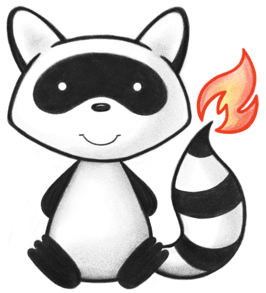
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum UnitsOfTimeEnum { 009 010 /** 011 * Code Value: <b>s</b> 012 */ 013 S("s", "http://unitsofmeasure.org"), 014 015 /** 016 * Code Value: <b>min</b> 017 */ 018 MIN("min", "http://unitsofmeasure.org"), 019 020 /** 021 * Code Value: <b>h</b> 022 */ 023 H("h", "http://unitsofmeasure.org"), 024 025 /** 026 * Code Value: <b>d</b> 027 */ 028 D("d", "http://unitsofmeasure.org"), 029 030 /** 031 * Code Value: <b>wk</b> 032 */ 033 WK("wk", "http://unitsofmeasure.org"), 034 035 /** 036 * Code Value: <b>mo</b> 037 */ 038 MO("mo", "http://unitsofmeasure.org"), 039 040 /** 041 * Code Value: <b>a</b> 042 */ 043 A("a", "http://unitsofmeasure.org"), 044 045 ; 046 047 /** 048 * Identifier for this Value Set: 049 * 050 */ 051 public static final String VALUESET_IDENTIFIER = ""; 052 053 /** 054 * Name for this Value Set: 055 * UnitsOfTime 056 */ 057 public static final String VALUESET_NAME = "UnitsOfTime"; 058 059 private static Map<String, UnitsOfTimeEnum> CODE_TO_ENUM = new HashMap<String, UnitsOfTimeEnum>(); 060 private static Map<String, Map<String, UnitsOfTimeEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, UnitsOfTimeEnum>>(); 061 062 private final String myCode; 063 private final String mySystem; 064 065 static { 066 for (UnitsOfTimeEnum next : UnitsOfTimeEnum.values()) { 067 CODE_TO_ENUM.put(next.getCode(), next); 068 069 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 070 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, UnitsOfTimeEnum>()); 071 } 072 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 073 } 074 } 075 076 /** 077 * Returns the code associated with this enumerated value 078 */ 079 public String getCode() { 080 return myCode; 081 } 082 083 /** 084 * Returns the code system associated with this enumerated value 085 */ 086 public String getSystem() { 087 return mySystem; 088 } 089 090 /** 091 * Returns the enumerated value associated with this code 092 */ 093 public static UnitsOfTimeEnum forCode(String theCode) { 094 UnitsOfTimeEnum retVal = CODE_TO_ENUM.get(theCode); 095 return retVal; 096 } 097 098 /** 099 * Converts codes to their respective enumerated values 100 */ 101 public static final IValueSetEnumBinder<UnitsOfTimeEnum> VALUESET_BINDER = new IValueSetEnumBinder<UnitsOfTimeEnum>() { 102 @Override 103 public String toCodeString(UnitsOfTimeEnum theEnum) { 104 return theEnum.getCode(); 105 } 106 107 @Override 108 public String toSystemString(UnitsOfTimeEnum theEnum) { 109 return theEnum.getSystem(); 110 } 111 112 @Override 113 public UnitsOfTimeEnum fromCodeString(String theCodeString) { 114 return CODE_TO_ENUM.get(theCodeString); 115 } 116 117 @Override 118 public UnitsOfTimeEnum fromCodeString(String theCodeString, String theSystemString) { 119 Map<String, UnitsOfTimeEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 120 if (map == null) { 121 return null; 122 } 123 return map.get(theCodeString); 124 } 125 126 }; 127 128 /** 129 * Constructor 130 */ 131 UnitsOfTimeEnum(String theCode, String theSystem) { 132 myCode = theCode; 133 mySystem = theSystem; 134 } 135 136 137}