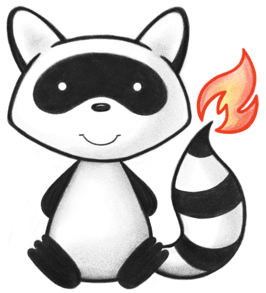
001package org.hl7.fhir.dstu3.formats; 002 003import java.io.IOException; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Mon, Apr 17, 2017 08:38+1000 for FHIR v3.0.x 035import org.hl7.fhir.dstu3.model.*; 036import org.hl7.fhir.exceptions.FHIRFormatError; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.utilities.xhtml.XhtmlNode; 039 040import com.google.gson.JsonArray; 041import com.google.gson.JsonObject; 042 043public class JsonParser extends JsonParserBase { 044 045 public JsonParser() { 046 super(); 047 } 048 049 public JsonParser(boolean allowUnknownContent) { 050 super(); 051 setAllowUnknownContent(allowUnknownContent); 052 } 053 054 055 protected void parseElementProperties(JsonObject json, Element element) throws IOException, FHIRFormatError { 056 super.parseElementProperties(json, element); 057 if (json.has("extension")) { 058 JsonArray array = json.getAsJsonArray("extension"); 059 for (int i = 0; i < array.size(); i++) { 060 element.getExtension().add(parseExtension(array.get(i).getAsJsonObject())); 061 } 062 }; 063 } 064 065 protected void parseBackboneProperties(JsonObject json, BackboneElement element) throws IOException, FHIRFormatError { 066 parseElementProperties(json, element); 067 if (json.has("modifierExtension")) { 068 JsonArray array = json.getAsJsonArray("modifierExtension"); 069 for (int i = 0; i < array.size(); i++) { 070 element.getModifierExtension().add(parseExtension(array.get(i).getAsJsonObject())); 071 } 072 } 073 } 074 075 protected void parseTypeProperties(JsonObject json, Element element) throws IOException, FHIRFormatError { 076 parseElementProperties(json, element); 077 } 078 079 @SuppressWarnings("unchecked") 080 protected <E extends Enum<E>> Enumeration<E> parseEnumeration(String s, E item, EnumFactory e) throws IOException, FHIRFormatError { 081 Enumeration<E> res = new Enumeration<E>(e); 082 if (s != null) 083 res.setValue((E) e.fromCode(s)); 084 return res; 085 } 086 087 protected DateType parseDate(String v) throws IOException, FHIRFormatError { 088 DateType res = new DateType(v); 089 return res; 090 } 091 092 protected DateTimeType parseDateTime(String v) throws IOException, FHIRFormatError { 093 DateTimeType res = new DateTimeType(v); 094 return res; 095 } 096 097 protected CodeType parseCode(String v) throws IOException, FHIRFormatError { 098 CodeType res = new CodeType(v); 099 return res; 100 } 101 102 protected StringType parseString(String v) throws IOException, FHIRFormatError { 103 StringType res = new StringType(v); 104 return res; 105 } 106 107 protected IntegerType parseInteger(java.lang.Long v) throws IOException, FHIRFormatError { 108 IntegerType res = new IntegerType(v); 109 return res; 110 } 111 112 protected OidType parseOid(String v) throws IOException, FHIRFormatError { 113 OidType res = new OidType(v); 114 return res; 115 } 116 117 protected UriType parseUri(String v) throws IOException, FHIRFormatError { 118 UriType res = new UriType(v); 119 return res; 120 } 121 122 protected UuidType parseUuid(String v) throws IOException, FHIRFormatError { 123 UuidType res = new UuidType(v); 124 return res; 125 } 126 127 protected InstantType parseInstant(String v) throws IOException, FHIRFormatError { 128 InstantType res = new InstantType(v); 129 return res; 130 } 131 132 protected BooleanType parseBoolean(java.lang.Boolean v) throws IOException, FHIRFormatError { 133 BooleanType res = new BooleanType(v); 134 return res; 135 } 136 137 protected Base64BinaryType parseBase64Binary(String v) throws IOException, FHIRFormatError { 138 Base64BinaryType res = new Base64BinaryType(v); 139 return res; 140 } 141 142 protected UnsignedIntType parseUnsignedInt(String v) throws IOException, FHIRFormatError { 143 UnsignedIntType res = new UnsignedIntType(v); 144 return res; 145 } 146 147 protected MarkdownType parseMarkdown(String v) throws IOException, FHIRFormatError { 148 MarkdownType res = new MarkdownType(v); 149 return res; 150 } 151 152 protected TimeType parseTime(String v) throws IOException, FHIRFormatError { 153 TimeType res = new TimeType(v); 154 return res; 155 } 156 157 protected IdType parseId(String v) throws IOException, FHIRFormatError { 158 IdType res = new IdType(v); 159 return res; 160 } 161 162 protected PositiveIntType parsePositiveInt(String v) throws IOException, FHIRFormatError { 163 PositiveIntType res = new PositiveIntType(v); 164 return res; 165 } 166 167 protected DecimalType parseDecimal(java.math.BigDecimal v) throws IOException, FHIRFormatError { 168 DecimalType res = new DecimalType(v); 169 return res; 170 } 171 172 protected Extension parseExtension(JsonObject json) throws IOException, FHIRFormatError { 173 Extension res = new Extension(); 174 parseExtensionProperties(json, res); 175 return res; 176 } 177 178 protected void parseExtensionProperties(JsonObject json, Extension res) throws IOException, FHIRFormatError { 179 parseTypeProperties(json, res); 180 if (json.has("url")) 181 res.setUrlElement(parseUri(json.get("url").getAsString())); 182 if (json.has("_url")) 183 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 184 Type value = parseType("value", json); 185 if (value != null) 186 res.setValue(value); 187 } 188 189 protected Narrative parseNarrative(JsonObject json) throws IOException, FHIRFormatError { 190 Narrative res = new Narrative(); 191 parseNarrativeProperties(json, res); 192 return res; 193 } 194 195 protected void parseNarrativeProperties(JsonObject json, Narrative res) throws IOException, FHIRFormatError { 196 parseTypeProperties(json, res); 197 if (json.has("status")) 198 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Narrative.NarrativeStatus.NULL, new Narrative.NarrativeStatusEnumFactory())); 199 if (json.has("_status")) 200 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 201 if (json.has("div")) 202 res.setDiv(parseXhtml(json.get("div").getAsString())); 203 } 204 205 protected Reference parseReference(JsonObject json) throws IOException, FHIRFormatError { 206 Reference res = new Reference(); 207 parseReferenceProperties(json, res); 208 return res; 209 } 210 211 protected void parseReferenceProperties(JsonObject json, Reference res) throws IOException, FHIRFormatError { 212 parseTypeProperties(json, res); 213 if (json.has("reference")) 214 res.setReferenceElement(parseString(json.get("reference").getAsString())); 215 if (json.has("_reference")) 216 parseElementProperties(getJObject(json, "_reference"), res.getReferenceElement()); 217 if (json.has("identifier")) 218 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 219 if (json.has("display")) 220 res.setDisplayElement(parseString(json.get("display").getAsString())); 221 if (json.has("_display")) 222 parseElementProperties(getJObject(json, "_display"), res.getDisplayElement()); 223 } 224 225 protected Quantity parseQuantity(JsonObject json) throws IOException, FHIRFormatError { 226 Quantity res = new Quantity(); 227 parseQuantityProperties(json, res); 228 return res; 229 } 230 231 protected void parseQuantityProperties(JsonObject json, Quantity res) throws IOException, FHIRFormatError { 232 parseTypeProperties(json, res); 233 if (json.has("value")) 234 res.setValueElement(parseDecimal(json.get("value").getAsBigDecimal())); 235 if (json.has("_value")) 236 parseElementProperties(getJObject(json, "_value"), res.getValueElement()); 237 if (json.has("comparator")) 238 res.setComparatorElement(parseEnumeration(json.get("comparator").getAsString(), Quantity.QuantityComparator.NULL, new Quantity.QuantityComparatorEnumFactory())); 239 if (json.has("_comparator")) 240 parseElementProperties(getJObject(json, "_comparator"), res.getComparatorElement()); 241 if (json.has("unit")) 242 res.setUnitElement(parseString(json.get("unit").getAsString())); 243 if (json.has("_unit")) 244 parseElementProperties(getJObject(json, "_unit"), res.getUnitElement()); 245 if (json.has("system")) 246 res.setSystemElement(parseUri(json.get("system").getAsString())); 247 if (json.has("_system")) 248 parseElementProperties(getJObject(json, "_system"), res.getSystemElement()); 249 if (json.has("code")) 250 res.setCodeElement(parseCode(json.get("code").getAsString())); 251 if (json.has("_code")) 252 parseElementProperties(getJObject(json, "_code"), res.getCodeElement()); 253 } 254 255 protected Period parsePeriod(JsonObject json) throws IOException, FHIRFormatError { 256 Period res = new Period(); 257 parsePeriodProperties(json, res); 258 return res; 259 } 260 261 protected void parsePeriodProperties(JsonObject json, Period res) throws IOException, FHIRFormatError { 262 parseTypeProperties(json, res); 263 if (json.has("start")) 264 res.setStartElement(parseDateTime(json.get("start").getAsString())); 265 if (json.has("_start")) 266 parseElementProperties(getJObject(json, "_start"), res.getStartElement()); 267 if (json.has("end")) 268 res.setEndElement(parseDateTime(json.get("end").getAsString())); 269 if (json.has("_end")) 270 parseElementProperties(getJObject(json, "_end"), res.getEndElement()); 271 } 272 273 protected Attachment parseAttachment(JsonObject json) throws IOException, FHIRFormatError { 274 Attachment res = new Attachment(); 275 parseAttachmentProperties(json, res); 276 return res; 277 } 278 279 protected void parseAttachmentProperties(JsonObject json, Attachment res) throws IOException, FHIRFormatError { 280 parseTypeProperties(json, res); 281 if (json.has("contentType")) 282 res.setContentTypeElement(parseCode(json.get("contentType").getAsString())); 283 if (json.has("_contentType")) 284 parseElementProperties(getJObject(json, "_contentType"), res.getContentTypeElement()); 285 if (json.has("language")) 286 res.setLanguageElement(parseCode(json.get("language").getAsString())); 287 if (json.has("_language")) 288 parseElementProperties(getJObject(json, "_language"), res.getLanguageElement()); 289 if (json.has("data")) 290 res.setDataElement(parseBase64Binary(json.get("data").getAsString())); 291 if (json.has("_data")) 292 parseElementProperties(getJObject(json, "_data"), res.getDataElement()); 293 if (json.has("url")) 294 res.setUrlElement(parseUri(json.get("url").getAsString())); 295 if (json.has("_url")) 296 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 297 if (json.has("size")) 298 res.setSizeElement(parseUnsignedInt(json.get("size").getAsString())); 299 if (json.has("_size")) 300 parseElementProperties(getJObject(json, "_size"), res.getSizeElement()); 301 if (json.has("hash")) 302 res.setHashElement(parseBase64Binary(json.get("hash").getAsString())); 303 if (json.has("_hash")) 304 parseElementProperties(getJObject(json, "_hash"), res.getHashElement()); 305 if (json.has("title")) 306 res.setTitleElement(parseString(json.get("title").getAsString())); 307 if (json.has("_title")) 308 parseElementProperties(getJObject(json, "_title"), res.getTitleElement()); 309 if (json.has("creation")) 310 res.setCreationElement(parseDateTime(json.get("creation").getAsString())); 311 if (json.has("_creation")) 312 parseElementProperties(getJObject(json, "_creation"), res.getCreationElement()); 313 } 314 315 protected Duration parseDuration(JsonObject json) throws IOException, FHIRFormatError { 316 Duration res = new Duration(); 317 parseDurationProperties(json, res); 318 return res; 319 } 320 321 protected void parseDurationProperties(JsonObject json, Duration res) throws IOException, FHIRFormatError { 322 parseQuantityProperties(json, res); 323 } 324 325 protected Count parseCount(JsonObject json) throws IOException, FHIRFormatError { 326 Count res = new Count(); 327 parseCountProperties(json, res); 328 return res; 329 } 330 331 protected void parseCountProperties(JsonObject json, Count res) throws IOException, FHIRFormatError { 332 parseQuantityProperties(json, res); 333 } 334 335 protected Range parseRange(JsonObject json) throws IOException, FHIRFormatError { 336 Range res = new Range(); 337 parseRangeProperties(json, res); 338 return res; 339 } 340 341 protected void parseRangeProperties(JsonObject json, Range res) throws IOException, FHIRFormatError { 342 parseTypeProperties(json, res); 343 if (json.has("low")) 344 res.setLow(parseSimpleQuantity(getJObject(json, "low"))); 345 if (json.has("high")) 346 res.setHigh(parseSimpleQuantity(getJObject(json, "high"))); 347 } 348 349 protected Annotation parseAnnotation(JsonObject json) throws IOException, FHIRFormatError { 350 Annotation res = new Annotation(); 351 parseAnnotationProperties(json, res); 352 return res; 353 } 354 355 protected void parseAnnotationProperties(JsonObject json, Annotation res) throws IOException, FHIRFormatError { 356 parseTypeProperties(json, res); 357 Type author = parseType("author", json); 358 if (author != null) 359 res.setAuthor(author); 360 if (json.has("time")) 361 res.setTimeElement(parseDateTime(json.get("time").getAsString())); 362 if (json.has("_time")) 363 parseElementProperties(getJObject(json, "_time"), res.getTimeElement()); 364 if (json.has("text")) 365 res.setTextElement(parseString(json.get("text").getAsString())); 366 if (json.has("_text")) 367 parseElementProperties(getJObject(json, "_text"), res.getTextElement()); 368 } 369 370 protected Money parseMoney(JsonObject json) throws IOException, FHIRFormatError { 371 Money res = new Money(); 372 parseMoneyProperties(json, res); 373 return res; 374 } 375 376 protected void parseMoneyProperties(JsonObject json, Money res) throws IOException, FHIRFormatError { 377 parseQuantityProperties(json, res); 378 } 379 380 protected Identifier parseIdentifier(JsonObject json) throws IOException, FHIRFormatError { 381 Identifier res = new Identifier(); 382 parseIdentifierProperties(json, res); 383 return res; 384 } 385 386 protected void parseIdentifierProperties(JsonObject json, Identifier res) throws IOException, FHIRFormatError { 387 parseTypeProperties(json, res); 388 if (json.has("use")) 389 res.setUseElement(parseEnumeration(json.get("use").getAsString(), Identifier.IdentifierUse.NULL, new Identifier.IdentifierUseEnumFactory())); 390 if (json.has("_use")) 391 parseElementProperties(getJObject(json, "_use"), res.getUseElement()); 392 if (json.has("type")) 393 res.setType(parseCodeableConcept(getJObject(json, "type"))); 394 if (json.has("system")) 395 res.setSystemElement(parseUri(json.get("system").getAsString())); 396 if (json.has("_system")) 397 parseElementProperties(getJObject(json, "_system"), res.getSystemElement()); 398 if (json.has("value")) 399 res.setValueElement(parseString(json.get("value").getAsString())); 400 if (json.has("_value")) 401 parseElementProperties(getJObject(json, "_value"), res.getValueElement()); 402 if (json.has("period")) 403 res.setPeriod(parsePeriod(getJObject(json, "period"))); 404 if (json.has("assigner")) 405 res.setAssigner(parseReference(getJObject(json, "assigner"))); 406 } 407 408 protected Coding parseCoding(JsonObject json) throws IOException, FHIRFormatError { 409 Coding res = new Coding(); 410 parseCodingProperties(json, res); 411 return res; 412 } 413 414 protected void parseCodingProperties(JsonObject json, Coding res) throws IOException, FHIRFormatError { 415 parseTypeProperties(json, res); 416 if (json.has("system")) 417 res.setSystemElement(parseUri(json.get("system").getAsString())); 418 if (json.has("_system")) 419 parseElementProperties(getJObject(json, "_system"), res.getSystemElement()); 420 if (json.has("version")) 421 res.setVersionElement(parseString(json.get("version").getAsString())); 422 if (json.has("_version")) 423 parseElementProperties(getJObject(json, "_version"), res.getVersionElement()); 424 if (json.has("code")) 425 res.setCodeElement(parseCode(json.get("code").getAsString())); 426 if (json.has("_code")) 427 parseElementProperties(getJObject(json, "_code"), res.getCodeElement()); 428 if (json.has("display")) 429 res.setDisplayElement(parseString(json.get("display").getAsString())); 430 if (json.has("_display")) 431 parseElementProperties(getJObject(json, "_display"), res.getDisplayElement()); 432 if (json.has("userSelected")) 433 res.setUserSelectedElement(parseBoolean(json.get("userSelected").getAsBoolean())); 434 if (json.has("_userSelected")) 435 parseElementProperties(getJObject(json, "_userSelected"), res.getUserSelectedElement()); 436 } 437 438 protected Signature parseSignature(JsonObject json) throws IOException, FHIRFormatError { 439 Signature res = new Signature(); 440 parseSignatureProperties(json, res); 441 return res; 442 } 443 444 protected void parseSignatureProperties(JsonObject json, Signature res) throws IOException, FHIRFormatError { 445 parseTypeProperties(json, res); 446 if (json.has("type")) { 447 JsonArray array = json.getAsJsonArray("type"); 448 for (int i = 0; i < array.size(); i++) { 449 res.getType().add(parseCoding(array.get(i).getAsJsonObject())); 450 } 451 }; 452 if (json.has("when")) 453 res.setWhenElement(parseInstant(json.get("when").getAsString())); 454 if (json.has("_when")) 455 parseElementProperties(getJObject(json, "_when"), res.getWhenElement()); 456 Type who = parseType("who", json); 457 if (who != null) 458 res.setWho(who); 459 Type onBehalfOf = parseType("onBehalfOf", json); 460 if (onBehalfOf != null) 461 res.setOnBehalfOf(onBehalfOf); 462 if (json.has("contentType")) 463 res.setContentTypeElement(parseCode(json.get("contentType").getAsString())); 464 if (json.has("_contentType")) 465 parseElementProperties(getJObject(json, "_contentType"), res.getContentTypeElement()); 466 if (json.has("blob")) 467 res.setBlobElement(parseBase64Binary(json.get("blob").getAsString())); 468 if (json.has("_blob")) 469 parseElementProperties(getJObject(json, "_blob"), res.getBlobElement()); 470 } 471 472 protected SampledData parseSampledData(JsonObject json) throws IOException, FHIRFormatError { 473 SampledData res = new SampledData(); 474 parseSampledDataProperties(json, res); 475 return res; 476 } 477 478 protected void parseSampledDataProperties(JsonObject json, SampledData res) throws IOException, FHIRFormatError { 479 parseTypeProperties(json, res); 480 if (json.has("origin")) 481 res.setOrigin(parseSimpleQuantity(getJObject(json, "origin"))); 482 if (json.has("period")) 483 res.setPeriodElement(parseDecimal(json.get("period").getAsBigDecimal())); 484 if (json.has("_period")) 485 parseElementProperties(getJObject(json, "_period"), res.getPeriodElement()); 486 if (json.has("factor")) 487 res.setFactorElement(parseDecimal(json.get("factor").getAsBigDecimal())); 488 if (json.has("_factor")) 489 parseElementProperties(getJObject(json, "_factor"), res.getFactorElement()); 490 if (json.has("lowerLimit")) 491 res.setLowerLimitElement(parseDecimal(json.get("lowerLimit").getAsBigDecimal())); 492 if (json.has("_lowerLimit")) 493 parseElementProperties(getJObject(json, "_lowerLimit"), res.getLowerLimitElement()); 494 if (json.has("upperLimit")) 495 res.setUpperLimitElement(parseDecimal(json.get("upperLimit").getAsBigDecimal())); 496 if (json.has("_upperLimit")) 497 parseElementProperties(getJObject(json, "_upperLimit"), res.getUpperLimitElement()); 498 if (json.has("dimensions")) 499 res.setDimensionsElement(parsePositiveInt(json.get("dimensions").getAsString())); 500 if (json.has("_dimensions")) 501 parseElementProperties(getJObject(json, "_dimensions"), res.getDimensionsElement()); 502 if (json.has("data")) 503 res.setDataElement(parseString(json.get("data").getAsString())); 504 if (json.has("_data")) 505 parseElementProperties(getJObject(json, "_data"), res.getDataElement()); 506 } 507 508 protected Ratio parseRatio(JsonObject json) throws IOException, FHIRFormatError { 509 Ratio res = new Ratio(); 510 parseRatioProperties(json, res); 511 return res; 512 } 513 514 protected void parseRatioProperties(JsonObject json, Ratio res) throws IOException, FHIRFormatError { 515 parseTypeProperties(json, res); 516 if (json.has("numerator")) 517 res.setNumerator(parseQuantity(getJObject(json, "numerator"))); 518 if (json.has("denominator")) 519 res.setDenominator(parseQuantity(getJObject(json, "denominator"))); 520 } 521 522 protected Distance parseDistance(JsonObject json) throws IOException, FHIRFormatError { 523 Distance res = new Distance(); 524 parseDistanceProperties(json, res); 525 return res; 526 } 527 528 protected void parseDistanceProperties(JsonObject json, Distance res) throws IOException, FHIRFormatError { 529 parseQuantityProperties(json, res); 530 } 531 532 protected Age parseAge(JsonObject json) throws IOException, FHIRFormatError { 533 Age res = new Age(); 534 parseAgeProperties(json, res); 535 return res; 536 } 537 538 protected void parseAgeProperties(JsonObject json, Age res) throws IOException, FHIRFormatError { 539 parseQuantityProperties(json, res); 540 } 541 542 protected CodeableConcept parseCodeableConcept(JsonObject json) throws IOException, FHIRFormatError { 543 CodeableConcept res = new CodeableConcept(); 544 parseCodeableConceptProperties(json, res); 545 return res; 546 } 547 548 protected void parseCodeableConceptProperties(JsonObject json, CodeableConcept res) throws IOException, FHIRFormatError { 549 parseTypeProperties(json, res); 550 if (json.has("coding")) { 551 JsonArray array = json.getAsJsonArray("coding"); 552 for (int i = 0; i < array.size(); i++) { 553 res.getCoding().add(parseCoding(array.get(i).getAsJsonObject())); 554 } 555 }; 556 if (json.has("text")) 557 res.setTextElement(parseString(json.get("text").getAsString())); 558 if (json.has("_text")) 559 parseElementProperties(getJObject(json, "_text"), res.getTextElement()); 560 } 561 562 protected SimpleQuantity parseSimpleQuantity(JsonObject json) throws IOException, FHIRFormatError { 563 SimpleQuantity res = new SimpleQuantity(); 564 parseSimpleQuantityProperties(json, res); 565 return res; 566 } 567 568 protected void parseSimpleQuantityProperties(JsonObject json, SimpleQuantity res) throws IOException, FHIRFormatError { 569 parseTypeProperties(json, res); 570 if (json.has("value")) 571 res.setValueElement(parseDecimal(json.get("value").getAsBigDecimal())); 572 if (json.has("_value")) 573 parseElementProperties(getJObject(json, "_value"), res.getValueElement()); 574 if (json.has("comparator")) 575 res.setComparatorElement(parseEnumeration(json.get("comparator").getAsString(), Quantity.QuantityComparator.NULL, new Quantity.QuantityComparatorEnumFactory())); 576 if (json.has("_comparator")) 577 parseElementProperties(getJObject(json, "_comparator"), res.getComparatorElement()); 578 if (json.has("unit")) 579 res.setUnitElement(parseString(json.get("unit").getAsString())); 580 if (json.has("_unit")) 581 parseElementProperties(getJObject(json, "_unit"), res.getUnitElement()); 582 if (json.has("system")) 583 res.setSystemElement(parseUri(json.get("system").getAsString())); 584 if (json.has("_system")) 585 parseElementProperties(getJObject(json, "_system"), res.getSystemElement()); 586 if (json.has("code")) 587 res.setCodeElement(parseCode(json.get("code").getAsString())); 588 if (json.has("_code")) 589 parseElementProperties(getJObject(json, "_code"), res.getCodeElement()); 590 } 591 592 protected Meta parseMeta(JsonObject json) throws IOException, FHIRFormatError { 593 Meta res = new Meta(); 594 parseMetaProperties(json, res); 595 return res; 596 } 597 598 protected void parseMetaProperties(JsonObject json, Meta res) throws IOException, FHIRFormatError { 599 parseElementProperties(json, res); 600 if (json.has("versionId")) 601 res.setVersionIdElement(parseId(json.get("versionId").getAsString())); 602 if (json.has("_versionId")) 603 parseElementProperties(getJObject(json, "_versionId"), res.getVersionIdElement()); 604 if (json.has("lastUpdated")) 605 res.setLastUpdatedElement(parseInstant(json.get("lastUpdated").getAsString())); 606 if (json.has("_lastUpdated")) 607 parseElementProperties(getJObject(json, "_lastUpdated"), res.getLastUpdatedElement()); 608 if (json.has("profile")) { 609 JsonArray array = json.getAsJsonArray("profile"); 610 for (int i = 0; i < array.size(); i++) { 611 if (array.get(i).isJsonNull()) { 612 res.getProfile().add(new UriType()); 613 } else { 614 res.getProfile().add(parseUri(array.get(i).getAsString())); 615 } 616 } 617 }; 618 if (json.has("_profile")) { 619 JsonArray array = json.getAsJsonArray("_profile"); 620 for (int i = 0; i < array.size(); i++) { 621 if (i == res.getProfile().size()) 622 res.getProfile().add(parseUri(null)); 623 if (array.get(i) instanceof JsonObject) 624 parseElementProperties(array.get(i).getAsJsonObject(), res.getProfile().get(i)); 625 } 626 }; 627 if (json.has("security")) { 628 JsonArray array = json.getAsJsonArray("security"); 629 for (int i = 0; i < array.size(); i++) { 630 res.getSecurity().add(parseCoding(array.get(i).getAsJsonObject())); 631 } 632 }; 633 if (json.has("tag")) { 634 JsonArray array = json.getAsJsonArray("tag"); 635 for (int i = 0; i < array.size(); i++) { 636 res.getTag().add(parseCoding(array.get(i).getAsJsonObject())); 637 } 638 }; 639 } 640 641 protected Address parseAddress(JsonObject json) throws IOException, FHIRFormatError { 642 Address res = new Address(); 643 parseAddressProperties(json, res); 644 return res; 645 } 646 647 protected void parseAddressProperties(JsonObject json, Address res) throws IOException, FHIRFormatError { 648 parseTypeProperties(json, res); 649 if (json.has("use")) 650 res.setUseElement(parseEnumeration(json.get("use").getAsString(), Address.AddressUse.NULL, new Address.AddressUseEnumFactory())); 651 if (json.has("_use")) 652 parseElementProperties(getJObject(json, "_use"), res.getUseElement()); 653 if (json.has("type")) 654 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), Address.AddressType.NULL, new Address.AddressTypeEnumFactory())); 655 if (json.has("_type")) 656 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 657 if (json.has("text")) 658 res.setTextElement(parseString(json.get("text").getAsString())); 659 if (json.has("_text")) 660 parseElementProperties(getJObject(json, "_text"), res.getTextElement()); 661 if (json.has("line")) { 662 JsonArray array = json.getAsJsonArray("line"); 663 for (int i = 0; i < array.size(); i++) { 664 if (array.get(i).isJsonNull()) { 665 res.getLine().add(new StringType()); 666 } else { 667 res.getLine().add(parseString(array.get(i).getAsString())); 668 } 669 } 670 }; 671 if (json.has("_line")) { 672 JsonArray array = json.getAsJsonArray("_line"); 673 for (int i = 0; i < array.size(); i++) { 674 if (i == res.getLine().size()) 675 res.getLine().add(parseString(null)); 676 if (array.get(i) instanceof JsonObject) 677 parseElementProperties(array.get(i).getAsJsonObject(), res.getLine().get(i)); 678 } 679 }; 680 if (json.has("city")) 681 res.setCityElement(parseString(json.get("city").getAsString())); 682 if (json.has("_city")) 683 parseElementProperties(getJObject(json, "_city"), res.getCityElement()); 684 if (json.has("district")) 685 res.setDistrictElement(parseString(json.get("district").getAsString())); 686 if (json.has("_district")) 687 parseElementProperties(getJObject(json, "_district"), res.getDistrictElement()); 688 if (json.has("state")) 689 res.setStateElement(parseString(json.get("state").getAsString())); 690 if (json.has("_state")) 691 parseElementProperties(getJObject(json, "_state"), res.getStateElement()); 692 if (json.has("postalCode")) 693 res.setPostalCodeElement(parseString(json.get("postalCode").getAsString())); 694 if (json.has("_postalCode")) 695 parseElementProperties(getJObject(json, "_postalCode"), res.getPostalCodeElement()); 696 if (json.has("country")) 697 res.setCountryElement(parseString(json.get("country").getAsString())); 698 if (json.has("_country")) 699 parseElementProperties(getJObject(json, "_country"), res.getCountryElement()); 700 if (json.has("period")) 701 res.setPeriod(parsePeriod(getJObject(json, "period"))); 702 } 703 704 protected TriggerDefinition parseTriggerDefinition(JsonObject json) throws IOException, FHIRFormatError { 705 TriggerDefinition res = new TriggerDefinition(); 706 parseTriggerDefinitionProperties(json, res); 707 return res; 708 } 709 710 protected void parseTriggerDefinitionProperties(JsonObject json, TriggerDefinition res) throws IOException, FHIRFormatError { 711 parseTypeProperties(json, res); 712 if (json.has("type")) 713 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), TriggerDefinition.TriggerType.NULL, new TriggerDefinition.TriggerTypeEnumFactory())); 714 if (json.has("_type")) 715 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 716 if (json.has("eventName")) 717 res.setEventNameElement(parseString(json.get("eventName").getAsString())); 718 if (json.has("_eventName")) 719 parseElementProperties(getJObject(json, "_eventName"), res.getEventNameElement()); 720 Type eventTiming = parseType("eventTiming", json); 721 if (eventTiming != null) 722 res.setEventTiming(eventTiming); 723 if (json.has("eventData")) 724 res.setEventData(parseDataRequirement(getJObject(json, "eventData"))); 725 } 726 727 protected Contributor parseContributor(JsonObject json) throws IOException, FHIRFormatError { 728 Contributor res = new Contributor(); 729 parseContributorProperties(json, res); 730 return res; 731 } 732 733 protected void parseContributorProperties(JsonObject json, Contributor res) throws IOException, FHIRFormatError { 734 parseTypeProperties(json, res); 735 if (json.has("type")) 736 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), Contributor.ContributorType.NULL, new Contributor.ContributorTypeEnumFactory())); 737 if (json.has("_type")) 738 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 739 if (json.has("name")) 740 res.setNameElement(parseString(json.get("name").getAsString())); 741 if (json.has("_name")) 742 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 743 if (json.has("contact")) { 744 JsonArray array = json.getAsJsonArray("contact"); 745 for (int i = 0; i < array.size(); i++) { 746 res.getContact().add(parseContactDetail(array.get(i).getAsJsonObject())); 747 } 748 }; 749 } 750 751 protected DataRequirement parseDataRequirement(JsonObject json) throws IOException, FHIRFormatError { 752 DataRequirement res = new DataRequirement(); 753 parseDataRequirementProperties(json, res); 754 return res; 755 } 756 757 protected void parseDataRequirementProperties(JsonObject json, DataRequirement res) throws IOException, FHIRFormatError { 758 parseTypeProperties(json, res); 759 if (json.has("type")) 760 res.setTypeElement(parseCode(json.get("type").getAsString())); 761 if (json.has("_type")) 762 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 763 if (json.has("profile")) { 764 JsonArray array = json.getAsJsonArray("profile"); 765 for (int i = 0; i < array.size(); i++) { 766 if (array.get(i).isJsonNull()) { 767 res.getProfile().add(new UriType()); 768 } else { 769 res.getProfile().add(parseUri(array.get(i).getAsString())); 770 } 771 } 772 }; 773 if (json.has("_profile")) { 774 JsonArray array = json.getAsJsonArray("_profile"); 775 for (int i = 0; i < array.size(); i++) { 776 if (i == res.getProfile().size()) 777 res.getProfile().add(parseUri(null)); 778 if (array.get(i) instanceof JsonObject) 779 parseElementProperties(array.get(i).getAsJsonObject(), res.getProfile().get(i)); 780 } 781 }; 782 if (json.has("mustSupport")) { 783 JsonArray array = json.getAsJsonArray("mustSupport"); 784 for (int i = 0; i < array.size(); i++) { 785 if (array.get(i).isJsonNull()) { 786 res.getMustSupport().add(new StringType()); 787 } else { 788 res.getMustSupport().add(parseString(array.get(i).getAsString())); 789 } 790 } 791 }; 792 if (json.has("_mustSupport")) { 793 JsonArray array = json.getAsJsonArray("_mustSupport"); 794 for (int i = 0; i < array.size(); i++) { 795 if (i == res.getMustSupport().size()) 796 res.getMustSupport().add(parseString(null)); 797 if (array.get(i) instanceof JsonObject) 798 parseElementProperties(array.get(i).getAsJsonObject(), res.getMustSupport().get(i)); 799 } 800 }; 801 if (json.has("codeFilter")) { 802 JsonArray array = json.getAsJsonArray("codeFilter"); 803 for (int i = 0; i < array.size(); i++) { 804 res.getCodeFilter().add(parseDataRequirementDataRequirementCodeFilterComponent(array.get(i).getAsJsonObject(), res)); 805 } 806 }; 807 if (json.has("dateFilter")) { 808 JsonArray array = json.getAsJsonArray("dateFilter"); 809 for (int i = 0; i < array.size(); i++) { 810 res.getDateFilter().add(parseDataRequirementDataRequirementDateFilterComponent(array.get(i).getAsJsonObject(), res)); 811 } 812 }; 813 } 814 815 protected DataRequirement.DataRequirementCodeFilterComponent parseDataRequirementDataRequirementCodeFilterComponent(JsonObject json, DataRequirement owner) throws IOException, FHIRFormatError { 816 DataRequirement.DataRequirementCodeFilterComponent res = new DataRequirement.DataRequirementCodeFilterComponent(); 817 parseDataRequirementDataRequirementCodeFilterComponentProperties(json, owner, res); 818 return res; 819 } 820 821 protected void parseDataRequirementDataRequirementCodeFilterComponentProperties(JsonObject json, DataRequirement owner, DataRequirement.DataRequirementCodeFilterComponent res) throws IOException, FHIRFormatError { 822 parseTypeProperties(json, res); 823 if (json.has("path")) 824 res.setPathElement(parseString(json.get("path").getAsString())); 825 if (json.has("_path")) 826 parseElementProperties(getJObject(json, "_path"), res.getPathElement()); 827 Type valueSet = parseType("valueSet", json); 828 if (valueSet != null) 829 res.setValueSet(valueSet); 830 if (json.has("valueCode")) { 831 JsonArray array = json.getAsJsonArray("valueCode"); 832 for (int i = 0; i < array.size(); i++) { 833 if (array.get(i).isJsonNull()) { 834 res.getValueCode().add(new CodeType()); 835 } else { 836 res.getValueCode().add(parseCode(array.get(i).getAsString())); 837 } 838 } 839 }; 840 if (json.has("_valueCode")) { 841 JsonArray array = json.getAsJsonArray("_valueCode"); 842 for (int i = 0; i < array.size(); i++) { 843 if (i == res.getValueCode().size()) 844 res.getValueCode().add(parseCode(null)); 845 if (array.get(i) instanceof JsonObject) 846 parseElementProperties(array.get(i).getAsJsonObject(), res.getValueCode().get(i)); 847 } 848 }; 849 if (json.has("valueCoding")) { 850 JsonArray array = json.getAsJsonArray("valueCoding"); 851 for (int i = 0; i < array.size(); i++) { 852 res.getValueCoding().add(parseCoding(array.get(i).getAsJsonObject())); 853 } 854 }; 855 if (json.has("valueCodeableConcept")) { 856 JsonArray array = json.getAsJsonArray("valueCodeableConcept"); 857 for (int i = 0; i < array.size(); i++) { 858 res.getValueCodeableConcept().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 859 } 860 }; 861 } 862 863 protected DataRequirement.DataRequirementDateFilterComponent parseDataRequirementDataRequirementDateFilterComponent(JsonObject json, DataRequirement owner) throws IOException, FHIRFormatError { 864 DataRequirement.DataRequirementDateFilterComponent res = new DataRequirement.DataRequirementDateFilterComponent(); 865 parseDataRequirementDataRequirementDateFilterComponentProperties(json, owner, res); 866 return res; 867 } 868 869 protected void parseDataRequirementDataRequirementDateFilterComponentProperties(JsonObject json, DataRequirement owner, DataRequirement.DataRequirementDateFilterComponent res) throws IOException, FHIRFormatError { 870 parseTypeProperties(json, res); 871 if (json.has("path")) 872 res.setPathElement(parseString(json.get("path").getAsString())); 873 if (json.has("_path")) 874 parseElementProperties(getJObject(json, "_path"), res.getPathElement()); 875 Type value = parseType("value", json); 876 if (value != null) 877 res.setValue(value); 878 } 879 880 protected Dosage parseDosage(JsonObject json) throws IOException, FHIRFormatError { 881 Dosage res = new Dosage(); 882 parseDosageProperties(json, res); 883 return res; 884 } 885 886 protected void parseDosageProperties(JsonObject json, Dosage res) throws IOException, FHIRFormatError { 887 parseTypeProperties(json, res); 888 if (json.has("sequence")) 889 res.setSequenceElement(parseInteger(json.get("sequence").getAsLong())); 890 if (json.has("_sequence")) 891 parseElementProperties(getJObject(json, "_sequence"), res.getSequenceElement()); 892 if (json.has("text")) 893 res.setTextElement(parseString(json.get("text").getAsString())); 894 if (json.has("_text")) 895 parseElementProperties(getJObject(json, "_text"), res.getTextElement()); 896 if (json.has("additionalInstruction")) { 897 JsonArray array = json.getAsJsonArray("additionalInstruction"); 898 for (int i = 0; i < array.size(); i++) { 899 res.getAdditionalInstruction().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 900 } 901 }; 902 if (json.has("patientInstruction")) 903 res.setPatientInstructionElement(parseString(json.get("patientInstruction").getAsString())); 904 if (json.has("_patientInstruction")) 905 parseElementProperties(getJObject(json, "_patientInstruction"), res.getPatientInstructionElement()); 906 if (json.has("timing")) 907 res.setTiming(parseTiming(getJObject(json, "timing"))); 908 Type asNeeded = parseType("asNeeded", json); 909 if (asNeeded != null) 910 res.setAsNeeded(asNeeded); 911 if (json.has("site")) 912 res.setSite(parseCodeableConcept(getJObject(json, "site"))); 913 if (json.has("route")) 914 res.setRoute(parseCodeableConcept(getJObject(json, "route"))); 915 if (json.has("method")) 916 res.setMethod(parseCodeableConcept(getJObject(json, "method"))); 917 Type dose = parseType("dose", json); 918 if (dose != null) 919 res.setDose(dose); 920 if (json.has("maxDosePerPeriod")) 921 res.setMaxDosePerPeriod(parseRatio(getJObject(json, "maxDosePerPeriod"))); 922 if (json.has("maxDosePerAdministration")) 923 res.setMaxDosePerAdministration(parseSimpleQuantity(getJObject(json, "maxDosePerAdministration"))); 924 if (json.has("maxDosePerLifetime")) 925 res.setMaxDosePerLifetime(parseSimpleQuantity(getJObject(json, "maxDosePerLifetime"))); 926 Type rate = parseType("rate", json); 927 if (rate != null) 928 res.setRate(rate); 929 } 930 931 protected RelatedArtifact parseRelatedArtifact(JsonObject json) throws IOException, FHIRFormatError { 932 RelatedArtifact res = new RelatedArtifact(); 933 parseRelatedArtifactProperties(json, res); 934 return res; 935 } 936 937 protected void parseRelatedArtifactProperties(JsonObject json, RelatedArtifact res) throws IOException, FHIRFormatError { 938 parseTypeProperties(json, res); 939 if (json.has("type")) 940 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), RelatedArtifact.RelatedArtifactType.NULL, new RelatedArtifact.RelatedArtifactTypeEnumFactory())); 941 if (json.has("_type")) 942 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 943 if (json.has("display")) 944 res.setDisplayElement(parseString(json.get("display").getAsString())); 945 if (json.has("_display")) 946 parseElementProperties(getJObject(json, "_display"), res.getDisplayElement()); 947 if (json.has("citation")) 948 res.setCitationElement(parseString(json.get("citation").getAsString())); 949 if (json.has("_citation")) 950 parseElementProperties(getJObject(json, "_citation"), res.getCitationElement()); 951 if (json.has("url")) 952 res.setUrlElement(parseUri(json.get("url").getAsString())); 953 if (json.has("_url")) 954 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 955 if (json.has("document")) 956 res.setDocument(parseAttachment(getJObject(json, "document"))); 957 if (json.has("resource")) 958 res.setResource(parseReference(getJObject(json, "resource"))); 959 } 960 961 protected ContactDetail parseContactDetail(JsonObject json) throws IOException, FHIRFormatError { 962 ContactDetail res = new ContactDetail(); 963 parseContactDetailProperties(json, res); 964 return res; 965 } 966 967 protected void parseContactDetailProperties(JsonObject json, ContactDetail res) throws IOException, FHIRFormatError { 968 parseTypeProperties(json, res); 969 if (json.has("name")) 970 res.setNameElement(parseString(json.get("name").getAsString())); 971 if (json.has("_name")) 972 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 973 if (json.has("telecom")) { 974 JsonArray array = json.getAsJsonArray("telecom"); 975 for (int i = 0; i < array.size(); i++) { 976 res.getTelecom().add(parseContactPoint(array.get(i).getAsJsonObject())); 977 } 978 }; 979 } 980 981 protected HumanName parseHumanName(JsonObject json) throws IOException, FHIRFormatError { 982 HumanName res = new HumanName(); 983 parseHumanNameProperties(json, res); 984 return res; 985 } 986 987 protected void parseHumanNameProperties(JsonObject json, HumanName res) throws IOException, FHIRFormatError { 988 parseTypeProperties(json, res); 989 if (json.has("use")) 990 res.setUseElement(parseEnumeration(json.get("use").getAsString(), HumanName.NameUse.NULL, new HumanName.NameUseEnumFactory())); 991 if (json.has("_use")) 992 parseElementProperties(getJObject(json, "_use"), res.getUseElement()); 993 if (json.has("text")) 994 res.setTextElement(parseString(json.get("text").getAsString())); 995 if (json.has("_text")) 996 parseElementProperties(getJObject(json, "_text"), res.getTextElement()); 997 if (json.has("family")) 998 res.setFamilyElement(parseString(json.get("family").getAsString())); 999 if (json.has("_family")) 1000 parseElementProperties(getJObject(json, "_family"), res.getFamilyElement()); 1001 if (json.has("given")) { 1002 JsonArray array = json.getAsJsonArray("given"); 1003 for (int i = 0; i < array.size(); i++) { 1004 if (array.get(i).isJsonNull()) { 1005 res.getGiven().add(new StringType()); 1006 } else { 1007 res.getGiven().add(parseString(array.get(i).getAsString())); 1008 } 1009 } 1010 }; 1011 if (json.has("_given")) { 1012 JsonArray array = json.getAsJsonArray("_given"); 1013 for (int i = 0; i < array.size(); i++) { 1014 if (i == res.getGiven().size()) 1015 res.getGiven().add(parseString(null)); 1016 if (array.get(i) instanceof JsonObject) 1017 parseElementProperties(array.get(i).getAsJsonObject(), res.getGiven().get(i)); 1018 } 1019 }; 1020 if (json.has("prefix")) { 1021 JsonArray array = json.getAsJsonArray("prefix"); 1022 for (int i = 0; i < array.size(); i++) { 1023 if (array.get(i).isJsonNull()) { 1024 res.getPrefix().add(new StringType()); 1025 } else { 1026 res.getPrefix().add(parseString(array.get(i).getAsString())); 1027 } 1028 } 1029 }; 1030 if (json.has("_prefix")) { 1031 JsonArray array = json.getAsJsonArray("_prefix"); 1032 for (int i = 0; i < array.size(); i++) { 1033 if (i == res.getPrefix().size()) 1034 res.getPrefix().add(parseString(null)); 1035 if (array.get(i) instanceof JsonObject) 1036 parseElementProperties(array.get(i).getAsJsonObject(), res.getPrefix().get(i)); 1037 } 1038 }; 1039 if (json.has("suffix")) { 1040 JsonArray array = json.getAsJsonArray("suffix"); 1041 for (int i = 0; i < array.size(); i++) { 1042 if (array.get(i).isJsonNull()) { 1043 res.getSuffix().add(new StringType()); 1044 } else { 1045 res.getSuffix().add(parseString(array.get(i).getAsString())); 1046 } 1047 } 1048 }; 1049 if (json.has("_suffix")) { 1050 JsonArray array = json.getAsJsonArray("_suffix"); 1051 for (int i = 0; i < array.size(); i++) { 1052 if (i == res.getSuffix().size()) 1053 res.getSuffix().add(parseString(null)); 1054 if (array.get(i) instanceof JsonObject) 1055 parseElementProperties(array.get(i).getAsJsonObject(), res.getSuffix().get(i)); 1056 } 1057 }; 1058 if (json.has("period")) 1059 res.setPeriod(parsePeriod(getJObject(json, "period"))); 1060 } 1061 1062 protected ContactPoint parseContactPoint(JsonObject json) throws IOException, FHIRFormatError { 1063 ContactPoint res = new ContactPoint(); 1064 parseContactPointProperties(json, res); 1065 return res; 1066 } 1067 1068 protected void parseContactPointProperties(JsonObject json, ContactPoint res) throws IOException, FHIRFormatError { 1069 parseTypeProperties(json, res); 1070 if (json.has("system")) 1071 res.setSystemElement(parseEnumeration(json.get("system").getAsString(), ContactPoint.ContactPointSystem.NULL, new ContactPoint.ContactPointSystemEnumFactory())); 1072 if (json.has("_system")) 1073 parseElementProperties(getJObject(json, "_system"), res.getSystemElement()); 1074 if (json.has("value")) 1075 res.setValueElement(parseString(json.get("value").getAsString())); 1076 if (json.has("_value")) 1077 parseElementProperties(getJObject(json, "_value"), res.getValueElement()); 1078 if (json.has("use")) 1079 res.setUseElement(parseEnumeration(json.get("use").getAsString(), ContactPoint.ContactPointUse.NULL, new ContactPoint.ContactPointUseEnumFactory())); 1080 if (json.has("_use")) 1081 parseElementProperties(getJObject(json, "_use"), res.getUseElement()); 1082 if (json.has("rank")) 1083 res.setRankElement(parsePositiveInt(json.get("rank").getAsString())); 1084 if (json.has("_rank")) 1085 parseElementProperties(getJObject(json, "_rank"), res.getRankElement()); 1086 if (json.has("period")) 1087 res.setPeriod(parsePeriod(getJObject(json, "period"))); 1088 } 1089 1090 protected UsageContext parseUsageContext(JsonObject json) throws IOException, FHIRFormatError { 1091 UsageContext res = new UsageContext(); 1092 parseUsageContextProperties(json, res); 1093 return res; 1094 } 1095 1096 protected void parseUsageContextProperties(JsonObject json, UsageContext res) throws IOException, FHIRFormatError { 1097 parseTypeProperties(json, res); 1098 if (json.has("code")) 1099 res.setCode(parseCoding(getJObject(json, "code"))); 1100 Type value = parseType("value", json); 1101 if (value != null) 1102 res.setValue(value); 1103 } 1104 1105 protected Timing parseTiming(JsonObject json) throws IOException, FHIRFormatError { 1106 Timing res = new Timing(); 1107 parseTimingProperties(json, res); 1108 return res; 1109 } 1110 1111 protected void parseTimingProperties(JsonObject json, Timing res) throws IOException, FHIRFormatError { 1112 parseTypeProperties(json, res); 1113 if (json.has("event")) { 1114 JsonArray array = json.getAsJsonArray("event"); 1115 for (int i = 0; i < array.size(); i++) { 1116 if (array.get(i).isJsonNull()) { 1117 res.getEvent().add(new DateTimeType()); 1118 } else { 1119 res.getEvent().add(parseDateTime(array.get(i).getAsString())); 1120 } 1121 } 1122 }; 1123 if (json.has("_event")) { 1124 JsonArray array = json.getAsJsonArray("_event"); 1125 for (int i = 0; i < array.size(); i++) { 1126 if (i == res.getEvent().size()) 1127 res.getEvent().add(parseDateTime(null)); 1128 if (array.get(i) instanceof JsonObject) 1129 parseElementProperties(array.get(i).getAsJsonObject(), res.getEvent().get(i)); 1130 } 1131 }; 1132 if (json.has("repeat")) 1133 res.setRepeat(parseTimingTimingRepeatComponent(getJObject(json, "repeat"), res)); 1134 if (json.has("code")) 1135 res.setCode(parseCodeableConcept(getJObject(json, "code"))); 1136 } 1137 1138 protected Timing.TimingRepeatComponent parseTimingTimingRepeatComponent(JsonObject json, Timing owner) throws IOException, FHIRFormatError { 1139 Timing.TimingRepeatComponent res = new Timing.TimingRepeatComponent(); 1140 parseTimingTimingRepeatComponentProperties(json, owner, res); 1141 return res; 1142 } 1143 1144 protected void parseTimingTimingRepeatComponentProperties(JsonObject json, Timing owner, Timing.TimingRepeatComponent res) throws IOException, FHIRFormatError { 1145 parseTypeProperties(json, res); 1146 Type bounds = parseType("bounds", json); 1147 if (bounds != null) 1148 res.setBounds(bounds); 1149 if (json.has("count")) 1150 res.setCountElement(parseInteger(json.get("count").getAsLong())); 1151 if (json.has("_count")) 1152 parseElementProperties(getJObject(json, "_count"), res.getCountElement()); 1153 if (json.has("countMax")) 1154 res.setCountMaxElement(parseInteger(json.get("countMax").getAsLong())); 1155 if (json.has("_countMax")) 1156 parseElementProperties(getJObject(json, "_countMax"), res.getCountMaxElement()); 1157 if (json.has("duration")) 1158 res.setDurationElement(parseDecimal(json.get("duration").getAsBigDecimal())); 1159 if (json.has("_duration")) 1160 parseElementProperties(getJObject(json, "_duration"), res.getDurationElement()); 1161 if (json.has("durationMax")) 1162 res.setDurationMaxElement(parseDecimal(json.get("durationMax").getAsBigDecimal())); 1163 if (json.has("_durationMax")) 1164 parseElementProperties(getJObject(json, "_durationMax"), res.getDurationMaxElement()); 1165 if (json.has("durationUnit")) 1166 res.setDurationUnitElement(parseEnumeration(json.get("durationUnit").getAsString(), Timing.UnitsOfTime.NULL, new Timing.UnitsOfTimeEnumFactory())); 1167 if (json.has("_durationUnit")) 1168 parseElementProperties(getJObject(json, "_durationUnit"), res.getDurationUnitElement()); 1169 if (json.has("frequency")) 1170 res.setFrequencyElement(parseInteger(json.get("frequency").getAsLong())); 1171 if (json.has("_frequency")) 1172 parseElementProperties(getJObject(json, "_frequency"), res.getFrequencyElement()); 1173 if (json.has("frequencyMax")) 1174 res.setFrequencyMaxElement(parseInteger(json.get("frequencyMax").getAsLong())); 1175 if (json.has("_frequencyMax")) 1176 parseElementProperties(getJObject(json, "_frequencyMax"), res.getFrequencyMaxElement()); 1177 if (json.has("period")) 1178 res.setPeriodElement(parseDecimal(json.get("period").getAsBigDecimal())); 1179 if (json.has("_period")) 1180 parseElementProperties(getJObject(json, "_period"), res.getPeriodElement()); 1181 if (json.has("periodMax")) 1182 res.setPeriodMaxElement(parseDecimal(json.get("periodMax").getAsBigDecimal())); 1183 if (json.has("_periodMax")) 1184 parseElementProperties(getJObject(json, "_periodMax"), res.getPeriodMaxElement()); 1185 if (json.has("periodUnit")) 1186 res.setPeriodUnitElement(parseEnumeration(json.get("periodUnit").getAsString(), Timing.UnitsOfTime.NULL, new Timing.UnitsOfTimeEnumFactory())); 1187 if (json.has("_periodUnit")) 1188 parseElementProperties(getJObject(json, "_periodUnit"), res.getPeriodUnitElement()); 1189 if (json.has("dayOfWeek")) { 1190 JsonArray array = json.getAsJsonArray("dayOfWeek"); 1191 for (int i = 0; i < array.size(); i++) { 1192 if (array.get(i).isJsonNull()) { 1193 res.getDayOfWeek().add(new Enumeration<Timing.DayOfWeek>()); 1194 } else { 1195 res.getDayOfWeek().add(parseEnumeration(array.get(i).getAsString(), Timing.DayOfWeek.NULL, new Timing.DayOfWeekEnumFactory())); 1196 } 1197 } 1198 }; 1199 if (json.has("_dayOfWeek")) { 1200 JsonArray array = json.getAsJsonArray("_dayOfWeek"); 1201 for (int i = 0; i < array.size(); i++) { 1202 if (i == res.getDayOfWeek().size()) 1203 res.getDayOfWeek().add(parseEnumeration(null, Timing.DayOfWeek.NULL, new Timing.DayOfWeekEnumFactory())); 1204 if (array.get(i) instanceof JsonObject) 1205 parseElementProperties(array.get(i).getAsJsonObject(), res.getDayOfWeek().get(i)); 1206 } 1207 }; 1208 if (json.has("timeOfDay")) { 1209 JsonArray array = json.getAsJsonArray("timeOfDay"); 1210 for (int i = 0; i < array.size(); i++) { 1211 if (array.get(i).isJsonNull()) { 1212 res.getTimeOfDay().add(new TimeType()); 1213 } else { 1214 res.getTimeOfDay().add(parseTime(array.get(i).getAsString())); 1215 } 1216 } 1217 }; 1218 if (json.has("_timeOfDay")) { 1219 JsonArray array = json.getAsJsonArray("_timeOfDay"); 1220 for (int i = 0; i < array.size(); i++) { 1221 if (i == res.getTimeOfDay().size()) 1222 res.getTimeOfDay().add(parseTime(null)); 1223 if (array.get(i) instanceof JsonObject) 1224 parseElementProperties(array.get(i).getAsJsonObject(), res.getTimeOfDay().get(i)); 1225 } 1226 }; 1227 if (json.has("when")) { 1228 JsonArray array = json.getAsJsonArray("when"); 1229 for (int i = 0; i < array.size(); i++) { 1230 if (array.get(i).isJsonNull()) { 1231 res.getWhen().add(new Enumeration<Timing.EventTiming>()); 1232 } else { 1233 res.getWhen().add(parseEnumeration(array.get(i).getAsString(), Timing.EventTiming.NULL, new Timing.EventTimingEnumFactory())); 1234 } 1235 } 1236 }; 1237 if (json.has("_when")) { 1238 JsonArray array = json.getAsJsonArray("_when"); 1239 for (int i = 0; i < array.size(); i++) { 1240 if (i == res.getWhen().size()) 1241 res.getWhen().add(parseEnumeration(null, Timing.EventTiming.NULL, new Timing.EventTimingEnumFactory())); 1242 if (array.get(i) instanceof JsonObject) 1243 parseElementProperties(array.get(i).getAsJsonObject(), res.getWhen().get(i)); 1244 } 1245 }; 1246 if (json.has("offset")) 1247 res.setOffsetElement(parseUnsignedInt(json.get("offset").getAsString())); 1248 if (json.has("_offset")) 1249 parseElementProperties(getJObject(json, "_offset"), res.getOffsetElement()); 1250 } 1251 1252 protected ElementDefinition parseElementDefinition(JsonObject json) throws IOException, FHIRFormatError { 1253 ElementDefinition res = new ElementDefinition(); 1254 parseElementDefinitionProperties(json, res); 1255 return res; 1256 } 1257 1258 protected void parseElementDefinitionProperties(JsonObject json, ElementDefinition res) throws IOException, FHIRFormatError { 1259 parseTypeProperties(json, res); 1260 if (json.has("path")) 1261 res.setPathElement(parseString(json.get("path").getAsString())); 1262 if (json.has("_path")) 1263 parseElementProperties(getJObject(json, "_path"), res.getPathElement()); 1264 if (json.has("representation")) { 1265 JsonArray array = json.getAsJsonArray("representation"); 1266 for (int i = 0; i < array.size(); i++) { 1267 if (array.get(i).isJsonNull()) { 1268 res.getRepresentation().add(new Enumeration<ElementDefinition.PropertyRepresentation>()); 1269 } else { 1270 res.getRepresentation().add(parseEnumeration(array.get(i).getAsString(), ElementDefinition.PropertyRepresentation.NULL, new ElementDefinition.PropertyRepresentationEnumFactory())); 1271 } 1272 } 1273 }; 1274 if (json.has("_representation")) { 1275 JsonArray array = json.getAsJsonArray("_representation"); 1276 for (int i = 0; i < array.size(); i++) { 1277 if (i == res.getRepresentation().size()) 1278 res.getRepresentation().add(parseEnumeration(null, ElementDefinition.PropertyRepresentation.NULL, new ElementDefinition.PropertyRepresentationEnumFactory())); 1279 if (array.get(i) instanceof JsonObject) 1280 parseElementProperties(array.get(i).getAsJsonObject(), res.getRepresentation().get(i)); 1281 } 1282 }; 1283 if (json.has("sliceName")) 1284 res.setSliceNameElement(parseString(json.get("sliceName").getAsString())); 1285 if (json.has("_sliceName")) 1286 parseElementProperties(getJObject(json, "_sliceName"), res.getSliceNameElement()); 1287 if (json.has("label")) 1288 res.setLabelElement(parseString(json.get("label").getAsString())); 1289 if (json.has("_label")) 1290 parseElementProperties(getJObject(json, "_label"), res.getLabelElement()); 1291 if (json.has("code")) { 1292 JsonArray array = json.getAsJsonArray("code"); 1293 for (int i = 0; i < array.size(); i++) { 1294 res.getCode().add(parseCoding(array.get(i).getAsJsonObject())); 1295 } 1296 }; 1297 if (json.has("slicing")) 1298 res.setSlicing(parseElementDefinitionElementDefinitionSlicingComponent(getJObject(json, "slicing"), res)); 1299 if (json.has("short")) 1300 res.setShortElement(parseString(json.get("short").getAsString())); 1301 if (json.has("_short")) 1302 parseElementProperties(getJObject(json, "_short"), res.getShortElement()); 1303 if (json.has("definition")) 1304 res.setDefinitionElement(parseMarkdown(json.get("definition").getAsString())); 1305 if (json.has("_definition")) 1306 parseElementProperties(getJObject(json, "_definition"), res.getDefinitionElement()); 1307 if (json.has("comment")) 1308 res.setCommentElement(parseMarkdown(json.get("comment").getAsString())); 1309 if (json.has("_comment")) 1310 parseElementProperties(getJObject(json, "_comment"), res.getCommentElement()); 1311 if (json.has("requirements")) 1312 res.setRequirementsElement(parseMarkdown(json.get("requirements").getAsString())); 1313 if (json.has("_requirements")) 1314 parseElementProperties(getJObject(json, "_requirements"), res.getRequirementsElement()); 1315 if (json.has("alias")) { 1316 JsonArray array = json.getAsJsonArray("alias"); 1317 for (int i = 0; i < array.size(); i++) { 1318 if (array.get(i).isJsonNull()) { 1319 res.getAlias().add(new StringType()); 1320 } else { 1321 res.getAlias().add(parseString(array.get(i).getAsString())); 1322 } 1323 } 1324 }; 1325 if (json.has("_alias")) { 1326 JsonArray array = json.getAsJsonArray("_alias"); 1327 for (int i = 0; i < array.size(); i++) { 1328 if (i == res.getAlias().size()) 1329 res.getAlias().add(parseString(null)); 1330 if (array.get(i) instanceof JsonObject) 1331 parseElementProperties(array.get(i).getAsJsonObject(), res.getAlias().get(i)); 1332 } 1333 }; 1334 if (json.has("min")) 1335 res.setMinElement(parseUnsignedInt(json.get("min").getAsString())); 1336 if (json.has("_min")) 1337 parseElementProperties(getJObject(json, "_min"), res.getMinElement()); 1338 if (json.has("max")) 1339 res.setMaxElement(parseString(json.get("max").getAsString())); 1340 if (json.has("_max")) 1341 parseElementProperties(getJObject(json, "_max"), res.getMaxElement()); 1342 if (json.has("base")) 1343 res.setBase(parseElementDefinitionElementDefinitionBaseComponent(getJObject(json, "base"), res)); 1344 if (json.has("contentReference")) 1345 res.setContentReferenceElement(parseUri(json.get("contentReference").getAsString())); 1346 if (json.has("_contentReference")) 1347 parseElementProperties(getJObject(json, "_contentReference"), res.getContentReferenceElement()); 1348 if (json.has("type")) { 1349 JsonArray array = json.getAsJsonArray("type"); 1350 for (int i = 0; i < array.size(); i++) { 1351 res.getType().add(parseElementDefinitionTypeRefComponent(array.get(i).getAsJsonObject(), res)); 1352 } 1353 }; 1354 Type defaultValue = parseType("defaultValue", json); 1355 if (defaultValue != null) 1356 res.setDefaultValue(defaultValue); 1357 if (json.has("meaningWhenMissing")) 1358 res.setMeaningWhenMissingElement(parseMarkdown(json.get("meaningWhenMissing").getAsString())); 1359 if (json.has("_meaningWhenMissing")) 1360 parseElementProperties(getJObject(json, "_meaningWhenMissing"), res.getMeaningWhenMissingElement()); 1361 if (json.has("orderMeaning")) 1362 res.setOrderMeaningElement(parseString(json.get("orderMeaning").getAsString())); 1363 if (json.has("_orderMeaning")) 1364 parseElementProperties(getJObject(json, "_orderMeaning"), res.getOrderMeaningElement()); 1365 Type fixed = parseType("fixed", json); 1366 if (fixed != null) 1367 res.setFixed(fixed); 1368 Type pattern = parseType("pattern", json); 1369 if (pattern != null) 1370 res.setPattern(pattern); 1371 if (json.has("example")) { 1372 JsonArray array = json.getAsJsonArray("example"); 1373 for (int i = 0; i < array.size(); i++) { 1374 res.getExample().add(parseElementDefinitionElementDefinitionExampleComponent(array.get(i).getAsJsonObject(), res)); 1375 } 1376 }; 1377 Type minValue = parseType("minValue", json); 1378 if (minValue != null) 1379 res.setMinValue(minValue); 1380 Type maxValue = parseType("maxValue", json); 1381 if (maxValue != null) 1382 res.setMaxValue(maxValue); 1383 if (json.has("maxLength")) 1384 res.setMaxLengthElement(parseInteger(json.get("maxLength").getAsLong())); 1385 if (json.has("_maxLength")) 1386 parseElementProperties(getJObject(json, "_maxLength"), res.getMaxLengthElement()); 1387 if (json.has("condition")) { 1388 JsonArray array = json.getAsJsonArray("condition"); 1389 for (int i = 0; i < array.size(); i++) { 1390 if (array.get(i).isJsonNull()) { 1391 res.getCondition().add(new IdType()); 1392 } else { 1393 res.getCondition().add(parseId(array.get(i).getAsString())); 1394 } 1395 } 1396 }; 1397 if (json.has("_condition")) { 1398 JsonArray array = json.getAsJsonArray("_condition"); 1399 for (int i = 0; i < array.size(); i++) { 1400 if (i == res.getCondition().size()) 1401 res.getCondition().add(parseId(null)); 1402 if (array.get(i) instanceof JsonObject) 1403 parseElementProperties(array.get(i).getAsJsonObject(), res.getCondition().get(i)); 1404 } 1405 }; 1406 if (json.has("constraint")) { 1407 JsonArray array = json.getAsJsonArray("constraint"); 1408 for (int i = 0; i < array.size(); i++) { 1409 res.getConstraint().add(parseElementDefinitionElementDefinitionConstraintComponent(array.get(i).getAsJsonObject(), res)); 1410 } 1411 }; 1412 if (json.has("mustSupport")) 1413 res.setMustSupportElement(parseBoolean(json.get("mustSupport").getAsBoolean())); 1414 if (json.has("_mustSupport")) 1415 parseElementProperties(getJObject(json, "_mustSupport"), res.getMustSupportElement()); 1416 if (json.has("isModifier")) 1417 res.setIsModifierElement(parseBoolean(json.get("isModifier").getAsBoolean())); 1418 if (json.has("_isModifier")) 1419 parseElementProperties(getJObject(json, "_isModifier"), res.getIsModifierElement()); 1420 if (json.has("isSummary")) 1421 res.setIsSummaryElement(parseBoolean(json.get("isSummary").getAsBoolean())); 1422 if (json.has("_isSummary")) 1423 parseElementProperties(getJObject(json, "_isSummary"), res.getIsSummaryElement()); 1424 if (json.has("binding")) 1425 res.setBinding(parseElementDefinitionElementDefinitionBindingComponent(getJObject(json, "binding"), res)); 1426 if (json.has("mapping")) { 1427 JsonArray array = json.getAsJsonArray("mapping"); 1428 for (int i = 0; i < array.size(); i++) { 1429 res.getMapping().add(parseElementDefinitionElementDefinitionMappingComponent(array.get(i).getAsJsonObject(), res)); 1430 } 1431 }; 1432 } 1433 1434 protected ElementDefinition.ElementDefinitionSlicingComponent parseElementDefinitionElementDefinitionSlicingComponent(JsonObject json, ElementDefinition owner) throws IOException, FHIRFormatError { 1435 ElementDefinition.ElementDefinitionSlicingComponent res = new ElementDefinition.ElementDefinitionSlicingComponent(); 1436 parseElementDefinitionElementDefinitionSlicingComponentProperties(json, owner, res); 1437 return res; 1438 } 1439 1440 protected void parseElementDefinitionElementDefinitionSlicingComponentProperties(JsonObject json, ElementDefinition owner, ElementDefinition.ElementDefinitionSlicingComponent res) throws IOException, FHIRFormatError { 1441 parseTypeProperties(json, res); 1442 if (json.has("discriminator")) { 1443 JsonArray array = json.getAsJsonArray("discriminator"); 1444 for (int i = 0; i < array.size(); i++) { 1445 res.getDiscriminator().add(parseElementDefinitionElementDefinitionSlicingDiscriminatorComponent(array.get(i).getAsJsonObject(), owner)); 1446 } 1447 }; 1448 if (json.has("description")) 1449 res.setDescriptionElement(parseString(json.get("description").getAsString())); 1450 if (json.has("_description")) 1451 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 1452 if (json.has("ordered")) 1453 res.setOrderedElement(parseBoolean(json.get("ordered").getAsBoolean())); 1454 if (json.has("_ordered")) 1455 parseElementProperties(getJObject(json, "_ordered"), res.getOrderedElement()); 1456 if (json.has("rules")) 1457 res.setRulesElement(parseEnumeration(json.get("rules").getAsString(), ElementDefinition.SlicingRules.NULL, new ElementDefinition.SlicingRulesEnumFactory())); 1458 if (json.has("_rules")) 1459 parseElementProperties(getJObject(json, "_rules"), res.getRulesElement()); 1460 } 1461 1462 protected ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent parseElementDefinitionElementDefinitionSlicingDiscriminatorComponent(JsonObject json, ElementDefinition owner) throws IOException, FHIRFormatError { 1463 ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent res = new ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent(); 1464 parseElementDefinitionElementDefinitionSlicingDiscriminatorComponentProperties(json, owner, res); 1465 return res; 1466 } 1467 1468 protected void parseElementDefinitionElementDefinitionSlicingDiscriminatorComponentProperties(JsonObject json, ElementDefinition owner, ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent res) throws IOException, FHIRFormatError { 1469 parseTypeProperties(json, res); 1470 if (json.has("type")) 1471 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), ElementDefinition.DiscriminatorType.NULL, new ElementDefinition.DiscriminatorTypeEnumFactory())); 1472 if (json.has("_type")) 1473 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 1474 if (json.has("path")) 1475 res.setPathElement(parseString(json.get("path").getAsString())); 1476 if (json.has("_path")) 1477 parseElementProperties(getJObject(json, "_path"), res.getPathElement()); 1478 } 1479 1480 protected ElementDefinition.ElementDefinitionBaseComponent parseElementDefinitionElementDefinitionBaseComponent(JsonObject json, ElementDefinition owner) throws IOException, FHIRFormatError { 1481 ElementDefinition.ElementDefinitionBaseComponent res = new ElementDefinition.ElementDefinitionBaseComponent(); 1482 parseElementDefinitionElementDefinitionBaseComponentProperties(json, owner, res); 1483 return res; 1484 } 1485 1486 protected void parseElementDefinitionElementDefinitionBaseComponentProperties(JsonObject json, ElementDefinition owner, ElementDefinition.ElementDefinitionBaseComponent res) throws IOException, FHIRFormatError { 1487 parseTypeProperties(json, res); 1488 if (json.has("path")) 1489 res.setPathElement(parseString(json.get("path").getAsString())); 1490 if (json.has("_path")) 1491 parseElementProperties(getJObject(json, "_path"), res.getPathElement()); 1492 if (json.has("min")) 1493 res.setMinElement(parseUnsignedInt(json.get("min").getAsString())); 1494 if (json.has("_min")) 1495 parseElementProperties(getJObject(json, "_min"), res.getMinElement()); 1496 if (json.has("max")) 1497 res.setMaxElement(parseString(json.get("max").getAsString())); 1498 if (json.has("_max")) 1499 parseElementProperties(getJObject(json, "_max"), res.getMaxElement()); 1500 } 1501 1502 protected ElementDefinition.TypeRefComponent parseElementDefinitionTypeRefComponent(JsonObject json, ElementDefinition owner) throws IOException, FHIRFormatError { 1503 ElementDefinition.TypeRefComponent res = new ElementDefinition.TypeRefComponent(); 1504 parseElementDefinitionTypeRefComponentProperties(json, owner, res); 1505 return res; 1506 } 1507 1508 protected void parseElementDefinitionTypeRefComponentProperties(JsonObject json, ElementDefinition owner, ElementDefinition.TypeRefComponent res) throws IOException, FHIRFormatError { 1509 parseTypeProperties(json, res); 1510 if (json.has("code")) 1511 res.setCodeElement(parseUri(json.get("code").getAsString())); 1512 if (json.has("_code")) 1513 parseElementProperties(getJObject(json, "_code"), res.getCodeElement()); 1514 if (json.has("profile")) 1515 res.setProfileElement(parseUri(json.get("profile").getAsString())); 1516 if (json.has("_profile")) 1517 parseElementProperties(getJObject(json, "_profile"), res.getProfileElement()); 1518 if (json.has("targetProfile")) 1519 res.setTargetProfileElement(parseUri(json.get("targetProfile").getAsString())); 1520 if (json.has("_targetProfile")) 1521 parseElementProperties(getJObject(json, "_targetProfile"), res.getTargetProfileElement()); 1522 if (json.has("aggregation")) { 1523 JsonArray array = json.getAsJsonArray("aggregation"); 1524 for (int i = 0; i < array.size(); i++) { 1525 if (array.get(i).isJsonNull()) { 1526 res.getAggregation().add(new Enumeration<ElementDefinition.AggregationMode>()); 1527 } else { 1528 res.getAggregation().add(parseEnumeration(array.get(i).getAsString(), ElementDefinition.AggregationMode.NULL, new ElementDefinition.AggregationModeEnumFactory())); 1529 } 1530 } 1531 }; 1532 if (json.has("_aggregation")) { 1533 JsonArray array = json.getAsJsonArray("_aggregation"); 1534 for (int i = 0; i < array.size(); i++) { 1535 if (i == res.getAggregation().size()) 1536 res.getAggregation().add(parseEnumeration(null, ElementDefinition.AggregationMode.NULL, new ElementDefinition.AggregationModeEnumFactory())); 1537 if (array.get(i) instanceof JsonObject) 1538 parseElementProperties(array.get(i).getAsJsonObject(), res.getAggregation().get(i)); 1539 } 1540 }; 1541 if (json.has("versioning")) 1542 res.setVersioningElement(parseEnumeration(json.get("versioning").getAsString(), ElementDefinition.ReferenceVersionRules.NULL, new ElementDefinition.ReferenceVersionRulesEnumFactory())); 1543 if (json.has("_versioning")) 1544 parseElementProperties(getJObject(json, "_versioning"), res.getVersioningElement()); 1545 } 1546 1547 protected ElementDefinition.ElementDefinitionExampleComponent parseElementDefinitionElementDefinitionExampleComponent(JsonObject json, ElementDefinition owner) throws IOException, FHIRFormatError { 1548 ElementDefinition.ElementDefinitionExampleComponent res = new ElementDefinition.ElementDefinitionExampleComponent(); 1549 parseElementDefinitionElementDefinitionExampleComponentProperties(json, owner, res); 1550 return res; 1551 } 1552 1553 protected void parseElementDefinitionElementDefinitionExampleComponentProperties(JsonObject json, ElementDefinition owner, ElementDefinition.ElementDefinitionExampleComponent res) throws IOException, FHIRFormatError { 1554 parseTypeProperties(json, res); 1555 if (json.has("label")) 1556 res.setLabelElement(parseString(json.get("label").getAsString())); 1557 if (json.has("_label")) 1558 parseElementProperties(getJObject(json, "_label"), res.getLabelElement()); 1559 Type value = parseType("value", json); 1560 if (value != null) 1561 res.setValue(value); 1562 } 1563 1564 protected ElementDefinition.ElementDefinitionConstraintComponent parseElementDefinitionElementDefinitionConstraintComponent(JsonObject json, ElementDefinition owner) throws IOException, FHIRFormatError { 1565 ElementDefinition.ElementDefinitionConstraintComponent res = new ElementDefinition.ElementDefinitionConstraintComponent(); 1566 parseElementDefinitionElementDefinitionConstraintComponentProperties(json, owner, res); 1567 return res; 1568 } 1569 1570 protected void parseElementDefinitionElementDefinitionConstraintComponentProperties(JsonObject json, ElementDefinition owner, ElementDefinition.ElementDefinitionConstraintComponent res) throws IOException, FHIRFormatError { 1571 parseTypeProperties(json, res); 1572 if (json.has("key")) 1573 res.setKeyElement(parseId(json.get("key").getAsString())); 1574 if (json.has("_key")) 1575 parseElementProperties(getJObject(json, "_key"), res.getKeyElement()); 1576 if (json.has("requirements")) 1577 res.setRequirementsElement(parseString(json.get("requirements").getAsString())); 1578 if (json.has("_requirements")) 1579 parseElementProperties(getJObject(json, "_requirements"), res.getRequirementsElement()); 1580 if (json.has("severity")) 1581 res.setSeverityElement(parseEnumeration(json.get("severity").getAsString(), ElementDefinition.ConstraintSeverity.NULL, new ElementDefinition.ConstraintSeverityEnumFactory())); 1582 if (json.has("_severity")) 1583 parseElementProperties(getJObject(json, "_severity"), res.getSeverityElement()); 1584 if (json.has("human")) 1585 res.setHumanElement(parseString(json.get("human").getAsString())); 1586 if (json.has("_human")) 1587 parseElementProperties(getJObject(json, "_human"), res.getHumanElement()); 1588 if (json.has("expression")) 1589 res.setExpressionElement(parseString(json.get("expression").getAsString())); 1590 if (json.has("_expression")) 1591 parseElementProperties(getJObject(json, "_expression"), res.getExpressionElement()); 1592 if (json.has("xpath")) 1593 res.setXpathElement(parseString(json.get("xpath").getAsString())); 1594 if (json.has("_xpath")) 1595 parseElementProperties(getJObject(json, "_xpath"), res.getXpathElement()); 1596 if (json.has("source")) 1597 res.setSourceElement(parseUri(json.get("source").getAsString())); 1598 if (json.has("_source")) 1599 parseElementProperties(getJObject(json, "_source"), res.getSourceElement()); 1600 } 1601 1602 protected ElementDefinition.ElementDefinitionBindingComponent parseElementDefinitionElementDefinitionBindingComponent(JsonObject json, ElementDefinition owner) throws IOException, FHIRFormatError { 1603 ElementDefinition.ElementDefinitionBindingComponent res = new ElementDefinition.ElementDefinitionBindingComponent(); 1604 parseElementDefinitionElementDefinitionBindingComponentProperties(json, owner, res); 1605 return res; 1606 } 1607 1608 protected void parseElementDefinitionElementDefinitionBindingComponentProperties(JsonObject json, ElementDefinition owner, ElementDefinition.ElementDefinitionBindingComponent res) throws IOException, FHIRFormatError { 1609 parseTypeProperties(json, res); 1610 if (json.has("strength")) 1611 res.setStrengthElement(parseEnumeration(json.get("strength").getAsString(), Enumerations.BindingStrength.NULL, new Enumerations.BindingStrengthEnumFactory())); 1612 if (json.has("_strength")) 1613 parseElementProperties(getJObject(json, "_strength"), res.getStrengthElement()); 1614 if (json.has("description")) 1615 res.setDescriptionElement(parseString(json.get("description").getAsString())); 1616 if (json.has("_description")) 1617 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 1618 Type valueSet = parseType("valueSet", json); 1619 if (valueSet != null) 1620 res.setValueSet(valueSet); 1621 } 1622 1623 protected ElementDefinition.ElementDefinitionMappingComponent parseElementDefinitionElementDefinitionMappingComponent(JsonObject json, ElementDefinition owner) throws IOException, FHIRFormatError { 1624 ElementDefinition.ElementDefinitionMappingComponent res = new ElementDefinition.ElementDefinitionMappingComponent(); 1625 parseElementDefinitionElementDefinitionMappingComponentProperties(json, owner, res); 1626 return res; 1627 } 1628 1629 protected void parseElementDefinitionElementDefinitionMappingComponentProperties(JsonObject json, ElementDefinition owner, ElementDefinition.ElementDefinitionMappingComponent res) throws IOException, FHIRFormatError { 1630 parseTypeProperties(json, res); 1631 if (json.has("identity")) 1632 res.setIdentityElement(parseId(json.get("identity").getAsString())); 1633 if (json.has("_identity")) 1634 parseElementProperties(getJObject(json, "_identity"), res.getIdentityElement()); 1635 if (json.has("language")) 1636 res.setLanguageElement(parseCode(json.get("language").getAsString())); 1637 if (json.has("_language")) 1638 parseElementProperties(getJObject(json, "_language"), res.getLanguageElement()); 1639 if (json.has("map")) 1640 res.setMapElement(parseString(json.get("map").getAsString())); 1641 if (json.has("_map")) 1642 parseElementProperties(getJObject(json, "_map"), res.getMapElement()); 1643 if (json.has("comment")) 1644 res.setCommentElement(parseString(json.get("comment").getAsString())); 1645 if (json.has("_comment")) 1646 parseElementProperties(getJObject(json, "_comment"), res.getCommentElement()); 1647 } 1648 1649 protected ParameterDefinition parseParameterDefinition(JsonObject json) throws IOException, FHIRFormatError { 1650 ParameterDefinition res = new ParameterDefinition(); 1651 parseParameterDefinitionProperties(json, res); 1652 return res; 1653 } 1654 1655 protected void parseParameterDefinitionProperties(JsonObject json, ParameterDefinition res) throws IOException, FHIRFormatError { 1656 parseTypeProperties(json, res); 1657 if (json.has("name")) 1658 res.setNameElement(parseCode(json.get("name").getAsString())); 1659 if (json.has("_name")) 1660 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 1661 if (json.has("use")) 1662 res.setUseElement(parseEnumeration(json.get("use").getAsString(), ParameterDefinition.ParameterUse.NULL, new ParameterDefinition.ParameterUseEnumFactory())); 1663 if (json.has("_use")) 1664 parseElementProperties(getJObject(json, "_use"), res.getUseElement()); 1665 if (json.has("min")) 1666 res.setMinElement(parseInteger(json.get("min").getAsLong())); 1667 if (json.has("_min")) 1668 parseElementProperties(getJObject(json, "_min"), res.getMinElement()); 1669 if (json.has("max")) 1670 res.setMaxElement(parseString(json.get("max").getAsString())); 1671 if (json.has("_max")) 1672 parseElementProperties(getJObject(json, "_max"), res.getMaxElement()); 1673 if (json.has("documentation")) 1674 res.setDocumentationElement(parseString(json.get("documentation").getAsString())); 1675 if (json.has("_documentation")) 1676 parseElementProperties(getJObject(json, "_documentation"), res.getDocumentationElement()); 1677 if (json.has("type")) 1678 res.setTypeElement(parseCode(json.get("type").getAsString())); 1679 if (json.has("_type")) 1680 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 1681 if (json.has("profile")) 1682 res.setProfile(parseReference(getJObject(json, "profile"))); 1683 } 1684 1685 protected void parseDomainResourceProperties(JsonObject json, DomainResource res) throws IOException, FHIRFormatError { 1686 parseResourceProperties(json, res); 1687 if (json.has("text")) 1688 res.setText(parseNarrative(getJObject(json, "text"))); 1689 if (json.has("contained")) { 1690 JsonArray array = json.getAsJsonArray("contained"); 1691 for (int i = 0; i < array.size(); i++) { 1692 res.getContained().add(parseResource(array.get(i).getAsJsonObject())); 1693 } 1694 }; 1695 if (json.has("extension")) { 1696 JsonArray array = json.getAsJsonArray("extension"); 1697 for (int i = 0; i < array.size(); i++) { 1698 res.getExtension().add(parseExtension(array.get(i).getAsJsonObject())); 1699 } 1700 }; 1701 if (json.has("modifierExtension")) { 1702 JsonArray array = json.getAsJsonArray("modifierExtension"); 1703 for (int i = 0; i < array.size(); i++) { 1704 res.getModifierExtension().add(parseExtension(array.get(i).getAsJsonObject())); 1705 } 1706 }; 1707 } 1708 1709 protected Parameters parseParameters(JsonObject json) throws IOException, FHIRFormatError { 1710 Parameters res = new Parameters(); 1711 parseParametersProperties(json, res); 1712 return res; 1713 } 1714 1715 protected void parseParametersProperties(JsonObject json, Parameters res) throws IOException, FHIRFormatError { 1716 parseResourceProperties(json, res); 1717 if (json.has("parameter")) { 1718 JsonArray array = json.getAsJsonArray("parameter"); 1719 for (int i = 0; i < array.size(); i++) { 1720 res.getParameter().add(parseParametersParametersParameterComponent(array.get(i).getAsJsonObject(), res)); 1721 } 1722 }; 1723 } 1724 1725 protected Parameters.ParametersParameterComponent parseParametersParametersParameterComponent(JsonObject json, Parameters owner) throws IOException, FHIRFormatError { 1726 Parameters.ParametersParameterComponent res = new Parameters.ParametersParameterComponent(); 1727 parseParametersParametersParameterComponentProperties(json, owner, res); 1728 return res; 1729 } 1730 1731 protected void parseParametersParametersParameterComponentProperties(JsonObject json, Parameters owner, Parameters.ParametersParameterComponent res) throws IOException, FHIRFormatError { 1732 parseBackboneProperties(json, res); 1733 if (json.has("name")) 1734 res.setNameElement(parseString(json.get("name").getAsString())); 1735 if (json.has("_name")) 1736 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 1737 Type value = parseType("value", json); 1738 if (value != null) 1739 res.setValue(value); 1740 if (json.has("resource")) 1741 res.setResource(parseResource(getJObject(json, "resource"))); 1742 if (json.has("part")) { 1743 JsonArray array = json.getAsJsonArray("part"); 1744 for (int i = 0; i < array.size(); i++) { 1745 res.getPart().add(parseParametersParametersParameterComponent(array.get(i).getAsJsonObject(), owner)); 1746 } 1747 }; 1748 } 1749 1750 protected void parseResourceProperties(JsonObject json, Resource res) throws IOException, FHIRFormatError { 1751 if (json.has("id")) 1752 res.setIdElement(parseId(json.get("id").getAsString())); 1753 if (json.has("_id")) 1754 parseElementProperties(getJObject(json, "_id"), res.getIdElement()); 1755 if (json.has("meta")) 1756 res.setMeta(parseMeta(getJObject(json, "meta"))); 1757 if (json.has("implicitRules")) 1758 res.setImplicitRulesElement(parseUri(json.get("implicitRules").getAsString())); 1759 if (json.has("_implicitRules")) 1760 parseElementProperties(getJObject(json, "_implicitRules"), res.getImplicitRulesElement()); 1761 if (json.has("language")) 1762 res.setLanguageElement(parseCode(json.get("language").getAsString())); 1763 if (json.has("_language")) 1764 parseElementProperties(getJObject(json, "_language"), res.getLanguageElement()); 1765 } 1766 1767 protected Account parseAccount(JsonObject json) throws IOException, FHIRFormatError { 1768 Account res = new Account(); 1769 parseAccountProperties(json, res); 1770 return res; 1771 } 1772 1773 protected void parseAccountProperties(JsonObject json, Account res) throws IOException, FHIRFormatError { 1774 parseDomainResourceProperties(json, res); 1775 if (json.has("identifier")) { 1776 JsonArray array = json.getAsJsonArray("identifier"); 1777 for (int i = 0; i < array.size(); i++) { 1778 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 1779 } 1780 }; 1781 if (json.has("status")) 1782 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Account.AccountStatus.NULL, new Account.AccountStatusEnumFactory())); 1783 if (json.has("_status")) 1784 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 1785 if (json.has("type")) 1786 res.setType(parseCodeableConcept(getJObject(json, "type"))); 1787 if (json.has("name")) 1788 res.setNameElement(parseString(json.get("name").getAsString())); 1789 if (json.has("_name")) 1790 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 1791 if (json.has("subject")) 1792 res.setSubject(parseReference(getJObject(json, "subject"))); 1793 if (json.has("period")) 1794 res.setPeriod(parsePeriod(getJObject(json, "period"))); 1795 if (json.has("active")) 1796 res.setActive(parsePeriod(getJObject(json, "active"))); 1797 if (json.has("balance")) 1798 res.setBalance(parseMoney(getJObject(json, "balance"))); 1799 if (json.has("coverage")) { 1800 JsonArray array = json.getAsJsonArray("coverage"); 1801 for (int i = 0; i < array.size(); i++) { 1802 res.getCoverage().add(parseAccountCoverageComponent(array.get(i).getAsJsonObject(), res)); 1803 } 1804 }; 1805 if (json.has("owner")) 1806 res.setOwner(parseReference(getJObject(json, "owner"))); 1807 if (json.has("description")) 1808 res.setDescriptionElement(parseString(json.get("description").getAsString())); 1809 if (json.has("_description")) 1810 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 1811 if (json.has("guarantor")) { 1812 JsonArray array = json.getAsJsonArray("guarantor"); 1813 for (int i = 0; i < array.size(); i++) { 1814 res.getGuarantor().add(parseAccountGuarantorComponent(array.get(i).getAsJsonObject(), res)); 1815 } 1816 }; 1817 } 1818 1819 protected Account.CoverageComponent parseAccountCoverageComponent(JsonObject json, Account owner) throws IOException, FHIRFormatError { 1820 Account.CoverageComponent res = new Account.CoverageComponent(); 1821 parseAccountCoverageComponentProperties(json, owner, res); 1822 return res; 1823 } 1824 1825 protected void parseAccountCoverageComponentProperties(JsonObject json, Account owner, Account.CoverageComponent res) throws IOException, FHIRFormatError { 1826 parseBackboneProperties(json, res); 1827 if (json.has("coverage")) 1828 res.setCoverage(parseReference(getJObject(json, "coverage"))); 1829 if (json.has("priority")) 1830 res.setPriorityElement(parsePositiveInt(json.get("priority").getAsString())); 1831 if (json.has("_priority")) 1832 parseElementProperties(getJObject(json, "_priority"), res.getPriorityElement()); 1833 } 1834 1835 protected Account.GuarantorComponent parseAccountGuarantorComponent(JsonObject json, Account owner) throws IOException, FHIRFormatError { 1836 Account.GuarantorComponent res = new Account.GuarantorComponent(); 1837 parseAccountGuarantorComponentProperties(json, owner, res); 1838 return res; 1839 } 1840 1841 protected void parseAccountGuarantorComponentProperties(JsonObject json, Account owner, Account.GuarantorComponent res) throws IOException, FHIRFormatError { 1842 parseBackboneProperties(json, res); 1843 if (json.has("party")) 1844 res.setParty(parseReference(getJObject(json, "party"))); 1845 if (json.has("onHold")) 1846 res.setOnHoldElement(parseBoolean(json.get("onHold").getAsBoolean())); 1847 if (json.has("_onHold")) 1848 parseElementProperties(getJObject(json, "_onHold"), res.getOnHoldElement()); 1849 if (json.has("period")) 1850 res.setPeriod(parsePeriod(getJObject(json, "period"))); 1851 } 1852 1853 protected ActivityDefinition parseActivityDefinition(JsonObject json) throws IOException, FHIRFormatError { 1854 ActivityDefinition res = new ActivityDefinition(); 1855 parseActivityDefinitionProperties(json, res); 1856 return res; 1857 } 1858 1859 protected void parseActivityDefinitionProperties(JsonObject json, ActivityDefinition res) throws IOException, FHIRFormatError { 1860 parseDomainResourceProperties(json, res); 1861 if (json.has("url")) 1862 res.setUrlElement(parseUri(json.get("url").getAsString())); 1863 if (json.has("_url")) 1864 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 1865 if (json.has("identifier")) { 1866 JsonArray array = json.getAsJsonArray("identifier"); 1867 for (int i = 0; i < array.size(); i++) { 1868 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 1869 } 1870 }; 1871 if (json.has("version")) 1872 res.setVersionElement(parseString(json.get("version").getAsString())); 1873 if (json.has("_version")) 1874 parseElementProperties(getJObject(json, "_version"), res.getVersionElement()); 1875 if (json.has("name")) 1876 res.setNameElement(parseString(json.get("name").getAsString())); 1877 if (json.has("_name")) 1878 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 1879 if (json.has("title")) 1880 res.setTitleElement(parseString(json.get("title").getAsString())); 1881 if (json.has("_title")) 1882 parseElementProperties(getJObject(json, "_title"), res.getTitleElement()); 1883 if (json.has("status")) 1884 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Enumerations.PublicationStatus.NULL, new Enumerations.PublicationStatusEnumFactory())); 1885 if (json.has("_status")) 1886 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 1887 if (json.has("experimental")) 1888 res.setExperimentalElement(parseBoolean(json.get("experimental").getAsBoolean())); 1889 if (json.has("_experimental")) 1890 parseElementProperties(getJObject(json, "_experimental"), res.getExperimentalElement()); 1891 if (json.has("date")) 1892 res.setDateElement(parseDateTime(json.get("date").getAsString())); 1893 if (json.has("_date")) 1894 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 1895 if (json.has("publisher")) 1896 res.setPublisherElement(parseString(json.get("publisher").getAsString())); 1897 if (json.has("_publisher")) 1898 parseElementProperties(getJObject(json, "_publisher"), res.getPublisherElement()); 1899 if (json.has("description")) 1900 res.setDescriptionElement(parseMarkdown(json.get("description").getAsString())); 1901 if (json.has("_description")) 1902 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 1903 if (json.has("purpose")) 1904 res.setPurposeElement(parseMarkdown(json.get("purpose").getAsString())); 1905 if (json.has("_purpose")) 1906 parseElementProperties(getJObject(json, "_purpose"), res.getPurposeElement()); 1907 if (json.has("usage")) 1908 res.setUsageElement(parseString(json.get("usage").getAsString())); 1909 if (json.has("_usage")) 1910 parseElementProperties(getJObject(json, "_usage"), res.getUsageElement()); 1911 if (json.has("approvalDate")) 1912 res.setApprovalDateElement(parseDate(json.get("approvalDate").getAsString())); 1913 if (json.has("_approvalDate")) 1914 parseElementProperties(getJObject(json, "_approvalDate"), res.getApprovalDateElement()); 1915 if (json.has("lastReviewDate")) 1916 res.setLastReviewDateElement(parseDate(json.get("lastReviewDate").getAsString())); 1917 if (json.has("_lastReviewDate")) 1918 parseElementProperties(getJObject(json, "_lastReviewDate"), res.getLastReviewDateElement()); 1919 if (json.has("effectivePeriod")) 1920 res.setEffectivePeriod(parsePeriod(getJObject(json, "effectivePeriod"))); 1921 if (json.has("useContext")) { 1922 JsonArray array = json.getAsJsonArray("useContext"); 1923 for (int i = 0; i < array.size(); i++) { 1924 res.getUseContext().add(parseUsageContext(array.get(i).getAsJsonObject())); 1925 } 1926 }; 1927 if (json.has("jurisdiction")) { 1928 JsonArray array = json.getAsJsonArray("jurisdiction"); 1929 for (int i = 0; i < array.size(); i++) { 1930 res.getJurisdiction().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 1931 } 1932 }; 1933 if (json.has("topic")) { 1934 JsonArray array = json.getAsJsonArray("topic"); 1935 for (int i = 0; i < array.size(); i++) { 1936 res.getTopic().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 1937 } 1938 }; 1939 if (json.has("contributor")) { 1940 JsonArray array = json.getAsJsonArray("contributor"); 1941 for (int i = 0; i < array.size(); i++) { 1942 res.getContributor().add(parseContributor(array.get(i).getAsJsonObject())); 1943 } 1944 }; 1945 if (json.has("contact")) { 1946 JsonArray array = json.getAsJsonArray("contact"); 1947 for (int i = 0; i < array.size(); i++) { 1948 res.getContact().add(parseContactDetail(array.get(i).getAsJsonObject())); 1949 } 1950 }; 1951 if (json.has("copyright")) 1952 res.setCopyrightElement(parseMarkdown(json.get("copyright").getAsString())); 1953 if (json.has("_copyright")) 1954 parseElementProperties(getJObject(json, "_copyright"), res.getCopyrightElement()); 1955 if (json.has("relatedArtifact")) { 1956 JsonArray array = json.getAsJsonArray("relatedArtifact"); 1957 for (int i = 0; i < array.size(); i++) { 1958 res.getRelatedArtifact().add(parseRelatedArtifact(array.get(i).getAsJsonObject())); 1959 } 1960 }; 1961 if (json.has("library")) { 1962 JsonArray array = json.getAsJsonArray("library"); 1963 for (int i = 0; i < array.size(); i++) { 1964 res.getLibrary().add(parseReference(array.get(i).getAsJsonObject())); 1965 } 1966 }; 1967 if (json.has("kind")) 1968 res.setKindElement(parseEnumeration(json.get("kind").getAsString(), ActivityDefinition.ActivityDefinitionKind.NULL, new ActivityDefinition.ActivityDefinitionKindEnumFactory())); 1969 if (json.has("_kind")) 1970 parseElementProperties(getJObject(json, "_kind"), res.getKindElement()); 1971 if (json.has("code")) 1972 res.setCode(parseCodeableConcept(getJObject(json, "code"))); 1973 Type timing = parseType("timing", json); 1974 if (timing != null) 1975 res.setTiming(timing); 1976 if (json.has("location")) 1977 res.setLocation(parseReference(getJObject(json, "location"))); 1978 if (json.has("participant")) { 1979 JsonArray array = json.getAsJsonArray("participant"); 1980 for (int i = 0; i < array.size(); i++) { 1981 res.getParticipant().add(parseActivityDefinitionActivityDefinitionParticipantComponent(array.get(i).getAsJsonObject(), res)); 1982 } 1983 }; 1984 Type product = parseType("product", json); 1985 if (product != null) 1986 res.setProduct(product); 1987 if (json.has("quantity")) 1988 res.setQuantity(parseSimpleQuantity(getJObject(json, "quantity"))); 1989 if (json.has("dosage")) { 1990 JsonArray array = json.getAsJsonArray("dosage"); 1991 for (int i = 0; i < array.size(); i++) { 1992 res.getDosage().add(parseDosage(array.get(i).getAsJsonObject())); 1993 } 1994 }; 1995 if (json.has("bodySite")) { 1996 JsonArray array = json.getAsJsonArray("bodySite"); 1997 for (int i = 0; i < array.size(); i++) { 1998 res.getBodySite().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 1999 } 2000 }; 2001 if (json.has("transform")) 2002 res.setTransform(parseReference(getJObject(json, "transform"))); 2003 if (json.has("dynamicValue")) { 2004 JsonArray array = json.getAsJsonArray("dynamicValue"); 2005 for (int i = 0; i < array.size(); i++) { 2006 res.getDynamicValue().add(parseActivityDefinitionActivityDefinitionDynamicValueComponent(array.get(i).getAsJsonObject(), res)); 2007 } 2008 }; 2009 } 2010 2011 protected ActivityDefinition.ActivityDefinitionParticipantComponent parseActivityDefinitionActivityDefinitionParticipantComponent(JsonObject json, ActivityDefinition owner) throws IOException, FHIRFormatError { 2012 ActivityDefinition.ActivityDefinitionParticipantComponent res = new ActivityDefinition.ActivityDefinitionParticipantComponent(); 2013 parseActivityDefinitionActivityDefinitionParticipantComponentProperties(json, owner, res); 2014 return res; 2015 } 2016 2017 protected void parseActivityDefinitionActivityDefinitionParticipantComponentProperties(JsonObject json, ActivityDefinition owner, ActivityDefinition.ActivityDefinitionParticipantComponent res) throws IOException, FHIRFormatError { 2018 parseBackboneProperties(json, res); 2019 if (json.has("type")) 2020 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), ActivityDefinition.ActivityParticipantType.NULL, new ActivityDefinition.ActivityParticipantTypeEnumFactory())); 2021 if (json.has("_type")) 2022 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 2023 if (json.has("role")) 2024 res.setRole(parseCodeableConcept(getJObject(json, "role"))); 2025 } 2026 2027 protected ActivityDefinition.ActivityDefinitionDynamicValueComponent parseActivityDefinitionActivityDefinitionDynamicValueComponent(JsonObject json, ActivityDefinition owner) throws IOException, FHIRFormatError { 2028 ActivityDefinition.ActivityDefinitionDynamicValueComponent res = new ActivityDefinition.ActivityDefinitionDynamicValueComponent(); 2029 parseActivityDefinitionActivityDefinitionDynamicValueComponentProperties(json, owner, res); 2030 return res; 2031 } 2032 2033 protected void parseActivityDefinitionActivityDefinitionDynamicValueComponentProperties(JsonObject json, ActivityDefinition owner, ActivityDefinition.ActivityDefinitionDynamicValueComponent res) throws IOException, FHIRFormatError { 2034 parseBackboneProperties(json, res); 2035 if (json.has("description")) 2036 res.setDescriptionElement(parseString(json.get("description").getAsString())); 2037 if (json.has("_description")) 2038 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 2039 if (json.has("path")) 2040 res.setPathElement(parseString(json.get("path").getAsString())); 2041 if (json.has("_path")) 2042 parseElementProperties(getJObject(json, "_path"), res.getPathElement()); 2043 if (json.has("language")) 2044 res.setLanguageElement(parseString(json.get("language").getAsString())); 2045 if (json.has("_language")) 2046 parseElementProperties(getJObject(json, "_language"), res.getLanguageElement()); 2047 if (json.has("expression")) 2048 res.setExpressionElement(parseString(json.get("expression").getAsString())); 2049 if (json.has("_expression")) 2050 parseElementProperties(getJObject(json, "_expression"), res.getExpressionElement()); 2051 } 2052 2053 protected AdverseEvent parseAdverseEvent(JsonObject json) throws IOException, FHIRFormatError { 2054 AdverseEvent res = new AdverseEvent(); 2055 parseAdverseEventProperties(json, res); 2056 return res; 2057 } 2058 2059 protected void parseAdverseEventProperties(JsonObject json, AdverseEvent res) throws IOException, FHIRFormatError { 2060 parseDomainResourceProperties(json, res); 2061 if (json.has("identifier")) 2062 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 2063 if (json.has("category")) 2064 res.setCategoryElement(parseEnumeration(json.get("category").getAsString(), AdverseEvent.AdverseEventCategory.NULL, new AdverseEvent.AdverseEventCategoryEnumFactory())); 2065 if (json.has("_category")) 2066 parseElementProperties(getJObject(json, "_category"), res.getCategoryElement()); 2067 if (json.has("type")) 2068 res.setType(parseCodeableConcept(getJObject(json, "type"))); 2069 if (json.has("subject")) 2070 res.setSubject(parseReference(getJObject(json, "subject"))); 2071 if (json.has("date")) 2072 res.setDateElement(parseDateTime(json.get("date").getAsString())); 2073 if (json.has("_date")) 2074 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 2075 if (json.has("reaction")) { 2076 JsonArray array = json.getAsJsonArray("reaction"); 2077 for (int i = 0; i < array.size(); i++) { 2078 res.getReaction().add(parseReference(array.get(i).getAsJsonObject())); 2079 } 2080 }; 2081 if (json.has("location")) 2082 res.setLocation(parseReference(getJObject(json, "location"))); 2083 if (json.has("seriousness")) 2084 res.setSeriousness(parseCodeableConcept(getJObject(json, "seriousness"))); 2085 if (json.has("outcome")) 2086 res.setOutcome(parseCodeableConcept(getJObject(json, "outcome"))); 2087 if (json.has("recorder")) 2088 res.setRecorder(parseReference(getJObject(json, "recorder"))); 2089 if (json.has("eventParticipant")) 2090 res.setEventParticipant(parseReference(getJObject(json, "eventParticipant"))); 2091 if (json.has("description")) 2092 res.setDescriptionElement(parseString(json.get("description").getAsString())); 2093 if (json.has("_description")) 2094 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 2095 if (json.has("suspectEntity")) { 2096 JsonArray array = json.getAsJsonArray("suspectEntity"); 2097 for (int i = 0; i < array.size(); i++) { 2098 res.getSuspectEntity().add(parseAdverseEventAdverseEventSuspectEntityComponent(array.get(i).getAsJsonObject(), res)); 2099 } 2100 }; 2101 if (json.has("subjectMedicalHistory")) { 2102 JsonArray array = json.getAsJsonArray("subjectMedicalHistory"); 2103 for (int i = 0; i < array.size(); i++) { 2104 res.getSubjectMedicalHistory().add(parseReference(array.get(i).getAsJsonObject())); 2105 } 2106 }; 2107 if (json.has("referenceDocument")) { 2108 JsonArray array = json.getAsJsonArray("referenceDocument"); 2109 for (int i = 0; i < array.size(); i++) { 2110 res.getReferenceDocument().add(parseReference(array.get(i).getAsJsonObject())); 2111 } 2112 }; 2113 if (json.has("study")) { 2114 JsonArray array = json.getAsJsonArray("study"); 2115 for (int i = 0; i < array.size(); i++) { 2116 res.getStudy().add(parseReference(array.get(i).getAsJsonObject())); 2117 } 2118 }; 2119 } 2120 2121 protected AdverseEvent.AdverseEventSuspectEntityComponent parseAdverseEventAdverseEventSuspectEntityComponent(JsonObject json, AdverseEvent owner) throws IOException, FHIRFormatError { 2122 AdverseEvent.AdverseEventSuspectEntityComponent res = new AdverseEvent.AdverseEventSuspectEntityComponent(); 2123 parseAdverseEventAdverseEventSuspectEntityComponentProperties(json, owner, res); 2124 return res; 2125 } 2126 2127 protected void parseAdverseEventAdverseEventSuspectEntityComponentProperties(JsonObject json, AdverseEvent owner, AdverseEvent.AdverseEventSuspectEntityComponent res) throws IOException, FHIRFormatError { 2128 parseBackboneProperties(json, res); 2129 if (json.has("instance")) 2130 res.setInstance(parseReference(getJObject(json, "instance"))); 2131 if (json.has("causality")) 2132 res.setCausalityElement(parseEnumeration(json.get("causality").getAsString(), AdverseEvent.AdverseEventCausality.NULL, new AdverseEvent.AdverseEventCausalityEnumFactory())); 2133 if (json.has("_causality")) 2134 parseElementProperties(getJObject(json, "_causality"), res.getCausalityElement()); 2135 if (json.has("causalityAssessment")) 2136 res.setCausalityAssessment(parseCodeableConcept(getJObject(json, "causalityAssessment"))); 2137 if (json.has("causalityProductRelatedness")) 2138 res.setCausalityProductRelatednessElement(parseString(json.get("causalityProductRelatedness").getAsString())); 2139 if (json.has("_causalityProductRelatedness")) 2140 parseElementProperties(getJObject(json, "_causalityProductRelatedness"), res.getCausalityProductRelatednessElement()); 2141 if (json.has("causalityMethod")) 2142 res.setCausalityMethod(parseCodeableConcept(getJObject(json, "causalityMethod"))); 2143 if (json.has("causalityAuthor")) 2144 res.setCausalityAuthor(parseReference(getJObject(json, "causalityAuthor"))); 2145 if (json.has("causalityResult")) 2146 res.setCausalityResult(parseCodeableConcept(getJObject(json, "causalityResult"))); 2147 } 2148 2149 protected AllergyIntolerance parseAllergyIntolerance(JsonObject json) throws IOException, FHIRFormatError { 2150 AllergyIntolerance res = new AllergyIntolerance(); 2151 parseAllergyIntoleranceProperties(json, res); 2152 return res; 2153 } 2154 2155 protected void parseAllergyIntoleranceProperties(JsonObject json, AllergyIntolerance res) throws IOException, FHIRFormatError { 2156 parseDomainResourceProperties(json, res); 2157 if (json.has("identifier")) { 2158 JsonArray array = json.getAsJsonArray("identifier"); 2159 for (int i = 0; i < array.size(); i++) { 2160 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 2161 } 2162 }; 2163 if (json.has("clinicalStatus")) 2164 res.setClinicalStatusElement(parseEnumeration(json.get("clinicalStatus").getAsString(), AllergyIntolerance.AllergyIntoleranceClinicalStatus.NULL, new AllergyIntolerance.AllergyIntoleranceClinicalStatusEnumFactory())); 2165 if (json.has("_clinicalStatus")) 2166 parseElementProperties(getJObject(json, "_clinicalStatus"), res.getClinicalStatusElement()); 2167 if (json.has("verificationStatus")) 2168 res.setVerificationStatusElement(parseEnumeration(json.get("verificationStatus").getAsString(), AllergyIntolerance.AllergyIntoleranceVerificationStatus.NULL, new AllergyIntolerance.AllergyIntoleranceVerificationStatusEnumFactory())); 2169 if (json.has("_verificationStatus")) 2170 parseElementProperties(getJObject(json, "_verificationStatus"), res.getVerificationStatusElement()); 2171 if (json.has("type")) 2172 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), AllergyIntolerance.AllergyIntoleranceType.NULL, new AllergyIntolerance.AllergyIntoleranceTypeEnumFactory())); 2173 if (json.has("_type")) 2174 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 2175 if (json.has("category")) { 2176 JsonArray array = json.getAsJsonArray("category"); 2177 for (int i = 0; i < array.size(); i++) { 2178 if (array.get(i).isJsonNull()) { 2179 res.getCategory().add(new Enumeration<AllergyIntolerance.AllergyIntoleranceCategory>()); 2180 } else { 2181 res.getCategory().add(parseEnumeration(array.get(i).getAsString(), AllergyIntolerance.AllergyIntoleranceCategory.NULL, new AllergyIntolerance.AllergyIntoleranceCategoryEnumFactory())); 2182 } 2183 } 2184 }; 2185 if (json.has("_category")) { 2186 JsonArray array = json.getAsJsonArray("_category"); 2187 for (int i = 0; i < array.size(); i++) { 2188 if (i == res.getCategory().size()) 2189 res.getCategory().add(parseEnumeration(null, AllergyIntolerance.AllergyIntoleranceCategory.NULL, new AllergyIntolerance.AllergyIntoleranceCategoryEnumFactory())); 2190 if (array.get(i) instanceof JsonObject) 2191 parseElementProperties(array.get(i).getAsJsonObject(), res.getCategory().get(i)); 2192 } 2193 }; 2194 if (json.has("criticality")) 2195 res.setCriticalityElement(parseEnumeration(json.get("criticality").getAsString(), AllergyIntolerance.AllergyIntoleranceCriticality.NULL, new AllergyIntolerance.AllergyIntoleranceCriticalityEnumFactory())); 2196 if (json.has("_criticality")) 2197 parseElementProperties(getJObject(json, "_criticality"), res.getCriticalityElement()); 2198 if (json.has("code")) 2199 res.setCode(parseCodeableConcept(getJObject(json, "code"))); 2200 if (json.has("patient")) 2201 res.setPatient(parseReference(getJObject(json, "patient"))); 2202 Type onset = parseType("onset", json); 2203 if (onset != null) 2204 res.setOnset(onset); 2205 if (json.has("assertedDate")) 2206 res.setAssertedDateElement(parseDateTime(json.get("assertedDate").getAsString())); 2207 if (json.has("_assertedDate")) 2208 parseElementProperties(getJObject(json, "_assertedDate"), res.getAssertedDateElement()); 2209 if (json.has("recorder")) 2210 res.setRecorder(parseReference(getJObject(json, "recorder"))); 2211 if (json.has("asserter")) 2212 res.setAsserter(parseReference(getJObject(json, "asserter"))); 2213 if (json.has("lastOccurrence")) 2214 res.setLastOccurrenceElement(parseDateTime(json.get("lastOccurrence").getAsString())); 2215 if (json.has("_lastOccurrence")) 2216 parseElementProperties(getJObject(json, "_lastOccurrence"), res.getLastOccurrenceElement()); 2217 if (json.has("note")) { 2218 JsonArray array = json.getAsJsonArray("note"); 2219 for (int i = 0; i < array.size(); i++) { 2220 res.getNote().add(parseAnnotation(array.get(i).getAsJsonObject())); 2221 } 2222 }; 2223 if (json.has("reaction")) { 2224 JsonArray array = json.getAsJsonArray("reaction"); 2225 for (int i = 0; i < array.size(); i++) { 2226 res.getReaction().add(parseAllergyIntoleranceAllergyIntoleranceReactionComponent(array.get(i).getAsJsonObject(), res)); 2227 } 2228 }; 2229 } 2230 2231 protected AllergyIntolerance.AllergyIntoleranceReactionComponent parseAllergyIntoleranceAllergyIntoleranceReactionComponent(JsonObject json, AllergyIntolerance owner) throws IOException, FHIRFormatError { 2232 AllergyIntolerance.AllergyIntoleranceReactionComponent res = new AllergyIntolerance.AllergyIntoleranceReactionComponent(); 2233 parseAllergyIntoleranceAllergyIntoleranceReactionComponentProperties(json, owner, res); 2234 return res; 2235 } 2236 2237 protected void parseAllergyIntoleranceAllergyIntoleranceReactionComponentProperties(JsonObject json, AllergyIntolerance owner, AllergyIntolerance.AllergyIntoleranceReactionComponent res) throws IOException, FHIRFormatError { 2238 parseBackboneProperties(json, res); 2239 if (json.has("substance")) 2240 res.setSubstance(parseCodeableConcept(getJObject(json, "substance"))); 2241 if (json.has("manifestation")) { 2242 JsonArray array = json.getAsJsonArray("manifestation"); 2243 for (int i = 0; i < array.size(); i++) { 2244 res.getManifestation().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 2245 } 2246 }; 2247 if (json.has("description")) 2248 res.setDescriptionElement(parseString(json.get("description").getAsString())); 2249 if (json.has("_description")) 2250 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 2251 if (json.has("onset")) 2252 res.setOnsetElement(parseDateTime(json.get("onset").getAsString())); 2253 if (json.has("_onset")) 2254 parseElementProperties(getJObject(json, "_onset"), res.getOnsetElement()); 2255 if (json.has("severity")) 2256 res.setSeverityElement(parseEnumeration(json.get("severity").getAsString(), AllergyIntolerance.AllergyIntoleranceSeverity.NULL, new AllergyIntolerance.AllergyIntoleranceSeverityEnumFactory())); 2257 if (json.has("_severity")) 2258 parseElementProperties(getJObject(json, "_severity"), res.getSeverityElement()); 2259 if (json.has("exposureRoute")) 2260 res.setExposureRoute(parseCodeableConcept(getJObject(json, "exposureRoute"))); 2261 if (json.has("note")) { 2262 JsonArray array = json.getAsJsonArray("note"); 2263 for (int i = 0; i < array.size(); i++) { 2264 res.getNote().add(parseAnnotation(array.get(i).getAsJsonObject())); 2265 } 2266 }; 2267 } 2268 2269 protected Appointment parseAppointment(JsonObject json) throws IOException, FHIRFormatError { 2270 Appointment res = new Appointment(); 2271 parseAppointmentProperties(json, res); 2272 return res; 2273 } 2274 2275 protected void parseAppointmentProperties(JsonObject json, Appointment res) throws IOException, FHIRFormatError { 2276 parseDomainResourceProperties(json, res); 2277 if (json.has("identifier")) { 2278 JsonArray array = json.getAsJsonArray("identifier"); 2279 for (int i = 0; i < array.size(); i++) { 2280 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 2281 } 2282 }; 2283 if (json.has("status")) 2284 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Appointment.AppointmentStatus.NULL, new Appointment.AppointmentStatusEnumFactory())); 2285 if (json.has("_status")) 2286 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 2287 if (json.has("serviceCategory")) 2288 res.setServiceCategory(parseCodeableConcept(getJObject(json, "serviceCategory"))); 2289 if (json.has("serviceType")) { 2290 JsonArray array = json.getAsJsonArray("serviceType"); 2291 for (int i = 0; i < array.size(); i++) { 2292 res.getServiceType().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 2293 } 2294 }; 2295 if (json.has("specialty")) { 2296 JsonArray array = json.getAsJsonArray("specialty"); 2297 for (int i = 0; i < array.size(); i++) { 2298 res.getSpecialty().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 2299 } 2300 }; 2301 if (json.has("appointmentType")) 2302 res.setAppointmentType(parseCodeableConcept(getJObject(json, "appointmentType"))); 2303 if (json.has("reason")) { 2304 JsonArray array = json.getAsJsonArray("reason"); 2305 for (int i = 0; i < array.size(); i++) { 2306 res.getReason().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 2307 } 2308 }; 2309 if (json.has("indication")) { 2310 JsonArray array = json.getAsJsonArray("indication"); 2311 for (int i = 0; i < array.size(); i++) { 2312 res.getIndication().add(parseReference(array.get(i).getAsJsonObject())); 2313 } 2314 }; 2315 if (json.has("priority")) 2316 res.setPriorityElement(parseUnsignedInt(json.get("priority").getAsString())); 2317 if (json.has("_priority")) 2318 parseElementProperties(getJObject(json, "_priority"), res.getPriorityElement()); 2319 if (json.has("description")) 2320 res.setDescriptionElement(parseString(json.get("description").getAsString())); 2321 if (json.has("_description")) 2322 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 2323 if (json.has("supportingInformation")) { 2324 JsonArray array = json.getAsJsonArray("supportingInformation"); 2325 for (int i = 0; i < array.size(); i++) { 2326 res.getSupportingInformation().add(parseReference(array.get(i).getAsJsonObject())); 2327 } 2328 }; 2329 if (json.has("start")) 2330 res.setStartElement(parseInstant(json.get("start").getAsString())); 2331 if (json.has("_start")) 2332 parseElementProperties(getJObject(json, "_start"), res.getStartElement()); 2333 if (json.has("end")) 2334 res.setEndElement(parseInstant(json.get("end").getAsString())); 2335 if (json.has("_end")) 2336 parseElementProperties(getJObject(json, "_end"), res.getEndElement()); 2337 if (json.has("minutesDuration")) 2338 res.setMinutesDurationElement(parsePositiveInt(json.get("minutesDuration").getAsString())); 2339 if (json.has("_minutesDuration")) 2340 parseElementProperties(getJObject(json, "_minutesDuration"), res.getMinutesDurationElement()); 2341 if (json.has("slot")) { 2342 JsonArray array = json.getAsJsonArray("slot"); 2343 for (int i = 0; i < array.size(); i++) { 2344 res.getSlot().add(parseReference(array.get(i).getAsJsonObject())); 2345 } 2346 }; 2347 if (json.has("created")) 2348 res.setCreatedElement(parseDateTime(json.get("created").getAsString())); 2349 if (json.has("_created")) 2350 parseElementProperties(getJObject(json, "_created"), res.getCreatedElement()); 2351 if (json.has("comment")) 2352 res.setCommentElement(parseString(json.get("comment").getAsString())); 2353 if (json.has("_comment")) 2354 parseElementProperties(getJObject(json, "_comment"), res.getCommentElement()); 2355 if (json.has("incomingReferral")) { 2356 JsonArray array = json.getAsJsonArray("incomingReferral"); 2357 for (int i = 0; i < array.size(); i++) { 2358 res.getIncomingReferral().add(parseReference(array.get(i).getAsJsonObject())); 2359 } 2360 }; 2361 if (json.has("participant")) { 2362 JsonArray array = json.getAsJsonArray("participant"); 2363 for (int i = 0; i < array.size(); i++) { 2364 res.getParticipant().add(parseAppointmentAppointmentParticipantComponent(array.get(i).getAsJsonObject(), res)); 2365 } 2366 }; 2367 if (json.has("requestedPeriod")) { 2368 JsonArray array = json.getAsJsonArray("requestedPeriod"); 2369 for (int i = 0; i < array.size(); i++) { 2370 res.getRequestedPeriod().add(parsePeriod(array.get(i).getAsJsonObject())); 2371 } 2372 }; 2373 } 2374 2375 protected Appointment.AppointmentParticipantComponent parseAppointmentAppointmentParticipantComponent(JsonObject json, Appointment owner) throws IOException, FHIRFormatError { 2376 Appointment.AppointmentParticipantComponent res = new Appointment.AppointmentParticipantComponent(); 2377 parseAppointmentAppointmentParticipantComponentProperties(json, owner, res); 2378 return res; 2379 } 2380 2381 protected void parseAppointmentAppointmentParticipantComponentProperties(JsonObject json, Appointment owner, Appointment.AppointmentParticipantComponent res) throws IOException, FHIRFormatError { 2382 parseBackboneProperties(json, res); 2383 if (json.has("type")) { 2384 JsonArray array = json.getAsJsonArray("type"); 2385 for (int i = 0; i < array.size(); i++) { 2386 res.getType().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 2387 } 2388 }; 2389 if (json.has("actor")) 2390 res.setActor(parseReference(getJObject(json, "actor"))); 2391 if (json.has("required")) 2392 res.setRequiredElement(parseEnumeration(json.get("required").getAsString(), Appointment.ParticipantRequired.NULL, new Appointment.ParticipantRequiredEnumFactory())); 2393 if (json.has("_required")) 2394 parseElementProperties(getJObject(json, "_required"), res.getRequiredElement()); 2395 if (json.has("status")) 2396 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Appointment.ParticipationStatus.NULL, new Appointment.ParticipationStatusEnumFactory())); 2397 if (json.has("_status")) 2398 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 2399 } 2400 2401 protected AppointmentResponse parseAppointmentResponse(JsonObject json) throws IOException, FHIRFormatError { 2402 AppointmentResponse res = new AppointmentResponse(); 2403 parseAppointmentResponseProperties(json, res); 2404 return res; 2405 } 2406 2407 protected void parseAppointmentResponseProperties(JsonObject json, AppointmentResponse res) throws IOException, FHIRFormatError { 2408 parseDomainResourceProperties(json, res); 2409 if (json.has("identifier")) { 2410 JsonArray array = json.getAsJsonArray("identifier"); 2411 for (int i = 0; i < array.size(); i++) { 2412 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 2413 } 2414 }; 2415 if (json.has("appointment")) 2416 res.setAppointment(parseReference(getJObject(json, "appointment"))); 2417 if (json.has("start")) 2418 res.setStartElement(parseInstant(json.get("start").getAsString())); 2419 if (json.has("_start")) 2420 parseElementProperties(getJObject(json, "_start"), res.getStartElement()); 2421 if (json.has("end")) 2422 res.setEndElement(parseInstant(json.get("end").getAsString())); 2423 if (json.has("_end")) 2424 parseElementProperties(getJObject(json, "_end"), res.getEndElement()); 2425 if (json.has("participantType")) { 2426 JsonArray array = json.getAsJsonArray("participantType"); 2427 for (int i = 0; i < array.size(); i++) { 2428 res.getParticipantType().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 2429 } 2430 }; 2431 if (json.has("actor")) 2432 res.setActor(parseReference(getJObject(json, "actor"))); 2433 if (json.has("participantStatus")) 2434 res.setParticipantStatusElement(parseEnumeration(json.get("participantStatus").getAsString(), AppointmentResponse.ParticipantStatus.NULL, new AppointmentResponse.ParticipantStatusEnumFactory())); 2435 if (json.has("_participantStatus")) 2436 parseElementProperties(getJObject(json, "_participantStatus"), res.getParticipantStatusElement()); 2437 if (json.has("comment")) 2438 res.setCommentElement(parseString(json.get("comment").getAsString())); 2439 if (json.has("_comment")) 2440 parseElementProperties(getJObject(json, "_comment"), res.getCommentElement()); 2441 } 2442 2443 protected AuditEvent parseAuditEvent(JsonObject json) throws IOException, FHIRFormatError { 2444 AuditEvent res = new AuditEvent(); 2445 parseAuditEventProperties(json, res); 2446 return res; 2447 } 2448 2449 protected void parseAuditEventProperties(JsonObject json, AuditEvent res) throws IOException, FHIRFormatError { 2450 parseDomainResourceProperties(json, res); 2451 if (json.has("type")) 2452 res.setType(parseCoding(getJObject(json, "type"))); 2453 if (json.has("subtype")) { 2454 JsonArray array = json.getAsJsonArray("subtype"); 2455 for (int i = 0; i < array.size(); i++) { 2456 res.getSubtype().add(parseCoding(array.get(i).getAsJsonObject())); 2457 } 2458 }; 2459 if (json.has("action")) 2460 res.setActionElement(parseEnumeration(json.get("action").getAsString(), AuditEvent.AuditEventAction.NULL, new AuditEvent.AuditEventActionEnumFactory())); 2461 if (json.has("_action")) 2462 parseElementProperties(getJObject(json, "_action"), res.getActionElement()); 2463 if (json.has("recorded")) 2464 res.setRecordedElement(parseInstant(json.get("recorded").getAsString())); 2465 if (json.has("_recorded")) 2466 parseElementProperties(getJObject(json, "_recorded"), res.getRecordedElement()); 2467 if (json.has("outcome")) 2468 res.setOutcomeElement(parseEnumeration(json.get("outcome").getAsString(), AuditEvent.AuditEventOutcome.NULL, new AuditEvent.AuditEventOutcomeEnumFactory())); 2469 if (json.has("_outcome")) 2470 parseElementProperties(getJObject(json, "_outcome"), res.getOutcomeElement()); 2471 if (json.has("outcomeDesc")) 2472 res.setOutcomeDescElement(parseString(json.get("outcomeDesc").getAsString())); 2473 if (json.has("_outcomeDesc")) 2474 parseElementProperties(getJObject(json, "_outcomeDesc"), res.getOutcomeDescElement()); 2475 if (json.has("purposeOfEvent")) { 2476 JsonArray array = json.getAsJsonArray("purposeOfEvent"); 2477 for (int i = 0; i < array.size(); i++) { 2478 res.getPurposeOfEvent().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 2479 } 2480 }; 2481 if (json.has("agent")) { 2482 JsonArray array = json.getAsJsonArray("agent"); 2483 for (int i = 0; i < array.size(); i++) { 2484 res.getAgent().add(parseAuditEventAuditEventAgentComponent(array.get(i).getAsJsonObject(), res)); 2485 } 2486 }; 2487 if (json.has("source")) 2488 res.setSource(parseAuditEventAuditEventSourceComponent(getJObject(json, "source"), res)); 2489 if (json.has("entity")) { 2490 JsonArray array = json.getAsJsonArray("entity"); 2491 for (int i = 0; i < array.size(); i++) { 2492 res.getEntity().add(parseAuditEventAuditEventEntityComponent(array.get(i).getAsJsonObject(), res)); 2493 } 2494 }; 2495 } 2496 2497 protected AuditEvent.AuditEventAgentComponent parseAuditEventAuditEventAgentComponent(JsonObject json, AuditEvent owner) throws IOException, FHIRFormatError { 2498 AuditEvent.AuditEventAgentComponent res = new AuditEvent.AuditEventAgentComponent(); 2499 parseAuditEventAuditEventAgentComponentProperties(json, owner, res); 2500 return res; 2501 } 2502 2503 protected void parseAuditEventAuditEventAgentComponentProperties(JsonObject json, AuditEvent owner, AuditEvent.AuditEventAgentComponent res) throws IOException, FHIRFormatError { 2504 parseBackboneProperties(json, res); 2505 if (json.has("role")) { 2506 JsonArray array = json.getAsJsonArray("role"); 2507 for (int i = 0; i < array.size(); i++) { 2508 res.getRole().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 2509 } 2510 }; 2511 if (json.has("reference")) 2512 res.setReference(parseReference(getJObject(json, "reference"))); 2513 if (json.has("userId")) 2514 res.setUserId(parseIdentifier(getJObject(json, "userId"))); 2515 if (json.has("altId")) 2516 res.setAltIdElement(parseString(json.get("altId").getAsString())); 2517 if (json.has("_altId")) 2518 parseElementProperties(getJObject(json, "_altId"), res.getAltIdElement()); 2519 if (json.has("name")) 2520 res.setNameElement(parseString(json.get("name").getAsString())); 2521 if (json.has("_name")) 2522 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 2523 if (json.has("requestor")) 2524 res.setRequestorElement(parseBoolean(json.get("requestor").getAsBoolean())); 2525 if (json.has("_requestor")) 2526 parseElementProperties(getJObject(json, "_requestor"), res.getRequestorElement()); 2527 if (json.has("location")) 2528 res.setLocation(parseReference(getJObject(json, "location"))); 2529 if (json.has("policy")) { 2530 JsonArray array = json.getAsJsonArray("policy"); 2531 for (int i = 0; i < array.size(); i++) { 2532 if (array.get(i).isJsonNull()) { 2533 res.getPolicy().add(new UriType()); 2534 } else { 2535 res.getPolicy().add(parseUri(array.get(i).getAsString())); 2536 } 2537 } 2538 }; 2539 if (json.has("_policy")) { 2540 JsonArray array = json.getAsJsonArray("_policy"); 2541 for (int i = 0; i < array.size(); i++) { 2542 if (i == res.getPolicy().size()) 2543 res.getPolicy().add(parseUri(null)); 2544 if (array.get(i) instanceof JsonObject) 2545 parseElementProperties(array.get(i).getAsJsonObject(), res.getPolicy().get(i)); 2546 } 2547 }; 2548 if (json.has("media")) 2549 res.setMedia(parseCoding(getJObject(json, "media"))); 2550 if (json.has("network")) 2551 res.setNetwork(parseAuditEventAuditEventAgentNetworkComponent(getJObject(json, "network"), owner)); 2552 if (json.has("purposeOfUse")) { 2553 JsonArray array = json.getAsJsonArray("purposeOfUse"); 2554 for (int i = 0; i < array.size(); i++) { 2555 res.getPurposeOfUse().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 2556 } 2557 }; 2558 } 2559 2560 protected AuditEvent.AuditEventAgentNetworkComponent parseAuditEventAuditEventAgentNetworkComponent(JsonObject json, AuditEvent owner) throws IOException, FHIRFormatError { 2561 AuditEvent.AuditEventAgentNetworkComponent res = new AuditEvent.AuditEventAgentNetworkComponent(); 2562 parseAuditEventAuditEventAgentNetworkComponentProperties(json, owner, res); 2563 return res; 2564 } 2565 2566 protected void parseAuditEventAuditEventAgentNetworkComponentProperties(JsonObject json, AuditEvent owner, AuditEvent.AuditEventAgentNetworkComponent res) throws IOException, FHIRFormatError { 2567 parseBackboneProperties(json, res); 2568 if (json.has("address")) 2569 res.setAddressElement(parseString(json.get("address").getAsString())); 2570 if (json.has("_address")) 2571 parseElementProperties(getJObject(json, "_address"), res.getAddressElement()); 2572 if (json.has("type")) 2573 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), AuditEvent.AuditEventAgentNetworkType.NULL, new AuditEvent.AuditEventAgentNetworkTypeEnumFactory())); 2574 if (json.has("_type")) 2575 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 2576 } 2577 2578 protected AuditEvent.AuditEventSourceComponent parseAuditEventAuditEventSourceComponent(JsonObject json, AuditEvent owner) throws IOException, FHIRFormatError { 2579 AuditEvent.AuditEventSourceComponent res = new AuditEvent.AuditEventSourceComponent(); 2580 parseAuditEventAuditEventSourceComponentProperties(json, owner, res); 2581 return res; 2582 } 2583 2584 protected void parseAuditEventAuditEventSourceComponentProperties(JsonObject json, AuditEvent owner, AuditEvent.AuditEventSourceComponent res) throws IOException, FHIRFormatError { 2585 parseBackboneProperties(json, res); 2586 if (json.has("site")) 2587 res.setSiteElement(parseString(json.get("site").getAsString())); 2588 if (json.has("_site")) 2589 parseElementProperties(getJObject(json, "_site"), res.getSiteElement()); 2590 if (json.has("identifier")) 2591 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 2592 if (json.has("type")) { 2593 JsonArray array = json.getAsJsonArray("type"); 2594 for (int i = 0; i < array.size(); i++) { 2595 res.getType().add(parseCoding(array.get(i).getAsJsonObject())); 2596 } 2597 }; 2598 } 2599 2600 protected AuditEvent.AuditEventEntityComponent parseAuditEventAuditEventEntityComponent(JsonObject json, AuditEvent owner) throws IOException, FHIRFormatError { 2601 AuditEvent.AuditEventEntityComponent res = new AuditEvent.AuditEventEntityComponent(); 2602 parseAuditEventAuditEventEntityComponentProperties(json, owner, res); 2603 return res; 2604 } 2605 2606 protected void parseAuditEventAuditEventEntityComponentProperties(JsonObject json, AuditEvent owner, AuditEvent.AuditEventEntityComponent res) throws IOException, FHIRFormatError { 2607 parseBackboneProperties(json, res); 2608 if (json.has("identifier")) 2609 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 2610 if (json.has("reference")) 2611 res.setReference(parseReference(getJObject(json, "reference"))); 2612 if (json.has("type")) 2613 res.setType(parseCoding(getJObject(json, "type"))); 2614 if (json.has("role")) 2615 res.setRole(parseCoding(getJObject(json, "role"))); 2616 if (json.has("lifecycle")) 2617 res.setLifecycle(parseCoding(getJObject(json, "lifecycle"))); 2618 if (json.has("securityLabel")) { 2619 JsonArray array = json.getAsJsonArray("securityLabel"); 2620 for (int i = 0; i < array.size(); i++) { 2621 res.getSecurityLabel().add(parseCoding(array.get(i).getAsJsonObject())); 2622 } 2623 }; 2624 if (json.has("name")) 2625 res.setNameElement(parseString(json.get("name").getAsString())); 2626 if (json.has("_name")) 2627 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 2628 if (json.has("description")) 2629 res.setDescriptionElement(parseString(json.get("description").getAsString())); 2630 if (json.has("_description")) 2631 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 2632 if (json.has("query")) 2633 res.setQueryElement(parseBase64Binary(json.get("query").getAsString())); 2634 if (json.has("_query")) 2635 parseElementProperties(getJObject(json, "_query"), res.getQueryElement()); 2636 if (json.has("detail")) { 2637 JsonArray array = json.getAsJsonArray("detail"); 2638 for (int i = 0; i < array.size(); i++) { 2639 res.getDetail().add(parseAuditEventAuditEventEntityDetailComponent(array.get(i).getAsJsonObject(), owner)); 2640 } 2641 }; 2642 } 2643 2644 protected AuditEvent.AuditEventEntityDetailComponent parseAuditEventAuditEventEntityDetailComponent(JsonObject json, AuditEvent owner) throws IOException, FHIRFormatError { 2645 AuditEvent.AuditEventEntityDetailComponent res = new AuditEvent.AuditEventEntityDetailComponent(); 2646 parseAuditEventAuditEventEntityDetailComponentProperties(json, owner, res); 2647 return res; 2648 } 2649 2650 protected void parseAuditEventAuditEventEntityDetailComponentProperties(JsonObject json, AuditEvent owner, AuditEvent.AuditEventEntityDetailComponent res) throws IOException, FHIRFormatError { 2651 parseBackboneProperties(json, res); 2652 if (json.has("type")) 2653 res.setTypeElement(parseString(json.get("type").getAsString())); 2654 if (json.has("_type")) 2655 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 2656 if (json.has("value")) 2657 res.setValueElement(parseBase64Binary(json.get("value").getAsString())); 2658 if (json.has("_value")) 2659 parseElementProperties(getJObject(json, "_value"), res.getValueElement()); 2660 } 2661 2662 protected Basic parseBasic(JsonObject json) throws IOException, FHIRFormatError { 2663 Basic res = new Basic(); 2664 parseBasicProperties(json, res); 2665 return res; 2666 } 2667 2668 protected void parseBasicProperties(JsonObject json, Basic res) throws IOException, FHIRFormatError { 2669 parseDomainResourceProperties(json, res); 2670 if (json.has("identifier")) { 2671 JsonArray array = json.getAsJsonArray("identifier"); 2672 for (int i = 0; i < array.size(); i++) { 2673 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 2674 } 2675 }; 2676 if (json.has("code")) 2677 res.setCode(parseCodeableConcept(getJObject(json, "code"))); 2678 if (json.has("subject")) 2679 res.setSubject(parseReference(getJObject(json, "subject"))); 2680 if (json.has("created")) 2681 res.setCreatedElement(parseDate(json.get("created").getAsString())); 2682 if (json.has("_created")) 2683 parseElementProperties(getJObject(json, "_created"), res.getCreatedElement()); 2684 if (json.has("author")) 2685 res.setAuthor(parseReference(getJObject(json, "author"))); 2686 } 2687 2688 protected Binary parseBinary(JsonObject json) throws IOException, FHIRFormatError { 2689 Binary res = new Binary(); 2690 parseBinaryProperties(json, res); 2691 return res; 2692 } 2693 2694 protected void parseBinaryProperties(JsonObject json, Binary res) throws IOException, FHIRFormatError { 2695 parseResourceProperties(json, res); 2696 if (json.has("contentType")) 2697 res.setContentTypeElement(parseCode(json.get("contentType").getAsString())); 2698 if (json.has("_contentType")) 2699 parseElementProperties(getJObject(json, "_contentType"), res.getContentTypeElement()); 2700 if (json.has("securityContext")) 2701 res.setSecurityContext(parseReference(getJObject(json, "securityContext"))); 2702 if (json.has("content")) 2703 res.setContentElement(parseBase64Binary(json.get("content").getAsString())); 2704 if (json.has("_content")) 2705 parseElementProperties(getJObject(json, "_content"), res.getContentElement()); 2706 } 2707 2708 protected BodySite parseBodySite(JsonObject json) throws IOException, FHIRFormatError { 2709 BodySite res = new BodySite(); 2710 parseBodySiteProperties(json, res); 2711 return res; 2712 } 2713 2714 protected void parseBodySiteProperties(JsonObject json, BodySite res) throws IOException, FHIRFormatError { 2715 parseDomainResourceProperties(json, res); 2716 if (json.has("identifier")) { 2717 JsonArray array = json.getAsJsonArray("identifier"); 2718 for (int i = 0; i < array.size(); i++) { 2719 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 2720 } 2721 }; 2722 if (json.has("active")) 2723 res.setActiveElement(parseBoolean(json.get("active").getAsBoolean())); 2724 if (json.has("_active")) 2725 parseElementProperties(getJObject(json, "_active"), res.getActiveElement()); 2726 if (json.has("code")) 2727 res.setCode(parseCodeableConcept(getJObject(json, "code"))); 2728 if (json.has("qualifier")) { 2729 JsonArray array = json.getAsJsonArray("qualifier"); 2730 for (int i = 0; i < array.size(); i++) { 2731 res.getQualifier().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 2732 } 2733 }; 2734 if (json.has("description")) 2735 res.setDescriptionElement(parseString(json.get("description").getAsString())); 2736 if (json.has("_description")) 2737 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 2738 if (json.has("image")) { 2739 JsonArray array = json.getAsJsonArray("image"); 2740 for (int i = 0; i < array.size(); i++) { 2741 res.getImage().add(parseAttachment(array.get(i).getAsJsonObject())); 2742 } 2743 }; 2744 if (json.has("patient")) 2745 res.setPatient(parseReference(getJObject(json, "patient"))); 2746 } 2747 2748 protected Bundle parseBundle(JsonObject json) throws IOException, FHIRFormatError { 2749 Bundle res = new Bundle(); 2750 parseBundleProperties(json, res); 2751 return res; 2752 } 2753 2754 protected void parseBundleProperties(JsonObject json, Bundle res) throws IOException, FHIRFormatError { 2755 parseResourceProperties(json, res); 2756 if (json.has("identifier")) 2757 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 2758 if (json.has("type")) 2759 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), Bundle.BundleType.NULL, new Bundle.BundleTypeEnumFactory())); 2760 if (json.has("_type")) 2761 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 2762 if (json.has("total")) 2763 res.setTotalElement(parseUnsignedInt(json.get("total").getAsString())); 2764 if (json.has("_total")) 2765 parseElementProperties(getJObject(json, "_total"), res.getTotalElement()); 2766 if (json.has("link")) { 2767 JsonArray array = json.getAsJsonArray("link"); 2768 for (int i = 0; i < array.size(); i++) { 2769 res.getLink().add(parseBundleBundleLinkComponent(array.get(i).getAsJsonObject(), res)); 2770 } 2771 }; 2772 if (json.has("entry")) { 2773 JsonArray array = json.getAsJsonArray("entry"); 2774 for (int i = 0; i < array.size(); i++) { 2775 res.getEntry().add(parseBundleBundleEntryComponent(array.get(i).getAsJsonObject(), res)); 2776 } 2777 }; 2778 if (json.has("signature")) 2779 res.setSignature(parseSignature(getJObject(json, "signature"))); 2780 } 2781 2782 protected Bundle.BundleLinkComponent parseBundleBundleLinkComponent(JsonObject json, Bundle owner) throws IOException, FHIRFormatError { 2783 Bundle.BundleLinkComponent res = new Bundle.BundleLinkComponent(); 2784 parseBundleBundleLinkComponentProperties(json, owner, res); 2785 return res; 2786 } 2787 2788 protected void parseBundleBundleLinkComponentProperties(JsonObject json, Bundle owner, Bundle.BundleLinkComponent res) throws IOException, FHIRFormatError { 2789 parseBackboneProperties(json, res); 2790 if (json.has("relation")) 2791 res.setRelationElement(parseString(json.get("relation").getAsString())); 2792 if (json.has("_relation")) 2793 parseElementProperties(getJObject(json, "_relation"), res.getRelationElement()); 2794 if (json.has("url")) 2795 res.setUrlElement(parseUri(json.get("url").getAsString())); 2796 if (json.has("_url")) 2797 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 2798 } 2799 2800 protected Bundle.BundleEntryComponent parseBundleBundleEntryComponent(JsonObject json, Bundle owner) throws IOException, FHIRFormatError { 2801 Bundle.BundleEntryComponent res = new Bundle.BundleEntryComponent(); 2802 parseBundleBundleEntryComponentProperties(json, owner, res); 2803 return res; 2804 } 2805 2806 protected void parseBundleBundleEntryComponentProperties(JsonObject json, Bundle owner, Bundle.BundleEntryComponent res) throws IOException, FHIRFormatError { 2807 parseBackboneProperties(json, res); 2808 if (json.has("link")) { 2809 JsonArray array = json.getAsJsonArray("link"); 2810 for (int i = 0; i < array.size(); i++) { 2811 res.getLink().add(parseBundleBundleLinkComponent(array.get(i).getAsJsonObject(), owner)); 2812 } 2813 }; 2814 if (json.has("fullUrl")) 2815 res.setFullUrlElement(parseUri(json.get("fullUrl").getAsString())); 2816 if (json.has("_fullUrl")) 2817 parseElementProperties(getJObject(json, "_fullUrl"), res.getFullUrlElement()); 2818 if (json.has("resource")) 2819 res.setResource(parseResource(getJObject(json, "resource"))); 2820 if (json.has("search")) 2821 res.setSearch(parseBundleBundleEntrySearchComponent(getJObject(json, "search"), owner)); 2822 if (json.has("request")) 2823 res.setRequest(parseBundleBundleEntryRequestComponent(getJObject(json, "request"), owner)); 2824 if (json.has("response")) 2825 res.setResponse(parseBundleBundleEntryResponseComponent(getJObject(json, "response"), owner)); 2826 } 2827 2828 protected Bundle.BundleEntrySearchComponent parseBundleBundleEntrySearchComponent(JsonObject json, Bundle owner) throws IOException, FHIRFormatError { 2829 Bundle.BundleEntrySearchComponent res = new Bundle.BundleEntrySearchComponent(); 2830 parseBundleBundleEntrySearchComponentProperties(json, owner, res); 2831 return res; 2832 } 2833 2834 protected void parseBundleBundleEntrySearchComponentProperties(JsonObject json, Bundle owner, Bundle.BundleEntrySearchComponent res) throws IOException, FHIRFormatError { 2835 parseBackboneProperties(json, res); 2836 if (json.has("mode")) 2837 res.setModeElement(parseEnumeration(json.get("mode").getAsString(), Bundle.SearchEntryMode.NULL, new Bundle.SearchEntryModeEnumFactory())); 2838 if (json.has("_mode")) 2839 parseElementProperties(getJObject(json, "_mode"), res.getModeElement()); 2840 if (json.has("score")) 2841 res.setScoreElement(parseDecimal(json.get("score").getAsBigDecimal())); 2842 if (json.has("_score")) 2843 parseElementProperties(getJObject(json, "_score"), res.getScoreElement()); 2844 } 2845 2846 protected Bundle.BundleEntryRequestComponent parseBundleBundleEntryRequestComponent(JsonObject json, Bundle owner) throws IOException, FHIRFormatError { 2847 Bundle.BundleEntryRequestComponent res = new Bundle.BundleEntryRequestComponent(); 2848 parseBundleBundleEntryRequestComponentProperties(json, owner, res); 2849 return res; 2850 } 2851 2852 protected void parseBundleBundleEntryRequestComponentProperties(JsonObject json, Bundle owner, Bundle.BundleEntryRequestComponent res) throws IOException, FHIRFormatError { 2853 parseBackboneProperties(json, res); 2854 if (json.has("method")) 2855 res.setMethodElement(parseEnumeration(json.get("method").getAsString(), Bundle.HTTPVerb.NULL, new Bundle.HTTPVerbEnumFactory())); 2856 if (json.has("_method")) 2857 parseElementProperties(getJObject(json, "_method"), res.getMethodElement()); 2858 if (json.has("url")) 2859 res.setUrlElement(parseUri(json.get("url").getAsString())); 2860 if (json.has("_url")) 2861 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 2862 if (json.has("ifNoneMatch")) 2863 res.setIfNoneMatchElement(parseString(json.get("ifNoneMatch").getAsString())); 2864 if (json.has("_ifNoneMatch")) 2865 parseElementProperties(getJObject(json, "_ifNoneMatch"), res.getIfNoneMatchElement()); 2866 if (json.has("ifModifiedSince")) 2867 res.setIfModifiedSinceElement(parseInstant(json.get("ifModifiedSince").getAsString())); 2868 if (json.has("_ifModifiedSince")) 2869 parseElementProperties(getJObject(json, "_ifModifiedSince"), res.getIfModifiedSinceElement()); 2870 if (json.has("ifMatch")) 2871 res.setIfMatchElement(parseString(json.get("ifMatch").getAsString())); 2872 if (json.has("_ifMatch")) 2873 parseElementProperties(getJObject(json, "_ifMatch"), res.getIfMatchElement()); 2874 if (json.has("ifNoneExist")) 2875 res.setIfNoneExistElement(parseString(json.get("ifNoneExist").getAsString())); 2876 if (json.has("_ifNoneExist")) 2877 parseElementProperties(getJObject(json, "_ifNoneExist"), res.getIfNoneExistElement()); 2878 } 2879 2880 protected Bundle.BundleEntryResponseComponent parseBundleBundleEntryResponseComponent(JsonObject json, Bundle owner) throws IOException, FHIRFormatError { 2881 Bundle.BundleEntryResponseComponent res = new Bundle.BundleEntryResponseComponent(); 2882 parseBundleBundleEntryResponseComponentProperties(json, owner, res); 2883 return res; 2884 } 2885 2886 protected void parseBundleBundleEntryResponseComponentProperties(JsonObject json, Bundle owner, Bundle.BundleEntryResponseComponent res) throws IOException, FHIRFormatError { 2887 parseBackboneProperties(json, res); 2888 if (json.has("status")) 2889 res.setStatusElement(parseString(json.get("status").getAsString())); 2890 if (json.has("_status")) 2891 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 2892 if (json.has("location")) 2893 res.setLocationElement(parseUri(json.get("location").getAsString())); 2894 if (json.has("_location")) 2895 parseElementProperties(getJObject(json, "_location"), res.getLocationElement()); 2896 if (json.has("etag")) 2897 res.setEtagElement(parseString(json.get("etag").getAsString())); 2898 if (json.has("_etag")) 2899 parseElementProperties(getJObject(json, "_etag"), res.getEtagElement()); 2900 if (json.has("lastModified")) 2901 res.setLastModifiedElement(parseInstant(json.get("lastModified").getAsString())); 2902 if (json.has("_lastModified")) 2903 parseElementProperties(getJObject(json, "_lastModified"), res.getLastModifiedElement()); 2904 if (json.has("outcome")) 2905 res.setOutcome(parseResource(getJObject(json, "outcome"))); 2906 } 2907 2908 protected CapabilityStatement parseCapabilityStatement(JsonObject json) throws IOException, FHIRFormatError { 2909 CapabilityStatement res = new CapabilityStatement(); 2910 parseCapabilityStatementProperties(json, res); 2911 return res; 2912 } 2913 2914 protected void parseCapabilityStatementProperties(JsonObject json, CapabilityStatement res) throws IOException, FHIRFormatError { 2915 parseDomainResourceProperties(json, res); 2916 if (json.has("url")) 2917 res.setUrlElement(parseUri(json.get("url").getAsString())); 2918 if (json.has("_url")) 2919 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 2920 if (json.has("version")) 2921 res.setVersionElement(parseString(json.get("version").getAsString())); 2922 if (json.has("_version")) 2923 parseElementProperties(getJObject(json, "_version"), res.getVersionElement()); 2924 if (json.has("name")) 2925 res.setNameElement(parseString(json.get("name").getAsString())); 2926 if (json.has("_name")) 2927 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 2928 if (json.has("title")) 2929 res.setTitleElement(parseString(json.get("title").getAsString())); 2930 if (json.has("_title")) 2931 parseElementProperties(getJObject(json, "_title"), res.getTitleElement()); 2932 if (json.has("status")) 2933 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Enumerations.PublicationStatus.NULL, new Enumerations.PublicationStatusEnumFactory())); 2934 if (json.has("_status")) 2935 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 2936 if (json.has("experimental")) 2937 res.setExperimentalElement(parseBoolean(json.get("experimental").getAsBoolean())); 2938 if (json.has("_experimental")) 2939 parseElementProperties(getJObject(json, "_experimental"), res.getExperimentalElement()); 2940 if (json.has("date")) 2941 res.setDateElement(parseDateTime(json.get("date").getAsString())); 2942 if (json.has("_date")) 2943 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 2944 if (json.has("publisher")) 2945 res.setPublisherElement(parseString(json.get("publisher").getAsString())); 2946 if (json.has("_publisher")) 2947 parseElementProperties(getJObject(json, "_publisher"), res.getPublisherElement()); 2948 if (json.has("contact")) { 2949 JsonArray array = json.getAsJsonArray("contact"); 2950 for (int i = 0; i < array.size(); i++) { 2951 res.getContact().add(parseContactDetail(array.get(i).getAsJsonObject())); 2952 } 2953 }; 2954 if (json.has("description")) 2955 res.setDescriptionElement(parseMarkdown(json.get("description").getAsString())); 2956 if (json.has("_description")) 2957 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 2958 if (json.has("useContext")) { 2959 JsonArray array = json.getAsJsonArray("useContext"); 2960 for (int i = 0; i < array.size(); i++) { 2961 res.getUseContext().add(parseUsageContext(array.get(i).getAsJsonObject())); 2962 } 2963 }; 2964 if (json.has("jurisdiction")) { 2965 JsonArray array = json.getAsJsonArray("jurisdiction"); 2966 for (int i = 0; i < array.size(); i++) { 2967 res.getJurisdiction().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 2968 } 2969 }; 2970 if (json.has("purpose")) 2971 res.setPurposeElement(parseMarkdown(json.get("purpose").getAsString())); 2972 if (json.has("_purpose")) 2973 parseElementProperties(getJObject(json, "_purpose"), res.getPurposeElement()); 2974 if (json.has("copyright")) 2975 res.setCopyrightElement(parseMarkdown(json.get("copyright").getAsString())); 2976 if (json.has("_copyright")) 2977 parseElementProperties(getJObject(json, "_copyright"), res.getCopyrightElement()); 2978 if (json.has("kind")) 2979 res.setKindElement(parseEnumeration(json.get("kind").getAsString(), CapabilityStatement.CapabilityStatementKind.NULL, new CapabilityStatement.CapabilityStatementKindEnumFactory())); 2980 if (json.has("_kind")) 2981 parseElementProperties(getJObject(json, "_kind"), res.getKindElement()); 2982 if (json.has("instantiates")) { 2983 JsonArray array = json.getAsJsonArray("instantiates"); 2984 for (int i = 0; i < array.size(); i++) { 2985 if (array.get(i).isJsonNull()) { 2986 res.getInstantiates().add(new UriType()); 2987 } else { 2988 res.getInstantiates().add(parseUri(array.get(i).getAsString())); 2989 } 2990 } 2991 }; 2992 if (json.has("_instantiates")) { 2993 JsonArray array = json.getAsJsonArray("_instantiates"); 2994 for (int i = 0; i < array.size(); i++) { 2995 if (i == res.getInstantiates().size()) 2996 res.getInstantiates().add(parseUri(null)); 2997 if (array.get(i) instanceof JsonObject) 2998 parseElementProperties(array.get(i).getAsJsonObject(), res.getInstantiates().get(i)); 2999 } 3000 }; 3001 if (json.has("software")) 3002 res.setSoftware(parseCapabilityStatementCapabilityStatementSoftwareComponent(getJObject(json, "software"), res)); 3003 if (json.has("implementation")) 3004 res.setImplementation(parseCapabilityStatementCapabilityStatementImplementationComponent(getJObject(json, "implementation"), res)); 3005 if (json.has("fhirVersion")) 3006 res.setFhirVersionElement(parseId(json.get("fhirVersion").getAsString())); 3007 if (json.has("_fhirVersion")) 3008 parseElementProperties(getJObject(json, "_fhirVersion"), res.getFhirVersionElement()); 3009 if (json.has("acceptUnknown")) 3010 res.setAcceptUnknownElement(parseEnumeration(json.get("acceptUnknown").getAsString(), CapabilityStatement.UnknownContentCode.NULL, new CapabilityStatement.UnknownContentCodeEnumFactory())); 3011 if (json.has("_acceptUnknown")) 3012 parseElementProperties(getJObject(json, "_acceptUnknown"), res.getAcceptUnknownElement()); 3013 if (json.has("format")) { 3014 JsonArray array = json.getAsJsonArray("format"); 3015 for (int i = 0; i < array.size(); i++) { 3016 if (array.get(i).isJsonNull()) { 3017 res.getFormat().add(new CodeType()); 3018 } else { 3019 res.getFormat().add(parseCode(array.get(i).getAsString())); 3020 } 3021 } 3022 }; 3023 if (json.has("_format")) { 3024 JsonArray array = json.getAsJsonArray("_format"); 3025 for (int i = 0; i < array.size(); i++) { 3026 if (i == res.getFormat().size()) 3027 res.getFormat().add(parseCode(null)); 3028 if (array.get(i) instanceof JsonObject) 3029 parseElementProperties(array.get(i).getAsJsonObject(), res.getFormat().get(i)); 3030 } 3031 }; 3032 if (json.has("patchFormat")) { 3033 JsonArray array = json.getAsJsonArray("patchFormat"); 3034 for (int i = 0; i < array.size(); i++) { 3035 if (array.get(i).isJsonNull()) { 3036 res.getPatchFormat().add(new CodeType()); 3037 } else { 3038 res.getPatchFormat().add(parseCode(array.get(i).getAsString())); 3039 } 3040 } 3041 }; 3042 if (json.has("_patchFormat")) { 3043 JsonArray array = json.getAsJsonArray("_patchFormat"); 3044 for (int i = 0; i < array.size(); i++) { 3045 if (i == res.getPatchFormat().size()) 3046 res.getPatchFormat().add(parseCode(null)); 3047 if (array.get(i) instanceof JsonObject) 3048 parseElementProperties(array.get(i).getAsJsonObject(), res.getPatchFormat().get(i)); 3049 } 3050 }; 3051 if (json.has("implementationGuide")) { 3052 JsonArray array = json.getAsJsonArray("implementationGuide"); 3053 for (int i = 0; i < array.size(); i++) { 3054 if (array.get(i).isJsonNull()) { 3055 res.getImplementationGuide().add(new UriType()); 3056 } else { 3057 res.getImplementationGuide().add(parseUri(array.get(i).getAsString())); 3058 } 3059 } 3060 }; 3061 if (json.has("_implementationGuide")) { 3062 JsonArray array = json.getAsJsonArray("_implementationGuide"); 3063 for (int i = 0; i < array.size(); i++) { 3064 if (i == res.getImplementationGuide().size()) 3065 res.getImplementationGuide().add(parseUri(null)); 3066 if (array.get(i) instanceof JsonObject) 3067 parseElementProperties(array.get(i).getAsJsonObject(), res.getImplementationGuide().get(i)); 3068 } 3069 }; 3070 if (json.has("profile")) { 3071 JsonArray array = json.getAsJsonArray("profile"); 3072 for (int i = 0; i < array.size(); i++) { 3073 res.getProfile().add(parseReference(array.get(i).getAsJsonObject())); 3074 } 3075 }; 3076 if (json.has("rest")) { 3077 JsonArray array = json.getAsJsonArray("rest"); 3078 for (int i = 0; i < array.size(); i++) { 3079 res.getRest().add(parseCapabilityStatementCapabilityStatementRestComponent(array.get(i).getAsJsonObject(), res)); 3080 } 3081 }; 3082 if (json.has("messaging")) { 3083 JsonArray array = json.getAsJsonArray("messaging"); 3084 for (int i = 0; i < array.size(); i++) { 3085 res.getMessaging().add(parseCapabilityStatementCapabilityStatementMessagingComponent(array.get(i).getAsJsonObject(), res)); 3086 } 3087 }; 3088 if (json.has("document")) { 3089 JsonArray array = json.getAsJsonArray("document"); 3090 for (int i = 0; i < array.size(); i++) { 3091 res.getDocument().add(parseCapabilityStatementCapabilityStatementDocumentComponent(array.get(i).getAsJsonObject(), res)); 3092 } 3093 }; 3094 } 3095 3096 protected CapabilityStatement.CapabilityStatementSoftwareComponent parseCapabilityStatementCapabilityStatementSoftwareComponent(JsonObject json, CapabilityStatement owner) throws IOException, FHIRFormatError { 3097 CapabilityStatement.CapabilityStatementSoftwareComponent res = new CapabilityStatement.CapabilityStatementSoftwareComponent(); 3098 parseCapabilityStatementCapabilityStatementSoftwareComponentProperties(json, owner, res); 3099 return res; 3100 } 3101 3102 protected void parseCapabilityStatementCapabilityStatementSoftwareComponentProperties(JsonObject json, CapabilityStatement owner, CapabilityStatement.CapabilityStatementSoftwareComponent res) throws IOException, FHIRFormatError { 3103 parseBackboneProperties(json, res); 3104 if (json.has("name")) 3105 res.setNameElement(parseString(json.get("name").getAsString())); 3106 if (json.has("_name")) 3107 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 3108 if (json.has("version")) 3109 res.setVersionElement(parseString(json.get("version").getAsString())); 3110 if (json.has("_version")) 3111 parseElementProperties(getJObject(json, "_version"), res.getVersionElement()); 3112 if (json.has("releaseDate")) 3113 res.setReleaseDateElement(parseDateTime(json.get("releaseDate").getAsString())); 3114 if (json.has("_releaseDate")) 3115 parseElementProperties(getJObject(json, "_releaseDate"), res.getReleaseDateElement()); 3116 } 3117 3118 protected CapabilityStatement.CapabilityStatementImplementationComponent parseCapabilityStatementCapabilityStatementImplementationComponent(JsonObject json, CapabilityStatement owner) throws IOException, FHIRFormatError { 3119 CapabilityStatement.CapabilityStatementImplementationComponent res = new CapabilityStatement.CapabilityStatementImplementationComponent(); 3120 parseCapabilityStatementCapabilityStatementImplementationComponentProperties(json, owner, res); 3121 return res; 3122 } 3123 3124 protected void parseCapabilityStatementCapabilityStatementImplementationComponentProperties(JsonObject json, CapabilityStatement owner, CapabilityStatement.CapabilityStatementImplementationComponent res) throws IOException, FHIRFormatError { 3125 parseBackboneProperties(json, res); 3126 if (json.has("description")) 3127 res.setDescriptionElement(parseString(json.get("description").getAsString())); 3128 if (json.has("_description")) 3129 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 3130 if (json.has("url")) 3131 res.setUrlElement(parseUri(json.get("url").getAsString())); 3132 if (json.has("_url")) 3133 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 3134 } 3135 3136 protected CapabilityStatement.CapabilityStatementRestComponent parseCapabilityStatementCapabilityStatementRestComponent(JsonObject json, CapabilityStatement owner) throws IOException, FHIRFormatError { 3137 CapabilityStatement.CapabilityStatementRestComponent res = new CapabilityStatement.CapabilityStatementRestComponent(); 3138 parseCapabilityStatementCapabilityStatementRestComponentProperties(json, owner, res); 3139 return res; 3140 } 3141 3142 protected void parseCapabilityStatementCapabilityStatementRestComponentProperties(JsonObject json, CapabilityStatement owner, CapabilityStatement.CapabilityStatementRestComponent res) throws IOException, FHIRFormatError { 3143 parseBackboneProperties(json, res); 3144 if (json.has("mode")) 3145 res.setModeElement(parseEnumeration(json.get("mode").getAsString(), CapabilityStatement.RestfulCapabilityMode.NULL, new CapabilityStatement.RestfulCapabilityModeEnumFactory())); 3146 if (json.has("_mode")) 3147 parseElementProperties(getJObject(json, "_mode"), res.getModeElement()); 3148 if (json.has("documentation")) 3149 res.setDocumentationElement(parseString(json.get("documentation").getAsString())); 3150 if (json.has("_documentation")) 3151 parseElementProperties(getJObject(json, "_documentation"), res.getDocumentationElement()); 3152 if (json.has("security")) 3153 res.setSecurity(parseCapabilityStatementCapabilityStatementRestSecurityComponent(getJObject(json, "security"), owner)); 3154 if (json.has("resource")) { 3155 JsonArray array = json.getAsJsonArray("resource"); 3156 for (int i = 0; i < array.size(); i++) { 3157 res.getResource().add(parseCapabilityStatementCapabilityStatementRestResourceComponent(array.get(i).getAsJsonObject(), owner)); 3158 } 3159 }; 3160 if (json.has("interaction")) { 3161 JsonArray array = json.getAsJsonArray("interaction"); 3162 for (int i = 0; i < array.size(); i++) { 3163 res.getInteraction().add(parseCapabilityStatementSystemInteractionComponent(array.get(i).getAsJsonObject(), owner)); 3164 } 3165 }; 3166 if (json.has("searchParam")) { 3167 JsonArray array = json.getAsJsonArray("searchParam"); 3168 for (int i = 0; i < array.size(); i++) { 3169 res.getSearchParam().add(parseCapabilityStatementCapabilityStatementRestResourceSearchParamComponent(array.get(i).getAsJsonObject(), owner)); 3170 } 3171 }; 3172 if (json.has("operation")) { 3173 JsonArray array = json.getAsJsonArray("operation"); 3174 for (int i = 0; i < array.size(); i++) { 3175 res.getOperation().add(parseCapabilityStatementCapabilityStatementRestOperationComponent(array.get(i).getAsJsonObject(), owner)); 3176 } 3177 }; 3178 if (json.has("compartment")) { 3179 JsonArray array = json.getAsJsonArray("compartment"); 3180 for (int i = 0; i < array.size(); i++) { 3181 if (array.get(i).isJsonNull()) { 3182 res.getCompartment().add(new UriType()); 3183 } else { 3184 res.getCompartment().add(parseUri(array.get(i).getAsString())); 3185 } 3186 } 3187 }; 3188 if (json.has("_compartment")) { 3189 JsonArray array = json.getAsJsonArray("_compartment"); 3190 for (int i = 0; i < array.size(); i++) { 3191 if (i == res.getCompartment().size()) 3192 res.getCompartment().add(parseUri(null)); 3193 if (array.get(i) instanceof JsonObject) 3194 parseElementProperties(array.get(i).getAsJsonObject(), res.getCompartment().get(i)); 3195 } 3196 }; 3197 } 3198 3199 protected CapabilityStatement.CapabilityStatementRestSecurityComponent parseCapabilityStatementCapabilityStatementRestSecurityComponent(JsonObject json, CapabilityStatement owner) throws IOException, FHIRFormatError { 3200 CapabilityStatement.CapabilityStatementRestSecurityComponent res = new CapabilityStatement.CapabilityStatementRestSecurityComponent(); 3201 parseCapabilityStatementCapabilityStatementRestSecurityComponentProperties(json, owner, res); 3202 return res; 3203 } 3204 3205 protected void parseCapabilityStatementCapabilityStatementRestSecurityComponentProperties(JsonObject json, CapabilityStatement owner, CapabilityStatement.CapabilityStatementRestSecurityComponent res) throws IOException, FHIRFormatError { 3206 parseBackboneProperties(json, res); 3207 if (json.has("cors")) 3208 res.setCorsElement(parseBoolean(json.get("cors").getAsBoolean())); 3209 if (json.has("_cors")) 3210 parseElementProperties(getJObject(json, "_cors"), res.getCorsElement()); 3211 if (json.has("service")) { 3212 JsonArray array = json.getAsJsonArray("service"); 3213 for (int i = 0; i < array.size(); i++) { 3214 res.getService().