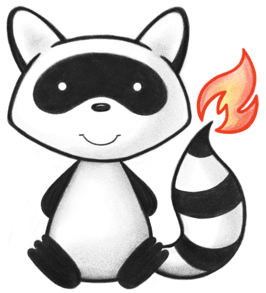
001package org.hl7.fhir.dstu3.context; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032 033 034import java.util.List; 035import java.util.Locale; 036import java.util.Set; 037 038import org.hl7.fhir.dstu3.formats.IParser; 039import org.hl7.fhir.dstu3.formats.ParserType; 040import org.hl7.fhir.dstu3.model.CodeSystem; 041import org.hl7.fhir.dstu3.model.CodeSystem.ConceptDefinitionComponent; 042import org.hl7.fhir.dstu3.model.CodeableConcept; 043import org.hl7.fhir.dstu3.model.Coding; 044import org.hl7.fhir.dstu3.model.ConceptMap; 045import org.hl7.fhir.dstu3.model.ExpansionProfile; 046import org.hl7.fhir.dstu3.model.MetadataResource; 047import org.hl7.fhir.dstu3.model.Resource; 048import org.hl7.fhir.dstu3.model.StructureDefinition; 049import org.hl7.fhir.dstu3.model.ValueSet; 050import org.hl7.fhir.dstu3.model.ValueSet.ConceptSetComponent; 051import org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionComponent; 052import org.hl7.fhir.dstu3.terminologies.ValueSetExpander.TerminologyServiceErrorClass; 053import org.hl7.fhir.dstu3.terminologies.ValueSetExpander.ValueSetExpansionOutcome; 054import org.hl7.fhir.dstu3.utils.INarrativeGenerator; 055import org.hl7.fhir.dstu3.utils.validation.IResourceValidator; 056import org.hl7.fhir.exceptions.FHIRException; 057import org.hl7.fhir.exceptions.TerminologyServiceException; 058import org.hl7.fhir.utilities.validation.ValidationMessage.IssueSeverity; 059 060 061/** 062 * This is the standard interface used for access to underlying FHIR 063 * services through the tools and utilities provided by the reference 064 * implementation. 065 * 066 * The functionality it provides is 067 * - get access to parsers, validators, narrative builders etc 068 * (you can't create these directly because they need access 069 * to the right context for their information) 070 * 071 * - find resources that the tools need to carry out their tasks 072 * 073 * - provide access to terminology services they need. 074 * (typically, these terminology service requests are just 075 * passed through to the local implementation's terminology 076 * service) 077 * 078 * @author Grahame 079 */ 080 081public interface IWorkerContext { 082 083 /** 084 * Get the versions of the definitions loaded in context 085 * @return 086 */ 087 public String getVersion(); 088 089 // -- Parsers (read and write instances) ---------------------------------------- 090 091 092 /** 093 * Get a parser to read/write instances. Use the defined type (will be extended 094 * as further types are added, though the only currently anticipate type is RDF) 095 * 096 * XML/JSON - the standard renderers 097 * XHTML - render the narrative only (generate it if necessary) 098 * 099 * @param type 100 * @return 101 */ 102 public IParser getParser(ParserType type); 103 104 /** 105 * Get a parser to read/write instances. Determine the type 106 * from the stated type. Supported value for type: 107 * - the recommended MIME types 108 * - variants of application/xml and application/json 109 * - _format values xml, json 110 * 111 * @param type 112 * @return 113 */ 114 public IParser getParser(String type); 115 116 /** 117 * Get a JSON parser 118 * 119 * @return 120 */ 121 public IParser newJsonParser(); 122 123 /** 124 * Get an XML parser 125 * 126 * @return 127 */ 128 public IParser newXmlParser(); 129 130 /** 131 * Get a generator that can generate narrative for the instance 132 * 133 * @return a prepared generator 134 */ 135 public INarrativeGenerator getNarrativeGenerator(String prefix, String basePath); 136 137 /** 138 * Get a validator that can check whether a resource is valid 139 * 140 * @return a prepared generator 141 * @throws FHIRException 142 * @ 143 */ 144 public IResourceValidator newValidator() throws FHIRException; 145 146 // -- resource fetchers --------------------------------------------------- 147 148 /** 149 * Find an identified resource. The most common use of this is to access the the 150 * standard conformance