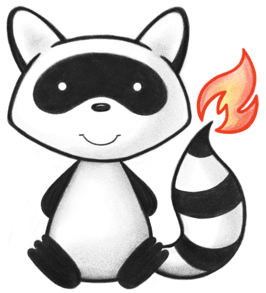
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum TimingAbbreviationEnum { 009 010 /** 011 * Display: <b>QD</b><br> 012 * Code Value: <b>QD</b> 013 * 014 * Every Day at institution specified times 015 */ 016 QD("QD", "http://hl7.org/fhir/timing-abbreviation"), 017 018 /** 019 * Display: <b>QOD</b><br> 020 * Code Value: <b>QOD</b> 021 * 022 * Every Other Day at institution specified times 023 */ 024 QOD("QOD", "http://hl7.org/fhir/timing-abbreviation"), 025 026 /** 027 * Display: <b>Q4H</b><br> 028 * Code Value: <b>Q4H</b> 029 * 030 * Every 4 hours at institution specified times 031 */ 032 Q4H("Q4H", "http://hl7.org/fhir/timing-abbreviation"), 033 034 /** 035 * Display: <b>Q6H</b><br> 036 * Code Value: <b>Q6H</b> 037 * 038 * Every 6 Hours at institution specified times 039 */ 040 Q6H("Q6H", "http://hl7.org/fhir/timing-abbreviation"), 041 042 /** 043 * Code Value: <b>BID</b> 044 */ 045 BID("BID", "http://hl7.org/fhir/v3/GTSAbbreviation"), 046 047 /** 048 * Code Value: <b>TID</b> 049 */ 050 TID("TID", "http://hl7.org/fhir/v3/GTSAbbreviation"), 051 052 /** 053 * Code Value: <b>QID</b> 054 */ 055 QID("QID", "http://hl7.org/fhir/v3/GTSAbbreviation"), 056 057 /** 058 * Code Value: <b>AM</b> 059 */ 060 AM("AM", "http://hl7.org/fhir/v3/GTSAbbreviation"), 061 062 /** 063 * Code Value: <b>PM</b> 064 */ 065 PM("PM", "http://hl7.org/fhir/v3/GTSAbbreviation"), 066 067 ; 068 069 /** 070 * Identifier for this Value Set: 071 * 072 */ 073 public static final String VALUESET_IDENTIFIER = ""; 074 075 /** 076 * Name for this Value Set: 077 * TimingAbbreviation 078 */ 079 public static final String VALUESET_NAME = "TimingAbbreviation"; 080 081 private static Map<String, TimingAbbreviationEnum> CODE_TO_ENUM = new HashMap<String, TimingAbbreviationEnum>(); 082 private static Map<String, Map<String, TimingAbbreviationEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, TimingAbbreviationEnum>>(); 083 084 private final String myCode; 085 private final String mySystem; 086 087 static { 088 for (TimingAbbreviationEnum next : TimingAbbreviationEnum.values()) { 089 CODE_TO_ENUM.put(next.getCode(), next); 090 091 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 092 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, TimingAbbreviationEnum>()); 093 } 094 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 095 } 096 } 097 098 /** 099 * Returns the code associated with this enumerated value 100 */ 101 public String getCode() { 102 return myCode; 103 } 104 105 /** 106 * Returns the code system associated with this enumerated value 107 */ 108 public String getSystem() { 109 return mySystem; 110 } 111 112 /** 113 * Returns the enumerated value associated with this code 114 */ 115 public static TimingAbbreviationEnum forCode(String theCode) { 116 TimingAbbreviationEnum retVal = CODE_TO_ENUM.get(theCode); 117 return retVal; 118 } 119 120 /** 121 * Converts codes to their respective enumerated values 122 */ 123 public static final IValueSetEnumBinder<TimingAbbreviationEnum> VALUESET_BINDER = new IValueSetEnumBinder<TimingAbbreviationEnum>() { 124 @Override 125 public String toCodeString(TimingAbbreviationEnum theEnum) { 126 return theEnum.getCode(); 127 } 128 129 @Override 130 public String toSystemString(TimingAbbreviationEnum theEnum) { 131 return theEnum.getSystem(); 132 } 133 134 @Override 135 public TimingAbbreviationEnum fromCodeString(String theCodeString) { 136 return CODE_TO_ENUM.get(theCodeString); 137 } 138 139 @Override 140 public TimingAbbreviationEnum fromCodeString(String theCodeString, String theSystemString) { 141 Map<String, TimingAbbreviationEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 142 if (map == null) { 143 return null; 144 } 145 return map.get(theCodeString); 146 } 147 148 }; 149 150 /** 151 * Constructor 152 */ 153 TimingAbbreviationEnum(String theCode, String theSystem) { 154 myCode = theCode; 155 mySystem = theSystem; 156 } 157 158 159}