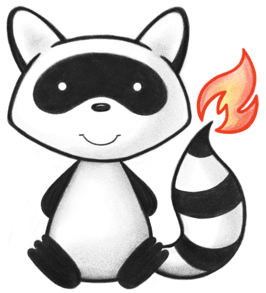
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum RemittanceOutcomeEnum { 009 010 /** 011 * Display: <b>Complete</b><br> 012 * Code Value: <b>complete</b> 013 * 014 * The processing completed without errors. 015 */ 016 COMPLETE("complete", "http://hl7.org/fhir/remittance-outcome"), 017 018 /** 019 * Display: <b>Error</b><br> 020 * Code Value: <b>error</b> 021 * 022 * The processing identified errors. 023 */ 024 ERROR("error", "http://hl7.org/fhir/remittance-outcome"), 025 026 ; 027 028 /** 029 * Identifier for this Value Set: 030 * 031 */ 032 public static final String VALUESET_IDENTIFIER = ""; 033 034 /** 035 * Name for this Value Set: 036 * RemittanceOutcome 037 */ 038 public static final String VALUESET_NAME = "RemittanceOutcome"; 039 040 private static Map<String, RemittanceOutcomeEnum> CODE_TO_ENUM = new HashMap<String, RemittanceOutcomeEnum>(); 041 private static Map<String, Map<String, RemittanceOutcomeEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, RemittanceOutcomeEnum>>(); 042 043 private final String myCode; 044 private final String mySystem; 045 046 static { 047 for (RemittanceOutcomeEnum next : RemittanceOutcomeEnum.values()) { 048 CODE_TO_ENUM.put(next.getCode(), next); 049 050 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 051 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, RemittanceOutcomeEnum>()); 052 } 053 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 054 } 055 } 056 057 /** 058 * Returns the code associated with this enumerated value 059 */ 060 public String getCode() { 061 return myCode; 062 } 063 064 /** 065 * Returns the code system associated with this enumerated value 066 */ 067 public String getSystem() { 068 return mySystem; 069 } 070 071 /** 072 * Returns the enumerated value associated with this code 073 */ 074 public static RemittanceOutcomeEnum forCode(String theCode) { 075 RemittanceOutcomeEnum retVal = CODE_TO_ENUM.get(theCode); 076 return retVal; 077 } 078 079 /** 080 * Converts codes to their respective enumerated values 081 */ 082 public static final IValueSetEnumBinder<RemittanceOutcomeEnum> VALUESET_BINDER = new IValueSetEnumBinder<RemittanceOutcomeEnum>() { 083 @Override 084 public String toCodeString(RemittanceOutcomeEnum theEnum) { 085 return theEnum.getCode(); 086 } 087 088 @Override 089 public String toSystemString(RemittanceOutcomeEnum theEnum) { 090 return theEnum.getSystem(); 091 } 092 093 @Override 094 public RemittanceOutcomeEnum fromCodeString(String theCodeString) { 095 return CODE_TO_ENUM.get(theCodeString); 096 } 097 098 @Override 099 public RemittanceOutcomeEnum fromCodeString(String theCodeString, String theSystemString) { 100 Map<String, RemittanceOutcomeEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 101 if (map == null) { 102 return null; 103 } 104 return map.get(theCodeString); 105 } 106 107 }; 108 109 /** 110 * Constructor 111 */ 112 RemittanceOutcomeEnum(String theCode, String theSystem) { 113 myCode = theCode; 114 mySystem = theSystem; 115 } 116 117 118}