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 3215 } 3216 }; 3217 if (json.has("description")) 3218 res.setDescriptionElement(parseString(json.get("description").getAsString())); 3219 if (json.has("_description")) 3220 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 3221 if (json.has("certificate")) { 3222 JsonArray array = json.getAsJsonArray("certificate"); 3223 for (int i = 0; i < array.size(); i++) { 3224 res.getCertificate().add(parseCapabilityStatementCapabilityStatementRestSecurityCertificateComponent(array.get(i).getAsJsonObject(), owner)); 3225 } 3226 }; 3227 } 3228 3229 protected CapabilityStatement.CapabilityStatementRestSecurityCertificateComponent parseCapabilityStatementCapabilityStatementRestSecurityCertificateComponent(JsonObject json, CapabilityStatement owner) throws IOException, FHIRFormatError { 3230 CapabilityStatement.CapabilityStatementRestSecurityCertificateComponent res = new CapabilityStatement.CapabilityStatementRestSecurityCertificateComponent(); 3231 parseCapabilityStatementCapabilityStatementRestSecurityCertificateComponentProperties(json, owner, res); 3232 return res; 3233 } 3234 3235 protected void parseCapabilityStatementCapabilityStatementRestSecurityCertificateComponentProperties(JsonObject json, CapabilityStatement owner, CapabilityStatement.CapabilityStatementRestSecurityCertificateComponent res) throws IOException, FHIRFormatError { 3236 parseBackboneProperties(json, res); 3237 if (json.has("type")) 3238 res.setTypeElement(parseCode(json.get("type").getAsString())); 3239 if (json.has("_type")) 3240 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 3241 if (json.has("blob")) 3242 res.setBlobElement(parseBase64Binary(json.get("blob").getAsString())); 3243 if (json.has("_blob")) 3244 parseElementProperties(getJObject(json, "_blob"), res.getBlobElement()); 3245 } 3246 3247 protected CapabilityStatement.CapabilityStatementRestResourceComponent parseCapabilityStatementCapabilityStatementRestResourceComponent(JsonObject json, CapabilityStatement owner) throws IOException, FHIRFormatError { 3248 CapabilityStatement.CapabilityStatementRestResourceComponent res = new CapabilityStatement.CapabilityStatementRestResourceComponent(); 3249 parseCapabilityStatementCapabilityStatementRestResourceComponentProperties(json, owner, res); 3250 return res; 3251 } 3252 3253 protected void parseCapabilityStatementCapabilityStatementRestResourceComponentProperties(JsonObject json, CapabilityStatement owner, CapabilityStatement.CapabilityStatementRestResourceComponent res) throws IOException, FHIRFormatError { 3254 parseBackboneProperties(json, res); 3255 if (json.has("type")) 3256 res.setTypeElement(parseCode(json.get("type").getAsString())); 3257 if (json.has("_type")) 3258 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 3259 if (json.has("profile")) 3260 res.setProfile(parseReference(getJObject(json, "profile"))); 3261 if (json.has("documentation")) 3262 res.setDocumentationElement(parseMarkdown(json.get("documentation").getAsString())); 3263 if (json.has("_documentation")) 3264 parseElementProperties(getJObject(json, "_documentation"), res.getDocumentationElement()); 3265 if (json.has("interaction")) { 3266 JsonArray array = json.getAsJsonArray("interaction"); 3267 for (int i = 0; i < array.size(); i++) { 3268 res.getInteraction().add(parseCapabilityStatementResourceInteractionComponent(array.get(i).getAsJsonObject(), owner)); 3269 } 3270 }; 3271 if (json.has("versioning")) 3272 res.setVersioningElement(parseEnumeration(json.get("versioning").getAsString(), CapabilityStatement.ResourceVersionPolicy.NULL, new CapabilityStatement.ResourceVersionPolicyEnumFactory())); 3273 if (json.has("_versioning")) 3274 parseElementProperties(getJObject(json, "_versioning"), res.getVersioningElement()); 3275 if (json.has("readHistory")) 3276 res.setReadHistoryElement(parseBoolean(json.get("readHistory").getAsBoolean())); 3277 if (json.has("_readHistory")) 3278 parseElementProperties(getJObject(json, "_readHistory"), res.getReadHistoryElement()); 3279 if (json.has("updateCreate")) 3280 res.setUpdateCreateElement(parseBoolean(json.get("updateCreate").getAsBoolean())); 3281 if (json.has("_updateCreate")) 3282 parseElementProperties(getJObject(json, "_updateCreate"), res.getUpdateCreateElement()); 3283 if (json.has("conditionalCreate")) 3284 res.setConditionalCreateElement(parseBoolean(json.get("conditionalCreate").getAsBoolean())); 3285 if (json.has("_conditionalCreate")) 3286 parseElementProperties(getJObject(json, "_conditionalCreate"), res.getConditionalCreateElement()); 3287 if (json.has("conditionalRead")) 3288 res.setConditionalReadElement(parseEnumeration(json.get("conditionalRead").getAsString(), CapabilityStatement.ConditionalReadStatus.NULL, new CapabilityStatement.ConditionalReadStatusEnumFactory())); 3289 if (json.has("_conditionalRead")) 3290 parseElementProperties(getJObject(json, "_conditionalRead"), res.getConditionalReadElement()); 3291 if (json.has("conditionalUpdate")) 3292 res.setConditionalUpdateElement(parseBoolean(json.get("conditionalUpdate").getAsBoolean())); 3293 if (json.has("_conditionalUpdate")) 3294 parseElementProperties(getJObject(json, "_conditionalUpdate"), res.getConditionalUpdateElement()); 3295 if (json.has("conditionalDelete")) 3296 res.setConditionalDeleteElement(parseEnumeration(json.get("conditionalDelete").getAsString(), CapabilityStatement.ConditionalDeleteStatus.NULL, new CapabilityStatement.ConditionalDeleteStatusEnumFactory())); 3297 if (json.has("_conditionalDelete")) 3298 parseElementProperties(getJObject(json, "_conditionalDelete"), res.getConditionalDeleteElement()); 3299 if (json.has("referencePolicy")) { 3300 JsonArray array = json.getAsJsonArray("referencePolicy"); 3301 for (int i = 0; i < array.size(); i++) { 3302 if (array.get(i).isJsonNull()) { 3303 res.getReferencePolicy().add(new Enumeration<CapabilityStatement.ReferenceHandlingPolicy>()); 3304 } else { 3305 res.getReferencePolicy().add(parseEnumeration(array.get(i).getAsString(), CapabilityStatement.ReferenceHandlingPolicy.NULL, new CapabilityStatement.ReferenceHandlingPolicyEnumFactory())); 3306 } 3307 } 3308 }; 3309 if (json.has("_referencePolicy")) { 3310 JsonArray array = json.getAsJsonArray("_referencePolicy"); 3311 for (int i = 0; i < array.size(); i++) { 3312 if (i == res.getReferencePolicy().size()) 3313 res.getReferencePolicy().add(parseEnumeration(null, CapabilityStatement.ReferenceHandlingPolicy.NULL, new CapabilityStatement.ReferenceHandlingPolicyEnumFactory())); 3314 if (array.get(i) instanceof JsonObject) 3315 parseElementProperties(array.get(i).getAsJsonObject(), res.getReferencePolicy().get(i)); 3316 } 3317 }; 3318 if (json.has("searchInclude")) { 3319 JsonArray array = json.getAsJsonArray("searchInclude"); 3320 for (int i = 0; i < array.size(); i++) { 3321 if (array.get(i).isJsonNull()) { 3322 res.getSearchInclude().add(new StringType()); 3323 } else { 3324 res.getSearchInclude().add(parseString(array.get(i).getAsString())); 3325 } 3326 } 3327 }; 3328 if (json.has("_searchInclude")) { 3329 JsonArray array = json.getAsJsonArray("_searchInclude"); 3330 for (int i = 0; i < array.size(); i++) { 3331 if (i == res.getSearchInclude().size()) 3332 res.getSearchInclude().add(parseString(null)); 3333 if (array.get(i) instanceof JsonObject) 3334 parseElementProperties(array.get(i).getAsJsonObject(), res.getSearchInclude().get(i)); 3335 } 3336 }; 3337 if (json.has("searchRevInclude")) { 3338 JsonArray array = json.getAsJsonArray("searchRevInclude"); 3339 for (int i = 0; i < array.size(); i++) { 3340 if (array.get(i).isJsonNull()) { 3341 res.getSearchRevInclude().add(new StringType()); 3342 } else { 3343 res.getSearchRevInclude().add(parseString(array.get(i).getAsString())); 3344 } 3345 } 3346 }; 3347 if (json.has("_searchRevInclude")) { 3348 JsonArray array = json.getAsJsonArray("_searchRevInclude"); 3349 for (int i = 0; i < array.size(); i++) { 3350 if (i == res.getSearchRevInclude().size()) 3351 res.getSearchRevInclude().add(parseString(null)); 3352 if (array.get(i) instanceof JsonObject) 3353 parseElementProperties(array.get(i).getAsJsonObject(), res.getSearchRevInclude().get(i)); 3354 } 3355 }; 3356 if (json.has("searchParam")) { 3357 JsonArray array = json.getAsJsonArray("searchParam"); 3358 for (int i = 0; i < array.size(); i++) { 3359 res.getSearchParam().add(parseCapabilityStatementCapabilityStatementRestResourceSearchParamComponent(array.get(i).getAsJsonObject(), owner)); 3360 } 3361 }; 3362 } 3363 3364 protected CapabilityStatement.ResourceInteractionComponent parseCapabilityStatementResourceInteractionComponent(JsonObject json, CapabilityStatement owner) throws IOException, FHIRFormatError { 3365 CapabilityStatement.ResourceInteractionComponent res = new CapabilityStatement.ResourceInteractionComponent(); 3366 parseCapabilityStatementResourceInteractionComponentProperties(json, owner, res); 3367 return res; 3368 } 3369 3370 protected void parseCapabilityStatementResourceInteractionComponentProperties(JsonObject json, CapabilityStatement owner, CapabilityStatement.ResourceInteractionComponent res) throws IOException, FHIRFormatError { 3371 parseBackboneProperties(json, res); 3372 if (json.has("code")) 3373 res.setCodeElement(parseEnumeration(json.get("code").getAsString(), CapabilityStatement.TypeRestfulInteraction.NULL, new CapabilityStatement.TypeRestfulInteractionEnumFactory())); 3374 if (json.has("_code")) 3375 parseElementProperties(getJObject(json, "_code"), res.getCodeElement()); 3376 if (json.has("documentation")) 3377 res.setDocumentationElement(parseString(json.get("documentation").getAsString())); 3378 if (json.has("_documentation")) 3379 parseElementProperties(getJObject(json, "_documentation"), res.getDocumentationElement()); 3380 } 3381 3382 protected CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent parseCapabilityStatementCapabilityStatementRestResourceSearchParamComponent(JsonObject json, CapabilityStatement owner) throws IOException, FHIRFormatError { 3383 CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent res = new CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent(); 3384 parseCapabilityStatementCapabilityStatementRestResourceSearchParamComponentProperties(json, owner, res); 3385 return res; 3386 } 3387 3388 protected void parseCapabilityStatementCapabilityStatementRestResourceSearchParamComponentProperties(JsonObject json, CapabilityStatement owner, CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent res) throws IOException, FHIRFormatError { 3389 parseBackboneProperties(json, res); 3390 if (json.has("name")) 3391 res.setNameElement(parseString(json.get("name").getAsString())); 3392 if (json.has("_name")) 3393 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 3394 if (json.has("definition")) 3395 res.setDefinitionElement(parseUri(json.get("definition").getAsString())); 3396 if (json.has("_definition")) 3397 parseElementProperties(getJObject(json, "_definition"), res.getDefinitionElement()); 3398 if (json.has("type")) 3399 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), Enumerations.SearchParamType.NULL, new Enumerations.SearchParamTypeEnumFactory())); 3400 if (json.has("_type")) 3401 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 3402 if (json.has("documentation")) 3403 res.setDocumentationElement(parseString(json.get("documentation").getAsString())); 3404 if (json.has("_documentation")) 3405 parseElementProperties(getJObject(json, "_documentation"), res.getDocumentationElement()); 3406 } 3407 3408 protected CapabilityStatement.SystemInteractionComponent parseCapabilityStatementSystemInteractionComponent(JsonObject json, CapabilityStatement owner) throws IOException, FHIRFormatError { 3409 CapabilityStatement.SystemInteractionComponent res = new CapabilityStatement.SystemInteractionComponent(); 3410 parseCapabilityStatementSystemInteractionComponentProperties(json, owner, res); 3411 return res; 3412 } 3413 3414 protected void parseCapabilityStatementSystemInteractionComponentProperties(JsonObject json, CapabilityStatement owner, CapabilityStatement.SystemInteractionComponent res) throws IOException, FHIRFormatError { 3415 parseBackboneProperties(json, res); 3416 if (json.has("code")) 3417 res.setCodeElement(parseEnumeration(json.get("code").getAsString(), CapabilityStatement.SystemRestfulInteraction.NULL, new CapabilityStatement.SystemRestfulInteractionEnumFactory())); 3418 if (json.has("_code")) 3419 parseElementProperties(getJObject(json, "_code"), res.getCodeElement()); 3420 if (json.has("documentation")) 3421 res.setDocumentationElement(parseString(json.get("documentation").getAsString())); 3422 if (json.has("_documentation")) 3423 parseElementProperties(getJObject(json, "_documentation"), res.getDocumentationElement()); 3424 } 3425 3426 protected CapabilityStatement.CapabilityStatementRestOperationComponent parseCapabilityStatementCapabilityStatementRestOperationComponent(JsonObject json, CapabilityStatement owner) throws IOException, FHIRFormatError { 3427 CapabilityStatement.CapabilityStatementRestOperationComponent res = new CapabilityStatement.CapabilityStatementRestOperationComponent(); 3428 parseCapabilityStatementCapabilityStatementRestOperationComponentProperties(json, owner, res); 3429 return res; 3430 } 3431 3432 protected void parseCapabilityStatementCapabilityStatementRestOperationComponentProperties(JsonObject json, CapabilityStatement owner, CapabilityStatement.CapabilityStatementRestOperationComponent res) throws IOException, FHIRFormatError { 3433 parseBackboneProperties(json, res); 3434 if (json.has("name")) 3435 res.setNameElement(parseString(json.get("name").getAsString())); 3436 if (json.has("_name")) 3437 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 3438 if (json.has("definition")) 3439 res.setDefinition(parseReference(getJObject(json, "definition"))); 3440 } 3441 3442 protected CapabilityStatement.CapabilityStatementMessagingComponent parseCapabilityStatementCapabilityStatementMessagingComponent(JsonObject json, CapabilityStatement owner) throws IOException, FHIRFormatError { 3443 CapabilityStatement.CapabilityStatementMessagingComponent res = new CapabilityStatement.CapabilityStatementMessagingComponent(); 3444 parseCapabilityStatementCapabilityStatementMessagingComponentProperties(json, owner, res); 3445 return res; 3446 } 3447 3448 protected void parseCapabilityStatementCapabilityStatementMessagingComponentProperties(JsonObject json, CapabilityStatement owner, CapabilityStatement.CapabilityStatementMessagingComponent res) throws IOException, FHIRFormatError { 3449 parseBackboneProperties(json, res); 3450 if (json.has("endpoint")) { 3451 JsonArray array = json.getAsJsonArray("endpoint"); 3452 for (int i = 0; i < array.size(); i++) { 3453 res.getEndpoint().add(parseCapabilityStatementCapabilityStatementMessagingEndpointComponent(array.get(i).getAsJsonObject(), owner)); 3454 } 3455 }; 3456 if (json.has("reliableCache")) 3457 res.setReliableCacheElement(parseUnsignedInt(json.get("reliableCache").getAsString())); 3458 if (json.has("_reliableCache")) 3459 parseElementProperties(getJObject(json, "_reliableCache"), res.getReliableCacheElement()); 3460 if (json.has("documentation")) 3461 res.setDocumentationElement(parseString(json.get("documentation").getAsString())); 3462 if (json.has("_documentation")) 3463 parseElementProperties(getJObject(json, "_documentation"), res.getDocumentationElement()); 3464 if (json.has("supportedMessage")) { 3465 JsonArray array = json.getAsJsonArray("supportedMessage"); 3466 for (int i = 0; i < array.size(); i++) { 3467 res.getSupportedMessage().add(parseCapabilityStatementCapabilityStatementMessagingSupportedMessageComponent(array.get(i).getAsJsonObject(), owner)); 3468 } 3469 }; 3470 if (json.has("event")) { 3471 JsonArray array = json.getAsJsonArray("event"); 3472 for (int i = 0; i < array.size(); i++) { 3473 res.getEvent().add(parseCapabilityStatementCapabilityStatementMessagingEventComponent(array.get(i).getAsJsonObject(), owner)); 3474 } 3475 }; 3476 } 3477 3478 protected CapabilityStatement.CapabilityStatementMessagingEndpointComponent parseCapabilityStatementCapabilityStatementMessagingEndpointComponent(JsonObject json, CapabilityStatement owner) throws IOException, FHIRFormatError { 3479 CapabilityStatement.CapabilityStatementMessagingEndpointComponent res = new CapabilityStatement.CapabilityStatementMessagingEndpointComponent(); 3480 parseCapabilityStatementCapabilityStatementMessagingEndpointComponentProperties(json, owner, res); 3481 return res; 3482 } 3483 3484 protected void parseCapabilityStatementCapabilityStatementMessagingEndpointComponentProperties(JsonObject json, CapabilityStatement owner, CapabilityStatement.CapabilityStatementMessagingEndpointComponent res) throws IOException, FHIRFormatError { 3485 parseBackboneProperties(json, res); 3486 if (json.has("protocol")) 3487 res.setProtocol(parseCoding(getJObject(json, "protocol"))); 3488 if (json.has("address")) 3489 res.setAddressElement(parseUri(json.get("address").getAsString())); 3490 if (json.has("_address")) 3491 parseElementProperties(getJObject(json, "_address"), res.getAddressElement()); 3492 } 3493 3494 protected CapabilityStatement.CapabilityStatementMessagingSupportedMessageComponent parseCapabilityStatementCapabilityStatementMessagingSupportedMessageComponent(JsonObject json, CapabilityStatement owner) throws IOException, FHIRFormatError { 3495 CapabilityStatement.CapabilityStatementMessagingSupportedMessageComponent res = new CapabilityStatement.CapabilityStatementMessagingSupportedMessageComponent(); 3496 parseCapabilityStatementCapabilityStatementMessagingSupportedMessageComponentProperties(json, owner, res); 3497 return res; 3498 } 3499 3500 protected void parseCapabilityStatementCapabilityStatementMessagingSupportedMessageComponentProperties(JsonObject json, CapabilityStatement owner, CapabilityStatement.CapabilityStatementMessagingSupportedMessageComponent res) throws IOException, FHIRFormatError { 3501 parseBackboneProperties(json, res); 3502 if (json.has("mode")) 3503 res.setModeElement(parseEnumeration(json.get("mode").getAsString(), CapabilityStatement.EventCapabilityMode.NULL, new CapabilityStatement.EventCapabilityModeEnumFactory())); 3504 if (json.has("_mode")) 3505 parseElementProperties(getJObject(json, "_mode"), res.getModeElement()); 3506 if (json.has("definition")) 3507 res.setDefinition(parseReference(getJObject(json, "definition"))); 3508 } 3509 3510 protected CapabilityStatement.CapabilityStatementMessagingEventComponent parseCapabilityStatementCapabilityStatementMessagingEventComponent(JsonObject json, CapabilityStatement owner) throws IOException, FHIRFormatError { 3511 CapabilityStatement.CapabilityStatementMessagingEventComponent res = new CapabilityStatement.CapabilityStatementMessagingEventComponent(); 3512 parseCapabilityStatementCapabilityStatementMessagingEventComponentProperties(json, owner, res); 3513 return res; 3514 } 3515 3516 protected void parseCapabilityStatementCapabilityStatementMessagingEventComponentProperties(JsonObject json, CapabilityStatement owner, CapabilityStatement.CapabilityStatementMessagingEventComponent res) throws IOException, FHIRFormatError { 3517 parseBackboneProperties(json, res); 3518 if (json.has("code")) 3519 res.setCode(parseCoding(getJObject(json, "code"))); 3520 if (json.has("category")) 3521 res.setCategoryElement(parseEnumeration(json.get("category").getAsString(), CapabilityStatement.MessageSignificanceCategory.NULL, new CapabilityStatement.MessageSignificanceCategoryEnumFactory())); 3522 if (json.has("_category")) 3523 parseElementProperties(getJObject(json, "_category"), res.getCategoryElement()); 3524 if (json.has("mode")) 3525 res.setModeElement(parseEnumeration(json.get("mode").getAsString(), CapabilityStatement.EventCapabilityMode.NULL, new CapabilityStatement.EventCapabilityModeEnumFactory())); 3526 if (json.has("_mode")) 3527 parseElementProperties(getJObject(json, "_mode"), res.getModeElement()); 3528 if (json.has("focus")) 3529 res.setFocusElement(parseCode(json.get("focus").getAsString())); 3530 if (json.has("_focus")) 3531 parseElementProperties(getJObject(json, "_focus"), res.getFocusElement()); 3532 if (json.has("request")) 3533 res.setRequest(parseReference(getJObject(json, "request"))); 3534 if (json.has("response")) 3535 res.setResponse(parseReference(getJObject(json, "response"))); 3536 if (json.has("documentation")) 3537 res.setDocumentationElement(parseString(json.get("documentation").getAsString())); 3538 if (json.has("_documentation")) 3539 parseElementProperties(getJObject(json, "_documentation"), res.getDocumentationElement()); 3540 } 3541 3542 protected CapabilityStatement.CapabilityStatementDocumentComponent parseCapabilityStatementCapabilityStatementDocumentComponent(JsonObject json, CapabilityStatement owner) throws IOException, FHIRFormatError { 3543 CapabilityStatement.CapabilityStatementDocumentComponent res = new CapabilityStatement.CapabilityStatementDocumentComponent(); 3544 parseCapabilityStatementCapabilityStatementDocumentComponentProperties(json, owner, res); 3545 return res; 3546 } 3547 3548 protected void parseCapabilityStatementCapabilityStatementDocumentComponentProperties(JsonObject json, CapabilityStatement owner, CapabilityStatement.CapabilityStatementDocumentComponent res) throws IOException, FHIRFormatError { 3549 parseBackboneProperties(json, res); 3550 if (json.has("mode")) 3551 res.setModeElement(parseEnumeration(json.get("mode").getAsString(), CapabilityStatement.DocumentMode.NULL, new CapabilityStatement.DocumentModeEnumFactory())); 3552 if (json.has("_mode")) 3553 parseElementProperties(getJObject(json, "_mode"), res.getModeElement()); 3554 if (json.has("documentation")) 3555 res.setDocumentationElement(parseString(json.get("documentation").getAsString())); 3556 if (json.has("_documentation")) 3557 parseElementProperties(getJObject(json, "_documentation"), res.getDocumentationElement()); 3558 if (json.has("profile")) 3559 res.setProfile(parseReference(getJObject(json, "profile"))); 3560 } 3561 3562 protected CarePlan parseCarePlan(JsonObject json) throws IOException, FHIRFormatError { 3563 CarePlan res = new CarePlan(); 3564 parseCarePlanProperties(json, res); 3565 return res; 3566 } 3567 3568 protected void parseCarePlanProperties(JsonObject json, CarePlan res) throws IOException, FHIRFormatError { 3569 parseDomainResourceProperties(json, res); 3570 if (json.has("identifier")) { 3571 JsonArray array = json.getAsJsonArray("identifier"); 3572 for (int i = 0; i < array.size(); i++) { 3573 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 3574 } 3575 }; 3576 if (json.has("definition")) { 3577 JsonArray array = json.getAsJsonArray("definition"); 3578 for (int i = 0; i < array.size(); i++) { 3579 res.getDefinition().add(parseReference(array.get(i).getAsJsonObject())); 3580 } 3581 }; 3582 if (json.has("basedOn")) { 3583 JsonArray array = json.getAsJsonArray("basedOn"); 3584 for (int i = 0; i < array.size(); i++) { 3585 res.getBasedOn().add(parseReference(array.get(i).getAsJsonObject())); 3586 } 3587 }; 3588 if (json.has("replaces")) { 3589 JsonArray array = json.getAsJsonArray("replaces"); 3590 for (int i = 0; i < array.size(); i++) { 3591 res.getReplaces().add(parseReference(array.get(i).getAsJsonObject())); 3592 } 3593 }; 3594 if (json.has("partOf")) { 3595 JsonArray array = json.getAsJsonArray("partOf"); 3596 for (int i = 0; i < array.size(); i++) { 3597 res.getPartOf().add(parseReference(array.get(i).getAsJsonObject())); 3598 } 3599 }; 3600 if (json.has("status")) 3601 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), CarePlan.CarePlanStatus.NULL, new CarePlan.CarePlanStatusEnumFactory())); 3602 if (json.has("_status")) 3603 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 3604 if (json.has("intent")) 3605 res.setIntentElement(parseEnumeration(json.get("intent").getAsString(), CarePlan.CarePlanIntent.NULL, new CarePlan.CarePlanIntentEnumFactory())); 3606 if (json.has("_intent")) 3607 parseElementProperties(getJObject(json, "_intent"), res.getIntentElement()); 3608 if (json.has("category")) { 3609 JsonArray array = json.getAsJsonArray("category"); 3610 for (int i = 0; i < array.size(); i++) { 3611 res.getCategory().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 3612 } 3613 }; 3614 if (json.has("title")) 3615 res.setTitleElement(parseString(json.get("title").getAsString())); 3616 if (json.has("_title")) 3617 parseElementProperties(getJObject(json, "_title"), res.getTitleElement()); 3618 if (json.has("description")) 3619 res.setDescriptionElement(parseString(json.get("description").getAsString())); 3620 if (json.has("_description")) 3621 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 3622 if (json.has("subject")) 3623 res.setSubject(parseReference(getJObject(json, "subject"))); 3624 if (json.has("context")) 3625 res.setContext(parseReference(getJObject(json, "context"))); 3626 if (json.has("period")) 3627 res.setPeriod(parsePeriod(getJObject(json, "period"))); 3628 if (json.has("author")) { 3629 JsonArray array = json.getAsJsonArray("author"); 3630 for (int i = 0; i < array.size(); i++) { 3631 res.getAuthor().add(parseReference(array.get(i).getAsJsonObject())); 3632 } 3633 }; 3634 if (json.has("careTeam")) { 3635 JsonArray array = json.getAsJsonArray("careTeam"); 3636 for (int i = 0; i < array.size(); i++) { 3637 res.getCareTeam().add(parseReference(array.get(i).getAsJsonObject())); 3638 } 3639 }; 3640 if (json.has("addresses")) { 3641 JsonArray array = json.getAsJsonArray("addresses"); 3642 for (int i = 0; i < array.size(); i++) { 3643 res.getAddresses().add(parseReference(array.get(i).getAsJsonObject())); 3644 } 3645 }; 3646 if (json.has("supportingInfo")) { 3647 JsonArray array = json.getAsJsonArray("supportingInfo"); 3648 for (int i = 0; i < array.size(); i++) { 3649 res.getSupportingInfo().add(parseReference(array.get(i).getAsJsonObject())); 3650 } 3651 }; 3652 if (json.has("goal")) { 3653 JsonArray array = json.getAsJsonArray("goal"); 3654 for (int i = 0; i < array.size(); i++) { 3655 res.getGoal().add(parseReference(array.get(i).getAsJsonObject())); 3656 } 3657 }; 3658 if (json.has("activity")) { 3659 JsonArray array = json.getAsJsonArray("activity"); 3660 for (int i = 0; i < array.size(); i++) { 3661 res.getActivity().add(parseCarePlanCarePlanActivityComponent(array.get(i).getAsJsonObject(), res)); 3662 } 3663 }; 3664 if (json.has("note")) { 3665 JsonArray array = json.getAsJsonArray("note"); 3666 for (int i = 0; i < array.size(); i++) { 3667 res.getNote().add(parseAnnotation(array.get(i).getAsJsonObject())); 3668 } 3669 }; 3670 } 3671 3672 protected CarePlan.CarePlanActivityComponent parseCarePlanCarePlanActivityComponent(JsonObject json, CarePlan owner) throws IOException, FHIRFormatError { 3673 CarePlan.CarePlanActivityComponent res = new CarePlan.CarePlanActivityComponent(); 3674 parseCarePlanCarePlanActivityComponentProperties(json, owner, res); 3675 return res; 3676 } 3677 3678 protected void parseCarePlanCarePlanActivityComponentProperties(JsonObject json, CarePlan owner, CarePlan.CarePlanActivityComponent res) throws IOException, FHIRFormatError { 3679 parseBackboneProperties(json, res); 3680 if (json.has("outcomeCodeableConcept")) { 3681 JsonArray array = json.getAsJsonArray("outcomeCodeableConcept"); 3682 for (int i = 0; i < array.size(); i++) { 3683 res.getOutcomeCodeableConcept().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 3684 } 3685 }; 3686 if (json.has("outcomeReference")) { 3687 JsonArray array = json.getAsJsonArray("outcomeReference"); 3688 for (int i = 0; i < array.size(); i++) { 3689 res.getOutcomeReference().add(parseReference(array.get(i).getAsJsonObject())); 3690 } 3691 }; 3692 if (json.has("progress")) { 3693 JsonArray array = json.getAsJsonArray("progress"); 3694 for (int i = 0; i < array.size(); i++) { 3695 res.getProgress().add(parseAnnotation(array.get(i).getAsJsonObject())); 3696 } 3697 }; 3698 if (json.has("reference")) 3699 res.setReference(parseReference(getJObject(json, "reference"))); 3700 if (json.has("detail")) 3701 res.setDetail(parseCarePlanCarePlanActivityDetailComponent(getJObject(json, "detail"), owner)); 3702 } 3703 3704 protected CarePlan.CarePlanActivityDetailComponent parseCarePlanCarePlanActivityDetailComponent(JsonObject json, CarePlan owner) throws IOException, FHIRFormatError { 3705 CarePlan.CarePlanActivityDetailComponent res = new CarePlan.CarePlanActivityDetailComponent(); 3706 parseCarePlanCarePlanActivityDetailComponentProperties(json, owner, res); 3707 return res; 3708 } 3709 3710 protected void parseCarePlanCarePlanActivityDetailComponentProperties(JsonObject json, CarePlan owner, CarePlan.CarePlanActivityDetailComponent res) throws IOException, FHIRFormatError { 3711 parseBackboneProperties(json, res); 3712 if (json.has("category")) 3713 res.setCategory(parseCodeableConcept(getJObject(json, "category"))); 3714 if (json.has("definition")) 3715 res.setDefinition(parseReference(getJObject(json, "definition"))); 3716 if (json.has("code")) 3717 res.setCode(parseCodeableConcept(getJObject(json, "code"))); 3718 if (json.has("reasonCode")) { 3719 JsonArray array = json.getAsJsonArray("reasonCode"); 3720 for (int i = 0; i < array.size(); i++) { 3721 res.getReasonCode().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 3722 } 3723 }; 3724 if (json.has("reasonReference")) { 3725 JsonArray array = json.getAsJsonArray("reasonReference"); 3726 for (int i = 0; i < array.size(); i++) { 3727 res.getReasonReference().add(parseReference(array.get(i).getAsJsonObject())); 3728 } 3729 }; 3730 if (json.has("goal")) { 3731 JsonArray array = json.getAsJsonArray("goal"); 3732 for (int i = 0; i < array.size(); i++) { 3733 res.getGoal().add(parseReference(array.get(i).getAsJsonObject())); 3734 } 3735 }; 3736 if (json.has("status")) 3737 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), CarePlan.CarePlanActivityStatus.NULL, new CarePlan.CarePlanActivityStatusEnumFactory())); 3738 if (json.has("_status")) 3739 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 3740 if (json.has("statusReason")) 3741 res.setStatusReasonElement(parseString(json.get("statusReason").getAsString())); 3742 if (json.has("_statusReason")) 3743 parseElementProperties(getJObject(json, "_statusReason"), res.getStatusReasonElement()); 3744 if (json.has("prohibited")) 3745 res.setProhibitedElement(parseBoolean(json.get("prohibited").getAsBoolean())); 3746 if (json.has("_prohibited")) 3747 parseElementProperties(getJObject(json, "_prohibited"), res.getProhibitedElement()); 3748 Type scheduled = parseType("scheduled", json); 3749 if (scheduled != null) 3750 res.setScheduled(scheduled); 3751 if (json.has("location")) 3752 res.setLocation(parseReference(getJObject(json, "location"))); 3753 if (json.has("performer")) { 3754 JsonArray array = json.getAsJsonArray("performer"); 3755 for (int i = 0; i < array.size(); i++) { 3756 res.getPerformer().add(parseReference(array.get(i).getAsJsonObject())); 3757 } 3758 }; 3759 Type product = parseType("product", json); 3760 if (product != null) 3761 res.setProduct(product); 3762 if (json.has("dailyAmount")) 3763 res.setDailyAmount(parseSimpleQuantity(getJObject(json, "dailyAmount"))); 3764 if (json.has("quantity")) 3765 res.setQuantity(parseSimpleQuantity(getJObject(json, "quantity"))); 3766 if (json.has("description")) 3767 res.setDescriptionElement(parseString(json.get("description").getAsString())); 3768 if (json.has("_description")) 3769 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 3770 } 3771 3772 protected CareTeam parseCareTeam(JsonObject json) throws IOException, FHIRFormatError { 3773 CareTeam res = new CareTeam(); 3774 parseCareTeamProperties(json, res); 3775 return res; 3776 } 3777 3778 protected void parseCareTeamProperties(JsonObject json, CareTeam res) throws IOException, FHIRFormatError { 3779 parseDomainResourceProperties(json, res); 3780 if (json.has("identifier")) { 3781 JsonArray array = json.getAsJsonArray("identifier"); 3782 for (int i = 0; i < array.size(); i++) { 3783 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 3784 } 3785 }; 3786 if (json.has("status")) 3787 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), CareTeam.CareTeamStatus.NULL, new CareTeam.CareTeamStatusEnumFactory())); 3788 if (json.has("_status")) 3789 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 3790 if (json.has("category")) { 3791 JsonArray array = json.getAsJsonArray("category"); 3792 for (int i = 0; i < array.size(); i++) { 3793 res.getCategory().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 3794 } 3795 }; 3796 if (json.has("name")) 3797 res.setNameElement(parseString(json.get("name").getAsString())); 3798 if (json.has("_name")) 3799 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 3800 if (json.has("subject")) 3801 res.setSubject(parseReference(getJObject(json, "subject"))); 3802 if (json.has("context")) 3803 res.setContext(parseReference(getJObject(json, "context"))); 3804 if (json.has("period")) 3805 res.setPeriod(parsePeriod(getJObject(json, "period"))); 3806 if (json.has("participant")) { 3807 JsonArray array = json.getAsJsonArray("participant"); 3808 for (int i = 0; i < array.size(); i++) { 3809 res.getParticipant().add(parseCareTeamCareTeamParticipantComponent(array.get(i).getAsJsonObject(), res)); 3810 } 3811 }; 3812 if (json.has("reasonCode")) { 3813 JsonArray array = json.getAsJsonArray("reasonCode"); 3814 for (int i = 0; i < array.size(); i++) { 3815 res.getReasonCode().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 3816 } 3817 }; 3818 if (json.has("reasonReference")) { 3819 JsonArray array = json.getAsJsonArray("reasonReference"); 3820 for (int i = 0; i < array.size(); i++) { 3821 res.getReasonReference().add(parseReference(array.get(i).getAsJsonObject())); 3822 } 3823 }; 3824 if (json.has("managingOrganization")) { 3825 JsonArray array = json.getAsJsonArray("managingOrganization"); 3826 for (int i = 0; i < array.size(); i++) { 3827 res.getManagingOrganization().add(parseReference(array.get(i).getAsJsonObject())); 3828 } 3829 }; 3830 if (json.has("note")) { 3831 JsonArray array = json.getAsJsonArray("note"); 3832 for (int i = 0; i < array.size(); i++) { 3833 res.getNote().add(parseAnnotation(array.get(i).getAsJsonObject())); 3834 } 3835 }; 3836 } 3837 3838 protected CareTeam.CareTeamParticipantComponent parseCareTeamCareTeamParticipantComponent(JsonObject json, CareTeam owner) throws IOException, FHIRFormatError { 3839 CareTeam.CareTeamParticipantComponent res = new CareTeam.CareTeamParticipantComponent(); 3840 parseCareTeamCareTeamParticipantComponentProperties(json, owner, res); 3841 return res; 3842 } 3843 3844 protected void parseCareTeamCareTeamParticipantComponentProperties(JsonObject json, CareTeam owner, CareTeam.CareTeamParticipantComponent res) throws IOException, FHIRFormatError { 3845 parseBackboneProperties(json, res); 3846 if (json.has("role")) 3847 res.setRole(parseCodeableConcept(getJObject(json, "role"))); 3848 if (json.has("member")) 3849 res.setMember(parseReference(getJObject(json, "member"))); 3850 if (json.has("onBehalfOf")) 3851 res.setOnBehalfOf(parseReference(getJObject(json, "onBehalfOf"))); 3852 if (json.has("period")) 3853 res.setPeriod(parsePeriod(getJObject(json, "period"))); 3854 } 3855 3856 protected ChargeItem parseChargeItem(JsonObject json) throws IOException, FHIRFormatError { 3857 ChargeItem res = new ChargeItem(); 3858 parseChargeItemProperties(json, res); 3859 return res; 3860 } 3861 3862 protected void parseChargeItemProperties(JsonObject json, ChargeItem res) throws IOException, FHIRFormatError { 3863 parseDomainResourceProperties(json, res); 3864 if (json.has("identifier")) 3865 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 3866 if (json.has("definition")) { 3867 JsonArray array = json.getAsJsonArray("definition"); 3868 for (int i = 0; i < array.size(); i++) { 3869 if (array.get(i).isJsonNull()) { 3870 res.getDefinition().add(new UriType()); 3871 } else { 3872 res.getDefinition().add(parseUri(array.get(i).getAsString())); 3873 } 3874 } 3875 }; 3876 if (json.has("_definition")) { 3877 JsonArray array = json.getAsJsonArray("_definition"); 3878 for (int i = 0; i < array.size(); i++) { 3879 if (i == res.getDefinition().size()) 3880 res.getDefinition().add(parseUri(null)); 3881 if (array.get(i) instanceof JsonObject) 3882 parseElementProperties(array.get(i).getAsJsonObject(), res.getDefinition().get(i)); 3883 } 3884 }; 3885 if (json.has("status")) 3886 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), ChargeItem.ChargeItemStatus.NULL, new ChargeItem.ChargeItemStatusEnumFactory())); 3887 if (json.has("_status")) 3888 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 3889 if (json.has("partOf")) { 3890 JsonArray array = json.getAsJsonArray("partOf"); 3891 for (int i = 0; i < array.size(); i++) { 3892 res.getPartOf().add(parseReference(array.get(i).getAsJsonObject())); 3893 } 3894 }; 3895 if (json.has("code")) 3896 res.setCode(parseCodeableConcept(getJObject(json, "code"))); 3897 if (json.has("subject")) 3898 res.setSubject(parseReference(getJObject(json, "subject"))); 3899 if (json.has("context")) 3900 res.setContext(parseReference(getJObject(json, "context"))); 3901 Type occurrence = parseType("occurrence", json); 3902 if (occurrence != null) 3903 res.setOccurrence(occurrence); 3904 if (json.has("participant")) { 3905 JsonArray array = json.getAsJsonArray("participant"); 3906 for (int i = 0; i < array.size(); i++) { 3907 res.getParticipant().add(parseChargeItemChargeItemParticipantComponent(array.get(i).getAsJsonObject(), res)); 3908 } 3909 }; 3910 if (json.has("performingOrganization")) 3911 res.setPerformingOrganization(parseReference(getJObject(json, "performingOrganization"))); 3912 if (json.has("requestingOrganization")) 3913 res.setRequestingOrganization(parseReference(getJObject(json, "requestingOrganization"))); 3914 if (json.has("quantity")) 3915 res.setQuantity(parseQuantity(getJObject(json, "quantity"))); 3916 if (json.has("bodysite")) { 3917 JsonArray array = json.getAsJsonArray("bodysite"); 3918 for (int i = 0; i < array.size(); i++) { 3919 res.getBodysite().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 3920 } 3921 }; 3922 if (json.has("factorOverride")) 3923 res.setFactorOverrideElement(parseDecimal(json.get("factorOverride").getAsBigDecimal())); 3924 if (json.has("_factorOverride")) 3925 parseElementProperties(getJObject(json, "_factorOverride"), res.getFactorOverrideElement()); 3926 if (json.has("priceOverride")) 3927 res.setPriceOverride(parseMoney(getJObject(json, "priceOverride"))); 3928 if (json.has("overrideReason")) 3929 res.setOverrideReasonElement(parseString(json.get("overrideReason").getAsString())); 3930 if (json.has("_overrideReason")) 3931 parseElementProperties(getJObject(json, "_overrideReason"), res.getOverrideReasonElement()); 3932 if (json.has("enterer")) 3933 res.setEnterer(parseReference(getJObject(json, "enterer"))); 3934 if (json.has("enteredDate")) 3935 res.setEnteredDateElement(parseDateTime(json.get("enteredDate").getAsString())); 3936 if (json.has("_enteredDate")) 3937 parseElementProperties(getJObject(json, "_enteredDate"), res.getEnteredDateElement()); 3938 if (json.has("reason")) { 3939 JsonArray array = json.getAsJsonArray("reason"); 3940 for (int i = 0; i < array.size(); i++) { 3941 res.getReason().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 3942 } 3943 }; 3944 if (json.has("service")) { 3945 JsonArray array = json.getAsJsonArray("service"); 3946 for (int i = 0; i < array.size(); i++) { 3947 res.getService().add(parseReference(array.get(i).getAsJsonObject())); 3948 } 3949 }; 3950 if (json.has("account")) { 3951 JsonArray array = json.getAsJsonArray("account"); 3952 for (int i = 0; i < array.size(); i++) { 3953 res.getAccount().add(parseReference(array.get(i).getAsJsonObject())); 3954 } 3955 }; 3956 if (json.has("note")) { 3957 JsonArray array = json.getAsJsonArray("note"); 3958 for (int i = 0; i < array.size(); i++) { 3959 res.getNote().add(parseAnnotation(array.get(i).getAsJsonObject())); 3960 } 3961 }; 3962 if (json.has("supportingInformation")) { 3963 JsonArray array = json.getAsJsonArray("supportingInformation"); 3964 for (int i = 0; i < array.size(); i++) { 3965 res.getSupportingInformation().add(parseReference(array.get(i).getAsJsonObject())); 3966 } 3967 }; 3968 } 3969 3970 protected ChargeItem.ChargeItemParticipantComponent parseChargeItemChargeItemParticipantComponent(JsonObject json, ChargeItem owner) throws IOException, FHIRFormatError { 3971 ChargeItem.ChargeItemParticipantComponent res = new ChargeItem.ChargeItemParticipantComponent(); 3972 parseChargeItemChargeItemParticipantComponentProperties(json, owner, res); 3973 return res; 3974 } 3975 3976 protected void parseChargeItemChargeItemParticipantComponentProperties(JsonObject json, ChargeItem owner, ChargeItem.ChargeItemParticipantComponent res) throws IOException, FHIRFormatError { 3977 parseBackboneProperties(json, res); 3978 if (json.has("role")) 3979 res.setRole(parseCodeableConcept(getJObject(json, "role"))); 3980 if (json.has("actor")) 3981 res.setActor(parseReference(getJObject(json, "actor"))); 3982 } 3983 3984 protected Claim parseClaim(JsonObject json) throws IOException, FHIRFormatError { 3985 Claim res = new Claim(); 3986 parseClaimProperties(json, res); 3987 return res; 3988 } 3989 3990 protected void parseClaimProperties(JsonObject json, Claim res) throws IOException, FHIRFormatError { 3991 parseDomainResourceProperties(json, res); 3992 if (json.has("identifier")) { 3993 JsonArray array = json.getAsJsonArray("identifier"); 3994 for (int i = 0; i < array.size(); i++) { 3995 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 3996 } 3997 }; 3998 if (json.has("status")) 3999 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Claim.ClaimStatus.NULL, new Claim.ClaimStatusEnumFactory())); 4000 if (json.has("_status")) 4001 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 4002 if (json.has("type")) 4003 res.setType(parseCodeableConcept(getJObject(json, "type"))); 4004 if (json.has("subType")) { 4005 JsonArray array = json.getAsJsonArray("subType"); 4006 for (int i = 0; i < array.size(); i++) { 4007 res.getSubType().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 4008 } 4009 }; 4010 if (json.has("use")) 4011 res.setUseElement(parseEnumeration(json.get("use").getAsString(), Claim.Use.NULL, new Claim.UseEnumFactory())); 4012 if (json.has("_use")) 4013 parseElementProperties(getJObject(json, "_use"), res.getUseElement()); 4014 if (json.has("patient")) 4015 res.setPatient(parseReference(getJObject(json, "patient"))); 4016 if (json.has("billablePeriod")) 4017 res.setBillablePeriod(parsePeriod(getJObject(json, "billablePeriod"))); 4018 if (json.has("created")) 4019 res.setCreatedElement(parseDateTime(json.get("created").getAsString())); 4020 if (json.has("_created")) 4021 parseElementProperties(getJObject(json, "_created"), res.getCreatedElement()); 4022 if (json.has("enterer")) 4023 res.setEnterer(parseReference(getJObject(json, "enterer"))); 4024 if (json.has("insurer")) 4025 res.setInsurer(parseReference(getJObject(json, "insurer"))); 4026 if (json.has("provider")) 4027 res.setProvider(parseReference(getJObject(json, "provider"))); 4028 if (json.has("organization")) 4029 res.setOrganization(parseReference(getJObject(json, "organization"))); 4030 if (json.has("priority")) 4031 res.setPriority(parseCodeableConcept(getJObject(json, "priority"))); 4032 if (json.has("fundsReserve")) 4033 res.setFundsReserve(parseCodeableConcept(getJObject(json, "fundsReserve"))); 4034 if (json.has("related")) { 4035 JsonArray array = json.getAsJsonArray("related"); 4036 for (int i = 0; i < array.size(); i++) { 4037 res.getRelated().add(parseClaimRelatedClaimComponent(array.get(i).getAsJsonObject(), res)); 4038 } 4039 }; 4040 if (json.has("prescription")) 4041 res.setPrescription(parseReference(getJObject(json, "prescription"))); 4042 if (json.has("originalPrescription")) 4043 res.setOriginalPrescription(parseReference(getJObject(json, "originalPrescription"))); 4044 if (json.has("payee")) 4045 res.setPayee(parseClaimPayeeComponent(getJObject(json, "payee"), res)); 4046 if (json.has("referral")) 4047 res.setReferral(parseReference(getJObject(json, "referral"))); 4048 if (json.has("facility")) 4049 res.setFacility(parseReference(getJObject(json, "facility"))); 4050 if (json.has("careTeam")) { 4051 JsonArray array = json.getAsJsonArray("careTeam"); 4052 for (int i = 0; i < array.size(); i++) { 4053 res.getCareTeam().add(parseClaimCareTeamComponent(array.get(i).getAsJsonObject(), res)); 4054 } 4055 }; 4056 if (json.has("information")) { 4057 JsonArray array = json.getAsJsonArray("information"); 4058 for (int i = 0; i < array.size(); i++) { 4059 res.getInformation().add(parseClaimSpecialConditionComponent(array.get(i).getAsJsonObject(), res)); 4060 } 4061 }; 4062 if (json.has("diagnosis")) { 4063 JsonArray array = json.getAsJsonArray("diagnosis"); 4064 for (int i = 0; i < array.size(); i++) { 4065 res.getDiagnosis().add(parseClaimDiagnosisComponent(array.get(i).getAsJsonObject(), res)); 4066 } 4067 }; 4068 if (json.has("procedure")) { 4069 JsonArray array = json.getAsJsonArray("procedure"); 4070 for (int i = 0; i < array.size(); i++) { 4071 res.getProcedure().add(parseClaimProcedureComponent(array.get(i).getAsJsonObject(), res)); 4072 } 4073 }; 4074 if (json.has("insurance")) { 4075 JsonArray array = json.getAsJsonArray("insurance"); 4076 for (int i = 0; i < array.size(); i++) { 4077 res.getInsurance().add(parseClaimInsuranceComponent(array.get(i).getAsJsonObject(), res)); 4078 } 4079 }; 4080 if (json.has("accident")) 4081 res.setAccident(parseClaimAccidentComponent(getJObject(json, "accident"), res)); 4082 if (json.has("employmentImpacted")) 4083 res.setEmploymentImpacted(parsePeriod(getJObject(json, "employmentImpacted"))); 4084 if (json.has("hospitalization")) 4085 res.setHospitalization(parsePeriod(getJObject(json, "hospitalization"))); 4086 if (json.has("item")) { 4087 JsonArray array = json.getAsJsonArray("item"); 4088 for (int i = 0; i < array.size(); i++) { 4089 res.getItem().add(parseClaimItemComponent(array.get(i).getAsJsonObject(), res)); 4090 } 4091 }; 4092 if (json.has("total")) 4093 res.setTotal(parseMoney(getJObject(json, "total"))); 4094 } 4095 4096 protected Claim.RelatedClaimComponent parseClaimRelatedClaimComponent(JsonObject json, Claim owner) throws IOException, FHIRFormatError { 4097 Claim.RelatedClaimComponent res = new Claim.RelatedClaimComponent(); 4098 parseClaimRelatedClaimComponentProperties(json, owner, res); 4099 return res; 4100 } 4101 4102 protected void parseClaimRelatedClaimComponentProperties(JsonObject json, Claim owner, Claim.RelatedClaimComponent res) throws IOException, FHIRFormatError { 4103 parseBackboneProperties(json, res); 4104 if (json.has("claim")) 4105 res.setClaim(parseReference(getJObject(json, "claim"))); 4106 if (json.has("relationship")) 4107 res.setRelationship(parseCodeableConcept(getJObject(json, "relationship"))); 4108 if (json.has("reference")) 4109 res.setReference(parseIdentifier(getJObject(json, "reference"))); 4110 } 4111 4112 protected Claim.PayeeComponent parseClaimPayeeComponent(JsonObject json, Claim owner) throws IOException, FHIRFormatError { 4113 Claim.PayeeComponent res = new Claim.PayeeComponent(); 4114 parseClaimPayeeComponentProperties(json, owner, res); 4115 return res; 4116 } 4117 4118 protected void parseClaimPayeeComponentProperties(JsonObject json, Claim owner, Claim.PayeeComponent res) throws IOException, FHIRFormatError { 4119 parseBackboneProperties(json, res); 4120 if (json.has("type")) 4121 res.setType(parseCodeableConcept(getJObject(json, "type"))); 4122 if (json.has("resourceType")) 4123 res.setResourceType(parseCoding(getJObject(json, "resourceType"))); 4124 if (json.has("party")) 4125 res.setParty(parseReference(getJObject(json, "party"))); 4126 } 4127 4128 protected Claim.CareTeamComponent parseClaimCareTeamComponent(JsonObject json, Claim owner) throws IOException, FHIRFormatError { 4129 Claim.CareTeamComponent res = new Claim.CareTeamComponent(); 4130 parseClaimCareTeamComponentProperties(json, owner, res); 4131 return res; 4132 } 4133 4134 protected void parseClaimCareTeamComponentProperties(JsonObject json, Claim owner, Claim.CareTeamComponent res) throws IOException, FHIRFormatError { 4135 parseBackboneProperties(json, res); 4136 if (json.has("sequence")) 4137 res.setSequenceElement(parsePositiveInt(json.get("sequence").getAsString())); 4138 if (json.has("_sequence")) 4139 parseElementProperties(getJObject(json, "_sequence"), res.getSequenceElement()); 4140 if (json.has("provider")) 4141 res.setProvider(parseReference(getJObject(json, "provider"))); 4142 if (json.has("responsible")) 4143 res.setResponsibleElement(parseBoolean(json.get("responsible").getAsBoolean())); 4144 if (json.has("_responsible")) 4145 parseElementProperties(getJObject(json, "_responsible"), res.getResponsibleElement()); 4146 if (json.has("role")) 4147 res.setRole(parseCodeableConcept(getJObject(json, "role"))); 4148 if (json.has("qualification")) 4149 res.setQualification(parseCodeableConcept(getJObject(json, "qualification"))); 4150 } 4151 4152 protected Claim.SpecialConditionComponent parseClaimSpecialConditionComponent(JsonObject json, Claim owner) throws IOException, FHIRFormatError { 4153 Claim.SpecialConditionComponent res = new Claim.SpecialConditionComponent(); 4154 parseClaimSpecialConditionComponentProperties(json, owner, res); 4155 return res; 4156 } 4157 4158 protected void parseClaimSpecialConditionComponentProperties(JsonObject json, Claim owner, Claim.SpecialConditionComponent res) throws IOException, FHIRFormatError { 4159 parseBackboneProperties(json, res); 4160 if (json.has("sequence")) 4161 res.setSequenceElement(parsePositiveInt(json.get("sequence").getAsString())); 4162 if (json.has("_sequence")) 4163 parseElementProperties(getJObject(json, "_sequence"), res.getSequenceElement()); 4164 if (json.has("category")) 4165 res.setCategory(parseCodeableConcept(getJObject(json, "category"))); 4166 if (json.has("code")) 4167 res.setCode(parseCodeableConcept(getJObject(json, "code"))); 4168 Type timing = parseType("timing", json); 4169 if (timing != null) 4170 res.setTiming(timing); 4171 Type value = parseType("value", json); 4172 if (value != null) 4173 res.setValue(value); 4174 if (json.has("reason")) 4175 res.setReason(parseCodeableConcept(getJObject(json, "reason"))); 4176 } 4177 4178 protected Claim.DiagnosisComponent parseClaimDiagnosisComponent(JsonObject json, Claim owner) throws IOException, FHIRFormatError { 4179 Claim.DiagnosisComponent res = new Claim.DiagnosisComponent(); 4180 parseClaimDiagnosisComponentProperties(json, owner, res); 4181 return res; 4182 } 4183 4184 protected void parseClaimDiagnosisComponentProperties(JsonObject json, Claim owner, Claim.DiagnosisComponent res) throws IOException, FHIRFormatError { 4185 parseBackboneProperties(json, res); 4186 if (json.has("sequence")) 4187 res.setSequenceElement(parsePositiveInt(json.get("sequence").getAsString())); 4188 if (json.has("_sequence")) 4189 parseElementProperties(getJObject(json, "_sequence"), res.getSequenceElement()); 4190 Type diagnosis = parseType("diagnosis", json); 4191 if (diagnosis != null) 4192 res.setDiagnosis(diagnosis); 4193 if (json.has("type")) { 4194 JsonArray array = json.getAsJsonArray("type"); 4195 for (int i = 0; i < array.size(); i++) { 4196 res.getType().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 4197 } 4198 }; 4199 if (json.has("packageCode")) 4200 res.setPackageCode(parseCodeableConcept(getJObject(json, "packageCode"))); 4201 } 4202 4203 protected Claim.ProcedureComponent parseClaimProcedureComponent(JsonObject json, Claim owner) throws IOException, FHIRFormatError { 4204 Claim.ProcedureComponent res = new Claim.ProcedureComponent(); 4205 parseClaimProcedureComponentProperties(json, owner, res); 4206 return res; 4207 } 4208 4209 protected void parseClaimProcedureComponentProperties(JsonObject json, Claim owner, Claim.ProcedureComponent res) throws IOException, FHIRFormatError { 4210 parseBackboneProperties(json, res); 4211 if (json.has("sequence")) 4212 res.setSequenceElement(parsePositiveInt(json.get("sequence").getAsString())); 4213 if (json.has("_sequence")) 4214 parseElementProperties(getJObject(json, "_sequence"), res.getSequenceElement()); 4215 if (json.has("date")) 4216 res.setDateElement(parseDateTime(json.get("date").getAsString())); 4217 if (json.has("_date")) 4218 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 4219 Type procedure = parseType("procedure", json); 4220 if (procedure != null) 4221 res.setProcedure(procedure); 4222 } 4223 4224 protected Claim.InsuranceComponent parseClaimInsuranceComponent(JsonObject json, Claim owner) throws IOException, FHIRFormatError { 4225 Claim.InsuranceComponent res = new Claim.InsuranceComponent(); 4226 parseClaimInsuranceComponentProperties(json, owner, res); 4227 return res; 4228 } 4229 4230 protected void parseClaimInsuranceComponentProperties(JsonObject json, Claim owner, Claim.InsuranceComponent res) throws IOException, FHIRFormatError { 4231 parseBackboneProperties(json, res); 4232 if (json.has("sequence")) 4233 res.setSequenceElement(parsePositiveInt(json.get("sequence").getAsString())); 4234 if (json.has("_sequence")) 4235 parseElementProperties(getJObject(json, "_sequence"), res.getSequenceElement()); 4236 if (json.has("focal")) 4237 res.setFocalElement(parseBoolean(json.get("focal").getAsBoolean())); 4238 if (json.has("_focal")) 4239 parseElementProperties(getJObject(json, "_focal"), res.getFocalElement()); 4240 if (json.has("coverage")) 4241 res.setCoverage(parseReference(getJObject(json, "coverage"))); 4242 if (json.has("businessArrangement")) 4243 res.setBusinessArrangementElement(parseString(json.get("businessArrangement").getAsString())); 4244 if (json.has("_businessArrangement")) 4245 parseElementProperties(getJObject(json, "_businessArrangement"), res.getBusinessArrangementElement()); 4246 if (json.has("preAuthRef")) { 4247 JsonArray array = json.getAsJsonArray("preAuthRef"); 4248 for (int i = 0; i < array.size(); i++) { 4249 if (array.get(i).isJsonNull()) { 4250 res.getPreAuthRef().add(new StringType()); 4251 } else { 4252 res.getPreAuthRef().add(parseString(array.get(i).getAsString())); 4253 } 4254 } 4255 }; 4256 if (json.has("_preAuthRef")) { 4257 JsonArray array = json.getAsJsonArray("_preAuthRef"); 4258 for (int i = 0; i < array.size(); i++) { 4259 if (i == res.getPreAuthRef().size()) 4260 res.getPreAuthRef().add(parseString(null)); 4261 if (array.get(i) instanceof JsonObject) 4262 parseElementProperties(array.get(i).getAsJsonObject(), res.getPreAuthRef().get(i)); 4263 } 4264 }; 4265 if (json.has("claimResponse")) 4266 res.setClaimResponse(parseReference(getJObject(json, "claimResponse"))); 4267 } 4268 4269 protected Claim.AccidentComponent parseClaimAccidentComponent(JsonObject json, Claim owner) throws IOException, FHIRFormatError { 4270 Claim.AccidentComponent res = new Claim.AccidentComponent(); 4271 parseClaimAccidentComponentProperties(json, owner, res); 4272 return res; 4273 } 4274 4275 protected void parseClaimAccidentComponentProperties(JsonObject json, Claim owner, Claim.AccidentComponent res) throws IOException, FHIRFormatError { 4276 parseBackboneProperties(json, res); 4277 if (json.has("date")) 4278 res.setDateElement(parseDate(json.get("date").getAsString())); 4279 if (json.has("_date")) 4280 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 4281 if (json.has("type")) 4282 res.setType(parseCodeableConcept(getJObject(json, "type"))); 4283 Type location = parseType("location", json); 4284 if (location != null) 4285 res.setLocation(location); 4286 } 4287 4288 protected Claim.ItemComponent parseClaimItemComponent(JsonObject json, Claim owner) throws IOException, FHIRFormatError { 4289 Claim.ItemComponent res = new Claim.ItemComponent(); 4290 parseClaimItemComponentProperties(json, owner, res); 4291 return res; 4292 } 4293 4294 protected void parseClaimItemComponentProperties(JsonObject json, Claim owner, Claim.ItemComponent res) throws IOException, FHIRFormatError { 4295 parseBackboneProperties(json, res); 4296 if (json.has("sequence")) 4297 res.setSequenceElement(parsePositiveInt(json.get("sequence").getAsString())); 4298 if (json.has("_sequence")) 4299 parseElementProperties(getJObject(json, "_sequence"), res.getSequenceElement()); 4300 if (json.has("careTeamLinkId")) { 4301 JsonArray array = json.getAsJsonArray("careTeamLinkId"); 4302 for (int i = 0; i < array.size(); i++) { 4303 if (array.get(i).isJsonNull()) { 4304 res.getCareTeamLinkId().add(new PositiveIntType()); 4305 } else { 4306 res.getCareTeamLinkId().add(parsePositiveInt(array.get(i).getAsString())); 4307 } 4308 } 4309 }; 4310 if (json.has("_careTeamLinkId")) { 4311 JsonArray array = json.getAsJsonArray("_careTeamLinkId"); 4312 for (int i = 0; i < array.size(); i++) { 4313 if (i == res.getCareTeamLinkId().size()) 4314 res.getCareTeamLinkId().add(parsePositiveInt(null)); 4315 if (array.get(i) instanceof JsonObject) 4316 parseElementProperties(array.get(i).getAsJsonObject(), res.getCareTeamLinkId().get(i)); 4317 } 4318 }; 4319 if (json.has("diagnosisLinkId")) { 4320 JsonArray array = json.getAsJsonArray("diagnosisLinkId"); 4321 for (int i = 0; i < array.size(); i++) { 4322 if (array.get(i).isJsonNull()) { 4323 res.getDiagnosisLinkId().add(new PositiveIntType()); 4324 } else { 4325 res.getDiagnosisLinkId().add(parsePositiveInt(array.get(i).getAsString())); 4326 } 4327 } 4328 }; 4329 if (json.has("_diagnosisLinkId")) { 4330 JsonArray array = json.getAsJsonArray("_diagnosisLinkId"); 4331 for (int i = 0; i < array.size(); i++) { 4332 if (i == res.getDiagnosisLinkId().size()) 4333 res.getDiagnosisLinkId().add(parsePositiveInt(null)); 4334 if (array.get(i) instanceof JsonObject) 4335 parseElementProperties(array.get(i).getAsJsonObject(), res.getDiagnosisLinkId().get(i)); 4336 } 4337 }; 4338 if (json.has("procedureLinkId")) { 4339 JsonArray array = json.getAsJsonArray("procedureLinkId"); 4340 for (int i = 0; i < array.size(); i++) { 4341 if (array.get(i).isJsonNull()) { 4342 res.getProcedureLinkId().add(new PositiveIntType()); 4343 } else { 4344 res.getProcedureLinkId().add(parsePositiveInt(array.get(i).getAsString())); 4345 } 4346 } 4347 }; 4348 if (json.has("_procedureLinkId")) { 4349 JsonArray array = json.getAsJsonArray("_procedureLinkId"); 4350 for (int i = 0; i < array.size(); i++) { 4351 if (i == res.getProcedureLinkId().size()) 4352 res.getProcedureLinkId().add(parsePositiveInt(null)); 4353 if (array.get(i) instanceof JsonObject) 4354 parseElementProperties(array.get(i).getAsJsonObject(), res.getProcedureLinkId().get(i)); 4355 } 4356 }; 4357 if (json.has("informationLinkId")) { 4358 JsonArray array = json.getAsJsonArray("informationLinkId"); 4359 for (int i = 0; i < array.size(); i++) { 4360 if (array.get(i).isJsonNull()) { 4361 res.getInformationLinkId().add(new PositiveIntType()); 4362 } else { 4363 res.getInformationLinkId().add(parsePositiveInt(array.get(i).getAsString())); 4364 } 4365 } 4366 }; 4367 if (json.has("_informationLinkId")) { 4368 JsonArray array = json.getAsJsonArray("_informationLinkId"); 4369 for (int i = 0; i < array.size(); i++) { 4370 if (i == res.getInformationLinkId().size()) 4371 res.getInformationLinkId().add(parsePositiveInt(null)); 4372 if (array.get(i) instanceof JsonObject) 4373 parseElementProperties(array.get(i).getAsJsonObject(), res.getInformationLinkId().get(i)); 4374 } 4375 }; 4376 if (json.has("revenue")) 4377 res.setRevenue(parseCodeableConcept(getJObject(json, "revenue"))); 4378 if (json.has("category")) 4379 res.setCategory(parseCodeableConcept(getJObject(json, "category"))); 4380 if (json.has("service")) 4381 res.setService(parseCodeableConcept(getJObject(json, "service"))); 4382 if (json.has("modifier")) { 4383 JsonArray array = json.getAsJsonArray("modifier"); 4384 for (int i = 0; i < array.size(); i++) { 4385 res.getModifier().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 4386 } 4387 }; 4388 if (json.has("programCode")) { 4389 JsonArray array = json.getAsJsonArray("programCode"); 4390 for (int i = 0; i < array.size(); i++) { 4391 res.getProgramCode().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 4392 } 4393 }; 4394 Type serviced = parseType("serviced", json); 4395 if (serviced != null) 4396 res.setServiced(serviced); 4397 Type location = parseType("location", json); 4398 if (location != null) 4399 res.setLocation(location); 4400 if (json.has("quantity")) 4401 res.setQuantity(parseSimpleQuantity(getJObject(json, "quantity"))); 4402 if (json.has("unitPrice")) 4403 res.setUnitPrice(parseMoney(getJObject(json, "unitPrice"))); 4404 if (json.has("factor")) 4405 res.setFactorElement(parseDecimal(json.get("factor").getAsBigDecimal())); 4406 if (json.has("_factor")) 4407 parseElementProperties(getJObject(json, "_factor"), res.getFactorElement()); 4408 if (json.has("net")) 4409 res.setNet(parseMoney(getJObject(json, "net"))); 4410 if (json.has("udi")) { 4411 JsonArray array = json.getAsJsonArray("udi"); 4412 for (int i = 0; i < array.size(); i++) { 4413 res.getUdi().add(parseReference(array.get(i).getAsJsonObject())); 4414 } 4415 }; 4416 if (json.has("bodySite")) 4417 res.setBodySite(parseCodeableConcept(getJObject(json, "bodySite"))); 4418 if (json.has("subSite")) { 4419 JsonArray array = json.getAsJsonArray("subSite"); 4420 for (int i = 0; i < array.size(); i++) { 4421 res.getSubSite().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 4422 } 4423 }; 4424 if (json.has("encounter")) { 4425 JsonArray array = json.getAsJsonArray("encounter"); 4426 for (int i = 0; i < array.size(); i++) { 4427 res.getEncounter().add(parseReference(array.get(i).getAsJsonObject())); 4428 } 4429 }; 4430 if (json.has("detail")) { 4431 JsonArray array = json.getAsJsonArray("detail"); 4432 for (int i = 0; i < array.size(); i++) { 4433 res.getDetail().add(parseClaimDetailComponent(array.get(i).getAsJsonObject(), owner)); 4434 } 4435 }; 4436 } 4437 4438 protected Claim.DetailComponent parseClaimDetailComponent(JsonObject json, Claim owner) throws IOException, FHIRFormatError { 4439 Claim.DetailComponent res = new Claim.DetailComponent(); 4440 parseClaimDetailComponentProperties(json, owner, res); 4441 return res; 4442 } 4443 4444 protected void parseClaimDetailComponentProperties(JsonObject json, Claim owner, Claim.DetailComponent res) throws IOException, FHIRFormatError { 4445 parseBackboneProperties(json, res); 4446 if (json.has("sequence")) 4447 res.setSequenceElement(parsePositiveInt(json.get("sequence").getAsString())); 4448 if (json.has("_sequence")) 4449 parseElementProperties(getJObject(json, "_sequence"), res.getSequenceElement()); 4450 if (json.has("revenue")) 4451 res.setRevenue(parseCodeableConcept(getJObject(json, "revenue"))); 4452 if (json.has("category")) 4453 res.setCategory(parseCodeableConcept(getJObject(json, "category"))); 4454 if (json.has("service")) 4455 res.setService(parseCodeableConcept(getJObject(json, "service"))); 4456 if (json.has("modifier")) { 4457 JsonArray array = json.getAsJsonArray("modifier"); 4458 for (int i = 0; i < array.size(); i++) { 4459 res.getModifier().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 4460 } 4461 }; 4462 if (json.has("programCode")) { 4463 JsonArray array = json.getAsJsonArray("programCode"); 4464 for (int i = 0; i < array.size(); i++) { 4465 res.getProgramCode().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 4466 } 4467 }; 4468 if (json.has("quantity")) 4469 res.setQuantity(parseSimpleQuantity(getJObject(json, "quantity"))); 4470 if (json.has("unitPrice")) 4471 res.setUnitPrice(parseMoney(getJObject(json, "unitPrice"))); 4472 if (json.has("factor")) 4473 res.setFactorElement(parseDecimal(json.get("factor").getAsBigDecimal())); 4474 if (json.has("_factor")) 4475 parseElementProperties(getJObject(json, "_factor"), res.getFactorElement()); 4476 if (json.has("net")) 4477 res.setNet(parseMoney(getJObject(json, "net"))); 4478 if (json.has("udi")) { 4479 JsonArray array = json.getAsJsonArray("udi"); 4480 for (int i = 0; i < array.size(); i++) { 4481 res.getUdi().add(parseReference(array.get(i).getAsJsonObject())); 4482 } 4483 }; 4484 if (json.has("subDetail")) { 4485 JsonArray array = json.getAsJsonArray("subDetail"); 4486 for (int i = 0; i < array.size(); i++) { 4487 res.getSubDetail().add(parseClaimSubDetailComponent(array.get(i).getAsJsonObject(), owner)); 4488 } 4489 }; 4490 } 4491 4492 protected Claim.SubDetailComponent parseClaimSubDetailComponent(JsonObject json, Claim owner) throws IOException, FHIRFormatError { 4493 Claim.SubDetailComponent res = new Claim.SubDetailComponent(); 4494 parseClaimSubDetailComponentProperties(json, owner, res); 4495 return res; 4496 } 4497 4498 protected void parseClaimSubDetailComponentProperties(JsonObject json, Claim owner, Claim.SubDetailComponent res) throws IOException, FHIRFormatError { 4499 parseBackboneProperties(json, res); 4500 if (json.has("sequence")) 4501 res.setSequenceElement(parsePositiveInt(json.get("sequence").getAsString())); 4502 if (json.has("_sequence")) 4503 parseElementProperties(getJObject(json, "_sequence"), res.getSequenceElement()); 4504 if (json.has("revenue")) 4505 res.setRevenue(parseCodeableConcept(getJObject(json, "revenue"))); 4506 if (json.has("category")) 4507 res.setCategory(parseCodeableConcept(getJObject(json, "category"))); 4508 if (json.has("service")) 4509 res.setService(parseCodeableConcept(getJObject(json, "service"))); 4510 if (json.has("modifier")) { 4511 JsonArray array = json.getAsJsonArray("modifier"); 4512 for (int i = 0; i < array.size(); i++) { 4513 res.getModifier().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 4514 } 4515 }; 4516 if (json.has("programCode")) { 4517 JsonArray array = json.getAsJsonArray("programCode"); 4518 for (int i = 0; i < array.size(); i++) { 4519 res.getProgramCode().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 4520 } 4521 }; 4522 if (json.has("quantity")) 4523 res.setQuantity(parseSimpleQuantity(getJObject(json, "quantity"))); 4524 if (json.has("unitPrice")) 4525 res.setUnitPrice(parseMoney(getJObject(json, "unitPrice"))); 4526 if (json.has("factor")) 4527 res.setFactorElement(parseDecimal(json.get("factor").getAsBigDecimal())); 4528 if (json.has("_factor")) 4529 parseElementProperties(getJObject(json, "_factor"), res.getFactorElement()); 4530 if (json.has("net")) 4531 res.setNet(parseMoney(getJObject(json, "net"))); 4532 if (json.has("udi")) { 4533 JsonArray array = json.getAsJsonArray("udi"); 4534 for (int i = 0; i < array.size(); i++) { 4535 res.getUdi().add(parseReference(array.get(i).getAsJsonObject())); 4536 } 4537 }; 4538 } 4539 4540 protected ClaimResponse parseClaimResponse(JsonObject json) throws IOException, FHIRFormatError { 4541 ClaimResponse res = new ClaimResponse(); 4542 parseClaimResponseProperties(json, res); 4543 return res; 4544 } 4545 4546 protected void parseClaimResponseProperties(JsonObject json, ClaimResponse res) throws IOException, FHIRFormatError { 4547 parseDomainResourceProperties(json, res); 4548 if (json.has("identifier")) { 4549 JsonArray array = json.getAsJsonArray("identifier"); 4550 for (int i = 0; i < array.size(); i++) { 4551 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 4552 } 4553 }; 4554 if (json.has("status")) 4555 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), ClaimResponse.ClaimResponseStatus.NULL, new ClaimResponse.ClaimResponseStatusEnumFactory())); 4556 if (json.has("_status")) 4557 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 4558 if (json.has("patient")) 4559 res.setPatient(parseReference(getJObject(json, "patient"))); 4560 if (json.has("created")) 4561 res.setCreatedElement(parseDateTime(json.get("created").getAsString())); 4562 if (json.has("_created")) 4563 parseElementProperties(getJObject(json, "_created"), res.getCreatedElement()); 4564 if (json.has("insurer")) 4565 res.setInsurer(parseReference(getJObject(json, "insurer"))); 4566 if (json.has("requestProvider")) 4567 res.setRequestProvider(parseReference(getJObject(json, "requestProvider"))); 4568 if (json.has("requestOrganization")) 4569 res.setRequestOrganization(parseReference(getJObject(json, "requestOrganization"))); 4570 if (json.has("request")) 4571 res.setRequest(parseReference(getJObject(json, "request"))); 4572 if (json.has("outcome")) 4573 res.setOutcome(parseCodeableConcept(getJObject(json, "outcome"))); 4574 if (json.has("disposition")) 4575 res.setDispositionElement(parseString(json.get("disposition").getAsString())); 4576 if (json.has("_disposition")) 4577 parseElementProperties(getJObject(json, "_disposition"), res.getDispositionElement()); 4578 if (json.has("payeeType")) 4579 res.setPayeeType(parseCodeableConcept(getJObject(json, "payeeType"))); 4580 if (json.has("item")) { 4581 JsonArray array = json.getAsJsonArray("item"); 4582 for (int i = 0; i < array.size(); i++) { 4583 res.getItem().add(parseClaimResponseItemComponent(array.get(i).getAsJsonObject(), res)); 4584 } 4585 }; 4586 if (json.has("addItem")) { 4587 JsonArray array = json.getAsJsonArray("addItem"); 4588 for (int i = 0; i < array.size(); i++) { 4589 res.getAddItem().add(parseClaimResponseAddedItemComponent(array.get(i).getAsJsonObject(), res)); 4590 } 4591 }; 4592 if (json.has("error")) { 4593 JsonArray array = json.getAsJsonArray("error"); 4594 for (int i = 0; i < array.size(); i++) { 4595 res.getError().add(parseClaimResponseErrorComponent(array.get(i).getAsJsonObject(), res)); 4596 } 4597 }; 4598 if (json.has("totalCost")) 4599 res.setTotalCost(parseMoney(getJObject(json, "totalCost"))); 4600 if (json.has("unallocDeductable")) 4601 res.setUnallocDeductable(parseMoney(getJObject(json, "unallocDeductable"))); 4602 if (json.has("totalBenefit")) 4603 res.setTotalBenefit(parseMoney(getJObject(json, "totalBenefit"))); 4604 if (json.has("payment")) 4605 res.setPayment(parseClaimResponsePaymentComponent(getJObject(json, "payment"), res)); 4606 if (json.has("reserved")) 4607 res.setReserved(parseCoding(getJObject(json, "reserved"))); 4608 if (json.has("form")) 4609 res.setForm(parseCodeableConcept(getJObject(json, "form"))); 4610 if (json.has("processNote")) { 4611 JsonArray array = json.getAsJsonArray("processNote"); 4612 for (int i = 0; i < array.size(); i++) { 4613 res.getProcessNote().add(parseClaimResponseNoteComponent(array.get(i).getAsJsonObject(), res)); 4614 } 4615 }; 4616 if (json.has("communicationRequest")) { 4617 JsonArray array = json.getAsJsonArray("communicationRequest"); 4618 for (int i = 0; i < array.size(); i++) { 4619 res.getCommunicationRequest().add(parseReference(array.get(i).getAsJsonObject())); 4620 } 4621 }; 4622 if (json.has("insurance")) { 4623 JsonArray array = json.getAsJsonArray("insurance"); 4624 for (int i = 0; i < array.size(); i++) { 4625 res.getInsurance().add(parseClaimResponseInsuranceComponent(array.get(i).getAsJsonObject(), res)); 4626 } 4627 }; 4628 } 4629 4630 protected ClaimResponse.ItemComponent parseClaimResponseItemComponent(JsonObject json, ClaimResponse owner) throws IOException, FHIRFormatError { 4631 ClaimResponse.ItemComponent res = new ClaimResponse.ItemComponent(); 4632 parseClaimResponseItemComponentProperties(json, owner, res); 4633 return res; 4634 } 4635 4636 protected void parseClaimResponseItemComponentProperties(JsonObject json, ClaimResponse owner, ClaimResponse.ItemComponent res) throws IOException, FHIRFormatError { 4637 parseBackboneProperties(json, res); 4638 if (json.has("sequenceLinkId")) 4639 res.setSequenceLinkIdElement(parsePositiveInt(json.get("sequenceLinkId").getAsString())); 4640 if (json.has("_sequenceLinkId")) 4641 parseElementProperties(getJObject(json, "_sequenceLinkId"), res.getSequenceLinkIdElement()); 4642 if (json.has("noteNumber")) { 4643 JsonArray array = json.getAsJsonArray("noteNumber"); 4644 for (int i = 0; i < array.size(); i++) { 4645 if (array.get(i).isJsonNull()) { 4646 res.getNoteNumber().add(new PositiveIntType()); 4647 } else { 4648 res.getNoteNumber().add(parsePositiveInt(array.get(i).getAsString())); 4649 } 4650 } 4651 }; 4652 if (json.has("_noteNumber")) { 4653 JsonArray array = json.getAsJsonArray("_noteNumber"); 4654 for (int i = 0; i < array.size(); i++) { 4655 if (i == res.getNoteNumber().size()) 4656 res.getNoteNumber().add(parsePositiveInt(null)); 4657 if (array.get(i) instanceof JsonObject) 4658 parseElementProperties(array.get(i).getAsJsonObject(), res.getNoteNumber().get(i)); 4659 } 4660 }; 4661 if (json.has("adjudication")) { 4662 JsonArray array = json.getAsJsonArray("adjudication"); 4663 for (int i = 0; i < array.size(); i++) { 4664 res.getAdjudication().add(parseClaimResponseAdjudicationComponent(array.get(i).getAsJsonObject(), owner)); 4665 } 4666 }; 4667 if (json.has("detail")) { 4668 JsonArray array = json.getAsJsonArray("detail"); 4669 for (int i = 0; i < array.size(); i++) { 4670 res.getDetail().add(parseClaimResponseItemDetailComponent(array.get(i).getAsJsonObject(), owner)); 4671 } 4672 }; 4673 } 4674 4675 protected ClaimResponse.AdjudicationComponent parseClaimResponseAdjudicationComponent(JsonObject json, ClaimResponse owner) throws IOException, FHIRFormatError { 4676 ClaimResponse.AdjudicationComponent res = new ClaimResponse.AdjudicationComponent(); 4677 parseClaimResponseAdjudicationComponentProperties(json, owner, res); 4678 return res; 4679 } 4680 4681 protected void parseClaimResponseAdjudicationComponentProperties(JsonObject json, ClaimResponse owner, ClaimResponse.AdjudicationComponent res) throws IOException, FHIRFormatError { 4682 parseBackboneProperties(json, res); 4683 if (json.has("category")) 4684 res.setCategory(parseCodeableConcept(getJObject(json, "category"))); 4685 if (json.has("reason")) 4686 res.setReason(parseCodeableConcept(getJObject(json, "reason"))); 4687 if (json.has("amount")) 4688 res.setAmount(parseMoney(getJObject(json, "amount"))); 4689 if (json.has("value")) 4690 res.setValueElement(parseDecimal(json.get("value").getAsBigDecimal())); 4691 if (json.has("_value")) 4692 parseElementProperties(getJObject(json, "_value"), res.getValueElement()); 4693 } 4694 4695 protected ClaimResponse.ItemDetailComponent parseClaimResponseItemDetailComponent(JsonObject json, ClaimResponse owner) throws IOException, FHIRFormatError { 4696 ClaimResponse.ItemDetailComponent res = new ClaimResponse.ItemDetailComponent(); 4697 parseClaimResponseItemDetailComponentProperties(json, owner, res); 4698 return res; 4699 } 4700 4701 protected void parseClaimResponseItemDetailComponentProperties(JsonObject json, ClaimResponse owner, ClaimResponse.ItemDetailComponent res) throws IOException, FHIRFormatError { 4702 parseBackboneProperties(json, res); 4703 if (json.has("sequenceLinkId")) 4704 res.setSequenceLinkIdElement(parsePositiveInt(json.get("sequenceLinkId").getAsString())); 4705 if (json.has("_sequenceLinkId")) 4706 parseElementProperties(getJObject(json, "_sequenceLinkId"), res.getSequenceLinkIdElement()); 4707 if (json.has("noteNumber")) { 4708 JsonArray array = json.getAsJsonArray("noteNumber"); 4709 for (int i = 0; i < array.size(); i++) { 4710 if (array.get(i).isJsonNull()) { 4711 res.getNoteNumber().add(new PositiveIntType()); 4712 } else { 4713 res.getNoteNumber().add(parsePositiveInt(array.get(i).getAsString())); 4714 } 4715 } 4716 }; 4717 if (json.has("_noteNumber")) { 4718 JsonArray array = json.getAsJsonArray("_noteNumber"); 4719 for (int i = 0; i < array.size(); i++) { 4720 if (i == res.getNoteNumber().size()) 4721 res.getNoteNumber().add(parsePositiveInt(null)); 4722 if (array.get(i) instanceof JsonObject) 4723 parseElementProperties(array.get(i).getAsJsonObject(), res.getNoteNumber().get(i)); 4724 } 4725 }; 4726 if (json.has("adjudication")) { 4727 JsonArray array = json.getAsJsonArray("adjudication"); 4728 for (int i = 0; i < array.size(); i++) { 4729 res.getAdjudication().add(parseClaimResponseAdjudicationComponent(array.get(i).getAsJsonObject(), owner)); 4730 } 4731 }; 4732 if (json.has("subDetail")) { 4733 JsonArray array = json.getAsJsonArray("subDetail"); 4734 for (int i = 0; i < array.size(); i++) { 4735 res.getSubDetail().add(parseClaimResponseSubDetailComponent(array.get(i).getAsJsonObject(), owner)); 4736 } 4737 }; 4738 } 4739 4740 protected ClaimResponse.SubDetailComponent parseClaimResponseSubDetailComponent(JsonObject json, ClaimResponse owner) throws IOException, FHIRFormatError { 4741 ClaimResponse.SubDetailComponent res = new ClaimResponse.SubDetailComponent(); 4742 parseClaimResponseSubDetailComponentProperties(json, owner, res); 4743 return res; 4744 } 4745 4746 protected void parseClaimResponseSubDetailComponentProperties(JsonObject json, ClaimResponse owner, ClaimResponse.SubDetailComponent res) throws IOException, FHIRFormatError { 4747 parseBackboneProperties(json, res); 4748 if (json.has("sequenceLinkId")) 4749 res.setSequenceLinkIdElement(parsePositiveInt(json.get("sequenceLinkId").getAsString())); 4750 if (json.has("_sequenceLinkId")) 4751 parseElementProperties(getJObject(json, "_sequenceLinkId"), res.getSequenceLinkIdElement()); 4752 if (json.has("noteNumber")) { 4753 JsonArray array = json.getAsJsonArray("noteNumber"); 4754 for (int i = 0; i < array.size(); i++) { 4755 if (array.get(i).isJsonNull()) { 4756 res.getNoteNumber().add(new PositiveIntType()); 4757 } else { 4758 res.getNoteNumber().add(parsePositiveInt(array.get(i).getAsString())); 4759 } 4760 } 4761 }; 4762 if (json.has("_noteNumber")) { 4763 JsonArray array = json.getAsJsonArray("_noteNumber"); 4764 for (int i = 0; i < array.size(); i++) { 4765 if (i == res.getNoteNumber().size()) 4766 res.getNoteNumber().add(parsePositiveInt(null)); 4767 if (array.get(i) instanceof JsonObject) 4768 parseElementProperties(array.get(i).getAsJsonObject(), res.getNoteNumber().get(i)); 4769 } 4770 }; 4771 if (json.has("adjudication")) { 4772 JsonArray array = json.getAsJsonArray("adjudication"); 4773 for (int i = 0; i < array.size(); i++) { 4774 res.getAdjudication().add(parseClaimResponseAdjudicationComponent(array.get(i).getAsJsonObject(), owner)); 4775 } 4776 }; 4777 } 4778 4779 protected ClaimResponse.AddedItemComponent parseClaimResponseAddedItemComponent(JsonObject json, ClaimResponse owner) throws IOException, FHIRFormatError { 4780 ClaimResponse.AddedItemComponent res = new ClaimResponse.AddedItemComponent(); 4781 parseClaimResponseAddedItemComponentProperties(json, owner, res); 4782 return res; 4783 } 4784 4785 protected void parseClaimResponseAddedItemComponentProperties(JsonObject json, ClaimResponse owner, ClaimResponse.AddedItemComponent res) throws IOException, FHIRFormatError { 4786 parseBackboneProperties(json, res); 4787 if (json.has("sequenceLinkId")) { 4788 JsonArray array = json.getAsJsonArray("sequenceLinkId"); 4789 for (int i = 0; i < array.size(); i++) { 4790 if (array.get(i).isJsonNull()) { 4791 res.getSequenceLinkId().add(new PositiveIntType()); 4792 } else { 4793 res.getSequenceLinkId().add(parsePositiveInt(array.get(i).getAsString())); 4794 } 4795 } 4796 }; 4797 if (json.has("_sequenceLinkId")) { 4798 JsonArray array = json.getAsJsonArray("_sequenceLinkId"); 4799 for (int i = 0; i < array.size(); i++) { 4800 if (i == res.getSequenceLinkId().size()) 4801 res.getSequenceLinkId().add(parsePositiveInt(null)); 4802 if (array.get(i) instanceof JsonObject) 4803 parseElementProperties(array.get(i).getAsJsonObject(), res.getSequenceLinkId().get(i)); 4804 } 4805 }; 4806 if (json.has("revenue")) 4807 res.setRevenue(parseCodeableConcept(getJObject(json, "revenue"))); 4808 if (json.has("category")) 4809 res.setCategory(parseCodeableConcept(getJObject(json, "category"))); 4810 if (json.has("service")) 4811 res.setService(parseCodeableConcept(getJObject(json, "service"))); 4812 if (json.has("modifier")) { 4813 JsonArray array = json.getAsJsonArray("modifier"); 4814 for (int i = 0; i < array.size(); i++) { 4815 res.getModifier().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 4816 } 4817 }; 4818 if (json.has("fee")) 4819 res.setFee(parseMoney(getJObject(json, "fee"))); 4820 if (json.has("noteNumber")) { 4821 JsonArray array = json.getAsJsonArray("noteNumber"); 4822 for (int i = 0; i < array.size(); i++) { 4823 if (array.get(i).isJsonNull()) { 4824 res.getNoteNumber().add(new PositiveIntType()); 4825 } else { 4826 res.getNoteNumber().add(parsePositiveInt(array.get(i).getAsString())); 4827 } 4828 } 4829 }; 4830 if (json.has("_noteNumber")) { 4831 JsonArray array = json.getAsJsonArray("_noteNumber"); 4832 for (int i = 0; i < array.size(); i++) { 4833 if (i == res.getNoteNumber().size()) 4834 res.getNoteNumber().add(parsePositiveInt(null)); 4835 if (array.get(i) instanceof JsonObject) 4836 parseElementProperties(array.get(i).getAsJsonObject(), res.getNoteNumber().get(i)); 4837 } 4838 }; 4839 if (json.has("adjudication")) { 4840 JsonArray array = json.getAsJsonArray("adjudication"); 4841 for (int i = 0; i < array.size(); i++) { 4842 res.getAdjudication().add(parseClaimResponseAdjudicationComponent(array.get(i).getAsJsonObject(), owner)); 4843 } 4844 }; 4845 if (json.has("detail")) { 4846 JsonArray array = json.getAsJsonArray("detail"); 4847 for (int i = 0; i < array.size(); i++) { 4848 res.getDetail().add(parseClaimResponseAddedItemsDetailComponent(array.get(i).getAsJsonObject(), owner)); 4849 } 4850 }; 4851 } 4852 4853 protected ClaimResponse.AddedItemsDetailComponent parseClaimResponseAddedItemsDetailComponent(JsonObject json, ClaimResponse owner) throws IOException, FHIRFormatError { 4854 ClaimResponse.AddedItemsDetailComponent res = new ClaimResponse.AddedItemsDetailComponent(); 4855 parseClaimResponseAddedItemsDetailComponentProperties(json, owner, res); 4856 return res; 4857 } 4858 4859 protected void parseClaimResponseAddedItemsDetailComponentProperties(JsonObject json, ClaimResponse owner, ClaimResponse.AddedItemsDetailComponent res) throws IOException, FHIRFormatError { 4860 parseBackboneProperties(json, res); 4861 if (json.has("revenue")) 4862 res.setRevenue(parseCodeableConcept(getJObject(json, "revenue"))); 4863 if (json.has("category")) 4864 res.setCategory(parseCodeableConcept(getJObject(json, "category"))); 4865 if (json.has("service")) 4866 res.setService(parseCodeableConcept(getJObject(json, "service"))); 4867 if (json.has("modifier")) { 4868 JsonArray array = json.getAsJsonArray("modifier"); 4869 for (int i = 0; i < array.size(); i++) { 4870 res.getModifier().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 4871 } 4872 }; 4873 if (json.has("fee")) 4874 res.setFee(parseMoney(getJObject(json, "fee"))); 4875 if (json.has("noteNumber")) { 4876 JsonArray array = json.getAsJsonArray("noteNumber"); 4877 for (int i = 0; i < array.size(); i++) { 4878 if (array.get(i).isJsonNull()) { 4879 res.getNoteNumber().add(new PositiveIntType()); 4880 } else { 4881 res.getNoteNumber().add(parsePositiveInt(array.get(i).getAsString())); 4882 } 4883 } 4884 }; 4885 if (json.has("_noteNumber")) { 4886 JsonArray array = json.getAsJsonArray("_noteNumber"); 4887 for (int i = 0; i < array.size(); i++) { 4888 if (i == res.getNoteNumber().size()) 4889 res.getNoteNumber().add(parsePositiveInt(null)); 4890 if (array.get(i) instanceof JsonObject) 4891 parseElementProperties(array.get(i).getAsJsonObject(), res.getNoteNumber().get(i)); 4892 } 4893 }; 4894 if (json.has("adjudication")) { 4895 JsonArray array = json.getAsJsonArray("adjudication"); 4896 for (int i = 0; i < array.size(); i++) { 4897 res.getAdjudication().add(parseClaimResponseAdjudicationComponent(array.get(i).getAsJsonObject(), owner)); 4898 } 4899 }; 4900 } 4901 4902 protected ClaimResponse.ErrorComponent parseClaimResponseErrorComponent(JsonObject json, ClaimResponse owner) throws IOException, FHIRFormatError { 4903 ClaimResponse.ErrorComponent res = new ClaimResponse.ErrorComponent(); 4904 parseClaimResponseErrorComponentProperties(json, owner, res); 4905 return res; 4906 } 4907 4908 protected void parseClaimResponseErrorComponentProperties(JsonObject json, ClaimResponse owner, ClaimResponse.ErrorComponent res) throws IOException, FHIRFormatError { 4909 parseBackboneProperties(json, res); 4910 if (json.has("sequenceLinkId")) 4911 res.setSequenceLinkIdElement(parsePositiveInt(json.get("sequenceLinkId").getAsString())); 4912 if (json.has("_sequenceLinkId")) 4913 parseElementProperties(getJObject(json, "_sequenceLinkId"), res.getSequenceLinkIdElement()); 4914 if (json.has("detailSequenceLinkId")) 4915 res.setDetailSequenceLinkIdElement(parsePositiveInt(json.get("detailSequenceLinkId").getAsString())); 4916 if (json.has("_detailSequenceLinkId")) 4917 parseElementProperties(getJObject(json, "_detailSequenceLinkId"), res.getDetailSequenceLinkIdElement()); 4918 if (json.has("subdetailSequenceLinkId")) 4919 res.setSubdetailSequenceLinkIdElement(parsePositiveInt(json.get("subdetailSequenceLinkId").getAsString())); 4920 if (json.has("_subdetailSequenceLinkId")) 4921 parseElementProperties(getJObject(json, "_subdetailSequenceLinkId"), res.getSubdetailSequenceLinkIdElement()); 4922 if (json.has("code")) 4923 res.setCode(parseCodeableConcept(getJObject(json, "code"))); 4924 } 4925 4926 protected ClaimResponse.PaymentComponent parseClaimResponsePaymentComponent(JsonObject json, ClaimResponse owner) throws IOException, FHIRFormatError { 4927 ClaimResponse.PaymentComponent res = new ClaimResponse.PaymentComponent(); 4928 parseClaimResponsePaymentComponentProperties(json, owner, res); 4929 return res; 4930 } 4931 4932 protected void parseClaimResponsePaymentComponentProperties(JsonObject json, ClaimResponse owner, ClaimResponse.PaymentComponent res) throws IOException, FHIRFormatError { 4933 parseBackboneProperties(json, res); 4934 if (json.has("type")) 4935 res.setType(parseCodeableConcept(getJObject(json, "type"))); 4936 if (json.has("adjustment")) 4937 res.setAdjustment(parseMoney(getJObject(json, "adjustment"))); 4938 if (json.has("adjustmentReason")) 4939 res.setAdjustmentReason(parseCodeableConcept(getJObject(json, "adjustmentReason"))); 4940 if (json.has("date")) 4941 res.setDateElement(parseDate(json.get("date").getAsString())); 4942 if (json.has("_date")) 4943 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 4944 if (json.has("amount")) 4945 res.setAmount(parseMoney(getJObject(json, "amount"))); 4946 if (json.has("identifier")) 4947 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 4948 } 4949 4950 protected ClaimResponse.NoteComponent parseClaimResponseNoteComponent(JsonObject json, ClaimResponse owner) throws IOException, FHIRFormatError { 4951 ClaimResponse.NoteComponent res = new ClaimResponse.NoteComponent(); 4952 parseClaimResponseNoteComponentProperties(json, owner, res); 4953 return res; 4954 } 4955 4956 protected void parseClaimResponseNoteComponentProperties(JsonObject json, ClaimResponse owner, ClaimResponse.NoteComponent res) throws IOException, FHIRFormatError { 4957 parseBackboneProperties(json, res); 4958 if (json.has("number")) 4959 res.setNumberElement(parsePositiveInt(json.get("number").getAsString())); 4960 if (json.has("_number")) 4961 parseElementProperties(getJObject(json, "_number"), res.getNumberElement()); 4962 if (json.has("type")) 4963 res.setType(parseCodeableConcept(getJObject(json, "type"))); 4964 if (json.has("text")) 4965 res.setTextElement(parseString(json.get("text").getAsString())); 4966 if (json.has("_text")) 4967 parseElementProperties(getJObject(json, "_text"), res.getTextElement()); 4968 if (json.has("language")) 4969 res.setLanguage(parseCodeableConcept(getJObject(json, "language"))); 4970 } 4971 4972 protected ClaimResponse.InsuranceComponent parseClaimResponseInsuranceComponent(JsonObject json, ClaimResponse owner) throws IOException, FHIRFormatError { 4973 ClaimResponse.InsuranceComponent res = new ClaimResponse.InsuranceComponent(); 4974 parseClaimResponseInsuranceComponentProperties(json, owner, res); 4975 return res; 4976 } 4977 4978 protected void parseClaimResponseInsuranceComponentProperties(JsonObject json, ClaimResponse owner, ClaimResponse.InsuranceComponent res) throws IOException, FHIRFormatError { 4979 parseBackboneProperties(json, res); 4980 if (json.has("sequence")) 4981 res.setSequenceElement(parsePositiveInt(json.get("sequence").getAsString())); 4982 if (json.has("_sequence")) 4983 parseElementProperties(getJObject(json, "_sequence"), res.getSequenceElement()); 4984 if (json.has("focal")) 4985 res.setFocalElement(parseBoolean(json.get("focal").getAsBoolean())); 4986 if (json.has("_focal")) 4987 parseElementProperties(getJObject(json, "_focal"), res.getFocalElement()); 4988 if (json.has("coverage")) 4989 res.setCoverage(parseReference(getJObject(json, "coverage"))); 4990 if (json.has("businessArrangement")) 4991 res.setBusinessArrangementElement(parseString(json.get("businessArrangement").getAsString())); 4992 if (json.has("_businessArrangement")) 4993 parseElementProperties(getJObject(json, "_businessArrangement"), res.getBusinessArrangementElement()); 4994 if (json.has("preAuthRef")) { 4995 JsonArray array = json.getAsJsonArray("preAuthRef"); 4996 for (int i = 0; i < array.size(); i++) { 4997 if (array.get(i).isJsonNull()) { 4998 res.getPreAuthRef().add(new StringType()); 4999 } else { 5000 res.getPreAuthRef().add(parseString(array.get(i).getAsString())); 5001 } 5002 } 5003 }; 5004 if (json.has("_preAuthRef")) { 5005 JsonArray array = json.getAsJsonArray("_preAuthRef"); 5006 for (int i = 0; i < array.size(); i++) { 5007 if (i == res.getPreAuthRef().size()) 5008 res.getPreAuthRef().add(parseString(null)); 5009 if (array.get(i) instanceof JsonObject) 5010 parseElementProperties(array.get(i).getAsJsonObject(), res.getPreAuthRef().get(i)); 5011 } 5012 }; 5013 if (json.has("claimResponse")) 5014 res.setClaimResponse(parseReference(getJObject(json, "claimResponse"))); 5015 } 5016 5017 protected ClinicalImpression parseClinicalImpression(JsonObject json) throws IOException, FHIRFormatError { 5018 ClinicalImpression res = new ClinicalImpression(); 5019 parseClinicalImpressionProperties(json, res); 5020 return res; 5021 } 5022 5023 protected void parseClinicalImpressionProperties(JsonObject json, ClinicalImpression res) throws IOException, FHIRFormatError { 5024 parseDomainResourceProperties(json, res); 5025 if (json.has("identifier")) { 5026 JsonArray array = json.getAsJsonArray("identifier"); 5027 for (int i = 0; i < array.size(); i++) { 5028 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 5029 } 5030 }; 5031 if (json.has("status")) 5032 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), ClinicalImpression.ClinicalImpressionStatus.NULL, new ClinicalImpression.ClinicalImpressionStatusEnumFactory())); 5033 if (json.has("_status")) 5034 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 5035 if (json.has("code")) 5036 res.setCode(parseCodeableConcept(getJObject(json, "code"))); 5037 if (json.has("description")) 5038 res.setDescriptionElement(parseString(json.get("description").getAsString())); 5039 if (json.has("_description")) 5040 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 5041 if (json.has("subject")) 5042 res.setSubject(parseReference(getJObject(json, "subject"))); 5043 if (json.has("context")) 5044 res.setContext(parseReference(getJObject(json, "context"))); 5045 Type effective = parseType("effective", json); 5046 if (effective != null) 5047 res.setEffective(effective); 5048 if (json.has("date")) 5049 res.setDateElement(parseDateTime(json.get("date").getAsString())); 5050 if (json.has("_date")) 5051 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 5052 if (json.has("assessor")) 5053 res.setAssessor(parseReference(getJObject(json, "assessor"))); 5054 if (json.has("previous")) 5055 res.setPrevious(parseReference(getJObject(json, "previous"))); 5056 if (json.has("problem")) { 5057 JsonArray array = json.getAsJsonArray("problem"); 5058 for (int i = 0; i < array.size(); i++) { 5059 res.getProblem().add(parseReference(array.get(i).getAsJsonObject())); 5060 } 5061 }; 5062 if (json.has("investigation")) { 5063 JsonArray array = json.getAsJsonArray("investigation"); 5064 for (int i = 0; i < array.size(); i++) { 5065 res.getInvestigation().add(parseClinicalImpressionClinicalImpressionInvestigationComponent(array.get(i).getAsJsonObject(), res)); 5066 } 5067 }; 5068 if (json.has("protocol")) { 5069 JsonArray array = json.getAsJsonArray("protocol"); 5070 for (int i = 0; i < array.size(); i++) { 5071 if (array.get(i).isJsonNull()) { 5072 res.getProtocol().add(new UriType()); 5073 } else { 5074 res.getProtocol().add(parseUri(array.get(i).getAsString())); 5075 } 5076 } 5077 }; 5078 if (json.has("_protocol")) { 5079 JsonArray array = json.getAsJsonArray("_protocol"); 5080 for (int i = 0; i < array.size(); i++) { 5081 if (i == res.getProtocol().size()) 5082 res.getProtocol().add(parseUri(null)); 5083 if (array.get(i) instanceof JsonObject) 5084 parseElementProperties(array.get(i).getAsJsonObject(), res.getProtocol().get(i)); 5085 } 5086 }; 5087 if (json.has("summary")) 5088 res.setSummaryElement(parseString(json.get("summary").getAsString())); 5089 if (json.has("_summary")) 5090 parseElementProperties(getJObject(json, "_summary"), res.getSummaryElement()); 5091 if (json.has("finding")) { 5092 JsonArray array = json.getAsJsonArray("finding"); 5093 for (int i = 0; i < array.size(); i++) { 5094 res.getFinding().add(parseClinicalImpressionClinicalImpressionFindingComponent(array.get(i).getAsJsonObject(), res)); 5095 } 5096 }; 5097 if (json.has("prognosisCodeableConcept")) { 5098 JsonArray array = json.getAsJsonArray("prognosisCodeableConcept"); 5099 for (int i = 0; i < array.size(); i++) { 5100 res.getPrognosisCodeableConcept().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 5101 } 5102 }; 5103 if (json.has("prognosisReference")) { 5104 JsonArray array = json.getAsJsonArray("prognosisReference"); 5105 for (int i = 0; i < array.size(); i++) { 5106 res.getPrognosisReference().add(parseReference(array.get(i).getAsJsonObject())); 5107 } 5108 }; 5109 if (json.has("action")) { 5110 JsonArray array = json.getAsJsonArray("action"); 5111 for (int i = 0; i < array.size(); i++) { 5112 res.getAction().add(parseReference(array.get(i).getAsJsonObject())); 5113 } 5114 }; 5115 if (json.has("note")) { 5116 JsonArray array = json.getAsJsonArray("note"); 5117 for (int i = 0; i < array.size(); i++) { 5118 res.getNote().add(parseAnnotation(array.get(i).getAsJsonObject())); 5119 } 5120 }; 5121 } 5122 5123 protected ClinicalImpression.ClinicalImpressionInvestigationComponent parseClinicalImpressionClinicalImpressionInvestigationComponent(JsonObject json, ClinicalImpression owner) throws IOException, FHIRFormatError { 5124 ClinicalImpression.ClinicalImpressionInvestigationComponent res = new ClinicalImpression.ClinicalImpressionInvestigationComponent(); 5125 parseClinicalImpressionClinicalImpressionInvestigationComponentProperties(json, owner, res); 5126 return res; 5127 } 5128 5129 protected void parseClinicalImpressionClinicalImpressionInvestigationComponentProperties(JsonObject json, ClinicalImpression owner, ClinicalImpression.ClinicalImpressionInvestigationComponent res) throws IOException, FHIRFormatError { 5130 parseBackboneProperties(json, res); 5131 if (json.has("code")) 5132 res.setCode(parseCodeableConcept(getJObject(json, "code"))); 5133 if (json.has("item")) { 5134 JsonArray array = json.getAsJsonArray("item"); 5135 for (int i = 0; i < array.size(); i++) { 5136 res.getItem().add(parseReference(array.get(i).getAsJsonObject())); 5137 } 5138 }; 5139 } 5140 5141 protected ClinicalImpression.ClinicalImpressionFindingComponent parseClinicalImpressionClinicalImpressionFindingComponent(JsonObject json, ClinicalImpression owner) throws IOException, FHIRFormatError { 5142 ClinicalImpression.ClinicalImpressionFindingComponent res = new ClinicalImpression.ClinicalImpressionFindingComponent(); 5143 parseClinicalImpressionClinicalImpressionFindingComponentProperties(json, owner, res); 5144 return res; 5145 } 5146 5147 protected void parseClinicalImpressionClinicalImpressionFindingComponentProperties(JsonObject json, ClinicalImpression owner, ClinicalImpression.ClinicalImpressionFindingComponent res) throws IOException, FHIRFormatError { 5148 parseBackboneProperties(json, res); 5149 Type item = parseType("item", json); 5150 if (item != null) 5151 res.setItem(item); 5152 if (json.has("basis")) 5153 res.setBasisElement(parseString(json.get("basis").getAsString())); 5154 if (json.has("_basis")) 5155 parseElementProperties(getJObject(json, "_basis"), res.getBasisElement()); 5156 } 5157 5158 protected CodeSystem parseCodeSystem(JsonObject json) throws IOException, FHIRFormatError { 5159 CodeSystem res = new CodeSystem(); 5160 parseCodeSystemProperties(json, res); 5161 return res; 5162 } 5163 5164 protected void parseCodeSystemProperties(JsonObject json, CodeSystem res) throws IOException, FHIRFormatError { 5165 parseDomainResourceProperties(json, res); 5166 if (json.has("url")) 5167 res.setUrlElement(parseUri(json.get("url").getAsString())); 5168 if (json.has("_url")) 5169 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 5170 if (json.has("identifier")) 5171 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 5172 if (json.has("version")) 5173 res.setVersionElement(parseString(json.get("version").getAsString())); 5174 if (json.has("_version")) 5175 parseElementProperties(getJObject(json, "_version"), res.getVersionElement()); 5176 if (json.has("name")) 5177 res.setNameElement(parseString(json.get("name").getAsString())); 5178 if (json.has("_name")) 5179 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 5180 if (json.has("title")) 5181 res.setTitleElement(parseString(json.get("title").getAsString())); 5182 if (json.has("_title")) 5183 parseElementProperties(getJObject(json, "_title"), res.getTitleElement()); 5184 if (json.has("status")) 5185 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Enumerations.PublicationStatus.NULL, new Enumerations.PublicationStatusEnumFactory())); 5186 if (json.has("_status")) 5187 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 5188 if (json.has("experimental")) 5189 res.setExperimentalElement(parseBoolean(json.get("experimental").getAsBoolean())); 5190 if (json.has("_experimental")) 5191 parseElementProperties(getJObject(json, "_experimental"), res.getExperimentalElement()); 5192 if (json.has("date")) 5193 res.setDateElement(parseDateTime(json.get("date").getAsString())); 5194 if (json.has("_date")) 5195 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 5196 if (json.has("publisher")) 5197 res.setPublisherElement(parseString(json.get("publisher").getAsString())); 5198 if (json.has("_publisher")) 5199 parseElementProperties(getJObject(json, "_publisher"), res.getPublisherElement()); 5200 if (json.has("contact")) { 5201 JsonArray array = json.getAsJsonArray("contact"); 5202 for (int i = 0; i < array.size(); i++) { 5203 res.getContact().add(parseContactDetail(array.get(i).getAsJsonObject())); 5204 } 5205 }; 5206 if (json.has("description")) 5207 res.setDescriptionElement(parseMarkdown(json.get("description").getAsString())); 5208 if (json.has("_description")) 5209 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 5210 if (json.has("useContext")) { 5211 JsonArray array = json.getAsJsonArray("useContext"); 5212 for (int i = 0; i < array.size(); i++) { 5213 res.getUseContext().add(parseUsageContext(array.get(i).getAsJsonObject())); 5214 } 5215 }; 5216 if (json.has("jurisdiction")) { 5217 JsonArray array = json.getAsJsonArray("jurisdiction"); 5218 for (int i = 0; i < array.size(); i++) { 5219 res.getJurisdiction().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 5220 } 5221 }; 5222 if (json.has("purpose")) 5223 res.setPurposeElement(parseMarkdown(json.get("purpose").getAsString())); 5224 if (json.has("_purpose")) 5225 parseElementProperties(getJObject(json, "_purpose"), res.getPurposeElement()); 5226 if (json.has("copyright")) 5227 res.setCopyrightElement(parseMarkdown(json.get("copyright").getAsString())); 5228 if (json.has("_copyright")) 5229 parseElementProperties(getJObject(json, "_copyright"), res.getCopyrightElement()); 5230 if (json.has("caseSensitive")) 5231 res.setCaseSensitiveElement(parseBoolean(json.get("caseSensitive").getAsBoolean())); 5232 if (json.has("_caseSensitive")) 5233 parseElementProperties(getJObject(json, "_caseSensitive"), res.getCaseSensitiveElement()); 5234 if (json.has("valueSet")) 5235 res.setValueSetElement(parseUri(json.get("valueSet").getAsString())); 5236 if (json.has("_valueSet")) 5237 parseElementProperties(getJObject(json, "_valueSet"), res.getValueSetElement()); 5238 if (json.has("hierarchyMeaning")) 5239 res.setHierarchyMeaningElement(parseEnumeration(json.get("hierarchyMeaning").getAsString(), CodeSystem.CodeSystemHierarchyMeaning.NULL, new CodeSystem.CodeSystemHierarchyMeaningEnumFactory())); 5240 if (json.has("_hierarchyMeaning")) 5241 parseElementProperties(getJObject(json, "_hierarchyMeaning"), res.getHierarchyMeaningElement()); 5242 if (json.has("compositional")) 5243 res.setCompositionalElement(parseBoolean(json.get("compositional").getAsBoolean())); 5244 if (json.has("_compositional")) 5245 parseElementProperties(getJObject(json, "_compositional"), res.getCompositionalElement()); 5246 if (json.has("versionNeeded")) 5247 res.setVersionNeededElement(parseBoolean(json.get("versionNeeded").getAsBoolean())); 5248 if (json.has("_versionNeeded")) 5249 parseElementProperties(getJObject(json, "_versionNeeded"), res.getVersionNeededElement()); 5250 if (json.has("content")) 5251 res.setContentElement(parseEnumeration(json.get("content").getAsString(), CodeSystem.CodeSystemContentMode.NULL, new CodeSystem.CodeSystemContentModeEnumFactory())); 5252 if (json.has("_content")) 5253 parseElementProperties(getJObject(json, "_content"), res.getContentElement()); 5254 if (json.has("count")) 5255 res.setCountElement(parseUnsignedInt(json.get("count").getAsString())); 5256 if (json.has("_count")) 5257 parseElementProperties(getJObject(json, "_count"), res.getCountElement()); 5258 if (json.has("filter")) { 5259 JsonArray array = json.getAsJsonArray("filter"); 5260 for (int i = 0; i < array.size(); i++) { 5261 res.getFilter().add(parseCodeSystemCodeSystemFilterComponent(array.get(i).getAsJsonObject(), res)); 5262 } 5263 }; 5264 if (json.has("property")) { 5265 JsonArray array = json.getAsJsonArray("property"); 5266 for (int i = 0; i < array.size(); i++) { 5267 res.getProperty().add(parseCodeSystemPropertyComponent(array.get(i).getAsJsonObject(), res)); 5268 } 5269 }; 5270 if (json.has("concept")) { 5271 JsonArray array = json.getAsJsonArray("concept"); 5272 for (int i = 0; i < array.size(); i++) { 5273 res.getConcept().add(parseCodeSystemConceptDefinitionComponent(array.get(i).getAsJsonObject(), res)); 5274 } 5275 }; 5276 } 5277 5278 protected CodeSystem.CodeSystemFilterComponent parseCodeSystemCodeSystemFilterComponent(JsonObject json, CodeSystem owner) throws IOException, FHIRFormatError { 5279 CodeSystem.CodeSystemFilterComponent res = new CodeSystem.CodeSystemFilterComponent(); 5280 parseCodeSystemCodeSystemFilterComponentProperties(json, owner, res); 5281 return res; 5282 } 5283 5284 protected void parseCodeSystemCodeSystemFilterComponentProperties(JsonObject json, CodeSystem owner, CodeSystem.CodeSystemFilterComponent res) throws IOException, FHIRFormatError { 5285 parseBackboneProperties(json, res); 5286 if (json.has("code")) 5287 res.setCodeElement(parseCode(json.get("code").getAsString())); 5288 if (json.has("_code")) 5289 parseElementProperties(getJObject(json, "_code"), res.getCodeElement()); 5290 if (json.has("description")) 5291 res.setDescriptionElement(parseString(json.get("description").getAsString())); 5292 if (json.has("_description")) 5293 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 5294 if (json.has("operator")) { 5295 JsonArray array = json.getAsJsonArray("operator"); 5296 for (int i = 0; i < array.size(); i++) { 5297 if (array.get(i).isJsonNull()) { 5298 res.getOperator().add(new Enumeration<CodeSystem.FilterOperator>()); 5299 } else { 5300 res.getOperator().add(parseEnumeration(array.get(i).getAsString(), CodeSystem.FilterOperator.NULL, new CodeSystem.FilterOperatorEnumFactory())); 5301 } 5302 } 5303 }; 5304 if (json.has("_operator")) { 5305 JsonArray array = json.getAsJsonArray("_operator"); 5306 for (int i = 0; i < array.size(); i++) { 5307 if (i == res.getOperator().size()) 5308 res.getOperator().add(parseEnumeration(null, CodeSystem.FilterOperator.NULL, new CodeSystem.FilterOperatorEnumFactory())); 5309 if (array.get(i) instanceof JsonObject) 5310 parseElementProperties(array.get(i).getAsJsonObject(), res.getOperator().get(i)); 5311 } 5312 }; 5313 if (json.has("value")) 5314 res.setValueElement(parseString(json.get("value").getAsString())); 5315 if (json.has("_value")) 5316 parseElementProperties(getJObject(json, "_value"), res.getValueElement()); 5317 } 5318 5319 protected CodeSystem.PropertyComponent parseCodeSystemPropertyComponent(JsonObject json, CodeSystem owner) throws IOException, FHIRFormatError { 5320 CodeSystem.PropertyComponent res = new CodeSystem.PropertyComponent(); 5321 parseCodeSystemPropertyComponentProperties(json, owner, res); 5322 return res; 5323 } 5324 5325 protected void parseCodeSystemPropertyComponentProperties(JsonObject json, CodeSystem owner, CodeSystem.PropertyComponent res) throws IOException, FHIRFormatError { 5326 parseBackboneProperties(json, res); 5327 if (json.has("code")) 5328 res.setCodeElement(parseCode(json.get("code").getAsString())); 5329 if (json.has("_code")) 5330 parseElementProperties(getJObject(json, "_code"), res.getCodeElement()); 5331 if (json.has("uri")) 5332 res.setUriElement(parseUri(json.get("uri").getAsString())); 5333 if (json.has("_uri")) 5334 parseElementProperties(getJObject(json, "_uri"), res.getUriElement()); 5335 if (json.has("description")) 5336 res.setDescriptionElement(parseString(json.get("description").getAsString())); 5337 if (json.has("_description")) 5338 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 5339 if (json.has("type")) 5340 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), CodeSystem.PropertyType.NULL, new CodeSystem.PropertyTypeEnumFactory())); 5341 if (json.has("_type")) 5342 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 5343 } 5344 5345 protected CodeSystem.ConceptDefinitionComponent parseCodeSystemConceptDefinitionComponent(JsonObject json, CodeSystem owner) throws IOException, FHIRFormatError { 5346 CodeSystem.ConceptDefinitionComponent res = new CodeSystem.ConceptDefinitionComponent(); 5347 parseCodeSystemConceptDefinitionComponentProperties(json, owner, res); 5348 return res; 5349 } 5350 5351 protected void parseCodeSystemConceptDefinitionComponentProperties(JsonObject json, CodeSystem owner, CodeSystem.ConceptDefinitionComponent res) throws IOException, FHIRFormatError { 5352 parseBackboneProperties(json, res); 5353 if (json.has("code")) 5354 res.setCodeElement(parseCode(json.get("code").getAsString())); 5355 if (json.has("_code")) 5356 parseElementProperties(getJObject(json, "_code"), res.getCodeElement()); 5357 if (json.has("display")) 5358 res.setDisplayElement(parseString(json.get("display").getAsString())); 5359 if (json.has("_display")) 5360 parseElementProperties(getJObject(json, "_display"), res.getDisplayElement()); 5361 if (json.has("definition")) 5362 res.setDefinitionElement(parseString(json.get("definition").getAsString())); 5363 if (json.has("_definition")) 5364 parseElementProperties(getJObject(json, "_definition"), res.getDefinitionElement()); 5365 if (json.has("designation")) { 5366 JsonArray array = json.getAsJsonArray("designation"); 5367 for (int i = 0; i < array.size(); i++) { 5368 res.getDesignation().add(parseCodeSystemConceptDefinitionDesignationComponent(array.get(i).getAsJsonObject(), owner)); 5369 } 5370 }; 5371 if (json.has("property")) { 5372 JsonArray array = json.getAsJsonArray("property"); 5373 for (int i = 0; i < array.size(); i++) { 5374 res.getProperty().add(parseCodeSystemConceptPropertyComponent(array.get(i).getAsJsonObject(), owner)); 5375 } 5376 }; 5377 if (json.has("concept")) { 5378 JsonArray array = json.getAsJsonArray("concept"); 5379 for (int i = 0; i < array.size(); i++) { 5380 res.getConcept().add(parseCodeSystemConceptDefinitionComponent(array.get(i).getAsJsonObject(), owner)); 5381 } 5382 }; 5383 } 5384 5385 protected CodeSystem.ConceptDefinitionDesignationComponent parseCodeSystemConceptDefinitionDesignationComponent(JsonObject json, CodeSystem owner) throws IOException, FHIRFormatError { 5386 CodeSystem.ConceptDefinitionDesignationComponent res = new CodeSystem.ConceptDefinitionDesignationComponent(); 5387 parseCodeSystemConceptDefinitionDesignationComponentProperties(json, owner, res); 5388 return res; 5389 } 5390 5391 protected void parseCodeSystemConceptDefinitionDesignationComponentProperties(JsonObject json, CodeSystem owner, CodeSystem.ConceptDefinitionDesignationComponent res) throws IOException, FHIRFormatError { 5392 parseBackboneProperties(json, res); 5393 if (json.has("language")) 5394 res.setLanguageElement(parseCode(json.get("language").getAsString())); 5395 if (json.has("_language")) 5396 parseElementProperties(getJObject(json, "_language"), res.getLanguageElement()); 5397 if (json.has("use")) 5398 res.setUse(parseCoding(getJObject(json, "use"))); 5399 if (json.has("value")) 5400 res.setValueElement(parseString(json.get("value").getAsString())); 5401 if (json.has("_value")) 5402 parseElementProperties(getJObject(json, "_value"), res.getValueElement()); 5403 } 5404 5405 protected CodeSystem.ConceptPropertyComponent parseCodeSystemConceptPropertyComponent(JsonObject json, CodeSystem owner) throws IOException, FHIRFormatError { 5406 CodeSystem.ConceptPropertyComponent res = new CodeSystem.ConceptPropertyComponent(); 5407 parseCodeSystemConceptPropertyComponentProperties(json, owner, res); 5408 return res; 5409 } 5410 5411 protected void parseCodeSystemConceptPropertyComponentProperties(JsonObject json, CodeSystem owner, CodeSystem.ConceptPropertyComponent res) throws IOException, FHIRFormatError { 5412 parseBackboneProperties(json, res); 5413 if (json.has("code")) 5414 res.setCodeElement(parseCode(json.get("code").getAsString())); 5415 if (json.has("_code")) 5416 parseElementProperties(getJObject(json, "_code"), res.getCodeElement()); 5417 Type value = parseType("value", json); 5418 if (value != null) 5419 res.setValue(value); 5420 } 5421 5422 protected Communication parseCommunication(JsonObject json) throws IOException, FHIRFormatError { 5423 Communication res = new Communication(); 5424 parseCommunicationProperties(json, res); 5425 return res; 5426 } 5427 5428 protected void parseCommunicationProperties(JsonObject json, Communication res) throws IOException, FHIRFormatError { 5429 parseDomainResourceProperties(json, res); 5430 if (json.has("identifier")) { 5431 JsonArray array = json.getAsJsonArray("identifier"); 5432 for (int i = 0; i < array.size(); i++) { 5433 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 5434 } 5435 }; 5436 if (json.has("definition")) { 5437 JsonArray array = json.getAsJsonArray("definition"); 5438 for (int i = 0; i < array.size(); i++) { 5439 res.getDefinition().add(parseReference(array.get(i).getAsJsonObject())); 5440 } 5441 }; 5442 if (json.has("basedOn")) { 5443 JsonArray array = json.getAsJsonArray("basedOn"); 5444 for (int i = 0; i < array.size(); i++) { 5445 res.getBasedOn().add(parseReference(array.get(i).getAsJsonObject())); 5446 } 5447 }; 5448 if (json.has("partOf")) { 5449 JsonArray array = json.getAsJsonArray("partOf"); 5450 for (int i = 0; i < array.size(); i++) { 5451 res.getPartOf().add(parseReference(array.get(i).getAsJsonObject())); 5452 } 5453 }; 5454 if (json.has("status")) 5455 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Communication.CommunicationStatus.NULL, new Communication.CommunicationStatusEnumFactory())); 5456 if (json.has("_status")) 5457 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 5458 if (json.has("notDone")) 5459 res.setNotDoneElement(parseBoolean(json.get("notDone").getAsBoolean())); 5460 if (json.has("_notDone")) 5461 parseElementProperties(getJObject(json, "_notDone"), res.getNotDoneElement()); 5462 if (json.has("notDoneReason")) 5463 res.setNotDoneReason(parseCodeableConcept(getJObject(json, "notDoneReason"))); 5464 if (json.has("category")) { 5465 JsonArray array = json.getAsJsonArray("category"); 5466 for (int i = 0; i < array.size(); i++) { 5467 res.getCategory().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 5468 } 5469 }; 5470 if (json.has("medium")) { 5471 JsonArray array = json.getAsJsonArray("medium"); 5472 for (int i = 0; i < array.size(); i++) { 5473 res.getMedium().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 5474 } 5475 }; 5476 if (json.has("subject")) 5477 res.setSubject(parseReference(getJObject(json, "subject"))); 5478 if (json.has("recipient")) { 5479 JsonArray array = json.getAsJsonArray("recipient"); 5480 for (int i = 0; i < array.size(); i++) { 5481 res.getRecipient().add(parseReference(array.get(i).getAsJsonObject())); 5482 } 5483 }; 5484 if (json.has("topic")) { 5485 JsonArray array = json.getAsJsonArray("topic"); 5486 for (int i = 0; i < array.size(); i++) { 5487 res.getTopic().add(parseReference(array.get(i).getAsJsonObject())); 5488 } 5489 }; 5490 if (json.has("context")) 5491 res.setContext(parseReference(getJObject(json, "context"))); 5492 if (json.has("sent")) 5493 res.setSentElement(parseDateTime(json.get("sent").getAsString())); 5494 if (json.has("_sent")) 5495 parseElementProperties(getJObject(json, "_sent"), res.getSentElement()); 5496 if (json.has("received")) 5497 res.setReceivedElement(parseDateTime(json.get("received").getAsString())); 5498 if (json.has("_received")) 5499 parseElementProperties(getJObject(json, "_received"), res.getReceivedElement()); 5500 if (json.has("sender")) 5501 res.setSender(parseReference(getJObject(json, "sender"))); 5502 if (json.has("reasonCode")) { 5503 JsonArray array = json.getAsJsonArray("reasonCode"); 5504 for (int i = 0; i < array.size(); i++) { 5505 res.getReasonCode().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 5506 } 5507 }; 5508 if (json.has("reasonReference")) { 5509 JsonArray array = json.getAsJsonArray("reasonReference"); 5510 for (int i = 0; i < array.size(); i++) { 5511 res.getReasonReference().add(parseReference(array.get(i).getAsJsonObject())); 5512 } 5513 }; 5514 if (json.has("payload")) { 5515 JsonArray array = json.getAsJsonArray("payload"); 5516 for (int i = 0; i < array.size(); i++) { 5517 res.getPayload().add(parseCommunicationCommunicationPayloadComponent(array.get(i).getAsJsonObject(), res)); 5518 } 5519 }; 5520 if (json.has("note")) { 5521 JsonArray array = json.getAsJsonArray("note"); 5522 for (int i = 0; i < array.size(); i++) { 5523 res.getNote().add(parseAnnotation(array.get(i).getAsJsonObject())); 5524 } 5525 }; 5526 } 5527 5528 protected Communication.CommunicationPayloadComponent parseCommunicationCommunicationPayloadComponent(JsonObject json, Communication owner) throws IOException, FHIRFormatError { 5529 Communication.CommunicationPayloadComponent res = new Communication.CommunicationPayloadComponent(); 5530 parseCommunicationCommunicationPayloadComponentProperties(json, owner, res); 5531 return res; 5532 } 5533 5534 protected void parseCommunicationCommunicationPayloadComponentProperties(JsonObject json, Communication owner, Communication.CommunicationPayloadComponent res) throws IOException, FHIRFormatError { 5535 parseBackboneProperties(json, res); 5536 Type content = parseType("content", json); 5537 if (content != null) 5538 res.setContent(content); 5539 } 5540 5541 protected CommunicationRequest parseCommunicationRequest(JsonObject json) throws IOException, FHIRFormatError { 5542 CommunicationRequest res = new CommunicationRequest(); 5543 parseCommunicationRequestProperties(json, res); 5544 return res; 5545 } 5546 5547 protected void parseCommunicationRequestProperties(JsonObject json, CommunicationRequest res) throws IOException, FHIRFormatError { 5548 parseDomainResourceProperties(json, res); 5549 if (json.has("identifier")) { 5550 JsonArray array = json.getAsJsonArray("identifier"); 5551 for (int i = 0; i < array.size(); i++) { 5552 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 5553 } 5554 }; 5555 if (json.has("basedOn")) { 5556 JsonArray array = json.getAsJsonArray("basedOn"); 5557 for (int i = 0; i < array.size(); i++) { 5558 res.getBasedOn().add(parseReference(array.get(i).getAsJsonObject())); 5559 } 5560 }; 5561 if (json.has("replaces")) { 5562 JsonArray array = json.getAsJsonArray("replaces"); 5563 for (int i = 0; i < array.size(); i++) { 5564 res.getReplaces().add(parseReference(array.get(i).getAsJsonObject())); 5565 } 5566 }; 5567 if (json.has("groupIdentifier")) 5568 res.setGroupIdentifier(parseIdentifier(getJObject(json, "groupIdentifier"))); 5569 if (json.has("status")) 5570 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), CommunicationRequest.CommunicationRequestStatus.NULL, new CommunicationRequest.CommunicationRequestStatusEnumFactory())); 5571 if (json.has("_status")) 5572 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 5573 if (json.has("category")) { 5574 JsonArray array = json.getAsJsonArray("category"); 5575 for (int i = 0; i < array.size(); i++) { 5576 res.getCategory().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 5577 } 5578 }; 5579 if (json.has("priority")) 5580 res.setPriorityElement(parseEnumeration(json.get("priority").getAsString(), CommunicationRequest.CommunicationPriority.NULL, new CommunicationRequest.CommunicationPriorityEnumFactory())); 5581 if (json.has("_priority")) 5582 parseElementProperties(getJObject(json, "_priority"), res.getPriorityElement()); 5583 if (json.has("medium")) { 5584 JsonArray array = json.getAsJsonArray("medium"); 5585 for (int i = 0; i < array.size(); i++) { 5586 res.getMedium().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 5587 } 5588 }; 5589 if (json.has("subject")) 5590 res.setSubject(parseReference(getJObject(json, "subject"))); 5591 if (json.has("recipient")) { 5592 JsonArray array = json.getAsJsonArray("recipient"); 5593 for (int i = 0; i < array.size(); i++) { 5594 res.getRecipient().add(parseReference(array.get(i).getAsJsonObject())); 5595 } 5596 }; 5597 if (json.has("topic")) { 5598 JsonArray array = json.getAsJsonArray("topic"); 5599 for (int i = 0; i < array.size(); i++) { 5600 res.getTopic().add(parseReference(array.get(i).getAsJsonObject())); 5601 } 5602 }; 5603 if (json.has("context")) 5604 res.setContext(parseReference(getJObject(json, "context"))); 5605 if (json.has("payload")) { 5606 JsonArray array = json.getAsJsonArray("payload"); 5607 for (int i = 0; i < array.size(); i++) { 5608 res.getPayload().add(parseCommunicationRequestCommunicationRequestPayloadComponent(array.get(i).getAsJsonObject(), res)); 5609 } 5610 }; 5611 Type occurrence = parseType("occurrence", json); 5612 if (occurrence != null) 5613 res.setOccurrence(occurrence); 5614 if (json.has("authoredOn")) 5615 res.setAuthoredOnElement(parseDateTime(json.get("authoredOn").getAsString())); 5616 if (json.has("_authoredOn")) 5617 parseElementProperties(getJObject(json, "_authoredOn"), res.getAuthoredOnElement()); 5618 if (json.has("sender")) 5619 res.setSender(parseReference(getJObject(json, "sender"))); 5620 if (json.has("requester")) 5621 res.setRequester(parseCommunicationRequestCommunicationRequestRequesterComponent(getJObject(json, "requester"), res)); 5622 if (json.has("reasonCode")) { 5623 JsonArray array = json.getAsJsonArray("reasonCode"); 5624 for (int i = 0; i < array.size(); i++) { 5625 res.getReasonCode().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 5626 } 5627 }; 5628 if (json.has("reasonReference")) { 5629 JsonArray array = json.getAsJsonArray("reasonReference"); 5630 for (int i = 0; i < array.size(); i++) { 5631 res.getReasonReference().add(parseReference(array.get(i).getAsJsonObject())); 5632 } 5633 }; 5634 if (json.has("note")) { 5635 JsonArray array = json.getAsJsonArray("note"); 5636 for (int i = 0; i < array.size(); i++) { 5637 res.getNote().add(parseAnnotation(array.get(i).getAsJsonObject())); 5638 } 5639 }; 5640 } 5641 5642 protected CommunicationRequest.CommunicationRequestPayloadComponent parseCommunicationRequestCommunicationRequestPayloadComponent(JsonObject json, CommunicationRequest owner) throws IOException, FHIRFormatError { 5643 CommunicationRequest.CommunicationRequestPayloadComponent res = new CommunicationRequest.CommunicationRequestPayloadComponent(); 5644 parseCommunicationRequestCommunicationRequestPayloadComponentProperties(json, owner, res); 5645 return res; 5646 } 5647 5648 protected void parseCommunicationRequestCommunicationRequestPayloadComponentProperties(JsonObject json, CommunicationRequest owner, CommunicationRequest.CommunicationRequestPayloadComponent res) throws IOException, FHIRFormatError { 5649 parseBackboneProperties(json, res); 5650 Type content = parseType("content", json); 5651 if (content != null) 5652 res.setContent(content); 5653 } 5654 5655 protected CommunicationRequest.CommunicationRequestRequesterComponent parseCommunicationRequestCommunicationRequestRequesterComponent(JsonObject json, CommunicationRequest owner) throws IOException, FHIRFormatError { 5656 CommunicationRequest.CommunicationRequestRequesterComponent res = new CommunicationRequest.CommunicationRequestRequesterComponent(); 5657 parseCommunicationRequestCommunicationRequestRequesterComponentProperties(json, owner, res); 5658 return res; 5659 } 5660 5661 protected void parseCommunicationRequestCommunicationRequestRequesterComponentProperties(JsonObject json, CommunicationRequest owner, CommunicationRequest.CommunicationRequestRequesterComponent res) throws IOException, FHIRFormatError { 5662 parseBackboneProperties(json, res); 5663 if (json.has("agent")) 5664 res.setAgent(parseReference(getJObject(json, "agent"))); 5665 if (json.has("onBehalfOf")) 5666 res.setOnBehalfOf(parseReference(getJObject(json, "onBehalfOf"))); 5667 } 5668 5669 protected CompartmentDefinition parseCompartmentDefinition(JsonObject json) throws IOException, FHIRFormatError { 5670 CompartmentDefinition res = new CompartmentDefinition(); 5671 parseCompartmentDefinitionProperties(json, res); 5672 return res; 5673 } 5674 5675 protected void parseCompartmentDefinitionProperties(JsonObject json, CompartmentDefinition res) throws IOException, FHIRFormatError { 5676 parseDomainResourceProperties(json, res); 5677 if (json.has("url")) 5678 res.setUrlElement(parseUri(json.get("url").getAsString())); 5679 if (json.has("_url")) 5680 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 5681 if (json.has("name")) 5682 res.setNameElement(parseString(json.get("name").getAsString())); 5683 if (json.has("_name")) 5684 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 5685 if (json.has("title")) 5686 res.setTitleElement(parseString(json.get("title").getAsString())); 5687 if (json.has("_title")) 5688 parseElementProperties(getJObject(json, "_title"), res.getTitleElement()); 5689 if (json.has("status")) 5690 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Enumerations.PublicationStatus.NULL, new Enumerations.PublicationStatusEnumFactory())); 5691 if (json.has("_status")) 5692 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 5693 if (json.has("experimental")) 5694 res.setExperimentalElement(parseBoolean(json.get("experimental").getAsBoolean())); 5695 if (json.has("_experimental")) 5696 parseElementProperties(getJObject(json, "_experimental"), res.getExperimentalElement()); 5697 if (json.has("date")) 5698 res.setDateElement(parseDateTime(json.get("date").getAsString())); 5699 if (json.has("_date")) 5700 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 5701 if (json.has("publisher")) 5702 res.setPublisherElement(parseString(json.get("publisher").getAsString())); 5703 if (json.has("_publisher")) 5704 parseElementProperties(getJObject(json, "_publisher"), res.getPublisherElement()); 5705 if (json.has("contact")) { 5706 JsonArray array = json.getAsJsonArray("contact"); 5707 for (int i = 0; i < array.size(); i++) { 5708 res.getContact().add(parseContactDetail(array.get(i).getAsJsonObject())); 5709 } 5710 }; 5711 if (json.has("description")) 5712 res.setDescriptionElement(parseMarkdown(json.get("description").getAsString())); 5713 if (json.has("_description")) 5714 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 5715 if (json.has("purpose")) 5716 res.setPurposeElement(parseMarkdown(json.get("purpose").getAsString())); 5717 if (json.has("_purpose")) 5718 parseElementProperties(getJObject(json, "_purpose"), res.getPurposeElement()); 5719 if (json.has("useContext")) { 5720 JsonArray array = json.getAsJsonArray("useContext"); 5721 for (int i = 0; i < array.size(); i++) { 5722 res.getUseContext().add(parseUsageContext(array.get(i).getAsJsonObject())); 5723 } 5724 }; 5725 if (json.has("jurisdiction")) { 5726 JsonArray array = json.getAsJsonArray("jurisdiction"); 5727 for (int i = 0; i < array.size(); i++) { 5728 res.getJurisdiction().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 5729 } 5730 }; 5731 if (json.has("code")) 5732 res.setCodeElement(parseEnumeration(json.get("code").getAsString(), CompartmentDefinition.CompartmentType.NULL, new CompartmentDefinition.CompartmentTypeEnumFactory())); 5733 if (json.has("_code")) 5734 parseElementProperties(getJObject(json, "_code"), res.getCodeElement()); 5735 if (json.has("search")) 5736 res.setSearchElement(parseBoolean(json.get("search").getAsBoolean())); 5737 if (json.has("_search")) 5738 parseElementProperties(getJObject(json, "_search"), res.getSearchElement()); 5739 if (json.has("resource")) { 5740 JsonArray array = json.getAsJsonArray("resource"); 5741 for (int i = 0; i < array.size(); i++) { 5742 res.getResource().add(parseCompartmentDefinitionCompartmentDefinitionResourceComponent(array.get(i).getAsJsonObject(), res)); 5743 } 5744 }; 5745 } 5746 5747 protected CompartmentDefinition.CompartmentDefinitionResourceComponent parseCompartmentDefinitionCompartmentDefinitionResourceComponent(JsonObject json, CompartmentDefinition owner) throws IOException, FHIRFormatError { 5748 CompartmentDefinition.CompartmentDefinitionResourceComponent res = new CompartmentDefinition.CompartmentDefinitionResourceComponent(); 5749 parseCompartmentDefinitionCompartmentDefinitionResourceComponentProperties(json, owner, res); 5750 return res; 5751 } 5752 5753 protected void parseCompartmentDefinitionCompartmentDefinitionResourceComponentProperties(JsonObject json, CompartmentDefinition owner, CompartmentDefinition.CompartmentDefinitionResourceComponent res) throws IOException, FHIRFormatError { 5754 parseBackboneProperties(json, res); 5755 if (json.has("code")) 5756 res.setCodeElement(parseCode(json.get("code").getAsString())); 5757 if (json.has("_code")) 5758 parseElementProperties(getJObject(json, "_code"), res.getCodeElement()); 5759 if (json.has("param")) { 5760 JsonArray array = json.getAsJsonArray("param"); 5761 for (int i = 0; i < array.size(); i++) { 5762 if (array.get(i).isJsonNull()) { 5763 res.getParam().add(new StringType()); 5764 } else { 5765 res.getParam().add(parseString(array.get(i).getAsString())); 5766 } 5767 } 5768 }; 5769 if (json.has("_param")) { 5770 JsonArray array = json.getAsJsonArray("_param"); 5771 for (int i = 0; i < array.size(); i++) { 5772 if (i == res.getParam().size()) 5773 res.getParam().add(parseString(null)); 5774 if (array.get(i) instanceof JsonObject) 5775 parseElementProperties(array.get(i).getAsJsonObject(), res.getParam().get(i)); 5776 } 5777 }; 5778 if (json.has("documentation")) 5779 res.setDocumentationElement(parseString(json.get("documentation").getAsString())); 5780 if (json.has("_documentation")) 5781 parseElementProperties(getJObject(json, "_documentation"), res.getDocumentationElement()); 5782 } 5783 5784 protected Composition parseComposition(JsonObject json) throws IOException, FHIRFormatError { 5785 Composition res = new Composition(); 5786 parseCompositionProperties(json, res); 5787 return res; 5788 } 5789 5790 protected void parseCompositionProperties(JsonObject json, Composition res) throws IOException, FHIRFormatError { 5791 parseDomainResourceProperties(json, res); 5792 if (json.has("identifier")) 5793 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 5794 if (json.has("status")) 5795 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Composition.CompositionStatus.NULL, new Composition.CompositionStatusEnumFactory())); 5796 if (json.has("_status")) 5797 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 5798 if (json.has("type")) 5799 res.setType(parseCodeableConcept(getJObject(json, "type"))); 5800 if (json.has("class")) 5801 res.setClass_(parseCodeableConcept(getJObject(json, "class"))); 5802 if (json.has("subject")) 5803 res.setSubject(parseReference(getJObject(json, "subject"))); 5804 if (json.has("encounter")) 5805 res.setEncounter(parseReference(getJObject(json, "encounter"))); 5806 if (json.has("date")) 5807 res.setDateElement(parseDateTime(json.get("date").getAsString())); 5808 if (json.has("_date")) 5809 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 5810 if (json.has("author")) { 5811 JsonArray array = json.getAsJsonArray("author"); 5812 for (int i = 0; i < array.size(); i++) { 5813 res.getAuthor().add(parseReference(array.get(i).getAsJsonObject())); 5814 } 5815 }; 5816 if (json.has("title")) 5817 res.setTitleElement(parseString(json.get("title").getAsString())); 5818 if (json.has("_title")) 5819 parseElementProperties(getJObject(json, "_title"), res.getTitleElement()); 5820 if (json.has("confidentiality")) 5821 res.setConfidentialityElement(parseEnumeration(json.get("confidentiality").getAsString(), Composition.DocumentConfidentiality.NULL, new Composition.DocumentConfidentialityEnumFactory())); 5822 if (json.has("_confidentiality")) 5823 parseElementProperties(getJObject(json, "_confidentiality"), res.getConfidentialityElement()); 5824 if (json.has("attester")) { 5825 JsonArray array = json.getAsJsonArray("attester"); 5826 for (int i = 0; i < array.size(); i++) { 5827 res.getAttester().add(parseCompositionCompositionAttesterComponent(array.get(i).getAsJsonObject(), res)); 5828 } 5829 }; 5830 if (json.has("custodian")) 5831 res.setCustodian(parseReference(getJObject(json, "custodian"))); 5832 if (json.has("relatesTo")) { 5833 JsonArray array = json.getAsJsonArray("relatesTo"); 5834 for (int i = 0; i < array.size(); i++) { 5835 res.getRelatesTo().add(parseCompositionCompositionRelatesToComponent(array.get(i).getAsJsonObject(), res)); 5836 } 5837 }; 5838 if (json.has("event")) { 5839 JsonArray array = json.getAsJsonArray("event"); 5840 for (int i = 0; i < array.size(); i++) { 5841 res.getEvent().add(parseCompositionCompositionEventComponent(array.get(i).getAsJsonObject(), res)); 5842 } 5843 }; 5844 if (json.has("section")) { 5845 JsonArray array = json.getAsJsonArray("section"); 5846 for (int i = 0; i < array.size(); i++) { 5847 res.getSection().add(parseCompositionSectionComponent(array.get(i).getAsJsonObject(), res)); 5848 } 5849 }; 5850 } 5851 5852 protected Composition.CompositionAttesterComponent parseCompositionCompositionAttesterComponent(JsonObject json, Composition owner) throws IOException, FHIRFormatError { 5853 Composition.CompositionAttesterComponent res = new Composition.CompositionAttesterComponent(); 5854 parseCompositionCompositionAttesterComponentProperties(json, owner, res); 5855 return res; 5856 } 5857 5858 protected void parseCompositionCompositionAttesterComponentProperties(JsonObject json, Composition owner, Composition.CompositionAttesterComponent res) throws IOException, FHIRFormatError { 5859 parseBackboneProperties(json, res); 5860 if (json.has("mode")) { 5861 JsonArray array = json.getAsJsonArray("mode"); 5862 for (int i = 0; i < array.size(); i++) { 5863 if (array.get(i).isJsonNull()) { 5864 res.getMode().add(new Enumeration<Composition.CompositionAttestationMode>()); 5865 } else { 5866 res.getMode().add(parseEnumeration(array.get(i).getAsString(), Composition.CompositionAttestationMode.NULL, new Composition.CompositionAttestationModeEnumFactory())); 5867 } 5868 } 5869 }; 5870 if (json.has("_mode")) { 5871 JsonArray array = json.getAsJsonArray("_mode"); 5872 for (int i = 0; i < array.size(); i++) { 5873 if (i == res.getMode().size()) 5874 res.getMode().add(parseEnumeration(null, Composition.CompositionAttestationMode.NULL, new Composition.CompositionAttestationModeEnumFactory())); 5875 if (array.get(i) instanceof JsonObject) 5876 parseElementProperties(array.get(i).getAsJsonObject(), res.getMode().get(i)); 5877 } 5878 }; 5879 if (json.has("time")) 5880 res.setTimeElement(parseDateTime(json.get("time").getAsString())); 5881 if (json.has("_time")) 5882 parseElementProperties(getJObject(json, "_time"), res.getTimeElement()); 5883 if (json.has("party")) 5884 res.setParty(parseReference(getJObject(json, "party"))); 5885 } 5886 5887 protected Composition.CompositionRelatesToComponent parseCompositionCompositionRelatesToComponent(JsonObject json, Composition owner) throws IOException, FHIRFormatError { 5888 Composition.CompositionRelatesToComponent res = new Composition.CompositionRelatesToComponent(); 5889 parseCompositionCompositionRelatesToComponentProperties(json, owner, res); 5890 return res; 5891 } 5892 5893 protected void parseCompositionCompositionRelatesToComponentProperties(JsonObject json, Composition owner, Composition.CompositionRelatesToComponent res) throws IOException, FHIRFormatError { 5894 parseBackboneProperties(json, res); 5895 if (json.has("code")) 5896 res.setCodeElement(parseEnumeration(json.get("code").getAsString(), Composition.DocumentRelationshipType.NULL, new Composition.DocumentRelationshipTypeEnumFactory())); 5897 if (json.has("_code")) 5898 parseElementProperties(getJObject(json, "_code"), res.getCodeElement()); 5899 Type target = parseType("target", json); 5900 if (target != null) 5901 res.setTarget(target); 5902 } 5903 5904 protected Composition.CompositionEventComponent parseCompositionCompositionEventComponent(JsonObject json, Composition owner) throws IOException, FHIRFormatError { 5905 Composition.CompositionEventComponent res = new Composition.CompositionEventComponent(); 5906 parseCompositionCompositionEventComponentProperties(json, owner, res); 5907 return res; 5908 } 5909 5910 protected void parseCompositionCompositionEventComponentProperties(JsonObject json, Composition owner, Composition.CompositionEventComponent res) throws IOException, FHIRFormatError { 5911 parseBackboneProperties(json, res); 5912 if (json.has("code")) { 5913 JsonArray array = json.getAsJsonArray("code"); 5914 for (int i = 0; i < array.size(); i++) { 5915 res.getCode().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 5916 } 5917 }; 5918 if (json.has("period")) 5919 res.setPeriod(parsePeriod(getJObject(json, "period"))); 5920 if (json.has("detail")) { 5921 JsonArray array = json.getAsJsonArray("detail"); 5922 for (int i = 0; i < array.size(); i++) { 5923 res.getDetail().add(parseReference(array.get(i).getAsJsonObject())); 5924 } 5925 }; 5926 } 5927 5928 protected Composition.SectionComponent parseCompositionSectionComponent(JsonObject json, Composition owner) throws IOException, FHIRFormatError { 5929 Composition.SectionComponent res = new Composition.SectionComponent(); 5930 parseCompositionSectionComponentProperties(json, owner, res); 5931 return res; 5932 } 5933 5934 protected void parseCompositionSectionComponentProperties(JsonObject json, Composition owner, Composition.SectionComponent res) throws IOException, FHIRFormatError { 5935 parseBackboneProperties(json, res); 5936 if (json.has("title")) 5937 res.setTitleElement(parseString(json.get("title").getAsString())); 5938 if (json.has("_title")) 5939 parseElementProperties(getJObject(json, "_title"), res.getTitleElement()); 5940 if (json.has("code")) 5941 res.setCode(parseCodeableConcept(getJObject(json, "code"))); 5942 if (json.has("text")) 5943 res.setText(parseNarrative(getJObject(json, "text"))); 5944 if (json.has("mode")) 5945 res.setModeElement(parseEnumeration(json.get("mode").getAsString(), Composition.SectionMode.NULL, new Composition.SectionModeEnumFactory())); 5946 if (json.has("_mode")) 5947 parseElementProperties(getJObject(json, "_mode"), res.getModeElement()); 5948 if (json.has("orderedBy")) 5949 res.setOrderedBy(parseCodeableConcept(getJObject(json, "orderedBy"))); 5950 if (json.has("entry")) { 5951 JsonArray array = json.getAsJsonArray("entry"); 5952 for (int i = 0; i < array.size(); i++) { 5953 res.getEntry().add(parseReference(array.get(i).getAsJsonObject())); 5954 } 5955 }; 5956 if (json.has("emptyReason")) 5957 res.setEmptyReason(parseCodeableConcept(getJObject(json, "emptyReason"))); 5958 if (json.has("section")) { 5959 JsonArray array = json.getAsJsonArray("section"); 5960 for (int i = 0; i < array.size(); i++) { 5961 res.getSection().add(parseCompositionSectionComponent(array.get(i).getAsJsonObject(), owner)); 5962 } 5963 }; 5964 } 5965 5966 protected ConceptMap parseConceptMap(JsonObject json) throws IOException, FHIRFormatError { 5967 ConceptMap res = new ConceptMap(); 5968 parseConceptMapProperties(json, res); 5969 return res; 5970 } 5971 5972 protected void parseConceptMapProperties(JsonObject json, ConceptMap res) throws IOException, FHIRFormatError { 5973 parseDomainResourceProperties(json, res); 5974 if (json.has("url")) 5975 res.setUrlElement(parseUri(json.get("url").getAsString())); 5976 if (json.has("_url")) 5977 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 5978 if (json.has("identifier")) 5979 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 5980 if (json.has("version")) 5981 res.setVersionElement(parseString(json.get("version").getAsString())); 5982 if (json.has("_version")) 5983 parseElementProperties(getJObject(json, "_version"), res.getVersionElement()); 5984 if (json.has("name")) 5985 res.setNameElement(parseString(json.get("name").getAsString())); 5986 if (json.has("_name")) 5987 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 5988 if (json.has("title")) 5989 res.setTitleElement(parseString(json.get("title").getAsString())); 5990 if (json.has("_title")) 5991 parseElementProperties(getJObject(json, "_title"), res.getTitleElement()); 5992 if (json.has("status")) 5993 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Enumerations.PublicationStatus.NULL, new Enumerations.PublicationStatusEnumFactory())); 5994 if (json.has("_status")) 5995 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 5996 if (json.has("experimental")) 5997 res.setExperimentalElement(parseBoolean(json.get("experimental").getAsBoolean())); 5998 if (json.has("_experimental")) 5999 parseElementProperties(getJObject(json, "_experimental"), res.getExperimentalElement()); 6000 if (json.has("date")) 6001 res.setDateElement(parseDateTime(json.get("date").getAsString())); 6002 if (json.has("_date")) 6003 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 6004 if (json.has("publisher")) 6005 res.setPublisherElement(parseString(json.get("publisher").getAsString())); 6006 if (json.has("_publisher")) 6007 parseElementProperties(getJObject(json, "_publisher"), res.getPublisherElement()); 6008 if (json.has("contact")) { 6009 JsonArray array = json.getAsJsonArray("contact"); 6010 for (int i = 0; i < array.size(); i++) { 6011 res.getContact().add(parseContactDetail(array.get(i).getAsJsonObject())); 6012 } 6013 }; 6014 if (json.has("description")) 6015 res.setDescriptionElement(parseMarkdown(json.get("description").getAsString())); 6016 if (json.has("_description")) 6017 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 6018 if (json.has("useContext")) { 6019 JsonArray array = json.getAsJsonArray("useContext"); 6020 for (int i = 0; i < array.size(); i++) { 6021 res.getUseContext().add(parseUsageContext(array.get(i).getAsJsonObject())); 6022 } 6023 }; 6024 if (json.has("jurisdiction")) { 6025 JsonArray array = json.getAsJsonArray("jurisdiction"); 6026 for (int i = 0; i < array.size(); i++) { 6027 res.getJurisdiction().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 6028 } 6029 }; 6030 if (json.has("purpose")) 6031 res.setPurposeElement(parseMarkdown(json.get("purpose").getAsString())); 6032 if (json.has("_purpose")) 6033 parseElementProperties(getJObject(json, "_purpose"), res.getPurposeElement()); 6034 if (json.has("copyright")) 6035 res.setCopyrightElement(parseMarkdown(json.get("copyright").getAsString())); 6036 if (json.has("_copyright")) 6037 parseElementProperties(getJObject(json, "_copyright"), res.getCopyrightElement()); 6038 Type source = parseType("source", json); 6039 if (source != null) 6040 res.setSource(source); 6041 Type target = parseType("target", json); 6042 if (target != null) 6043 res.setTarget(target); 6044 if (json.has("group")) { 6045 JsonArray array = json.getAsJsonArray("group"); 6046 for (int i = 0; i < array.size(); i++) { 6047 res.getGroup().add(parseConceptMapConceptMapGroupComponent(array.get(i).getAsJsonObject(), res)); 6048 } 6049 }; 6050 } 6051 6052 protected ConceptMap.ConceptMapGroupComponent parseConceptMapConceptMapGroupComponent(JsonObject json, ConceptMap owner) throws IOException, FHIRFormatError { 6053 ConceptMap.ConceptMapGroupComponent res = new ConceptMap.ConceptMapGroupComponent(); 6054 parseConceptMapConceptMapGroupComponentProperties(json, owner, res); 6055 return res; 6056 } 6057 6058 protected void parseConceptMapConceptMapGroupComponentProperties(JsonObject json, ConceptMap owner, ConceptMap.ConceptMapGroupComponent res) throws IOException, FHIRFormatError { 6059 parseBackboneProperties(json, res); 6060 if (json.has("source")) 6061 res.setSourceElement(parseUri(json.get("source").getAsString())); 6062 if (json.has("_source")) 6063 parseElementProperties(getJObject(json, "_source"), res.getSourceElement()); 6064 if (json.has("sourceVersion")) 6065 res.setSourceVersionElement(parseString(json.get("sourceVersion").getAsString())); 6066 if (json.has("_sourceVersion")) 6067 parseElementProperties(getJObject(json, "_sourceVersion"), res.getSourceVersionElement()); 6068 if (json.has("target")) 6069 res.setTargetElement(parseUri(json.get("target").getAsString())); 6070 if (json.has("_target")) 6071 parseElementProperties(getJObject(json, "_target"), res.getTargetElement()); 6072 if (json.has("targetVersion")) 6073 res.setTargetVersionElement(parseString(json.get("targetVersion").getAsString())); 6074 if (json.has("_targetVersion")) 6075 parseElementProperties(getJObject(json, "_targetVersion"), res.getTargetVersionElement()); 6076 if (json.has("element")) { 6077 JsonArray array = json.getAsJsonArray("element"); 6078 for (int i = 0; i < array.size(); i++) { 6079 res.getElement().add(parseConceptMapSourceElementComponent(array.get(i).getAsJsonObject(), owner)); 6080 } 6081 }; 6082 if (json.has("unmapped")) 6083 res.setUnmapped(parseConceptMapConceptMapGroupUnmappedComponent(getJObject(json, "unmapped"), owner)); 6084 } 6085 6086 protected ConceptMap.SourceElementComponent parseConceptMapSourceElementComponent(JsonObject json, ConceptMap owner) throws IOException, FHIRFormatError { 6087 ConceptMap.SourceElementComponent res = new ConceptMap.SourceElementComponent(); 6088 parseConceptMapSourceElementComponentProperties(json, owner, res); 6089 return res; 6090 } 6091 6092 protected void parseConceptMapSourceElementComponentProperties(JsonObject json, ConceptMap owner, ConceptMap.SourceElementComponent res) throws IOException, FHIRFormatError { 6093 parseBackboneProperties(json, res); 6094 if (json.has("code")) 6095 res.setCodeElement(parseCode(json.get("code").getAsString())); 6096 if (json.has("_code")) 6097 parseElementProperties(getJObject(json, "_code"), res.getCodeElement()); 6098 if (json.has("display")) 6099 res.setDisplayElement(parseString(json.get("display").getAsString())); 6100 if (json.has("_display")) 6101 parseElementProperties(getJObject(json, "_display"), res.getDisplayElement()); 6102 if (json.has("target")) { 6103 JsonArray array = json.getAsJsonArray("target"); 6104 for (int i = 0; i < array.size(); i++) { 6105 res.getTarget().add(parseConceptMapTargetElementComponent(array.get(i).getAsJsonObject(), owner)); 6106 } 6107 }; 6108 } 6109 6110 protected ConceptMap.TargetElementComponent parseConceptMapTargetElementComponent(JsonObject json, ConceptMap owner) throws IOException, FHIRFormatError { 6111 ConceptMap.TargetElementComponent res = new ConceptMap.TargetElementComponent(); 6112 parseConceptMapTargetElementComponentProperties(json, owner, res); 6113 return res; 6114 } 6115 6116 protected void parseConceptMapTargetElementComponentProperties(JsonObject json, ConceptMap owner, ConceptMap.TargetElementComponent res) throws IOException, FHIRFormatError { 6117 parseBackboneProperties(json, res); 6118 if (json.has("code")) 6119 res.setCodeElement(parseCode(json.get("code").getAsString())); 6120 if (json.has("_code")) 6121 parseElementProperties(getJObject(json, "_code"), res.getCodeElement()); 6122 if (json.has("display")) 6123 res.setDisplayElement(parseString(json.get("display").getAsString())); 6124 if (json.has("_display")) 6125 parseElementProperties(getJObject(json, "_display"), res.getDisplayElement()); 6126 if (json.has("equivalence")) 6127 res.setEquivalenceElement(parseEnumeration(json.get("equivalence").getAsString(), Enumerations.ConceptMapEquivalence.NULL, new Enumerations.ConceptMapEquivalenceEnumFactory())); 6128 if (json.has("_equivalence")) 6129 parseElementProperties(getJObject(json, "_equivalence"), res.getEquivalenceElement()); 6130 if (json.has("comment")) 6131 res.setCommentElement(parseString(json.get("comment").getAsString())); 6132 if (json.has("_comment")) 6133 parseElementProperties(getJObject(json, "_comment"), res.getCommentElement()); 6134 if (json.has("dependsOn")) { 6135 JsonArray array = json.getAsJsonArray("dependsOn"); 6136 for (int i = 0; i < array.size(); i++) { 6137 res.getDependsOn().add(parseConceptMapOtherElementComponent(array.get(i).getAsJsonObject(), owner)); 6138 } 6139 }; 6140 if (json.has("product")) { 6141 JsonArray array = json.getAsJsonArray("product"); 6142 for (int i = 0; i < array.size(); i++) { 6143 res.getProduct().add(parseConceptMapOtherElementComponent(array.get(i).getAsJsonObject(), owner)); 6144 } 6145 }; 6146 } 6147 6148 protected ConceptMap.OtherElementComponent parseConceptMapOtherElementComponent(JsonObject json, ConceptMap owner) throws IOException, FHIRFormatError { 6149 ConceptMap.OtherElementComponent res = new ConceptMap.OtherElementComponent(); 6150 parseConceptMapOtherElementComponentProperties(json, owner, res); 6151 return res; 6152 } 6153 6154 protected void parseConceptMapOtherElementComponentProperties(JsonObject json, ConceptMap owner, ConceptMap.OtherElementComponent res) throws IOException, FHIRFormatError { 6155 parseBackboneProperties(json, res); 6156 if (json.has("property")) 6157 res.setPropertyElement(parseUri(json.get("property").getAsString())); 6158 if (json.has("_property")) 6159 parseElementProperties(getJObject(json, "_property"), res.getPropertyElement()); 6160 if (json.has("system")) 6161 res.setSystemElement(parseUri(json.get("system").getAsString())); 6162 if (json.has("_system")) 6163 parseElementProperties(getJObject(json, "_system"), res.getSystemElement()); 6164 if (json.has("code")) 6165 res.setCodeElement(parseString(json.get("code").getAsString())); 6166 if (json.has("_code")) 6167 parseElementProperties(getJObject(json, "_code"), res.getCodeElement()); 6168 if (json.has("display")) 6169 res.setDisplayElement(parseString(json.get("display").getAsString())); 6170 if (json.has("_display")) 6171 parseElementProperties(getJObject(json, "_display"), res.getDisplayElement()); 6172 } 6173 6174 protected ConceptMap.ConceptMapGroupUnmappedComponent parseConceptMapConceptMapGroupUnmappedComponent(JsonObject json, ConceptMap owner) throws IOException, FHIRFormatError { 6175 ConceptMap.ConceptMapGroupUnmappedComponent res = new ConceptMap.ConceptMapGroupUnmappedComponent(); 6176 parseConceptMapConceptMapGroupUnmappedComponentProperties(json, owner, res); 6177 return res; 6178 } 6179 6180 protected void parseConceptMapConceptMapGroupUnmappedComponentProperties(JsonObject json, ConceptMap owner, ConceptMap.ConceptMapGroupUnmappedComponent res) throws IOException, FHIRFormatError { 6181 parseBackboneProperties(json, res); 6182 if (json.has("mode")) 6183 res.setModeElement(parseEnumeration(json.get("mode").getAsString(), ConceptMap.ConceptMapGroupUnmappedMode.NULL, new ConceptMap.ConceptMapGroupUnmappedModeEnumFactory())); 6184 if (json.has("_mode")) 6185 parseElementProperties(getJObject(json, "_mode"), res.getModeElement()); 6186 if (json.has("code")) 6187 res.setCodeElement(parseCode(json.get("code").getAsString())); 6188 if (json.has("_code")) 6189 parseElementProperties(getJObject(json, "_code"), res.getCodeElement()); 6190 if (json.has("display")) 6191 res.setDisplayElement(parseString(json.get("display").getAsString())); 6192 if (json.has("_display")) 6193 parseElementProperties(getJObject(json, "_display"), res.getDisplayElement()); 6194 if (json.has("url")) 6195 res.setUrlElement(parseUri(json.get("url").getAsString())); 6196 if (json.has("_url")) 6197 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 6198 } 6199 6200 protected Condition parseCondition(JsonObject json) throws IOException, FHIRFormatError { 6201 Condition res = new Condition(); 6202 parseConditionProperties(json, res); 6203 return res; 6204 } 6205 6206 protected void parseConditionProperties(JsonObject json, Condition res) throws IOException, FHIRFormatError { 6207 parseDomainResourceProperties(json, res); 6208 if (json.has("identifier")) { 6209 JsonArray array = json.getAsJsonArray("identifier"); 6210 for (int i = 0; i < array.size(); i++) { 6211 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 6212 } 6213 }; 6214 if (json.has("clinicalStatus")) 6215 res.setClinicalStatusElement(parseEnumeration(json.get("clinicalStatus").getAsString(), Condition.ConditionClinicalStatus.NULL, new Condition.ConditionClinicalStatusEnumFactory())); 6216 if (json.has("_clinicalStatus")) 6217 parseElementProperties(getJObject(json, "_clinicalStatus"), res.getClinicalStatusElement()); 6218 if (json.has("verificationStatus")) 6219 res.setVerificationStatusElement(parseEnumeration(json.get("verificationStatus").getAsString(), Condition.ConditionVerificationStatus.NULL, new Condition.ConditionVerificationStatusEnumFactory())); 6220 if (json.has("_verificationStatus")) 6221 parseElementProperties(getJObject(json, "_verificationStatus"), res.getVerificationStatusElement()); 6222 if (json.has("category")) { 6223 JsonArray array = json.getAsJsonArray("category"); 6224 for (int i = 0; i < array.size(); i++) { 6225 res.getCategory().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 6226 } 6227 }; 6228 if (json.has("severity")) 6229 res.setSeverity(parseCodeableConcept(getJObject(json, "severity"))); 6230 if (json.has("code")) 6231 res.setCode(parseCodeableConcept(getJObject(json, "code"))); 6232 if (json.has("bodySite")) { 6233 JsonArray array = json.getAsJsonArray("bodySite"); 6234 for (int i = 0; i < array.size(); i++) { 6235 res.getBodySite().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 6236 } 6237 }; 6238 if (json.has("subject")) 6239 res.setSubject(parseReference(getJObject(json, "subject"))); 6240 if (json.has("context")) 6241 res.setContext(parseReference(getJObject(json, "context"))); 6242 Type onset = parseType("onset", json); 6243 if (onset != null) 6244 res.setOnset(onset); 6245 Type abatement = parseType("abatement", json); 6246 if (abatement != null) 6247 res.setAbatement(abatement); 6248 if (json.has("assertedDate")) 6249 res.setAssertedDateElement(parseDateTime(json.get("assertedDate").getAsString())); 6250 if (json.has("_assertedDate")) 6251 parseElementProperties(getJObject(json, "_assertedDate"), res.getAssertedDateElement()); 6252 if (json.has("asserter")) 6253 res.setAsserter(parseReference(getJObject(json, "asserter"))); 6254 if (json.has("stage")) 6255 res.setStage(parseConditionConditionStageComponent(getJObject(json, "stage"), res)); 6256 if (json.has("evidence")) { 6257 JsonArray array = json.getAsJsonArray("evidence"); 6258 for (int i = 0; i < array.size(); i++) { 6259 res.getEvidence().add(parseConditionConditionEvidenceComponent(array.get(i).getAsJsonObject(), res)); 6260 } 6261 }; 6262 if (json.has("note")) { 6263 JsonArray array = json.getAsJsonArray("note"); 6264 for (int i = 0; i < array.size(); i++) { 6265 res.getNote().add(parseAnnotation(array.get(i).getAsJsonObject())); 6266 } 6267 }; 6268 } 6269 6270 protected Condition.ConditionStageComponent parseConditionConditionStageComponent(JsonObject json, Condition owner) throws IOException, FHIRFormatError { 6271 Condition.ConditionStageComponent res = new Condition.ConditionStageComponent(); 6272 parseConditionConditionStageComponentProperties(json, owner, res); 6273 return res; 6274 } 6275 6276 protected void parseConditionConditionStageComponentProperties(JsonObject json, Condition owner, Condition.ConditionStageComponent res) throws IOException, FHIRFormatError { 6277 parseBackboneProperties(json, res); 6278 if (json.has("summary")) 6279 res.setSummary(parseCodeableConcept(getJObject(json, "summary"))); 6280 if (json.has("assessment")) { 6281 JsonArray array = json.getAsJsonArray("assessment"); 6282 for (int i = 0; i < array.size(); i++) { 6283 res.getAssessment().add(parseReference(array.get(i).getAsJsonObject())); 6284 } 6285 }; 6286 } 6287 6288 protected Condition.ConditionEvidenceComponent parseConditionConditionEvidenceComponent(JsonObject json, Condition owner) throws IOException, FHIRFormatError { 6289 Condition.ConditionEvidenceComponent res = new Condition.ConditionEvidenceComponent(); 6290 parseConditionConditionEvidenceComponentProperties(json, owner, res); 6291 return res; 6292 } 6293 6294 protected void parseConditionConditionEvidenceComponentProperties(JsonObject json, Condition owner, Condition.ConditionEvidenceComponent res) throws IOException, FHIRFormatError { 6295 parseBackboneProperties(json, res); 6296 if (json.has("code")) { 6297 JsonArray array = json.getAsJsonArray("code"); 6298 for (int i = 0; i < array.size(); i++) { 6299 res.getCode().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 6300 } 6301 }; 6302 if (json.has("detail")) { 6303 JsonArray array = json.getAsJsonArray("detail"); 6304 for (int i = 0; i < array.size(); i++) { 6305 res.getDetail().add(parseReference(array.get(i).getAsJsonObject())); 6306 } 6307 }; 6308 } 6309 6310 protected Consent parseConsent(JsonObject json) throws IOException, FHIRFormatError { 6311 Consent res = new Consent(); 6312 parseConsentProperties(json, res); 6313 return res; 6314 } 6315 6316 protected void parseConsentProperties(JsonObject json, Consent res) throws IOException, FHIRFormatError { 6317 parseDomainResourceProperties(json, res); 6318 if (json.has("identifier")) 6319 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 6320 if (json.has("status")) 6321 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Consent.ConsentState.NULL, new Consent.ConsentStateEnumFactory())); 6322 if (json.has("_status")) 6323 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 6324 if (json.has("category")) { 6325 JsonArray array = json.getAsJsonArray("category"); 6326 for (int i = 0; i < array.size(); i++) { 6327 res.getCategory().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 6328 } 6329 }; 6330 if (json.has("patient")) 6331 res.setPatient(parseReference(getJObject(json, "patient"))); 6332 if (json.has("period")) 6333 res.setPeriod(parsePeriod(getJObject(json, "period"))); 6334 if (json.has("dateTime")) 6335 res.setDateTimeElement(parseDateTime(json.get("dateTime").getAsString())); 6336 if (json.has("_dateTime")) 6337 parseElementProperties(getJObject(json, "_dateTime"), res.getDateTimeElement()); 6338 if (json.has("consentingParty")) { 6339 JsonArray array = json.getAsJsonArray("consentingParty"); 6340 for (int i = 0; i < array.size(); i++) { 6341 res.getConsentingParty().add(parseReference(array.get(i).getAsJsonObject())); 6342 } 6343 }; 6344 if (json.has("actor")) { 6345 JsonArray array = json.getAsJsonArray("actor"); 6346 for (int i = 0; i < array.size(); i++) { 6347 res.getActor().add(parseConsentConsentActorComponent(array.get(i).getAsJsonObject(), res)); 6348 } 6349 }; 6350 if (json.has("action")) { 6351 JsonArray array = json.getAsJsonArray("action"); 6352 for (int i = 0; i < array.size(); i++) { 6353 res.getAction().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 6354 } 6355 }; 6356 if (json.has("organization")) { 6357 JsonArray array = json.getAsJsonArray("organization"); 6358 for (int i = 0; i < array.size(); i++) { 6359 res.getOrganization().add(parseReference(array.get(i).getAsJsonObject())); 6360 } 6361 }; 6362 Type source = parseType("source", json); 6363 if (source != null) 6364 res.setSource(source); 6365 if (json.has("policy")) { 6366 JsonArray array = json.getAsJsonArray("policy"); 6367 for (int i = 0; i < array.size(); i++) { 6368 res.getPolicy().add(parseConsentConsentPolicyComponent(array.get(i).getAsJsonObject(), res)); 6369 } 6370 }; 6371 if (json.has("policyRule")) 6372 res.setPolicyRuleElement(parseUri(json.get("policyRule").getAsString())); 6373 if (json.has("_policyRule")) 6374 parseElementProperties(getJObject(json, "_policyRule"), res.getPolicyRuleElement()); 6375 if (json.has("securityLabel")) { 6376 JsonArray array = json.getAsJsonArray("securityLabel"); 6377 for (int i = 0; i < array.size(); i++) { 6378 res.getSecurityLabel().add(parseCoding(array.get(i).getAsJsonObject())); 6379 } 6380 }; 6381 if (json.has("purpose")) { 6382 JsonArray array = json.getAsJsonArray("purpose"); 6383 for (int i = 0; i < array.size(); i++) { 6384 res.getPurpose().add(parseCoding(array.get(i).getAsJsonObject())); 6385 } 6386 }; 6387 if (json.has("dataPeriod")) 6388 res.setDataPeriod(parsePeriod(getJObject(json, "dataPeriod"))); 6389 if (json.has("data")) { 6390 JsonArray array = json.getAsJsonArray("data"); 6391 for (int i = 0; i < array.size(); i++) { 6392 res.getData().add(parseConsentConsentDataComponent(array.get(i).getAsJsonObject(), res)); 6393 } 6394 }; 6395 if (json.has("except")) { 6396 JsonArray array = json.getAsJsonArray("except"); 6397 for (int i = 0; i < array.size(); i++) { 6398 res.getExcept().add(parseConsentExceptComponent(array.get(i).getAsJsonObject(), res)); 6399 } 6400 }; 6401 } 6402 6403 protected Consent.ConsentActorComponent parseConsentConsentActorComponent(JsonObject json, Consent owner) throws IOException, FHIRFormatError { 6404 Consent.ConsentActorComponent res = new Consent.ConsentActorComponent(); 6405 parseConsentConsentActorComponentProperties(json, owner, res); 6406 return res; 6407 } 6408 6409 protected void parseConsentConsentActorComponentProperties(JsonObject json, Consent owner, Consent.ConsentActorComponent res) throws IOException, FHIRFormatError { 6410 parseBackboneProperties(json, res); 6411 if (json.has("role")) 6412 res.setRole(parseCodeableConcept(getJObject(json, "role"))); 6413 if (json.has("reference")) 6414 res.setReference(parseReference(getJObject(json, "reference"))); 6415 } 6416 6417 protected Consent.ConsentPolicyComponent parseConsentConsentPolicyComponent(JsonObject json, Consent owner) throws IOException, FHIRFormatError { 6418 Consent.ConsentPolicyComponent res = new Consent.ConsentPolicyComponent(); 6419 parseConsentConsentPolicyComponentProperties(json, owner, res); 6420 return res; 6421 } 6422 6423 protected void parseConsentConsentPolicyComponentProperties(JsonObject json, Consent owner, Consent.ConsentPolicyComponent res) throws IOException, FHIRFormatError { 6424 parseBackboneProperties(json, res); 6425 if (json.has("authority")) 6426 res.setAuthorityElement(parseUri(json.get("authority").getAsString())); 6427 if (json.has("_authority")) 6428 parseElementProperties(getJObject(json, "_authority"), res.getAuthorityElement()); 6429 if (json.has("uri")) 6430 res.setUriElement(parseUri(json.get("uri").getAsString())); 6431 if (json.has("_uri")) 6432 parseElementProperties(getJObject(json, "_uri"), res.getUriElement()); 6433 } 6434 6435 protected Consent.ConsentDataComponent parseConsentConsentDataComponent(JsonObject json, Consent owner) throws IOException, FHIRFormatError { 6436 Consent.ConsentDataComponent res = new Consent.ConsentDataComponent(); 6437 parseConsentConsentDataComponentProperties(json, owner, res); 6438 return res; 6439 } 6440 6441 protected void parseConsentConsentDataComponentProperties(JsonObject json, Consent owner, Consent.ConsentDataComponent res) throws IOException, FHIRFormatError { 6442 parseBackboneProperties(json, res); 6443 if (json.has("meaning")) 6444 res.setMeaningElement(parseEnumeration(json.get("meaning").getAsString(), Consent.ConsentDataMeaning.NULL, new Consent.ConsentDataMeaningEnumFactory())); 6445 if (json.has("_meaning")) 6446 parseElementProperties(getJObject(json, "_meaning"), res.getMeaningElement()); 6447 if (json.has("reference")) 6448 res.setReference(parseReference(getJObject(json, "reference"))); 6449 } 6450 6451 protected Consent.ExceptComponent parseConsentExceptComponent(JsonObject json, Consent owner) throws IOException, FHIRFormatError { 6452 Consent.ExceptComponent res = new Consent.ExceptComponent(); 6453 parseConsentExceptComponentProperties(json, owner, res); 6454 return res; 6455 } 6456 6457 protected void parseConsentExceptComponentProperties(JsonObject json, Consent owner, Consent.ExceptComponent res) throws IOException, FHIRFormatError { 6458 parseBackboneProperties(json, res); 6459 if (json.has("type")) 6460 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), Consent.ConsentExceptType.NULL, new Consent.ConsentExceptTypeEnumFactory())); 6461 if (json.has("_type")) 6462 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 6463 if (json.has("period")) 6464 res.setPeriod(parsePeriod(getJObject(json, "period"))); 6465 if (json.has("actor")) { 6466 JsonArray array = json.getAsJsonArray("actor"); 6467 for (int i = 0; i < array.size(); i++) { 6468 res.getActor().add(parseConsentExceptActorComponent(array.get(i).getAsJsonObject(), owner)); 6469 } 6470 }; 6471 if (json.has("action")) { 6472 JsonArray array = json.getAsJsonArray("action"); 6473 for (int i = 0; i < array.size(); i++) { 6474 res.getAction().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 6475 } 6476 }; 6477 if (json.has("securityLabel")) { 6478 JsonArray array = json.getAsJsonArray("securityLabel"); 6479 for (int i = 0; i < array.size(); i++) { 6480 res.getSecurityLabel().add(parseCoding(array.get(i).getAsJsonObject())); 6481 } 6482 }; 6483 if (json.has("purpose")) { 6484 JsonArray array = json.getAsJsonArray("purpose"); 6485 for (int i = 0; i < array.size(); i++) { 6486 res.getPurpose().add(parseCoding(array.get(i).getAsJsonObject())); 6487 } 6488 }; 6489 if (json.has("class")) { 6490 JsonArray array = json.getAsJsonArray("class"); 6491 for (int i = 0; i < array.size(); i++) { 6492 res.getClass_().add(parseCoding(array.get(i).getAsJsonObject())); 6493 } 6494 }; 6495 if (json.has("code")) { 6496 JsonArray array = json.getAsJsonArray("code"); 6497 for (int i = 0; i < array.size(); i++) { 6498 res.getCode().add(parseCoding(array.get(i).getAsJsonObject())); 6499 } 6500 }; 6501 if (json.has("dataPeriod")) 6502 res.setDataPeriod(parsePeriod(getJObject(json, "dataPeriod"))); 6503 if (json.has("data")) { 6504 JsonArray array = json.getAsJsonArray("data"); 6505 for (int i = 0; i < array.size(); i++) { 6506 res.getData().add(parseConsentExceptDataComponent(array.get(i).getAsJsonObject(), owner)); 6507 } 6508 }; 6509 } 6510 6511 protected Consent.ExceptActorComponent parseConsentExceptActorComponent(JsonObject json, Consent owner) throws IOException, FHIRFormatError { 6512 Consent.ExceptActorComponent res = new Consent.ExceptActorComponent(); 6513 parseConsentExceptActorComponentProperties(json, owner, res); 6514 return res; 6515 } 6516 6517 protected void parseConsentExceptActorComponentProperties(JsonObject json, Consent owner, Consent.ExceptActorComponent res) throws IOException, FHIRFormatError { 6518 parseBackboneProperties(json, res); 6519 if (json.has("role")) 6520 res.setRole(parseCodeableConcept(getJObject(json, "role"))); 6521 if (json.has("reference")) 6522 res.setReference(parseReference(getJObject(json, "reference"))); 6523 } 6524 6525 protected Consent.ExceptDataComponent parseConsentExceptDataComponent(JsonObject json, Consent owner) throws IOException, FHIRFormatError { 6526 Consent.ExceptDataComponent res = new Consent.ExceptDataComponent(); 6527 parseConsentExceptDataComponentProperties(json, owner, res); 6528 return res; 6529 } 6530 6531 protected void parseConsentExceptDataComponentProperties(JsonObject json, Consent owner, Consent.ExceptDataComponent res) throws IOException, FHIRFormatError { 6532 parseBackboneProperties(json, res); 6533 if (json.has("meaning")) 6534 res.setMeaningElement(parseEnumeration(json.get("meaning").getAsString(), Consent.ConsentDataMeaning.NULL, new Consent.ConsentDataMeaningEnumFactory())); 6535 if (json.has("_meaning")) 6536 parseElementProperties(getJObject(json, "_meaning"), res.getMeaningElement()); 6537 if (json.has("reference")) 6538 res.setReference(parseReference(getJObject(json, "reference"))); 6539 } 6540 6541 protected Contract parseContract(JsonObject json) throws IOException, FHIRFormatError { 6542 Contract res = new Contract(); 6543 parseContractProperties(json, res); 6544 return res; 6545 } 6546 6547 protected void parseContractProperties(JsonObject json, Contract res) throws IOException, FHIRFormatError { 6548 parseDomainResourceProperties(json, res); 6549 if (json.has("identifier")) 6550 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 6551 if (json.has("status")) 6552 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Contract.ContractStatus.NULL, new Contract.ContractStatusEnumFactory())); 6553 if (json.has("_status")) 6554 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 6555 if (json.has("issued")) 6556 res.setIssuedElement(parseDateTime(json.get("issued").getAsString())); 6557 if (json.has("_issued")) 6558 parseElementProperties(getJObject(json, "_issued"), res.getIssuedElement()); 6559 if (json.has("applies")) 6560 res.setApplies(parsePeriod(getJObject(json, "applies"))); 6561 if (json.has("subject")) { 6562 JsonArray array = json.getAsJsonArray("subject"); 6563 for (int i = 0; i < array.size(); i++) { 6564 res.getSubject().add(parseReference(array.get(i).getAsJsonObject())); 6565 } 6566 }; 6567 if (json.has("topic")) { 6568 JsonArray array = json.getAsJsonArray("topic"); 6569 for (int i = 0; i < array.size(); i++) { 6570 res.getTopic().add(parseReference(array.get(i).getAsJsonObject())); 6571 } 6572 }; 6573 if (json.has("authority")) { 6574 JsonArray array = json.getAsJsonArray("authority"); 6575 for (int i = 0; i < array.size(); i++) { 6576 res.getAuthority().add(parseReference(array.get(i).getAsJsonObject())); 6577 } 6578 }; 6579 if (json.has("domain")) { 6580 JsonArray array = json.getAsJsonArray("domain"); 6581 for (int i = 0; i < array.size(); i++) { 6582 res.getDomain().add(parseReference(array.get(i).getAsJsonObject())); 6583 } 6584 }; 6585 if (json.has("type")) 6586 res.setType(parseCodeableConcept(getJObject(json, "type"))); 6587 if (json.has("subType")) { 6588 JsonArray array = json.getAsJsonArray("subType"); 6589 for (int i = 0; i < array.size(); i++) { 6590 res.getSubType().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 6591 } 6592 }; 6593 if (json.has("action")) { 6594 JsonArray array = json.getAsJsonArray("action"); 6595 for (int i = 0; i < array.size(); i++) { 6596 res.getAction().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 6597 } 6598 }; 6599 if (json.has("actionReason")) { 6600 JsonArray array = json.getAsJsonArray("actionReason"); 6601 for (int i = 0; i < array.size(); i++) { 6602 res.getActionReason().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 6603 } 6604 }; 6605 if (json.has("decisionType")) 6606 res.setDecisionType(parseCodeableConcept(getJObject(json, "decisionType"))); 6607 if (json.has("contentDerivative")) 6608 res.setContentDerivative(parseCodeableConcept(getJObject(json, "contentDerivative"))); 6609 if (json.has("securityLabel")) { 6610 JsonArray array = json.getAsJsonArray("securityLabel"); 6611 for (int i = 0; i < array.size(); i++) { 6612 res.getSecurityLabel().add(parseCoding(array.get(i).getAsJsonObject())); 6613 } 6614 }; 6615 if (json.has("agent")) { 6616 JsonArray array = json.getAsJsonArray("agent"); 6617 for (int i = 0; i < array.size(); i++) { 6618 res.getAgent().add(parseContractAgentComponent(array.get(i).getAsJsonObject(), res)); 6619 } 6620 }; 6621 if (json.has("signer")) { 6622 JsonArray array = json.getAsJsonArray("signer"); 6623 for (int i = 0; i < array.size(); i++) { 6624 res.getSigner().add(parseContractSignatoryComponent(array.get(i).getAsJsonObject(), res)); 6625 } 6626 }; 6627 if (json.has("valuedItem")) { 6628 JsonArray array = json.getAsJsonArray("valuedItem"); 6629 for (int i = 0; i < array.size(); i++) { 6630 res.getValuedItem().add(parseContractValuedItemComponent(array.get(i).getAsJsonObject(), res)); 6631 } 6632 }; 6633 if (json.has("term")) { 6634 JsonArray array = json.getAsJsonArray("term"); 6635 for (int i = 0; i < array.size(); i++) { 6636 res.getTerm().add(parseContractTermComponent(array.get(i).getAsJsonObject(), res)); 6637 } 6638 }; 6639 Type binding = parseType("binding", json); 6640 if (binding != null) 6641 res.setBinding(binding); 6642 if (json.has("friendly")) { 6643 JsonArray array = json.getAsJsonArray("friendly"); 6644 for (int i = 0; i < array.size(); i++) { 6645 res.getFriendly().add(parseContractFriendlyLanguageComponent(array.get(i).getAsJsonObject(), res)); 6646 } 6647 }; 6648 if (json.has("legal")) { 6649 JsonArray array = json.getAsJsonArray("legal"); 6650 for (int i = 0; i < array.size(); i++) { 6651 res.getLegal().add(parseContractLegalLanguageComponent(array.get(i).getAsJsonObject(), res)); 6652 } 6653 }; 6654 if (json.has("rule")) { 6655 JsonArray array = json.getAsJsonArray("rule"); 6656 for (int i = 0; i < array.size(); i++) { 6657 res.getRule().add(parseContractComputableLanguageComponent(array.get(i).getAsJsonObject(), res)); 6658 } 6659 }; 6660 } 6661 6662 protected Contract.AgentComponent parseContractAgentComponent(JsonObject json, Contract owner) throws IOException, FHIRFormatError { 6663 Contract.AgentComponent res = new Contract.AgentComponent(); 6664 parseContractAgentComponentProperties(json, owner, res); 6665 return res; 6666 } 6667 6668 protected void parseContractAgentComponentProperties(JsonObject json, Contract owner, Contract.AgentComponent res) throws IOException, FHIRFormatError { 6669 parseBackboneProperties(json, res); 6670 if (json.has("actor")) 6671 res.setActor(parseReference(getJObject(json, "actor"))); 6672 if (json.has("role")) { 6673 JsonArray array = json.getAsJsonArray("role"); 6674 for (int i = 0; i < array.size(); i++) { 6675 res.getRole().