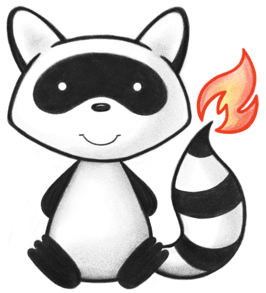
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum SearchEntryModeEnum { 009 010 /** 011 * Display: <b>Match</b><br> 012 * Code Value: <b>match</b> 013 * 014 * This resource matched the search specification. 015 */ 016 MATCH("match", "http://hl7.org/fhir/search-entry-mode"), 017 018 /** 019 * Display: <b>Include</b><br> 020 * Code Value: <b>include</b> 021 * 022 * This resource is returned because it is referred to from another resource in the search set. 023 */ 024 INCLUDE("include", "http://hl7.org/fhir/search-entry-mode"), 025 026 /** 027 * Display: <b>Outcome</b><br> 028 * Code Value: <b>outcome</b> 029 * 030 * An OperationOutcome that provides additional information about the processing of a search. 031 */ 032 OUTCOME("outcome", "http://hl7.org/fhir/search-entry-mode"), 033 034 ; 035 036 /** 037 * Identifier for this Value Set: 038 * 039 */ 040 public static final String VALUESET_IDENTIFIER = ""; 041 042 /** 043 * Name for this Value Set: 044 * SearchEntryMode 045 */ 046 public static final String VALUESET_NAME = "SearchEntryMode"; 047 048 private static Map<String, SearchEntryModeEnum> CODE_TO_ENUM = new HashMap<String, SearchEntryModeEnum>(); 049 private static Map<String, Map<String, SearchEntryModeEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, SearchEntryModeEnum>>(); 050 051 private final String myCode; 052 private final String mySystem; 053 054 static { 055 for (SearchEntryModeEnum next : SearchEntryModeEnum.values()) { 056 CODE_TO_ENUM.put(next.getCode(), next); 057 058 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 059 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, SearchEntryModeEnum>()); 060 } 061 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 062 } 063 } 064 065 /** 066 * Returns the code associated with this enumerated value 067 */ 068 public String getCode() { 069 return myCode; 070 } 071 072 /** 073 * Returns the code system associated with this enumerated value 074 */ 075 public String getSystem() { 076 return mySystem; 077 } 078 079 /** 080 * Returns the enumerated value associated with this code 081 */ 082 public static SearchEntryModeEnum forCode(String theCode) { 083 SearchEntryModeEnum retVal = CODE_TO_ENUM.get(theCode); 084 return retVal; 085 } 086 087 /** 088 * Converts codes to their respective enumerated values 089 */ 090 public static final IValueSetEnumBinder<SearchEntryModeEnum> VALUESET_BINDER = new IValueSetEnumBinder<SearchEntryModeEnum>() { 091 @Override 092 public String toCodeString(SearchEntryModeEnum theEnum) { 093 return theEnum.getCode(); 094 } 095 096 @Override 097 public String toSystemString(SearchEntryModeEnum theEnum) { 098 return theEnum.getSystem(); 099 } 100 101 @Override 102 public SearchEntryModeEnum fromCodeString(String theCodeString) { 103 return CODE_TO_ENUM.get(theCodeString); 104 } 105 106 @Override 107 public SearchEntryModeEnum fromCodeString(String theCodeString, String theSystemString) { 108 Map<String, SearchEntryModeEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 109 if (map == null) { 110 return null; 111 } 112 return map.get(theCodeString); 113 } 114 115 }; 116 117 /** 118 * Constructor 119 */ 120 SearchEntryModeEnum(String theCode, String theSystem) { 121 myCode = theCode; 122 mySystem = theSystem; 123 } 124 125 126}