resources that are part of the standard - structure 151 * definitions, value sets, concept maps, etc. 152 * 153 * Also, the narrative generator uses this, and may access any kind of resource 154 * 155 * The URI is called speculatively for things that might exist, so not finding 156 * a matching resouce, return null, not an error 157 * 158 * The URI can have one of 3 formats: 159 * - a full URL e.g. http://acme.org/fhir/ValueSet/[id] 160 * - a relative URL e.g. ValueSet/[id] 161 * - a logical id e.g. [id] 162 * 163 * It's an error if the second form doesn't agree with class_. It's an 164 * error if class_ is null for the last form 165 * 166 * @param resource 167 * @param Reference 168 * @return 169 * @throws FHIRException 170 * @throws Exception 171 */ 172 public <T extends Resource> T fetchResource(Class<T> class_, String uri); 173 public <T extends Resource> T fetchResourceWithException(Class<T> class_, String uri) throws FHIRException; 174 175 /** 176 * find whether a resource is available. 177 * 178 * Implementations of the interface can assume that if hasResource ruturns 179 * true, the resource will usually be fetched subsequently 180 * 181 * @param class_ 182 * @param uri 183 * @return 184 */ 185 public <T extends Resource> boolean hasResource(Class<T> class_, String uri); 186 187 // -- profile services --------------------------------------------------------- 188 189 public List<String> getResourceNames(); 190 public Set<String> getResourceNamesAsSet(); 191 public List<String> getTypeNames(); 192 public List<StructureDefinition> allStructures(); 193 public List<MetadataResource> allConformanceResources(); 194 195 // -- Terminology services ------------------------------------------------------ 196 197 public ExpansionProfile getExpansionProfile(); 198 public void setExpansionProfile(ExpansionProfile expProfile); 199 200 // these are the terminology services used internally by the tools 201 /** 202 * Find the code system definition for the nominated system uri. 203 * return null if there isn't one (then the tool might try 204 * supportsSystem) 205 * 206 * @param system 207 * @return 208 */ 209 public CodeSystem fetchCodeSystem(String system); 210 211 /** 212 * True if the underlying terminology service provider will do 213 * expansion and code validation for the terminology. Corresponds 214 * to the extension 215 * 216 * http://hl7.org/fhir/StructureDefinition/capabilitystatement-supported-system 217 * 218 * in the Conformance resource 219 * 220 * @param system 221 * @return 222 * @throws Exception 223 */ 224 public boolean supportsSystem(String system) throws TerminologyServiceException; 225 226 /** 227 * find concept maps for a source 228 * @param url 229 * @return 230 */ 231 public List<ConceptMap> findMapsForSource(String url); 232 233 /** 234 * ValueSet Expansion - see $expand 235 * 236 * @param source 237 * @return 238 */ 239 public ValueSetExpansionOutcome expandVS(ValueSet source, boolean cacheOk, boolean heiarchical); 240 241 /** 242 * Value set expanion inside the internal expansion engine - used 243 * for references to supported system (see "supportsSystem") for 244 * which there is no value set. 245 * 246 * @param inc 247 * @return 248 * @throws FHIRException 249 */ 250 public ValueSetExpansionComponent expandVS(ConceptSetComponent inc, boolean heiarchical) throws TerminologyServiceException; 251 252 Locale getLocale(); 253 254 void setLocale(Locale locale); 255 256 String formatMessage(String theMessage, Object... theMessageArguments); 257 258 void setValidationMessageLanguage(Locale locale); 259 260 public class ValidationResult { 261 private ConceptDefinitionComponent definition; 262 private IssueSeverity severity; 263 private String message; 264 private TerminologyServiceErrorClass errorClass; 265 266 public ValidationResult(IssueSeverity severity, String message) { 267 this.severity = severity; 268 this.message = message; 269 } 270 271 public ValidationResult(ConceptDefinitionComponent definition) { 272 