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 6676 } 6677 }; 6678 } 6679 6680 protected Contract.SignatoryComponent parseContractSignatoryComponent(JsonObject json, Contract owner) throws IOException, FHIRFormatError { 6681 Contract.SignatoryComponent res = new Contract.SignatoryComponent(); 6682 parseContractSignatoryComponentProperties(json, owner, res); 6683 return res; 6684 } 6685 6686 protected void parseContractSignatoryComponentProperties(JsonObject json, Contract owner, Contract.SignatoryComponent res) throws IOException, FHIRFormatError { 6687 parseBackboneProperties(json, res); 6688 if (json.has("type")) 6689 res.setType(parseCoding(getJObject(json, "type"))); 6690 if (json.has("party")) 6691 res.setParty(parseReference(getJObject(json, "party"))); 6692 if (json.has("signature")) { 6693 JsonArray array = json.getAsJsonArray("signature"); 6694 for (int i = 0; i < array.size(); i++) { 6695 res.getSignature().add(parseSignature(array.get(i).getAsJsonObject())); 6696 } 6697 }; 6698 } 6699 6700 protected Contract.ValuedItemComponent parseContractValuedItemComponent(JsonObject json, Contract owner) throws IOException, FHIRFormatError { 6701 Contract.ValuedItemComponent res = new Contract.ValuedItemComponent(); 6702 parseContractValuedItemComponentProperties(json, owner, res); 6703 return res; 6704 } 6705 6706 protected void parseContractValuedItemComponentProperties(JsonObject json, Contract owner, Contract.ValuedItemComponent res) throws IOException, FHIRFormatError { 6707 parseBackboneProperties(json, res); 6708 Type entity = parseType("entity", json); 6709 if (entity != null) 6710 res.setEntity(entity); 6711 if (json.has("identifier")) 6712 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 6713 if (json.has("effectiveTime")) 6714 res.setEffectiveTimeElement(parseDateTime(json.get("effectiveTime").getAsString())); 6715 if (json.has("_effectiveTime")) 6716 parseElementProperties(getJObject(json, "_effectiveTime"), res.getEffectiveTimeElement()); 6717 if (json.has("quantity")) 6718 res.setQuantity(parseSimpleQuantity(getJObject(json, "quantity"))); 6719 if (json.has("unitPrice")) 6720 res.setUnitPrice(parseMoney(getJObject(json, "unitPrice"))); 6721 if (json.has("factor")) 6722 res.setFactorElement(parseDecimal(json.get("factor").getAsBigDecimal())); 6723 if (json.has("_factor")) 6724 parseElementProperties(getJObject(json, "_factor"), res.getFactorElement()); 6725 if (json.has("points")) 6726 res.setPointsElement(parseDecimal(json.get("points").getAsBigDecimal())); 6727 if (json.has("_points")) 6728 parseElementProperties(getJObject(json, "_points"), res.getPointsElement()); 6729 if (json.has("net")) 6730 res.setNet(parseMoney(getJObject(json, "net"))); 6731 } 6732 6733 protected Contract.TermComponent parseContractTermComponent(JsonObject json, Contract owner) throws IOException, FHIRFormatError { 6734 Contract.TermComponent res = new Contract.TermComponent(); 6735 parseContractTermComponentProperties(json, owner, res); 6736 return res; 6737 } 6738 6739 protected void parseContractTermComponentProperties(JsonObject json, Contract owner, Contract.TermComponent res) throws IOException, FHIRFormatError { 6740 parseBackboneProperties(json, res); 6741 if (json.has("identifier")) 6742 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 6743 if (json.has("issued")) 6744 res.setIssuedElement(parseDateTime(json.get("issued").getAsString())); 6745 if (json.has("_issued")) 6746 parseElementProperties(getJObject(json, "_issued"), res.getIssuedElement()); 6747 if (json.has("applies")) 6748 res.setApplies(parsePeriod(getJObject(json, "applies"))); 6749 if (json.has("type")) 6750 res.setType(parseCodeableConcept(getJObject(json, "type"))); 6751 if (json.has("subType")) 6752 res.setSubType(parseCodeableConcept(getJObject(json, "subType"))); 6753 if (json.has("topic")) { 6754 JsonArray array = json.getAsJsonArray("topic"); 6755 for (int i = 0; i < array.size(); i++) { 6756 res.getTopic().add(parseReference(array.get(i).getAsJsonObject())); 6757 } 6758 }; 6759 if (json.has("action")) { 6760 JsonArray array = json.getAsJsonArray("action"); 6761 for (int i = 0; i < array.size(); i++) { 6762 res.getAction().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 6763 } 6764 }; 6765 if (json.has("actionReason")) { 6766 JsonArray array = json.getAsJsonArray("actionReason"); 6767 for (int i = 0; i < array.size(); i++) { 6768 res.getActionReason().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 6769 } 6770 }; 6771 if (json.has("securityLabel")) { 6772 JsonArray array = json.getAsJsonArray("securityLabel"); 6773 for (int i = 0; i < array.size(); i++) { 6774 res.getSecurityLabel().add(parseCoding(array.get(i).getAsJsonObject())); 6775 } 6776 }; 6777 if (json.has("agent")) { 6778 JsonArray array = json.getAsJsonArray("agent"); 6779 for (int i = 0; i < array.size(); i++) { 6780 res.getAgent().add(parseContractTermAgentComponent(array.get(i).getAsJsonObject(), owner)); 6781 } 6782 }; 6783 if (json.has("text")) 6784 res.setTextElement(parseString(json.get("text").getAsString())); 6785 if (json.has("_text")) 6786 parseElementProperties(getJObject(json, "_text"), res.getTextElement()); 6787 if (json.has("valuedItem")) { 6788 JsonArray array = json.getAsJsonArray("valuedItem"); 6789 for (int i = 0; i < array.size(); i++) { 6790 res.getValuedItem().add(parseContractTermValuedItemComponent(array.get(i).getAsJsonObject(), owner)); 6791 } 6792 }; 6793 if (json.has("group")) { 6794 JsonArray array = json.getAsJsonArray("group"); 6795 for (int i = 0; i < array.size(); i++) { 6796 res.getGroup().add(parseContractTermComponent(array.get(i).getAsJsonObject(), owner)); 6797 } 6798 }; 6799 } 6800 6801 protected Contract.TermAgentComponent parseContractTermAgentComponent(JsonObject json, Contract owner) throws IOException, FHIRFormatError { 6802 Contract.TermAgentComponent res = new Contract.TermAgentComponent(); 6803 parseContractTermAgentComponentProperties(json, owner, res); 6804 return res; 6805 } 6806 6807 protected void parseContractTermAgentComponentProperties(JsonObject json, Contract owner, Contract.TermAgentComponent res) throws IOException, FHIRFormatError { 6808 parseBackboneProperties(json, res); 6809 if (json.has("actor")) 6810 res.setActor(parseReference(getJObject(json, "actor"))); 6811 if (json.has("role")) { 6812 JsonArray array = json.getAsJsonArray("role"); 6813 for (int i = 0; i < array.size(); i++) { 6814 res.getRole().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 6815 } 6816 }; 6817 } 6818 6819 protected Contract.TermValuedItemComponent parseContractTermValuedItemComponent(JsonObject json, Contract owner) throws IOException, FHIRFormatError { 6820 Contract.TermValuedItemComponent res = new Contract.TermValuedItemComponent(); 6821 parseContractTermValuedItemComponentProperties(json, owner, res); 6822 return res; 6823 } 6824 6825 protected void parseContractTermValuedItemComponentProperties(JsonObject json, Contract owner, Contract.TermValuedItemComponent res) throws IOException, FHIRFormatError { 6826 parseBackboneProperties(json, res); 6827 Type entity = parseType("entity", json); 6828 if (entity != null) 6829 res.setEntity(entity); 6830 if (json.has("identifier")) 6831 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 6832 if (json.has("effectiveTime")) 6833 res.setEffectiveTimeElement(parseDateTime(json.get("effectiveTime").getAsString())); 6834 if (json.has("_effectiveTime")) 6835 parseElementProperties(getJObject(json, "_effectiveTime"), res.getEffectiveTimeElement()); 6836 if (json.has("quantity")) 6837 res.setQuantity(parseSimpleQuantity(getJObject(json, "quantity"))); 6838 if (json.has("unitPrice")) 6839 res.setUnitPrice(parseMoney(getJObject(json, "unitPrice"))); 6840 if (json.has("factor")) 6841 res.setFactorElement(parseDecimal(json.get("factor").getAsBigDecimal())); 6842 if (json.has("_factor")) 6843 parseElementProperties(getJObject(json, "_factor"), res.getFactorElement()); 6844 if (json.has("points")) 6845 res.setPointsElement(parseDecimal(json.get("points").getAsBigDecimal())); 6846 if (json.has("_points")) 6847 parseElementProperties(getJObject(json, "_points"), res.getPointsElement()); 6848 if (json.has("net")) 6849 res.setNet(parseMoney(getJObject(json, "net"))); 6850 } 6851 6852 protected Contract.FriendlyLanguageComponent parseContractFriendlyLanguageComponent(JsonObject json, Contract owner) throws IOException, FHIRFormatError { 6853 Contract.FriendlyLanguageComponent res = new Contract.FriendlyLanguageComponent(); 6854 parseContractFriendlyLanguageComponentProperties(json, owner, res); 6855 return res; 6856 } 6857 6858 protected void parseContractFriendlyLanguageComponentProperties(JsonObject json, Contract owner, Contract.FriendlyLanguageComponent res) throws IOException, FHIRFormatError { 6859 parseBackboneProperties(json, res); 6860 Type content = parseType("content", json); 6861 if (content != null) 6862 res.setContent(content); 6863 } 6864 6865 protected Contract.LegalLanguageComponent parseContractLegalLanguageComponent(JsonObject json, Contract owner) throws IOException, FHIRFormatError { 6866 Contract.LegalLanguageComponent res = new Contract.LegalLanguageComponent(); 6867 parseContractLegalLanguageComponentProperties(json, owner, res); 6868 return res; 6869 } 6870 6871 protected void parseContractLegalLanguageComponentProperties(JsonObject json, Contract owner, Contract.LegalLanguageComponent res) throws IOException, FHIRFormatError { 6872 parseBackboneProperties(json, res); 6873 Type content = parseType("content", json); 6874 if (content != null) 6875 res.setContent(content); 6876 } 6877 6878 protected Contract.ComputableLanguageComponent parseContractComputableLanguageComponent(JsonObject json, Contract owner) throws IOException, FHIRFormatError { 6879 Contract.ComputableLanguageComponent res = new Contract.ComputableLanguageComponent(); 6880 parseContractComputableLanguageComponentProperties(json, owner, res); 6881 return res; 6882 } 6883 6884 protected void parseContractComputableLanguageComponentProperties(JsonObject json, Contract owner, Contract.ComputableLanguageComponent res) throws IOException, FHIRFormatError { 6885 parseBackboneProperties(json, res); 6886 Type content = parseType("content", json); 6887 if (content != null) 6888 res.setContent(content); 6889 } 6890 6891 protected Coverage parseCoverage(JsonObject json) throws IOException, FHIRFormatError { 6892 Coverage res = new Coverage(); 6893 parseCoverageProperties(json, res); 6894 return res; 6895 } 6896 6897 protected void parseCoverageProperties(JsonObject json, Coverage res) throws IOException, FHIRFormatError { 6898 parseDomainResourceProperties(json, res); 6899 if (json.has("identifier")) { 6900 JsonArray array = json.getAsJsonArray("identifier"); 6901 for (int i = 0; i < array.size(); i++) { 6902 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 6903 } 6904 }; 6905 if (json.has("status")) 6906 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Coverage.CoverageStatus.NULL, new Coverage.CoverageStatusEnumFactory())); 6907 if (json.has("_status")) 6908 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 6909 if (json.has("type")) 6910 res.setType(parseCodeableConcept(getJObject(json, "type"))); 6911 if (json.has("policyHolder")) 6912 res.setPolicyHolder(parseReference(getJObject(json, "policyHolder"))); 6913 if (json.has("subscriber")) 6914 res.setSubscriber(parseReference(getJObject(json, "subscriber"))); 6915 if (json.has("subscriberId")) 6916 res.setSubscriberIdElement(parseString(json.get("subscriberId").getAsString())); 6917 if (json.has("_subscriberId")) 6918 parseElementProperties(getJObject(json, "_subscriberId"), res.getSubscriberIdElement()); 6919 if (json.has("beneficiary")) 6920 res.setBeneficiary(parseReference(getJObject(json, "beneficiary"))); 6921 if (json.has("relationship")) 6922 res.setRelationship(parseCodeableConcept(getJObject(json, "relationship"))); 6923 if (json.has("period")) 6924 res.setPeriod(parsePeriod(getJObject(json, "period"))); 6925 if (json.has("payor")) { 6926 JsonArray array = json.getAsJsonArray("payor"); 6927 for (int i = 0; i < array.size(); i++) { 6928 res.getPayor().add(parseReference(array.get(i).getAsJsonObject())); 6929 } 6930 }; 6931 if (json.has("grouping")) 6932 res.setGrouping(parseCoverageGroupComponent(getJObject(json, "grouping"), res)); 6933 if (json.has("dependent")) 6934 res.setDependentElement(parseString(json.get("dependent").getAsString())); 6935 if (json.has("_dependent")) 6936 parseElementProperties(getJObject(json, "_dependent"), res.getDependentElement()); 6937 if (json.has("sequence")) 6938 res.setSequenceElement(parseString(json.get("sequence").getAsString())); 6939 if (json.has("_sequence")) 6940 parseElementProperties(getJObject(json, "_sequence"), res.getSequenceElement()); 6941 if (json.has("order")) 6942 res.setOrderElement(parsePositiveInt(json.get("order").getAsString())); 6943 if (json.has("_order")) 6944 parseElementProperties(getJObject(json, "_order"), res.getOrderElement()); 6945 if (json.has("network")) 6946 res.setNetworkElement(parseString(json.get("network").getAsString())); 6947 if (json.has("_network")) 6948 parseElementProperties(getJObject(json, "_network"), res.getNetworkElement()); 6949 if (json.has("contract")) { 6950 JsonArray array = json.getAsJsonArray("contract"); 6951 for (int i = 0; i < array.size(); i++) { 6952 res.getContract().add(parseReference(array.get(i).getAsJsonObject())); 6953 } 6954 }; 6955 } 6956 6957 protected Coverage.GroupComponent parseCoverageGroupComponent(JsonObject json, Coverage owner) throws IOException, FHIRFormatError { 6958 Coverage.GroupComponent res = new Coverage.GroupComponent(); 6959 parseCoverageGroupComponentProperties(json, owner, res); 6960 return res; 6961 } 6962 6963 protected void parseCoverageGroupComponentProperties(JsonObject json, Coverage owner, Coverage.GroupComponent res) throws IOException, FHIRFormatError { 6964 parseBackboneProperties(json, res); 6965 if (json.has("group")) 6966 res.setGroupElement(parseString(json.get("group").getAsString())); 6967 if (json.has("_group")) 6968 parseElementProperties(getJObject(json, "_group"), res.getGroupElement()); 6969 if (json.has("groupDisplay")) 6970 res.setGroupDisplayElement(parseString(json.get("groupDisplay").getAsString())); 6971 if (json.has("_groupDisplay")) 6972 parseElementProperties(getJObject(json, "_groupDisplay"), res.getGroupDisplayElement()); 6973 if (json.has("subGroup")) 6974 res.setSubGroupElement(parseString(json.get("subGroup").getAsString())); 6975 if (json.has("_subGroup")) 6976 parseElementProperties(getJObject(json, "_subGroup"), res.getSubGroupElement()); 6977 if (json.has("subGroupDisplay")) 6978 res.setSubGroupDisplayElement(parseString(json.get("subGroupDisplay").getAsString())); 6979 if (json.has("_subGroupDisplay")) 6980 parseElementProperties(getJObject(json, "_subGroupDisplay"), res.getSubGroupDisplayElement()); 6981 if (json.has("plan")) 6982 res.setPlanElement(parseString(json.get("plan").getAsString())); 6983 if (json.has("_plan")) 6984 parseElementProperties(getJObject(json, "_plan"), res.getPlanElement()); 6985 if (json.has("planDisplay")) 6986 res.setPlanDisplayElement(parseString(json.get("planDisplay").getAsString())); 6987 if (json.has("_planDisplay")) 6988 parseElementProperties(getJObject(json, "_planDisplay"), res.getPlanDisplayElement()); 6989 if (json.has("subPlan")) 6990 res.setSubPlanElement(parseString(json.get("subPlan").getAsString())); 6991 if (json.has("_subPlan")) 6992 parseElementProperties(getJObject(json, "_subPlan"), res.getSubPlanElement()); 6993 if (json.has("subPlanDisplay")) 6994 res.setSubPlanDisplayElement(parseString(json.get("subPlanDisplay").getAsString())); 6995 if (json.has("_subPlanDisplay")) 6996 parseElementProperties(getJObject(json, "_subPlanDisplay"), res.getSubPlanDisplayElement()); 6997 if (json.has("class")) 6998 res.setClass_Element(parseString(json.get("class").getAsString())); 6999 if (json.has("_class")) 7000 parseElementProperties(getJObject(json, "_class"), res.getClass_Element()); 7001 if (json.has("classDisplay")) 7002 res.setClassDisplayElement(parseString(json.get("classDisplay").getAsString())); 7003 if (json.has("_classDisplay")) 7004 parseElementProperties(getJObject(json, "_classDisplay"), res.getClassDisplayElement()); 7005 if (json.has("subClass")) 7006 res.setSubClassElement(parseString(json.get("subClass").getAsString())); 7007 if (json.has("_subClass")) 7008 parseElementProperties(getJObject(json, "_subClass"), res.getSubClassElement()); 7009 if (json.has("subClassDisplay")) 7010 res.setSubClassDisplayElement(parseString(json.get("subClassDisplay").getAsString())); 7011 if (json.has("_subClassDisplay")) 7012 parseElementProperties(getJObject(json, "_subClassDisplay"), res.getSubClassDisplayElement()); 7013 } 7014 7015 protected DataElement parseDataElement(JsonObject json) throws IOException, FHIRFormatError { 7016 DataElement res = new DataElement(); 7017 parseDataElementProperties(json, res); 7018 return res; 7019 } 7020 7021 protected void parseDataElementProperties(JsonObject json, DataElement res) throws IOException, FHIRFormatError { 7022 parseDomainResourceProperties(json, res); 7023 if (json.has("url")) 7024 res.setUrlElement(parseUri(json.get("url").getAsString())); 7025 if (json.has("_url")) 7026 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 7027 if (json.has("identifier")) { 7028 JsonArray array = json.getAsJsonArray("identifier"); 7029 for (int i = 0; i < array.size(); i++) { 7030 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 7031 } 7032 }; 7033 if (json.has("version")) 7034 res.setVersionElement(parseString(json.get("version").getAsString())); 7035 if (json.has("_version")) 7036 parseElementProperties(getJObject(json, "_version"), res.getVersionElement()); 7037 if (json.has("status")) 7038 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Enumerations.PublicationStatus.NULL, new Enumerations.PublicationStatusEnumFactory())); 7039 if (json.has("_status")) 7040 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 7041 if (json.has("experimental")) 7042 res.setExperimentalElement(parseBoolean(json.get("experimental").getAsBoolean())); 7043 if (json.has("_experimental")) 7044 parseElementProperties(getJObject(json, "_experimental"), res.getExperimentalElement()); 7045 if (json.has("date")) 7046 res.setDateElement(parseDateTime(json.get("date").getAsString())); 7047 if (json.has("_date")) 7048 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 7049 if (json.has("publisher")) 7050 res.setPublisherElement(parseString(json.get("publisher").getAsString())); 7051 if (json.has("_publisher")) 7052 parseElementProperties(getJObject(json, "_publisher"), res.getPublisherElement()); 7053 if (json.has("name")) 7054 res.setNameElement(parseString(json.get("name").getAsString())); 7055 if (json.has("_name")) 7056 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 7057 if (json.has("title")) 7058 res.setTitleElement(parseString(json.get("title").getAsString())); 7059 if (json.has("_title")) 7060 parseElementProperties(getJObject(json, "_title"), res.getTitleElement()); 7061 if (json.has("contact")) { 7062 JsonArray array = json.getAsJsonArray("contact"); 7063 for (int i = 0; i < array.size(); i++) { 7064 res.getContact().add(parseContactDetail(array.get(i).getAsJsonObject())); 7065 } 7066 }; 7067 if (json.has("useContext")) { 7068 JsonArray array = json.getAsJsonArray("useContext"); 7069 for (int i = 0; i < array.size(); i++) { 7070 res.getUseContext().add(parseUsageContext(array.get(i).getAsJsonObject())); 7071 } 7072 }; 7073 if (json.has("jurisdiction")) { 7074 JsonArray array = json.getAsJsonArray("jurisdiction"); 7075 for (int i = 0; i < array.size(); i++) { 7076 res.getJurisdiction().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 7077 } 7078 }; 7079 if (json.has("copyright")) 7080 res.setCopyrightElement(parseMarkdown(json.get("copyright").getAsString())); 7081 if (json.has("_copyright")) 7082 parseElementProperties(getJObject(json, "_copyright"), res.getCopyrightElement()); 7083 if (json.has("stringency")) 7084 res.setStringencyElement(parseEnumeration(json.get("stringency").getAsString(), DataElement.DataElementStringency.NULL, new DataElement.DataElementStringencyEnumFactory())); 7085 if (json.has("_stringency")) 7086 parseElementProperties(getJObject(json, "_stringency"), res.getStringencyElement()); 7087 if (json.has("mapping")) { 7088 JsonArray array = json.getAsJsonArray("mapping"); 7089 for (int i = 0; i < array.size(); i++) { 7090 res.getMapping().add(parseDataElementDataElementMappingComponent(array.get(i).getAsJsonObject(), res)); 7091 } 7092 }; 7093 if (json.has("element")) { 7094 JsonArray array = json.getAsJsonArray("element"); 7095 for (int i = 0; i < array.size(); i++) { 7096 res.getElement().add(parseElementDefinition(array.get(i).getAsJsonObject())); 7097 } 7098 }; 7099 } 7100 7101 protected DataElement.DataElementMappingComponent parseDataElementDataElementMappingComponent(JsonObject json, DataElement owner) throws IOException, FHIRFormatError { 7102 DataElement.DataElementMappingComponent res = new DataElement.DataElementMappingComponent(); 7103 parseDataElementDataElementMappingComponentProperties(json, owner, res); 7104 return res; 7105 } 7106 7107 protected void parseDataElementDataElementMappingComponentProperties(JsonObject json, DataElement owner, DataElement.DataElementMappingComponent res) throws IOException, FHIRFormatError { 7108 parseBackboneProperties(json, res); 7109 if (json.has("identity")) 7110 res.setIdentityElement(parseId(json.get("identity").getAsString())); 7111 if (json.has("_identity")) 7112 parseElementProperties(getJObject(json, "_identity"), res.getIdentityElement()); 7113 if (json.has("uri")) 7114 res.setUriElement(parseUri(json.get("uri").getAsString())); 7115 if (json.has("_uri")) 7116 parseElementProperties(getJObject(json, "_uri"), res.getUriElement()); 7117 if (json.has("name")) 7118 res.setNameElement(parseString(json.get("name").getAsString())); 7119 if (json.has("_name")) 7120 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 7121 if (json.has("comment")) 7122 res.setCommentElement(parseString(json.get("comment").getAsString())); 7123 if (json.has("_comment")) 7124 parseElementProperties(getJObject(json, "_comment"), res.getCommentElement()); 7125 } 7126 7127 protected DetectedIssue parseDetectedIssue(JsonObject json) throws IOException, FHIRFormatError { 7128 DetectedIssue res = new DetectedIssue(); 7129 parseDetectedIssueProperties(json, res); 7130 return res; 7131 } 7132 7133 protected void parseDetectedIssueProperties(JsonObject json, DetectedIssue res) throws IOException, FHIRFormatError { 7134 parseDomainResourceProperties(json, res); 7135 if (json.has("identifier")) 7136 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 7137 if (json.has("status")) 7138 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), DetectedIssue.DetectedIssueStatus.NULL, new DetectedIssue.DetectedIssueStatusEnumFactory())); 7139 if (json.has("_status")) 7140 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 7141 if (json.has("category")) 7142 res.setCategory(parseCodeableConcept(getJObject(json, "category"))); 7143 if (json.has("severity")) 7144 res.setSeverityElement(parseEnumeration(json.get("severity").getAsString(), DetectedIssue.DetectedIssueSeverity.NULL, new DetectedIssue.DetectedIssueSeverityEnumFactory())); 7145 if (json.has("_severity")) 7146 parseElementProperties(getJObject(json, "_severity"), res.getSeverityElement()); 7147 if (json.has("patient")) 7148 res.setPatient(parseReference(getJObject(json, "patient"))); 7149 if (json.has("date")) 7150 res.setDateElement(parseDateTime(json.get("date").getAsString())); 7151 if (json.has("_date")) 7152 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 7153 if (json.has("author")) 7154 res.setAuthor(parseReference(getJObject(json, "author"))); 7155 if (json.has("implicated")) { 7156 JsonArray array = json.getAsJsonArray("implicated"); 7157 for (int i = 0; i < array.size(); i++) { 7158 res.getImplicated().add(parseReference(array.get(i).getAsJsonObject())); 7159 } 7160 }; 7161 if (json.has("detail")) 7162 res.setDetailElement(parseString(json.get("detail").getAsString())); 7163 if (json.has("_detail")) 7164 parseElementProperties(getJObject(json, "_detail"), res.getDetailElement()); 7165 if (json.has("reference")) 7166 res.setReferenceElement(parseUri(json.get("reference").getAsString())); 7167 if (json.has("_reference")) 7168 parseElementProperties(getJObject(json, "_reference"), res.getReferenceElement()); 7169 if (json.has("mitigation")) { 7170 JsonArray array = json.getAsJsonArray("mitigation"); 7171 for (int i = 0; i < array.size(); i++) { 7172 res.getMitigation().add(parseDetectedIssueDetectedIssueMitigationComponent(array.get(i).getAsJsonObject(), res)); 7173 } 7174 }; 7175 } 7176 7177 protected DetectedIssue.DetectedIssueMitigationComponent parseDetectedIssueDetectedIssueMitigationComponent(JsonObject json, DetectedIssue owner) throws IOException, FHIRFormatError { 7178 DetectedIssue.DetectedIssueMitigationComponent res = new DetectedIssue.DetectedIssueMitigationComponent(); 7179 parseDetectedIssueDetectedIssueMitigationComponentProperties(json, owner, res); 7180 return res; 7181 } 7182 7183 protected void parseDetectedIssueDetectedIssueMitigationComponentProperties(JsonObject json, DetectedIssue owner, DetectedIssue.DetectedIssueMitigationComponent res) throws IOException, FHIRFormatError { 7184 parseBackboneProperties(json, res); 7185 if (json.has("action")) 7186 res.setAction(parseCodeableConcept(getJObject(json, "action"))); 7187 if (json.has("date")) 7188 res.setDateElement(parseDateTime(json.get("date").getAsString())); 7189 if (json.has("_date")) 7190 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 7191 if (json.has("author")) 7192 res.setAuthor(parseReference(getJObject(json, "author"))); 7193 } 7194 7195 protected Device parseDevice(JsonObject json) throws IOException, FHIRFormatError { 7196 Device res = new Device(); 7197 parseDeviceProperties(json, res); 7198 return res; 7199 } 7200 7201 protected void parseDeviceProperties(JsonObject json, Device res) throws IOException, FHIRFormatError { 7202 parseDomainResourceProperties(json, res); 7203 if (json.has("identifier")) { 7204 JsonArray array = json.getAsJsonArray("identifier"); 7205 for (int i = 0; i < array.size(); i++) { 7206 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 7207 } 7208 }; 7209 if (json.has("udi")) 7210 res.setUdi(parseDeviceDeviceUdiComponent(getJObject(json, "udi"), res)); 7211 if (json.has("status")) 7212 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Device.FHIRDeviceStatus.NULL, new Device.FHIRDeviceStatusEnumFactory())); 7213 if (json.has("_status")) 7214 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 7215 if (json.has("type")) 7216 res.setType(parseCodeableConcept(getJObject(json, "type"))); 7217 if (json.has("lotNumber")) 7218 res.setLotNumberElement(parseString(json.get("lotNumber").getAsString())); 7219 if (json.has("_lotNumber")) 7220 parseElementProperties(getJObject(json, "_lotNumber"), res.getLotNumberElement()); 7221 if (json.has("manufacturer")) 7222 res.setManufacturerElement(parseString(json.get("manufacturer").getAsString())); 7223 if (json.has("_manufacturer")) 7224 parseElementProperties(getJObject(json, "_manufacturer"), res.getManufacturerElement()); 7225 if (json.has("manufactureDate")) 7226 res.setManufactureDateElement(parseDateTime(json.get("manufactureDate").getAsString())); 7227 if (json.has("_manufactureDate")) 7228 parseElementProperties(getJObject(json, "_manufactureDate"), res.getManufactureDateElement()); 7229 if (json.has("expirationDate")) 7230 res.setExpirationDateElement(parseDateTime(json.get("expirationDate").getAsString())); 7231 if (json.has("_expirationDate")) 7232 parseElementProperties(getJObject(json, "_expirationDate"), res.getExpirationDateElement()); 7233 if (json.has("model")) 7234 res.setModelElement(parseString(json.get("model").getAsString())); 7235 if (json.has("_model")) 7236 parseElementProperties(getJObject(json, "_model"), res.getModelElement()); 7237 if (json.has("version")) 7238 res.setVersionElement(parseString(json.get("version").getAsString())); 7239 if (json.has("_version")) 7240 parseElementProperties(getJObject(json, "_version"), res.getVersionElement()); 7241 if (json.has("patient")) 7242 res.setPatient(parseReference(getJObject(json, "patient"))); 7243 if (json.has("owner")) 7244 res.setOwner(parseReference(getJObject(json, "owner"))); 7245 if (json.has("contact")) { 7246 JsonArray array = json.getAsJsonArray("contact"); 7247 for (int i = 0; i < array.size(); i++) { 7248 res.getContact().add(parseContactPoint(array.get(i).getAsJsonObject())); 7249 } 7250 }; 7251 if (json.has("location")) 7252 res.setLocation(parseReference(getJObject(json, "location"))); 7253 if (json.has("url")) 7254 res.setUrlElement(parseUri(json.get("url").getAsString())); 7255 if (json.has("_url")) 7256 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 7257 if (json.has("note")) { 7258 JsonArray array = json.getAsJsonArray("note"); 7259 for (int i = 0; i < array.size(); i++) { 7260 res.getNote().add(parseAnnotation(array.get(i).getAsJsonObject())); 7261 } 7262 }; 7263 if (json.has("safety")) { 7264 JsonArray array = json.getAsJsonArray("safety"); 7265 for (int i = 0; i < array.size(); i++) { 7266 res.getSafety().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 7267 } 7268 }; 7269 } 7270 7271 protected Device.DeviceUdiComponent parseDeviceDeviceUdiComponent(JsonObject json, Device owner) throws IOException, FHIRFormatError { 7272 Device.DeviceUdiComponent res = new Device.DeviceUdiComponent(); 7273 parseDeviceDeviceUdiComponentProperties(json, owner, res); 7274 return res; 7275 } 7276 7277 protected void parseDeviceDeviceUdiComponentProperties(JsonObject json, Device owner, Device.DeviceUdiComponent res) throws IOException, FHIRFormatError { 7278 parseBackboneProperties(json, res); 7279 if (json.has("deviceIdentifier")) 7280 res.setDeviceIdentifierElement(parseString(json.get("deviceIdentifier").getAsString())); 7281 if (json.has("_deviceIdentifier")) 7282 parseElementProperties(getJObject(json, "_deviceIdentifier"), res.getDeviceIdentifierElement()); 7283 if (json.has("name")) 7284 res.setNameElement(parseString(json.get("name").getAsString())); 7285 if (json.has("_name")) 7286 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 7287 if (json.has("jurisdiction")) 7288 res.setJurisdictionElement(parseUri(json.get("jurisdiction").getAsString())); 7289 if (json.has("_jurisdiction")) 7290 parseElementProperties(getJObject(json, "_jurisdiction"), res.getJurisdictionElement()); 7291 if (json.has("carrierHRF")) 7292 res.setCarrierHRFElement(parseString(json.get("carrierHRF").getAsString())); 7293 if (json.has("_carrierHRF")) 7294 parseElementProperties(getJObject(json, "_carrierHRF"), res.getCarrierHRFElement()); 7295 if (json.has("carrierAIDC")) 7296 res.setCarrierAIDCElement(parseBase64Binary(json.get("carrierAIDC").getAsString())); 7297 if (json.has("_carrierAIDC")) 7298 parseElementProperties(getJObject(json, "_carrierAIDC"), res.getCarrierAIDCElement()); 7299 if (json.has("issuer")) 7300 res.setIssuerElement(parseUri(json.get("issuer").getAsString())); 7301 if (json.has("_issuer")) 7302 parseElementProperties(getJObject(json, "_issuer"), res.getIssuerElement()); 7303 if (json.has("entryType")) 7304 res.setEntryTypeElement(parseEnumeration(json.get("entryType").getAsString(), Device.UDIEntryType.NULL, new Device.UDIEntryTypeEnumFactory())); 7305 if (json.has("_entryType")) 7306 parseElementProperties(getJObject(json, "_entryType"), res.getEntryTypeElement()); 7307 } 7308 7309 protected DeviceComponent parseDeviceComponent(JsonObject json) throws IOException, FHIRFormatError { 7310 DeviceComponent res = new DeviceComponent(); 7311 parseDeviceComponentProperties(json, res); 7312 return res; 7313 } 7314 7315 protected void parseDeviceComponentProperties(JsonObject json, DeviceComponent res) throws IOException, FHIRFormatError { 7316 parseDomainResourceProperties(json, res); 7317 if (json.has("identifier")) 7318 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 7319 if (json.has("type")) 7320 res.setType(parseCodeableConcept(getJObject(json, "type"))); 7321 if (json.has("lastSystemChange")) 7322 res.setLastSystemChangeElement(parseInstant(json.get("lastSystemChange").getAsString())); 7323 if (json.has("_lastSystemChange")) 7324 parseElementProperties(getJObject(json, "_lastSystemChange"), res.getLastSystemChangeElement()); 7325 if (json.has("source")) 7326 res.setSource(parseReference(getJObject(json, "source"))); 7327 if (json.has("parent")) 7328 res.setParent(parseReference(getJObject(json, "parent"))); 7329 if (json.has("operationalStatus")) { 7330 JsonArray array = json.getAsJsonArray("operationalStatus"); 7331 for (int i = 0; i < array.size(); i++) { 7332 res.getOperationalStatus().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 7333 } 7334 }; 7335 if (json.has("parameterGroup")) 7336 res.setParameterGroup(parseCodeableConcept(getJObject(json, "parameterGroup"))); 7337 if (json.has("measurementPrinciple")) 7338 res.setMeasurementPrincipleElement(parseEnumeration(json.get("measurementPrinciple").getAsString(), DeviceComponent.MeasmntPrinciple.NULL, new DeviceComponent.MeasmntPrincipleEnumFactory())); 7339 if (json.has("_measurementPrinciple")) 7340 parseElementProperties(getJObject(json, "_measurementPrinciple"), res.getMeasurementPrincipleElement()); 7341 if (json.has("productionSpecification")) { 7342 JsonArray array = json.getAsJsonArray("productionSpecification"); 7343 for (int i = 0; i < array.size(); i++) { 7344 res.getProductionSpecification().add(parseDeviceComponentDeviceComponentProductionSpecificationComponent(array.get(i).getAsJsonObject(), res)); 7345 } 7346 }; 7347 if (json.has("languageCode")) 7348 res.setLanguageCode(parseCodeableConcept(getJObject(json, "languageCode"))); 7349 } 7350 7351 protected DeviceComponent.DeviceComponentProductionSpecificationComponent parseDeviceComponentDeviceComponentProductionSpecificationComponent(JsonObject json, DeviceComponent owner) throws IOException, FHIRFormatError { 7352 DeviceComponent.DeviceComponentProductionSpecificationComponent res = new DeviceComponent.DeviceComponentProductionSpecificationComponent(); 7353 parseDeviceComponentDeviceComponentProductionSpecificationComponentProperties(json, owner, res); 7354 return res; 7355 } 7356 7357 protected void parseDeviceComponentDeviceComponentProductionSpecificationComponentProperties(JsonObject json, DeviceComponent owner, DeviceComponent.DeviceComponentProductionSpecificationComponent res) throws IOException, FHIRFormatError { 7358 parseBackboneProperties(json, res); 7359 if (json.has("specType")) 7360 res.setSpecType(parseCodeableConcept(getJObject(json, "specType"))); 7361 if (json.has("componentId")) 7362 res.setComponentId(parseIdentifier(getJObject(json, "componentId"))); 7363 if (json.has("productionSpec")) 7364 res.setProductionSpecElement(parseString(json.get("productionSpec").getAsString())); 7365 if (json.has("_productionSpec")) 7366 parseElementProperties(getJObject(json, "_productionSpec"), res.getProductionSpecElement()); 7367 } 7368 7369 protected DeviceMetric parseDeviceMetric(JsonObject json) throws IOException, FHIRFormatError { 7370 DeviceMetric res = new DeviceMetric(); 7371 parseDeviceMetricProperties(json, res); 7372 return res; 7373 } 7374 7375 protected void parseDeviceMetricProperties(JsonObject json, DeviceMetric res) throws IOException, FHIRFormatError { 7376 parseDomainResourceProperties(json, res); 7377 if (json.has("identifier")) 7378 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 7379 if (json.has("type")) 7380 res.setType(parseCodeableConcept(getJObject(json, "type"))); 7381 if (json.has("unit")) 7382 res.setUnit(parseCodeableConcept(getJObject(json, "unit"))); 7383 if (json.has("source")) 7384 res.setSource(parseReference(getJObject(json, "source"))); 7385 if (json.has("parent")) 7386 res.setParent(parseReference(getJObject(json, "parent"))); 7387 if (json.has("operationalStatus")) 7388 res.setOperationalStatusElement(parseEnumeration(json.get("operationalStatus").getAsString(), DeviceMetric.DeviceMetricOperationalStatus.NULL, new DeviceMetric.DeviceMetricOperationalStatusEnumFactory())); 7389 if (json.has("_operationalStatus")) 7390 parseElementProperties(getJObject(json, "_operationalStatus"), res.getOperationalStatusElement()); 7391 if (json.has("color")) 7392 res.setColorElement(parseEnumeration(json.get("color").getAsString(), DeviceMetric.DeviceMetricColor.NULL, new DeviceMetric.DeviceMetricColorEnumFactory())); 7393 if (json.has("_color")) 7394 parseElementProperties(getJObject(json, "_color"), res.getColorElement()); 7395 if (json.has("category")) 7396 res.setCategoryElement(parseEnumeration(json.get("category").getAsString(), DeviceMetric.DeviceMetricCategory.NULL, new DeviceMetric.DeviceMetricCategoryEnumFactory())); 7397 if (json.has("_category")) 7398 parseElementProperties(getJObject(json, "_category"), res.getCategoryElement()); 7399 if (json.has("measurementPeriod")) 7400 res.setMeasurementPeriod(parseTiming(getJObject(json, "measurementPeriod"))); 7401 if (json.has("calibration")) { 7402 JsonArray array = json.getAsJsonArray("calibration"); 7403 for (int i = 0; i < array.size(); i++) { 7404 res.getCalibration().add(parseDeviceMetricDeviceMetricCalibrationComponent(array.get(i).getAsJsonObject(), res)); 7405 } 7406 }; 7407 } 7408 7409 protected DeviceMetric.DeviceMetricCalibrationComponent parseDeviceMetricDeviceMetricCalibrationComponent(JsonObject json, DeviceMetric owner) throws IOException, FHIRFormatError { 7410 DeviceMetric.DeviceMetricCalibrationComponent res = new DeviceMetric.DeviceMetricCalibrationComponent(); 7411 parseDeviceMetricDeviceMetricCalibrationComponentProperties(json, owner, res); 7412 return res; 7413 } 7414 7415 protected void parseDeviceMetricDeviceMetricCalibrationComponentProperties(JsonObject json, DeviceMetric owner, DeviceMetric.DeviceMetricCalibrationComponent res) throws IOException, FHIRFormatError { 7416 parseBackboneProperties(json, res); 7417 if (json.has("type")) 7418 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), DeviceMetric.DeviceMetricCalibrationType.NULL, new DeviceMetric.DeviceMetricCalibrationTypeEnumFactory())); 7419 if (json.has("_type")) 7420 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 7421 if (json.has("state")) 7422 res.setStateElement(parseEnumeration(json.get("state").getAsString(), DeviceMetric.DeviceMetricCalibrationState.NULL, new DeviceMetric.DeviceMetricCalibrationStateEnumFactory())); 7423 if (json.has("_state")) 7424 parseElementProperties(getJObject(json, "_state"), res.getStateElement()); 7425 if (json.has("time")) 7426 res.setTimeElement(parseInstant(json.get("time").getAsString())); 7427 if (json.has("_time")) 7428 parseElementProperties(getJObject(json, "_time"), res.getTimeElement()); 7429 } 7430 7431 protected DeviceRequest parseDeviceRequest(JsonObject json) throws IOException, FHIRFormatError { 7432 DeviceRequest res = new DeviceRequest(); 7433 parseDeviceRequestProperties(json, res); 7434 return res; 7435 } 7436 7437 protected void parseDeviceRequestProperties(JsonObject json, DeviceRequest res) throws IOException, FHIRFormatError { 7438 parseDomainResourceProperties(json, res); 7439 if (json.has("identifier")) { 7440 JsonArray array = json.getAsJsonArray("identifier"); 7441 for (int i = 0; i < array.size(); i++) { 7442 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 7443 } 7444 }; 7445 if (json.has("definition")) { 7446 JsonArray array = json.getAsJsonArray("definition"); 7447 for (int i = 0; i < array.size(); i++) { 7448 res.getDefinition().add(parseReference(array.get(i).getAsJsonObject())); 7449 } 7450 }; 7451 if (json.has("basedOn")) { 7452 JsonArray array = json.getAsJsonArray("basedOn"); 7453 for (int i = 0; i < array.size(); i++) { 7454 res.getBasedOn().add(parseReference(array.get(i).getAsJsonObject())); 7455 } 7456 }; 7457 if (json.has("priorRequest")) { 7458 JsonArray array = json.getAsJsonArray("priorRequest"); 7459 for (int i = 0; i < array.size(); i++) { 7460 res.getPriorRequest().add(parseReference(array.get(i).getAsJsonObject())); 7461 } 7462 }; 7463 if (json.has("groupIdentifier")) 7464 res.setGroupIdentifier(parseIdentifier(getJObject(json, "groupIdentifier"))); 7465 if (json.has("status")) 7466 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), DeviceRequest.DeviceRequestStatus.NULL, new DeviceRequest.DeviceRequestStatusEnumFactory())); 7467 if (json.has("_status")) 7468 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 7469 if (json.has("intent")) 7470 res.setIntent(parseCodeableConcept(getJObject(json, "intent"))); 7471 if (json.has("priority")) 7472 res.setPriorityElement(parseEnumeration(json.get("priority").getAsString(), DeviceRequest.RequestPriority.NULL, new DeviceRequest.RequestPriorityEnumFactory())); 7473 if (json.has("_priority")) 7474 parseElementProperties(getJObject(json, "_priority"), res.getPriorityElement()); 7475 Type code = parseType("code", json); 7476 if (code != null) 7477 res.setCode(code); 7478 if (json.has("subject")) 7479 res.setSubject(parseReference(getJObject(json, "subject"))); 7480 if (json.has("context")) 7481 res.setContext(parseReference(getJObject(json, "context"))); 7482 Type occurrence = parseType("occurrence", json); 7483 if (occurrence != null) 7484 res.setOccurrence(occurrence); 7485 if (json.has("authoredOn")) 7486 res.setAuthoredOnElement(parseDateTime(json.get("authoredOn").getAsString())); 7487 if (json.has("_authoredOn")) 7488 parseElementProperties(getJObject(json, "_authoredOn"), res.getAuthoredOnElement()); 7489 if (json.has("requester")) 7490 res.setRequester(parseDeviceRequestDeviceRequestRequesterComponent(getJObject(json, "requester"), res)); 7491 if (json.has("performerType")) 7492 res.setPerformerType(parseCodeableConcept(getJObject(json, "performerType"))); 7493 if (json.has("performer")) 7494 res.setPerformer(parseReference(getJObject(json, "performer"))); 7495 if (json.has("reasonCode")) { 7496 JsonArray array = json.getAsJsonArray("reasonCode"); 7497 for (int i = 0; i < array.size(); i++) { 7498 res.getReasonCode().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 7499 } 7500 }; 7501 if (json.has("reasonReference")) { 7502 JsonArray array = json.getAsJsonArray("reasonReference"); 7503 for (int i = 0; i < array.size(); i++) { 7504 res.getReasonReference().add(parseReference(array.get(i).getAsJsonObject())); 7505 } 7506 }; 7507 if (json.has("supportingInfo")) { 7508 JsonArray array = json.getAsJsonArray("supportingInfo"); 7509 for (int i = 0; i < array.size(); i++) { 7510 res.getSupportingInfo().add(parseReference(array.get(i).getAsJsonObject())); 7511 } 7512 }; 7513 if (json.has("note")) { 7514 JsonArray array = json.getAsJsonArray("note"); 7515 for (int i = 0; i < array.size(); i++) { 7516 res.getNote().add(parseAnnotation(array.get(i).getAsJsonObject())); 7517 } 7518 }; 7519 if (json.has("relevantHistory")) { 7520 JsonArray array = json.getAsJsonArray("relevantHistory"); 7521 for (int i = 0; i < array.size(); i++) { 7522 res.getRelevantHistory().add(parseReference(array.get(i).getAsJsonObject())); 7523 } 7524 }; 7525 } 7526 7527 protected DeviceRequest.DeviceRequestRequesterComponent parseDeviceRequestDeviceRequestRequesterComponent(JsonObject json, DeviceRequest owner) throws IOException, FHIRFormatError { 7528 DeviceRequest.DeviceRequestRequesterComponent res = new DeviceRequest.DeviceRequestRequesterComponent(); 7529 parseDeviceRequestDeviceRequestRequesterComponentProperties(json, owner, res); 7530 return res; 7531 } 7532 7533 protected void parseDeviceRequestDeviceRequestRequesterComponentProperties(JsonObject json, DeviceRequest owner, DeviceRequest.DeviceRequestRequesterComponent res) throws IOException, FHIRFormatError { 7534 parseBackboneProperties(json, res); 7535 if (json.has("agent")) 7536 res.setAgent(parseReference(getJObject(json, "agent"))); 7537 if (json.has("onBehalfOf")) 7538 res.setOnBehalfOf(parseReference(getJObject(json, "onBehalfOf"))); 7539 } 7540 7541 protected DeviceUseStatement parseDeviceUseStatement(JsonObject json) throws IOException, FHIRFormatError { 7542 DeviceUseStatement res = new DeviceUseStatement(); 7543 parseDeviceUseStatementProperties(json, res); 7544 return res; 7545 } 7546 7547 protected void parseDeviceUseStatementProperties(JsonObject json, DeviceUseStatement res) throws IOException, FHIRFormatError { 7548 parseDomainResourceProperties(json, res); 7549 if (json.has("identifier")) { 7550 JsonArray array = json.getAsJsonArray("identifier"); 7551 for (int i = 0; i < array.size(); i++) { 7552 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 7553 } 7554 }; 7555 if (json.has("status")) 7556 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), DeviceUseStatement.DeviceUseStatementStatus.NULL, new DeviceUseStatement.DeviceUseStatementStatusEnumFactory())); 7557 if (json.has("_status")) 7558 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 7559 if (json.has("subject")) 7560 res.setSubject(parseReference(getJObject(json, "subject"))); 7561 if (json.has("whenUsed")) 7562 res.setWhenUsed(parsePeriod(getJObject(json, "whenUsed"))); 7563 Type timing = parseType("timing", json); 7564 if (timing != null) 7565 res.setTiming(timing); 7566 if (json.has("recordedOn")) 7567 res.setRecordedOnElement(parseDateTime(json.get("recordedOn").getAsString())); 7568 if (json.has("_recordedOn")) 7569 parseElementProperties(getJObject(json, "_recordedOn"), res.getRecordedOnElement()); 7570 if (json.has("source")) 7571 res.setSource(parseReference(getJObject(json, "source"))); 7572 if (json.has("device")) 7573 res.setDevice(parseReference(getJObject(json, "device"))); 7574 if (json.has("indication")) { 7575 JsonArray array = json.getAsJsonArray("indication"); 7576 for (int i = 0; i < array.size(); i++) { 7577 res.getIndication().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 7578 } 7579 }; 7580 if (json.has("bodySite")) 7581 res.setBodySite(parseCodeableConcept(getJObject(json, "bodySite"))); 7582 if (json.has("note")) { 7583 JsonArray array = json.getAsJsonArray("note"); 7584 for (int i = 0; i < array.size(); i++) { 7585 res.getNote().add(parseAnnotation(array.get(i).getAsJsonObject())); 7586 } 7587 }; 7588 } 7589 7590 protected DiagnosticReport parseDiagnosticReport(JsonObject json) throws IOException, FHIRFormatError { 7591 DiagnosticReport res = new DiagnosticReport(); 7592 parseDiagnosticReportProperties(json, res); 7593 return res; 7594 } 7595 7596 protected void parseDiagnosticReportProperties(JsonObject json, DiagnosticReport res) throws IOException, FHIRFormatError { 7597 parseDomainResourceProperties(json, res); 7598 if (json.has("identifier")) { 7599 JsonArray array = json.getAsJsonArray("identifier"); 7600 for (int i = 0; i < array.size(); i++) { 7601 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 7602 } 7603 }; 7604 if (json.has("basedOn")) { 7605 JsonArray array = json.getAsJsonArray("basedOn"); 7606 for (int i = 0; i < array.size(); i++) { 7607 res.getBasedOn().add(parseReference(array.get(i).getAsJsonObject())); 7608 } 7609 }; 7610 if (json.has("status")) 7611 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), DiagnosticReport.DiagnosticReportStatus.NULL, new DiagnosticReport.DiagnosticReportStatusEnumFactory())); 7612 if (json.has("_status")) 7613 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 7614 if (json.has("category")) 7615 res.setCategory(parseCodeableConcept(getJObject(json, "category"))); 7616 if (json.has("code")) 7617 res.setCode(parseCodeableConcept(getJObject(json, "code"))); 7618 if (json.has("subject")) 7619 res.setSubject(parseReference(getJObject(json, "subject"))); 7620 if (json.has("context")) 7621 res.setContext(parseReference(getJObject(json, "context"))); 7622 Type effective = parseType("effective", json); 7623 if (effective != null) 7624 res.setEffective(effective); 7625 if (json.has("issued")) 7626 res.setIssuedElement(parseInstant(json.get("issued").getAsString())); 7627 if (json.has("_issued")) 7628 parseElementProperties(getJObject(json, "_issued"), res.getIssuedElement()); 7629 if (json.has("performer")) { 7630 JsonArray array = json.getAsJsonArray("performer"); 7631 for (int i = 0; i < array.size(); i++) { 7632 res.getPerformer().add(parseDiagnosticReportDiagnosticReportPerformerComponent(array.get(i).getAsJsonObject(), res)); 7633 } 7634 }; 7635 if (json.has("specimen")) { 7636 JsonArray array = json.getAsJsonArray("specimen"); 7637 for (int i = 0; i < array.size(); i++) { 7638 res.getSpecimen().add(parseReference(array.get(i).getAsJsonObject())); 7639 } 7640 }; 7641 if (json.has("result")) { 7642 JsonArray array = json.getAsJsonArray("result"); 7643 for (int i = 0; i < array.size(); i++) { 7644 res.getResult().add(parseReference(array.get(i).getAsJsonObject())); 7645 } 7646 }; 7647 if (json.has("imagingStudy")) { 7648 JsonArray array = json.getAsJsonArray("imagingStudy"); 7649 for (int i = 0; i < array.size(); i++) { 7650 res.getImagingStudy().add(parseReference(array.get(i).getAsJsonObject())); 7651 } 7652 }; 7653 if (json.has("image")) { 7654 JsonArray array = json.getAsJsonArray("image"); 7655 for (int i = 0; i < array.size(); i++) { 7656 res.getImage().add(parseDiagnosticReportDiagnosticReportImageComponent(array.get(i).getAsJsonObject(), res)); 7657 } 7658 }; 7659 if (json.has("conclusion")) 7660 res.setConclusionElement(parseString(json.get("conclusion").getAsString())); 7661 if (json.has("_conclusion")) 7662 parseElementProperties(getJObject(json, "_conclusion"), res.getConclusionElement()); 7663 if (json.has("codedDiagnosis")) { 7664 JsonArray array = json.getAsJsonArray("codedDiagnosis"); 7665 for (int i = 0; i < array.size(); i++) { 7666 res.getCodedDiagnosis().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 7667 } 7668 }; 7669 if (json.has("presentedForm")) { 7670 JsonArray array = json.getAsJsonArray("presentedForm"); 7671 for (int i = 0; i < array.size(); i++) { 7672 res.getPresentedForm().add(parseAttachment(array.get(i).getAsJsonObject())); 7673 } 7674 }; 7675 } 7676 7677 protected DiagnosticReport.DiagnosticReportPerformerComponent parseDiagnosticReportDiagnosticReportPerformerComponent(JsonObject json, DiagnosticReport owner) throws IOException, FHIRFormatError { 7678 DiagnosticReport.DiagnosticReportPerformerComponent res = new DiagnosticReport.DiagnosticReportPerformerComponent(); 7679 parseDiagnosticReportDiagnosticReportPerformerComponentProperties(json, owner, res); 7680 return res; 7681 } 7682 7683 protected void parseDiagnosticReportDiagnosticReportPerformerComponentProperties(JsonObject json, DiagnosticReport owner, DiagnosticReport.DiagnosticReportPerformerComponent res) throws IOException, FHIRFormatError { 7684 parseBackboneProperties(json, res); 7685 if (json.has("role")) 7686 res.setRole(parseCodeableConcept(getJObject(json, "role"))); 7687 if (json.has("actor")) 7688 res.setActor(parseReference(getJObject(json, "actor"))); 7689 } 7690 7691 protected DiagnosticReport.DiagnosticReportImageComponent parseDiagnosticReportDiagnosticReportImageComponent(JsonObject json, DiagnosticReport owner) throws IOException, FHIRFormatError { 7692 DiagnosticReport.DiagnosticReportImageComponent res = new DiagnosticReport.DiagnosticReportImageComponent(); 7693 parseDiagnosticReportDiagnosticReportImageComponentProperties(json, owner, res); 7694 return res; 7695 } 7696 7697 protected void parseDiagnosticReportDiagnosticReportImageComponentProperties(JsonObject json, DiagnosticReport owner, DiagnosticReport.DiagnosticReportImageComponent res) throws IOException, FHIRFormatError { 7698 parseBackboneProperties(json, res); 7699 if (json.has("comment")) 7700 res.setCommentElement(parseString(json.get("comment").getAsString())); 7701 if (json.has("_comment")) 7702 parseElementProperties(getJObject(json, "_comment"), res.getCommentElement()); 7703 if (json.has("link")) 7704 res.setLink(parseReference(getJObject(json, "link"))); 7705 } 7706 7707 protected DocumentManifest parseDocumentManifest(JsonObject json) throws IOException, FHIRFormatError { 7708 DocumentManifest res = new DocumentManifest(); 7709 parseDocumentManifestProperties(json, res); 7710 return res; 7711 } 7712 7713 protected void parseDocumentManifestProperties(JsonObject json, DocumentManifest res) throws IOException, FHIRFormatError { 7714 parseDomainResourceProperties(json, res); 7715 if (json.has("masterIdentifier")) 7716 res.setMasterIdentifier(parseIdentifier(getJObject(json, "masterIdentifier"))); 7717 if (json.has("identifier")) { 7718 JsonArray array = json.getAsJsonArray("identifier"); 7719 for (int i = 0; i < array.size(); i++) { 7720 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 7721 } 7722 }; 7723 if (json.has("status")) 7724 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Enumerations.DocumentReferenceStatus.NULL, new Enumerations.DocumentReferenceStatusEnumFactory())); 7725 if (json.has("_status")) 7726 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 7727 if (json.has("type")) 7728 res.setType(parseCodeableConcept(getJObject(json, "type"))); 7729 if (json.has("subject")) 7730 res.setSubject(parseReference(getJObject(json, "subject"))); 7731 if (json.has("created")) 7732 res.setCreatedElement(parseDateTime(json.get("created").getAsString())); 7733 if (json.has("_created")) 7734 parseElementProperties(getJObject(json, "_created"), res.getCreatedElement()); 7735 if (json.has("author")) { 7736 JsonArray array = json.getAsJsonArray("author"); 7737 for (int i = 0; i < array.size(); i++) { 7738 res.getAuthor().add(parseReference(array.get(i).getAsJsonObject())); 7739 } 7740 }; 7741 if (json.has("recipient")) { 7742 JsonArray array = json.getAsJsonArray("recipient"); 7743 for (int i = 0; i < array.size(); i++) { 7744 res.getRecipient().add(parseReference(array.get(i).getAsJsonObject())); 7745 } 7746 }; 7747 if (json.has("source")) 7748 res.setSourceElement(parseUri(json.get("source").getAsString())); 7749 if (json.has("_source")) 7750 parseElementProperties(getJObject(json, "_source"), res.getSourceElement()); 7751 if (json.has("description")) 7752 res.setDescriptionElement(parseString(json.get("description").getAsString())); 7753 if (json.has("_description")) 7754 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 7755 if (json.has("content")) { 7756 JsonArray array = json.getAsJsonArray("content"); 7757 for (int i = 0; i < array.size(); i++) { 7758 res.getContent().add(parseDocumentManifestDocumentManifestContentComponent(array.get(i).getAsJsonObject(), res)); 7759 } 7760 }; 7761 if (json.has("related")) { 7762 JsonArray array = json.getAsJsonArray("related"); 7763 for (int i = 0; i < array.size(); i++) { 7764 res.getRelated().add(parseDocumentManifestDocumentManifestRelatedComponent(array.get(i).getAsJsonObject(), res)); 7765 } 7766 }; 7767 } 7768 7769 protected DocumentManifest.DocumentManifestContentComponent parseDocumentManifestDocumentManifestContentComponent(JsonObject json, DocumentManifest owner) throws IOException, FHIRFormatError { 7770 DocumentManifest.DocumentManifestContentComponent res = new DocumentManifest.DocumentManifestContentComponent(); 7771 parseDocumentManifestDocumentManifestContentComponentProperties(json, owner, res); 7772 return res; 7773 } 7774 7775 protected void parseDocumentManifestDocumentManifestContentComponentProperties(JsonObject json, DocumentManifest owner, DocumentManifest.DocumentManifestContentComponent res) throws IOException, FHIRFormatError { 7776 parseBackboneProperties(json, res); 7777 Type p = parseType("p", json); 7778 if (p != null) 7779 res.setP(p); 7780 } 7781 7782 protected DocumentManifest.DocumentManifestRelatedComponent parseDocumentManifestDocumentManifestRelatedComponent(JsonObject json, DocumentManifest owner) throws IOException, FHIRFormatError { 7783 DocumentManifest.DocumentManifestRelatedComponent res = new DocumentManifest.DocumentManifestRelatedComponent(); 7784 parseDocumentManifestDocumentManifestRelatedComponentProperties(json, owner, res); 7785 return res; 7786 } 7787 7788 protected void parseDocumentManifestDocumentManifestRelatedComponentProperties(JsonObject json, DocumentManifest owner, DocumentManifest.DocumentManifestRelatedComponent res) throws IOException, FHIRFormatError { 7789 parseBackboneProperties(json, res); 7790 if (json.has("identifier")) 7791 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 7792 if (json.has("ref")) 7793 res.setRef(parseReference(getJObject(json, "ref"))); 7794 } 7795 7796 protected DocumentReference parseDocumentReference(JsonObject json) throws IOException, FHIRFormatError { 7797 DocumentReference res = new DocumentReference(); 7798 parseDocumentReferenceProperties(json, res); 7799 return res; 7800 } 7801 7802 protected void parseDocumentReferenceProperties(JsonObject json, DocumentReference res) throws IOException, FHIRFormatError { 7803 parseDomainResourceProperties(json, res); 7804 if (json.has("masterIdentifier")) 7805 res.setMasterIdentifier(parseIdentifier(getJObject(json, "masterIdentifier"))); 7806 if (json.has("identifier")) { 7807 JsonArray array = json.getAsJsonArray("identifier"); 7808 for (int i = 0; i < array.size(); i++) { 7809 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 7810 } 7811 }; 7812 if (json.has("status")) 7813 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Enumerations.DocumentReferenceStatus.NULL, new Enumerations.DocumentReferenceStatusEnumFactory())); 7814 if (json.has("_status")) 7815 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 7816 if (json.has("docStatus")) 7817 res.setDocStatusElement(parseEnumeration(json.get("docStatus").getAsString(), DocumentReference.ReferredDocumentStatus.NULL, new DocumentReference.ReferredDocumentStatusEnumFactory())); 7818 if (json.has("_docStatus")) 7819 parseElementProperties(getJObject(json, "_docStatus"), res.getDocStatusElement()); 7820 if (json.has("type")) 7821 res.setType(parseCodeableConcept(getJObject(json, "type"))); 7822 if (json.has("class")) 7823 res.setClass_(parseCodeableConcept(getJObject(json, "class"))); 7824 if (json.has("subject")) 7825 res.setSubject(parseReference(getJObject(json, "subject"))); 7826 if (json.has("created")) 7827 res.setCreatedElement(parseDateTime(json.get("created").getAsString())); 7828 if (json.has("_created")) 7829 parseElementProperties(getJObject(json, "_created"), res.getCreatedElement()); 7830 if (json.has("indexed")) 7831 res.setIndexedElement(parseInstant(json.get("indexed").getAsString())); 7832 if (json.has("_indexed")) 7833 parseElementProperties(getJObject(json, "_indexed"), res.getIndexedElement()); 7834 if (json.has("author")) { 7835 JsonArray array = json.getAsJsonArray("author"); 7836 for (int i = 0; i < array.size(); i++) { 7837 res.getAuthor().add(parseReference(array.get(i).getAsJsonObject())); 7838 } 7839 }; 7840 if (json.has("authenticator")) 7841 res.setAuthenticator(parseReference(getJObject(json, "authenticator"))); 7842 if (json.has("custodian")) 7843 res.setCustodian(parseReference(getJObject(json, "custodian"))); 7844 if (json.has("relatesTo")) { 7845 JsonArray array = json.getAsJsonArray("relatesTo"); 7846 for (int i = 0; i < array.size(); i++) { 7847 res.getRelatesTo().add(parseDocumentReferenceDocumentReferenceRelatesToComponent(array.get(i).getAsJsonObject(), res)); 7848 } 7849 }; 7850 if (json.has("description")) 7851 res.setDescriptionElement(parseString(json.get("description").getAsString())); 7852 if (json.has("_description")) 7853 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 7854 if (json.has("securityLabel")) { 7855 JsonArray array = json.getAsJsonArray("securityLabel"); 7856 for (int i = 0; i < array.size(); i++) { 7857 res.getSecurityLabel().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 7858 } 7859 }; 7860 if (json.has("content")) { 7861 JsonArray array = json.getAsJsonArray("content"); 7862 for (int i = 0; i < array.size(); i++) { 7863 res.getContent().add(parseDocumentReferenceDocumentReferenceContentComponent(array.get(i).getAsJsonObject(), res)); 7864 } 7865 }; 7866 if (json.has("context")) 7867 res.setContext(parseDocumentReferenceDocumentReferenceContextComponent(getJObject(json, "context"), res)); 7868 } 7869 7870 protected DocumentReference.DocumentReferenceRelatesToComponent parseDocumentReferenceDocumentReferenceRelatesToComponent(JsonObject json, DocumentReference owner) throws IOException, FHIRFormatError { 7871 DocumentReference.DocumentReferenceRelatesToComponent res = new DocumentReference.DocumentReferenceRelatesToComponent(); 7872 parseDocumentReferenceDocumentReferenceRelatesToComponentProperties(json, owner, res); 7873 return res; 7874 } 7875 7876 protected void parseDocumentReferenceDocumentReferenceRelatesToComponentProperties(JsonObject json, DocumentReference owner, DocumentReference.DocumentReferenceRelatesToComponent res) throws IOException, FHIRFormatError { 7877 parseBackboneProperties(json, res); 7878 if (json.has("code")) 7879 res.setCodeElement(parseEnumeration(json.get("code").getAsString(), DocumentReference.DocumentRelationshipType.NULL, new DocumentReference.DocumentRelationshipTypeEnumFactory())); 7880 if (json.has("_code")) 7881 parseElementProperties(getJObject(json, "_code"), res.getCodeElement()); 7882 if (json.has("target")) 7883 res.setTarget(parseReference(getJObject(json, "target"))); 7884 } 7885 7886 protected DocumentReference.DocumentReferenceContentComponent parseDocumentReferenceDocumentReferenceContentComponent(JsonObject json, DocumentReference owner) throws IOException, FHIRFormatError { 7887 DocumentReference.DocumentReferenceContentComponent res = new DocumentReference.DocumentReferenceContentComponent(); 7888 parseDocumentReferenceDocumentReferenceContentComponentProperties(json, owner, res); 7889 return res; 7890 } 7891 7892 protected void parseDocumentReferenceDocumentReferenceContentComponentProperties(JsonObject json, DocumentReference owner, DocumentReference.DocumentReferenceContentComponent res) throws IOException, FHIRFormatError { 7893 parseBackboneProperties(json, res); 7894 if (json.has("attachment")) 7895 res.setAttachment(parseAttachment(getJObject(json, "attachment"))); 7896 if (json.has("format")) 7897 res.setFormat(parseCoding(getJObject(json, "format"))); 7898 } 7899 7900 protected DocumentReference.DocumentReferenceContextComponent parseDocumentReferenceDocumentReferenceContextComponent(JsonObject json, DocumentReference owner) throws IOException, FHIRFormatError { 7901 DocumentReference.DocumentReferenceContextComponent res = new DocumentReference.DocumentReferenceContextComponent(); 7902 parseDocumentReferenceDocumentReferenceContextComponentProperties(json, owner, res); 7903 return res; 7904 } 7905 7906 protected void parseDocumentReferenceDocumentReferenceContextComponentProperties(JsonObject json, DocumentReference owner, DocumentReference.DocumentReferenceContextComponent res) throws IOException, FHIRFormatError { 7907 parseBackboneProperties(json, res); 7908 if (json.has("encounter")) 7909 res.setEncounter(parseReference(getJObject(json, "encounter"))); 7910 if (json.has("event")) { 7911 JsonArray array = json.getAsJsonArray("event"); 7912 for (int i = 0; i < array.size(); i++) { 7913 res.getEvent().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 7914 } 7915 }; 7916 if (json.has("period")) 7917 res.setPeriod(parsePeriod(getJObject(json, "period"))); 7918 if (json.has("facilityType")) 7919 res.setFacilityType(parseCodeableConcept(getJObject(json, "facilityType"))); 7920 if (json.has("practiceSetting")) 7921 res.setPracticeSetting(parseCodeableConcept(getJObject(json, "practiceSetting"))); 7922 if (json.has("sourcePatientInfo")) 7923 res.setSourcePatientInfo(parseReference(getJObject(json, "sourcePatientInfo"))); 7924 if (json.has("related")) { 7925 JsonArray array = json.getAsJsonArray("related"); 7926 for (int i = 0; i < array.size(); i++) { 7927 res.getRelated().add(parseDocumentReferenceDocumentReferenceContextRelatedComponent(array.get(i).getAsJsonObject(), owner)); 7928 } 7929 }; 7930 } 7931 7932 protected DocumentReference.DocumentReferenceContextRelatedComponent parseDocumentReferenceDocumentReferenceContextRelatedComponent(JsonObject json, DocumentReference owner) throws IOException, FHIRFormatError { 7933 DocumentReference.DocumentReferenceContextRelatedComponent res = new DocumentReference.DocumentReferenceContextRelatedComponent(); 7934 parseDocumentReferenceDocumentReferenceContextRelatedComponentProperties(json, owner, res); 7935 return res; 7936 } 7937 7938 protected void parseDocumentReferenceDocumentReferenceContextRelatedComponentProperties(JsonObject json, DocumentReference owner, DocumentReference.DocumentReferenceContextRelatedComponent res) throws IOException, FHIRFormatError { 7939 parseBackboneProperties(json, res); 7940 if (json.has("identifier")) 7941 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 7942 if (json.has("ref")) 7943 res.setRef(parseReference(getJObject(json, "ref"))); 7944 } 7945 7946 protected EligibilityRequest parseEligibilityRequest(JsonObject json) throws IOException, FHIRFormatError { 7947 EligibilityRequest res = new EligibilityRequest(); 7948 parseEligibilityRequestProperties(json, res); 7949 return res; 7950 } 7951 7952 protected void parseEligibilityRequestProperties(JsonObject json, EligibilityRequest res) throws IOException, FHIRFormatError { 7953 parseDomainResourceProperties(json, res); 7954 if (json.has("identifier")) { 7955 JsonArray array = json.getAsJsonArray("identifier"); 7956 for (int i = 0; i < array.size(); i++) { 7957 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 7958 } 7959 }; 7960 if (json.has("status")) 7961 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), EligibilityRequest.EligibilityRequestStatus.NULL, new EligibilityRequest.EligibilityRequestStatusEnumFactory())); 7962 if (json.has("_status")) 7963 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 7964 if (json.has("priority")) 7965 res.setPriority(parseCodeableConcept(getJObject(json, "priority"))); 7966 if (json.has("patient")) 7967 res.setPatient(parseReference(getJObject(json, "patient"))); 7968 Type serviced = parseType("serviced", json); 7969 if (serviced != null) 7970 res.setServiced(serviced); 7971 if (json.has("created")) 7972 res.setCreatedElement(parseDateTime(json.get("created").getAsString())); 7973 if (json.has("_created")) 7974 parseElementProperties(getJObject(json, "_created"), res.getCreatedElement()); 7975 if (json.has("enterer")) 7976 res.setEnterer(parseReference(getJObject(json, "enterer"))); 7977 if (json.has("provider")) 7978 res.setProvider(parseReference(getJObject(json, "provider"))); 7979 if (json.has("organization")) 7980 res.setOrganization(parseReference(getJObject(json, "organization"))); 7981 if (json.has("insurer")) 7982 res.setInsurer(parseReference(getJObject(json, "insurer"))); 7983 if (json.has("facility")) 7984 res.setFacility(parseReference(getJObject(json, "facility"))); 7985 if (json.has("coverage")) 7986 res.setCoverage(parseReference(getJObject(json, "coverage"))); 7987 if (json.has("businessArrangement")) 7988 res.setBusinessArrangementElement(parseString(json.get("businessArrangement").getAsString())); 7989 if (json.has("_businessArrangement")) 7990 parseElementProperties(getJObject(json, "_businessArrangement"), res.getBusinessArrangementElement()); 7991 if (json.has("benefitCategory")) 7992 res.setBenefitCategory(parseCodeableConcept(getJObject(json, "benefitCategory"))); 7993 if (json.has("benefitSubCategory")) 7994 res.setBenefitSubCategory(parseCodeableConcept(getJObject(json, "benefitSubCategory"))); 7995 } 7996 7997 protected EligibilityResponse parseEligibilityResponse(JsonObject json) throws IOException, FHIRFormatError { 7998 EligibilityResponse res = new EligibilityResponse(); 7999 parseEligibilityResponseProperties(json, res); 8000 return res; 8001 } 8002 8003 protected void parseEligibilityResponseProperties(JsonObject json, EligibilityResponse res) throws IOException, FHIRFormatError { 8004 parseDomainResourceProperties(json, res); 8005 if (json.has("identifier")) { 8006 JsonArray array = json.getAsJsonArray("identifier"); 8007 for (int i = 0; i < array.size(); i++) { 8008 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 8009 } 8010 }; 8011 if (json.has("status")) 8012 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), EligibilityResponse.EligibilityResponseStatus.NULL, new EligibilityResponse.EligibilityResponseStatusEnumFactory())); 8013 if (json.has("_status")) 8014 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 8015 if (json.has("created")) 8016 res.setCreatedElement(parseDateTime(json.get("created").getAsString())); 8017 if (json.has("_created")) 8018 parseElementProperties(getJObject(json, "_created"), res.getCreatedElement()); 8019 if (json.has("requestProvider")) 8020 res.setRequestProvider(parseReference(getJObject(json, "requestProvider"))); 8021 if (json.has("requestOrganization")) 8022 res.setRequestOrganization(parseReference(getJObject(json, "requestOrganization"))); 8023 if (json.has("request")) 8024 res.setRequest(parseReference(getJObject(json, "request"))); 8025 if (json.has("outcome")) 8026 res.setOutcome(parseCodeableConcept(getJObject(json, "outcome"))); 8027 if (json.has("disposition")) 8028 res.setDispositionElement(parseString(json.get("disposition").getAsString())); 8029 if (json.has("_disposition")) 8030 parseElementProperties(getJObject(json, "_disposition"), res.getDispositionElement()); 8031 if (json.has("insurer")) 8032 res.setInsurer(parseReference(getJObject(json, "insurer"))); 8033 if (json.has("inforce")) 8034 res.setInforceElement(parseBoolean(json.get("inforce").getAsBoolean())); 8035 if (json.has("_inforce")) 8036 parseElementProperties(getJObject(json, "_inforce"), res.getInforceElement()); 8037 if (json.has("insurance")) { 8038 JsonArray array = json.getAsJsonArray("insurance"); 8039 for (int i = 0; i < array.size(); i++) { 8040 res.getInsurance().add(parseEligibilityResponseInsuranceComponent(array.get(i).getAsJsonObject(), res)); 8041 } 8042 }; 8043 if (json.has("form")) 8044 res.setForm(parseCodeableConcept(getJObject(json, "form"))); 8045 if (json.has("error")) { 8046 JsonArray array = json.getAsJsonArray("error"); 8047 for (int i = 0; i < array.size(); i++) { 8048 res.getError().add(parseEligibilityResponseErrorsComponent(array.get(i).getAsJsonObject(), res)); 8049 } 8050 }; 8051 } 8052 8053 protected EligibilityResponse.InsuranceComponent parseEligibilityResponseInsuranceComponent(JsonObject json, EligibilityResponse owner) throws IOException, FHIRFormatError { 8054 EligibilityResponse.InsuranceComponent res = new EligibilityResponse.InsuranceComponent(); 8055 parseEligibilityResponseInsuranceComponentProperties(json, owner, res); 8056 return res; 8057 } 8058 8059 protected void parseEligibilityResponseInsuranceComponentProperties(JsonObject json, EligibilityResponse owner, EligibilityResponse.InsuranceComponent res) throws IOException, FHIRFormatError { 8060 parseBackboneProperties(json, res); 8061 if (json.has("coverage")) 8062 res.setCoverage(parseReference(getJObject(json, "coverage"))); 8063 if (json.has("contract")) 8064 res.setContract(parseReference(getJObject(json, "contract"))); 8065 if (json.has("benefitBalance")) { 8066 JsonArray array = json.getAsJsonArray("benefitBalance"); 8067 for (int i = 0; i < array.size(); i++) { 8068 res.getBenefitBalance().add(parseEligibilityResponseBenefitsComponent(array.get(i).getAsJsonObject(), owner)); 8069 } 8070 }; 8071 } 8072 8073 protected EligibilityResponse.BenefitsComponent parseEligibilityResponseBenefitsComponent(JsonObject json, EligibilityResponse owner) throws IOException, FHIRFormatError { 8074 EligibilityResponse.BenefitsComponent res = new EligibilityResponse.BenefitsComponent(); 8075 parseEligibilityResponseBenefitsComponentProperties(json, owner, res); 8076 return res; 8077 } 8078 8079 protected void parseEligibilityResponseBenefitsComponentProperties(JsonObject json, EligibilityResponse owner, EligibilityResponse.BenefitsComponent res) throws IOException, FHIRFormatError { 8080 parseBackboneProperties(json, res); 8081 if (json.has("category")) 8082 res.setCategory(parseCodeableConcept(getJObject(json, "category"))); 8083 if (json.has("subCategory")) 8084 res.setSubCategory(parseCodeableConcept(getJObject(json, "subCategory"))); 8085 if (json.has("excluded")) 8086 res.setExcludedElement(parseBoolean(json.get("excluded").getAsBoolean())); 8087 if (json.has("_excluded")) 8088 parseElementProperties(getJObject(json, "_excluded"), res.getExcludedElement()); 8089 if (json.has("name")) 8090 res.setNameElement(parseString(json.get("name").getAsString())); 8091 if (json.has("_name")) 8092 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 8093 if (json.has("description")) 8094 res.setDescriptionElement(parseString(json.get("description").getAsString())); 8095 if (json.has("_description")) 8096 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 8097 if (json.has("network")) 8098 res.setNetwork(parseCodeableConcept(getJObject(json, "network"))); 8099 if (json.has("unit")) 8100 res.setUnit(parseCodeableConcept(getJObject(json, "unit"))); 8101 if (json.has("term")) 8102 res.setTerm(parseCodeableConcept(getJObject(json, "term"))); 8103 if (json.has("financial")) { 8104 JsonArray array = json.getAsJsonArray("financial"); 8105 for (int i = 0; i < array.size(); i++) { 8106 res.getFinancial().add(parseEligibilityResponseBenefitComponent(array.get(i).getAsJsonObject(), owner)); 8107 } 8108 }; 8109 } 8110 8111 protected EligibilityResponse.BenefitComponent parseEligibilityResponseBenefitComponent(JsonObject json, EligibilityResponse owner) throws IOException, FHIRFormatError { 8112 EligibilityResponse.BenefitComponent res = new EligibilityResponse.BenefitComponent(); 8113 parseEligibilityResponseBenefitComponentProperties(json, owner, res); 8114 return res; 8115 } 8116 8117 protected void parseEligibilityResponseBenefitComponentProperties(JsonObject json, EligibilityResponse owner, EligibilityResponse.BenefitComponent res) throws IOException, FHIRFormatError { 8118 parseBackboneProperties(json, res); 8119 if (json.has("type")) 8120 res.setType(parseCodeableConcept(getJObject(json, "type"))); 8121 Type allowed = parseType("allowed", json); 8122 if (allowed != null) 8123 res.setAllowed(allowed); 8124 Type used = parseType("used", json); 8125 if (used != null) 8126 res.setUsed(used); 8127 } 8128 8129 protected EligibilityResponse.ErrorsComponent parseEligibilityResponseErrorsComponent(JsonObject json, EligibilityResponse owner) throws IOException, FHIRFormatError { 8130 EligibilityResponse.ErrorsComponent res = new EligibilityResponse.ErrorsComponent(); 8131 parseEligibilityResponseErrorsComponentProperties(json, owner, res); 8132 return res; 8133 } 8134 8135 protected void parseEligibilityResponseErrorsComponentProperties(JsonObject json, EligibilityResponse owner, EligibilityResponse.ErrorsComponent res) throws IOException, FHIRFormatError { 8136 parseBackboneProperties(json, res); 8137 if (json.has("code")) 8138 res.setCode(parseCodeableConcept(getJObject(json, "code"))); 8139 } 8140 8141 protected Encounter parseEncounter(JsonObject json) throws IOException, FHIRFormatError { 8142 Encounter res = new Encounter(); 8143 parseEncounterProperties(json, res); 8144 return res; 8145 } 8146 8147 protected void parseEncounterProperties(JsonObject json, Encounter res) throws IOException, FHIRFormatError { 8148 parseDomainResourceProperties(json, res); 8149 if (json.has("identifier")) { 8150 JsonArray array = json.getAsJsonArray("identifier"); 8151 for (int i = 0; i < array.size(); i++) { 8152 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 8153 } 8154 }; 8155 if (json.has("status")) 8156 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Encounter.EncounterStatus.NULL, new Encounter.EncounterStatusEnumFactory())); 8157 if (json.has("_status")) 8158 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 8159 if (json.has("statusHistory")) { 8160 JsonArray array = json.getAsJsonArray("statusHistory"); 8161 for (int i = 0; i < array.size(); i++) { 8162 res.getStatusHistory().add(parseEncounterStatusHistoryComponent(array.get(i).getAsJsonObject(), res)); 8163 } 8164 }; 8165 if (json.has("class")) 8166 res.setClass_(parseCoding(getJObject(json, "class"))); 8167 if (json.has("classHistory")) { 8168 JsonArray array = json.getAsJsonArray("classHistory"); 8169 for (int i = 0; i < array.size(); i++) { 8170 res.getClassHistory().add(parseEncounterClassHistoryComponent(array.get(i).getAsJsonObject(), res)); 8171 } 8172 }; 8173 if (json.has("type")) { 8174 JsonArray array = json.getAsJsonArray("type"); 8175 for (int i = 0; i < array.size(); i++) { 8176 res.getType().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 8177 } 8178 }; 8179 if (json.has("priority")) 8180 res.setPriority(parseCodeableConcept(getJObject(json, "priority"))); 8181 if (json.has("subject")) 8182 res.setSubject(parseReference(getJObject(json, "subject"))); 8183 if (json.has("episodeOfCare")) { 8184 JsonArray array = json.getAsJsonArray("episodeOfCare"); 8185 for (int i = 0; i < array.size(); i++) { 8186 res.getEpisodeOfCare().add(parseReference(array.get(i).getAsJsonObject())); 8187 } 8188 }; 8189 if (json.has("incomingReferral")) { 8190 JsonArray array = json.getAsJsonArray("incomingReferral"); 8191 for (int i = 0; i < array.size(); i++) { 8192 res.getIncomingReferral().add(parseReference(array.get(i).getAsJsonObject())); 8193 } 8194 }; 8195 if (json.has("participant")) { 8196 JsonArray array = json.getAsJsonArray("participant"); 8197 for (int i = 0; i < array.size(); i++) { 8198 res.getParticipant().add(parseEncounterEncounterParticipantComponent(array.get(i).getAsJsonObject(), res)); 8199 } 8200 }; 8201 if (json.has("appointment")) 8202 res.setAppointment(parseReference(getJObject(json, "appointment"))); 8203 if (json.has("period")) 8204 res.setPeriod(parsePeriod(getJObject(json, "period"))); 8205 if (json.has("length")) 8206 res.setLength(parseDuration(getJObject(json, "length"))); 8207 if (json.has("reason")) { 8208 JsonArray array = json.getAsJsonArray("reason"); 8209 for (int i = 0; i < array.size(); i++) { 8210 res.getReason().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 8211 } 8212 }; 8213 if (json.has("diagnosis")) { 8214 JsonArray array = json.getAsJsonArray("diagnosis"); 8215 for (int i = 0; i < array.size(); i++) { 8216 res.getDiagnosis().add(parseEncounterDiagnosisComponent(array.get(i).getAsJsonObject(), res)); 8217 } 8218 }; 8219 if (json.has("account")) { 8220 JsonArray array = json.getAsJsonArray("account"); 8221 for (int i = 0; i < array.size(); i++) { 8222 res.getAccount().add(parseReference(array.get(i).getAsJsonObject())); 8223 } 8224 }; 8225 if (json.has("hospitalization")) 8226 res.setHospitalization(parseEncounterEncounterHospitalizationComponent(getJObject(json, "hospitalization"), res)); 8227 if (json.has("location")) { 8228 JsonArray array = json.getAsJsonArray("location"); 8229 for (int i = 0; i < array.size(); i++) { 8230 res.getLocation().add(parseEncounterEncounterLocationComponent(array.get(i).getAsJsonObject(), res)); 8231 } 8232 }; 8233 if (json.has("serviceProvider")) 8234 res.setServiceProvider(parseReference(getJObject(json, "serviceProvider"))); 8235 if (json.has("partOf")) 8236 res.setPartOf(parseReference(getJObject(json, "partOf"))); 8237 } 8238 8239 protected Encounter.StatusHistoryComponent parseEncounterStatusHistoryComponent(JsonObject json, Encounter owner) throws IOException, FHIRFormatError { 8240 Encounter.StatusHistoryComponent res = new Encounter.StatusHistoryComponent(); 8241 parseEncounterStatusHistoryComponentProperties(json, owner, res); 8242 return res; 8243 } 8244 8245 protected void parseEncounterStatusHistoryComponentProperties(JsonObject json, Encounter owner, Encounter.StatusHistoryComponent res) throws IOException, FHIRFormatError { 8246 parseBackboneProperties(json, res); 8247 if (json.has("status")) 8248 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Encounter.EncounterStatus.NULL, new Encounter.EncounterStatusEnumFactory())); 8249 if (json.has("_status")) 8250 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 8251 if (json.has("period")) 8252 res.setPeriod(parsePeriod(getJObject(json, "period"))); 8253 } 8254 8255 protected Encounter.ClassHistoryComponent parseEncounterClassHistoryComponent(JsonObject json, Encounter owner) throws IOException, FHIRFormatError { 8256 Encounter.ClassHistoryComponent res = new Encounter.ClassHistoryComponent(); 8257 parseEncounterClassHistoryComponentProperties(json, owner, res); 8258 return res; 8259 } 8260 8261 protected void parseEncounterClassHistoryComponentProperties(JsonObject json, Encounter owner, Encounter.ClassHistoryComponent res) throws IOException, FHIRFormatError { 8262 parseBackboneProperties(json, res); 8263 if (json.has("class")) 8264 res.setClass_(parseCoding(getJObject(json, "class"))); 8265 if (json.has("period")) 8266 res.setPeriod(parsePeriod(getJObject(json, "period"))); 8267 } 8268 8269 protected Encounter.EncounterParticipantComponent parseEncounterEncounterParticipantComponent(JsonObject json, Encounter owner) throws IOException, FHIRFormatError { 8270 Encounter.EncounterParticipantComponent res = new Encounter.EncounterParticipantComponent(); 8271 parseEncounterEncounterParticipantComponentProperties(json, owner, res); 8272 return res; 8273 } 8274 8275 protected void parseEncounterEncounterParticipantComponentProperties(JsonObject json, Encounter owner, Encounter.EncounterParticipantComponent res) throws IOException, FHIRFormatError { 8276 parseBackboneProperties(json, res); 8277 if (json.has("type")) { 8278 JsonArray array = json.getAsJsonArray("type"); 8279 for (int i = 0; i < array.size(); i++) { 8280 res.getType().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 8281 } 8282 }; 8283 if (json.has("period")) 8284 res.setPeriod(parsePeriod(getJObject(json, "period"))); 8285 if (json.has("individual")) 8286 res.setIndividual(parseReference(getJObject(json, "individual"))); 8287 } 8288 8289 protected Encounter.DiagnosisComponent parseEncounterDiagnosisComponent(JsonObject json, Encounter owner) throws IOException, FHIRFormatError { 8290 Encounter.DiagnosisComponent res = new Encounter.DiagnosisComponent(); 8291 parseEncounterDiagnosisComponentProperties(json, owner, res); 8292 return res; 8293 } 8294 8295 protected void parseEncounterDiagnosisComponentProperties(JsonObject json, Encounter owner, Encounter.DiagnosisComponent res) throws IOException, FHIRFormatError { 8296 parseBackboneProperties(json, res); 8297 if (json.has("condition")) 8298 res.setCondition(parseReference(getJObject(json, "condition"))); 8299 if (json.has("role")) 8300 res.setRole(parseCodeableConcept(getJObject(json, "role"))); 8301 if (json.has("rank")) 8302 res.setRankElement(parsePositiveInt(json.get("rank").getAsString())); 8303 if (json.has("_rank")) 8304 parseElementProperties(getJObject(json, "_rank"), res.getRankElement()); 8305 } 8306 8307 protected Encounter.EncounterHospitalizationComponent parseEncounterEncounterHospitalizationComponent(JsonObject json, Encounter owner) throws IOException, FHIRFormatError { 8308 Encounter.EncounterHospitalizationComponent res = new Encounter.EncounterHospitalizationComponent(); 8309 parseEncounterEncounterHospitalizationComponentProperties(json, owner, res); 8310 return res; 8311 } 8312 8313 protected void parseEncounterEncounterHospitalizationComponentProperties(JsonObject json, Encounter owner, Encounter.EncounterHospitalizationComponent res) throws IOException, FHIRFormatError { 8314 parseBackboneProperties(json, res); 8315 if (json.has("preAdmissionIdentifier")) 8316 res.setPreAdmissionIdentifier(parseIdentifier(getJObject(json, "preAdmissionIdentifier"))); 8317 if (json.has("origin")) 8318 res.setOrigin(parseReference(getJObject(json, "origin"))); 8319 if (json.has("admitSource")) 8320 res.setAdmitSource(parseCodeableConcept(getJObject(json, "admitSource"))); 8321 if (json.has("reAdmission")) 8322 res.setReAdmission(parseCodeableConcept(getJObject(json, "reAdmission"))); 8323 if (json.has("dietPreference")) { 8324 JsonArray array = json.getAsJsonArray("dietPreference"); 8325 for (int i = 0; i < array.size(); i++) { 8326 res.getDietPreference().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 8327 } 8328 }; 8329 if (json.has("specialCourtesy")) { 8330 JsonArray array = json.getAsJsonArray("specialCourtesy"); 8331 for (int i = 0; i < array.size(); i++) { 8332 res.getSpecialCourtesy().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 8333 } 8334 }; 8335 if (json.has("specialArrangement")) { 8336 JsonArray array = json.getAsJsonArray("specialArrangement"); 8337 for (int i = 0; i < array.size(); i++) { 8338 res.getSpecialArrangement().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 8339 } 8340 }; 8341 if (json.has("destination")) 8342 res.setDestination(parseReference(getJObject(json, "destination"))); 8343 if (json.has("dischargeDisposition")) 8344 res.setDischargeDisposition(parseCodeableConcept(getJObject(json, "dischargeDisposition"))); 8345 } 8346 8347 protected Encounter.EncounterLocationComponent parseEncounterEncounterLocationComponent(JsonObject json, Encounter owner) throws IOException, FHIRFormatError { 8348 Encounter.EncounterLocationComponent res = new Encounter.EncounterLocationComponent(); 8349 parseEncounterEncounterLocationComponentProperties(json, owner, res); 8350 return res; 8351 } 8352 8353 protected void parseEncounterEncounterLocationComponentProperties(JsonObject json, Encounter owner, Encounter.EncounterLocationComponent res) throws IOException, FHIRFormatError { 8354 parseBackboneProperties(json, res); 8355 if (json.has("location")) 8356 res.setLocation(parseReference(getJObject(json, "location"))); 8357 if (json.has("status")) 8358 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Encounter.EncounterLocationStatus.NULL, new Encounter.EncounterLocationStatusEnumFactory())); 8359 if (json.has("_status")) 8360 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 8361 if (json.has("period")) 8362 res.setPeriod(parsePeriod(getJObject(json, "period"))); 8363 } 8364 8365 protected Endpoint parseEndpoint(JsonObject json) throws IOException, FHIRFormatError { 8366 Endpoint res = new Endpoint(); 8367 parseEndpointProperties(json, res); 8368 return res; 8369 } 8370 8371 protected void parseEndpointProperties(JsonObject json, Endpoint res) throws IOException, FHIRFormatError { 8372 parseDomainResourceProperties(json, res); 8373 if (json.has("identifier")) { 8374 JsonArray array = json.getAsJsonArray("identifier"); 8375 for (int i = 0; i < array.size(); i++) { 8376 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 8377 } 8378 }; 8379 if (json.has("status")) 8380 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Endpoint.EndpointStatus.NULL, new Endpoint.EndpointStatusEnumFactory())); 8381 if (json.has("_status")) 8382 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 8383 if (json.has("connectionType")) 8384 res.setConnectionType(parseCoding(getJObject(json, "connectionType"))); 8385 if (json.has("name")) 8386 res.setNameElement(parseString(json.get("name").getAsString())); 8387 if (json.has("_name")) 8388 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 8389 if (json.has("managingOrganization")) 8390 res.setManagingOrganization(parseReference(getJObject(json, "managingOrganization"))); 8391 if (json.has("contact")) { 8392 JsonArray array = json.getAsJsonArray("contact"); 8393 for (int i = 0; i < array.size(); i++) { 8394 res.getContact().add(parseContactPoint(array.get(i).getAsJsonObject())); 8395 } 8396 }; 8397 if (json.has("period")) 8398 res.setPeriod(parsePeriod(getJObject(json, "period"))); 8399 if (json.has("payloadType")) { 8400 JsonArray array = json.getAsJsonArray("payloadType"); 8401 for (int i = 0; i < array.size(); i++) { 8402 res.getPayloadType().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 8403 } 8404 }; 8405 if (json.has("payloadMimeType")) { 8406 JsonArray array = json.getAsJsonArray("payloadMimeType"); 8407 for (int i = 0; i < array.size(); i++) { 8408 if (array.get(i).isJsonNull()) { 8409 res.getPayloadMimeType().add(new CodeType()); 8410 } else { 8411 res.getPayloadMimeType().add(parseCode(array.get(i).getAsString())); 8412 } 8413 } 8414 }; 8415 if (json.has("_payloadMimeType")) { 8416 JsonArray array = json.getAsJsonArray("_payloadMimeType"); 8417 for (int i = 0; i < array.size(); i++) { 8418 if (i == res.getPayloadMimeType().size()) 8419 res.getPayloadMimeType().add(parseCode(null)); 8420 if (array.get(i) instanceof JsonObject) 8421 parseElementProperties(array.get(i).getAsJsonObject(), res.getPayloadMimeType().get(i)); 8422 } 8423 }; 8424 if (json.has("address")) 8425 res.setAddressElement(parseUri(json.get("address").getAsString())); 8426 if (json.has("_address")) 8427 parseElementProperties(getJObject(json, "_address"), res.getAddressElement()); 8428 if (json.has("header")) { 8429 JsonArray array = json.getAsJsonArray("header"); 8430 for (int i = 0; i < array.size(); i++) { 8431 if (array.get(i).isJsonNull()) { 8432 res.getHeader().add(new StringType()); 8433 } else { 8434 res.getHeader().add(parseString(array.get(i).getAsString())); 8435 } 8436 } 8437 }; 8438 if (json.has("_header")) { 8439 JsonArray array = json.getAsJsonArray("_header"); 8440 for (int i = 0; i < array.size(); i++) { 8441 if (i == res.getHeader().size()) 8442 res.getHeader().add(parseString(null)); 8443 if (array.get(i) instanceof JsonObject) 8444 parseElementProperties(array.get(i).getAsJsonObject(), res.getHeader().get(i)); 8445 } 8446 }; 8447 } 8448 8449 protected EnrollmentRequest parseEnrollmentRequest(JsonObject json) throws IOException, FHIRFormatError { 8450 EnrollmentRequest res = new EnrollmentRequest(); 8451 parseEnrollmentRequestProperties(json, res); 8452 return res; 8453 } 8454 8455 protected void parseEnrollmentRequestProperties(JsonObject json, EnrollmentRequest res) throws IOException, FHIRFormatError { 8456 parseDomainResourceProperties(json, res); 8457 if (json.has("identifier")) { 8458 JsonArray array = json.getAsJsonArray("identifier"); 8459 for (int i = 0; i < array.size(); i++) { 8460 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 8461 } 8462 }; 8463 if (json.has("status")) 8464 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), EnrollmentRequest.EnrollmentRequestStatus.NULL, new EnrollmentRequest.EnrollmentRequestStatusEnumFactory())); 8465 if (json.has("_status")) 8466 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 8467 if (json.has("created")) 8468 res.setCreatedElement(parseDateTime(json.get("created").getAsString())); 8469 if (json.has("_created")) 8470 parseElementProperties(getJObject(json, "_created"), res.getCreatedElement()); 8471 if (json.has("insurer")) 8472 res.setInsurer(parseReference(getJObject(json, "insurer"))); 8473 if (json.has("provider")) 8474 res.setProvider(parseReference(getJObject(json, "provider"))); 8475 if (json.has("organization")) 8476 res.setOrganization(parseReference(getJObject(json, "organization"))); 8477 if (json.has("subject")) 8478 res.setSubject(parseReference(getJObject(json, "subject"))); 8479 if (json.has("coverage")) 8480 res.setCoverage(parseReference(getJObject(json, "coverage"))); 8481 } 8482 8483 protected EnrollmentResponse parseEnrollmentResponse(JsonObject json) throws IOException, FHIRFormatError { 8484 EnrollmentResponse res = new EnrollmentResponse(); 8485 parseEnrollmentResponseProperties(json, res); 8486 return res; 8487 } 8488 8489 protected void parseEnrollmentResponseProperties(JsonObject json, EnrollmentResponse res) throws IOException, FHIRFormatError { 8490 parseDomainResourceProperties(json, res); 8491 if (json.has("identifier")) { 8492 JsonArray array = json.getAsJsonArray("identifier"); 8493 for (int i = 0; i < array.size(); i++) { 8494 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 8495 } 8496 }; 8497 if (json.has("status")) 8498 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), EnrollmentResponse.EnrollmentResponseStatus.NULL, new EnrollmentResponse.EnrollmentResponseStatusEnumFactory())); 8499 if (json.has("_status")) 8500 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 8501 if (json.has("request")) 8502 res.setRequest(parseReference(getJObject(json, "request"))); 8503 if (json.has("outcome")) 8504 res.setOutcome(parseCodeableConcept(getJObject(json, "outcome"))); 8505 if (json.has("disposition")) 8506 res.setDispositionElement(parseString(json.get("disposition").getAsString())); 8507 if (json.has("_disposition")) 8508 parseElementProperties(getJObject(json, "_disposition"), res.getDispositionElement()); 8509 if (json.has("created")) 8510 res.setCreatedElement(parseDateTime(json.get("created").getAsString())); 8511 if (json.has("_created")) 8512 parseElementProperties(getJObject(json, "_created"), res.getCreatedElement()); 8513 if (json.has("organization")) 8514 res.setOrganization(parseReference(getJObject(json, "organization"))); 8515 if (json.has("requestProvider")) 8516 res.setRequestProvider(parseReference(getJObject(json, "requestProvider"))); 8517 if (json.has("requestOrganization")) 8518 res.setRequestOrganization(parseReference(getJObject(json, "requestOrganization"))); 8519 } 8520 8521 protected EpisodeOfCare parseEpisodeOfCare(JsonObject json) throws IOException, FHIRFormatError { 8522 EpisodeOfCare res = new EpisodeOfCare(); 8523 parseEpisodeOfCareProperties(json, res); 8524 return res; 8525 } 8526 8527 protected void parseEpisodeOfCareProperties(JsonObject json, EpisodeOfCare res) throws IOException, FHIRFormatError { 8528 parseDomainResourceProperties(json, res); 8529 if (json.has("identifier")) { 8530 JsonArray array = json.getAsJsonArray("identifier"); 8531 for (int i = 0; i < array.size(); i++) { 8532 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 8533 } 8534 }; 8535 if (json.has("status")) 8536 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), EpisodeOfCare.EpisodeOfCareStatus.NULL, new EpisodeOfCare.EpisodeOfCareStatusEnumFactory())); 8537 if (json.has("_status")) 8538 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 8539 if (json.has("statusHistory")) { 8540 JsonArray array = json.getAsJsonArray("statusHistory"); 8541 for (int i = 0; i < array.size(); i++) { 8542 res.getStatusHistory().add(parseEpisodeOfCareEpisodeOfCareStatusHistoryComponent(array.get(i).getAsJsonObject(), res)); 8543 } 8544 }; 8545 if (json.has("type")) { 8546 JsonArray array = json.getAsJsonArray("type"); 8547 for (int i = 0; i < array.size(); i++) { 8548 res.getType().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 8549 } 8550 }; 8551 if (json.has("diagnosis")) { 8552 JsonArray array = json.getAsJsonArray("diagnosis"); 8553 for (int i = 0; i < array.size(); i++) { 8554 res.getDiagnosis().add(parseEpisodeOfCareDiagnosisComponent(array.get(i).getAsJsonObject(), res)); 8555 } 8556 }; 8557 if (json.has("patient")) 8558 res.setPatient(parseReference(getJObject(json, "patient"))); 8559 if (json.has("managingOrganization")) 8560 res.setManagingOrganization(parseReference(getJObject(json, "managingOrganization"))); 8561 if (json.has("period")) 8562 res.setPeriod(parsePeriod(getJObject(json, "period"))); 8563 if (json.has("referralRequest")) { 8564 JsonArray array = json.getAsJsonArray("referralRequest"); 8565 for (int i = 0; i < array.size(); i++) { 8566 res.getReferralRequest().add(parseReference(array.get(i).getAsJsonObject())); 8567 } 8568 }; 8569 if (json.has("careManager")) 8570 res.setCareManager(parseReference(getJObject(json, "careManager"))); 8571 if (json.has("team")) { 8572 JsonArray array = json.getAsJsonArray("team"); 8573 for (int i = 0; i < array.size(); i++) { 8574 res.getTeam().add(parseReference(array.get(i).getAsJsonObject())); 8575 } 8576 }; 8577 if (json.has("account")) { 8578 JsonArray array = json.getAsJsonArray("account"); 8579 for (int i = 0; i < array.size(); i++) { 8580 res.getAccount().add(parseReference(array.get(i).getAsJsonObject())); 8581 } 8582 }; 8583 } 8584 8585 protected EpisodeOfCare.EpisodeOfCareStatusHistoryComponent parseEpisodeOfCareEpisodeOfCareStatusHistoryComponent(JsonObject json, EpisodeOfCare owner) throws IOException, FHIRFormatError { 8586 EpisodeOfCare.EpisodeOfCareStatusHistoryComponent res = new EpisodeOfCare.EpisodeOfCareStatusHistoryComponent(); 8587 parseEpisodeOfCareEpisodeOfCareStatusHistoryComponentProperties(json, owner, res); 8588 return res; 8589 } 8590 8591 protected void parseEpisodeOfCareEpisodeOfCareStatusHistoryComponentProperties(JsonObject json, EpisodeOfCare owner, EpisodeOfCare.EpisodeOfCareStatusHistoryComponent res) throws IOException, FHIRFormatError { 8592 parseBackboneProperties(json, res); 8593 if (json.has("status")) 8594 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), EpisodeOfCare.EpisodeOfCareStatus.NULL, new EpisodeOfCare.EpisodeOfCareStatusEnumFactory())); 8595 if (json.has("_status")) 8596 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 8597 if (json.has("period")) 8598 res.setPeriod(parsePeriod(getJObject(json, "period"))); 8599 } 8600 8601 protected EpisodeOfCare.DiagnosisComponent parseEpisodeOfCareDiagnosisComponent(JsonObject json, EpisodeOfCare owner) throws IOException, FHIRFormatError { 8602 EpisodeOfCare.DiagnosisComponent res = new EpisodeOfCare.DiagnosisComponent(); 8603 parseEpisodeOfCareDiagnosisComponentProperties(json, owner, res); 8604 return res; 8605 } 8606 8607 protected void parseEpisodeOfCareDiagnosisComponentProperties(JsonObject json, EpisodeOfCare owner, EpisodeOfCare.DiagnosisComponent res) throws IOException, FHIRFormatError { 8608 parseBackboneProperties(json, res); 8609 if (json.has("condition")) 8610 res.setCondition(parseReference(getJObject(json, "condition"))); 8611 if (json.has("role")) 8612 res.setRole(parseCodeableConcept(getJObject(json, "role"))); 8613 if (json.has("rank")) 8614 res.setRankElement(parsePositiveInt(json.get("rank").getAsString())); 8615 if (json.has("_rank")) 8616 parseElementProperties(getJObject(json, "_rank"), res.getRankElement()); 8617 } 8618 8619 protected ExpansionProfile parseExpansionProfile(JsonObject json) throws IOException, FHIRFormatError { 8620 ExpansionProfile res = new ExpansionProfile(); 8621 parseExpansionProfileProperties(json, res); 8622 return res; 8623 } 8624 8625 protected void parseExpansionProfileProperties(JsonObject json, ExpansionProfile res) throws IOException, FHIRFormatError { 8626 parseDomainResourceProperties(json, res); 8627 if (json.has("url")) 8628 res.setUrlElement(parseUri(json.get("url").getAsString())); 8629 if (json.has("_url")) 8630 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 8631 if (json.has("identifier")) 8632 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 8633 if (json.has("version")) 8634 res.setVersionElement(parseString(json.get("version").getAsString())); 8635 if (json.has("_version")) 8636 parseElementProperties(getJObject(json, "_version"), res.getVersionElement()); 8637 if (json.has("name")) 8638 res.setNameElement(parseString(json.get("name").getAsString())); 8639 if (json.has("_name")) 8640 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 8641 if (json.has("status")) 8642 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Enumerations.PublicationStatus.NULL, new Enumerations.PublicationStatusEnumFactory())); 8643 if (json.has("_status")) 8644 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 8645 if (json.has("experimental")) 8646 res.setExperimentalElement(parseBoolean(json.get("experimental").getAsBoolean())); 8647 if (json.has("_experimental")) 8648 parseElementProperties(getJObject(json, "_experimental"), res.getExperimentalElement()); 8649 if (json.has("date")) 8650 res.setDateElement(parseDateTime(json.get("date").getAsString())); 8651 if (json.has("_date")) 8652 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 8653 if (json.has("publisher")) 8654 res.setPublisherElement(parseString(json.get("publisher").getAsString())); 8655 if (json.has("_publisher")) 8656 parseElementProperties(getJObject(json, "_publisher"), res.getPublisherElement()); 8657 if (json.has("contact")) { 8658 JsonArray array = json.getAsJsonArray("contact"); 8659 for (int i = 0; i < array.size(); i++) { 8660 res.getContact().add(parseContactDetail(array.get(i).getAsJsonObject())); 8661 } 8662 }; 8663 if (json.has("description")) 8664 res.setDescriptionElement(parseMarkdown(json.get("description").getAsString())); 8665 if (json.has("_description")) 8666 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 8667 if (json.has("useContext")) { 8668 JsonArray array = json.getAsJsonArray("useContext"); 8669 for (int i = 0; i < array.size(); i++) { 8670 res.getUseContext().add(parseUsageContext(array.get(i).getAsJsonObject())); 8671 } 8672 }; 8673 if (json.has("jurisdiction")) { 8674 JsonArray array = json.getAsJsonArray("jurisdiction"); 8675 for (int i = 0; i < array.size(); i++) { 8676 res.getJurisdiction().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 8677 } 8678 }; 8679 if (json.has("fixedVersion")) { 8680 JsonArray array = json.getAsJsonArray("fixedVersion"); 8681 for (int i = 0; i < array.size(); i++) { 8682 res.getFixedVersion().add(parseExpansionProfileExpansionProfileFixedVersionComponent(array.get(i).getAsJsonObject(), res)); 8683 } 8684 }; 8685 if (json.has("excludedSystem")) 8686 res.setExcludedSystem(parseExpansionProfileExpansionProfileExcludedSystemComponent(getJObject(json, "excludedSystem"), res)); 8687 if (json.has("includeDesignations")) 8688 res.setIncludeDesignationsElement(parseBoolean(json.get("includeDesignations").getAsBoolean())); 8689 if (json.has("_includeDesignations")) 8690 parseElementProperties(getJObject(json, "_includeDesignations"), res.getIncludeDesignationsElement()); 8691 if (json.has("designation")) 8692 res.setDesignation(parseExpansionProfileExpansionProfileDesignationComponent(getJObject(json, "designation"), res)); 8693 if (json.has("includeDefinition")) 8694 res.setIncludeDefinitionElement(parseBoolean(json.get("includeDefinition").getAsBoolean())); 8695 if (json.has("_includeDefinition")) 8696 parseElementProperties(getJObject(json, "_includeDefinition"), res.getIncludeDefinitionElement()); 8697 if (json.has("activeOnly")) 8698 res.setActiveOnlyElement(parseBoolean(json.get("activeOnly").getAsBoolean())); 8699 if (json.has("_activeOnly")) 8700 parseElementProperties(getJObject(json, "_activeOnly"), res.getActiveOnlyElement()); 8701 if (json.has("excludeNested")) 8702 res.setExcludeNestedElement(parseBoolean(json.get("excludeNested").getAsBoolean())); 8703 if (json.has("_excludeNested")) 8704 parseElementProperties(getJObject(json, "_excludeNested"), res.getExcludeNestedElement()); 8705 if (json.has("excludeNotForUI")) 8706 res.setExcludeNotForUIElement(parseBoolean(json.get("excludeNotForUI").getAsBoolean())); 8707 if (json.has("_excludeNotForUI")) 8708 parseElementProperties(getJObject(json, "_excludeNotForUI"), res.getExcludeNotForUIElement()); 8709 if (json.has("excludePostCoordinated")) 8710 res.setExcludePostCoordinatedElement(parseBoolean(json.get("excludePostCoordinated").getAsBoolean())); 8711 if (json.has("_excludePostCoordinated")) 8712 parseElementProperties(getJObject(json, "_excludePostCoordinated"), res.getExcludePostCoordinatedElement()); 8713 if (json.has("displayLanguage")) 8714 res.setDisplayLanguageElement(parseCode(json.get("displayLanguage").getAsString())); 8715 if (json.has("_displayLanguage")) 8716 parseElementProperties(getJObject(json, "_displayLanguage"), res.getDisplayLanguageElement()); 8717 if (json.has("limitedExpansion")) 8718 res.setLimitedExpansionElement(parseBoolean(json.get("limitedExpansion").getAsBoolean())); 8719 if (json.has("_limitedExpansion")) 8720 parseElementProperties(getJObject(json, "_limitedExpansion"), res.getLimitedExpansionElement()); 8721 } 8722 8723 protected ExpansionProfile.ExpansionProfileFixedVersionComponent parseExpansionProfileExpansionProfileFixedVersionComponent(JsonObject json, ExpansionProfile owner) throws IOException, FHIRFormatError { 8724 ExpansionProfile.ExpansionProfileFixedVersionComponent res = new ExpansionProfile.ExpansionProfileFixedVersionComponent(); 8725 parseExpansionProfileExpansionProfileFixedVersionComponentProperties(json, owner, res); 8726 return res; 8727 } 8728 8729 protected void parseExpansionProfileExpansionProfileFixedVersionComponentProperties(JsonObject json, ExpansionProfile owner, ExpansionProfile.ExpansionProfileFixedVersionComponent res) throws IOException, FHIRFormatError { 8730 parseBackboneProperties(json, res); 8731 if (json.has("system")) 8732 res.setSystemElement(parseUri(json.get("system").getAsString())); 8733 if (json.has("_system")) 8734 parseElementProperties(getJObject(json, "_system"), res.getSystemElement()); 8735 if (json.has("version")) 8736 res.setVersionElement(parseString(json.get("version").getAsString())); 8737 if (json.has("_version")) 8738 parseElementProperties(getJObject(json, "_version"), res.getVersionElement()); 8739 if (json.has("mode")) 8740 res.setModeElement(parseEnumeration(json.get("mode").getAsString(), ExpansionProfile.SystemVersionProcessingMode.NULL, new ExpansionProfile.SystemVersionProcessingModeEnumFactory())); 8741 if (json.has("_mode")) 8742 parseElementProperties(getJObject(json, "_mode"), res.getModeElement()); 8743 } 8744 8745 protected ExpansionProfile.ExpansionProfileExcludedSystemComponent parseExpansionProfileExpansionProfileExcludedSystemComponent(JsonObject json, ExpansionProfile owner) throws IOException, FHIRFormatError { 8746 ExpansionProfile.ExpansionProfileExcludedSystemComponent res = new ExpansionProfile.ExpansionProfileExcludedSystemComponent(); 8747 parseExpansionProfileExpansionProfileExcludedSystemComponentProperties(json, owner, res); 8748 return res; 8749 } 8750 8751 protected void parseExpansionProfileExpansionProfileExcludedSystemComponentProperties(JsonObject json, ExpansionProfile owner, ExpansionProfile.ExpansionProfileExcludedSystemComponent res) throws IOException, FHIRFormatError { 8752 parseBackboneProperties(json, res); 8753 if (json.has("system")) 8754 res.setSystemElement(parseUri(json.get("system").getAsString())); 8755 if (json.has("_system")) 8756 parseElementProperties(getJObject(json, "_system"), res.getSystemElement()); 8757 if (json.has("version")) 8758 res.setVersionElement(parseString(json.get("version").getAsString())); 8759 if (json.has("_version")) 8760 parseElementProperties(getJObject(json, "_version"), res.getVersionElement()); 8761 } 8762 8763 protected ExpansionProfile.ExpansionProfileDesignationComponent parseExpansionProfileExpansionProfileDesignationComponent(JsonObject json, ExpansionProfile owner) throws IOException, FHIRFormatError { 8764 ExpansionProfile.ExpansionProfileDesignationComponent res = new ExpansionProfile.ExpansionProfileDesignationComponent(); 8765 parseExpansionProfileExpansionProfileDesignationComponentProperties(json, owner, res); 8766 return res; 8767 } 8768 8769 protected void parseExpansionProfileExpansionProfileDesignationComponentProperties(JsonObject json, ExpansionProfile owner, ExpansionProfile.ExpansionProfileDesignationComponent res) throws IOException, FHIRFormatError { 8770 parseBackboneProperties(json, res); 8771 if (json.has("include")) 8772 res.setInclude(parseExpansionProfileDesignationIncludeComponent(getJObject(json, "include"), owner)); 8773 if (json.has("exclude")) 8774 res.setExclude(parseExpansionProfileDesignationExcludeComponent(getJObject(json, "exclude"), owner)); 8775 } 8776 8777 protected ExpansionProfile.DesignationIncludeComponent parseExpansionProfileDesignationIncludeComponent(JsonObject json, ExpansionProfile owner) throws IOException, FHIRFormatError { 8778 ExpansionProfile.DesignationIncludeComponent res = new ExpansionProfile.DesignationIncludeComponent(); 8779 parseExpansionProfileDesignationIncludeComponentProperties(json, owner, res); 8780 return res; 8781 } 8782 8783 protected void parseExpansionProfileDesignationIncludeComponentProperties(JsonObject json, ExpansionProfile owner, ExpansionProfile.DesignationIncludeComponent res) throws IOException, FHIRFormatError { 8784 parseBackboneProperties(json, res); 8785 if (json.has("designation")) { 8786 JsonArray array = json.getAsJsonArray("designation"); 8787 for (int i = 0; i < array.size(); i++) { 8788 res.getDesignation().add(parseExpansionProfileDesignationIncludeDesignationComponent(array.get(i).getAsJsonObject(), owner)); 8789 } 8790 }; 8791 } 8792 8793 protected ExpansionProfile.DesignationIncludeDesignationComponent parseExpansionProfileDesignationIncludeDesignationComponent(JsonObject json, ExpansionProfile owner) throws IOException, FHIRFormatError { 8794 ExpansionProfile.DesignationIncludeDesignationComponent res = new ExpansionProfile.DesignationIncludeDesignationComponent(); 8795 parseExpansionProfileDesignationIncludeDesignationComponentProperties(json, owner, res); 8796 return res; 8797 } 8798 8799 protected void parseExpansionProfileDesignationIncludeDesignationComponentProperties(JsonObject json, ExpansionProfile owner, ExpansionProfile.DesignationIncludeDesignationComponent res) throws IOException, FHIRFormatError { 8800 parseBackboneProperties(json, res); 8801 if (json.has("language")) 8802 res.setLanguageElement(parseCode(json.get("language").getAsString())); 8803 if (json.has("_language")) 8804 parseElementProperties(getJObject(json, "_language"), res.getLanguageElement()); 8805 if (json.has("use")) 8806 res.setUse(parseCoding(getJObject(json, "use"))); 8807 } 8808 8809 protected ExpansionProfile.DesignationExcludeComponent parseExpansionProfileDesignationExcludeComponent(JsonObject json, ExpansionProfile owner) throws IOException, FHIRFormatError { 8810 ExpansionProfile.DesignationExcludeComponent res = new ExpansionProfile.DesignationExcludeComponent(); 8811 parseExpansionProfileDesignationExcludeComponentProperties(json, owner, res); 8812 return res; 8813 } 8814 8815 protected void parseExpansionProfileDesignationExcludeComponentProperties(JsonObject json, ExpansionProfile owner, ExpansionProfile.DesignationExcludeComponent res) throws IOException, FHIRFormatError { 8816 parseBackboneProperties(json, res); 8817 if (json.has("designation")) { 8818 JsonArray array = json.getAsJsonArray("designation"); 8819 for (int i = 0; i < array.size(); i++) { 8820 res.getDesignation().add(parseExpansionProfileDesignationExcludeDesignationComponent(array.get(i).getAsJsonObject(), owner)); 8821 } 8822 }; 8823 } 8824 8825 protected ExpansionProfile.DesignationExcludeDesignationComponent parseExpansionProfileDesignationExcludeDesignationComponent(JsonObject json, ExpansionProfile owner) throws IOException, FHIRFormatError { 8826 ExpansionProfile.DesignationExcludeDesignationComponent res = new ExpansionProfile.DesignationExcludeDesignationComponent(); 8827 parseExpansionProfileDesignationExcludeDesignationComponentProperties(json, owner, res); 8828 return res; 8829 } 8830 8831 protected void parseExpansionProfileDesignationExcludeDesignationComponentProperties(JsonObject json, ExpansionProfile owner, ExpansionProfile.DesignationExcludeDesignationComponent res) throws IOException, FHIRFormatError { 8832 parseBackboneProperties(json, res); 8833 if (json.has("language")) 8834 res.setLanguageElement(parseCode(json.get("language").getAsString())); 8835 if (json.has("_language")) 8836 parseElementProperties(getJObject(json, "_language"), res.getLanguageElement()); 8837 if (json.has("use")) 8838 res.setUse(parseCoding(getJObject(json, "use"))); 8839 } 8840 8841 protected ExplanationOfBenefit parseExplanationOfBenefit(JsonObject json) throws IOException, FHIRFormatError { 8842 ExplanationOfBenefit res = new ExplanationOfBenefit(); 8843 parseExplanationOfBenefitProperties(json, res); 8844 return res; 8845 } 8846 8847 protected void parseExplanationOfBenefitProperties(JsonObject json, ExplanationOfBenefit res) throws IOException, FHIRFormatError { 8848 parseDomainResourceProperties(json, res); 8849 if (json.has("identifier")) { 8850 JsonArray array = json.getAsJsonArray("identifier"); 8851 for (int i = 0; i < array.size(); i++) { 8852 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 8853 } 8854 }; 8855 if (json.has("status")) 8856 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), ExplanationOfBenefit.ExplanationOfBenefitStatus.NULL, new ExplanationOfBenefit.ExplanationOfBenefitStatusEnumFactory())); 8857 if (json.has("_status")) 8858 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 8859 if (json.has("type")) 8860 res.setType(parseCodeableConcept(getJObject(json, "type"))); 8861 if (json.has("subType")) { 8862 JsonArray array = json.getAsJsonArray("subType"); 8863 for (int i = 0; i < array.size(); i++) { 8864 res.getSubType().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 8865 } 8866 }; 8867 if (json.has("patient")) 8868 res.setPatient(parseReference(getJObject(json, "patient"))); 8869 if (json.has("billablePeriod")) 8870 res.setBillablePeriod(parsePeriod(getJObject(json, "billablePeriod"))); 8871 if (json.has("created")) 8872 res.setCreatedElement(parseDateTime(json.get("created").getAsString())); 8873 if (json.has("_created")) 8874 parseElementProperties(getJObject(json, "_created"), res.getCreatedElement()); 8875 if (json.has("enterer")) 8876 res.setEnterer(parseReference(getJObject(json, "enterer"))); 8877 if (json.has("insurer")) 8878 res.setInsurer(parseReference(getJObject(json, "insurer"))); 8879 if (json.has("provider")) 8880 res.setProvider(parseReference(getJObject(json, "provider"))); 8881 if (json.has("organization")) 8882 res.setOrganization(parseReference(getJObject(json, "organization"))); 8883 if (json.has("referral")) 8884 res.setReferral(parseReference(getJObject(json, "referral"))); 8885 if (json.has("facility")) 8886 res.setFacility(parseReference(getJObject(json, "facility"))); 8887 if (json.has("claim")) 8888 res.setClaim(parseReference(getJObject(json, "claim"))); 8889 if (json.has("claimResponse")) 8890 res.setClaimResponse(parseReference(getJObject(json, "claimResponse"))); 8891 if (json.has("outcome")) 8892 res.setOutcome(parseCodeableConcept(getJObject(json, "outcome"))); 8893 if (json.has("disposition")) 8894 res.setDispositionElement(parseString(json.get("disposition").getAsString())); 8895 if (json.has("_disposition")) 8896 parseElementProperties(getJObject(json, "_disposition"), res.getDispositionElement()); 8897 if (json.has("related")) { 8898 JsonArray array = json.getAsJsonArray("related"); 8899 for (int i = 0; i < array.size(); i++) { 8900 res.getRelated().add(parseExplanationOfBenefitRelatedClaimComponent(array.get(i).getAsJsonObject(), res)); 8901 } 8902 }; 8903 if (json.has("prescription")) 8904 res.setPrescription(parseReference(getJObject(json, "prescription"))); 8905 if (json.has("originalPrescription")) 8906 res.setOriginalPrescription(parseReference(getJObject(json, "originalPrescription"))); 8907 if (json.has("payee")) 8908 res.setPayee(parseExplanationOfBenefitPayeeComponent(getJObject(json, "payee"), res)); 8909 if (json.has("information")) { 8910 JsonArray array = json.getAsJsonArray("information"); 8911 for (int i = 0; i < array.size(); i++) { 8912 res.getInformation().add(parseExplanationOfBenefitSupportingInformationComponent(array.get(i).getAsJsonObject(), res)); 8913 } 8914 }; 8915 if (json.has("careTeam")) { 8916 JsonArray array = json.getAsJsonArray("careTeam"); 8917 for (int i = 0; i < array.size(); i++) { 8918 res.getCareTeam().add(parseExplanationOfBenefitCareTeamComponent(array.get(i).getAsJsonObject(), res)); 8919 } 8920 }; 8921 if (json.has("diagnosis")) { 8922 JsonArray array = json.getAsJsonArray("diagnosis"); 8923 for (int i = 0; i < array.size(); i++) { 8924 res.getDiagnosis().add(parseExplanationOfBenefitDiagnosisComponent(array.get(i).getAsJsonObject(), res)); 8925 } 8926 }; 8927 if (json.has("procedure")) { 8928 JsonArray array = json.getAsJsonArray("procedure"); 8929 for (int i = 0; i < array.size(); i++) { 8930 res.getProcedure().add(parseExplanationOfBenefitProcedureComponent(array.get(i).getAsJsonObject(), res)); 8931 } 8932 }; 8933 if (json.has("precedence")) 8934 res.setPrecedenceElement(parsePositiveInt(json.get("precedence").getAsString())); 8935 if (json.has("_precedence")) 8936 parseElementProperties(getJObject(json, "_precedence"), res.getPrecedenceElement()); 8937 if (json.has("insurance")) 8938 res.setInsurance(parseExplanationOfBenefitInsuranceComponent(getJObject(json, "insurance"), res)); 8939 if (json.has("accident")) 8940 res.setAccident(parseExplanationOfBenefitAccidentComponent(getJObject(json, "accident"), res)); 8941 if (json.has("employmentImpacted")) 8942 res.setEmploymentImpacted(parsePeriod(getJObject(json, "employmentImpacted"))); 8943 if (json.has("hospitalization")) 8944 res.setHospitalization(parsePeriod(getJObject(json, "hospitalization"))); 8945 if (json.has("item")) { 8946 JsonArray array = json.getAsJsonArray("item"); 8947 for (int i = 0; i < array.size(); i++) { 8948 res.getItem().add(parseExplanationOfBenefitItemComponent(array.get(i).getAsJsonObject(), res)); 8949 } 8950 }; 8951 if (json.has("addItem")) { 8952 JsonArray array = json.getAsJsonArray("addItem"); 8953 for (int i = 0; i < array.size(); i++) { 8954 res.getAddItem().add(parseExplanationOfBenefitAddedItemComponent(array.get(i).getAsJsonObject(), res)); 8955 } 8956 }; 8957 if (json.has("totalCost")) 8958 res.setTotalCost(parseMoney(getJObject(json, "totalCost"))); 8959 if (json.has("unallocDeductable")) 8960 res.setUnallocDeductable(parseMoney(getJObject(json, "unallocDeductable"))); 8961 if (json.has("totalBenefit")) 8962 res.setTotalBenefit(parseMoney(getJObject(json, "totalBenefit"))); 8963 if (json.has("payment")) 8964 res.setPayment(parseExplanationOfBenefitPaymentComponent(getJObject(json, "payment"), res)); 8965 if (json.has("form")) 8966 res.setForm(parseCodeableConcept(getJObject(json, "form"))); 8967 if (json.has("processNote")) { 8968 JsonArray array = json.getAsJsonArray("processNote"); 8969 for (int i = 0; i < array.size(); i++) { 8970 res.getProcessNote().add(parseExplanationOfBenefitNoteComponent(array.get(i).getAsJsonObject(), res)); 8971 } 8972 }; 8973 if (json.has("benefitBalance")) { 8974 JsonArray array = json.getAsJsonArray("benefitBalance"); 8975 for (int i = 0; i < array.size(); i++) { 8976 res.getBenefitBalance().add(parseExplanationOfBenefitBenefitBalanceComponent(array.get(i).getAsJsonObject(), res)); 8977 } 8978 }; 8979 } 8980 8981 protected ExplanationOfBenefit.RelatedClaimComponent parseExplanationOfBenefitRelatedClaimComponent(JsonObject json, ExplanationOfBenefit owner) throws IOException, FHIRFormatError { 8982 ExplanationOfBenefit.RelatedClaimComponent res = new ExplanationOfBenefit.RelatedClaimComponent(); 8983 parseExplanationOfBenefitRelatedClaimComponentProperties(json, owner, res); 8984 return res; 8985 } 8986 8987 protected void parseExplanationOfBenefitRelatedClaimComponentProperties(JsonObject json, ExplanationOfBenefit owner, ExplanationOfBenefit.RelatedClaimComponent res) throws IOException, FHIRFormatError { 8988 parseBackboneProperties(json, res); 8989 if (json.has("claim")) 8990 res.setClaim(parseReference(getJObject(json, "claim"))); 8991 if (json.has("relationship")) 8992 res.setRelationship(parseCodeableConcept(getJObject(json, "relationship"))); 8993 if (json.has("reference")) 8994 res.setReference(parseIdentifier(getJObject(json, "reference"))); 8995 } 8996 8997 protected ExplanationOfBenefit.PayeeComponent parseExplanationOfBenefitPayeeComponent(JsonObject json, ExplanationOfBenefit owner) throws IOException, FHIRFormatError { 8998 ExplanationOfBenefit.PayeeComponent res = new ExplanationOfBenefit.PayeeComponent(); 8999 parseExplanationOfBenefitPayeeComponentProperties(json, owner, res); 9000 return res; 9001 } 9002 9003 protected void parseExplanationOfBenefitPayeeComponentProperties(JsonObject json, ExplanationOfBenefit owner, ExplanationOfBenefit.PayeeComponent res) throws IOException, FHIRFormatError { 9004 parseBackboneProperties(json, res); 9005 if (json.has("type")) 9006 res.setType(parseCodeableConcept(getJObject(json, "type"))); 9007 if (json.has("resourceType")) 9008 res.setResourceType(parseCodeableConcept(getJObject(json, "resourceType"))); 9009 if (json.has("party")) 9010 res.setParty(parseReference(getJObject(json, "party"))); 9011 } 9012 9013 protected ExplanationOfBenefit.SupportingInformationComponent parseExplanationOfBenefitSupportingInformationComponent(JsonObject json, ExplanationOfBenefit owner) throws IOException, FHIRFormatError { 9014 ExplanationOfBenefit.SupportingInformationComponent res = new ExplanationOfBenefit.SupportingInformationComponent(); 9015 parseExplanationOfBenefitSupportingInformationComponentProperties(json, owner, res); 9016 return res; 9017 } 9018 9019 protected void parseExplanationOfBenefitSupportingInformationComponentProperties(JsonObject json, ExplanationOfBenefit owner, ExplanationOfBenefit.SupportingInformationComponent res) throws IOException, FHIRFormatError { 9020 parseBackboneProperties(json, res); 9021 if (json.has("sequence")) 9022 res.setSequenceElement(parsePositiveInt(json.get("sequence").getAsString())); 9023 if (json.has("_sequence")) 9024 parseElementProperties(getJObject(json, "_sequence"), res.getSequenceElement()); 9025 if (json.has("category")) 9026 res.setCategory(parseCodeableConcept(getJObject(json, "category"))); 9027 if (json.has("code")) 9028 res.setCode(parseCodeableConcept(getJObject(json, "code"))); 9029 Type timing = parseType("timing", json); 9030 if (timing != null) 9031 res.setTiming(timing); 9032 Type value = parseType("value", json); 9033 if (value != null) 9034 res.setValue(value); 9035 if (json.has("reason")) 9036 res.setReason(parseCoding(getJObject(json, "reason"))); 9037 } 9038 9039 protected ExplanationOfBenefit.CareTeamComponent parseExplanationOfBenefitCareTeamComponent(JsonObject json, ExplanationOfBenefit owner) throws IOException, FHIRFormatError { 9040 ExplanationOfBenefit.CareTeamComponent res = new ExplanationOfBenefit.CareTeamComponent(); 9041 parseExplanationOfBenefitCareTeamComponentProperties(json, owner, res); 9042 return res; 9043 } 9044 9045 protected void parseExplanationOfBenefitCareTeamComponentProperties(JsonObject json, ExplanationOfBenefit owner, ExplanationOfBenefit.CareTeamComponent res) throws IOException, FHIRFormatError { 9046 parseBackboneProperties(json, res); 9047 if (json.has("sequence")) 9048 res.setSequenceElement(parsePositiveInt(json.get("sequence").getAsString())); 9049 if (json.has("_sequence")) 9050 parseElementProperties(getJObject(json, "_sequence"), res.getSequenceElement()); 9051 if (json.has("provider")) 9052 res.setProvider(parseReference(getJObject(json, "provider"))); 9053 if (json.has("responsible")) 9054 res.setResponsibleElement(parseBoolean(json.get("responsible").getAsBoolean())); 9055 if (json.has("_responsible")) 9056 parseElementProperties(getJObject(json, "_responsible"), res.getResponsibleElement()); 9057 if (json.has("role")) 9058 res.setRole(parseCodeableConcept(getJObject(json, "role"))); 9059 if (json.has("qualification")) 9060 res.setQualification(parseCodeableConcept(getJObject(json, "qualification"))); 9061 } 9062 9063 protected ExplanationOfBenefit.DiagnosisComponent parseExplanationOfBenefitDiagnosisComponent(JsonObject json, ExplanationOfBenefit owner) throws IOException, FHIRFormatError { 9064 ExplanationOfBenefit.DiagnosisComponent res = new ExplanationOfBenefit.DiagnosisComponent(); 9065 parseExplanationOfBenefitDiagnosisComponentProperties(json, owner, res); 9066 return res; 9067 } 9068 9069 protected void parseExplanationOfBenefitDiagnosisComponentProperties(JsonObject json, ExplanationOfBenefit owner, ExplanationOfBenefit.DiagnosisComponent res) throws IOException, FHIRFormatError { 9070 parseBackboneProperties(json, res); 9071 if (json.has("sequence")) 9072 res.setSequenceElement(parsePositiveInt(json.get("sequence").getAsString())); 9073 if (json.has("_sequence")) 9074 parseElementProperties(getJObject(json, "_sequence"), res.getSequenceElement()); 9075 Type diagnosis = parseType("diagnosis", json); 9076 if (diagnosis != null) 9077 res.setDiagnosis(diagnosis); 9078 if (json.has("type")) { 9079 JsonArray array = json.getAsJsonArray("type"); 9080 for (int i = 0; i < array.size(); i++) { 9081 res.getType().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 9082 } 9083 }; 9084 if (json.has("packageCode")) 9085 res.setPackageCode(parseCodeableConcept(getJObject(json, "packageCode"))); 9086 } 9087 9088 protected ExplanationOfBenefit.ProcedureComponent parseExplanationOfBenefitProcedureComponent(JsonObject json, ExplanationOfBenefit owner) throws IOException, FHIRFormatError { 9089 ExplanationOfBenefit.ProcedureComponent res = new ExplanationOfBenefit.ProcedureComponent(); 9090 parseExplanationOfBenefitProcedureComponentProperties(json, owner, res); 9091 return res; 9092 } 9093 9094 protected void parseExplanationOfBenefitProcedureComponentProperties(JsonObject json, ExplanationOfBenefit owner, ExplanationOfBenefit.ProcedureComponent res) throws IOException, FHIRFormatError { 9095 parseBackboneProperties(json, res); 9096 if (json.has("sequence")) 9097 res.setSequenceElement(parsePositiveInt(json.get("sequence").getAsString())); 9098 if (json.has("_sequence")) 9099 parseElementProperties(getJObject(json, "_sequence"), res.getSequenceElement()); 9100 if (json.has("date")) 9101 res.setDateElement(parseDateTime(json.get("date").getAsString())); 9102 if (json.has("_date")) 9103 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 9104 Type procedure = parseType("procedure", json); 9105 if (procedure != null) 9106 res.setProcedure(procedure); 9107 } 9108 9109 protected ExplanationOfBenefit.InsuranceComponent parseExplanationOfBenefitInsuranceComponent(JsonObject json, ExplanationOfBenefit owner) throws IOException, FHIRFormatError { 9110 ExplanationOfBenefit.InsuranceComponent res = new ExplanationOfBenefit.InsuranceComponent(); 9111 parseExplanationOfBenefitInsuranceComponentProperties(json, owner, res); 9112 return res; 9113 } 9114 9115 protected void parseExplanationOfBenefitInsuranceComponentProperties(JsonObject json, ExplanationOfBenefit owner, ExplanationOfBenefit.InsuranceComponent res) throws IOException, FHIRFormatError { 9116 parseBackboneProperties(json, res); 9117 if (json.has("coverage")) 9118 res.setCoverage(parseReference(getJObject(json, "coverage"))); 9119 if (json.has("preAuthRef")) { 9120 JsonArray array = json.getAsJsonArray("preAuthRef"); 9121 for (int i = 0; i < array.size(); i++) { 9122 if (array.get(i).isJsonNull()) { 9123 res.getPreAuthRef().add(new StringType()); 9124 } else { 9125 res.getPreAuthRef().add(parseString(array.get(i).getAsString())); 9126 } 9127 } 9128 }; 9129 if (json.has("_preAuthRef")) { 9130 JsonArray array = json.getAsJsonArray("_preAuthRef"); 9131 for (int i = 0; i < array.size(); i++) { 9132 if (i == res.getPreAuthRef().size()) 9133 res.getPreAuthRef().add(parseString(null)); 9134 if (array.get(i) instanceof JsonObject) 9135 parseElementProperties(array.get(i).getAsJsonObject(), res.getPreAuthRef().get(i)); 9136 } 9137 }; 9138 } 9139 9140 protected ExplanationOfBenefit.AccidentComponent parseExplanationOfBenefitAccidentComponent(JsonObject json, ExplanationOfBenefit owner) throws IOException, FHIRFormatError { 9141 ExplanationOfBenefit.AccidentComponent res = new ExplanationOfBenefit.AccidentComponent(); 9142 parseExplanationOfBenefitAccidentComponentProperties(json, owner, res); 9143 return res; 9144 } 9145 9146 protected void parseExplanationOfBenefitAccidentComponentProperties(JsonObject json, ExplanationOfBenefit owner, ExplanationOfBenefit.AccidentComponent res) throws IOException, FHIRFormatError { 9147 parseBackboneProperties(json, res); 9148 if (json.has("date")) 9149 res.setDateElement(parseDate(json.get("date").getAsString())); 9150 if (json.has("_date")) 9151 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 9152 if (json.has("type")) 9153 res.setType(parseCodeableConcept(getJObject(json, "type"))); 9154 Type location = parseType("location", json); 9155 if (location != null) 9156 res.setLocation(location); 9157 } 9158 9159 protected ExplanationOfBenefit.ItemComponent parseExplanationOfBenefitItemComponent(JsonObject json, ExplanationOfBenefit owner) throws IOException, FHIRFormatError { 9160 ExplanationOfBenefit.ItemComponent res = new ExplanationOfBenefit.ItemComponent(); 9161 parseExplanationOfBenefitItemComponentProperties(json, owner, res); 9162 return res; 9163 } 9164 9165 protected void parseExplanationOfBenefitItemComponentProperties(JsonObject json, ExplanationOfBenefit owner, ExplanationOfBenefit.ItemComponent res) throws IOException, FHIRFormatError { 9166 parseBackboneProperties(json, res); 9167 if (json.has("sequence")) 9168 res.setSequenceElement(parsePositiveInt(json.get("sequence").getAsString())); 9169 if (json.has("_sequence")) 9170 parseElementProperties(getJObject(json, "_sequence"), res.getSequenceElement()); 9171 if (json.has("careTeamLinkId")) { 9172 JsonArray array = json.getAsJsonArray("careTeamLinkId"); 9173 for (int i = 0; i < array.size(); i++) { 9174 if (array.get(i).isJsonNull()) { 9175 res.getCareTeamLinkId().add(new PositiveIntType()); 9176 } else { 9177 res.getCareTeamLinkId().add(parsePositiveInt(array.get(i).getAsString())); 9178 } 9179 } 9180 }; 9181 if (json.has("_careTeamLinkId")) { 9182 JsonArray array = json.getAsJsonArray("_careTeamLinkId"); 9183 for (int i = 0; i < array.size(); i++) { 9184 if (i == res.getCareTeamLinkId().size()) 9185 res.getCareTeamLinkId().add(parsePositiveInt(null)); 9186 if (array.get(i) instanceof JsonObject) 9187 parseElementProperties(array.get(i).getAsJsonObject(), res.getCareTeamLinkId().get(i)); 9188 } 9189 }; 9190 if (json.has("diagnosisLinkId")) { 9191 JsonArray array = json.getAsJsonArray("diagnosisLinkId"); 9192 for (int i = 0; i < array.size(); i++) { 9193 if (array.get(i).isJsonNull()) { 9194 res.getDiagnosisLinkId().add(new PositiveIntType()); 9195 } else { 9196 res.getDiagnosisLinkId().add(parsePositiveInt(array.get(i).getAsString())); 9197 } 9198 } 9199 }; 9200 if (json.has("_diagnosisLinkId")) { 9201 JsonArray array = json.getAsJsonArray("_diagnosisLinkId"); 9202 for (int i = 0; i < array.size(); i++) { 9203 if (i == res.getDiagnosisLinkId().size()) 9204 res.getDiagnosisLinkId().add(parsePositiveInt(null)); 9205 if (array.get(i) instanceof JsonObject) 9206 parseElementProperties(array.get(i).getAsJsonObject(), res.getDiagnosisLinkId().get(i)); 9207 } 9208 }; 9209 if (json.has("procedureLinkId")) { 9210 JsonArray array = json.getAsJsonArray("procedureLinkId"); 9211 for (int i = 0; i < array.size(); i++) { 9212 if (array.get(i).isJsonNull()) { 9213 res.getProcedureLinkId().add(new PositiveIntType()); 9214 } else { 9215 res.getProcedureLinkId().add(parsePositiveInt(array.get(i).getAsString())); 9216 } 9217 } 9218 }; 9219 if (json.has("_procedureLinkId")) { 9220 JsonArray array = json.getAsJsonArray("_procedureLinkId"); 9221 for (int i = 0; i < array.size(); i++) { 9222 if (i == res.getProcedureLinkId().size()) 9223 res.getProcedureLinkId().add(parsePositiveInt(null)); 9224 if (array.get(i) instanceof JsonObject) 9225 parseElementProperties(array.get(i).getAsJsonObject(), res.getProcedureLinkId().get(i)); 9226 } 9227 }; 9228 if (json.has("informationLinkId")) { 9229 JsonArray array = json.getAsJsonArray("informationLinkId"); 9230 for (int i = 0; i < array.size(); i++) { 9231 if (array.get(i).isJsonNull()) { 9232 res.getInformationLinkId().add(new PositiveIntType()); 9233 } else { 9234 res.getInformationLinkId().add(parsePositiveInt(array.get(i).getAsString())); 9235 } 9236 } 9237 }; 9238 if (json.has("_informationLinkId")) { 9239 JsonArray array = json.getAsJsonArray("_informationLinkId"); 9240 for (int i = 0; i < array.size(); i++) { 9241 if (i == res.getInformationLinkId().size()) 9242 res.getInformationLinkId().add(parsePositiveInt(null)); 9243 if (array.get(i) instanceof JsonObject) 9244 parseElementProperties(array.get(i).getAsJsonObject(), res.getInformationLinkId().get(i)); 9245 } 9246 }; 9247 if (json.has("revenue")) 9248 res.setRevenue(parseCodeableConcept(getJObject(json, "revenue"))); 9249 if (json.has("category")) 9250 res.setCategory(parseCodeableConcept(getJObject(json, "category"))); 9251 if (json.has("service")) 9252 res.setService(parseCodeableConcept(getJObject(json, "service"))); 9253 if (json.has("modifier")) { 9254 JsonArray array = json.getAsJsonArray("modifier"); 9255 for (int i = 0; i < array.size(); i++) { 9256 res.getModifier().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 9257 } 9258 }; 9259 if (json.has("programCode")) { 9260 JsonArray array = json.getAsJsonArray("programCode"); 9261 for (int i = 0; i < array.size(); i++) { 9262 res.getProgramCode().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 9263 } 9264 }; 9265 Type serviced = parseType("serviced", json); 9266 if (serviced != null) 9267 res.setServiced(serviced); 9268 Type location = parseType("location", json); 9269 if (location != null) 9270 res.setLocation(location); 9271 if (json.has("quantity")) 9272 res.setQuantity(parseSimpleQuantity(getJObject(json, "quantity"))); 9273 if (json.has("unitPrice")) 9274 res.setUnitPrice(parseMoney(getJObject(json, "unitPrice"))); 9275 if (json.has("factor")) 9276 res.setFactorElement(parseDecimal(json.get("factor").getAsBigDecimal())); 9277 if (json.has("_factor")) 9278 parseElementProperties(getJObject(json, "_factor"), res.getFactorElement()); 9279 if (json.has("net")) 9280 res.setNet(parseMoney(getJObject(json, "net"))); 9281 if (json.has("udi")) { 9282 JsonArray array = json.getAsJsonArray("udi"); 9283 for (int i = 0; i < array.size(); i++) { 9284 res.getUdi().add(parseReference(array.get(i).getAsJsonObject())); 9285 } 9286 }; 9287 if (json.has("bodySite")) 9288 res.setBodySite(parseCodeableConcept(getJObject(json, "bodySite"))); 9289 if (json.has("subSite")) { 9290 JsonArray array = json.getAsJsonArray("subSite"); 9291 for (int i = 0; i < array.size(); i++) { 9292 res.getSubSite().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 9293 } 9294 }; 9295 if (json.has("encounter")) { 9296 JsonArray array = json.getAsJsonArray("encounter"); 9297 for (int i = 0; i < array.size(); i++) { 9298 res.getEncounter().add(parseReference(array.get(i).getAsJsonObject())); 9299 } 9300 }; 9301 if (json.has("noteNumber")) { 9302 JsonArray array = json.getAsJsonArray("noteNumber"); 9303 for (int i = 0; i < array.size(); i++) { 9304 if (array.get(i).isJsonNull()) { 9305 res.getNoteNumber().add(new PositiveIntType()); 9306 } else { 9307 res.getNoteNumber().add(parsePositiveInt(array.get(i).getAsString())); 9308 } 9309 } 9310 }; 9311 if (json.has("_noteNumber")) { 9312 JsonArray array = json.getAsJsonArray("_noteNumber"); 9313 for (int i = 0; i < array.size(); i++) { 9314 if (i == res.getNoteNumber().size()) 9315 res.getNoteNumber().add(parsePositiveInt(null)); 9316 if (array.get(i) instanceof JsonObject) 9317 parseElementProperties(array.get(i).getAsJsonObject(), res.getNoteNumber().get(i)); 9318 } 9319 }; 9320 if (json.has("adjudication")) { 9321 JsonArray array = json.getAsJsonArray("adjudication"); 9322 for (int i = 0; i < array.size(); i++) { 9323 res.getAdjudication().add(parseExplanationOfBenefitAdjudicationComponent(array.get(i).getAsJsonObject(), owner)); 9324 } 9325 }; 9326 if (json.has("detail")) { 9327 JsonArray array = json.getAsJsonArray("detail"); 9328 for (int i = 0; i < array.size(); i++) { 9329 res.getDetail().add(parseExplanationOfBenefitDetailComponent(array.get(i).getAsJsonObject(), owner)); 9330 } 9331 }; 9332 } 9333 9334 protected ExplanationOfBenefit.AdjudicationComponent parseExplanationOfBenefitAdjudicationComponent(JsonObject json, ExplanationOfBenefit owner) throws IOException, FHIRFormatError { 9335 ExplanationOfBenefit.AdjudicationComponent res = new ExplanationOfBenefit.AdjudicationComponent(); 9336 parseExplanationOfBenefitAdjudicationComponentProperties(json, owner, res); 9337 return res; 9338 } 9339 9340 protected void parseExplanationOfBenefitAdjudicationComponentProperties(JsonObject json, ExplanationOfBenefit owner, ExplanationOfBenefit.AdjudicationComponent res) throws IOException, FHIRFormatError { 9341 parseBackboneProperties(json, res); 9342 if (json.has("category")) 9343 res.setCategory(parseCodeableConcept(getJObject(json, "category"))); 9344 if (json.has("reason")) 9345 res.setReason(parseCodeableConcept(getJObject(json, "reason"))); 9346 if (json.has("amount")) 9347 res.setAmount(parseMoney(getJObject(json, "amount"))); 9348 if (json.has("value")) 9349 res.setValueElement(parseDecimal(json.get("value").getAsBigDecimal())); 9350 if (json.has("_value")) 9351 parseElementProperties(getJObject(json, "_value"), res.getValueElement()); 9352 } 9353 9354 protected ExplanationOfBenefit.DetailComponent parseExplanationOfBenefitDetailComponent(JsonObject json, ExplanationOfBenefit owner) throws IOException, FHIRFormatError { 9355 ExplanationOfBenefit.DetailComponent res = new ExplanationOfBenefit.DetailComponent(); 9356 parseExplanationOfBenefitDetailComponentProperties(json, owner, res); 9357 return res; 9358 } 9359 9360 protected void parseExplanationOfBenefitDetailComponentProperties(JsonObject json, ExplanationOfBenefit owner, ExplanationOfBenefit.DetailComponent res) throws IOException, FHIRFormatError { 9361 parseBackboneProperties(json, res); 9362 if (json.has("sequence")) 9363 res.setSequenceElement(parsePositiveInt(json.get("sequence").getAsString())); 9364 if (json.has("_sequence")) 9365 parseElementProperties(getJObject(json, "_sequence"), res.getSequenceElement()); 9366 if (json.has("type")) 9367 res.setType(parseCodeableConcept(getJObject(json, "type"))); 9368 if (json.has("revenue")) 9369 res.setRevenue(parseCodeableConcept(getJObject(json, "revenue"))); 9370 if (json.has("category")) 9371 res.setCategory(parseCodeableConcept(getJObject(json, "category"))); 9372 if (json.has("service")) 9373 res.setService(parseCodeableConcept(getJObject(json, "service"))); 9374 if (json.has("modifier")) { 9375 JsonArray array = json.getAsJsonArray("modifier"); 9376 for (int i = 0; i < array.size(); i++) { 9377 res.getModifier().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 9378 } 9379 }; 9380 if (json.has("programCode")) { 9381 JsonArray array = json.getAsJsonArray("programCode"); 9382 for (int i = 0; i < array.size(); i++) { 9383 res.getProgramCode().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 9384 } 9385 }; 9386 if (json.has("quantity")) 9387 res.setQuantity(parseSimpleQuantity(getJObject(json, "quantity"))); 9388 if (json.has("unitPrice")) 9389 res.setUnitPrice(parseMoney(getJObject(json, "unitPrice"))); 9390 if (json.has("factor")) 9391 res.setFactorElement(parseDecimal(json.get("factor").getAsBigDecimal())); 9392 if (json.has("_factor")) 9393 parseElementProperties(getJObject(json, "_factor"), res.getFactorElement()); 9394 if (json.has("net")) 9395 res.setNet(parseMoney(getJObject(json, "net"))); 9396 if (json.has("udi")) { 9397 JsonArray array = json.getAsJsonArray("udi"); 9398 for (int i = 0; i < array.size(); i++) { 9399 res.getUdi().add(parseReference(array.get(i).getAsJsonObject())); 9400 } 9401 }; 9402 if (json.has("noteNumber")) { 9403 JsonArray array = json.getAsJsonArray("noteNumber"); 9404 for (int i = 0; i < array.size(); i++) { 9405 if (array.get(i).isJsonNull()) { 9406 res.getNoteNumber().add(new PositiveIntType()); 9407 } else { 9408 res.getNoteNumber().add(parsePositiveInt(array.get(i).getAsString())); 9409 } 9410 } 9411 }; 9412 if (json.has("_noteNumber")) { 9413 JsonArray array = json.getAsJsonArray("_noteNumber"); 9414 for (int i = 0; i < array.size(); i++) { 9415 if (i == res.getNoteNumber().size()) 9416 res.getNoteNumber().add(parsePositiveInt(null)); 9417 if (array.get(i) instanceof JsonObject) 9418 parseElementProperties(array.get(i).getAsJsonObject(), res.getNoteNumber().get(i)); 9419 } 9420 }; 9421 if (json.has("adjudication")) { 9422 JsonArray array = json.getAsJsonArray("adjudication"); 9423 for (int i = 0; i < array.size(); i++) { 9424 res.getAdjudication().add(parseExplanationOfBenefitAdjudicationComponent(array.get(i).getAsJsonObject(), owner)); 9425 } 9426 }; 9427 if (json.has("subDetail")) { 9428 JsonArray array = json.getAsJsonArray("subDetail"); 9429 for (int i = 0; i < array.size(); i++) { 9430 res.getSubDetail().add(parseExplanationOfBenefitSubDetailComponent(array.get(i).getAsJsonObject(), owner)); 9431 } 9432 }; 9433 } 9434 9435 protected ExplanationOfBenefit.SubDetailComponent parseExplanationOfBenefitSubDetailComponent(JsonObject json, ExplanationOfBenefit owner) throws IOException, FHIRFormatError { 9436 ExplanationOfBenefit.SubDetailComponent res = new ExplanationOfBenefit.SubDetailComponent(); 9437 parseExplanationOfBenefitSubDetailComponentProperties(json, owner, res); 9438 return res; 9439 } 9440 9441 protected void parseExplanationOfBenefitSubDetailComponentProperties(JsonObject json, ExplanationOfBenefit owner, ExplanationOfBenefit.SubDetailComponent res) throws IOException, FHIRFormatError { 9442 parseBackboneProperties(json, res); 9443 if (json.has("sequence")) 9444 res.setSequenceElement(parsePositiveInt(json.get("sequence").getAsString())); 9445 if (json.has("_sequence")) 9446 parseElementProperties(getJObject(json, "_sequence"), res.getSequenceElement()); 9447 if (json.has("type")) 9448 res.setType(parseCodeableConcept(getJObject(json, "type"))); 9449 if (json.has("revenue")) 9450 res.setRevenue(parseCodeableConcept(getJObject(json, "revenue"))); 9451 if (json.has("category")) 9452 res.setCategory(parseCodeableConcept(getJObject(json, "category"))); 9453 if (json.has("service")) 9454 res.setService(parseCodeableConcept(getJObject(json, "service"))); 9455 if (json.has("modifier")) { 9456 JsonArray array = json.getAsJsonArray("modifier"); 9457 for (int i = 0; i < array.size(); i++) { 9458 res.getModifier().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 9459 } 9460 }; 9461 if (json.has("programCode")) { 9462 JsonArray array = json.getAsJsonArray("programCode"); 9463 for (int i = 0; i < array.size(); i++) { 9464 res.getProgramCode().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 9465 } 9466 }; 9467 if (json.has("quantity")) 9468 res.setQuantity(parseSimpleQuantity(getJObject(json, "quantity"))); 9469 if (json.has("unitPrice")) 9470 res.setUnitPrice(parseMoney(getJObject(json, "unitPrice"))); 9471 if (json.has("factor")) 9472 res.setFactorElement(parseDecimal(json.get("factor").getAsBigDecimal())); 9473 if (json.has("_factor")) 9474 parseElementProperties(getJObject(json, "_factor"), res.getFactorElement()); 9475 if (json.has("net")) 9476 res.setNet(parseMoney(getJObject(json, "net"))); 9477 if (json.has("udi")) { 9478 JsonArray array = json.getAsJsonArray("udi"); 9479 for (int i = 0; i < array.size(); i++) { 9480 res.getUdi().add(parseReference(array.get(i).getAsJsonObject())); 9481 } 9482 }; 9483 if (json.has("noteNumber")) { 9484 JsonArray array = json.getAsJsonArray("noteNumber"); 9485 for (int i = 0; i < array.size(); i++) { 9486 if (array.get(i).isJsonNull()) { 9487 res.getNoteNumber().add(new PositiveIntType()); 9488 } else { 9489 res.getNoteNumber().add(parsePositiveInt(array.get(i).getAsString())); 9490 } 9491 } 9492 }; 9493 if (json.has("_noteNumber")) { 9494 JsonArray array = json.getAsJsonArray("_noteNumber"); 9495 for (int i = 0; i < array.size(); i++) { 9496 if (i == res.getNoteNumber().size()) 9497 res.getNoteNumber().add(parsePositiveInt(null)); 9498 if (array.get(i) instanceof JsonObject) 9499 parseElementProperties(array.get(i).getAsJsonObject(), res.getNoteNumber().get(i)); 9500 } 9501 }; 9502 if (json.has("adjudication")) { 9503 JsonArray array = json.getAsJsonArray("adjudication"); 9504 for (int i = 0; i < array.size(); i++) { 9505 res.getAdjudication().add(parseExplanationOfBenefitAdjudicationComponent(array.get(i).getAsJsonObject(), owner)); 9506 } 9507 }; 9508 } 9509 9510 protected ExplanationOfBenefit.AddedItemComponent parseExplanationOfBenefitAddedItemComponent(JsonObject json, ExplanationOfBenefit owner) throws IOException, FHIRFormatError { 9511 ExplanationOfBenefit.AddedItemComponent res = new ExplanationOfBenefit.AddedItemComponent(); 9512 parseExplanationOfBenefitAddedItemComponentProperties(json, owner, res); 9513 return res; 9514 } 9515 9516 protected void parseExplanationOfBenefitAddedItemComponentProperties(JsonObject json, ExplanationOfBenefit owner, ExplanationOfBenefit.AddedItemComponent res) throws IOException, FHIRFormatError { 9517 parseBackboneProperties(json, res); 9518 if (json.has("sequenceLinkId")) { 9519 JsonArray array = json.getAsJsonArray("sequenceLinkId"); 9520 for (int i = 0; i < array.size(); i++) { 9521 if (array.get(i).isJsonNull()) { 9522 res.getSequenceLinkId().add(new PositiveIntType()); 9523 } else { 9524 res.getSequenceLinkId().add(parsePositiveInt(array.get(i).getAsString())); 9525 } 9526 } 9527 }; 9528 if (json.has("_sequenceLinkId")) { 9529 JsonArray array = json.getAsJsonArray("_sequenceLinkId"); 9530 for (int i = 0; i < array.size(); i++) { 9531 if (i == res.getSequenceLinkId().size()) 9532 res.getSequenceLinkId().add(parsePositiveInt(null)); 9533 if (array.get(i) instanceof JsonObject) 9534 parseElementProperties(array.get(i).getAsJsonObject(), res.getSequenceLinkId().get(i)); 9535 } 9536 }; 9537 if (json.has("revenue")) 9538 res.setRevenue(parseCodeableConcept(getJObject(json, "revenue"))); 9539 if (json.has("category")) 9540 res.setCategory(parseCodeableConcept(getJObject(json, "category"))); 9541 if (json.has("service")) 9542 res.setService(parseCodeableConcept(getJObject(json, "service"))); 9543 if (json.has("modifier")) { 9544 JsonArray array = json.getAsJsonArray("modifier"); 9545 for (int i = 0; i < array.size(); i++) { 9546 res.getModifier().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 9547 } 9548 }; 9549 if (json.has("fee")) 9550 res.setFee(parseMoney(getJObject(json, "fee"))); 9551 if (json.has("noteNumber")) { 9552 JsonArray array = json.getAsJsonArray("noteNumber"); 9553 for (int i = 0; i < array.size(); i++) { 9554 if (array.get(i).isJsonNull()) { 9555 res.getNoteNumber().add(new PositiveIntType()); 9556 } else { 9557 res.getNoteNumber().add(parsePositiveInt(array.get(i).getAsString())); 9558 } 9559 } 9560 }; 9561 if (json.has("_noteNumber")) { 9562 JsonArray array = json.getAsJsonArray("_noteNumber"); 9563 for (int i = 0; i < array.size(); i++) { 9564 if (i == res.getNoteNumber().size()) 9565 res.getNoteNumber().add(parsePositiveInt(null)); 9566 if (array.get(i) instanceof JsonObject) 9567 parseElementProperties(array.get(i).getAsJsonObject(), res.getNoteNumber().get(i)); 9568 } 9569 }; 9570 if (json.has("adjudication")) { 9571 JsonArray array = json.getAsJsonArray("adjudication"); 9572 for (int i = 0; i < array.size(); i++) { 9573 res.getAdjudication().add(parseExplanationOfBenefitAdjudicationComponent(array.get(i).getAsJsonObject(), owner)); 9574 } 9575 }; 9576 if (json.has("detail")) { 9577 JsonArray array = json.getAsJsonArray("detail"); 9578 for (int i = 0; i < array.size(); i++) { 9579 res.getDetail().add(parseExplanationOfBenefitAddedItemsDetailComponent(array.get(i).getAsJsonObject(), owner)); 9580 } 9581 }; 9582 } 9583 9584 protected ExplanationOfBenefit.AddedItemsDetailComponent parseExplanationOfBenefitAddedItemsDetailComponent(JsonObject json, ExplanationOfBenefit owner) throws IOException, FHIRFormatError { 9585 ExplanationOfBenefit.AddedItemsDetailComponent res = new ExplanationOfBenefit.AddedItemsDetailComponent(); 9586 parseExplanationOfBenefitAddedItemsDetailComponentProperties(json, owner, res); 9587 return res; 9588 } 9589 9590 protected void parseExplanationOfBenefitAddedItemsDetailComponentProperties(JsonObject json, ExplanationOfBenefit owner, ExplanationOfBenefit.AddedItemsDetailComponent res) throws IOException, FHIRFormatError { 9591 parseBackboneProperties(json, res); 9592 if (json.has("revenue")) 9593 res.setRevenue(parseCodeableConcept(getJObject(json, "revenue"))); 9594 if (json.has("category")) 9595 res.setCategory(parseCodeableConcept(getJObject(json, "category"))); 9596 if (json.has("service")) 9597 res.setService(parseCodeableConcept(getJObject(json, "service"))); 9598 if (json.has("modifier")) { 9599 JsonArray array = json.getAsJsonArray("modifier"); 9600 for (int i = 0; i < array.size(); i++) { 9601 res.getModifier().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 9602 } 9603 }; 9604 if (json.has("fee")) 9605 res.setFee(parseMoney(getJObject(json, "fee"))); 9606 if (json.has("noteNumber")) { 9607 JsonArray array = json.getAsJsonArray("noteNumber"); 9608 for (int i = 0; i < array.size(); i++) { 9609 if (array.get(i).isJsonNull()) { 9610 res.getNoteNumber().add(new PositiveIntType()); 9611 } else { 9612 res.getNoteNumber().add(parsePositiveInt(array.get(i).getAsString())); 9613 } 9614 } 9615 }; 9616 if (json.has("_noteNumber")) { 9617 JsonArray array = json.getAsJsonArray("_noteNumber"); 9618 for (int i = 0; i < array.size(); i++) { 9619 if (i == res.getNoteNumber().size()) 9620 res.getNoteNumber().add(parsePositiveInt(null)); 9621 if (array.get(i) instanceof JsonObject) 9622 parseElementProperties(array.get(i).getAsJsonObject(), res.getNoteNumber().get(i)); 9623 } 9624 }; 9625 if (json.has("adjudication")) { 9626 JsonArray array = json.getAsJsonArray("adjudication"); 9627 for (int i = 0; i < array.size(); i++) { 9628 res.getAdjudication().add(parseExplanationOfBenefitAdjudicationComponent(array.get(i).getAsJsonObject(), owner)); 9629 } 9630 }; 9631 } 9632 9633 protected ExplanationOfBenefit.PaymentComponent parseExplanationOfBenefitPaymentComponent(JsonObject json, ExplanationOfBenefit owner) throws IOException, FHIRFormatError { 9634 ExplanationOfBenefit.PaymentComponent res = new ExplanationOfBenefit.PaymentComponent(); 9635 parseExplanationOfBenefitPaymentComponentProperties(json, owner, res); 9636 return res; 9637 } 9638 9639 protected void parseExplanationOfBenefitPaymentComponentProperties(JsonObject json, ExplanationOfBenefit owner, ExplanationOfBenefit.PaymentComponent res) throws IOException, FHIRFormatError { 9640 parseBackboneProperties(json, res); 9641 if (json.has("type")) 9642 res.setType(parseCodeableConcept(getJObject(json, "type"))); 9643 if (json.has("adjustment")) 9644 res.setAdjustment(parseMoney(getJObject(json, "adjustment"))); 9645 if (json.has("adjustmentReason")) 9646 res.setAdjustmentReason(parseCodeableConcept(getJObject(json, "adjustmentReason"))); 9647 if (json.has("date")) 9648 res.setDateElement(parseDate(json.get("date").getAsString())); 9649 if (json.has("_date")) 9650 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 9651 if (json.has("amount")) 9652 res.setAmount(parseMoney(getJObject(json, "amount"))); 9653 if (json.has("identifier")) 9654 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 9655 } 9656 9657 protected ExplanationOfBenefit.NoteComponent parseExplanationOfBenefitNoteComponent(JsonObject json, ExplanationOfBenefit owner) throws IOException, FHIRFormatError { 9658 ExplanationOfBenefit.NoteComponent res = new ExplanationOfBenefit.NoteComponent(); 9659 parseExplanationOfBenefitNoteComponentProperties(json, owner, res); 9660 return res; 9661 } 9662 9663 protected void parseExplanationOfBenefitNoteComponentProperties(JsonObject json, ExplanationOfBenefit owner, ExplanationOfBenefit.NoteComponent res) throws IOException, FHIRFormatError { 9664 parseBackboneProperties(json, res); 9665 if (json.has("number")) 9666 res.setNumberElement(parsePositiveInt(json.get("number").getAsString())); 9667 if (json.has("_number")) 9668 parseElementProperties(getJObject(json, "_number"), res.getNumberElement()); 9669 if (json.has("type")) 9670 res.setType(parseCodeableConcept(getJObject(json, "type"))); 9671 if (json.has("text")) 9672 res.setTextElement(parseString(json.get("text").getAsString())); 9673 if (json.has("_text")) 9674 parseElementProperties(getJObject(json, "_text"), res.getTextElement()); 9675 if (json.has("language")) 9676 res.setLanguage(parseCodeableConcept(getJObject(json, "language"))); 9677 } 9678 9679 protected ExplanationOfBenefit.BenefitBalanceComponent parseExplanationOfBenefitBenefitBalanceComponent(JsonObject json, ExplanationOfBenefit owner) throws IOException, FHIRFormatError { 9680 ExplanationOfBenefit.BenefitBalanceComponent res = new ExplanationOfBenefit.BenefitBalanceComponent(); 9681 parseExplanationOfBenefitBenefitBalanceComponentProperties(json, owner, res); 9682 return res; 9683 } 9684 9685 protected void parseExplanationOfBenefitBenefitBalanceComponentProperties(JsonObject json, ExplanationOfBenefit owner, ExplanationOfBenefit.BenefitBalanceComponent res) throws IOException, FHIRFormatError { 9686 parseBackboneProperties(json, res); 9687 if (json.has("category")) 9688 res.setCategory(parseCodeableConcept(getJObject(json, "category"))); 9689 if (json.has("subCategory")) 9690 res.setSubCategory(parseCodeableConcept(getJObject(json, "subCategory"))); 9691 if (json.has("excluded")) 9692 res.setExcludedElement(parseBoolean(json.get("excluded").getAsBoolean())); 9693 if (json.has("_excluded")) 9694 parseElementProperties(getJObject(json, "_excluded"), res.getExcludedElement()); 9695 if (json.has("name")) 9696 res.setNameElement(parseString(json.get("name").getAsString())); 9697 if (json.has("_name")) 9698 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 9699 if (json.has("description")) 9700 res.setDescriptionElement(parseString(json.get("description").getAsString())); 9701 if (json.has("_description")) 9702 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 9703 if (json.has("network")) 9704 res.setNetwork(parseCodeableConcept(getJObject(json, "network"))); 9705 if (json.has("unit")) 9706 res.setUnit(parseCodeableConcept(getJObject(json, "unit"))); 9707 if (json.has("term")) 9708 res.setTerm(parseCodeableConcept(getJObject(json, "term"))); 9709 if (json.has("financial")) { 9710 JsonArray array = json.getAsJsonArray("financial"); 9711 for (int i = 0; i < array.size(); i++) { 9712 res.getFinancial().add(parseExplanationOfBenefitBenefitComponent(array.get(i).getAsJsonObject(), owner)); 9713 } 9714 }; 9715 } 9716 9717 protected ExplanationOfBenefit.BenefitComponent parseExplanationOfBenefitBenefitComponent(JsonObject json, ExplanationOfBenefit owner) throws IOException, FHIRFormatError { 9718 ExplanationOfBenefit.BenefitComponent res = new ExplanationOfBenefit.BenefitComponent(); 9719 parseExplanationOfBenefitBenefitComponentProperties(json, owner, res); 9720 return res; 9721 } 9722 9723 protected void parseExplanationOfBenefitBenefitComponentProperties(JsonObject json, ExplanationOfBenefit owner, ExplanationOfBenefit.BenefitComponent res) throws IOException, FHIRFormatError { 9724 parseBackboneProperties(json, res); 9725 if (json.has("type")) 9726 res.setType(parseCodeableConcept(getJObject(json, "type"))); 9727 Type allowed = parseType("allowed", json); 9728 if (allowed != null) 9729 res.setAllowed(allowed); 9730 Type used = parseType("used", json); 9731 if (used != null) 9732 res.setUsed(used); 9733 } 9734 9735 protected FamilyMemberHistory parseFamilyMemberHistory(JsonObject json) throws IOException, FHIRFormatError { 9736 FamilyMemberHistory res = new FamilyMemberHistory(); 9737 parseFamilyMemberHistoryProperties(json, res); 9738 return res; 9739 } 9740 9741 protected void parseFamilyMemberHistoryProperties(JsonObject json, FamilyMemberHistory res) throws IOException, FHIRFormatError { 9742 parseDomainResourceProperties(json, res); 9743 if (json.has("identifier")) { 9744 JsonArray array = json.getAsJsonArray("identifier"); 9745 for (int i = 0; i < array.size(); i++) { 9746 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 9747 } 9748 }; 9749 if (json.has("definition")) { 9750 JsonArray array = json.getAsJsonArray("definition"); 9751 for (int i = 0; i < array.size(); i++) { 9752 res.getDefinition().add(parseReference(array.get(i).getAsJsonObject())); 9753 } 9754 }; 9755 if (json.has("status")) 9756 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), FamilyMemberHistory.FamilyHistoryStatus.NULL, new FamilyMemberHistory.FamilyHistoryStatusEnumFactory())); 9757 if (json.has("_status")) 9758 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 9759 if (json.has("notDone")) 9760 res.setNotDoneElement(parseBoolean(json.get("notDone").getAsBoolean())); 9761 if (json.has("_notDone")) 9762 parseElementProperties(getJObject(json, "_notDone"), res.getNotDoneElement()); 9763 if (json.has("notDoneReason")) 9764 res.setNotDoneReason(parseCodeableConcept(getJObject(json, "notDoneReason"))); 9765 if (json.has("patient")) 9766 res.setPatient(parseReference(getJObject(json, "patient"))); 9767 if (json.has("date")) 9768 res.setDateElement(parseDateTime(json.get("date").getAsString())); 9769 if (json.has("_date")) 9770 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 9771 if (json.has("name")) 9772 res.setNameElement(parseString(json.get("name").getAsString())); 9773 if (json.has("_name")) 9774 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 9775 if (json.has("relationship")) 9776 res.setRelationship(parseCodeableConcept(getJObject(json, "relationship"))); 9777 if (json.has("gender")) 9778 res.setGenderElement(parseEnumeration(json.get("gender").getAsString(), Enumerations.AdministrativeGender.NULL, new Enumerations.AdministrativeGenderEnumFactory())); 9779 if (json.has("_gender")) 9780 parseElementProperties(getJObject(json, "_gender"), res.getGenderElement()); 9781 Type born = parseType("born", json); 9782 if (born != null) 9783 res.setBorn(born); 9784 Type age = parseType("age", json); 9785 if (age != null) 9786 res.setAge(age); 9787 if (json.has("estimatedAge")) 9788 res.setEstimatedAgeElement(parseBoolean(json.get("estimatedAge").getAsBoolean())); 9789 if (json.has("_estimatedAge")) 9790 parseElementProperties(getJObject(json, "_estimatedAge"), res.getEstimatedAgeElement()); 9791 Type deceased = parseType("deceased", json); 9792 if (deceased != null) 9793 res.setDeceased(deceased); 9794 if (json.has("reasonCode")) { 9795 JsonArray array = json.getAsJsonArray("reasonCode"); 9796 for (int i = 0; i < array.size(); i++) { 9797 res.getReasonCode().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 9798 } 9799 }; 9800 if (json.has("reasonReference")) { 9801 JsonArray array = json.getAsJsonArray("reasonReference"); 9802 for (int i = 0; i < array.size(); i++) { 9803 res.getReasonReference().add(parseReference(array.get(i).getAsJsonObject())); 9804 } 9805 }; 9806 if (json.has("note")) { 9807 JsonArray array = json.getAsJsonArray("note"); 9808 for (int i = 0; i < array.size(); i++) { 9809 res.getNote().add(parseAnnotation(array.get(i).getAsJsonObject())); 9810 } 9811 }; 9812 if (json.has("condition")) { 9813 JsonArray array = json.getAsJsonArray("condition"); 9814 for (int i = 0; i < array.size(); i++) { 9815 res.getCondition().add(parseFamilyMemberHistoryFamilyMemberHistoryConditionComponent(array.get(i).getAsJsonObject(), res)); 9816 } 9817 }; 9818 } 9819 9820 protected FamilyMemberHistory.FamilyMemberHistoryConditionComponent parseFamilyMemberHistoryFamilyMemberHistoryConditionComponent(JsonObject json, FamilyMemberHistory owner) throws IOException, FHIRFormatError { 9821 FamilyMemberHistory.FamilyMemberHistoryConditionComponent res = new FamilyMemberHistory.FamilyMemberHistoryConditionComponent(); 9822 parseFamilyMemberHistoryFamilyMemberHistoryConditionComponentProperties(json, owner, res); 9823 return res; 9824 } 9825 9826 protected void parseFamilyMemberHistoryFamilyMemberHistoryConditionComponentProperties(JsonObject json, FamilyMemberHistory owner, FamilyMemberHistory.FamilyMemberHistoryConditionComponent res) throws IOException, FHIRFormatError { 9827 parseBackboneProperties(json, res); 9828 if (json.has("code")) 9829 res.setCode(parseCodeableConcept(getJObject(json, "code"))); 9830 if (json.has("outcome")) 9831 res.setOutcome(parseCodeableConcept(getJObject(json, "outcome"))); 9832 Type onset = parseType("onset", json); 9833 if (onset != null) 9834 res.setOnset(onset); 9835 if (json.has("note")) { 9836 JsonArray array = json.getAsJsonArray("note"); 9837 for (int i = 0; i < array.size(); i++) { 9838 res.getNote().add(parseAnnotation(array.get(i).getAsJsonObject())); 9839 } 9840 }; 9841 } 9842 9843 protected Flag parseFlag(JsonObject json) throws IOException, FHIRFormatError { 9844 Flag res = new Flag(); 9845 parseFlagProperties(json, res); 9846 return res; 9847 } 9848 9849 protected void parseFlagProperties(JsonObject json, Flag res) throws IOException, FHIRFormatError { 9850 parseDomainResourceProperties(json, res); 9851 if (json.has("identifier")) { 9852 JsonArray array = json.getAsJsonArray("identifier"); 9853 for (int i = 0; i < array.size(); i++) { 9854 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 9855 } 9856 }; 9857 if (json.has("status")) 9858 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Flag.FlagStatus.NULL, new Flag.FlagStatusEnumFactory())); 9859 if (json.has("_status")) 9860 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 9861 if (json.has("category")) 9862 res.setCategory(parseCodeableConcept(getJObject(json, "category"))); 9863 if (json.has("code")) 9864 res.setCode(parseCodeableConcept(getJObject(json, "code"))); 9865 if (json.has("subject")) 9866 res.setSubject(parseReference(getJObject(json, "subject"))); 9867 if (json.has("period")) 9868 res.setPeriod(parsePeriod(getJObject(json, "period"))); 9869 if (json.has("encounter")) 9870 res.setEncounter(parseReference(getJObject(json, "encounter"))); 9871 if (json.has("author")) 9872 res.setAuthor(parseReference(getJObject(json, "author"))); 9873 } 9874 9875 protected Goal parseGoal(JsonObject json) throws IOException, FHIRFormatError { 9876 Goal res = new Goal(); 9877 parseGoalProperties(json, res); 9878 return res; 9879 } 9880 9881 protected void parseGoalProperties(JsonObject json, Goal res) throws IOException, FHIRFormatError { 9882 parseDomainResourceProperties(json, res); 9883 if (json.has("identifier")) { 9884 JsonArray array = json.getAsJsonArray("identifier"); 9885 for (int i = 0; i < array.size(); i++) { 9886 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 9887 } 9888 }; 9889 if (json.has("status")) 9890 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Goal.GoalStatus.NULL, new Goal.GoalStatusEnumFactory())); 9891 if (json.has("_status")) 9892 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 9893 if (json.has("category")) { 9894 JsonArray array = json.getAsJsonArray("category"); 9895 for (int i = 0; i < array.size(); i++) { 9896 res.getCategory().