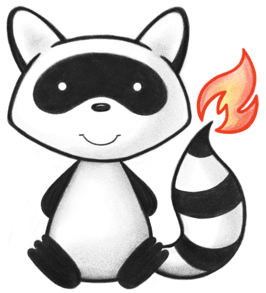
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum SubstanceCategoryCodesEnum { 009 010 /** 011 * Display: <b>Allergen</b><br> 012 * Code Value: <b>allergen</b> 013 * 014 * A substance that causes an allergic reaction. 015 */ 016 ALLERGEN("allergen", "http://hl7.org/fhir/substance-category"), 017 018 /** 019 * Display: <b>Biological Substance</b><br> 020 * Code Value: <b>biological</b> 021 * 022 * A substance that is produced by or extracted from a biological source. 023 */ 024 BIOLOGICAL_SUBSTANCE("biological", "http://hl7.org/fhir/substance-category"), 025 026 /** 027 * Display: <b>Body Substance</b><br> 028 * Code Value: <b>body</b> 029 * 030 * A substance that comes directly from a human or an animal (e.g. blood, urine, feces, tears, etc.). 031 */ 032 BODY_SUBSTANCE("body", "http://hl7.org/fhir/substance-category"), 033 034 /** 035 * Display: <b>Chemical</b><br> 036 * Code Value: <b>chemical</b> 037 * 038 * Any organic or inorganic substance of a particular molecular identity, including -- (i) any combination of such substances occurring in whole or in part as a result of a chemical reaction or occurring in nature and (ii) any element or uncombined radical (http://www.epa.gov/opptintr/import-export/pubs/importguide.pdf). 039 */ 040 CHEMICAL("chemical", "http://hl7.org/fhir/substance-category"), 041 042 /** 043 * Display: <b>Dietary Substance</b><br> 044 * Code Value: <b>food</b> 045 * 046 * A food, dietary ingredient, or dietary supplement for human or animal. 047 */ 048 DIETARY_SUBSTANCE("food", "http://hl7.org/fhir/substance-category"), 049 050 /** 051 * Display: <b>Drug or Medicament</b><br> 052 * Code Value: <b>drug</b> 053 * 054 * A substance intended for use in the diagnosis, cure, mitigation, treatment, or prevention of disease in man or other animals (Federal Food Drug and Cosmetic Act). 055 */ 056 DRUG_OR_MEDICAMENT("drug", "http://hl7.org/fhir/substance-category"), 057 058 /** 059 * Display: <b>Material</b><br> 060 * Code Value: <b>material</b> 061 * 062 * A finished product which is not normally ingested, absorbed or injected (e.g. steel, iron, wood, plastic and paper). 063 */ 064 MATERIAL("material", "http://hl7.org/fhir/substance-category"), 065 066 ; 067 068 /** 069 * Identifier for this Value Set: 070 * 071 */ 072 public static final String VALUESET_IDENTIFIER = ""; 073 074 /** 075 * Name for this Value Set: 076 * Substance Category Codes 077 */ 078 public static final String VALUESET_NAME = "Substance Category Codes"; 079 080 private static Map<String, SubstanceCategoryCodesEnum> CODE_TO_ENUM = new HashMap<String, SubstanceCategoryCodesEnum>(); 081 private static Map<String, Map<String, SubstanceCategoryCodesEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, SubstanceCategoryCodesEnum>>(); 082 083 private final String myCode; 084 private final String mySystem; 085 086 static { 087 for (SubstanceCategoryCodesEnum next : SubstanceCategoryCodesEnum.values()) { 088 CODE_TO_ENUM.put(next.getCode(), next); 089 090 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 091 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, SubstanceCategoryCodesEnum>()); 092 } 093 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 094 } 095 } 096 097 /** 098 * Returns the code associated with this enumerated value 099 */ 100 public String getCode() { 101 return myCode; 102 } 103 104 /** 105 * Returns the code system associated with this enumerated value 106 */ 107 public String getSystem() { 108 return mySystem; 109 } 110 111 /** 112 * Returns the enumerated value associated with this code 113 */ 114 public static SubstanceCategoryCodesEnum forCode(String theCode) { 115 SubstanceCategoryCodesEnum retVal = CODE_TO_ENUM.get(theCode); 116 return retVal; 117 } 118 119 /** 120 * Converts codes to their respective enumerated values 121 */ 122 public static final IValueSetEnumBinder<SubstanceCategoryCodesEnum> VALUESET_BINDER = new IValueSetEnumBinder<SubstanceCategoryCodesEnum>() { 123 @Override 124 public String toCodeString(SubstanceCategoryCodesEnum theEnum) { 125 return theEnum.getCode(); 126 } 127 128 @Override 129 public String toSystemString(SubstanceCategoryCodesEnum theEnum) { 130 return theEnum.getSystem(); 131 } 132 133 @Override 134 public SubstanceCategoryCodesEnum fromCodeString(String theCodeString) { 135 return CODE_TO_ENUM.get(theCodeString); 136 } 137 138 @Override 139 public SubstanceCategoryCodesEnum fromCodeString(String theCodeString, String theSystemString) { 140 Map<String, SubstanceCategoryCodesEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 141 if (map == null) { 142 return null; 143 } 144 return map.get(theCodeString); 145 } 146 147 }; 148 149 /** 150 * Constructor 151 */ 152 SubstanceCategoryCodesEnum(String theCode, String theSystem) { 153 myCode = theCode; 154 mySystem = theSystem; 155 } 156 157 158}