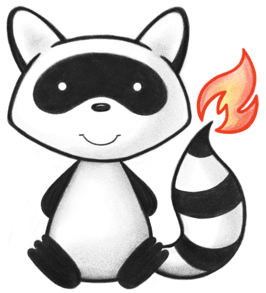
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum RulesetCodesEnum { 009 010 /** 011 * Code Value: <b>x12-4010</b> 012 */ 013 X12_4010("x12-4010", "http://hl7.org/fhir/ruleset"), 014 015 /** 016 * Code Value: <b>x12-5010</b> 017 */ 018 X12_5010("x12-5010", "http://hl7.org/fhir/ruleset"), 019 020 /** 021 * Code Value: <b>x12-7010</b> 022 */ 023 X12_7010("x12-7010", "http://hl7.org/fhir/ruleset"), 024 025 /** 026 * Code Value: <b>cdanet-v2</b> 027 */ 028 CDANET_V2("cdanet-v2", "http://hl7.org/fhir/ruleset"), 029 030 /** 031 * Code Value: <b>cdanet-v4</b> 032 */ 033 CDANET_V4("cdanet-v4", "http://hl7.org/fhir/ruleset"), 034 035 /** 036 * Code Value: <b>cpha-3</b> 037 */ 038 CPHA_3("cpha-3", "http://hl7.org/fhir/ruleset"), 039 040 ; 041 042 /** 043 * Identifier for this Value Set: 044 * 045 */ 046 public static final String VALUESET_IDENTIFIER = ""; 047 048 /** 049 * Name for this Value Set: 050 * Ruleset Codes 051 */ 052 public static final String VALUESET_NAME = "Ruleset Codes"; 053 054 private static Map<String, RulesetCodesEnum> CODE_TO_ENUM = new HashMap<String, RulesetCodesEnum>(); 055 private static Map<String, Map<String, RulesetCodesEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, RulesetCodesEnum>>(); 056 057 private final String myCode; 058 private final String mySystem; 059 060 static { 061 for (RulesetCodesEnum next : RulesetCodesEnum.values()) { 062 CODE_TO_ENUM.put(next.getCode(), next); 063 064 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 065 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, RulesetCodesEnum>()); 066 } 067 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 068 } 069 } 070 071 /** 072 * Returns the code associated with this enumerated value 073 */ 074 public String getCode() { 075 return myCode; 076 } 077 078 /** 079 * Returns the code system associated with this enumerated value 080 */ 081 public String getSystem() { 082 return mySystem; 083 } 084 085 /** 086 * Returns the enumerated value associated with this code 087 */ 088 public static RulesetCodesEnum forCode(String theCode) { 089 RulesetCodesEnum retVal = CODE_TO_ENUM.get(theCode); 090 return retVal; 091 } 092 093 /** 094 * Converts codes to their respective enumerated values 095 */ 096 public static final IValueSetEnumBinder<RulesetCodesEnum> VALUESET_BINDER = new IValueSetEnumBinder<RulesetCodesEnum>() { 097 @Override 098 public String toCodeString(RulesetCodesEnum theEnum) { 099 return theEnum.getCode(); 100 } 101 102 @Override 103 public String toSystemString(RulesetCodesEnum theEnum) { 104 return theEnum.getSystem(); 105 } 106 107 @Override 108 public RulesetCodesEnum fromCodeString(String theCodeString) { 109 return CODE_TO_ENUM.get(theCodeString); 110 } 111 112 @Override 113 public RulesetCodesEnum fromCodeString(String theCodeString, String theSystemString) { 114 Map<String, RulesetCodesEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 115 if (map == null) { 116 return null; 117 } 118 return map.get(theCodeString); 119 } 120 121 }; 122 123 /** 124 * Constructor 125 */ 126 RulesetCodesEnum(String theCode, String theSystem) { 127 myCode = theCode; 128 mySystem = theSystem; 129 } 130 131 132}