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 9897 } 9898 }; 9899 if (json.has("priority")) 9900 res.setPriority(parseCodeableConcept(getJObject(json, "priority"))); 9901 if (json.has("description")) 9902 res.setDescription(parseCodeableConcept(getJObject(json, "description"))); 9903 if (json.has("subject")) 9904 res.setSubject(parseReference(getJObject(json, "subject"))); 9905 Type start = parseType("start", json); 9906 if (start != null) 9907 res.setStart(start); 9908 if (json.has("target")) 9909 res.setTarget(parseGoalGoalTargetComponent(getJObject(json, "target"), res)); 9910 if (json.has("statusDate")) 9911 res.setStatusDateElement(parseDate(json.get("statusDate").getAsString())); 9912 if (json.has("_statusDate")) 9913 parseElementProperties(getJObject(json, "_statusDate"), res.getStatusDateElement()); 9914 if (json.has("statusReason")) 9915 res.setStatusReasonElement(parseString(json.get("statusReason").getAsString())); 9916 if (json.has("_statusReason")) 9917 parseElementProperties(getJObject(json, "_statusReason"), res.getStatusReasonElement()); 9918 if (json.has("expressedBy")) 9919 res.setExpressedBy(parseReference(getJObject(json, "expressedBy"))); 9920 if (json.has("addresses")) { 9921 JsonArray array = json.getAsJsonArray("addresses"); 9922 for (int i = 0; i < array.size(); i++) { 9923 res.getAddresses().add(parseReference(array.get(i).getAsJsonObject())); 9924 } 9925 }; 9926 if (json.has("note")) { 9927 JsonArray array = json.getAsJsonArray("note"); 9928 for (int i = 0; i < array.size(); i++) { 9929 res.getNote().add(parseAnnotation(array.get(i).getAsJsonObject())); 9930 } 9931 }; 9932 if (json.has("outcomeCode")) { 9933 JsonArray array = json.getAsJsonArray("outcomeCode"); 9934 for (int i = 0; i < array.size(); i++) { 9935 res.getOutcomeCode().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 9936 } 9937 }; 9938 if (json.has("outcomeReference")) { 9939 JsonArray array = json.getAsJsonArray("outcomeReference"); 9940 for (int i = 0; i < array.size(); i++) { 9941 res.getOutcomeReference().add(parseReference(array.get(i).getAsJsonObject())); 9942 } 9943 }; 9944 } 9945 9946 protected Goal.GoalTargetComponent parseGoalGoalTargetComponent(JsonObject json, Goal owner) throws IOException, FHIRFormatError { 9947 Goal.GoalTargetComponent res = new Goal.GoalTargetComponent(); 9948 parseGoalGoalTargetComponentProperties(json, owner, res); 9949 return res; 9950 } 9951 9952 protected void parseGoalGoalTargetComponentProperties(JsonObject json, Goal owner, Goal.GoalTargetComponent res) throws IOException, FHIRFormatError { 9953 parseBackboneProperties(json, res); 9954 if (json.has("measure")) 9955 res.setMeasure(parseCodeableConcept(getJObject(json, "measure"))); 9956 Type detail = parseType("detail", json); 9957 if (detail != null) 9958 res.setDetail(detail); 9959 Type due = parseType("due", json); 9960 if (due != null) 9961 res.setDue(due); 9962 } 9963 9964 protected GraphDefinition parseGraphDefinition(JsonObject json) throws IOException, FHIRFormatError { 9965 GraphDefinition res = new GraphDefinition(); 9966 parseGraphDefinitionProperties(json, res); 9967 return res; 9968 } 9969 9970 protected void parseGraphDefinitionProperties(JsonObject json, GraphDefinition res) throws IOException, FHIRFormatError { 9971 parseDomainResourceProperties(json, res); 9972 if (json.has("url")) 9973 res.setUrlElement(parseUri(json.get("url").getAsString())); 9974 if (json.has("_url")) 9975 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 9976 if (json.has("version")) 9977 res.setVersionElement(parseString(json.get("version").getAsString())); 9978 if (json.has("_version")) 9979 parseElementProperties(getJObject(json, "_version"), res.getVersionElement()); 9980 if (json.has("name")) 9981 res.setNameElement(parseString(json.get("name").getAsString())); 9982 if (json.has("_name")) 9983 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 9984 if (json.has("status")) 9985 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Enumerations.PublicationStatus.NULL, new Enumerations.PublicationStatusEnumFactory())); 9986 if (json.has("_status")) 9987 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 9988 if (json.has("experimental")) 9989 res.setExperimentalElement(parseBoolean(json.get("experimental").getAsBoolean())); 9990 if (json.has("_experimental")) 9991 parseElementProperties(getJObject(json, "_experimental"), res.getExperimentalElement()); 9992 if (json.has("date")) 9993 res.setDateElement(parseDateTime(json.get("date").getAsString())); 9994 if (json.has("_date")) 9995 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 9996 if (json.has("publisher")) 9997 res.setPublisherElement(parseString(json.get("publisher").getAsString())); 9998 if (json.has("_publisher")) 9999 parseElementProperties(getJObject(json, "_publisher"), res.getPublisherElement()); 10000 if (json.has("contact")) { 10001 JsonArray array = json.getAsJsonArray("contact"); 10002 for (int i = 0; i < array.size(); i++) { 10003 res.getContact().add(parseContactDetail(array.get(i).getAsJsonObject())); 10004 } 10005 }; 10006 if (json.has("description")) 10007 res.setDescriptionElement(parseMarkdown(json.get("description").getAsString())); 10008 if (json.has("_description")) 10009 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 10010 if (json.has("useContext")) { 10011 JsonArray array = json.getAsJsonArray("useContext"); 10012 for (int i = 0; i < array.size(); i++) { 10013 res.getUseContext().add(parseUsageContext(array.get(i).getAsJsonObject())); 10014 } 10015 }; 10016 if (json.has("jurisdiction")) { 10017 JsonArray array = json.getAsJsonArray("jurisdiction"); 10018 for (int i = 0; i < array.size(); i++) { 10019 res.getJurisdiction().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 10020 } 10021 }; 10022 if (json.has("purpose")) 10023 res.setPurposeElement(parseMarkdown(json.get("purpose").getAsString())); 10024 if (json.has("_purpose")) 10025 parseElementProperties(getJObject(json, "_purpose"), res.getPurposeElement()); 10026 if (json.has("start")) 10027 res.setStartElement(parseCode(json.get("start").getAsString())); 10028 if (json.has("_start")) 10029 parseElementProperties(getJObject(json, "_start"), res.getStartElement()); 10030 if (json.has("profile")) 10031 res.setProfileElement(parseUri(json.get("profile").getAsString())); 10032 if (json.has("_profile")) 10033 parseElementProperties(getJObject(json, "_profile"), res.getProfileElement()); 10034 if (json.has("link")) { 10035 JsonArray array = json.getAsJsonArray("link"); 10036 for (int i = 0; i < array.size(); i++) { 10037 res.getLink().add(parseGraphDefinitionGraphDefinitionLinkComponent(array.get(i).getAsJsonObject(), res)); 10038 } 10039 }; 10040 } 10041 10042 protected GraphDefinition.GraphDefinitionLinkComponent parseGraphDefinitionGraphDefinitionLinkComponent(JsonObject json, GraphDefinition owner) throws IOException, FHIRFormatError { 10043 GraphDefinition.GraphDefinitionLinkComponent res = new GraphDefinition.GraphDefinitionLinkComponent(); 10044 parseGraphDefinitionGraphDefinitionLinkComponentProperties(json, owner, res); 10045 return res; 10046 } 10047 10048 protected void parseGraphDefinitionGraphDefinitionLinkComponentProperties(JsonObject json, GraphDefinition owner, GraphDefinition.GraphDefinitionLinkComponent res) throws IOException, FHIRFormatError { 10049 parseBackboneProperties(json, res); 10050 if (json.has("path")) 10051 res.setPathElement(parseString(json.get("path").getAsString())); 10052 if (json.has("_path")) 10053 parseElementProperties(getJObject(json, "_path"), res.getPathElement()); 10054 if (json.has("sliceName")) 10055 res.setSliceNameElement(parseString(json.get("sliceName").getAsString())); 10056 if (json.has("_sliceName")) 10057 parseElementProperties(getJObject(json, "_sliceName"), res.getSliceNameElement()); 10058 if (json.has("min")) 10059 res.setMinElement(parseInteger(json.get("min").getAsLong())); 10060 if (json.has("_min")) 10061 parseElementProperties(getJObject(json, "_min"), res.getMinElement()); 10062 if (json.has("max")) 10063 res.setMaxElement(parseString(json.get("max").getAsString())); 10064 if (json.has("_max")) 10065 parseElementProperties(getJObject(json, "_max"), res.getMaxElement()); 10066 if (json.has("description")) 10067 res.setDescriptionElement(parseString(json.get("description").getAsString())); 10068 if (json.has("_description")) 10069 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 10070 if (json.has("target")) { 10071 JsonArray array = json.getAsJsonArray("target"); 10072 for (int i = 0; i < array.size(); i++) { 10073 res.getTarget().add(parseGraphDefinitionGraphDefinitionLinkTargetComponent(array.get(i).getAsJsonObject(), owner)); 10074 } 10075 }; 10076 } 10077 10078 protected GraphDefinition.GraphDefinitionLinkTargetComponent parseGraphDefinitionGraphDefinitionLinkTargetComponent(JsonObject json, GraphDefinition owner) throws IOException, FHIRFormatError { 10079 GraphDefinition.GraphDefinitionLinkTargetComponent res = new GraphDefinition.GraphDefinitionLinkTargetComponent(); 10080 parseGraphDefinitionGraphDefinitionLinkTargetComponentProperties(json, owner, res); 10081 return res; 10082 } 10083 10084 protected void parseGraphDefinitionGraphDefinitionLinkTargetComponentProperties(JsonObject json, GraphDefinition owner, GraphDefinition.GraphDefinitionLinkTargetComponent res) throws IOException, FHIRFormatError { 10085 parseBackboneProperties(json, res); 10086 if (json.has("type")) 10087 res.setTypeElement(parseCode(json.get("type").getAsString())); 10088 if (json.has("_type")) 10089 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 10090 if (json.has("profile")) 10091 res.setProfileElement(parseUri(json.get("profile").getAsString())); 10092 if (json.has("_profile")) 10093 parseElementProperties(getJObject(json, "_profile"), res.getProfileElement()); 10094 if (json.has("compartment")) { 10095 JsonArray array = json.getAsJsonArray("compartment"); 10096 for (int i = 0; i < array.size(); i++) { 10097 res.getCompartment().add(parseGraphDefinitionGraphDefinitionLinkTargetCompartmentComponent(array.get(i).getAsJsonObject(), owner)); 10098 } 10099 }; 10100 if (json.has("link")) { 10101 JsonArray array = json.getAsJsonArray("link"); 10102 for (int i = 0; i < array.size(); i++) { 10103 res.getLink().add(parseGraphDefinitionGraphDefinitionLinkComponent(array.get(i).getAsJsonObject(), owner)); 10104 } 10105 }; 10106 } 10107 10108 protected GraphDefinition.GraphDefinitionLinkTargetCompartmentComponent parseGraphDefinitionGraphDefinitionLinkTargetCompartmentComponent(JsonObject json, GraphDefinition owner) throws IOException, FHIRFormatError { 10109 GraphDefinition.GraphDefinitionLinkTargetCompartmentComponent res = new GraphDefinition.GraphDefinitionLinkTargetCompartmentComponent(); 10110 parseGraphDefinitionGraphDefinitionLinkTargetCompartmentComponentProperties(json, owner, res); 10111 return res; 10112 } 10113 10114 protected void parseGraphDefinitionGraphDefinitionLinkTargetCompartmentComponentProperties(JsonObject json, GraphDefinition owner, GraphDefinition.GraphDefinitionLinkTargetCompartmentComponent res) throws IOException, FHIRFormatError { 10115 parseBackboneProperties(json, res); 10116 if (json.has("code")) 10117 res.setCodeElement(parseEnumeration(json.get("code").getAsString(), GraphDefinition.CompartmentCode.NULL, new GraphDefinition.CompartmentCodeEnumFactory())); 10118 if (json.has("_code")) 10119 parseElementProperties(getJObject(json, "_code"), res.getCodeElement()); 10120 if (json.has("rule")) 10121 res.setRuleElement(parseEnumeration(json.get("rule").getAsString(), GraphDefinition.GraphCompartmentRule.NULL, new GraphDefinition.GraphCompartmentRuleEnumFactory())); 10122 if (json.has("_rule")) 10123 parseElementProperties(getJObject(json, "_rule"), res.getRuleElement()); 10124 if (json.has("expression")) 10125 res.setExpressionElement(parseString(json.get("expression").getAsString())); 10126 if (json.has("_expression")) 10127 parseElementProperties(getJObject(json, "_expression"), res.getExpressionElement()); 10128 if (json.has("description")) 10129 res.setDescriptionElement(parseString(json.get("description").getAsString())); 10130 if (json.has("_description")) 10131 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 10132 } 10133 10134 protected Group parseGroup(JsonObject json) throws IOException, FHIRFormatError { 10135 Group res = new Group(); 10136 parseGroupProperties(json, res); 10137 return res; 10138 } 10139 10140 protected void parseGroupProperties(JsonObject json, Group res) throws IOException, FHIRFormatError { 10141 parseDomainResourceProperties(json, res); 10142 if (json.has("identifier")) { 10143 JsonArray array = json.getAsJsonArray("identifier"); 10144 for (int i = 0; i < array.size(); i++) { 10145 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 10146 } 10147 }; 10148 if (json.has("active")) 10149 res.setActiveElement(parseBoolean(json.get("active").getAsBoolean())); 10150 if (json.has("_active")) 10151 parseElementProperties(getJObject(json, "_active"), res.getActiveElement()); 10152 if (json.has("type")) 10153 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), Group.GroupType.NULL, new Group.GroupTypeEnumFactory())); 10154 if (json.has("_type")) 10155 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 10156 if (json.has("actual")) 10157 res.setActualElement(parseBoolean(json.get("actual").getAsBoolean())); 10158 if (json.has("_actual")) 10159 parseElementProperties(getJObject(json, "_actual"), res.getActualElement()); 10160 if (json.has("code")) 10161 res.setCode(parseCodeableConcept(getJObject(json, "code"))); 10162 if (json.has("name")) 10163 res.setNameElement(parseString(json.get("name").getAsString())); 10164 if (json.has("_name")) 10165 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 10166 if (json.has("quantity")) 10167 res.setQuantityElement(parseUnsignedInt(json.get("quantity").getAsString())); 10168 if (json.has("_quantity")) 10169 parseElementProperties(getJObject(json, "_quantity"), res.getQuantityElement()); 10170 if (json.has("characteristic")) { 10171 JsonArray array = json.getAsJsonArray("characteristic"); 10172 for (int i = 0; i < array.size(); i++) { 10173 res.getCharacteristic().add(parseGroupGroupCharacteristicComponent(array.get(i).getAsJsonObject(), res)); 10174 } 10175 }; 10176 if (json.has("member")) { 10177 JsonArray array = json.getAsJsonArray("member"); 10178 for (int i = 0; i < array.size(); i++) { 10179 res.getMember().add(parseGroupGroupMemberComponent(array.get(i).getAsJsonObject(), res)); 10180 } 10181 }; 10182 } 10183 10184 protected Group.GroupCharacteristicComponent parseGroupGroupCharacteristicComponent(JsonObject json, Group owner) throws IOException, FHIRFormatError { 10185 Group.GroupCharacteristicComponent res = new Group.GroupCharacteristicComponent(); 10186 parseGroupGroupCharacteristicComponentProperties(json, owner, res); 10187 return res; 10188 } 10189 10190 protected void parseGroupGroupCharacteristicComponentProperties(JsonObject json, Group owner, Group.GroupCharacteristicComponent res) throws IOException, FHIRFormatError { 10191 parseBackboneProperties(json, res); 10192 if (json.has("code")) 10193 res.setCode(parseCodeableConcept(getJObject(json, "code"))); 10194 Type value = parseType("value", json); 10195 if (value != null) 10196 res.setValue(value); 10197 if (json.has("exclude")) 10198 res.setExcludeElement(parseBoolean(json.get("exclude").getAsBoolean())); 10199 if (json.has("_exclude")) 10200 parseElementProperties(getJObject(json, "_exclude"), res.getExcludeElement()); 10201 if (json.has("period")) 10202 res.setPeriod(parsePeriod(getJObject(json, "period"))); 10203 } 10204 10205 protected Group.GroupMemberComponent parseGroupGroupMemberComponent(JsonObject json, Group owner) throws IOException, FHIRFormatError { 10206 Group.GroupMemberComponent res = new Group.GroupMemberComponent(); 10207 parseGroupGroupMemberComponentProperties(json, owner, res); 10208 return res; 10209 } 10210 10211 protected void parseGroupGroupMemberComponentProperties(JsonObject json, Group owner, Group.GroupMemberComponent res) throws IOException, FHIRFormatError { 10212 parseBackboneProperties(json, res); 10213 if (json.has("entity")) 10214 res.setEntity(parseReference(getJObject(json, "entity"))); 10215 if (json.has("period")) 10216 res.setPeriod(parsePeriod(getJObject(json, "period"))); 10217 if (json.has("inactive")) 10218 res.setInactiveElement(parseBoolean(json.get("inactive").getAsBoolean())); 10219 if (json.has("_inactive")) 10220 parseElementProperties(getJObject(json, "_inactive"), res.getInactiveElement()); 10221 } 10222 10223 protected GuidanceResponse parseGuidanceResponse(JsonObject json) throws IOException, FHIRFormatError { 10224 GuidanceResponse res = new GuidanceResponse(); 10225 parseGuidanceResponseProperties(json, res); 10226 return res; 10227 } 10228 10229 protected void parseGuidanceResponseProperties(JsonObject json, GuidanceResponse res) throws IOException, FHIRFormatError { 10230 parseDomainResourceProperties(json, res); 10231 if (json.has("requestId")) 10232 res.setRequestIdElement(parseId(json.get("requestId").getAsString())); 10233 if (json.has("_requestId")) 10234 parseElementProperties(getJObject(json, "_requestId"), res.getRequestIdElement()); 10235 if (json.has("identifier")) 10236 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 10237 if (json.has("module")) 10238 res.setModule(parseReference(getJObject(json, "module"))); 10239 if (json.has("status")) 10240 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), GuidanceResponse.GuidanceResponseStatus.NULL, new GuidanceResponse.GuidanceResponseStatusEnumFactory())); 10241 if (json.has("_status")) 10242 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 10243 if (json.has("subject")) 10244 res.setSubject(parseReference(getJObject(json, "subject"))); 10245 if (json.has("context")) 10246 res.setContext(parseReference(getJObject(json, "context"))); 10247 if (json.has("occurrenceDateTime")) 10248 res.setOccurrenceDateTimeElement(parseDateTime(json.get("occurrenceDateTime").getAsString())); 10249 if (json.has("_occurrenceDateTime")) 10250 parseElementProperties(getJObject(json, "_occurrenceDateTime"), res.getOccurrenceDateTimeElement()); 10251 if (json.has("performer")) 10252 res.setPerformer(parseReference(getJObject(json, "performer"))); 10253 Type reason = parseType("reason", json); 10254 if (reason != null) 10255 res.setReason(reason); 10256 if (json.has("note")) { 10257 JsonArray array = json.getAsJsonArray("note"); 10258 for (int i = 0; i < array.size(); i++) { 10259 res.getNote().add(parseAnnotation(array.get(i).getAsJsonObject())); 10260 } 10261 }; 10262 if (json.has("evaluationMessage")) { 10263 JsonArray array = json.getAsJsonArray("evaluationMessage"); 10264 for (int i = 0; i < array.size(); i++) { 10265 res.getEvaluationMessage().add(parseReference(array.get(i).getAsJsonObject())); 10266 } 10267 }; 10268 if (json.has("outputParameters")) 10269 res.setOutputParameters(parseReference(getJObject(json, "outputParameters"))); 10270 if (json.has("result")) 10271 res.setResult(parseReference(getJObject(json, "result"))); 10272 if (json.has("dataRequirement")) { 10273 JsonArray array = json.getAsJsonArray("dataRequirement"); 10274 for (int i = 0; i < array.size(); i++) { 10275 res.getDataRequirement().add(parseDataRequirement(array.get(i).getAsJsonObject())); 10276 } 10277 }; 10278 } 10279 10280 protected HealthcareService parseHealthcareService(JsonObject json) throws IOException, FHIRFormatError { 10281 HealthcareService res = new HealthcareService(); 10282 parseHealthcareServiceProperties(json, res); 10283 return res; 10284 } 10285 10286 protected void parseHealthcareServiceProperties(JsonObject json, HealthcareService res) throws IOException, FHIRFormatError { 10287 parseDomainResourceProperties(json, res); 10288 if (json.has("identifier")) { 10289 JsonArray array = json.getAsJsonArray("identifier"); 10290 for (int i = 0; i < array.size(); i++) { 10291 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 10292 } 10293 }; 10294 if (json.has("active")) 10295 res.setActiveElement(parseBoolean(json.get("active").getAsBoolean())); 10296 if (json.has("_active")) 10297 parseElementProperties(getJObject(json, "_active"), res.getActiveElement()); 10298 if (json.has("providedBy")) 10299 res.setProvidedBy(parseReference(getJObject(json, "providedBy"))); 10300 if (json.has("category")) 10301 res.setCategory(parseCodeableConcept(getJObject(json, "category"))); 10302 if (json.has("type")) { 10303 JsonArray array = json.getAsJsonArray("type"); 10304 for (int i = 0; i < array.size(); i++) { 10305 res.getType().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 10306 } 10307 }; 10308 if (json.has("specialty")) { 10309 JsonArray array = json.getAsJsonArray("specialty"); 10310 for (int i = 0; i < array.size(); i++) { 10311 res.getSpecialty().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 10312 } 10313 }; 10314 if (json.has("location")) { 10315 JsonArray array = json.getAsJsonArray("location"); 10316 for (int i = 0; i < array.size(); i++) { 10317 res.getLocation().add(parseReference(array.get(i).getAsJsonObject())); 10318 } 10319 }; 10320 if (json.has("name")) 10321 res.setNameElement(parseString(json.get("name").getAsString())); 10322 if (json.has("_name")) 10323 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 10324 if (json.has("comment")) 10325 res.setCommentElement(parseString(json.get("comment").getAsString())); 10326 if (json.has("_comment")) 10327 parseElementProperties(getJObject(json, "_comment"), res.getCommentElement()); 10328 if (json.has("extraDetails")) 10329 res.setExtraDetailsElement(parseString(json.get("extraDetails").getAsString())); 10330 if (json.has("_extraDetails")) 10331 parseElementProperties(getJObject(json, "_extraDetails"), res.getExtraDetailsElement()); 10332 if (json.has("photo")) 10333 res.setPhoto(parseAttachment(getJObject(json, "photo"))); 10334 if (json.has("telecom")) { 10335 JsonArray array = json.getAsJsonArray("telecom"); 10336 for (int i = 0; i < array.size(); i++) { 10337 res.getTelecom().add(parseContactPoint(array.get(i).getAsJsonObject())); 10338 } 10339 }; 10340 if (json.has("coverageArea")) { 10341 JsonArray array = json.getAsJsonArray("coverageArea"); 10342 for (int i = 0; i < array.size(); i++) { 10343 res.getCoverageArea().add(parseReference(array.get(i).getAsJsonObject())); 10344 } 10345 }; 10346 if (json.has("serviceProvisionCode")) { 10347 JsonArray array = json.getAsJsonArray("serviceProvisionCode"); 10348 for (int i = 0; i < array.size(); i++) { 10349 res.getServiceProvisionCode().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 10350 } 10351 }; 10352 if (json.has("eligibility")) 10353 res.setEligibility(parseCodeableConcept(getJObject(json, "eligibility"))); 10354 if (json.has("eligibilityNote")) 10355 res.setEligibilityNoteElement(parseString(json.get("eligibilityNote").getAsString())); 10356 if (json.has("_eligibilityNote")) 10357 parseElementProperties(getJObject(json, "_eligibilityNote"), res.getEligibilityNoteElement()); 10358 if (json.has("programName")) { 10359 JsonArray array = json.getAsJsonArray("programName"); 10360 for (int i = 0; i < array.size(); i++) { 10361 if (array.get(i).isJsonNull()) { 10362 res.getProgramName().add(new StringType()); 10363 } else { 10364 res.getProgramName().add(parseString(array.get(i).getAsString())); 10365 } 10366 } 10367 }; 10368 if (json.has("_programName")) { 10369 JsonArray array = json.getAsJsonArray("_programName"); 10370 for (int i = 0; i < array.size(); i++) { 10371 if (i == res.getProgramName().size()) 10372 res.getProgramName().add(parseString(null)); 10373 if (array.get(i) instanceof JsonObject) 10374 parseElementProperties(array.get(i).getAsJsonObject(), res.getProgramName().get(i)); 10375 } 10376 }; 10377 if (json.has("characteristic")) { 10378 JsonArray array = json.getAsJsonArray("characteristic"); 10379 for (int i = 0; i < array.size(); i++) { 10380 res.getCharacteristic().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 10381 } 10382 }; 10383 if (json.has("referralMethod")) { 10384 JsonArray array = json.getAsJsonArray("referralMethod"); 10385 for (int i = 0; i < array.size(); i++) { 10386 res.getReferralMethod().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 10387 } 10388 }; 10389 if (json.has("appointmentRequired")) 10390 res.setAppointmentRequiredElement(parseBoolean(json.get("appointmentRequired").getAsBoolean())); 10391 if (json.has("_appointmentRequired")) 10392 parseElementProperties(getJObject(json, "_appointmentRequired"), res.getAppointmentRequiredElement()); 10393 if (json.has("availableTime")) { 10394 JsonArray array = json.getAsJsonArray("availableTime"); 10395 for (int i = 0; i < array.size(); i++) { 10396 res.getAvailableTime().add(parseHealthcareServiceHealthcareServiceAvailableTimeComponent(array.get(i).getAsJsonObject(), res)); 10397 } 10398 }; 10399 if (json.has("notAvailable")) { 10400 JsonArray array = json.getAsJsonArray("notAvailable"); 10401 for (int i = 0; i < array.size(); i++) { 10402 res.getNotAvailable().add(parseHealthcareServiceHealthcareServiceNotAvailableComponent(array.get(i).getAsJsonObject(), res)); 10403 } 10404 }; 10405 if (json.has("availabilityExceptions")) 10406 res.setAvailabilityExceptionsElement(parseString(json.get("availabilityExceptions").getAsString())); 10407 if (json.has("_availabilityExceptions")) 10408 parseElementProperties(getJObject(json, "_availabilityExceptions"), res.getAvailabilityExceptionsElement()); 10409 if (json.has("endpoint")) { 10410 JsonArray array = json.getAsJsonArray("endpoint"); 10411 for (int i = 0; i < array.size(); i++) { 10412 res.getEndpoint().add(parseReference(array.get(i).getAsJsonObject())); 10413 } 10414 }; 10415 } 10416 10417 protected HealthcareService.HealthcareServiceAvailableTimeComponent parseHealthcareServiceHealthcareServiceAvailableTimeComponent(JsonObject json, HealthcareService owner) throws IOException, FHIRFormatError { 10418 HealthcareService.HealthcareServiceAvailableTimeComponent res = new HealthcareService.HealthcareServiceAvailableTimeComponent(); 10419 parseHealthcareServiceHealthcareServiceAvailableTimeComponentProperties(json, owner, res); 10420 return res; 10421 } 10422 10423 protected void parseHealthcareServiceHealthcareServiceAvailableTimeComponentProperties(JsonObject json, HealthcareService owner, HealthcareService.HealthcareServiceAvailableTimeComponent res) throws IOException, FHIRFormatError { 10424 parseBackboneProperties(json, res); 10425 if (json.has("daysOfWeek")) { 10426 JsonArray array = json.getAsJsonArray("daysOfWeek"); 10427 for (int i = 0; i < array.size(); i++) { 10428 if (array.get(i).isJsonNull()) { 10429 res.getDaysOfWeek().add(new Enumeration<HealthcareService.DaysOfWeek>()); 10430 } else { 10431 res.getDaysOfWeek().add(parseEnumeration(array.get(i).getAsString(), HealthcareService.DaysOfWeek.NULL, new HealthcareService.DaysOfWeekEnumFactory())); 10432 } 10433 } 10434 }; 10435 if (json.has("_daysOfWeek")) { 10436 JsonArray array = json.getAsJsonArray("_daysOfWeek"); 10437 for (int i = 0; i < array.size(); i++) { 10438 if (i == res.getDaysOfWeek().size()) 10439 res.getDaysOfWeek().add(parseEnumeration(null, HealthcareService.DaysOfWeek.NULL, new HealthcareService.DaysOfWeekEnumFactory())); 10440 if (array.get(i) instanceof JsonObject) 10441 parseElementProperties(array.get(i).getAsJsonObject(), res.getDaysOfWeek().get(i)); 10442 } 10443 }; 10444 if (json.has("allDay")) 10445 res.setAllDayElement(parseBoolean(json.get("allDay").getAsBoolean())); 10446 if (json.has("_allDay")) 10447 parseElementProperties(getJObject(json, "_allDay"), res.getAllDayElement()); 10448 if (json.has("availableStartTime")) 10449 res.setAvailableStartTimeElement(parseTime(json.get("availableStartTime").getAsString())); 10450 if (json.has("_availableStartTime")) 10451 parseElementProperties(getJObject(json, "_availableStartTime"), res.getAvailableStartTimeElement()); 10452 if (json.has("availableEndTime")) 10453 res.setAvailableEndTimeElement(parseTime(json.get("availableEndTime").getAsString())); 10454 if (json.has("_availableEndTime")) 10455 parseElementProperties(getJObject(json, "_availableEndTime"), res.getAvailableEndTimeElement()); 10456 } 10457 10458 protected HealthcareService.HealthcareServiceNotAvailableComponent parseHealthcareServiceHealthcareServiceNotAvailableComponent(JsonObject json, HealthcareService owner) throws IOException, FHIRFormatError { 10459 HealthcareService.HealthcareServiceNotAvailableComponent res = new HealthcareService.HealthcareServiceNotAvailableComponent(); 10460 parseHealthcareServiceHealthcareServiceNotAvailableComponentProperties(json, owner, res); 10461 return res; 10462 } 10463 10464 protected void parseHealthcareServiceHealthcareServiceNotAvailableComponentProperties(JsonObject json, HealthcareService owner, HealthcareService.HealthcareServiceNotAvailableComponent res) throws IOException, FHIRFormatError { 10465 parseBackboneProperties(json, res); 10466 if (json.has("description")) 10467 res.setDescriptionElement(parseString(json.get("description").getAsString())); 10468 if (json.has("_description")) 10469 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 10470 if (json.has("during")) 10471 res.setDuring(parsePeriod(getJObject(json, "during"))); 10472 } 10473 10474 protected ImagingManifest parseImagingManifest(JsonObject json) throws IOException, FHIRFormatError { 10475 ImagingManifest res = new ImagingManifest(); 10476 parseImagingManifestProperties(json, res); 10477 return res; 10478 } 10479 10480 protected void parseImagingManifestProperties(JsonObject json, ImagingManifest res) throws IOException, FHIRFormatError { 10481 parseDomainResourceProperties(json, res); 10482 if (json.has("identifier")) 10483 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 10484 if (json.has("patient")) 10485 res.setPatient(parseReference(getJObject(json, "patient"))); 10486 if (json.has("authoringTime")) 10487 res.setAuthoringTimeElement(parseDateTime(json.get("authoringTime").getAsString())); 10488 if (json.has("_authoringTime")) 10489 parseElementProperties(getJObject(json, "_authoringTime"), res.getAuthoringTimeElement()); 10490 if (json.has("author")) 10491 res.setAuthor(parseReference(getJObject(json, "author"))); 10492 if (json.has("description")) 10493 res.setDescriptionElement(parseString(json.get("description").getAsString())); 10494 if (json.has("_description")) 10495 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 10496 if (json.has("study")) { 10497 JsonArray array = json.getAsJsonArray("study"); 10498 for (int i = 0; i < array.size(); i++) { 10499 res.getStudy().add(parseImagingManifestStudyComponent(array.get(i).getAsJsonObject(), res)); 10500 } 10501 }; 10502 } 10503 10504 protected ImagingManifest.StudyComponent parseImagingManifestStudyComponent(JsonObject json, ImagingManifest owner) throws IOException, FHIRFormatError { 10505 ImagingManifest.StudyComponent res = new ImagingManifest.StudyComponent(); 10506 parseImagingManifestStudyComponentProperties(json, owner, res); 10507 return res; 10508 } 10509 10510 protected void parseImagingManifestStudyComponentProperties(JsonObject json, ImagingManifest owner, ImagingManifest.StudyComponent res) throws IOException, FHIRFormatError { 10511 parseBackboneProperties(json, res); 10512 if (json.has("uid")) 10513 res.setUidElement(parseOid(json.get("uid").getAsString())); 10514 if (json.has("_uid")) 10515 parseElementProperties(getJObject(json, "_uid"), res.getUidElement()); 10516 if (json.has("imagingStudy")) 10517 res.setImagingStudy(parseReference(getJObject(json, "imagingStudy"))); 10518 if (json.has("endpoint")) { 10519 JsonArray array = json.getAsJsonArray("endpoint"); 10520 for (int i = 0; i < array.size(); i++) { 10521 res.getEndpoint().add(parseReference(array.get(i).getAsJsonObject())); 10522 } 10523 }; 10524 if (json.has("series")) { 10525 JsonArray array = json.getAsJsonArray("series"); 10526 for (int i = 0; i < array.size(); i++) { 10527 res.getSeries().add(parseImagingManifestSeriesComponent(array.get(i).getAsJsonObject(), owner)); 10528 } 10529 }; 10530 } 10531 10532 protected ImagingManifest.SeriesComponent parseImagingManifestSeriesComponent(JsonObject json, ImagingManifest owner) throws IOException, FHIRFormatError { 10533 ImagingManifest.SeriesComponent res = new ImagingManifest.SeriesComponent(); 10534 parseImagingManifestSeriesComponentProperties(json, owner, res); 10535 return res; 10536 } 10537 10538 protected void parseImagingManifestSeriesComponentProperties(JsonObject json, ImagingManifest owner, ImagingManifest.SeriesComponent res) throws IOException, FHIRFormatError { 10539 parseBackboneProperties(json, res); 10540 if (json.has("uid")) 10541 res.setUidElement(parseOid(json.get("uid").getAsString())); 10542 if (json.has("_uid")) 10543 parseElementProperties(getJObject(json, "_uid"), res.getUidElement()); 10544 if (json.has("endpoint")) { 10545 JsonArray array = json.getAsJsonArray("endpoint"); 10546 for (int i = 0; i < array.size(); i++) { 10547 res.getEndpoint().add(parseReference(array.get(i).getAsJsonObject())); 10548 } 10549 }; 10550 if (json.has("instance")) { 10551 JsonArray array = json.getAsJsonArray("instance"); 10552 for (int i = 0; i < array.size(); i++) { 10553 res.getInstance().add(parseImagingManifestInstanceComponent(array.get(i).getAsJsonObject(), owner)); 10554 } 10555 }; 10556 } 10557 10558 protected ImagingManifest.InstanceComponent parseImagingManifestInstanceComponent(JsonObject json, ImagingManifest owner) throws IOException, FHIRFormatError { 10559 ImagingManifest.InstanceComponent res = new ImagingManifest.InstanceComponent(); 10560 parseImagingManifestInstanceComponentProperties(json, owner, res); 10561 return res; 10562 } 10563 10564 protected void parseImagingManifestInstanceComponentProperties(JsonObject json, ImagingManifest owner, ImagingManifest.InstanceComponent res) throws IOException, FHIRFormatError { 10565 parseBackboneProperties(json, res); 10566 if (json.has("sopClass")) 10567 res.setSopClassElement(parseOid(json.get("sopClass").getAsString())); 10568 if (json.has("_sopClass")) 10569 parseElementProperties(getJObject(json, "_sopClass"), res.getSopClassElement()); 10570 if (json.has("uid")) 10571 res.setUidElement(parseOid(json.get("uid").getAsString())); 10572 if (json.has("_uid")) 10573 parseElementProperties(getJObject(json, "_uid"), res.getUidElement()); 10574 } 10575 10576 protected ImagingStudy parseImagingStudy(JsonObject json) throws IOException, FHIRFormatError { 10577 ImagingStudy res = new ImagingStudy(); 10578 parseImagingStudyProperties(json, res); 10579 return res; 10580 } 10581 10582 protected void parseImagingStudyProperties(JsonObject json, ImagingStudy res) throws IOException, FHIRFormatError { 10583 parseDomainResourceProperties(json, res); 10584 if (json.has("uid")) 10585 res.setUidElement(parseOid(json.get("uid").getAsString())); 10586 if (json.has("_uid")) 10587 parseElementProperties(getJObject(json, "_uid"), res.getUidElement()); 10588 if (json.has("accession")) 10589 res.setAccession(parseIdentifier(getJObject(json, "accession"))); 10590 if (json.has("identifier")) { 10591 JsonArray array = json.getAsJsonArray("identifier"); 10592 for (int i = 0; i < array.size(); i++) { 10593 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 10594 } 10595 }; 10596 if (json.has("availability")) 10597 res.setAvailabilityElement(parseEnumeration(json.get("availability").getAsString(), ImagingStudy.InstanceAvailability.NULL, new ImagingStudy.InstanceAvailabilityEnumFactory())); 10598 if (json.has("_availability")) 10599 parseElementProperties(getJObject(json, "_availability"), res.getAvailabilityElement()); 10600 if (json.has("modalityList")) { 10601 JsonArray array = json.getAsJsonArray("modalityList"); 10602 for (int i = 0; i < array.size(); i++) { 10603 res.getModalityList().add(parseCoding(array.get(i).getAsJsonObject())); 10604 } 10605 }; 10606 if (json.has("patient")) 10607 res.setPatient(parseReference(getJObject(json, "patient"))); 10608 if (json.has("context")) 10609 res.setContext(parseReference(getJObject(json, "context"))); 10610 if (json.has("started")) 10611 res.setStartedElement(parseDateTime(json.get("started").getAsString())); 10612 if (json.has("_started")) 10613 parseElementProperties(getJObject(json, "_started"), res.getStartedElement()); 10614 if (json.has("basedOn")) { 10615 JsonArray array = json.getAsJsonArray("basedOn"); 10616 for (int i = 0; i < array.size(); i++) { 10617 res.getBasedOn().add(parseReference(array.get(i).getAsJsonObject())); 10618 } 10619 }; 10620 if (json.has("referrer")) 10621 res.setReferrer(parseReference(getJObject(json, "referrer"))); 10622 if (json.has("interpreter")) { 10623 JsonArray array = json.getAsJsonArray("interpreter"); 10624 for (int i = 0; i < array.size(); i++) { 10625 res.getInterpreter().add(parseReference(array.get(i).getAsJsonObject())); 10626 } 10627 }; 10628 if (json.has("endpoint")) { 10629 JsonArray array = json.getAsJsonArray("endpoint"); 10630 for (int i = 0; i < array.size(); i++) { 10631 res.getEndpoint().add(parseReference(array.get(i).getAsJsonObject())); 10632 } 10633 }; 10634 if (json.has("numberOfSeries")) 10635 res.setNumberOfSeriesElement(parseUnsignedInt(json.get("numberOfSeries").getAsString())); 10636 if (json.has("_numberOfSeries")) 10637 parseElementProperties(getJObject(json, "_numberOfSeries"), res.getNumberOfSeriesElement()); 10638 if (json.has("numberOfInstances")) 10639 res.setNumberOfInstancesElement(parseUnsignedInt(json.get("numberOfInstances").getAsString())); 10640 if (json.has("_numberOfInstances")) 10641 parseElementProperties(getJObject(json, "_numberOfInstances"), res.getNumberOfInstancesElement()); 10642 if (json.has("procedureReference")) { 10643 JsonArray array = json.getAsJsonArray("procedureReference"); 10644 for (int i = 0; i < array.size(); i++) { 10645 res.getProcedureReference().add(parseReference(array.get(i).getAsJsonObject())); 10646 } 10647 }; 10648 if (json.has("procedureCode")) { 10649 JsonArray array = json.getAsJsonArray("procedureCode"); 10650 for (int i = 0; i < array.size(); i++) { 10651 res.getProcedureCode().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 10652 } 10653 }; 10654 if (json.has("reason")) 10655 res.setReason(parseCodeableConcept(getJObject(json, "reason"))); 10656 if (json.has("description")) 10657 res.setDescriptionElement(parseString(json.get("description").getAsString())); 10658 if (json.has("_description")) 10659 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 10660 if (json.has("series")) { 10661 JsonArray array = json.getAsJsonArray("series"); 10662 for (int i = 0; i < array.size(); i++) { 10663 res.getSeries().add(parseImagingStudyImagingStudySeriesComponent(array.get(i).getAsJsonObject(), res)); 10664 } 10665 }; 10666 } 10667 10668 protected ImagingStudy.ImagingStudySeriesComponent parseImagingStudyImagingStudySeriesComponent(JsonObject json, ImagingStudy owner) throws IOException, FHIRFormatError { 10669 ImagingStudy.ImagingStudySeriesComponent res = new ImagingStudy.ImagingStudySeriesComponent(); 10670 parseImagingStudyImagingStudySeriesComponentProperties(json, owner, res); 10671 return res; 10672 } 10673 10674 protected void parseImagingStudyImagingStudySeriesComponentProperties(JsonObject json, ImagingStudy owner, ImagingStudy.ImagingStudySeriesComponent res) throws IOException, FHIRFormatError { 10675 parseBackboneProperties(json, res); 10676 if (json.has("uid")) 10677 res.setUidElement(parseOid(json.get("uid").getAsString())); 10678 if (json.has("_uid")) 10679 parseElementProperties(getJObject(json, "_uid"), res.getUidElement()); 10680 if (json.has("number")) 10681 res.setNumberElement(parseUnsignedInt(json.get("number").getAsString())); 10682 if (json.has("_number")) 10683 parseElementProperties(getJObject(json, "_number"), res.getNumberElement()); 10684 if (json.has("modality")) 10685 res.setModality(parseCoding(getJObject(json, "modality"))); 10686 if (json.has("description")) 10687 res.setDescriptionElement(parseString(json.get("description").getAsString())); 10688 if (json.has("_description")) 10689 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 10690 if (json.has("numberOfInstances")) 10691 res.setNumberOfInstancesElement(parseUnsignedInt(json.get("numberOfInstances").getAsString())); 10692 if (json.has("_numberOfInstances")) 10693 parseElementProperties(getJObject(json, "_numberOfInstances"), res.getNumberOfInstancesElement()); 10694 if (json.has("availability")) 10695 res.setAvailabilityElement(parseEnumeration(json.get("availability").getAsString(), ImagingStudy.InstanceAvailability.NULL, new ImagingStudy.InstanceAvailabilityEnumFactory())); 10696 if (json.has("_availability")) 10697 parseElementProperties(getJObject(json, "_availability"), res.getAvailabilityElement()); 10698 if (json.has("endpoint")) { 10699 JsonArray array = json.getAsJsonArray("endpoint"); 10700 for (int i = 0; i < array.size(); i++) { 10701 res.getEndpoint().add(parseReference(array.get(i).getAsJsonObject())); 10702 } 10703 }; 10704 if (json.has("bodySite")) 10705 res.setBodySite(parseCoding(getJObject(json, "bodySite"))); 10706 if (json.has("laterality")) 10707 res.setLaterality(parseCoding(getJObject(json, "laterality"))); 10708 if (json.has("started")) 10709 res.setStartedElement(parseDateTime(json.get("started").getAsString())); 10710 if (json.has("_started")) 10711 parseElementProperties(getJObject(json, "_started"), res.getStartedElement()); 10712 if (json.has("performer")) { 10713 JsonArray array = json.getAsJsonArray("performer"); 10714 for (int i = 0; i < array.size(); i++) { 10715 res.getPerformer().add(parseReference(array.get(i).getAsJsonObject())); 10716 } 10717 }; 10718 if (json.has("instance")) { 10719 JsonArray array = json.getAsJsonArray("instance"); 10720 for (int i = 0; i < array.size(); i++) { 10721 res.getInstance().add(parseImagingStudyImagingStudySeriesInstanceComponent(array.get(i).getAsJsonObject(), owner)); 10722 } 10723 }; 10724 } 10725 10726 protected ImagingStudy.ImagingStudySeriesInstanceComponent parseImagingStudyImagingStudySeriesInstanceComponent(JsonObject json, ImagingStudy owner) throws IOException, FHIRFormatError { 10727 ImagingStudy.ImagingStudySeriesInstanceComponent res = new ImagingStudy.ImagingStudySeriesInstanceComponent(); 10728 parseImagingStudyImagingStudySeriesInstanceComponentProperties(json, owner, res); 10729 return res; 10730 } 10731 10732 protected void parseImagingStudyImagingStudySeriesInstanceComponentProperties(JsonObject json, ImagingStudy owner, ImagingStudy.ImagingStudySeriesInstanceComponent res) throws IOException, FHIRFormatError { 10733 parseBackboneProperties(json, res); 10734 if (json.has("uid")) 10735 res.setUidElement(parseOid(json.get("uid").getAsString())); 10736 if (json.has("_uid")) 10737 parseElementProperties(getJObject(json, "_uid"), res.getUidElement()); 10738 if (json.has("number")) 10739 res.setNumberElement(parseUnsignedInt(json.get("number").getAsString())); 10740 if (json.has("_number")) 10741 parseElementProperties(getJObject(json, "_number"), res.getNumberElement()); 10742 if (json.has("sopClass")) 10743 res.setSopClassElement(parseOid(json.get("sopClass").getAsString())); 10744 if (json.has("_sopClass")) 10745 parseElementProperties(getJObject(json, "_sopClass"), res.getSopClassElement()); 10746 if (json.has("title")) 10747 res.setTitleElement(parseString(json.get("title").getAsString())); 10748 if (json.has("_title")) 10749 parseElementProperties(getJObject(json, "_title"), res.getTitleElement()); 10750 } 10751 10752 protected Immunization parseImmunization(JsonObject json) throws IOException, FHIRFormatError { 10753 Immunization res = new Immunization(); 10754 parseImmunizationProperties(json, res); 10755 return res; 10756 } 10757 10758 protected void parseImmunizationProperties(JsonObject json, Immunization res) throws IOException, FHIRFormatError { 10759 parseDomainResourceProperties(json, res); 10760 if (json.has("identifier")) { 10761 JsonArray array = json.getAsJsonArray("identifier"); 10762 for (int i = 0; i < array.size(); i++) { 10763 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 10764 } 10765 }; 10766 if (json.has("status")) 10767 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Immunization.ImmunizationStatus.NULL, new Immunization.ImmunizationStatusEnumFactory())); 10768 if (json.has("_status")) 10769 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 10770 if (json.has("notGiven")) 10771 res.setNotGivenElement(parseBoolean(json.get("notGiven").getAsBoolean())); 10772 if (json.has("_notGiven")) 10773 parseElementProperties(getJObject(json, "_notGiven"), res.getNotGivenElement()); 10774 if (json.has("vaccineCode")) 10775 res.setVaccineCode(parseCodeableConcept(getJObject(json, "vaccineCode"))); 10776 if (json.has("patient")) 10777 res.setPatient(parseReference(getJObject(json, "patient"))); 10778 if (json.has("encounter")) 10779 res.setEncounter(parseReference(getJObject(json, "encounter"))); 10780 if (json.has("date")) 10781 res.setDateElement(parseDateTime(json.get("date").getAsString())); 10782 if (json.has("_date")) 10783 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 10784 if (json.has("primarySource")) 10785 res.setPrimarySourceElement(parseBoolean(json.get("primarySource").getAsBoolean())); 10786 if (json.has("_primarySource")) 10787 parseElementProperties(getJObject(json, "_primarySource"), res.getPrimarySourceElement()); 10788 if (json.has("reportOrigin")) 10789 res.setReportOrigin(parseCodeableConcept(getJObject(json, "reportOrigin"))); 10790 if (json.has("location")) 10791 res.setLocation(parseReference(getJObject(json, "location"))); 10792 if (json.has("manufacturer")) 10793 res.setManufacturer(parseReference(getJObject(json, "manufacturer"))); 10794 if (json.has("lotNumber")) 10795 res.setLotNumberElement(parseString(json.get("lotNumber").getAsString())); 10796 if (json.has("_lotNumber")) 10797 parseElementProperties(getJObject(json, "_lotNumber"), res.getLotNumberElement()); 10798 if (json.has("expirationDate")) 10799 res.setExpirationDateElement(parseDate(json.get("expirationDate").getAsString())); 10800 if (json.has("_expirationDate")) 10801 parseElementProperties(getJObject(json, "_expirationDate"), res.getExpirationDateElement()); 10802 if (json.has("site")) 10803 res.setSite(parseCodeableConcept(getJObject(json, "site"))); 10804 if (json.has("route")) 10805 res.setRoute(parseCodeableConcept(getJObject(json, "route"))); 10806 if (json.has("doseQuantity")) 10807 res.setDoseQuantity(parseSimpleQuantity(getJObject(json, "doseQuantity"))); 10808 if (json.has("practitioner")) { 10809 JsonArray array = json.getAsJsonArray("practitioner"); 10810 for (int i = 0; i < array.size(); i++) { 10811 res.getPractitioner().add(parseImmunizationImmunizationPractitionerComponent(array.get(i).getAsJsonObject(), res)); 10812 } 10813 }; 10814 if (json.has("note")) { 10815 JsonArray array = json.getAsJsonArray("note"); 10816 for (int i = 0; i < array.size(); i++) { 10817 res.getNote().add(parseAnnotation(array.get(i).getAsJsonObject())); 10818 } 10819 }; 10820 if (json.has("explanation")) 10821 res.setExplanation(parseImmunizationImmunizationExplanationComponent(getJObject(json, "explanation"), res)); 10822 if (json.has("reaction")) { 10823 JsonArray array = json.getAsJsonArray("reaction"); 10824 for (int i = 0; i < array.size(); i++) { 10825 res.getReaction().add(parseImmunizationImmunizationReactionComponent(array.get(i).getAsJsonObject(), res)); 10826 } 10827 }; 10828 if (json.has("vaccinationProtocol")) { 10829 JsonArray array = json.getAsJsonArray("vaccinationProtocol"); 10830 for (int i = 0; i < array.size(); i++) { 10831 res.getVaccinationProtocol().add(parseImmunizationImmunizationVaccinationProtocolComponent(array.get(i).getAsJsonObject(), res)); 10832 } 10833 }; 10834 } 10835 10836 protected Immunization.ImmunizationPractitionerComponent parseImmunizationImmunizationPractitionerComponent(JsonObject json, Immunization owner) throws IOException, FHIRFormatError { 10837 Immunization.ImmunizationPractitionerComponent res = new Immunization.ImmunizationPractitionerComponent(); 10838 parseImmunizationImmunizationPractitionerComponentProperties(json, owner, res); 10839 return res; 10840 } 10841 10842 protected void parseImmunizationImmunizationPractitionerComponentProperties(JsonObject json, Immunization owner, Immunization.ImmunizationPractitionerComponent res) throws IOException, FHIRFormatError { 10843 parseBackboneProperties(json, res); 10844 if (json.has("role")) 10845 res.setRole(parseCodeableConcept(getJObject(json, "role"))); 10846 if (json.has("actor")) 10847 res.setActor(parseReference(getJObject(json, "actor"))); 10848 } 10849 10850 protected Immunization.ImmunizationExplanationComponent parseImmunizationImmunizationExplanationComponent(JsonObject json, Immunization owner) throws IOException, FHIRFormatError { 10851 Immunization.ImmunizationExplanationComponent res = new Immunization.ImmunizationExplanationComponent(); 10852 parseImmunizationImmunizationExplanationComponentProperties(json, owner, res); 10853 return res; 10854 } 10855 10856 protected void parseImmunizationImmunizationExplanationComponentProperties(JsonObject json, Immunization owner, Immunization.ImmunizationExplanationComponent res) throws IOException, FHIRFormatError { 10857 parseBackboneProperties(json, res); 10858 if (json.has("reason")) { 10859 JsonArray array = json.getAsJsonArray("reason"); 10860 for (int i = 0; i < array.size(); i++) { 10861 res.getReason().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 10862 } 10863 }; 10864 if (json.has("reasonNotGiven")) { 10865 JsonArray array = json.getAsJsonArray("reasonNotGiven"); 10866 for (int i = 0; i < array.size(); i++) { 10867 res.getReasonNotGiven().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 10868 } 10869 }; 10870 } 10871 10872 protected Immunization.ImmunizationReactionComponent parseImmunizationImmunizationReactionComponent(JsonObject json, Immunization owner) throws IOException, FHIRFormatError { 10873 Immunization.ImmunizationReactionComponent res = new Immunization.ImmunizationReactionComponent(); 10874 parseImmunizationImmunizationReactionComponentProperties(json, owner, res); 10875 return res; 10876 } 10877 10878 protected void parseImmunizationImmunizationReactionComponentProperties(JsonObject json, Immunization owner, Immunization.ImmunizationReactionComponent res) throws IOException, FHIRFormatError { 10879 parseBackboneProperties(json, res); 10880 if (json.has("date")) 10881 res.setDateElement(parseDateTime(json.get("date").getAsString())); 10882 if (json.has("_date")) 10883 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 10884 if (json.has("detail")) 10885 res.setDetail(parseReference(getJObject(json, "detail"))); 10886 if (json.has("reported")) 10887 res.setReportedElement(parseBoolean(json.get("reported").getAsBoolean())); 10888 if (json.has("_reported")) 10889 parseElementProperties(getJObject(json, "_reported"), res.getReportedElement()); 10890 } 10891 10892 protected Immunization.ImmunizationVaccinationProtocolComponent parseImmunizationImmunizationVaccinationProtocolComponent(JsonObject json, Immunization owner) throws IOException, FHIRFormatError { 10893 Immunization.ImmunizationVaccinationProtocolComponent res = new Immunization.ImmunizationVaccinationProtocolComponent(); 10894 parseImmunizationImmunizationVaccinationProtocolComponentProperties(json, owner, res); 10895 return res; 10896 } 10897 10898 protected void parseImmunizationImmunizationVaccinationProtocolComponentProperties(JsonObject json, Immunization owner, Immunization.ImmunizationVaccinationProtocolComponent res) throws IOException, FHIRFormatError { 10899 parseBackboneProperties(json, res); 10900 if (json.has("doseSequence")) 10901 res.setDoseSequenceElement(parsePositiveInt(json.get("doseSequence").getAsString())); 10902 if (json.has("_doseSequence")) 10903 parseElementProperties(getJObject(json, "_doseSequence"), res.getDoseSequenceElement()); 10904 if (json.has("description")) 10905 res.setDescriptionElement(parseString(json.get("description").getAsString())); 10906 if (json.has("_description")) 10907 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 10908 if (json.has("authority")) 10909 res.setAuthority(parseReference(getJObject(json, "authority"))); 10910 if (json.has("series")) 10911 res.setSeriesElement(parseString(json.get("series").getAsString())); 10912 if (json.has("_series")) 10913 parseElementProperties(getJObject(json, "_series"), res.getSeriesElement()); 10914 if (json.has("seriesDoses")) 10915 res.setSeriesDosesElement(parsePositiveInt(json.get("seriesDoses").getAsString())); 10916 if (json.has("_seriesDoses")) 10917 parseElementProperties(getJObject(json, "_seriesDoses"), res.getSeriesDosesElement()); 10918 if (json.has("targetDisease")) { 10919 JsonArray array = json.getAsJsonArray("targetDisease"); 10920 for (int i = 0; i < array.size(); i++) { 10921 res.getTargetDisease().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 10922 } 10923 }; 10924 if (json.has("doseStatus")) 10925 res.setDoseStatus(parseCodeableConcept(getJObject(json, "doseStatus"))); 10926 if (json.has("doseStatusReason")) 10927 res.setDoseStatusReason(parseCodeableConcept(getJObject(json, "doseStatusReason"))); 10928 } 10929 10930 protected ImmunizationRecommendation parseImmunizationRecommendation(JsonObject json) throws IOException, FHIRFormatError { 10931 ImmunizationRecommendation res = new ImmunizationRecommendation(); 10932 parseImmunizationRecommendationProperties(json, res); 10933 return res; 10934 } 10935 10936 protected void parseImmunizationRecommendationProperties(JsonObject json, ImmunizationRecommendation res) throws IOException, FHIRFormatError { 10937 parseDomainResourceProperties(json, res); 10938 if (json.has("identifier")) { 10939 JsonArray array = json.getAsJsonArray("identifier"); 10940 for (int i = 0; i < array.size(); i++) { 10941 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 10942 } 10943 }; 10944 if (json.has("patient")) 10945 res.setPatient(parseReference(getJObject(json, "patient"))); 10946 if (json.has("recommendation")) { 10947 JsonArray array = json.getAsJsonArray("recommendation"); 10948 for (int i = 0; i < array.size(); i++) { 10949 res.getRecommendation().add(parseImmunizationRecommendationImmunizationRecommendationRecommendationComponent(array.get(i).getAsJsonObject(), res)); 10950 } 10951 }; 10952 } 10953 10954 protected ImmunizationRecommendation.ImmunizationRecommendationRecommendationComponent parseImmunizationRecommendationImmunizationRecommendationRecommendationComponent(JsonObject json, ImmunizationRecommendation owner) throws IOException, FHIRFormatError { 10955 ImmunizationRecommendation.ImmunizationRecommendationRecommendationComponent res = new ImmunizationRecommendation.ImmunizationRecommendationRecommendationComponent(); 10956 parseImmunizationRecommendationImmunizationRecommendationRecommendationComponentProperties(json, owner, res); 10957 return res; 10958 } 10959 10960 protected void parseImmunizationRecommendationImmunizationRecommendationRecommendationComponentProperties(JsonObject json, ImmunizationRecommendation owner, ImmunizationRecommendation.ImmunizationRecommendationRecommendationComponent res) throws IOException, FHIRFormatError { 10961 parseBackboneProperties(json, res); 10962 if (json.has("date")) 10963 res.setDateElement(parseDateTime(json.get("date").getAsString())); 10964 if (json.has("_date")) 10965 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 10966 if (json.has("vaccineCode")) 10967 res.setVaccineCode(parseCodeableConcept(getJObject(json, "vaccineCode"))); 10968 if (json.has("targetDisease")) 10969 res.setTargetDisease(parseCodeableConcept(getJObject(json, "targetDisease"))); 10970 if (json.has("doseNumber")) 10971 res.setDoseNumberElement(parsePositiveInt(json.get("doseNumber").getAsString())); 10972 if (json.has("_doseNumber")) 10973 parseElementProperties(getJObject(json, "_doseNumber"), res.getDoseNumberElement()); 10974 if (json.has("forecastStatus")) 10975 res.setForecastStatus(parseCodeableConcept(getJObject(json, "forecastStatus"))); 10976 if (json.has("dateCriterion")) { 10977 JsonArray array = json.getAsJsonArray("dateCriterion"); 10978 for (int i = 0; i < array.size(); i++) { 10979 res.getDateCriterion().add(parseImmunizationRecommendationImmunizationRecommendationRecommendationDateCriterionComponent(array.get(i).getAsJsonObject(), owner)); 10980 } 10981 }; 10982 if (json.has("protocol")) 10983 res.setProtocol(parseImmunizationRecommendationImmunizationRecommendationRecommendationProtocolComponent(getJObject(json, "protocol"), owner)); 10984 if (json.has("supportingImmunization")) { 10985 JsonArray array = json.getAsJsonArray("supportingImmunization"); 10986 for (int i = 0; i < array.size(); i++) { 10987 res.getSupportingImmunization().add(parseReference(array.get(i).getAsJsonObject())); 10988 } 10989 }; 10990 if (json.has("supportingPatientInformation")) { 10991 JsonArray array = json.getAsJsonArray("supportingPatientInformation"); 10992 for (int i = 0; i < array.size(); i++) { 10993 res.getSupportingPatientInformation().add(parseReference(array.get(i).getAsJsonObject())); 10994 } 10995 }; 10996 } 10997 10998 protected ImmunizationRecommendation.ImmunizationRecommendationRecommendationDateCriterionComponent parseImmunizationRecommendationImmunizationRecommendationRecommendationDateCriterionComponent(JsonObject json, ImmunizationRecommendation owner) throws IOException, FHIRFormatError { 10999 ImmunizationRecommendation.ImmunizationRecommendationRecommendationDateCriterionComponent res = new ImmunizationRecommendation.ImmunizationRecommendationRecommendationDateCriterionComponent(); 11000 parseImmunizationRecommendationImmunizationRecommendationRecommendationDateCriterionComponentProperties(json, owner, res); 11001 return res; 11002 } 11003 11004 protected void parseImmunizationRecommendationImmunizationRecommendationRecommendationDateCriterionComponentProperties(JsonObject json, ImmunizationRecommendation owner, ImmunizationRecommendation.ImmunizationRecommendationRecommendationDateCriterionComponent res) throws IOException, FHIRFormatError { 11005 parseBackboneProperties(json, res); 11006 if (json.has("code")) 11007 res.setCode(parseCodeableConcept(getJObject(json, "code"))); 11008 if (json.has("value")) 11009 res.setValueElement(parseDateTime(json.get("value").getAsString())); 11010 if (json.has("_value")) 11011 parseElementProperties(getJObject(json, "_value"), res.getValueElement()); 11012 } 11013 11014 protected ImmunizationRecommendation.ImmunizationRecommendationRecommendationProtocolComponent parseImmunizationRecommendationImmunizationRecommendationRecommendationProtocolComponent(JsonObject json, ImmunizationRecommendation owner) throws IOException, FHIRFormatError { 11015 ImmunizationRecommendation.ImmunizationRecommendationRecommendationProtocolComponent res = new ImmunizationRecommendation.ImmunizationRecommendationRecommendationProtocolComponent(); 11016 parseImmunizationRecommendationImmunizationRecommendationRecommendationProtocolComponentProperties(json, owner, res); 11017 return res; 11018 } 11019 11020 protected void parseImmunizationRecommendationImmunizationRecommendationRecommendationProtocolComponentProperties(JsonObject json, ImmunizationRecommendation owner, ImmunizationRecommendation.ImmunizationRecommendationRecommendationProtocolComponent res) throws IOException, FHIRFormatError { 11021 parseBackboneProperties(json, res); 11022 if (json.has("doseSequence")) 11023 res.setDoseSequenceElement(parsePositiveInt(json.get("doseSequence").getAsString())); 11024 if (json.has("_doseSequence")) 11025 parseElementProperties(getJObject(json, "_doseSequence"), res.getDoseSequenceElement()); 11026 if (json.has("description")) 11027 res.setDescriptionElement(parseString(json.get("description").getAsString())); 11028 if (json.has("_description")) 11029 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 11030 if (json.has("authority")) 11031 res.setAuthority(parseReference(getJObject(json, "authority"))); 11032 if (json.has("series")) 11033 res.setSeriesElement(parseString(json.get("series").getAsString())); 11034 if (json.has("_series")) 11035 parseElementProperties(getJObject(json, "_series"), res.getSeriesElement()); 11036 } 11037 11038 protected ImplementationGuide parseImplementationGuide(JsonObject json) throws IOException, FHIRFormatError { 11039 ImplementationGuide res = new ImplementationGuide(); 11040 parseImplementationGuideProperties(json, res); 11041 return res; 11042 } 11043 11044 protected void parseImplementationGuideProperties(JsonObject json, ImplementationGuide res) throws IOException, FHIRFormatError { 11045 parseDomainResourceProperties(json, res); 11046 if (json.has("url")) 11047 res.setUrlElement(parseUri(json.get("url").getAsString())); 11048 if (json.has("_url")) 11049 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 11050 if (json.has("version")) 11051 res.setVersionElement(parseString(json.get("version").getAsString())); 11052 if (json.has("_version")) 11053 parseElementProperties(getJObject(json, "_version"), res.getVersionElement()); 11054 if (json.has("name")) 11055 res.setNameElement(parseString(json.get("name").getAsString())); 11056 if (json.has("_name")) 11057 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 11058 if (json.has("status")) 11059 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Enumerations.PublicationStatus.NULL, new Enumerations.PublicationStatusEnumFactory())); 11060 if (json.has("_status")) 11061 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 11062 if (json.has("experimental")) 11063 res.setExperimentalElement(parseBoolean(json.get("experimental").getAsBoolean())); 11064 if (json.has("_experimental")) 11065 parseElementProperties(getJObject(json, "_experimental"), res.getExperimentalElement()); 11066 if (json.has("date")) 11067 res.setDateElement(parseDateTime(json.get("date").getAsString())); 11068 if (json.has("_date")) 11069 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 11070 if (json.has("publisher")) 11071 res.setPublisherElement(parseString(json.get("publisher").getAsString())); 11072 if (json.has("_publisher")) 11073 parseElementProperties(getJObject(json, "_publisher"), res.getPublisherElement()); 11074 if (json.has("contact")) { 11075 JsonArray array = json.getAsJsonArray("contact"); 11076 for (int i = 0; i < array.size(); i++) { 11077 res.getContact().add(parseContactDetail(array.get(i).getAsJsonObject())); 11078 } 11079 }; 11080 if (json.has("description")) 11081 res.setDescriptionElement(parseMarkdown(json.get("description").getAsString())); 11082 if (json.has("_description")) 11083 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 11084 if (json.has("useContext")) { 11085 JsonArray array = json.getAsJsonArray("useContext"); 11086 for (int i = 0; i < array.size(); i++) { 11087 res.getUseContext().add(parseUsageContext(array.get(i).getAsJsonObject())); 11088 } 11089 }; 11090 if (json.has("jurisdiction")) { 11091 JsonArray array = json.getAsJsonArray("jurisdiction"); 11092 for (int i = 0; i < array.size(); i++) { 11093 res.getJurisdiction().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 11094 } 11095 }; 11096 if (json.has("copyright")) 11097 res.setCopyrightElement(parseMarkdown(json.get("copyright").getAsString())); 11098 if (json.has("_copyright")) 11099 parseElementProperties(getJObject(json, "_copyright"), res.getCopyrightElement()); 11100 if (json.has("fhirVersion")) 11101 res.setFhirVersionElement(parseId(json.get("fhirVersion").getAsString())); 11102 if (json.has("_fhirVersion")) 11103 parseElementProperties(getJObject(json, "_fhirVersion"), res.getFhirVersionElement()); 11104 if (json.has("dependency")) { 11105 JsonArray array = json.getAsJsonArray("dependency"); 11106 for (int i = 0; i < array.size(); i++) { 11107 res.getDependency().add(parseImplementationGuideImplementationGuideDependencyComponent(array.get(i).getAsJsonObject(), res)); 11108 } 11109 }; 11110 if (json.has("package")) { 11111 JsonArray array = json.getAsJsonArray("package"); 11112 for (int i = 0; i < array.size(); i++) { 11113 res.getPackage().add(parseImplementationGuideImplementationGuidePackageComponent(array.get(i).getAsJsonObject(), res)); 11114 } 11115 }; 11116 if (json.has("global")) { 11117 JsonArray array = json.getAsJsonArray("global"); 11118 for (int i = 0; i < array.size(); i++) { 11119 res.getGlobal().add(parseImplementationGuideImplementationGuideGlobalComponent(array.get(i).getAsJsonObject(), res)); 11120 } 11121 }; 11122 if (json.has("binary")) { 11123 JsonArray array = json.getAsJsonArray("binary"); 11124 for (int i = 0; i < array.size(); i++) { 11125 if (array.get(i).isJsonNull()) { 11126 res.getBinary().add(new UriType()); 11127 } else { 11128 res.getBinary().add(parseUri(array.get(i).getAsString())); 11129 } 11130 } 11131 }; 11132 if (json.has("_binary")) { 11133 JsonArray array = json.getAsJsonArray("_binary"); 11134 for (int i = 0; i < array.size(); i++) { 11135 if (i == res.getBinary().size()) 11136 res.getBinary().add(parseUri(null)); 11137 if (array.get(i) instanceof JsonObject) 11138 parseElementProperties(array.get(i).getAsJsonObject(), res.getBinary().get(i)); 11139 } 11140 }; 11141 if (json.has("page")) 11142 res.setPage(parseImplementationGuideImplementationGuidePageComponent(getJObject(json, "page"), res)); 11143 } 11144 11145 protected ImplementationGuide.ImplementationGuideDependencyComponent parseImplementationGuideImplementationGuideDependencyComponent(JsonObject json, ImplementationGuide owner) throws IOException, FHIRFormatError { 11146 ImplementationGuide.ImplementationGuideDependencyComponent res = new ImplementationGuide.ImplementationGuideDependencyComponent(); 11147 parseImplementationGuideImplementationGuideDependencyComponentProperties(json, owner, res); 11148 return res; 11149 } 11150 11151 protected void parseImplementationGuideImplementationGuideDependencyComponentProperties(JsonObject json, ImplementationGuide owner, ImplementationGuide.ImplementationGuideDependencyComponent res) throws IOException, FHIRFormatError { 11152 parseBackboneProperties(json, res); 11153 if (json.has("type")) 11154 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), ImplementationGuide.GuideDependencyType.NULL, new ImplementationGuide.GuideDependencyTypeEnumFactory())); 11155 if (json.has("_type")) 11156 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 11157 if (json.has("uri")) 11158 res.setUriElement(parseUri(json.get("uri").getAsString())); 11159 if (json.has("_uri")) 11160 parseElementProperties(getJObject(json, "_uri"), res.getUriElement()); 11161 } 11162 11163 protected ImplementationGuide.ImplementationGuidePackageComponent parseImplementationGuideImplementationGuidePackageComponent(JsonObject json, ImplementationGuide owner) throws IOException, FHIRFormatError { 11164 ImplementationGuide.ImplementationGuidePackageComponent res = new ImplementationGuide.ImplementationGuidePackageComponent(); 11165 parseImplementationGuideImplementationGuidePackageComponentProperties(json, owner, res); 11166 return res; 11167 } 11168 11169 protected void parseImplementationGuideImplementationGuidePackageComponentProperties(JsonObject json, ImplementationGuide owner, ImplementationGuide.ImplementationGuidePackageComponent res) throws IOException, FHIRFormatError { 11170 parseBackboneProperties(json, res); 11171 if (json.has("name")) 11172 res.setNameElement(parseString(json.get("name").getAsString())); 11173 if (json.has("_name")) 11174 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 11175 if (json.has("description")) 11176 res.setDescriptionElement(parseString(json.get("description").getAsString())); 11177 if (json.has("_description")) 11178 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 11179 if (json.has("resource")) { 11180 JsonArray array = json.getAsJsonArray("resource"); 11181 for (int i = 0; i < array.size(); i++) { 11182 res.getResource().add(parseImplementationGuideImplementationGuidePackageResourceComponent(array.get(i).getAsJsonObject(), owner)); 11183 } 11184 }; 11185 } 11186 11187 protected ImplementationGuide.ImplementationGuidePackageResourceComponent parseImplementationGuideImplementationGuidePackageResourceComponent(JsonObject json, ImplementationGuide owner) throws IOException, FHIRFormatError { 11188 ImplementationGuide.ImplementationGuidePackageResourceComponent res = new ImplementationGuide.ImplementationGuidePackageResourceComponent(); 11189 parseImplementationGuideImplementationGuidePackageResourceComponentProperties(json, owner, res); 11190 return res; 11191 } 11192 11193 protected void parseImplementationGuideImplementationGuidePackageResourceComponentProperties(JsonObject json, ImplementationGuide owner, ImplementationGuide.ImplementationGuidePackageResourceComponent res) throws IOException, FHIRFormatError { 11194 parseBackboneProperties(json, res); 11195 if (json.has("example")) 11196 res.setExampleElement(parseBoolean(json.get("example").getAsBoolean())); 11197 if (json.has("_example")) 11198 parseElementProperties(getJObject(json, "_example"), res.getExampleElement()); 11199 if (json.has("name")) 11200 res.setNameElement(parseString(json.get("name").getAsString())); 11201 if (json.has("_name")) 11202 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 11203 if (json.has("description")) 11204 res.setDescriptionElement(parseString(json.get("description").getAsString())); 11205 if (json.has("_description")) 11206 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 11207 if (json.has("acronym")) 11208 res.setAcronymElement(parseString(json.get("acronym").getAsString())); 11209 if (json.has("_acronym")) 11210 parseElementProperties(getJObject(json, "_acronym"), res.getAcronymElement()); 11211 Type source = parseType("source", json); 11212 if (source != null) 11213 res.setSource(source); 11214 if (json.has("exampleFor")) 11215 res.setExampleFor(parseReference(getJObject(json, "exampleFor"))); 11216 } 11217 11218 protected ImplementationGuide.ImplementationGuideGlobalComponent parseImplementationGuideImplementationGuideGlobalComponent(JsonObject json, ImplementationGuide owner) throws IOException, FHIRFormatError { 11219 ImplementationGuide.ImplementationGuideGlobalComponent res = new ImplementationGuide.ImplementationGuideGlobalComponent(); 11220 parseImplementationGuideImplementationGuideGlobalComponentProperties(json, owner, res); 11221 return res; 11222 } 11223 11224 protected void parseImplementationGuideImplementationGuideGlobalComponentProperties(JsonObject json, ImplementationGuide owner, ImplementationGuide.ImplementationGuideGlobalComponent res) throws IOException, FHIRFormatError { 11225 parseBackboneProperties(json, res); 11226 if (json.has("type")) 11227 res.setTypeElement(parseCode(json.get("type").getAsString())); 11228 if (json.has("_type")) 11229 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 11230 if (json.has("profile")) 11231 res.setProfile(parseReference(getJObject(json, "profile"))); 11232 } 11233 11234 protected ImplementationGuide.ImplementationGuidePageComponent parseImplementationGuideImplementationGuidePageComponent(JsonObject json, ImplementationGuide owner) throws IOException, FHIRFormatError { 11235 ImplementationGuide.ImplementationGuidePageComponent res = new ImplementationGuide.ImplementationGuidePageComponent(); 11236 parseImplementationGuideImplementationGuidePageComponentProperties(json, owner, res); 11237 return res; 11238 } 11239 11240 protected void parseImplementationGuideImplementationGuidePageComponentProperties(JsonObject json, ImplementationGuide owner, ImplementationGuide.ImplementationGuidePageComponent res) throws IOException, FHIRFormatError { 11241 parseBackboneProperties(json, res); 11242 if (json.has("source")) 11243 res.setSourceElement(parseUri(json.get("source").getAsString())); 11244 if (json.has("_source")) 11245 parseElementProperties(getJObject(json, "_source"), res.getSourceElement()); 11246 if (json.has("title")) 11247 res.setTitleElement(parseString(json.get("title").getAsString())); 11248 if (json.has("_title")) 11249 parseElementProperties(getJObject(json, "_title"), res.getTitleElement()); 11250 if (json.has("kind")) 11251 res.setKindElement(parseEnumeration(json.get("kind").getAsString(), ImplementationGuide.GuidePageKind.NULL, new ImplementationGuide.GuidePageKindEnumFactory())); 11252 if (json.has("_kind")) 11253 parseElementProperties(getJObject(json, "_kind"), res.getKindElement()); 11254 if (json.has("type")) { 11255 JsonArray array = json.getAsJsonArray("type"); 11256 for (int i = 0; i < array.size(); i++) { 11257 if (array.get(i).isJsonNull()) { 11258 res.getType().add(new CodeType()); 11259 } else { 11260 res.getType().add(parseCode(array.get(i).getAsString())); 11261 } 11262 } 11263 }; 11264 if (json.has("_type")) { 11265 JsonArray array = json.getAsJsonArray("_type"); 11266 for (int i = 0; i < array.size(); i++) { 11267 if (i == res.getType().size()) 11268 res.getType().add(parseCode(null)); 11269 if (array.get(i) instanceof JsonObject) 11270 parseElementProperties(array.get(i).getAsJsonObject(), res.getType().get(i)); 11271 } 11272 }; 11273 if (json.has("package")) { 11274 JsonArray array = json.getAsJsonArray("package"); 11275 for (int i = 0; i < array.size(); i++) { 11276 if (array.get(i).isJsonNull()) { 11277 res.getPackage().add(new StringType()); 11278 } else { 11279 res.getPackage().add(parseString(array.get(i).getAsString())); 11280 } 11281 } 11282 }; 11283 if (json.has("_package")) { 11284 JsonArray array = json.getAsJsonArray("_package"); 11285 for (int i = 0; i < array.size(); i++) { 11286 if (i == res.getPackage().size()) 11287 res.getPackage().add(parseString(null)); 11288 if (array.get(i) instanceof JsonObject) 11289 parseElementProperties(array.get(i).getAsJsonObject(), res.getPackage().get(i)); 11290 } 11291 }; 11292 if (json.has("format")) 11293 res.setFormatElement(parseCode(json.get("format").getAsString())); 11294 if (json.has("_format")) 11295 parseElementProperties(getJObject(json, "_format"), res.getFormatElement()); 11296 if (json.has("page")) { 11297 JsonArray array = json.getAsJsonArray("page"); 11298 for (int i = 0; i < array.size(); i++) { 11299 res.getPage().add(parseImplementationGuideImplementationGuidePageComponent(array.get(i).getAsJsonObject(), owner)); 11300 } 11301 }; 11302 } 11303 11304 protected Library parseLibrary(JsonObject json) throws IOException, FHIRFormatError { 11305 Library res = new Library(); 11306 parseLibraryProperties(json, res); 11307 return res; 11308 } 11309 11310 protected void parseLibraryProperties(JsonObject json, Library res) throws IOException, FHIRFormatError { 11311 parseDomainResourceProperties(json, res); 11312 if (json.has("url")) 11313 res.setUrlElement(parseUri(json.get("url").getAsString())); 11314 if (json.has("_url")) 11315 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 11316 if (json.has("identifier")) { 11317 JsonArray array = json.getAsJsonArray("identifier"); 11318 for (int i = 0; i < array.size(); i++) { 11319 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 11320 } 11321 }; 11322 if (json.has("version")) 11323 res.setVersionElement(parseString(json.get("version").getAsString())); 11324 if (json.has("_version")) 11325 parseElementProperties(getJObject(json, "_version"), res.getVersionElement()); 11326 if (json.has("name")) 11327 res.setNameElement(parseString(json.get("name").getAsString())); 11328 if (json.has("_name")) 11329 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 11330 if (json.has("title")) 11331 res.setTitleElement(parseString(json.get("title").getAsString())); 11332 if (json.has("_title")) 11333 parseElementProperties(getJObject(json, "_title"), res.getTitleElement()); 11334 if (json.has("status")) 11335 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Enumerations.PublicationStatus.NULL, new Enumerations.PublicationStatusEnumFactory())); 11336 if (json.has("_status")) 11337 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 11338 if (json.has("experimental")) 11339 res.setExperimentalElement(parseBoolean(json.get("experimental").getAsBoolean())); 11340 if (json.has("_experimental")) 11341 parseElementProperties(getJObject(json, "_experimental"), res.getExperimentalElement()); 11342 if (json.has("type")) 11343 res.setType(parseCodeableConcept(getJObject(json, "type"))); 11344 if (json.has("date")) 11345 res.setDateElement(parseDateTime(json.get("date").getAsString())); 11346 if (json.has("_date")) 11347 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 11348 if (json.has("publisher")) 11349 res.setPublisherElement(parseString(json.get("publisher").getAsString())); 11350 if (json.has("_publisher")) 11351 parseElementProperties(getJObject(json, "_publisher"), res.getPublisherElement()); 11352 if (json.has("description")) 11353 res.setDescriptionElement(parseMarkdown(json.get("description").getAsString())); 11354 if (json.has("_description")) 11355 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 11356 if (json.has("purpose")) 11357 res.setPurposeElement(parseMarkdown(json.get("purpose").getAsString())); 11358 if (json.has("_purpose")) 11359 parseElementProperties(getJObject(json, "_purpose"), res.getPurposeElement()); 11360 if (json.has("usage")) 11361 res.setUsageElement(parseString(json.get("usage").getAsString())); 11362 if (json.has("_usage")) 11363 parseElementProperties(getJObject(json, "_usage"), res.getUsageElement()); 11364 if (json.has("approvalDate")) 11365 res.setApprovalDateElement(parseDate(json.get("approvalDate").getAsString())); 11366 if (json.has("_approvalDate")) 11367 parseElementProperties(getJObject(json, "_approvalDate"), res.getApprovalDateElement()); 11368 if (json.has("lastReviewDate")) 11369 res.setLastReviewDateElement(parseDate(json.get("lastReviewDate").getAsString())); 11370 if (json.has("_lastReviewDate")) 11371 parseElementProperties(getJObject(json, "_lastReviewDate"), res.getLastReviewDateElement()); 11372 if (json.has("effectivePeriod")) 11373 res.setEffectivePeriod(parsePeriod(getJObject(json, "effectivePeriod"))); 11374 if (json.has("useContext")) { 11375 JsonArray array = json.getAsJsonArray("useContext"); 11376 for (int i = 0; i < array.size(); i++) { 11377 res.getUseContext().add(parseUsageContext(array.get(i).getAsJsonObject())); 11378 } 11379 }; 11380 if (json.has("jurisdiction")) { 11381 JsonArray array = json.getAsJsonArray("jurisdiction"); 11382 for (int i = 0; i < array.size(); i++) { 11383 res.getJurisdiction().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 11384 } 11385 }; 11386 if (json.has("topic")) { 11387 JsonArray array = json.getAsJsonArray("topic"); 11388 for (int i = 0; i < array.size(); i++) { 11389 res.getTopic().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 11390 } 11391 }; 11392 if (json.has("contributor")) { 11393 JsonArray array = json.getAsJsonArray("contributor"); 11394 for (int i = 0; i < array.size(); i++) { 11395 res.getContributor().add(parseContributor(array.get(i).getAsJsonObject())); 11396 } 11397 }; 11398 if (json.has("contact")) { 11399 JsonArray array = json.getAsJsonArray("contact"); 11400 for (int i = 0; i < array.size(); i++) { 11401 res.getContact().add(parseContactDetail(array.get(i).getAsJsonObject())); 11402 } 11403 }; 11404 if (json.has("copyright")) 11405 res.setCopyrightElement(parseMarkdown(json.get("copyright").getAsString())); 11406 if (json.has("_copyright")) 11407 parseElementProperties(getJObject(json, "_copyright"), res.getCopyrightElement()); 11408 if (json.has("relatedArtifact")) { 11409 JsonArray array = json.getAsJsonArray("relatedArtifact"); 11410 for (int i = 0; i < array.size(); i++) { 11411 res.getRelatedArtifact().add(parseRelatedArtifact(array.get(i).getAsJsonObject())); 11412 } 11413 }; 11414 if (json.has("parameter")) { 11415 JsonArray array = json.getAsJsonArray("parameter"); 11416 for (int i = 0; i < array.size(); i++) { 11417 res.getParameter().add(parseParameterDefinition(array.get(i).getAsJsonObject())); 11418 } 11419 }; 11420 if (json.has("dataRequirement")) { 11421 JsonArray array = json.getAsJsonArray("dataRequirement"); 11422 for (int i = 0; i < array.size(); i++) { 11423 res.getDataRequirement().add(parseDataRequirement(array.get(i).getAsJsonObject())); 11424 } 11425 }; 11426 if (json.has("content")) { 11427 JsonArray array = json.getAsJsonArray("content"); 11428 for (int i = 0; i < array.size(); i++) { 11429 res.getContent().add(parseAttachment(array.get(i).getAsJsonObject())); 11430 } 11431 }; 11432 } 11433 11434 protected Linkage parseLinkage(JsonObject json) throws IOException, FHIRFormatError { 11435 Linkage res = new Linkage(); 11436 parseLinkageProperties(json, res); 11437 return res; 11438 } 11439 11440 protected void parseLinkageProperties(JsonObject json, Linkage res) throws IOException, FHIRFormatError { 11441 parseDomainResourceProperties(json, res); 11442 if (json.has("active")) 11443 res.setActiveElement(parseBoolean(json.get("active").getAsBoolean())); 11444 if (json.has("_active")) 11445 parseElementProperties(getJObject(json, "_active"), res.getActiveElement()); 11446 if (json.has("author")) 11447 res.setAuthor(parseReference(getJObject(json, "author"))); 11448 if (json.has("item")) { 11449 JsonArray array = json.getAsJsonArray("item"); 11450 for (int i = 0; i < array.size(); i++) { 11451 res.getItem().add(parseLinkageLinkageItemComponent(array.get(i).getAsJsonObject(), res)); 11452 } 11453 }; 11454 } 11455 11456 protected Linkage.LinkageItemComponent parseLinkageLinkageItemComponent(JsonObject json, Linkage owner) throws IOException, FHIRFormatError { 11457 Linkage.LinkageItemComponent res = new Linkage.LinkageItemComponent(); 11458 parseLinkageLinkageItemComponentProperties(json, owner, res); 11459 return res; 11460 } 11461 11462 protected void parseLinkageLinkageItemComponentProperties(JsonObject json, Linkage owner, Linkage.LinkageItemComponent res) throws IOException, FHIRFormatError { 11463 parseBackboneProperties(json, res); 11464 if (json.has("type")) 11465 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), Linkage.LinkageType.NULL, new Linkage.LinkageTypeEnumFactory())); 11466 if (json.has("_type")) 11467 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 11468 if (json.has("resource")) 11469 res.setResource(parseReference(getJObject(json, "resource"))); 11470 } 11471 11472 protected ListResource parseListResource(JsonObject json) throws IOException, FHIRFormatError { 11473 ListResource res = new ListResource(); 11474 parseListResourceProperties(json, res); 11475 return res; 11476 } 11477 11478 protected void parseListResourceProperties(JsonObject json, ListResource res) throws IOException, FHIRFormatError { 11479 parseDomainResourceProperties(json, res); 11480 if (json.has("identifier")) { 11481 JsonArray array = json.getAsJsonArray("identifier"); 11482 for (int i = 0; i < array.size(); i++) { 11483 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 11484 } 11485 }; 11486 if (json.has("status")) 11487 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), ListResource.ListStatus.NULL, new ListResource.ListStatusEnumFactory())); 11488 if (json.has("_status")) 11489 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 11490 if (json.has("mode")) 11491 res.setModeElement(parseEnumeration(json.get("mode").getAsString(), ListResource.ListMode.NULL, new ListResource.ListModeEnumFactory())); 11492 if (json.has("_mode")) 11493 parseElementProperties(getJObject(json, "_mode"), res.getModeElement()); 11494 if (json.has("title")) 11495 res.setTitleElement(parseString(json.get("title").getAsString())); 11496 if (json.has("_title")) 11497 parseElementProperties(getJObject(json, "_title"), res.getTitleElement()); 11498 if (json.has("code")) 11499 res.setCode(parseCodeableConcept(getJObject(json, "code"))); 11500 if (json.has("subject")) 11501 res.setSubject(parseReference(getJObject(json, "subject"))); 11502 if (json.has("encounter")) 11503 res.setEncounter(parseReference(getJObject(json, "encounter"))); 11504 if (json.has("date")) 11505 res.setDateElement(parseDateTime(json.get("date").getAsString())); 11506 if (json.has("_date")) 11507 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 11508 if (json.has("source")) 11509 res.setSource(parseReference(getJObject(json, "source"))); 11510 if (json.has("orderedBy")) 11511 res.setOrderedBy(parseCodeableConcept(getJObject(json, "orderedBy"))); 11512 if (json.has("note")) { 11513 JsonArray array = json.getAsJsonArray("note"); 11514 for (int i = 0; i < array.size(); i++) { 11515 res.getNote().add(parseAnnotation(array.get(i).getAsJsonObject())); 11516 } 11517 }; 11518 if (json.has("entry")) { 11519 JsonArray array = json.getAsJsonArray("entry"); 11520 for (int i = 0; i < array.size(); i++) { 11521 res.getEntry().add(parseListResourceListEntryComponent(array.get(i).getAsJsonObject(), res)); 11522 } 11523 }; 11524 if (json.has("emptyReason")) 11525 res.setEmptyReason(parseCodeableConcept(getJObject(json, "emptyReason"))); 11526 } 11527 11528 protected ListResource.ListEntryComponent parseListResourceListEntryComponent(JsonObject json, ListResource owner) throws IOException, FHIRFormatError { 11529 ListResource.ListEntryComponent res = new ListResource.ListEntryComponent(); 11530 parseListResourceListEntryComponentProperties(json, owner, res); 11531 return res; 11532 } 11533 11534 protected void parseListResourceListEntryComponentProperties(JsonObject json, ListResource owner, ListResource.ListEntryComponent res) throws IOException, FHIRFormatError { 11535 parseBackboneProperties(json, res); 11536 if (json.has("flag")) 11537 res.setFlag(parseCodeableConcept(getJObject(json, "flag"))); 11538 if (json.has("deleted")) 11539 res.setDeletedElement(parseBoolean(json.get("deleted").getAsBoolean())); 11540 if (json.has("_deleted")) 11541 parseElementProperties(getJObject(json, "_deleted"), res.getDeletedElement()); 11542 if (json.has("date")) 11543 res.setDateElement(parseDateTime(json.get("date").getAsString())); 11544 if (json.has("_date")) 11545 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 11546 if (json.has("item")) 11547 res.setItem(parseReference(getJObject(json, "item"))); 11548 } 11549 11550 protected Location parseLocation(JsonObject json) throws IOException, FHIRFormatError { 11551 Location res = new Location(); 11552 parseLocationProperties(json, res); 11553 return res; 11554 } 11555 11556 protected void parseLocationProperties(JsonObject json, Location res) throws IOException, FHIRFormatError { 11557 parseDomainResourceProperties(json, res); 11558 if (json.has("identifier")) { 11559 JsonArray array = json.getAsJsonArray("identifier"); 11560 for (int i = 0; i < array.size(); i++) { 11561 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 11562 } 11563 }; 11564 if (json.has("status")) 11565 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Location.LocationStatus.NULL, new Location.LocationStatusEnumFactory())); 11566 if (json.has("_status")) 11567 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 11568 if (json.has("operationalStatus")) 11569 res.setOperationalStatus(parseCoding(getJObject(json, "operationalStatus"))); 11570 if (json.has("name")) 11571 res.setNameElement(parseString(json.get("name").getAsString())); 11572 if (json.has("_name")) 11573 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 11574 if (json.has("alias")) { 11575 JsonArray array = json.getAsJsonArray("alias"); 11576 for (int i = 0; i < array.size(); i++) { 11577 if (array.get(i).isJsonNull()) { 11578 res.getAlias().add(new StringType()); 11579 } else { 11580 res.getAlias().add(parseString(array.get(i).getAsString())); 11581 } 11582 } 11583 }; 11584 if (json.has("_alias")) { 11585 JsonArray array = json.getAsJsonArray("_alias"); 11586 for (int i = 0; i < array.size(); i++) { 11587 if (i == res.getAlias().size()) 11588 res.getAlias().add(parseString(null)); 11589 if (array.get(i) instanceof JsonObject) 11590 parseElementProperties(array.get(i).getAsJsonObject(), res.getAlias().get(i)); 11591 } 11592 }; 11593 if (json.has("description")) 11594 res.setDescriptionElement(parseString(json.get("description").getAsString())); 11595 if (json.has("_description")) 11596 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 11597 if (json.has("mode")) 11598 res.setModeElement(parseEnumeration(json.get("mode").getAsString(), Location.LocationMode.NULL, new Location.LocationModeEnumFactory())); 11599 if (json.has("_mode")) 11600 parseElementProperties(getJObject(json, "_mode"), res.getModeElement()); 11601 if (json.has("type")) 11602 res.setType(parseCodeableConcept(getJObject(json, "type"))); 11603 if (json.has("telecom")) { 11604 JsonArray array = json.getAsJsonArray("telecom"); 11605 for (int i = 0; i < array.size(); i++) { 11606 res.getTelecom().add(parseContactPoint(array.get(i).getAsJsonObject())); 11607 } 11608 }; 11609 if (json.has("address")) 11610 res.setAddress(parseAddress(getJObject(json, "address"))); 11611 if (json.has("physicalType")) 11612 res.setPhysicalType(parseCodeableConcept(getJObject(json, "physicalType"))); 11613 if (json.has("position")) 11614 res.setPosition(parseLocationLocationPositionComponent(getJObject(json, "position"), res)); 11615 if (json.has("managingOrganization")) 11616 res.setManagingOrganization(parseReference(getJObject(json, "managingOrganization"))); 11617 if (json.has("partOf")) 11618 res.setPartOf(parseReference(getJObject(json, "partOf"))); 11619 if (json.has("endpoint")) { 11620 JsonArray array = json.getAsJsonArray("endpoint"); 11621 for (int i = 0; i < array.size(); i++) { 11622 res.getEndpoint().add(parseReference(array.get(i).getAsJsonObject())); 11623 } 11624 }; 11625 } 11626 11627 protected Location.LocationPositionComponent parseLocationLocationPositionComponent(JsonObject json, Location owner) throws IOException, FHIRFormatError { 11628 Location.LocationPositionComponent res = new Location.LocationPositionComponent(); 11629 parseLocationLocationPositionComponentProperties(json, owner, res); 11630 return res; 11631 } 11632 11633 protected void parseLocationLocationPositionComponentProperties(JsonObject json, Location owner, Location.LocationPositionComponent res) throws IOException, FHIRFormatError { 11634 parseBackboneProperties(json, res); 11635 if (json.has("longitude")) 11636 res.setLongitudeElement(parseDecimal(json.get("longitude").getAsBigDecimal())); 11637 if (json.has("_longitude")) 11638 parseElementProperties(getJObject(json, "_longitude"), res.getLongitudeElement()); 11639 if (json.has("latitude")) 11640 res.setLatitudeElement(parseDecimal(json.get("latitude").getAsBigDecimal())); 11641 if (json.has("_latitude")) 11642 parseElementProperties(getJObject(json, "_latitude"), res.getLatitudeElement()); 11643 if (json.has("altitude")) 11644 res.setAltitudeElement(parseDecimal(json.get("altitude").getAsBigDecimal())); 11645 if (json.has("_altitude")) 11646 parseElementProperties(getJObject(json, "_altitude"), res.getAltitudeElement()); 11647 } 11648 11649 protected Measure parseMeasure(JsonObject json) throws IOException, FHIRFormatError { 11650 Measure res = new Measure(); 11651 parseMeasureProperties(json, res); 11652 return res; 11653 } 11654 11655 protected void parseMeasureProperties(JsonObject json, Measure res) throws IOException, FHIRFormatError { 11656 parseDomainResourceProperties(json, res); 11657 if (json.has("url")) 11658 res.setUrlElement(parseUri(json.get("url").getAsString())); 11659 if (json.has("_url")) 11660 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 11661 if (json.has("identifier")) { 11662 JsonArray array = json.getAsJsonArray("identifier"); 11663 for (int i = 0; i < array.size(); i++) { 11664 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 11665 } 11666 }; 11667 if (json.has("version")) 11668 res.setVersionElement(parseString(json.get("version").getAsString())); 11669 if (json.has("_version")) 11670 parseElementProperties(getJObject(json, "_version"), res.getVersionElement()); 11671 if (json.has("name")) 11672 res.setNameElement(parseString(json.get("name").getAsString())); 11673 if (json.has("_name")) 11674 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 11675 if (json.has("title")) 11676 res.setTitleElement(parseString(json.get("title").getAsString())); 11677 if (json.has("_title")) 11678 parseElementProperties(getJObject(json, "_title"), res.getTitleElement()); 11679 if (json.has("status")) 11680 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Enumerations.PublicationStatus.NULL, new Enumerations.PublicationStatusEnumFactory())); 11681 if (json.has("_status")) 11682 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 11683 if (json.has("experimental")) 11684 res.setExperimentalElement(parseBoolean(json.get("experimental").getAsBoolean())); 11685 if (json.has("_experimental")) 11686 parseElementProperties(getJObject(json, "_experimental"), res.getExperimentalElement()); 11687 if (json.has("date")) 11688 res.setDateElement(parseDateTime(json.get("date").getAsString())); 11689 if (json.has("_date")) 11690 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 11691 if (json.has("publisher")) 11692 res.setPublisherElement(parseString(json.get("publisher").getAsString())); 11693 if (json.has("_publisher")) 11694 parseElementProperties(getJObject(json, "_publisher"), res.getPublisherElement()); 11695 if (json.has("description")) 11696 res.setDescriptionElement(parseMarkdown(json.get("description").getAsString())); 11697 if (json.has("_description")) 11698 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 11699 if (json.has("purpose")) 11700 res.setPurposeElement(parseMarkdown(json.get("purpose").getAsString())); 11701 if (json.has("_purpose")) 11702 parseElementProperties(getJObject(json, "_purpose"), res.getPurposeElement()); 11703 if (json.has("usage")) 11704 res.setUsageElement(parseString(json.get("usage").getAsString())); 11705 if (json.has("_usage")) 11706 parseElementProperties(getJObject(json, "_usage"), res.getUsageElement()); 11707 if (json.has("approvalDate")) 11708 res.setApprovalDateElement(parseDate(json.get("approvalDate").getAsString())); 11709 if (json.has("_approvalDate")) 11710 parseElementProperties(getJObject(json, "_approvalDate"), res.getApprovalDateElement()); 11711 if (json.has("lastReviewDate")) 11712 res.setLastReviewDateElement(parseDate(json.get("lastReviewDate").getAsString())); 11713 if (json.has("_lastReviewDate")) 11714 parseElementProperties(getJObject(json, "_lastReviewDate"), res.getLastReviewDateElement()); 11715 if (json.has("effectivePeriod")) 11716 res.setEffectivePeriod(parsePeriod(getJObject(json, "effectivePeriod"))); 11717 if (json.has("useContext")) { 11718 JsonArray array = json.getAsJsonArray("useContext"); 11719 for (int i = 0; i < array.size(); i++) { 11720 res.getUseContext().add(parseUsageContext(array.get(i).getAsJsonObject())); 11721 } 11722 }; 11723 if (json.has("jurisdiction")) { 11724 JsonArray array = json.getAsJsonArray("jurisdiction"); 11725 for (int i = 0; i < array.size(); i++) { 11726 res.getJurisdiction().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 11727 } 11728 }; 11729 if (json.has("topic")) { 11730 JsonArray array = json.getAsJsonArray("topic"); 11731 for (int i = 0; i < array.size(); i++) { 11732 res.getTopic().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 11733 } 11734 }; 11735 if (json.has("contributor")) { 11736 JsonArray array = json.getAsJsonArray("contributor"); 11737 for (int i = 0; i < array.size(); i++) { 11738 res.getContributor().add(parseContributor(array.get(i).getAsJsonObject())); 11739 } 11740 }; 11741 if (json.has("contact")) { 11742 JsonArray array = json.getAsJsonArray("contact"); 11743 for (int i = 0; i < array.size(); i++) { 11744 res.getContact().add(parseContactDetail(array.get(i).getAsJsonObject())); 11745 } 11746 }; 11747 if (json.has("copyright")) 11748 res.setCopyrightElement(parseMarkdown(json.get("copyright").getAsString())); 11749 if (json.has("_copyright")) 11750 parseElementProperties(getJObject(json, "_copyright"), res.getCopyrightElement()); 11751 if (json.has("relatedArtifact")) { 11752 JsonArray array = json.getAsJsonArray("relatedArtifact"); 11753 for (int i = 0; i < array.size(); i++) { 11754 res.getRelatedArtifact().add(parseRelatedArtifact(array.get(i).getAsJsonObject())); 11755 } 11756 }; 11757 if (json.has("library")) { 11758 JsonArray array = json.getAsJsonArray("library"); 11759 for (int i = 0; i < array.size(); i++) { 11760 res.getLibrary().add(parseReference(array.get(i).getAsJsonObject())); 11761 } 11762 }; 11763 if (json.has("disclaimer")) 11764 res.setDisclaimerElement(parseMarkdown(json.get("disclaimer").getAsString())); 11765 if (json.has("_disclaimer")) 11766 parseElementProperties(getJObject(json, "_disclaimer"), res.getDisclaimerElement()); 11767 if (json.has("scoring")) 11768 res.setScoring(parseCodeableConcept(getJObject(json, "scoring"))); 11769 if (json.has("compositeScoring")) 11770 res.setCompositeScoring(parseCodeableConcept(getJObject(json, "compositeScoring"))); 11771 if (json.has("type")) { 11772 JsonArray array = json.getAsJsonArray("type"); 11773 for (int i = 0; i < array.size(); i++) { 11774 res.getType().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 11775 } 11776 }; 11777 if (json.has("riskAdjustment")) 11778 res.setRiskAdjustmentElement(parseString(json.get("riskAdjustment").getAsString())); 11779 if (json.has("_riskAdjustment")) 11780 parseElementProperties(getJObject(json, "_riskAdjustment"), res.getRiskAdjustmentElement()); 11781 if (json.has("rateAggregation")) 11782 res.setRateAggregationElement(parseString(json.get("rateAggregation").getAsString())); 11783 if (json.has("_rateAggregation")) 11784 parseElementProperties(getJObject(json, "_rateAggregation"), res.getRateAggregationElement()); 11785 if (json.has("rationale")) 11786 res.setRationaleElement(parseMarkdown(json.get("rationale").getAsString())); 11787 if (json.has("_rationale")) 11788 parseElementProperties(getJObject(json, "_rationale"), res.getRationaleElement()); 11789 if (json.has("clinicalRecommendationStatement")) 11790 res.setClinicalRecommendationStatementElement(parseMarkdown(json.get("clinicalRecommendationStatement").getAsString())); 11791 if (json.has("_clinicalRecommendationStatement")) 11792 parseElementProperties(getJObject(json, "_clinicalRecommendationStatement"), res.getClinicalRecommendationStatementElement()); 11793 if (json.has("improvementNotation")) 11794 res.setImprovementNotationElement(parseString(json.get("improvementNotation").getAsString())); 11795 if (json.has("_improvementNotation")) 11796 parseElementProperties(getJObject(json, "_improvementNotation"), res.getImprovementNotationElement()); 11797 if (json.has("definition")) { 11798 JsonArray array = json.getAsJsonArray("definition"); 11799 for (int i = 0; i < array.size(); i++) { 11800 if (array.get(i).isJsonNull()) { 11801 res.getDefinition().add(new MarkdownType()); 11802 } else { 11803 res.getDefinition().add(parseMarkdown(array.get(i).getAsString())); 11804 } 11805 } 11806 }; 11807 if (json.has("_definition")) { 11808 JsonArray array = json.getAsJsonArray("_definition"); 11809 for (int i = 0; i < array.size(); i++) { 11810 if (i == res.getDefinition().size()) 11811 res.getDefinition().add(parseMarkdown(null)); 11812 if (array.get(i) instanceof JsonObject) 11813 parseElementProperties(array.get(i).getAsJsonObject(), res.getDefinition().get(i)); 11814 } 11815 }; 11816 if (json.has("guidance")) 11817 res.setGuidanceElement(parseMarkdown(json.get("guidance").getAsString())); 11818 if (json.has("_guidance")) 11819 parseElementProperties(getJObject(json, "_guidance"), res.getGuidanceElement()); 11820 if (json.has("set")) 11821 res.setSetElement(parseString(json.get("set").getAsString())); 11822 if (json.has("_set")) 11823 parseElementProperties(getJObject(json, "_set"), res.getSetElement()); 11824 if (json.has("group")) { 11825 JsonArray array = json.getAsJsonArray("group"); 11826 for (int i = 0; i < array.size(); i++) { 11827 res.getGroup().add(parseMeasureMeasureGroupComponent(array.get(i).getAsJsonObject(), res)); 11828 } 11829 }; 11830 if (json.has("supplementalData")) { 11831 JsonArray array = json.getAsJsonArray("supplementalData"); 11832 for (int i = 0; i < array.size(); i++) { 11833 res.getSupplementalData().add(parseMeasureMeasureSupplementalDataComponent(array.get(i).getAsJsonObject(), res)); 11834 } 11835 }; 11836 } 11837 11838 protected Measure.MeasureGroupComponent parseMeasureMeasureGroupComponent(JsonObject json, Measure owner) throws IOException, FHIRFormatError { 11839 Measure.MeasureGroupComponent res = new Measure.MeasureGroupComponent(); 11840 parseMeasureMeasureGroupComponentProperties(json, owner, res); 11841 return res; 11842 } 11843 11844 protected void parseMeasureMeasureGroupComponentProperties(JsonObject json, Measure owner, Measure.MeasureGroupComponent res) throws IOException, FHIRFormatError { 11845 parseBackboneProperties(json, res); 11846 if (json.has("identifier")) 11847 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 11848 if (json.has("name")) 11849 res.setNameElement(parseString(json.get("name").getAsString())); 11850 if (json.has("_name")) 11851 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 11852 if (json.has("description")) 11853 res.setDescriptionElement(parseString(json.get("description").getAsString())); 11854 if (json.has("_description")) 11855 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 11856 if (json.has("population")) { 11857 JsonArray array = json.getAsJsonArray("population"); 11858 for (int i = 0; i < array.size(); i++) { 11859 res.getPopulation().add(parseMeasureMeasureGroupPopulationComponent(array.get(i).getAsJsonObject(), owner)); 11860 } 11861 }; 11862 if (json.has("stratifier")) { 11863 JsonArray array = json.getAsJsonArray("stratifier"); 11864 for (int i = 0; i < array.size(); i++) { 11865 res.getStratifier().add(parseMeasureMeasureGroupStratifierComponent(array.get(i).getAsJsonObject(), owner)); 11866 } 11867 }; 11868 } 11869 11870 protected Measure.MeasureGroupPopulationComponent parseMeasureMeasureGroupPopulationComponent(JsonObject json, Measure owner) throws IOException, FHIRFormatError { 11871 Measure.MeasureGroupPopulationComponent res = new Measure.MeasureGroupPopulationComponent(); 11872 parseMeasureMeasureGroupPopulationComponentProperties(json, owner, res); 11873 return res; 11874 } 11875 11876 protected void parseMeasureMeasureGroupPopulationComponentProperties(JsonObject json, Measure owner, Measure.MeasureGroupPopulationComponent res) throws IOException, FHIRFormatError { 11877 parseBackboneProperties(json, res); 11878 if (json.has("identifier")) 11879 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 11880 if (json.has("code")) 11881 res.setCode(parseCodeableConcept(getJObject(json, "code"))); 11882 if (json.has("name")) 11883 res.setNameElement(parseString(json.get("name").getAsString())); 11884 if (json.has("_name")) 11885 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 11886 if (json.has("description")) 11887 res.setDescriptionElement(parseString(json.get("description").getAsString())); 11888 if (json.has("_description")) 11889 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 11890 if (json.has("criteria")) 11891 res.setCriteriaElement(parseString(json.get("criteria").getAsString())); 11892 if (json.has("_criteria")) 11893 parseElementProperties(getJObject(json, "_criteria"), res.getCriteriaElement()); 11894 } 11895 11896 protected Measure.MeasureGroupStratifierComponent parseMeasureMeasureGroupStratifierComponent(JsonObject json, Measure owner) throws IOException, FHIRFormatError { 11897 Measure.MeasureGroupStratifierComponent res = new Measure.MeasureGroupStratifierComponent(); 11898 parseMeasureMeasureGroupStratifierComponentProperties(json, owner, res); 11899 return res; 11900 } 11901 11902 protected void parseMeasureMeasureGroupStratifierComponentProperties(JsonObject json, Measure owner, Measure.MeasureGroupStratifierComponent res) throws IOException, FHIRFormatError { 11903 parseBackboneProperties(json, res); 11904 if (json.has("identifier")) 11905 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 11906 if (json.has("criteria")) 11907 res.setCriteriaElement(parseString(json.get("criteria").getAsString())); 11908 if (json.has("_criteria")) 11909 parseElementProperties(getJObject(json, "_criteria"), res.getCriteriaElement()); 11910 if (json.has("path")) 11911 res.setPathElement(parseString(json.get("path").getAsString())); 11912 if (json.has("_path")) 11913 parseElementProperties(getJObject(json, "_path"), res.getPathElement()); 11914 } 11915 11916 protected Measure.MeasureSupplementalDataComponent parseMeasureMeasureSupplementalDataComponent(JsonObject json, Measure owner) throws IOException, FHIRFormatError { 11917 Measure.MeasureSupplementalDataComponent res = new Measure.MeasureSupplementalDataComponent(); 11918 parseMeasureMeasureSupplementalDataComponentProperties(json, owner, res); 11919 return res; 11920 } 11921 11922 protected void parseMeasureMeasureSupplementalDataComponentProperties(JsonObject json, Measure owner, Measure.MeasureSupplementalDataComponent res) throws IOException, FHIRFormatError { 11923 parseBackboneProperties(json, res); 11924 if (json.has("identifier")) 11925 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 11926 if (json.has("usage")) { 11927 JsonArray array = json.getAsJsonArray("usage"); 11928 for (int i = 0; i < array.size(); i++) { 11929 res.getUsage().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 11930 } 11931 }; 11932 if (json.has("criteria")) 11933 res.setCriteriaElement(parseString(json.get("criteria").getAsString())); 11934 if (json.has("_criteria")) 11935 parseElementProperties(getJObject(json, "_criteria"), res.getCriteriaElement()); 11936 if (json.has("path")) 11937 res.setPathElement(parseString(json.get("path").getAsString())); 11938 if (json.has("_path")) 11939 parseElementProperties(getJObject(json, "_path"), res.getPathElement()); 11940 } 11941 11942 protected MeasureReport parseMeasureReport(JsonObject json) throws IOException, FHIRFormatError { 11943 MeasureReport res = new MeasureReport(); 11944 parseMeasureReportProperties(json, res); 11945 return res; 11946 } 11947 11948 protected void parseMeasureReportProperties(JsonObject json, MeasureReport res) throws IOException, FHIRFormatError { 11949 parseDomainResourceProperties(json, res); 11950 if (json.has("identifier")) 11951 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 11952 if (json.has("status")) 11953 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), MeasureReport.MeasureReportStatus.NULL, new MeasureReport.MeasureReportStatusEnumFactory())); 11954 if (json.has("_status")) 11955 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 11956 if (json.has("type")) 11957 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), MeasureReport.MeasureReportType.NULL, new MeasureReport.MeasureReportTypeEnumFactory())); 11958 if (json.has("_type")) 11959 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 11960 if (json.has("measure")) 11961 res.setMeasure(parseReference(getJObject(json, "measure"))); 11962 if (json.has("patient")) 11963 res.setPatient(parseReference(getJObject(json, "patient"))); 11964 if (json.has("date")) 11965 res.setDateElement(parseDateTime(json.get("date").getAsString())); 11966 if (json.has("_date")) 11967 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 11968 if (json.has("reportingOrganization")) 11969 res.setReportingOrganization(parseReference(getJObject(json, "reportingOrganization"))); 11970 if (json.has("period")) 11971 res.setPeriod(parsePeriod(getJObject(json, "period"))); 11972 if (json.has("group")) { 11973 JsonArray array = json.getAsJsonArray("group"); 11974 for (int i = 0; i < array.size(); i++) { 11975 res.getGroup().add(parseMeasureReportMeasureReportGroupComponent(array.get(i).getAsJsonObject(), res)); 11976 } 11977 }; 11978 if (json.has("evaluatedResources")) 11979 res.setEvaluatedResources(parseReference(getJObject(json, "evaluatedResources"))); 11980 } 11981 11982 protected MeasureReport.MeasureReportGroupComponent parseMeasureReportMeasureReportGroupComponent(JsonObject json, MeasureReport owner) throws IOException, FHIRFormatError { 11983 MeasureReport.MeasureReportGroupComponent res = new MeasureReport.MeasureReportGroupComponent(); 11984 parseMeasureReportMeasureReportGroupComponentProperties(json, owner, res); 11985 return res; 11986 } 11987 11988 protected void parseMeasureReportMeasureReportGroupComponentProperties(JsonObject json, MeasureReport owner, MeasureReport.MeasureReportGroupComponent res) throws IOException, FHIRFormatError { 11989 parseBackboneProperties(json, res); 11990 if (json.has("identifier")) 11991 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 11992 if (json.has("population")) { 11993 JsonArray array = json.getAsJsonArray("population"); 11994 for (int i = 0; i < array.size(); i++) { 11995 res.getPopulation().add(parseMeasureReportMeasureReportGroupPopulationComponent(array.get(i).getAsJsonObject(), owner)); 11996 } 11997 }; 11998 if (json.has("measureScore")) 11999 res.setMeasureScoreElement(parseDecimal(json.get("measureScore").getAsBigDecimal())); 12000 if (json.has("_measureScore")) 12001 parseElementProperties(getJObject(json, "_measureScore"), res.getMeasureScoreElement()); 12002 if (json.has("stratifier")) { 12003 JsonArray array = json.getAsJsonArray("stratifier"); 12004 for (int i = 0; i < array.size(); i++) { 12005 res.getStratifier().add(parseMeasureReportMeasureReportGroupStratifierComponent(array.get(i).getAsJsonObject(), owner)); 12006 } 12007 }; 12008 } 12009 12010 protected MeasureReport.MeasureReportGroupPopulationComponent parseMeasureReportMeasureReportGroupPopulationComponent(JsonObject json, MeasureReport owner) throws IOException, FHIRFormatError { 12011 MeasureReport.MeasureReportGroupPopulationComponent res = new MeasureReport.MeasureReportGroupPopulationComponent(); 12012 parseMeasureReportMeasureReportGroupPopulationComponentProperties(json, owner, res); 12013 return res; 12014 } 12015 12016 protected void parseMeasureReportMeasureReportGroupPopulationComponentProperties(JsonObject json, MeasureReport owner, MeasureReport.MeasureReportGroupPopulationComponent res) throws IOException, FHIRFormatError { 12017 parseBackboneProperties(json, res); 12018 if (json.has("identifier")) 12019 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 12020 if (json.has("code")) 12021 res.setCode(parseCodeableConcept(getJObject(json, "code"))); 12022 if (json.has("count")) 12023 res.setCountElement(parseInteger(json.get("count").getAsLong())); 12024 if (json.has("_count")) 12025 parseElementProperties(getJObject(json, "_count"), res.getCountElement()); 12026 if (json.has("patients")) 12027 res.setPatients(parseReference(getJObject(json, "patients"))); 12028 } 12029 12030 protected MeasureReport.MeasureReportGroupStratifierComponent parseMeasureReportMeasureReportGroupStratifierComponent(JsonObject json, MeasureReport owner) throws IOException, FHIRFormatError { 12031 MeasureReport.MeasureReportGroupStratifierComponent res = new MeasureReport.MeasureReportGroupStratifierComponent(); 12032 parseMeasureReportMeasureReportGroupStratifierComponentProperties(json, owner, res); 12033 return res; 12034 } 12035 12036 protected void parseMeasureReportMeasureReportGroupStratifierComponentProperties(JsonObject json, MeasureReport owner, MeasureReport.MeasureReportGroupStratifierComponent res) throws IOException, FHIRFormatError { 12037 parseBackboneProperties(json, res); 12038 if (json.has("identifier")) 12039 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 12040 if (json.has("stratum")) { 12041 JsonArray array = json.getAsJsonArray("stratum"); 12042 for (int i = 0; i < array.size(); i++) { 12043 res.getStratum().add(parseMeasureReportStratifierGroupComponent(array.get(i).getAsJsonObject(), owner)); 12044 } 12045 }; 12046 } 12047 12048 protected MeasureReport.StratifierGroupComponent parseMeasureReportStratifierGroupComponent(JsonObject json, MeasureReport owner) throws IOException, FHIRFormatError { 12049 MeasureReport.StratifierGroupComponent res = new MeasureReport.StratifierGroupComponent(); 12050 parseMeasureReportStratifierGroupComponentProperties(json, owner, res); 12051 return res; 12052 } 12053 12054 protected void parseMeasureReportStratifierGroupComponentProperties(JsonObject json, MeasureReport owner, MeasureReport.StratifierGroupComponent res) throws IOException, FHIRFormatError { 12055 parseBackboneProperties(json, res); 12056 if (json.has("value")) 12057 res.setValueElement(parseString(json.get("value").getAsString())); 12058 if (json.has("_value")) 12059 parseElementProperties(getJObject(json, "_value"), res.getValueElement()); 12060 if (json.has("population")) { 12061 JsonArray array = json.getAsJsonArray("population"); 12062 for (int i = 0; i < array.size(); i++) { 12063 res.getPopulation().add(parseMeasureReportStratifierGroupPopulationComponent(array.get(i).getAsJsonObject(), owner)); 12064 } 12065 }; 12066 if (json.has("measureScore")) 12067 res.setMeasureScoreElement(parseDecimal(json.get("measureScore").getAsBigDecimal())); 12068 if (json.has("_measureScore")) 12069 parseElementProperties(getJObject(json, "_measureScore"), res.getMeasureScoreElement()); 12070 } 12071 12072 protected MeasureReport.StratifierGroupPopulationComponent parseMeasureReportStratifierGroupPopulationComponent(JsonObject json, MeasureReport owner) throws IOException, FHIRFormatError { 12073 MeasureReport.StratifierGroupPopulationComponent res = new MeasureReport.StratifierGroupPopulationComponent(); 12074 parseMeasureReportStratifierGroupPopulationComponentProperties(json, owner, res); 12075 return res; 12076 } 12077 12078 protected void parseMeasureReportStratifierGroupPopulationComponentProperties(JsonObject json, MeasureReport owner, MeasureReport.StratifierGroupPopulationComponent res) throws IOException, FHIRFormatError { 12079 parseBackboneProperties(json, res); 12080 if (json.has("identifier")) 12081 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 12082 if (json.has("code")) 12083 res.setCode(parseCodeableConcept(getJObject(json, "code"))); 12084 if (json.has("count")) 12085 res.setCountElement(parseInteger(json.get("count").getAsLong())); 12086 if (json.has("_count")) 12087 parseElementProperties(getJObject(json, "_count"), res.getCountElement()); 12088 if (json.has("patients")) 12089 res.setPatients(parseReference(getJObject(json, "patients"))); 12090 } 12091 12092 protected Media parseMedia(JsonObject json) throws IOException, FHIRFormatError { 12093 Media res = new Media(); 12094 parseMediaProperties(json, res); 12095 return res; 12096 } 12097 12098 protected void parseMediaProperties(JsonObject json, Media res) throws IOException, FHIRFormatError { 12099 parseDomainResourceProperties(json, res); 12100 if (json.has("identifier")) { 12101 JsonArray array = json.getAsJsonArray("identifier"); 12102 for (int i = 0; i < array.size(); i++) { 12103 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 12104 } 12105 }; 12106 if (json.has("basedOn")) { 12107 JsonArray array = json.getAsJsonArray("basedOn"); 12108 for (int i = 0; i < array.size(); i++) { 12109 res.getBasedOn().add(parseReference(array.get(i).getAsJsonObject())); 12110 } 12111 }; 12112 if (json.has("type")) 12113 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), Media.DigitalMediaType.NULL, new Media.DigitalMediaTypeEnumFactory())); 12114 if (json.has("_type")) 12115 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 12116 if (json.has("subtype")) 12117 res.setSubtype(parseCodeableConcept(getJObject(json, "subtype"))); 12118 if (json.has("view")) 12119 res.setView(parseCodeableConcept(getJObject(json, "view"))); 12120 if (json.has("subject")) 12121 res.setSubject(parseReference(getJObject(json, "subject"))); 12122 if (json.has("context")) 12123 res.setContext(parseReference(getJObject(json, "context"))); 12124 Type occurrence = parseType("occurrence", json); 12125 if (occurrence != null) 12126 res.setOccurrence(occurrence); 12127 if (json.has("operator")) 12128 res.setOperator(parseReference(getJObject(json, "operator"))); 12129 if (json.has("reasonCode")) { 12130 JsonArray array = json.getAsJsonArray("reasonCode"); 12131 for (int i = 0; i < array.size(); i++) { 12132 res.getReasonCode().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 12133 } 12134 }; 12135 if (json.has("bodySite")) 12136 res.setBodySite(parseCodeableConcept(getJObject(json, "bodySite"))); 12137 if (json.has("device")) 12138 res.setDevice(parseReference(getJObject(json, "device"))); 12139 if (json.has("height")) 12140 res.setHeightElement(parsePositiveInt(json.get("height").getAsString())); 12141 if (json.has("_height")) 12142 parseElementProperties(getJObject(json, "_height"), res.getHeightElement()); 12143 if (json.has("width")) 12144 res.setWidthElement(parsePositiveInt(json.get("width").getAsString())); 12145 if (json.has("_width")) 12146 parseElementProperties(getJObject(json, "_width"), res.getWidthElement()); 12147 if (json.has("frames")) 12148 res.setFramesElement(parsePositiveInt(json.get("frames").getAsString())); 12149 if (json.has("_frames")) 12150 parseElementProperties(getJObject(json, "_frames"), res.getFramesElement()); 12151 if (json.has("duration")) 12152 res.setDurationElement(parseUnsignedInt(json.get("duration").getAsString())); 12153 if (json.has("_duration")) 12154 parseElementProperties(getJObject(json, "_duration"), res.getDurationElement()); 12155 if (json.has("content")) 12156 res.setContent(parseAttachment(getJObject(json, "content"))); 12157 if (json.has("note")) { 12158 JsonArray array = json.getAsJsonArray("note"); 12159 for (int i = 0; i < array.size(); i++) { 12160 res.getNote().add(parseAnnotation(array.get(i).getAsJsonObject())); 12161 } 12162 }; 12163 } 12164 12165 protected Medication parseMedication(JsonObject json) throws IOException, FHIRFormatError { 12166 Medication res = new Medication(); 12167 parseMedicationProperties(json, res); 12168 return res; 12169 } 12170 12171 protected void parseMedicationProperties(JsonObject json, Medication res) throws IOException, FHIRFormatError { 12172 parseDomainResourceProperties(json, res); 12173 if (json.has("code")) 12174 res.setCode(parseCodeableConcept(getJObject(json, "code"))); 12175 if (json.has("status")) 12176 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Medication.MedicationStatus.NULL, new Medication.MedicationStatusEnumFactory())); 12177 if (json.has("_status")) 12178 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 12179 if (json.has("isBrand")) 12180 res.setIsBrandElement(parseBoolean(json.get("isBrand").getAsBoolean())); 12181 if (json.has("_isBrand")) 12182 parseElementProperties(getJObject(json, "_isBrand"), res.getIsBrandElement()); 12183 if (json.has("isOverTheCounter")) 12184 res.setIsOverTheCounterElement(parseBoolean(json.get("isOverTheCounter").getAsBoolean())); 12185 if (json.has("_isOverTheCounter")) 12186 parseElementProperties(getJObject(json, "_isOverTheCounter"), res.getIsOverTheCounterElement()); 12187 if (json.has("manufacturer")) 12188 res.setManufacturer(parseReference(getJObject(json, "manufacturer"))); 12189 if (json.has("form")) 12190 res.setForm(parseCodeableConcept(getJObject(json, "form"))); 12191 if (json.has("ingredient")) { 12192 JsonArray array = json.getAsJsonArray("ingredient"); 12193 for (int i = 0; i < array.size(); i++) { 12194 res.getIngredient().add(parseMedicationMedicationIngredientComponent(array.get(i).getAsJsonObject(), res)); 12195 } 12196 }; 12197 if (json.has("package")) 12198 res.setPackage(parseMedicationMedicationPackageComponent(getJObject(json, "package"), res)); 12199 if (json.has("image")) { 12200 JsonArray array = json.getAsJsonArray("image"); 12201 for (int i = 0; i < array.size(); i++) { 12202 res.getImage().add(parseAttachment(array.get(i).getAsJsonObject())); 12203 } 12204 }; 12205 } 12206 12207 protected Medication.MedicationIngredientComponent parseMedicationMedicationIngredientComponent(JsonObject json, Medication owner) throws IOException, FHIRFormatError { 12208 Medication.MedicationIngredientComponent res = new Medication.MedicationIngredientComponent(); 12209 parseMedicationMedicationIngredientComponentProperties(json, owner, res); 12210 return res; 12211 } 12212 12213 protected void parseMedicationMedicationIngredientComponentProperties(JsonObject json, Medication owner, Medication.MedicationIngredientComponent res) throws IOException, FHIRFormatError { 12214 parseBackboneProperties(json, res); 12215 Type item = parseType("item", json); 12216 if (item != null) 12217 res.setItem(item); 12218 if (json.has("isActive")) 12219 res.setIsActiveElement(parseBoolean(json.get("isActive").getAsBoolean())); 12220 if (json.has("_isActive")) 12221 parseElementProperties(getJObject(json, "_isActive"), res.getIsActiveElement()); 12222 if (json.has("amount")) 12223 res.setAmount(parseRatio(getJObject(json, "amount"))); 12224 } 12225 12226 protected Medication.MedicationPackageComponent parseMedicationMedicationPackageComponent(JsonObject json, Medication owner) throws IOException, FHIRFormatError { 12227 Medication.MedicationPackageComponent res = new Medication.MedicationPackageComponent(); 12228 parseMedicationMedicationPackageComponentProperties(json, owner, res); 12229 return res; 12230 } 12231 12232 protected void parseMedicationMedicationPackageComponentProperties(JsonObject json, Medication owner, Medication.MedicationPackageComponent res) throws IOException, FHIRFormatError { 12233 parseBackboneProperties(json, res); 12234 if (json.has("container")) 12235 res.setContainer(parseCodeableConcept(getJObject(json, "container"))); 12236 if (json.has("content")) { 12237 JsonArray array = json.getAsJsonArray("content"); 12238 for (int i = 0; i < array.size(); i++) { 12239 res.getContent().add(parseMedicationMedicationPackageContentComponent(array.get(i).getAsJsonObject(), owner)); 12240 } 12241 }; 12242 if (json.has("batch")) { 12243 JsonArray array = json.getAsJsonArray("batch"); 12244 for (int i = 0; i < array.size(); i++) { 12245 res.getBatch().add(parseMedicationMedicationPackageBatchComponent(array.get(i).getAsJsonObject(), owner)); 12246 } 12247 }; 12248 } 12249 12250 protected Medication.MedicationPackageContentComponent parseMedicationMedicationPackageContentComponent(JsonObject json, Medication owner) throws IOException, FHIRFormatError { 12251 Medication.MedicationPackageContentComponent res = new Medication.MedicationPackageContentComponent(); 12252 parseMedicationMedicationPackageContentComponentProperties(json, owner, res); 12253 return res; 12254 } 12255 12256 protected void parseMedicationMedicationPackageContentComponentProperties(JsonObject json, Medication owner, Medication.MedicationPackageContentComponent res) throws IOException, FHIRFormatError { 12257 parseBackboneProperties(json, res); 12258 Type item = parseType("item", json); 12259 if (item != null) 12260 res.setItem(item); 12261 if (json.has("amount")) 12262 res.setAmount(parseSimpleQuantity(getJObject(json, "amount"))); 12263 } 12264 12265 protected Medication.MedicationPackageBatchComponent parseMedicationMedicationPackageBatchComponent(JsonObject json, Medication owner) throws IOException, FHIRFormatError { 12266 Medication.MedicationPackageBatchComponent res = new Medication.MedicationPackageBatchComponent(); 12267 parseMedicationMedicationPackageBatchComponentProperties(json, owner, res); 12268 return res; 12269 } 12270 12271 protected void parseMedicationMedicationPackageBatchComponentProperties(JsonObject json, Medication owner, Medication.MedicationPackageBatchComponent res) throws IOException, FHIRFormatError { 12272 parseBackboneProperties(json, res); 12273 if (json.has("lotNumber")) 12274 res.setLotNumberElement(parseString(json.get("lotNumber").getAsString())); 12275 if (json.has("_lotNumber")) 12276 parseElementProperties(getJObject(json, "_lotNumber"), res.getLotNumberElement()); 12277 if (json.has("expirationDate")) 12278 res.setExpirationDateElement(parseDateTime(json.get("expirationDate").getAsString())); 12279 if (json.has("_expirationDate")) 12280 parseElementProperties(getJObject(json, "_expirationDate"), res.getExpirationDateElement()); 12281 } 12282 12283 protected MedicationAdministration parseMedicationAdministration(JsonObject json) throws IOException, FHIRFormatError { 12284 MedicationAdministration res = new MedicationAdministration(); 12285 parseMedicationAdministrationProperties(json, res); 12286 return res; 12287 } 12288 12289 protected void parseMedicationAdministrationProperties(JsonObject json, MedicationAdministration res) throws IOException, FHIRFormatError { 12290 parseDomainResourceProperties(json, res); 12291 if (json.has("identifier")) { 12292 JsonArray array = json.getAsJsonArray("identifier"); 12293 for (int i = 0; i < array.size(); i++) { 12294 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 12295 } 12296 }; 12297 if (json.has("definition")) { 12298 JsonArray array = json.getAsJsonArray("definition"); 12299 for (int i = 0; i < array.size(); i++) { 12300 res.getDefinition().add(parseReference(array.get(i).getAsJsonObject())); 12301 } 12302 }; 12303 if (json.has("partOf")) { 12304 JsonArray array = json.getAsJsonArray("partOf"); 12305 for (int i = 0; i < array.size(); i++) { 12306 res.getPartOf().add(parseReference(array.get(i).getAsJsonObject())); 12307 } 12308 }; 12309 if (json.has("status")) 12310 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), MedicationAdministration.MedicationAdministrationStatus.NULL, new MedicationAdministration.MedicationAdministrationStatusEnumFactory())); 12311 if (json.has("_status")) 12312 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 12313 if (json.has("category")) 12314 res.setCategory(parseCodeableConcept(getJObject(json, "category"))); 12315 Type medication = parseType("medication", json); 12316 if (medication != null) 12317 res.setMedication(medication); 12318 if (json.has("subject")) 12319 res.setSubject(parseReference(getJObject(json, "subject"))); 12320 if (json.has("context")) 12321 res.setContext(parseReference(getJObject(json, "context"))); 12322 if (json.has("supportingInformation")) { 12323 JsonArray array = json.getAsJsonArray("supportingInformation"); 12324 for (int i = 0; i < array.size(); i++) { 12325 res.getSupportingInformation().add(parseReference(array.get(i).getAsJsonObject())); 12326 } 12327 }; 12328 Type effective = parseType("effective", json); 12329 if (effective != null) 12330 res.setEffective(effective); 12331 if (json.has("performer")) { 12332 JsonArray array = json.getAsJsonArray("performer"); 12333 for (int i = 0; i < array.size(); i++) { 12334 res.getPerformer().add(parseMedicationAdministrationMedicationAdministrationPerformerComponent(array.get(i).getAsJsonObject(), res)); 12335 } 12336 }; 12337 if (json.has("notGiven")) 12338 res.setNotGivenElement(parseBoolean(json.get("notGiven").getAsBoolean())); 12339 if (json.has("_notGiven")) 12340 parseElementProperties(getJObject(json, "_notGiven"), res.getNotGivenElement()); 12341 if (json.has("reasonNotGiven")) { 12342 JsonArray array = json.getAsJsonArray("reasonNotGiven"); 12343 for (int i = 0; i < array.size(); i++) { 12344 res.getReasonNotGiven().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 12345 } 12346 }; 12347 if (json.has("reasonCode")) { 12348 JsonArray array = json.getAsJsonArray("reasonCode"); 12349 for (int i = 0; i < array.size(); i++) { 12350 res.getReasonCode().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 12351 } 12352 }; 12353 if (json.has("reasonReference")) { 12354 JsonArray array = json.getAsJsonArray("reasonReference"); 12355 for (int i = 0; i < array.size(); i++) { 12356 res.getReasonReference().add(parseReference(array.get(i).getAsJsonObject())); 12357 } 12358 }; 12359 if (json.has("prescription")) 12360 res.setPrescription(parseReference(getJObject(json, "prescription"))); 12361 if (json.has("device")) { 12362 JsonArray array = json.getAsJsonArray("device"); 12363 for (int i = 0; i < array.size(); i++) { 12364 res.getDevice().add(parseReference(array.get(i).getAsJsonObject())); 12365 } 12366 }; 12367 if (json.has("note")) { 12368 JsonArray array = json.getAsJsonArray("note"); 12369 for (int i = 0; i < array.size(); i++) { 12370 res.getNote().add(parseAnnotation(array.get(i).getAsJsonObject())); 12371 } 12372 }; 12373 if (json.has("dosage")) 12374 res.setDosage(parseMedicationAdministrationMedicationAdministrationDosageComponent(getJObject(json, "dosage"), res)); 12375 if (json.has("eventHistory")) { 12376 JsonArray array = json.getAsJsonArray("eventHistory"); 12377 for (int i = 0; i < array.size(); i++) { 12378 res.getEventHistory().add(parseReference(array.get(i).getAsJsonObject())); 12379 } 12380 }; 12381 } 12382 12383 protected MedicationAdministration.MedicationAdministrationPerformerComponent parseMedicationAdministrationMedicationAdministrationPerformerComponent(JsonObject json, MedicationAdministration owner) throws IOException, FHIRFormatError { 12384 MedicationAdministration.MedicationAdministrationPerformerComponent res = new MedicationAdministration.MedicationAdministrationPerformerComponent(); 12385 parseMedicationAdministrationMedicationAdministrationPerformerComponentProperties(json, owner, res); 12386 return res; 12387 } 12388 12389 protected void parseMedicationAdministrationMedicationAdministrationPerformerComponentProperties(JsonObject json, MedicationAdministration owner, MedicationAdministration.MedicationAdministrationPerformerComponent res) throws IOException, FHIRFormatError { 12390 parseBackboneProperties(json, res); 12391 if (json.has("actor")) 12392 res.setActor(parseReference(getJObject(json, "actor"))); 12393 if (json.has("onBehalfOf")) 12394 res.setOnBehalfOf(parseReference(getJObject(json, "onBehalfOf"))); 12395 } 12396 12397 protected MedicationAdministration.MedicationAdministrationDosageComponent parseMedicationAdministrationMedicationAdministrationDosageComponent(JsonObject json, MedicationAdministration owner) throws IOException, FHIRFormatError { 12398 MedicationAdministration.MedicationAdministrationDosageComponent res = new MedicationAdministration.MedicationAdministrationDosageComponent(); 12399 parseMedicationAdministrationMedicationAdministrationDosageComponentProperties(json, owner, res); 12400 return res; 12401 } 12402 12403 protected void parseMedicationAdministrationMedicationAdministrationDosageComponentProperties(JsonObject json, MedicationAdministration owner, MedicationAdministration.MedicationAdministrationDosageComponent res) throws IOException, FHIRFormatError { 12404 parseBackboneProperties(json, res); 12405 if (json.has("text")) 12406 res.setTextElement(parseString(json.get("text").getAsString())); 12407 if (json.has("_text")) 12408 parseElementProperties(getJObject(json, "_text"), res.getTextElement()); 12409 if (json.has("site")) 12410 res.setSite(parseCodeableConcept(getJObject(json, "site"))); 12411 if (json.has("route")) 12412 res.setRoute(parseCodeableConcept(getJObject(json, "route"))); 12413 if (json.has("method")) 12414 res.setMethod(parseCodeableConcept(getJObject(json, "method"))); 12415 if (json.has("dose")) 12416 res.setDose(parseSimpleQuantity(getJObject(json, "dose"))); 12417 Type rate = parseType("rate", json); 12418 if (rate != null) 12419 res.setRate(rate); 12420 } 12421 12422 protected MedicationDispense parseMedicationDispense(JsonObject json) throws IOException, FHIRFormatError { 12423 MedicationDispense res = new MedicationDispense(); 12424 parseMedicationDispenseProperties(json, res); 12425 return res; 12426 } 12427 12428 protected void parseMedicationDispenseProperties(JsonObject json, MedicationDispense res) throws IOException, FHIRFormatError { 12429 parseDomainResourceProperties(json, res); 12430 if (json.has("identifier")) { 12431 JsonArray array = json.getAsJsonArray("identifier"); 12432 for (int i = 0; i < array.size(); i++) { 12433 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 12434 } 12435 }; 12436 if (json.has("partOf")) { 12437 JsonArray array = json.getAsJsonArray("partOf"); 12438 for (int i = 0; i < array.size(); i++) { 12439 res.getPartOf().add(parseReference(array.get(i).getAsJsonObject())); 12440 } 12441 }; 12442 if (json.has("status")) 12443 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), MedicationDispense.MedicationDispenseStatus.NULL, new MedicationDispense.MedicationDispenseStatusEnumFactory())); 12444 if (json.has("_status")) 12445 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 12446 if (json.has("category")) 12447 res.setCategory(parseCodeableConcept(getJObject(json, "category"))); 12448 Type medication = parseType("medication", json); 12449 if (medication != null) 12450 res.setMedication(medication); 12451 if (json.has("subject")) 12452 res.setSubject(parseReference(getJObject(json, "subject"))); 12453 if (json.has("context")) 12454 res.setContext(parseReference(getJObject(json, "context"))); 12455 if (json.has("supportingInformation")) { 12456 JsonArray array = json.getAsJsonArray("supportingInformation"); 12457 for (int i = 0; i < array.size(); i++) { 12458 res.getSupportingInformation().add(parseReference(array.get(i).getAsJsonObject())); 12459 } 12460 }; 12461 if (json.has("performer")) { 12462 JsonArray array = json.getAsJsonArray("performer"); 12463 for (int i = 0; i < array.size(); i++) { 12464 res.getPerformer().add(parseMedicationDispenseMedicationDispensePerformerComponent(array.get(i).getAsJsonObject(), res)); 12465 } 12466 }; 12467 if (json.has("authorizingPrescription")) { 12468 JsonArray array = json.getAsJsonArray("authorizingPrescription"); 12469 for (int i = 0; i < array.size(); i++) { 12470 res.getAuthorizingPrescription().add(parseReference(array.get(i).getAsJsonObject())); 12471 } 12472 }; 12473 if (json.has("type")) 12474 res.setType(parseCodeableConcept(getJObject(json, "type"))); 12475 if (json.has("quantity")) 12476 res.setQuantity(parseSimpleQuantity(getJObject(json, "quantity"))); 12477 if (json.has("daysSupply")) 12478 res.setDaysSupply(parseSimpleQuantity(getJObject(json, "daysSupply"))); 12479 if (json.has("whenPrepared")) 12480 res.setWhenPreparedElement(parseDateTime(json.get("whenPrepared").getAsString())); 12481 if (json.has("_whenPrepared")) 12482 parseElementProperties(getJObject(json, "_whenPrepared"), res.getWhenPreparedElement()); 12483 if (json.has("whenHandedOver")) 12484 res.setWhenHandedOverElement(parseDateTime(json.get("whenHandedOver").getAsString())); 12485 if (json.has("_whenHandedOver")) 12486 parseElementProperties(getJObject(json, "_whenHandedOver"), res.getWhenHandedOverElement()); 12487 if (json.has("destination")) 12488 res.setDestination(parseReference(getJObject(json, "destination"))); 12489 if (json.has("receiver")) { 12490 JsonArray array = json.getAsJsonArray("receiver"); 12491 for (int i = 0; i < array.size(); i++) { 12492 res.getReceiver().add(parseReference(array.get(i).getAsJsonObject())); 12493 } 12494 }; 12495 if (json.has("note")) { 12496 JsonArray array = json.getAsJsonArray("note"); 12497 for (int i = 0; i < array.size(); i++) { 12498 res.getNote().add(parseAnnotation(array.get(i).getAsJsonObject())); 12499 } 12500 }; 12501 if (json.has("dosageInstruction")) { 12502 JsonArray array = json.getAsJsonArray("dosageInstruction"); 12503 for (int i = 0; i < array.size(); i++) { 12504 res.getDosageInstruction().add(parseDosage(array.get(i).getAsJsonObject())); 12505 } 12506 }; 12507 if (json.has("substitution")) 12508 res.setSubstitution(parseMedicationDispenseMedicationDispenseSubstitutionComponent(getJObject(json, "substitution"), res)); 12509 if (json.has("detectedIssue")) { 12510 JsonArray array = json.getAsJsonArray("detectedIssue"); 12511 for (int i = 0; i < array.size(); i++) { 12512 res.getDetectedIssue().add(parseReference(array.get(i).getAsJsonObject())); 12513 } 12514 }; 12515 if (json.has("notDone")) 12516 res.setNotDoneElement(parseBoolean(json.get("notDone").getAsBoolean())); 12517 if (json.has("_notDone")) 12518 parseElementProperties(getJObject(json, "_notDone"), res.getNotDoneElement()); 12519 Type notDoneReason = parseType("notDoneReason", json); 12520 if (notDoneReason != null) 12521 res.setNotDoneReason(notDoneReason); 12522 if (json.has("eventHistory")) { 12523 JsonArray array = json.getAsJsonArray("eventHistory"); 12524 for (int i = 0; i < array.size(); i++) { 12525 res.getEventHistory().add(parseReference(array.get(i).getAsJsonObject())); 12526 } 12527 }; 12528 } 12529 12530 protected MedicationDispense.MedicationDispensePerformerComponent parseMedicationDispenseMedicationDispensePerformerComponent(JsonObject json, MedicationDispense owner) throws IOException, FHIRFormatError { 12531 MedicationDispense.MedicationDispensePerformerComponent res = new MedicationDispense.MedicationDispensePerformerComponent(); 12532 parseMedicationDispenseMedicationDispensePerformerComponentProperties(json, owner, res); 12533 return res; 12534 } 12535 12536 protected void parseMedicationDispenseMedicationDispensePerformerComponentProperties(JsonObject json, MedicationDispense owner, MedicationDispense.MedicationDispensePerformerComponent res) throws IOException, FHIRFormatError { 12537 parseBackboneProperties(json, res); 12538 if (json.has("actor")) 12539 res.setActor(parseReference(getJObject(json, "actor"))); 12540 if (json.has("onBehalfOf")) 12541 res.setOnBehalfOf(parseReference(getJObject(json, "onBehalfOf"))); 12542 } 12543 12544 protected MedicationDispense.MedicationDispenseSubstitutionComponent parseMedicationDispenseMedicationDispenseSubstitutionComponent(JsonObject json, MedicationDispense owner) throws IOException, FHIRFormatError { 12545 MedicationDispense.MedicationDispenseSubstitutionComponent res = new MedicationDispense.MedicationDispenseSubstitutionComponent(); 12546 parseMedicationDispenseMedicationDispenseSubstitutionComponentProperties(json, owner, res); 12547 return res; 12548 } 12549 12550 protected void parseMedicationDispenseMedicationDispenseSubstitutionComponentProperties(JsonObject json, MedicationDispense owner, MedicationDispense.MedicationDispenseSubstitutionComponent res) throws IOException, FHIRFormatError { 12551 parseBackboneProperties(json, res); 12552 if (json.has("wasSubstituted")) 12553 res.setWasSubstitutedElement(parseBoolean(json.get("wasSubstituted").getAsBoolean())); 12554 if (json.has("_wasSubstituted")) 12555 parseElementProperties(getJObject(json, "_wasSubstituted"), res.getWasSubstitutedElement()); 12556 if (json.has("type")) 12557 res.setType(parseCodeableConcept(getJObject(json, "type"))); 12558 if (json.has("reason")) { 12559 JsonArray array = json.getAsJsonArray("reason"); 12560 for (int i = 0; i < array.size(); i++) { 12561 res.getReason().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 12562 } 12563 }; 12564 if (json.has("responsibleParty")) { 12565 JsonArray array = json.getAsJsonArray("responsibleParty"); 12566 for (int i = 0; i < array.size(); i++) { 12567 res.getResponsibleParty().add(parseReference(array.get(i).getAsJsonObject())); 12568 } 12569 }; 12570 } 12571 12572 protected MedicationRequest parseMedicationRequest(JsonObject json) throws IOException, FHIRFormatError { 12573 MedicationRequest res = new MedicationRequest(); 12574 parseMedicationRequestProperties(json, res); 12575 return res; 12576 } 12577 12578 protected void parseMedicationRequestProperties(JsonObject json, MedicationRequest res) throws IOException, FHIRFormatError { 12579 parseDomainResourceProperties(json, res); 12580 if (json.has("identifier")) { 12581 JsonArray array = json.getAsJsonArray("identifier"); 12582 for (int i = 0; i < array.size(); i++) { 12583 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 12584 } 12585 }; 12586 if (json.has("definition")) { 12587 JsonArray array = json.getAsJsonArray("definition"); 12588 for (int i = 0; i < array.size(); i++) { 12589 res.getDefinition().add(parseReference(array.get(i).getAsJsonObject())); 12590 } 12591 }; 12592 if (json.has("basedOn")) { 12593 JsonArray array = json.getAsJsonArray("basedOn"); 12594 for (int i = 0; i < array.size(); i++) { 12595 res.getBasedOn().add(parseReference(array.get(i).getAsJsonObject())); 12596 } 12597 }; 12598 if (json.has("groupIdentifier")) 12599 res.setGroupIdentifier(parseIdentifier(getJObject(json, "groupIdentifier"))); 12600 if (json.has("status")) 12601 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), MedicationRequest.MedicationRequestStatus.NULL, new MedicationRequest.MedicationRequestStatusEnumFactory())); 12602 if (json.has("_status")) 12603 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 12604 if (json.has("intent")) 12605 res.setIntentElement(parseEnumeration(json.get("intent").getAsString(), MedicationRequest.MedicationRequestIntent.NULL, new MedicationRequest.MedicationRequestIntentEnumFactory())); 12606 if (json.has("_intent")) 12607 parseElementProperties(getJObject(json, "_intent"), res.getIntentElement()); 12608 if (json.has("category")) 12609 res.setCategory(parseCodeableConcept(getJObject(json, "category"))); 12610 if (json.has("priority")) 12611 res.setPriorityElement(parseEnumeration(json.get("priority").getAsString(), MedicationRequest.MedicationRequestPriority.NULL, new MedicationRequest.MedicationRequestPriorityEnumFactory())); 12612 if (json.has("_priority")) 12613 parseElementProperties(getJObject(json, "_priority"), res.getPriorityElement()); 12614 Type medication = parseType("medication", json); 12615 if (medication != null) 12616 res.setMedication(medication); 12617 if (json.has("subject")) 12618 res.setSubject(parseReference(getJObject(json, "subject"))); 12619 if (json.has("context")) 12620 res.setContext(parseReference(getJObject(json, "context"))); 12621 if (json.has("supportingInformation")) { 12622 JsonArray array = json.getAsJsonArray("supportingInformation"); 12623 for (int i = 0; i < array.size(); i++) { 12624 res.getSupportingInformation().add(parseReference(array.get(i).getAsJsonObject())); 12625 } 12626 }; 12627 if (json.has("authoredOn")) 12628 res.setAuthoredOnElement(parseDateTime(json.get("authoredOn").getAsString())); 12629 if (json.has("_authoredOn")) 12630 parseElementProperties(getJObject(json, "_authoredOn"), res.getAuthoredOnElement()); 12631 if (json.has("requester")) 12632 res.setRequester(parseMedicationRequestMedicationRequestRequesterComponent(getJObject(json, "requester"), res)); 12633 if (json.has("recorder")) 12634 res.setRecorder(parseReference(getJObject(json, "recorder"))); 12635 if (json.has("reasonCode")) { 12636 JsonArray array = json.getAsJsonArray("reasonCode"); 12637 for (int i = 0; i < array.size(); i++) { 12638 res.getReasonCode().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 12639 } 12640 }; 12641 if (json.has("reasonReference")) { 12642 JsonArray array = json.getAsJsonArray("reasonReference"); 12643 for (int i = 0; i < array.size(); i++) { 12644 res.getReasonReference().add(parseReference(array.get(i).getAsJsonObject())); 12645 } 12646 }; 12647 if (json.has("note")) { 12648 JsonArray array = json.getAsJsonArray("note"); 12649 for (int i = 0; i < array.size(); i++) { 12650 res.getNote().add(parseAnnotation(array.get(i).getAsJsonObject())); 12651 } 12652 }; 12653 if (json.has("dosageInstruction")) { 12654 JsonArray array = json.getAsJsonArray("dosageInstruction"); 12655 for (int i = 0; i < array.size(); i++) { 12656 res.getDosageInstruction().add(parseDosage(array.get(i).getAsJsonObject())); 12657 } 12658 }; 12659 if (json.has("dispenseRequest")) 12660 res.setDispenseRequest(parseMedicationRequestMedicationRequestDispenseRequestComponent(getJObject(json, "dispenseRequest"), res)); 12661 if (json.has("substitution")) 12662 res.setSubstitution(parseMedicationRequestMedicationRequestSubstitutionComponent(getJObject(json, "substitution"), res)); 12663 if (json.has("priorPrescription")) 12664 res.setPriorPrescription(parseReference(getJObject(json, "priorPrescription"))); 12665 if (json.has("detectedIssue")) { 12666 JsonArray array = json.getAsJsonArray("detectedIssue"); 12667 for (int i = 0; i < array.size(); i++) { 12668 res.getDetectedIssue().add(parseReference(array.get(i).getAsJsonObject())); 12669 } 12670 }; 12671 if (json.has("eventHistory")) { 12672 JsonArray array = json.getAsJsonArray("eventHistory"); 12673 for (int i = 0; i < array.size(); i++) { 12674 res.getEventHistory().add(parseReference(array.get(i).getAsJsonObject())); 12675 } 12676 }; 12677 } 12678 12679 protected MedicationRequest.MedicationRequestRequesterComponent parseMedicationRequestMedicationRequestRequesterComponent(JsonObject json, MedicationRequest owner) throws IOException, FHIRFormatError { 12680 MedicationRequest.MedicationRequestRequesterComponent res = new MedicationRequest.MedicationRequestRequesterComponent(); 12681 parseMedicationRequestMedicationRequestRequesterComponentProperties(json, owner, res); 12682 return res; 12683 } 12684 12685 protected void parseMedicationRequestMedicationRequestRequesterComponentProperties(JsonObject json, MedicationRequest owner, MedicationRequest.MedicationRequestRequesterComponent res) throws IOException, FHIRFormatError { 12686 parseBackboneProperties(json, res); 12687 if (json.has("agent")) 12688 res.setAgent(parseReference(getJObject(json, "agent"))); 12689 if (json.has("onBehalfOf")) 12690 res.setOnBehalfOf(parseReference(getJObject(json, "onBehalfOf"))); 12691 } 12692 12693 protected MedicationRequest.MedicationRequestDispenseRequestComponent parseMedicationRequestMedicationRequestDispenseRequestComponent(JsonObject json, MedicationRequest owner) throws IOException, FHIRFormatError { 12694 MedicationRequest.MedicationRequestDispenseRequestComponent res = new MedicationRequest.MedicationRequestDispenseRequestComponent(); 12695 parseMedicationRequestMedicationRequestDispenseRequestComponentProperties(json, owner, res); 12696 return res; 12697 } 12698 12699 protected void parseMedicationRequestMedicationRequestDispenseRequestComponentProperties(JsonObject json, MedicationRequest owner, MedicationRequest.MedicationRequestDispenseRequestComponent res) throws IOException, FHIRFormatError { 12700 parseBackboneProperties(json, res); 12701 if (json.has("validityPeriod")) 12702 res.setValidityPeriod(parsePeriod(getJObject(json, "validityPeriod"))); 12703 if (json.has("numberOfRepeatsAllowed")) 12704 res.setNumberOfRepeatsAllowedElement(parsePositiveInt(json.get("numberOfRepeatsAllowed").getAsString())); 12705 if (json.has("_numberOfRepeatsAllowed")) 12706 parseElementProperties(getJObject(json, "_numberOfRepeatsAllowed"), res.getNumberOfRepeatsAllowedElement()); 12707 if (json.has("quantity")) 12708 res.setQuantity(parseSimpleQuantity(getJObject(json, "quantity"))); 12709 if (json.has("expectedSupplyDuration")) 12710 res.setExpectedSupplyDuration(parseDuration(getJObject(json, "expectedSupplyDuration"))); 12711 if (json.has("performer")) 12712 res.setPerformer(parseReference(getJObject(json, "performer"))); 12713 } 12714 12715 protected MedicationRequest.MedicationRequestSubstitutionComponent parseMedicationRequestMedicationRequestSubstitutionComponent(JsonObject json, MedicationRequest owner) throws IOException, FHIRFormatError { 12716 MedicationRequest.MedicationRequestSubstitutionComponent res = new MedicationRequest.MedicationRequestSubstitutionComponent(); 12717 parseMedicationRequestMedicationRequestSubstitutionComponentProperties(json, owner, res); 12718 return res; 12719 } 12720 12721 protected void parseMedicationRequestMedicationRequestSubstitutionComponentProperties(JsonObject json, MedicationRequest owner, MedicationRequest.MedicationRequestSubstitutionComponent res) throws IOException, FHIRFormatError { 12722 parseBackboneProperties(json, res); 12723 if (json.has("allowed")) 12724 res.setAllowedElement(parseBoolean(json.get("allowed").getAsBoolean())); 12725 if (json.has("_allowed")) 12726 parseElementProperties(getJObject(json, "_allowed"), res.getAllowedElement()); 12727 if (json.has("reason")) 12728 res.setReason(parseCodeableConcept(getJObject(json, "reason"))); 12729 } 12730 12731 protected MedicationStatement parseMedicationStatement(JsonObject json) throws IOException, FHIRFormatError { 12732 MedicationStatement res = new MedicationStatement(); 12733 parseMedicationStatementProperties(json, res); 12734 return res; 12735 } 12736 12737 protected void parseMedicationStatementProperties(JsonObject json, MedicationStatement res) throws IOException, FHIRFormatError { 12738 parseDomainResourceProperties(json, res); 12739 if (json.has("identifier")) { 12740 JsonArray array = json.getAsJsonArray("identifier"); 12741 for (int i = 0; i < array.size(); i++) { 12742 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 12743 } 12744 }; 12745 if (json.has("basedOn")) { 12746 JsonArray array = json.getAsJsonArray("basedOn"); 12747 for (int i = 0; i < array.size(); i++) { 12748 res.getBasedOn().add(parseReference(array.get(i).getAsJsonObject())); 12749 } 12750 }; 12751 if (json.has("partOf")) { 12752 JsonArray array = json.getAsJsonArray("partOf"); 12753 for (int i = 0; i < array.size(); i++) { 12754 res.getPartOf().add(parseReference(array.get(i).getAsJsonObject())); 12755 } 12756 }; 12757 if (json.has("context")) 12758 res.setContext(parseReference(getJObject(json, "context"))); 12759 if (json.has("status")) 12760 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), MedicationStatement.MedicationStatementStatus.NULL, new MedicationStatement.MedicationStatementStatusEnumFactory())); 12761 if (json.has("_status")) 12762 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 12763 if (json.has("category")) 12764 res.setCategory(parseCodeableConcept(getJObject(json, "category"))); 12765 Type medication = parseType("medication", json); 12766 if (medication != null) 12767 res.setMedication(medication); 12768 Type effective = parseType("effective", json); 12769 if (effective != null) 12770 res.setEffective(effective); 12771 if (json.has("dateAsserted")) 12772 res.setDateAssertedElement(parseDateTime(json.get("dateAsserted").getAsString())); 12773 if (json.has("_dateAsserted")) 12774 parseElementProperties(getJObject(json, "_dateAsserted"), res.getDateAssertedElement()); 12775 if (json.has("informationSource")) 12776 res.setInformationSource(parseReference(getJObject(json, "informationSource"))); 12777 if (json.has("subject")) 12778 res.setSubject(parseReference(getJObject(json, "subject"))); 12779 if (json.has("derivedFrom")) { 12780 JsonArray array = json.getAsJsonArray("derivedFrom"); 12781 for (int i = 0; i < array.size(); i++) { 12782 res.getDerivedFrom().add(parseReference(array.get(i).getAsJsonObject())); 12783 } 12784 }; 12785 if (json.has("taken")) 12786 res.setTakenElement(parseEnumeration(json.get("taken").getAsString(), MedicationStatement.MedicationStatementTaken.NULL, new MedicationStatement.MedicationStatementTakenEnumFactory())); 12787 if (json.has("_taken")) 12788 parseElementProperties(getJObject(json, "_taken"), res.getTakenElement()); 12789 if (json.has("reasonNotTaken")) { 12790 JsonArray array = json.getAsJsonArray("reasonNotTaken"); 12791 for (int i = 0; i < array.size(); i++) { 12792 res.getReasonNotTaken().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 12793 } 12794 }; 12795 if (json.has("reasonCode")) { 12796 JsonArray array = json.getAsJsonArray("reasonCode"); 12797 for (int i = 0; i < array.size(); i++) { 12798 res.getReasonCode().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 12799 } 12800 }; 12801 if (json.has("reasonReference")) { 12802 JsonArray array = json.getAsJsonArray("reasonReference"); 12803 for (int i = 0; i < array.size(); i++) { 12804 res.getReasonReference().add(parseReference(array.get(i).getAsJsonObject())); 12805 } 12806 }; 12807 if (json.has("note")) { 12808 JsonArray array = json.getAsJsonArray("note"); 12809 for (int i = 0; i < array.size(); i++) { 12810 res.getNote().add(parseAnnotation(array.get(i).getAsJsonObject())); 12811 } 12812 }; 12813 if (json.has("dosage")) { 12814 JsonArray array = json.getAsJsonArray("dosage"); 12815 for (int i = 0; i < array.size(); i++) { 12816 res.getDosage().add(parseDosage(array.get(i).getAsJsonObject())); 12817 } 12818 }; 12819 } 12820 12821 protected MessageDefinition parseMessageDefinition(JsonObject json) throws IOException, FHIRFormatError { 12822 MessageDefinition res = new MessageDefinition(); 12823 parseMessageDefinitionProperties(json, res); 12824 return res; 12825 } 12826 12827 protected void parseMessageDefinitionProperties(JsonObject json, MessageDefinition res) throws IOException, FHIRFormatError { 12828 parseDomainResourceProperties(json, res); 12829 if (json.has("url")) 12830 res.setUrlElement(parseUri(json.get("url").getAsString())); 12831 if (json.has("_url")) 12832 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 12833 if (json.has("identifier")) 12834 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 12835 if (json.has("version")) 12836 res.setVersionElement(parseString(json.get("version").getAsString())); 12837 if (json.has("_version")) 12838 parseElementProperties(getJObject(json, "_version"), res.getVersionElement()); 12839 if (json.has("name")) 12840 res.setNameElement(parseString(json.get("name").getAsString())); 12841 if (json.has("_name")) 12842 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 12843 if (json.has("title")) 12844 res.setTitleElement(parseString(json.get("title").getAsString())); 12845 if (json.has("_title")) 12846 parseElementProperties(getJObject(json, "_title"), res.getTitleElement()); 12847 if (json.has("status")) 12848 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Enumerations.PublicationStatus.NULL, new Enumerations.PublicationStatusEnumFactory())); 12849 if (json.has("_status")) 12850 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 12851 if (json.has("experimental")) 12852 res.setExperimentalElement(parseBoolean(json.get("experimental").getAsBoolean())); 12853 if (json.has("_experimental")) 12854 parseElementProperties(getJObject(json, "_experimental"), res.getExperimentalElement()); 12855 if (json.has("date")) 12856 res.setDateElement(parseDateTime(json.get("date").getAsString())); 12857 if (json.has("_date")) 12858 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 12859 if (json.has("publisher")) 12860 res.setPublisherElement(parseString(json.get("publisher").getAsString())); 12861 if (json.has("_publisher")) 12862 parseElementProperties(getJObject(json, "_publisher"), res.getPublisherElement()); 12863 if (json.has("contact")) { 12864 JsonArray array = json.getAsJsonArray("contact"); 12865 for (int i = 0; i < array.size(); i++) { 12866 res.getContact().add(parseContactDetail(array.get(i).getAsJsonObject())); 12867 } 12868 }; 12869 if (json.has("description")) 12870 res.setDescriptionElement(parseMarkdown(json.get("description").getAsString())); 12871 if (json.has("_description")) 12872 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 12873 if (json.has("useContext")) { 12874 JsonArray array = json.getAsJsonArray("useContext"); 12875 for (int i = 0; i < array.size(); i++) { 12876 res.getUseContext().add(parseUsageContext(array.get(i).getAsJsonObject())); 12877 } 12878 }; 12879 if (json.has("jurisdiction")) { 12880 JsonArray array = json.getAsJsonArray("jurisdiction"); 12881 for (int i = 0; i < array.size(); i++) { 12882 res.getJurisdiction().