this.definition = definition; 273 } 274 275 public ValidationResult(IssueSeverity severity, String message, ConceptDefinitionComponent definition) { 276 this.severity = severity; 277 this.message = message; 278 this.definition = definition; 279 } 280 281 public ValidationResult(IssueSeverity severity, String message, TerminologyServiceErrorClass errorClass) { 282 this.severity = severity; 283 this.message = message; 284 this.errorClass = errorClass; 285 } 286 287 public boolean isOk() { 288 return definition != null; 289 } 290 291 public String getDisplay() { 292// We don't want to return question-marks because that prevents something more useful from being displayed (e.g. the code) if there's no display value 293// return definition == null ? "??" : definition.getDisplay(); 294 return definition == null ? null : definition.getDisplay(); 295 } 296 297 public ConceptDefinitionComponent asConceptDefinition() { 298 return definition; 299 } 300 301 public IssueSeverity getSeverity() { 302 return severity; 303 } 304 305 public String getMessage() { 306 return message; 307 } 308 309 public boolean IsNoService() { 310 return errorClass == TerminologyServiceErrorClass.NOSERVICE; 311 } 312 313 public TerminologyServiceErrorClass getErrorClass() { 314 return errorClass; 315 } 316 317 318 } 319 320 /** 321 * Validation of a code - consult the terminology service 322 * to see whether it is known. If known, return a description of it 323 * 324 * note: always return a result, with either an error or a code description 325 * 326 * corresponds to 2 terminology service calls: $validate-code and $lookup 327 * 328 * @param system 329 * @param code 330 * @param display 331 * @return 332 */ 333 public ValidationResult validateCode(String system, String code, String display); 334 335 /** 336 * Validation of a code - consult the terminology service 337 * to see whether it is known. If known, return a description of it 338 * Also, check whether it's in the provided value set 339 * 340 * note: always return a result, with either an error or a code description, or both (e.g. known code, but not in the value set) 341 * 342 * corresponds to 2 terminology service calls: $validate-code and $lookup 343 * 344 * @param system 345 * @param code 346 * @param display 347 * @return 348 */ 349 public ValidationResult validateCode(String system, String code, String display, ValueSet vs); 350 public ValidationResult validateCode(Coding code, ValueSet vs); 351 public ValidationResult validateCode(CodeableConcept code, ValueSet vs); 352 353 /** 354 * Validation of a code - consult the terminology service 355 * to see whether it is known. If known, return a description of it 356 * Also, check whether it's in the provided value set fragment (for supported systems with no value set definition) 357 * 358 * note: always return a result, with either an error or a code description, or both (e.g. known code, but not in the value set) 359 * 360 * corresponds to 2 terminology service calls: $validate-code and $lookup 361 * 362 * @param system 363 * @param code 364 * @param display 365 * @return 366 */ 367 public ValidationResult validateCode(String system, String code, String display, ConceptSetComponent vsi); 368 369 /** 370 * returns the recommended tla for the type 371 * 372 * @param name 373 * @return 374 */ 375 public String getAbbreviation(String name); 376 377 // return a set of types that have tails 378 public Set<String> typeTails(); 379 380 public String oid2Uri(String code); 381 382 public boolean hasCache(); 383 384 public interface ILoggingService { 385 public enum LogCategory { 386 PROGRESS, TX, INIT, CONTEXT, HTML 387 } 388 public void logMessage(String message); // status messages, always display 389 public void logDebugMessage(LogCategory category, String message); // verbose; only when debugging 390 } 391 392 public void setLogger(ILoggingService logger); 393 394 public boolean isNoTerminologyServer(); 395 public StructureDefinition fetchTypeDefinition(String typeName); 396 397}