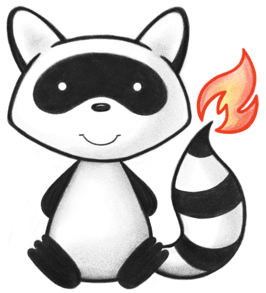
001package org.hl7.fhir.dstu3.formats; 002 003 004 005 006 007import java.io.IOException; 008 009/* 010 Copyright (c) 2011+, HL7, Inc. 011 All rights reserved. 012 013 Redistribution and use in source and binary forms, with or without modification, 014 are permitted provided that the following conditions are met: 015 016 * Redistributions of source code must retain the above copyright notice, this 017 list of conditions and the following disclaimer. 018 * Redistributions in binary form must reproduce the above copyright notice, 019 this list of conditions and the following disclaimer in the documentation 020 and/or other materials provided with the distribution. 021 * Neither the name of HL7 nor the names of its contributors may be used to 022 endorse or promote products derived from this software without specific 023 prior written permission. 024 025 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 026 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 027 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 028 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 029 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 030 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 031 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 032 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 033 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 034 POSSIBILITY OF SUCH DAMAGE. 035 036*/ 037 038 039import java.io.InputStream; 040import java.io.OutputStream; 041import java.io.UnsupportedEncodingException; 042 043import org.hl7.fhir.dstu3.model.Resource; 044import org.hl7.fhir.dstu3.model.Type; 045import org.hl7.fhir.exceptions.FHIRFormatError; 046import org.xmlpull.v1.XmlPullParserException; 047 048 049/** 050 * General interface - either an XML or JSON parser: read or write instances 051 * 052 * Defined to allow a factory to create a parser of the right type 053 */ 054public interface IParser { 055 056 /** 057 * check what kind of parser this is 058 * 059 * @return what kind of parser this is 060 */ 061 public ParserType getType(); 062 063 // -- Parser Configuration ---------------------------------- 064 /** 065 * Whether to parse or ignore comments - either reading or writing 066 */ 067 public boolean getHandleComments(); 068 public IParser setHandleComments(boolean value); 069 070 /** 071 * @param allowUnknownContent Whether to throw an exception if unknown content is found (or just skip it) when parsing 072 */ 073 public boolean isAllowUnknownContent(); 074 public IParser setAllowUnknownContent(boolean value); 075 076 077 public enum OutputStyle { 078 /** 079 * Produce normal output - no whitespace, except in HTML where whitespace is untouched 080 */ 081 NORMAL, 082 083 /** 084 * Produce pretty output - human readable whitespace, HTML whitespace untouched 085 */ 086 PRETTY, 087 088 /** 089 * Produce canonical output - no comments, no whitspace, HTML whitespace normlised, JSON attributes sorted alphabetically (slightly slower) 090 */ 091 CANONICAL, 092 } 093 094 /** 095 * Writing: 096 */ 097 public OutputStyle getOutputStyle(); 098 public IParser setOutputStyle(OutputStyle value); 099 100 /** 101 * This method is used by the publication tooling to stop the xhrtml narrative being generated. 102 * It is not valid to use in production use. The tooling uses it to generate json/xml representations in html that are not cluttered by escaped html representations of the html representation 103 */ 104 public IParser setSuppressXhtml(String message); 105 106 // -- Reading methods ---------------------------------------- 107 108 /** 109 * parse content that is known to be a resource 110 * @throws XmlPullParserException 111 * @throws FHIRFormatError 112 * @throws IOException 113 */ 114 public Resource parse(InputStream input) throws IOException, FHIRFormatError; 115 116 /** 117 * parse content that is known to be a resource 118 * @throws UnsupportedEncodingException 119 * @throws IOException 120 * @throws FHIRFormatError 121 */ 122 public Resource parse(String input) throws UnsupportedEncodingException, FHIRFormatError, IOException; 123 124 /** 125 * parse content that is known to be a resource 126 * @throws IOException 127 * @throws FHIRFormatError 128 */ 129 public Resource parse(byte[] bytes) throws FHIRFormatError, IOException; 130 131 /** 132 * This is used to parse a type - a fragment of a resource. 133 * There's no reason to use this in production - it's used 134 * in the build tools 135 * 136 * Not supported by all implementations 137 * 138 * @param input 139 * @param knownType. if this is blank, the parser may try to infer the type (xml only) 140 * @return 141 * @throws XmlPullParserException 142 * @throws FHIRFormatError 143 * @throws IOException 144 */ 145 public Type parseType(InputStream input, String knownType) throws IOException, FHIRFormatError; 146 /** 147 * This is used to parse a type - a fragment of a resource. 148 * There's no reason to use this in production - it's used 149 * in the build tools 150 * 151 * Not supported by all implementations 152 * 153 * @param input 154 * @param knownType. if this is blank, the parser may try to infer the type (xml only) 155 * @return 156 * @throws UnsupportedEncodingException 157 * @throws IOException 158 * @throws FHIRFormatError 159 */ 160 public Type parseType(String input, String knownType) throws UnsupportedEncodingException, FHIRFormatError, IOException; 161 /** 162 * This is used to parse a type - a fragment of a resource. 163 * There's no reason to use this in production - it's used 164 * in the build tools 165 * 166 * Not supported by all implementations 167 * 168 * @param input 169 * @param knownType. if this is blank, the parser may try to infer the type (xml only) 170 * @return 171 * @throws IOException 172 * @throws FHIRFormatError 173 */ 174 public Type parseType(byte[] bytes, String knownType) throws FHIRFormatError, IOException; 175 176 // -- Writing methods ---------------------------------------- 177 178 /** 179 * Compose a resource to a stream, possibly using pretty presentation for a human reader (used in the spec, for example, but not normally in production) 180 * @throws IOException 181 */ 182 public void compose(OutputStream stream, Resource resource) throws IOException; 183 184 /** 185 * Compose a resource to a stream, possibly using pretty presentation for a human reader (used in the spec, for example, but not normally in production) 186 * @throws IOException 187 */ 188 public String composeString(Resource resource) throws IOException; 189 190 /** 191 * Compose a resource to a stream, possibly using pretty presentation for a human reader (used in the spec, for example, but not normally in production) 192 * @throws IOException 193 */ 194 public byte[] composeBytes(Resource resource) throws IOException; 195 196 197 /** 198 * Compose a type to a stream, possibly using pretty presentation for a human reader (used in the spec, for example, but not normally in production) 199 * 200 * Not supported by all implementations. rootName is ignored in the JSON format 201 * @throws XmlPullParserException 202 * @throws FHIRFormatError 203 * @throws IOException 204 */ 205 public void compose(OutputStream stream, Type type, String rootName) throws IOException; 206 207 /** 208 * Compose a type to a stream, possibly using pretty presentation for a human reader (used in the spec, for example, but not normally in production) 209 * 210 * Not supported by all implementations. rootName is ignored in the JSON format 211 * @throws IOException 212 */ 213 public String composeString(Type type, String rootName) throws IOException; 214 215 /** 216 * Compose a type to a stream, possibly using pretty presentation for a human reader (used in the spec, for example, but not normally in production) 217 * 218 * Not supported by all implementations. rootName is ignored in the JSON format 219 * @throws IOException 220 */ 221 public byte[] composeBytes(Type type, String rootName) throws IOException; 222 223 224}