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 12883 } 12884 }; 12885 if (json.has("purpose")) 12886 res.setPurposeElement(parseMarkdown(json.get("purpose").getAsString())); 12887 if (json.has("_purpose")) 12888 parseElementProperties(getJObject(json, "_purpose"), res.getPurposeElement()); 12889 if (json.has("copyright")) 12890 res.setCopyrightElement(parseMarkdown(json.get("copyright").getAsString())); 12891 if (json.has("_copyright")) 12892 parseElementProperties(getJObject(json, "_copyright"), res.getCopyrightElement()); 12893 if (json.has("base")) 12894 res.setBase(parseReference(getJObject(json, "base"))); 12895 if (json.has("parent")) { 12896 JsonArray array = json.getAsJsonArray("parent"); 12897 for (int i = 0; i < array.size(); i++) { 12898 res.getParent().add(parseReference(array.get(i).getAsJsonObject())); 12899 } 12900 }; 12901 if (json.has("replaces")) { 12902 JsonArray array = json.getAsJsonArray("replaces"); 12903 for (int i = 0; i < array.size(); i++) { 12904 res.getReplaces().add(parseReference(array.get(i).getAsJsonObject())); 12905 } 12906 }; 12907 if (json.has("event")) 12908 res.setEvent(parseCoding(getJObject(json, "event"))); 12909 if (json.has("category")) 12910 res.setCategoryElement(parseEnumeration(json.get("category").getAsString(), MessageDefinition.MessageSignificanceCategory.NULL, new MessageDefinition.MessageSignificanceCategoryEnumFactory())); 12911 if (json.has("_category")) 12912 parseElementProperties(getJObject(json, "_category"), res.getCategoryElement()); 12913 if (json.has("focus")) { 12914 JsonArray array = json.getAsJsonArray("focus"); 12915 for (int i = 0; i < array.size(); i++) { 12916 res.getFocus().add(parseMessageDefinitionMessageDefinitionFocusComponent(array.get(i).getAsJsonObject(), res)); 12917 } 12918 }; 12919 if (json.has("responseRequired")) 12920 res.setResponseRequiredElement(parseBoolean(json.get("responseRequired").getAsBoolean())); 12921 if (json.has("_responseRequired")) 12922 parseElementProperties(getJObject(json, "_responseRequired"), res.getResponseRequiredElement()); 12923 if (json.has("allowedResponse")) { 12924 JsonArray array = json.getAsJsonArray("allowedResponse"); 12925 for (int i = 0; i < array.size(); i++) { 12926 res.getAllowedResponse().add(parseMessageDefinitionMessageDefinitionAllowedResponseComponent(array.get(i).getAsJsonObject(), res)); 12927 } 12928 }; 12929 } 12930 12931 protected MessageDefinition.MessageDefinitionFocusComponent parseMessageDefinitionMessageDefinitionFocusComponent(JsonObject json, MessageDefinition owner) throws IOException, FHIRFormatError { 12932 MessageDefinition.MessageDefinitionFocusComponent res = new MessageDefinition.MessageDefinitionFocusComponent(); 12933 parseMessageDefinitionMessageDefinitionFocusComponentProperties(json, owner, res); 12934 return res; 12935 } 12936 12937 protected void parseMessageDefinitionMessageDefinitionFocusComponentProperties(JsonObject json, MessageDefinition owner, MessageDefinition.MessageDefinitionFocusComponent res) throws IOException, FHIRFormatError { 12938 parseBackboneProperties(json, res); 12939 if (json.has("code")) 12940 res.setCodeElement(parseCode(json.get("code").getAsString())); 12941 if (json.has("_code")) 12942 parseElementProperties(getJObject(json, "_code"), res.getCodeElement()); 12943 if (json.has("profile")) 12944 res.setProfile(parseReference(getJObject(json, "profile"))); 12945 if (json.has("min")) 12946 res.setMinElement(parseUnsignedInt(json.get("min").getAsString())); 12947 if (json.has("_min")) 12948 parseElementProperties(getJObject(json, "_min"), res.getMinElement()); 12949 if (json.has("max")) 12950 res.setMaxElement(parseString(json.get("max").getAsString())); 12951 if (json.has("_max")) 12952 parseElementProperties(getJObject(json, "_max"), res.getMaxElement()); 12953 } 12954 12955 protected MessageDefinition.MessageDefinitionAllowedResponseComponent parseMessageDefinitionMessageDefinitionAllowedResponseComponent(JsonObject json, MessageDefinition owner) throws IOException, FHIRFormatError { 12956 MessageDefinition.MessageDefinitionAllowedResponseComponent res = new MessageDefinition.MessageDefinitionAllowedResponseComponent(); 12957 parseMessageDefinitionMessageDefinitionAllowedResponseComponentProperties(json, owner, res); 12958 return res; 12959 } 12960 12961 protected void parseMessageDefinitionMessageDefinitionAllowedResponseComponentProperties(JsonObject json, MessageDefinition owner, MessageDefinition.MessageDefinitionAllowedResponseComponent res) throws IOException, FHIRFormatError { 12962 parseBackboneProperties(json, res); 12963 if (json.has("message")) 12964 res.setMessage(parseReference(getJObject(json, "message"))); 12965 if (json.has("situation")) 12966 res.setSituationElement(parseMarkdown(json.get("situation").getAsString())); 12967 if (json.has("_situation")) 12968 parseElementProperties(getJObject(json, "_situation"), res.getSituationElement()); 12969 } 12970 12971 protected MessageHeader parseMessageHeader(JsonObject json) throws IOException, FHIRFormatError { 12972 MessageHeader res = new MessageHeader(); 12973 parseMessageHeaderProperties(json, res); 12974 return res; 12975 } 12976 12977 protected void parseMessageHeaderProperties(JsonObject json, MessageHeader res) throws IOException, FHIRFormatError { 12978 parseDomainResourceProperties(json, res); 12979 if (json.has("event")) 12980 res.setEvent(parseCoding(getJObject(json, "event"))); 12981 if (json.has("destination")) { 12982 JsonArray array = json.getAsJsonArray("destination"); 12983 for (int i = 0; i < array.size(); i++) { 12984 res.getDestination().add(parseMessageHeaderMessageDestinationComponent(array.get(i).getAsJsonObject(), res)); 12985 } 12986 }; 12987 if (json.has("receiver")) 12988 res.setReceiver(parseReference(getJObject(json, "receiver"))); 12989 if (json.has("sender")) 12990 res.setSender(parseReference(getJObject(json, "sender"))); 12991 if (json.has("timestamp")) 12992 res.setTimestampElement(parseInstant(json.get("timestamp").getAsString())); 12993 if (json.has("_timestamp")) 12994 parseElementProperties(getJObject(json, "_timestamp"), res.getTimestampElement()); 12995 if (json.has("enterer")) 12996 res.setEnterer(parseReference(getJObject(json, "enterer"))); 12997 if (json.has("author")) 12998 res.setAuthor(parseReference(getJObject(json, "author"))); 12999 if (json.has("source")) 13000 res.setSource(parseMessageHeaderMessageSourceComponent(getJObject(json, "source"), res)); 13001 if (json.has("responsible")) 13002 res.setResponsible(parseReference(getJObject(json, "responsible"))); 13003 if (json.has("reason")) 13004 res.setReason(parseCodeableConcept(getJObject(json, "reason"))); 13005 if (json.has("response")) 13006 res.setResponse(parseMessageHeaderMessageHeaderResponseComponent(getJObject(json, "response"), res)); 13007 if (json.has("focus")) { 13008 JsonArray array = json.getAsJsonArray("focus"); 13009 for (int i = 0; i < array.size(); i++) { 13010 res.getFocus().add(parseReference(array.get(i).getAsJsonObject())); 13011 } 13012 }; 13013 } 13014 13015 protected MessageHeader.MessageDestinationComponent parseMessageHeaderMessageDestinationComponent(JsonObject json, MessageHeader owner) throws IOException, FHIRFormatError { 13016 MessageHeader.MessageDestinationComponent res = new MessageHeader.MessageDestinationComponent(); 13017 parseMessageHeaderMessageDestinationComponentProperties(json, owner, res); 13018 return res; 13019 } 13020 13021 protected void parseMessageHeaderMessageDestinationComponentProperties(JsonObject json, MessageHeader owner, MessageHeader.MessageDestinationComponent res) throws IOException, FHIRFormatError { 13022 parseBackboneProperties(json, res); 13023 if (json.has("name")) 13024 res.setNameElement(parseString(json.get("name").getAsString())); 13025 if (json.has("_name")) 13026 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 13027 if (json.has("target")) 13028 res.setTarget(parseReference(getJObject(json, "target"))); 13029 if (json.has("endpoint")) 13030 res.setEndpointElement(parseUri(json.get("endpoint").getAsString())); 13031 if (json.has("_endpoint")) 13032 parseElementProperties(getJObject(json, "_endpoint"), res.getEndpointElement()); 13033 } 13034 13035 protected MessageHeader.MessageSourceComponent parseMessageHeaderMessageSourceComponent(JsonObject json, MessageHeader owner) throws IOException, FHIRFormatError { 13036 MessageHeader.MessageSourceComponent res = new MessageHeader.MessageSourceComponent(); 13037 parseMessageHeaderMessageSourceComponentProperties(json, owner, res); 13038 return res; 13039 } 13040 13041 protected void parseMessageHeaderMessageSourceComponentProperties(JsonObject json, MessageHeader owner, MessageHeader.MessageSourceComponent res) throws IOException, FHIRFormatError { 13042 parseBackboneProperties(json, res); 13043 if (json.has("name")) 13044 res.setNameElement(parseString(json.get("name").getAsString())); 13045 if (json.has("_name")) 13046 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 13047 if (json.has("software")) 13048 res.setSoftwareElement(parseString(json.get("software").getAsString())); 13049 if (json.has("_software")) 13050 parseElementProperties(getJObject(json, "_software"), res.getSoftwareElement()); 13051 if (json.has("version")) 13052 res.setVersionElement(parseString(json.get("version").getAsString())); 13053 if (json.has("_version")) 13054 parseElementProperties(getJObject(json, "_version"), res.getVersionElement()); 13055 if (json.has("contact")) 13056 res.setContact(parseContactPoint(getJObject(json, "contact"))); 13057 if (json.has("endpoint")) 13058 res.setEndpointElement(parseUri(json.get("endpoint").getAsString())); 13059 if (json.has("_endpoint")) 13060 parseElementProperties(getJObject(json, "_endpoint"), res.getEndpointElement()); 13061 } 13062 13063 protected MessageHeader.MessageHeaderResponseComponent parseMessageHeaderMessageHeaderResponseComponent(JsonObject json, MessageHeader owner) throws IOException, FHIRFormatError { 13064 MessageHeader.MessageHeaderResponseComponent res = new MessageHeader.MessageHeaderResponseComponent(); 13065 parseMessageHeaderMessageHeaderResponseComponentProperties(json, owner, res); 13066 return res; 13067 } 13068 13069 protected void parseMessageHeaderMessageHeaderResponseComponentProperties(JsonObject json, MessageHeader owner, MessageHeader.MessageHeaderResponseComponent res) throws IOException, FHIRFormatError { 13070 parseBackboneProperties(json, res); 13071 if (json.has("identifier")) 13072 res.setIdentifierElement(parseId(json.get("identifier").getAsString())); 13073 if (json.has("_identifier")) 13074 parseElementProperties(getJObject(json, "_identifier"), res.getIdentifierElement()); 13075 if (json.has("code")) 13076 res.setCodeElement(parseEnumeration(json.get("code").getAsString(), MessageHeader.ResponseType.NULL, new MessageHeader.ResponseTypeEnumFactory())); 13077 if (json.has("_code")) 13078 parseElementProperties(getJObject(json, "_code"), res.getCodeElement()); 13079 if (json.has("details")) 13080 res.setDetails(parseReference(getJObject(json, "details"))); 13081 } 13082 13083 protected NamingSystem parseNamingSystem(JsonObject json) throws IOException, FHIRFormatError { 13084 NamingSystem res = new NamingSystem(); 13085 parseNamingSystemProperties(json, res); 13086 return res; 13087 } 13088 13089 protected void parseNamingSystemProperties(JsonObject json, NamingSystem res) throws IOException, FHIRFormatError { 13090 parseDomainResourceProperties(json, res); 13091 if (json.has("name")) 13092 res.setNameElement(parseString(json.get("name").getAsString())); 13093 if (json.has("_name")) 13094 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 13095 if (json.has("status")) 13096 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Enumerations.PublicationStatus.NULL, new Enumerations.PublicationStatusEnumFactory())); 13097 if (json.has("_status")) 13098 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 13099 if (json.has("kind")) 13100 res.setKindElement(parseEnumeration(json.get("kind").getAsString(), NamingSystem.NamingSystemType.NULL, new NamingSystem.NamingSystemTypeEnumFactory())); 13101 if (json.has("_kind")) 13102 parseElementProperties(getJObject(json, "_kind"), res.getKindElement()); 13103 if (json.has("date")) 13104 res.setDateElement(parseDateTime(json.get("date").getAsString())); 13105 if (json.has("_date")) 13106 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 13107 if (json.has("publisher")) 13108 res.setPublisherElement(parseString(json.get("publisher").getAsString())); 13109 if (json.has("_publisher")) 13110 parseElementProperties(getJObject(json, "_publisher"), res.getPublisherElement()); 13111 if (json.has("contact")) { 13112 JsonArray array = json.getAsJsonArray("contact"); 13113 for (int i = 0; i < array.size(); i++) { 13114 res.getContact().add(parseContactDetail(array.get(i).getAsJsonObject())); 13115 } 13116 }; 13117 if (json.has("responsible")) 13118 res.setResponsibleElement(parseString(json.get("responsible").getAsString())); 13119 if (json.has("_responsible")) 13120 parseElementProperties(getJObject(json, "_responsible"), res.getResponsibleElement()); 13121 if (json.has("type")) 13122 res.setType(parseCodeableConcept(getJObject(json, "type"))); 13123 if (json.has("description")) 13124 res.setDescriptionElement(parseMarkdown(json.get("description").getAsString())); 13125 if (json.has("_description")) 13126 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 13127 if (json.has("useContext")) { 13128 JsonArray array = json.getAsJsonArray("useContext"); 13129 for (int i = 0; i < array.size(); i++) { 13130 res.getUseContext().add(parseUsageContext(array.get(i).getAsJsonObject())); 13131 } 13132 }; 13133 if (json.has("jurisdiction")) { 13134 JsonArray array = json.getAsJsonArray("jurisdiction"); 13135 for (int i = 0; i < array.size(); i++) { 13136 res.getJurisdiction().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 13137 } 13138 }; 13139 if (json.has("usage")) 13140 res.setUsageElement(parseString(json.get("usage").getAsString())); 13141 if (json.has("_usage")) 13142 parseElementProperties(getJObject(json, "_usage"), res.getUsageElement()); 13143 if (json.has("uniqueId")) { 13144 JsonArray array = json.getAsJsonArray("uniqueId"); 13145 for (int i = 0; i < array.size(); i++) { 13146 res.getUniqueId().add(parseNamingSystemNamingSystemUniqueIdComponent(array.get(i).getAsJsonObject(), res)); 13147 } 13148 }; 13149 if (json.has("replacedBy")) 13150 res.setReplacedBy(parseReference(getJObject(json, "replacedBy"))); 13151 } 13152 13153 protected NamingSystem.NamingSystemUniqueIdComponent parseNamingSystemNamingSystemUniqueIdComponent(JsonObject json, NamingSystem owner) throws IOException, FHIRFormatError { 13154 NamingSystem.NamingSystemUniqueIdComponent res = new NamingSystem.NamingSystemUniqueIdComponent(); 13155 parseNamingSystemNamingSystemUniqueIdComponentProperties(json, owner, res); 13156 return res; 13157 } 13158 13159 protected void parseNamingSystemNamingSystemUniqueIdComponentProperties(JsonObject json, NamingSystem owner, NamingSystem.NamingSystemUniqueIdComponent res) throws IOException, FHIRFormatError { 13160 parseBackboneProperties(json, res); 13161 if (json.has("type")) 13162 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), NamingSystem.NamingSystemIdentifierType.NULL, new NamingSystem.NamingSystemIdentifierTypeEnumFactory())); 13163 if (json.has("_type")) 13164 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 13165 if (json.has("value")) 13166 res.setValueElement(parseString(json.get("value").getAsString())); 13167 if (json.has("_value")) 13168 parseElementProperties(getJObject(json, "_value"), res.getValueElement()); 13169 if (json.has("preferred")) 13170 res.setPreferredElement(parseBoolean(json.get("preferred").getAsBoolean())); 13171 if (json.has("_preferred")) 13172 parseElementProperties(getJObject(json, "_preferred"), res.getPreferredElement()); 13173 if (json.has("comment")) 13174 res.setCommentElement(parseString(json.get("comment").getAsString())); 13175 if (json.has("_comment")) 13176 parseElementProperties(getJObject(json, "_comment"), res.getCommentElement()); 13177 if (json.has("period")) 13178 res.setPeriod(parsePeriod(getJObject(json, "period"))); 13179 } 13180 13181 protected NutritionOrder parseNutritionOrder(JsonObject json) throws IOException, FHIRFormatError { 13182 NutritionOrder res = new NutritionOrder(); 13183 parseNutritionOrderProperties(json, res); 13184 return res; 13185 } 13186 13187 protected void parseNutritionOrderProperties(JsonObject json, NutritionOrder res) throws IOException, FHIRFormatError { 13188 parseDomainResourceProperties(json, res); 13189 if (json.has("identifier")) { 13190 JsonArray array = json.getAsJsonArray("identifier"); 13191 for (int i = 0; i < array.size(); i++) { 13192 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 13193 } 13194 }; 13195 if (json.has("status")) 13196 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), NutritionOrder.NutritionOrderStatus.NULL, new NutritionOrder.NutritionOrderStatusEnumFactory())); 13197 if (json.has("_status")) 13198 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 13199 if (json.has("patient")) 13200 res.setPatient(parseReference(getJObject(json, "patient"))); 13201 if (json.has("encounter")) 13202 res.setEncounter(parseReference(getJObject(json, "encounter"))); 13203 if (json.has("dateTime")) 13204 res.setDateTimeElement(parseDateTime(json.get("dateTime").getAsString())); 13205 if (json.has("_dateTime")) 13206 parseElementProperties(getJObject(json, "_dateTime"), res.getDateTimeElement()); 13207 if (json.has("orderer")) 13208 res.setOrderer(parseReference(getJObject(json, "orderer"))); 13209 if (json.has("allergyIntolerance")) { 13210 JsonArray array = json.getAsJsonArray("allergyIntolerance"); 13211 for (int i = 0; i < array.size(); i++) { 13212 res.getAllergyIntolerance().add(parseReference(array.get(i).getAsJsonObject())); 13213 } 13214 }; 13215 if (json.has("foodPreferenceModifier")) { 13216 JsonArray array = json.getAsJsonArray("foodPreferenceModifier"); 13217 for (int i = 0; i < array.size(); i++) { 13218 res.getFoodPreferenceModifier().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 13219 } 13220 }; 13221 if (json.has("excludeFoodModifier")) { 13222 JsonArray array = json.getAsJsonArray("excludeFoodModifier"); 13223 for (int i = 0; i < array.size(); i++) { 13224 res.getExcludeFoodModifier().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 13225 } 13226 }; 13227 if (json.has("oralDiet")) 13228 res.setOralDiet(parseNutritionOrderNutritionOrderOralDietComponent(getJObject(json, "oralDiet"), res)); 13229 if (json.has("supplement")) { 13230 JsonArray array = json.getAsJsonArray("supplement"); 13231 for (int i = 0; i < array.size(); i++) { 13232 res.getSupplement().add(parseNutritionOrderNutritionOrderSupplementComponent(array.get(i).getAsJsonObject(), res)); 13233 } 13234 }; 13235 if (json.has("enteralFormula")) 13236 res.setEnteralFormula(parseNutritionOrderNutritionOrderEnteralFormulaComponent(getJObject(json, "enteralFormula"), res)); 13237 } 13238 13239 protected NutritionOrder.NutritionOrderOralDietComponent parseNutritionOrderNutritionOrderOralDietComponent(JsonObject json, NutritionOrder owner) throws IOException, FHIRFormatError { 13240 NutritionOrder.NutritionOrderOralDietComponent res = new NutritionOrder.NutritionOrderOralDietComponent(); 13241 parseNutritionOrderNutritionOrderOralDietComponentProperties(json, owner, res); 13242 return res; 13243 } 13244 13245 protected void parseNutritionOrderNutritionOrderOralDietComponentProperties(JsonObject json, NutritionOrder owner, NutritionOrder.NutritionOrderOralDietComponent res) throws IOException, FHIRFormatError { 13246 parseBackboneProperties(json, res); 13247 if (json.has("type")) { 13248 JsonArray array = json.getAsJsonArray("type"); 13249 for (int i = 0; i < array.size(); i++) { 13250 res.getType().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 13251 } 13252 }; 13253 if (json.has("schedule")) { 13254 JsonArray array = json.getAsJsonArray("schedule"); 13255 for (int i = 0; i < array.size(); i++) { 13256 res.getSchedule().add(parseTiming(array.get(i).getAsJsonObject())); 13257 } 13258 }; 13259 if (json.has("nutrient")) { 13260 JsonArray array = json.getAsJsonArray("nutrient"); 13261 for (int i = 0; i < array.size(); i++) { 13262 res.getNutrient().add(parseNutritionOrderNutritionOrderOralDietNutrientComponent(array.get(i).getAsJsonObject(), owner)); 13263 } 13264 }; 13265 if (json.has("texture")) { 13266 JsonArray array = json.getAsJsonArray("texture"); 13267 for (int i = 0; i < array.size(); i++) { 13268 res.getTexture().add(parseNutritionOrderNutritionOrderOralDietTextureComponent(array.get(i).getAsJsonObject(), owner)); 13269 } 13270 }; 13271 if (json.has("fluidConsistencyType")) { 13272 JsonArray array = json.getAsJsonArray("fluidConsistencyType"); 13273 for (int i = 0; i < array.size(); i++) { 13274 res.getFluidConsistencyType().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 13275 } 13276 }; 13277 if (json.has("instruction")) 13278 res.setInstructionElement(parseString(json.get("instruction").getAsString())); 13279 if (json.has("_instruction")) 13280 parseElementProperties(getJObject(json, "_instruction"), res.getInstructionElement()); 13281 } 13282 13283 protected NutritionOrder.NutritionOrderOralDietNutrientComponent parseNutritionOrderNutritionOrderOralDietNutrientComponent(JsonObject json, NutritionOrder owner) throws IOException, FHIRFormatError { 13284 NutritionOrder.NutritionOrderOralDietNutrientComponent res = new NutritionOrder.NutritionOrderOralDietNutrientComponent(); 13285 parseNutritionOrderNutritionOrderOralDietNutrientComponentProperties(json, owner, res); 13286 return res; 13287 } 13288 13289 protected void parseNutritionOrderNutritionOrderOralDietNutrientComponentProperties(JsonObject json, NutritionOrder owner, NutritionOrder.NutritionOrderOralDietNutrientComponent res) throws IOException, FHIRFormatError { 13290 parseBackboneProperties(json, res); 13291 if (json.has("modifier")) 13292 res.setModifier(parseCodeableConcept(getJObject(json, "modifier"))); 13293 if (json.has("amount")) 13294 res.setAmount(parseSimpleQuantity(getJObject(json, "amount"))); 13295 } 13296 13297 protected NutritionOrder.NutritionOrderOralDietTextureComponent parseNutritionOrderNutritionOrderOralDietTextureComponent(JsonObject json, NutritionOrder owner) throws IOException, FHIRFormatError { 13298 NutritionOrder.NutritionOrderOralDietTextureComponent res = new NutritionOrder.NutritionOrderOralDietTextureComponent(); 13299 parseNutritionOrderNutritionOrderOralDietTextureComponentProperties(json, owner, res); 13300 return res; 13301 } 13302 13303 protected void parseNutritionOrderNutritionOrderOralDietTextureComponentProperties(JsonObject json, NutritionOrder owner, NutritionOrder.NutritionOrderOralDietTextureComponent res) throws IOException, FHIRFormatError { 13304 parseBackboneProperties(json, res); 13305 if (json.has("modifier")) 13306 res.setModifier(parseCodeableConcept(getJObject(json, "modifier"))); 13307 if (json.has("foodType")) 13308 res.setFoodType(parseCodeableConcept(getJObject(json, "foodType"))); 13309 } 13310 13311 protected NutritionOrder.NutritionOrderSupplementComponent parseNutritionOrderNutritionOrderSupplementComponent(JsonObject json, NutritionOrder owner) throws IOException, FHIRFormatError { 13312 NutritionOrder.NutritionOrderSupplementComponent res = new NutritionOrder.NutritionOrderSupplementComponent(); 13313 parseNutritionOrderNutritionOrderSupplementComponentProperties(json, owner, res); 13314 return res; 13315 } 13316 13317 protected void parseNutritionOrderNutritionOrderSupplementComponentProperties(JsonObject json, NutritionOrder owner, NutritionOrder.NutritionOrderSupplementComponent res) throws IOException, FHIRFormatError { 13318 parseBackboneProperties(json, res); 13319 if (json.has("type")) 13320 res.setType(parseCodeableConcept(getJObject(json, "type"))); 13321 if (json.has("productName")) 13322 res.setProductNameElement(parseString(json.get("productName").getAsString())); 13323 if (json.has("_productName")) 13324 parseElementProperties(getJObject(json, "_productName"), res.getProductNameElement()); 13325 if (json.has("schedule")) { 13326 JsonArray array = json.getAsJsonArray("schedule"); 13327 for (int i = 0; i < array.size(); i++) { 13328 res.getSchedule().add(parseTiming(array.get(i).getAsJsonObject())); 13329 } 13330 }; 13331 if (json.has("quantity")) 13332 res.setQuantity(parseSimpleQuantity(getJObject(json, "quantity"))); 13333 if (json.has("instruction")) 13334 res.setInstructionElement(parseString(json.get("instruction").getAsString())); 13335 if (json.has("_instruction")) 13336 parseElementProperties(getJObject(json, "_instruction"), res.getInstructionElement()); 13337 } 13338 13339 protected NutritionOrder.NutritionOrderEnteralFormulaComponent parseNutritionOrderNutritionOrderEnteralFormulaComponent(JsonObject json, NutritionOrder owner) throws IOException, FHIRFormatError { 13340 NutritionOrder.NutritionOrderEnteralFormulaComponent res = new NutritionOrder.NutritionOrderEnteralFormulaComponent(); 13341 parseNutritionOrderNutritionOrderEnteralFormulaComponentProperties(json, owner, res); 13342 return res; 13343 } 13344 13345 protected void parseNutritionOrderNutritionOrderEnteralFormulaComponentProperties(JsonObject json, NutritionOrder owner, NutritionOrder.NutritionOrderEnteralFormulaComponent res) throws IOException, FHIRFormatError { 13346 parseBackboneProperties(json, res); 13347 if (json.has("baseFormulaType")) 13348 res.setBaseFormulaType(parseCodeableConcept(getJObject(json, "baseFormulaType"))); 13349 if (json.has("baseFormulaProductName")) 13350 res.setBaseFormulaProductNameElement(parseString(json.get("baseFormulaProductName").getAsString())); 13351 if (json.has("_baseFormulaProductName")) 13352 parseElementProperties(getJObject(json, "_baseFormulaProductName"), res.getBaseFormulaProductNameElement()); 13353 if (json.has("additiveType")) 13354 res.setAdditiveType(parseCodeableConcept(getJObject(json, "additiveType"))); 13355 if (json.has("additiveProductName")) 13356 res.setAdditiveProductNameElement(parseString(json.get("additiveProductName").getAsString())); 13357 if (json.has("_additiveProductName")) 13358 parseElementProperties(getJObject(json, "_additiveProductName"), res.getAdditiveProductNameElement()); 13359 if (json.has("caloricDensity")) 13360 res.setCaloricDensity(parseSimpleQuantity(getJObject(json, "caloricDensity"))); 13361 if (json.has("routeofAdministration")) 13362 res.setRouteofAdministration(parseCodeableConcept(getJObject(json, "routeofAdministration"))); 13363 if (json.has("administration")) { 13364 JsonArray array = json.getAsJsonArray("administration"); 13365 for (int i = 0; i < array.size(); i++) { 13366 res.getAdministration().add(parseNutritionOrderNutritionOrderEnteralFormulaAdministrationComponent(array.get(i).getAsJsonObject(), owner)); 13367 } 13368 }; 13369 if (json.has("maxVolumeToDeliver")) 13370 res.setMaxVolumeToDeliver(parseSimpleQuantity(getJObject(json, "maxVolumeToDeliver"))); 13371 if (json.has("administrationInstruction")) 13372 res.setAdministrationInstructionElement(parseString(json.get("administrationInstruction").getAsString())); 13373 if (json.has("_administrationInstruction")) 13374 parseElementProperties(getJObject(json, "_administrationInstruction"), res.getAdministrationInstructionElement()); 13375 } 13376 13377 protected NutritionOrder.NutritionOrderEnteralFormulaAdministrationComponent parseNutritionOrderNutritionOrderEnteralFormulaAdministrationComponent(JsonObject json, NutritionOrder owner) throws IOException, FHIRFormatError { 13378 NutritionOrder.NutritionOrderEnteralFormulaAdministrationComponent res = new NutritionOrder.NutritionOrderEnteralFormulaAdministrationComponent(); 13379 parseNutritionOrderNutritionOrderEnteralFormulaAdministrationComponentProperties(json, owner, res); 13380 return res; 13381 } 13382 13383 protected void parseNutritionOrderNutritionOrderEnteralFormulaAdministrationComponentProperties(JsonObject json, NutritionOrder owner, NutritionOrder.NutritionOrderEnteralFormulaAdministrationComponent res) throws IOException, FHIRFormatError { 13384 parseBackboneProperties(json, res); 13385 if (json.has("schedule")) 13386 res.setSchedule(parseTiming(getJObject(json, "schedule"))); 13387 if (json.has("quantity")) 13388 res.setQuantity(parseSimpleQuantity(getJObject(json, "quantity"))); 13389 Type rate = parseType("rate", json); 13390 if (rate != null) 13391 res.setRate(rate); 13392 } 13393 13394 protected Observation parseObservation(JsonObject json) throws IOException, FHIRFormatError { 13395 Observation res = new Observation(); 13396 parseObservationProperties(json, res); 13397 return res; 13398 } 13399 13400 protected void parseObservationProperties(JsonObject json, Observation res) throws IOException, FHIRFormatError { 13401 parseDomainResourceProperties(json, res); 13402 if (json.has("identifier")) { 13403 JsonArray array = json.getAsJsonArray("identifier"); 13404 for (int i = 0; i < array.size(); i++) { 13405 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 13406 } 13407 }; 13408 if (json.has("basedOn")) { 13409 JsonArray array = json.getAsJsonArray("basedOn"); 13410 for (int i = 0; i < array.size(); i++) { 13411 res.getBasedOn().add(parseReference(array.get(i).getAsJsonObject())); 13412 } 13413 }; 13414 if (json.has("status")) 13415 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Observation.ObservationStatus.NULL, new Observation.ObservationStatusEnumFactory())); 13416 if (json.has("_status")) 13417 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 13418 if (json.has("category")) { 13419 JsonArray array = json.getAsJsonArray("category"); 13420 for (int i = 0; i < array.size(); i++) { 13421 res.getCategory().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 13422 } 13423 }; 13424 if (json.has("code")) 13425 res.setCode(parseCodeableConcept(getJObject(json, "code"))); 13426 if (json.has("subject")) 13427 res.setSubject(parseReference(getJObject(json, "subject"))); 13428 if (json.has("context")) 13429 res.setContext(parseReference(getJObject(json, "context"))); 13430 Type effective = parseType("effective", json); 13431 if (effective != null) 13432 res.setEffective(effective); 13433 if (json.has("issued")) 13434 res.setIssuedElement(parseInstant(json.get("issued").getAsString())); 13435 if (json.has("_issued")) 13436 parseElementProperties(getJObject(json, "_issued"), res.getIssuedElement()); 13437 if (json.has("performer")) { 13438 JsonArray array = json.getAsJsonArray("performer"); 13439 for (int i = 0; i < array.size(); i++) { 13440 res.getPerformer().add(parseReference(array.get(i).getAsJsonObject())); 13441 } 13442 }; 13443 Type value = parseType("value", json); 13444 if (value != null) 13445 res.setValue(value); 13446 if (json.has("dataAbsentReason")) 13447 res.setDataAbsentReason(parseCodeableConcept(getJObject(json, "dataAbsentReason"))); 13448 if (json.has("interpretation")) 13449 res.setInterpretation(parseCodeableConcept(getJObject(json, "interpretation"))); 13450 if (json.has("comment")) 13451 res.setCommentElement(parseString(json.get("comment").getAsString())); 13452 if (json.has("_comment")) 13453 parseElementProperties(getJObject(json, "_comment"), res.getCommentElement()); 13454 if (json.has("bodySite")) 13455 res.setBodySite(parseCodeableConcept(getJObject(json, "bodySite"))); 13456 if (json.has("method")) 13457 res.setMethod(parseCodeableConcept(getJObject(json, "method"))); 13458 if (json.has("specimen")) 13459 res.setSpecimen(parseReference(getJObject(json, "specimen"))); 13460 if (json.has("device")) 13461 res.setDevice(parseReference(getJObject(json, "device"))); 13462 if (json.has("referenceRange")) { 13463 JsonArray array = json.getAsJsonArray("referenceRange"); 13464 for (int i = 0; i < array.size(); i++) { 13465 res.getReferenceRange().add(parseObservationObservationReferenceRangeComponent(array.get(i).getAsJsonObject(), res)); 13466 } 13467 }; 13468 if (json.has("related")) { 13469 JsonArray array = json.getAsJsonArray("related"); 13470 for (int i = 0; i < array.size(); i++) { 13471 res.getRelated().add(parseObservationObservationRelatedComponent(array.get(i).getAsJsonObject(), res)); 13472 } 13473 }; 13474 if (json.has("component")) { 13475 JsonArray array = json.getAsJsonArray("component"); 13476 for (int i = 0; i < array.size(); i++) { 13477 res.getComponent().add(parseObservationObservationComponentComponent(array.get(i).getAsJsonObject(), res)); 13478 } 13479 }; 13480 } 13481 13482 protected Observation.ObservationReferenceRangeComponent parseObservationObservationReferenceRangeComponent(JsonObject json, Observation owner) throws IOException, FHIRFormatError { 13483 Observation.ObservationReferenceRangeComponent res = new Observation.ObservationReferenceRangeComponent(); 13484 parseObservationObservationReferenceRangeComponentProperties(json, owner, res); 13485 return res; 13486 } 13487 13488 protected void parseObservationObservationReferenceRangeComponentProperties(JsonObject json, Observation owner, Observation.ObservationReferenceRangeComponent res) throws IOException, FHIRFormatError { 13489 parseBackboneProperties(json, res); 13490 if (json.has("low")) 13491 res.setLow(parseSimpleQuantity(getJObject(json, "low"))); 13492 if (json.has("high")) 13493 res.setHigh(parseSimpleQuantity(getJObject(json, "high"))); 13494 if (json.has("type")) 13495 res.setType(parseCodeableConcept(getJObject(json, "type"))); 13496 if (json.has("appliesTo")) { 13497 JsonArray array = json.getAsJsonArray("appliesTo"); 13498 for (int i = 0; i < array.size(); i++) { 13499 res.getAppliesTo().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 13500 } 13501 }; 13502 if (json.has("age")) 13503 res.setAge(parseRange(getJObject(json, "age"))); 13504 if (json.has("text")) 13505 res.setTextElement(parseString(json.get("text").getAsString())); 13506 if (json.has("_text")) 13507 parseElementProperties(getJObject(json, "_text"), res.getTextElement()); 13508 } 13509 13510 protected Observation.ObservationRelatedComponent parseObservationObservationRelatedComponent(JsonObject json, Observation owner) throws IOException, FHIRFormatError { 13511 Observation.ObservationRelatedComponent res = new Observation.ObservationRelatedComponent(); 13512 parseObservationObservationRelatedComponentProperties(json, owner, res); 13513 return res; 13514 } 13515 13516 protected void parseObservationObservationRelatedComponentProperties(JsonObject json, Observation owner, Observation.ObservationRelatedComponent res) throws IOException, FHIRFormatError { 13517 parseBackboneProperties(json, res); 13518 if (json.has("type")) 13519 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), Observation.ObservationRelationshipType.NULL, new Observation.ObservationRelationshipTypeEnumFactory())); 13520 if (json.has("_type")) 13521 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 13522 if (json.has("target")) 13523 res.setTarget(parseReference(getJObject(json, "target"))); 13524 } 13525 13526 protected Observation.ObservationComponentComponent parseObservationObservationComponentComponent(JsonObject json, Observation owner) throws IOException, FHIRFormatError { 13527 Observation.ObservationComponentComponent res = new Observation.ObservationComponentComponent(); 13528 parseObservationObservationComponentComponentProperties(json, owner, res); 13529 return res; 13530 } 13531 13532 protected void parseObservationObservationComponentComponentProperties(JsonObject json, Observation owner, Observation.ObservationComponentComponent res) throws IOException, FHIRFormatError { 13533 parseBackboneProperties(json, res); 13534 if (json.has("code")) 13535 res.setCode(parseCodeableConcept(getJObject(json, "code"))); 13536 Type value = parseType("value", json); 13537 if (value != null) 13538 res.setValue(value); 13539 if (json.has("dataAbsentReason")) 13540 res.setDataAbsentReason(parseCodeableConcept(getJObject(json, "dataAbsentReason"))); 13541 if (json.has("interpretation")) 13542 res.setInterpretation(parseCodeableConcept(getJObject(json, "interpretation"))); 13543 if (json.has("referenceRange")) { 13544 JsonArray array = json.getAsJsonArray("referenceRange"); 13545 for (int i = 0; i < array.size(); i++) { 13546 res.getReferenceRange().add(parseObservationObservationReferenceRangeComponent(array.get(i).getAsJsonObject(), owner)); 13547 } 13548 }; 13549 } 13550 13551 protected OperationDefinition parseOperationDefinition(JsonObject json) throws IOException, FHIRFormatError { 13552 OperationDefinition res = new OperationDefinition(); 13553 parseOperationDefinitionProperties(json, res); 13554 return res; 13555 } 13556 13557 protected void parseOperationDefinitionProperties(JsonObject json, OperationDefinition res) throws IOException, FHIRFormatError { 13558 parseDomainResourceProperties(json, res); 13559 if (json.has("url")) 13560 res.setUrlElement(parseUri(json.get("url").getAsString())); 13561 if (json.has("_url")) 13562 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 13563 if (json.has("version")) 13564 res.setVersionElement(parseString(json.get("version").getAsString())); 13565 if (json.has("_version")) 13566 parseElementProperties(getJObject(json, "_version"), res.getVersionElement()); 13567 if (json.has("name")) 13568 res.setNameElement(parseString(json.get("name").getAsString())); 13569 if (json.has("_name")) 13570 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 13571 if (json.has("status")) 13572 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Enumerations.PublicationStatus.NULL, new Enumerations.PublicationStatusEnumFactory())); 13573 if (json.has("_status")) 13574 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 13575 if (json.has("kind")) 13576 res.setKindElement(parseEnumeration(json.get("kind").getAsString(), OperationDefinition.OperationKind.NULL, new OperationDefinition.OperationKindEnumFactory())); 13577 if (json.has("_kind")) 13578 parseElementProperties(getJObject(json, "_kind"), res.getKindElement()); 13579 if (json.has("experimental")) 13580 res.setExperimentalElement(parseBoolean(json.get("experimental").getAsBoolean())); 13581 if (json.has("_experimental")) 13582 parseElementProperties(getJObject(json, "_experimental"), res.getExperimentalElement()); 13583 if (json.has("date")) 13584 res.setDateElement(parseDateTime(json.get("date").getAsString())); 13585 if (json.has("_date")) 13586 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 13587 if (json.has("publisher")) 13588 res.setPublisherElement(parseString(json.get("publisher").getAsString())); 13589 if (json.has("_publisher")) 13590 parseElementProperties(getJObject(json, "_publisher"), res.getPublisherElement()); 13591 if (json.has("contact")) { 13592 JsonArray array = json.getAsJsonArray("contact"); 13593 for (int i = 0; i < array.size(); i++) { 13594 res.getContact().add(parseContactDetail(array.get(i).getAsJsonObject())); 13595 } 13596 }; 13597 if (json.has("description")) 13598 res.setDescriptionElement(parseMarkdown(json.get("description").getAsString())); 13599 if (json.has("_description")) 13600 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 13601 if (json.has("useContext")) { 13602 JsonArray array = json.getAsJsonArray("useContext"); 13603 for (int i = 0; i < array.size(); i++) { 13604 res.getUseContext().add(parseUsageContext(array.get(i).getAsJsonObject())); 13605 } 13606 }; 13607 if (json.has("jurisdiction")) { 13608 JsonArray array = json.getAsJsonArray("jurisdiction"); 13609 for (int i = 0; i < array.size(); i++) { 13610 res.getJurisdiction().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 13611 } 13612 }; 13613 if (json.has("purpose")) 13614 res.setPurposeElement(parseMarkdown(json.get("purpose").getAsString())); 13615 if (json.has("_purpose")) 13616 parseElementProperties(getJObject(json, "_purpose"), res.getPurposeElement()); 13617 if (json.has("idempotent")) 13618 res.setIdempotentElement(parseBoolean(json.get("idempotent").getAsBoolean())); 13619 if (json.has("_idempotent")) 13620 parseElementProperties(getJObject(json, "_idempotent"), res.getIdempotentElement()); 13621 if (json.has("code")) 13622 res.setCodeElement(parseCode(json.get("code").getAsString())); 13623 if (json.has("_code")) 13624 parseElementProperties(getJObject(json, "_code"), res.getCodeElement()); 13625 if (json.has("comment")) 13626 res.setCommentElement(parseString(json.get("comment").getAsString())); 13627 if (json.has("_comment")) 13628 parseElementProperties(getJObject(json, "_comment"), res.getCommentElement()); 13629 if (json.has("base")) 13630 res.setBase(parseReference(getJObject(json, "base"))); 13631 if (json.has("resource")) { 13632 JsonArray array = json.getAsJsonArray("resource"); 13633 for (int i = 0; i < array.size(); i++) { 13634 if (array.get(i).isJsonNull()) { 13635 res.getResource().add(new CodeType()); 13636 } else { 13637 res.getResource().add(parseCode(array.get(i).getAsString())); 13638 } 13639 } 13640 }; 13641 if (json.has("_resource")) { 13642 JsonArray array = json.getAsJsonArray("_resource"); 13643 for (int i = 0; i < array.size(); i++) { 13644 if (i == res.getResource().size()) 13645 res.getResource().add(parseCode(null)); 13646 if (array.get(i) instanceof JsonObject) 13647 parseElementProperties(array.get(i).getAsJsonObject(), res.getResource().get(i)); 13648 } 13649 }; 13650 if (json.has("system")) 13651 res.setSystemElement(parseBoolean(json.get("system").getAsBoolean())); 13652 if (json.has("_system")) 13653 parseElementProperties(getJObject(json, "_system"), res.getSystemElement()); 13654 if (json.has("type")) 13655 res.setTypeElement(parseBoolean(json.get("type").getAsBoolean())); 13656 if (json.has("_type")) 13657 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 13658 if (json.has("instance")) 13659 res.setInstanceElement(parseBoolean(json.get("instance").getAsBoolean())); 13660 if (json.has("_instance")) 13661 parseElementProperties(getJObject(json, "_instance"), res.getInstanceElement()); 13662 if (json.has("parameter")) { 13663 JsonArray array = json.getAsJsonArray("parameter"); 13664 for (int i = 0; i < array.size(); i++) { 13665 res.getParameter().add(parseOperationDefinitionOperationDefinitionParameterComponent(array.get(i).getAsJsonObject(), res)); 13666 } 13667 }; 13668 if (json.has("overload")) { 13669 JsonArray array = json.getAsJsonArray("overload"); 13670 for (int i = 0; i < array.size(); i++) { 13671 res.getOverload().add(parseOperationDefinitionOperationDefinitionOverloadComponent(array.get(i).getAsJsonObject(), res)); 13672 } 13673 }; 13674 } 13675 13676 protected OperationDefinition.OperationDefinitionParameterComponent parseOperationDefinitionOperationDefinitionParameterComponent(JsonObject json, OperationDefinition owner) throws IOException, FHIRFormatError { 13677 OperationDefinition.OperationDefinitionParameterComponent res = new OperationDefinition.OperationDefinitionParameterComponent(); 13678 parseOperationDefinitionOperationDefinitionParameterComponentProperties(json, owner, res); 13679 return res; 13680 } 13681 13682 protected void parseOperationDefinitionOperationDefinitionParameterComponentProperties(JsonObject json, OperationDefinition owner, OperationDefinition.OperationDefinitionParameterComponent res) throws IOException, FHIRFormatError { 13683 parseBackboneProperties(json, res); 13684 if (json.has("name")) 13685 res.setNameElement(parseCode(json.get("name").getAsString())); 13686 if (json.has("_name")) 13687 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 13688 if (json.has("use")) 13689 res.setUseElement(parseEnumeration(json.get("use").getAsString(), OperationDefinition.OperationParameterUse.NULL, new OperationDefinition.OperationParameterUseEnumFactory())); 13690 if (json.has("_use")) 13691 parseElementProperties(getJObject(json, "_use"), res.getUseElement()); 13692 if (json.has("min")) 13693 res.setMinElement(parseInteger(json.get("min").getAsLong())); 13694 if (json.has("_min")) 13695 parseElementProperties(getJObject(json, "_min"), res.getMinElement()); 13696 if (json.has("max")) 13697 res.setMaxElement(parseString(json.get("max").getAsString())); 13698 if (json.has("_max")) 13699 parseElementProperties(getJObject(json, "_max"), res.getMaxElement()); 13700 if (json.has("documentation")) 13701 res.setDocumentationElement(parseString(json.get("documentation").getAsString())); 13702 if (json.has("_documentation")) 13703 parseElementProperties(getJObject(json, "_documentation"), res.getDocumentationElement()); 13704 if (json.has("type")) 13705 res.setTypeElement(parseCode(json.get("type").getAsString())); 13706 if (json.has("_type")) 13707 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 13708 if (json.has("searchType")) 13709 res.setSearchTypeElement(parseEnumeration(json.get("searchType").getAsString(), Enumerations.SearchParamType.NULL, new Enumerations.SearchParamTypeEnumFactory())); 13710 if (json.has("_searchType")) 13711 parseElementProperties(getJObject(json, "_searchType"), res.getSearchTypeElement()); 13712 if (json.has("profile")) 13713 res.setProfile(parseReference(getJObject(json, "profile"))); 13714 if (json.has("binding")) 13715 res.setBinding(parseOperationDefinitionOperationDefinitionParameterBindingComponent(getJObject(json, "binding"), owner)); 13716 if (json.has("part")) { 13717 JsonArray array = json.getAsJsonArray("part"); 13718 for (int i = 0; i < array.size(); i++) { 13719 res.getPart().add(parseOperationDefinitionOperationDefinitionParameterComponent(array.get(i).getAsJsonObject(), owner)); 13720 } 13721 }; 13722 } 13723 13724 protected OperationDefinition.OperationDefinitionParameterBindingComponent parseOperationDefinitionOperationDefinitionParameterBindingComponent(JsonObject json, OperationDefinition owner) throws IOException, FHIRFormatError { 13725 OperationDefinition.OperationDefinitionParameterBindingComponent res = new OperationDefinition.OperationDefinitionParameterBindingComponent(); 13726 parseOperationDefinitionOperationDefinitionParameterBindingComponentProperties(json, owner, res); 13727 return res; 13728 } 13729 13730 protected void parseOperationDefinitionOperationDefinitionParameterBindingComponentProperties(JsonObject json, OperationDefinition owner, OperationDefinition.OperationDefinitionParameterBindingComponent res) throws IOException, FHIRFormatError { 13731 parseBackboneProperties(json, res); 13732 if (json.has("strength")) 13733 res.setStrengthElement(parseEnumeration(json.get("strength").getAsString(), Enumerations.BindingStrength.NULL, new Enumerations.BindingStrengthEnumFactory())); 13734 if (json.has("_strength")) 13735 parseElementProperties(getJObject(json, "_strength"), res.getStrengthElement()); 13736 Type valueSet = parseType("valueSet", json); 13737 if (valueSet != null) 13738 res.setValueSet(valueSet); 13739 } 13740 13741 protected OperationDefinition.OperationDefinitionOverloadComponent parseOperationDefinitionOperationDefinitionOverloadComponent(JsonObject json, OperationDefinition owner) throws IOException, FHIRFormatError { 13742 OperationDefinition.OperationDefinitionOverloadComponent res = new OperationDefinition.OperationDefinitionOverloadComponent(); 13743 parseOperationDefinitionOperationDefinitionOverloadComponentProperties(json, owner, res); 13744 return res; 13745 } 13746 13747 protected void parseOperationDefinitionOperationDefinitionOverloadComponentProperties(JsonObject json, OperationDefinition owner, OperationDefinition.OperationDefinitionOverloadComponent res) throws IOException, FHIRFormatError { 13748 parseBackboneProperties(json, res); 13749 if (json.has("parameterName")) { 13750 JsonArray array = json.getAsJsonArray("parameterName"); 13751 for (int i = 0; i < array.size(); i++) { 13752 if (array.get(i).isJsonNull()) { 13753 res.getParameterName().add(new StringType()); 13754 } else { 13755 res.getParameterName().add(parseString(array.get(i).getAsString())); 13756 } 13757 } 13758 }; 13759 if (json.has("_parameterName")) { 13760 JsonArray array = json.getAsJsonArray("_parameterName"); 13761 for (int i = 0; i < array.size(); i++) { 13762 if (i == res.getParameterName().size()) 13763 res.getParameterName().add(parseString(null)); 13764 if (array.get(i) instanceof JsonObject) 13765 parseElementProperties(array.get(i).getAsJsonObject(), res.getParameterName().get(i)); 13766 } 13767 }; 13768 if (json.has("comment")) 13769 res.setCommentElement(parseString(json.get("comment").getAsString())); 13770 if (json.has("_comment")) 13771 parseElementProperties(getJObject(json, "_comment"), res.getCommentElement()); 13772 } 13773 13774 protected OperationOutcome parseOperationOutcome(JsonObject json) throws IOException, FHIRFormatError { 13775 OperationOutcome res = new OperationOutcome(); 13776 parseOperationOutcomeProperties(json, res); 13777 return res; 13778 } 13779 13780 protected void parseOperationOutcomeProperties(JsonObject json, OperationOutcome res) throws IOException, FHIRFormatError { 13781 parseDomainResourceProperties(json, res); 13782 if (json.has("issue")) { 13783 JsonArray array = json.getAsJsonArray("issue"); 13784 for (int i = 0; i < array.size(); i++) { 13785 res.getIssue().add(parseOperationOutcomeOperationOutcomeIssueComponent(array.get(i).getAsJsonObject(), res)); 13786 } 13787 }; 13788 } 13789 13790 protected OperationOutcome.OperationOutcomeIssueComponent parseOperationOutcomeOperationOutcomeIssueComponent(JsonObject json, OperationOutcome owner) throws IOException, FHIRFormatError { 13791 OperationOutcome.OperationOutcomeIssueComponent res = new OperationOutcome.OperationOutcomeIssueComponent(); 13792 parseOperationOutcomeOperationOutcomeIssueComponentProperties(json, owner, res); 13793 return res; 13794 } 13795 13796 protected void parseOperationOutcomeOperationOutcomeIssueComponentProperties(JsonObject json, OperationOutcome owner, OperationOutcome.OperationOutcomeIssueComponent res) throws IOException, FHIRFormatError { 13797 parseBackboneProperties(json, res); 13798 if (json.has("severity")) 13799 res.setSeverityElement(parseEnumeration(json.get("severity").getAsString(), OperationOutcome.IssueSeverity.NULL, new OperationOutcome.IssueSeverityEnumFactory())); 13800 if (json.has("_severity")) 13801 parseElementProperties(getJObject(json, "_severity"), res.getSeverityElement()); 13802 if (json.has("code")) 13803 res.setCodeElement(parseEnumeration(json.get("code").getAsString(), OperationOutcome.IssueType.NULL, new OperationOutcome.IssueTypeEnumFactory())); 13804 if (json.has("_code")) 13805 parseElementProperties(getJObject(json, "_code"), res.getCodeElement()); 13806 if (json.has("details")) 13807 res.setDetails(parseCodeableConcept(getJObject(json, "details"))); 13808 if (json.has("diagnostics")) 13809 res.setDiagnosticsElement(parseString(json.get("diagnostics").getAsString())); 13810 if (json.has("_diagnostics")) 13811 parseElementProperties(getJObject(json, "_diagnostics"), res.getDiagnosticsElement()); 13812 if (json.has("location")) { 13813 JsonArray array = json.getAsJsonArray("location"); 13814 for (int i = 0; i < array.size(); i++) { 13815 if (array.get(i).isJsonNull()) { 13816 res.getLocation().add(new StringType()); 13817 } else { 13818 res.getLocation().add(parseString(array.get(i).getAsString())); 13819 } 13820 } 13821 }; 13822 if (json.has("_location")) { 13823 JsonArray array = json.getAsJsonArray("_location"); 13824 for (int i = 0; i < array.size(); i++) { 13825 if (i == res.getLocation().size()) 13826 res.getLocation().add(parseString(null)); 13827 if (array.get(i) instanceof JsonObject) 13828 parseElementProperties(array.get(i).getAsJsonObject(), res.getLocation().get(i)); 13829 } 13830 }; 13831 if (json.has("expression")) { 13832 JsonArray array = json.getAsJsonArray("expression"); 13833 for (int i = 0; i < array.size(); i++) { 13834 if (array.get(i).isJsonNull()) { 13835 res.getExpression().add(new StringType()); 13836 } else { 13837 res.getExpression().add(parseString(array.get(i).getAsString())); 13838 } 13839 } 13840 }; 13841 if (json.has("_expression")) { 13842 JsonArray array = json.getAsJsonArray("_expression"); 13843 for (int i = 0; i < array.size(); i++) { 13844 if (i == res.getExpression().size()) 13845 res.getExpression().add(parseString(null)); 13846 if (array.get(i) instanceof JsonObject) 13847 parseElementProperties(array.get(i).getAsJsonObject(), res.getExpression().get(i)); 13848 } 13849 }; 13850 } 13851 13852 protected Organization parseOrganization(JsonObject json) throws IOException, FHIRFormatError { 13853 Organization res = new Organization(); 13854 parseOrganizationProperties(json, res); 13855 return res; 13856 } 13857 13858 protected void parseOrganizationProperties(JsonObject json, Organization res) throws IOException, FHIRFormatError { 13859 parseDomainResourceProperties(json, res); 13860 if (json.has("identifier")) { 13861 JsonArray array = json.getAsJsonArray("identifier"); 13862 for (int i = 0; i < array.size(); i++) { 13863 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 13864 } 13865 }; 13866 if (json.has("active")) 13867 res.setActiveElement(parseBoolean(json.get("active").getAsBoolean())); 13868 if (json.has("_active")) 13869 parseElementProperties(getJObject(json, "_active"), res.getActiveElement()); 13870 if (json.has("type")) { 13871 JsonArray array = json.getAsJsonArray("type"); 13872 for (int i = 0; i < array.size(); i++) { 13873 res.getType().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 13874 } 13875 }; 13876 if (json.has("name")) 13877 res.setNameElement(parseString(json.get("name").getAsString())); 13878 if (json.has("_name")) 13879 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 13880 if (json.has("alias")) { 13881 JsonArray array = json.getAsJsonArray("alias"); 13882 for (int i = 0; i < array.size(); i++) { 13883 if (array.get(i).isJsonNull()) { 13884 res.getAlias().add(new StringType()); 13885 } else { 13886 res.getAlias().add(parseString(array.get(i).getAsString())); 13887 } 13888 } 13889 }; 13890 if (json.has("_alias")) { 13891 JsonArray array = json.getAsJsonArray("_alias"); 13892 for (int i = 0; i < array.size(); i++) { 13893 if (i == res.getAlias().size()) 13894 res.getAlias().add(parseString(null)); 13895 if (array.get(i) instanceof JsonObject) 13896 parseElementProperties(array.get(i).getAsJsonObject(), res.getAlias().get(i)); 13897 } 13898 }; 13899 if (json.has("telecom")) { 13900 JsonArray array = json.getAsJsonArray("telecom"); 13901 for (int i = 0; i < array.size(); i++) { 13902 res.getTelecom().add(parseContactPoint(array.get(i).getAsJsonObject())); 13903 } 13904 }; 13905 if (json.has("address")) { 13906 JsonArray array = json.getAsJsonArray("address"); 13907 for (int i = 0; i < array.size(); i++) { 13908 res.getAddress().add(parseAddress(array.get(i).getAsJsonObject())); 13909 } 13910 }; 13911 if (json.has("partOf")) 13912 res.setPartOf(parseReference(getJObject(json, "partOf"))); 13913 if (json.has("contact")) { 13914 JsonArray array = json.getAsJsonArray("contact"); 13915 for (int i = 0; i < array.size(); i++) { 13916 res.getContact().add(parseOrganizationOrganizationContactComponent(array.get(i).getAsJsonObject(), res)); 13917 } 13918 }; 13919 if (json.has("endpoint")) { 13920 JsonArray array = json.getAsJsonArray("endpoint"); 13921 for (int i = 0; i < array.size(); i++) { 13922 res.getEndpoint().add(parseReference(array.get(i).getAsJsonObject())); 13923 } 13924 }; 13925 } 13926 13927 protected Organization.OrganizationContactComponent parseOrganizationOrganizationContactComponent(JsonObject json, Organization owner) throws IOException, FHIRFormatError { 13928 Organization.OrganizationContactComponent res = new Organization.OrganizationContactComponent(); 13929 parseOrganizationOrganizationContactComponentProperties(json, owner, res); 13930 return res; 13931 } 13932 13933 protected void parseOrganizationOrganizationContactComponentProperties(JsonObject json, Organization owner, Organization.OrganizationContactComponent res) throws IOException, FHIRFormatError { 13934 parseBackboneProperties(json, res); 13935 if (json.has("purpose")) 13936 res.setPurpose(parseCodeableConcept(getJObject(json, "purpose"))); 13937 if (json.has("name")) 13938 res.setName(parseHumanName(getJObject(json, "name"))); 13939 if (json.has("telecom")) { 13940 JsonArray array = json.getAsJsonArray("telecom"); 13941 for (int i = 0; i < array.size(); i++) { 13942 res.getTelecom().add(parseContactPoint(array.get(i).getAsJsonObject())); 13943 } 13944 }; 13945 if (json.has("address")) 13946 res.setAddress(parseAddress(getJObject(json, "address"))); 13947 } 13948 13949 protected Patient parsePatient(JsonObject json) throws IOException, FHIRFormatError { 13950 Patient res = new Patient(); 13951 parsePatientProperties(json, res); 13952 return res; 13953 } 13954 13955 protected void parsePatientProperties(JsonObject json, Patient res) throws IOException, FHIRFormatError { 13956 parseDomainResourceProperties(json, res); 13957 if (json.has("identifier")) { 13958 JsonArray array = json.getAsJsonArray("identifier"); 13959 for (int i = 0; i < array.size(); i++) { 13960 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 13961 } 13962 }; 13963 if (json.has("active")) 13964 res.setActiveElement(parseBoolean(json.get("active").getAsBoolean())); 13965 if (json.has("_active")) 13966 parseElementProperties(getJObject(json, "_active"), res.getActiveElement()); 13967 if (json.has("name")) { 13968 JsonArray array = json.getAsJsonArray("name"); 13969 for (int i = 0; i < array.size(); i++) { 13970 res.getName().add(parseHumanName(array.get(i).getAsJsonObject())); 13971 } 13972 }; 13973 if (json.has("telecom")) { 13974 JsonArray array = json.getAsJsonArray("telecom"); 13975 for (int i = 0; i < array.size(); i++) { 13976 res.getTelecom().add(parseContactPoint(array.get(i).getAsJsonObject())); 13977 } 13978 }; 13979 if (json.has("gender")) 13980 res.setGenderElement(parseEnumeration(json.get("gender").getAsString(), Enumerations.AdministrativeGender.NULL, new Enumerations.AdministrativeGenderEnumFactory())); 13981 if (json.has("_gender")) 13982 parseElementProperties(getJObject(json, "_gender"), res.getGenderElement()); 13983 if (json.has("birthDate")) 13984 res.setBirthDateElement(parseDate(json.get("birthDate").getAsString())); 13985 if (json.has("_birthDate")) 13986 parseElementProperties(getJObject(json, "_birthDate"), res.getBirthDateElement()); 13987 Type deceased = parseType("deceased", json); 13988 if (deceased != null) 13989 res.setDeceased(deceased); 13990 if (json.has("address")) { 13991 JsonArray array = json.getAsJsonArray("address"); 13992 for (int i = 0; i < array.size(); i++) { 13993 res.getAddress().add(parseAddress(array.get(i).getAsJsonObject())); 13994 } 13995 }; 13996 if (json.has("maritalStatus")) 13997 res.setMaritalStatus(parseCodeableConcept(getJObject(json, "maritalStatus"))); 13998 Type multipleBirth = parseType("multipleBirth", json); 13999 if (multipleBirth != null) 14000 res.setMultipleBirth(multipleBirth); 14001 if (json.has("photo")) { 14002 JsonArray array = json.getAsJsonArray("photo"); 14003 for (int i = 0; i < array.size(); i++) { 14004 res.getPhoto().add(parseAttachment(array.get(i).getAsJsonObject())); 14005 } 14006 }; 14007 if (json.has("contact")) { 14008 JsonArray array = json.getAsJsonArray("contact"); 14009 for (int i = 0; i < array.size(); i++) { 14010 res.getContact().add(parsePatientContactComponent(array.get(i).getAsJsonObject(), res)); 14011 } 14012 }; 14013 if (json.has("animal")) 14014 res.setAnimal(parsePatientAnimalComponent(getJObject(json, "animal"), res)); 14015 if (json.has("communication")) { 14016 JsonArray array = json.getAsJsonArray("communication"); 14017 for (int i = 0; i < array.size(); i++) { 14018 res.getCommunication().add(parsePatientPatientCommunicationComponent(array.get(i).getAsJsonObject(), res)); 14019 } 14020 }; 14021 if (json.has("generalPractitioner")) { 14022 JsonArray array = json.getAsJsonArray("generalPractitioner"); 14023 for (int i = 0; i < array.size(); i++) { 14024 res.getGeneralPractitioner().add(parseReference(array.get(i).getAsJsonObject())); 14025 } 14026 }; 14027 if (json.has("managingOrganization")) 14028 res.setManagingOrganization(parseReference(getJObject(json, "managingOrganization"))); 14029 if (json.has("link")) { 14030 JsonArray array = json.getAsJsonArray("link"); 14031 for (int i = 0; i < array.size(); i++) { 14032 res.getLink().add(parsePatientPatientLinkComponent(array.get(i).getAsJsonObject(), res)); 14033 } 14034 }; 14035 } 14036 14037 protected Patient.ContactComponent parsePatientContactComponent(JsonObject json, Patient owner) throws IOException, FHIRFormatError { 14038 Patient.ContactComponent res = new Patient.ContactComponent(); 14039 parsePatientContactComponentProperties(json, owner, res); 14040 return res; 14041 } 14042 14043 protected void parsePatientContactComponentProperties(JsonObject json, Patient owner, Patient.ContactComponent res) throws IOException, FHIRFormatError { 14044 parseBackboneProperties(json, res); 14045 if (json.has("relationship")) { 14046 JsonArray array = json.getAsJsonArray("relationship"); 14047 for (int i = 0; i < array.size(); i++) { 14048 res.getRelationship().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 14049 } 14050 }; 14051 if (json.has("name")) 14052 res.setName(parseHumanName(getJObject(json, "name"))); 14053 if (json.has("telecom")) { 14054 JsonArray array = json.getAsJsonArray("telecom"); 14055 for (int i = 0; i < array.size(); i++) { 14056 res.getTelecom().add(parseContactPoint(array.get(i).getAsJsonObject())); 14057 } 14058 }; 14059 if (json.has("address")) 14060 res.setAddress(parseAddress(getJObject(json, "address"))); 14061 if (json.has("gender")) 14062 res.setGenderElement(parseEnumeration(json.get("gender").getAsString(), Enumerations.AdministrativeGender.NULL, new Enumerations.AdministrativeGenderEnumFactory())); 14063 if (json.has("_gender")) 14064 parseElementProperties(getJObject(json, "_gender"), res.getGenderElement()); 14065 if (json.has("organization")) 14066 res.setOrganization(parseReference(getJObject(json, "organization"))); 14067 if (json.has("period")) 14068 res.setPeriod(parsePeriod(getJObject(json, "period"))); 14069 } 14070 14071 protected Patient.AnimalComponent parsePatientAnimalComponent(JsonObject json, Patient owner) throws IOException, FHIRFormatError { 14072 Patient.AnimalComponent res = new Patient.AnimalComponent(); 14073 parsePatientAnimalComponentProperties(json, owner, res); 14074 return res; 14075 } 14076 14077 protected void parsePatientAnimalComponentProperties(JsonObject json, Patient owner, Patient.AnimalComponent res) throws IOException, FHIRFormatError { 14078 parseBackboneProperties(json, res); 14079 if (json.has("species")) 14080 res.setSpecies(parseCodeableConcept(getJObject(json, "species"))); 14081 if (json.has("breed")) 14082 res.setBreed(parseCodeableConcept(getJObject(json, "breed"))); 14083 if (json.has("genderStatus")) 14084 res.setGenderStatus(parseCodeableConcept(getJObject(json, "genderStatus"))); 14085 } 14086 14087 protected Patient.PatientCommunicationComponent parsePatientPatientCommunicationComponent(JsonObject json, Patient owner) throws IOException, FHIRFormatError { 14088 Patient.PatientCommunicationComponent res = new Patient.PatientCommunicationComponent(); 14089 parsePatientPatientCommunicationComponentProperties(json, owner, res); 14090 return res; 14091 } 14092 14093 protected void parsePatientPatientCommunicationComponentProperties(JsonObject json, Patient owner, Patient.PatientCommunicationComponent res) throws IOException, FHIRFormatError { 14094 parseBackboneProperties(json, res); 14095 if (json.has("language")) 14096 res.setLanguage(parseCodeableConcept(getJObject(json, "language"))); 14097 if (json.has("preferred")) 14098 res.setPreferredElement(parseBoolean(json.get("preferred").getAsBoolean())); 14099 if (json.has("_preferred")) 14100 parseElementProperties(getJObject(json, "_preferred"), res.getPreferredElement()); 14101 } 14102 14103 protected Patient.PatientLinkComponent parsePatientPatientLinkComponent(JsonObject json, Patient owner) throws IOException, FHIRFormatError { 14104 Patient.PatientLinkComponent res = new Patient.PatientLinkComponent(); 14105 parsePatientPatientLinkComponentProperties(json, owner, res); 14106 return res; 14107 } 14108 14109 protected void parsePatientPatientLinkComponentProperties(JsonObject json, Patient owner, Patient.PatientLinkComponent res) throws IOException, FHIRFormatError { 14110 parseBackboneProperties(json, res); 14111 if (json.has("other")) 14112 res.setOther(parseReference(getJObject(json, "other"))); 14113 if (json.has("type")) 14114 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), Patient.LinkType.NULL, new Patient.LinkTypeEnumFactory())); 14115 if (json.has("_type")) 14116 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 14117 } 14118 14119 protected PaymentNotice parsePaymentNotice(JsonObject json) throws IOException, FHIRFormatError { 14120 PaymentNotice res = new PaymentNotice(); 14121 parsePaymentNoticeProperties(json, res); 14122 return res; 14123 } 14124 14125 protected void parsePaymentNoticeProperties(JsonObject json, PaymentNotice res) throws IOException, FHIRFormatError { 14126 parseDomainResourceProperties(json, res); 14127 if (json.has("identifier")) { 14128 JsonArray array = json.getAsJsonArray("identifier"); 14129 for (int i = 0; i < array.size(); i++) { 14130 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 14131 } 14132 }; 14133 if (json.has("status")) 14134 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), PaymentNotice.PaymentNoticeStatus.NULL, new PaymentNotice.PaymentNoticeStatusEnumFactory())); 14135 if (json.has("_status")) 14136 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 14137 if (json.has("request")) 14138 res.setRequest(parseReference(getJObject(json, "request"))); 14139 if (json.has("response")) 14140 res.setResponse(parseReference(getJObject(json, "response"))); 14141 if (json.has("statusDate")) 14142 res.setStatusDateElement(parseDate(json.get("statusDate").getAsString())); 14143 if (json.has("_statusDate")) 14144 parseElementProperties(getJObject(json, "_statusDate"), res.getStatusDateElement()); 14145 if (json.has("created")) 14146 res.setCreatedElement(parseDateTime(json.get("created").getAsString())); 14147 if (json.has("_created")) 14148 parseElementProperties(getJObject(json, "_created"), res.getCreatedElement()); 14149 if (json.has("target")) 14150 res.setTarget(parseReference(getJObject(json, "target"))); 14151 if (json.has("provider")) 14152 res.setProvider(parseReference(getJObject(json, "provider"))); 14153 if (json.has("organization")) 14154 res.setOrganization(parseReference(getJObject(json, "organization"))); 14155 if (json.has("paymentStatus")) 14156 res.setPaymentStatus(parseCodeableConcept(getJObject(json, "paymentStatus"))); 14157 } 14158 14159 protected PaymentReconciliation parsePaymentReconciliation(JsonObject json) throws IOException, FHIRFormatError { 14160 PaymentReconciliation res = new PaymentReconciliation(); 14161 parsePaymentReconciliationProperties(json, res); 14162 return res; 14163 } 14164 14165 protected void parsePaymentReconciliationProperties(JsonObject json, PaymentReconciliation res) throws IOException, FHIRFormatError { 14166 parseDomainResourceProperties(json, res); 14167 if (json.has("identifier")) { 14168 JsonArray array = json.getAsJsonArray("identifier"); 14169 for (int i = 0; i < array.size(); i++) { 14170 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 14171 } 14172 }; 14173 if (json.has("status")) 14174 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), PaymentReconciliation.PaymentReconciliationStatus.NULL, new PaymentReconciliation.PaymentReconciliationStatusEnumFactory())); 14175 if (json.has("_status")) 14176 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 14177 if (json.has("period")) 14178 res.setPeriod(parsePeriod(getJObject(json, "period"))); 14179 if (json.has("created")) 14180 res.setCreatedElement(parseDateTime(json.get("created").getAsString())); 14181 if (json.has("_created")) 14182 parseElementProperties(getJObject(json, "_created"), res.getCreatedElement()); 14183 if (json.has("organization")) 14184 res.setOrganization(parseReference(getJObject(json, "organization"))); 14185 if (json.has("request")) 14186 res.setRequest(parseReference(getJObject(json, "request"))); 14187 if (json.has("outcome")) 14188 res.setOutcome(parseCodeableConcept(getJObject(json, "outcome"))); 14189 if (json.has("disposition")) 14190 res.setDispositionElement(parseString(json.get("disposition").getAsString())); 14191 if (json.has("_disposition")) 14192 parseElementProperties(getJObject(json, "_disposition"), res.getDispositionElement()); 14193 if (json.has("requestProvider")) 14194 res.setRequestProvider(parseReference(getJObject(json, "requestProvider"))); 14195 if (json.has("requestOrganization")) 14196 res.setRequestOrganization(parseReference(getJObject(json, "requestOrganization"))); 14197 if (json.has("detail")) { 14198 JsonArray array = json.getAsJsonArray("detail"); 14199 for (int i = 0; i < array.size(); i++) { 14200 res.getDetail().add(parsePaymentReconciliationDetailsComponent(array.get(i).getAsJsonObject(), res)); 14201 } 14202 }; 14203 if (json.has("form")) 14204 res.setForm(parseCodeableConcept(getJObject(json, "form"))); 14205 if (json.has("total")) 14206 res.setTotal(parseMoney(getJObject(json, "total"))); 14207 if (json.has("processNote")) { 14208 JsonArray array = json.getAsJsonArray("processNote"); 14209 for (int i = 0; i < array.size(); i++) { 14210 res.getProcessNote().add(parsePaymentReconciliationNotesComponent(array.get(i).getAsJsonObject(), res)); 14211 } 14212 }; 14213 } 14214 14215 protected PaymentReconciliation.DetailsComponent parsePaymentReconciliationDetailsComponent(JsonObject json, PaymentReconciliation owner) throws IOException, FHIRFormatError { 14216 PaymentReconciliation.DetailsComponent res = new PaymentReconciliation.DetailsComponent(); 14217 parsePaymentReconciliationDetailsComponentProperties(json, owner, res); 14218 return res; 14219 } 14220 14221 protected void parsePaymentReconciliationDetailsComponentProperties(JsonObject json, PaymentReconciliation owner, PaymentReconciliation.DetailsComponent res) throws IOException, FHIRFormatError { 14222 parseBackboneProperties(json, res); 14223 if (json.has("type")) 14224 res.setType(parseCodeableConcept(getJObject(json, "type"))); 14225 if (json.has("request")) 14226 res.setRequest(parseReference(getJObject(json, "request"))); 14227 if (json.has("response")) 14228 res.setResponse(parseReference(getJObject(json, "response"))); 14229 if (json.has("submitter")) 14230 res.setSubmitter(parseReference(getJObject(json, "submitter"))); 14231 if (json.has("payee")) 14232 res.setPayee(parseReference(getJObject(json, "payee"))); 14233 if (json.has("date")) 14234 res.setDateElement(parseDate(json.get("date").getAsString())); 14235 if (json.has("_date")) 14236 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 14237 if (json.has("amount")) 14238 res.setAmount(parseMoney(getJObject(json, "amount"))); 14239 } 14240 14241 protected PaymentReconciliation.NotesComponent parsePaymentReconciliationNotesComponent(JsonObject json, PaymentReconciliation owner) throws IOException, FHIRFormatError { 14242 PaymentReconciliation.NotesComponent res = new PaymentReconciliation.NotesComponent(); 14243 parsePaymentReconciliationNotesComponentProperties(json, owner, res); 14244 return res; 14245 } 14246 14247 protected void parsePaymentReconciliationNotesComponentProperties(JsonObject json, PaymentReconciliation owner, PaymentReconciliation.NotesComponent res) throws IOException, FHIRFormatError { 14248 parseBackboneProperties(json, res); 14249 if (json.has("type")) 14250 res.setType(parseCodeableConcept(getJObject(json, "type"))); 14251 if (json.has("text")) 14252 res.setTextElement(parseString(json.get("text").getAsString())); 14253 if (json.has("_text")) 14254 parseElementProperties(getJObject(json, "_text"), res.getTextElement()); 14255 } 14256 14257 protected Person parsePerson(JsonObject json) throws IOException, FHIRFormatError { 14258 Person res = new Person(); 14259 parsePersonProperties(json, res); 14260 return res; 14261 } 14262 14263 protected void parsePersonProperties(JsonObject json, Person res) throws IOException, FHIRFormatError { 14264 parseDomainResourceProperties(json, res); 14265 if (json.has("identifier")) { 14266 JsonArray array = json.getAsJsonArray("identifier"); 14267 for (int i = 0; i < array.size(); i++) { 14268 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 14269 } 14270 }; 14271 if (json.has("name")) { 14272 JsonArray array = json.getAsJsonArray("name"); 14273 for (int i = 0; i < array.size(); i++) { 14274 res.getName().add(parseHumanName(array.get(i).getAsJsonObject())); 14275 } 14276 }; 14277 if (json.has("telecom")) { 14278 JsonArray array = json.getAsJsonArray("telecom"); 14279 for (int i = 0; i < array.size(); i++) { 14280 res.getTelecom().add(parseContactPoint(array.get(i).getAsJsonObject())); 14281 } 14282 }; 14283 if (json.has("gender")) 14284 res.setGenderElement(parseEnumeration(json.get("gender").getAsString(), Enumerations.AdministrativeGender.NULL, new Enumerations.AdministrativeGenderEnumFactory())); 14285 if (json.has("_gender")) 14286 parseElementProperties(getJObject(json, "_gender"), res.getGenderElement()); 14287 if (json.has("birthDate")) 14288 res.setBirthDateElement(parseDate(json.get("birthDate").getAsString())); 14289 if (json.has("_birthDate")) 14290 parseElementProperties(getJObject(json, "_birthDate"), res.getBirthDateElement()); 14291 if (json.has("address")) { 14292 JsonArray array = json.getAsJsonArray("address"); 14293 for (int i = 0; i < array.size(); i++) { 14294 res.getAddress().add(parseAddress(array.get(i).getAsJsonObject())); 14295 } 14296 }; 14297 if (json.has("photo")) 14298 res.setPhoto(parseAttachment(getJObject(json, "photo"))); 14299 if (json.has("managingOrganization")) 14300 res.setManagingOrganization(parseReference(getJObject(json, "managingOrganization"))); 14301 if (json.has("active")) 14302 res.setActiveElement(parseBoolean(json.get("active").getAsBoolean())); 14303 if (json.has("_active")) 14304 parseElementProperties(getJObject(json, "_active"), res.getActiveElement()); 14305 if (json.has("link")) { 14306 JsonArray array = json.getAsJsonArray("link"); 14307 for (int i = 0; i < array.size(); i++) { 14308 res.getLink().add(parsePersonPersonLinkComponent(array.get(i).getAsJsonObject(), res)); 14309 } 14310 }; 14311 } 14312 14313 protected Person.PersonLinkComponent parsePersonPersonLinkComponent(JsonObject json, Person owner) throws IOException, FHIRFormatError { 14314 Person.PersonLinkComponent res = new Person.PersonLinkComponent(); 14315 parsePersonPersonLinkComponentProperties(json, owner, res); 14316 return res; 14317 } 14318 14319 protected void parsePersonPersonLinkComponentProperties(JsonObject json, Person owner, Person.PersonLinkComponent res) throws IOException, FHIRFormatError { 14320 parseBackboneProperties(json, res); 14321 if (json.has("target")) 14322 res.setTarget(parseReference(getJObject(json, "target"))); 14323 if (json.has("assurance")) 14324 res.setAssuranceElement(parseEnumeration(json.get("assurance").getAsString(), Person.IdentityAssuranceLevel.NULL, new Person.IdentityAssuranceLevelEnumFactory())); 14325 if (json.has("_assurance")) 14326 parseElementProperties(getJObject(json, "_assurance"), res.getAssuranceElement()); 14327 } 14328 14329 protected PlanDefinition parsePlanDefinition(JsonObject json) throws IOException, FHIRFormatError { 14330 PlanDefinition res = new PlanDefinition(); 14331 parsePlanDefinitionProperties(json, res); 14332 return res; 14333 } 14334 14335 protected void parsePlanDefinitionProperties(JsonObject json, PlanDefinition res) throws IOException, FHIRFormatError { 14336 parseDomainResourceProperties(json, res); 14337 if (json.has("url")) 14338 res.setUrlElement(parseUri(json.get("url").getAsString())); 14339 if (json.has("_url")) 14340 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 14341 if (json.has("identifier")) { 14342 JsonArray array = json.getAsJsonArray("identifier"); 14343 for (int i = 0; i < array.size(); i++) { 14344 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 14345 } 14346 }; 14347 if (json.has("version")) 14348 res.setVersionElement(parseString(json.get("version").getAsString())); 14349 if (json.has("_version")) 14350 parseElementProperties(getJObject(json, "_version"), res.getVersionElement()); 14351 if (json.has("name")) 14352 res.setNameElement(parseString(json.get("name").getAsString())); 14353 if (json.has("_name")) 14354 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 14355 if (json.has("title")) 14356 res.setTitleElement(parseString(json.get("title").getAsString())); 14357 if (json.has("_title")) 14358 parseElementProperties(getJObject(json, "_title"), res.getTitleElement()); 14359 if (json.has("type")) 14360 res.setType(parseCodeableConcept(getJObject(json, "type"))); 14361 if (json.has("status")) 14362 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Enumerations.PublicationStatus.NULL, new Enumerations.PublicationStatusEnumFactory())); 14363 if (json.has("_status")) 14364 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 14365 if (json.has("experimental")) 14366 res.setExperimentalElement(parseBoolean(json.get("experimental").getAsBoolean())); 14367 if (json.has("_experimental")) 14368 parseElementProperties(getJObject(json, "_experimental"), res.getExperimentalElement()); 14369 if (json.has("date")) 14370 res.setDateElement(parseDateTime(json.get("date").getAsString())); 14371 if (json.has("_date")) 14372 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 14373 if (json.has("publisher")) 14374 res.setPublisherElement(parseString(json.get("publisher").getAsString())); 14375 if (json.has("_publisher")) 14376 parseElementProperties(getJObject(json, "_publisher"), res.getPublisherElement()); 14377 if (json.has("description")) 14378 res.setDescriptionElement(parseMarkdown(json.get("description").getAsString())); 14379 if (json.has("_description")) 14380 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 14381 if (json.has("purpose")) 14382 res.setPurposeElement(parseMarkdown(json.get("purpose").getAsString())); 14383 if (json.has("_purpose")) 14384 parseElementProperties(getJObject(json, "_purpose"), res.getPurposeElement()); 14385 if (json.has("usage")) 14386 res.setUsageElement(parseString(json.get("usage").getAsString())); 14387 if (json.has("_usage")) 14388 parseElementProperties(getJObject(json, "_usage"), res.getUsageElement()); 14389 if (json.has("approvalDate")) 14390 res.setApprovalDateElement(parseDate(json.get("approvalDate").getAsString())); 14391 if (json.has("_approvalDate")) 14392 parseElementProperties(getJObject(json, "_approvalDate"), res.getApprovalDateElement()); 14393 if (json.has("lastReviewDate")) 14394 res.setLastReviewDateElement(parseDate(json.get("lastReviewDate").getAsString())); 14395 if (json.has("_lastReviewDate")) 14396 parseElementProperties(getJObject(json, "_lastReviewDate"), res.getLastReviewDateElement()); 14397 if (json.has("effectivePeriod")) 14398 res.setEffectivePeriod(parsePeriod(getJObject(json, "effectivePeriod"))); 14399 if (json.has("useContext")) { 14400 JsonArray array = json.getAsJsonArray("useContext"); 14401 for (int i = 0; i < array.size(); i++) { 14402 res.getUseContext().add(parseUsageContext(array.get(i).getAsJsonObject())); 14403 } 14404 }; 14405 if (json.has("jurisdiction")) { 14406 JsonArray array = json.getAsJsonArray("jurisdiction"); 14407 for (int i = 0; i < array.size(); i++) { 14408 res.getJurisdiction().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 14409 } 14410 }; 14411 if (json.has("topic")) { 14412 JsonArray array = json.getAsJsonArray("topic"); 14413 for (int i = 0; i < array.size(); i++) { 14414 res.getTopic().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 14415 } 14416 }; 14417 if (json.has("contributor")) { 14418 JsonArray array = json.getAsJsonArray("contributor"); 14419 for (int i = 0; i < array.size(); i++) { 14420 res.getContributor().add(parseContributor(array.get(i).getAsJsonObject())); 14421 } 14422 }; 14423 if (json.has("contact")) { 14424 JsonArray array = json.getAsJsonArray("contact"); 14425 for (int i = 0; i < array.size(); i++) { 14426 res.getContact().add(parseContactDetail(array.get(i).getAsJsonObject())); 14427 } 14428 }; 14429 if (json.has("copyright")) 14430 res.setCopyrightElement(parseMarkdown(json.get("copyright").getAsString())); 14431 if (json.has("_copyright")) 14432 parseElementProperties(getJObject(json, "_copyright"), res.getCopyrightElement()); 14433 if (json.has("relatedArtifact")) { 14434 JsonArray array = json.getAsJsonArray("relatedArtifact"); 14435 for (int i = 0; i < array.size(); i++) { 14436 res.getRelatedArtifact().add(parseRelatedArtifact(array.get(i).getAsJsonObject())); 14437 } 14438 }; 14439 if (json.has("library")) { 14440 JsonArray array = json.getAsJsonArray("library"); 14441 for (int i = 0; i < array.size(); i++) { 14442 res.getLibrary().add(parseReference(array.get(i).getAsJsonObject())); 14443 } 14444 }; 14445 if (json.has("goal")) { 14446 JsonArray array = json.getAsJsonArray("goal"); 14447 for (int i = 0; i < array.size(); i++) { 14448 res.getGoal().add(parsePlanDefinitionPlanDefinitionGoalComponent(array.get(i).getAsJsonObject(), res)); 14449 } 14450 }; 14451 if (json.has("action")) { 14452 JsonArray array = json.getAsJsonArray("action"); 14453 for (int i = 0; i < array.size(); i++) { 14454 res.getAction().add(parsePlanDefinitionPlanDefinitionActionComponent(array.get(i).getAsJsonObject(), res)); 14455 } 14456 }; 14457 } 14458 14459 protected PlanDefinition.PlanDefinitionGoalComponent parsePlanDefinitionPlanDefinitionGoalComponent(JsonObject json, PlanDefinition owner) throws IOException, FHIRFormatError { 14460 PlanDefinition.PlanDefinitionGoalComponent res = new PlanDefinition.PlanDefinitionGoalComponent(); 14461 parsePlanDefinitionPlanDefinitionGoalComponentProperties(json, owner, res); 14462 return res; 14463 } 14464 14465 protected void parsePlanDefinitionPlanDefinitionGoalComponentProperties(JsonObject json, PlanDefinition owner, PlanDefinition.PlanDefinitionGoalComponent res) throws IOException, FHIRFormatError { 14466 parseBackboneProperties(json, res); 14467 if (json.has("category")) 14468 res.setCategory(parseCodeableConcept(getJObject(json, "category"))); 14469 if (json.has("description")) 14470 res.setDescription(parseCodeableConcept(getJObject(json, "description"))); 14471 if (json.has("priority")) 14472 res.setPriority(parseCodeableConcept(getJObject(json, "priority"))); 14473 if (json.has("start")) 14474 res.setStart(parseCodeableConcept(getJObject(json, "start"))); 14475 if (json.has("addresses")) { 14476 JsonArray array = json.getAsJsonArray("addresses"); 14477 for (int i = 0; i < array.size(); i++) { 14478 res.getAddresses().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 14479 } 14480 }; 14481 if (json.has("documentation")) { 14482 JsonArray array = json.getAsJsonArray("documentation"); 14483 for (int i = 0; i < array.size(); i++) { 14484 res.getDocumentation().add(parseRelatedArtifact(array.get(i).getAsJsonObject())); 14485 } 14486 }; 14487 if (json.has("target")) { 14488 JsonArray array = json.getAsJsonArray("target"); 14489 for (int i = 0; i < array.size(); i++) { 14490 res.getTarget().add(parsePlanDefinitionPlanDefinitionGoalTargetComponent(array.get(i).getAsJsonObject(), owner)); 14491 } 14492 }; 14493 } 14494 14495 protected PlanDefinition.PlanDefinitionGoalTargetComponent parsePlanDefinitionPlanDefinitionGoalTargetComponent(JsonObject json, PlanDefinition owner) throws IOException, FHIRFormatError { 14496 PlanDefinition.PlanDefinitionGoalTargetComponent res = new PlanDefinition.PlanDefinitionGoalTargetComponent(); 14497 parsePlanDefinitionPlanDefinitionGoalTargetComponentProperties(json, owner, res); 14498 return res; 14499 } 14500 14501 protected void parsePlanDefinitionPlanDefinitionGoalTargetComponentProperties(JsonObject json, PlanDefinition owner, PlanDefinition.PlanDefinitionGoalTargetComponent res) throws IOException, FHIRFormatError { 14502 parseBackboneProperties(json, res); 14503 if (json.has("measure")) 14504 res.setMeasure(parseCodeableConcept(getJObject(json, "measure"))); 14505 Type detail = parseType("detail", json); 14506 if (detail != null) 14507 res.setDetail(detail); 14508 if (json.has("due")) 14509 res.setDue(parseDuration(getJObject(json, "due"))); 14510 } 14511 14512 protected PlanDefinition.PlanDefinitionActionComponent parsePlanDefinitionPlanDefinitionActionComponent(JsonObject json, PlanDefinition owner) throws IOException, FHIRFormatError { 14513 PlanDefinition.PlanDefinitionActionComponent res = new PlanDefinition.PlanDefinitionActionComponent(); 14514 parsePlanDefinitionPlanDefinitionActionComponentProperties(json, owner, res); 14515 return res; 14516 } 14517 14518 protected void parsePlanDefinitionPlanDefinitionActionComponentProperties(JsonObject json, PlanDefinition owner, PlanDefinition.PlanDefinitionActionComponent res) throws IOException, FHIRFormatError { 14519 parseBackboneProperties(json, res); 14520 if (json.has("label")) 14521 res.setLabelElement(parseString(json.get("label").getAsString())); 14522 if (json.has("_label")) 14523 parseElementProperties(getJObject(json, "_label"), res.getLabelElement()); 14524 if (json.has("title")) 14525 res.setTitleElement(parseString(json.get("title").getAsString())); 14526 if (json.has("_title")) 14527 parseElementProperties(getJObject(json, "_title"), res.getTitleElement()); 14528 if (json.has("description")) 14529 res.setDescriptionElement(parseString(json.get("description").getAsString())); 14530 if (json.has("_description")) 14531 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 14532 if (json.has("textEquivalent")) 14533 res.setTextEquivalentElement(parseString(json.get("textEquivalent").getAsString())); 14534 if (json.has("_textEquivalent")) 14535 parseElementProperties(getJObject(json, "_textEquivalent"), res.getTextEquivalentElement()); 14536 if (json.has("code")) { 14537 JsonArray array = json.getAsJsonArray("code"); 14538 for (int i = 0; i < array.size(); i++) { 14539 res.getCode().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 14540 } 14541 }; 14542 if (json.has("reason")) { 14543 JsonArray array = json.getAsJsonArray("reason"); 14544 for (int i = 0; i < array.size(); i++) { 14545 res.getReason().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 14546 } 14547 }; 14548 if (json.has("documentation")) { 14549 JsonArray array = json.getAsJsonArray("documentation"); 14550 for (int i = 0; i < array.size(); i++) { 14551 res.getDocumentation().add(parseRelatedArtifact(array.get(i).getAsJsonObject())); 14552 } 14553 }; 14554 if (json.has("goalId")) { 14555 JsonArray array = json.getAsJsonArray("goalId"); 14556 for (int i = 0; i < array.size(); i++) { 14557 if (array.get(i).isJsonNull()) { 14558 res.getGoalId().add(new IdType()); 14559 } else { 14560 res.getGoalId().add(parseId(array.get(i).getAsString())); 14561 } 14562 } 14563 }; 14564 if (json.has("_goalId")) { 14565 JsonArray array = json.getAsJsonArray("_goalId"); 14566 for (int i = 0; i < array.size(); i++) { 14567 if (i == res.getGoalId().size()) 14568 res.getGoalId().add(parseId(null)); 14569 if (array.get(i) instanceof JsonObject) 14570 parseElementProperties(array.get(i).getAsJsonObject(), res.getGoalId().get(i)); 14571 } 14572 }; 14573 if (json.has("triggerDefinition")) { 14574 JsonArray array = json.getAsJsonArray("triggerDefinition"); 14575 for (int i = 0; i < array.size(); i++) { 14576 res.getTriggerDefinition().add(parseTriggerDefinition(array.get(i).getAsJsonObject())); 14577 } 14578 }; 14579 if (json.has("condition")) { 14580 JsonArray array = json.getAsJsonArray("condition"); 14581 for (int i = 0; i < array.size(); i++) { 14582 res.getCondition().add(parsePlanDefinitionPlanDefinitionActionConditionComponent(array.get(i).getAsJsonObject(), owner)); 14583 } 14584 }; 14585 if (json.has("input")) { 14586 JsonArray array = json.getAsJsonArray("input"); 14587 for (int i = 0; i < array.size(); i++) { 14588 res.getInput().add(parseDataRequirement(array.get(i).getAsJsonObject())); 14589 } 14590 }; 14591 if (json.has("output")) { 14592 JsonArray array = json.getAsJsonArray("output"); 14593 for (int i = 0; i < array.size(); i++) { 14594 res.getOutput().add(parseDataRequirement(array.get(i).getAsJsonObject())); 14595 } 14596 }; 14597 if (json.has("relatedAction")) { 14598 JsonArray array = json.getAsJsonArray("relatedAction"); 14599 for (int i = 0; i < array.size(); i++) { 14600 res.getRelatedAction().add(parsePlanDefinitionPlanDefinitionActionRelatedActionComponent(array.get(i).getAsJsonObject(), owner)); 14601 } 14602 }; 14603 Type timing = parseType("timing", json); 14604 if (timing != null) 14605 res.setTiming(timing); 14606 if (json.has("participant")) { 14607 JsonArray array = json.getAsJsonArray("participant"); 14608 for (int i = 0; i < array.size(); i++) { 14609 res.getParticipant().add(parsePlanDefinitionPlanDefinitionActionParticipantComponent(array.get(i).getAsJsonObject(), owner)); 14610 } 14611 }; 14612 if (json.has("type")) 14613 res.setType(parseCoding(getJObject(json, "type"))); 14614 if (json.has("groupingBehavior")) 14615 res.setGroupingBehaviorElement(parseEnumeration(json.get("groupingBehavior").getAsString(), PlanDefinition.ActionGroupingBehavior.NULL, new PlanDefinition.ActionGroupingBehaviorEnumFactory())); 14616 if (json.has("_groupingBehavior")) 14617 parseElementProperties(getJObject(json, "_groupingBehavior"), res.getGroupingBehaviorElement()); 14618 if (json.has("selectionBehavior")) 14619 res.setSelectionBehaviorElement(parseEnumeration(json.get("selectionBehavior").getAsString(), PlanDefinition.ActionSelectionBehavior.NULL, new PlanDefinition.ActionSelectionBehaviorEnumFactory())); 14620 if (json.has("_selectionBehavior")) 14621 parseElementProperties(getJObject(json, "_selectionBehavior"), res.getSelectionBehaviorElement()); 14622 if (json.has("requiredBehavior")) 14623 res.setRequiredBehaviorElement(parseEnumeration(json.get("requiredBehavior").getAsString(), PlanDefinition.ActionRequiredBehavior.NULL, new PlanDefinition.ActionRequiredBehaviorEnumFactory())); 14624 if (json.has("_requiredBehavior")) 14625 parseElementProperties(getJObject(json, "_requiredBehavior"), res.getRequiredBehaviorElement()); 14626 if (json.has("precheckBehavior")) 14627 res.setPrecheckBehaviorElement(parseEnumeration(json.get("precheckBehavior").getAsString(), PlanDefinition.ActionPrecheckBehavior.NULL, new PlanDefinition.ActionPrecheckBehaviorEnumFactory())); 14628 if (json.has("_precheckBehavior")) 14629 parseElementProperties(getJObject(json, "_precheckBehavior"), res.getPrecheckBehaviorElement()); 14630 if (json.has("cardinalityBehavior")) 14631 res.setCardinalityBehaviorElement(parseEnumeration(json.get("cardinalityBehavior").getAsString(), PlanDefinition.ActionCardinalityBehavior.NULL, new PlanDefinition.ActionCardinalityBehaviorEnumFactory())); 14632 if (json.has("_cardinalityBehavior")) 14633 parseElementProperties(getJObject(json, "_cardinalityBehavior"), res.getCardinalityBehaviorElement()); 14634 if (json.has("definition")) 14635 res.setDefinition(parseReference(getJObject(json, "definition"))); 14636 if (json.has("transform")) 14637 res.setTransform(parseReference(getJObject(json, "transform"))); 14638 if (json.has("dynamicValue")) { 14639 JsonArray array = json.getAsJsonArray("dynamicValue"); 14640 for (int i = 0; i < array.size(); i++) { 14641 res.getDynamicValue().add(parsePlanDefinitionPlanDefinitionActionDynamicValueComponent(array.get(i).getAsJsonObject(), owner)); 14642 } 14643 }; 14644 if (json.has("action")) { 14645 JsonArray array = json.getAsJsonArray("action"); 14646 for (int i = 0; i < array.size(); i++) { 14647 res.getAction().add(parsePlanDefinitionPlanDefinitionActionComponent(array.get(i).getAsJsonObject(), owner)); 14648 } 14649 }; 14650 } 14651 14652 protected PlanDefinition.PlanDefinitionActionConditionComponent parsePlanDefinitionPlanDefinitionActionConditionComponent(JsonObject json, PlanDefinition owner) throws IOException, FHIRFormatError { 14653 PlanDefinition.PlanDefinitionActionConditionComponent res = new PlanDefinition.PlanDefinitionActionConditionComponent(); 14654 parsePlanDefinitionPlanDefinitionActionConditionComponentProperties(json, owner, res); 14655 return res; 14656 } 14657 14658 protected void parsePlanDefinitionPlanDefinitionActionConditionComponentProperties(JsonObject json, PlanDefinition owner, PlanDefinition.PlanDefinitionActionConditionComponent res) throws IOException, FHIRFormatError { 14659 parseBackboneProperties(json, res); 14660 if (json.has("kind")) 14661 res.setKindElement(parseEnumeration(json.get("kind").getAsString(), PlanDefinition.ActionConditionKind.NULL, new PlanDefinition.ActionConditionKindEnumFactory())); 14662 if (json.has("_kind")) 14663 parseElementProperties(getJObject(json, "_kind"), res.getKindElement()); 14664 if (json.has("description")) 14665 res.setDescriptionElement(parseString(json.get("description").getAsString())); 14666 if (json.has("_description")) 14667 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 14668 if (json.has("language")) 14669 res.setLanguageElement(parseString(json.get("language").getAsString())); 14670 if (json.has("_language")) 14671 parseElementProperties(getJObject(json, "_language"), res.getLanguageElement()); 14672 if (json.has("expression")) 14673 res.setExpressionElement(parseString(json.get("expression").getAsString())); 14674 if (json.has("_expression")) 14675 parseElementProperties(getJObject(json, "_expression"), res.getExpressionElement()); 14676 } 14677 14678 protected PlanDefinition.PlanDefinitionActionRelatedActionComponent parsePlanDefinitionPlanDefinitionActionRelatedActionComponent(JsonObject json, PlanDefinition owner) throws IOException, FHIRFormatError { 14679 PlanDefinition.PlanDefinitionActionRelatedActionComponent res = new PlanDefinition.PlanDefinitionActionRelatedActionComponent(); 14680 parsePlanDefinitionPlanDefinitionActionRelatedActionComponentProperties(json, owner, res); 14681 return res; 14682 } 14683 14684 protected void parsePlanDefinitionPlanDefinitionActionRelatedActionComponentProperties(JsonObject json, PlanDefinition owner, PlanDefinition.PlanDefinitionActionRelatedActionComponent res) throws IOException, FHIRFormatError { 14685 parseBackboneProperties(json, res); 14686 if (json.has("actionId")) 14687 res.setActionIdElement(parseId(json.get("actionId").getAsString())); 14688 if (json.has("_actionId")) 14689 parseElementProperties(getJObject(json, "_actionId"), res.getActionIdElement()); 14690 if (json.has("relationship")) 14691 res.setRelationshipElement(parseEnumeration(json.get("relationship").getAsString(), PlanDefinition.ActionRelationshipType.NULL, new PlanDefinition.ActionRelationshipTypeEnumFactory())); 14692 if (json.has("_relationship")) 14693 parseElementProperties(getJObject(json, "_relationship"), res.getRelationshipElement()); 14694 Type offset = parseType("offset", json); 14695 if (offset != null) 14696 res.setOffset(offset); 14697 } 14698 14699 protected PlanDefinition.PlanDefinitionActionParticipantComponent parsePlanDefinitionPlanDefinitionActionParticipantComponent(JsonObject json, PlanDefinition owner) throws IOException, FHIRFormatError { 14700 PlanDefinition.PlanDefinitionActionParticipantComponent res = new PlanDefinition.PlanDefinitionActionParticipantComponent(); 14701 parsePlanDefinitionPlanDefinitionActionParticipantComponentProperties(json, owner, res); 14702 return res; 14703 } 14704 14705 protected void parsePlanDefinitionPlanDefinitionActionParticipantComponentProperties(JsonObject json, PlanDefinition owner, PlanDefinition.PlanDefinitionActionParticipantComponent res) throws IOException, FHIRFormatError { 14706 parseBackboneProperties(json, res); 14707 if (json.has("type")) 14708 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), PlanDefinition.ActionParticipantType.NULL, new PlanDefinition.ActionParticipantTypeEnumFactory())); 14709 if (json.has("_type")) 14710 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 14711 if (json.has("role")) 14712 res.setRole(parseCodeableConcept(getJObject(json, "role"))); 14713 } 14714 14715 protected PlanDefinition.PlanDefinitionActionDynamicValueComponent parsePlanDefinitionPlanDefinitionActionDynamicValueComponent(JsonObject json, PlanDefinition owner) throws IOException, FHIRFormatError { 14716 PlanDefinition.PlanDefinitionActionDynamicValueComponent res = new PlanDefinition.PlanDefinitionActionDynamicValueComponent(); 14717 parsePlanDefinitionPlanDefinitionActionDynamicValueComponentProperties(json, owner, res); 14718 return res; 14719 } 14720 14721 protected void parsePlanDefinitionPlanDefinitionActionDynamicValueComponentProperties(JsonObject json, PlanDefinition owner, PlanDefinition.PlanDefinitionActionDynamicValueComponent res) throws IOException, FHIRFormatError { 14722 parseBackboneProperties(json, res); 14723 if (json.has("description")) 14724 res.setDescriptionElement(parseString(json.get("description").getAsString())); 14725 if (json.has("_description")) 14726 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 14727 if (json.has("path")) 14728 res.setPathElement(parseString(json.get("path").getAsString())); 14729 if (json.has("_path")) 14730 parseElementProperties(getJObject(json, "_path"), res.getPathElement()); 14731 if (json.has("language")) 14732 res.setLanguageElement(parseString(json.get("language").getAsString())); 14733 if (json.has("_language")) 14734 parseElementProperties(getJObject(json, "_language"), res.getLanguageElement()); 14735 if (json.has("expression")) 14736 res.setExpressionElement(parseString(json.get("expression").getAsString())); 14737 if (json.has("_expression")) 14738 parseElementProperties(getJObject(json, "_expression"), res.getExpressionElement()); 14739 } 14740 14741 protected Practitioner parsePractitioner(JsonObject json) throws IOException, FHIRFormatError { 14742 Practitioner res = new Practitioner(); 14743 parsePractitionerProperties(json, res); 14744 return res; 14745 } 14746 14747 protected void parsePractitionerProperties(JsonObject json, Practitioner res) throws IOException, FHIRFormatError { 14748 parseDomainResourceProperties(json, res); 14749 if (json.has("identifier")) { 14750 JsonArray array = json.getAsJsonArray("identifier"); 14751 for (int i = 0; i < array.size(); i++) { 14752 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 14753 } 14754 }; 14755 if (json.has("active")) 14756 res.setActiveElement(parseBoolean(json.get("active").getAsBoolean())); 14757 if (json.has("_active")) 14758 parseElementProperties(getJObject(json, "_active"), res.getActiveElement()); 14759 if (json.has("name")) { 14760 JsonArray array = json.getAsJsonArray("name"); 14761 for (int i = 0; i < array.size(); i++) { 14762 res.getName().add(parseHumanName(array.get(i).getAsJsonObject())); 14763 } 14764 }; 14765 if (json.has("telecom")) { 14766 JsonArray array = json.getAsJsonArray("telecom"); 14767 for (int i = 0; i < array.size(); i++) { 14768 res.getTelecom().add(parseContactPoint(array.get(i).getAsJsonObject())); 14769 } 14770 }; 14771 if (json.has("address")) { 14772 JsonArray array = json.getAsJsonArray("address"); 14773 for (int i = 0; i < array.size(); i++) { 14774 res.getAddress().add(parseAddress(array.get(i).getAsJsonObject())); 14775 } 14776 }; 14777 if (json.has("gender")) 14778 res.setGenderElement(parseEnumeration(json.get("gender").getAsString(), Enumerations.AdministrativeGender.NULL, new Enumerations.AdministrativeGenderEnumFactory())); 14779 if (json.has("_gender")) 14780 parseElementProperties(getJObject(json, "_gender"), res.getGenderElement()); 14781 if (json.has("birthDate")) 14782 res.setBirthDateElement(parseDate(json.get("birthDate").getAsString())); 14783 if (json.has("_birthDate")) 14784 parseElementProperties(getJObject(json, "_birthDate"), res.getBirthDateElement()); 14785 if (json.has("photo")) { 14786 JsonArray array = json.getAsJsonArray("photo"); 14787 for (int i = 0; i < array.size(); i++) { 14788 res.getPhoto().add(parseAttachment(array.get(i).getAsJsonObject())); 14789 } 14790 }; 14791 if (json.has("qualification")) { 14792 JsonArray array = json.getAsJsonArray("qualification"); 14793 for (int i = 0; i < array.size(); i++) { 14794 res.getQualification().add(parsePractitionerPractitionerQualificationComponent(array.get(i).getAsJsonObject(), res)); 14795 } 14796 }; 14797 if (json.has("communication")) { 14798 JsonArray array = json.getAsJsonArray("communication"); 14799 for (int i = 0; i < array.size(); i++) { 14800 res.getCommunication().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 14801 } 14802 }; 14803 } 14804 14805 protected Practitioner.PractitionerQualificationComponent parsePractitionerPractitionerQualificationComponent(JsonObject json, Practitioner owner) throws IOException, FHIRFormatError { 14806 Practitioner.PractitionerQualificationComponent res = new Practitioner.PractitionerQualificationComponent(); 14807 parsePractitionerPractitionerQualificationComponentProperties(json, owner, res); 14808 return res; 14809 } 14810 14811 protected void parsePractitionerPractitionerQualificationComponentProperties(JsonObject json, Practitioner owner, Practitioner.PractitionerQualificationComponent res) throws IOException, FHIRFormatError { 14812 parseBackboneProperties(json, res); 14813 if (json.has("identifier")) { 14814 JsonArray array = json.getAsJsonArray("identifier"); 14815 for (int i = 0; i < array.size(); i++) { 14816 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 14817 } 14818 }; 14819 if (json.has("code")) 14820 res.setCode(parseCodeableConcept(getJObject(json, "code"))); 14821 if (json.has("period")) 14822 res.setPeriod(parsePeriod(getJObject(json, "period"))); 14823 if (json.has("issuer")) 14824 res.setIssuer(parseReference(getJObject(json, "issuer"))); 14825 } 14826 14827 protected PractitionerRole parsePractitionerRole(JsonObject json) throws IOException, FHIRFormatError { 14828 PractitionerRole res = new PractitionerRole(); 14829 parsePractitionerRoleProperties(json, res); 14830 return res; 14831 } 14832 14833 protected void parsePractitionerRoleProperties(JsonObject json, PractitionerRole res) throws IOException, FHIRFormatError { 14834 parseDomainResourceProperties(json, res); 14835 if (json.has("identifier")) { 14836 JsonArray array = json.getAsJsonArray("identifier"); 14837 for (int i = 0; i < array.size(); i++) { 14838 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 14839 } 14840 }; 14841 if (json.has("active")) 14842 res.setActiveElement(parseBoolean(json.get("active").getAsBoolean())); 14843 if (json.has("_active")) 14844 parseElementProperties(getJObject(json, "_active"), res.getActiveElement()); 14845 if (json.has("period")) 14846 res.setPeriod(parsePeriod(getJObject(json, "period"))); 14847 if (json.has("practitioner")) 14848 res.setPractitioner(parseReference(getJObject(json, "practitioner"))); 14849 if (json.has("organization")) 14850 res.setOrganization(parseReference(getJObject(json, "organization"))); 14851 if (json.has("code")) { 14852 JsonArray array = json.getAsJsonArray("code"); 14853 for (int i = 0; i < array.size(); i++) { 14854 res.getCode().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 14855 } 14856 }; 14857 if (json.has("specialty")) { 14858 JsonArray array = json.getAsJsonArray("specialty"); 14859 for (int i = 0; i < array.size(); i++) { 14860 res.getSpecialty().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 14861 } 14862 }; 14863 if (json.has("location")) { 14864 JsonArray array = json.getAsJsonArray("location"); 14865 for (int i = 0; i < array.size(); i++) { 14866 res.getLocation().add(parseReference(array.get(i).getAsJsonObject())); 14867 } 14868 }; 14869 if (json.has("healthcareService")) { 14870 JsonArray array = json.getAsJsonArray("healthcareService"); 14871 for (int i = 0; i < array.size(); i++) { 14872 res.getHealthcareService().add(parseReference(array.get(i).getAsJsonObject())); 14873 } 14874 }; 14875 if (json.has("telecom")) { 14876 JsonArray array = json.getAsJsonArray("telecom"); 14877 for (int i = 0; i < array.size(); i++) { 14878 res.getTelecom().add(parseContactPoint(array.get(i).getAsJsonObject())); 14879 } 14880 }; 14881 if (json.has("availableTime")) { 14882 JsonArray array = json.getAsJsonArray("availableTime"); 14883 for (int i = 0; i < array.size(); i++) { 14884 res.getAvailableTime().add(parsePractitionerRolePractitionerRoleAvailableTimeComponent(array.get(i).getAsJsonObject(), res)); 14885 } 14886 }; 14887 if (json.has("notAvailable")) { 14888 JsonArray array = json.getAsJsonArray("notAvailable"); 14889 for (int i = 0; i < array.size(); i++) { 14890 res.getNotAvailable().add(parsePractitionerRolePractitionerRoleNotAvailableComponent(array.get(i).getAsJsonObject(), res)); 14891 } 14892 }; 14893 if (json.has("availabilityExceptions")) 14894 res.setAvailabilityExceptionsElement(parseString(json.get("availabilityExceptions").getAsString())); 14895 if (json.has("_availabilityExceptions")) 14896 parseElementProperties(getJObject(json, "_availabilityExceptions"), res.getAvailabilityExceptionsElement()); 14897 if (json.has("endpoint")) { 14898 JsonArray array = json.getAsJsonArray("endpoint"); 14899 for (int i = 0; i < array.size(); i++) { 14900 res.getEndpoint().add(parseReference(array.get(i).getAsJsonObject())); 14901 } 14902 }; 14903 } 14904 14905 protected PractitionerRole.PractitionerRoleAvailableTimeComponent parsePractitionerRolePractitionerRoleAvailableTimeComponent(JsonObject json, PractitionerRole owner) throws IOException, FHIRFormatError { 14906 PractitionerRole.PractitionerRoleAvailableTimeComponent res = new PractitionerRole.PractitionerRoleAvailableTimeComponent(); 14907 parsePractitionerRolePractitionerRoleAvailableTimeComponentProperties(json, owner, res); 14908 return res; 14909 } 14910 14911 protected void parsePractitionerRolePractitionerRoleAvailableTimeComponentProperties(JsonObject json, PractitionerRole owner, PractitionerRole.PractitionerRoleAvailableTimeComponent res) throws IOException, FHIRFormatError { 14912 parseBackboneProperties(json, res); 14913 if (json.has("daysOfWeek")) { 14914 JsonArray array = json.getAsJsonArray("daysOfWeek"); 14915 for (int i = 0; i < array.size(); i++) { 14916 if (array.get(i).isJsonNull()) { 14917 res.getDaysOfWeek().add(new Enumeration<PractitionerRole.DaysOfWeek>()); 14918 } else { 14919 res.getDaysOfWeek().add(parseEnumeration(array.get(i).getAsString(), PractitionerRole.DaysOfWeek.NULL, new PractitionerRole.DaysOfWeekEnumFactory())); 14920 } 14921 } 14922 }; 14923 if (json.has("_daysOfWeek")) { 14924 JsonArray array = json.getAsJsonArray("_daysOfWeek"); 14925 for (int i = 0; i < array.size(); i++) { 14926 if (i == res.getDaysOfWeek().size()) 14927 res.getDaysOfWeek().add(parseEnumeration(null, PractitionerRole.DaysOfWeek.NULL, new PractitionerRole.DaysOfWeekEnumFactory())); 14928 if (array.get(i) instanceof JsonObject) 14929 parseElementProperties(array.get(i).getAsJsonObject(), res.getDaysOfWeek().get(i)); 14930 } 14931 }; 14932 if (json.has("allDay")) 14933 res.setAllDayElement(parseBoolean(json.get("allDay").getAsBoolean())); 14934 if (json.has("_allDay")) 14935 parseElementProperties(getJObject(json, "_allDay"), res.getAllDayElement()); 14936 if (json.has("availableStartTime")) 14937 res.setAvailableStartTimeElement(parseTime(json.get("availableStartTime").getAsString())); 14938 if (json.has("_availableStartTime")) 14939 parseElementProperties(getJObject(json, "_availableStartTime"), res.getAvailableStartTimeElement()); 14940 if (json.has("availableEndTime")) 14941 res.setAvailableEndTimeElement(parseTime(json.get("availableEndTime").getAsString())); 14942 if (json.has("_availableEndTime")) 14943 parseElementProperties(getJObject(json, "_availableEndTime"), res.getAvailableEndTimeElement()); 14944 } 14945 14946 protected PractitionerRole.PractitionerRoleNotAvailableComponent parsePractitionerRolePractitionerRoleNotAvailableComponent(JsonObject json, PractitionerRole owner) throws IOException, FHIRFormatError { 14947 PractitionerRole.PractitionerRoleNotAvailableComponent res = new PractitionerRole.PractitionerRoleNotAvailableComponent(); 14948 parsePractitionerRolePractitionerRoleNotAvailableComponentProperties(json, owner, res); 14949 return res; 14950 } 14951 14952 protected void parsePractitionerRolePractitionerRoleNotAvailableComponentProperties(JsonObject json, PractitionerRole owner, PractitionerRole.PractitionerRoleNotAvailableComponent res) throws IOException, FHIRFormatError { 14953 parseBackboneProperties(json, res); 14954 if (json.has("description")) 14955 res.setDescriptionElement(parseString(json.get("description").getAsString())); 14956 if (json.has("_description")) 14957 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 14958 if (json.has("during")) 14959 res.setDuring(parsePeriod(getJObject(json, "during"))); 14960 } 14961 14962 protected Procedure parseProcedure(JsonObject json) throws IOException, FHIRFormatError { 14963 Procedure res = new Procedure(); 14964 parseProcedureProperties(json, res); 14965 return res; 14966 } 14967 14968 protected void parseProcedureProperties(JsonObject json, Procedure res) throws IOException, FHIRFormatError { 14969 parseDomainResourceProperties(json, res); 14970 if (json.has("identifier")) { 14971 JsonArray array = json.getAsJsonArray("identifier"); 14972 for (int i = 0; i < array.size(); i++) { 14973 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 14974 } 14975 }; 14976 if (json.has("definition")) { 14977 JsonArray array = json.getAsJsonArray("definition"); 14978 for (int i = 0; i < array.size(); i++) { 14979 res.getDefinition().add(parseReference(array.get(i).getAsJsonObject())); 14980 } 14981 }; 14982 if (json.has("basedOn")) { 14983 JsonArray array = json.getAsJsonArray("basedOn"); 14984 for (int i = 0; i < array.size(); i++) { 14985 res.getBasedOn().add(parseReference(array.get(i).getAsJsonObject())); 14986 } 14987 }; 14988 if (json.has("partOf")) { 14989 JsonArray array = json.getAsJsonArray("partOf"); 14990 for (int i = 0; i < array.size(); i++) { 14991 res.getPartOf().add(parseReference(array.get(i).getAsJsonObject())); 14992 } 14993 }; 14994 if (json.has("status")) 14995 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Procedure.ProcedureStatus.NULL, new Procedure.ProcedureStatusEnumFactory())); 14996 if (json.has("_status")) 14997 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 14998 if (json.has("notDone")) 14999 res.setNotDoneElement(parseBoolean(json.get("notDone").getAsBoolean())); 15000 if (json.has("_notDone")) 15001 parseElementProperties(getJObject(json, "_notDone"), res.getNotDoneElement()); 15002 if (json.has("notDoneReason")) 15003 res.setNotDoneReason(parseCodeableConcept(getJObject(json, "notDoneReason"))); 15004 if (json.has("category")) 15005 res.setCategory(parseCodeableConcept(getJObject(json, "category"))); 15006 if (json.has("code")) 15007 res.setCode(parseCodeableConcept(getJObject(json, "code"))); 15008 if (json.has("subject")) 15009 res.setSubject(parseReference(getJObject(json, "subject"))); 15010 if (json.has("context")) 15011 res.setContext(parseReference(getJObject(json, "context"))); 15012 Type performed = parseType("performed", json); 15013 if (performed != null) 15014 res.setPerformed(performed); 15015 if (json.has("performer")) { 15016 JsonArray array = json.getAsJsonArray("performer"); 15017 for (int i = 0; i < array.size(); i++) { 15018 res.getPerformer().add(parseProcedureProcedurePerformerComponent(array.get(i).getAsJsonObject(), res)); 15019 } 15020 }; 15021 if (json.has("location")) 15022 res.setLocation(parseReference(getJObject(json, "location"))); 15023 if (json.has("reasonCode")) { 15024 JsonArray array = json.getAsJsonArray("reasonCode"); 15025 for (int i = 0; i < array.size(); i++) { 15026 res.getReasonCode().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 15027 } 15028 }; 15029 if (json.has("reasonReference")) { 15030 JsonArray array = json.getAsJsonArray("reasonReference"); 15031 for (int i = 0; i < array.size(); i++) { 15032 res.getReasonReference().add(parseReference(array.get(i).getAsJsonObject())); 15033 } 15034 }; 15035 if (json.has("bodySite")) { 15036 JsonArray array = json.getAsJsonArray("bodySite"); 15037 for (int i = 0; i < array.size(); i++) { 15038 res.getBodySite().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 15039 } 15040 }; 15041 if (json.has("outcome")) 15042 res.setOutcome(parseCodeableConcept(getJObject(json, "outcome"))); 15043 if (json.has("report")) { 15044 JsonArray array = json.getAsJsonArray("report"); 15045 for (int i = 0; i < array.size(); i++) { 15046 res.getReport().add(parseReference(array.get(i).getAsJsonObject())); 15047 } 15048 }; 15049 if (json.has("complication")) { 15050 JsonArray array = json.getAsJsonArray("complication"); 15051 for (int i = 0; i < array.size(); i++) { 15052 res.getComplication().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 15053 } 15054 }; 15055 if (json.has("complicationDetail")) { 15056 JsonArray array = json.getAsJsonArray("complicationDetail"); 15057 for (int i = 0; i < array.size(); i++) { 15058 res.getComplicationDetail().add(parseReference(array.get(i).getAsJsonObject())); 15059 } 15060 }; 15061 if (json.has("followUp")) { 15062 JsonArray array = json.getAsJsonArray("followUp"); 15063 for (int i = 0; i < array.size(); i++) { 15064 res.getFollowUp().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 15065 } 15066 }; 15067 if (json.has("note")) { 15068 JsonArray array = json.getAsJsonArray("note"); 15069 for (int i = 0; i < array.size(); i++) { 15070 res.getNote().add(parseAnnotation(array.get(i).getAsJsonObject())); 15071 } 15072 }; 15073 if (json.has("focalDevice")) { 15074 JsonArray array = json.getAsJsonArray("focalDevice"); 15075 for (int i = 0; i < array.size(); i++) { 15076 res.getFocalDevice().add(parseProcedureProcedureFocalDeviceComponent(array.get(i).getAsJsonObject(), res)); 15077 } 15078 }; 15079 if (json.has("usedReference")) { 15080 JsonArray array = json.getAsJsonArray("usedReference"); 15081 for (int i = 0; i < array.size(); i++) { 15082 res.getUsedReference().add(parseReference(array.get(i).getAsJsonObject())); 15083 } 15084 }; 15085 if (json.has("usedCode")) { 15086 JsonArray array = json.getAsJsonArray("usedCode"); 15087 for (int i = 0; i < array.size(); i++) { 15088 res.getUsedCode().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 15089 } 15090 }; 15091 } 15092 15093 protected Procedure.ProcedurePerformerComponent parseProcedureProcedurePerformerComponent(JsonObject json, Procedure owner) throws IOException, FHIRFormatError { 15094 Procedure.ProcedurePerformerComponent res = new Procedure.ProcedurePerformerComponent(); 15095 parseProcedureProcedurePerformerComponentProperties(json, owner, res); 15096 return res; 15097 } 15098 15099 protected void parseProcedureProcedurePerformerComponentProperties(JsonObject json, Procedure owner, Procedure.ProcedurePerformerComponent res) throws IOException, FHIRFormatError { 15100 parseBackboneProperties(json, res); 15101 if (json.has("role")) 15102 res.setRole(parseCodeableConcept(getJObject(json, "role"))); 15103 if (json.has("actor")) 15104 res.setActor(parseReference(getJObject(json, "actor"))); 15105 if (json.has("onBehalfOf")) 15106 res.setOnBehalfOf(parseReference(getJObject(json, "onBehalfOf"))); 15107 } 15108 15109 protected Procedure.ProcedureFocalDeviceComponent parseProcedureProcedureFocalDeviceComponent(JsonObject json, Procedure owner) throws IOException, FHIRFormatError { 15110 Procedure.ProcedureFocalDeviceComponent res = new Procedure.ProcedureFocalDeviceComponent(); 15111 parseProcedureProcedureFocalDeviceComponentProperties(json, owner, res); 15112 return res; 15113 } 15114 15115 protected void parseProcedureProcedureFocalDeviceComponentProperties(JsonObject json, Procedure owner, Procedure.ProcedureFocalDeviceComponent res) throws IOException, FHIRFormatError { 15116 parseBackboneProperties(json, res); 15117 if (json.has("action")) 15118 res.setAction(parseCodeableConcept(getJObject(json, "action"))); 15119 if (json.has("manipulated")) 15120 res.setManipulated(parseReference(getJObject(json, "manipulated"))); 15121 } 15122 15123 protected ProcedureRequest parseProcedureRequest(JsonObject json) throws IOException, FHIRFormatError { 15124 ProcedureRequest res = new ProcedureRequest(); 15125 parseProcedureRequestProperties(json, res); 15126 return res; 15127 } 15128 15129 protected void parseProcedureRequestProperties(JsonObject json, ProcedureRequest res) throws IOException, FHIRFormatError { 15130 parseDomainResourceProperties(json, res); 15131 if (json.has("identifier")) { 15132 JsonArray array = json.getAsJsonArray("identifier"); 15133 for (int i = 0; i < array.size(); i++) { 15134 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 15135 } 15136 }; 15137 if (json.has("definition")) { 15138 JsonArray array = json.getAsJsonArray("definition"); 15139 for (int i = 0; i < array.size(); i++) { 15140 res.getDefinition().add(parseReference(array.get(i).getAsJsonObject())); 15141 } 15142 }; 15143 if (json.has("basedOn")) { 15144 JsonArray array = json.getAsJsonArray("basedOn"); 15145 for (int i = 0; i < array.size(); i++) { 15146 res.getBasedOn().add(parseReference(array.get(i).getAsJsonObject())); 15147 } 15148 }; 15149 if (json.has("replaces")) { 15150 JsonArray array = json.getAsJsonArray("replaces"); 15151 for (int i = 0; i < array.size(); i++) { 15152 res.getReplaces().add(parseReference(array.get(i).getAsJsonObject())); 15153 } 15154 }; 15155 if (json.has("requisition")) 15156 res.setRequisition(parseIdentifier(getJObject(json, "requisition"))); 15157 if (json.has("status")) 15158 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), ProcedureRequest.ProcedureRequestStatus.NULL, new ProcedureRequest.ProcedureRequestStatusEnumFactory())); 15159 if (json.has("_status")) 15160 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 15161 if (json.has("intent")) 15162 res.setIntentElement(parseEnumeration(json.get("intent").getAsString(), ProcedureRequest.ProcedureRequestIntent.NULL, new ProcedureRequest.ProcedureRequestIntentEnumFactory())); 15163 if (json.has("_intent")) 15164 parseElementProperties(getJObject(json, "_intent"), res.getIntentElement()); 15165 if (json.has("priority")) 15166 res.setPriorityElement(parseEnumeration(json.get("priority").getAsString(), ProcedureRequest.ProcedureRequestPriority.NULL, new ProcedureRequest.ProcedureRequestPriorityEnumFactory())); 15167 if (json.has("_priority")) 15168 parseElementProperties(getJObject(json, "_priority"), res.getPriorityElement()); 15169 if (json.has("doNotPerform")) 15170 res.setDoNotPerformElement(parseBoolean(json.get("doNotPerform").getAsBoolean())); 15171 if (json.has("_doNotPerform")) 15172 parseElementProperties(getJObject(json, "_doNotPerform"), res.getDoNotPerformElement()); 15173 if (json.has("category")) { 15174 JsonArray array = json.getAsJsonArray("category"); 15175 for (int i = 0; i < array.size(); i++) { 15176 res.getCategory().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 15177 } 15178 }; 15179 if (json.has("code")) 15180 res.setCode(parseCodeableConcept(getJObject(json, "code"))); 15181 if (json.has("subject")) 15182 res.setSubject(parseReference(getJObject(json, "subject"))); 15183 if (json.has("context")) 15184 res.setContext(parseReference(getJObject(json, "context"))); 15185 Type occurrence = parseType("occurrence", json); 15186 if (occurrence != null) 15187 res.setOccurrence(occurrence); 15188 Type asNeeded = parseType("asNeeded", json); 15189 if (asNeeded != null) 15190 res.setAsNeeded(asNeeded); 15191 if (json.has("authoredOn")) 15192 res.setAuthoredOnElement(parseDateTime(json.get("authoredOn").getAsString())); 15193 if (json.has("_authoredOn")) 15194 parseElementProperties(getJObject(json, "_authoredOn"), res.getAuthoredOnElement()); 15195 if (json.has("requester")) 15196 res.setRequester(parseProcedureRequestProcedureRequestRequesterComponent(getJObject(json, "requester"), res)); 15197 if (json.has("performerType")) 15198 res.setPerformerType(parseCodeableConcept(getJObject(json, "performerType"))); 15199 if (json.has("performer")) 15200 res.setPerformer(parseReference(getJObject(json, "performer"))); 15201 if (json.has("reasonCode")) { 15202 JsonArray array = json.getAsJsonArray("reasonCode"); 15203 for (int i = 0; i < array.size(); i++) { 15204 res.getReasonCode().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 15205 } 15206 }; 15207 if (json.has("reasonReference")) { 15208 JsonArray array = json.getAsJsonArray("reasonReference"); 15209 for (int i = 0; i < array.size(); i++) { 15210 res.getReasonReference().add(parseReference(array.get(i).getAsJsonObject())); 15211 } 15212 }; 15213 if (json.has("supportingInfo")) { 15214 JsonArray array = json.getAsJsonArray("supportingInfo"); 15215 for (int i = 0; i < array.size(); i++) { 15216 res.getSupportingInfo().add(parseReference(array.get(i).getAsJsonObject())); 15217 } 15218 }; 15219 if (json.has("specimen")) { 15220 JsonArray array = json.getAsJsonArray("specimen"); 15221 for (int i = 0; i < array.size(); i++) { 15222 res.getSpecimen().add(parseReference(array.get(i).getAsJsonObject())); 15223 } 15224 }; 15225 if (json.has("bodySite")) { 15226 JsonArray array = json.getAsJsonArray("bodySite"); 15227 for (int i = 0; i < array.size(); i++) { 15228 res.getBodySite().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 15229 } 15230 }; 15231 if (json.has("note")) { 15232 JsonArray array = json.getAsJsonArray("note"); 15233 for (int i = 0; i < array.size(); i++) { 15234 res.getNote().add(parseAnnotation(array.get(i).getAsJsonObject())); 15235 } 15236 }; 15237 if (json.has("relevantHistory")) { 15238 JsonArray array = json.getAsJsonArray("relevantHistory"); 15239 for (int i = 0; i < array.size(); i++) { 15240 res.getRelevantHistory().add(parseReference(array.get(i).getAsJsonObject())); 15241 } 15242 }; 15243 } 15244 15245 protected ProcedureRequest.ProcedureRequestRequesterComponent parseProcedureRequestProcedureRequestRequesterComponent(JsonObject json, ProcedureRequest owner) throws IOException, FHIRFormatError { 15246 ProcedureRequest.ProcedureRequestRequesterComponent res = new ProcedureRequest.ProcedureRequestRequesterComponent(); 15247 parseProcedureRequestProcedureRequestRequesterComponentProperties(json, owner, res); 15248 return res; 15249 } 15250 15251 protected void parseProcedureRequestProcedureRequestRequesterComponentProperties(JsonObject json, ProcedureRequest owner, ProcedureRequest.ProcedureRequestRequesterComponent res) throws IOException, FHIRFormatError { 15252 parseBackboneProperties(json, res); 15253 if (json.has("agent")) 15254 res.setAgent(parseReference(getJObject(json, "agent"))); 15255 if (json.has("onBehalfOf")) 15256 res.setOnBehalfOf(parseReference(getJObject(json, "onBehalfOf"))); 15257 } 15258 15259 protected ProcessRequest parseProcessRequest(JsonObject json) throws IOException, FHIRFormatError { 15260 ProcessRequest res = new ProcessRequest(); 15261 parseProcessRequestProperties(json, res); 15262 return res; 15263 } 15264 15265 protected void parseProcessRequestProperties(JsonObject json, ProcessRequest res) throws IOException, FHIRFormatError { 15266 parseDomainResourceProperties(json, res); 15267 if (json.has("identifier")) { 15268 JsonArray array = json.getAsJsonArray("identifier"); 15269 for (int i = 0; i < array.size(); i++) { 15270 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 15271 } 15272 }; 15273 if (json.has("status")) 15274 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), ProcessRequest.ProcessRequestStatus.NULL, new ProcessRequest.ProcessRequestStatusEnumFactory())); 15275 if (json.has("_status")) 15276 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 15277 if (json.has("action")) 15278 res.setActionElement(parseEnumeration(json.get("action").getAsString(), ProcessRequest.ActionList.NULL, new ProcessRequest.ActionListEnumFactory())); 15279 if (json.has("_action")) 15280 parseElementProperties(getJObject(json, "_action"), res.getActionElement()); 15281 if (json.has("target")) 15282 res.setTarget(parseReference(getJObject(json, "target"))); 15283 if (json.has("created")) 15284 res.setCreatedElement(parseDateTime(json.get("created").getAsString())); 15285 if (json.has("_created")) 15286 parseElementProperties(getJObject(json, "_created"), res.getCreatedElement()); 15287 if (json.has("provider")) 15288 res.setProvider(parseReference(getJObject(json, "provider"))); 15289 if (json.has("organization")) 15290 res.setOrganization(parseReference(getJObject(json, "organization"))); 15291 if (json.has("request")) 15292 res.setRequest(parseReference(getJObject(json, "request"))); 15293 if (json.has("response")) 15294 res.setResponse(parseReference(getJObject(json, "response"))); 15295 if (json.has("nullify")) 15296 res.setNullifyElement(parseBoolean(json.get("nullify").getAsBoolean())); 15297 if (json.has("_nullify")) 15298 parseElementProperties(getJObject(json, "_nullify"), res.getNullifyElement()); 15299 if (json.has("reference")) 15300 res.setReferenceElement(parseString(json.get("reference").getAsString())); 15301 if (json.has("_reference")) 15302 parseElementProperties(getJObject(json, "_reference"), res.getReferenceElement()); 15303 if (json.has("item")) { 15304 JsonArray array = json.getAsJsonArray("item"); 15305 for (int i = 0; i < array.size(); i++) { 15306 res.getItem().add(parseProcessRequestItemsComponent(array.get(i).getAsJsonObject(), res)); 15307 } 15308 }; 15309 if (json.has("include")) { 15310 JsonArray array = json.getAsJsonArray("include"); 15311 for (int i = 0; i < array.size(); i++) { 15312 if (array.get(i).isJsonNull()) { 15313 res.getInclude().add(new StringType()); 15314 } else { 15315 res.getInclude().add(parseString(array.get(i).getAsString())); 15316 } 15317 } 15318 }; 15319 if (json.has("_include")) { 15320 JsonArray array = json.getAsJsonArray("_include"); 15321 for (int i = 0; i < array.size(); i++) { 15322 if (i == res.getInclude().size()) 15323 res.getInclude().add(parseString(null)); 15324 if (array.get(i) instanceof JsonObject) 15325 parseElementProperties(array.get(i).getAsJsonObject(), res.getInclude().get(i)); 15326 } 15327 }; 15328 if (json.has("exclude")) { 15329 JsonArray array = json.getAsJsonArray("exclude"); 15330 for (int i = 0; i < array.size(); i++) { 15331 if (array.get(i).isJsonNull()) { 15332 res.getExclude().add(new StringType()); 15333 } else { 15334 res.getExclude().add(parseString(array.get(i).getAsString())); 15335 } 15336 } 15337 }; 15338 if (json.has("_exclude")) { 15339 JsonArray array = json.getAsJsonArray("_exclude"); 15340 for (int i = 0; i < array.size(); i++) { 15341 if (i == res.getExclude().size()) 15342 res.getExclude().add(parseString(null)); 15343 if (array.get(i) instanceof JsonObject) 15344 parseElementProperties(array.get(i).getAsJsonObject(), res.getExclude().get(i)); 15345 } 15346 }; 15347 if (json.has("period")) 15348 res.setPeriod(parsePeriod(getJObject(json, "period"))); 15349 } 15350 15351 protected ProcessRequest.ItemsComponent parseProcessRequestItemsComponent(JsonObject json, ProcessRequest owner) throws IOException, FHIRFormatError { 15352 ProcessRequest.ItemsComponent res = new ProcessRequest.ItemsComponent(); 15353 parseProcessRequestItemsComponentProperties(json, owner, res); 15354 return res; 15355 } 15356 15357 protected void parseProcessRequestItemsComponentProperties(JsonObject json, ProcessRequest owner, ProcessRequest.ItemsComponent res) throws IOException, FHIRFormatError { 15358 parseBackboneProperties(json, res); 15359 if (json.has("sequenceLinkId")) 15360 res.setSequenceLinkIdElement(parseInteger(json.get("sequenceLinkId").getAsLong())); 15361 if (json.has("_sequenceLinkId")) 15362 parseElementProperties(getJObject(json, "_sequenceLinkId"), res.getSequenceLinkIdElement()); 15363 } 15364 15365 protected ProcessResponse parseProcessResponse(JsonObject json) throws IOException, FHIRFormatError { 15366 ProcessResponse res = new ProcessResponse(); 15367 parseProcessResponseProperties(json, res); 15368 return res; 15369 } 15370 15371 protected void parseProcessResponseProperties(JsonObject json, ProcessResponse res) throws IOException, FHIRFormatError { 15372 parseDomainResourceProperties(json, res); 15373 if (json.has("identifier")) { 15374 JsonArray array = json.getAsJsonArray("identifier"); 15375 for (int i = 0; i < array.size(); i++) { 15376 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 15377 } 15378 }; 15379 if (json.has("status")) 15380 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), ProcessResponse.ProcessResponseStatus.NULL, new ProcessResponse.ProcessResponseStatusEnumFactory())); 15381 if (json.has("_status")) 15382 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 15383 if (json.has("created")) 15384 res.setCreatedElement(parseDateTime(json.get("created").getAsString())); 15385 if (json.has("_created")) 15386 parseElementProperties(getJObject(json, "_created"), res.getCreatedElement()); 15387 if (json.has("organization")) 15388 res.setOrganization(parseReference(getJObject(json, "organization"))); 15389 if (json.has("request")) 15390 res.setRequest(parseReference(getJObject(json, "request"))); 15391 if (json.has("outcome")) 15392 res.setOutcome(parseCodeableConcept(getJObject(json, "outcome"))); 15393 if (json.has("disposition")) 15394 res.setDispositionElement(parseString(json.get("disposition").getAsString())); 15395 if (json.has("_disposition")) 15396 parseElementProperties(getJObject(json, "_disposition"), res.getDispositionElement()); 15397 if (json.has("requestProvider")) 15398 res.setRequestProvider(parseReference(getJObject(json, "requestProvider"))); 15399 if (json.has("requestOrganization")) 15400 res.setRequestOrganization(parseReference(getJObject(json, "requestOrganization"))); 15401 if (json.has("form")) 15402 res.setForm(parseCodeableConcept(getJObject(json, "form"))); 15403 if (json.has("processNote")) { 15404 JsonArray array = json.getAsJsonArray("processNote"); 15405 for (int i = 0; i < array.size(); i++) { 15406 res.getProcessNote().add(parseProcessResponseProcessResponseProcessNoteComponent(array.get(i).getAsJsonObject(), res)); 15407 } 15408 }; 15409 if (json.has("error")) { 15410 JsonArray array = json.getAsJsonArray("error"); 15411 for (int i = 0; i < array.size(); i++) { 15412 res.getError().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 15413 } 15414 }; 15415 if (json.has("communicationRequest")) { 15416 JsonArray array = json.getAsJsonArray("communicationRequest"); 15417 for (int i = 0; i < array.size(); i++) { 15418 res.getCommunicationRequest().add(parseReference(array.get(i).getAsJsonObject())); 15419 } 15420 }; 15421 } 15422 15423 protected ProcessResponse.ProcessResponseProcessNoteComponent parseProcessResponseProcessResponseProcessNoteComponent(JsonObject json, ProcessResponse owner) throws IOException, FHIRFormatError { 15424 ProcessResponse.ProcessResponseProcessNoteComponent res = new ProcessResponse.ProcessResponseProcessNoteComponent(); 15425 parseProcessResponseProcessResponseProcessNoteComponentProperties(json, owner, res); 15426 return res; 15427 } 15428 15429 protected void parseProcessResponseProcessResponseProcessNoteComponentProperties(JsonObject json, ProcessResponse owner, ProcessResponse.ProcessResponseProcessNoteComponent res) throws IOException, FHIRFormatError { 15430 parseBackboneProperties(json, res); 15431 if (json.has("type")) 15432 res.setType(parseCodeableConcept(getJObject(json, "type"))); 15433 if (json.has("text")) 15434 res.setTextElement(parseString(json.get("text").getAsString())); 15435 if (json.has("_text")) 15436 parseElementProperties(getJObject(json, "_text"), res.getTextElement()); 15437 } 15438 15439 protected Provenance parseProvenance(JsonObject json) throws IOException, FHIRFormatError { 15440 Provenance res = new Provenance(); 15441 parseProvenanceProperties(json, res); 15442 return res; 15443 } 15444 15445 protected void parseProvenanceProperties(JsonObject json, Provenance res) throws IOException, FHIRFormatError { 15446 parseDomainResourceProperties(json, res); 15447 if (json.has("target")) { 15448 JsonArray array = json.getAsJsonArray("target"); 15449 for (int i = 0; i < array.size(); i++) { 15450 res.getTarget().add(parseReference(array.get(i).getAsJsonObject())); 15451 } 15452 }; 15453 if (json.has("period")) 15454 res.setPeriod(parsePeriod(getJObject(json, "period"))); 15455 if (json.has("recorded")) 15456 res.setRecordedElement(parseInstant(json.get("recorded").getAsString())); 15457 if (json.has("_recorded")) 15458 parseElementProperties(getJObject(json, "_recorded"), res.getRecordedElement()); 15459 if (json.has("policy")) { 15460 JsonArray array = json.getAsJsonArray("policy"); 15461 for (int i = 0; i < array.size(); i++) { 15462 if (array.get(i).isJsonNull()) { 15463 res.getPolicy().add(new UriType()); 15464 } else { 15465 res.getPolicy().add(parseUri(array.get(i).getAsString())); 15466 } 15467 } 15468 }; 15469 if (json.has("_policy")) { 15470 JsonArray array = json.getAsJsonArray("_policy"); 15471 for (int i = 0; i < array.size(); i++) { 15472 if (i == res.getPolicy().size()) 15473 res.getPolicy().add(parseUri(null)); 15474 if (array.get(i) instanceof JsonObject) 15475 parseElementProperties(array.get(i).getAsJsonObject(), res.getPolicy().get(i)); 15476 } 15477 }; 15478 if (json.has("location")) 15479 res.setLocation(parseReference(getJObject(json, "location"))); 15480 if (json.has("reason")) { 15481 JsonArray array = json.getAsJsonArray("reason"); 15482 for (int i = 0; i < array.size(); i++) { 15483 res.getReason().add(parseCoding(array.get(i).getAsJsonObject())); 15484 } 15485 }; 15486 if (json.has("activity")) 15487 res.setActivity(parseCoding(getJObject(json, "activity"))); 15488 if (json.has("agent")) { 15489 JsonArray array = json.getAsJsonArray("agent"); 15490 for (int i = 0; i < array.size(); i++) { 15491 res.getAgent().add(parseProvenanceProvenanceAgentComponent(array.get(i).getAsJsonObject(), res)); 15492 } 15493 }; 15494 if (json.has("entity")) { 15495 JsonArray array = json.getAsJsonArray("entity"); 15496 for (int i = 0; i < array.size(); i++) { 15497 res.getEntity().add(parseProvenanceProvenanceEntityComponent(array.get(i).getAsJsonObject(), res)); 15498 } 15499 }; 15500 if (json.has("signature")) { 15501 JsonArray array = json.getAsJsonArray("signature"); 15502 for (int i = 0; i < array.size(); i++) { 15503 res.getSignature().add(parseSignature(array.get(i).getAsJsonObject())); 15504 } 15505 }; 15506 } 15507 15508 protected Provenance.ProvenanceAgentComponent parseProvenanceProvenanceAgentComponent(JsonObject json, Provenance owner) throws IOException, FHIRFormatError { 15509 Provenance.ProvenanceAgentComponent res = new Provenance.ProvenanceAgentComponent(); 15510 parseProvenanceProvenanceAgentComponentProperties(json, owner, res); 15511 return res; 15512 } 15513 15514 protected void parseProvenanceProvenanceAgentComponentProperties(JsonObject json, Provenance owner, Provenance.ProvenanceAgentComponent res) throws IOException, FHIRFormatError { 15515 parseBackboneProperties(json, res); 15516 if (json.has("role")) { 15517 JsonArray array = json.getAsJsonArray("role"); 15518 for (int i = 0; i < array.size(); i++) { 15519 res.getRole().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 15520 } 15521 }; 15522 Type who = parseType("who", json); 15523 if (who != null) 15524 res.setWho(who); 15525 Type onBehalfOf = parseType("onBehalfOf", json); 15526 if (onBehalfOf != null) 15527 res.setOnBehalfOf(onBehalfOf); 15528 if (json.has("relatedAgentType")) 15529 res.setRelatedAgentType(parseCodeableConcept(getJObject(json, "relatedAgentType"))); 15530 } 15531 15532 protected Provenance.ProvenanceEntityComponent parseProvenanceProvenanceEntityComponent(JsonObject json, Provenance owner) throws IOException, FHIRFormatError { 15533 Provenance.ProvenanceEntityComponent res = new Provenance.ProvenanceEntityComponent(); 15534 parseProvenanceProvenanceEntityComponentProperties(json, owner, res); 15535 return res; 15536 } 15537 15538 protected void parseProvenanceProvenanceEntityComponentProperties(JsonObject json, Provenance owner, Provenance.ProvenanceEntityComponent res) throws IOException, FHIRFormatError { 15539 parseBackboneProperties(json, res); 15540 if (json.has("role")) 15541 res.setRoleElement(parseEnumeration(json.get("role").getAsString(), Provenance.ProvenanceEntityRole.NULL, new Provenance.ProvenanceEntityRoleEnumFactory())); 15542 if (json.has("_role")) 15543 parseElementProperties(getJObject(json, "_role"), res.getRoleElement()); 15544 Type what = parseType("what", json); 15545 if (what != null) 15546 res.setWhat(what); 15547 if (json.has("agent")) { 15548 JsonArray array = json.getAsJsonArray("agent"); 15549 for (int i = 0; i < array.size(); i++) { 15550 res.getAgent().add(parseProvenanceProvenanceAgentComponent(array.get(i).getAsJsonObject(), owner)); 15551 } 15552 }; 15553 } 15554 15555 protected Questionnaire parseQuestionnaire(JsonObject json) throws IOException, FHIRFormatError { 15556 Questionnaire res = new Questionnaire(); 15557 parseQuestionnaireProperties(json, res); 15558 return res; 15559 } 15560 15561 protected void parseQuestionnaireProperties(JsonObject json, Questionnaire res) throws IOException, FHIRFormatError { 15562 parseDomainResourceProperties(json, res); 15563 if (json.has("url")) 15564 res.setUrlElement(parseUri(json.get("url").getAsString())); 15565 if (json.has("_url")) 15566 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 15567 if (json.has("identifier")) { 15568 JsonArray array = json.getAsJsonArray("identifier"); 15569 for (int i = 0; i < array.size(); i++) { 15570 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 15571 } 15572 }; 15573 if (json.has("version")) 15574 res.setVersionElement(parseString(json.get("version").getAsString())); 15575 if (json.has("_version")) 15576 parseElementProperties(getJObject(json, "_version"), res.getVersionElement()); 15577 if (json.has("name")) 15578 res.setNameElement(parseString(json.get("name").getAsString())); 15579 if (json.has("_name")) 15580 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 15581 if (json.has("title")) 15582 res.setTitleElement(parseString(json.get("title").getAsString())); 15583 if (json.has("_title")) 15584 parseElementProperties(getJObject(json, "_title"), res.getTitleElement()); 15585 if (json.has("status")) 15586 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Enumerations.PublicationStatus.NULL, new Enumerations.PublicationStatusEnumFactory())); 15587 if (json.has("_status")) 15588 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 15589 if (json.has("experimental")) 15590 res.setExperimentalElement(parseBoolean(json.get("experimental").getAsBoolean())); 15591 if (json.has("_experimental")) 15592 parseElementProperties(getJObject(json, "_experimental"), res.getExperimentalElement()); 15593 if (json.has("date")) 15594 res.setDateElement(parseDateTime(json.get("date").getAsString())); 15595 if (json.has("_date")) 15596 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 15597 if (json.has("publisher")) 15598 res.setPublisherElement(parseString(json.get("publisher").getAsString())); 15599 if (json.has("_publisher")) 15600 parseElementProperties(getJObject(json, "_publisher"), res.getPublisherElement()); 15601 if (json.has("description")) 15602 res.setDescriptionElement(parseMarkdown(json.get("description").getAsString())); 15603 if (json.has("_description")) 15604 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 15605 if (json.has("purpose")) 15606 res.setPurposeElement(parseMarkdown(json.get("purpose").getAsString())); 15607 if (json.has("_purpose")) 15608 parseElementProperties(getJObject(json, "_purpose"), res.getPurposeElement()); 15609 if (json.has("approvalDate")) 15610 res.setApprovalDateElement(parseDate(json.get("approvalDate").getAsString())); 15611 if (json.has("_approvalDate")) 15612 parseElementProperties(getJObject(json, "_approvalDate"), res.getApprovalDateElement()); 15613 if (json.has("lastReviewDate")) 15614 res.setLastReviewDateElement(parseDate(json.get("lastReviewDate").getAsString())); 15615 if (json.has("_lastReviewDate")) 15616 parseElementProperties(getJObject(json, "_lastReviewDate"), res.getLastReviewDateElement()); 15617 if (json.has("effectivePeriod")) 15618 res.setEffectivePeriod(parsePeriod(getJObject(json, "effectivePeriod"))); 15619 if (json.has("useContext")) { 15620 JsonArray array = json.getAsJsonArray("useContext"); 15621 for (int i = 0; i < array.size(); i++) { 15622 res.getUseContext().add(parseUsageContext(array.get(i).getAsJsonObject())); 15623 } 15624 }; 15625 if (json.has("jurisdiction")) { 15626 JsonArray array = json.getAsJsonArray("jurisdiction"); 15627 for (int i = 0; i < array.size(); i++) { 15628 res.getJurisdiction().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 15629 } 15630 }; 15631 if (json.has("contact")) { 15632 JsonArray array = json.getAsJsonArray("contact"); 15633 for (int i = 0; i < array.size(); i++) { 15634 res.getContact().add(parseContactDetail(array.get(i).getAsJsonObject())); 15635 } 15636 }; 15637 if (json.has("copyright")) 15638 res.setCopyrightElement(parseMarkdown(json.get("copyright").getAsString())); 15639 if (json.has("_copyright")) 15640 parseElementProperties(getJObject(json, "_copyright"), res.getCopyrightElement()); 15641 if (json.has("code")) { 15642 JsonArray array = json.getAsJsonArray("code"); 15643 for (int i = 0; i < array.size(); i++) { 15644 res.getCode().add(parseCoding(array.get(i).getAsJsonObject())); 15645 } 15646 }; 15647 if (json.has("subjectType")) { 15648 JsonArray array = json.getAsJsonArray("subjectType"); 15649 for (int i = 0; i < array.size(); i++) { 15650 if (array.get(i).isJsonNull()) { 15651 res.getSubjectType().add(new CodeType()); 15652 } else { 15653 res.getSubjectType().add(parseCode(array.get(i).getAsString())); 15654 } 15655 } 15656 }; 15657 if (json.has("_subjectType")) { 15658 JsonArray array = json.getAsJsonArray("_subjectType"); 15659 for (int i = 0; i < array.size(); i++) { 15660 if (i == res.getSubjectType().size()) 15661 res.getSubjectType().add(parseCode(null)); 15662 if (array.get(i) instanceof JsonObject) 15663 parseElementProperties(array.get(i).getAsJsonObject(), res.getSubjectType().get(i)); 15664 } 15665 }; 15666 if (json.has("item")) { 15667 JsonArray array = json.getAsJsonArray("item"); 15668 for (int i = 0; i < array.size(); i++) { 15669 res.getItem().add(parseQuestionnaireQuestionnaireItemComponent(array.get(i).getAsJsonObject(), res)); 15670 } 15671 }; 15672 } 15673 15674 protected Questionnaire.QuestionnaireItemComponent parseQuestionnaireQuestionnaireItemComponent(JsonObject json, Questionnaire owner) throws IOException, FHIRFormatError { 15675 Questionnaire.QuestionnaireItemComponent res = new Questionnaire.QuestionnaireItemComponent(); 15676 parseQuestionnaireQuestionnaireItemComponentProperties(json, owner, res); 15677 return res; 15678 } 15679 15680 protected void parseQuestionnaireQuestionnaireItemComponentProperties(JsonObject json, Questionnaire owner, Questionnaire.QuestionnaireItemComponent res) throws IOException, FHIRFormatError { 15681 parseBackboneProperties(json, res); 15682 if (json.has("linkId")) 15683 res.setLinkIdElement(parseString(json.get("linkId").getAsString())); 15684 if (json.has("_linkId")) 15685 parseElementProperties(getJObject(json, "_linkId"), res.getLinkIdElement()); 15686 if (json.has("definition")) 15687 res.setDefinitionElement(parseUri(json.get("definition").getAsString())); 15688 if (json.has("_definition")) 15689 parseElementProperties(getJObject(json, "_definition"), res.getDefinitionElement()); 15690 if (json.has("code")) { 15691 JsonArray array = json.getAsJsonArray("code"); 15692 for (int i = 0; i < array.size(); i++) { 15693 res.getCode().add(parseCoding(array.get(i).getAsJsonObject())); 15694 } 15695 }; 15696 if (json.has("prefix")) 15697 res.setPrefixElement(parseString(json.get("prefix").getAsString())); 15698 if (json.has("_prefix")) 15699 parseElementProperties(getJObject(json, "_prefix"), res.getPrefixElement()); 15700 if (json.has("text")) 15701 res.setTextElement(parseString(json.get("text").getAsString())); 15702 if (json.has("_text")) 15703 parseElementProperties(getJObject(json, "_text"), res.getTextElement()); 15704 if (json.has("type")) 15705 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), Questionnaire.QuestionnaireItemType.NULL, new Questionnaire.QuestionnaireItemTypeEnumFactory())); 15706 if (json.has("_type")) 15707 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 15708 if (json.has("enableWhen")) { 15709 JsonArray array = json.getAsJsonArray("enableWhen"); 15710 for (int i = 0; i < array.size(); i++) { 15711 res.getEnableWhen().add(parseQuestionnaireQuestionnaireItemEnableWhenComponent(array.get(i).getAsJsonObject(), owner)); 15712 } 15713 }; 15714 if (json.has("required")) 15715 res.setRequiredElement(parseBoolean(json.get("required").getAsBoolean())); 15716 if (json.has("_required")) 15717 parseElementProperties(getJObject(json, "_required"), res.getRequiredElement()); 15718 if (json.has("repeats")) 15719 res.setRepeatsElement(parseBoolean(json.get("repeats").getAsBoolean())); 15720 if (json.has("_repeats")) 15721 parseElementProperties(getJObject(json, "_repeats"), res.getRepeatsElement()); 15722 if (json.has("readOnly")) 15723 res.setReadOnlyElement(parseBoolean(json.get("readOnly").getAsBoolean())); 15724 if (json.has("_readOnly")) 15725 parseElementProperties(getJObject(json, "_readOnly"), res.getReadOnlyElement()); 15726 if (json.has("maxLength")) 15727 res.setMaxLengthElement(parseInteger(json.get("maxLength").getAsLong())); 15728 if (json.has("_maxLength")) 15729 parseElementProperties(getJObject(json, "_maxLength"), res.getMaxLengthElement()); 15730 if (json.has("options")) 15731 res.setOptions(parseReference(getJObject(json, "options"))); 15732 if (json.has("option")) { 15733 JsonArray array = json.getAsJsonArray("option"); 15734 for (int i = 0; i < array.size(); i++) { 15735 res.getOption().add(parseQuestionnaireQuestionnaireItemOptionComponent(array.get(i).getAsJsonObject(), owner)); 15736 } 15737 }; 15738 Type initial = parseType("initial", json); 15739 if (initial != null) 15740 res.setInitial(initial); 15741 if (json.has("item")) { 15742 JsonArray array = json.getAsJsonArray("item"); 15743 for (int i = 0; i < array.size(); i++) { 15744 res.getItem().add(parseQuestionnaireQuestionnaireItemComponent(array.get(i).getAsJsonObject(), owner)); 15745 } 15746 }; 15747 } 15748 15749 protected Questionnaire.QuestionnaireItemEnableWhenComponent parseQuestionnaireQuestionnaireItemEnableWhenComponent(JsonObject json, Questionnaire owner) throws IOException, FHIRFormatError { 15750 Questionnaire.QuestionnaireItemEnableWhenComponent res = new Questionnaire.QuestionnaireItemEnableWhenComponent(); 15751 parseQuestionnaireQuestionnaireItemEnableWhenComponentProperties(json, owner, res); 15752 return res; 15753 } 15754 15755 protected void parseQuestionnaireQuestionnaireItemEnableWhenComponentProperties(JsonObject json, Questionnaire owner, Questionnaire.QuestionnaireItemEnableWhenComponent res) throws IOException, FHIRFormatError { 15756 parseBackboneProperties(json, res); 15757 if (json.has("question")) 15758 res.setQuestionElement(parseString(json.get("question").getAsString())); 15759 if (json.has("_question")) 15760 parseElementProperties(getJObject(json, "_question"), res.getQuestionElement()); 15761 if (json.has("hasAnswer")) 15762 res.setHasAnswerElement(parseBoolean(json.get("hasAnswer").getAsBoolean())); 15763 if (json.has("_hasAnswer")) 15764 parseElementProperties(getJObject(json, "_hasAnswer"), res.getHasAnswerElement()); 15765 Type answer = parseType("answer", json); 15766 if (answer != null) 15767 res.setAnswer(answer); 15768 } 15769 15770 protected Questionnaire.QuestionnaireItemOptionComponent parseQuestionnaireQuestionnaireItemOptionComponent(JsonObject json, Questionnaire owner) throws IOException, FHIRFormatError { 15771 Questionnaire.QuestionnaireItemOptionComponent res = new Questionnaire.QuestionnaireItemOptionComponent(); 15772 parseQuestionnaireQuestionnaireItemOptionComponentProperties(json, owner, res); 15773 return res; 15774 } 15775 15776 protected void parseQuestionnaireQuestionnaireItemOptionComponentProperties(JsonObject json, Questionnaire owner, Questionnaire.QuestionnaireItemOptionComponent res) throws IOException, FHIRFormatError { 15777 parseBackboneProperties(json, res); 15778 Type value = parseType("value", json); 15779 if (value != null) 15780 res.setValue(value); 15781 } 15782 15783 protected QuestionnaireResponse parseQuestionnaireResponse(JsonObject json) throws IOException, FHIRFormatError { 15784 QuestionnaireResponse res = new QuestionnaireResponse(); 15785 parseQuestionnaireResponseProperties(json, res); 15786 return res; 15787 } 15788 15789 protected void parseQuestionnaireResponseProperties(JsonObject json, QuestionnaireResponse res) throws IOException, FHIRFormatError { 15790 parseDomainResourceProperties(json, res); 15791 if (json.has("identifier")) 15792 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 15793 if (json.has("basedOn")) { 15794 JsonArray array = json.getAsJsonArray("basedOn"); 15795 for (int i = 0; i < array.size(); i++) { 15796 res.getBasedOn().add(parseReference(array.get(i).getAsJsonObject())); 15797 } 15798 }; 15799 if (json.has("parent")) { 15800 JsonArray array = json.getAsJsonArray("parent"); 15801 for (int i = 0; i < array.size(); i++) { 15802 res.getParent().add(parseReference(array.get(i).getAsJsonObject())); 15803 } 15804 }; 15805 if (json.has("questionnaire")) 15806 res.setQuestionnaire(parseReference(getJObject(json, "questionnaire"))); 15807 if (json.has("status")) 15808 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), QuestionnaireResponse.QuestionnaireResponseStatus.NULL, new QuestionnaireResponse.QuestionnaireResponseStatusEnumFactory())); 15809 if (json.has("_status")) 15810 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 15811 if (json.has("subject")) 15812 res.setSubject(parseReference(getJObject(json, "subject"))); 15813 if (json.has("context")) 15814 res.setContext(parseReference(getJObject(json, "context"))); 15815 if (json.has("authored")) 15816 res.setAuthoredElement(parseDateTime(json.get("authored").getAsString())); 15817 if (json.has("_authored")) 15818 parseElementProperties(getJObject(json, "_authored"), res.getAuthoredElement()); 15819 if (json.has("author")) 15820 res.setAuthor(parseReference(getJObject(json, "author"))); 15821 if (json.has("source")) 15822 res.setSource(parseReference(getJObject(json, "source"))); 15823 if (json.has("item")) { 15824 JsonArray array = json.getAsJsonArray("item"); 15825 for (int i = 0; i < array.size(); i++) { 15826 res.getItem().add(parseQuestionnaireResponseQuestionnaireResponseItemComponent(array.get(i).getAsJsonObject(), res)); 15827 } 15828 }; 15829 } 15830 15831 protected QuestionnaireResponse.QuestionnaireResponseItemComponent parseQuestionnaireResponseQuestionnaireResponseItemComponent(JsonObject json, QuestionnaireResponse owner) throws IOException, FHIRFormatError { 15832 QuestionnaireResponse.QuestionnaireResponseItemComponent res = new QuestionnaireResponse.QuestionnaireResponseItemComponent(); 15833 parseQuestionnaireResponseQuestionnaireResponseItemComponentProperties(json, owner, res); 15834 return res; 15835 } 15836 15837 protected void parseQuestionnaireResponseQuestionnaireResponseItemComponentProperties(JsonObject json, QuestionnaireResponse owner, QuestionnaireResponse.QuestionnaireResponseItemComponent res) throws IOException, FHIRFormatError { 15838 parseBackboneProperties(json, res); 15839 if (json.has("linkId")) 15840 res.setLinkIdElement(parseString(json.get("linkId").getAsString())); 15841 if (json.has("_linkId")) 15842 parseElementProperties(getJObject(json, "_linkId"), res.getLinkIdElement()); 15843 if (json.has("definition")) 15844 res.setDefinitionElement(parseUri(json.get("definition").getAsString())); 15845 if (json.has("_definition")) 15846 parseElementProperties(getJObject(json, "_definition"), res.getDefinitionElement()); 15847 if (json.has("text")) 15848 res.setTextElement(parseString(json.get("text").getAsString())); 15849 if (json.has("_text")) 15850 parseElementProperties(getJObject(json, "_text"), res.getTextElement()); 15851 if (json.has("subject")) 15852 res.setSubject(parseReference(getJObject(json, "subject"))); 15853 if (json.has("answer")) { 15854 JsonArray array = json.getAsJsonArray("answer"); 15855 for (int i = 0; i < array.size(); i++) { 15856 res.getAnswer().add(parseQuestionnaireResponseQuestionnaireResponseItemAnswerComponent(array.get(i).getAsJsonObject(), owner)); 15857 } 15858 }; 15859 if (json.has("item")) { 15860 JsonArray array = json.getAsJsonArray("item"); 15861 for (int i = 0; i < array.size(); i++) { 15862 res.getItem().add(parseQuestionnaireResponseQuestionnaireResponseItemComponent(array.get(i).getAsJsonObject(), owner)); 15863 } 15864 }; 15865 } 15866 15867 protected QuestionnaireResponse.QuestionnaireResponseItemAnswerComponent parseQuestionnaireResponseQuestionnaireResponseItemAnswerComponent(JsonObject json, QuestionnaireResponse owner) throws IOException, FHIRFormatError { 15868 QuestionnaireResponse.QuestionnaireResponseItemAnswerComponent res = new QuestionnaireResponse.QuestionnaireResponseItemAnswerComponent(); 15869 parseQuestionnaireResponseQuestionnaireResponseItemAnswerComponentProperties(json, owner, res); 15870 return res; 15871 } 15872 15873 protected void parseQuestionnaireResponseQuestionnaireResponseItemAnswerComponentProperties(JsonObject json, QuestionnaireResponse owner, QuestionnaireResponse.QuestionnaireResponseItemAnswerComponent res) throws IOException, FHIRFormatError { 15874 parseBackboneProperties(json, res); 15875 Type value = parseType("value", json); 15876 if (value != null) 15877 res.setValue(value); 15878 if (json.has("item")) { 15879 JsonArray array = json.getAsJsonArray("item"); 15880 for (int i = 0; i < array.size(); i++) { 15881 res.getItem().add(parseQuestionnaireResponseQuestionnaireResponseItemComponent(array.get(i).getAsJsonObject(), owner)); 15882 } 15883 }; 15884 } 15885 15886 protected ReferralRequest parseReferralRequest(JsonObject json) throws IOException, FHIRFormatError { 15887 ReferralRequest res = new ReferralRequest(); 15888 parseReferralRequestProperties(json, res); 15889 return res; 15890 } 15891 15892 protected void parseReferralRequestProperties(JsonObject json, ReferralRequest res) throws IOException, FHIRFormatError { 15893 parseDomainResourceProperties(json, res); 15894 if (json.has("identifier")) { 15895 JsonArray array = json.getAsJsonArray("identifier"); 15896 for (int i = 0; i < array.size(); i++) { 15897 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 15898 } 15899 }; 15900 if (json.has("definition")) { 15901 JsonArray array = json.getAsJsonArray("definition"); 15902 for (int i = 0; i < array.size(); i++) { 15903 res.getDefinition().add(parseReference(array.get(i).getAsJsonObject())); 15904 } 15905 }; 15906 if (json.has("basedOn")) { 15907 JsonArray array = json.getAsJsonArray("basedOn"); 15908 for (int i = 0; i < array.size(); i++) { 15909 res.getBasedOn().add(parseReference(array.get(i).getAsJsonObject())); 15910 } 15911 }; 15912 if (json.has("replaces")) { 15913 JsonArray array = json.getAsJsonArray("replaces"); 15914 for (int i = 0; i < array.size(); i++) { 15915 res.getReplaces().add(parseReference(array.get(i).getAsJsonObject())); 15916 } 15917 }; 15918 if (json.has("groupIdentifier")) 15919 res.setGroupIdentifier(parseIdentifier(getJObject(json, "groupIdentifier"))); 15920 if (json.has("status")) 15921 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), ReferralRequest.ReferralRequestStatus.NULL, new ReferralRequest.ReferralRequestStatusEnumFactory())); 15922 if (json.has("_status")) 15923 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 15924 if (json.has("intent")) 15925 res.setIntentElement(parseEnumeration(json.get("intent").getAsString(), ReferralRequest.ReferralCategory.NULL, new ReferralRequest.ReferralCategoryEnumFactory())); 15926 if (json.has("_intent")) 15927 parseElementProperties(getJObject(json, "_intent"), res.getIntentElement()); 15928 if (json.has("type")) 15929 res.setType(parseCodeableConcept(getJObject(json, "type"))); 15930 if (json.has("priority")) 15931 res.setPriorityElement(parseEnumeration(json.get("priority").getAsString(), ReferralRequest.ReferralPriority.NULL, new ReferralRequest.ReferralPriorityEnumFactory())); 15932 if (json.has("_priority")) 15933 parseElementProperties(getJObject(json, "_priority"), res.getPriorityElement()); 15934 if (json.has("serviceRequested")) { 15935 JsonArray array = json.getAsJsonArray("serviceRequested"); 15936 for (int i = 0; i < array.size(); i++) { 15937 res.getServiceRequested().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 15938 } 15939 }; 15940 if (json.has("subject")) 15941 res.setSubject(parseReference(getJObject(json, "subject"))); 15942 if (json.has("context")) 15943 res.setContext(parseReference(getJObject(json, "context"))); 15944 Type occurrence = parseType("occurrence", json); 15945 if (occurrence != null) 15946 res.setOccurrence(occurrence); 15947 if (json.has("authoredOn")) 15948 res.setAuthoredOnElement(parseDateTime(json.get("authoredOn").getAsString())); 15949 if (json.has("_authoredOn")) 15950 parseElementProperties(getJObject(json, "_authoredOn"), res.getAuthoredOnElement()); 15951 if (json.has("requester")) 15952 res.setRequester(parseReferralRequestReferralRequestRequesterComponent(getJObject(json, "requester"), res)); 15953 if (json.has("specialty")) 15954 res.setSpecialty(parseCodeableConcept(getJObject(json, "specialty"))); 15955 if (json.has("recipient")) { 15956 JsonArray array = json.getAsJsonArray("recipient"); 15957 for (int i = 0; i < array.size(); i++) { 15958 res.getRecipient().add(parseReference(array.get(i).getAsJsonObject())); 15959 } 15960 }; 15961 if (json.has("reasonCode")) { 15962 JsonArray array = json.getAsJsonArray("reasonCode"); 15963 for (int i = 0; i < array.size(); i++) { 15964 res.getReasonCode().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 15965 } 15966 }; 15967 if (json.has("reasonReference")) { 15968 JsonArray array = json.getAsJsonArray("reasonReference"); 15969 for (int i = 0; i < array.size(); i++) { 15970 res.getReasonReference().add(parseReference(array.get(i).getAsJsonObject())); 15971 } 15972 }; 15973 if (json.has("description")) 15974 res.setDescriptionElement(parseString(json.get("description").getAsString())); 15975 if (json.has("_description")) 15976 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 15977 if (json.has("supportingInfo")) { 15978 JsonArray array = json.getAsJsonArray("supportingInfo"); 15979 for (int i = 0; i < array.size(); i++) { 15980 res.getSupportingInfo().add(parseReference(array.get(i).getAsJsonObject())); 15981 } 15982 }; 15983 if (json.has("note")) { 15984 JsonArray array = json.getAsJsonArray("note"); 15985 for (int i = 0; i < array.size(); i++) { 15986 res.getNote().add(parseAnnotation(array.get(i).getAsJsonObject())); 15987 } 15988 }; 15989 if (json.has("relevantHistory")) { 15990 JsonArray array = json.getAsJsonArray("relevantHistory"); 15991 for (int i = 0; i < array.size(); i++) { 15992 res.getRelevantHistory().add(parseReference(array.get(i).getAsJsonObject())); 15993 } 15994 }; 15995 } 15996 15997 protected ReferralRequest.ReferralRequestRequesterComponent parseReferralRequestReferralRequestRequesterComponent(JsonObject json, ReferralRequest owner) throws IOException, FHIRFormatError { 15998 ReferralRequest.ReferralRequestRequesterComponent res = new ReferralRequest.ReferralRequestRequesterComponent(); 15999 parseReferralRequestReferralRequestRequesterComponentProperties(json, owner, res); 16000 return res; 16001 } 16002 16003 protected void parseReferralRequestReferralRequestRequesterComponentProperties(JsonObject json, ReferralRequest owner, ReferralRequest.ReferralRequestRequesterComponent res) throws IOException, FHIRFormatError { 16004 parseBackboneProperties(json, res); 16005 if (json.has("agent")) 16006 res.setAgent(parseReference(getJObject(json, "agent"))); 16007 if (json.has("onBehalfOf")) 16008 res.setOnBehalfOf(parseReference(getJObject(json, "onBehalfOf"))); 16009 } 16010 16011 protected RelatedPerson parseRelatedPerson(JsonObject json) throws IOException, FHIRFormatError { 16012 RelatedPerson res = new RelatedPerson(); 16013 parseRelatedPersonProperties(json, res); 16014 return res; 16015 } 16016 16017 protected void parseRelatedPersonProperties(JsonObject json, RelatedPerson res) throws IOException, FHIRFormatError { 16018 parseDomainResourceProperties(json, res); 16019 if (json.has("identifier")) { 16020 JsonArray array = json.getAsJsonArray("identifier"); 16021 for (int i = 0; i < array.size(); i++) { 16022 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 16023 } 16024 }; 16025 if (json.has("active")) 16026 res.setActiveElement(parseBoolean(json.get("active").getAsBoolean())); 16027 if (json.has("_active")) 16028 parseElementProperties(getJObject(json, "_active"), res.getActiveElement()); 16029 if (json.has("patient")) 16030 res.setPatient(parseReference(getJObject(json, "patient"))); 16031 if (json.has("relationship")) 16032 res.setRelationship(parseCodeableConcept(getJObject(json, "relationship"))); 16033 if (json.has("name")) { 16034 JsonArray array = json.getAsJsonArray("name"); 16035 for (int i = 0; i < array.size(); i++) { 16036 res.getName().add(parseHumanName(array.get(i).getAsJsonObject())); 16037 } 16038 }; 16039 if (json.has("telecom")) { 16040 JsonArray array = json.getAsJsonArray("telecom"); 16041 for (int i = 0; i < array.size(); i++) { 16042 res.getTelecom().add(parseContactPoint(array.get(i).getAsJsonObject())); 16043 } 16044 }; 16045 if (json.has("gender")) 16046 res.setGenderElement(parseEnumeration(json.get("gender").getAsString(), Enumerations.AdministrativeGender.NULL, new Enumerations.AdministrativeGenderEnumFactory())); 16047 if (json.has("_gender")) 16048 parseElementProperties(getJObject(json, "_gender"), res.getGenderElement()); 16049 if (json.has("birthDate")) 16050 res.setBirthDateElement(parseDate(json.get("birthDate").getAsString())); 16051 if (json.has("_birthDate")) 16052 parseElementProperties(getJObject(json, "_birthDate"), res.getBirthDateElement()); 16053 if (json.has("address")) { 16054 JsonArray array = json.getAsJsonArray("address"); 16055 for (int i = 0; i < array.size(); i++) { 16056 res.getAddress().add(parseAddress(array.get(i).getAsJsonObject())); 16057 } 16058 }; 16059 if (json.has("photo")) { 16060 JsonArray array = json.getAsJsonArray("photo"); 16061 for (int i = 0; i < array.size(); i++) { 16062 res.getPhoto().add(parseAttachment(array.get(i).getAsJsonObject())); 16063 } 16064 }; 16065 if (json.has("period")) 16066 res.setPeriod(parsePeriod(getJObject(json, "period"))); 16067 } 16068 16069 protected RequestGroup parseRequestGroup(JsonObject json) throws IOException, FHIRFormatError { 16070 RequestGroup res = new RequestGroup(); 16071 parseRequestGroupProperties(json, res); 16072 return res; 16073 } 16074 16075 protected void parseRequestGroupProperties(JsonObject json, RequestGroup res) throws IOException, FHIRFormatError { 16076 parseDomainResourceProperties(json, res); 16077 if (json.has("identifier")) { 16078 JsonArray array = json.getAsJsonArray("identifier"); 16079 for (int i = 0; i < array.size(); i++) { 16080 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 16081 } 16082 }; 16083 if (json.has("definition")) { 16084 JsonArray array = json.getAsJsonArray("definition"); 16085 for (int i = 0; i < array.size(); i++) { 16086 res.getDefinition().add(parseReference(array.get(i).getAsJsonObject())); 16087 } 16088 }; 16089 if (json.has("basedOn")) { 16090 JsonArray array = json.getAsJsonArray("basedOn"); 16091 for (int i = 0; i < array.size(); i++) { 16092 res.getBasedOn().add(parseReference(array.get(i).getAsJsonObject())); 16093 } 16094 }; 16095 if (json.has("replaces")) { 16096 JsonArray array = json.getAsJsonArray("replaces"); 16097 for (int i = 0; i < array.size(); i++) { 16098 res.getReplaces().add(parseReference(array.get(i).getAsJsonObject())); 16099 } 16100 }; 16101 if (json.has("groupIdentifier")) 16102 res.setGroupIdentifier(parseIdentifier(getJObject(json, "groupIdentifier"))); 16103 if (json.has("status")) 16104 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), RequestGroup.RequestStatus.NULL, new RequestGroup.RequestStatusEnumFactory())); 16105 if (json.has("_status")) 16106 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 16107 if (json.has("intent")) 16108 res.setIntentElement(parseEnumeration(json.get("intent").getAsString(), RequestGroup.RequestIntent.NULL, new RequestGroup.RequestIntentEnumFactory())); 16109 if (json.has("_intent")) 16110 parseElementProperties(getJObject(json, "_intent"), res.getIntentElement()); 16111 if (json.has("priority")) 16112 res.setPriorityElement(parseEnumeration(json.get("priority").getAsString(), RequestGroup.RequestPriority.NULL, new RequestGroup.RequestPriorityEnumFactory())); 16113 if (json.has("_priority")) 16114 parseElementProperties(getJObject(json, "_priority"), res.getPriorityElement()); 16115 if (json.has("subject")) 16116 res.setSubject(parseReference(getJObject(json, "subject"))); 16117 if (json.has("context")) 16118 res.setContext(parseReference(getJObject(json, "context"))); 16119 if (json.has("authoredOn")) 16120 res.setAuthoredOnElement(parseDateTime(json.get("authoredOn").getAsString())); 16121 if (json.has("_authoredOn")) 16122 parseElementProperties(getJObject(json, "_authoredOn"), res.getAuthoredOnElement()); 16123 if (json.has("author")) 16124 res.setAuthor(parseReference(getJObject(json, "author"))); 16125 Type reason = parseType("reason", json); 16126 if (reason != null) 16127 res.setReason(reason); 16128 if (json.has("note")) { 16129 JsonArray array = json.getAsJsonArray("note"); 16130 for (int i = 0; i < array.size(); i++) { 16131 res.getNote().add(parseAnnotation(array.get(i).getAsJsonObject())); 16132 } 16133 }; 16134 if (json.has("action")) { 16135 JsonArray array = json.getAsJsonArray("action"); 16136 for (int i = 0; i < array.size(); i++) { 16137 res.getAction().add(parseRequestGroupRequestGroupActionComponent(array.get(i).getAsJsonObject(), res)); 16138 } 16139 }; 16140 } 16141 16142 protected RequestGroup.RequestGroupActionComponent parseRequestGroupRequestGroupActionComponent(JsonObject json, RequestGroup owner) throws IOException, FHIRFormatError { 16143 RequestGroup.RequestGroupActionComponent res = new RequestGroup.RequestGroupActionComponent(); 16144 parseRequestGroupRequestGroupActionComponentProperties(json, owner, res); 16145 return res; 16146 } 16147 16148 protected void parseRequestGroupRequestGroupActionComponentProperties(JsonObject json, RequestGroup owner, RequestGroup.RequestGroupActionComponent res) throws IOException, FHIRFormatError { 16149 parseBackboneProperties(json, res); 16150 if (json.has("label")) 16151 res.setLabelElement(parseString(json.get("label").getAsString())); 16152 if (json.has("_label")) 16153 parseElementProperties(getJObject(json, "_label"), res.getLabelElement()); 16154 if (json.has("title")) 16155 res.setTitleElement(parseString(json.get("title").getAsString())); 16156 if (json.has("_title")) 16157 parseElementProperties(getJObject(json, "_title"), res.getTitleElement()); 16158 if (json.has("description")) 16159 res.setDescriptionElement(parseString(json.get("description").getAsString())); 16160 if (json.has("_description")) 16161 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 16162 if (json.has("textEquivalent")) 16163 res.setTextEquivalentElement(parseString(json.get("textEquivalent").getAsString())); 16164 if (json.has("_textEquivalent")) 16165 parseElementProperties(getJObject(json, "_textEquivalent"), res.getTextEquivalentElement()); 16166 if (json.has("code")) { 16167 JsonArray array = json.getAsJsonArray("code"); 16168 for (int i = 0; i < array.size(); i++) { 16169 res.getCode().