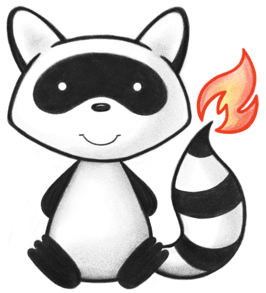
001package org.hl7.fhir.dstu3.elementmodel; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032 033 034import java.io.ByteArrayInputStream; 035import java.io.ByteArrayOutputStream; 036import java.io.IOException; 037import java.util.ArrayList; 038import java.util.List; 039 040import org.hl7.fhir.dstu3.conformance.ProfileUtilities; 041import org.hl7.fhir.dstu3.context.IWorkerContext; 042import org.hl7.fhir.dstu3.formats.IParser.OutputStyle; 043import org.hl7.fhir.dstu3.model.Base; 044import org.hl7.fhir.dstu3.model.CodeableConcept; 045import org.hl7.fhir.dstu3.model.Coding; 046import org.hl7.fhir.dstu3.model.ElementDefinition; 047import org.hl7.fhir.dstu3.model.Factory; 048import org.hl7.fhir.dstu3.model.Identifier; 049import org.hl7.fhir.dstu3.model.PrimitiveType; 050import org.hl7.fhir.dstu3.model.Reference; 051import org.hl7.fhir.dstu3.model.Resource; 052import org.hl7.fhir.dstu3.model.StructureDefinition; 053import org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionKind; 054import org.hl7.fhir.dstu3.model.Type; 055import org.hl7.fhir.exceptions.DefinitionException; 056import org.hl7.fhir.exceptions.FHIRException; 057import org.hl7.fhir.exceptions.FHIRFormatError; 058 059 060 061@Deprecated 062public class ObjectConverter { 063 064 private IWorkerContext context; 065 066 public ObjectConverter(IWorkerContext context) { 067 this.context = context; 068 } 069 070 public Element convert(Resource ig) throws IOException, FHIRFormatError, DefinitionException { 071 if (ig == null) 072 return null; 073 ByteArrayOutputStream bs = new ByteArrayOutputStream(); 074 org.hl7.fhir.dstu3.formats.JsonParser jp = new org.hl7.fhir.dstu3.formats.JsonParser(); 075 jp.compose(bs, ig); 076 ByteArrayInputStream bi = new ByteArrayInputStream(bs.toByteArray()); 077 return new JsonParser(context).parse(bi); 078 } 079 080 public Element convert(Property property, Type type) throws FHIRException { 081 return convertElement(property, type); 082 } 083 084 private Element convertElement(Property property, Base base) throws FHIRException { 085 if (base == null) 086 return null; 087 String tn = base.fhirType(); 088 StructureDefinition sd = context.fetchTypeDefinition(tn); 089 if (sd == null) 090 throw new FHIRException("Unable to find definition for type "+tn); 091 Element res = new Element(property.getName(), property); 092 if (sd.getKind() == StructureDefinitionKind.PRIMITIVETYPE) 093 res.setValue(((PrimitiveType) base).asStringValue()); 094 095 List<ElementDefinition> children = ProfileUtilities.getChildMap(sd, sd.getSnapshot().getElementFirstRep()); 096 for (ElementDefinition child : children) { 097 String n = tail(child.getPath()); 098 if (sd.getKind() != StructureDefinitionKind.PRIMITIVETYPE || !"value".equals(n)) { 099 Base[] values = base.getProperty(n.hashCode(), n, false); 100 if (values != null) 101 for (Base value : values) { 102 res.getChildren().add(convertElement(new Property(context, child, sd), value)); 103 } 104 } 105 } 106 return res; 107 } 108 109 private String tail(String path) { 110 if (path.contains(".")) 111 return path.substring(path.lastIndexOf('.')+1); 112 else 113 return path; 114 } 115 116 public Type convertToType(Element element) throws FHIRException { 117 Type b = new Factory().create(element.fhirType()); 118 if (b instanceof PrimitiveType) { 119 ((PrimitiveType) b).setValueAsString(element.primitiveValue()); 120 } else { 121 for (Element child : element.getChildren()) { 122 b.setProperty(child.getName(), convertToType(child)); 123 } 124 } 125 return b; 126 } 127 128 public Resource convert(Element element) throws FHIRException { 129 ByteArrayOutputStream bo = new ByteArrayOutputStream(); 130 try { 131 new JsonParser(context).compose(element, bo, OutputStyle.NORMAL, null); 132 return new org.hl7.fhir.dstu3.formats.JsonParser().parse(bo.toByteArray()); 133 } catch (IOException e) { 134 // won't happen 135 throw new FHIRException(e); 136 } 137 138 } 139 140 public static CodeableConcept readAsCodeableConcept(Element element) { 141 CodeableConcept cc = new CodeableConcept(); 142 List<Element> list = new ArrayList<Element>(); 143 element.getNamedChildren("coding", list); 144 for (Element item : list) 145 cc.addCoding(readAsCoding(item)); 146 cc.setText(element.getNamedChildValue("text")); 147 return cc; 148 } 149 150 public static Coding readAsCoding(Element item) { 151 Coding c = new Coding(); 152 c.setSystem(item.getNamedChildValue("system")); 153 c.setVersion(item.getNamedChildValue("version")); 154 c.setCode(item.getNamedChildValue("code")); 155 c.setDisplay(item.getNamedChildValue("display")); 156 return c; 157 } 158 159 public static Identifier readAsIdentifier(Element item) { 160 Identifier r = new Identifier(); 161 r.setSystem(item.getNamedChildValue("system")); 162 r.setValue(item.getNamedChildValue("value")); 163 return r; 164 } 165 166 public static Reference readAsReference(Element item) { 167 Reference r = new Reference(); 168 r.setDisplay(item.getNamedChildValue("display")); 169 r.setReference(item.getNamedChildValue("reference")); 170 List<Element> identifier = item.getChildrenByName("identifier"); 171 if (identifier.isEmpty() == false) { 172 r.setIdentifier(readAsIdentifier(identifier.get(0))); 173 } 174 return r; 175 } 176 177}