getSystem(); 083 } 084 085 @Override 086 public PropertyRepresentationEnum fromCodeString(String theCodeString) { 087 return CODE_TO_ENUM.get(theCodeString); 088 } 089 090 @Override 091 public PropertyRepresentationEnum fromCodeString(String theCodeString, String theSystemString) { 092 Map<String, PropertyRepresentationEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 093 if (map == null) { 094 return null; 095 } 096 return map.get(theCodeString); 097 } 098 099 }; 100 101 /** 102 * Constructor 103 */ 104 PropertyRepresentationEnum(String theCode, String theSystem) { 105 myCode = theCode; 106 mySystem = theSystem; 107 } 108 109 110}