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 16170 } 16171 }; 16172 if (json.has("documentation")) { 16173 JsonArray array = json.getAsJsonArray("documentation"); 16174 for (int i = 0; i < array.size(); i++) { 16175 res.getDocumentation().add(parseRelatedArtifact(array.get(i).getAsJsonObject())); 16176 } 16177 }; 16178 if (json.has("condition")) { 16179 JsonArray array = json.getAsJsonArray("condition"); 16180 for (int i = 0; i < array.size(); i++) { 16181 res.getCondition().add(parseRequestGroupRequestGroupActionConditionComponent(array.get(i).getAsJsonObject(), owner)); 16182 } 16183 }; 16184 if (json.has("relatedAction")) { 16185 JsonArray array = json.getAsJsonArray("relatedAction"); 16186 for (int i = 0; i < array.size(); i++) { 16187 res.getRelatedAction().add(parseRequestGroupRequestGroupActionRelatedActionComponent(array.get(i).getAsJsonObject(), owner)); 16188 } 16189 }; 16190 Type timing = parseType("timing", json); 16191 if (timing != null) 16192 res.setTiming(timing); 16193 if (json.has("participant")) { 16194 JsonArray array = json.getAsJsonArray("participant"); 16195 for (int i = 0; i < array.size(); i++) { 16196 res.getParticipant().add(parseReference(array.get(i).getAsJsonObject())); 16197 } 16198 }; 16199 if (json.has("type")) 16200 res.setType(parseCoding(getJObject(json, "type"))); 16201 if (json.has("groupingBehavior")) 16202 res.setGroupingBehaviorElement(parseEnumeration(json.get("groupingBehavior").getAsString(), RequestGroup.ActionGroupingBehavior.NULL, new RequestGroup.ActionGroupingBehaviorEnumFactory())); 16203 if (json.has("_groupingBehavior")) 16204 parseElementProperties(getJObject(json, "_groupingBehavior"), res.getGroupingBehaviorElement()); 16205 if (json.has("selectionBehavior")) 16206 res.setSelectionBehaviorElement(parseEnumeration(json.get("selectionBehavior").getAsString(), RequestGroup.ActionSelectionBehavior.NULL, new RequestGroup.ActionSelectionBehaviorEnumFactory())); 16207 if (json.has("_selectionBehavior")) 16208 parseElementProperties(getJObject(json, "_selectionBehavior"), res.getSelectionBehaviorElement()); 16209 if (json.has("requiredBehavior")) 16210 res.setRequiredBehaviorElement(parseEnumeration(json.get("requiredBehavior").getAsString(), RequestGroup.ActionRequiredBehavior.NULL, new RequestGroup.ActionRequiredBehaviorEnumFactory())); 16211 if (json.has("_requiredBehavior")) 16212 parseElementProperties(getJObject(json, "_requiredBehavior"), res.getRequiredBehaviorElement()); 16213 if (json.has("precheckBehavior")) 16214 res.setPrecheckBehaviorElement(parseEnumeration(json.get("precheckBehavior").getAsString(), RequestGroup.ActionPrecheckBehavior.NULL, new RequestGroup.ActionPrecheckBehaviorEnumFactory())); 16215 if (json.has("_precheckBehavior")) 16216 parseElementProperties(getJObject(json, "_precheckBehavior"), res.getPrecheckBehaviorElement()); 16217 if (json.has("cardinalityBehavior")) 16218 res.setCardinalityBehaviorElement(parseEnumeration(json.get("cardinalityBehavior").getAsString(), RequestGroup.ActionCardinalityBehavior.NULL, new RequestGroup.ActionCardinalityBehaviorEnumFactory())); 16219 if (json.has("_cardinalityBehavior")) 16220 parseElementProperties(getJObject(json, "_cardinalityBehavior"), res.getCardinalityBehaviorElement()); 16221 if (json.has("resource")) 16222 res.setResource(parseReference(getJObject(json, "resource"))); 16223 if (json.has("action")) { 16224 JsonArray array = json.getAsJsonArray("action"); 16225 for (int i = 0; i < array.size(); i++) { 16226 res.getAction().add(parseRequestGroupRequestGroupActionComponent(array.get(i).getAsJsonObject(), owner)); 16227 } 16228 }; 16229 } 16230 16231 protected RequestGroup.RequestGroupActionConditionComponent parseRequestGroupRequestGroupActionConditionComponent(JsonObject json, RequestGroup owner) throws IOException, FHIRFormatError { 16232 RequestGroup.RequestGroupActionConditionComponent res = new RequestGroup.RequestGroupActionConditionComponent(); 16233 parseRequestGroupRequestGroupActionConditionComponentProperties(json, owner, res); 16234 return res; 16235 } 16236 16237 protected void parseRequestGroupRequestGroupActionConditionComponentProperties(JsonObject json, RequestGroup owner, RequestGroup.RequestGroupActionConditionComponent res) throws IOException, FHIRFormatError { 16238 parseBackboneProperties(json, res); 16239 if (json.has("kind")) 16240 res.setKindElement(parseEnumeration(json.get("kind").getAsString(), RequestGroup.ActionConditionKind.NULL, new RequestGroup.ActionConditionKindEnumFactory())); 16241 if (json.has("_kind")) 16242 parseElementProperties(getJObject(json, "_kind"), res.getKindElement()); 16243 if (json.has("description")) 16244 res.setDescriptionElement(parseString(json.get("description").getAsString())); 16245 if (json.has("_description")) 16246 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 16247 if (json.has("language")) 16248 res.setLanguageElement(parseString(json.get("language").getAsString())); 16249 if (json.has("_language")) 16250 parseElementProperties(getJObject(json, "_language"), res.getLanguageElement()); 16251 if (json.has("expression")) 16252 res.setExpressionElement(parseString(json.get("expression").getAsString())); 16253 if (json.has("_expression")) 16254 parseElementProperties(getJObject(json, "_expression"), res.getExpressionElement()); 16255 } 16256 16257 protected RequestGroup.RequestGroupActionRelatedActionComponent parseRequestGroupRequestGroupActionRelatedActionComponent(JsonObject json, RequestGroup owner) throws IOException, FHIRFormatError { 16258 RequestGroup.RequestGroupActionRelatedActionComponent res = new RequestGroup.RequestGroupActionRelatedActionComponent(); 16259 parseRequestGroupRequestGroupActionRelatedActionComponentProperties(json, owner, res); 16260 return res; 16261 } 16262 16263 protected void parseRequestGroupRequestGroupActionRelatedActionComponentProperties(JsonObject json, RequestGroup owner, RequestGroup.RequestGroupActionRelatedActionComponent res) throws IOException, FHIRFormatError { 16264 parseBackboneProperties(json, res); 16265 if (json.has("actionId")) 16266 res.setActionIdElement(parseId(json.get("actionId").getAsString())); 16267 if (json.has("_actionId")) 16268 parseElementProperties(getJObject(json, "_actionId"), res.getActionIdElement()); 16269 if (json.has("relationship")) 16270 res.setRelationshipElement(parseEnumeration(json.get("relationship").getAsString(), RequestGroup.ActionRelationshipType.NULL, new RequestGroup.ActionRelationshipTypeEnumFactory())); 16271 if (json.has("_relationship")) 16272 parseElementProperties(getJObject(json, "_relationship"), res.getRelationshipElement()); 16273 Type offset = parseType("offset", json); 16274 if (offset != null) 16275 res.setOffset(offset); 16276 } 16277 16278 protected ResearchStudy parseResearchStudy(JsonObject json) throws IOException, FHIRFormatError { 16279 ResearchStudy res = new ResearchStudy(); 16280 parseResearchStudyProperties(json, res); 16281 return res; 16282 } 16283 16284 protected void parseResearchStudyProperties(JsonObject json, ResearchStudy res) throws IOException, FHIRFormatError { 16285 parseDomainResourceProperties(json, res); 16286 if (json.has("identifier")) { 16287 JsonArray array = json.getAsJsonArray("identifier"); 16288 for (int i = 0; i < array.size(); i++) { 16289 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 16290 } 16291 }; 16292 if (json.has("title")) 16293 res.setTitleElement(parseString(json.get("title").getAsString())); 16294 if (json.has("_title")) 16295 parseElementProperties(getJObject(json, "_title"), res.getTitleElement()); 16296 if (json.has("protocol")) { 16297 JsonArray array = json.getAsJsonArray("protocol"); 16298 for (int i = 0; i < array.size(); i++) { 16299 res.getProtocol().add(parseReference(array.get(i).getAsJsonObject())); 16300 } 16301 }; 16302 if (json.has("partOf")) { 16303 JsonArray array = json.getAsJsonArray("partOf"); 16304 for (int i = 0; i < array.size(); i++) { 16305 res.getPartOf().add(parseReference(array.get(i).getAsJsonObject())); 16306 } 16307 }; 16308 if (json.has("status")) 16309 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), ResearchStudy.ResearchStudyStatus.NULL, new ResearchStudy.ResearchStudyStatusEnumFactory())); 16310 if (json.has("_status")) 16311 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 16312 if (json.has("category")) { 16313 JsonArray array = json.getAsJsonArray("category"); 16314 for (int i = 0; i < array.size(); i++) { 16315 res.getCategory().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 16316 } 16317 }; 16318 if (json.has("focus")) { 16319 JsonArray array = json.getAsJsonArray("focus"); 16320 for (int i = 0; i < array.size(); i++) { 16321 res.getFocus().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 16322 } 16323 }; 16324 if (json.has("contact")) { 16325 JsonArray array = json.getAsJsonArray("contact"); 16326 for (int i = 0; i < array.size(); i++) { 16327 res.getContact().add(parseContactDetail(array.get(i).getAsJsonObject())); 16328 } 16329 }; 16330 if (json.has("relatedArtifact")) { 16331 JsonArray array = json.getAsJsonArray("relatedArtifact"); 16332 for (int i = 0; i < array.size(); i++) { 16333 res.getRelatedArtifact().add(parseRelatedArtifact(array.get(i).getAsJsonObject())); 16334 } 16335 }; 16336 if (json.has("keyword")) { 16337 JsonArray array = json.getAsJsonArray("keyword"); 16338 for (int i = 0; i < array.size(); i++) { 16339 res.getKeyword().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 16340 } 16341 }; 16342 if (json.has("jurisdiction")) { 16343 JsonArray array = json.getAsJsonArray("jurisdiction"); 16344 for (int i = 0; i < array.size(); i++) { 16345 res.getJurisdiction().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 16346 } 16347 }; 16348 if (json.has("description")) 16349 res.setDescriptionElement(parseMarkdown(json.get("description").getAsString())); 16350 if (json.has("_description")) 16351 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 16352 if (json.has("enrollment")) { 16353 JsonArray array = json.getAsJsonArray("enrollment"); 16354 for (int i = 0; i < array.size(); i++) { 16355 res.getEnrollment().add(parseReference(array.get(i).getAsJsonObject())); 16356 } 16357 }; 16358 if (json.has("period")) 16359 res.setPeriod(parsePeriod(getJObject(json, "period"))); 16360 if (json.has("sponsor")) 16361 res.setSponsor(parseReference(getJObject(json, "sponsor"))); 16362 if (json.has("principalInvestigator")) 16363 res.setPrincipalInvestigator(parseReference(getJObject(json, "principalInvestigator"))); 16364 if (json.has("site")) { 16365 JsonArray array = json.getAsJsonArray("site"); 16366 for (int i = 0; i < array.size(); i++) { 16367 res.getSite().add(parseReference(array.get(i).getAsJsonObject())); 16368 } 16369 }; 16370 if (json.has("reasonStopped")) 16371 res.setReasonStopped(parseCodeableConcept(getJObject(json, "reasonStopped"))); 16372 if (json.has("note")) { 16373 JsonArray array = json.getAsJsonArray("note"); 16374 for (int i = 0; i < array.size(); i++) { 16375 res.getNote().add(parseAnnotation(array.get(i).getAsJsonObject())); 16376 } 16377 }; 16378 if (json.has("arm")) { 16379 JsonArray array = json.getAsJsonArray("arm"); 16380 for (int i = 0; i < array.size(); i++) { 16381 res.getArm().add(parseResearchStudyResearchStudyArmComponent(array.get(i).getAsJsonObject(), res)); 16382 } 16383 }; 16384 } 16385 16386 protected ResearchStudy.ResearchStudyArmComponent parseResearchStudyResearchStudyArmComponent(JsonObject json, ResearchStudy owner) throws IOException, FHIRFormatError { 16387 ResearchStudy.ResearchStudyArmComponent res = new ResearchStudy.ResearchStudyArmComponent(); 16388 parseResearchStudyResearchStudyArmComponentProperties(json, owner, res); 16389 return res; 16390 } 16391 16392 protected void parseResearchStudyResearchStudyArmComponentProperties(JsonObject json, ResearchStudy owner, ResearchStudy.ResearchStudyArmComponent res) throws IOException, FHIRFormatError { 16393 parseBackboneProperties(json, res); 16394 if (json.has("name")) 16395 res.setNameElement(parseString(json.get("name").getAsString())); 16396 if (json.has("_name")) 16397 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 16398 if (json.has("code")) 16399 res.setCode(parseCodeableConcept(getJObject(json, "code"))); 16400 if (json.has("description")) 16401 res.setDescriptionElement(parseString(json.get("description").getAsString())); 16402 if (json.has("_description")) 16403 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 16404 } 16405 16406 protected ResearchSubject parseResearchSubject(JsonObject json) throws IOException, FHIRFormatError { 16407 ResearchSubject res = new ResearchSubject(); 16408 parseResearchSubjectProperties(json, res); 16409 return res; 16410 } 16411 16412 protected void parseResearchSubjectProperties(JsonObject json, ResearchSubject res) throws IOException, FHIRFormatError { 16413 parseDomainResourceProperties(json, res); 16414 if (json.has("identifier")) 16415 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 16416 if (json.has("status")) 16417 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), ResearchSubject.ResearchSubjectStatus.NULL, new ResearchSubject.ResearchSubjectStatusEnumFactory())); 16418 if (json.has("_status")) 16419 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 16420 if (json.has("period")) 16421 res.setPeriod(parsePeriod(getJObject(json, "period"))); 16422 if (json.has("study")) 16423 res.setStudy(parseReference(getJObject(json, "study"))); 16424 if (json.has("individual")) 16425 res.setIndividual(parseReference(getJObject(json, "individual"))); 16426 if (json.has("assignedArm")) 16427 res.setAssignedArmElement(parseString(json.get("assignedArm").getAsString())); 16428 if (json.has("_assignedArm")) 16429 parseElementProperties(getJObject(json, "_assignedArm"), res.getAssignedArmElement()); 16430 if (json.has("actualArm")) 16431 res.setActualArmElement(parseString(json.get("actualArm").getAsString())); 16432 if (json.has("_actualArm")) 16433 parseElementProperties(getJObject(json, "_actualArm"), res.getActualArmElement()); 16434 if (json.has("consent")) 16435 res.setConsent(parseReference(getJObject(json, "consent"))); 16436 } 16437 16438 protected RiskAssessment parseRiskAssessment(JsonObject json) throws IOException, FHIRFormatError { 16439 RiskAssessment res = new RiskAssessment(); 16440 parseRiskAssessmentProperties(json, res); 16441 return res; 16442 } 16443 16444 protected void parseRiskAssessmentProperties(JsonObject json, RiskAssessment res) throws IOException, FHIRFormatError { 16445 parseDomainResourceProperties(json, res); 16446 if (json.has("identifier")) 16447 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 16448 if (json.has("basedOn")) 16449 res.setBasedOn(parseReference(getJObject(json, "basedOn"))); 16450 if (json.has("parent")) 16451 res.setParent(parseReference(getJObject(json, "parent"))); 16452 if (json.has("status")) 16453 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), RiskAssessment.RiskAssessmentStatus.NULL, new RiskAssessment.RiskAssessmentStatusEnumFactory())); 16454 if (json.has("_status")) 16455 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 16456 if (json.has("method")) 16457 res.setMethod(parseCodeableConcept(getJObject(json, "method"))); 16458 if (json.has("code")) 16459 res.setCode(parseCodeableConcept(getJObject(json, "code"))); 16460 if (json.has("subject")) 16461 res.setSubject(parseReference(getJObject(json, "subject"))); 16462 if (json.has("context")) 16463 res.setContext(parseReference(getJObject(json, "context"))); 16464 Type occurrence = parseType("occurrence", json); 16465 if (occurrence != null) 16466 res.setOccurrence(occurrence); 16467 if (json.has("condition")) 16468 res.setCondition(parseReference(getJObject(json, "condition"))); 16469 if (json.has("performer")) 16470 res.setPerformer(parseReference(getJObject(json, "performer"))); 16471 Type reason = parseType("reason", json); 16472 if (reason != null) 16473 res.setReason(reason); 16474 if (json.has("basis")) { 16475 JsonArray array = json.getAsJsonArray("basis"); 16476 for (int i = 0; i < array.size(); i++) { 16477 res.getBasis().add(parseReference(array.get(i).getAsJsonObject())); 16478 } 16479 }; 16480 if (json.has("prediction")) { 16481 JsonArray array = json.getAsJsonArray("prediction"); 16482 for (int i = 0; i < array.size(); i++) { 16483 res.getPrediction().add(parseRiskAssessmentRiskAssessmentPredictionComponent(array.get(i).getAsJsonObject(), res)); 16484 } 16485 }; 16486 if (json.has("mitigation")) 16487 res.setMitigationElement(parseString(json.get("mitigation").getAsString())); 16488 if (json.has("_mitigation")) 16489 parseElementProperties(getJObject(json, "_mitigation"), res.getMitigationElement()); 16490 if (json.has("comment")) 16491 res.setCommentElement(parseString(json.get("comment").getAsString())); 16492 if (json.has("_comment")) 16493 parseElementProperties(getJObject(json, "_comment"), res.getCommentElement()); 16494 } 16495 16496 protected RiskAssessment.RiskAssessmentPredictionComponent parseRiskAssessmentRiskAssessmentPredictionComponent(JsonObject json, RiskAssessment owner) throws IOException, FHIRFormatError { 16497 RiskAssessment.RiskAssessmentPredictionComponent res = new RiskAssessment.RiskAssessmentPredictionComponent(); 16498 parseRiskAssessmentRiskAssessmentPredictionComponentProperties(json, owner, res); 16499 return res; 16500 } 16501 16502 protected void parseRiskAssessmentRiskAssessmentPredictionComponentProperties(JsonObject json, RiskAssessment owner, RiskAssessment.RiskAssessmentPredictionComponent res) throws IOException, FHIRFormatError { 16503 parseBackboneProperties(json, res); 16504 if (json.has("outcome")) 16505 res.setOutcome(parseCodeableConcept(getJObject(json, "outcome"))); 16506 Type probability = parseType("probability", json); 16507 if (probability != null) 16508 res.setProbability(probability); 16509 if (json.has("qualitativeRisk")) 16510 res.setQualitativeRisk(parseCodeableConcept(getJObject(json, "qualitativeRisk"))); 16511 if (json.has("relativeRisk")) 16512 res.setRelativeRiskElement(parseDecimal(json.get("relativeRisk").getAsBigDecimal())); 16513 if (json.has("_relativeRisk")) 16514 parseElementProperties(getJObject(json, "_relativeRisk"), res.getRelativeRiskElement()); 16515 Type when = parseType("when", json); 16516 if (when != null) 16517 res.setWhen(when); 16518 if (json.has("rationale")) 16519 res.setRationaleElement(parseString(json.get("rationale").getAsString())); 16520 if (json.has("_rationale")) 16521 parseElementProperties(getJObject(json, "_rationale"), res.getRationaleElement()); 16522 } 16523 16524 protected Schedule parseSchedule(JsonObject json) throws IOException, FHIRFormatError { 16525 Schedule res = new Schedule(); 16526 parseScheduleProperties(json, res); 16527 return res; 16528 } 16529 16530 protected void parseScheduleProperties(JsonObject json, Schedule res) throws IOException, FHIRFormatError { 16531 parseDomainResourceProperties(json, res); 16532 if (json.has("identifier")) { 16533 JsonArray array = json.getAsJsonArray("identifier"); 16534 for (int i = 0; i < array.size(); i++) { 16535 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 16536 } 16537 }; 16538 if (json.has("active")) 16539 res.setActiveElement(parseBoolean(json.get("active").getAsBoolean())); 16540 if (json.has("_active")) 16541 parseElementProperties(getJObject(json, "_active"), res.getActiveElement()); 16542 if (json.has("serviceCategory")) 16543 res.setServiceCategory(parseCodeableConcept(getJObject(json, "serviceCategory"))); 16544 if (json.has("serviceType")) { 16545 JsonArray array = json.getAsJsonArray("serviceType"); 16546 for (int i = 0; i < array.size(); i++) { 16547 res.getServiceType().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 16548 } 16549 }; 16550 if (json.has("specialty")) { 16551 JsonArray array = json.getAsJsonArray("specialty"); 16552 for (int i = 0; i < array.size(); i++) { 16553 res.getSpecialty().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 16554 } 16555 }; 16556 if (json.has("actor")) { 16557 JsonArray array = json.getAsJsonArray("actor"); 16558 for (int i = 0; i < array.size(); i++) { 16559 res.getActor().add(parseReference(array.get(i).getAsJsonObject())); 16560 } 16561 }; 16562 if (json.has("planningHorizon")) 16563 res.setPlanningHorizon(parsePeriod(getJObject(json, "planningHorizon"))); 16564 if (json.has("comment")) 16565 res.setCommentElement(parseString(json.get("comment").getAsString())); 16566 if (json.has("_comment")) 16567 parseElementProperties(getJObject(json, "_comment"), res.getCommentElement()); 16568 } 16569 16570 protected SearchParameter parseSearchParameter(JsonObject json) throws IOException, FHIRFormatError { 16571 SearchParameter res = new SearchParameter(); 16572 parseSearchParameterProperties(json, res); 16573 return res; 16574 } 16575 16576 protected void parseSearchParameterProperties(JsonObject json, SearchParameter res) throws IOException, FHIRFormatError { 16577 parseDomainResourceProperties(json, res); 16578 if (json.has("url")) 16579 res.setUrlElement(parseUri(json.get("url").getAsString())); 16580 if (json.has("_url")) 16581 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 16582 if (json.has("version")) 16583 res.setVersionElement(parseString(json.get("version").getAsString())); 16584 if (json.has("_version")) 16585 parseElementProperties(getJObject(json, "_version"), res.getVersionElement()); 16586 if (json.has("name")) 16587 res.setNameElement(parseString(json.get("name").getAsString())); 16588 if (json.has("_name")) 16589 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 16590 if (json.has("status")) 16591 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Enumerations.PublicationStatus.NULL, new Enumerations.PublicationStatusEnumFactory())); 16592 if (json.has("_status")) 16593 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 16594 if (json.has("experimental")) 16595 res.setExperimentalElement(parseBoolean(json.get("experimental").getAsBoolean())); 16596 if (json.has("_experimental")) 16597 parseElementProperties(getJObject(json, "_experimental"), res.getExperimentalElement()); 16598 if (json.has("date")) 16599 res.setDateElement(parseDateTime(json.get("date").getAsString())); 16600 if (json.has("_date")) 16601 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 16602 if (json.has("publisher")) 16603 res.setPublisherElement(parseString(json.get("publisher").getAsString())); 16604 if (json.has("_publisher")) 16605 parseElementProperties(getJObject(json, "_publisher"), res.getPublisherElement()); 16606 if (json.has("contact")) { 16607 JsonArray array = json.getAsJsonArray("contact"); 16608 for (int i = 0; i < array.size(); i++) { 16609 res.getContact().add(parseContactDetail(array.get(i).getAsJsonObject())); 16610 } 16611 }; 16612 if (json.has("useContext")) { 16613 JsonArray array = json.getAsJsonArray("useContext"); 16614 for (int i = 0; i < array.size(); i++) { 16615 res.getUseContext().add(parseUsageContext(array.get(i).getAsJsonObject())); 16616 } 16617 }; 16618 if (json.has("jurisdiction")) { 16619 JsonArray array = json.getAsJsonArray("jurisdiction"); 16620 for (int i = 0; i < array.size(); i++) { 16621 res.getJurisdiction().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 16622 } 16623 }; 16624 if (json.has("purpose")) 16625 res.setPurposeElement(parseMarkdown(json.get("purpose").getAsString())); 16626 if (json.has("_purpose")) 16627 parseElementProperties(getJObject(json, "_purpose"), res.getPurposeElement()); 16628 if (json.has("code")) 16629 res.setCodeElement(parseCode(json.get("code").getAsString())); 16630 if (json.has("_code")) 16631 parseElementProperties(getJObject(json, "_code"), res.getCodeElement()); 16632 if (json.has("base")) { 16633 JsonArray array = json.getAsJsonArray("base"); 16634 for (int i = 0; i < array.size(); i++) { 16635 if (array.get(i).isJsonNull()) { 16636 res.getBase().add(new CodeType()); 16637 } else { 16638 res.getBase().add(parseCode(array.get(i).getAsString())); 16639 } 16640 } 16641 }; 16642 if (json.has("_base")) { 16643 JsonArray array = json.getAsJsonArray("_base"); 16644 for (int i = 0; i < array.size(); i++) { 16645 if (i == res.getBase().size()) 16646 res.getBase().add(parseCode(null)); 16647 if (array.get(i) instanceof JsonObject) 16648 parseElementProperties(array.get(i).getAsJsonObject(), res.getBase().get(i)); 16649 } 16650 }; 16651 if (json.has("type")) 16652 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), Enumerations.SearchParamType.NULL, new Enumerations.SearchParamTypeEnumFactory())); 16653 if (json.has("_type")) 16654 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 16655 if (json.has("derivedFrom")) 16656 res.setDerivedFromElement(parseUri(json.get("derivedFrom").getAsString())); 16657 if (json.has("_derivedFrom")) 16658 parseElementProperties(getJObject(json, "_derivedFrom"), res.getDerivedFromElement()); 16659 if (json.has("description")) 16660 res.setDescriptionElement(parseMarkdown(json.get("description").getAsString())); 16661 if (json.has("_description")) 16662 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 16663 if (json.has("expression")) 16664 res.setExpressionElement(parseString(json.get("expression").getAsString())); 16665 if (json.has("_expression")) 16666 parseElementProperties(getJObject(json, "_expression"), res.getExpressionElement()); 16667 if (json.has("xpath")) 16668 res.setXpathElement(parseString(json.get("xpath").getAsString())); 16669 if (json.has("_xpath")) 16670 parseElementProperties(getJObject(json, "_xpath"), res.getXpathElement()); 16671 if (json.has("xpathUsage")) 16672 res.setXpathUsageElement(parseEnumeration(json.get("xpathUsage").getAsString(), SearchParameter.XPathUsageType.NULL, new SearchParameter.XPathUsageTypeEnumFactory())); 16673 if (json.has("_xpathUsage")) 16674 parseElementProperties(getJObject(json, "_xpathUsage"), res.getXpathUsageElement()); 16675 if (json.has("target")) { 16676 JsonArray array = json.getAsJsonArray("target"); 16677 for (int i = 0; i < array.size(); i++) { 16678 if (array.get(i).isJsonNull()) { 16679 res.getTarget().add(new CodeType()); 16680 } else { 16681 res.getTarget().add(parseCode(array.get(i).getAsString())); 16682 } 16683 } 16684 }; 16685 if (json.has("_target")) { 16686 JsonArray array = json.getAsJsonArray("_target"); 16687 for (int i = 0; i < array.size(); i++) { 16688 if (i == res.getTarget().size()) 16689 res.getTarget().add(parseCode(null)); 16690 if (array.get(i) instanceof JsonObject) 16691 parseElementProperties(array.get(i).getAsJsonObject(), res.getTarget().get(i)); 16692 } 16693 }; 16694 if (json.has("comparator")) { 16695 JsonArray array = json.getAsJsonArray("comparator"); 16696 for (int i = 0; i < array.size(); i++) { 16697 if (array.get(i).isJsonNull()) { 16698 res.getComparator().add(new Enumeration<SearchParameter.SearchComparator>()); 16699 } else { 16700 res.getComparator().add(parseEnumeration(array.get(i).getAsString(), SearchParameter.SearchComparator.NULL, new SearchParameter.SearchComparatorEnumFactory())); 16701 } 16702 } 16703 }; 16704 if (json.has("_comparator")) { 16705 JsonArray array = json.getAsJsonArray("_comparator"); 16706 for (int i = 0; i < array.size(); i++) { 16707 if (i == res.getComparator().size()) 16708 res.getComparator().add(parseEnumeration(null, SearchParameter.SearchComparator.NULL, new SearchParameter.SearchComparatorEnumFactory())); 16709 if (array.get(i) instanceof JsonObject) 16710 parseElementProperties(array.get(i).getAsJsonObject(), res.getComparator().get(i)); 16711 } 16712 }; 16713 if (json.has("modifier")) { 16714 JsonArray array = json.getAsJsonArray("modifier"); 16715 for (int i = 0; i < array.size(); i++) { 16716 if (array.get(i).isJsonNull()) { 16717 res.getModifier().add(new Enumeration<SearchParameter.SearchModifierCode>()); 16718 } else { 16719 res.getModifier().add(parseEnumeration(array.get(i).getAsString(), SearchParameter.SearchModifierCode.NULL, new SearchParameter.SearchModifierCodeEnumFactory())); 16720 } 16721 } 16722 }; 16723 if (json.has("_modifier")) { 16724 JsonArray array = json.getAsJsonArray("_modifier"); 16725 for (int i = 0; i < array.size(); i++) { 16726 if (i == res.getModifier().size()) 16727 res.getModifier().add(parseEnumeration(null, SearchParameter.SearchModifierCode.NULL, new SearchParameter.SearchModifierCodeEnumFactory())); 16728 if (array.get(i) instanceof JsonObject) 16729 parseElementProperties(array.get(i).getAsJsonObject(), res.getModifier().get(i)); 16730 } 16731 }; 16732 if (json.has("chain")) { 16733 JsonArray array = json.getAsJsonArray("chain"); 16734 for (int i = 0; i < array.size(); i++) { 16735 if (array.get(i).isJsonNull()) { 16736 res.getChain().add(new StringType()); 16737 } else { 16738 res.getChain().add(parseString(array.get(i).getAsString())); 16739 } 16740 } 16741 }; 16742 if (json.has("_chain")) { 16743 JsonArray array = json.getAsJsonArray("_chain"); 16744 for (int i = 0; i < array.size(); i++) { 16745 if (i == res.getChain().size()) 16746 res.getChain().add(parseString(null)); 16747 if (array.get(i) instanceof JsonObject) 16748 parseElementProperties(array.get(i).getAsJsonObject(), res.getChain().get(i)); 16749 } 16750 }; 16751 if (json.has("component")) { 16752 JsonArray array = json.getAsJsonArray("component"); 16753 for (int i = 0; i < array.size(); i++) { 16754 res.getComponent().add(parseSearchParameterSearchParameterComponentComponent(array.get(i).getAsJsonObject(), res)); 16755 } 16756 }; 16757 } 16758 16759 protected SearchParameter.SearchParameterComponentComponent parseSearchParameterSearchParameterComponentComponent(JsonObject json, SearchParameter owner) throws IOException, FHIRFormatError { 16760 SearchParameter.SearchParameterComponentComponent res = new SearchParameter.SearchParameterComponentComponent(); 16761 parseSearchParameterSearchParameterComponentComponentProperties(json, owner, res); 16762 return res; 16763 } 16764 16765 protected void parseSearchParameterSearchParameterComponentComponentProperties(JsonObject json, SearchParameter owner, SearchParameter.SearchParameterComponentComponent res) throws IOException, FHIRFormatError { 16766 parseBackboneProperties(json, res); 16767 if (json.has("definition")) 16768 res.setDefinition(parseReference(getJObject(json, "definition"))); 16769 if (json.has("expression")) 16770 res.setExpressionElement(parseString(json.get("expression").getAsString())); 16771 if (json.has("_expression")) 16772 parseElementProperties(getJObject(json, "_expression"), res.getExpressionElement()); 16773 } 16774 16775 protected Sequence parseSequence(JsonObject json) throws IOException, FHIRFormatError { 16776 Sequence res = new Sequence(); 16777 parseSequenceProperties(json, res); 16778 return res; 16779 } 16780 16781 protected void parseSequenceProperties(JsonObject json, Sequence res) throws IOException, FHIRFormatError { 16782 parseDomainResourceProperties(json, res); 16783 if (json.has("identifier")) { 16784 JsonArray array = json.getAsJsonArray("identifier"); 16785 for (int i = 0; i < array.size(); i++) { 16786 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 16787 } 16788 }; 16789 if (json.has("type")) 16790 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), Sequence.SequenceType.NULL, new Sequence.SequenceTypeEnumFactory())); 16791 if (json.has("_type")) 16792 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 16793 if (json.has("coordinateSystem")) 16794 res.setCoordinateSystemElement(parseInteger(json.get("coordinateSystem").getAsLong())); 16795 if (json.has("_coordinateSystem")) 16796 parseElementProperties(getJObject(json, "_coordinateSystem"), res.getCoordinateSystemElement()); 16797 if (json.has("patient")) 16798 res.setPatient(parseReference(getJObject(json, "patient"))); 16799 if (json.has("specimen")) 16800 res.setSpecimen(parseReference(getJObject(json, "specimen"))); 16801 if (json.has("device")) 16802 res.setDevice(parseReference(getJObject(json, "device"))); 16803 if (json.has("performer")) 16804 res.setPerformer(parseReference(getJObject(json, "performer"))); 16805 if (json.has("quantity")) 16806 res.setQuantity(parseQuantity(getJObject(json, "quantity"))); 16807 if (json.has("referenceSeq")) 16808 res.setReferenceSeq(parseSequenceSequenceReferenceSeqComponent(getJObject(json, "referenceSeq"), res)); 16809 if (json.has("variant")) { 16810 JsonArray array = json.getAsJsonArray("variant"); 16811 for (int i = 0; i < array.size(); i++) { 16812 res.getVariant().add(parseSequenceSequenceVariantComponent(array.get(i).getAsJsonObject(), res)); 16813 } 16814 }; 16815 if (json.has("observedSeq")) 16816 res.setObservedSeqElement(parseString(json.get("observedSeq").getAsString())); 16817 if (json.has("_observedSeq")) 16818 parseElementProperties(getJObject(json, "_observedSeq"), res.getObservedSeqElement()); 16819 if (json.has("quality")) { 16820 JsonArray array = json.getAsJsonArray("quality"); 16821 for (int i = 0; i < array.size(); i++) { 16822 res.getQuality().add(parseSequenceSequenceQualityComponent(array.get(i).getAsJsonObject(), res)); 16823 } 16824 }; 16825 if (json.has("readCoverage")) 16826 res.setReadCoverageElement(parseInteger(json.get("readCoverage").getAsLong())); 16827 if (json.has("_readCoverage")) 16828 parseElementProperties(getJObject(json, "_readCoverage"), res.getReadCoverageElement()); 16829 if (json.has("repository")) { 16830 JsonArray array = json.getAsJsonArray("repository"); 16831 for (int i = 0; i < array.size(); i++) { 16832 res.getRepository().add(parseSequenceSequenceRepositoryComponent(array.get(i).getAsJsonObject(), res)); 16833 } 16834 }; 16835 if (json.has("pointer")) { 16836 JsonArray array = json.getAsJsonArray("pointer"); 16837 for (int i = 0; i < array.size(); i++) { 16838 res.getPointer().add(parseReference(array.get(i).getAsJsonObject())); 16839 } 16840 }; 16841 } 16842 16843 protected Sequence.SequenceReferenceSeqComponent parseSequenceSequenceReferenceSeqComponent(JsonObject json, Sequence owner) throws IOException, FHIRFormatError { 16844 Sequence.SequenceReferenceSeqComponent res = new Sequence.SequenceReferenceSeqComponent(); 16845 parseSequenceSequenceReferenceSeqComponentProperties(json, owner, res); 16846 return res; 16847 } 16848 16849 protected void parseSequenceSequenceReferenceSeqComponentProperties(JsonObject json, Sequence owner, Sequence.SequenceReferenceSeqComponent res) throws IOException, FHIRFormatError { 16850 parseBackboneProperties(json, res); 16851 if (json.has("chromosome")) 16852 res.setChromosome(parseCodeableConcept(getJObject(json, "chromosome"))); 16853 if (json.has("genomeBuild")) 16854 res.setGenomeBuildElement(parseString(json.get("genomeBuild").getAsString())); 16855 if (json.has("_genomeBuild")) 16856 parseElementProperties(getJObject(json, "_genomeBuild"), res.getGenomeBuildElement()); 16857 if (json.has("referenceSeqId")) 16858 res.setReferenceSeqId(parseCodeableConcept(getJObject(json, "referenceSeqId"))); 16859 if (json.has("referenceSeqPointer")) 16860 res.setReferenceSeqPointer(parseReference(getJObject(json, "referenceSeqPointer"))); 16861 if (json.has("referenceSeqString")) 16862 res.setReferenceSeqStringElement(parseString(json.get("referenceSeqString").getAsString())); 16863 if (json.has("_referenceSeqString")) 16864 parseElementProperties(getJObject(json, "_referenceSeqString"), res.getReferenceSeqStringElement()); 16865 if (json.has("strand")) 16866 res.setStrandElement(parseInteger(json.get("strand").getAsLong())); 16867 if (json.has("_strand")) 16868 parseElementProperties(getJObject(json, "_strand"), res.getStrandElement()); 16869 if (json.has("windowStart")) 16870 res.setWindowStartElement(parseInteger(json.get("windowStart").getAsLong())); 16871 if (json.has("_windowStart")) 16872 parseElementProperties(getJObject(json, "_windowStart"), res.getWindowStartElement()); 16873 if (json.has("windowEnd")) 16874 res.setWindowEndElement(parseInteger(json.get("windowEnd").getAsLong())); 16875 if (json.has("_windowEnd")) 16876 parseElementProperties(getJObject(json, "_windowEnd"), res.getWindowEndElement()); 16877 } 16878 16879 protected Sequence.SequenceVariantComponent parseSequenceSequenceVariantComponent(JsonObject json, Sequence owner) throws IOException, FHIRFormatError { 16880 Sequence.SequenceVariantComponent res = new Sequence.SequenceVariantComponent(); 16881 parseSequenceSequenceVariantComponentProperties(json, owner, res); 16882 return res; 16883 } 16884 16885 protected void parseSequenceSequenceVariantComponentProperties(JsonObject json, Sequence owner, Sequence.SequenceVariantComponent res) throws IOException, FHIRFormatError { 16886 parseBackboneProperties(json, res); 16887 if (json.has("start")) 16888 res.setStartElement(parseInteger(json.get("start").getAsLong())); 16889 if (json.has("_start")) 16890 parseElementProperties(getJObject(json, "_start"), res.getStartElement()); 16891 if (json.has("end")) 16892 res.setEndElement(parseInteger(json.get("end").getAsLong())); 16893 if (json.has("_end")) 16894 parseElementProperties(getJObject(json, "_end"), res.getEndElement()); 16895 if (json.has("observedAllele")) 16896 res.setObservedAlleleElement(parseString(json.get("observedAllele").getAsString())); 16897 if (json.has("_observedAllele")) 16898 parseElementProperties(getJObject(json, "_observedAllele"), res.getObservedAlleleElement()); 16899 if (json.has("referenceAllele")) 16900 res.setReferenceAlleleElement(parseString(json.get("referenceAllele").getAsString())); 16901 if (json.has("_referenceAllele")) 16902 parseElementProperties(getJObject(json, "_referenceAllele"), res.getReferenceAlleleElement()); 16903 if (json.has("cigar")) 16904 res.setCigarElement(parseString(json.get("cigar").getAsString())); 16905 if (json.has("_cigar")) 16906 parseElementProperties(getJObject(json, "_cigar"), res.getCigarElement()); 16907 if (json.has("variantPointer")) 16908 res.setVariantPointer(parseReference(getJObject(json, "variantPointer"))); 16909 } 16910 16911 protected Sequence.SequenceQualityComponent parseSequenceSequenceQualityComponent(JsonObject json, Sequence owner) throws IOException, FHIRFormatError { 16912 Sequence.SequenceQualityComponent res = new Sequence.SequenceQualityComponent(); 16913 parseSequenceSequenceQualityComponentProperties(json, owner, res); 16914 return res; 16915 } 16916 16917 protected void parseSequenceSequenceQualityComponentProperties(JsonObject json, Sequence owner, Sequence.SequenceQualityComponent res) throws IOException, FHIRFormatError { 16918 parseBackboneProperties(json, res); 16919 if (json.has("type")) 16920 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), Sequence.QualityType.NULL, new Sequence.QualityTypeEnumFactory())); 16921 if (json.has("_type")) 16922 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 16923 if (json.has("standardSequence")) 16924 res.setStandardSequence(parseCodeableConcept(getJObject(json, "standardSequence"))); 16925 if (json.has("start")) 16926 res.setStartElement(parseInteger(json.get("start").getAsLong())); 16927 if (json.has("_start")) 16928 parseElementProperties(getJObject(json, "_start"), res.getStartElement()); 16929 if (json.has("end")) 16930 res.setEndElement(parseInteger(json.get("end").getAsLong())); 16931 if (json.has("_end")) 16932 parseElementProperties(getJObject(json, "_end"), res.getEndElement()); 16933 if (json.has("score")) 16934 res.setScore(parseQuantity(getJObject(json, "score"))); 16935 if (json.has("method")) 16936 res.setMethod(parseCodeableConcept(getJObject(json, "method"))); 16937 if (json.has("truthTP")) 16938 res.setTruthTPElement(parseDecimal(json.get("truthTP").getAsBigDecimal())); 16939 if (json.has("_truthTP")) 16940 parseElementProperties(getJObject(json, "_truthTP"), res.getTruthTPElement()); 16941 if (json.has("queryTP")) 16942 res.setQueryTPElement(parseDecimal(json.get("queryTP").getAsBigDecimal())); 16943 if (json.has("_queryTP")) 16944 parseElementProperties(getJObject(json, "_queryTP"), res.getQueryTPElement()); 16945 if (json.has("truthFN")) 16946 res.setTruthFNElement(parseDecimal(json.get("truthFN").getAsBigDecimal())); 16947 if (json.has("_truthFN")) 16948 parseElementProperties(getJObject(json, "_truthFN"), res.getTruthFNElement()); 16949 if (json.has("queryFP")) 16950 res.setQueryFPElement(parseDecimal(json.get("queryFP").getAsBigDecimal())); 16951 if (json.has("_queryFP")) 16952 parseElementProperties(getJObject(json, "_queryFP"), res.getQueryFPElement()); 16953 if (json.has("gtFP")) 16954 res.setGtFPElement(parseDecimal(json.get("gtFP").getAsBigDecimal())); 16955 if (json.has("_gtFP")) 16956 parseElementProperties(getJObject(json, "_gtFP"), res.getGtFPElement()); 16957 if (json.has("precision")) 16958 res.setPrecisionElement(parseDecimal(json.get("precision").getAsBigDecimal())); 16959 if (json.has("_precision")) 16960 parseElementProperties(getJObject(json, "_precision"), res.getPrecisionElement()); 16961 if (json.has("recall")) 16962 res.setRecallElement(parseDecimal(json.get("recall").getAsBigDecimal())); 16963 if (json.has("_recall")) 16964 parseElementProperties(getJObject(json, "_recall"), res.getRecallElement()); 16965 if (json.has("fScore")) 16966 res.setFScoreElement(parseDecimal(json.get("fScore").getAsBigDecimal())); 16967 if (json.has("_fScore")) 16968 parseElementProperties(getJObject(json, "_fScore"), res.getFScoreElement()); 16969 } 16970 16971 protected Sequence.SequenceRepositoryComponent parseSequenceSequenceRepositoryComponent(JsonObject json, Sequence owner) throws IOException, FHIRFormatError { 16972 Sequence.SequenceRepositoryComponent res = new Sequence.SequenceRepositoryComponent(); 16973 parseSequenceSequenceRepositoryComponentProperties(json, owner, res); 16974 return res; 16975 } 16976 16977 protected void parseSequenceSequenceRepositoryComponentProperties(JsonObject json, Sequence owner, Sequence.SequenceRepositoryComponent res) throws IOException, FHIRFormatError { 16978 parseBackboneProperties(json, res); 16979 if (json.has("type")) 16980 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), Sequence.RepositoryType.NULL, new Sequence.RepositoryTypeEnumFactory())); 16981 if (json.has("_type")) 16982 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 16983 if (json.has("url")) 16984 res.setUrlElement(parseUri(json.get("url").getAsString())); 16985 if (json.has("_url")) 16986 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 16987 if (json.has("name")) 16988 res.setNameElement(parseString(json.get("name").getAsString())); 16989 if (json.has("_name")) 16990 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 16991 if (json.has("datasetId")) 16992 res.setDatasetIdElement(parseString(json.get("datasetId").getAsString())); 16993 if (json.has("_datasetId")) 16994 parseElementProperties(getJObject(json, "_datasetId"), res.getDatasetIdElement()); 16995 if (json.has("variantsetId")) 16996 res.setVariantsetIdElement(parseString(json.get("variantsetId").getAsString())); 16997 if (json.has("_variantsetId")) 16998 parseElementProperties(getJObject(json, "_variantsetId"), res.getVariantsetIdElement()); 16999 if (json.has("readsetId")) 17000 res.setReadsetIdElement(parseString(json.get("readsetId").getAsString())); 17001 if (json.has("_readsetId")) 17002 parseElementProperties(getJObject(json, "_readsetId"), res.getReadsetIdElement()); 17003 } 17004 17005 protected ServiceDefinition parseServiceDefinition(JsonObject json) throws IOException, FHIRFormatError { 17006 ServiceDefinition res = new ServiceDefinition(); 17007 parseServiceDefinitionProperties(json, res); 17008 return res; 17009 } 17010 17011 protected void parseServiceDefinitionProperties(JsonObject json, ServiceDefinition res) throws IOException, FHIRFormatError { 17012 parseDomainResourceProperties(json, res); 17013 if (json.has("url")) 17014 res.setUrlElement(parseUri(json.get("url").getAsString())); 17015 if (json.has("_url")) 17016 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 17017 if (json.has("identifier")) { 17018 JsonArray array = json.getAsJsonArray("identifier"); 17019 for (int i = 0; i < array.size(); i++) { 17020 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 17021 } 17022 }; 17023 if (json.has("version")) 17024 res.setVersionElement(parseString(json.get("version").getAsString())); 17025 if (json.has("_version")) 17026 parseElementProperties(getJObject(json, "_version"), res.getVersionElement()); 17027 if (json.has("name")) 17028 res.setNameElement(parseString(json.get("name").getAsString())); 17029 if (json.has("_name")) 17030 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 17031 if (json.has("title")) 17032 res.setTitleElement(parseString(json.get("title").getAsString())); 17033 if (json.has("_title")) 17034 parseElementProperties(getJObject(json, "_title"), res.getTitleElement()); 17035 if (json.has("status")) 17036 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Enumerations.PublicationStatus.NULL, new Enumerations.PublicationStatusEnumFactory())); 17037 if (json.has("_status")) 17038 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 17039 if (json.has("experimental")) 17040 res.setExperimentalElement(parseBoolean(json.get("experimental").getAsBoolean())); 17041 if (json.has("_experimental")) 17042 parseElementProperties(getJObject(json, "_experimental"), res.getExperimentalElement()); 17043 if (json.has("date")) 17044 res.setDateElement(parseDateTime(json.get("date").getAsString())); 17045 if (json.has("_date")) 17046 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 17047 if (json.has("publisher")) 17048 res.setPublisherElement(parseString(json.get("publisher").getAsString())); 17049 if (json.has("_publisher")) 17050 parseElementProperties(getJObject(json, "_publisher"), res.getPublisherElement()); 17051 if (json.has("description")) 17052 res.setDescriptionElement(parseMarkdown(json.get("description").getAsString())); 17053 if (json.has("_description")) 17054 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 17055 if (json.has("purpose")) 17056 res.setPurposeElement(parseMarkdown(json.get("purpose").getAsString())); 17057 if (json.has("_purpose")) 17058 parseElementProperties(getJObject(json, "_purpose"), res.getPurposeElement()); 17059 if (json.has("usage")) 17060 res.setUsageElement(parseString(json.get("usage").getAsString())); 17061 if (json.has("_usage")) 17062 parseElementProperties(getJObject(json, "_usage"), res.getUsageElement()); 17063 if (json.has("approvalDate")) 17064 res.setApprovalDateElement(parseDate(json.get("approvalDate").getAsString())); 17065 if (json.has("_approvalDate")) 17066 parseElementProperties(getJObject(json, "_approvalDate"), res.getApprovalDateElement()); 17067 if (json.has("lastReviewDate")) 17068 res.setLastReviewDateElement(parseDate(json.get("lastReviewDate").getAsString())); 17069 if (json.has("_lastReviewDate")) 17070 parseElementProperties(getJObject(json, "_lastReviewDate"), res.getLastReviewDateElement()); 17071 if (json.has("effectivePeriod")) 17072 res.setEffectivePeriod(parsePeriod(getJObject(json, "effectivePeriod"))); 17073 if (json.has("useContext")) { 17074 JsonArray array = json.getAsJsonArray("useContext"); 17075 for (int i = 0; i < array.size(); i++) { 17076 res.getUseContext().add(parseUsageContext(array.get(i).getAsJsonObject())); 17077 } 17078 }; 17079 if (json.has("jurisdiction")) { 17080 JsonArray array = json.getAsJsonArray("jurisdiction"); 17081 for (int i = 0; i < array.size(); i++) { 17082 res.getJurisdiction().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 17083 } 17084 }; 17085 if (json.has("topic")) { 17086 JsonArray array = json.getAsJsonArray("topic"); 17087 for (int i = 0; i < array.size(); i++) { 17088 res.getTopic().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 17089 } 17090 }; 17091 if (json.has("contributor")) { 17092 JsonArray array = json.getAsJsonArray("contributor"); 17093 for (int i = 0; i < array.size(); i++) { 17094 res.getContributor().add(parseContributor(array.get(i).getAsJsonObject())); 17095 } 17096 }; 17097 if (json.has("contact")) { 17098 JsonArray array = json.getAsJsonArray("contact"); 17099 for (int i = 0; i < array.size(); i++) { 17100 res.getContact().add(parseContactDetail(array.get(i).getAsJsonObject())); 17101 } 17102 }; 17103 if (json.has("copyright")) 17104 res.setCopyrightElement(parseMarkdown(json.get("copyright").getAsString())); 17105 if (json.has("_copyright")) 17106 parseElementProperties(getJObject(json, "_copyright"), res.getCopyrightElement()); 17107 if (json.has("relatedArtifact")) { 17108 JsonArray array = json.getAsJsonArray("relatedArtifact"); 17109 for (int i = 0; i < array.size(); i++) { 17110 res.getRelatedArtifact().add(parseRelatedArtifact(array.get(i).getAsJsonObject())); 17111 } 17112 }; 17113 if (json.has("trigger")) { 17114 JsonArray array = json.getAsJsonArray("trigger"); 17115 for (int i = 0; i < array.size(); i++) { 17116 res.getTrigger().add(parseTriggerDefinition(array.get(i).getAsJsonObject())); 17117 } 17118 }; 17119 if (json.has("dataRequirement")) { 17120 JsonArray array = json.getAsJsonArray("dataRequirement"); 17121 for (int i = 0; i < array.size(); i++) { 17122 res.getDataRequirement().add(parseDataRequirement(array.get(i).getAsJsonObject())); 17123 } 17124 }; 17125 if (json.has("operationDefinition")) 17126 res.setOperationDefinition(parseReference(getJObject(json, "operationDefinition"))); 17127 } 17128 17129 protected Slot parseSlot(JsonObject json) throws IOException, FHIRFormatError { 17130 Slot res = new Slot(); 17131 parseSlotProperties(json, res); 17132 return res; 17133 } 17134 17135 protected void parseSlotProperties(JsonObject json, Slot res) throws IOException, FHIRFormatError { 17136 parseDomainResourceProperties(json, res); 17137 if (json.has("identifier")) { 17138 JsonArray array = json.getAsJsonArray("identifier"); 17139 for (int i = 0; i < array.size(); i++) { 17140 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 17141 } 17142 }; 17143 if (json.has("serviceCategory")) 17144 res.setServiceCategory(parseCodeableConcept(getJObject(json, "serviceCategory"))); 17145 if (json.has("serviceType")) { 17146 JsonArray array = json.getAsJsonArray("serviceType"); 17147 for (int i = 0; i < array.size(); i++) { 17148 res.getServiceType().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 17149 } 17150 }; 17151 if (json.has("specialty")) { 17152 JsonArray array = json.getAsJsonArray("specialty"); 17153 for (int i = 0; i < array.size(); i++) { 17154 res.getSpecialty().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 17155 } 17156 }; 17157 if (json.has("appointmentType")) 17158 res.setAppointmentType(parseCodeableConcept(getJObject(json, "appointmentType"))); 17159 if (json.has("schedule")) 17160 res.setSchedule(parseReference(getJObject(json, "schedule"))); 17161 if (json.has("status")) 17162 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Slot.SlotStatus.NULL, new Slot.SlotStatusEnumFactory())); 17163 if (json.has("_status")) 17164 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 17165 if (json.has("start")) 17166 res.setStartElement(parseInstant(json.get("start").getAsString())); 17167 if (json.has("_start")) 17168 parseElementProperties(getJObject(json, "_start"), res.getStartElement()); 17169 if (json.has("end")) 17170 res.setEndElement(parseInstant(json.get("end").getAsString())); 17171 if (json.has("_end")) 17172 parseElementProperties(getJObject(json, "_end"), res.getEndElement()); 17173 if (json.has("overbooked")) 17174 res.setOverbookedElement(parseBoolean(json.get("overbooked").getAsBoolean())); 17175 if (json.has("_overbooked")) 17176 parseElementProperties(getJObject(json, "_overbooked"), res.getOverbookedElement()); 17177 if (json.has("comment")) 17178 res.setCommentElement(parseString(json.get("comment").getAsString())); 17179 if (json.has("_comment")) 17180 parseElementProperties(getJObject(json, "_comment"), res.getCommentElement()); 17181 } 17182 17183 protected Specimen parseSpecimen(JsonObject json) throws IOException, FHIRFormatError { 17184 Specimen res = new Specimen(); 17185 parseSpecimenProperties(json, res); 17186 return res; 17187 } 17188 17189 protected void parseSpecimenProperties(JsonObject json, Specimen res) throws IOException, FHIRFormatError { 17190 parseDomainResourceProperties(json, res); 17191 if (json.has("identifier")) { 17192 JsonArray array = json.getAsJsonArray("identifier"); 17193 for (int i = 0; i < array.size(); i++) { 17194 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 17195 } 17196 }; 17197 if (json.has("accessionIdentifier")) 17198 res.setAccessionIdentifier(parseIdentifier(getJObject(json, "accessionIdentifier"))); 17199 if (json.has("status")) 17200 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Specimen.SpecimenStatus.NULL, new Specimen.SpecimenStatusEnumFactory())); 17201 if (json.has("_status")) 17202 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 17203 if (json.has("type")) 17204 res.setType(parseCodeableConcept(getJObject(json, "type"))); 17205 if (json.has("subject")) 17206 res.setSubject(parseReference(getJObject(json, "subject"))); 17207 if (json.has("receivedTime")) 17208 res.setReceivedTimeElement(parseDateTime(json.get("receivedTime").getAsString())); 17209 if (json.has("_receivedTime")) 17210 parseElementProperties(getJObject(json, "_receivedTime"), res.getReceivedTimeElement()); 17211 if (json.has("parent")) { 17212 JsonArray array = json.getAsJsonArray("parent"); 17213 for (int i = 0; i < array.size(); i++) { 17214 res.getParent().add(parseReference(array.get(i).getAsJsonObject())); 17215 } 17216 }; 17217 if (json.has("request")) { 17218 JsonArray array = json.getAsJsonArray("request"); 17219 for (int i = 0; i < array.size(); i++) { 17220 res.getRequest().add(parseReference(array.get(i).getAsJsonObject())); 17221 } 17222 }; 17223 if (json.has("collection")) 17224 res.setCollection(parseSpecimenSpecimenCollectionComponent(getJObject(json, "collection"), res)); 17225 if (json.has("processing")) { 17226 JsonArray array = json.getAsJsonArray("processing"); 17227 for (int i = 0; i < array.size(); i++) { 17228 res.getProcessing().add(parseSpecimenSpecimenProcessingComponent(array.get(i).getAsJsonObject(), res)); 17229 } 17230 }; 17231 if (json.has("container")) { 17232 JsonArray array = json.getAsJsonArray("container"); 17233 for (int i = 0; i < array.size(); i++) { 17234 res.getContainer().add(parseSpecimenSpecimenContainerComponent(array.get(i).getAsJsonObject(), res)); 17235 } 17236 }; 17237 if (json.has("note")) { 17238 JsonArray array = json.getAsJsonArray("note"); 17239 for (int i = 0; i < array.size(); i++) { 17240 res.getNote().add(parseAnnotation(array.get(i).getAsJsonObject())); 17241 } 17242 }; 17243 } 17244 17245 protected Specimen.SpecimenCollectionComponent parseSpecimenSpecimenCollectionComponent(JsonObject json, Specimen owner) throws IOException, FHIRFormatError { 17246 Specimen.SpecimenCollectionComponent res = new Specimen.SpecimenCollectionComponent(); 17247 parseSpecimenSpecimenCollectionComponentProperties(json, owner, res); 17248 return res; 17249 } 17250 17251 protected void parseSpecimenSpecimenCollectionComponentProperties(JsonObject json, Specimen owner, Specimen.SpecimenCollectionComponent res) throws IOException, FHIRFormatError { 17252 parseBackboneProperties(json, res); 17253 if (json.has("collector")) 17254 res.setCollector(parseReference(getJObject(json, "collector"))); 17255 Type collected = parseType("collected", json); 17256 if (collected != null) 17257 res.setCollected(collected); 17258 if (json.has("quantity")) 17259 res.setQuantity(parseSimpleQuantity(getJObject(json, "quantity"))); 17260 if (json.has("method")) 17261 res.setMethod(parseCodeableConcept(getJObject(json, "method"))); 17262 if (json.has("bodySite")) 17263 res.setBodySite(parseCodeableConcept(getJObject(json, "bodySite"))); 17264 } 17265 17266 protected Specimen.SpecimenProcessingComponent parseSpecimenSpecimenProcessingComponent(JsonObject json, Specimen owner) throws IOException, FHIRFormatError { 17267 Specimen.SpecimenProcessingComponent res = new Specimen.SpecimenProcessingComponent(); 17268 parseSpecimenSpecimenProcessingComponentProperties(json, owner, res); 17269 return res; 17270 } 17271 17272 protected void parseSpecimenSpecimenProcessingComponentProperties(JsonObject json, Specimen owner, Specimen.SpecimenProcessingComponent res) throws IOException, FHIRFormatError { 17273 parseBackboneProperties(json, res); 17274 if (json.has("description")) 17275 res.setDescriptionElement(parseString(json.get("description").getAsString())); 17276 if (json.has("_description")) 17277 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 17278 if (json.has("procedure")) 17279 res.setProcedure(parseCodeableConcept(getJObject(json, "procedure"))); 17280 if (json.has("additive")) { 17281 JsonArray array = json.getAsJsonArray("additive"); 17282 for (int i = 0; i < array.size(); i++) { 17283 res.getAdditive().add(parseReference(array.get(i).getAsJsonObject())); 17284 } 17285 }; 17286 Type time = parseType("time", json); 17287 if (time != null) 17288 res.setTime(time); 17289 } 17290 17291 protected Specimen.SpecimenContainerComponent parseSpecimenSpecimenContainerComponent(JsonObject json, Specimen owner) throws IOException, FHIRFormatError { 17292 Specimen.SpecimenContainerComponent res = new Specimen.SpecimenContainerComponent(); 17293 parseSpecimenSpecimenContainerComponentProperties(json, owner, res); 17294 return res; 17295 } 17296 17297 protected void parseSpecimenSpecimenContainerComponentProperties(JsonObject json, Specimen owner, Specimen.SpecimenContainerComponent res) throws IOException, FHIRFormatError { 17298 parseBackboneProperties(json, res); 17299 if (json.has("identifier")) { 17300 JsonArray array = json.getAsJsonArray("identifier"); 17301 for (int i = 0; i < array.size(); i++) { 17302 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 17303 } 17304 }; 17305 if (json.has("description")) 17306 res.setDescriptionElement(parseString(json.get("description").getAsString())); 17307 if (json.has("_description")) 17308 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 17309 if (json.has("type")) 17310 res.setType(parseCodeableConcept(getJObject(json, "type"))); 17311 if (json.has("capacity")) 17312 res.setCapacity(parseSimpleQuantity(getJObject(json, "capacity"))); 17313 if (json.has("specimenQuantity")) 17314 res.setSpecimenQuantity(parseSimpleQuantity(getJObject(json, "specimenQuantity"))); 17315 Type additive = parseType("additive", json); 17316 if (additive != null) 17317 res.setAdditive(additive); 17318 } 17319 17320 protected StructureDefinition parseStructureDefinition(JsonObject json) throws IOException, FHIRFormatError { 17321 StructureDefinition res = new StructureDefinition(); 17322 parseStructureDefinitionProperties(json, res); 17323 return res; 17324 } 17325 17326 protected void parseStructureDefinitionProperties(JsonObject json, StructureDefinition res) throws IOException, FHIRFormatError { 17327 parseDomainResourceProperties(json, res); 17328 if (json.has("url")) 17329 res.setUrlElement(parseUri(json.get("url").getAsString())); 17330 if (json.has("_url")) 17331 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 17332 if (json.has("identifier")) { 17333 JsonArray array = json.getAsJsonArray("identifier"); 17334 for (int i = 0; i < array.size(); i++) { 17335 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 17336 } 17337 }; 17338 if (json.has("version")) 17339 res.setVersionElement(parseString(json.get("version").getAsString())); 17340 if (json.has("_version")) 17341 parseElementProperties(getJObject(json, "_version"), res.getVersionElement()); 17342 if (json.has("name")) 17343 res.setNameElement(parseString(json.get("name").getAsString())); 17344 if (json.has("_name")) 17345 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 17346 if (json.has("title")) 17347 res.setTitleElement(parseString(json.get("title").getAsString())); 17348 if (json.has("_title")) 17349 parseElementProperties(getJObject(json, "_title"), res.getTitleElement()); 17350 if (json.has("status")) 17351 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Enumerations.PublicationStatus.NULL, new Enumerations.PublicationStatusEnumFactory())); 17352 if (json.has("_status")) 17353 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 17354 if (json.has("experimental")) 17355 res.setExperimentalElement(parseBoolean(json.get("experimental").getAsBoolean())); 17356 if (json.has("_experimental")) 17357 parseElementProperties(getJObject(json, "_experimental"), res.getExperimentalElement()); 17358 if (json.has("date")) 17359 res.setDateElement(parseDateTime(json.get("date").getAsString())); 17360 if (json.has("_date")) 17361 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 17362 if (json.has("publisher")) 17363 res.setPublisherElement(parseString(json.get("publisher").getAsString())); 17364 if (json.has("_publisher")) 17365 parseElementProperties(getJObject(json, "_publisher"), res.getPublisherElement()); 17366 if (json.has("contact")) { 17367 JsonArray array = json.getAsJsonArray("contact"); 17368 for (int i = 0; i < array.size(); i++) { 17369 res.getContact().add(parseContactDetail(array.get(i).getAsJsonObject())); 17370 } 17371 }; 17372 if (json.has("description")) 17373 res.setDescriptionElement(parseMarkdown(json.get("description").getAsString())); 17374 if (json.has("_description")) 17375 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 17376 if (json.has("useContext")) { 17377 JsonArray array = json.getAsJsonArray("useContext"); 17378 for (int i = 0; i < array.size(); i++) { 17379 res.getUseContext().add(parseUsageContext(array.get(i).getAsJsonObject())); 17380 } 17381 }; 17382 if (json.has("jurisdiction")) { 17383 JsonArray array = json.getAsJsonArray("jurisdiction"); 17384 for (int i = 0; i < array.size(); i++) { 17385 res.getJurisdiction().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 17386 } 17387 }; 17388 if (json.has("purpose")) 17389 res.setPurposeElement(parseMarkdown(json.get("purpose").getAsString())); 17390 if (json.has("_purpose")) 17391 parseElementProperties(getJObject(json, "_purpose"), res.getPurposeElement()); 17392 if (json.has("copyright")) 17393 res.setCopyrightElement(parseMarkdown(json.get("copyright").getAsString())); 17394 if (json.has("_copyright")) 17395 parseElementProperties(getJObject(json, "_copyright"), res.getCopyrightElement()); 17396 if (json.has("keyword")) { 17397 JsonArray array = json.getAsJsonArray("keyword"); 17398 for (int i = 0; i < array.size(); i++) { 17399 res.getKeyword().add(parseCoding(array.get(i).getAsJsonObject())); 17400 } 17401 }; 17402 if (json.has("fhirVersion")) 17403 res.setFhirVersionElement(parseId(json.get("fhirVersion").getAsString())); 17404 if (json.has("_fhirVersion")) 17405 parseElementProperties(getJObject(json, "_fhirVersion"), res.getFhirVersionElement()); 17406 if (json.has("mapping")) { 17407 JsonArray array = json.getAsJsonArray("mapping"); 17408 for (int i = 0; i < array.size(); i++) { 17409 res.getMapping().add(parseStructureDefinitionStructureDefinitionMappingComponent(array.get(i).getAsJsonObject(), res)); 17410 } 17411 }; 17412 if (json.has("kind")) 17413 res.setKindElement(parseEnumeration(json.get("kind").getAsString(), StructureDefinition.StructureDefinitionKind.NULL, new StructureDefinition.StructureDefinitionKindEnumFactory())); 17414 if (json.has("_kind")) 17415 parseElementProperties(getJObject(json, "_kind"), res.getKindElement()); 17416 if (json.has("abstract")) 17417 res.setAbstractElement(parseBoolean(json.get("abstract").getAsBoolean())); 17418 if (json.has("_abstract")) 17419 parseElementProperties(getJObject(json, "_abstract"), res.getAbstractElement()); 17420 if (json.has("contextType")) 17421 res.setContextTypeElement(parseEnumeration(json.get("contextType").getAsString(), StructureDefinition.ExtensionContext.NULL, new StructureDefinition.ExtensionContextEnumFactory())); 17422 if (json.has("_contextType")) 17423 parseElementProperties(getJObject(json, "_contextType"), res.getContextTypeElement()); 17424 if (json.has("context")) { 17425 JsonArray array = json.getAsJsonArray("context"); 17426 for (int i = 0; i < array.size(); i++) { 17427 if (array.get(i).isJsonNull()) { 17428 res.getContext().add(new StringType()); 17429 } else { 17430 res.getContext().add(parseString(array.get(i).getAsString())); 17431 } 17432 } 17433 }; 17434 if (json.has("_context")) { 17435 JsonArray array = json.getAsJsonArray("_context"); 17436 for (int i = 0; i < array.size(); i++) { 17437 if (i == res.getContext().size()) 17438 res.getContext().add(parseString(null)); 17439 if (array.get(i) instanceof JsonObject) 17440 parseElementProperties(array.get(i).getAsJsonObject(), res.getContext().get(i)); 17441 } 17442 }; 17443 if (json.has("contextInvariant")) { 17444 JsonArray array = json.getAsJsonArray("contextInvariant"); 17445 for (int i = 0; i < array.size(); i++) { 17446 if (array.get(i).isJsonNull()) { 17447 res.getContextInvariant().add(new StringType()); 17448 } else { 17449 res.getContextInvariant().add(parseString(array.get(i).getAsString())); 17450 } 17451 } 17452 }; 17453 if (json.has("_contextInvariant")) { 17454 JsonArray array = json.getAsJsonArray("_contextInvariant"); 17455 for (int i = 0; i < array.size(); i++) { 17456 if (i == res.getContextInvariant().size()) 17457 res.getContextInvariant().add(parseString(null)); 17458 if (array.get(i) instanceof JsonObject) 17459 parseElementProperties(array.get(i).getAsJsonObject(), res.getContextInvariant().get(i)); 17460 } 17461 }; 17462 if (json.has("type")) 17463 res.setTypeElement(parseCode(json.get("type").getAsString())); 17464 if (json.has("_type")) 17465 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 17466 if (json.has("baseDefinition")) 17467 res.setBaseDefinitionElement(parseUri(json.get("baseDefinition").getAsString())); 17468 if (json.has("_baseDefinition")) 17469 parseElementProperties(getJObject(json, "_baseDefinition"), res.getBaseDefinitionElement()); 17470 if (json.has("derivation")) 17471 res.setDerivationElement(parseEnumeration(json.get("derivation").getAsString(), StructureDefinition.TypeDerivationRule.NULL, new StructureDefinition.TypeDerivationRuleEnumFactory())); 17472 if (json.has("_derivation")) 17473 parseElementProperties(getJObject(json, "_derivation"), res.getDerivationElement()); 17474 if (json.has("snapshot")) 17475 res.setSnapshot(parseStructureDefinitionStructureDefinitionSnapshotComponent(getJObject(json, "snapshot"), res)); 17476 if (json.has("differential")) 17477 res.setDifferential(parseStructureDefinitionStructureDefinitionDifferentialComponent(getJObject(json, "differential"), res)); 17478 } 17479 17480 protected StructureDefinition.StructureDefinitionMappingComponent parseStructureDefinitionStructureDefinitionMappingComponent(JsonObject json, StructureDefinition owner) throws IOException, FHIRFormatError { 17481 StructureDefinition.StructureDefinitionMappingComponent res = new StructureDefinition.StructureDefinitionMappingComponent(); 17482 parseStructureDefinitionStructureDefinitionMappingComponentProperties(json, owner, res); 17483 return res; 17484 } 17485 17486 protected void parseStructureDefinitionStructureDefinitionMappingComponentProperties(JsonObject json, StructureDefinition owner, StructureDefinition.StructureDefinitionMappingComponent res) throws IOException, FHIRFormatError { 17487 parseBackboneProperties(json, res); 17488 if (json.has("identity")) 17489 res.setIdentityElement(parseId(json.get("identity").getAsString())); 17490 if (json.has("_identity")) 17491 parseElementProperties(getJObject(json, "_identity"), res.getIdentityElement()); 17492 if (json.has("uri")) 17493 res.setUriElement(parseUri(json.get("uri").getAsString())); 17494 if (json.has("_uri")) 17495 parseElementProperties(getJObject(json, "_uri"), res.getUriElement()); 17496 if (json.has("name")) 17497 res.setNameElement(parseString(json.get("name").getAsString())); 17498 if (json.has("_name")) 17499 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 17500 if (json.has("comment")) 17501 res.setCommentElement(parseString(json.get("comment").getAsString())); 17502 if (json.has("_comment")) 17503 parseElementProperties(getJObject(json, "_comment"), res.getCommentElement()); 17504 } 17505 17506 protected StructureDefinition.StructureDefinitionSnapshotComponent parseStructureDefinitionStructureDefinitionSnapshotComponent(JsonObject json, StructureDefinition owner) throws IOException, FHIRFormatError { 17507 StructureDefinition.StructureDefinitionSnapshotComponent res = new StructureDefinition.StructureDefinitionSnapshotComponent(); 17508 parseStructureDefinitionStructureDefinitionSnapshotComponentProperties(json, owner, res); 17509 return res; 17510 } 17511 17512 protected void parseStructureDefinitionStructureDefinitionSnapshotComponentProperties(JsonObject json, StructureDefinition owner, StructureDefinition.StructureDefinitionSnapshotComponent res) throws IOException, FHIRFormatError { 17513 parseBackboneProperties(json, res); 17514 if (json.has("element")) { 17515 JsonArray array = json.getAsJsonArray("element"); 17516 for (int i = 0; i < array.size(); i++) { 17517 res.getElement().add(parseElementDefinition(array.get(i).getAsJsonObject())); 17518 } 17519 }; 17520 } 17521 17522 protected StructureDefinition.StructureDefinitionDifferentialComponent parseStructureDefinitionStructureDefinitionDifferentialComponent(JsonObject json, StructureDefinition owner) throws IOException, FHIRFormatError { 17523 StructureDefinition.StructureDefinitionDifferentialComponent res = new StructureDefinition.StructureDefinitionDifferentialComponent(); 17524 parseStructureDefinitionStructureDefinitionDifferentialComponentProperties(json, owner, res); 17525 return res; 17526 } 17527 17528 protected void parseStructureDefinitionStructureDefinitionDifferentialComponentProperties(JsonObject json, StructureDefinition owner, StructureDefinition.StructureDefinitionDifferentialComponent res) throws IOException, FHIRFormatError { 17529 parseBackboneProperties(json, res); 17530 if (json.has("element")) { 17531 JsonArray array = json.getAsJsonArray("element"); 17532 for (int i = 0; i < array.size(); i++) { 17533 res.getElement().add(parseElementDefinition(array.get(i).getAsJsonObject())); 17534 } 17535 }; 17536 } 17537 17538 protected StructureMap parseStructureMap(JsonObject json) throws IOException, FHIRFormatError { 17539 StructureMap res = new StructureMap(); 17540 parseStructureMapProperties(json, res); 17541 return res; 17542 } 17543 17544 protected void parseStructureMapProperties(JsonObject json, StructureMap res) throws IOException, FHIRFormatError { 17545 parseDomainResourceProperties(json, res); 17546 if (json.has("url")) 17547 res.setUrlElement(parseUri(json.get("url").getAsString())); 17548 if (json.has("_url")) 17549 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 17550 if (json.has("identifier")) { 17551 JsonArray array = json.getAsJsonArray("identifier"); 17552 for (int i = 0; i < array.size(); i++) { 17553 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 17554 } 17555 }; 17556 if (json.has("version")) 17557 res.setVersionElement(parseString(json.get("version").getAsString())); 17558 if (json.has("_version")) 17559 parseElementProperties(getJObject(json, "_version"), res.getVersionElement()); 17560 if (json.has("name")) 17561 res.setNameElement(parseString(json.get("name").getAsString())); 17562 if (json.has("_name")) 17563 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 17564 if (json.has("title")) 17565 res.setTitleElement(parseString(json.get("title").getAsString())); 17566 if (json.has("_title")) 17567 parseElementProperties(getJObject(json, "_title"), res.getTitleElement()); 17568 if (json.has("status")) 17569 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Enumerations.PublicationStatus.NULL, new Enumerations.PublicationStatusEnumFactory())); 17570 if (json.has("_status")) 17571 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 17572 if (json.has("experimental")) 17573 res.setExperimentalElement(parseBoolean(json.get("experimental").getAsBoolean())); 17574 if (json.has("_experimental")) 17575 parseElementProperties(getJObject(json, "_experimental"), res.getExperimentalElement()); 17576 if (json.has("date")) 17577 res.setDateElement(parseDateTime(json.get("date").getAsString())); 17578 if (json.has("_date")) 17579 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 17580 if (json.has("publisher")) 17581 res.setPublisherElement(parseString(json.get("publisher").getAsString())); 17582 if (json.has("_publisher")) 17583 parseElementProperties(getJObject(json, "_publisher"), res.getPublisherElement()); 17584 if (json.has("contact")) { 17585 JsonArray array = json.getAsJsonArray("contact"); 17586 for (int i = 0; i < array.size(); i++) { 17587 res.getContact().add(parseContactDetail(array.get(i).getAsJsonObject())); 17588 } 17589 }; 17590 if (json.has("description")) 17591 res.setDescriptionElement(parseMarkdown(json.get("description").getAsString())); 17592 if (json.has("_description")) 17593 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 17594 if (json.has("useContext")) { 17595 JsonArray array = json.getAsJsonArray("useContext"); 17596 for (int i = 0; i < array.size(); i++) { 17597 res.getUseContext().add(parseUsageContext(array.get(i).getAsJsonObject())); 17598 } 17599 }; 17600 if (json.has("jurisdiction")) { 17601 JsonArray array = json.getAsJsonArray("jurisdiction"); 17602 for (int i = 0; i < array.size(); i++) { 17603 res.getJurisdiction().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 17604 } 17605 }; 17606 if (json.has("purpose")) 17607 res.setPurposeElement(parseMarkdown(json.get("purpose").getAsString())); 17608 if (json.has("_purpose")) 17609 parseElementProperties(getJObject(json, "_purpose"), res.getPurposeElement()); 17610 if (json.has("copyright")) 17611 res.setCopyrightElement(parseMarkdown(json.get("copyright").getAsString())); 17612 if (json.has("_copyright")) 17613 parseElementProperties(getJObject(json, "_copyright"), res.getCopyrightElement()); 17614 if (json.has("structure")) { 17615 JsonArray array = json.getAsJsonArray("structure"); 17616 for (int i = 0; i < array.size(); i++) { 17617 res.getStructure().add(parseStructureMapStructureMapStructureComponent(array.get(i).getAsJsonObject(), res)); 17618 } 17619 }; 17620 if (json.has("import")) { 17621 JsonArray array = json.getAsJsonArray("import"); 17622 for (int i = 0; i < array.size(); i++) { 17623 if (array.get(i).isJsonNull()) { 17624 res.getImport().add(new UriType()); 17625 } else { 17626 res.getImport().add(parseUri(array.get(i).getAsString())); 17627 } 17628 } 17629 }; 17630 if (json.has("_import")) { 17631 JsonArray array = json.getAsJsonArray("_import"); 17632 for (int i = 0; i < array.size(); i++) { 17633 if (i == res.getImport().size()) 17634 res.getImport().add(parseUri(null)); 17635 if (array.get(i) instanceof JsonObject) 17636 parseElementProperties(array.get(i).getAsJsonObject(), res.getImport().get(i)); 17637 } 17638 }; 17639 if (json.has("group")) { 17640 JsonArray array = json.getAsJsonArray("group"); 17641 for (int i = 0; i < array.size(); i++) { 17642 res.getGroup().add(parseStructureMapStructureMapGroupComponent(array.get(i).getAsJsonObject(), res)); 17643 } 17644 }; 17645 } 17646 17647 protected StructureMap.StructureMapStructureComponent parseStructureMapStructureMapStructureComponent(JsonObject json, StructureMap owner) throws IOException, FHIRFormatError { 17648 StructureMap.StructureMapStructureComponent res = new StructureMap.StructureMapStructureComponent(); 17649 parseStructureMapStructureMapStructureComponentProperties(json, owner, res); 17650 return res; 17651 } 17652 17653 protected void parseStructureMapStructureMapStructureComponentProperties(JsonObject json, StructureMap owner, StructureMap.StructureMapStructureComponent res) throws IOException, FHIRFormatError { 17654 parseBackboneProperties(json, res); 17655 if (json.has("url")) 17656 res.setUrlElement(parseUri(json.get("url").getAsString())); 17657 if (json.has("_url")) 17658 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 17659 if (json.has("mode")) 17660 res.setModeElement(parseEnumeration(json.get("mode").getAsString(), StructureMap.StructureMapModelMode.NULL, new StructureMap.StructureMapModelModeEnumFactory())); 17661 if (json.has("_mode")) 17662 parseElementProperties(getJObject(json, "_mode"), res.getModeElement()); 17663 if (json.has("alias")) 17664 res.setAliasElement(parseString(json.get("alias").getAsString())); 17665 if (json.has("_alias")) 17666 parseElementProperties(getJObject(json, "_alias"), res.getAliasElement()); 17667 if (json.has("documentation")) 17668 res.setDocumentationElement(parseString(json.get("documentation").getAsString())); 17669 if (json.has("_documentation")) 17670 parseElementProperties(getJObject(json, "_documentation"), res.getDocumentationElement()); 17671 } 17672 17673 protected StructureMap.StructureMapGroupComponent parseStructureMapStructureMapGroupComponent(JsonObject json, StructureMap owner) throws IOException, FHIRFormatError { 17674 StructureMap.StructureMapGroupComponent res = new StructureMap.StructureMapGroupComponent(); 17675 parseStructureMapStructureMapGroupComponentProperties(json, owner, res); 17676 return res; 17677 } 17678 17679 protected void parseStructureMapStructureMapGroupComponentProperties(JsonObject json, StructureMap owner, StructureMap.StructureMapGroupComponent res) throws IOException, FHIRFormatError { 17680 parseBackboneProperties(json, res); 17681 if (json.has("name")) 17682 res.setNameElement(parseId(json.get("name").getAsString())); 17683 if (json.has("_name")) 17684 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 17685 if (json.has("extends")) 17686 res.setExtendsElement(parseId(json.get("extends").getAsString())); 17687 if (json.has("_extends")) 17688 parseElementProperties(getJObject(json, "_extends"), res.getExtendsElement()); 17689 if (json.has("typeMode")) 17690 res.setTypeModeElement(parseEnumeration(json.get("typeMode").getAsString(), StructureMap.StructureMapGroupTypeMode.NULL, new StructureMap.StructureMapGroupTypeModeEnumFactory())); 17691 if (json.has("_typeMode")) 17692 parseElementProperties(getJObject(json, "_typeMode"), res.getTypeModeElement()); 17693 if (json.has("documentation")) 17694 res.setDocumentationElement(parseString(json.get("documentation").getAsString())); 17695 if (json.has("_documentation")) 17696 parseElementProperties(getJObject(json, "_documentation"), res.getDocumentationElement()); 17697 if (json.has("input")) { 17698 JsonArray array = json.getAsJsonArray("input"); 17699 for (int i = 0; i < array.size(); i++) { 17700 res.getInput().add(parseStructureMapStructureMapGroupInputComponent(array.get(i).getAsJsonObject(), owner)); 17701 } 17702 }; 17703 if (json.has("rule")) { 17704 JsonArray array = json.getAsJsonArray("rule"); 17705 for (int i = 0; i < array.size(); i++) { 17706 res.getRule().add(parseStructureMapStructureMapGroupRuleComponent(array.get(i).getAsJsonObject(), owner)); 17707 } 17708 }; 17709 } 17710 17711 protected StructureMap.StructureMapGroupInputComponent parseStructureMapStructureMapGroupInputComponent(JsonObject json, StructureMap owner) throws IOException, FHIRFormatError { 17712 StructureMap.StructureMapGroupInputComponent res = new StructureMap.StructureMapGroupInputComponent(); 17713 parseStructureMapStructureMapGroupInputComponentProperties(json, owner, res); 17714 return res; 17715 } 17716 17717 protected void parseStructureMapStructureMapGroupInputComponentProperties(JsonObject json, StructureMap owner, StructureMap.StructureMapGroupInputComponent res) throws IOException, FHIRFormatError { 17718 parseBackboneProperties(json, res); 17719 if (json.has("name")) 17720 res.setNameElement(parseId(json.get("name").getAsString())); 17721 if (json.has("_name")) 17722 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 17723 if (json.has("type")) 17724 res.setTypeElement(parseString(json.get("type").getAsString())); 17725 if (json.has("_type")) 17726 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 17727 if (json.has("mode")) 17728 res.setModeElement(parseEnumeration(json.get("mode").getAsString(), StructureMap.StructureMapInputMode.NULL, new StructureMap.StructureMapInputModeEnumFactory())); 17729 if (json.has("_mode")) 17730 parseElementProperties(getJObject(json, "_mode"), res.getModeElement()); 17731 if (json.has("documentation")) 17732 res.setDocumentationElement(parseString(json.get("documentation").getAsString())); 17733 if (json.has("_documentation")) 17734 parseElementProperties(getJObject(json, "_documentation"), res.getDocumentationElement()); 17735 } 17736 17737 protected StructureMap.StructureMapGroupRuleComponent parseStructureMapStructureMapGroupRuleComponent(JsonObject json, StructureMap owner) throws IOException, FHIRFormatError { 17738 StructureMap.StructureMapGroupRuleComponent res = new StructureMap.StructureMapGroupRuleComponent(); 17739 parseStructureMapStructureMapGroupRuleComponentProperties(json, owner, res); 17740 return res; 17741 } 17742 17743 protected void parseStructureMapStructureMapGroupRuleComponentProperties(JsonObject json, StructureMap owner, StructureMap.StructureMapGroupRuleComponent res) throws IOException, FHIRFormatError { 17744 parseBackboneProperties(json, res); 17745 if (json.has("name")) 17746 res.setNameElement(parseId(json.get("name").getAsString())); 17747 if (json.has("_name")) 17748 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 17749 if (json.has("source")) { 17750 JsonArray array = json.getAsJsonArray("source"); 17751 for (int i = 0; i < array.size(); i++) { 17752 res.getSource().add(parseStructureMapStructureMapGroupRuleSourceComponent(array.get(i).getAsJsonObject(), owner)); 17753 } 17754 }; 17755 if (json.has("target")) { 17756 JsonArray array = json.getAsJsonArray("target"); 17757 for (int i = 0; i < array.size(); i++) { 17758 res.getTarget().add(parseStructureMapStructureMapGroupRuleTargetComponent(array.get(i).getAsJsonObject(), owner)); 17759 } 17760 }; 17761 if (json.has("rule")) { 17762 JsonArray array = json.getAsJsonArray("rule"); 17763 for (int i = 0; i < array.size(); i++) { 17764 res.getRule().add(parseStructureMapStructureMapGroupRuleComponent(array.get(i).getAsJsonObject(), owner)); 17765 } 17766 }; 17767 if (json.has("dependent")) { 17768 JsonArray array = json.getAsJsonArray("dependent"); 17769 for (int i = 0; i < array.size(); i++) { 17770 res.getDependent().add(parseStructureMapStructureMapGroupRuleDependentComponent(array.get(i).getAsJsonObject(), owner)); 17771 } 17772 }; 17773 if (json.has("documentation")) 17774 res.setDocumentationElement(parseString(json.get("documentation").getAsString())); 17775 if (json.has("_documentation")) 17776 parseElementProperties(getJObject(json, "_documentation"), res.getDocumentationElement()); 17777 } 17778 17779 protected StructureMap.StructureMapGroupRuleSourceComponent parseStructureMapStructureMapGroupRuleSourceComponent(JsonObject json, StructureMap owner) throws IOException, FHIRFormatError { 17780 StructureMap.StructureMapGroupRuleSourceComponent res = new StructureMap.StructureMapGroupRuleSourceComponent(); 17781 parseStructureMapStructureMapGroupRuleSourceComponentProperties(json, owner, res); 17782 return res; 17783 } 17784 17785 protected void parseStructureMapStructureMapGroupRuleSourceComponentProperties(JsonObject json, StructureMap owner, StructureMap.StructureMapGroupRuleSourceComponent res) throws IOException, FHIRFormatError { 17786 parseBackboneProperties(json, res); 17787 if (json.has("context")) 17788 res.setContextElement(parseId(json.get("context").getAsString())); 17789 if (json.has("_context")) 17790 parseElementProperties(getJObject(json, "_context"), res.getContextElement()); 17791 if (json.has("min")) 17792 res.setMinElement(parseInteger(json.get("min").getAsLong())); 17793 if (json.has("_min")) 17794 parseElementProperties(getJObject(json, "_min"), res.getMinElement()); 17795 if (json.has("max")) 17796 res.setMaxElement(parseString(json.get("max").getAsString())); 17797 if (json.has("_max")) 17798 parseElementProperties(getJObject(json, "_max"), res.getMaxElement()); 17799 if (json.has("type")) 17800 res.setTypeElement(parseString(json.get("type").getAsString())); 17801 if (json.has("_type")) 17802 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 17803 Type defaultValue = parseType("defaultValue", json); 17804 if (defaultValue != null) 17805 res.setDefaultValue(defaultValue); 17806 if (json.has("element")) 17807 res.setElementElement(parseString(json.get("element").getAsString())); 17808 if (json.has("_element")) 17809 parseElementProperties(getJObject(json, "_element"), res.getElementElement()); 17810 if (json.has("listMode")) 17811 res.setListModeElement(parseEnumeration(json.get("listMode").getAsString(), StructureMap.StructureMapSourceListMode.NULL, new StructureMap.StructureMapSourceListModeEnumFactory())); 17812 if (json.has("_listMode")) 17813 parseElementProperties(getJObject(json, "_listMode"), res.getListModeElement()); 17814 if (json.has("variable")) 17815 res.setVariableElement(parseId(json.get("variable").getAsString())); 17816 if (json.has("_variable")) 17817 parseElementProperties(getJObject(json, "_variable"), res.getVariableElement()); 17818 if (json.has("condition")) 17819 res.setConditionElement(parseString(json.get("condition").getAsString())); 17820 if (json.has("_condition")) 17821 parseElementProperties(getJObject(json, "_condition"), res.getConditionElement()); 17822 if (json.has("check")) 17823 res.setCheckElement(parseString(json.get("check").getAsString())); 17824 if (json.has("_check")) 17825 parseElementProperties(getJObject(json, "_check"), res.getCheckElement()); 17826 } 17827 17828 protected StructureMap.StructureMapGroupRuleTargetComponent parseStructureMapStructureMapGroupRuleTargetComponent(JsonObject json, StructureMap owner) throws IOException, FHIRFormatError { 17829 StructureMap.StructureMapGroupRuleTargetComponent res = new StructureMap.StructureMapGroupRuleTargetComponent(); 17830 parseStructureMapStructureMapGroupRuleTargetComponentProperties(json, owner, res); 17831 return res; 17832 } 17833 17834 protected void parseStructureMapStructureMapGroupRuleTargetComponentProperties(JsonObject json, StructureMap owner, StructureMap.StructureMapGroupRuleTargetComponent res) throws IOException, FHIRFormatError { 17835 parseBackboneProperties(json, res); 17836 if (json.has("context")) 17837 res.setContextElement(parseId(json.get("context").getAsString())); 17838 if (json.has("_context")) 17839 parseElementProperties(getJObject(json, "_context"), res.getContextElement()); 17840 if (json.has("contextType")) 17841 res.setContextTypeElement(parseEnumeration(json.get("contextType").getAsString(), StructureMap.StructureMapContextType.NULL, new StructureMap.StructureMapContextTypeEnumFactory())); 17842 if (json.has("_contextType")) 17843 parseElementProperties(getJObject(json, "_contextType"), res.getContextTypeElement()); 17844 if (json.has("element")) 17845 res.setElementElement(parseString(json.get("element").getAsString())); 17846 if (json.has("_element")) 17847 parseElementProperties(getJObject(json, "_element"), res.getElementElement()); 17848 if (json.has("variable")) 17849 res.setVariableElement(parseId(json.get("variable").getAsString())); 17850 if (json.has("_variable")) 17851 parseElementProperties(getJObject(json, "_variable"), res.getVariableElement()); 17852 if (json.has("listMode")) { 17853 JsonArray array = json.getAsJsonArray("listMode"); 17854 for (int i = 0; i < array.size(); i++) { 17855 if (array.get(i).isJsonNull()) { 17856 res.getListMode().add(new Enumeration<StructureMap.StructureMapTargetListMode>()); 17857 } else { 17858 res.getListMode().add(parseEnumeration(array.get(i).getAsString(), StructureMap.StructureMapTargetListMode.NULL, new StructureMap.StructureMapTargetListModeEnumFactory())); 17859 } 17860 } 17861 }; 17862 if (json.has("_listMode")) { 17863 JsonArray array = json.getAsJsonArray("_listMode"); 17864 for (int i = 0; i < array.size(); i++) { 17865 if (i == res.getListMode().size()) 17866 res.getListMode().add(parseEnumeration(null, StructureMap.StructureMapTargetListMode.NULL, new StructureMap.StructureMapTargetListModeEnumFactory())); 17867 if (array.get(i) instanceof JsonObject) 17868 parseElementProperties(array.get(i).getAsJsonObject(), res.getListMode().get(i)); 17869 } 17870 }; 17871 if (json.has("listRuleId")) 17872 res.setListRuleIdElement(parseId(json.get("listRuleId").getAsString())); 17873 if (json.has("_listRuleId")) 17874 parseElementProperties(getJObject(json, "_listRuleId"), res.getListRuleIdElement()); 17875 if (json.has("transform")) 17876 res.setTransformElement(parseEnumeration(json.get("transform").getAsString(), StructureMap.StructureMapTransform.NULL, new StructureMap.StructureMapTransformEnumFactory())); 17877 if (json.has("_transform")) 17878 parseElementProperties(getJObject(json, "_transform"), res.getTransformElement()); 17879 if (json.has("parameter")) { 17880 JsonArray array = json.getAsJsonArray("parameter"); 17881 for (int i = 0; i < array.size(); i++) { 17882 res.getParameter().add(parseStructureMapStructureMapGroupRuleTargetParameterComponent(array.get(i).getAsJsonObject(), owner)); 17883 } 17884 }; 17885 } 17886 17887 protected StructureMap.StructureMapGroupRuleTargetParameterComponent parseStructureMapStructureMapGroupRuleTargetParameterComponent(JsonObject json, StructureMap owner) throws IOException, FHIRFormatError { 17888 StructureMap.StructureMapGroupRuleTargetParameterComponent res = new StructureMap.StructureMapGroupRuleTargetParameterComponent(); 17889 parseStructureMapStructureMapGroupRuleTargetParameterComponentProperties(json, owner, res); 17890 return res; 17891 } 17892 17893 protected void parseStructureMapStructureMapGroupRuleTargetParameterComponentProperties(JsonObject json, StructureMap owner, StructureMap.StructureMapGroupRuleTargetParameterComponent res) throws IOException, FHIRFormatError { 17894 parseBackboneProperties(json, res); 17895 Type value = parseType("value", json); 17896 if (value != null) 17897 res.setValue(value); 17898 } 17899 17900 protected StructureMap.StructureMapGroupRuleDependentComponent parseStructureMapStructureMapGroupRuleDependentComponent(JsonObject json, StructureMap owner) throws IOException, FHIRFormatError { 17901 StructureMap.StructureMapGroupRuleDependentComponent res = new StructureMap.StructureMapGroupRuleDependentComponent(); 17902 parseStructureMapStructureMapGroupRuleDependentComponentProperties(json, owner, res); 17903 return res; 17904 } 17905 17906 protected void parseStructureMapStructureMapGroupRuleDependentComponentProperties(JsonObject json, StructureMap owner, StructureMap.StructureMapGroupRuleDependentComponent res) throws IOException, FHIRFormatError { 17907 parseBackboneProperties(json, res); 17908 if (json.has("name")) 17909 res.setNameElement(parseId(json.get("name").getAsString())); 17910 if (json.has("_name")) 17911 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 17912 if (json.has("variable")) { 17913 JsonArray array = json.getAsJsonArray("variable"); 17914 for (int i = 0; i < array.size(); i++) { 17915 if (array.get(i).isJsonNull()) { 17916 res.getVariable().add(new StringType()); 17917 } else { 17918 res.getVariable().add(parseString(array.get(i).getAsString())); 17919 } 17920 } 17921 }; 17922 if (json.has("_variable")) { 17923 JsonArray array = json.getAsJsonArray("_variable"); 17924 for (int i = 0; i < array.size(); i++) { 17925 if (i == res.getVariable().size()) 17926 res.getVariable().add(parseString(null)); 17927 if (array.get(i) instanceof JsonObject) 17928 parseElementProperties(array.get(i).getAsJsonObject(), res.getVariable().get(i)); 17929 } 17930 }; 17931 } 17932 17933 protected Subscription parseSubscription(JsonObject json) throws IOException, FHIRFormatError { 17934 Subscription res = new Subscription(); 17935 parseSubscriptionProperties(json, res); 17936 return res; 17937 } 17938 17939 protected void parseSubscriptionProperties(JsonObject json, Subscription res) throws IOException, FHIRFormatError { 17940 parseDomainResourceProperties(json, res); 17941 if (json.has("status")) 17942 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Subscription.SubscriptionStatus.NULL, new Subscription.SubscriptionStatusEnumFactory())); 17943 if (json.has("_status")) 17944 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 17945 if (json.has("contact")) { 17946 JsonArray array = json.getAsJsonArray("contact"); 17947 for (int i = 0; i < array.size(); i++) { 17948 res.getContact().add(parseContactPoint(array.get(i).getAsJsonObject())); 17949 } 17950 }; 17951 if (json.has("end")) 17952 res.setEndElement(parseInstant(json.get("end").getAsString())); 17953 if (json.has("_end")) 17954 parseElementProperties(getJObject(json, "_end"), res.getEndElement()); 17955 if (json.has("reason")) 17956 res.setReasonElement(parseString(json.get("reason").getAsString())); 17957 if (json.has("_reason")) 17958 parseElementProperties(getJObject(json, "_reason"), res.getReasonElement()); 17959 if (json.has("criteria")) 17960 res.setCriteriaElement(parseString(json.get("criteria").getAsString())); 17961 if (json.has("_criteria")) 17962 parseElementProperties(getJObject(json, "_criteria"), res.getCriteriaElement()); 17963 if (json.has("error")) 17964 res.setErrorElement(parseString(json.get("error").getAsString())); 17965 if (json.has("_error")) 17966 parseElementProperties(getJObject(json, "_error"), res.getErrorElement()); 17967 if (json.has("channel")) 17968 res.setChannel(parseSubscriptionSubscriptionChannelComponent(getJObject(json, "channel"), res)); 17969 if (json.has("tag")) { 17970 JsonArray array = json.getAsJsonArray("tag"); 17971 for (int i = 0; i < array.size(); i++) { 17972 res.getTag().add(parseCoding(array.get(i).getAsJsonObject())); 17973 } 17974 }; 17975 } 17976 17977 protected Subscription.SubscriptionChannelComponent parseSubscriptionSubscriptionChannelComponent(JsonObject json, Subscription owner) throws IOException, FHIRFormatError { 17978 Subscription.SubscriptionChannelComponent res = new Subscription.SubscriptionChannelComponent(); 17979 parseSubscriptionSubscriptionChannelComponentProperties(json, owner, res); 17980 return res; 17981 } 17982 17983 protected void parseSubscriptionSubscriptionChannelComponentProperties(JsonObject json, Subscription owner, Subscription.SubscriptionChannelComponent res) throws IOException, FHIRFormatError { 17984 parseBackboneProperties(json, res); 17985 if (json.has("type")) 17986 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), Subscription.SubscriptionChannelType.NULL, new Subscription.SubscriptionChannelTypeEnumFactory())); 17987 if (json.has("_type")) 17988 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 17989 if (json.has("endpoint")) 17990 res.setEndpointElement(parseUri(json.get("endpoint").getAsString())); 17991 if (json.has("_endpoint")) 17992 parseElementProperties(getJObject(json, "_endpoint"), res.getEndpointElement()); 17993 if (json.has("payload")) 17994 res.setPayloadElement(parseString(json.get("payload").getAsString())); 17995 if (json.has("_payload")) 17996 parseElementProperties(getJObject(json, "_payload"), res.getPayloadElement()); 17997 if (json.has("header")) { 17998 JsonArray array = json.getAsJsonArray("header"); 17999 for (int i = 0; i < array.size(); i++) { 18000 if (array.get(i).isJsonNull()) { 18001 res.getHeader().add(new StringType()); 18002 } else { 18003 res.getHeader().add(parseString(array.get(i).getAsString())); 18004 } 18005 } 18006 }; 18007 if (json.has("_header")) { 18008 JsonArray array = json.getAsJsonArray("_header"); 18009 for (int i = 0; i < array.size(); i++) { 18010 if (i == res.getHeader().size()) 18011 res.getHeader().add(parseString(null)); 18012 if (array.get(i) instanceof JsonObject) 18013 parseElementProperties(array.get(i).getAsJsonObject(), res.getHeader().get(i)); 18014 } 18015 }; 18016 } 18017 18018 protected Substance parseSubstance(JsonObject json) throws IOException, FHIRFormatError { 18019 Substance res = new Substance(); 18020 parseSubstanceProperties(json, res); 18021 return res; 18022 } 18023 18024 protected void parseSubstanceProperties(JsonObject json, Substance res) throws IOException, FHIRFormatError { 18025 parseDomainResourceProperties(json, res); 18026 if (json.has("identifier")) { 18027 JsonArray array = json.getAsJsonArray("identifier"); 18028 for (int i = 0; i < array.size(); i++) { 18029 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 18030 } 18031 }; 18032 if (json.has("status")) 18033 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Substance.FHIRSubstanceStatus.NULL, new Substance.FHIRSubstanceStatusEnumFactory())); 18034 if (json.has("_status")) 18035 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 18036 if (json.has("category")) { 18037 JsonArray array = json.getAsJsonArray("category"); 18038 for (int i = 0; i < array.size(); i++) { 18039 res.getCategory().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 18040 } 18041 }; 18042 if (json.has("code")) 18043 res.setCode(parseCodeableConcept(getJObject(json, "code"))); 18044 if (json.has("description")) 18045 res.setDescriptionElement(parseString(json.get("description").getAsString())); 18046 if (json.has("_description")) 18047 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 18048 if (json.has("instance")) { 18049 JsonArray array = json.getAsJsonArray("instance"); 18050 for (int i = 0; i < array.size(); i++) { 18051 res.getInstance().add(parseSubstanceSubstanceInstanceComponent(array.get(i).getAsJsonObject(), res)); 18052 } 18053 }; 18054 if (json.has("ingredient")) { 18055 JsonArray array = json.getAsJsonArray("ingredient"); 18056 for (int i = 0; i < array.size(); i++) { 18057 res.getIngredient().add(parseSubstanceSubstanceIngredientComponent(array.get(i).getAsJsonObject(), res)); 18058 } 18059 }; 18060 } 18061 18062 protected Substance.SubstanceInstanceComponent parseSubstanceSubstanceInstanceComponent(JsonObject json, Substance owner) throws IOException, FHIRFormatError { 18063 Substance.SubstanceInstanceComponent res = new Substance.SubstanceInstanceComponent(); 18064 parseSubstanceSubstanceInstanceComponentProperties(json, owner, res); 18065 return res; 18066 } 18067 18068 protected void parseSubstanceSubstanceInstanceComponentProperties(JsonObject json, Substance owner, Substance.SubstanceInstanceComponent res) throws IOException, FHIRFormatError { 18069 parseBackboneProperties(json, res); 18070 if (json.has("identifier")) 18071 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 18072 if (json.has("expiry")) 18073 res.setExpiryElement(parseDateTime(json.get("expiry").getAsString())); 18074 if (json.has("_expiry")) 18075 parseElementProperties(getJObject(json, "_expiry"), res.getExpiryElement()); 18076 if (json.has("quantity")) 18077 res.setQuantity(parseSimpleQuantity(getJObject(json, "quantity"))); 18078 } 18079 18080 protected Substance.SubstanceIngredientComponent parseSubstanceSubstanceIngredientComponent(JsonObject json, Substance owner) throws IOException, FHIRFormatError { 18081 Substance.SubstanceIngredientComponent res = new Substance.SubstanceIngredientComponent(); 18082 parseSubstanceSubstanceIngredientComponentProperties(json, owner, res); 18083 return res; 18084 } 18085 18086 protected void parseSubstanceSubstanceIngredientComponentProperties(JsonObject json, Substance owner, Substance.SubstanceIngredientComponent res) throws IOException, FHIRFormatError { 18087 parseBackboneProperties(json, res); 18088 if (json.has("quantity")) 18089 res.setQuantity(parseRatio(getJObject(json, "quantity"))); 18090 Type substance = parseType("substance", json); 18091 if (substance != null) 18092 res.setSubstance(substance); 18093 } 18094 18095 protected SupplyDelivery parseSupplyDelivery(JsonObject json) throws IOException, FHIRFormatError { 18096 SupplyDelivery res = new SupplyDelivery(); 18097 parseSupplyDeliveryProperties(json, res); 18098 return res; 18099 } 18100 18101 protected void parseSupplyDeliveryProperties(JsonObject json, SupplyDelivery res) throws IOException, FHIRFormatError { 18102 parseDomainResourceProperties(json, res); 18103 if (json.has("identifier")) 18104 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 18105 if (json.has("basedOn")) { 18106 JsonArray array = json.getAsJsonArray("basedOn"); 18107 for (int i = 0; i < array.size(); i++) { 18108 res.getBasedOn().add(parseReference(array.get(i).getAsJsonObject())); 18109 } 18110 }; 18111 if (json.has("partOf")) { 18112 JsonArray array = json.getAsJsonArray("partOf"); 18113 for (int i = 0; i < array.size(); i++) { 18114 res.getPartOf().add(parseReference(array.get(i).getAsJsonObject())); 18115 } 18116 }; 18117 if (json.has("status")) 18118 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), SupplyDelivery.SupplyDeliveryStatus.NULL, new SupplyDelivery.SupplyDeliveryStatusEnumFactory())); 18119 if (json.has("_status")) 18120 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 18121 if (json.has("patient")) 18122 res.setPatient(parseReference(getJObject(json, "patient"))); 18123 if (json.has("type")) 18124 res.setType(parseCodeableConcept(getJObject(json, "type"))); 18125 if (json.has("suppliedItem")) 18126 res.setSuppliedItem(parseSupplyDeliverySupplyDeliverySuppliedItemComponent(getJObject(json, "suppliedItem"), res)); 18127 Type occurrence = parseType("occurrence", json); 18128 if (occurrence != null) 18129 res.setOccurrence(occurrence); 18130 if (json.has("supplier")) 18131 res.setSupplier(parseReference(getJObject(json, "supplier"))); 18132 if (json.has("destination")) 18133 res.setDestination(parseReference(getJObject(json, "destination"))); 18134 if (json.has("receiver")) { 18135 JsonArray array = json.getAsJsonArray("receiver"); 18136 for (int i = 0; i < array.size(); i++) { 18137 res.getReceiver().add(parseReference(array.get(i).getAsJsonObject())); 18138 } 18139 }; 18140 } 18141 18142 protected SupplyDelivery.SupplyDeliverySuppliedItemComponent parseSupplyDeliverySupplyDeliverySuppliedItemComponent(JsonObject json, SupplyDelivery owner) throws IOException, FHIRFormatError { 18143 SupplyDelivery.SupplyDeliverySuppliedItemComponent res = new SupplyDelivery.SupplyDeliverySuppliedItemComponent(); 18144 parseSupplyDeliverySupplyDeliverySuppliedItemComponentProperties(json, owner, res); 18145 return res; 18146 } 18147 18148 protected void parseSupplyDeliverySupplyDeliverySuppliedItemComponentProperties(JsonObject json, SupplyDelivery owner, SupplyDelivery.SupplyDeliverySuppliedItemComponent res) throws IOException, FHIRFormatError { 18149 parseBackboneProperties(json, res); 18150 if (json.has("quantity")) 18151 res.setQuantity(parseSimpleQuantity(getJObject(json, "quantity"))); 18152 Type item = parseType("item", json); 18153 if (item != null) 18154 res.setItem(item); 18155 } 18156 18157 protected SupplyRequest parseSupplyRequest(JsonObject json) throws IOException, FHIRFormatError { 18158 SupplyRequest res = new SupplyRequest(); 18159 parseSupplyRequestProperties(json, res); 18160 return res; 18161 } 18162 18163 protected void parseSupplyRequestProperties(JsonObject json, SupplyRequest res) throws IOException, FHIRFormatError { 18164 parseDomainResourceProperties(json, res); 18165 if (json.has("identifier")) 18166 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 18167 if (json.has("status")) 18168 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), SupplyRequest.SupplyRequestStatus.NULL, new SupplyRequest.SupplyRequestStatusEnumFactory())); 18169 if (json.has("_status")) 18170 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 18171 if (json.has("category")) 18172 res.setCategory(parseCodeableConcept(getJObject(json, "category"))); 18173 if (json.has("priority")) 18174 res.setPriorityElement(parseEnumeration(json.get("priority").getAsString(), SupplyRequest.RequestPriority.NULL, new SupplyRequest.RequestPriorityEnumFactory())); 18175 if (json.has("_priority")) 18176 parseElementProperties(getJObject(json, "_priority"), res.getPriorityElement()); 18177 if (json.has("orderedItem")) 18178 res.setOrderedItem(parseSupplyRequestSupplyRequestOrderedItemComponent(getJObject(json, "orderedItem"), res)); 18179 Type occurrence = parseType("occurrence", json); 18180 if (occurrence != null) 18181 res.setOccurrence(occurrence); 18182 if (json.has("authoredOn")) 18183 res.setAuthoredOnElement(parseDateTime(json.get("authoredOn").getAsString())); 18184 if (json.has("_authoredOn")) 18185 parseElementProperties(getJObject(json, "_authoredOn"), res.getAuthoredOnElement()); 18186 if (json.has("requester")) 18187 res.setRequester(parseSupplyRequestSupplyRequestRequesterComponent(getJObject(json, "requester"), res)); 18188 if (json.has("supplier")) { 18189 JsonArray array = json.getAsJsonArray("supplier"); 18190 for (int i = 0; i < array.size(); i++) { 18191 res.getSupplier().add(parseReference(array.get(i).getAsJsonObject())); 18192 } 18193 }; 18194 Type reason = parseType("reason", json); 18195 if (reason != null) 18196 res.setReason(reason); 18197 if (json.has("deliverFrom")) 18198 res.setDeliverFrom(parseReference(getJObject(json, "deliverFrom"))); 18199 if (json.has("deliverTo")) 18200 res.setDeliverTo(parseReference(getJObject(json, "deliverTo"))); 18201 } 18202 18203 protected SupplyRequest.SupplyRequestOrderedItemComponent parseSupplyRequestSupplyRequestOrderedItemComponent(JsonObject json, SupplyRequest owner) throws IOException, FHIRFormatError { 18204 SupplyRequest.SupplyRequestOrderedItemComponent res = new SupplyRequest.SupplyRequestOrderedItemComponent(); 18205 parseSupplyRequestSupplyRequestOrderedItemComponentProperties(json, owner, res); 18206 return res; 18207 } 18208 18209 protected void parseSupplyRequestSupplyRequestOrderedItemComponentProperties(JsonObject json, SupplyRequest owner, SupplyRequest.SupplyRequestOrderedItemComponent res) throws IOException, FHIRFormatError { 18210 parseBackboneProperties(json, res); 18211 if (json.has("quantity")) 18212 res.setQuantity(parseQuantity(getJObject(json, "quantity"))); 18213 Type item = parseType("item", json); 18214 if (item != null) 18215 res.setItem(item); 18216 } 18217 18218 protected SupplyRequest.SupplyRequestRequesterComponent parseSupplyRequestSupplyRequestRequesterComponent(JsonObject json, SupplyRequest owner) throws IOException, FHIRFormatError { 18219 SupplyRequest.SupplyRequestRequesterComponent res = new SupplyRequest.SupplyRequestRequesterComponent(); 18220 parseSupplyRequestSupplyRequestRequesterComponentProperties(json, owner, res); 18221 return res; 18222 } 18223 18224 protected void parseSupplyRequestSupplyRequestRequesterComponentProperties(JsonObject json, SupplyRequest owner, SupplyRequest.SupplyRequestRequesterComponent res) throws IOException, FHIRFormatError { 18225 parseBackboneProperties(json, res); 18226 if (json.has("agent")) 18227 res.setAgent(parseReference(getJObject(json, "agent"))); 18228 if (json.has("onBehalfOf")) 18229 res.setOnBehalfOf(parseReference(getJObject(json, "onBehalfOf"))); 18230 } 18231 18232 protected Task parseTask(JsonObject json) throws IOException, FHIRFormatError { 18233 Task res = new Task(); 18234 parseTaskProperties(json, res); 18235 return res; 18236 } 18237 18238 protected void parseTaskProperties(JsonObject json, Task res) throws IOException, FHIRFormatError { 18239 parseDomainResourceProperties(json, res); 18240 if (json.has("identifier")) { 18241 JsonArray array = json.getAsJsonArray("identifier"); 18242 for (int i = 0; i < array.size(); i++) { 18243 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 18244 } 18245 }; 18246 Type definition = parseType("definition", json); 18247 if (definition != null) 18248 res.setDefinition(definition); 18249 if (json.has("basedOn")) { 18250 JsonArray array = json.getAsJsonArray("basedOn"); 18251 for (int i = 0; i < array.size(); i++) { 18252 res.getBasedOn().add(parseReference(array.get(i).getAsJsonObject())); 18253 } 18254 }; 18255 if (json.has("groupIdentifier")) 18256 res.setGroupIdentifier(parseIdentifier(getJObject(json, "groupIdentifier"))); 18257 if (json.has("partOf")) { 18258 JsonArray array = json.getAsJsonArray("partOf"); 18259 for (int i = 0; i < array.size(); i++) { 18260 res.getPartOf().add(parseReference(array.get(i).getAsJsonObject())); 18261 } 18262 }; 18263 if (json.has("status")) 18264 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Task.TaskStatus.NULL, new Task.TaskStatusEnumFactory())); 18265 if (json.has("_status")) 18266 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 18267 if (json.has("statusReason")) 18268 res.setStatusReason(parseCodeableConcept(getJObject(json, "statusReason"))); 18269 if (json.has("businessStatus")) 18270 res.setBusinessStatus(parseCodeableConcept(getJObject(json, "businessStatus"))); 18271 if (json.has("intent")) 18272 res.setIntentElement(parseEnumeration(json.get("intent").getAsString(), Task.TaskIntent.NULL, new Task.TaskIntentEnumFactory())); 18273 if (json.has("_intent")) 18274 parseElementProperties(getJObject(json, "_intent"), res.getIntentElement()); 18275 if (json.has("priority")) 18276 res.setPriorityElement(parseEnumeration(json.get("priority").getAsString(), Task.TaskPriority.NULL, new Task.TaskPriorityEnumFactory())); 18277 if (json.has("_priority")) 18278 parseElementProperties(getJObject(json, "_priority"), res.getPriorityElement()); 18279 if (json.has("code")) 18280 res.setCode(parseCodeableConcept(getJObject(json, "code"))); 18281 if (json.has("description")) 18282 res.setDescriptionElement(parseString(json.get("description").getAsString())); 18283 if (json.has("_description")) 18284 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 18285 if (json.has("focus")) 18286 res.setFocus(parseReference(getJObject(json, "focus"))); 18287 if (json.has("for")) 18288 res.setFor(parseReference(getJObject(json, "for"))); 18289 if (json.has("context")) 18290 res.setContext(parseReference(getJObject(json, "context"))); 18291 if (json.has("executionPeriod")) 18292 res.setExecutionPeriod(parsePeriod(getJObject(json, "executionPeriod"))); 18293 if (json.has("authoredOn")) 18294 res.setAuthoredOnElement(parseDateTime(json.get("authoredOn").getAsString())); 18295 if (json.has("_authoredOn")) 18296 parseElementProperties(getJObject(json, "_authoredOn"), res.getAuthoredOnElement()); 18297 if (json.has("lastModified")) 18298 res.setLastModifiedElement(parseDateTime(json.get("lastModified").getAsString())); 18299 if (json.has("_lastModified")) 18300 parseElementProperties(getJObject(json, "_lastModified"), res.getLastModifiedElement()); 18301 if (json.has("requester")) 18302 res.setRequester(parseTaskTaskRequesterComponent(getJObject(json, "requester"), res)); 18303 if (json.has("performerType")) { 18304 JsonArray array = json.getAsJsonArray("performerType"); 18305 for (int i = 0; i < array.size(); i++) { 18306 res.getPerformerType().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 18307 } 18308 }; 18309 if (json.has("owner")) 18310 res.setOwner(parseReference(getJObject(json, "owner"))); 18311 if (json.has("reason")) 18312 res.setReason(parseCodeableConcept(getJObject(json, "reason"))); 18313 if (json.has("note")) { 18314 JsonArray array = json.getAsJsonArray("note"); 18315 for (int i = 0; i < array.size(); i++) { 18316 res.getNote().add(parseAnnotation(array.get(i).getAsJsonObject())); 18317 } 18318 }; 18319 if (json.has("relevantHistory")) { 18320 JsonArray array = json.getAsJsonArray("relevantHistory"); 18321 for (int i = 0; i < array.size(); i++) { 18322 res.getRelevantHistory().add(parseReference(array.get(i).getAsJsonObject())); 18323 } 18324 }; 18325 if (json.has("restriction")) 18326 res.setRestriction(parseTaskTaskRestrictionComponent(getJObject(json, "restriction"), res)); 18327 if (json.has("input")) { 18328 JsonArray array = json.getAsJsonArray("input"); 18329 for (int i = 0; i < array.size(); i++) { 18330 res.getInput().add(parseTaskParameterComponent(array.get(i).getAsJsonObject(), res)); 18331 } 18332 }; 18333 if (json.has("output")) { 18334 JsonArray array = json.getAsJsonArray("output"); 18335 for (int i = 0; i < array.size(); i++) { 18336 res.getOutput().add(parseTaskTaskOutputComponent(array.get(i).getAsJsonObject(), res)); 18337 } 18338 }; 18339 } 18340 18341 protected Task.TaskRequesterComponent parseTaskTaskRequesterComponent(JsonObject json, Task owner) throws IOException, FHIRFormatError { 18342 Task.TaskRequesterComponent res = new Task.TaskRequesterComponent(); 18343 parseTaskTaskRequesterComponentProperties(json, owner, res); 18344 return res; 18345 } 18346 18347 protected void parseTaskTaskRequesterComponentProperties(JsonObject json, Task owner, Task.TaskRequesterComponent res) throws IOException, FHIRFormatError { 18348 parseBackboneProperties(json, res); 18349 if (json.has("agent")) 18350 res.setAgent(parseReference(getJObject(json, "agent"))); 18351 if (json.has("onBehalfOf")) 18352 res.setOnBehalfOf(parseReference(getJObject(json, "onBehalfOf"))); 18353 } 18354 18355 protected Task.TaskRestrictionComponent parseTaskTaskRestrictionComponent(JsonObject json, Task owner) throws IOException, FHIRFormatError { 18356 Task.TaskRestrictionComponent res = new Task.TaskRestrictionComponent(); 18357 parseTaskTaskRestrictionComponentProperties(json, owner, res); 18358 return res; 18359 } 18360 18361 protected void parseTaskTaskRestrictionComponentProperties(JsonObject json, Task owner, Task.TaskRestrictionComponent res) throws IOException, FHIRFormatError { 18362 parseBackboneProperties(json, res); 18363 if (json.has("repetitions")) 18364 res.setRepetitionsElement(parsePositiveInt(json.get("repetitions").getAsString())); 18365 if (json.has("_repetitions")) 18366 parseElementProperties(getJObject(json, "_repetitions"), res.getRepetitionsElement()); 18367 if (json.has("period")) 18368 res.setPeriod(parsePeriod(getJObject(json, "period"))); 18369 if (json.has("recipient")) { 18370 JsonArray array = json.getAsJsonArray("recipient"); 18371 for (int i = 0; i < array.size(); i++) { 18372 res.getRecipient().add(parseReference(array.get(i).getAsJsonObject())); 18373 } 18374 }; 18375 } 18376 18377 protected Task.ParameterComponent parseTaskParameterComponent(JsonObject json, Task owner) throws IOException, FHIRFormatError { 18378 Task.ParameterComponent res = new Task.ParameterComponent(); 18379 parseTaskParameterComponentProperties(json, owner, res); 18380 return res; 18381 } 18382 18383 protected void parseTaskParameterComponentProperties(JsonObject json, Task owner, Task.ParameterComponent res) throws IOException, FHIRFormatError { 18384 parseBackboneProperties(json, res); 18385 if (json.has("type")) 18386 res.setType(parseCodeableConcept(getJObject(json, "type"))); 18387 Type value = parseType("value", json); 18388 if (value != null) 18389 res.setValue(value); 18390 } 18391 18392 protected Task.TaskOutputComponent parseTaskTaskOutputComponent(JsonObject json, Task owner) throws IOException, FHIRFormatError { 18393 Task.TaskOutputComponent res = new Task.TaskOutputComponent(); 18394 parseTaskTaskOutputComponentProperties(json, owner, res); 18395 return res; 18396 } 18397 18398 protected void parseTaskTaskOutputComponentProperties(JsonObject json, Task owner, Task.TaskOutputComponent res) throws IOException, FHIRFormatError { 18399 parseBackboneProperties(json, res); 18400 if (json.has("type")) 18401 res.setType(parseCodeableConcept(getJObject(json, "type"))); 18402 Type value = parseType("value", json); 18403 if (value != null) 18404 res.setValue(value); 18405 } 18406 18407 protected TestReport parseTestReport(JsonObject json) throws IOException, FHIRFormatError { 18408 TestReport res = new TestReport(); 18409 parseTestReportProperties(json, res); 18410 return res; 18411 } 18412 18413 protected void parseTestReportProperties(JsonObject json, TestReport res) throws IOException, FHIRFormatError { 18414 parseDomainResourceProperties(json, res); 18415 if (json.has("identifier")) 18416 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 18417 if (json.has("name")) 18418 res.setNameElement(parseString(json.get("name").getAsString())); 18419 if (json.has("_name")) 18420 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 18421 if (json.has("status")) 18422 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), TestReport.TestReportStatus.NULL, new TestReport.TestReportStatusEnumFactory())); 18423 if (json.has("_status")) 18424 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 18425 if (json.has("testScript")) 18426 res.setTestScript(parseReference(getJObject(json, "testScript"))); 18427 if (json.has("result")) 18428 res.setResultElement(parseEnumeration(json.get("result").getAsString(), TestReport.TestReportResult.NULL, new TestReport.TestReportResultEnumFactory())); 18429 if (json.has("_result")) 18430 parseElementProperties(getJObject(json, "_result"), res.getResultElement()); 18431 if (json.has("score")) 18432 res.setScoreElement(parseDecimal(json.get("score").getAsBigDecimal())); 18433 if (json.has("_score")) 18434 parseElementProperties(getJObject(json, "_score"), res.getScoreElement()); 18435 if (json.has("tester")) 18436 res.setTesterElement(parseString(json.get("tester").getAsString())); 18437 if (json.has("_tester")) 18438 parseElementProperties(getJObject(json, "_tester"), res.getTesterElement()); 18439 if (json.has("issued")) 18440 res.setIssuedElement(parseDateTime(json.get("issued").getAsString())); 18441 if (json.has("_issued")) 18442 parseElementProperties(getJObject(json, "_issued"), res.getIssuedElement()); 18443 if (json.has("participant")) { 18444 JsonArray array = json.getAsJsonArray("participant"); 18445 for (int i = 0; i < array.size(); i++) { 18446 res.getParticipant().add(parseTestReportTestReportParticipantComponent(array.get(i).getAsJsonObject(), res)); 18447 } 18448 }; 18449 if (json.has("setup")) 18450 res.setSetup(parseTestReportTestReportSetupComponent(getJObject(json, "setup"), res)); 18451 if (json.has("test")) { 18452 JsonArray array = json.getAsJsonArray("test"); 18453 for (int i = 0; i < array.size(); i++) { 18454 res.getTest().add(parseTestReportTestReportTestComponent(array.get(i).getAsJsonObject(), res)); 18455 } 18456 }; 18457 if (json.has("teardown")) 18458 res.setTeardown(parseTestReportTestReportTeardownComponent(getJObject(json, "teardown"), res)); 18459 } 18460 18461 protected TestReport.TestReportParticipantComponent parseTestReportTestReportParticipantComponent(JsonObject json, TestReport owner) throws IOException, FHIRFormatError { 18462 TestReport.TestReportParticipantComponent res = new TestReport.TestReportParticipantComponent(); 18463 parseTestReportTestReportParticipantComponentProperties(json, owner, res); 18464 return res; 18465 } 18466 18467 protected void parseTestReportTestReportParticipantComponentProperties(JsonObject json, TestReport owner, TestReport.TestReportParticipantComponent res) throws IOException, FHIRFormatError { 18468 parseBackboneProperties(json, res); 18469 if (json.has("type")) 18470 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), TestReport.TestReportParticipantType.NULL, new TestReport.TestReportParticipantTypeEnumFactory())); 18471 if (json.has("_type")) 18472 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 18473 if (json.has("uri")) 18474 res.setUriElement(parseUri(json.get("uri").getAsString())); 18475 if (json.has("_uri")) 18476 parseElementProperties(getJObject(json, "_uri"), res.getUriElement()); 18477 if (json.has("display")) 18478 res.setDisplayElement(parseString(json.get("display").getAsString())); 18479 if (json.has("_display")) 18480 parseElementProperties(getJObject(json, "_display"), res.getDisplayElement()); 18481 } 18482 18483 protected TestReport.TestReportSetupComponent parseTestReportTestReportSetupComponent(JsonObject json, TestReport owner) throws IOException, FHIRFormatError { 18484 TestReport.TestReportSetupComponent res = new TestReport.TestReportSetupComponent(); 18485 parseTestReportTestReportSetupComponentProperties(json, owner, res); 18486 return res; 18487 } 18488 18489 protected void parseTestReportTestReportSetupComponentProperties(JsonObject json, TestReport owner, TestReport.TestReportSetupComponent res) throws IOException, FHIRFormatError { 18490 parseBackboneProperties(json, res); 18491 if (json.has("action")) { 18492 JsonArray array = json.getAsJsonArray("action"); 18493 for (int i = 0; i < array.size(); i++) { 18494 res.getAction().add(parseTestReportSetupActionComponent(array.get(i).getAsJsonObject(), owner)); 18495 } 18496 }; 18497 } 18498 18499 protected TestReport.SetupActionComponent parseTestReportSetupActionComponent(JsonObject json, TestReport owner) throws IOException, FHIRFormatError { 18500 TestReport.SetupActionComponent res = new TestReport.SetupActionComponent(); 18501 parseTestReportSetupActionComponentProperties(json, owner, res); 18502 return res; 18503 } 18504 18505 protected void parseTestReportSetupActionComponentProperties(JsonObject json, TestReport owner, TestReport.SetupActionComponent res) throws IOException, FHIRFormatError { 18506 parseBackboneProperties(json, res); 18507 if (json.has("operation")) 18508 res.setOperation(parseTestReportSetupActionOperationComponent(getJObject(json, "operation"), owner)); 18509 if (json.has("assert")) 18510 res.setAssert(parseTestReportSetupActionAssertComponent(getJObject(json, "assert"), owner)); 18511 } 18512 18513 protected TestReport.SetupActionOperationComponent parseTestReportSetupActionOperationComponent(JsonObject json, TestReport owner) throws IOException, FHIRFormatError { 18514 TestReport.SetupActionOperationComponent res = new TestReport.SetupActionOperationComponent(); 18515 parseTestReportSetupActionOperationComponentProperties(json, owner, res); 18516 return res; 18517 } 18518 18519 protected void parseTestReportSetupActionOperationComponentProperties(JsonObject json, TestReport owner, TestReport.SetupActionOperationComponent res) throws IOException, FHIRFormatError { 18520 parseBackboneProperties(json, res); 18521 if (json.has("result")) 18522 res.setResultElement(parseEnumeration(json.get("result").getAsString(), TestReport.TestReportActionResult.NULL, new TestReport.TestReportActionResultEnumFactory())); 18523 if (json.has("_result")) 18524 parseElementProperties(getJObject(json, "_result"), res.getResultElement()); 18525 if (json.has("message")) 18526 res.setMessageElement(parseMarkdown(json.get("message").getAsString())); 18527 if (json.has("_message")) 18528 parseElementProperties(getJObject(json, "_message"), res.getMessageElement()); 18529 if (json.has("detail")) 18530 res.setDetailElement(parseUri(json.get("detail").getAsString())); 18531 if (json.has("_detail")) 18532 parseElementProperties(getJObject(json, "_detail"), res.getDetailElement()); 18533 } 18534 18535 protected TestReport.SetupActionAssertComponent parseTestReportSetupActionAssertComponent(JsonObject json, TestReport owner) throws IOException, FHIRFormatError { 18536 TestReport.SetupActionAssertComponent res = new TestReport.SetupActionAssertComponent(); 18537 parseTestReportSetupActionAssertComponentProperties(json, owner, res); 18538 return res; 18539 } 18540 18541 protected void parseTestReportSetupActionAssertComponentProperties(JsonObject json, TestReport owner, TestReport.SetupActionAssertComponent res) throws IOException, FHIRFormatError { 18542 parseBackboneProperties(json, res); 18543 if (json.has("result")) 18544 res.setResultElement(parseEnumeration(json.get("result").getAsString(), TestReport.TestReportActionResult.NULL, new TestReport.TestReportActionResultEnumFactory())); 18545 if (json.has("_result")) 18546 parseElementProperties(getJObject(json, "_result"), res.getResultElement()); 18547 if (json.has("message")) 18548 res.setMessageElement(parseMarkdown(json.get("message").getAsString())); 18549 if (json.has("_message")) 18550 parseElementProperties(getJObject(json, "_message"), res.getMessageElement()); 18551 if (json.has("detail")) 18552 res.setDetailElement(parseString(json.get("detail").getAsString())); 18553 if (json.has("_detail")) 18554 parseElementProperties(getJObject(json, "_detail"), res.getDetailElement()); 18555 } 18556 18557 protected TestReport.TestReportTestComponent parseTestReportTestReportTestComponent(JsonObject json, TestReport owner) throws IOException, FHIRFormatError { 18558 TestReport.TestReportTestComponent res = new TestReport.TestReportTestComponent(); 18559 parseTestReportTestReportTestComponentProperties(json, owner, res); 18560 return res; 18561 } 18562 18563 protected void parseTestReportTestReportTestComponentProperties(JsonObject json, TestReport owner, TestReport.TestReportTestComponent res) throws IOException, FHIRFormatError { 18564 parseBackboneProperties(json, res); 18565 if (json.has("name")) 18566 res.setNameElement(parseString(json.get("name").getAsString())); 18567 if (json.has("_name")) 18568 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 18569 if (json.has("description")) 18570 res.setDescriptionElement(parseString(json.get("description").getAsString())); 18571 if (json.has("_description")) 18572 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 18573 if (json.has("action")) { 18574 JsonArray array = json.getAsJsonArray("action"); 18575 for (int i = 0; i < array.size(); i++) { 18576 res.getAction().add(parseTestReportTestActionComponent(array.get(i).getAsJsonObject(), owner)); 18577 } 18578 }; 18579 } 18580 18581 protected TestReport.TestActionComponent parseTestReportTestActionComponent(JsonObject json, TestReport owner) throws IOException, FHIRFormatError { 18582 TestReport.TestActionComponent res = new TestReport.TestActionComponent(); 18583 parseTestReportTestActionComponentProperties(json, owner, res); 18584 return res; 18585 } 18586 18587 protected void parseTestReportTestActionComponentProperties(JsonObject json, TestReport owner, TestReport.TestActionComponent res) throws IOException, FHIRFormatError { 18588 parseBackboneProperties(json, res); 18589 if (json.has("operation")) 18590 res.setOperation(parseTestReportSetupActionOperationComponent(getJObject(json, "operation"), owner)); 18591 if (json.has("assert")) 18592 res.setAssert(parseTestReportSetupActionAssertComponent(getJObject(json, "assert"), owner)); 18593 } 18594 18595 protected TestReport.TestReportTeardownComponent parseTestReportTestReportTeardownComponent(JsonObject json, TestReport owner) throws IOException, FHIRFormatError { 18596 TestReport.TestReportTeardownComponent res = new TestReport.TestReportTeardownComponent(); 18597 parseTestReportTestReportTeardownComponentProperties(json, owner, res); 18598 return res; 18599 } 18600 18601 protected void parseTestReportTestReportTeardownComponentProperties(JsonObject json, TestReport owner, TestReport.TestReportTeardownComponent res) throws IOException, FHIRFormatError { 18602 parseBackboneProperties(json, res); 18603 if (json.has("action")) { 18604 JsonArray array = json.getAsJsonArray("action"); 18605 for (int i = 0; i < array.size(); i++) { 18606 res.getAction().add(parseTestReportTeardownActionComponent(array.get(i).getAsJsonObject(), owner)); 18607 } 18608 }; 18609 } 18610 18611 protected TestReport.TeardownActionComponent parseTestReportTeardownActionComponent(JsonObject json, TestReport owner) throws IOException, FHIRFormatError { 18612 TestReport.TeardownActionComponent res = new TestReport.TeardownActionComponent(); 18613 parseTestReportTeardownActionComponentProperties(json, owner, res); 18614 return res; 18615 } 18616 18617 protected void parseTestReportTeardownActionComponentProperties(JsonObject json, TestReport owner, TestReport.TeardownActionComponent res) throws IOException, FHIRFormatError { 18618 parseBackboneProperties(json, res); 18619 if (json.has("operation")) 18620 res.setOperation(parseTestReportSetupActionOperationComponent(getJObject(json, "operation"), owner)); 18621 } 18622 18623 protected TestScript parseTestScript(JsonObject json) throws IOException, FHIRFormatError { 18624 TestScript res = new TestScript(); 18625 parseTestScriptProperties(json, res); 18626 return res; 18627 } 18628 18629 protected void parseTestScriptProperties(JsonObject json, TestScript res) throws IOException, FHIRFormatError { 18630 parseDomainResourceProperties(json, res); 18631 if (json.has("url")) 18632 res.setUrlElement(parseUri(json.get("url").getAsString())); 18633 if (json.has("_url")) 18634 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 18635 if (json.has("identifier")) 18636 res.setIdentifier(parseIdentifier(getJObject(json, "identifier"))); 18637 if (json.has("version")) 18638 res.setVersionElement(parseString(json.get("version").getAsString())); 18639 if (json.has("_version")) 18640 parseElementProperties(getJObject(json, "_version"), res.getVersionElement()); 18641 if (json.has("name")) 18642 res.setNameElement(parseString(json.get("name").getAsString())); 18643 if (json.has("_name")) 18644 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 18645 if (json.has("title")) 18646 res.setTitleElement(parseString(json.get("title").getAsString())); 18647 if (json.has("_title")) 18648 parseElementProperties(getJObject(json, "_title"), res.getTitleElement()); 18649 if (json.has("status")) 18650 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Enumerations.PublicationStatus.NULL, new Enumerations.PublicationStatusEnumFactory())); 18651 if (json.has("_status")) 18652 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 18653 if (json.has("experimental")) 18654 res.setExperimentalElement(parseBoolean(json.get("experimental").getAsBoolean())); 18655 if (json.has("_experimental")) 18656 parseElementProperties(getJObject(json, "_experimental"), res.getExperimentalElement()); 18657 if (json.has("date")) 18658 res.setDateElement(parseDateTime(json.get("date").getAsString())); 18659 if (json.has("_date")) 18660 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 18661 if (json.has("publisher")) 18662 res.setPublisherElement(parseString(json.get("publisher").getAsString())); 18663 if (json.has("_publisher")) 18664 parseElementProperties(getJObject(json, "_publisher"), res.getPublisherElement()); 18665 if (json.has("contact")) { 18666 JsonArray array = json.getAsJsonArray("contact"); 18667 for (int i = 0; i < array.size(); i++) { 18668 res.getContact().add(parseContactDetail(array.get(i).getAsJsonObject())); 18669 } 18670 }; 18671 if (json.has("description")) 18672 res.setDescriptionElement(parseMarkdown(json.get("description").getAsString())); 18673 if (json.has("_description")) 18674 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 18675 if (json.has("useContext")) { 18676 JsonArray array = json.getAsJsonArray("useContext"); 18677 for (int i = 0; i < array.size(); i++) { 18678 res.getUseContext().add(parseUsageContext(array.get(i).getAsJsonObject())); 18679 } 18680 }; 18681 if (json.has("jurisdiction")) { 18682 JsonArray array = json.getAsJsonArray("jurisdiction"); 18683 for (int i = 0; i < array.size(); i++) { 18684 res.getJurisdiction().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 18685 } 18686 }; 18687 if (json.has("purpose")) 18688 res.setPurposeElement(parseMarkdown(json.get("purpose").getAsString())); 18689 if (json.has("_purpose")) 18690 parseElementProperties(getJObject(json, "_purpose"), res.getPurposeElement()); 18691 if (json.has("copyright")) 18692 res.setCopyrightElement(parseMarkdown(json.get("copyright").getAsString())); 18693 if (json.has("_copyright")) 18694 parseElementProperties(getJObject(json, "_copyright"), res.getCopyrightElement()); 18695 if (json.has("origin")) { 18696 JsonArray array = json.getAsJsonArray("origin"); 18697 for (int i = 0; i < array.size(); i++) { 18698 res.getOrigin().add(parseTestScriptTestScriptOriginComponent(array.get(i).getAsJsonObject(), res)); 18699 } 18700 }; 18701 if (json.has("destination")) { 18702 JsonArray array = json.getAsJsonArray("destination"); 18703 for (int i = 0; i < array.size(); i++) { 18704 res.getDestination().add(parseTestScriptTestScriptDestinationComponent(array.get(i).getAsJsonObject(), res)); 18705 } 18706 }; 18707 if (json.has("metadata")) 18708 res.setMetadata(parseTestScriptTestScriptMetadataComponent(getJObject(json, "metadata"), res)); 18709 if (json.has("fixture")) { 18710 JsonArray array = json.getAsJsonArray("fixture"); 18711 for (int i = 0; i < array.size(); i++) { 18712 res.getFixture().add(parseTestScriptTestScriptFixtureComponent(array.get(i).getAsJsonObject(), res)); 18713 } 18714 }; 18715 if (json.has("profile")) { 18716 JsonArray array = json.getAsJsonArray("profile"); 18717 for (int i = 0; i < array.size(); i++) { 18718 res.getProfile().add(parseReference(array.get(i).getAsJsonObject())); 18719 } 18720 }; 18721 if (json.has("variable")) { 18722 JsonArray array = json.getAsJsonArray("variable"); 18723 for (int i = 0; i < array.size(); i++) { 18724 res.getVariable().add(parseTestScriptTestScriptVariableComponent(array.get(i).getAsJsonObject(), res)); 18725 } 18726 }; 18727 if (json.has("rule")) { 18728 JsonArray array = json.getAsJsonArray("rule"); 18729 for (int i = 0; i < array.size(); i++) { 18730 res.getRule().add(parseTestScriptTestScriptRuleComponent(array.get(i).getAsJsonObject(), res)); 18731 } 18732 }; 18733 if (json.has("ruleset")) { 18734 JsonArray array = json.getAsJsonArray("ruleset"); 18735 for (int i = 0; i < array.size(); i++) { 18736 res.getRuleset().add(parseTestScriptTestScriptRulesetComponent(array.get(i).getAsJsonObject(), res)); 18737 } 18738 }; 18739 if (json.has("setup")) 18740 res.setSetup(parseTestScriptTestScriptSetupComponent(getJObject(json, "setup"), res)); 18741 if (json.has("test")) { 18742 JsonArray array = json.getAsJsonArray("test"); 18743 for (int i = 0; i < array.size(); i++) { 18744 res.getTest().add(parseTestScriptTestScriptTestComponent(array.get(i).getAsJsonObject(), res)); 18745 } 18746 }; 18747 if (json.has("teardown")) 18748 res.setTeardown(parseTestScriptTestScriptTeardownComponent(getJObject(json, "teardown"), res)); 18749 } 18750 18751 protected TestScript.TestScriptOriginComponent parseTestScriptTestScriptOriginComponent(JsonObject json, TestScript owner) throws IOException, FHIRFormatError { 18752 TestScript.TestScriptOriginComponent res = new TestScript.TestScriptOriginComponent(); 18753 parseTestScriptTestScriptOriginComponentProperties(json, owner, res); 18754 return res; 18755 } 18756 18757 protected void parseTestScriptTestScriptOriginComponentProperties(JsonObject json, TestScript owner, TestScript.TestScriptOriginComponent res) throws IOException, FHIRFormatError { 18758 parseBackboneProperties(json, res); 18759 if (json.has("index")) 18760 res.setIndexElement(parseInteger(json.get("index").getAsLong())); 18761 if (json.has("_index")) 18762 parseElementProperties(getJObject(json, "_index"), res.getIndexElement()); 18763 if (json.has("profile")) 18764 res.setProfile(parseCoding(getJObject(json, "profile"))); 18765 } 18766 18767 protected TestScript.TestScriptDestinationComponent parseTestScriptTestScriptDestinationComponent(JsonObject json, TestScript owner) throws IOException, FHIRFormatError { 18768 TestScript.TestScriptDestinationComponent res = new TestScript.TestScriptDestinationComponent(); 18769 parseTestScriptTestScriptDestinationComponentProperties(json, owner, res); 18770 return res; 18771 } 18772 18773 protected void parseTestScriptTestScriptDestinationComponentProperties(JsonObject json, TestScript owner, TestScript.TestScriptDestinationComponent res) throws IOException, FHIRFormatError { 18774 parseBackboneProperties(json, res); 18775 if (json.has("index")) 18776 res.setIndexElement(parseInteger(json.get("index").getAsLong())); 18777 if (json.has("_index")) 18778 parseElementProperties(getJObject(json, "_index"), res.getIndexElement()); 18779 if (json.has("profile")) 18780 res.setProfile(parseCoding(getJObject(json, "profile"))); 18781 } 18782 18783 protected TestScript.TestScriptMetadataComponent parseTestScriptTestScriptMetadataComponent(JsonObject json, TestScript owner) throws IOException, FHIRFormatError { 18784 TestScript.TestScriptMetadataComponent res = new TestScript.TestScriptMetadataComponent(); 18785 parseTestScriptTestScriptMetadataComponentProperties(json, owner, res); 18786 return res; 18787 } 18788 18789 protected void parseTestScriptTestScriptMetadataComponentProperties(JsonObject json, TestScript owner, TestScript.TestScriptMetadataComponent res) throws IOException, FHIRFormatError { 18790 parseBackboneProperties(json, res); 18791 if (json.has("link")) { 18792 JsonArray array = json.getAsJsonArray("link"); 18793 for (int i = 0; i < array.size(); i++) { 18794 res.getLink().add(parseTestScriptTestScriptMetadataLinkComponent(array.get(i).getAsJsonObject(), owner)); 18795 } 18796 }; 18797 if (json.has("capability")) { 18798 JsonArray array = json.getAsJsonArray("capability"); 18799 for (int i = 0; i < array.size(); i++) { 18800 res.getCapability().add(parseTestScriptTestScriptMetadataCapabilityComponent(array.get(i).getAsJsonObject(), owner)); 18801 } 18802 }; 18803 } 18804 18805 protected TestScript.TestScriptMetadataLinkComponent parseTestScriptTestScriptMetadataLinkComponent(JsonObject json, TestScript owner) throws IOException, FHIRFormatError { 18806 TestScript.TestScriptMetadataLinkComponent res = new TestScript.TestScriptMetadataLinkComponent(); 18807 parseTestScriptTestScriptMetadataLinkComponentProperties(json, owner, res); 18808 return res; 18809 } 18810 18811 protected void parseTestScriptTestScriptMetadataLinkComponentProperties(JsonObject json, TestScript owner, TestScript.TestScriptMetadataLinkComponent res) throws IOException, FHIRFormatError { 18812 parseBackboneProperties(json, res); 18813 if (json.has("url")) 18814 res.setUrlElement(parseUri(json.get("url").getAsString())); 18815 if (json.has("_url")) 18816 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 18817 if (json.has("description")) 18818 res.setDescriptionElement(parseString(json.get("description").getAsString())); 18819 if (json.has("_description")) 18820 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 18821 } 18822 18823 protected TestScript.TestScriptMetadataCapabilityComponent parseTestScriptTestScriptMetadataCapabilityComponent(JsonObject json, TestScript owner) throws IOException, FHIRFormatError { 18824 TestScript.TestScriptMetadataCapabilityComponent res = new TestScript.TestScriptMetadataCapabilityComponent(); 18825 parseTestScriptTestScriptMetadataCapabilityComponentProperties(json, owner, res); 18826 return res; 18827 } 18828 18829 protected void parseTestScriptTestScriptMetadataCapabilityComponentProperties(JsonObject json, TestScript owner, TestScript.TestScriptMetadataCapabilityComponent res) throws IOException, FHIRFormatError { 18830 parseBackboneProperties(json, res); 18831 if (json.has("required")) 18832 res.setRequiredElement(parseBoolean(json.get("required").getAsBoolean())); 18833 if (json.has("_required")) 18834 parseElementProperties(getJObject(json, "_required"), res.getRequiredElement()); 18835 if (json.has("validated")) 18836 res.setValidatedElement(parseBoolean(json.get("validated").getAsBoolean())); 18837 if (json.has("_validated")) 18838 parseElementProperties(getJObject(json, "_validated"), res.getValidatedElement()); 18839 if (json.has("description")) 18840 res.setDescriptionElement(parseString(json.get("description").getAsString())); 18841 if (json.has("_description")) 18842 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 18843 if (json.has("origin")) { 18844 JsonArray array = json.getAsJsonArray("origin"); 18845 for (int i = 0; i < array.size(); i++) { 18846 if (array.get(i).isJsonNull()) { 18847 res.getOrigin().add(new IntegerType()); 18848 } else { 18849 res.getOrigin().add(parseInteger(array.get(i).getAsLong())); 18850 } 18851 } 18852 }; 18853 if (json.has("_origin")) { 18854 JsonArray array = json.getAsJsonArray("_origin"); 18855 for (int i = 0; i < array.size(); i++) { 18856 if (i == res.getOrigin().size()) 18857 res.getOrigin().add(parseInteger(null)); 18858 if (array.get(i) instanceof JsonObject) 18859 parseElementProperties(array.get(i).getAsJsonObject(), res.getOrigin().get(i)); 18860 } 18861 }; 18862 if (json.has("destination")) 18863 res.setDestinationElement(parseInteger(json.get("destination").getAsLong())); 18864 if (json.has("_destination")) 18865 parseElementProperties(getJObject(json, "_destination"), res.getDestinationElement()); 18866 if (json.has("link")) { 18867 JsonArray array = json.getAsJsonArray("link"); 18868 for (int i = 0; i < array.size(); i++) { 18869 if (array.get(i).isJsonNull()) { 18870 res.getLink().add(new UriType()); 18871 } else { 18872 res.getLink().add(parseUri(array.get(i).getAsString())); 18873 } 18874 } 18875 }; 18876 if (json.has("_link")) { 18877 JsonArray array = json.getAsJsonArray("_link"); 18878 for (int i = 0; i < array.size(); i++) { 18879 if (i == res.getLink().size()) 18880 res.getLink().add(parseUri(null)); 18881 if (array.get(i) instanceof JsonObject) 18882 parseElementProperties(array.get(i).getAsJsonObject(), res.getLink().get(i)); 18883 } 18884 }; 18885 if (json.has("capabilities")) 18886 res.setCapabilities(parseReference(getJObject(json, "capabilities"))); 18887 } 18888 18889 protected TestScript.TestScriptFixtureComponent parseTestScriptTestScriptFixtureComponent(JsonObject json, TestScript owner) throws IOException, FHIRFormatError { 18890 TestScript.TestScriptFixtureComponent res = new TestScript.TestScriptFixtureComponent(); 18891 parseTestScriptTestScriptFixtureComponentProperties(json, owner, res); 18892 return res; 18893 } 18894 18895 protected void parseTestScriptTestScriptFixtureComponentProperties(JsonObject json, TestScript owner, TestScript.TestScriptFixtureComponent res) throws IOException, FHIRFormatError { 18896 parseBackboneProperties(json, res); 18897 if (json.has("autocreate")) 18898 res.setAutocreateElement(parseBoolean(json.get("autocreate").getAsBoolean())); 18899 if (json.has("_autocreate")) 18900 parseElementProperties(getJObject(json, "_autocreate"), res.getAutocreateElement()); 18901 if (json.has("autodelete")) 18902 res.setAutodeleteElement(parseBoolean(json.get("autodelete").getAsBoolean())); 18903 if (json.has("_autodelete")) 18904 parseElementProperties(getJObject(json, "_autodelete"), res.getAutodeleteElement()); 18905 if (json.has("resource")) 18906 res.setResource(parseReference(getJObject(json, "resource"))); 18907 } 18908 18909 protected TestScript.TestScriptVariableComponent parseTestScriptTestScriptVariableComponent(JsonObject json, TestScript owner) throws IOException, FHIRFormatError { 18910 TestScript.TestScriptVariableComponent res = new TestScript.TestScriptVariableComponent(); 18911 parseTestScriptTestScriptVariableComponentProperties(json, owner, res); 18912 return res; 18913 } 18914 18915 protected void parseTestScriptTestScriptVariableComponentProperties(JsonObject json, TestScript owner, TestScript.TestScriptVariableComponent res) throws IOException, FHIRFormatError { 18916 parseBackboneProperties(json, res); 18917 if (json.has("name")) 18918 res.setNameElement(parseString(json.get("name").getAsString())); 18919 if (json.has("_name")) 18920 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 18921 if (json.has("defaultValue")) 18922 res.setDefaultValueElement(parseString(json.get("defaultValue").getAsString())); 18923 if (json.has("_defaultValue")) 18924 parseElementProperties(getJObject(json, "_defaultValue"), res.getDefaultValueElement()); 18925 if (json.has("description")) 18926 res.setDescriptionElement(parseString(json.get("description").getAsString())); 18927 if (json.has("_description")) 18928 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 18929 if (json.has("expression")) 18930 res.setExpressionElement(parseString(json.get("expression").getAsString())); 18931 if (json.has("_expression")) 18932 parseElementProperties(getJObject(json, "_expression"), res.getExpressionElement()); 18933 if (json.has("headerField")) 18934 res.setHeaderFieldElement(parseString(json.get("headerField").getAsString())); 18935 if (json.has("_headerField")) 18936 parseElementProperties(getJObject(json, "_headerField"), res.getHeaderFieldElement()); 18937 if (json.has("hint")) 18938 res.setHintElement(parseString(json.get("hint").getAsString())); 18939 if (json.has("_hint")) 18940 parseElementProperties(getJObject(json, "_hint"), res.getHintElement()); 18941 if (json.has("path")) 18942 res.setPathElement(parseString(json.get("path").getAsString())); 18943 if (json.has("_path")) 18944 parseElementProperties(getJObject(json, "_path"), res.getPathElement()); 18945 if (json.has("sourceId")) 18946 res.setSourceIdElement(parseId(json.get("sourceId").getAsString())); 18947 if (json.has("_sourceId")) 18948 parseElementProperties(getJObject(json, "_sourceId"), res.getSourceIdElement()); 18949 } 18950 18951 protected TestScript.TestScriptRuleComponent parseTestScriptTestScriptRuleComponent(JsonObject json, TestScript owner) throws IOException, FHIRFormatError { 18952 TestScript.TestScriptRuleComponent res = new TestScript.TestScriptRuleComponent(); 18953 parseTestScriptTestScriptRuleComponentProperties(json, owner, res); 18954 return res; 18955 } 18956 18957 protected void parseTestScriptTestScriptRuleComponentProperties(JsonObject json, TestScript owner, TestScript.TestScriptRuleComponent res) throws IOException, FHIRFormatError { 18958 parseBackboneProperties(json, res); 18959 if (json.has("resource")) 18960 res.setResource(parseReference(getJObject(json, "resource"))); 18961 if (json.has("param")) { 18962 JsonArray array = json.getAsJsonArray("param"); 18963 for (int i = 0; i < array.size(); i++) { 18964 res.getParam().add(parseTestScriptRuleParamComponent(array.get(i).getAsJsonObject(), owner)); 18965 } 18966 }; 18967 } 18968 18969 protected TestScript.RuleParamComponent parseTestScriptRuleParamComponent(JsonObject json, TestScript owner) throws IOException, FHIRFormatError { 18970 TestScript.RuleParamComponent res = new TestScript.RuleParamComponent(); 18971 parseTestScriptRuleParamComponentProperties(json, owner, res); 18972 return res; 18973 } 18974 18975 protected void parseTestScriptRuleParamComponentProperties(JsonObject json, TestScript owner, TestScript.RuleParamComponent res) throws IOException, FHIRFormatError { 18976 parseBackboneProperties(json, res); 18977 if (json.has("name")) 18978 res.setNameElement(parseString(json.get("name").getAsString())); 18979 if (json.has("_name")) 18980 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 18981 if (json.has("value")) 18982 res.setValueElement(parseString(json.get("value").getAsString())); 18983 if (json.has("_value")) 18984 parseElementProperties(getJObject(json, "_value"), res.getValueElement()); 18985 } 18986 18987 protected TestScript.TestScriptRulesetComponent parseTestScriptTestScriptRulesetComponent(JsonObject json, TestScript owner) throws IOException, FHIRFormatError { 18988 TestScript.TestScriptRulesetComponent res = new TestScript.TestScriptRulesetComponent(); 18989 parseTestScriptTestScriptRulesetComponentProperties(json, owner, res); 18990 return res; 18991 } 18992 18993 protected void parseTestScriptTestScriptRulesetComponentProperties(JsonObject json, TestScript owner, TestScript.TestScriptRulesetComponent res) throws IOException, FHIRFormatError { 18994 parseBackboneProperties(json, res); 18995 if (json.has("resource")) 18996 res.setResource(parseReference(getJObject(json, "resource"))); 18997 if (json.has("rule")) { 18998 JsonArray array = json.getAsJsonArray("rule"); 18999 for (int i = 0; i < array.size(); i++) { 19000 res.getRule().add(parseTestScriptRulesetRuleComponent(array.get(i).getAsJsonObject(), owner)); 19001 } 19002 }; 19003 } 19004 19005 protected TestScript.RulesetRuleComponent parseTestScriptRulesetRuleComponent(JsonObject json, TestScript owner) throws IOException, FHIRFormatError { 19006 TestScript.RulesetRuleComponent res = new TestScript.RulesetRuleComponent(); 19007 parseTestScriptRulesetRuleComponentProperties(json, owner, res); 19008 return res; 19009 } 19010 19011 protected void parseTestScriptRulesetRuleComponentProperties(JsonObject json, TestScript owner, TestScript.RulesetRuleComponent res) throws IOException, FHIRFormatError { 19012 parseBackboneProperties(json, res); 19013 if (json.has("ruleId")) 19014 res.setRuleIdElement(parseId(json.get("ruleId").getAsString())); 19015 if (json.has("_ruleId")) 19016 parseElementProperties(getJObject(json, "_ruleId"), res.getRuleIdElement()); 19017 if (json.has("param")) { 19018 JsonArray array = json.getAsJsonArray("param"); 19019 for (int i = 0; i < array.size(); i++) { 19020 res.getParam().add(parseTestScriptRulesetRuleParamComponent(array.get(i).getAsJsonObject(), owner)); 19021 } 19022 }; 19023 } 19024 19025 protected TestScript.RulesetRuleParamComponent parseTestScriptRulesetRuleParamComponent(JsonObject json, TestScript owner) throws IOException, FHIRFormatError { 19026 TestScript.RulesetRuleParamComponent res = new TestScript.RulesetRuleParamComponent(); 19027 parseTestScriptRulesetRuleParamComponentProperties(json, owner, res); 19028 return res; 19029 } 19030 19031 protected void parseTestScriptRulesetRuleParamComponentProperties(JsonObject json, TestScript owner, TestScript.RulesetRuleParamComponent res) throws IOException, FHIRFormatError { 19032 parseBackboneProperties(json, res); 19033 if (json.has("name")) 19034 res.setNameElement(parseString(json.get("name").getAsString())); 19035 if (json.has("_name")) 19036 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 19037 if (json.has("value")) 19038 res.setValueElement(parseString(json.get("value").getAsString())); 19039 if (json.has("_value")) 19040 parseElementProperties(getJObject(json, "_value"), res.getValueElement()); 19041 } 19042 19043 protected TestScript.TestScriptSetupComponent parseTestScriptTestScriptSetupComponent(JsonObject json, TestScript owner) throws IOException, FHIRFormatError { 19044 TestScript.TestScriptSetupComponent res = new TestScript.TestScriptSetupComponent(); 19045 parseTestScriptTestScriptSetupComponentProperties(json, owner, res); 19046 return res; 19047 } 19048 19049 protected void parseTestScriptTestScriptSetupComponentProperties(JsonObject json, TestScript owner, TestScript.TestScriptSetupComponent res) throws IOException, FHIRFormatError { 19050 parseBackboneProperties(json, res); 19051 if (json.has("action")) { 19052 JsonArray array = json.getAsJsonArray("action"); 19053 for (int i = 0; i < array.size(); i++) { 19054 res.getAction().add(parseTestScriptSetupActionComponent(array.get(i).getAsJsonObject(), owner)); 19055 } 19056 }; 19057 } 19058 19059 protected TestScript.SetupActionComponent parseTestScriptSetupActionComponent(JsonObject json, TestScript owner) throws IOException, FHIRFormatError { 19060 TestScript.SetupActionComponent res = new TestScript.SetupActionComponent(); 19061 parseTestScriptSetupActionComponentProperties(json, owner, res); 19062 return res; 19063 } 19064 19065 protected void parseTestScriptSetupActionComponentProperties(JsonObject json, TestScript owner, TestScript.SetupActionComponent res) throws IOException, FHIRFormatError { 19066 parseBackboneProperties(json, res); 19067 if (json.has("operation")) 19068 res.setOperation(parseTestScriptSetupActionOperationComponent(getJObject(json, "operation"), owner)); 19069 if (json.has("assert")) 19070 res.setAssert(parseTestScriptSetupActionAssertComponent(getJObject(json, "assert"), owner)); 19071 } 19072 19073 protected TestScript.SetupActionOperationComponent parseTestScriptSetupActionOperationComponent(JsonObject json, TestScript owner) throws IOException, FHIRFormatError { 19074 TestScript.SetupActionOperationComponent res = new TestScript.SetupActionOperationComponent(); 19075 parseTestScriptSetupActionOperationComponentProperties(json, owner, res); 19076 return res; 19077 } 19078 19079 protected void parseTestScriptSetupActionOperationComponentProperties(JsonObject json, TestScript owner, TestScript.SetupActionOperationComponent res) throws IOException, FHIRFormatError { 19080 parseBackboneProperties(json, res); 19081 if (json.has("type")) 19082 res.setType(parseCoding(getJObject(json, "type"))); 19083 if (json.has("resource")) 19084 res.setResourceElement(parseCode(json.get("resource").getAsString())); 19085 if (json.has("_resource")) 19086 parseElementProperties(getJObject(json, "_resource"), res.getResourceElement()); 19087 if (json.has("label")) 19088 res.setLabelElement(parseString(json.get("label").getAsString())); 19089 if (json.has("_label")) 19090 parseElementProperties(getJObject(json, "_label"), res.getLabelElement()); 19091 if (json.has("description")) 19092 res.setDescriptionElement(parseString(json.get("description").getAsString())); 19093 if (json.has("_description")) 19094 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 19095 if (json.has("accept")) 19096 res.setAcceptElement(parseEnumeration(json.get("accept").getAsString(), TestScript.ContentType.NULL, new TestScript.ContentTypeEnumFactory())); 19097 if (json.has("_accept")) 19098 parseElementProperties(getJObject(json, "_accept"), res.getAcceptElement()); 19099 if (json.has("contentType")) 19100 res.setContentTypeElement(parseEnumeration(json.get("contentType").getAsString(), TestScript.ContentType.NULL, new TestScript.ContentTypeEnumFactory())); 19101 if (json.has("_contentType")) 19102 parseElementProperties(getJObject(json, "_contentType"), res.getContentTypeElement()); 19103 if (json.has("destination")) 19104 res.setDestinationElement(parseInteger(json.get("destination").getAsLong())); 19105 if (json.has("_destination")) 19106 parseElementProperties(getJObject(json, "_destination"), res.getDestinationElement()); 19107 if (json.has("encodeRequestUrl")) 19108 res.setEncodeRequestUrlElement(parseBoolean(json.get("encodeRequestUrl").getAsBoolean())); 19109 if (json.has("_encodeRequestUrl")) 19110 parseElementProperties(getJObject(json, "_encodeRequestUrl"), res.getEncodeRequestUrlElement()); 19111 if (json.has("origin")) 19112 res.setOriginElement(parseInteger(json.get("origin").getAsLong())); 19113 if (json.has("_origin")) 19114 parseElementProperties(getJObject(json, "_origin"), res.getOriginElement()); 19115 if (json.has("params")) 19116 res.setParamsElement(parseString(json.get("params").getAsString())); 19117 if (json.has("_params")) 19118 parseElementProperties(getJObject(json, "_params"), res.getParamsElement()); 19119 if (json.has("requestHeader")) { 19120 JsonArray array = json.getAsJsonArray("requestHeader"); 19121 for (int i = 0; i < array.size(); i++) { 19122 res.getRequestHeader().add(parseTestScriptSetupActionOperationRequestHeaderComponent(array.get(i).getAsJsonObject(), owner)); 19123 } 19124 }; 19125 if (json.has("requestId")) 19126 res.setRequestIdElement(parseId(json.get("requestId").getAsString())); 19127 if (json.has("_requestId")) 19128 parseElementProperties(getJObject(json, "_requestId"), res.getRequestIdElement()); 19129 if (json.has("responseId")) 19130 res.setResponseIdElement(parseId(json.get("responseId").getAsString())); 19131 if (json.has("_responseId")) 19132 parseElementProperties(getJObject(json, "_responseId"), res.getResponseIdElement()); 19133 if (json.has("sourceId")) 19134 res.setSourceIdElement(parseId(json.get("sourceId").getAsString())); 19135 if (json.has("_sourceId")) 19136 parseElementProperties(getJObject(json, "_sourceId"), res.getSourceIdElement()); 19137 if (json.has("targetId")) 19138 res.setTargetIdElement(parseId(json.get("targetId").getAsString())); 19139 if (json.has("_targetId")) 19140 parseElementProperties(getJObject(json, "_targetId"), res.getTargetIdElement()); 19141 if (json.has("url")) 19142 res.setUrlElement(parseString(json.get("url").getAsString())); 19143 if (json.has("_url")) 19144 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 19145 } 19146 19147 protected TestScript.SetupActionOperationRequestHeaderComponent parseTestScriptSetupActionOperationRequestHeaderComponent(JsonObject json, TestScript owner) throws IOException, FHIRFormatError { 19148 TestScript.SetupActionOperationRequestHeaderComponent res = new TestScript.SetupActionOperationRequestHeaderComponent(); 19149 parseTestScriptSetupActionOperationRequestHeaderComponentProperties(json, owner, res); 19150 return res; 19151 } 19152 19153 protected void parseTestScriptSetupActionOperationRequestHeaderComponentProperties(JsonObject json, TestScript owner, TestScript.SetupActionOperationRequestHeaderComponent res) throws IOException, FHIRFormatError { 19154 parseBackboneProperties(json, res); 19155 if (json.has("field")) 19156 res.setFieldElement(parseString(json.get("field").getAsString())); 19157 if (json.has("_field")) 19158 parseElementProperties(getJObject(json, "_field"), res.getFieldElement()); 19159 if (json.has("value")) 19160 res.setValueElement(parseString(json.get("value").getAsString())); 19161 if (json.has("_value")) 19162 parseElementProperties(getJObject(json, "_value"), res.getValueElement()); 19163 } 19164 19165 protected TestScript.SetupActionAssertComponent parseTestScriptSetupActionAssertComponent(JsonObject json, TestScript owner) throws IOException, FHIRFormatError { 19166 TestScript.SetupActionAssertComponent res = new TestScript.SetupActionAssertComponent(); 19167 parseTestScriptSetupActionAssertComponentProperties(json, owner, res); 19168 return res; 19169 } 19170 19171 protected void parseTestScriptSetupActionAssertComponentProperties(JsonObject json, TestScript owner, TestScript.SetupActionAssertComponent res) throws IOException, FHIRFormatError { 19172 parseBackboneProperties(json, res); 19173 if (json.has("label")) 19174 res.setLabelElement(parseString(json.get("label").getAsString())); 19175 if (json.has("_label")) 19176 parseElementProperties(getJObject(json, "_label"), res.getLabelElement()); 19177 if (json.has("description")) 19178 res.setDescriptionElement(parseString(json.get("description").getAsString())); 19179 if (json.has("_description")) 19180 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 19181 if (json.has("direction")) 19182 res.setDirectionElement(parseEnumeration(json.get("direction").getAsString(), TestScript.AssertionDirectionType.NULL, new TestScript.AssertionDirectionTypeEnumFactory())); 19183 if (json.has("_direction")) 19184 parseElementProperties(getJObject(json, "_direction"), res.getDirectionElement()); 19185 if (json.has("compareToSourceId")) 19186 res.setCompareToSourceIdElement(parseString(json.get("compareToSourceId").getAsString())); 19187 if (json.has("_compareToSourceId")) 19188 parseElementProperties(getJObject(json, "_compareToSourceId"), res.getCompareToSourceIdElement()); 19189 if (json.has("compareToSourceExpression")) 19190 res.setCompareToSourceExpressionElement(parseString(json.get("compareToSourceExpression").getAsString())); 19191 if (json.has("_compareToSourceExpression")) 19192 parseElementProperties(getJObject(json, "_compareToSourceExpression"), res.getCompareToSourceExpressionElement()); 19193 if (json.has("compareToSourcePath")) 19194 res.setCompareToSourcePathElement(parseString(json.get("compareToSourcePath").getAsString())); 19195 if (json.has("_compareToSourcePath")) 19196 parseElementProperties(getJObject(json, "_compareToSourcePath"), res.getCompareToSourcePathElement()); 19197 if (json.has("contentType")) 19198 res.setContentTypeElement(parseEnumeration(json.get("contentType").getAsString(), TestScript.ContentType.NULL, new TestScript.ContentTypeEnumFactory())); 19199 if (json.has("_contentType")) 19200 parseElementProperties(getJObject(json, "_contentType"), res.getContentTypeElement()); 19201 if (json.has("expression")) 19202 res.setExpressionElement(parseString(json.get("expression").getAsString())); 19203 if (json.has("_expression")) 19204 parseElementProperties(getJObject(json, "_expression"), res.getExpressionElement()); 19205 if (json.has("headerField")) 19206 res.setHeaderFieldElement(parseString(json.get("headerField").getAsString())); 19207 if (json.has("_headerField")) 19208 parseElementProperties(getJObject(json, "_headerField"), res.getHeaderFieldElement()); 19209 if (json.has("minimumId")) 19210 res.setMinimumIdElement(parseString(json.get("minimumId").getAsString())); 19211 if (json.has("_minimumId")) 19212 parseElementProperties(getJObject(json, "_minimumId"), res.getMinimumIdElement()); 19213 if (json.has("navigationLinks")) 19214 res.setNavigationLinksElement(parseBoolean(json.get("navigationLinks").getAsBoolean())); 19215 if (json.has("_navigationLinks")) 19216 parseElementProperties(getJObject(json, "_navigationLinks"), res.getNavigationLinksElement()); 19217 if (json.has("operator")) 19218 res.setOperatorElement(parseEnumeration(json.get("operator").getAsString(), TestScript.AssertionOperatorType.NULL, new TestScript.AssertionOperatorTypeEnumFactory())); 19219 if (json.has("_operator")) 19220 parseElementProperties(getJObject(json, "_operator"), res.getOperatorElement()); 19221 if (json.has("path")) 19222 res.setPathElement(parseString(json.get("path").getAsString())); 19223 if (json.has("_path")) 19224 parseElementProperties(getJObject(json, "_path"), res.getPathElement()); 19225 if (json.has("requestMethod")) 19226 res.setRequestMethodElement(parseEnumeration(json.get("requestMethod").getAsString(), TestScript.TestScriptRequestMethodCode.NULL, new TestScript.TestScriptRequestMethodCodeEnumFactory())); 19227 if (json.has("_requestMethod")) 19228 parseElementProperties(getJObject(json, "_requestMethod"), res.getRequestMethodElement()); 19229 if (json.has("requestURL")) 19230 res.setRequestURLElement(parseString(json.get("requestURL").getAsString())); 19231 if (json.has("_requestURL")) 19232 parseElementProperties(getJObject(json, "_requestURL"), res.getRequestURLElement()); 19233 if (json.has("resource")) 19234 res.setResourceElement(parseCode(json.get("resource").getAsString())); 19235 if (json.has("_resource")) 19236 parseElementProperties(getJObject(json, "_resource"), res.getResourceElement()); 19237 if (json.has("response")) 19238 res.setResponseElement(parseEnumeration(json.get("response").getAsString(), TestScript.AssertionResponseTypes.NULL, new TestScript.AssertionResponseTypesEnumFactory())); 19239 if (json.has("_response")) 19240 parseElementProperties(getJObject(json, "_response"), res.getResponseElement()); 19241 if (json.has("responseCode")) 19242 res.setResponseCodeElement(parseString(json.get("responseCode").getAsString())); 19243 if (json.has("_responseCode")) 19244 parseElementProperties(getJObject(json, "_responseCode"), res.getResponseCodeElement()); 19245 if (json.has("rule")) 19246 res.setRule(parseTestScriptActionAssertRuleComponent(getJObject(json, "rule"), owner)); 19247 if (json.has("ruleset")) 19248 res.setRuleset(parseTestScriptActionAssertRulesetComponent(getJObject(json, "ruleset"), owner)); 19249 if (json.has("sourceId")) 19250 res.setSourceIdElement(parseId(json.get("sourceId").getAsString())); 19251 if (json.has("_sourceId")) 19252 parseElementProperties(getJObject(json, "_sourceId"), res.getSourceIdElement()); 19253 if (json.has("validateProfileId")) 19254 res.setValidateProfileIdElement(parseId(json.get("validateProfileId").getAsString())); 19255 if (json.has("_validateProfileId")) 19256 parseElementProperties(getJObject(json, "_validateProfileId"), res.getValidateProfileIdElement()); 19257 if (json.has("value")) 19258 res.setValueElement(parseString(json.get("value").getAsString())); 19259 if (json.has("_value")) 19260 parseElementProperties(getJObject(json, "_value"), res.getValueElement()); 19261 if (json.has("warningOnly")) 19262 res.setWarningOnlyElement(parseBoolean(json.get("warningOnly").getAsBoolean())); 19263 if (json.has("_warningOnly")) 19264 parseElementProperties(getJObject(json, "_warningOnly"), res.getWarningOnlyElement()); 19265 } 19266 19267 protected TestScript.ActionAssertRuleComponent parseTestScriptActionAssertRuleComponent(JsonObject json, TestScript owner) throws IOException, FHIRFormatError { 19268 TestScript.ActionAssertRuleComponent res = new TestScript.ActionAssertRuleComponent(); 19269 parseTestScriptActionAssertRuleComponentProperties(json, owner, res); 19270 return res; 19271 } 19272 19273 protected void parseTestScriptActionAssertRuleComponentProperties(JsonObject json, TestScript owner, TestScript.ActionAssertRuleComponent res) throws IOException, FHIRFormatError { 19274 parseBackboneProperties(json, res); 19275 if (json.has("ruleId")) 19276 res.setRuleIdElement(parseId(json.get("ruleId").getAsString())); 19277 if (json.has("_ruleId")) 19278 parseElementProperties(getJObject(json, "_ruleId"), res.getRuleIdElement()); 19279 if (json.has("param")) { 19280 JsonArray array = json.getAsJsonArray("param"); 19281 for (int i = 0; i < array.size(); i++) { 19282 res.getParam().add(parseTestScriptActionAssertRuleParamComponent(array.get(i).getAsJsonObject(), owner)); 19283 } 19284 }; 19285 } 19286 19287 protected TestScript.ActionAssertRuleParamComponent parseTestScriptActionAssertRuleParamComponent(JsonObject json, TestScript owner) throws IOException, FHIRFormatError { 19288 TestScript.ActionAssertRuleParamComponent res = new TestScript.ActionAssertRuleParamComponent(); 19289 parseTestScriptActionAssertRuleParamComponentProperties(json, owner, res); 19290 return res; 19291 } 19292 19293 protected void parseTestScriptActionAssertRuleParamComponentProperties(JsonObject json, TestScript owner, TestScript.ActionAssertRuleParamComponent res) throws IOException, FHIRFormatError { 19294 parseBackboneProperties(json, res); 19295 if (json.has("name")) 19296 res.setNameElement(parseString(json.get("name").getAsString())); 19297 if (json.has("_name")) 19298 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 19299 if (json.has("value")) 19300 res.setValueElement(parseString(json.get("value").getAsString())); 19301 if (json.has("_value")) 19302 parseElementProperties(getJObject(json, "_value"), res.getValueElement()); 19303 } 19304 19305 protected TestScript.ActionAssertRulesetComponent parseTestScriptActionAssertRulesetComponent(JsonObject json, TestScript owner) throws IOException, FHIRFormatError { 19306 TestScript.ActionAssertRulesetComponent res = new TestScript.ActionAssertRulesetComponent(); 19307 parseTestScriptActionAssertRulesetComponentProperties(json, owner, res); 19308 return res; 19309 } 19310 19311 protected void parseTestScriptActionAssertRulesetComponentProperties(JsonObject json, TestScript owner, TestScript.ActionAssertRulesetComponent res) throws IOException, FHIRFormatError { 19312 parseBackboneProperties(json, res); 19313 if (json.has("rulesetId")) 19314 res.setRulesetIdElement(parseId(json.get("rulesetId").getAsString())); 19315 if (json.has("_rulesetId")) 19316 parseElementProperties(getJObject(json, "_rulesetId"), res.getRulesetIdElement()); 19317 if (json.has("rule")) { 19318 JsonArray array = json.getAsJsonArray("rule"); 19319 for (int i = 0; i < array.size(); i++) { 19320 res.getRule().add(parseTestScriptActionAssertRulesetRuleComponent(array.get(i).getAsJsonObject(), owner)); 19321 } 19322 }; 19323 } 19324 19325 protected TestScript.ActionAssertRulesetRuleComponent parseTestScriptActionAssertRulesetRuleComponent(JsonObject json, TestScript owner) throws IOException, FHIRFormatError { 19326 TestScript.ActionAssertRulesetRuleComponent res = new TestScript.ActionAssertRulesetRuleComponent(); 19327 parseTestScriptActionAssertRulesetRuleComponentProperties(json, owner, res); 19328 return res; 19329 } 19330 19331 protected void parseTestScriptActionAssertRulesetRuleComponentProperties(JsonObject json, TestScript owner, TestScript.ActionAssertRulesetRuleComponent res) throws IOException, FHIRFormatError { 19332 parseBackboneProperties(json, res); 19333 if (json.has("ruleId")) 19334 res.setRuleIdElement(parseId(json.get("ruleId").getAsString())); 19335 if (json.has("_ruleId")) 19336 parseElementProperties(getJObject(json, "_ruleId"), res.getRuleIdElement()); 19337 if (json.has("param")) { 19338 JsonArray array = json.getAsJsonArray("param"); 19339 for (int i = 0; i < array.size(); i++) { 19340 res.getParam().add(parseTestScriptActionAssertRulesetRuleParamComponent(array.get(i).getAsJsonObject(), owner)); 19341 } 19342 }; 19343 } 19344 19345 protected TestScript.ActionAssertRulesetRuleParamComponent parseTestScriptActionAssertRulesetRuleParamComponent(JsonObject json, TestScript owner) throws IOException, FHIRFormatError { 19346 TestScript.ActionAssertRulesetRuleParamComponent res = new TestScript.ActionAssertRulesetRuleParamComponent(); 19347 parseTestScriptActionAssertRulesetRuleParamComponentProperties(json, owner, res); 19348 return res; 19349 } 19350 19351 protected void parseTestScriptActionAssertRulesetRuleParamComponentProperties(JsonObject json, TestScript owner, TestScript.ActionAssertRulesetRuleParamComponent res) throws IOException, FHIRFormatError { 19352 parseBackboneProperties(json, res); 19353 if (json.has("name")) 19354 res.setNameElement(parseString(json.get("name").getAsString())); 19355 if (json.has("_name")) 19356 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 19357 if (json.has("value")) 19358 res.setValueElement(parseString(json.get("value").getAsString())); 19359 if (json.has("_value")) 19360 parseElementProperties(getJObject(json, "_value"), res.getValueElement()); 19361 } 19362 19363 protected TestScript.TestScriptTestComponent parseTestScriptTestScriptTestComponent(JsonObject json, TestScript owner) throws IOException, FHIRFormatError { 19364 TestScript.TestScriptTestComponent res = new TestScript.TestScriptTestComponent(); 19365 parseTestScriptTestScriptTestComponentProperties(json, owner, res); 19366 return res; 19367 } 19368 19369 protected void parseTestScriptTestScriptTestComponentProperties(JsonObject json, TestScript owner, TestScript.TestScriptTestComponent res) throws IOException, FHIRFormatError { 19370 parseBackboneProperties(json, res); 19371 if (json.has("name")) 19372 res.setNameElement(parseString(json.get("name").getAsString())); 19373 if (json.has("_name")) 19374 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 19375 if (json.has("description")) 19376 res.setDescriptionElement(parseString(json.get("description").getAsString())); 19377 if (json.has("_description")) 19378 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 19379 if (json.has("action")) { 19380 JsonArray array = json.getAsJsonArray("action"); 19381 for (int i = 0; i < array.size(); i++) { 19382 res.getAction().add(parseTestScriptTestActionComponent(array.get(i).getAsJsonObject(), owner)); 19383 } 19384 }; 19385 } 19386 19387 protected TestScript.TestActionComponent parseTestScriptTestActionComponent(JsonObject json, TestScript owner) throws IOException, FHIRFormatError { 19388 TestScript.TestActionComponent res = new TestScript.TestActionComponent(); 19389 parseTestScriptTestActionComponentProperties(json, owner, res); 19390 return res; 19391 } 19392 19393 protected void parseTestScriptTestActionComponentProperties(JsonObject json, TestScript owner, TestScript.TestActionComponent res) throws IOException, FHIRFormatError { 19394 parseBackboneProperties(json, res); 19395 if (json.has("operation")) 19396 res.setOperation(parseTestScriptSetupActionOperationComponent(getJObject(json, "operation"), owner)); 19397 if (json.has("assert")) 19398 res.setAssert(parseTestScriptSetupActionAssertComponent(getJObject(json, "assert"), owner)); 19399 } 19400 19401 protected TestScript.TestScriptTeardownComponent parseTestScriptTestScriptTeardownComponent(JsonObject json, TestScript owner) throws IOException, FHIRFormatError { 19402 TestScript.TestScriptTeardownComponent res = new TestScript.TestScriptTeardownComponent(); 19403 parseTestScriptTestScriptTeardownComponentProperties(json, owner, res); 19404 return res; 19405 } 19406 19407 protected void parseTestScriptTestScriptTeardownComponentProperties(JsonObject json, TestScript owner, TestScript.TestScriptTeardownComponent res) throws IOException, FHIRFormatError { 19408 parseBackboneProperties(json, res); 19409 if (json.has("action")) { 19410 JsonArray array = json.getAsJsonArray("action"); 19411 for (int i = 0; i < array.size(); i++) { 19412 res.getAction().add(parseTestScriptTeardownActionComponent(array.get(i).getAsJsonObject(), owner)); 19413 } 19414 }; 19415 } 19416 19417 protected TestScript.TeardownActionComponent parseTestScriptTeardownActionComponent(JsonObject json, TestScript owner) throws IOException, FHIRFormatError { 19418 TestScript.TeardownActionComponent res = new TestScript.TeardownActionComponent(); 19419 parseTestScriptTeardownActionComponentProperties(json, owner, res); 19420 return res; 19421 } 19422 19423 protected void parseTestScriptTeardownActionComponentProperties(JsonObject json, TestScript owner, TestScript.TeardownActionComponent res) throws IOException, FHIRFormatError { 19424 parseBackboneProperties(json, res); 19425 if (json.has("operation")) 19426 res.setOperation(parseTestScriptSetupActionOperationComponent(getJObject(json, "operation"), owner)); 19427 } 19428 19429 protected ValueSet parseValueSet(JsonObject json) throws IOException, FHIRFormatError { 19430 ValueSet res = new ValueSet(); 19431 parseValueSetProperties(json, res); 19432 return res; 19433 } 19434 19435 protected void parseValueSetProperties(JsonObject json, ValueSet res) throws IOException, FHIRFormatError { 19436 parseDomainResourceProperties(json, res); 19437 if (json.has("url")) 19438 res.setUrlElement(parseUri(json.get("url").getAsString())); 19439 if (json.has("_url")) 19440 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 19441 if (json.has("identifier")) { 19442 JsonArray array = json.getAsJsonArray("identifier"); 19443 for (int i = 0; i < array.size(); i++) { 19444 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 19445 } 19446 }; 19447 if (json.has("version")) 19448 res.setVersionElement(parseString(json.get("version").getAsString())); 19449 if (json.has("_version")) 19450 parseElementProperties(getJObject(json, "_version"), res.getVersionElement()); 19451 if (json.has("name")) 19452 res.setNameElement(parseString(json.get("name").getAsString())); 19453 if (json.has("_name")) 19454 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 19455 if (json.has("title")) 19456 res.setTitleElement(parseString(json.get("title").getAsString())); 19457 if (json.has("_title")) 19458 parseElementProperties(getJObject(json, "_title"), res.getTitleElement()); 19459 if (json.has("status")) 19460 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Enumerations.PublicationStatus.NULL, new Enumerations.PublicationStatusEnumFactory())); 19461 if (json.has("_status")) 19462 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 19463 if (json.has("experimental")) 19464 res.setExperimentalElement(parseBoolean(json.get("experimental").getAsBoolean())); 19465 if (json.has("_experimental")) 19466 parseElementProperties(getJObject(json, "_experimental"), res.getExperimentalElement()); 19467 if (json.has("date")) 19468 res.setDateElement(parseDateTime(json.get("date").getAsString())); 19469 if (json.has("_date")) 19470 parseElementProperties(getJObject(json, "_date"), res.getDateElement()); 19471 if (json.has("publisher")) 19472 res.setPublisherElement(parseString(json.get("publisher").getAsString())); 19473 if (json.has("_publisher")) 19474 parseElementProperties(getJObject(json, "_publisher"), res.getPublisherElement()); 19475 if (json.has("contact")) { 19476 JsonArray array = json.getAsJsonArray("contact"); 19477 for (int i = 0; i < array.size(); i++) { 19478 res.getContact().add(parseContactDetail(array.get(i).getAsJsonObject())); 19479 } 19480 }; 19481 if (json.has("description")) 19482 res.setDescriptionElement(parseMarkdown(json.get("description").getAsString())); 19483 if (json.has("_description")) 19484 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 19485 if (json.has("useContext")) { 19486 JsonArray array = json.getAsJsonArray("useContext"); 19487 for (int i = 0; i < array.size(); i++) { 19488 res.getUseContext().add(parseUsageContext(array.get(i).getAsJsonObject())); 19489 } 19490 }; 19491 if (json.has("jurisdiction")) { 19492 JsonArray array = json.getAsJsonArray("jurisdiction"); 19493 for (int i = 0; i < array.size(); i++) { 19494 res.getJurisdiction().