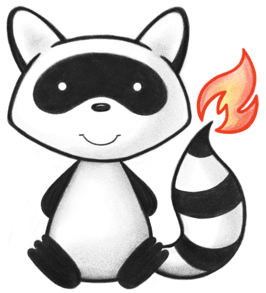
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum SystemRestfulInteractionEnum { 009 010 /** 011 * Code Value: <b>transaction</b> 012 */ 013 TRANSACTION("transaction", "http://hl7.org/fhir/restful-interaction"), 014 015 /** 016 * Code Value: <b>search-system</b> 017 */ 018 SEARCH_SYSTEM("search-system", "http://hl7.org/fhir/restful-interaction"), 019 020 /** 021 * Code Value: <b>history-system</b> 022 */ 023 HISTORY_SYSTEM("history-system", "http://hl7.org/fhir/restful-interaction"), 024 025 ; 026 027 /** 028 * Identifier for this Value Set: 029 * 030 */ 031 public static final String VALUESET_IDENTIFIER = ""; 032 033 /** 034 * Name for this Value Set: 035 * SystemRestfulInteraction 036 */ 037 public static final String VALUESET_NAME = "SystemRestfulInteraction"; 038 039 private static Map<String, SystemRestfulInteractionEnum> CODE_TO_ENUM = new HashMap<String, SystemRestfulInteractionEnum>(); 040 private static Map<String, Map<String, SystemRestfulInteractionEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, SystemRestfulInteractionEnum>>(); 041 042 private final String myCode; 043 private final String mySystem; 044 045 static { 046 for (SystemRestfulInteractionEnum next : SystemRestfulInteractionEnum.values()) { 047 CODE_TO_ENUM.put(next.getCode(), next); 048 049 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 050 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, SystemRestfulInteractionEnum>()); 051 } 052 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 053 } 054 } 055 056 /** 057 * Returns the code associated with this enumerated value 058 */ 059 public String getCode() { 060 return myCode; 061 } 062 063 /** 064 * Returns the code system associated with this enumerated value 065 */ 066 public String getSystem() { 067 return mySystem; 068 } 069 070 /** 071 * Returns the enumerated value associated with this code 072 */ 073 public static SystemRestfulInteractionEnum forCode(String theCode) { 074 SystemRestfulInteractionEnum retVal = CODE_TO_ENUM.get(theCode); 075 return retVal; 076 } 077 078 /** 079 * Converts codes to their respective enumerated values 080 */ 081 public static final IValueSetEnumBinder<SystemRestfulInteractionEnum> VALUESET_BINDER = new IValueSetEnumBinder<SystemRestfulInteractionEnum>() { 082 @Override 083 public String toCodeString(SystemRestfulInteractionEnum theEnum) { 084 return theEnum.getCode(); 085 } 086 087 @Override 088 public String toSystemString(SystemRestfulInteractionEnum theEnum) { 089 return theEnum.getSystem(); 090 } 091 092 @Override 093 public SystemRestfulInteractionEnum fromCodeString(String theCodeString) { 094 return CODE_TO_ENUM.get(theCodeString); 095 } 096 097 @Override 098 public SystemRestfulInteractionEnum fromCodeString(String theCodeString, String theSystemString) { 099 Map<String, SystemRestfulInteractionEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 100 if (map == null) { 101 return null; 102 } 103 return map.get(theCodeString); 104 } 105 106 }; 107 108 /** 109 * Constructor 110 */ 111 SystemRestfulInteractionEnum(String theCode, String theSystem) { 112 myCode = theCode; 113 mySystem = theSystem; 114 } 115 116 117}