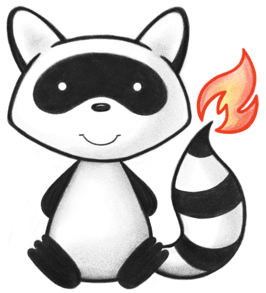
001package org.hl7.fhir.dstu3.fhirpath; 002 003import java.util.HashMap; 004import java.util.List; 005import java.util.Map; 006 007import org.hl7.fhir.dstu3.model.Base; 008import org.hl7.fhir.exceptions.FHIRException; 009 010public class FHIRPathUtilityClasses { 011 012 013 public static class ExecutionContext { 014 private Object appInfo; 015 private Base resource; 016 private Base context; 017 private Base thisItem; 018 private Map<String, Base> aliases; 019 020 public ExecutionContext(Object appInfo, Base resource, Base context, Map<String, Base> aliases, Base thisItem) { 021 this.appInfo = appInfo; 022 this.context = context; 023 this.resource = resource; 024 this.aliases = aliases; 025 this.thisItem = thisItem; 026 } 027 public Base getResource() { 028 return resource; 029 } 030 public Base getThisItem() { 031 return thisItem; 032 } 033 public void addAlias(String name, List<Base> focus) throws FHIRException { 034 if (aliases == null) 035 aliases = new HashMap<String, Base>(); 036 else 037 aliases = new HashMap<String, Base>(aliases); // clone it, since it's going to change 038 if (focus.size() > 1) 039 throw new FHIRException("Attempt to alias a collection, not a singleton"); 040 aliases.put(name, focus.size() == 0 ? null : focus.get(0)); 041 } 042 public Base getAlias(String name) { 043 return aliases == null ? null : aliases.get(name); 044 } 045 public Object getAppInfo() { 046 return appInfo; 047 } 048 public Base getContext() { 049 return context; 050 } 051 public Map<String, Base> getAliases() { 052 return aliases; 053 } 054 055 } 056 057 public static class ExecutionTypeContext { 058 private Object appInfo; 059 private String resource; 060 private String context; 061 private TypeDetails thisItem; 062 063 064 public ExecutionTypeContext(Object appInfo, String resource, String context, TypeDetails thisItem) { 065 super(); 066 this.appInfo = appInfo; 067 this.resource = resource; 068 this.context = context; 069 this.thisItem = thisItem; 070 071 } 072 public String getResource() { 073 return resource; 074 } 075 public TypeDetails getThisItem() { 076 return thisItem; 077 } 078 public Object getAppInfo() { 079 return appInfo; 080 } 081 public String getContext() { 082 return context; 083 } 084 085 086 } 087 088 public static class FunctionDetails { 089 private String description; 090 private int minParameters; 091 private int maxParameters; 092 public FunctionDetails(String description, int minParameters, int maxParameters) { 093 super(); 094 this.description = description; 095 this.minParameters = minParameters; 096 this.maxParameters = maxParameters; 097 } 098 public String getDescription() { 099 return description; 100 } 101 public int getMinParameters() { 102 return minParameters; 103 } 104 public int getMaxParameters() { 105 return maxParameters; 106 } 107 108 } 109}