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 19495 } 19496 }; 19497 if (json.has("immutable")) 19498 res.setImmutableElement(parseBoolean(json.get("immutable").getAsBoolean())); 19499 if (json.has("_immutable")) 19500 parseElementProperties(getJObject(json, "_immutable"), res.getImmutableElement()); 19501 if (json.has("purpose")) 19502 res.setPurposeElement(parseMarkdown(json.get("purpose").getAsString())); 19503 if (json.has("_purpose")) 19504 parseElementProperties(getJObject(json, "_purpose"), res.getPurposeElement()); 19505 if (json.has("copyright")) 19506 res.setCopyrightElement(parseMarkdown(json.get("copyright").getAsString())); 19507 if (json.has("_copyright")) 19508 parseElementProperties(getJObject(json, "_copyright"), res.getCopyrightElement()); 19509 if (json.has("extensible")) 19510 res.setExtensibleElement(parseBoolean(json.get("extensible").getAsBoolean())); 19511 if (json.has("_extensible")) 19512 parseElementProperties(getJObject(json, "_extensible"), res.getExtensibleElement()); 19513 if (json.has("compose")) 19514 res.setCompose(parseValueSetValueSetComposeComponent(getJObject(json, "compose"), res)); 19515 if (json.has("expansion")) 19516 res.setExpansion(parseValueSetValueSetExpansionComponent(getJObject(json, "expansion"), res)); 19517 } 19518 19519 protected ValueSet.ValueSetComposeComponent parseValueSetValueSetComposeComponent(JsonObject json, ValueSet owner) throws IOException, FHIRFormatError { 19520 ValueSet.ValueSetComposeComponent res = new ValueSet.ValueSetComposeComponent(); 19521 parseValueSetValueSetComposeComponentProperties(json, owner, res); 19522 return res; 19523 } 19524 19525 protected void parseValueSetValueSetComposeComponentProperties(JsonObject json, ValueSet owner, ValueSet.ValueSetComposeComponent res) throws IOException, FHIRFormatError { 19526 parseBackboneProperties(json, res); 19527 if (json.has("lockedDate")) 19528 res.setLockedDateElement(parseDate(json.get("lockedDate").getAsString())); 19529 if (json.has("_lockedDate")) 19530 parseElementProperties(getJObject(json, "_lockedDate"), res.getLockedDateElement()); 19531 if (json.has("inactive")) 19532 res.setInactiveElement(parseBoolean(json.get("inactive").getAsBoolean())); 19533 if (json.has("_inactive")) 19534 parseElementProperties(getJObject(json, "_inactive"), res.getInactiveElement()); 19535 if (json.has("include")) { 19536 JsonArray array = json.getAsJsonArray("include"); 19537 for (int i = 0; i < array.size(); i++) { 19538 res.getInclude().add(parseValueSetConceptSetComponent(array.get(i).getAsJsonObject(), owner)); 19539 } 19540 }; 19541 if (json.has("exclude")) { 19542 JsonArray array = json.getAsJsonArray("exclude"); 19543 for (int i = 0; i < array.size(); i++) { 19544 res.getExclude().add(parseValueSetConceptSetComponent(array.get(i).getAsJsonObject(), owner)); 19545 } 19546 }; 19547 } 19548 19549 protected ValueSet.ConceptSetComponent parseValueSetConceptSetComponent(JsonObject json, ValueSet owner) throws IOException, FHIRFormatError { 19550 ValueSet.ConceptSetComponent res = new ValueSet.ConceptSetComponent(); 19551 parseValueSetConceptSetComponentProperties(json, owner, res); 19552 return res; 19553 } 19554 19555 protected void parseValueSetConceptSetComponentProperties(JsonObject json, ValueSet owner, ValueSet.ConceptSetComponent res) throws IOException, FHIRFormatError { 19556 parseBackboneProperties(json, res); 19557 if (json.has("system")) 19558 res.setSystemElement(parseUri(json.get("system").getAsString())); 19559 if (json.has("_system")) 19560 parseElementProperties(getJObject(json, "_system"), res.getSystemElement()); 19561 if (json.has("version")) 19562 res.setVersionElement(parseString(json.get("version").getAsString())); 19563 if (json.has("_version")) 19564 parseElementProperties(getJObject(json, "_version"), res.getVersionElement()); 19565 if (json.has("concept")) { 19566 JsonArray array = json.getAsJsonArray("concept"); 19567 for (int i = 0; i < array.size(); i++) { 19568 res.getConcept().add(parseValueSetConceptReferenceComponent(array.get(i).getAsJsonObject(), owner)); 19569 } 19570 }; 19571 if (json.has("filter")) { 19572 JsonArray array = json.getAsJsonArray("filter"); 19573 for (int i = 0; i < array.size(); i++) { 19574 res.getFilter().add(parseValueSetConceptSetFilterComponent(array.get(i).getAsJsonObject(), owner)); 19575 } 19576 }; 19577 if (json.has("valueSet")) { 19578 JsonArray array = json.getAsJsonArray("valueSet"); 19579 for (int i = 0; i < array.size(); i++) { 19580 if (array.get(i).isJsonNull()) { 19581 res.getValueSet().add(new UriType()); 19582 } else { 19583 res.getValueSet().add(parseUri(array.get(i).getAsString())); 19584 } 19585 } 19586 }; 19587 if (json.has("_valueSet")) { 19588 JsonArray array = json.getAsJsonArray("_valueSet"); 19589 for (int i = 0; i < array.size(); i++) { 19590 if (i == res.getValueSet().size()) 19591 res.getValueSet().add(parseUri(null)); 19592 if (array.get(i) instanceof JsonObject) 19593 parseElementProperties(array.get(i).getAsJsonObject(), res.getValueSet().get(i)); 19594 } 19595 }; 19596 } 19597 19598 protected ValueSet.ConceptReferenceComponent parseValueSetConceptReferenceComponent(JsonObject json, ValueSet owner) throws IOException, FHIRFormatError { 19599 ValueSet.ConceptReferenceComponent res = new ValueSet.ConceptReferenceComponent(); 19600 parseValueSetConceptReferenceComponentProperties(json, owner, res); 19601 return res; 19602 } 19603 19604 protected void parseValueSetConceptReferenceComponentProperties(JsonObject json, ValueSet owner, ValueSet.ConceptReferenceComponent res) throws IOException, FHIRFormatError { 19605 parseBackboneProperties(json, res); 19606 if (json.has("code")) 19607 res.setCodeElement(parseCode(json.get("code").getAsString())); 19608 if (json.has("_code")) 19609 parseElementProperties(getJObject(json, "_code"), res.getCodeElement()); 19610 if (json.has("display")) 19611 res.setDisplayElement(parseString(json.get("display").getAsString())); 19612 if (json.has("_display")) 19613 parseElementProperties(getJObject(json, "_display"), res.getDisplayElement()); 19614 if (json.has("designation")) { 19615 JsonArray array = json.getAsJsonArray("designation"); 19616 for (int i = 0; i < array.size(); i++) { 19617 res.getDesignation().add(parseValueSetConceptReferenceDesignationComponent(array.get(i).getAsJsonObject(), owner)); 19618 } 19619 }; 19620 } 19621 19622 protected ValueSet.ConceptReferenceDesignationComponent parseValueSetConceptReferenceDesignationComponent(JsonObject json, ValueSet owner) throws IOException, FHIRFormatError { 19623 ValueSet.ConceptReferenceDesignationComponent res = new ValueSet.ConceptReferenceDesignationComponent(); 19624 parseValueSetConceptReferenceDesignationComponentProperties(json, owner, res); 19625 return res; 19626 } 19627 19628 protected void parseValueSetConceptReferenceDesignationComponentProperties(JsonObject json, ValueSet owner, ValueSet.ConceptReferenceDesignationComponent res) throws IOException, FHIRFormatError { 19629 parseBackboneProperties(json, res); 19630 if (json.has("language")) 19631 res.setLanguageElement(parseCode(json.get("language").getAsString())); 19632 if (json.has("_language")) 19633 parseElementProperties(getJObject(json, "_language"), res.getLanguageElement()); 19634 if (json.has("use")) 19635 res.setUse(parseCoding(getJObject(json, "use"))); 19636 if (json.has("value")) 19637 res.setValueElement(parseString(json.get("value").getAsString())); 19638 if (json.has("_value")) 19639 parseElementProperties(getJObject(json, "_value"), res.getValueElement()); 19640 } 19641 19642 protected ValueSet.ConceptSetFilterComponent parseValueSetConceptSetFilterComponent(JsonObject json, ValueSet owner) throws IOException, FHIRFormatError { 19643 ValueSet.ConceptSetFilterComponent res = new ValueSet.ConceptSetFilterComponent(); 19644 parseValueSetConceptSetFilterComponentProperties(json, owner, res); 19645 return res; 19646 } 19647 19648 protected void parseValueSetConceptSetFilterComponentProperties(JsonObject json, ValueSet owner, ValueSet.ConceptSetFilterComponent res) throws IOException, FHIRFormatError { 19649 parseBackboneProperties(json, res); 19650 if (json.has("property")) 19651 res.setPropertyElement(parseCode(json.get("property").getAsString())); 19652 if (json.has("_property")) 19653 parseElementProperties(getJObject(json, "_property"), res.getPropertyElement()); 19654 if (json.has("op")) 19655 res.setOpElement(parseEnumeration(json.get("op").getAsString(), ValueSet.FilterOperator.NULL, new ValueSet.FilterOperatorEnumFactory())); 19656 if (json.has("_op")) 19657 parseElementProperties(getJObject(json, "_op"), res.getOpElement()); 19658 if (json.has("value")) 19659 res.setValueElement(parseCode(json.get("value").getAsString())); 19660 if (json.has("_value")) 19661 parseElementProperties(getJObject(json, "_value"), res.getValueElement()); 19662 } 19663 19664 protected ValueSet.ValueSetExpansionComponent parseValueSetValueSetExpansionComponent(JsonObject json, ValueSet owner) throws IOException, FHIRFormatError { 19665 ValueSet.ValueSetExpansionComponent res = new ValueSet.ValueSetExpansionComponent(); 19666 parseValueSetValueSetExpansionComponentProperties(json, owner, res); 19667 return res; 19668 } 19669 19670 protected void parseValueSetValueSetExpansionComponentProperties(JsonObject json, ValueSet owner, ValueSet.ValueSetExpansionComponent res) throws IOException, FHIRFormatError { 19671 parseBackboneProperties(json, res); 19672 if (json.has("identifier")) 19673 res.setIdentifierElement(parseUri(json.get("identifier").getAsString())); 19674 if (json.has("_identifier")) 19675 parseElementProperties(getJObject(json, "_identifier"), res.getIdentifierElement()); 19676 if (json.has("timestamp")) 19677 res.setTimestampElement(parseDateTime(json.get("timestamp").getAsString())); 19678 if (json.has("_timestamp")) 19679 parseElementProperties(getJObject(json, "_timestamp"), res.getTimestampElement()); 19680 if (json.has("total")) 19681 res.setTotalElement(parseInteger(json.get("total").getAsLong())); 19682 if (json.has("_total")) 19683 parseElementProperties(getJObject(json, "_total"), res.getTotalElement()); 19684 if (json.has("offset")) 19685 res.setOffsetElement(parseInteger(json.get("offset").getAsLong())); 19686 if (json.has("_offset")) 19687 parseElementProperties(getJObject(json, "_offset"), res.getOffsetElement()); 19688 if (json.has("parameter")) { 19689 JsonArray array = json.getAsJsonArray("parameter"); 19690 for (int i = 0; i < array.size(); i++) { 19691 res.getParameter().add(parseValueSetValueSetExpansionParameterComponent(array.get(i).getAsJsonObject(), owner)); 19692 } 19693 }; 19694 if (json.has("contains")) { 19695 JsonArray array = json.getAsJsonArray("contains"); 19696 for (int i = 0; i < array.size(); i++) { 19697 res.getContains().add(parseValueSetValueSetExpansionContainsComponent(array.get(i).getAsJsonObject(), owner)); 19698 } 19699 }; 19700 } 19701 19702 protected ValueSet.ValueSetExpansionParameterComponent parseValueSetValueSetExpansionParameterComponent(JsonObject json, ValueSet owner) throws IOException, FHIRFormatError { 19703 ValueSet.ValueSetExpansionParameterComponent res = new ValueSet.ValueSetExpansionParameterComponent(); 19704 parseValueSetValueSetExpansionParameterComponentProperties(json, owner, res); 19705 return res; 19706 } 19707 19708 protected void parseValueSetValueSetExpansionParameterComponentProperties(JsonObject json, ValueSet owner, ValueSet.ValueSetExpansionParameterComponent res) throws IOException, FHIRFormatError { 19709 parseBackboneProperties(json, res); 19710 if (json.has("name")) 19711 res.setNameElement(parseString(json.get("name").getAsString())); 19712 if (json.has("_name")) 19713 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 19714 Type value = parseType("value", json); 19715 if (value != null) 19716 res.setValue(value); 19717 } 19718 19719 protected ValueSet.ValueSetExpansionContainsComponent parseValueSetValueSetExpansionContainsComponent(JsonObject json, ValueSet owner) throws IOException, FHIRFormatError { 19720 ValueSet.ValueSetExpansionContainsComponent res = new ValueSet.ValueSetExpansionContainsComponent(); 19721 parseValueSetValueSetExpansionContainsComponentProperties(json, owner, res); 19722 return res; 19723 } 19724 19725 protected void parseValueSetValueSetExpansionContainsComponentProperties(JsonObject json, ValueSet owner, ValueSet.ValueSetExpansionContainsComponent res) throws IOException, FHIRFormatError { 19726 parseBackboneProperties(json, res); 19727 if (json.has("system")) 19728 res.setSystemElement(parseUri(json.get("system").getAsString())); 19729 if (json.has("_system")) 19730 parseElementProperties(getJObject(json, "_system"), res.getSystemElement()); 19731 if (json.has("abstract")) 19732 res.setAbstractElement(parseBoolean(json.get("abstract").getAsBoolean())); 19733 if (json.has("_abstract")) 19734 parseElementProperties(getJObject(json, "_abstract"), res.getAbstractElement()); 19735 if (json.has("inactive")) 19736 res.setInactiveElement(parseBoolean(json.get("inactive").getAsBoolean())); 19737 if (json.has("_inactive")) 19738 parseElementProperties(getJObject(json, "_inactive"), res.getInactiveElement()); 19739 if (json.has("version")) 19740 res.setVersionElement(parseString(json.get("version").getAsString())); 19741 if (json.has("_version")) 19742 parseElementProperties(getJObject(json, "_version"), res.getVersionElement()); 19743 if (json.has("code")) 19744 res.setCodeElement(parseCode(json.get("code").getAsString())); 19745 if (json.has("_code")) 19746 parseElementProperties(getJObject(json, "_code"), res.getCodeElement()); 19747 if (json.has("display")) 19748 res.setDisplayElement(parseString(json.get("display").getAsString())); 19749 if (json.has("_display")) 19750 parseElementProperties(getJObject(json, "_display"), res.getDisplayElement()); 19751 if (json.has("designation")) { 19752 JsonArray array = json.getAsJsonArray("designation"); 19753 for (int i = 0; i < array.size(); i++) { 19754 res.getDesignation().add(parseValueSetConceptReferenceDesignationComponent(array.get(i).getAsJsonObject(), owner)); 19755 } 19756 }; 19757 if (json.has("contains")) { 19758 JsonArray array = json.getAsJsonArray("contains"); 19759 for (int i = 0; i < array.size(); i++) { 19760 res.getContains().add(parseValueSetValueSetExpansionContainsComponent(array.get(i).getAsJsonObject(), owner)); 19761 } 19762 }; 19763 } 19764 19765 protected VisionPrescription parseVisionPrescription(JsonObject json) throws IOException, FHIRFormatError { 19766 VisionPrescription res = new VisionPrescription(); 19767 parseVisionPrescriptionProperties(json, res); 19768 return res; 19769 } 19770 19771 protected void parseVisionPrescriptionProperties(JsonObject json, VisionPrescription res) throws IOException, FHIRFormatError { 19772 parseDomainResourceProperties(json, res); 19773 if (json.has("identifier")) { 19774 JsonArray array = json.getAsJsonArray("identifier"); 19775 for (int i = 0; i < array.size(); i++) { 19776 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 19777 } 19778 }; 19779 if (json.has("status")) 19780 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), VisionPrescription.VisionStatus.NULL, new VisionPrescription.VisionStatusEnumFactory())); 19781 if (json.has("_status")) 19782 parseElementProperties(getJObject(json, "_status"), res.getStatusElement()); 19783 if (json.has("patient")) 19784 res.setPatient(parseReference(getJObject(json, "patient"))); 19785 if (json.has("encounter")) 19786 res.setEncounter(parseReference(getJObject(json, "encounter"))); 19787 if (json.has("dateWritten")) 19788 res.setDateWrittenElement(parseDateTime(json.get("dateWritten").getAsString())); 19789 if (json.has("_dateWritten")) 19790 parseElementProperties(getJObject(json, "_dateWritten"), res.getDateWrittenElement()); 19791 if (json.has("prescriber")) 19792 res.setPrescriber(parseReference(getJObject(json, "prescriber"))); 19793 Type reason = parseType("reason", json); 19794 if (reason != null) 19795 res.setReason(reason); 19796 if (json.has("dispense")) { 19797 JsonArray array = json.getAsJsonArray("dispense"); 19798 for (int i = 0; i < array.size(); i++) { 19799 res.getDispense().add(parseVisionPrescriptionVisionPrescriptionDispenseComponent(array.get(i).getAsJsonObject(), res)); 19800 } 19801 }; 19802 } 19803 19804 protected VisionPrescription.VisionPrescriptionDispenseComponent parseVisionPrescriptionVisionPrescriptionDispenseComponent(JsonObject json, VisionPrescription owner) throws IOException, FHIRFormatError { 19805 VisionPrescription.VisionPrescriptionDispenseComponent res = new VisionPrescription.VisionPrescriptionDispenseComponent(); 19806 parseVisionPrescriptionVisionPrescriptionDispenseComponentProperties(json, owner, res); 19807 return res; 19808 } 19809 19810 protected void parseVisionPrescriptionVisionPrescriptionDispenseComponentProperties(JsonObject json, VisionPrescription owner, VisionPrescription.VisionPrescriptionDispenseComponent res) throws IOException, FHIRFormatError { 19811 parseBackboneProperties(json, res); 19812 if (json.has("product")) 19813 res.setProduct(parseCodeableConcept(getJObject(json, "product"))); 19814 if (json.has("eye")) 19815 res.setEyeElement(parseEnumeration(json.get("eye").getAsString(), VisionPrescription.VisionEyes.NULL, new VisionPrescription.VisionEyesEnumFactory())); 19816 if (json.has("_eye")) 19817 parseElementProperties(getJObject(json, "_eye"), res.getEyeElement()); 19818 if (json.has("sphere")) 19819 res.setSphereElement(parseDecimal(json.get("sphere").getAsBigDecimal())); 19820 if (json.has("_sphere")) 19821 parseElementProperties(getJObject(json, "_sphere"), res.getSphereElement()); 19822 if (json.has("cylinder")) 19823 res.setCylinderElement(parseDecimal(json.get("cylinder").getAsBigDecimal())); 19824 if (json.has("_cylinder")) 19825 parseElementProperties(getJObject(json, "_cylinder"), res.getCylinderElement()); 19826 if (json.has("axis")) 19827 res.setAxisElement(parseInteger(json.get("axis").getAsLong())); 19828 if (json.has("_axis")) 19829 parseElementProperties(getJObject(json, "_axis"), res.getAxisElement()); 19830 if (json.has("prism")) 19831 res.setPrismElement(parseDecimal(json.get("prism").getAsBigDecimal())); 19832 if (json.has("_prism")) 19833 parseElementProperties(getJObject(json, "_prism"), res.getPrismElement()); 19834 if (json.has("base")) 19835 res.setBaseElement(parseEnumeration(json.get("base").getAsString(), VisionPrescription.VisionBase.NULL, new VisionPrescription.VisionBaseEnumFactory())); 19836 if (json.has("_base")) 19837 parseElementProperties(getJObject(json, "_base"), res.getBaseElement()); 19838 if (json.has("add")) 19839 res.setAddElement(parseDecimal(json.get("add").getAsBigDecimal())); 19840 if (json.has("_add")) 19841 parseElementProperties(getJObject(json, "_add"), res.getAddElement()); 19842 if (json.has("power")) 19843 res.setPowerElement(parseDecimal(json.get("power").getAsBigDecimal())); 19844 if (json.has("_power")) 19845 parseElementProperties(getJObject(json, "_power"), res.getPowerElement()); 19846 if (json.has("backCurve")) 19847 res.setBackCurveElement(parseDecimal(json.get("backCurve").getAsBigDecimal())); 19848 if (json.has("_backCurve")) 19849 parseElementProperties(getJObject(json, "_backCurve"), res.getBackCurveElement()); 19850 if (json.has("diameter")) 19851 res.setDiameterElement(parseDecimal(json.get("diameter").getAsBigDecimal())); 19852 if (json.has("_diameter")) 19853 parseElementProperties(getJObject(json, "_diameter"), res.getDiameterElement()); 19854 if (json.has("duration")) 19855 res.setDuration(parseSimpleQuantity(getJObject(json, "duration"))); 19856 if (json.has("color")) 19857 res.setColorElement(parseString(json.get("color").getAsString())); 19858 if (json.has("_color")) 19859 parseElementProperties(getJObject(json, "_color"), res.getColorElement()); 19860 if (json.has("brand")) 19861 res.setBrandElement(parseString(json.get("brand").getAsString())); 19862 if (json.has("_brand")) 19863 parseElementProperties(getJObject(json, "_brand"), res.getBrandElement()); 19864 if (json.has("note")) { 19865 JsonArray array = json.getAsJsonArray("note"); 19866 for (int i = 0; i < array.size(); i++) { 19867 res.getNote().add(parseAnnotation(array.get(i).getAsJsonObject())); 19868 } 19869 }; 19870 } 19871 19872 @Override 19873 protected Resource parseResource(JsonObject json) throws IOException, FHIRFormatError { 19874 if (!json.has("resourceType")) { 19875 throw new FHIRFormatError("Unable to find resource type - maybe not a FHIR resource?"); 19876 } 19877 String t = json.get("resourceType").getAsString(); 19878 if (Utilities.noString(t)) 19879 throw new FHIRFormatError("Unable to find resource type - maybe not a FHIR resource?"); 19880 if (t.equals("Parameters")) 19881 return parseParameters(json); 19882 else if (t.equals("Account")) 19883 return parseAccount(json); 19884 else if (t.equals("ActivityDefinition")) 19885 return parseActivityDefinition(json); 19886 else if (t.equals("AdverseEvent")) 19887 return parseAdverseEvent(json); 19888 else if (t.equals("AllergyIntolerance")) 19889 return parseAllergyIntolerance(json); 19890 else if (t.equals("Appointment")) 19891 return parseAppointment(json); 19892 else if (t.equals("AppointmentResponse")) 19893 return parseAppointmentResponse(json); 19894 else if (t.equals("AuditEvent")) 19895 return parseAuditEvent(json); 19896 else if (t.equals("Basic")) 19897 return parseBasic(json); 19898 else if (t.equals("Binary")) 19899 return parseBinary(json); 19900 else if (t.equals("BodySite")) 19901 return parseBodySite(json); 19902 else if (t.equals("Bundle")) 19903 return parseBundle(json); 19904 else if (t.equals("CapabilityStatement")) 19905 return parseCapabilityStatement(json); 19906 else if (t.equals("CarePlan")) 19907 return parseCarePlan(json); 19908 else if (t.equals("CareTeam")) 19909 return parseCareTeam(json); 19910 else if (t.equals("ChargeItem")) 19911 return parseChargeItem(json); 19912 else if (t.equals("Claim")) 19913 return parseClaim(json); 19914 else if (t.equals("ClaimResponse")) 19915 return parseClaimResponse(json); 19916 else if (t.equals("ClinicalImpression")) 19917 return parseClinicalImpression(json); 19918 else if (t.equals("CodeSystem")) 19919 return parseCodeSystem(json); 19920 else if (t.equals("Communication")) 19921 return parseCommunication(json); 19922 else if (t.equals("CommunicationRequest")) 19923 return parseCommunicationRequest(json); 19924 else if (t.equals("CompartmentDefinition")) 19925 return parseCompartmentDefinition(json); 19926 else if (t.equals("Composition")) 19927 return parseComposition(json); 19928 else if (t.equals("ConceptMap")) 19929 return parseConceptMap(json); 19930 else if (t.equals("Condition")) 19931 return parseCondition(json); 19932 else if (t.equals("Consent")) 19933 return parseConsent(json); 19934 else if (t.equals("Contract")) 19935 return parseContract(json); 19936 else if (t.equals("Coverage")) 19937 return parseCoverage(json); 19938 else if (t.equals("DataElement")) 19939 return parseDataElement(json); 19940 else if (t.equals("DetectedIssue")) 19941 return parseDetectedIssue(json); 19942 else if (t.equals("Device")) 19943 return parseDevice(json); 19944 else if (t.equals("DeviceComponent")) 19945 return parseDeviceComponent(json); 19946 else if (t.equals("DeviceMetric")) 19947 return parseDeviceMetric(json); 19948 else if (t.equals("DeviceRequest")) 19949 return parseDeviceRequest(json); 19950 else if (t.equals("DeviceUseStatement")) 19951 return parseDeviceUseStatement(json); 19952 else if (t.equals("DiagnosticReport")) 19953 return parseDiagnosticReport(json); 19954 else if (t.equals("DocumentManifest")) 19955 return parseDocumentManifest(json); 19956 else if (t.equals("DocumentReference")) 19957 return parseDocumentReference(json); 19958 else if (t.equals("EligibilityRequest")) 19959 return parseEligibilityRequest(json); 19960 else if (t.equals("EligibilityResponse")) 19961 return parseEligibilityResponse(json); 19962 else if (t.equals("Encounter")) 19963 return parseEncounter(json); 19964 else if (t.equals("Endpoint")) 19965 return parseEndpoint(json); 19966 else if (t.equals("EnrollmentRequest")) 19967 return parseEnrollmentRequest(json); 19968 else if (t.equals("EnrollmentResponse")) 19969 return parseEnrollmentResponse(json); 19970 else if (t.equals("EpisodeOfCare")) 19971 return parseEpisodeOfCare(json); 19972 else if (t.equals("ExpansionProfile")) 19973 return parseExpansionProfile(json); 19974 else if (t.equals("ExplanationOfBenefit")) 19975 return parseExplanationOfBenefit(json); 19976 else if (t.equals("FamilyMemberHistory")) 19977 return parseFamilyMemberHistory(json); 19978 else if (t.equals("Flag")) 19979 return parseFlag(json); 19980 else if (t.equals("Goal")) 19981 return parseGoal(json); 19982 else if (t.equals("GraphDefinition")) 19983 return parseGraphDefinition(json); 19984 else if (t.equals("Group")) 19985 return parseGroup(json); 19986 else if (t.equals("GuidanceResponse")) 19987 return parseGuidanceResponse(json); 19988 else if (t.equals("HealthcareService")) 19989 return parseHealthcareService(json); 19990 else if (t.equals("ImagingManifest")) 19991 return parseImagingManifest(json); 19992 else if (t.equals("ImagingStudy")) 19993 return parseImagingStudy(json); 19994 else if (t.equals("Immunization")) 19995 return parseImmunization(json); 19996 else if (t.equals("ImmunizationRecommendation")) 19997 return parseImmunizationRecommendation(json); 19998 else if (t.equals("ImplementationGuide")) 19999 return parseImplementationGuide(json); 20000 else if (t.equals("Library")) 20001 return parseLibrary(json); 20002 else if (t.equals("Linkage")) 20003 return parseLinkage(json); 20004 else if (t.equals("List")) 20005 return parseListResource(json); 20006 else if (t.equals("Location")) 20007 return parseLocation(json); 20008 else if (t.equals("Measure")) 20009 return parseMeasure(json); 20010 else if (t.equals("MeasureReport")) 20011 return parseMeasureReport(json); 20012 else if (t.equals("Media")) 20013 return parseMedia(json); 20014 else if (t.equals("Medication")) 20015 return parseMedication(json); 20016 else if (t.equals("MedicationAdministration")) 20017 return parseMedicationAdministration(json); 20018 else if (t.equals("MedicationDispense")) 20019 return parseMedicationDispense(json); 20020 else if (t.equals("MedicationRequest")) 20021 return parseMedicationRequest(json); 20022 else if (t.equals("MedicationStatement")) 20023 return parseMedicationStatement(json); 20024 else if (t.equals("MessageDefinition")) 20025 return parseMessageDefinition(json); 20026 else if (t.equals("MessageHeader")) 20027 return parseMessageHeader(json); 20028 else if (t.equals("NamingSystem")) 20029 return parseNamingSystem(json); 20030 else if (t.equals("NutritionOrder")) 20031 return parseNutritionOrder(json); 20032 else if (t.equals("Observation")) 20033 return parseObservation(json); 20034 else if (t.equals("OperationDefinition")) 20035 return parseOperationDefinition(json); 20036 else if (t.equals("OperationOutcome")) 20037 return parseOperationOutcome(json); 20038 else if (t.equals("Organization")) 20039 return parseOrganization(json); 20040 else if (t.equals("Patient")) 20041 return parsePatient(json); 20042 else if (t.equals("PaymentNotice")) 20043 return parsePaymentNotice(json); 20044 else if (t.equals("PaymentReconciliation")) 20045 return parsePaymentReconciliation(json); 20046 else if (t.equals("Person")) 20047 return parsePerson(json); 20048 else if (t.equals("PlanDefinition")) 20049 return parsePlanDefinition(json); 20050 else if (t.equals("Practitioner")) 20051 return parsePractitioner(json); 20052 else if (t.equals("PractitionerRole")) 20053 return parsePractitionerRole(json); 20054 else if (t.equals("Procedure")) 20055 return parseProcedure(json); 20056 else if (t.equals("ProcedureRequest")) 20057 return parseProcedureRequest(json); 20058 else if (t.equals("ProcessRequest")) 20059 return parseProcessRequest(json); 20060 else if (t.equals("ProcessResponse")) 20061 return parseProcessResponse(json); 20062 else if (t.equals("Provenance")) 20063 return parseProvenance(json); 20064 else if (t.equals("Questionnaire")) 20065 return parseQuestionnaire(json); 20066 else if (t.equals("QuestionnaireResponse")) 20067 return parseQuestionnaireResponse(json); 20068 else if (t.equals("ReferralRequest")) 20069 return parseReferralRequest(json); 20070 else if (t.equals("RelatedPerson")) 20071 return parseRelatedPerson(json); 20072 else if (t.equals("RequestGroup")) 20073 return parseRequestGroup(json); 20074 else if (t.equals("ResearchStudy")) 20075 return parseResearchStudy(json); 20076 else if (t.equals("ResearchSubject")) 20077 return parseResearchSubject(json); 20078 else if (t.equals("RiskAssessment")) 20079 return parseRiskAssessment(json); 20080 else if (t.equals("Schedule")) 20081 return parseSchedule(json); 20082 else if (t.equals("SearchParameter")) 20083 return parseSearchParameter(json); 20084 else if (t.equals("Sequence")) 20085 return parseSequence(json); 20086 else if (t.equals("ServiceDefinition")) 20087 return parseServiceDefinition(json); 20088 else if (t.equals("Slot")) 20089 return parseSlot(json); 20090 else if (t.equals("Specimen")) 20091 return parseSpecimen(json); 20092 else if (t.equals("StructureDefinition")) 20093 return parseStructureDefinition(json); 20094 else if (t.equals("StructureMap")) 20095 return parseStructureMap(json); 20096 else if (t.equals("Subscription")) 20097 return parseSubscription(json); 20098 else if (t.equals("Substance")) 20099 return parseSubstance(json); 20100 else if (t.equals("SupplyDelivery")) 20101 return parseSupplyDelivery(json); 20102 else if (t.equals("SupplyRequest")) 20103 return parseSupplyRequest(json); 20104 else if (t.equals("Task")) 20105 return parseTask(json); 20106 else if (t.equals("TestReport")) 20107 return parseTestReport(json); 20108 else if (t.equals("TestScript")) 20109 return parseTestScript(json); 20110 else if (t.equals("ValueSet")) 20111 return parseValueSet(json); 20112 else if (t.equals("VisionPrescription")) 20113 return parseVisionPrescription(json); 20114 else if (t.equals("Binary")) 20115 return parseBinary(json); 20116 throw new FHIRFormatError("Unknown.Unrecognised resource type '"+t+"' (in property 'resourceType')"); 20117 } 20118 20119 protected Type parseType(String prefix, JsonObject json) throws IOException, FHIRFormatError { 20120 if (json.has(prefix+"Reference")) 20121 return parseReference(getJObject(json, prefix+"Reference")); 20122 else if (json.has(prefix+"Quantity")) 20123 return parseQuantity(getJObject(json, prefix+"Quantity")); 20124 else if (json.has(prefix+"Period")) 20125 return parsePeriod(getJObject(json, prefix+"Period")); 20126 else if (json.has(prefix+"Attachment")) 20127 return parseAttachment(getJObject(json, prefix+"Attachment")); 20128 else if (json.has(prefix+"Duration")) 20129 return parseDuration(getJObject(json, prefix+"Duration")); 20130 else if (json.has(prefix+"Count")) 20131 return parseCount(getJObject(json, prefix+"Count")); 20132 else if (json.has(prefix+"Range")) 20133 return parseRange(getJObject(json, prefix+"Range")); 20134 else if (json.has(prefix+"Annotation")) 20135 return parseAnnotation(getJObject(json, prefix+"Annotation")); 20136 else if (json.has(prefix+"Money")) 20137 return parseMoney(getJObject(json, prefix+"Money")); 20138 else if (json.has(prefix+"Identifier")) 20139 return parseIdentifier(getJObject(json, prefix+"Identifier")); 20140 else if (json.has(prefix+"Coding")) 20141 return parseCoding(getJObject(json, prefix+"Coding")); 20142 else if (json.has(prefix+"Signature")) 20143 return parseSignature(getJObject(json, prefix+"Signature")); 20144 else if (json.has(prefix+"SampledData")) 20145 return parseSampledData(getJObject(json, prefix+"SampledData")); 20146 else if (json.has(prefix+"Ratio")) 20147 return parseRatio(getJObject(json, prefix+"Ratio")); 20148 else if (json.has(prefix+"Distance")) 20149 return parseDistance(getJObject(json, prefix+"Distance")); 20150 else if (json.has(prefix+"Age")) 20151 return parseAge(getJObject(json, prefix+"Age")); 20152 else if (json.has(prefix+"CodeableConcept")) 20153 return parseCodeableConcept(getJObject(json, prefix+"CodeableConcept")); 20154 else if (json.has(prefix+"SimpleQuantity")) 20155 return parseSimpleQuantity(getJObject(json, prefix+"SimpleQuantity")); 20156 else if (json.has(prefix+"Meta")) 20157 return parseMeta(getJObject(json, prefix+"Meta")); 20158 else if (json.has(prefix+"Address")) 20159 return parseAddress(getJObject(json, prefix+"Address")); 20160 else if (json.has(prefix+"TriggerDefinition")) 20161 return parseTriggerDefinition(getJObject(json, prefix+"TriggerDefinition")); 20162 else if (json.has(prefix+"Contributor")) 20163 return parseContributor(getJObject(json, prefix+"Contributor")); 20164 else if (json.has(prefix+"DataRequirement")) 20165 return parseDataRequirement(getJObject(json, prefix+"DataRequirement")); 20166 else if (json.has(prefix+"Dosage")) 20167 return parseDosage(getJObject(json, prefix+"Dosage")); 20168 else if (json.has(prefix+"RelatedArtifact")) 20169 return parseRelatedArtifact(getJObject(json, prefix+"RelatedArtifact")); 20170 else if (json.has(prefix+"ContactDetail")) 20171 return parseContactDetail(getJObject(json, prefix+"ContactDetail")); 20172 else if (json.has(prefix+"HumanName")) 20173 return parseHumanName(getJObject(json, prefix+"HumanName")); 20174 else if (json.has(prefix+"ContactPoint")) 20175 return parseContactPoint(getJObject(json, prefix+"ContactPoint")); 20176 else if (json.has(prefix+"UsageContext")) 20177 return parseUsageContext(getJObject(json, prefix+"UsageContext")); 20178 else if (json.has(prefix+"Timing")) 20179 return parseTiming(getJObject(json, prefix+"Timing")); 20180 else if (json.has(prefix+"ElementDefinition")) 20181 return parseElementDefinition(getJObject(json, prefix+"ElementDefinition")); 20182 else if (json.has(prefix+"ParameterDefinition")) 20183 return parseParameterDefinition(getJObject(json, prefix+"ParameterDefinition")); 20184 else if (json.has(prefix+"Date") || json.has("_"+prefix+"Date")) { 20185 Type t = json.has(prefix+"Date") ? parseDate(json.get(prefix+"Date").getAsString()) : new DateType(); 20186 if (json.has("_"+prefix+"Date")) 20187 parseElementProperties(getJObject(json, "_"+prefix+"Date"), t); 20188 return t; 20189 } 20190 else if (json.has(prefix+"DateTime") || json.has("_"+prefix+"DateTime")) { 20191 Type t = json.has(prefix+"DateTime") ? parseDateTime(json.get(prefix+"DateTime").getAsString()) : new DateTimeType(); 20192 if (json.has("_"+prefix+"DateTime")) 20193 parseElementProperties(getJObject(json, "_"+prefix+"DateTime"), t); 20194 return t; 20195 } 20196 else if (json.has(prefix+"Code") || json.has("_"+prefix+"Code")) { 20197 Type t = json.has(prefix+"Code") ? parseCode(json.get(prefix+"Code").getAsString()) : new CodeType(); 20198 if (json.has("_"+prefix+"Code")) 20199 parseElementProperties(getJObject(json, "_"+prefix+"Code"), t); 20200 return t; 20201 } 20202 else if (json.has(prefix+"String") || json.has("_"+prefix+"String")) { 20203 Type t = json.has(prefix+"String") ? parseString(json.get(prefix+"String").getAsString()) : new StringType(); 20204 if (json.has("_"+prefix+"String")) 20205 parseElementProperties(getJObject(json, "_"+prefix+"String"), t); 20206 return t; 20207 } 20208 else if (json.has(prefix+"Integer") || json.has("_"+prefix+"Integer")) { 20209 Type t = json.has(prefix+"Integer") ? parseInteger(json.get(prefix+"Integer").getAsLong()) : new IntegerType(); 20210 if (json.has("_"+prefix+"Integer")) 20211 parseElementProperties(getJObject(json, "_"+prefix+"Integer"), t); 20212 return t; 20213 } 20214 else if (json.has(prefix+"Oid") || json.has("_"+prefix+"Oid")) { 20215 Type t = json.has(prefix+"Oid") ? parseOid(json.get(prefix+"Oid").getAsString()) : new OidType(); 20216 if (json.has("_"+prefix+"Oid")) 20217 parseElementProperties(getJObject(json, "_"+prefix+"Oid"), t); 20218 return t; 20219 } 20220 else if (json.has(prefix+"Uri") || json.has("_"+prefix+"Uri")) { 20221 Type t = json.has(prefix+"Uri") ? parseUri(json.get(prefix+"Uri").getAsString()) : new UriType(); 20222 if (json.has("_"+prefix+"Uri")) 20223 parseElementProperties(getJObject(json, "_"+prefix+"Uri"), t); 20224 return t; 20225 } 20226 else if (json.has(prefix+"Uuid") || json.has("_"+prefix+"Uuid")) { 20227 Type t = json.has(prefix+"Uuid") ? parseUuid(json.get(prefix+"Uuid").getAsString()) : new UuidType(); 20228 if (json.has("_"+prefix+"Uuid")) 20229 parseElementProperties(getJObject(json, "_"+prefix+"Uuid"), t); 20230 return t; 20231 } 20232 else if (json.has(prefix+"Instant") || json.has("_"+prefix+"Instant")) { 20233 Type t = json.has(prefix+"Instant") ? parseInstant(json.get(prefix+"Instant").getAsString()) : new InstantType(); 20234 if (json.has("_"+prefix+"Instant")) 20235 parseElementProperties(getJObject(json, "_"+prefix+"Instant"), t); 20236 return t; 20237 } 20238 else if (json.has(prefix+"Boolean") || json.has("_"+prefix+"Boolean")) { 20239 Type t = json.has(prefix+"Boolean") ? parseBoolean(json.get(prefix+"Boolean").getAsBoolean()) : new BooleanType(); 20240 if (json.has("_"+prefix+"Boolean")) 20241 parseElementProperties(getJObject(json, "_"+prefix+"Boolean"), t); 20242 return t; 20243 } 20244 else if (json.has(prefix+"Base64Binary") || json.has("_"+prefix+"Base64Binary")) { 20245 Type t = json.has(prefix+"Base64Binary") ? parseBase64Binary(json.get(prefix+"Base64Binary").getAsString()) : new Base64BinaryType(); 20246 if (json.has("_"+prefix+"Base64Binary")) 20247 parseElementProperties(getJObject(json, "_"+prefix+"Base64Binary"), t); 20248 return t; 20249 } 20250 else if (json.has(prefix+"UnsignedInt") || json.has("_"+prefix+"UnsignedInt")) { 20251 Type t = json.has(prefix+"UnsignedInt") ? parseUnsignedInt(json.get(prefix+"UnsignedInt").getAsString()) : new UnsignedIntType(); 20252 if (json.has("_"+prefix+"UnsignedInt")) 20253 parseElementProperties(getJObject(json, "_"+prefix+"UnsignedInt"), t); 20254 return t; 20255 } 20256 else if (json.has(prefix+"Markdown") || json.has("_"+prefix+"Markdown")) { 20257 Type t = json.has(prefix+"Markdown") ? parseMarkdown(json.get(prefix+"Markdown").getAsString()) : new MarkdownType(); 20258 if (json.has("_"+prefix+"Markdown")) 20259 parseElementProperties(getJObject(json, "_"+prefix+"Markdown"), t); 20260 return t; 20261 } 20262 else if (json.has(prefix+"Time") || json.has("_"+prefix+"Time")) { 20263 Type t = json.has(prefix+"Time") ? parseTime(json.get(prefix+"Time").getAsString()) : new TimeType(); 20264 if (json.has("_"+prefix+"Time")) 20265 parseElementProperties(getJObject(json, "_"+prefix+"Time"), t); 20266 return t; 20267 } 20268 else if (json.has(prefix+"Id") || json.has("_"+prefix+"Id")) { 20269 Type t = json.has(prefix+"Id") ? parseId(json.get(prefix+"Id").getAsString()) : new IdType(); 20270 if (json.has("_"+prefix+"Id")) 20271 parseElementProperties(getJObject(json, "_"+prefix+"Id"), t); 20272 return t; 20273 } 20274 else if (json.has(prefix+"PositiveInt") || json.has("_"+prefix+"PositiveInt")) { 20275 Type t = json.has(prefix+"PositiveInt") ? parsePositiveInt(json.get(prefix+"PositiveInt").getAsString()) : new PositiveIntType(); 20276 if (json.has("_"+prefix+"PositiveInt")) 20277 parseElementProperties(getJObject(json, "_"+prefix+"PositiveInt"), t); 20278 return t; 20279 } 20280 else if (json.has(prefix+"Decimal") || json.has("_"+prefix+"Decimal")) { 20281 Type t = json.has(prefix+"Decimal") ? parseDecimal(json.get(prefix+"Decimal").getAsBigDecimal()) : new DecimalType(); 20282 if (json.has("_"+prefix+"Decimal")) 20283 parseElementProperties(getJObject(json, "_"+prefix+"Decimal"), t); 20284 return t; 20285 } 20286 return null; 20287 } 20288 20289 protected Type parseType(JsonObject json, String type) throws IOException, FHIRFormatError { 20290 if (type.equals("Reference")) 20291 return parseReference(json); 20292 else if (type.equals("Quantity")) 20293 return parseQuantity(json); 20294 else if (type.equals("Period")) 20295 return parsePeriod(json); 20296 else if (type.equals("Attachment")) 20297 return parseAttachment(json); 20298 else if (type.equals("Duration")) 20299 return parseDuration(json); 20300 else if (type.equals("Count")) 20301 return parseCount(json); 20302 else if (type.equals("Range")) 20303 return parseRange(json); 20304 else if (type.equals("Annotation")) 20305 return parseAnnotation(json); 20306 else if (type.equals("Money")) 20307 return parseMoney(json); 20308 else if (type.equals("Identifier")) 20309 return parseIdentifier(json); 20310 else if (type.equals("Coding")) 20311 return parseCoding(json); 20312 else if (type.equals("Signature")) 20313 return parseSignature(json); 20314 else if (type.equals("SampledData")) 20315 return parseSampledData(json); 20316 else if (type.equals("Ratio")) 20317 return parseRatio(json); 20318 else if (type.equals("Distance")) 20319 return parseDistance(json); 20320 else if (type.equals("Age")) 20321 return parseAge(json); 20322 else if (type.equals("CodeableConcept")) 20323 return parseCodeableConcept(json); 20324 else if (type.equals("SimpleQuantity")) 20325 return parseSimpleQuantity(json); 20326 else if (type.equals("Meta")) 20327 return parseMeta(json); 20328 else if (type.equals("Address")) 20329 return parseAddress(json); 20330 else if (type.equals("TriggerDefinition")) 20331 return parseTriggerDefinition(json); 20332 else if (type.equals("Contributor")) 20333 return parseContributor(json); 20334 else if (type.equals("DataRequirement")) 20335 return parseDataRequirement(json); 20336 else if (type.equals("Dosage")) 20337 return parseDosage(json); 20338 else if (type.equals("RelatedArtifact")) 20339 return parseRelatedArtifact(json); 20340 else if (type.equals("ContactDetail")) 20341 return parseContactDetail(json); 20342 else if (type.equals("HumanName")) 20343 return parseHumanName(json); 20344 else if (type.equals("ContactPoint")) 20345 return parseContactPoint(json); 20346 else if (type.equals("UsageContext")) 20347 return parseUsageContext(json); 20348 else if (type.equals("Timing")) 20349 return parseTiming(json); 20350 else if (type.equals("ElementDefinition")) 20351 return parseElementDefinition(json); 20352 else if (type.equals("ParameterDefinition")) 20353 return parseParameterDefinition(json); 20354 throw new FHIRFormatError("Unknown Type "+type); 20355 } 20356 20357 protected boolean hasTypeName(JsonObject json, String prefix) { 20358 if (json.has(prefix+"Reference")) 20359 return true; 20360 if (json.has(prefix+"Quantity")) 20361 return true; 20362 if (json.has(prefix+"Period")) 20363 return true; 20364 if (json.has(prefix+"Attachment")) 20365 return true; 20366 if (json.has(prefix+"Duration")) 20367 return true; 20368 if (json.has(prefix+"Count")) 20369 return true; 20370 if (json.has(prefix+"Range")) 20371 return true; 20372 if (json.has(prefix+"Annotation")) 20373 return true; 20374 if (json.has(prefix+"Money")) 20375 return true; 20376 if (json.has(prefix+"Identifier")) 20377 return true; 20378 if (json.has(prefix+"Coding")) 20379 return true; 20380 if (json.has(prefix+"Signature")) 20381 return true; 20382 if (json.has(prefix+"SampledData")) 20383 return true; 20384 if (json.has(prefix+"Ratio")) 20385 return true; 20386 if (json.has(prefix+"Distance")) 20387 return true; 20388 if (json.has(prefix+"Age")) 20389 return true; 20390 if (json.has(prefix+"CodeableConcept")) 20391 return true; 20392 if (json.has(prefix+"SimpleQuantity")) 20393 return true; 20394 if (json.has(prefix+"Meta")) 20395 return true; 20396 if (json.has(prefix+"Address")) 20397 return true; 20398 if (json.has(prefix+"TriggerDefinition")) 20399 return true; 20400 if (json.has(prefix+"Contributor")) 20401 return true; 20402 if (json.has(prefix+"DataRequirement")) 20403 return true; 20404 if (json.has(prefix+"Dosage")) 20405 return true; 20406 if (json.has(prefix+"RelatedArtifact")) 20407 return true; 20408 if (json.has(prefix+"ContactDetail")) 20409 return true; 20410 if (json.has(prefix+"HumanName")) 20411 return true; 20412 if (json.has(prefix+"ContactPoint")) 20413 return true; 20414 if (json.has(prefix+"UsageContext")) 20415 return true; 20416 if (json.has(prefix+"Timing")) 20417 return true; 20418 if (json.has(prefix+"ElementDefinition")) 20419 return true; 20420 if (json.has(prefix+"ParameterDefinition")) 20421 return true; 20422 if (json.has(prefix+"Parameters")) 20423 return true; 20424 if (json.has(prefix+"Account")) 20425 return true; 20426 if (json.has(prefix+"ActivityDefinition")) 20427 return true; 20428 if (json.has(prefix+"AdverseEvent")) 20429 return true; 20430 if (json.has(prefix+"AllergyIntolerance")) 20431 return true; 20432 if (json.has(prefix+"Appointment")) 20433 return true; 20434 if (json.has(prefix+"AppointmentResponse")) 20435 return true; 20436 if (json.has(prefix+"AuditEvent")) 20437 return true; 20438 if (json.has(prefix+"Basic")) 20439 return true; 20440 if (json.has(prefix+"Binary")) 20441 return true; 20442 if (json.has(prefix+"BodySite")) 20443 return true; 20444 if (json.has(prefix+"Bundle")) 20445 return true; 20446 if (json.has(prefix+"CapabilityStatement")) 20447 return true; 20448 if (json.has(prefix+"CarePlan")) 20449 return true; 20450 if (json.has(prefix+"CareTeam")) 20451 return true; 20452 if (json.has(prefix+"ChargeItem")) 20453 return true; 20454 if (json.has(prefix+"Claim")) 20455 return true; 20456 if (json.has(prefix+"ClaimResponse")) 20457 return true; 20458 if (json.has(prefix+"ClinicalImpression")) 20459 return true; 20460 if (json.has(prefix+"CodeSystem")) 20461 return true; 20462 if (json.has(prefix+"Communication")) 20463 return true; 20464 if (json.has(prefix+"CommunicationRequest")) 20465 return true; 20466 if (json.has(prefix+"CompartmentDefinition")) 20467 return true; 20468 if (json.has(prefix+"Composition")) 20469 return true; 20470 if (json.has(prefix+"ConceptMap")) 20471 return true; 20472 if (json.has(prefix+"Condition")) 20473 return true; 20474 if (json.has(prefix+"Consent")) 20475 return true; 20476 if (json.has(prefix+"Contract")) 20477 return true; 20478 if (json.has(prefix+"Coverage")) 20479 return true; 20480 if (json.has(prefix+"DataElement")) 20481 return true; 20482 if (json.has(prefix+"DetectedIssue")) 20483 return true; 20484 if (json.has(prefix+"Device")) 20485 return true; 20486 if (json.has(prefix+"DeviceComponent")) 20487 return true; 20488 if (json.has(prefix+"DeviceMetric")) 20489 return true; 20490 if (json.has(prefix+"DeviceRequest")) 20491 return true; 20492 if (json.has(prefix+"DeviceUseStatement")) 20493 return true; 20494 if (json.has(prefix+"DiagnosticReport")) 20495 return true; 20496 if (json.has(prefix+"DocumentManifest")) 20497 return true; 20498 if (json.has(prefix+"DocumentReference")) 20499 return true; 20500 if (json.has(prefix+"EligibilityRequest")) 20501 return true; 20502 if (json.has(prefix+"EligibilityResponse")) 20503 return true; 20504 if (json.has(prefix+"Encounter")) 20505 return true; 20506 if (json.has(prefix+"Endpoint")) 20507 return true; 20508 if (json.has(prefix+"EnrollmentRequest")) 20509 return true; 20510 if (json.has(prefix+"EnrollmentResponse")) 20511 return true; 20512 if (json.has(prefix+"EpisodeOfCare")) 20513 return true; 20514 if (json.has(prefix+"ExpansionProfile")) 20515 return true; 20516 if (json.has(prefix+"ExplanationOfBenefit")) 20517 return true; 20518 if (json.has(prefix+"FamilyMemberHistory")) 20519 return true; 20520 if (json.has(prefix+"Flag")) 20521 return true; 20522 if (json.has(prefix+"Goal")) 20523 return true; 20524 if (json.has(prefix+"GraphDefinition")) 20525 return true; 20526 if (json.has(prefix+"Group")) 20527 return true; 20528 if (json.has(prefix+"GuidanceResponse")) 20529 return true; 20530 if (json.has(prefix+"HealthcareService")) 20531 return true; 20532 if (json.has(prefix+"ImagingManifest")) 20533 return true; 20534 if (json.has(prefix+"ImagingStudy")) 20535 return true; 20536 if (json.has(prefix+"Immunization")) 20537 return true; 20538 if (json.has(prefix+"ImmunizationRecommendation")) 20539 return true; 20540 if (json.has(prefix+"ImplementationGuide")) 20541 return true; 20542 if (json.has(prefix+"Library")) 20543 return true; 20544 if (json.has(prefix+"Linkage")) 20545 return true; 20546 if (json.has(prefix+"List")) 20547 return true; 20548 if (json.has(prefix+"Location")) 20549 return true; 20550 if (json.has(prefix+"Measure")) 20551 return true; 20552 if (json.has(prefix+"MeasureReport")) 20553 return true; 20554 if (json.has(prefix+"Media")) 20555 return true; 20556 if (json.has(prefix+"Medication")) 20557 return true; 20558 if (json.has(prefix+"MedicationAdministration")) 20559 return true; 20560 if (json.has(prefix+"MedicationDispense")) 20561 return true; 20562 if (json.has(prefix+"MedicationRequest")) 20563 return true; 20564 if (json.has(prefix+"MedicationStatement")) 20565 return true; 20566 if (json.has(prefix+"MessageDefinition")) 20567 return true; 20568 if (json.has(prefix+"MessageHeader")) 20569 return true; 20570 if (json.has(prefix+"NamingSystem")) 20571 return true; 20572 if (json.has(prefix+"NutritionOrder")) 20573 return true; 20574 if (json.has(prefix+"Observation")) 20575 return true; 20576 if (json.has(prefix+"OperationDefinition")) 20577 return true; 20578 if (json.has(prefix+"OperationOutcome")) 20579 return true; 20580 if (json.has(prefix+"Organization")) 20581 return true; 20582 if (json.has(prefix+"Patient")) 20583 return true; 20584 if (json.has(prefix+"PaymentNotice")) 20585 return true; 20586 if (json.has(prefix+"PaymentReconciliation")) 20587 return true; 20588 if (json.has(prefix+"Person")) 20589 return true; 20590 if (json.has(prefix+"PlanDefinition")) 20591 return true; 20592 if (json.has(prefix+"Practitioner")) 20593 return true; 20594 if (json.has(prefix+"PractitionerRole")) 20595 return true; 20596 if (json.has(prefix+"Procedure")) 20597 return true; 20598 if (json.has(prefix+"ProcedureRequest")) 20599 return true; 20600 if (json.has(prefix+"ProcessRequest")) 20601 return true; 20602 if (json.has(prefix+"ProcessResponse")) 20603 return true; 20604 if (json.has(prefix+"Provenance")) 20605 return true; 20606 if (json.has(prefix+"Questionnaire")) 20607 return true; 20608 if (json.has(prefix+"QuestionnaireResponse")) 20609 return true; 20610 if (json.has(prefix+"ReferralRequest")) 20611 return true; 20612 if (json.has(prefix+"RelatedPerson")) 20613 return true; 20614 if (json.has(prefix+"RequestGroup")) 20615 return true; 20616 if (json.has(prefix+"ResearchStudy")) 20617 return true; 20618 if (json.has(prefix+"ResearchSubject")) 20619 return true; 20620 if (json.has(prefix+"RiskAssessment")) 20621 return true; 20622 if (json.has(prefix+"Schedule")) 20623 return true; 20624 if (json.has(prefix+"SearchParameter")) 20625 return true; 20626 if (json.has(prefix+"Sequence")) 20627 return true; 20628 if (json.has(prefix+"ServiceDefinition")) 20629 return true; 20630 if (json.has(prefix+"Slot")) 20631 return true; 20632 if (json.has(prefix+"Specimen")) 20633 return true; 20634 if (json.has(prefix+"StructureDefinition")) 20635 return true; 20636 if (json.has(prefix+"StructureMap")) 20637 return true; 20638 if (json.has(prefix+"Subscription")) 20639 return true; 20640 if (json.has(prefix+"Substance")) 20641 return true; 20642 if (json.has(prefix+"SupplyDelivery")) 20643 return true; 20644 if (json.has(prefix+"SupplyRequest")) 20645 return true; 20646 if (json.has(prefix+"Task")) 20647 return true; 20648 if (json.has(prefix+"TestReport")) 20649 return true; 20650 if (json.has(prefix+"TestScript")) 20651 return true; 20652 if (json.has(prefix+"ValueSet")) 20653 return true; 20654 if (json.has(prefix+"VisionPrescription")) 20655 return true; 20656 if (json.has(prefix+"Date") || json.has("_"+prefix+"Date")) 20657 return true; 20658 if (json.has(prefix+"DateTime") || json.has("_"+prefix+"DateTime")) 20659 return true; 20660 if (json.has(prefix+"Code") || json.has("_"+prefix+"Code")) 20661 return true; 20662 if (json.has(prefix+"String") || json.has("_"+prefix+"String")) 20663 return true; 20664 if (json.has(prefix+"Integer") || json.has("_"+prefix+"Integer")) 20665 return true; 20666 if (json.has(prefix+"Oid") || json.has("_"+prefix+"Oid")) 20667 return true; 20668 if (json.has(prefix+"Uri") || json.has("_"+prefix+"Uri")) 20669 return true; 20670 if (json.has(prefix+"Uuid") || json.has("_"+prefix+"Uuid")) 20671 return true; 20672 if (json.has(prefix+"Instant") || json.has("_"+prefix+"Instant")) 20673 return true; 20674 if (json.has(prefix+"Boolean") || json.has("_"+prefix+"Boolean")) 20675 return true; 20676 if (json.has(prefix+"Base64Binary") || json.has("_"+prefix+"Base64Binary")) 20677 return true; 20678 if (json.has(prefix+"UnsignedInt") || json.has("_"+prefix+"UnsignedInt")) 20679 return true; 20680 if (json.has(prefix+"Markdown") || json.has("_"+prefix+"Markdown")) 20681 return true; 20682 if (json.has(prefix+"Time") || json.has("_"+prefix+"Time")) 20683 return true; 20684 if (json.has(prefix+"Id") || json.has("_"+prefix+"Id")) 20685 return true; 20686 if (json.has(prefix+"PositiveInt") || json.has("_"+prefix+"PositiveInt")) 20687 return true; 20688 if (json.has(prefix+"Decimal") || json.has("_"+prefix+"Decimal")) 20689 return true; 20690 return false; 20691 } 20692 protected void composeElement(Element element) throws IOException { 20693 if (element.hasId()) 20694 prop("id", element.getId()); 20695 if (makeComments(element)) { 20696 openArray("fhir_comments"); 20697 for (String s : element.getFormatCommentsPre()) 20698 prop(null, s); 20699 for (String s : element.getFormatCommentsPost()) 20700 prop(null, s); 20701 closeArray(); 20702 } 20703 if (element.hasExtension()) { 20704 openArray("extension"); 20705 for (Extension e : element.getExtension()) 20706 composeExtension(null, e); 20707 closeArray(); 20708 } 20709 } 20710 20711 protected void composeBackbone(BackboneElement element) throws IOException { 20712 composeElement(element); 20713 if (element.hasModifierExtension()) { 20714 openArray("modifierExtension"); 20715 for (Extension e : element.getModifierExtension()) 20716 composeExtension(null, e); 20717 closeArray(); 20718 } 20719 } 20720 20721 protected <E extends Enum<E>> void composeEnumerationCore(String name, Enumeration<E> value, EnumFactory e, boolean inArray) throws IOException { 20722 if (value != null && value.getValue() != null) { 20723 prop(name, e.toCode(value.getValue())); 20724 } else if (inArray) 20725 writeNull(name); 20726 } 20727 20728 protected <E extends Enum<E>> void composeEnumerationExtras(String name, Enumeration<E> value, EnumFactory e, boolean inArray) throws IOException { 20729 if (value != null && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 20730 open(inArray ? null : "_"+name); 20731 composeElement(value); 20732 close(); 20733 } else if (inArray) 20734 writeNull(name); 20735 } 20736 20737 protected void composeDateCore(String name, DateType value, boolean inArray) throws IOException { 20738 if (value != null && value.hasValue()) { 20739 prop(name, value.asStringValue()); 20740 } 20741 else if (inArray) 20742 writeNull(name); 20743 } 20744 20745 protected void composeDateExtras(String name, DateType value, boolean inArray) throws IOException { 20746 if (value != null && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 20747 open(inArray ? null : "_"+name); 20748 composeElement(value); 20749 close(); 20750 } 20751 else if (inArray) 20752 writeNull(name); 20753 } 20754 20755 protected void composeDateTimeCore(String name, DateTimeType value, boolean inArray) throws IOException { 20756 if (value != null && value.hasValue()) { 20757 prop(name, value.asStringValue()); 20758 } 20759 else if (inArray) 20760 writeNull(name); 20761 } 20762 20763 protected void composeDateTimeExtras(String name, DateTimeType value, boolean inArray) throws IOException { 20764 if (value != null && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 20765 open(inArray ? null : "_"+name); 20766 composeElement(value); 20767 close(); 20768 } 20769 else if (inArray) 20770 writeNull(name); 20771 } 20772 20773 protected void composeCodeCore(String name, CodeType value, boolean inArray) throws IOException { 20774 if (value != null && value.hasValue()) { 20775 prop(name, toString(value.getValue())); 20776 } 20777 else if (inArray) 20778 writeNull(name); 20779 } 20780 20781 protected void composeCodeExtras(String name, CodeType value, boolean inArray) throws IOException { 20782 if (value != null && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 20783 open(inArray ? null : "_"+name); 20784 composeElement(value); 20785 close(); 20786 } 20787 else if (inArray) 20788 writeNull(name); 20789 } 20790 20791 protected void composeStringCore(String name, StringType value, boolean inArray) throws IOException { 20792 if (value != null && value.hasValue()) { 20793 prop(name, toString(value.getValue())); 20794 } 20795 else if (inArray) 20796 writeNull(name); 20797 } 20798 20799 protected void composeStringExtras(String name, StringType value, boolean inArray) throws IOException { 20800 if (value != null && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 20801 open(inArray ? null : "_"+name); 20802 composeElement(value); 20803 close(); 20804 } 20805 else if (inArray) 20806 writeNull(name); 20807 } 20808 20809 protected void composeIntegerCore(String name, IntegerType value, boolean inArray) throws IOException { 20810 if (value != null && value.hasValue()) { 20811 prop(name, Integer.valueOf(value.getValue())); 20812 } 20813 else if (inArray) 20814 writeNull(name); 20815 } 20816 20817 protected void composeIntegerExtras(String name, IntegerType value, boolean inArray) throws IOException { 20818 if (value != null && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 20819 open(inArray ? null : "_"+name); 20820 composeElement(value); 20821 close(); 20822 } 20823 else if (inArray) 20824 writeNull(name); 20825 } 20826 20827 protected void composeOidCore(String name, OidType value, boolean inArray) throws IOException { 20828 if (value != null && value.hasValue()) { 20829 prop(name, toString(value.getValue())); 20830 } 20831 else if (inArray) 20832 writeNull(name); 20833 } 20834 20835 protected void composeOidExtras(String name, OidType value, boolean inArray) throws IOException { 20836 if (value != null && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 20837 open(inArray ? null : "_"+name); 20838 composeElement(value); 20839 close(); 20840 } 20841 else if (inArray) 20842 writeNull(name); 20843 } 20844 20845 protected void composeUriCore(String name, UriType value, boolean inArray) throws IOException { 20846 if (value != null && value.hasValue()) { 20847 prop(name, toString(value.getValue())); 20848 } 20849 else if (inArray) 20850 writeNull(name); 20851 } 20852 20853 protected void composeUriExtras(String name, UriType value, boolean inArray) throws IOException { 20854 if (value != null && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 20855 open(inArray ? null : "_"+name); 20856 composeElement(value); 20857 close(); 20858 } 20859 else if (inArray) 20860 writeNull(name); 20861 } 20862 20863 protected void composeUuidCore(String name, UuidType value, boolean inArray) throws IOException { 20864 if (value != null && value.hasValue()) { 20865 prop(name, toString(value.getValue())); 20866 } 20867 else if (inArray) 20868 writeNull(name); 20869 } 20870 20871 protected void composeUuidExtras(String name, UuidType value, boolean inArray) throws IOException { 20872 if (value != null && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 20873 open(inArray ? null : "_"+name); 20874 composeElement(value); 20875 close(); 20876 } 20877 else if (inArray) 20878 writeNull(name); 20879 } 20880 20881 protected void composeInstantCore(String name, InstantType value, boolean inArray) throws IOException { 20882 if (value != null && value.hasValue()) { 20883 prop(name, value.asStringValue()); 20884 } 20885 else if (inArray) 20886 writeNull(name); 20887 } 20888 20889 protected void composeInstantExtras(String name, InstantType value, boolean inArray) throws IOException { 20890 if (value != null && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 20891 open(inArray ? null : "_"+name); 20892 composeElement(value); 20893 close(); 20894 } 20895 else if (inArray) 20896 writeNull(name); 20897 } 20898 20899 protected void composeBooleanCore(String name, BooleanType value, boolean inArray) throws IOException { 20900 if (value != null && value.hasValue()) { 20901 prop(name, value.getValue()); 20902 } 20903 else if (inArray) 20904 writeNull(name); 20905 } 20906 20907 protected void composeBooleanExtras(String name, BooleanType value, boolean inArray) throws IOException { 20908 if (value != null && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 20909 open(inArray ? null : "_"+name); 20910 composeElement(value); 20911 close(); 20912 } 20913 else if (inArray) 20914 writeNull(name); 20915 } 20916 20917 protected void composeBase64BinaryCore(String name, Base64BinaryType value, boolean inArray) throws IOException { 20918 if (value != null && value.hasValue()) { 20919 prop(name, toString(value.getValue())); 20920 } 20921 else if (inArray) 20922 writeNull(name); 20923 } 20924 20925 protected void composeBase64BinaryExtras(String name, Base64BinaryType value, boolean inArray) throws IOException { 20926 if (value != null && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 20927 open(inArray ? null : "_"+name); 20928 composeElement(value); 20929 close(); 20930 } 20931 else if (inArray) 20932 writeNull(name); 20933 } 20934 20935 protected void composeUnsignedIntCore(String name, UnsignedIntType value, boolean inArray) throws IOException { 20936 if (value != null && value.hasValue()) { 20937 prop(name, Integer.valueOf(value.getValue())); 20938 } 20939 else if (inArray) 20940 writeNull(name); 20941 } 20942 20943 protected void composeUnsignedIntExtras(String name, UnsignedIntType value, boolean inArray) throws IOException { 20944 if (value != null && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 20945 open(inArray ? null : "_"+name); 20946 composeElement(value); 20947 close(); 20948 } 20949 else if (inArray) 20950 writeNull(name); 20951 } 20952 20953 protected void composeMarkdownCore(String name, MarkdownType value, boolean inArray) throws IOException { 20954 if (value != null && value.hasValue()) { 20955 prop(name, toString(value.getValue())); 20956 } 20957 else if (inArray) 20958 writeNull(name); 20959 } 20960 20961 protected void composeMarkdownExtras(String name, MarkdownType value, boolean inArray) throws IOException { 20962 if (value != null && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 20963 open(inArray ? null : "_"+name); 20964 composeElement(value); 20965 close(); 20966 } 20967 else if (inArray) 20968 writeNull(name); 20969 } 20970 20971 protected void composeTimeCore(String name, TimeType value, boolean inArray) throws IOException { 20972 if (value != null && value.hasValue()) { 20973 prop(name, value.asStringValue()); 20974 } 20975 else if (inArray) 20976 writeNull(name); 20977 } 20978 20979 protected void composeTimeExtras(String name, TimeType value, boolean inArray) throws IOException { 20980 if (value != null && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 20981 open(inArray ? null : "_"+name); 20982 composeElement(value); 20983 close(); 20984 } 20985 else if (inArray) 20986 writeNull(name); 20987 } 20988 20989 protected void composeIdCore(String name, IdType value, boolean inArray) throws IOException { 20990 if (value != null && value.hasValue()) { 20991 prop(name, toString(value.getValue())); 20992 } 20993 else if (inArray) 20994 writeNull(name); 20995 } 20996 20997 protected void composeIdExtras(String name, IdType value, boolean inArray) throws IOException { 20998 if (value != null && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 20999 open(inArray ? null : "_"+name); 21000 composeElement(value); 21001 close(); 21002 } 21003 else if (inArray) 21004 writeNull(name); 21005 } 21006 21007 protected void composePositiveIntCore(String name, PositiveIntType value, boolean inArray) throws IOException { 21008 if (value != null && value.hasValue()) { 21009 prop(name, Integer.valueOf(value.getValue())); 21010 } 21011 else if (inArray) 21012 writeNull(name); 21013 } 21014 21015 protected void composePositiveIntExtras(String name, PositiveIntType value, boolean inArray) throws IOException { 21016 if (value != null && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 21017 open(inArray ? null : "_"+name); 21018 composeElement(value); 21019 close(); 21020 } 21021 else if (inArray) 21022 writeNull(name); 21023 } 21024 21025 protected void composeDecimalCore(String name, DecimalType value, boolean inArray) throws IOException { 21026 if (value != null && value.hasValue()) { 21027 prop(name, value.getValue()); 21028 } 21029 else if (inArray) 21030 writeNull(name); 21031 } 21032 21033 protected void composeDecimalExtras(String name, DecimalType value, boolean inArray) throws IOException { 21034 if (value != null && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 21035 open(inArray ? null : "_"+name); 21036 composeElement(value); 21037 close(); 21038 } 21039 else if (inArray) 21040 writeNull(name); 21041 } 21042 21043 protected void composeExtension(String name, Extension element) throws IOException { 21044 if (element != null) { 21045 open(name); 21046 composeExtensionInner(element); 21047 close(); 21048 } 21049 } 21050 21051 protected void composeExtensionInner(Extension element) throws IOException { 21052 composeElement(element); 21053 if (element.hasUrlElement()) { 21054 composeUriCore("url", element.getUrlElement(), false); 21055 composeUriExtras("url", element.getUrlElement(), false); 21056 } 21057 if (element.hasValue()) { 21058 composeType("value", element.getValue()); 21059 } 21060 } 21061 21062 protected void composeNarrative(String name, Narrative element) throws IOException { 21063 if (element != null) { 21064 open(name); 21065 composeNarrativeInner(element); 21066 close(); 21067 } 21068 } 21069 21070 protected void composeNarrativeInner(Narrative element) throws IOException { 21071 composeElement(element); 21072 if (element.hasStatusElement()) { 21073 composeEnumerationCore("status", element.getStatusElement(), new Narrative.NarrativeStatusEnumFactory(), false); 21074 composeEnumerationExtras("status", element.getStatusElement(), new Narrative.NarrativeStatusEnumFactory(), false); 21075 } 21076 if (element.hasDiv()) { 21077 XhtmlNode node = element.getDiv(); 21078 if (node.getNsDecl() == null) { 21079 node.attribute("xmlns", XHTML_NS); 21080 } 21081 composeXhtml("div", node); 21082 } 21083 } 21084 21085 protected void composeDuration(String name, Duration element) throws IOException { 21086 if (element != null) { 21087 open(name); 21088 composeDurationInner(element); 21089 close(); 21090 } 21091 } 21092 21093 protected void composeDurationInner(Duration element) throws IOException { 21094 composeQuantityInner(element); 21095 } 21096 21097 protected void composeCount(String name, Count element) throws IOException { 21098 if (element != null) { 21099 open(name); 21100 composeCountInner(element); 21101 close(); 21102 } 21103 } 21104 21105 protected void composeCountInner(Count element) throws IOException { 21106 composeQuantityInner(element); 21107 } 21108 21109 protected void composeMoney(String name, Money element) throws IOException { 21110 if (element != null) { 21111 open(name); 21112 composeMoneyInner(element); 21113 close(); 21114 } 21115 } 21116 21117 protected void composeMoneyInner(Money element) throws IOException { 21118 composeQuantityInner(element); 21119 } 21120 21121 protected void composeDistance(String name, Distance element) throws IOException { 21122 if (element != null) { 21123 open(name); 21124 composeDistanceInner(element); 21125 close(); 21126 } 21127 } 21128 21129 protected void composeDistanceInner(Distance element) throws IOException { 21130 composeQuantityInner(element); 21131 } 21132 21133 protected void composeAge(String name, Age element) throws IOException { 21134 if (element != null) { 21135 open(name); 21136 composeAgeInner(element); 21137 close(); 21138 } 21139 } 21140 21141 protected void composeAgeInner(Age element) throws IOException { 21142 composeQuantityInner(element); 21143 } 21144 21145 protected void composeReference(String name, Reference element) throws IOException { 21146 if (element != null) { 21147 open(name); 21148 composeReferenceInner(element); 21149 close(); 21150 } 21151 } 21152 21153 protected void composeReferenceInner(Reference element) throws IOException { 21154 composeElement(element); 21155 if (element.hasReferenceElement()) { 21156 composeStringCore("reference", element.getReferenceElement(), false); 21157 composeStringExtras("reference", element.getReferenceElement(), false); 21158 } 21159 if (element.hasIdentifier()) { 21160 composeIdentifier("identifier", element.getIdentifier()); 21161 } 21162 if (element.hasDisplayElement()) { 21163 composeStringCore("display", element.getDisplayElement(), false); 21164 composeStringExtras("display", element.getDisplayElement(), false); 21165 } 21166 } 21167 21168 protected void composeQuantity(String name, Quantity element) throws IOException { 21169 if (element != null) { 21170 open(name); 21171 composeQuantityInner(element); 21172 close(); 21173 } 21174 } 21175 21176 protected void composeQuantityInner(Quantity element) throws IOException { 21177 composeElement(element); 21178 if (element.hasValueElement()) { 21179 composeDecimalCore("value", element.getValueElement(), false); 21180 composeDecimalExtras("value", element.getValueElement(), false); 21181 } 21182 if (element.hasComparatorElement()) { 21183 composeEnumerationCore("comparator", element.getComparatorElement(), new Quantity.QuantityComparatorEnumFactory(), false); 21184 composeEnumerationExtras("comparator", element.getComparatorElement(), new Quantity.QuantityComparatorEnumFactory(), false); 21185 } 21186 if (element.hasUnitElement()) { 21187 composeStringCore("unit", element.getUnitElement(), false); 21188 composeStringExtras("unit", element.getUnitElement(), false); 21189 } 21190 if (element.hasSystemElement()) { 21191 composeUriCore("system", element.getSystemElement(), false); 21192 composeUriExtras("system", element.getSystemElement(), false); 21193 } 21194 if (element.hasCodeElement()) { 21195 composeCodeCore("code", element.getCodeElement(), false); 21196 composeCodeExtras("code", element.getCodeElement(), false); 21197 } 21198 } 21199 21200 protected void composePeriod(String name, Period element) throws IOException { 21201 if (element != null) { 21202 open(name); 21203 composePeriodInner(element); 21204 close(); 21205 } 21206 } 21207 21208 protected void composePeriodInner(Period element) throws IOException { 21209 composeElement(element); 21210 if (element.hasStartElement()) { 21211 composeDateTimeCore("start", element.getStartElement(), false); 21212 composeDateTimeExtras("start", element.getStartElement(), false); 21213 } 21214 if (element.hasEndElement()) { 21215 composeDateTimeCore("end", element.getEndElement(), false); 21216 composeDateTimeExtras("end", element.getEndElement(), false); 21217 } 21218 } 21219 21220 protected void composeAttachment(String name, Attachment element) throws IOException { 21221 if (element != null) { 21222 open(name); 21223 composeAttachmentInner(element); 21224 close(); 21225 } 21226 } 21227 21228 protected void composeAttachmentInner(Attachment element) throws IOException { 21229 composeElement(element); 21230 if (element.hasContentTypeElement()) { 21231 composeCodeCore("contentType", element.getContentTypeElement(), false); 21232 composeCodeExtras("contentType", element.getContentTypeElement(), false); 21233 } 21234 if (element.hasLanguageElement()) { 21235 composeCodeCore("language", element.getLanguageElement(), false); 21236 composeCodeExtras("language", element.getLanguageElement(), false); 21237 } 21238 if (element.hasDataElement()) { 21239 composeBase64BinaryCore("data", element.getDataElement(), false); 21240 composeBase64BinaryExtras("data", element.getDataElement(), false); 21241 } 21242 if (element.hasUrlElement()) { 21243 composeUriCore("url", element.getUrlElement(), false); 21244 composeUriExtras("url", element.getUrlElement(), false); 21245 } 21246 if (element.hasSizeElement()) { 21247 composeUnsignedIntCore("size", element.getSizeElement(), false); 21248 composeUnsignedIntExtras("size", element.getSizeElement(), false); 21249 } 21250 if (element.hasHashElement()) { 21251 composeBase64BinaryCore("hash", element.getHashElement(), false); 21252 composeBase64BinaryExtras("hash", element.getHashElement(), false); 21253 } 21254 if (element.hasTitleElement()) { 21255 composeStringCore("title", element.getTitleElement(), false); 21256 composeStringExtras("title", element.getTitleElement(), false); 21257 } 21258 if (element.hasCreationElement()) { 21259 composeDateTimeCore("creation", element.getCreationElement(), false); 21260 composeDateTimeExtras("creation", element.getCreationElement(), false); 21261 } 21262 } 21263 21264 protected void composeRange(String name, Range element) throws IOException { 21265 if (element != null) { 21266 open(name); 21267 composeRangeInner(element); 21268 close(); 21269 } 21270 } 21271 21272 protected void composeRangeInner(Range element) throws IOException { 21273 composeElement(element); 21274 if (element.hasLow()) { 21275 composeSimpleQuantity("low", element.getLow()); 21276 } 21277 if (element.hasHigh()) { 21278 composeSimpleQuantity("high", element.getHigh()); 21279 } 21280 } 21281 21282 protected void composeAnnotation(String name, Annotation element) throws IOException { 21283 if (element != null) { 21284 open(name); 21285 composeAnnotationInner(element); 21286 close(); 21287 } 21288 } 21289 21290 protected void composeAnnotationInner(Annotation element) throws IOException { 21291 composeElement(element); 21292 if (element.hasAuthor()) { 21293 composeType("author", element.getAuthor()); 21294 } 21295 if (element.hasTimeElement()) { 21296 composeDateTimeCore("time", element.getTimeElement(), false); 21297 composeDateTimeExtras("time", element.getTimeElement(), false); 21298 } 21299 if (element.hasTextElement()) { 21300 composeStringCore("text", element.getTextElement(), false); 21301 composeStringExtras("text", element.getTextElement(), false); 21302 } 21303 } 21304 21305 protected void composeIdentifier(String name, Identifier element) throws IOException { 21306 if (element != null) { 21307 open(name); 21308 composeIdentifierInner(element); 21309 close(); 21310 } 21311 } 21312 21313 protected void composeIdentifierInner(Identifier element) throws IOException { 21314 composeElement(element); 21315 if (element.hasUseElement()) { 21316 composeEnumerationCore("use", element.getUseElement(), new Identifier.IdentifierUseEnumFactory(), false); 21317 composeEnumerationExtras("use", element.getUseElement(), new Identifier.IdentifierUseEnumFactory(), false); 21318 } 21319 if (element.hasType()) { 21320 composeCodeableConcept("type", element.getType()); 21321 } 21322 if (element.hasSystemElement()) { 21323 composeUriCore("system", element.getSystemElement(), false); 21324 composeUriExtras("system", element.getSystemElement(), false); 21325 } 21326 if (element.hasValueElement()) { 21327 composeStringCore("value", element.getValueElement(), false); 21328 composeStringExtras("value", element.getValueElement(), false); 21329 } 21330 if (element.hasPeriod()) { 21331 composePeriod("period", element.getPeriod()); 21332 } 21333 if (element.hasAssigner()) { 21334 composeReference("assigner", element.getAssigner()); 21335 } 21336 } 21337 21338 protected void composeCoding(String name, Coding element) throws IOException { 21339 if (element != null) { 21340 open(name); 21341 composeCodingInner(element); 21342 close(); 21343 } 21344 } 21345 21346 protected void composeCodingInner(Coding element) throws IOException { 21347 composeElement(element); 21348 if (element.hasSystemElement()) { 21349 composeUriCore("system", element.getSystemElement(), false); 21350 composeUriExtras("system", element.getSystemElement(), false); 21351 } 21352 if (element.hasVersionElement()) { 21353 composeStringCore("version", element.getVersionElement(), false); 21354 composeStringExtras("version", element.getVersionElement(), false); 21355 } 21356 if (element.hasCodeElement()) { 21357 composeCodeCore("code", element.getCodeElement(), false); 21358 composeCodeExtras("code", element.getCodeElement(), false); 21359 } 21360 if (element.hasDisplayElement()) { 21361 composeStringCore("display", element.getDisplayElement(), false); 21362 composeStringExtras("display", element.getDisplayElement(), false); 21363 } 21364 if (element.hasUserSelectedElement()) { 21365 composeBooleanCore("userSelected", element.getUserSelectedElement(), false); 21366 composeBooleanExtras("userSelected", element.getUserSelectedElement(), false); 21367 } 21368 } 21369 21370 protected void composeSignature(String name, Signature element) throws IOException { 21371 if (element != null) { 21372 open(name); 21373 composeSignatureInner(element); 21374 close(); 21375 } 21376 } 21377 21378 protected void composeSignatureInner(Signature element) throws IOException { 21379 composeElement(element); 21380 if (element.hasType()) { 21381 openArray("type"); 21382 for (Coding e : element.getType()) 21383 composeCoding(null, e); 21384 closeArray(); 21385 }; 21386 if (element.hasWhenElement()) { 21387 composeInstantCore("when", element.getWhenElement(), false); 21388 composeInstantExtras("when", element.getWhenElement(), false); 21389 } 21390 if (element.hasWho()) { 21391 composeType("who", element.getWho()); 21392 } 21393 if (element.hasOnBehalfOf()) { 21394 composeType("onBehalfOf", element.getOnBehalfOf()); 21395 } 21396 if (element.hasContentTypeElement()) { 21397 composeCodeCore("contentType", element.getContentTypeElement(), false); 21398 composeCodeExtras("contentType", element.getContentTypeElement(), false); 21399 } 21400 if (element.hasBlobElement()) { 21401 composeBase64BinaryCore("blob", element.getBlobElement(), false); 21402 composeBase64BinaryExtras("blob", element.getBlobElement(), false); 21403 } 21404 } 21405 21406 protected void composeSampledData(String name, SampledData element) throws IOException { 21407 if (element != null) { 21408 open(name); 21409 composeSampledDataInner(element); 21410 close(); 21411 } 21412 } 21413 21414 protected void composeSampledDataInner(SampledData element) throws IOException { 21415 composeElement(element); 21416 if (element.hasOrigin()) { 21417 composeSimpleQuantity("origin", element.getOrigin()); 21418 } 21419 if (element.hasPeriodElement()) { 21420 composeDecimalCore("period", element.getPeriodElement(), false); 21421 composeDecimalExtras("period", element.getPeriodElement(), false); 21422 } 21423 if (element.hasFactorElement()) { 21424 composeDecimalCore("factor", element.getFactorElement(), false); 21425 composeDecimalExtras("factor", element.getFactorElement(), false); 21426 } 21427 if (element.hasLowerLimitElement()) { 21428 composeDecimalCore("lowerLimit", element.getLowerLimitElement(), false); 21429 composeDecimalExtras("lowerLimit", element.getLowerLimitElement(), false); 21430 } 21431 if (element.hasUpperLimitElement()) { 21432 composeDecimalCore("upperLimit", element.getUpperLimitElement(), false); 21433 composeDecimalExtras("upperLimit", element.getUpperLimitElement(), false); 21434 } 21435 if (element.hasDimensionsElement()) { 21436 composePositiveIntCore("dimensions", element.getDimensionsElement(), false); 21437 composePositiveIntExtras("dimensions", element.getDimensionsElement(), false); 21438 } 21439 if (element.hasDataElement()) { 21440 composeStringCore("data", element.getDataElement(), false); 21441 composeStringExtras("data", element.getDataElement(), false); 21442 } 21443 } 21444 21445 protected void composeRatio(String name, Ratio element) throws IOException { 21446 if (element != null) { 21447 open(name); 21448 composeRatioInner(element); 21449 close(); 21450 } 21451 } 21452 21453 protected void composeRatioInner(Ratio element) throws IOException { 21454 composeElement(element); 21455 if (element.hasNumerator()) { 21456 composeQuantity("numerator", element.getNumerator()); 21457 } 21458 if (element.hasDenominator()) { 21459 composeQuantity("denominator", element.getDenominator()); 21460 } 21461 } 21462 21463 protected void composeCodeableConcept(String name, CodeableConcept element) throws IOException { 21464 if (element != null) { 21465 open(name); 21466 composeCodeableConceptInner(element); 21467 close(); 21468 } 21469 } 21470 21471 protected void composeCodeableConceptInner(CodeableConcept element) throws IOException { 21472 composeElement(element); 21473 if (element.hasCoding()) { 21474 openArray("coding"); 21475 for (Coding e : element.getCoding()) 21476 composeCoding(null, e); 21477 closeArray(); 21478 }; 21479 if (element.hasTextElement()) { 21480 composeStringCore("text", element.getTextElement(), false); 21481 composeStringExtras("text", element.getTextElement(), false); 21482 } 21483 } 21484 21485 protected void composeSimpleQuantity(String name, SimpleQuantity element) throws IOException { 21486 if (element != null) { 21487 open(name); 21488 composeSimpleQuantityInner(element); 21489 close(); 21490 } 21491 } 21492 21493 protected void composeSimpleQuantityInner(SimpleQuantity element) throws IOException { 21494 composeElement(element); 21495 if (element.hasValueElement()) { 21496 composeDecimalCore("value", element.getValueElement(), false); 21497 composeDecimalExtras("value", element.getValueElement(), false); 21498 } 21499 if (element.hasComparatorElement()) { 21500 composeEnumerationCore("comparator", element.getComparatorElement(), new SimpleQuantity.QuantityComparatorEnumFactory(), false); 21501 composeEnumerationExtras("comparator", element.getComparatorElement(), new SimpleQuantity.QuantityComparatorEnumFactory(), false); 21502 } 21503 if (element.hasUnitElement()) { 21504 composeStringCore("unit", element.getUnitElement(), false); 21505 composeStringExtras("unit", element.getUnitElement(), false); 21506 } 21507 if (element.hasSystemElement()) { 21508 composeUriCore("system", element.getSystemElement(), false); 21509 composeUriExtras("system", element.getSystemElement(), false); 21510 } 21511 if (element.hasCodeElement()) { 21512 composeCodeCore("code", element.getCodeElement(), false); 21513 composeCodeExtras("code", element.getCodeElement(), false); 21514 } 21515 } 21516 21517 protected void composeMeta(String name, Meta element) throws IOException { 21518 if (element != null) { 21519 open(name); 21520 composeMetaInner(element); 21521 close(); 21522 } 21523 } 21524 21525 protected void composeMetaInner(Meta element) throws IOException { 21526 composeElement(element); 21527 if (element.hasVersionIdElement()) { 21528 composeIdCore("versionId", element.getVersionIdElement(), false); 21529 composeIdExtras("versionId", element.getVersionIdElement(), false); 21530 } 21531 if (element.hasLastUpdatedElement()) { 21532 composeInstantCore("lastUpdated", element.getLastUpdatedElement(), false); 21533 composeInstantExtras("lastUpdated", element.getLastUpdatedElement(), false); 21534 } 21535 if (element.hasProfile()) { 21536 openArray("profile"); 21537 for (UriType e : element.getProfile()) 21538 composeUriCore(null, e, true); 21539 closeArray(); 21540 if (anyHasExtras(element.getProfile())) { 21541 openArray("_profile"); 21542 for (UriType e : element.getProfile()) 21543 composeUriExtras(null, e, true); 21544 closeArray(); 21545 } 21546 }; 21547 if (element.hasSecurity()) { 21548 openArray("security"); 21549 for (Coding e : element.getSecurity()) 21550 composeCoding(null, e); 21551 closeArray(); 21552 }; 21553 if (element.hasTag()) { 21554 openArray("tag"); 21555 for (Coding e : element.getTag()) 21556 composeCoding(null, e); 21557 closeArray(); 21558 }; 21559 } 21560 21561 protected void composeAddress(String name, Address element) throws IOException { 21562 if (element != null) { 21563 open(name); 21564 composeAddressInner(element); 21565 close(); 21566 } 21567 } 21568 21569 protected void composeAddressInner(Address element) throws IOException { 21570 composeElement(element); 21571 if (element.hasUseElement()) { 21572 composeEnumerationCore("use", element.getUseElement(), new Address.AddressUseEnumFactory(), false); 21573 composeEnumerationExtras("use", element.getUseElement(), new Address.AddressUseEnumFactory(), false); 21574 } 21575 if (element.hasTypeElement()) { 21576 composeEnumerationCore("type", element.getTypeElement(), new Address.AddressTypeEnumFactory(), false); 21577 composeEnumerationExtras("type", element.getTypeElement(), new Address.AddressTypeEnumFactory(), false); 21578 } 21579 if (element.hasTextElement()) { 21580 composeStringCore("text", element.getTextElement(), false); 21581 composeStringExtras("text", element.getTextElement(), false); 21582 } 21583 if (element.hasLine()) { 21584 openArray("line"); 21585 for (StringType e : element.getLine()) 21586 composeStringCore(null, e, true); 21587 closeArray(); 21588 if (anyHasExtras(element.getLine())) { 21589 openArray("_line"); 21590 for (StringType e : element.getLine()) 21591 composeStringExtras(null, e, true); 21592 closeArray(); 21593 } 21594 }; 21595 if (element.hasCityElement()) { 21596 composeStringCore("city", element.getCityElement(), false); 21597 composeStringExtras("city", element.getCityElement(), false); 21598 } 21599 if (element.hasDistrictElement()) { 21600 composeStringCore("district", element.getDistrictElement(), false); 21601 composeStringExtras("district", element.getDistrictElement(), false); 21602 } 21603 if (element.hasStateElement()) { 21604 composeStringCore("state", element.getStateElement(), false); 21605 composeStringExtras("state", element.getStateElement(), false); 21606 } 21607 if (element.hasPostalCodeElement()) { 21608 composeStringCore("postalCode", element.getPostalCodeElement(), false); 21609 composeStringExtras("postalCode", element.getPostalCodeElement(), false); 21610 } 21611 if (element.hasCountryElement()) { 21612 composeStringCore("country", element.getCountryElement(), false); 21613 composeStringExtras("country", element.getCountryElement(), false); 21614 } 21615 if (element.hasPeriod()) { 21616 composePeriod("period", element.getPeriod()); 21617 } 21618 } 21619 21620 protected void composeTriggerDefinition(String name, TriggerDefinition element) throws IOException { 21621 if (element != null) { 21622 open(name); 21623 composeTriggerDefinitionInner(element); 21624 close(); 21625 } 21626 } 21627 21628 protected void composeTriggerDefinitionInner(TriggerDefinition element) throws IOException { 21629 composeElement(element); 21630 if (element.hasTypeElement()) { 21631 composeEnumerationCore("type", element.getTypeElement(), new TriggerDefinition.TriggerTypeEnumFactory(), false); 21632 composeEnumerationExtras("type", element.getTypeElement(), new TriggerDefinition.TriggerTypeEnumFactory(), false); 21633 } 21634 if (element.hasEventNameElement()) { 21635 composeStringCore("eventName", element.getEventNameElement(), false); 21636 composeStringExtras("eventName", element.getEventNameElement(), false); 21637 } 21638 if (element.hasEventTiming()) { 21639 composeType("eventTiming", element.getEventTiming()); 21640 } 21641 if (element.hasEventData()) { 21642 composeDataRequirement("eventData", element.getEventData()); 21643 } 21644 } 21645 21646 protected void composeContributor(String name, Contributor element) throws IOException { 21647 if (element != null) { 21648 open(name); 21649 composeContributorInner(element); 21650 close(); 21651 } 21652 } 21653 21654 protected void composeContributorInner(Contributor element) throws IOException { 21655 composeElement(element); 21656 if (element.hasTypeElement()) { 21657 composeEnumerationCore("type", element.getTypeElement(), new Contributor.ContributorTypeEnumFactory(), false); 21658 composeEnumerationExtras("type", element.getTypeElement(), new Contributor.ContributorTypeEnumFactory(), false); 21659 } 21660 if (element.hasNameElement()) { 21661 composeStringCore("name", element.getNameElement(), false); 21662 composeStringExtras("name", element.getNameElement(), false); 21663 } 21664 if (element.hasContact()) { 21665 openArray("contact"); 21666 for (ContactDetail e : element.getContact()) 21667 composeContactDetail(null, e); 21668 closeArray(); 21669 }; 21670 } 21671 21672 protected void composeDataRequirement(String name, DataRequirement element) throws IOException { 21673 if (element != null) { 21674 open(name); 21675 composeDataRequirementInner(element); 21676 close(); 21677 } 21678 } 21679 21680 protected void composeDataRequirementInner(DataRequirement element) throws IOException { 21681 composeElement(element); 21682 if (element.hasTypeElement()) { 21683 composeCodeCore("type", element.getTypeElement(), false); 21684 composeCodeExtras("type", element.getTypeElement(), false); 21685 } 21686 if (element.hasProfile()) { 21687 openArray("profile"); 21688 for (UriType e : element.getProfile()) 21689 composeUriCore(null, e, true); 21690 closeArray(); 21691 if (anyHasExtras(element.getProfile())) { 21692 openArray("_profile"); 21693 for (UriType e : element.getProfile()) 21694 composeUriExtras(null, e, true); 21695 closeArray(); 21696 } 21697 }; 21698 if (element.hasMustSupport()) { 21699 openArray("mustSupport"); 21700 for (StringType e : element.getMustSupport()) 21701 composeStringCore(null, e, true); 21702 closeArray(); 21703 if (anyHasExtras(element.getMustSupport())) { 21704 openArray("_mustSupport"); 21705 for (StringType e : element.getMustSupport()) 21706 composeStringExtras(null, e, true); 21707 closeArray(); 21708 } 21709 }; 21710 if (element.hasCodeFilter()) { 21711 openArray("codeFilter"); 21712 for (DataRequirement.DataRequirementCodeFilterComponent e : element.getCodeFilter()) 21713 composeDataRequirementDataRequirementCodeFilterComponent(null, e); 21714 closeArray(); 21715 }; 21716 if (element.hasDateFilter()) { 21717 openArray("dateFilter"); 21718 for (DataRequirement.DataRequirementDateFilterComponent e : element.getDateFilter()) 21719 composeDataRequirementDataRequirementDateFilterComponent(null, e); 21720 closeArray(); 21721 }; 21722 } 21723 21724 protected void composeDataRequirementDataRequirementCodeFilterComponent(String name, DataRequirement.DataRequirementCodeFilterComponent element) throws IOException { 21725 if (element != null) { 21726 open(name); 21727 composeDataRequirementDataRequirementCodeFilterComponentInner(element); 21728 close(); 21729 } 21730 } 21731 21732 protected void composeDataRequirementDataRequirementCodeFilterComponentInner(DataRequirement.DataRequirementCodeFilterComponent element) throws IOException { 21733 composeElement(element); 21734 if (element.hasPathElement()) { 21735 composeStringCore("path", element.getPathElement(), false); 21736 composeStringExtras("path", element.getPathElement(), false); 21737 } 21738 if (element.hasValueSet()) { 21739 composeType("valueSet", element.getValueSet()); 21740 } 21741 if (element.hasValueCode()) { 21742 openArray("valueCode"); 21743 for (CodeType e : element.getValueCode()) 21744 composeCodeCore(null, e, true); 21745 closeArray(); 21746 if (anyHasExtras(element.getValueCode())) { 21747 openArray("_valueCode"); 21748 for (CodeType e : element.getValueCode()) 21749 composeCodeExtras(null, e, true); 21750 closeArray(); 21751 } 21752 }; 21753 if (element.hasValueCoding()) { 21754 openArray("valueCoding"); 21755 for (Coding e : element.getValueCoding()) 21756 composeCoding(null, e); 21757 closeArray(); 21758 }; 21759 if (element.hasValueCodeableConcept()) { 21760 openArray("valueCodeableConcept"); 21761 for (CodeableConcept e : element.getValueCodeableConcept()) 21762 composeCodeableConcept(null, e); 21763 closeArray(); 21764 }; 21765 } 21766 21767 protected void composeDataRequirementDataRequirementDateFilterComponent(String name, DataRequirement.DataRequirementDateFilterComponent element) throws IOException { 21768 if (element != null) { 21769 open(name); 21770 composeDataRequirementDataRequirementDateFilterComponentInner(element); 21771 close(); 21772 } 21773 } 21774 21775 protected void composeDataRequirementDataRequirementDateFilterComponentInner(DataRequirement.DataRequirementDateFilterComponent element) throws IOException { 21776 composeElement(element); 21777 if (element.hasPathElement()) { 21778 composeStringCore("path", element.getPathElement(), false); 21779 composeStringExtras("path", element.getPathElement(), false); 21780 } 21781 if (element.hasValue()) { 21782 composeType("value", element.getValue()); 21783 } 21784 } 21785 21786 protected void composeDosage(String name, Dosage element) throws IOException { 21787 if (element != null) { 21788 open(name); 21789 composeDosageInner(element); 21790 close(); 21791 } 21792 } 21793 21794 protected void composeDosageInner(Dosage element) throws IOException { 21795 composeElement(element); 21796 if (element.hasSequenceElement()) { 21797 composeIntegerCore("sequence", element.getSequenceElement(), false); 21798 composeIntegerExtras("sequence", element.getSequenceElement(), false); 21799 } 21800 if (element.hasTextElement()) { 21801 composeStringCore("text", element.getTextElement(), false); 21802 composeStringExtras("text", element.getTextElement(), false); 21803 } 21804 if (element.hasAdditionalInstruction()) { 21805 openArray("additionalInstruction"); 21806 for (CodeableConcept e : element.getAdditionalInstruction()) 21807 composeCodeableConcept(null, e); 21808 closeArray(); 21809 }; 21810 if (element.hasPatientInstructionElement()) { 21811 composeStringCore("patientInstruction", element.getPatientInstructionElement(), false); 21812 composeStringExtras("patientInstruction", element.getPatientInstructionElement(), false); 21813 } 21814 if (element.hasTiming()) { 21815 composeTiming("timing", element.getTiming()); 21816 } 21817 if (element.hasAsNeeded()) { 21818 composeType("asNeeded", element.getAsNeeded()); 21819 } 21820 if (element.hasSite()) { 21821 composeCodeableConcept("site", element.getSite()); 21822 } 21823 if (element.hasRoute()) { 21824 composeCodeableConcept("route", element.getRoute()); 21825 } 21826 if (element.hasMethod()) { 21827 composeCodeableConcept("method", element.getMethod()); 21828 } 21829 if (element.hasDose()) { 21830 composeType("dose", element.getDose()); 21831 } 21832 if (element.hasMaxDosePerPeriod()) { 21833 composeRatio("maxDosePerPeriod", element.getMaxDosePerPeriod()); 21834 } 21835 if (element.hasMaxDosePerAdministration()) { 21836 composeSimpleQuantity("maxDosePerAdministration", element.getMaxDosePerAdministration()); 21837 } 21838 if (element.hasMaxDosePerLifetime()) { 21839 composeSimpleQuantity("maxDosePerLifetime", element.getMaxDosePerLifetime()); 21840 } 21841 if (element.hasRate()) { 21842 composeType("rate", element.getRate()); 21843 } 21844 } 21845 21846 protected void composeRelatedArtifact(String name, RelatedArtifact element) throws IOException { 21847 if (element != null) { 21848 open(name); 21849 composeRelatedArtifactInner(element); 21850 close(); 21851 } 21852 } 21853 21854 protected void composeRelatedArtifactInner(RelatedArtifact element) throws IOException { 21855 composeElement(element); 21856 if (element.hasTypeElement()) { 21857 composeEnumerationCore("type", element.getTypeElement(), new RelatedArtifact.RelatedArtifactTypeEnumFactory(), false); 21858 composeEnumerationExtras("type", element.getTypeElement(), new RelatedArtifact.RelatedArtifactTypeEnumFactory(), false); 21859 } 21860 if (element.hasDisplayElement()) { 21861 composeStringCore("display", element.getDisplayElement(), false); 21862 composeStringExtras("display", element.getDisplayElement(), false); 21863 } 21864 if (element.hasCitationElement()) { 21865 composeStringCore("citation", element.getCitationElement(), false); 21866 composeStringExtras("citation", element.getCitationElement(), false); 21867 } 21868 if (element.hasUrlElement()) { 21869 composeUriCore("url", element.getUrlElement(), false); 21870 composeUriExtras("url", element.getUrlElement(), false); 21871 } 21872 if (element.hasDocument()) { 21873 composeAttachment("document", element.getDocument()); 21874 } 21875 if (element.hasResource()) { 21876 composeReference("resource", element.getResource()); 21877 } 21878 } 21879 21880 protected void composeContactDetail(String name, ContactDetail element) throws IOException { 21881 if (element != null) { 21882 open(name); 21883 composeContactDetailInner(element); 21884 close(); 21885 } 21886 } 21887 21888 protected void composeContactDetailInner(ContactDetail element) throws IOException { 21889 composeElement(element); 21890 if (element.hasNameElement()) { 21891 composeStringCore("name", element.getNameElement(), false); 21892 composeStringExtras("name", element.getNameElement(), false); 21893 } 21894 if (element.hasTelecom()) { 21895 openArray("telecom"); 21896 for (ContactPoint e : element.getTelecom()) 21897 composeContactPoint(null, e); 21898 closeArray(); 21899 }; 21900 } 21901 21902 protected void composeHumanName(String name, HumanName element) throws IOException { 21903 if (element != null) { 21904 open(name); 21905 composeHumanNameInner(element); 21906 close(); 21907 } 21908 } 21909 21910 protected void composeHumanNameInner(HumanName element) throws IOException { 21911 composeElement(element); 21912 if (element.hasUseElement()) { 21913 composeEnumerationCore("use", element.getUseElement(), new HumanName.NameUseEnumFactory(), false); 21914 composeEnumerationExtras("use", element.getUseElement(), new HumanName.NameUseEnumFactory(), false); 21915 } 21916 if (element.hasTextElement()) { 21917 composeStringCore("text", element.getTextElement(), false); 21918 composeStringExtras("text", element.getTextElement(), false); 21919 } 21920 if (element.hasFamilyElement()) { 21921 composeStringCore("family", element.getFamilyElement(), false); 21922 composeStringExtras("family", element.getFamilyElement(), false); 21923 } 21924 if (element.hasGiven()) { 21925 openArray("given"); 21926 for (StringType e : element.getGiven()) 21927 composeStringCore(null, e, true); 21928 closeArray(); 21929 if (anyHasExtras(element.getGiven())) { 21930 openArray("_given"); 21931 for (StringType e : element.getGiven()) 21932 composeStringExtras(null, e, true); 21933 closeArray(); 21934 } 21935 }; 21936 if (element.hasPrefix()) { 21937 openArray("prefix"); 21938 for (StringType e : element.getPrefix()) 21939 composeStringCore(null, e, true); 21940 closeArray(); 21941 if (anyHasExtras(element.getPrefix())) { 21942 openArray("_prefix"); 21943 for (StringType e : element.getPrefix()) 21944 composeStringExtras(null, e, true); 21945 closeArray(); 21946 } 21947 }; 21948 if (element.hasSuffix()) { 21949 openArray("suffix"); 21950 for (StringType e : element.getSuffix()) 21951 composeStringCore(null, e, true); 21952 closeArray(); 21953 if (anyHasExtras(element.getSuffix())) { 21954 openArray("_suffix"); 21955 for (StringType e : element.getSuffix()) 21956 composeStringExtras(null, e, true); 21957 closeArray(); 21958 } 21959 }; 21960 if (element.hasPeriod()) { 21961 composePeriod("period", element.getPeriod()); 21962 } 21963 } 21964 21965 protected void composeContactPoint(String name, ContactPoint element) throws IOException { 21966 if (element != null) { 21967 open(name); 21968 composeContactPointInner(element); 21969 close(); 21970 } 21971 } 21972 21973 protected void composeContactPointInner(ContactPoint element) throws IOException { 21974 composeElement(element); 21975 if (element.hasSystemElement()) { 21976 composeEnumerationCore("system", element.getSystemElement(), new ContactPoint.ContactPointSystemEnumFactory(), false); 21977 composeEnumerationExtras("system", element.getSystemElement(), new ContactPoint.ContactPointSystemEnumFactory(), false); 21978 } 21979 if (element.hasValueElement()) { 21980 composeStringCore("value", element.getValueElement(), false); 21981 composeStringExtras("value", element.getValueElement(), false); 21982 } 21983 if (element.hasUseElement()) { 21984 composeEnumerationCore("use", element.getUseElement(), new ContactPoint.ContactPointUseEnumFactory(), false); 21985 composeEnumerationExtras("use", element.getUseElement(), new ContactPoint.ContactPointUseEnumFactory(), false); 21986 } 21987 if (element.hasRankElement()) { 21988 composePositiveIntCore("rank", element.getRankElement(), false); 21989 composePositiveIntExtras("rank", element.getRankElement(), false); 21990 } 21991 if (element.hasPeriod()) { 21992 composePeriod("period", element.getPeriod()); 21993 } 21994 } 21995 21996 protected void composeUsageContext(String name, UsageContext element) throws IOException { 21997 if (element != null) { 21998 open(name); 21999 composeUsageContextInner(element); 22000 close(); 22001 } 22002 } 22003 22004 protected void composeUsageContextInner(UsageContext element) throws IOException { 22005 composeElement(element); 22006 if (element.hasCode()) { 22007 composeCoding("code", element.getCode()); 22008 } 22009 if (element.hasValue()) { 22010 composeType("value", element.getValue()); 22011 } 22012 } 22013 22014 protected void composeTiming(String name, Timing element) throws IOException { 22015 if (element != null) { 22016 open(name); 22017 composeTimingInner(element); 22018 close(); 22019 } 22020 } 22021 22022 protected void composeTimingInner(Timing element) throws IOException { 22023 composeElement(element); 22024 if (element.hasEvent()) { 22025 openArray("event"); 22026 for (DateTimeType e : element.getEvent()) 22027 composeDateTimeCore(null, e, true); 22028 closeArray(); 22029 if (anyHasExtras(element.getEvent())) { 22030 openArray("_event"); 22031 for (DateTimeType e : element.getEvent()) 22032 composeDateTimeExtras(null, e, true); 22033 closeArray(); 22034 } 22035 }; 22036 if (element.hasRepeat()) { 22037 composeTimingTimingRepeatComponent("repeat", element.getRepeat()); 22038 } 22039 if (element.hasCode()) { 22040 composeCodeableConcept("code", element.getCode()); 22041 } 22042 } 22043 22044 protected void composeTimingTimingRepeatComponent(String name, Timing.TimingRepeatComponent element) throws IOException { 22045 if (element != null) { 22046 open(name); 22047 composeTimingTimingRepeatComponentInner(element); 22048 close(); 22049 } 22050 } 22051 22052 protected void composeTimingTimingRepeatComponentInner(Timing.TimingRepeatComponent element) throws IOException { 22053 composeElement(element); 22054 if (element.hasBounds()) { 22055 composeType("bounds", element.getBounds()); 22056 } 22057 if (element.hasCountElement()) { 22058 composeIntegerCore("count", element.getCountElement(), false); 22059 composeIntegerExtras("count", element.getCountElement(), false); 22060 } 22061 if (element.hasCountMaxElement()) { 22062 composeIntegerCore("countMax", element.getCountMaxElement(), false); 22063 composeIntegerExtras("countMax", element.getCountMaxElement(), false); 22064 } 22065 if (element.hasDurationElement()) { 22066 composeDecimalCore("duration", element.getDurationElement(), false); 22067 composeDecimalExtras("duration", element.getDurationElement(), false); 22068 } 22069 if (element.hasDurationMaxElement()) { 22070 composeDecimalCore("durationMax", element.getDurationMaxElement(), false); 22071 composeDecimalExtras("durationMax", element.getDurationMaxElement(), false); 22072 } 22073 if (element.hasDurationUnitElement()) { 22074 composeEnumerationCore("durationUnit", element.getDurationUnitElement(), new Timing.UnitsOfTimeEnumFactory(), false); 22075 composeEnumerationExtras("durationUnit", element.getDurationUnitElement(), new Timing.UnitsOfTimeEnumFactory(), false); 22076 } 22077 if (element.hasFrequencyElement()) { 22078 composeIntegerCore("frequency", element.getFrequencyElement(), false); 22079 composeIntegerExtras("frequency", element.getFrequencyElement(), false); 22080 } 22081 if (element.hasFrequencyMaxElement()) { 22082 composeIntegerCore("frequencyMax", element.getFrequencyMaxElement(), false); 22083 composeIntegerExtras("frequencyMax", element.getFrequencyMaxElement(), false); 22084 } 22085 if (element.hasPeriodElement()) { 22086 composeDecimalCore("period", element.getPeriodElement(), false); 22087 composeDecimalExtras("period", element.getPeriodElement(), false); 22088 } 22089 if (element.hasPeriodMaxElement()) { 22090 composeDecimalCore("periodMax", element.getPeriodMaxElement(), false); 22091 composeDecimalExtras("periodMax", element.getPeriodMaxElement(), false); 22092 } 22093 if (element.hasPeriodUnitElement()) { 22094 composeEnumerationCore("periodUnit", element.getPeriodUnitElement(), new Timing.UnitsOfTimeEnumFactory(), false); 22095 composeEnumerationExtras("periodUnit", element.getPeriodUnitElement(), new Timing.UnitsOfTimeEnumFactory(), false); 22096 } 22097 if (element.hasDayOfWeek()) { 22098 openArray("dayOfWeek"); 22099 for (Enumeration<Timing.DayOfWeek> e : element.getDayOfWeek()) 22100 composeEnumerationCore(null, e, new Timing.DayOfWeekEnumFactory(), true); 22101 closeArray(); 22102 if (anyHasExtras(element.getDayOfWeek())) { 22103 openArray("_dayOfWeek"); 22104 for (Enumeration<Timing.DayOfWeek> e : element.getDayOfWeek()) 22105 composeEnumerationExtras(null, e, new Timing.DayOfWeekEnumFactory(), true); 22106 closeArray(); 22107 } 22108 }; 22109 if (element.hasTimeOfDay()) { 22110 openArray("timeOfDay"); 22111 for (TimeType e : element.getTimeOfDay()) 22112 composeTimeCore(null, e, true); 22113 closeArray(); 22114 if (anyHasExtras(element.getTimeOfDay())) { 22115 openArray("_timeOfDay"); 22116 for (TimeType e : element.getTimeOfDay()) 22117 composeTimeExtras(null, e, true); 22118 closeArray(); 22119 } 22120 }; 22121 if (element.hasWhen()) { 22122 openArray("when"); 22123 for (Enumeration<Timing.EventTiming> e : element.getWhen()) 22124 composeEnumerationCore(null, e, new Timing.EventTimingEnumFactory(), true); 22125 closeArray(); 22126 if (anyHasExtras(element.getWhen())) { 22127 openArray("_when"); 22128 for (Enumeration<Timing.EventTiming> e : element.getWhen()) 22129 composeEnumerationExtras(null, e, new Timing.EventTimingEnumFactory(), true); 22130 closeArray(); 22131 } 22132 }; 22133 if (element.hasOffsetElement()) { 22134 composeUnsignedIntCore("offset", element.getOffsetElement(), false); 22135 composeUnsignedIntExtras("offset", element.getOffsetElement(), false); 22136 } 22137 } 22138 22139 protected void composeElementDefinition(String name, ElementDefinition element) throws IOException { 22140 if (element != null) { 22141 open(name); 22142 composeElementDefinitionInner(element); 22143 close(); 22144 } 22145 } 22146 22147 protected void composeElementDefinitionInner(ElementDefinition element) throws IOException { 22148 composeElement(element); 22149 if (element.hasPathElement()) { 22150 composeStringCore("path", element.getPathElement(), false); 22151 composeStringExtras("path", element.getPathElement(), false); 22152 } 22153 if (element.hasRepresentation()) { 22154 openArray("representation"); 22155 for (Enumeration<ElementDefinition.PropertyRepresentation> e : element.getRepresentation()) 22156 composeEnumerationCore(null, e, new ElementDefinition.PropertyRepresentationEnumFactory(), true); 22157 closeArray(); 22158 if (anyHasExtras(element.getRepresentation())) { 22159 openArray("_representation"); 22160 for (Enumeration<ElementDefinition.PropertyRepresentation> e : element.getRepresentation()) 22161 composeEnumerationExtras(null, e, new ElementDefinition.PropertyRepresentationEnumFactory(), true); 22162 closeArray(); 22163 } 22164 }; 22165 if (element.hasSliceNameElement()) { 22166 composeStringCore("sliceName", element.getSliceNameElement(), false); 22167 composeStringExtras("sliceName", element.getSliceNameElement(), false); 22168 } 22169 if (element.hasLabelElement()) { 22170 composeStringCore("label", element.getLabelElement(), false); 22171 composeStringExtras("label", element.getLabelElement(), false); 22172 } 22173 if (element.hasCode()) { 22174 openArray("code"); 22175 for (Coding e : element.getCode()) 22176 composeCoding(null, e); 22177 closeArray(); 22178 }; 22179 if (element.hasSlicing()) { 22180 composeElementDefinitionElementDefinitionSlicingComponent("slicing", element.getSlicing()); 22181 } 22182 if (element.hasShortElement()) { 22183 composeStringCore("short", element.getShortElement(), false); 22184 composeStringExtras("short", element.getShortElement(), false); 22185 } 22186 if (element.hasDefinitionElement()) { 22187 composeMarkdownCore("definition", element.getDefinitionElement(), false); 22188 composeMarkdownExtras("definition", element.getDefinitionElement(), false); 22189 } 22190 if (element.hasCommentElement()) { 22191 composeMarkdownCore("comment", element.getCommentElement(), false); 22192 composeMarkdownExtras("comment", element.getCommentElement(), false); 22193 } 22194 if (element.hasRequirementsElement()) { 22195 composeMarkdownCore("requirements", element.getRequirementsElement(), false); 22196 composeMarkdownExtras("requirements", element.getRequirementsElement(), false); 22197 } 22198 if (element.hasAlias()) { 22199 openArray("alias"); 22200 for (StringType e : element.getAlias()) 22201 composeStringCore(null, e, true); 22202 closeArray(); 22203 if (anyHasExtras(element.getAlias())) { 22204 openArray("_alias"); 22205 for (StringType e : element.getAlias()) 22206 composeStringExtras(null, e, true); 22207 closeArray(); 22208 } 22209 }; 22210 if (element.hasMinElement()) { 22211 composeUnsignedIntCore("min", element.getMinElement(), false); 22212 composeUnsignedIntExtras("min", element.getMinElement(), false); 22213 } 22214 if (element.hasMaxElement()) { 22215 composeStringCore("max", element.getMaxElement(), false); 22216 composeStringExtras("max", element.getMaxElement(), false); 22217 } 22218 if (element.hasBase()) { 22219 composeElementDefinitionElementDefinitionBaseComponent("base", element.getBase()); 22220 } 22221 if (element.hasContentReferenceElement()) { 22222 composeUriCore("contentReference", element.getContentReferenceElement(), false); 22223 composeUriExtras("contentReference", element.getContentReferenceElement(), false); 22224 } 22225 if (element.hasType()) { 22226 openArray("type"); 22227 for (ElementDefinition.TypeRefComponent e : element.getType()) 22228 composeElementDefinitionTypeRefComponent(null, e); 22229 closeArray(); 22230 }; 22231 if (element.hasDefaultValue()) { 22232 composeType("defaultValue", element.getDefaultValue()); 22233 } 22234 if (element.hasMeaningWhenMissingElement()) { 22235 composeMarkdownCore("meaningWhenMissing", element.getMeaningWhenMissingElement(), false); 22236 composeMarkdownExtras("meaningWhenMissing", element.getMeaningWhenMissingElement(), false); 22237 } 22238 if (element.hasOrderMeaningElement()) { 22239 composeStringCore("orderMeaning", element.getOrderMeaningElement(), false); 22240 composeStringExtras("orderMeaning", element.getOrderMeaningElement(), false); 22241 } 22242 if (element.hasFixed()) { 22243 composeType("fixed", element.getFixed()); 22244 } 22245 if (element.hasPattern()) { 22246 composeType("pattern", element.getPattern()); 22247 } 22248 if (element.hasExample()) { 22249 openArray("example"); 22250 for (ElementDefinition.ElementDefinitionExampleComponent e : element.getExample()) 22251 composeElementDefinitionElementDefinitionExampleComponent(null, e); 22252 closeArray(); 22253 }; 22254 if (element.hasMinValue()) { 22255 composeType("minValue", element.getMinValue()); 22256 } 22257 if (element.hasMaxValue()) { 22258 composeType("maxValue", element.getMaxValue()); 22259 } 22260 if (element.hasMaxLengthElement()) { 22261 composeIntegerCore("maxLength", element.getMaxLengthElement(), false); 22262 composeIntegerExtras("maxLength", element.getMaxLengthElement(), false); 22263 } 22264 if (element.hasCondition()) { 22265 openArray("condition"); 22266 for (IdType e : element.getCondition()) 22267 composeIdCore(null, e, true); 22268 closeArray(); 22269 if (anyHasExtras(element.getCondition())) { 22270 openArray("_condition"); 22271 for (IdType e : element.getCondition()) 22272 composeIdExtras(null, e, true); 22273 closeArray(); 22274 } 22275 }; 22276 if (element.hasConstraint()) { 22277 openArray("constraint"); 22278 for (ElementDefinition.ElementDefinitionConstraintComponent e : element.getConstraint()) 22279 composeElementDefinitionElementDefinitionConstraintComponent(null, e); 22280 closeArray(); 22281 }; 22282 if (element.hasMustSupportElement()) { 22283 composeBooleanCore("mustSupport", element.getMustSupportElement(), false); 22284 composeBooleanExtras("mustSupport", element.getMustSupportElement(), false); 22285 } 22286 if (element.hasIsModifierElement()) { 22287 composeBooleanCore("isModifier", element.getIsModifierElement(), false); 22288 composeBooleanExtras("isModifier", element.getIsModifierElement(), false); 22289 } 22290 if (element.hasIsSummaryElement()) { 22291 composeBooleanCore("isSummary", element.getIsSummaryElement(), false); 22292 composeBooleanExtras("isSummary", element.getIsSummaryElement(), false); 22293 } 22294 if (element.hasBinding()) { 22295 composeElementDefinitionElementDefinitionBindingComponent("binding", element.getBinding()); 22296 } 22297 if (element.hasMapping()) { 22298 openArray("mapping"); 22299 for (ElementDefinition.ElementDefinitionMappingComponent e : element.getMapping()) 22300 composeElementDefinitionElementDefinitionMappingComponent(null, e); 22301 closeArray(); 22302 }; 22303 } 22304 22305 protected void composeElementDefinitionElementDefinitionSlicingComponent(String name, ElementDefinition.ElementDefinitionSlicingComponent element) throws IOException { 22306 if (element != null) { 22307 open(name); 22308 composeElementDefinitionElementDefinitionSlicingComponentInner(element); 22309 close(); 22310 } 22311 } 22312 22313 protected void composeElementDefinitionElementDefinitionSlicingComponentInner(ElementDefinition.ElementDefinitionSlicingComponent element) throws IOException { 22314 composeElement(element); 22315 if (element.hasDiscriminator()) { 22316 openArray("discriminator"); 22317 for (ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent e : element.getDiscriminator()) 22318 composeElementDefinitionElementDefinitionSlicingDiscriminatorComponent(null, e); 22319 closeArray(); 22320 }; 22321 if (element.hasDescriptionElement()) { 22322 composeStringCore("description", element.getDescriptionElement(), false); 22323 composeStringExtras("description", element.getDescriptionElement(), false); 22324 } 22325 if (element.hasOrderedElement()) { 22326 composeBooleanCore("ordered", element.getOrderedElement(), false); 22327 composeBooleanExtras("ordered", element.getOrderedElement(), false); 22328 } 22329 if (element.hasRulesElement()) { 22330 composeEnumerationCore("rules", element.getRulesElement(), new ElementDefinition.SlicingRulesEnumFactory(), false); 22331 composeEnumerationExtras("rules", element.getRulesElement(), new ElementDefinition.SlicingRulesEnumFactory(), false); 22332 } 22333 } 22334 22335 protected void composeElementDefinitionElementDefinitionSlicingDiscriminatorComponent(String name, ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent element) throws IOException { 22336 if (element != null) { 22337 open(name); 22338 composeElementDefinitionElementDefinitionSlicingDiscriminatorComponentInner(element); 22339 close(); 22340 } 22341 } 22342 22343 protected void composeElementDefinitionElementDefinitionSlicingDiscriminatorComponentInner(ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent element) throws IOException { 22344 composeElement(element); 22345 if (element.hasTypeElement()) { 22346 composeEnumerationCore("type", element.getTypeElement(), new ElementDefinition.DiscriminatorTypeEnumFactory(), false); 22347 composeEnumerationExtras("type", element.getTypeElement(), new ElementDefinition.DiscriminatorTypeEnumFactory(), false); 22348 } 22349 if (element.hasPathElement()) { 22350 composeStringCore("path", element.getPathElement(), false); 22351 composeStringExtras("path", element.getPathElement(), false); 22352 } 22353 } 22354 22355 protected void composeElementDefinitionElementDefinitionBaseComponent(String name, ElementDefinition.ElementDefinitionBaseComponent element) throws IOException { 22356 if (element != null) { 22357 open(name); 22358 composeElementDefinitionElementDefinitionBaseComponentInner(element); 22359 close(); 22360 } 22361 } 22362 22363 protected void composeElementDefinitionElementDefinitionBaseComponentInner(ElementDefinition.ElementDefinitionBaseComponent element) throws IOException { 22364 composeElement(element); 22365 if (element.hasPathElement()) { 22366 composeStringCore("path", element.getPathElement(), false); 22367 composeStringExtras("path", element.getPathElement(), false); 22368 } 22369 if (element.hasMinElement()) { 22370 composeUnsignedIntCore("min", element.getMinElement(), false); 22371 composeUnsignedIntExtras("min", element.getMinElement(), false); 22372 } 22373 if (element.hasMaxElement()) { 22374 composeStringCore("max", element.getMaxElement(), false); 22375 composeStringExtras("max", element.getMaxElement(), false); 22376 } 22377 } 22378 22379 protected void composeElementDefinitionTypeRefComponent(String name, ElementDefinition.TypeRefComponent element) throws IOException { 22380 if (element != null) { 22381 open(name); 22382 composeElementDefinitionTypeRefComponentInner(element); 22383 close(); 22384 } 22385 } 22386 22387 protected void composeElementDefinitionTypeRefComponentInner(ElementDefinition.TypeRefComponent element) throws IOException { 22388 composeElement(element); 22389 if (element.hasCodeElement()) { 22390 composeUriCore("code", element.getCodeElement(), false); 22391 composeUriExtras("code", element.getCodeElement(), false); 22392 } 22393 if (element.hasProfileElement()) { 22394 composeUriCore("profile", element.getProfileElement(), false); 22395 composeUriExtras("profile", element.getProfileElement(), false); 22396 } 22397 if (element.hasTargetProfileElement()) { 22398 composeUriCore("targetProfile", element.getTargetProfileElement(), false); 22399 composeUriExtras("targetProfile", element.getTargetProfileElement(), false); 22400 } 22401 if (element.hasAggregation()) { 22402 openArray("aggregation"); 22403 for (Enumeration<ElementDefinition.AggregationMode> e : element.getAggregation()) 22404 composeEnumerationCore(null, e, new ElementDefinition.AggregationModeEnumFactory(), true); 22405 closeArray(); 22406 if (anyHasExtras(element.getAggregation())) { 22407 openArray("_aggregation"); 22408 for (Enumeration<ElementDefinition.AggregationMode> e : element.getAggregation()) 22409 composeEnumerationExtras(null, e, new ElementDefinition.AggregationModeEnumFactory(), true); 22410 closeArray(); 22411 } 22412 }; 22413 if (element.hasVersioningElement()) { 22414 composeEnumerationCore("versioning", element.getVersioningElement(), new ElementDefinition.ReferenceVersionRulesEnumFactory(), false); 22415 composeEnumerationExtras("versioning", element.getVersioningElement(), new ElementDefinition.ReferenceVersionRulesEnumFactory(), false); 22416 } 22417 } 22418 22419 protected void composeElementDefinitionElementDefinitionExampleComponent(String name, ElementDefinition.ElementDefinitionExampleComponent element) throws IOException { 22420 if (element != null) { 22421 open(name); 22422 composeElementDefinitionElementDefinitionExampleComponentInner(element); 22423 close(); 22424 } 22425 } 22426 22427 protected void composeElementDefinitionElementDefinitionExampleComponentInner(ElementDefinition.ElementDefinitionExampleComponent element) throws IOException { 22428 composeElement(element); 22429 if (element.hasLabelElement()) { 22430 composeStringCore("label", element.getLabelElement(), false); 22431 composeStringExtras("label", element.getLabelElement(), false); 22432 } 22433 if (element.hasValue()) { 22434 composeType("value", element.getValue()); 22435 } 22436 } 22437 22438 protected void composeElementDefinitionElementDefinitionConstraintComponent(String name, ElementDefinition.ElementDefinitionConstraintComponent element) throws IOException { 22439 if (element != null) { 22440 open(name); 22441 composeElementDefinitionElementDefinitionConstraintComponentInner(element); 22442 close(); 22443 } 22444 } 22445 22446 protected void composeElementDefinitionElementDefinitionConstraintComponentInner(ElementDefinition.ElementDefinitionConstraintComponent element) throws IOException { 22447 composeElement(element); 22448 if (element.hasKeyElement()) { 22449 composeIdCore("key", element.getKeyElement(), false); 22450 composeIdExtras("key", element.getKeyElement(), false); 22451 } 22452 if (element.hasRequirementsElement()) { 22453 composeStringCore("requirements", element.getRequirementsElement(), false); 22454 composeStringExtras("requirements", element.getRequirementsElement(), false); 22455 } 22456 if (element.hasSeverityElement()) { 22457 composeEnumerationCore("severity", element.getSeverityElement(), new ElementDefinition.ConstraintSeverityEnumFactory(), false); 22458 composeEnumerationExtras("severity", element.getSeverityElement(), new ElementDefinition.ConstraintSeverityEnumFactory(), false); 22459 } 22460 if (element.hasHumanElement()) { 22461 composeStringCore("human", element.getHumanElement(), false); 22462 composeStringExtras("human", element.getHumanElement(), false); 22463 } 22464 if (element.hasExpressionElement()) { 22465 composeStringCore("expression", element.getExpressionElement(), false); 22466 composeStringExtras("expression", element.getExpressionElement(), false); 22467 } 22468 if (element.hasXpathElement()) { 22469 composeStringCore("xpath", element.getXpathElement(), false); 22470 composeStringExtras("xpath", element.getXpathElement(), false); 22471 } 22472 if (element.hasSourceElement()) { 22473 composeUriCore("source", element.getSourceElement(), false); 22474 composeUriExtras("source", element.getSourceElement(), false); 22475 } 22476 } 22477 22478 protected void composeElementDefinitionElementDefinitionBindingComponent(String name, ElementDefinition.ElementDefinitionBindingComponent element) throws IOException { 22479 if (element != null) { 22480 open(name); 22481 composeElementDefinitionElementDefinitionBindingComponentInner(element); 22482 close(); 22483 } 22484 } 22485 22486 protected void composeElementDefinitionElementDefinitionBindingComponentInner(ElementDefinition.ElementDefinitionBindingComponent element) throws IOException { 22487 composeElement(element); 22488 if (element.hasStrengthElement()) { 22489 composeEnumerationCore("strength", element.getStrengthElement(), new Enumerations.BindingStrengthEnumFactory(), false); 22490 composeEnumerationExtras("strength", element.getStrengthElement(), new Enumerations.BindingStrengthEnumFactory(), false); 22491 } 22492 if (element.hasDescriptionElement()) { 22493 composeStringCore("description", element.getDescriptionElement(), false); 22494 composeStringExtras("description", element.getDescriptionElement(), false); 22495 } 22496 if (element.hasValueSet()) { 22497 composeType("valueSet", element.getValueSet()); 22498 } 22499 } 22500 22501 protected void composeElementDefinitionElementDefinitionMappingComponent(String name, ElementDefinition.ElementDefinitionMappingComponent element) throws IOException { 22502 if (element != null) { 22503 open(name); 22504 composeElementDefinitionElementDefinitionMappingComponentInner(element); 22505 close(); 22506 } 22507 } 22508 22509 protected void composeElementDefinitionElementDefinitionMappingComponentInner(ElementDefinition.ElementDefinitionMappingComponent element) throws IOException { 22510 composeElement(element); 22511 if (element.hasIdentityElement()) { 22512 composeIdCore("identity", element.getIdentityElement(), false); 22513 composeIdExtras("identity", element.getIdentityElement(), false); 22514 } 22515 if (element.hasLanguageElement()) { 22516 composeCodeCore("language", element.getLanguageElement(), false); 22517 composeCodeExtras("language", element.getLanguageElement(), false); 22518 } 22519 if (element.hasMapElement()) { 22520 composeStringCore("map", element.getMapElement(), false); 22521 composeStringExtras("map", element.getMapElement(), false); 22522 } 22523 if (element.hasCommentElement()) { 22524 composeStringCore("comment", element.getCommentElement(), false); 22525 composeStringExtras("comment", element.getCommentElement(), false); 22526 } 22527 } 22528 22529 protected void composeParameterDefinition(String name, ParameterDefinition element) throws IOException { 22530 if (element != null) { 22531 open(name); 22532 composeParameterDefinitionInner(element); 22533 close(); 22534 } 22535 } 22536 22537 protected void composeParameterDefinitionInner(ParameterDefinition element) throws IOException { 22538 composeElement(element); 22539 if (element.hasNameElement()) { 22540 composeCodeCore("name", element.getNameElement(), false); 22541 composeCodeExtras("name", element.getNameElement(), false); 22542 } 22543 if (element.hasUseElement()) { 22544 composeEnumerationCore("use", element.getUseElement(), new ParameterDefinition.ParameterUseEnumFactory(), false); 22545 composeEnumerationExtras("use", element.getUseElement(), new ParameterDefinition.ParameterUseEnumFactory(), false); 22546 } 22547 if (element.hasMinElement()) { 22548 composeIntegerCore("min", element.getMinElement(), false); 22549 composeIntegerExtras("min", element.getMinElement(), false); 22550 } 22551 if (element.hasMaxElement()) { 22552 composeStringCore("max", element.getMaxElement(), false); 22553 composeStringExtras("max", element.getMaxElement(), false); 22554 } 22555 if (element.hasDocumentationElement()) { 22556 composeStringCore("documentation", element.getDocumentationElement(), false); 22557 composeStringExtras("documentation", element.getDocumentationElement(), false); 22558 } 22559 if (element.hasTypeElement()) { 22560 composeCodeCore("type", element.getTypeElement(), false); 22561 composeCodeExtras("type", element.getTypeElement(), false); 22562 } 22563 if (element.hasProfile()) { 22564 composeReference("profile", element.getProfile()); 22565 } 22566 } 22567 22568 protected void composeDomainResourceElements(DomainResource element) throws IOException { 22569 composeResourceElements(element); 22570 if (element.hasText()) { 22571 composeNarrative("text", element.getText()); 22572 } 22573 if (element.hasContained()) { 22574 openArray("contained"); 22575 for (Resource e : element.getContained()) { 22576 open(null); 22577 composeResource(e); 22578 close(); 22579 } 22580 closeArray(); 22581 }; 22582 if (element.hasExtension()) { 22583 openArray("extension"); 22584 for (Extension e : element.getExtension()) 22585 composeExtension(null, e); 22586 closeArray(); 22587 }; 22588 if (element.hasModifierExtension()) { 22589 openArray("modifierExtension"); 22590 for (Extension e : element.getModifierExtension()) 22591 composeExtension(null, e); 22592 closeArray(); 22593 }; 22594 } 22595 22596 protected void composeParameters(String name, Parameters element) throws IOException { 22597 if (element != null) { 22598 prop("resourceType", name); 22599 composeParametersInner(element); 22600 } 22601 } 22602 22603 protected void composeParametersInner(Parameters element) throws IOException { 22604 composeResourceElements(element); 22605 if (element.hasParameter()) { 22606 openArray("parameter"); 22607 for (Parameters.ParametersParameterComponent e : element.getParameter()) 22608 composeParametersParametersParameterComponent(null, e); 22609 closeArray(); 22610 }; 22611 } 22612 22613 protected void composeParametersParametersParameterComponent(String name, Parameters.ParametersParameterComponent element) throws IOException { 22614 if (element != null) { 22615 open(name); 22616 composeParametersParametersParameterComponentInner(element); 22617 close(); 22618 } 22619 } 22620 22621 protected void composeParametersParametersParameterComponentInner(Parameters.ParametersParameterComponent element) throws IOException { 22622 composeBackbone(element); 22623 if (element.hasNameElement()) { 22624 composeStringCore("name", element.getNameElement(), false); 22625 composeStringExtras("name", element.getNameElement(), false); 22626 } 22627 if (element.hasValue()) { 22628 composeType("value", element.getValue()); 22629 } 22630 if (element.hasResource()) { 22631 open("resource"); 22632 composeResource(element.getResource()); 22633 close(); 22634 } 22635 if (element.hasPart()) { 22636 openArray("part"); 22637 for (Parameters.ParametersParameterComponent e : element.getPart()) 22638 composeParametersParametersParameterComponent(null, e); 22639 closeArray(); 22640 }; 22641 } 22642 22643 protected void composeResourceElements(Resource element) throws IOException { 22644 if (element.hasIdElement()) { 22645 composeIdCore("id", element.getIdElement(), false); 22646 composeIdExtras("id", element.getIdElement(), false); 22647 } 22648 if (element.hasMeta()) { 22649 composeMeta("meta", element.getMeta()); 22650 } 22651 if (element.hasImplicitRulesElement()) { 22652 composeUriCore("implicitRules", element.getImplicitRulesElement(), false); 22653 composeUriExtras("implicitRules", element.getImplicitRulesElement(), false); 22654 } 22655 if (element.hasLanguageElement()) { 22656 composeCodeCore("language", element.getLanguageElement(), false); 22657 composeCodeExtras("language", element.getLanguageElement(), false); 22658 } 22659 } 22660 22661 protected void composeAccount(String name, Account element) throws IOException { 22662 if (element != null) { 22663 prop("resourceType", name); 22664 composeAccountInner(element); 22665 } 22666 } 22667 22668 protected void composeAccountInner(Account element) throws IOException { 22669 composeDomainResourceElements(element); 22670 if (element.hasIdentifier()) { 22671 openArray("identifier"); 22672 for (Identifier e : element.getIdentifier()) 22673 composeIdentifier(null, e); 22674 closeArray(); 22675 }; 22676 if (element.hasStatusElement()) { 22677 composeEnumerationCore("status", element.getStatusElement(), new Account.AccountStatusEnumFactory(), false); 22678 composeEnumerationExtras("status", element.getStatusElement(), new Account.AccountStatusEnumFactory(), false); 22679 } 22680 if (element.hasType()) { 22681 composeCodeableConcept("type", element.getType()); 22682 } 22683 if (element.hasNameElement()) { 22684 composeStringCore("name", element.getNameElement(), false); 22685 composeStringExtras("name", element.getNameElement(), false); 22686 } 22687 if (element.hasSubject()) { 22688 composeReference("subject", element.getSubject()); 22689 } 22690 if (element.hasPeriod()) { 22691 composePeriod("period", element.getPeriod()); 22692 } 22693 if (element.hasActive()) { 22694 composePeriod("active", element.getActive()); 22695 } 22696 if (element.hasBalance()) { 22697 composeMoney("balance", element.getBalance()); 22698 } 22699 if (element.hasCoverage()) { 22700 openArray("coverage"); 22701 for (Account.CoverageComponent e : element.getCoverage()) 22702 composeAccountCoverageComponent(null, e); 22703 closeArray(); 22704 }; 22705 if (element.hasOwner()) { 22706 composeReference("owner", element.getOwner()); 22707 } 22708 if (element.hasDescriptionElement()) { 22709 composeStringCore("description", element.getDescriptionElement(), false); 22710 composeStringExtras("description", element.getDescriptionElement(), false); 22711 } 22712 if (element.hasGuarantor()) { 22713 openArray("guarantor"); 22714 for (Account.GuarantorComponent e : element.getGuarantor()) 22715 composeAccountGuarantorComponent(null, e); 22716 closeArray(); 22717 }; 22718 } 22719 22720 protected void composeAccountCoverageComponent(String name, Account.CoverageComponent element) throws IOException { 22721 if (element != null) { 22722 open(name); 22723 composeAccountCoverageComponentInner(element); 22724 close(); 22725 } 22726 } 22727 22728 protected void composeAccountCoverageComponentInner(Account.CoverageComponent element) throws IOException { 22729 composeBackbone(element); 22730 if (element.hasCoverage()) { 22731 composeReference("coverage", element.getCoverage()); 22732 } 22733 if (element.hasPriorityElement()) { 22734 composePositiveIntCore("priority", element.getPriorityElement(), false); 22735 composePositiveIntExtras("priority", element.getPriorityElement(), false); 22736 } 22737 } 22738 22739 protected void composeAccountGuarantorComponent(String name, Account.GuarantorComponent element) throws IOException { 22740 if (element != null) { 22741 open(name); 22742 composeAccountGuarantorComponentInner(element); 22743 close(); 22744 } 22745 } 22746 22747 protected void composeAccountGuarantorComponentInner(Account.GuarantorComponent element) throws IOException { 22748 composeBackbone(element); 22749 if (element.hasParty()) { 22750 composeReference("party", element.getParty()); 22751 } 22752 if (element.hasOnHoldElement()) { 22753 composeBooleanCore("onHold", element.getOnHoldElement(), false); 22754 composeBooleanExtras("onHold", element.getOnHoldElement(), false); 22755 } 22756 if (element.hasPeriod()) { 22757 composePeriod("period", element.getPeriod()); 22758 } 22759 } 22760 22761 protected void composeActivityDefinition(String name, ActivityDefinition element) throws IOException { 22762 if (element != null) { 22763 prop("resourceType", name); 22764 composeActivityDefinitionInner(element); 22765 } 22766 } 22767 22768 protected void composeActivityDefinitionInner(ActivityDefinition element) throws IOException { 22769 composeDomainResourceElements(element); 22770 if (element.hasUrlElement()) { 22771 composeUriCore("url", element.getUrlElement(), false); 22772 composeUriExtras("url", element.getUrlElement(), false); 22773 } 22774 if (element.hasIdentifier()) { 22775 openArray("identifier"); 22776 for (Identifier e : element.getIdentifier()) 22777 composeIdentifier(null, e); 22778 closeArray(); 22779 }; 22780 if (element.hasVersionElement()) { 22781 composeStringCore("version", element.getVersionElement(), false); 22782 composeStringExtras("version", element.getVersionElement(), false); 22783 } 22784 if (element.hasNameElement()) { 22785 composeStringCore("name", element.getNameElement(), false); 22786 composeStringExtras("name", element.getNameElement(), false); 22787 } 22788 if (element.hasTitleElement()) { 22789 composeStringCore("title", element.getTitleElement(), false); 22790 composeStringExtras("title", element.getTitleElement(), false); 22791 } 22792 if (element.hasStatusElement()) { 22793 composeEnumerationCore("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 22794 composeEnumerationExtras("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 22795 } 22796 if (element.hasExperimentalElement()) { 22797 composeBooleanCore("experimental", element.getExperimentalElement(), false); 22798 composeBooleanExtras("experimental", element.getExperimentalElement(), false); 22799 } 22800 if (element.hasDateElement()) { 22801 composeDateTimeCore("date", element.getDateElement(), false); 22802 composeDateTimeExtras("date", element.getDateElement(), false); 22803 } 22804 if (element.hasPublisherElement()) { 22805 composeStringCore("publisher", element.getPublisherElement(), false); 22806 composeStringExtras("publisher", element.getPublisherElement(), false); 22807 } 22808 if (element.hasDescriptionElement()) { 22809 composeMarkdownCore("description", element.getDescriptionElement(), false); 22810 composeMarkdownExtras("description", element.getDescriptionElement(), false); 22811 } 22812 if (element.hasPurposeElement()) { 22813 composeMarkdownCore("purpose", element.getPurposeElement(), false); 22814 composeMarkdownExtras("purpose", element.getPurposeElement(), false); 22815 } 22816 if (element.hasUsageElement()) { 22817 composeStringCore("usage", element.getUsageElement(), false); 22818 composeStringExtras("usage", element.getUsageElement(), false); 22819 } 22820 if (element.hasApprovalDateElement()) { 22821 composeDateCore("approvalDate", element.getApprovalDateElement(), false); 22822 composeDateExtras("approvalDate", element.getApprovalDateElement(), false); 22823 } 22824 if (element.hasLastReviewDateElement()) { 22825 composeDateCore("lastReviewDate", element.getLastReviewDateElement(), false); 22826 composeDateExtras("lastReviewDate", element.getLastReviewDateElement(), false); 22827 } 22828 if (element.hasEffectivePeriod()) { 22829 composePeriod("effectivePeriod", element.getEffectivePeriod()); 22830 } 22831 if (element.hasUseContext()) { 22832 openArray("useContext"); 22833 for (UsageContext e : element.getUseContext()) 22834 composeUsageContext(null, e); 22835 closeArray(); 22836 }; 22837 if (element.hasJurisdiction()) { 22838 openArray("jurisdiction"); 22839 for (CodeableConcept e : element.getJurisdiction()) 22840 composeCodeableConcept(null, e); 22841 closeArray(); 22842 }; 22843 if (element.hasTopic()) { 22844 openArray("topic"); 22845 for (CodeableConcept e : element.getTopic()) 22846 composeCodeableConcept(null, e); 22847 closeArray(); 22848 }; 22849 if (element.hasContributor()) { 22850 openArray("contributor"); 22851 for (Contributor e : element.getContributor()) 22852 composeContributor(null, e); 22853 closeArray(); 22854 }; 22855 if (element.hasContact()) { 22856 openArray("contact"); 22857 for (ContactDetail e : element.getContact()) 22858 composeContactDetail(null, e); 22859 closeArray(); 22860 }; 22861 if (element.hasCopyrightElement()) { 22862 composeMarkdownCore("copyright", element.getCopyrightElement(), false); 22863 composeMarkdownExtras("copyright", element.getCopyrightElement(), false); 22864 } 22865 if (element.hasRelatedArtifact()) { 22866 openArray("relatedArtifact"); 22867 for (RelatedArtifact e : element.getRelatedArtifact()) 22868 composeRelatedArtifact(null, e); 22869 closeArray(); 22870 }; 22871 if (element.hasLibrary()) { 22872 openArray("library"); 22873 for (Reference e : element.getLibrary()) 22874 composeReference(null, e); 22875 closeArray(); 22876 }; 22877 if (element.hasKindElement()) { 22878 composeEnumerationCore("kind", element.getKindElement(), new ActivityDefinition.ActivityDefinitionKindEnumFactory(), false); 22879 composeEnumerationExtras("kind", element.getKindElement(), new ActivityDefinition.ActivityDefinitionKindEnumFactory(), false); 22880 } 22881 if (element.hasCode()) { 22882 composeCodeableConcept("code", element.getCode()); 22883 } 22884 if (element.hasTiming()) { 22885 composeType("timing", element.getTiming()); 22886 } 22887 if (element.hasLocation()) { 22888 composeReference("location", element.getLocation()); 22889 } 22890 if (element.hasParticipant()) { 22891 openArray("participant"); 22892 for (ActivityDefinition.ActivityDefinitionParticipantComponent e : element.getParticipant()) 22893 composeActivityDefinitionActivityDefinitionParticipantComponent(null, e); 22894 closeArray(); 22895 }; 22896 if (element.hasProduct()) { 22897 composeType("product", element.getProduct()); 22898 } 22899 if (element.hasQuantity()) { 22900 composeSimpleQuantity("quantity", element.getQuantity()); 22901 } 22902 if (element.hasDosage()) { 22903 openArray("dosage"); 22904 for (Dosage e : element.getDosage()) 22905 composeDosage(null, e); 22906 closeArray(); 22907 }; 22908 if (element.hasBodySite()) { 22909 openArray("bodySite"); 22910 for (CodeableConcept e : element.getBodySite()) 22911 composeCodeableConcept(null, e); 22912 closeArray(); 22913 }; 22914 if (element.hasTransform()) { 22915 composeReference("transform", element.getTransform()); 22916 } 22917 if (element.hasDynamicValue()) { 22918 openArray("dynamicValue"); 22919 for (ActivityDefinition.ActivityDefinitionDynamicValueComponent e : element.getDynamicValue()) 22920 composeActivityDefinitionActivityDefinitionDynamicValueComponent(null, e); 22921 closeArray(); 22922 }; 22923 } 22924 22925 protected void composeActivityDefinitionActivityDefinitionParticipantComponent(String name, ActivityDefinition.ActivityDefinitionParticipantComponent element) throws IOException { 22926 if (element != null) { 22927 open(name); 22928 composeActivityDefinitionActivityDefinitionParticipantComponentInner(element); 22929 close(); 22930 } 22931 } 22932 22933 protected void composeActivityDefinitionActivityDefinitionParticipantComponentInner(ActivityDefinition.ActivityDefinitionParticipantComponent element) throws IOException { 22934 composeBackbone(element); 22935 if (element.hasTypeElement()) { 22936 composeEnumerationCore("type", element.getTypeElement(), new ActivityDefinition.ActivityParticipantTypeEnumFactory(), false); 22937 composeEnumerationExtras("type", element.getTypeElement(), new ActivityDefinition.ActivityParticipantTypeEnumFactory(), false); 22938 } 22939 if (element.hasRole()) { 22940 composeCodeableConcept("role", element.getRole()); 22941 } 22942 } 22943 22944 protected void composeActivityDefinitionActivityDefinitionDynamicValueComponent(String name, ActivityDefinition.ActivityDefinitionDynamicValueComponent element) throws IOException { 22945 if (element != null) { 22946 open(name); 22947 composeActivityDefinitionActivityDefinitionDynamicValueComponentInner(element); 22948 close(); 22949 } 22950 } 22951 22952 protected void composeActivityDefinitionActivityDefinitionDynamicValueComponentInner(ActivityDefinition.ActivityDefinitionDynamicValueComponent element) throws IOException { 22953 composeBackbone(element); 22954 if (element.hasDescriptionElement()) { 22955 composeStringCore("description", element.getDescriptionElement(), false); 22956 composeStringExtras("description", element.getDescriptionElement(), false); 22957 } 22958 if (element.hasPathElement()) { 22959 composeStringCore("path", element.getPathElement(), false); 22960 composeStringExtras("path", element.getPathElement(), false); 22961 } 22962 if (element.hasLanguageElement()) { 22963 composeStringCore("language", element.getLanguageElement(), false); 22964 composeStringExtras("language", element.getLanguageElement(), false); 22965 } 22966 if (element.hasExpressionElement()) { 22967 composeStringCore("expression", element.getExpressionElement(), false); 22968 composeStringExtras("expression", element.getExpressionElement(), false); 22969 } 22970 } 22971 22972 protected void composeAdverseEvent(String name, AdverseEvent element) throws IOException { 22973 if (element != null) { 22974 prop("resourceType", name); 22975 composeAdverseEventInner(element); 22976 } 22977 } 22978 22979 protected void composeAdverseEventInner(AdverseEvent element) throws IOException { 22980 composeDomainResourceElements(element); 22981 if (element.hasIdentifier()) { 22982 composeIdentifier("identifier", element.getIdentifier()); 22983 } 22984 if (element.hasCategoryElement()) { 22985 composeEnumerationCore("category", element.getCategoryElement(), new AdverseEvent.AdverseEventCategoryEnumFactory(), false); 22986 composeEnumerationExtras("category", element.getCategoryElement(), new AdverseEvent.AdverseEventCategoryEnumFactory(), false); 22987 } 22988 if (element.hasType()) { 22989 composeCodeableConcept("type", element.getType()); 22990 } 22991 if (element.hasSubject()) { 22992 composeReference("subject", element.getSubject()); 22993 } 22994 if (element.hasDateElement()) { 22995 composeDateTimeCore("date", element.getDateElement(), false); 22996 composeDateTimeExtras("date", element.getDateElement(), false); 22997 } 22998 if (element.hasReaction()) { 22999 openArray("reaction"); 23000 for (Reference e : element.getReaction()) 23001 composeReference(null, e); 23002 closeArray(); 23003 }; 23004 if (element.hasLocation()) { 23005 composeReference("location", element.getLocation()); 23006 } 23007 if (element.hasSeriousness()) { 23008 composeCodeableConcept("seriousness", element.getSeriousness()); 23009 } 23010 if (element.hasOutcome()) { 23011 composeCodeableConcept("outcome", element.getOutcome()); 23012 } 23013 if (element.hasRecorder()) { 23014 composeReference("recorder", element.getRecorder()); 23015 } 23016 if (element.hasEventParticipant()) { 23017 composeReference("eventParticipant", element.getEventParticipant()); 23018 } 23019 if (element.hasDescriptionElement()) { 23020 composeStringCore("description", element.getDescriptionElement(), false); 23021 composeStringExtras("description", element.getDescriptionElement(), false); 23022 } 23023 if (element.hasSuspectEntity()) { 23024 openArray("suspectEntity"); 23025 for (AdverseEvent.AdverseEventSuspectEntityComponent e : element.getSuspectEntity()) 23026 composeAdverseEventAdverseEventSuspectEntityComponent(null, e); 23027 closeArray(); 23028 }; 23029 if (element.hasSubjectMedicalHistory()) { 23030 openArray("subjectMedicalHistory"); 23031 for (Reference e : element.getSubjectMedicalHistory()) 23032 composeReference(null, e); 23033 closeArray(); 23034 }; 23035 if (element.hasReferenceDocument()) { 23036 openArray("referenceDocument"); 23037 for (Reference e : element.getReferenceDocument()) 23038 composeReference(null, e); 23039 closeArray(); 23040 }; 23041 if (element.hasStudy()) { 23042 openArray("study"); 23043 for (Reference e : element.getStudy()) 23044 composeReference(null, e); 23045 closeArray(); 23046 }; 23047 } 23048 23049 protected void composeAdverseEventAdverseEventSuspectEntityComponent(String name, AdverseEvent.AdverseEventSuspectEntityComponent element) throws IOException { 23050 if (element != null) { 23051 open(name); 23052 composeAdverseEventAdverseEventSuspectEntityComponentInner(element); 23053 close(); 23054 } 23055 } 23056 23057 protected void composeAdverseEventAdverseEventSuspectEntityComponentInner(AdverseEvent.AdverseEventSuspectEntityComponent element) throws IOException { 23058 composeBackbone(element); 23059 if (element.hasInstance()) { 23060 composeReference("instance", element.getInstance()); 23061 } 23062 if (element.hasCausalityElement()) { 23063 composeEnumerationCore("causality", element.getCausalityElement(), new AdverseEvent.AdverseEventCausalityEnumFactory(), false); 23064 composeEnumerationExtras("causality", element.getCausalityElement(), new AdverseEvent.AdverseEventCausalityEnumFactory(), false); 23065 } 23066 if (element.hasCausalityAssessment()) { 23067 composeCodeableConcept("causalityAssessment", element.getCausalityAssessment()); 23068 } 23069 if (element.hasCausalityProductRelatednessElement()) { 23070 composeStringCore("causalityProductRelatedness", element.getCausalityProductRelatednessElement(), false); 23071 composeStringExtras("causalityProductRelatedness", element.getCausalityProductRelatednessElement(), false); 23072 } 23073 if (element.hasCausalityMethod()) { 23074 composeCodeableConcept("causalityMethod", element.getCausalityMethod()); 23075 } 23076 if (element.hasCausalityAuthor()) { 23077 composeReference("causalityAuthor", element.getCausalityAuthor()); 23078 } 23079 if (element.hasCausalityResult()) { 23080 composeCodeableConcept("causalityResult", element.getCausalityResult()); 23081 } 23082 } 23083 23084 protected void composeAllergyIntolerance(String name, AllergyIntolerance element) throws IOException { 23085 if (element != null) { 23086 prop("resourceType", name); 23087 composeAllergyIntoleranceInner(element); 23088 } 23089 } 23090 23091 protected void composeAllergyIntoleranceInner(AllergyIntolerance element) throws IOException { 23092 composeDomainResourceElements(element); 23093 if (element.hasIdentifier()) { 23094 openArray("identifier"); 23095 for (Identifier e : element.getIdentifier()) 23096 composeIdentifier(null, e); 23097 closeArray(); 23098 }; 23099 if (element.hasClinicalStatusElement()) { 23100 composeEnumerationCore("clinicalStatus", element.getClinicalStatusElement(), new AllergyIntolerance.AllergyIntoleranceClinicalStatusEnumFactory(), false); 23101 composeEnumerationExtras("clinicalStatus", element.getClinicalStatusElement(), new AllergyIntolerance.AllergyIntoleranceClinicalStatusEnumFactory(), false); 23102 } 23103 if (element.hasVerificationStatusElement()) { 23104 composeEnumerationCore("verificationStatus", element.getVerificationStatusElement(), new AllergyIntolerance.AllergyIntoleranceVerificationStatusEnumFactory(), false); 23105 composeEnumerationExtras("verificationStatus", element.getVerificationStatusElement(), new AllergyIntolerance.AllergyIntoleranceVerificationStatusEnumFactory(), false); 23106 } 23107 if (element.hasTypeElement()) { 23108 composeEnumerationCore("type", element.getTypeElement(), new AllergyIntolerance.AllergyIntoleranceTypeEnumFactory(), false); 23109 composeEnumerationExtras("type", element.getTypeElement(), new AllergyIntolerance.AllergyIntoleranceTypeEnumFactory(), false); 23110 } 23111 if (element.hasCategory()) { 23112 openArray("category"); 23113 for (Enumeration<AllergyIntolerance.AllergyIntoleranceCategory> e : element.getCategory()) 23114 composeEnumerationCore(null, e, new AllergyIntolerance.AllergyIntoleranceCategoryEnumFactory(), true); 23115 closeArray(); 23116 if (anyHasExtras(element.getCategory())) { 23117 openArray("_category"); 23118 for (Enumeration<AllergyIntolerance.AllergyIntoleranceCategory> e : element.getCategory()) 23119 composeEnumerationExtras(null, e, new AllergyIntolerance.AllergyIntoleranceCategoryEnumFactory(), true); 23120 closeArray(); 