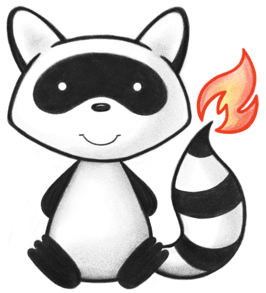
001package org.hl7.fhir.dstu3.elementmodel; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032 033 034import java.io.IOException; 035import java.io.InputStream; 036import java.io.OutputStream; 037import java.util.List; 038 039import org.hl7.fhir.dstu3.context.IWorkerContext; 040import org.hl7.fhir.dstu3.formats.FormatUtilities; 041import org.hl7.fhir.dstu3.formats.IParser.OutputStyle; 042import org.hl7.fhir.dstu3.model.StructureDefinition; 043import org.hl7.fhir.dstu3.utils.ToolingExtensions; 044import org.hl7.fhir.exceptions.DefinitionException; 045import org.hl7.fhir.exceptions.FHIRException; 046import org.hl7.fhir.exceptions.FHIRFormatError; 047import org.hl7.fhir.utilities.Utilities; 048import org.hl7.fhir.utilities.validation.ValidationMessage; 049import org.hl7.fhir.utilities.validation.ValidationMessage.IssueSeverity; 050import org.hl7.fhir.utilities.validation.ValidationMessage.IssueType; 051import org.hl7.fhir.utilities.validation.ValidationMessage.Source; 052 053@Deprecated 054public abstract class ParserBase { 055 056 public interface ILinkResolver { 057 String resolveType(String type); 058 String resolveProperty(Property property); 059 String resolvePage(String string); 060 } 061 062 public enum ValidationPolicy { NONE, QUICK, EVERYTHING } 063 064 public boolean isPrimitive(String code) { 065 return Utilities.existsInList(code, "boolean", "integer", "string", "decimal", "uri", "base64Binary", "instant", "date", "dateTime", "time", "code", "oid", "id", "markdown", "unsignedInt", "positiveInt", "xhtml"); 066 067// StructureDefinition sd = context.fetchTypeDefinition(code); 068// return sd != null && sd.getKind() == StructureDefinitionKind.PRIMITIVETYPE; 069 } 070 071 protected IWorkerContext context; 072 protected ValidationPolicy policy; 073 protected List<ValidationMessage> errors; 074 protected ILinkResolver linkResolver; 075 076 public ParserBase(IWorkerContext context) { 077 super(); 078 this.context = context; 079 policy = ValidationPolicy.NONE; 080 } 081 082 public void setupValidation(ValidationPolicy policy, List<ValidationMessage> errors) { 083 this.policy = policy; 084 this.errors = errors; 085 } 086 087 public abstract Element parse(InputStream stream) throws IOException, FHIRFormatError, DefinitionException, FHIRException; 088 089 public abstract void compose(Element e, OutputStream destination, OutputStyle style, String base) throws FHIRException, IOException; 090 091 092 public void logError(int line, int col, String path, IssueType type, String message, IssueSeverity level) throws FHIRFormatError { 093 if (policy == ValidationPolicy.EVERYTHING) { 094 ValidationMessage msg = new ValidationMessage(Source.InstanceValidator, type, line, col, path, message, level); 095 errors.add(msg); 096 } else if (level == IssueSeverity.FATAL || (level == IssueSeverity.ERROR && policy == ValidationPolicy.QUICK)) 097 throw new FHIRFormatError(message+String.format(" at line %d col %d", line, col)); 098 } 099 100 101 protected StructureDefinition getDefinition(int line, int col, String ns, String name) throws FHIRFormatError { 102 if (ns == null) { 103 logError(line, col, name, IssueType.STRUCTURE, "This cannot be parsed as a FHIR object (no namespace)", IssueSeverity.FATAL); 104 return null; 105 } 106 if (name == null) { 107 logError(line, col, name, IssueType.STRUCTURE, "This cannot be parsed as a FHIR object (no name)", IssueSeverity.FATAL); 108 return null; 109 } 110 for (StructureDefinition sd : context.allStructures()) { 111 if (name.equals(sd.getIdElement().getIdPart())) { 112 if((ns == null || ns.equals(FormatUtilities.FHIR_NS)) && !ToolingExtensions.hasExtension(sd, "http://hl7.org/fhir/StructureDefinition/elementdefinition-namespace")) 113 return sd; 114 String sns = ToolingExtensions.readStringExtension(sd, "http://hl7.org/fhir/StructureDefinition/elementdefinition-namespace"); 115 if (ns != null && ns.equals(sns)) 116 return sd; 117 } 118 } 119 logError(line, col, name, IssueType.STRUCTURE, "This does not appear to be a FHIR resource (unknown namespace/name '"+ns+"::"+name+"')", IssueSeverity.FATAL); 120 return null; 121 } 122 123 protected StructureDefinition getDefinition(int line, int col, String name) throws FHIRFormatError { 124 if (name == null) { 125 logError(line, col, name, IssueType.STRUCTURE, "This cannot be parsed as a FHIR object (no name)", IssueSeverity.FATAL); 126 return null; 127 } 128 // first pass: only look at base definitions 129 for (StructureDefinition sd : context.allStructures()) { 130 if (sd.getUrl().equals("http://hl7.org/fhir/StructureDefinition/"+name)) { 131 return sd; 132 } 133 } 134 for (StructureDefinition sd : context.allStructures()) { 135 if (name.equals(sd.getIdElement().getIdPart())) { 136 return sd; 137 } 138 } 139 logError(line, col, name, IssueType.STRUCTURE, "This does not appear to be a FHIR resource (unknown name '"+name+"')", IssueSeverity.FATAL); 140 return null; 141 } 142 143 public ILinkResolver getLinkResolver() { 144 return linkResolver; 145 } 146 147 public ParserBase setLinkResolver(ILinkResolver linkResolver) { 148 this.linkResolver = linkResolver; 149 return this; 150 } 151 152 153 154 155}