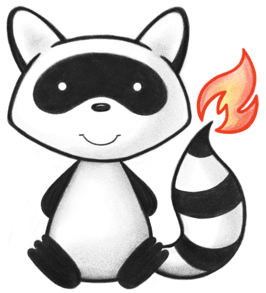
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum ReferralMethodEnum { 009 010 /** 011 * Display: <b>Fax</b><br> 012 * Code Value: <b>fax</b> 013 * 014 * Referrals may be accepted by fax. 015 */ 016 FAX("fax", "http://hl7.org/fhir/service-referral-method"), 017 018 /** 019 * Display: <b>Phone</b><br> 020 * Code Value: <b>phone</b> 021 * 022 * Referrals may be accepted over the phone from a practitioner. 023 */ 024 PHONE("phone", "http://hl7.org/fhir/service-referral-method"), 025 026 /** 027 * Display: <b>Secure Messaging</b><br> 028 * Code Value: <b>elec</b> 029 * 030 * Referrals may be accepted via a secure messaging system. To determine the types of secure messaging systems supported, refer to the identifiers collection. Callers will need to understand the specific identifier system used to know that they are able to transmit messages. 031 */ 032 SECURE_MESSAGING("elec", "http://hl7.org/fhir/service-referral-method"), 033 034 /** 035 * Display: <b>Secure Email</b><br> 036 * Code Value: <b>semail</b> 037 * 038 * Referrals may be accepted via a secure email. To send please enrypt with the services public key. 039 */ 040 SECURE_EMAIL("semail", "http://hl7.org/fhir/service-referral-method"), 041 042 /** 043 * Display: <b>Mail</b><br> 044 * Code Value: <b>mail</b> 045 * 046 * Referrals may be accepted via regular postage (or hand delivered). 047 */ 048 MAIL("mail", "http://hl7.org/fhir/service-referral-method"), 049 050 ; 051 052 /** 053 * Identifier for this Value Set: 054 * 055 */ 056 public static final String VALUESET_IDENTIFIER = ""; 057 058 /** 059 * Name for this Value Set: 060 * ReferralMethod 061 */ 062 public static final String VALUESET_NAME = "ReferralMethod"; 063 064 private static Map<String, ReferralMethodEnum> CODE_TO_ENUM = new HashMap<String, ReferralMethodEnum>(); 065 private static Map<String, Map<String, ReferralMethodEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, ReferralMethodEnum>>(); 066 067 private final String myCode; 068 private final String mySystem; 069 070 static { 071 for (ReferralMethodEnum next : ReferralMethodEnum.values()) { 072 CODE_TO_ENUM.put(next.getCode(), next); 073 074 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 075 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, ReferralMethodEnum>()); 076 } 077 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 078 } 079 } 080 081 /** 082 * Returns the code associated with this enumerated value 083 */ 084 public String getCode() { 085 return myCode; 086 } 087 088 /** 089 * Returns the code system associated with this enumerated value 090 */ 091 public String getSystem() { 092 return mySystem; 093 } 094 095 /** 096 * Returns the enumerated value associated with this code 097 */ 098 public static ReferralMethodEnum forCode(String theCode) { 099 ReferralMethodEnum retVal = CODE_TO_ENUM.get(theCode); 100 return retVal; 101 } 102 103 /** 104 * Converts codes to their respective enumerated values 105 */ 106 public static final IValueSetEnumBinder<ReferralMethodEnum> VALUESET_BINDER = new IValueSetEnumBinder<ReferralMethodEnum>() { 107 @Override 108 public String toCodeString(ReferralMethodEnum theEnum) { 109 return theEnum.getCode(); 110 } 111 112 @Override 113 public String toSystemString(ReferralMethodEnum theEnum) { 114 return theEnum.getSystem(); 115 } 116 117 @Override 118 public ReferralMethodEnum fromCodeString(String theCodeString) { 119 return CODE_TO_ENUM.get(theCodeString); 120 } 121 122 @Override 123 public ReferralMethodEnum fromCodeString(String theCodeString, String theSystemString) { 124 Map<String, ReferralMethodEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 125 if (map == null) { 126 return null; 127 } 128 return map.get(theCodeString); 129 } 130 131 }; 132 133 /** 134 * Constructor 135 */ 136 ReferralMethodEnum(String theCode, String theSystem) { 137 myCode = theCode; 138 mySystem = theSystem; 139 } 140 141 142}