23121 } 23122 }; 23123 if (element.hasCriticalityElement()) { 23124 composeEnumerationCore("criticality", element.getCriticalityElement(), new AllergyIntolerance.AllergyIntoleranceCriticalityEnumFactory(), false); 23125 composeEnumerationExtras("criticality", element.getCriticalityElement(), new AllergyIntolerance.AllergyIntoleranceCriticalityEnumFactory(), false); 23126 } 23127 if (element.hasCode()) { 23128 composeCodeableConcept("code", element.getCode()); 23129 } 23130 if (element.hasPatient()) { 23131 composeReference("patient", element.getPatient()); 23132 } 23133 if (element.hasOnset()) { 23134 composeType("onset", element.getOnset()); 23135 } 23136 if (element.hasAssertedDateElement()) { 23137 composeDateTimeCore("assertedDate", element.getAssertedDateElement(), false); 23138 composeDateTimeExtras("assertedDate", element.getAssertedDateElement(), false); 23139 } 23140 if (element.hasRecorder()) { 23141 composeReference("recorder", element.getRecorder()); 23142 } 23143 if (element.hasAsserter()) { 23144 composeReference("asserter", element.getAsserter()); 23145 } 23146 if (element.hasLastOccurrenceElement()) { 23147 composeDateTimeCore("lastOccurrence", element.getLastOccurrenceElement(), false); 23148 composeDateTimeExtras("lastOccurrence", element.getLastOccurrenceElement(), false); 23149 } 23150 if (element.hasNote()) { 23151 openArray("note"); 23152 for (Annotation e : element.getNote()) 23153 composeAnnotation(null, e); 23154 closeArray(); 23155 }; 23156 if (element.hasReaction()) { 23157 openArray("reaction"); 23158 for (AllergyIntolerance.AllergyIntoleranceReactionComponent e : element.getReaction()) 23159 composeAllergyIntoleranceAllergyIntoleranceReactionComponent(null, e); 23160 closeArray(); 23161 }; 23162 } 23163 23164 protected void composeAllergyIntoleranceAllergyIntoleranceReactionComponent(String name, AllergyIntolerance.AllergyIntoleranceReactionComponent element) throws IOException { 23165 if (element != null) { 23166 open(name); 23167 composeAllergyIntoleranceAllergyIntoleranceReactionComponentInner(element); 23168 close(); 23169 } 23170 } 23171 23172 protected void composeAllergyIntoleranceAllergyIntoleranceReactionComponentInner(AllergyIntolerance.AllergyIntoleranceReactionComponent element) throws IOException { 23173 composeBackbone(element); 23174 if (element.hasSubstance()) { 23175 composeCodeableConcept("substance", element.getSubstance()); 23176 } 23177 if (element.hasManifestation()) { 23178 openArray("manifestation"); 23179 for (CodeableConcept e : element.getManifestation()) 23180 composeCodeableConcept(null, e); 23181 closeArray(); 23182 }; 23183 if (element.hasDescriptionElement()) { 23184 composeStringCore("description", element.getDescriptionElement(), false); 23185 composeStringExtras("description", element.getDescriptionElement(), false); 23186 } 23187 if (element.hasOnsetElement()) { 23188 composeDateTimeCore("onset", element.getOnsetElement(), false); 23189 composeDateTimeExtras("onset", element.getOnsetElement(), false); 23190 } 23191 if (element.hasSeverityElement()) { 23192 composeEnumerationCore("severity", element.getSeverityElement(), new AllergyIntolerance.AllergyIntoleranceSeverityEnumFactory(), false); 23193 composeEnumerationExtras("severity", element.getSeverityElement(), new AllergyIntolerance.AllergyIntoleranceSeverityEnumFactory(), false); 23194 } 23195 if (element.hasExposureRoute()) { 23196 composeCodeableConcept("exposureRoute", element.getExposureRoute()); 23197 } 23198 if (element.hasNote()) { 23199 openArray("note"); 23200 for (Annotation e : element.getNote()) 23201 composeAnnotation(null, e); 23202 closeArray(); 23203 }; 23204 } 23205 23206 protected void composeAppointment(String name, Appointment element) throws IOException { 23207 if (element != null) { 23208 prop("resourceType", name); 23209 composeAppointmentInner(element); 23210 } 23211 } 23212 23213 protected void composeAppointmentInner(Appointment element) throws IOException { 23214 composeDomainResourceElements(element); 23215 if (element.hasIdentifier()) { 23216 openArray("identifier"); 23217 for (Identifier e : element.getIdentifier()) 23218 composeIdentifier(null, e); 23219 closeArray(); 23220 }; 23221 if (element.hasStatusElement()) { 23222 composeEnumerationCore("status", element.getStatusElement(), new Appointment.AppointmentStatusEnumFactory(), false); 23223 composeEnumerationExtras("status", element.getStatusElement(), new Appointment.AppointmentStatusEnumFactory(), false); 23224 } 23225 if (element.hasServiceCategory()) { 23226 composeCodeableConcept("serviceCategory", element.getServiceCategory()); 23227 } 23228 if (element.hasServiceType()) { 23229 openArray("serviceType"); 23230 for (CodeableConcept e : element.getServiceType()) 23231 composeCodeableConcept(null, e); 23232 closeArray(); 23233 }; 23234 if (element.hasSpecialty()) { 23235 openArray("specialty"); 23236 for (CodeableConcept e : element.getSpecialty()) 23237 composeCodeableConcept(null, e); 23238 closeArray(); 23239 }; 23240 if (element.hasAppointmentType()) { 23241 composeCodeableConcept("appointmentType", element.getAppointmentType()); 23242 } 23243 if (element.hasReason()) { 23244 openArray("reason"); 23245 for (CodeableConcept e : element.getReason()) 23246 composeCodeableConcept(null, e); 23247 closeArray(); 23248 }; 23249 if (element.hasIndication()) { 23250 openArray("indication"); 23251 for (Reference e : element.getIndication()) 23252 composeReference(null, e); 23253 closeArray(); 23254 }; 23255 if (element.hasPriorityElement()) { 23256 composeUnsignedIntCore("priority", element.getPriorityElement(), false); 23257 composeUnsignedIntExtras("priority", element.getPriorityElement(), false); 23258 } 23259 if (element.hasDescriptionElement()) { 23260 composeStringCore("description", element.getDescriptionElement(), false); 23261 composeStringExtras("description", element.getDescriptionElement(), false); 23262 } 23263 if (element.hasSupportingInformation()) { 23264 openArray("supportingInformation"); 23265 for (Reference e : element.getSupportingInformation()) 23266 composeReference(null, e); 23267 closeArray(); 23268 }; 23269 if (element.hasStartElement()) { 23270 composeInstantCore("start", element.getStartElement(), false); 23271 composeInstantExtras("start", element.getStartElement(), false); 23272 } 23273 if (element.hasEndElement()) { 23274 composeInstantCore("end", element.getEndElement(), false); 23275 composeInstantExtras("end", element.getEndElement(), false); 23276 } 23277 if (element.hasMinutesDurationElement()) { 23278 composePositiveIntCore("minutesDuration", element.getMinutesDurationElement(), false); 23279 composePositiveIntExtras("minutesDuration", element.getMinutesDurationElement(), false); 23280 } 23281 if (element.hasSlot()) { 23282 openArray("slot"); 23283 for (Reference e : element.getSlot()) 23284 composeReference(null, e); 23285 closeArray(); 23286 }; 23287 if (element.hasCreatedElement()) { 23288 composeDateTimeCore("created", element.getCreatedElement(), false); 23289 composeDateTimeExtras("created", element.getCreatedElement(), false); 23290 } 23291 if (element.hasCommentElement()) { 23292 composeStringCore("comment", element.getCommentElement(), false); 23293 composeStringExtras("comment", element.getCommentElement(), false); 23294 } 23295 if (element.hasIncomingReferral()) { 23296 openArray("incomingReferral"); 23297 for (Reference e : element.getIncomingReferral()) 23298 composeReference(null, e); 23299 closeArray(); 23300 }; 23301 if (element.hasParticipant()) { 23302 openArray("participant"); 23303 for (Appointment.AppointmentParticipantComponent e : element.getParticipant()) 23304 composeAppointmentAppointmentParticipantComponent(null, e); 23305 closeArray(); 23306 }; 23307 if (element.hasRequestedPeriod()) { 23308 openArray("requestedPeriod"); 23309 for (Period e : element.getRequestedPeriod()) 23310 composePeriod(null, e); 23311 closeArray(); 23312 }; 23313 } 23314 23315 protected void composeAppointmentAppointmentParticipantComponent(String name, Appointment.AppointmentParticipantComponent element) throws IOException { 23316 if (element != null) { 23317 open(name); 23318 composeAppointmentAppointmentParticipantComponentInner(element); 23319 close(); 23320 } 23321 } 23322 23323 protected void composeAppointmentAppointmentParticipantComponentInner(Appointment.AppointmentParticipantComponent element) throws IOException { 23324 composeBackbone(element); 23325 if (element.hasType()) { 23326 openArray("type"); 23327 for (CodeableConcept e : element.getType()) 23328 composeCodeableConcept(null, e); 23329 closeArray(); 23330 }; 23331 if (element.hasActor()) { 23332 composeReference("actor", element.getActor()); 23333 } 23334 if (element.hasRequiredElement()) { 23335 composeEnumerationCore("required", element.getRequiredElement(), new Appointment.ParticipantRequiredEnumFactory(), false); 23336 composeEnumerationExtras("required", element.getRequiredElement(), new Appointment.ParticipantRequiredEnumFactory(), false); 23337 } 23338 if (element.hasStatusElement()) { 23339 composeEnumerationCore("status", element.getStatusElement(), new Appointment.ParticipationStatusEnumFactory(), false); 23340 composeEnumerationExtras("status", element.getStatusElement(), new Appointment.ParticipationStatusEnumFactory(), false); 23341 } 23342 } 23343 23344 protected void composeAppointmentResponse(String name, AppointmentResponse element) throws IOException { 23345 if (element != null) { 23346 prop("resourceType", name); 23347 composeAppointmentResponseInner(element); 23348 } 23349 } 23350 23351 protected void composeAppointmentResponseInner(AppointmentResponse element) throws IOException { 23352 composeDomainResourceElements(element); 23353 if (element.hasIdentifier()) { 23354 openArray("identifier"); 23355 for (Identifier e : element.getIdentifier()) 23356 composeIdentifier(null, e); 23357 closeArray(); 23358 }; 23359 if (element.hasAppointment()) { 23360 composeReference("appointment", element.getAppointment()); 23361 } 23362 if (element.hasStartElement()) { 23363 composeInstantCore("start", element.getStartElement(), false); 23364 composeInstantExtras("start", element.getStartElement(), false); 23365 } 23366 if (element.hasEndElement()) { 23367 composeInstantCore("end", element.getEndElement(), false); 23368 composeInstantExtras("end", element.getEndElement(), false); 23369 } 23370 if (element.hasParticipantType()) { 23371 openArray("participantType"); 23372 for (CodeableConcept e : element.getParticipantType()) 23373 composeCodeableConcept(null, e); 23374 closeArray(); 23375 }; 23376 if (element.hasActor()) { 23377 composeReference("actor", element.getActor()); 23378 } 23379 if (element.hasParticipantStatusElement()) { 23380 composeEnumerationCore("participantStatus", element.getParticipantStatusElement(), new AppointmentResponse.ParticipantStatusEnumFactory(), false); 23381 composeEnumerationExtras("participantStatus", element.getParticipantStatusElement(), new AppointmentResponse.ParticipantStatusEnumFactory(), false); 23382 } 23383 if (element.hasCommentElement()) { 23384 composeStringCore("comment", element.getCommentElement(), false); 23385 composeStringExtras("comment", element.getCommentElement(), false); 23386 } 23387 } 23388 23389 protected void composeAuditEvent(String name, AuditEvent element) throws IOException { 23390 if (element != null) { 23391 prop("resourceType", name); 23392 composeAuditEventInner(element); 23393 } 23394 } 23395 23396 protected void composeAuditEventInner(AuditEvent element) throws IOException { 23397 composeDomainResourceElements(element); 23398 if (element.hasType()) { 23399 composeCoding("type", element.getType()); 23400 } 23401 if (element.hasSubtype()) { 23402 openArray("subtype"); 23403 for (Coding e : element.getSubtype()) 23404 composeCoding(null, e); 23405 closeArray(); 23406 }; 23407 if (element.hasActionElement()) { 23408 composeEnumerationCore("action", element.getActionElement(), new AuditEvent.AuditEventActionEnumFactory(), false); 23409 composeEnumerationExtras("action", element.getActionElement(), new AuditEvent.AuditEventActionEnumFactory(), false); 23410 } 23411 if (element.hasRecordedElement()) { 23412 composeInstantCore("recorded", element.getRecordedElement(), false); 23413 composeInstantExtras("recorded", element.getRecordedElement(), false); 23414 } 23415 if (element.hasOutcomeElement()) { 23416 composeEnumerationCore("outcome", element.getOutcomeElement(), new AuditEvent.AuditEventOutcomeEnumFactory(), false); 23417 composeEnumerationExtras("outcome", element.getOutcomeElement(), new AuditEvent.AuditEventOutcomeEnumFactory(), false); 23418 } 23419 if (element.hasOutcomeDescElement()) { 23420 composeStringCore("outcomeDesc", element.getOutcomeDescElement(), false); 23421 composeStringExtras("outcomeDesc", element.getOutcomeDescElement(), false); 23422 } 23423 if (element.hasPurposeOfEvent()) { 23424 openArray("purposeOfEvent"); 23425 for (CodeableConcept e : element.getPurposeOfEvent()) 23426 composeCodeableConcept(null, e); 23427 closeArray(); 23428 }; 23429 if (element.hasAgent()) { 23430 openArray("agent"); 23431 for (AuditEvent.AuditEventAgentComponent e : element.getAgent()) 23432 composeAuditEventAuditEventAgentComponent(null, e); 23433 closeArray(); 23434 }; 23435 if (element.hasSource()) { 23436 composeAuditEventAuditEventSourceComponent("source", element.getSource()); 23437 } 23438 if (element.hasEntity()) { 23439 openArray("entity"); 23440 for (AuditEvent.AuditEventEntityComponent e : element.getEntity()) 23441 composeAuditEventAuditEventEntityComponent(null, e); 23442 closeArray(); 23443 }; 23444 } 23445 23446 protected void composeAuditEventAuditEventAgentComponent(String name, AuditEvent.AuditEventAgentComponent element) throws IOException { 23447 if (element != null) { 23448 open(name); 23449 composeAuditEventAuditEventAgentComponentInner(element); 23450 close(); 23451 } 23452 } 23453 23454 protected void composeAuditEventAuditEventAgentComponentInner(AuditEvent.AuditEventAgentComponent element) throws IOException { 23455 composeBackbone(element); 23456 if (element.hasRole()) { 23457 openArray("role"); 23458 for (CodeableConcept e : element.getRole()) 23459 composeCodeableConcept(null, e); 23460 closeArray(); 23461 }; 23462 if (element.hasReference()) { 23463 composeReference("reference", element.getReference()); 23464 } 23465 if (element.hasUserId()) { 23466 composeIdentifier("userId", element.getUserId()); 23467 } 23468 if (element.hasAltIdElement()) { 23469 composeStringCore("altId", element.getAltIdElement(), false); 23470 composeStringExtras("altId", element.getAltIdElement(), false); 23471 } 23472 if (element.hasNameElement()) { 23473 composeStringCore("name", element.getNameElement(), false); 23474 composeStringExtras("name", element.getNameElement(), false); 23475 } 23476 if (element.hasRequestorElement()) { 23477 composeBooleanCore("requestor", element.getRequestorElement(), false); 23478 composeBooleanExtras("requestor", element.getRequestorElement(), false); 23479 } 23480 if (element.hasLocation()) { 23481 composeReference("location", element.getLocation()); 23482 } 23483 if (element.hasPolicy()) { 23484 openArray("policy"); 23485 for (UriType e : element.getPolicy()) 23486 composeUriCore(null, e, true); 23487 closeArray(); 23488 if (anyHasExtras(element.getPolicy())) { 23489 openArray("_policy"); 23490 for (UriType e : element.getPolicy()) 23491 composeUriExtras(null, e, true); 23492 closeArray(); 23493 } 23494 }; 23495 if (element.hasMedia()) { 23496 composeCoding("media", element.getMedia()); 23497 } 23498 if (element.hasNetwork()) { 23499 composeAuditEventAuditEventAgentNetworkComponent("network", element.getNetwork()); 23500 } 23501 if (element.hasPurposeOfUse()) { 23502 openArray("purposeOfUse"); 23503 for (CodeableConcept e : element.getPurposeOfUse()) 23504 composeCodeableConcept(null, e); 23505 closeArray(); 23506 }; 23507 } 23508 23509 protected void composeAuditEventAuditEventAgentNetworkComponent(String name, AuditEvent.AuditEventAgentNetworkComponent element) throws IOException { 23510 if (element != null) { 23511 open(name); 23512 composeAuditEventAuditEventAgentNetworkComponentInner(element); 23513 close(); 23514 } 23515 } 23516 23517 protected void composeAuditEventAuditEventAgentNetworkComponentInner(AuditEvent.AuditEventAgentNetworkComponent element) throws IOException { 23518 composeBackbone(element); 23519 if (element.hasAddressElement()) { 23520 composeStringCore("address", element.getAddressElement(), false); 23521 composeStringExtras("address", element.getAddressElement(), false); 23522 } 23523 if (element.hasTypeElement()) { 23524 composeEnumerationCore("type", element.getTypeElement(), new AuditEvent.AuditEventAgentNetworkTypeEnumFactory(), false); 23525 composeEnumerationExtras("type", element.getTypeElement(), new AuditEvent.AuditEventAgentNetworkTypeEnumFactory(), false); 23526 } 23527 } 23528 23529 protected void composeAuditEventAuditEventSourceComponent(String name, AuditEvent.AuditEventSourceComponent element) throws IOException { 23530 if (element != null) { 23531 open(name); 23532 composeAuditEventAuditEventSourceComponentInner(element); 23533 close(); 23534 } 23535 } 23536 23537 protected void composeAuditEventAuditEventSourceComponentInner(AuditEvent.AuditEventSourceComponent element) throws IOException { 23538 composeBackbone(element); 23539 if (element.hasSiteElement()) { 23540 composeStringCore("site", element.getSiteElement(), false); 23541 composeStringExtras("site", element.getSiteElement(), false); 23542 } 23543 if (element.hasIdentifier()) { 23544 composeIdentifier("identifier", element.getIdentifier()); 23545 } 23546 if (element.hasType()) { 23547 openArray("type"); 23548 for (Coding e : element.getType()) 23549 composeCoding(null, e); 23550 closeArray(); 23551 }; 23552 } 23553 23554 protected void composeAuditEventAuditEventEntityComponent(String name, AuditEvent.AuditEventEntityComponent element) throws IOException { 23555 if (element != null) { 23556 open(name); 23557 composeAuditEventAuditEventEntityComponentInner(element); 23558 close(); 23559 } 23560 } 23561 23562 protected void composeAuditEventAuditEventEntityComponentInner(AuditEvent.AuditEventEntityComponent element) throws IOException { 23563 composeBackbone(element); 23564 if (element.hasIdentifier()) { 23565 composeIdentifier("identifier", element.getIdentifier()); 23566 } 23567 if (element.hasReference()) { 23568 composeReference("reference", element.getReference()); 23569 } 23570 if (element.hasType()) { 23571 composeCoding("type", element.getType()); 23572 } 23573 if (element.hasRole()) { 23574 composeCoding("role", element.getRole()); 23575 } 23576 if (element.hasLifecycle()) { 23577 composeCoding("lifecycle", element.getLifecycle()); 23578 } 23579 if (element.hasSecurityLabel()) { 23580 openArray("securityLabel"); 23581 for (Coding e : element.getSecurityLabel()) 23582 composeCoding(null, e); 23583 closeArray(); 23584 }; 23585 if (element.hasNameElement()) { 23586 composeStringCore("name", element.getNameElement(), false); 23587 composeStringExtras("name", element.getNameElement(), false); 23588 } 23589 if (element.hasDescriptionElement()) { 23590 composeStringCore("description", element.getDescriptionElement(), false); 23591 composeStringExtras("description", element.getDescriptionElement(), false); 23592 } 23593 if (element.hasQueryElement()) { 23594 composeBase64BinaryCore("query", element.getQueryElement(), false); 23595 composeBase64BinaryExtras("query", element.getQueryElement(), false); 23596 } 23597 if (element.hasDetail()) { 23598 openArray("detail"); 23599 for (AuditEvent.AuditEventEntityDetailComponent e : element.getDetail()) 23600 composeAuditEventAuditEventEntityDetailComponent(null, e); 23601 closeArray(); 23602 }; 23603 } 23604 23605 protected void composeAuditEventAuditEventEntityDetailComponent(String name, AuditEvent.AuditEventEntityDetailComponent element) throws IOException { 23606 if (element != null) { 23607 open(name); 23608 composeAuditEventAuditEventEntityDetailComponentInner(element); 23609 close(); 23610 } 23611 } 23612 23613 protected void composeAuditEventAuditEventEntityDetailComponentInner(AuditEvent.AuditEventEntityDetailComponent element) throws IOException { 23614 composeBackbone(element); 23615 if (element.hasTypeElement()) { 23616 composeStringCore("type", element.getTypeElement(), false); 23617 composeStringExtras("type", element.getTypeElement(), false); 23618 } 23619 if (element.hasValueElement()) { 23620 composeBase64BinaryCore("value", element.getValueElement(), false); 23621 composeBase64BinaryExtras("value", element.getValueElement(), false); 23622 } 23623 } 23624 23625 protected void composeBasic(String name, Basic element) throws IOException { 23626 if (element != null) { 23627 prop("resourceType", name); 23628 composeBasicInner(element); 23629 } 23630 } 23631 23632 protected void composeBasicInner(Basic element) throws IOException { 23633 composeDomainResourceElements(element); 23634 if (element.hasIdentifier()) { 23635 openArray("identifier"); 23636 for (Identifier e : element.getIdentifier()) 23637 composeIdentifier(null, e); 23638 closeArray(); 23639 }; 23640 if (element.hasCode()) { 23641 composeCodeableConcept("code", element.getCode()); 23642 } 23643 if (element.hasSubject()) { 23644 composeReference("subject", element.getSubject()); 23645 } 23646 if (element.hasCreatedElement()) { 23647 composeDateCore("created", element.getCreatedElement(), false); 23648 composeDateExtras("created", element.getCreatedElement(), false); 23649 } 23650 if (element.hasAuthor()) { 23651 composeReference("author", element.getAuthor()); 23652 } 23653 } 23654 23655 protected void composeBinary(String name, Binary element) throws IOException { 23656 if (element != null) { 23657 prop("resourceType", name); 23658 composeBinaryInner(element); 23659 } 23660 } 23661 23662 protected void composeBinaryInner(Binary element) throws IOException { 23663 composeResourceElements(element); 23664 if (element.hasContentTypeElement()) { 23665 composeCodeCore("contentType", element.getContentTypeElement(), false); 23666 composeCodeExtras("contentType", element.getContentTypeElement(), false); 23667 } 23668 if (element.hasSecurityContext()) { 23669 composeReference("securityContext", element.getSecurityContext()); 23670 } 23671 if (element.hasContentElement()) { 23672 composeBase64BinaryCore("content", element.getContentElement(), false); 23673 composeBase64BinaryExtras("content", element.getContentElement(), false); 23674 } 23675 } 23676 23677 protected void composeBodySite(String name, BodySite element) throws IOException { 23678 if (element != null) { 23679 prop("resourceType", name); 23680 composeBodySiteInner(element); 23681 } 23682 } 23683 23684 protected void composeBodySiteInner(BodySite element) throws IOException { 23685 composeDomainResourceElements(element); 23686 if (element.hasIdentifier()) { 23687 openArray("identifier"); 23688 for (Identifier e : element.getIdentifier()) 23689 composeIdentifier(null, e); 23690 closeArray(); 23691 }; 23692 if (element.hasActiveElement()) { 23693 composeBooleanCore("active", element.getActiveElement(), false); 23694 composeBooleanExtras("active", element.getActiveElement(), false); 23695 } 23696 if (element.hasCode()) { 23697 composeCodeableConcept("code", element.getCode()); 23698 } 23699 if (element.hasQualifier()) { 23700 openArray("qualifier"); 23701 for (CodeableConcept e : element.getQualifier()) 23702 composeCodeableConcept(null, e); 23703 closeArray(); 23704 }; 23705 if (element.hasDescriptionElement()) { 23706 composeStringCore("description", element.getDescriptionElement(), false); 23707 composeStringExtras("description", element.getDescriptionElement(), false); 23708 } 23709 if (element.hasImage()) { 23710 openArray("image"); 23711 for (Attachment e : element.getImage()) 23712 composeAttachment(null, e); 23713 closeArray(); 23714 }; 23715 if (element.hasPatient()) { 23716 composeReference("patient", element.getPatient()); 23717 } 23718 } 23719 23720 protected void composeBundle(String name, Bundle element) throws IOException { 23721 if (element != null) { 23722 prop("resourceType", name); 23723 composeBundleInner(element); 23724 } 23725 } 23726 23727 protected void composeBundleInner(Bundle element) throws IOException { 23728 composeResourceElements(element); 23729 if (element.hasIdentifier()) { 23730 composeIdentifier("identifier", element.getIdentifier()); 23731 } 23732 if (element.hasTypeElement()) { 23733 composeEnumerationCore("type", element.getTypeElement(), new Bundle.BundleTypeEnumFactory(), false); 23734 composeEnumerationExtras("type", element.getTypeElement(), new Bundle.BundleTypeEnumFactory(), false); 23735 } 23736 if (element.hasTotalElement()) { 23737 composeUnsignedIntCore("total", element.getTotalElement(), false); 23738 composeUnsignedIntExtras("total", element.getTotalElement(), false); 23739 } 23740 if (element.hasLink()) { 23741 openArray("link"); 23742 for (Bundle.BundleLinkComponent e : element.getLink()) 23743 composeBundleBundleLinkComponent(null, e); 23744 closeArray(); 23745 }; 23746 if (element.hasEntry()) { 23747 openArray("entry"); 23748 for (Bundle.BundleEntryComponent e : element.getEntry()) 23749 composeBundleBundleEntryComponent(null, e); 23750 closeArray(); 23751 }; 23752 if (element.hasSignature()) { 23753 composeSignature("signature", element.getSignature()); 23754 } 23755 } 23756 23757 protected void composeBundleBundleLinkComponent(String name, Bundle.BundleLinkComponent element) throws IOException { 23758 if (element != null) { 23759 open(name); 23760 composeBundleBundleLinkComponentInner(element); 23761 close(); 23762 } 23763 } 23764 23765 protected void composeBundleBundleLinkComponentInner(Bundle.BundleLinkComponent element) throws IOException { 23766 composeBackbone(element); 23767 if (element.hasRelationElement()) { 23768 composeStringCore("relation", element.getRelationElement(), false); 23769 composeStringExtras("relation", element.getRelationElement(), false); 23770 } 23771 if (element.hasUrlElement()) { 23772 composeUriCore("url", element.getUrlElement(), false); 23773 composeUriExtras("url", element.getUrlElement(), false); 23774 } 23775 } 23776 23777 protected void composeBundleBundleEntryComponent(String name, Bundle.BundleEntryComponent element) throws IOException { 23778 if (element != null) { 23779 open(name); 23780 composeBundleBundleEntryComponentInner(element); 23781 close(); 23782 } 23783 } 23784 23785 protected void composeBundleBundleEntryComponentInner(Bundle.BundleEntryComponent element) throws IOException { 23786 composeBackbone(element); 23787 if (element.hasLink()) { 23788 openArray("link"); 23789 for (Bundle.BundleLinkComponent e : element.getLink()) 23790 composeBundleBundleLinkComponent(null, e); 23791 closeArray(); 23792 }; 23793 if (element.hasFullUrlElement()) { 23794 composeUriCore("fullUrl", element.getFullUrlElement(), false); 23795 composeUriExtras("fullUrl", element.getFullUrlElement(), false); 23796 } 23797 if (element.hasResource()) { 23798 open("resource"); 23799 composeResource(element.getResource()); 23800 close(); 23801 } 23802 if (element.hasSearch()) { 23803 composeBundleBundleEntrySearchComponent("search", element.getSearch()); 23804 } 23805 if (element.hasRequest()) { 23806 composeBundleBundleEntryRequestComponent("request", element.getRequest()); 23807 } 23808 if (element.hasResponse()) { 23809 composeBundleBundleEntryResponseComponent("response", element.getResponse()); 23810 } 23811 } 23812 23813 protected void composeBundleBundleEntrySearchComponent(String name, Bundle.BundleEntrySearchComponent element) throws IOException { 23814 if (element != null) { 23815 open(name); 23816 composeBundleBundleEntrySearchComponentInner(element); 23817 close(); 23818 } 23819 } 23820 23821 protected void composeBundleBundleEntrySearchComponentInner(Bundle.BundleEntrySearchComponent element) throws IOException { 23822 composeBackbone(element); 23823 if (element.hasModeElement()) { 23824 composeEnumerationCore("mode", element.getModeElement(), new Bundle.SearchEntryModeEnumFactory(), false); 23825 composeEnumerationExtras("mode", element.getModeElement(), new Bundle.SearchEntryModeEnumFactory(), false); 23826 } 23827 if (element.hasScoreElement()) { 23828 composeDecimalCore("score", element.getScoreElement(), false); 23829 composeDecimalExtras("score", element.getScoreElement(), false); 23830 } 23831 } 23832 23833 protected void composeBundleBundleEntryRequestComponent(String name, Bundle.BundleEntryRequestComponent element) throws IOException { 23834 if (element != null) { 23835 open(name); 23836 composeBundleBundleEntryRequestComponentInner(element); 23837 close(); 23838 } 23839 } 23840 23841 protected void composeBundleBundleEntryRequestComponentInner(Bundle.BundleEntryRequestComponent element) throws IOException { 23842 composeBackbone(element); 23843 if (element.hasMethodElement()) { 23844 composeEnumerationCore("method", element.getMethodElement(), new Bundle.HTTPVerbEnumFactory(), false); 23845 composeEnumerationExtras("method", element.getMethodElement(), new Bundle.HTTPVerbEnumFactory(), false); 23846 } 23847 if (element.hasUrlElement()) { 23848 composeUriCore("url", element.getUrlElement(), false); 23849 composeUriExtras("url", element.getUrlElement(), false); 23850 } 23851 if (element.hasIfNoneMatchElement()) { 23852 composeStringCore("ifNoneMatch", element.getIfNoneMatchElement(), false); 23853 composeStringExtras("ifNoneMatch", element.getIfNoneMatchElement(), false); 23854 } 23855 if (element.hasIfModifiedSinceElement()) { 23856 composeInstantCore("ifModifiedSince", element.getIfModifiedSinceElement(), false); 23857 composeInstantExtras("ifModifiedSince", element.getIfModifiedSinceElement(), false); 23858 } 23859 if (element.hasIfMatchElement()) { 23860 composeStringCore("ifMatch", element.getIfMatchElement(), false); 23861 composeStringExtras("ifMatch", element.getIfMatchElement(), false); 23862 } 23863 if (element.hasIfNoneExistElement()) { 23864 composeStringCore("ifNoneExist", element.getIfNoneExistElement(), false); 23865 composeStringExtras("ifNoneExist", element.getIfNoneExistElement(), false); 23866 } 23867 } 23868 23869 protected void composeBundleBundleEntryResponseComponent(String name, Bundle.BundleEntryResponseComponent element) throws IOException { 23870 if (element != null) { 23871 open(name); 23872 composeBundleBundleEntryResponseComponentInner(element); 23873 close(); 23874 } 23875 } 23876 23877 protected void composeBundleBundleEntryResponseComponentInner(Bundle.BundleEntryResponseComponent element) throws IOException { 23878 composeBackbone(element); 23879 if (element.hasStatusElement()) { 23880 composeStringCore("status", element.getStatusElement(), false); 23881 composeStringExtras("status", element.getStatusElement(), false); 23882 } 23883 if (element.hasLocationElement()) { 23884 composeUriCore("location", element.getLocationElement(), false); 23885 composeUriExtras("location", element.getLocationElement(), false); 23886 } 23887 if (element.hasEtagElement()) { 23888 composeStringCore("etag", element.getEtagElement(), false); 23889 composeStringExtras("etag", element.getEtagElement(), false); 23890 } 23891 if (element.hasLastModifiedElement()) { 23892 composeInstantCore("lastModified", element.getLastModifiedElement(), false); 23893 composeInstantExtras("lastModified", element.getLastModifiedElement(), false); 23894 } 23895 if (element.hasOutcome()) { 23896 open("outcome"); 23897 composeResource(element.getOutcome()); 23898 close(); 23899 } 23900 } 23901 23902 protected void composeCapabilityStatement(String name, CapabilityStatement element) throws IOException { 23903 if (element != null) { 23904 prop("resourceType", name); 23905 composeCapabilityStatementInner(element); 23906 } 23907 } 23908 23909 protected void composeCapabilityStatementInner(CapabilityStatement element) throws IOException { 23910 composeDomainResourceElements(element); 23911 if (element.hasUrlElement()) { 23912 composeUriCore("url", element.getUrlElement(), false); 23913 composeUriExtras("url", element.getUrlElement(), false); 23914 } 23915 if (element.hasVersionElement()) { 23916 composeStringCore("version", element.getVersionElement(), false); 23917 composeStringExtras("version", element.getVersionElement(), false); 23918 } 23919 if (element.hasNameElement()) { 23920 composeStringCore("name", element.getNameElement(), false); 23921 composeStringExtras("name", element.getNameElement(), false); 23922 } 23923 if (element.hasTitleElement()) { 23924 composeStringCore("title", element.getTitleElement(), false); 23925 composeStringExtras("title", element.getTitleElement(), false); 23926 } 23927 if (element.hasStatusElement()) { 23928 composeEnumerationCore("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 23929 composeEnumerationExtras("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 23930 } 23931 if (element.hasExperimentalElement()) { 23932 composeBooleanCore("experimental", element.getExperimentalElement(), false); 23933 composeBooleanExtras("experimental", element.getExperimentalElement(), false); 23934 } 23935 if (element.hasDateElement()) { 23936 composeDateTimeCore("date", element.getDateElement(), false); 23937 composeDateTimeExtras("date", element.getDateElement(), false); 23938 } 23939 if (element.hasPublisherElement()) { 23940 composeStringCore("publisher", element.getPublisherElement(), false); 23941 composeStringExtras("publisher", element.getPublisherElement(), false); 23942 } 23943 if (element.hasContact()) { 23944 openArray("contact"); 23945 for (ContactDetail e : element.getContact()) 23946 composeContactDetail(null, e); 23947 closeArray(); 23948 }; 23949 if (element.hasDescriptionElement()) { 23950 composeMarkdownCore("description", element.getDescriptionElement(), false); 23951 composeMarkdownExtras("description", element.getDescriptionElement(), false); 23952 } 23953 if (element.hasUseContext()) { 23954 openArray("useContext"); 23955 for (UsageContext e : element.getUseContext()) 23956 composeUsageContext(null, e); 23957 closeArray(); 23958 }; 23959 if (element.hasJurisdiction()) { 23960 openArray("jurisdiction"); 23961 for (CodeableConcept e : element.getJurisdiction()) 23962 composeCodeableConcept(null, e); 23963 closeArray(); 23964 }; 23965 if (element.hasPurposeElement()) { 23966 composeMarkdownCore("purpose", element.getPurposeElement(), false); 23967 composeMarkdownExtras("purpose", element.getPurposeElement(), false); 23968 } 23969 if (element.hasCopyrightElement()) { 23970 composeMarkdownCore("copyright", element.getCopyrightElement(), false); 23971 composeMarkdownExtras("copyright", element.getCopyrightElement(), false); 23972 } 23973 if (element.hasKindElement()) { 23974 composeEnumerationCore("kind", element.getKindElement(), new CapabilityStatement.CapabilityStatementKindEnumFactory(), false); 23975 composeEnumerationExtras("kind", element.getKindElement(), new CapabilityStatement.CapabilityStatementKindEnumFactory(), false); 23976 } 23977 if (element.hasInstantiates()) { 23978 openArray("instantiates"); 23979 for (UriType e : element.getInstantiates()) 23980 composeUriCore(null, e, true); 23981 closeArray(); 23982 if (anyHasExtras(element.getInstantiates())) { 23983 openArray("_instantiates"); 23984 for (UriType e : element.getInstantiates()) 23985 composeUriExtras(null, e, true); 23986 closeArray(); 23987 } 23988 }; 23989 if (element.hasSoftware()) { 23990 composeCapabilityStatementCapabilityStatementSoftwareComponent("software", element.getSoftware()); 23991 } 23992 if (element.hasImplementation()) { 23993 composeCapabilityStatementCapabilityStatementImplementationComponent("implementation", element.getImplementation()); 23994 } 23995 if (element.hasFhirVersionElement()) { 23996 composeIdCore("fhirVersion", element.getFhirVersionElement(), false); 23997 composeIdExtras("fhirVersion", element.getFhirVersionElement(), false); 23998 } 23999 if (element.hasAcceptUnknownElement()) { 24000 composeEnumerationCore("acceptUnknown", element.getAcceptUnknownElement(), new CapabilityStatement.UnknownContentCodeEnumFactory(), false); 24001 composeEnumerationExtras("acceptUnknown", element.getAcceptUnknownElement(), new CapabilityStatement.UnknownContentCodeEnumFactory(), false); 24002 } 24003 if (element.hasFormat()) { 24004 openArray("format"); 24005 for (CodeType e : element.getFormat()) 24006 composeCodeCore(null, e, true); 24007 closeArray(); 24008 if (anyHasExtras(element.getFormat())) { 24009 openArray("_format"); 24010 for (CodeType e : element.getFormat()) 24011 composeCodeExtras(null, e, true); 24012 closeArray(); 24013 } 24014 }; 24015 if (element.hasPatchFormat()) { 24016 openArray("patchFormat"); 24017 for (CodeType e : element.getPatchFormat()) 24018 composeCodeCore(null, e, true); 24019 closeArray(); 24020 if (anyHasExtras(element.getPatchFormat())) { 24021 openArray("_patchFormat"); 24022 for (CodeType e : element.getPatchFormat()) 24023 composeCodeExtras(null, e, true); 24024 closeArray(); 24025 } 24026 }; 24027 if (element.hasImplementationGuide()) { 24028 openArray("implementationGuide"); 24029 for (UriType e : element.getImplementationGuide()) 24030 composeUriCore(null, e, true); 24031 closeArray(); 24032 if (anyHasExtras(element.getImplementationGuide())) { 24033 openArray("_implementationGuide"); 24034 for (UriType e : element.getImplementationGuide()) 24035 composeUriExtras(null, e, true); 24036 closeArray(); 24037 } 24038 }; 24039 if (element.hasProfile()) { 24040 openArray("profile"); 24041 for (Reference e : element.getProfile()) 24042 composeReference(null, e); 24043 closeArray(); 24044 }; 24045 if (element.hasRest()) { 24046 openArray("rest"); 24047 for (CapabilityStatement.CapabilityStatementRestComponent e : element.getRest()) 24048 composeCapabilityStatementCapabilityStatementRestComponent(null, e); 24049 closeArray(); 24050 }; 24051 if (element.hasMessaging()) { 24052 openArray("messaging"); 24053 for (CapabilityStatement.CapabilityStatementMessagingComponent e : element.getMessaging()) 24054 composeCapabilityStatementCapabilityStatementMessagingComponent(null, e); 24055 closeArray(); 24056 }; 24057 if (element.hasDocument()) { 24058 openArray("document"); 24059 for (CapabilityStatement.CapabilityStatementDocumentComponent e : element.getDocument()) 24060 composeCapabilityStatementCapabilityStatementDocumentComponent(null, e); 24061 closeArray(); 24062 }; 24063 } 24064 24065 protected void composeCapabilityStatementCapabilityStatementSoftwareComponent(String name, CapabilityStatement.CapabilityStatementSoftwareComponent element) throws IOException { 24066 if (element != null) { 24067 open(name); 24068 composeCapabilityStatementCapabilityStatementSoftwareComponentInner(element); 24069 close(); 24070 } 24071 } 24072 24073 protected void composeCapabilityStatementCapabilityStatementSoftwareComponentInner(CapabilityStatement.CapabilityStatementSoftwareComponent element) throws IOException { 24074 composeBackbone(element); 24075 if (element.hasNameElement()) { 24076 composeStringCore("name", element.getNameElement(), false); 24077 composeStringExtras("name", element.getNameElement(), false); 24078 } 24079 if (element.hasVersionElement()) { 24080 composeStringCore("version", element.getVersionElement(), false); 24081 composeStringExtras("version", element.getVersionElement(), false); 24082 } 24083 if (element.hasReleaseDateElement()) { 24084 composeDateTimeCore("releaseDate", element.getReleaseDateElement(), false); 24085 composeDateTimeExtras("releaseDate", element.getReleaseDateElement(), false); 24086 } 24087 } 24088 24089 protected void composeCapabilityStatementCapabilityStatementImplementationComponent(String name, CapabilityStatement.CapabilityStatementImplementationComponent element) throws IOException { 24090 if (element != null) { 24091 open(name); 24092 composeCapabilityStatementCapabilityStatementImplementationComponentInner(element); 24093 close(); 24094 } 24095 } 24096 24097 protected void composeCapabilityStatementCapabilityStatementImplementationComponentInner(CapabilityStatement.CapabilityStatementImplementationComponent element) throws IOException { 24098 composeBackbone(element); 24099 if (element.hasDescriptionElement()) { 24100 composeStringCore("description", element.getDescriptionElement(), false); 24101 composeStringExtras("description", element.getDescriptionElement(), false); 24102 } 24103 if (element.hasUrlElement()) { 24104 composeUriCore("url", element.getUrlElement(), false); 24105 composeUriExtras("url", element.getUrlElement(), false); 24106 } 24107 } 24108 24109 protected void composeCapabilityStatementCapabilityStatementRestComponent(String name, CapabilityStatement.CapabilityStatementRestComponent element) throws IOException { 24110 if (element != null) { 24111 open(name); 24112 composeCapabilityStatementCapabilityStatementRestComponentInner(element); 24113 close(); 24114 } 24115 } 24116 24117 protected void composeCapabilityStatementCapabilityStatementRestComponentInner(CapabilityStatement.CapabilityStatementRestComponent element) throws IOException { 24118 composeBackbone(element); 24119 if (element.hasModeElement()) { 24120 composeEnumerationCore("mode", element.getModeElement(), new CapabilityStatement.RestfulCapabilityModeEnumFactory(), false); 24121 composeEnumerationExtras("mode", element.getModeElement(), new CapabilityStatement.RestfulCapabilityModeEnumFactory(), false); 24122 } 24123 if (element.hasDocumentationElement()) { 24124 composeStringCore("documentation", element.getDocumentationElement(), false); 24125 composeStringExtras("documentation", element.getDocumentationElement(), false); 24126 } 24127 if (element.hasSecurity()) { 24128 composeCapabilityStatementCapabilityStatementRestSecurityComponent("security", element.getSecurity()); 24129 } 24130 if (element.hasResource()) { 24131 openArray("resource"); 24132 for (CapabilityStatement.CapabilityStatementRestResourceComponent e : element.getResource()) 24133 composeCapabilityStatementCapabilityStatementRestResourceComponent(null, e); 24134 closeArray(); 24135 }; 24136 if (element.hasInteraction()) { 24137 openArray("interaction"); 24138 for (CapabilityStatement.SystemInteractionComponent e : element.getInteraction()) 24139 composeCapabilityStatementSystemInteractionComponent(null, e); 24140 closeArray(); 24141 }; 24142 if (element.hasSearchParam()) { 24143 openArray("searchParam"); 24144 for (CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent e : element.getSearchParam()) 24145 composeCapabilityStatementCapabilityStatementRestResourceSearchParamComponent(null, e); 24146 closeArray(); 24147 }; 24148 if (element.hasOperation()) { 24149 openArray("operation"); 24150 for (CapabilityStatement.CapabilityStatementRestOperationComponent e : element.getOperation()) 24151 composeCapabilityStatementCapabilityStatementRestOperationComponent(null, e); 24152 closeArray(); 24153 }; 24154 if (element.hasCompartment()) { 24155 openArray("compartment"); 24156 for (UriType e : element.getCompartment()) 24157 composeUriCore(null, e, true); 24158 closeArray(); 24159 if (anyHasExtras(element.getCompartment())) { 24160 openArray("_compartment"); 24161 for (UriType e : element.getCompartment()) 24162 composeUriExtras(null, e, true); 24163 closeArray(); 24164 } 24165 }; 24166 } 24167 24168 protected void composeCapabilityStatementCapabilityStatementRestSecurityComponent(String name, CapabilityStatement.CapabilityStatementRestSecurityComponent element) throws IOException { 24169 if (element != null) { 24170 open(name); 24171 composeCapabilityStatementCapabilityStatementRestSecurityComponentInner(element); 24172 close(); 24173 } 24174 } 24175 24176 protected void composeCapabilityStatementCapabilityStatementRestSecurityComponentInner(CapabilityStatement.CapabilityStatementRestSecurityComponent element) throws IOException { 24177 composeBackbone(element); 24178 if (element.hasCorsElement()) { 24179 composeBooleanCore("cors", element.getCorsElement(), false); 24180 composeBooleanExtras("cors", element.getCorsElement(), false); 24181 } 24182 if (element.hasService()) { 24183 openArray("service"); 24184 for (CodeableConcept e : element.getService()) 24185 composeCodeableConcept(null, e); 24186 closeArray(); 24187 }; 24188 if (element.hasDescriptionElement()) { 24189 composeStringCore("description", element.getDescriptionElement(), false); 24190 composeStringExtras("description", element.getDescriptionElement(), false); 24191 } 24192 if (element.hasCertificate()) { 24193 openArray("certificate"); 24194 for (CapabilityStatement.CapabilityStatementRestSecurityCertificateComponent e : element.getCertificate()) 24195 composeCapabilityStatementCapabilityStatementRestSecurityCertificateComponent(null, e); 24196 closeArray(); 24197 }; 24198 } 24199 24200 protected void composeCapabilityStatementCapabilityStatementRestSecurityCertificateComponent(String name, CapabilityStatement.CapabilityStatementRestSecurityCertificateComponent element) throws IOException { 24201 if (element != null) { 24202 open(name); 24203 composeCapabilityStatementCapabilityStatementRestSecurityCertificateComponentInner(element); 24204 close(); 24205 } 24206 } 24207 24208 protected void composeCapabilityStatementCapabilityStatementRestSecurityCertificateComponentInner(CapabilityStatement.CapabilityStatementRestSecurityCertificateComponent element) throws IOException { 24209 composeBackbone(element); 24210 if (element.hasTypeElement()) { 24211 composeCodeCore("type", element.getTypeElement(), false); 24212 composeCodeExtras("type", element.getTypeElement(), false); 24213 } 24214 if (element.hasBlobElement()) { 24215 composeBase64BinaryCore("blob", element.getBlobElement(), false); 24216 composeBase64BinaryExtras("blob", element.getBlobElement(), false); 24217 } 24218 } 24219 24220 protected void composeCapabilityStatementCapabilityStatementRestResourceComponent(String name, CapabilityStatement.CapabilityStatementRestResourceComponent element) throws IOException { 24221 if (element != null) { 24222 open(name); 24223 composeCapabilityStatementCapabilityStatementRestResourceComponentInner(element); 24224 close(); 24225 } 24226 } 24227 24228 protected void composeCapabilityStatementCapabilityStatementRestResourceComponentInner(CapabilityStatement.CapabilityStatementRestResourceComponent element) throws IOException { 24229 composeBackbone(element); 24230 if (element.hasTypeElement()) { 24231 composeCodeCore("type", element.getTypeElement(), false); 24232 composeCodeExtras("type", element.getTypeElement(), false); 24233 } 24234 if (element.hasProfile()) { 24235 composeReference("profile", element.getProfile()); 24236 } 24237 if (element.hasDocumentationElement()) { 24238 composeMarkdownCore("documentation", element.getDocumentationElement(), false); 24239 composeMarkdownExtras("documentation", element.getDocumentationElement(), false); 24240 } 24241 if (element.hasInteraction()) { 24242 openArray("interaction"); 24243 for (CapabilityStatement.ResourceInteractionComponent e : element.getInteraction()) 24244 composeCapabilityStatementResourceInteractionComponent(null, e); 24245 closeArray(); 24246 }; 24247 if (element.hasVersioningElement()) { 24248 composeEnumerationCore("versioning", element.getVersioningElement(), new CapabilityStatement.ResourceVersionPolicyEnumFactory(), false); 24249 composeEnumerationExtras("versioning", element.getVersioningElement(), new CapabilityStatement.ResourceVersionPolicyEnumFactory(), false); 24250 } 24251 if (element.hasReadHistoryElement()) { 24252 composeBooleanCore("readHistory", element.getReadHistoryElement(), false); 24253 composeBooleanExtras("readHistory", element.getReadHistoryElement(), false); 24254 } 24255 if (element.hasUpdateCreateElement()) { 24256 composeBooleanCore("updateCreate", element.getUpdateCreateElement(), false); 24257 composeBooleanExtras("updateCreate", element.getUpdateCreateElement(), false); 24258 } 24259 if (element.hasConditionalCreateElement()) { 24260 composeBooleanCore("conditionalCreate", element.getConditionalCreateElement(), false); 24261 composeBooleanExtras("conditionalCreate", element.getConditionalCreateElement(), false); 24262 } 24263 if (element.hasConditionalReadElement()) { 24264 composeEnumerationCore("conditionalRead", element.getConditionalReadElement(), new CapabilityStatement.ConditionalReadStatusEnumFactory(), false); 24265 composeEnumerationExtras("conditionalRead", element.getConditionalReadElement(), new CapabilityStatement.ConditionalReadStatusEnumFactory(), false); 24266 } 24267 if (element.hasConditionalUpdateElement()) { 24268 composeBooleanCore("conditionalUpdate", element.getConditionalUpdateElement(), false); 24269 composeBooleanExtras("conditionalUpdate", element.getConditionalUpdateElement(), false); 24270 } 24271 if (element.hasConditionalDeleteElement()) { 24272 composeEnumerationCore("conditionalDelete", element.getConditionalDeleteElement(), new CapabilityStatement.ConditionalDeleteStatusEnumFactory(), false); 24273 composeEnumerationExtras("conditionalDelete", element.getConditionalDeleteElement(), new CapabilityStatement.ConditionalDeleteStatusEnumFactory(), false); 24274 } 24275 if (element.hasReferencePolicy()) { 24276 openArray("referencePolicy"); 24277 for (Enumeration<CapabilityStatement.ReferenceHandlingPolicy> e : element.getReferencePolicy()) 24278 composeEnumerationCore(null, e, new CapabilityStatement.ReferenceHandlingPolicyEnumFactory(), true); 24279 closeArray(); 24280 if (anyHasExtras(element.getReferencePolicy())) { 24281 openArray("_referencePolicy"); 24282 for (Enumeration<CapabilityStatement.ReferenceHandlingPolicy> e : element.getReferencePolicy()) 24283 composeEnumerationExtras(null, e, new CapabilityStatement.ReferenceHandlingPolicyEnumFactory(), true); 24284 closeArray(); 24285 } 24286 }; 24287 if (element.hasSearchInclude()) { 24288 openArray("searchInclude"); 24289 for (StringType e : element.getSearchInclude()) 24290 composeStringCore(null, e, true); 24291 closeArray(); 24292 if (anyHasExtras(element.getSearchInclude())) { 24293 openArray("_searchInclude"); 24294 for (StringType e : element.getSearchInclude()) 24295 composeStringExtras(null, e, true); 24296 closeArray(); 24297 } 24298 }; 24299 if (element.hasSearchRevInclude()) { 24300 openArray("searchRevInclude"); 24301 for (StringType e : element.getSearchRevInclude()) 24302 composeStringCore(null, e, true); 24303 closeArray(); 24304 if (anyHasExtras(element.getSearchRevInclude())) { 24305 openArray("_searchRevInclude"); 24306 for (StringType e : element.getSearchRevInclude()) 24307 composeStringExtras(null, e, true); 24308 closeArray(); 24309 } 24310 }; 24311 if (element.hasSearchParam()) { 24312 openArray("searchParam"); 24313 for (CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent e : element.getSearchParam()) 24314 composeCapabilityStatementCapabilityStatementRestResourceSearchParamComponent(null, e); 24315 closeArray(); 24316 }; 24317 } 24318 24319 protected void composeCapabilityStatementResourceInteractionComponent(String name, CapabilityStatement.ResourceInteractionComponent element) throws IOException { 24320 if (element != null) { 24321 open(name); 24322 composeCapabilityStatementResourceInteractionComponentInner(element); 24323 close(); 24324 } 24325 } 24326 24327 protected void composeCapabilityStatementResourceInteractionComponentInner(CapabilityStatement.ResourceInteractionComponent element) throws IOException { 24328 composeBackbone(element); 24329 if (element.hasCodeElement()) { 24330 composeEnumerationCore("code", element.getCodeElement(), new CapabilityStatement.TypeRestfulInteractionEnumFactory(), false); 24331 composeEnumerationExtras("code", element.getCodeElement(), new CapabilityStatement.TypeRestfulInteractionEnumFactory(), false); 24332 } 24333 if (element.hasDocumentationElement()) { 24334 composeStringCore("documentation", element.getDocumentationElement(), false); 24335 composeStringExtras("documentation", element.getDocumentationElement(), false); 24336 } 24337 } 24338 24339 protected void composeCapabilityStatementCapabilityStatementRestResourceSearchParamComponent(String name, CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent element) throws IOException { 24340 if (element != null) { 24341 open(name); 24342 composeCapabilityStatementCapabilityStatementRestResourceSearchParamComponentInner(element); 24343 close(); 24344 } 24345 } 24346 24347 protected void composeCapabilityStatementCapabilityStatementRestResourceSearchParamComponentInner(CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent element) throws IOException { 24348 composeBackbone(element); 24349 if (element.hasNameElement()) { 24350 composeStringCore("name", element.getNameElement(), false); 24351 composeStringExtras("name", element.getNameElement(), false); 24352 } 24353 if (element.hasDefinitionElement()) { 24354 composeUriCore("definition", element.getDefinitionElement(), false); 24355 composeUriExtras("definition", element.getDefinitionElement(), false); 24356 } 24357 if (element.hasTypeElement()) { 24358 composeEnumerationCore("type", element.getTypeElement(), new Enumerations.SearchParamTypeEnumFactory(), false); 24359 composeEnumerationExtras("type", element.getTypeElement(), new Enumerations.SearchParamTypeEnumFactory(), false); 24360 } 24361 if (element.hasDocumentationElement()) { 24362 composeStringCore("documentation", element.getDocumentationElement(), false); 24363 composeStringExtras("documentation", element.getDocumentationElement(), false); 24364 } 24365 } 24366 24367 protected void composeCapabilityStatementSystemInteractionComponent(String name, CapabilityStatement.SystemInteractionComponent element) throws IOException { 24368 if (element != null) { 24369 open(name); 24370 composeCapabilityStatementSystemInteractionComponentInner(element); 24371 close(); 24372 } 24373 } 24374 24375 protected void composeCapabilityStatementSystemInteractionComponentInner(CapabilityStatement.SystemInteractionComponent element) throws IOException { 24376 composeBackbone(element); 24377 if (element.hasCodeElement()) { 24378 composeEnumerationCore("code", element.getCodeElement(), new CapabilityStatement.SystemRestfulInteractionEnumFactory(), false); 24379 composeEnumerationExtras("code", element.getCodeElement(), new CapabilityStatement.SystemRestfulInteractionEnumFactory(), false); 24380 } 24381 if (element.hasDocumentationElement()) { 24382 composeStringCore("documentation", element.getDocumentationElement(), false); 24383 composeStringExtras("documentation", element.getDocumentationElement(), false); 24384 } 24385 } 24386 24387 protected void composeCapabilityStatementCapabilityStatementRestOperationComponent(String name, CapabilityStatement.CapabilityStatementRestOperationComponent element) throws IOException { 24388 if (element != null) { 24389 open(name); 24390 composeCapabilityStatementCapabilityStatementRestOperationComponentInner(element); 24391 close(); 24392 } 24393 } 24394 24395 protected void composeCapabilityStatementCapabilityStatementRestOperationComponentInner(CapabilityStatement.CapabilityStatementRestOperationComponent element) throws IOException { 24396 composeBackbone(element); 24397 if (element.hasNameElement()) { 24398 composeStringCore("name", element.getNameElement(), false); 24399 composeStringExtras("name", element.getNameElement(), false); 24400 } 24401 if (element.hasDefinition()) { 24402 composeReference("definition", element.getDefinition()); 24403 } 24404 } 24405 24406 protected void composeCapabilityStatementCapabilityStatementMessagingComponent(String name, CapabilityStatement.CapabilityStatementMessagingComponent element) throws IOException { 24407 if (element != null) { 24408 open(name); 24409 composeCapabilityStatementCapabilityStatementMessagingComponentInner(element); 24410 close(); 24411 } 24412 } 24413 24414 protected void composeCapabilityStatementCapabilityStatementMessagingComponentInner(CapabilityStatement.CapabilityStatementMessagingComponent element) throws IOException { 24415 composeBackbone(element); 24416 if (element.hasEndpoint()) { 24417 openArray("endpoint"); 24418 for (CapabilityStatement.CapabilityStatementMessagingEndpointComponent e : element.getEndpoint()) 24419 composeCapabilityStatementCapabilityStatementMessagingEndpointComponent(null, e); 24420 closeArray(); 24421 }; 24422 if (element.hasReliableCacheElement()) { 24423 composeUnsignedIntCore("reliableCache", element.getReliableCacheElement(), false); 24424 composeUnsignedIntExtras("reliableCache", element.getReliableCacheElement(), false); 24425 } 24426 if (element.hasDocumentationElement()) { 24427 composeStringCore("documentation", element.getDocumentationElement(), false); 24428 composeStringExtras("documentation", element.getDocumentationElement(), false); 24429 } 24430 if (element.hasSupportedMessage()) { 24431 openArray("supportedMessage"); 24432 for (CapabilityStatement.CapabilityStatementMessagingSupportedMessageComponent e : element.getSupportedMessage()) 24433 composeCapabilityStatementCapabilityStatementMessagingSupportedMessageComponent(null, e); 24434 closeArray(); 24435 }; 24436 if (element.hasEvent()) { 24437 openArray("event"); 24438 for (CapabilityStatement.CapabilityStatementMessagingEventComponent e : element.getEvent()) 24439 composeCapabilityStatementCapabilityStatementMessagingEventComponent(null, e); 24440 closeArray(); 24441 }; 24442 } 24443 24444 protected void composeCapabilityStatementCapabilityStatementMessagingEndpointComponent(String name, CapabilityStatement.CapabilityStatementMessagingEndpointComponent element) throws IOException { 24445 if (element != null) { 24446 open(name); 24447 composeCapabilityStatementCapabilityStatementMessagingEndpointComponentInner(element); 24448 close(); 24449 } 24450 } 24451 24452 protected void composeCapabilityStatementCapabilityStatementMessagingEndpointComponentInner(CapabilityStatement.CapabilityStatementMessagingEndpointComponent element) throws IOException { 24453 composeBackbone(element); 24454 if (element.hasProtocol()) { 24455 composeCoding("protocol", element.getProtocol()); 24456 } 24457 if (element.hasAddressElement()) { 24458 composeUriCore("address", element.getAddressElement(), false); 24459 composeUriExtras("address", element.getAddressElement(), false); 24460 } 24461 } 24462 24463 protected void composeCapabilityStatementCapabilityStatementMessagingSupportedMessageComponent(String name, CapabilityStatement.CapabilityStatementMessagingSupportedMessageComponent element) throws IOException { 24464 if (element != null) { 24465 open(name); 24466 composeCapabilityStatementCapabilityStatementMessagingSupportedMessageComponentInner(element); 24467 close(); 24468 } 24469 } 24470 24471 protected void composeCapabilityStatementCapabilityStatementMessagingSupportedMessageComponentInner(CapabilityStatement.CapabilityStatementMessagingSupportedMessageComponent element) throws IOException { 24472 composeBackbone(element); 24473 if (element.hasModeElement()) { 24474 composeEnumerationCore("mode", element.getModeElement(), new CapabilityStatement.EventCapabilityModeEnumFactory(), false); 24475 composeEnumerationExtras("mode", element.getModeElement(), new CapabilityStatement.EventCapabilityModeEnumFactory(), false); 24476 } 24477 if (element.hasDefinition()) { 24478 composeReference("definition", element.getDefinition()); 24479 } 24480 } 24481 24482 protected void composeCapabilityStatementCapabilityStatementMessagingEventComponent(String name, CapabilityStatement.CapabilityStatementMessagingEventComponent element) throws IOException { 24483 if (element != null) { 24484 open(name); 24485 composeCapabilityStatementCapabilityStatementMessagingEventComponentInner(element); 24486 close(); 24487 } 24488 } 24489 24490 protected void composeCapabilityStatementCapabilityStatementMessagingEventComponentInner(CapabilityStatement.CapabilityStatementMessagingEventComponent element) throws IOException { 24491 composeBackbone(element); 24492 if (element.hasCode()) { 24493 composeCoding("code", element.getCode()); 24494 } 24495 if (element.hasCategoryElement()) { 24496 composeEnumerationCore("category", element.getCategoryElement(), new CapabilityStatement.MessageSignificanceCategoryEnumFactory(), false); 24497 composeEnumerationExtras("category", element.getCategoryElement(), new CapabilityStatement.MessageSignificanceCategoryEnumFactory(), false); 24498 } 24499 if (element.hasModeElement()) { 24500 composeEnumerationCore("mode", element.getModeElement(), new CapabilityStatement.EventCapabilityModeEnumFactory(), false); 24501 composeEnumerationExtras("mode", element.getModeElement(), new CapabilityStatement.EventCapabilityModeEnumFactory(), false); 24502 } 24503 if (element.hasFocusElement()) { 24504 composeCodeCore("focus", element.getFocusElement(), false); 24505 composeCodeExtras("focus", element.getFocusElement(), false); 24506 } 24507 if (element.hasRequest()) { 24508 composeReference("request", element.getRequest()); 24509 } 24510 if (element.hasResponse()) { 24511 composeReference("response", element.getResponse()); 24512 } 24513 if (element.hasDocumentationElement()) { 24514 composeStringCore("documentation", element.getDocumentationElement(), false); 24515 composeStringExtras("documentation", element.getDocumentationElement(), false); 24516 } 24517 } 24518 24519 protected void composeCapabilityStatementCapabilityStatementDocumentComponent(String name, CapabilityStatement.CapabilityStatementDocumentComponent element) throws IOException { 24520 if (element != null) { 24521 open(name); 24522 composeCapabilityStatementCapabilityStatementDocumentComponentInner(element); 24523 close(); 24524 } 24525 } 24526 24527 protected void composeCapabilityStatementCapabilityStatementDocumentComponentInner(CapabilityStatement.CapabilityStatementDocumentComponent element) throws IOException { 24528 composeBackbone(element); 24529 if (element.hasModeElement()) { 24530 composeEnumerationCore("mode", element.getModeElement(), new CapabilityStatement.DocumentModeEnumFactory(), false); 24531 composeEnumerationExtras("mode", element.getModeElement(), new CapabilityStatement.DocumentModeEnumFactory(), false); 24532 } 24533 if (element.hasDocumentationElement()) { 24534 composeStringCore("documentation", element.getDocumentationElement(), false); 24535 composeStringExtras("documentation", element.getDocumentationElement(), false); 24536 } 24537 if (element.hasProfile()) { 24538 composeReference("profile", element.getProfile()); 24539 } 24540 } 24541 24542 protected void composeCarePlan(String name, CarePlan element) throws IOException { 24543 if (element != null) { 24544 prop("resourceType", name); 24545 composeCarePlanInner(element); 24546 } 24547 } 24548 24549 protected void composeCarePlanInner(CarePlan element) throws IOException { 24550 composeDomainResourceElements(element); 24551 if (element.hasIdentifier()) { 24552 openArray("identifier"); 24553 for (Identifier e : element.getIdentifier()) 24554 composeIdentifier(null, e); 24555 closeArray(); 24556 }; 24557 if (element.hasDefinition()) { 24558 openArray("definition"); 24559 for (Reference e : element.getDefinition()) 24560 composeReference(null, e); 24561 closeArray(); 24562 }; 24563 if (element.hasBasedOn()) { 24564 openArray("basedOn"); 24565 for (Reference e : element.getBasedOn()) 24566 composeReference(null, e); 24567 closeArray(); 24568 }; 24569 if (element.hasReplaces()) { 24570 openArray("replaces"); 24571 for (Reference e : element.getReplaces()) 24572 composeReference(null, e); 24573 closeArray(); 24574 }; 24575 if (element.hasPartOf()) { 24576 openArray("partOf"); 24577 for (Reference e : element.getPartOf()) 24578 composeReference(null, e); 24579 closeArray(); 24580 }; 24581 if (element.hasStatusElement()) { 24582 composeEnumerationCore("status", element.getStatusElement(), new CarePlan.CarePlanStatusEnumFactory(), false); 24583 composeEnumerationExtras("status", element.getStatusElement(), new CarePlan.CarePlanStatusEnumFactory(), false); 24584 } 24585 if (element.hasIntentElement()) { 24586 composeEnumerationCore("intent", element.getIntentElement(), new CarePlan.CarePlanIntentEnumFactory(), false); 24587 composeEnumerationExtras("intent", element.getIntentElement(), new CarePlan.CarePlanIntentEnumFactory(), false); 24588 } 24589 if (element.hasCategory()) { 24590 openArray("category"); 24591 for (CodeableConcept e : element.getCategory()) 24592 composeCodeableConcept(null, e); 24593 closeArray(); 24594 }; 24595 if (element.hasTitleElement()) { 24596 composeStringCore("title", element.getTitleElement(), false); 24597 composeStringExtras("title", element.getTitleElement(), false); 24598 } 24599 if (element.hasDescriptionElement()) { 24600 composeStringCore("description", element.getDescriptionElement(), false); 24601 composeStringExtras("description", element.getDescriptionElement(), false); 24602 } 24603 if (element.hasSubject()) { 24604 composeReference("subject", element.getSubject()); 24605 } 24606 if (element.hasContext()) { 24607 composeReference("context", element.getContext()); 24608 } 24609 if (element.hasPeriod()) { 24610 composePeriod("period", element.getPeriod()); 24611 } 24612 if (element.hasAuthor()) { 24613 openArray("author"); 24614 for (Reference e : element.getAuthor()) 24615 composeReference(null, e); 24616 closeArray(); 24617 }; 24618 if (element.hasCareTeam()) { 24619 openArray("careTeam"); 24620 for (Reference e : element.getCareTeam()) 24621 composeReference(null, e); 24622 closeArray(); 24623 }; 24624 if (element.hasAddresses()) { 24625 openArray("addresses"); 24626 for (Reference e : element.getAddresses()) 24627 composeReference(null, e); 24628 closeArray(); 24629 }; 24630 if (element.hasSupportingInfo()) { 24631 openArray("supportingInfo"); 24632 for (Reference e : element.getSupportingInfo()) 24633 composeReference(null, e); 24634 closeArray(); 24635 }; 24636 if (element.hasGoal()) { 24637 openArray("goal"); 24638 for (Reference e : element.getGoal()) 24639 composeReference(null, e); 24640 closeArray(); 24641 }; 24642 if (element.hasActivity()) { 24643 openArray("activity"); 24644 for (CarePlan.CarePlanActivityComponent e : element.getActivity()) 24645 composeCarePlanCarePlanActivityComponent(null, e); 24646 closeArray(); 24647 }; 24648 if (element.hasNote()) { 24649 openArray("note"); 24650 for (Annotation e : element.getNote()) 24651 composeAnnotation(null, e); 24652 closeArray(); 24653 }; 24654 } 24655 24656 protected void composeCarePlanCarePlanActivityComponent(String name, CarePlan.CarePlanActivityComponent element) throws IOException { 24657 if (element != null) { 24658 open(name); 24659 composeCarePlanCarePlanActivityComponentInner(element); 24660 close(); 24661 } 24662 } 24663 24664 protected void composeCarePlanCarePlanActivityComponentInner(CarePlan.CarePlanActivityComponent element) throws IOException { 24665 composeBackbone(element); 24666 if (element.hasOutcomeCodeableConcept()) { 24667 openArray("outcomeCodeableConcept"); 24668 for (CodeableConcept e : element.getOutcomeCodeableConcept()) 24669 composeCodeableConcept(null, e); 24670 closeArray(); 24671 }; 24672 if (element.hasOutcomeReference()) { 24673 openArray("outcomeReference"); 24674 for (Reference e : element.getOutcomeReference()) 24675 composeReference(null, e); 24676 closeArray(); 24677 }; 24678 if (element.hasProgress()) { 24679 openArray("progress"); 24680 for (Annotation e : element.getProgress()) 24681 composeAnnotation(null, e); 24682 closeArray(); 24683 }; 24684 if (element.hasReference()) { 24685 composeReference("reference", element.getReference()); 24686 } 24687 if (element.hasDetail()) { 24688 composeCarePlanCarePlanActivityDetailComponent("detail", element.getDetail()); 24689 } 24690 } 24691 24692 protected void composeCarePlanCarePlanActivityDetailComponent(String name, CarePlan.CarePlanActivityDetailComponent element) throws IOException { 24693 if (element != null) { 24694 open(name); 24695 composeCarePlanCarePlanActivityDetailComponentInner(element); 24696 close(); 24697 } 24698 } 24699 24700 protected void composeCarePlanCarePlanActivityDetailComponentInner(CarePlan.CarePlanActivityDetailComponent element) throws IOException { 24701 composeBackbone(element); 24702 if (element.hasCategory()) { 24703 composeCodeableConcept("category", element.getCategory()); 24704 } 24705 if (element.hasDefinition()) { 24706 composeReference("definition", element.getDefinition()); 24707 } 24708 if (element.hasCode()) { 24709 composeCodeableConcept("code", element.getCode()); 24710 } 24711 if (element.hasReasonCode()) { 24712 openArray("reasonCode"); 24713 for (CodeableConcept e : element.getReasonCode()) 24714 composeCodeableConcept(null, e); 24715 closeArray(); 24716 }; 24717 if (element.hasReasonReference()) { 24718 openArray("reasonReference"); 24719 for (Reference e : element.getReasonReference()) 24720 composeReference(null, e); 24721 closeArray(); 24722 }; 24723 if (element.hasGoal()) { 24724 openArray("goal"); 24725 for (Reference e : element.getGoal()) 24726 composeReference(null, e); 24727 closeArray(); 24728 }; 24729 if (element.hasStatusElement()) { 24730 composeEnumerationCore("status", element.getStatusElement(), new CarePlan.CarePlanActivityStatusEnumFactory(), false); 24731 composeEnumerationExtras("status", element.getStatusElement(), new CarePlan.CarePlanActivityStatusEnumFactory(), false); 24732 } 24733 if (element.hasStatusReasonElement()) { 24734 composeStringCore("statusReason", element.getStatusReasonElement(), false); 24735 composeStringExtras("statusReason", element.getStatusReasonElement(), false); 24736 } 24737 if (element.hasProhibitedElement()) { 24738 composeBooleanCore("prohibited", element.getProhibitedElement(), false); 24739 composeBooleanExtras("prohibited", element.getProhibitedElement(), false); 24740 } 24741 if (element.hasScheduled()) { 24742 composeType("scheduled", element.getScheduled()); 24743 } 24744 if (element.hasLocation()) { 24745 composeReference("location", element.getLocation()); 24746 } 24747 if (element.hasPerformer()) { 24748 openArray("performer"); 24749 for (Reference e : element.getPerformer()) 24750 composeReference(null, e); 24751 closeArray(); 24752 }; 24753 if (element.hasProduct()) { 24754 composeType("product", element.getProduct()); 24755 } 24756 if (element.hasDailyAmount()) { 24757 composeSimpleQuantity("dailyAmount", element.getDailyAmount()); 24758 } 24759 if (element.hasQuantity()) { 24760 composeSimpleQuantity("quantity", element.getQuantity()); 24761 } 24762 if (element.hasDescriptionElement()) { 24763 composeStringCore("description", element.getDescriptionElement(), false); 24764 composeStringExtras("description", element.getDescriptionElement(), false); 24765 } 24766 } 24767 24768 protected void composeCareTeam(String name, CareTeam element) throws IOException { 24769 if (element != null) { 24770 prop("resourceType", name); 24771 composeCareTeamInner(element); 24772 } 24773 } 24774 24775 protected void composeCareTeamInner(CareTeam element) throws IOException { 24776 composeDomainResourceElements(element); 24777 if (element.hasIdentifier()) { 24778 openArray("identifier"); 24779 for (Identifier e : element.getIdentifier()) 24780 composeIdentifier(null, e); 24781 closeArray(); 24782 }; 24783 if (element.hasStatusElement()) { 24784 composeEnumerationCore("status", element.getStatusElement(), new CareTeam.CareTeamStatusEnumFactory(), false); 24785 composeEnumerationExtras("status", element.getStatusElement(), new CareTeam.CareTeamStatusEnumFactory(), false); 24786 } 24787 if (element.hasCategory()) { 24788 openArray("category"); 24789 for (CodeableConcept e : element.getCategory()) 24790 composeCodeableConcept(null, e); 24791 closeArray(); 24792 }; 24793 if (element.hasNameElement()) { 24794 composeStringCore("name", element.getNameElement(), false); 24795 composeStringExtras("name", element.getNameElement(), false); 24796 } 24797 if (element.hasSubject()) { 24798 composeReference("subject", element.getSubject()); 24799 } 24800 if (element.hasContext()) { 24801 composeReference("context", element.getContext()); 24802 } 24803 if (element.hasPeriod()) { 24804 composePeriod("period", element.getPeriod()); 24805 } 24806 if (element.hasParticipant()) { 24807 openArray("participant"); 24808 for (CareTeam.CareTeamParticipantComponent e : element.getParticipant()) 24809 composeCareTeamCareTeamParticipantComponent(null, e); 24810 closeArray(); 24811 }; 24812 if (element.hasReasonCode()) { 24813 openArray("reasonCode"); 24814 for (CodeableConcept e : element.getReasonCode()) 24815 composeCodeableConcept(null, e); 24816 closeArray(); 24817 }; 24818 if (element.hasReasonReference()) { 24819 openArray("reasonReference"); 24820 for (Reference e : element.getReasonReference()) 24821 composeReference(null, e); 24822 closeArray(); 24823 }; 24824 if (element.hasManagingOrganization()) { 24825 openArray("managingOrganization"); 24826 for (Reference e : element.getManagingOrganization()) 24827 composeReference(null, e); 24828 closeArray(); 24829 }; 24830 if (element.hasNote()) { 24831 openArray("note"); 24832 for (Annotation e : element.getNote()) 24833 composeAnnotation(null, e); 24834 closeArray(); 24835 }; 24836 } 24837 24838 protected void composeCareTeamCareTeamParticipantComponent(String name, CareTeam.CareTeamParticipantComponent element) throws IOException { 24839 if (element != null) { 24840 open(name); 24841 composeCareTeamCareTeamParticipantComponentInner(element); 24842 close(); 24843 } 24844 } 24845 24846 protected void composeCareTeamCareTeamParticipantComponentInner(CareTeam.CareTeamParticipantComponent element) throws IOException { 24847 composeBackbone(element); 24848 if (element.hasRole()) { 24849 composeCodeableConcept("role", element.getRole()); 24850 } 24851 if (element.hasMember()) { 24852 composeReference("member", element.getMember()); 24853 } 24854 if (element.hasOnBehalfOf()) { 24855 composeReference("onBehalfOf", element.getOnBehalfOf()); 24856 } 24857 if (element.hasPeriod()) { 24858 composePeriod("period", element.getPeriod()); 24859 } 24860 } 24861 24862 protected void composeChargeItem(String name, ChargeItem element) throws IOException { 24863 if (element != null) { 24864 prop("resourceType", name); 24865 composeChargeItemInner(element); 24866 } 24867 } 24868 24869 protected void composeChargeItemInner(ChargeItem element) throws IOException { 24870 composeDomainResourceElements(element); 24871 if (element.hasIdentifier()) { 24872 composeIdentifier("identifier", element.getIdentifier()); 24873 } 24874 if (element.hasDefinition()) { 24875 openArray("definition"); 24876 for (UriType e : element.getDefinition()) 24877 composeUriCore(null, e, true); 24878 closeArray(); 24879 if (anyHasExtras(element.getDefinition())) { 24880 openArray("_definition"); 24881 for (UriType e : element.getDefinition()) 24882 composeUriExtras(null, e, true); 24883 closeArray(); 24884 } 24885 }; 24886 if (element.hasStatusElement()) { 24887 composeEnumerationCore("status", element.getStatusElement(), new ChargeItem.ChargeItemStatusEnumFactory(), false); 24888 composeEnumerationExtras("status", element.getStatusElement(), new ChargeItem.ChargeItemStatusEnumFactory(), false); 24889 } 24890 if (element.hasPartOf()) { 24891 openArray("partOf"); 24892 for (Reference e : element.getPartOf()) 24893 composeReference(null, e); 24894 closeArray(); 24895 }; 24896 if (element.hasCode()) { 24897 composeCodeableConcept("code", element.getCode()); 24898 } 24899 if (element.hasSubject()) { 24900 composeReference("subject", element.getSubject()); 24901 } 24902 if (element.hasContext()) { 24903 composeReference("context", element.getContext()); 24904 } 24905 if (element.hasOccurrence()) { 24906 composeType("occurrence", element.getOccurrence()); 24907 } 24908 if (element.hasParticipant()) { 24909 openArray("participant"); 24910 for (ChargeItem.ChargeItemParticipantComponent e : element.getParticipant()) 24911 composeChargeItemChargeItemParticipantComponent(null, e); 24912 closeArray(); 24913 }; 24914 if (element.hasPerformingOrganization()) { 24915 composeReference("performingOrganization", element.getPerformingOrganization()); 24916 } 24917 if (element.hasRequestingOrganization()) { 24918 composeReference("requestingOrganization", element.getRequestingOrganization()); 24919 } 24920 if (element.hasQuantity()) { 24921 composeQuantity("quantity", element.getQuantity()); 24922 } 24923 if (element.hasBodysite()) { 24924 openArray("bodysite"); 24925 for (CodeableConcept e : element.getBodysite()) 24926 composeCodeableConcept(null, e); 24927 closeArray(); 24928 }; 24929 if (element.hasFactorOverrideElement()) { 24930 composeDecimalCore("factorOverride", element.getFactorOverrideElement(), false); 24931 composeDecimalExtras("factorOverride", element.getFactorOverrideElement(), false); 24932 } 24933 if (element.hasPriceOverride()) { 24934 composeMoney("priceOverride", element.getPriceOverride()); 24935 } 24936 if (element.hasOverrideReasonElement()) { 24937 composeStringCore("overrideReason", element.getOverrideReasonElement(), false); 24938 composeStringExtras("overrideReason", element.getOverrideReasonElement(), false); 24939 } 24940 if (element.hasEnterer()) { 24941 composeReference("enterer", element.getEnterer()); 24942 } 24943 if (element.hasEnteredDateElement()) { 24944 composeDateTimeCore("enteredDate", element.getEnteredDateElement(), false); 24945 composeDateTimeExtras("enteredDate", element.getEnteredDateElement(), false); 24946 } 24947 if (element.hasReason()) { 24948 openArray("reason"); 24949 for (CodeableConcept e : element.getReason()) 24950 composeCodeableConcept(null, e); 24951 closeArray(); 24952 }; 24953 if (element.hasService()) { 24954 openArray("service"); 24955 for (Reference e : element.getService()) 24956 composeReference(null, e); 24957 closeArray(); 24958 }; 24959 if (element.hasAccount()) { 24960 openArray("account"); 24961 for (Reference e : element.getAccount()) 24962 composeReference(null, e); 24963 closeArray(); 24964 }; 24965 if (element.hasNote()) { 24966 openArray("note"); 24967 for (Annotation e : element.getNote()) 24968 composeAnnotation(null, e); 24969 closeArray(); 24970 }; 24971 if (element.hasSupportingInformation()) { 24972 openArray("supportingInformation"); 24973 for (Reference e : element.getSupportingInformation()) 24974 composeReference(null, e); 24975 closeArray(); 24976 }; 24977 } 24978 24979 protected void composeChargeItemChargeItemParticipantComponent(String name, ChargeItem.ChargeItemParticipantComponent element) throws IOException { 24980 if (element != null) { 24981 open(name); 24982 composeChargeItemChargeItemParticipantComponentInner(element); 24983 close(); 24984 } 24985 } 24986 24987 protected void composeChargeItemChargeItemParticipantComponentInner(ChargeItem.ChargeItemParticipantComponent element) throws IOException { 24988 composeBackbone(element); 24989 if (element.hasRole()) { 24990 composeCodeableConcept("role", element.getRole()); 24991 } 24992 if (element.hasActor()) { 24993 composeReference("actor", element.getActor()); 24994 } 24995 } 24996 24997 protected void composeClaim(String name, Claim element) throws IOException { 24998 if (element != null) { 24999 prop("resourceType", name); 25000 composeClaimInner(element); 25001 } 25002 } 25003 25004 protected void composeClaimInner(Claim element) throws IOException { 25005 composeDomainResourceElements(element); 25006 if (element.hasIdentifier()) { 25007 openArray("identifier"); 25008 for (Identifier e : element.getIdentifier()) 25009 composeIdentifier(null, e); 25010 closeArray(); 25011 }; 25012 if (element.hasStatusElement()) { 25013 composeEnumerationCore("status", element.getStatusElement(), new Claim.ClaimStatusEnumFactory(), false); 25014 composeEnumerationExtras("status", element.getStatusElement(), new Claim.ClaimStatusEnumFactory(), false); 25015 } 25016 if (element.hasType()) { 25017 composeCodeableConcept("type", element.getType()); 25018 } 25019 if (element.hasSubType()) { 25020 openArray("subType"); 25021 for (CodeableConcept e : element.getSubType()) 25022 composeCodeableConcept(null, e); 25023 closeArray(); 25024 }; 25025 if (element.hasUseElement()) { 25026 composeEnumerationCore("use", element.getUseElement(), new Claim.UseEnumFactory(), false); 25027 composeEnumerationExtras("use", element.getUseElement(), new Claim.UseEnumFactory(), false); 25028 } 25029 if (element.hasPatient()) { 25030 composeReference("patient", element.getPatient()); 25031 } 25032 if (element.hasBillablePeriod()) { 25033 composePeriod("billablePeriod", element.getBillablePeriod()); 25034 } 25035 if (element.hasCreatedElement()) { 25036 composeDateTimeCore("created", element.getCreatedElement(), false); 25037 composeDateTimeExtras("created", element.getCreatedElement(), false); 25038 } 25039 if (element.hasEnterer()) { 25040 composeReference("enterer", element.getEnterer()); 25041 } 25042 if (element.hasInsurer()) { 25043 composeReference("insurer", element.getInsurer()); 25044 } 25045 if (element.hasProvider()) { 25046 composeReference("provider", element.getProvider()); 25047 } 25048 if (element.hasOrganization()) { 25049 composeReference("organization", element.getOrganization()); 25050 } 25051 if (element.hasPriority()) { 25052 composeCodeableConcept("priority", element.getPriority()); 25053 } 25054 if (element.hasFundsReserve()) { 25055 composeCodeableConcept("fundsReserve", element.getFundsReserve()); 25056 } 25057 if (element.hasRelated()) { 25058 openArray("related"); 25059 for (Claim.RelatedClaimComponent e : element.getRelated()) 25060 composeClaimRelatedClaimComponent(null, e); 25061 closeArray(); 25062 }; 25063 if (element.hasPrescription()) { 25064 composeReference("prescription", element.getPrescription()); 25065 } 25066 if (element.hasOriginalPrescription()) { 25067 composeReference("originalPrescription", element.getOriginalPrescription()); 25068 } 25069 if (element.hasPayee()) { 25070 composeClaimPayeeComponent("payee", element.getPayee()); 25071 } 25072 if (element.hasReferral()) { 25073 composeReference("referral", element.getReferral()); 25074 } 25075 if (element.hasFacility()) { 25076 composeReference("facility", element.getFacility()); 25077 } 25078 if (element.hasCareTeam()) { 25079 openArray("careTeam"); 25080 for (Claim.CareTeamComponent e : element.getCareTeam()) 25081 composeClaimCareTeamComponent(null, e); 25082 closeArray(); 25083 }; 25084 if (element.hasInformation()) { 25085 openArray("information"); 25086 for (Claim.SpecialConditionComponent e : element.getInformation()) 25087 composeClaimSpecialConditionComponent(null, e); 25088 closeArray(); 25089 }; 25090 if (element.hasDiagnosis()) { 25091 openArray("diagnosis"); 25092 for (Claim.DiagnosisComponent e : element.getDiagnosis()) 25093 composeClaimDiagnosisComponent(null, e); 25094 closeArray(); 25095 }; 25096 if (element.hasProcedure()) { 25097 openArray("procedure"); 25098 for (Claim.ProcedureComponent e : element.getProcedure()) 25099 composeClaimProcedureComponent(null, e); 25100 closeArray(); 25101 }; 25102 if (element.hasInsurance()) { 25103 openArray("insurance"); 25104 for (Claim.InsuranceComponent e : element.getInsurance()) 25105 composeClaimInsuranceComponent(null, e); 25106 closeArray(); 25107 }; 25108 if (element.hasAccident()) { 25109 composeClaimAccidentComponent("accident", element.getAccident()); 25110 } 25111 if (element.hasEmploymentImpacted()) { 25112 composePeriod("employmentImpacted", element.getEmploymentImpacted()); 25113 } 25114 if (element.hasHospitalization()) { 25115 composePeriod("hospitalization", element.getHospitalization()); 25116 } 25117 if (element.hasItem()) { 25118 openArray("item"); 25119 for (Claim.ItemComponent e : element.getItem()) 25120 composeClaimItemComponent(null, e); 25121 closeArray(); 25122 }; 25123 if (element.hasTotal()) { 25124 composeMoney("total", element.getTotal()); 25125 } 25126 } 25127 25128 protected void composeClaimRelatedClaimComponent(String name, Claim.RelatedClaimComponent element) throws IOException { 25129 if (element != null) { 25130 open(name); 25131 composeClaimRelatedClaimComponentInner(element); 25132 close(); 25133 } 25134 } 25135 25136 protected void composeClaimRelatedClaimComponentInner(Claim.RelatedClaimComponent element) throws IOException { 25137 composeBackbone(element); 25138 if (element.hasClaim()) { 25139 composeReference("claim", element.getClaim()); 25140 } 25141 if (element.hasRelationship()) { 25142 composeCodeableConcept("relationship", element.getRelationship()); 25143 } 25144 if (element.hasReference()) { 25145 composeIdentifier("reference", element.getReference()); 25146 } 25147 } 25148 25149 protected void composeClaimPayeeComponent(String name, Claim.PayeeComponent element) throws IOException { 25150 if (element != null) { 25151 open(name); 25152 composeClaimPayeeComponentInner(element); 25153 close(); 25154 } 25155 } 25156 25157 protected void composeClaimPayeeComponentInner(Claim.PayeeComponent element) throws IOException { 25158 composeBackbone(element); 25159 if (element.hasType()) { 25160 composeCodeableConcept("type", element.getType()); 25161 } 25162 if (element.hasResourceType()) { 25163 composeCoding("resourceType", element.getResourceType()); 25164 } 25165 if (element.hasParty()) { 25166 composeReference("party", element.getParty()); 25167 } 25168 } 25169 25170 protected void composeClaimCareTeamComponent(String name, Claim.CareTeamComponent element) throws IOException { 25171 if (element != null) { 25172 open(name); 25173 composeClaimCareTeamComponentInner(element); 25174 close(); 25175 } 25176 } 25177 25178 protected void composeClaimCareTeamComponentInner(Claim.CareTeamComponent element) throws IOException { 25179 composeBackbone(element); 25180 if (element.hasSequenceElement()) { 25181 composePositiveIntCore("sequence", element.getSequenceElement(), false); 25182 composePositiveIntExtras("sequence", element.getSequenceElement(), false); 25183 } 25184 if (element.hasProvider()) { 25185 composeReference("provider", element.getProvider()); 25186 } 25187 if (element.hasResponsibleElement()) { 25188 composeBooleanCore("responsible", element.getResponsibleElement(), false); 25189 composeBooleanExtras("responsible", element.getResponsibleElement(), false); 25190 } 25191 if (element.hasRole()) { 25192 composeCodeableConcept("role", element.getRole()); 25193 } 25194 if (element.hasQualification()) { 25195 composeCodeableConcept("qualification", element.getQualification()); 25196 } 25197 } 25198 25199 protected void composeClaimSpecialConditionComponent(String name, Claim.SpecialConditionComponent element) throws IOException { 25200 if (element != null) { 25201 open(name); 25202 composeClaimSpecialConditionComponentInner(element); 25203 close(); 25204 } 25205 } 25206 25207 protected void composeClaimSpecialConditionComponentInner(Claim.SpecialConditionComponent element) throws IOException { 25208 composeBackbone(element); 25209 if (element.hasSequenceElement()) { 25210 composePositiveIntCore("sequence", element.getSequenceElement(), false); 25211 composePositiveIntExtras("sequence", element.getSequenceElement(), false); 25212 } 25213 if (element.hasCategory()) { 25214 composeCodeableConcept("category", element.getCategory()); 25215 } 25216 if (element.hasCode()) { 25217 composeCodeableConcept("code", element.getCode()); 25218 } 25219 if (element.hasTiming()) { 25220 composeType("timing", element.getTiming()); 25221 } 25222 if (element.hasValue()) { 25223 composeType("value", element.getValue()); 25224 } 25225 if (element.hasReason()) { 25226 composeCodeableConcept("reason", element.getReason()); 25227 } 25228 } 25229 25230 protected void composeClaimDiagnosisComponent(String name, Claim.DiagnosisComponent element) throws IOException { 25231 if (element != null) { 25232 open(name); 25233 composeClaimDiagnosisComponentInner(element); 25234 close(); 25235 } 25236 } 25237 25238 protected void composeClaimDiagnosisComponentInner(Claim.DiagnosisComponent element) throws IOException { 25239 composeBackbone(element); 25240 if (element.hasSequenceElement()) { 25241 composePositiveIntCore("sequence", element.getSequenceElement(), false); 25242 composePositiveIntExtras("sequence", element.getSequenceElement(), false); 25243 } 25244 if (element.hasDiagnosis()) { 25245 composeType("diagnosis", element.getDiagnosis()); 25246 } 25247 if (element.hasType()) { 25248 openArray("type"); 25249 for (CodeableConcept e : element.getType()) 25250 composeCodeableConcept(null, e); 25251 closeArray(); 25252 }; 25253 if (element.hasPackageCode()) { 25254 composeCodeableConcept("packageCode", element.getPackageCode()); 25255 } 25256 } 25257 25258 protected void composeClaimProcedureComponent(String name, Claim.ProcedureComponent element) throws IOException { 25259 if (element != null) { 25260 open(name); 25261 composeClaimProcedureComponentInner(element); 25262 close(); 25263 } 25264 } 25265 25266 protected void composeClaimProcedureComponentInner(Claim.ProcedureComponent element) throws IOException { 25267 composeBackbone(element); 25268 if (element.hasSequenceElement()) { 25269 composePositiveIntCore("sequence", element.getSequenceElement(), false); 25270 composePositiveIntExtras("sequence", element.getSequenceElement(), false); 25271 } 25272 if (element.hasDateElement()) { 25273 composeDateTimeCore("date", element.getDateElement(), false); 25274 composeDateTimeExtras("date", element.getDateElement(), false); 25275 } 25276 if (element.hasProcedure()) { 25277 composeType("procedure", element.getProcedure()); 25278 } 25279 } 25280 25281 protected void composeClaimInsuranceComponent(String name, Claim.InsuranceComponent element) throws IOException { 25282 if (element != null) { 25283 open(name); 25284 composeClaimInsuranceComponentInner(element); 25285 close(); 25286 } 25287 } 25288 25289 protected void composeClaimInsuranceComponentInner(Claim.InsuranceComponent element) throws IOException { 25290 composeBackbone(element); 25291 if (element.hasSequenceElement()) { 25292 composePositiveIntCore("sequence", element.getSequenceElement(), false); 25293 composePositiveIntExtras("sequence", element.getSequenceElement(), false); 25294 } 25295 if (element.hasFocalElement()) { 25296 composeBooleanCore("focal", element.getFocalElement(), false); 25297 composeBooleanExtras("focal", element.getFocalElement(), false); 25298 } 25299 if (element.hasCoverage()) { 25300 composeReference("coverage", element.getCoverage()); 25301 } 25302 if (element.hasBusinessArrangementElement()) { 25303 composeStringCore("businessArrangement", element.getBusinessArrangementElement(), false); 25304 composeStringExtras("businessArrangement", element.getBusinessArrangementElement(), false); 25305 } 25306 if (element.hasPreAuthRef()) { 25307 openArray("preAuthRef"); 25308 for (StringType e : element.getPreAuthRef()) 25309 composeStringCore(null, e, true); 25310 closeArray(); 25311 if (anyHasExtras(element.getPreAuthRef())) { 25312 openArray("_preAuthRef"); 25313 for (StringType e : element.getPreAuthRef()) 25314 composeStringExtras(null, e, true); 25315 closeArray(); 25316 } 25317 }; 25318 if (element.hasClaimResponse()) { 25319 composeReference("claimResponse", element.getClaimResponse()); 25320 } 25321 } 25322 25323 protected void composeClaimAccidentComponent(String name, Claim.AccidentComponent element) throws IOException { 25324 if (element != null) { 25325 open(name); 25326 composeClaimAccidentComponentInner(element); 25327 close(); 25328 } 25329 } 25330 25331 protected void composeClaimAccidentComponentInner(Claim.AccidentComponent element) throws IOException { 25332 composeBackbone(element); 25333 if (element.hasDateElement()) { 25334 composeDateCore("date", element.getDateElement(), false); 25335 composeDateExtras("date", element.getDateElement(), false); 25336 } 25337 if (element.hasType()) { 25338 composeCodeableConcept("type", element.getType()); 25339 } 25340 if (element.hasLocation()) { 25341 composeType("location", element.getLocation()); 25342 } 25343 } 25344 25345 protected void composeClaimItemComponent(String name, Claim.ItemComponent element) throws IOException { 25346 if (element != null) { 25347 open(name); 25348 composeClaimItemComponentInner(element); 25349 close(); 25350 } 25351 } 25352 25353 protected void composeClaimItemComponentInner(Claim.ItemComponent element) throws IOException { 25354 composeBackbone(element); 25355 if (element.hasSequenceElement()) { 25356 composePositiveIntCore("sequence", element.getSequenceElement(), false); 25357 composePositiveIntExtras("sequence", element.getSequenceElement(), false); 25358 } 25359 if (element.hasCareTeamLinkId()) { 25360 openArray("careTeamLinkId"); 25361 for (PositiveIntType e : element.getCareTeamLinkId()) 25362 composePositiveIntCore(null, e, true); 25363 closeArray(); 25364 if (anyHasExtras(element.getCareTeamLinkId())) { 25365 openArray("_careTeamLinkId"); 25366 for (PositiveIntType e : element.getCareTeamLinkId()) 25367 composePositiveIntExtras(null, e, true); 25368 closeArray(); 25369 } 25370 }; 25371 if (element.hasDiagnosisLinkId()) { 25372 openArray("diagnosisLinkId"); 25373 for (PositiveIntType e : element.getDiagnosisLinkId()) 25374 composePositiveIntCore(null, e, true); 25375 closeArray(); 25376 if (anyHasExtras(element.getDiagnosisLinkId())) { 25377 openArray("_diagnosisLinkId"); 25378 for (PositiveIntType e : element.getDiagnosisLinkId()) 25379 composePositiveIntExtras(null, e, true); 25380 closeArray(); 25381 } 25382 }; 25383 if (element.hasProcedureLinkId()) { 25384 openArray("procedureLinkId"); 25385 for (PositiveIntType e : element.getProcedureLinkId()) 25386 composePositiveIntCore(null, e, true); 25387 closeArray(); 25388 if (anyHasExtras(element.getProcedureLinkId())) { 25389 openArray("_procedureLinkId"); 25390 for (PositiveIntType e : element.getProcedureLinkId()) 25391 composePositiveIntExtras(null, e, true); 25392 closeArray(); 25393 } 25394 }; 25395 if (element.hasInformationLinkId()) { 25396 openArray("informationLinkId"); 25397 for (PositiveIntType e : element.getInformationLinkId()) 25398 composePositiveIntCore(null, e, true); 25399 closeArray(); 25400 if (anyHasExtras(element.getInformationLinkId())) { 25401 openArray("_informationLinkId"); 25402 for (PositiveIntType e : element.getInformationLinkId()) 25403 composePositiveIntExtras(null, e, true); 25404 closeArray(); 25405 } 25406 }; 25407 if (element.hasRevenue()) { 25408 composeCodeableConcept("revenue", element.getRevenue()); 25409 } 25410 if (element.hasCategory()) { 25411 composeCodeableConcept("category", element.getCategory()); 25412 } 25413 if (element.hasService()) { 25414 composeCodeableConcept("service", element.getService()); 25415 } 25416 if (element.hasModifier()) { 25417 openArray("modifier"); 25418 for (CodeableConcept e : element.getModifier()) 25419 composeCodeableConcept(null, e); 25420 closeArray(); 25421 }; 25422 if (element.hasProgramCode()) { 25423 openArray("programCode"); 25424 for (CodeableConcept e : element.getProgramCode()) 25425 composeCodeableConcept(null, e); 25426 closeArray(); 25427 }; 25428 if (element.hasServiced()) { 25429 composeType("serviced", element.getServiced()); 25430 } 25431 if (element.hasLocation()) { 25432 composeType("location", element.getLocation()); 25433 } 25434 if (element.hasQuantity()) { 25435 composeSimpleQuantity("quantity", element.getQuantity()); 25436 } 25437 if (element.hasUnitPrice()) { 25438 composeMoney("unitPrice", element.getUnitPrice()); 25439 } 25440 if (element.hasFactorElement()) { 25441 composeDecimalCore("factor", element.getFactorElement(), false); 25442 composeDecimalExtras("factor", element.getFactorElement(), false); 25443 } 25444 if (element.hasNet()) { 25445 composeMoney("net", element.getNet()); 25446 } 25447 if (element.hasUdi()) { 25448 openArray("udi"); 25449 for (Reference e : element.getUdi()) 25450 composeReference(null, e); 25451 closeArray(); 25452 }; 25453 if (element.hasBodySite()) { 25454 composeCodeableConcept("bodySite", element.getBodySite()); 25455 } 25456 if (element.hasSubSite()) { 25457 openArray("subSite"); 25458 for (CodeableConcept e : element.getSubSite()) 25459 composeCodeableConcept(null, e); 25460 closeArray(); 25461 }; 25462 if (element.hasEncounter()) { 25463 openArray("encounter"); 25464 for (Reference e : element.getEncounter()) 25465 composeReference(null, e); 25466 closeArray(); 25467 }; 25468 if (element.hasDetail()) { 25469 openArray("detail"); 25470 for (Claim.DetailComponent e : element.getDetail()) 25471 composeClaimDetailComponent(null, e); 25472 closeArray(); 25473 }; 25474 } 25475 25476 protected void composeClaimDetailComponent(String name, Claim.DetailComponent element) throws IOException { 25477 if (element != null) { 25478 open(name); 25479 composeClaimDetailComponentInner(element); 25480 close(); 25481 } 25482 } 25483 25484 protected void composeClaimDetailComponentInner(Claim.DetailComponent element) throws IOException { 25485 composeBackbone(element); 25486 if (element.hasSequenceElement()) { 25487 composePositiveIntCore("sequence", element.getSequenceElement(), false); 25488 composePositiveIntExtras("sequence", element.getSequenceElement(), false); 25489 } 25490 if (element.hasRevenue()) { 25491 composeCodeableConcept("revenue", element.getRevenue()); 25492 } 25493 if (element.hasCategory()) { 25494 composeCodeableConcept("category", element.getCategory()); 25495 } 25496 if (element.hasService()) { 25497 composeCodeableConcept("service", element.getService()); 25498 } 25499 if (element.hasModifier()) { 25500 openArray("modifier"); 25501 for (CodeableConcept e : element.getModifier()) 25502 composeCodeableConcept(null, e); 25503 closeArray(); 25504 }; 25505 if (element.hasProgramCode()) { 25506 openArray("programCode"); 25507 for (CodeableConcept e : element.getProgramCode()) 25508 composeCodeableConcept(null, e); 25509 closeArray(); 25510 }; 25511 if (element.hasQuantity()) { 25512 composeSimpleQuantity("quantity", element.getQuantity()); 25513 } 25514 if (element.hasUnitPrice()) { 25515 composeMoney("unitPrice", element.getUnitPrice()); 25516 } 25517 if (element.hasFactorElement()) { 25518 composeDecimalCore("factor", element.getFactorElement(), false); 25519 composeDecimalExtras("factor", element.getFactorElement(), false); 25520 } 25521 if (element.hasNet()) { 25522 composeMoney("net", element.getNet()); 25523 } 25524 if (element.hasUdi()) { 25525 openArray("udi"); 25526 for (Reference e : element.getUdi()) 25527 composeReference(null, e); 25528 closeArray(); 25529 }; 25530 if (element.hasSubDetail()) { 25531 openArray("subDetail"); 25532 for (Claim.SubDetailComponent e : element.getSubDetail()) 25533 composeClaimSubDetailComponent(null, e); 25534 closeArray(); 25535 }; 25536 } 25537 25538 protected void composeClaimSubDetailComponent(String name, Claim.SubDetailComponent element) throws IOException { 25539 if (element != null) { 25540 open(name); 25541 composeClaimSubDetailComponentInner(element); 25542 close(); 25543 } 25544 } 25545 25546 protected void composeClaimSubDetailComponentInner(Claim.SubDetailComponent element) throws IOException { 25547 composeBackbone(element); 25548 if (element.hasSequenceElement()) { 25549 composePositiveIntCore("sequence", element.getSequenceElement(), false); 25550 composePositiveIntExtras("sequence", element.getSequenceElement(), false); 25551 } 25552 if (element.hasRevenue()) { 25553 composeCodeableConcept("revenue", element.getRevenue()); 25554 } 25555 if (element.hasCategory()) { 25556 composeCodeableConcept("category", element.getCategory()); 25557 } 25558 if (element.hasService()) { 25559 composeCodeableConcept("service", element.getService()); 25560 } 25561 if (element.hasModifier()) { 25562 openArray("modifier"); 25563 for (CodeableConcept e : element.getModifier()) 25564 composeCodeableConcept(null, e); 25565 closeArray(); 25566 }; 25567 if (element.hasProgramCode()) { 25568 openArray("programCode"); 25569 for (CodeableConcept e : element.getProgramCode()) 25570 composeCodeableConcept(null, e); 25571 closeArray(); 25572 }; 25573 if (element.hasQuantity()) { 25574 composeSimpleQuantity("quantity", element.getQuantity()); 25575 } 25576 if (element.hasUnitPrice()) { 25577 composeMoney("unitPrice", element.getUnitPrice()); 25578 } 25579 if (element.hasFactorElement()) { 25580 composeDecimalCore("factor", element.getFactorElement(), false); 25581 composeDecimalExtras("factor", element.getFactorElement(), false); 25582 } 25583 if (element.hasNet()) { 25584 composeMoney("net", element.getNet()); 25585 } 25586 if (element.hasUdi()) { 25587 openArray("udi"); 25588 for (Reference e : element.getUdi()) 25589 composeReference(null, e); 25590 closeArray(); 25591 }; 25592 } 25593 25594 protected void composeClaimResponse(String name, ClaimResponse element) throws IOException { 25595 if (element != null) { 25596 prop("resourceType", name); 25597 composeClaimResponseInner(element); 25598 } 25599 } 25600 25601 protected void composeClaimResponseInner(ClaimResponse element) throws IOException { 25602 composeDomainResourceElements(element); 25603 if (element.hasIdentifier()) { 25604 openArray("identifier"); 25605 for (Identifier e : element.getIdentifier()) 25606 composeIdentifier(null, e); 25607 closeArray(); 25608 }; 25609 if (element.hasStatusElement()) { 25610 composeEnumerationCore("status", element.getStatusElement(), new ClaimResponse.ClaimResponseStatusEnumFactory(), false); 25611 composeEnumerationExtras("status", element.getStatusElement(), new ClaimResponse.ClaimResponseStatusEnumFactory(), false); 25612 } 25613 if (element.hasPatient()) { 25614 composeReference("patient", element.getPatient()); 25615 } 25616 if (element.hasCreatedElement()) { 25617 composeDateTimeCore("created", element.getCreatedElement(), false); 25618 composeDateTimeExtras("created", element.getCreatedElement(), false); 25619 } 25620 if (element.hasInsurer()) { 25621 composeReference("insurer", element.getInsurer()); 25622 } 25623 if (element.hasRequestProvider()) { 25624 composeReference("requestProvider", element.getRequestProvider()); 25625 } 25626 if (element.hasRequestOrganization()) { 25627 composeReference("requestOrganization", element.getRequestOrganization()); 25628 } 25629 if (element.hasRequest()) { 25630 composeReference("request", element.getRequest()); 25631 } 25632 if (element.hasOutcome()) { 25633 composeCodeableConcept("outcome", element.getOutcome()); 25634 } 25635 if (element.hasDispositionElement()) { 25636 composeStringCore("disposition", element.getDispositionElement(), false); 25637 composeStringExtras("disposition", element.getDispositionElement(), false); 25638 } 25639 if (element.hasPayeeType()) { 25640 composeCodeableConcept("payeeType", element.getPayeeType()); 25641 } 25642 if (element.hasItem()) { 25643 openArray("item"); 25644 for (ClaimResponse.ItemComponent e : element.getItem()) 25645 composeClaimResponseItemComponent(null, e); 25646 closeArray(); 25647 }; 25648 if (element.hasAddItem()) { 25649 openArray("addItem"); 25650 for (ClaimResponse.AddedItemComponent e : element.getAddItem()) 25651 composeClaimResponseAddedItemComponent(null, e); 25652 closeArray(); 25653 }; 25654 if (element.hasError()) { 25655 openArray("error"); 25656 for (ClaimResponse.ErrorComponent e : element.getError()) 25657 composeClaimResponseErrorComponent(null, e); 25658 closeArray(); 25659 }; 25660 if (element.hasTotalCost()) { 25661 composeMoney("totalCost", element.getTotalCost()); 25662 } 25663 if (element.hasUnallocDeductable()) { 25664 composeMoney("unallocDeductable", element.getUnallocDeductable()); 25665 } 25666 if (element.hasTotalBenefit()) { 25667 composeMoney("totalBenefit", element.getTotalBenefit()); 25668 } 25669 if (element.hasPayment()) { 25670 composeClaimResponsePaymentComponent("payment", element.getPayment()); 25671 } 25672 if (element.hasReserved()) { 25673 composeCoding("reserved", element.getReserved()); 25674 } 25675 if (element.hasForm()) { 25676 composeCodeableConcept("form", element.getForm()); 25677 } 25678 if (element.hasProcessNote()) { 25679 openArray("processNote"); 25680 for (ClaimResponse.NoteComponent e : element.getProcessNote()) 25681 composeClaimResponseNoteComponent(null, e); 25682 closeArray(); 25683 }; 25684 if (element.hasCommunicationRequest()) { 25685 openArray("communicationRequest"); 25686 for (Reference e : element.getCommunicationRequest()) 25687 composeReference(null, e); 25688 closeArray(); 25689 }; 25690 if (element.hasInsurance()) { 25691 openArray("insurance"); 25692 for (ClaimResponse.InsuranceComponent e : element.getInsurance()) 25693 composeClaimResponseInsuranceComponent(null, e); 25694 closeArray(); 25695 }; 25696 } 25697 25698 protected void composeClaimResponseItemComponent(String name, ClaimResponse.ItemComponent element) throws IOException { 25699 if (element != null) { 25700 open(name); 25701 composeClaimResponseItemComponentInner(element); 25702 close(); 25703 } 25704 } 25705 25706 protected void composeClaimResponseItemComponentInner(ClaimResponse.ItemComponent element) throws IOException { 25707 composeBackbone(element); 25708 if (element.hasSequenceLinkIdElement()) { 25709 composePositiveIntCore("sequenceLinkId", element.getSequenceLinkIdElement(), false); 25710 composePositiveIntExtras("sequenceLinkId", element.getSequenceLinkIdElement(), false); 25711 } 25712 if (element.hasNoteNumber()) { 25713 openArray("noteNumber"); 25714 for (PositiveIntType e : element.getNoteNumber()) 25715 composePositiveIntCore(null, e, true); 25716 closeArray(); 25717 if (anyHasExtras(element.getNoteNumber())) { 25718 openArray("_noteNumber"); 25719 for (PositiveIntType e : element.getNoteNumber()) 25720 composePositiveIntExtras(null, e, true); 25721 closeArray(); 25722 } 25723 }; 25724 if (element.hasAdjudication()) { 25725 openArray("adjudication"); 25726 for (ClaimResponse.AdjudicationComponent e : element.getAdjudication()) 25727 composeClaimResponseAdjudicationComponent(null, e); 25728 closeArray(); 25729 }; 25730 if (element.hasDetail()) { 25731 openArray("detail"); 25732 for (ClaimResponse.ItemDetailComponent e : element.getDetail()) 25733 composeClaimResponseItemDetailComponent(null, e); 25734 closeArray(); 25735 }; 25736 } 25737 25738 protected void composeClaimResponseAdjudicationComponent(String name, ClaimResponse.AdjudicationComponent element) throws IOException { 25739 if (element != null) { 25740 open(name); 25741 composeClaimResponseAdjudicationComponentInner(element); 25742 close(); 25743 } 25744 } 25745 25746 protected void composeClaimResponseAdjudicationComponentInner(ClaimResponse.AdjudicationComponent element) throws IOException { 25747 composeBackbone(element); 25748 if (element.hasCategory()) { 25749 composeCodeableConcept("category", element.getCategory()); 25750 } 25751 if (element.hasReason()) { 25752 composeCodeableConcept("reason", element.getReason()); 25753 } 25754 if (element.hasAmount()) { 25755 composeMoney("amount", element.getAmount()); 25756 } 25757 if (element.hasValueElement()) { 25758 composeDecimalCore("value", element.getValueElement(), false); 25759 composeDecimalExtras("value", element.getValueElement(), false); 25760 } 25761 } 25762 25763 protected void composeClaimResponseItemDetailComponent(String name, ClaimResponse.ItemDetailComponent element) throws IOException { 25764 if (element != null) { 25765 open(name); 25766 composeClaimResponseItemDetailComponentInner(element); 25767 close(); 25768 } 25769 } 25770 25771 protected void composeClaimResponseItemDetailComponentInner(ClaimResponse.ItemDetailComponent element) throws IOException { 25772 composeBackbone(element); 25773 if (element.hasSequenceLinkIdElement()) { 25774 composePositiveIntCore("sequenceLinkId", element.getSequenceLinkIdElement(), false); 25775 composePositiveIntExtras("sequenceLinkId", element.getSequenceLinkIdElement(), false); 25776 } 25777 if (element.hasNoteNumber()) { 25778 openArray("noteNumber"); 25779 for (PositiveIntType e : element.getNoteNumber()) 25780 composePositiveIntCore(null, e, true); 25781 closeArray(); 25782 if (anyHasExtras(element.getNoteNumber())) { 25783 openArray("_noteNumber"); 25784 for (PositiveIntType e : element.getNoteNumber()) 25785 composePositiveIntExtras(null, e, true); 25786 closeArray(); 25787 } 25788 }; 25789 if (element.hasAdjudication()) { 25790 openArray("adjudication"); 25791 for (ClaimResponse.AdjudicationComponent e : element.getAdjudication()) 25792 composeClaimResponseAdjudicationComponent(null, e); 25793 closeArray(); 25794 }; 25795 if (element.hasSubDetail()) { 25796 openArray("subDetail"); 25797 for (ClaimResponse.SubDetailComponent e : element.getSubDetail()) 25798 composeClaimResponseSubDetailComponent(null, e); 25799 closeArray(); 25800 }; 25801 } 25802 25803 protected void composeClaimResponseSubDetailComponent(String name, ClaimResponse.SubDetailComponent element) throws IOException { 25804 if (element != null) { 25805 open(name); 25806 composeClaimResponseSubDetailComponentInner(element); 25807 close(); 25808 } 25809 } 25810 25811 protected void composeClaimResponseSubDetailComponentInner(ClaimResponse.SubDetailComponent element) throws IOException { 25812 composeBackbone(element); 25813 if (element.hasSequenceLinkIdElement()) { 25814 composePositiveIntCore("sequenceLinkId", element.getSequenceLinkIdElement(), false); 25815 composePositiveIntExtras("sequenceLinkId", element.getSequenceLinkIdElement(), false); 25816 } 25817 if (element.hasNoteNumber()) { 25818 openArray("noteNumber"); 25819 for (PositiveIntType e : element.getNoteNumber()) 25820 composePositiveIntCore(null, e, true); 25821 closeArray(); 25822 if (anyHasExtras(element.getNoteNumber())) { 25823 openArray("_noteNumber"); 25824 for (PositiveIntType e : element.getNoteNumber()) 25825 composePositiveIntExtras(null, e, true); 25826 closeArray(); 25827 } 25828 }; 25829 if (element.hasAdjudication()) { 25830 openArray("adjudication"); 25831 for (ClaimResponse.AdjudicationComponent e : element.getAdjudication()) 25832 composeClaimResponseAdjudicationComponent(null, e); 25833 closeArray(); 25834 }; 25835 } 25836 25837 protected void composeClaimResponseAddedItemComponent(String name, ClaimResponse.AddedItemComponent element) throws IOException { 25838 if (element != null) { 25839 open(name); 25840 composeClaimResponseAddedItemComponentInner(element); 25841 close(); 25842 } 25843 } 25844 25845 protected void composeClaimResponseAddedItemComponentInner(ClaimResponse.AddedItemComponent element) throws IOException { 25846 composeBackbone(element); 25847 if (element.hasSequenceLinkId()) { 25848 openArray("sequenceLinkId"); 25849 for (PositiveIntType e : element.getSequenceLinkId()) 25850 composePositiveIntCore(null, e, true); 25851 closeArray(); 25852 if (anyHasExtras(element.getSequenceLinkId())) { 25853 openArray("_sequenceLinkId"); 25854 for (PositiveIntType e : element.getSequenceLinkId()) 25855 composePositiveIntExtras(null, e, true); 25856 closeArray(); 25857 } 25858 }; 25859 if (element.hasRevenue()) { 25860 composeCodeableConcept("revenue", element.getRevenue()); 25861 } 25862 if (element.hasCategory()) { 25863 composeCodeableConcept("category", element.getCategory()); 25864 } 25865 if (element.hasService()) { 25866 composeCodeableConcept("service", element.getService()); 25867 } 25868 if (element.hasModifier()) { 25869 openArray("modifier"); 25870 for (CodeableConcept e : element.getModifier()) 25871 composeCodeableConcept(null, e); 25872 closeArray(); 25873 }; 25874 if (element.hasFee()) { 25875 composeMoney("fee", element.getFee()); 25876 } 25877 if (element.hasNoteNumber()) { 25878 openArray("noteNumber"); 25879 for (PositiveIntType e : element.getNoteNumber()) 25880 composePositiveIntCore(null, e, true); 25881 closeArray(); 25882 if (anyHasExtras(element.getNoteNumber())) { 25883 openArray("_noteNumber"); 25884 for (PositiveIntType e : element.getNoteNumber()) 25885 composePositiveIntExtras(null, e, true); 25886 closeArray(); 25887 } 25888 }; 25889 if (element.hasAdjudication()) { 25890 openArray("adjudication"); 25891 for (ClaimResponse.AdjudicationComponent e : element.getAdjudication()) 25892 composeClaimResponseAdjudicationComponent(null, e); 25893 closeArray(); 25894 }; 25895 if (element.hasDetail()) { 25896 openArray("detail"); 25897 for (ClaimResponse.AddedItemsDetailComponent e : element.getDetail()) 25898 composeClaimResponseAddedItemsDetailComponent(null, e); 25899 closeArray(); 25900 }; 25901 } 25902 25903 protected void composeClaimResponseAddedItemsDetailComponent(String name, ClaimResponse.AddedItemsDetailComponent element) throws IOException { 25904 if (element != null) { 25905 open(name); 25906 composeClaimResponseAddedItemsDetailComponentInner(element); 25907 close(); 25908 } 25909 } 25910 25911 protected void composeClaimResponseAddedItemsDetailComponentInner(ClaimResponse.AddedItemsDetailComponent element) throws IOException { 25912 composeBackbone(element); 25913 if (element.hasRevenue()) { 25914 composeCodeableConcept("revenue", element.getRevenue()); 25915 } 25916 if (element.hasCategory()) { 25917 composeCodeableConcept("category", element.getCategory()); 25918 } 25919 if (element.hasService()) { 25920 composeCodeableConcept("service", element.getService()); 25921 } 25922 if (element.hasModifier()) { 25923 openArray("modifier"); 25924 for (CodeableConcept e : element.getModifier()) 25925 composeCodeableConcept(null, e); 25926 closeArray(); 25927 }; 25928 if (element.hasFee()) { 25929 composeMoney("fee", element.getFee()); 25930 } 25931 if (element.hasNoteNumber()) { 25932 openArray("noteNumber"); 25933 for (PositiveIntType e : element.getNoteNumber()) 25934 composePositiveIntCore(null, e, true); 25935 closeArray(); 25936 if (anyHasExtras(element.getNoteNumber())) { 25937 openArray("_noteNumber"); 25938 for (PositiveIntType e : element.getNoteNumber()) 25939 composePositiveIntExtras(null, e, true); 25940 closeArray(); 25941 } 25942 }; 25943 if (element.hasAdjudication()) { 25944 openArray("adjudication"); 25945 for (ClaimResponse.AdjudicationComponent e : element.getAdjudication()) 25946 composeClaimResponseAdjudicationComponent(null, e); 25947 closeArray(); 25948 }; 25949 } 25950 25951 protected void composeClaimResponseErrorComponent(String name, ClaimResponse.ErrorComponent element) throws IOException { 25952 if (element != null) { 25953 open(name); 25954 composeClaimResponseErrorComponentInner(element); 25955 close(); 25956 } 25957 } 25958 25959 protected void composeClaimResponseErrorComponentInner(ClaimResponse.ErrorComponent element) throws IOException { 25960 composeBackbone(element); 25961 if (element.hasSequenceLinkIdElement()) { 25962 composePositiveIntCore("sequenceLinkId", element.getSequenceLinkIdElement(), false); 25963 composePositiveIntExtras("sequenceLinkId", element.getSequenceLinkIdElement(), false); 25964 } 25965 if (element.hasDetailSequenceLinkIdElement()) { 25966 composePositiveIntCore("detailSequenceLinkId", element.getDetailSequenceLinkIdElement(), false); 25967 composePositiveIntExtras("detailSequenceLinkId", element.getDetailSequenceLinkIdElement(), false); 25968 } 25969 if (element.hasSubdetailSequenceLinkIdElement()) { 25970 composePositiveIntCore("subdetailSequenceLinkId", element.getSubdetailSequenceLinkIdElement(), false); 25971 composePositiveIntExtras("subdetailSequenceLinkId", element.getSubdetailSequenceLinkIdElement(), false); 25972 } 25973 if (element.hasCode()) { 25974 composeCodeableConcept("code", element.getCode()); 25975 } 25976 } 25977 25978 protected void composeClaimResponsePaymentComponent(String name, ClaimResponse.PaymentComponent element) throws IOException { 25979 if (element != null) { 25980 open(name); 25981 composeClaimResponsePaymentComponentInner(element); 25982 close(); 25983 } 25984 } 25985 25986 protected void composeClaimResponsePaymentComponentInner(ClaimResponse.PaymentComponent element) throws IOException { 25987 composeBackbone(element); 25988 if (element.hasType()) { 25989 composeCodeableConcept("type", element.getType()); 25990 } 25991 if (element.hasAdjustment()) { 25992 composeMoney("adjustment", element.getAdjustment()); 25993 } 25994 if (element.hasAdjustmentReason()) { 25995 composeCodeableConcept("adjustmentReason", element.getAdjustmentReason()); 25996 } 25997 if (element.hasDateElement()) { 25998 composeDateCore("date", element.getDateElement(), false); 25999 composeDateExtras("date", element.getDateElement(), false); 26000 } 26001 if (element.hasAmount()) { 26002 composeMoney("amount", element.getAmount()); 26003 } 26004 if (element.hasIdentifier()) { 26005 composeIdentifier("identifier", element.getIdentifier()); 26006 } 26007 } 26008 26009 protected void composeClaimResponseNoteComponent(String name, ClaimResponse.NoteComponent element) throws IOException { 26010 if (element != null) { 26011 open(name); 26012 composeClaimResponseNoteComponentInner(element); 26013 close(); 26014 } 26015 } 26016 26017 protected void composeClaimResponseNoteComponentInner(ClaimResponse.NoteComponent element) throws IOException { 26018 composeBackbone(element); 26019 if (element.hasNumberElement()) { 26020 composePositiveIntCore("number", element.getNumberElement(), false); 26021 composePositiveIntExtras("number", element.getNumberElement(), false); 26022 } 26023 if (element.hasType()) { 26024 composeCodeableConcept("type", element.getType()); 26025 } 26026 if (element.hasTextElement()) { 26027 composeStringCore("text", element.getTextElement(), false); 26028 composeStringExtras("text", element.getTextElement(), false); 26029 } 26030 if (element.hasLanguage()) { 26031 composeCodeableConcept("language", element.getLanguage()); 26032 } 26033 } 26034 26035 protected void composeClaimResponseInsuranceComponent(String name, ClaimResponse.InsuranceComponent element) throws IOException { 26036 if (element != null) { 26037 open(name); 26038 composeClaimResponseInsuranceComponentInner(element); 26039 close(); 26040 } 26041 } 26042 26043 protected void composeClaimResponseInsuranceComponentInner(ClaimResponse.InsuranceComponent element) throws IOException { 26044 composeBackbone(element); 26045 if (element.hasSequenceElement()) { 26046 composePositiveIntCore("sequence", element.getSequenceElement(), false); 26047 composePositiveIntExtras("sequence", element.getSequenceElement(), false); 26048 } 26049 if (element.hasFocalElement()) { 26050 composeBooleanCore("focal", element.getFocalElement(), false); 26051 composeBooleanExtras("focal", element.getFocalElement(), false); 26052 } 26053 if (element.hasCoverage()) { 26054 composeReference("coverage", element.getCoverage()); 26055 } 26056 if (element.hasBusinessArrangementElement()) { 26057 composeStringCore("businessArrangement", element.getBusinessArrangementElement(), false); 26058 composeStringExtras("businessArrangement", element.getBusinessArrangementElement(), false); 26059 } 26060 if (element.hasPreAuthRef()) { 26061 openArray("preAuthRef"); 26062 for (StringType e : element.getPreAuthRef()) 26063 composeStringCore(null, e, true); 26064 closeArray(); 26065 if (anyHasExtras(element.getPreAuthRef())) { 26066 openArray("_preAuthRef"); 26067 for (StringType e : element.getPreAuthRef()) 26068 composeStringExtras(null, e, true); 26069 closeArray(); 26070 } 26071 }; 26072 if (element.hasClaimResponse()) { 26073 composeReference("claimResponse", element.getClaimResponse()); 26074 } 26075 } 26076 26077 protected void composeClinicalImpression(String name, ClinicalImpression element) throws IOException { 26078 if (element != null) { 26079 prop("resourceType", name); 26080 composeClinicalImpressionInner(element); 26081 } 26082 } 26083 26084 protected void composeClinicalImpressionInner(ClinicalImpression element) throws IOException { 26085 composeDomainResourceElements(element); 26086 if (element.hasIdentifier()) { 26087 openArray("identifier"); 26088 for (Identifier e : element.getIdentifier()) 26089 composeIdentifier(null, e); 26090 closeArray(); 26091 }; 26092 if (element.hasStatusElement()) { 26093 composeEnumerationCore("status", element.getStatusElement(), new ClinicalImpression.ClinicalImpressionStatusEnumFactory(), false); 26094 composeEnumerationExtras("status", element.getStatusElement(), new ClinicalImpression.ClinicalImpressionStatusEnumFactory(), false); 26095 } 26096 if (element.hasCode()) { 26097 composeCodeableConcept("code", element.getCode()); 26098 } 26099 if (element.hasDescriptionElement()) { 26100 composeStringCore("description", element.getDescriptionElement(), false); 26101 composeStringExtras("description", element.getDescriptionElement(), false); 26102 } 26103 if (element.hasSubject()) { 26104 composeReference("subject", element.getSubject()); 26105 } 26106 if (element.hasContext()) { 26107 composeReference("context", element.getContext()); 26108 } 26109 if (element.hasEffective()) { 26110 composeType("effective", element.getEffective()); 26111 } 26112 if (element.hasDateElement()) { 26113 composeDateTimeCore("date", element.getDateElement(), false); 26114 composeDateTimeExtras("date", element.getDateElement(), false); 26115 } 26116 if (element.hasAssessor()) { 26117 composeReference("assessor", element.getAssessor()); 26118 } 26119 if (element.hasPrevious()) { 26120 composeReference("previous", element.getPrevious()); 26121 } 26122 if (element.hasProblem()) { 26123 openArray("problem"); 26124 for (Reference e : element.getProblem()) 26125 composeReference(null, e); 26126 closeArray(); 26127 }; 26128 if (element.hasInvestigation()) { 26129 openArray("investigation"); 26130 for (ClinicalImpression.ClinicalImpressionInvestigationComponent e : element.getInvestigation()) 26131 composeClinicalImpressionClinicalImpressionInvestigationComponent(null, e); 26132 closeArray(); 26133 }; 26134 if (element.hasProtocol()) { 26135 openArray("protocol"); 26136 for (UriType e : element.getProtocol()) 26137 composeUriCore(null, e, true); 26138 closeArray(); 26139 if (anyHasExtras(element.getProtocol())) { 26140 openArray("_protocol"); 26141 for (UriType e : element.getProtocol()) 26142 composeUriExtras(null, e, true); 26143 closeArray(); 26144 } 26145 }; 26146 if (element.hasSummaryElement()) { 26147 composeStringCore("summary", element.getSummaryElement(), false); 26148 composeStringExtras("summary", element.getSummaryElement(), false); 26149 } 26150 if (element.hasFinding()) { 26151 openArray("finding"); 26152 for (ClinicalImpression.ClinicalImpressionFindingComponent e : element.getFinding()) 26153 composeClinicalImpressionClinicalImpressionFindingComponent(null, e); 26154 closeArray(); 26155 }; 26156 if (element.hasPrognosisCodeableConcept()) { 26157 openArray("prognosisCodeableConcept"); 26158 for (CodeableConcept e : element.getPrognosisCodeableConcept()) 26159 composeCodeableConcept(null, e); 26160 closeArray(); 26161 }; 26162 if (element.hasPrognosisReference()) { 26163 openArray("prognosisReference"); 26164 for (Reference e : element.getPrognosisReference()) 26165 composeReference(null, e); 26166 closeArray(); 26167 }; 26168 if (element.hasAction()) { 26169 openArray("action"); 26170 for (Reference e : element.getAction()) 26171 composeReference(null, e); 26172 closeArray(); 26173 }; 26174 if (element.hasNote()) { 26175 openArray("note"); 26176 for (Annotation e : element.getNote()) 26177 composeAnnotation(null, e); 26178 closeArray(); 26179 }; 26180 } 26181 26182 protected void composeClinicalImpressionClinicalImpressionInvestigationComponent(String name, ClinicalImpression.ClinicalImpressionInvestigationComponent element) throws IOException { 26183 if (element != null) { 26184 open(name); 26185 composeClinicalImpressionClinicalImpressionInvestigationComponentInner(element); 26186 close(); 26187 } 26188 } 26189 26190 protected void composeClinicalImpressionClinicalImpressionInvestigationComponentInner(ClinicalImpression.ClinicalImpressionInvestigationComponent element) throws IOException { 26191 composeBackbone(element); 26192 if (element.hasCode()) { 26193 composeCodeableConcept("code", element.getCode()); 26194 } 26195 if (element.hasItem()) { 26196 openArray("item"); 26197 for (Reference e : element.getItem()) 26198 composeReference(null, e); 26199 closeArray(); 26200 }; 26201 } 26202 26203 protected void composeClinicalImpressionClinicalImpressionFindingComponent(String name, ClinicalImpression.ClinicalImpressionFindingComponent element) throws IOException { 26204 if (element != null) { 26205 open(name); 26206 composeClinicalImpressionClinicalImpressionFindingComponentInner(element); 26207 close(); 26208 } 26209 } 26210 26211 protected void composeClinicalImpressionClinicalImpressionFindingComponentInner(ClinicalImpression.ClinicalImpressionFindingComponent element) throws IOException { 26212 composeBackbone(element); 26213 if (element.hasItem()) { 26214 composeType("item", element.getItem()); 26215 } 26216 if (element.hasBasisElement()) { 26217 composeStringCore("basis", element.getBasisElement(), false); 26218 composeStringExtras("basis", element.getBasisElement(), false); 26219 } 26220 } 26221 26222 protected void composeCodeSystem(String name, CodeSystem element) throws IOException { 26223 if (element != null) { 26224 prop("resourceType", name); 26225 composeCodeSystemInner(element); 26226 } 26227 } 26228 26229 protected void composeCodeSystemInner(CodeSystem element) throws IOException { 26230 composeDomainResourceElements(element); 26231 if (element.hasUrlElement()) { 26232 composeUriCore("url", element.getUrlElement(), false); 26233 composeUriExtras("url", element.getUrlElement(), false); 26234 } 26235 if (element.hasIdentifier()) { 26236 composeIdentifier("identifier", element.getIdentifier()); 26237 } 26238 if (element.hasVersionElement()) { 26239 composeStringCore("version", element.getVersionElement(), false); 26240 composeStringExtras("version", element.getVersionElement(), false); 26241 } 26242 if (element.hasNameElement()) { 26243 composeStringCore("name", element.getNameElement(), false); 26244 composeStringExtras("name", element.getNameElement(), false); 26245 } 26246 if (element.hasTitleElement()) { 26247 composeStringCore("title", element.getTitleElement(), false); 26248 composeStringExtras("title", element.getTitleElement(), false); 26249 } 26250 if (element.hasStatusElement()) { 26251 composeEnumerationCore("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 26252 composeEnumerationExtras("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 26253 } 26254 if (element.hasExperimentalElement()) { 26255 composeBooleanCore("experimental", element.getExperimentalElement(), false); 26256 composeBooleanExtras("experimental", element.getExperimentalElement(), false); 26257 } 26258 if (element.hasDateElement()) { 26259 composeDateTimeCore("date", element.getDateElement(), false); 26260 composeDateTimeExtras("date", element.getDateElement(), false); 26261 } 26262 if (element.hasPublisherElement()) { 26263 composeStringCore("publisher", element.getPublisherElement(), false); 26264 composeStringExtras("publisher", element.getPublisherElement(), false); 26265 } 26266 if (element.hasContact()) { 26267 openArray("contact"); 26268 for (ContactDetail e : element.getContact()) 26269 composeContactDetail(null, e); 26270 closeArray(); 26271 }; 26272 if (element.hasDescriptionElement()) { 26273 composeMarkdownCore("description", element.getDescriptionElement(), false); 26274 composeMarkdownExtras("description", element.getDescriptionElement(), false); 26275 } 26276 if (element.hasUseContext()) { 26277 openArray("useContext"); 26278 for (UsageContext e : element.getUseContext()) 26279 composeUsageContext(null, e); 26280 closeArray(); 26281 }; 26282 if (element.hasJurisdiction()) { 26283 openArray("jurisdiction"); 26284 for (CodeableConcept e : element.getJurisdiction()) 26285 composeCodeableConcept(null, e); 26286 closeArray(); 26287 }; 26288 if (element.hasPurposeElement()) { 26289 composeMarkdownCore("purpose", element.getPurposeElement(), false); 26290 composeMarkdownExtras("purpose", element.getPurposeElement(), false); 26291 } 26292 if (element.hasCopyrightElement()) { 26293 composeMarkdownCore("copyright", element.getCopyrightElement(), false); 26294 composeMarkdownExtras("copyright", element.getCopyrightElement(), false); 26295 } 26296 if (element.hasCaseSensitiveElement()) { 26297 composeBooleanCore("caseSensitive", element.getCaseSensitiveElement(), false); 26298 composeBooleanExtras("caseSensitive", element.getCaseSensitiveElement(), false); 26299 } 26300 if (element.hasValueSetElement()) { 26301 composeUriCore("valueSet", element.getValueSetElement(), false); 26302 composeUriExtras("valueSet", element.getValueSetElement(), false); 26303 } 26304 if (element.hasHierarchyMeaningElement()) { 26305 composeEnumerationCore("hierarchyMeaning", element.getHierarchyMeaningElement(), new CodeSystem.CodeSystemHierarchyMeaningEnumFactory(), false); 26306 composeEnumerationExtras("hierarchyMeaning", element.getHierarchyMeaningElement(), new CodeSystem.CodeSystemHierarchyMeaningEnumFactory(), false); 26307 } 26308 if (element.hasCompositionalElement()) { 26309 composeBooleanCore("compositional", element.getCompositionalElement(), false); 26310 composeBooleanExtras("compositional", element.getCompositionalElement(), false); 26311 } 26312 if (element.hasVersionNeededElement()) { 26313 composeBooleanCore("versionNeeded", element.getVersionNeededElement(), false); 26314 composeBooleanExtras("versionNeeded", element.getVersionNeededElement(), false); 26315 } 26316 if (element.hasContentElement()) { 26317 composeEnumerationCore("content", element.getContentElement(), new CodeSystem.CodeSystemContentModeEnumFactory(), false); 26318 composeEnumerationExtras("content", element.getContentElement(), new CodeSystem.CodeSystemContentModeEnumFactory(), false); 26319 } 26320 if (element.hasCountElement()) { 26321 composeUnsignedIntCore("count", element.getCountElement(), false); 26322 composeUnsignedIntExtras("count", element.getCountElement(), false); 26323 } 26324 if (element.hasFilter()) { 26325 openArray("filter"); 26326 for (CodeSystem.CodeSystemFilterComponent e : element.getFilter()) 26327 composeCodeSystemCodeSystemFilterComponent(null, e); 26328 closeArray(); 26329 }; 26330 if (element.hasProperty()) { 26331 openArray("property"); 26332 for (CodeSystem.PropertyComponent e : element.getProperty()) 26333 composeCodeSystemPropertyComponent(null, e); 26334 closeArray(); 26335 }; 26336 if (element.hasConcept()) { 26337 openArray("concept"); 26338 for (CodeSystem.ConceptDefinitionComponent e : element.getConcept()) 26339 composeCodeSystemConceptDefinitionComponent(null, e); 26340 closeArray(); 26341 }; 26342 } 26343 26344 protected void composeCodeSystemCodeSystemFilterComponent(String name, CodeSystem.CodeSystemFilterComponent element) throws IOException { 26345 if (element != null) { 26346 open(name); 26347 composeCodeSystemCodeSystemFilterComponentInner(element); 26348 close(); 26349 } 26350 } 26351 26352 protected void composeCodeSystemCodeSystemFilterComponentInner(CodeSystem.CodeSystemFilterComponent element) throws IOException { 26353 composeBackbone(element); 26354 if (element.hasCodeElement()) { 26355 composeCodeCore("code", element.getCodeElement(), false); 26356 composeCodeExtras("code", element.getCodeElement(), false); 26357 } 26358 if (element.hasDescriptionElement()) { 26359 composeStringCore("description", element.getDescriptionElement(), false); 26360 composeStringExtras("description", element.getDescriptionElement(), false); 26361 } 26362 if (element.hasOperator()) { 26363 openArray("operator"); 26364 for (Enumeration<CodeSystem.FilterOperator> e : element.getOperator()) 26365 composeEnumerationCore(null, e, new CodeSystem.FilterOperatorEnumFactory(), true); 26366 closeArray(); 26367 if (anyHasExtras(element.getOperator())) { 26368 openArray("_operator"); 26369 for (Enumeration<CodeSystem.FilterOperator> e : element.getOperator()) 26370 composeEnumerationExtras(null, e, new CodeSystem.FilterOperatorEnumFactory(), true); 26371 closeArray(); 26372 } 26373 }; 26374 if (element.hasValueElement()) { 26375 composeStringCore("value", element.getValueElement(), false); 26376 composeStringExtras("value", element.getValueElement(), false); 26377 } 26378 } 26379 26380 protected void composeCodeSystemPropertyComponent(String name, CodeSystem.PropertyComponent element) throws IOException { 26381 if (element != null) { 26382 open(name); 26383 composeCodeSystemPropertyComponentInner(element); 26384 close(); 26385 } 26386 } 26387 26388 protected void composeCodeSystemPropertyComponentInner(CodeSystem.PropertyComponent element) throws IOException { 26389 composeBackbone(element); 26390 if (element.hasCodeElement()) { 26391 composeCodeCore("code", element.getCodeElement(), false); 26392 composeCodeExtras("code", element.getCodeElement(), false); 26393 } 26394 if (element.hasUriElement()) { 26395 composeUriCore("uri", element.getUriElement(), false); 26396 composeUriExtras("uri", element.getUriElement(), false); 26397 } 26398 if (element.hasDescriptionElement()) { 26399 composeStringCore("description", element.getDescriptionElement(), false); 26400 composeStringExtras("description", element.getDescriptionElement(), false); 26401 } 26402 if (element.hasTypeElement()) { 26403 composeEnumerationCore("type", element.getTypeElement(), new CodeSystem.PropertyTypeEnumFactory(), false); 26404 composeEnumerationExtras("type", element.getTypeElement(), new CodeSystem.PropertyTypeEnumFactory(), false); 26405 } 26406 } 26407 26408 protected void composeCodeSystemConceptDefinitionComponent(String name, CodeSystem.ConceptDefinitionComponent element) throws IOException { 26409 if (element != null) { 26410 open(name); 26411 composeCodeSystemConceptDefinitionComponentInner(element); 26412 close(); 26413 } 26414 } 26415 26416 protected void composeCodeSystemConceptDefinitionComponentInner(CodeSystem.ConceptDefinitionComponent element) throws IOException { 26417 composeBackbone(element); 26418 if (element.hasCodeElement()) { 26419 composeCodeCore("code", element.getCodeElement(), false); 26420 composeCodeExtras("code", element.getCodeElement(), false); 26421 } 26422 if (element.hasDisplayElement()) { 26423 composeStringCore("display", element.getDisplayElement(), false); 26424 composeStringExtras("display", element.getDisplayElement(), false); 26425 } 26426 if (element.hasDefinitionElement()) { 26427 composeStringCore("definition", element.getDefinitionElement(), false); 26428 composeStringExtras("definition", element.getDefinitionElement(), false); 26429 } 26430 if (element.hasDesignation()) { 26431 openArray("designation"); 26432 for (CodeSystem.ConceptDefinitionDesignationComponent e : element.getDesignation()) 26433 composeCodeSystemConceptDefinitionDesignationComponent(null, e); 26434 closeArray(); 26435 }; 26436 if (element.hasProperty()) { 26437 openArray("property"); 26438 for (CodeSystem.ConceptPropertyComponent e : element.getProperty()) 26439 composeCodeSystemConceptPropertyComponent(null, e); 26440 closeArray(); 26441 }; 26442 if (element.hasConcept()) { 26443 openArray("concept"); 26444 for (CodeSystem.ConceptDefinitionComponent e : element.getConcept()) 26445 composeCodeSystemConceptDefinitionComponent(null, e); 26446 closeArray(); 26447 }; 26448 } 26449 26450 protected void composeCodeSystemConceptDefinitionDesignationComponent(String name, CodeSystem.ConceptDefinitionDesignationComponent element) throws IOException { 26451 if (element != null) { 26452 open(name); 26453 composeCodeSystemConceptDefinitionDesignationComponentInner(element); 26454 close(); 26455 } 26456 } 26457 26458 protected void composeCodeSystemConceptDefinitionDesignationComponentInner(CodeSystem.ConceptDefinitionDesignationComponent element) throws IOException { 26459 composeBackbone(element); 26460 if (element.hasLanguageElement()) { 26461 composeCodeCore("language", element.getLanguageElement(), false); 26462 composeCodeExtras("language", element.getLanguageElement(), false); 26463 } 26464 if (element.hasUse()) { 26465 composeCoding("use", element.getUse()); 26466 } 26467 if (element.hasValueElement()) { 26468 composeStringCore("value", element.getValueElement(), false); 26469 composeStringExtras("value", element.getValueElement(), false); 26470 } 26471 } 26472 26473 protected void composeCodeSystemConceptPropertyComponent(String name, CodeSystem.ConceptPropertyComponent element) throws IOException { 26474 if (element != null) { 26475 open(name); 26476 composeCodeSystemConceptPropertyComponentInner(element); 26477 close(); 26478 } 26479 } 26480 26481 protected void composeCodeSystemConceptPropertyComponentInner(CodeSystem.ConceptPropertyComponent element) throws IOException { 26482 composeBackbone(element); 26483 if (element.hasCodeElement()) { 26484 composeCodeCore("code", element.getCodeElement(), false); 26485 composeCodeExtras("code", element.getCodeElement(), false); 26486 } 26487 if (element.hasValue()) { 26488 composeType("value", element.getValue()); 26489 } 26490 } 26491 26492 protected void composeCommunication(String name, Communication element) throws IOException { 26493 if (element != null) { 26494 prop("resourceType", name); 26495 composeCommunicationInner(element); 26496 } 26497 } 26498 26499 protected void composeCommunicationInner(Communication element) throws IOException { 26500 composeDomainResourceElements(element); 26501 if (element.hasIdentifier()) { 26502 openArray("identifier"); 26503 for (Identifier e : element.getIdentifier()) 26504 composeIdentifier(null, e); 26505 closeArray(); 26506 }; 26507 if (element.hasDefinition()) { 26508 openArray("definition"); 26509 for (Reference e : element.getDefinition()) 26510 composeReference(null, e); 26511 closeArray(); 26512 }; 26513 if (element.hasBasedOn()) { 26514 openArray("basedOn"); 26515 for (Reference e : element.getBasedOn()) 26516 composeReference(null, e); 26517 closeArray(); 26518 }; 26519 if (element.hasPartOf()) { 26520 openArray("partOf"); 26521 for (Reference e : element.getPartOf()) 26522 composeReference(null, e); 26523 closeArray(); 26524 }; 26525 if (element.hasStatusElement()) { 26526 composeEnumerationCore("status", element.getStatusElement(), new Communication.CommunicationStatusEnumFactory(), false); 26527 composeEnumerationExtras("status", element.getStatusElement(), new Communication.CommunicationStatusEnumFactory(), false); 26528 } 26529 if (element.hasNotDoneElement()) { 26530 composeBooleanCore("notDone", element.getNotDoneElement(), false); 26531 composeBooleanExtras("notDone", element.getNotDoneElement(), false); 26532 } 26533 if (element.hasNotDoneReason()) { 26534 composeCodeableConcept("notDoneReason", element.getNotDoneReason()); 26535 } 26536 if (element.hasCategory()) { 26537 openArray("category"); 26538 for (CodeableConcept e : element.getCategory()) 26539 composeCodeableConcept(null, e); 26540 closeArray(); 26541 }; 26542 if (element.hasMedium()) { 26543 openArray("medium"); 26544 for (CodeableConcept e : element.getMedium()) 26545 composeCodeableConcept(null, e); 26546 closeArray(); 26547 }; 26548 if (element.hasSubject()) { 26549 composeReference("subject", element.getSubject()); 26550 } 26551 if (element.hasRecipient()) { 26552 openArray("recipient"); 26553 for (Reference e : element.getRecipient()) 26554 composeReference(null, e); 26555 closeArray(); 26556 }; 26557 if (element.hasTopic()) { 26558 openArray("topic"); 26559 for (Reference e : element.getTopic()) 26560 composeReference(null, e); 26561 closeArray(); 26562 }; 26563 if (element.hasContext()) { 26564 composeReference("context", element.getContext()); 26565 } 26566 if (element.hasSentElement()) { 26567 composeDateTimeCore("sent", element.getSentElement(), false); 26568 composeDateTimeExtras("sent", element.getSentElement(), false); 26569 } 26570 if (element.hasReceivedElement()) { 26571 composeDateTimeCore("received", element.getReceivedElement(), false); 26572 composeDateTimeExtras("received", element.getReceivedElement(), false); 26573 } 26574 if (element.hasSender()) { 26575 composeReference("sender", element.getSender()); 26576 } 26577 if (element.hasReasonCode()) { 26578 openArray("reasonCode"); 26579 for (CodeableConcept e : element.getReasonCode()) 26580 composeCodeableConcept(null, e); 26581 closeArray(); 26582 }; 26583 if (element.hasReasonReference()) { 26584 openArray("reasonReference"); 26585 for (Reference e : element.getReasonReference()) 26586 composeReference(null, e); 26587 closeArray(); 26588 }; 26589 if (element.hasPayload()) { 26590 openArray("payload"); 26591 for (Communication.CommunicationPayloadComponent e : element.getPayload()) 26592 composeCommunicationCommunicationPayloadComponent(null, e); 26593 closeArray(); 26594 }; 26595 if (element.hasNote()) { 26596 openArray("note"); 26597 for (Annotation e : element.getNote()) 26598 composeAnnotation(null, e); 26599 closeArray(); 26600 }; 26601 } 26602 26603 protected void composeCommunicationCommunicationPayloadComponent(String name, Communication.CommunicationPayloadComponent element) throws IOException { 26604 if (element != null) { 26605 open(name); 26606 composeCommunicationCommunicationPayloadComponentInner(element); 26607 close(); 26608 } 26609 } 26610 26611 protected void composeCommunicationCommunicationPayloadComponentInner(Communication.CommunicationPayloadComponent element) throws IOException { 26612 composeBackbone(element); 26613 if (element.hasContent()) { 26614 composeType("content", element.getContent()); 26615 } 26616 } 26617 26618 protected void composeCommunicationRequest(String name, CommunicationRequest element) throws IOException { 26619 if (element != null) { 26620 prop("resourceType", name); 26621 composeCommunicationRequestInner(element); 26622 } 26623 } 26624 26625 protected void composeCommunicationRequestInner(CommunicationRequest element) throws IOException { 26626 composeDomainResourceElements(element); 26627 if (element.hasIdentifier()) { 26628 openArray("identifier"); 26629 for (Identifier e : element.getIdentifier()) 26630 composeIdentifier(null, e); 26631 closeArray(); 26632 }; 26633 if (element.hasBasedOn()) { 26634 openArray("basedOn"); 26635 for (Reference e : element.getBasedOn()) 26636 composeReference(null, e); 26637 closeArray(); 26638 }; 26639 if (element.hasReplaces()) { 26640 openArray("replaces"); 26641 for (Reference e : element.getReplaces()) 26642 composeReference(null, e); 26643 closeArray(); 26644 }; 26645 if (element.hasGroupIdentifier()) { 26646 composeIdentifier("groupIdentifier", element.getGroupIdentifier()); 26647 } 26648 if (element.hasStatusElement()) { 26649 composeEnumerationCore("status", element.getStatusElement(), new CommunicationRequest.CommunicationRequestStatusEnumFactory(), false); 26650 composeEnumerationExtras("status", element.getStatusElement(), new CommunicationRequest.CommunicationRequestStatusEnumFactory(), false); 26651 } 26652 if (element.hasCategory()) { 26653 openArray("category"); 26654 for (CodeableConcept e : element.getCategory()) 26655 composeCodeableConcept(null, e); 26656 closeArray(); 26657 }; 26658 if (element.hasPriorityElement()) { 26659 composeEnumerationCore("priority", element.getPriorityElement(), new CommunicationRequest.CommunicationPriorityEnumFactory(), false); 26660 composeEnumerationExtras("priority", element.getPriorityElement(), new CommunicationRequest.CommunicationPriorityEnumFactory(), false); 26661 } 26662 if (element.hasMedium()) { 26663 openArray("medium"); 26664 for (CodeableConcept e : element.getMedium()) 26665 composeCodeableConcept(null, e); 26666 closeArray(); 26667 }; 26668 if (element.hasSubject()) { 26669 composeReference("subject", element.getSubject()); 26670 } 26671 if (element.hasRecipient()) { 26672 openArray("recipient"); 26673 for (Reference e : element.getRecipient()) 26674 composeReference(null, e); 26675 closeArray(); 26676 }; 26677 if (element.hasTopic()) { 26678 openArray("topic"); 26679 for (Reference e : element.getTopic()) 26680 composeReference(null, e); 26681 closeArray(); 26682 }; 26683 if (element.hasContext()) { 26684 composeReference("context", element.getContext()); 26685 } 26686 if (element.hasPayload()) { 26687 openArray("payload"); 26688 for (CommunicationRequest.CommunicationRequestPayloadComponent e : element.getPayload()) 26689 composeCommunicationRequestCommunicationRequestPayloadComponent(null, e); 26690 closeArray(); 26691 }; 26692 if (element.hasOccurrence()) { 26693 composeType("occurrence", element.getOccurrence()); 26694 } 26695 if (element.hasAuthoredOnElement()) { 26696 composeDateTimeCore("authoredOn", element.getAuthoredOnElement(), false); 26697 composeDateTimeExtras("authoredOn", element.getAuthoredOnElement(), false); 26698 } 26699 if (element.hasSender()) { 26700 composeReference("sender", element.getSender()); 26701 } 26702 if (element.hasRequester()) { 26703 composeCommunicationRequestCommunicationRequestRequesterComponent("requester", element.getRequester()); 26704 } 26705 if (element.hasReasonCode()) { 26706 openArray("reasonCode"); 26707 for (CodeableConcept e : element.getReasonCode()) 26708 composeCodeableConcept(null, e); 26709 closeArray(); 26710 }; 26711 if (element.hasReasonReference()) { 26712 openArray("reasonReference"); 26713 for (Reference e : element.getReasonReference()) 26714 composeReference(null, e); 26715 closeArray(); 26716 }; 26717 if (element.hasNote()) { 26718 openArray("note"); 26719 for (Annotation e : element.getNote()) 26720 composeAnnotation(null, e); 26721 closeArray(); 26722 }; 26723 } 26724 26725 protected void composeCommunicationRequestCommunicationRequestPayloadComponent(String name, CommunicationRequest.CommunicationRequestPayloadComponent element) throws IOException { 26726 if (element != null) { 26727 open(name); 26728 composeCommunicationRequestCommunicationRequestPayloadComponentInner(element); 26729 close(); 26730 } 26731 } 26732 26733 protected void composeCommunicationRequestCommunicationRequestPayloadComponentInner(CommunicationRequest.CommunicationRequestPayloadComponent element) throws IOException { 26734 composeBackbone(element); 26735 if (element.hasContent()) { 26736 composeType("content", element.getContent()); 26737 } 26738 } 26739 26740 protected void composeCommunicationRequestCommunicationRequestRequesterComponent(String name, CommunicationRequest.CommunicationRequestRequesterComponent element) throws IOException { 26741 if (element != null) { 26742 open(name); 26743 composeCommunicationRequestCommunicationRequestRequesterComponentInner(element); 26744 close(); 26745 } 26746 } 26747 26748 protected void composeCommunicationRequestCommunicationRequestRequesterComponentInner(CommunicationRequest.CommunicationRequestRequesterComponent element) throws IOException { 26749 composeBackbone(element); 26750 if (element.hasAgent()) { 26751 composeReference("agent", element.getAgent()); 26752 } 26753 if (element.hasOnBehalfOf()) { 26754 composeReference("onBehalfOf", element.getOnBehalfOf()); 26755 } 26756 } 26757 26758 protected void composeCompartmentDefinition(String name, CompartmentDefinition element) throws IOException { 26759 if (element != null) { 26760 prop("resourceType", name); 26761 composeCompartmentDefinitionInner(element); 26762 } 26763 } 26764 26765 protected void composeCompartmentDefinitionInner(CompartmentDefinition element) throws IOException { 26766 composeDomainResourceElements(element); 26767 if (element.hasUrlElement()) { 26768 composeUriCore("url", element.getUrlElement(), false); 26769 composeUriExtras("url", element.getUrlElement(), false); 26770 } 26771 if (element.hasNameElement()) { 26772 composeStringCore("name", element.getNameElement(), false); 26773 composeStringExtras("name", element.getNameElement(), false); 26774 } 26775 if (element.hasTitleElement()) { 26776 composeStringCore("title", element.getTitleElement(), false); 26777 composeStringExtras("title", element.getTitleElement(), false); 26778 } 26779 if (element.hasStatusElement()) { 26780 composeEnumerationCore("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 26781 composeEnumerationExtras("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 26782 } 26783 if (element.hasExperimentalElement()) { 26784 composeBooleanCore("experimental", element.getExperimentalElement(), false); 26785 composeBooleanExtras("experimental", element.getExperimentalElement(), false); 26786 } 26787 if (element.hasDateElement()) { 26788 composeDateTimeCore("date", element.getDateElement(), false); 26789 composeDateTimeExtras("date", element.getDateElement(), false); 26790 } 26791 if (element.hasPublisherElement()) { 26792 composeStringCore("publisher", element.getPublisherElement(), false); 26793 composeStringExtras("publisher", element.getPublisherElement(), false); 26794 } 26795 if (element.hasContact()) { 26796 openArray("contact"); 26797 for (ContactDetail e : element.getContact()) 26798 composeContactDetail(null, e); 26799 closeArray(); 26800 }; 26801 if (element.hasDescriptionElement()) { 26802 composeMarkdownCore("description", element.getDescriptionElement(), false); 26803 composeMarkdownExtras("description", element.getDescriptionElement(), false); 26804 } 26805 if (element.hasPurposeElement()) { 26806 composeMarkdownCore("purpose", element.getPurposeElement(), false); 26807 composeMarkdownExtras("purpose", element.getPurposeElement(), false); 26808 } 26809 if (element.hasUseContext()) { 26810 openArray("useContext"); 26811 for (UsageContext e : element.getUseContext()) 26812 composeUsageContext(null, e); 26813 closeArray(); 26814 }; 26815 if (element.hasJurisdiction()) { 26816 openArray("jurisdiction"); 26817 for (CodeableConcept e : element.getJurisdiction()) 26818 composeCodeableConcept(null, e); 26819 closeArray(); 26820 }; 26821 if (element.hasCodeElement()) { 26822 composeEnumerationCore("code", element.getCodeElement(), new CompartmentDefinition.CompartmentTypeEnumFactory(), false); 26823 composeEnumerationExtras("code", element.getCodeElement(), new CompartmentDefinition.CompartmentTypeEnumFactory(), false); 26824 } 26825 if (element.hasSearchElement()) { 26826 composeBooleanCore("search", element.getSearchElement(), false); 26827 composeBooleanExtras("search", element.getSearchElement(), false); 26828 } 26829 if (element.hasResource()) { 26830 openArray("resource"); 26831 for (CompartmentDefinition.CompartmentDefinitionResourceComponent e : element.getResource()) 26832 composeCompartmentDefinitionCompartmentDefinitionResourceComponent(null, e); 26833 closeArray(); 26834 }; 26835 } 26836 26837 protected void composeCompartmentDefinitionCompartmentDefinitionResourceComponent(String name, CompartmentDefinition.CompartmentDefinitionResourceComponent element) throws IOException { 26838 if (element != null) { 26839 open(name); 26840 composeCompartmentDefinitionCompartmentDefinitionResourceComponentInner(element); 26841 close(); 26842 } 26843 } 26844 26845 protected void composeCompartmentDefinitionCompartmentDefinitionResourceComponentInner(CompartmentDefinition.CompartmentDefinitionResourceComponent element) throws IOException { 26846 composeBackbone(element); 26847 if (element.hasCodeElement()) { 26848 composeCodeCore("code", element.getCodeElement(), false); 26849 composeCodeExtras("code", element.getCodeElement(), false); 26850 } 26851 if (element.hasParam()) { 26852 openArray("param"); 26853 for (StringType e : element.getParam()) 26854 composeStringCore(null, e, true); 26855 closeArray(); 26856 if (anyHasExtras(element.getParam())) { 26857 openArray("_param"); 26858 for (StringType e : element.getParam()) 26859 composeStringExtras(null, e, true); 26860 closeArray(); 26861 } 26862 }; 26863 if (element.hasDocumentationElement()) { 26864 composeStringCore("documentation", element.getDocumentationElement(), false); 26865 composeStringExtras("documentation", element.getDocumentationElement(), false); 26866 } 26867 } 26868 26869 protected void composeComposition(String name, Composition element) throws IOException { 26870 if (element != null) { 26871 prop("resourceType", name); 26872 composeCompositionInner(element); 26873 } 26874 } 26875 26876 protected void composeCompositionInner(Composition element) throws IOException { 26877 composeDomainResourceElements(element); 26878 if (element.hasIdentifier()) { 26879 composeIdentifier("identifier", element.getIdentifier()); 26880 } 26881 if (element.hasStatusElement()) { 26882 composeEnumerationCore("status", element.getStatusElement(), new Composition.CompositionStatusEnumFactory(), false); 26883 composeEnumerationExtras("status", element.getStatusElement(), new Composition.CompositionStatusEnumFactory(), false); 26884 } 26885 if (element.hasType()) { 26886 composeCodeableConcept("type", element.getType()); 26887 } 26888 if (element.hasClass_()) { 26889 composeCodeableConcept("class", element.getClass_()); 26890 } 26891 if (element.hasSubject()) { 26892 composeReference("subject", element.getSubject()); 26893 } 26894 if (element.hasEncounter()) { 26895 composeReference("encounter", element.getEncounter()); 26896 } 26897 if (element.hasDateElement()) { 26898 composeDateTimeCore("date", element.getDateElement(), false); 26899 composeDateTimeExtras("date", element.getDateElement(), false); 26900 } 26901 if (element.hasAuthor()) { 26902 openArray("author"); 26903 for (Reference e : element.getAuthor()) 26904 composeReference(null, e); 26905 closeArray(); 26906 }; 26907 if (element.hasTitleElement()) { 26908 composeStringCore("title", element.getTitleElement(), false); 26909 composeStringExtras("title", element.getTitleElement(), false); 26910 } 26911 if (element.hasConfidentialityElement()) { 26912 composeEnumerationCore("confidentiality", element.getConfidentialityElement(), new Composition.DocumentConfidentialityEnumFactory(), false); 26913 composeEnumerationExtras("confidentiality", element.getConfidentialityElement(), new Composition.DocumentConfidentialityEnumFactory(), false); 26914 } 26915 if (element.hasAttester()) { 26916 openArray("attester"); 26917 for (Composition.CompositionAttesterComponent e : element.getAttester()) 26918 composeCompositionCompositionAttesterComponent(null, e); 26919 closeArray(); 26920 }; 26921 if (element.hasCustodian()) { 26922 composeReference("custodian", element.getCustodian()); 26923 } 26924 if (element.hasRelatesTo()) { 26925 openArray("relatesTo"); 26926 for (Composition.CompositionRelatesToComponent e : element.getRelatesTo()) 26927 composeCompositionCompositionRelatesToComponent(null, e); 26928 closeArray(); 26929 }; 26930 if (element.hasEvent()) { 26931 openArray("event"); 26932 for (Composition.CompositionEventComponent e : element.getEvent()) 26933 composeCompositionCompositionEventComponent(null, e); 26934 closeArray(); 26935 }; 26936 if (element.hasSection()) { 26937 openArray("section"); 26938 for (Composition.SectionComponent e : element.getSection()) 26939 composeCompositionSectionComponent(null, e); 26940 closeArray(); 26941 }; 26942 } 26943 26944 protected void composeCompositionCompositionAttesterComponent(String name, Composition.CompositionAttesterComponent element) throws IOException { 26945 if (element != null) { 26946 open(name); 26947 composeCompositionCompositionAttesterComponentInner(element); 26948 close(); 26949 } 26950 } 26951 26952 protected void composeCompositionCompositionAttesterComponentInner(Composition.CompositionAttesterComponent element) throws IOException { 26953 composeBackbone(element); 26954 if (element.hasMode()) { 26955 openArray("mode"); 26956 for (Enumeration<Composition.CompositionAttestationMode> e : element.getMode()) 26957 composeEnumerationCore(null, e, new Composition.CompositionAttestationModeEnumFactory(), true); 26958 closeArray(); 26959 if (anyHasExtras(element.getMode())) { 26960 openArray("_mode"); 26961 for (Enumeration<Composition.CompositionAttestationMode> e : element.getMode()) 26962 composeEnumerationExtras(null, e, new Composition.CompositionAttestationModeEnumFactory(), true); 26963 closeArray(); 26964 } 26965 }; 26966 if (element.hasTimeElement()) { 26967 composeDateTimeCore("time", element.getTimeElement(), false); 26968 composeDateTimeExtras("time", element.getTimeElement(), false); 26969 } 26970 if (element.hasParty()) { 26971 composeReference("party", element.getParty()); 26972 } 26973 } 26974 26975 protected void composeCompositionCompositionRelatesToComponent(String name, Composition.CompositionRelatesToComponent element) throws IOException { 26976 if (element != null) { 26977 open(name); 26978 composeCompositionCompositionRelatesToComponentInner(element); 26979 close(); 26980 } 26981 } 26982 26983 protected void composeCompositionCompositionRelatesToComponentInner(Composition.CompositionRelatesToComponent element) throws IOException { 26984 composeBackbone(element); 26985 if (element.hasCodeElement()) { 26986 composeEnumerationCore("code", element.getCodeElement(), new Composition.DocumentRelationshipTypeEnumFactory(), false); 26987 composeEnumerationExtras("code", element.getCodeElement(), new Composition.DocumentRelationshipTypeEnumFactory(), false); 26988 } 26989 if (element.hasTarget()) { 26990 composeType("target", element.getTarget()); 26991 } 26992 } 26993 26994 protected void composeCompositionCompositionEventComponent(String name, Composition.CompositionEventComponent element) throws IOException { 26995 if (element != null) { 26996 open(name); 26997 composeCompositionCompositionEventComponentInner(element); 26998 close(); 26999 } 27000 } 27001 27002 protected void composeCompositionCompositionEventComponentInner(Composition.CompositionEventComponent element) throws IOException { 27003 composeBackbone(element); 27004 if (element.hasCode()) { 27005 openArray("code"); 27006 for (CodeableConcept e : element.getCode()) 27007 composeCodeableConcept(null, e); 27008 closeArray(); 27009 }; 27010 if (element.hasPeriod()) { 27011 composePeriod("period", element.getPeriod()); 27012 } 27013 if (element.hasDetail()) { 27014 openArray("detail"); 27015 for (Reference e : element.getDetail()) 27016 composeReference(null, e); 27017 closeArray(); 27018 }; 27019 } 27020 27021 protected void composeCompositionSectionComponent(String name, Composition.SectionComponent element) throws IOException { 27022 if (element != null) { 27023 open(name); 27024 composeCompositionSectionComponentInner(element); 27025 close(); 27026 } 27027 } 27028 27029 protected void composeCompositionSectionComponentInner(Composition.SectionComponent element) throws IOException { 27030 composeBackbone(element); 27031 if (element.hasTitleElement()) { 27032 composeStringCore("title", element.getTitleElement(), false); 27033 composeStringExtras("title", element.getTitleElement(), false); 27034 } 27035 if (element.hasCode()) { 27036 composeCodeableConcept("code", element.getCode()); 27037 } 27038 if (element.hasText()) { 27039 composeNarrative("text", element.getText()); 27040 } 27041 if (element.hasModeElement()) { 27042 composeEnumerationCore("mode", element.getModeElement(), new Composition.SectionModeEnumFactory(), false); 27043 composeEnumerationExtras("mode", element.getModeElement(), new Composition.SectionModeEnumFactory(), false); 27044 } 27045 if (element.hasOrderedBy()) { 27046 composeCodeableConcept("orderedBy", element.getOrderedBy()); 27047 } 27048 if (element.hasEntry()) { 27049 openArray("entry"); 27050 for (Reference e : element.getEntry()) 27051 composeReference(null, e); 27052 closeArray(); 27053 }; 27054 if (element.hasEmptyReason()) { 27055 composeCodeableConcept("emptyReason", element.getEmptyReason()); 27056 } 27057 if (element.hasSection()) { 27058 openArray("section"); 27059 for (Composition.SectionComponent e : element.getSection()) 27060 composeCompositionSectionComponent(null, e); 27061 closeArray(); 27062 }; 27063 } 27064 27065 protected void composeConceptMap(String name, ConceptMap element) throws IOException { 27066 if (element != null) { 27067 prop("resourceType", name); 27068 composeConceptMapInner(element); 27069 } 27070 } 27071 27072 protected void composeConceptMapInner(ConceptMap element) throws IOException { 27073 composeDomainResourceElements(element); 27074 if (element.hasUrlElement()) { 27075 composeUriCore("url", element.getUrlElement(), false); 27076 composeUriExtras("url", element.getUrlElement(), false); 27077 } 27078 if (element.hasIdentifier()) { 27079 composeIdentifier("identifier", element.getIdentifier()); 27080 } 27081 if (element.hasVersionElement()) { 27082 composeStringCore("version", element.getVersionElement(), false); 27083 composeStringExtras("version", element.getVersionElement(), false); 27084 } 27085 if (element.hasNameElement()) { 27086 composeStringCore("name", element.getNameElement(), false); 27087 composeStringExtras("name", element.getNameElement(), false); 27088 } 27089 if (element.hasTitleElement()) { 27090 composeStringCore("title", element.getTitleElement(), false); 27091 composeStringExtras("title", element.getTitleElement(), false); 27092 } 27093 if (element.hasStatusElement()) { 27094 composeEnumerationCore("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 27095 composeEnumerationExtras("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 27096 } 27097 if (element.hasExperimentalElement()) { 27098 composeBooleanCore("experimental", element.getExperimentalElement(), false); 27099 composeBooleanExtras("experimental", element.getExperimentalElement(), false); 27100 } 27101 if (element.hasDateElement()) { 27102 composeDateTimeCore("date", element.getDateElement(), false); 27103 composeDateTimeExtras("date", element.getDateElement(), false); 27104 } 27105 if (element.hasPublisherElement()) { 27106 composeStringCore("publisher", element.getPublisherElement(), false); 27107 composeStringExtras("publisher", element.getPublisherElement(), false); 27108 } 27109 if (element.hasContact()) { 27110 openArray("contact"); 27111 for (ContactDetail e : element.getContact()) 27112 composeContactDetail(null, e); 27113 closeArray(); 27114 }; 27115 if (element.hasDescriptionElement()) { 27116 composeMarkdownCore("description", element.getDescriptionElement(), false); 27117 composeMarkdownExtras("description", element.getDescriptionElement(), false); 27118 } 27119 if (element.hasUseContext()) { 27120 openArray("useContext"); 27121 for (UsageContext e : element.getUseContext()) 27122 composeUsageContext(null, e); 27123 closeArray(); 27124 }; 27125 if (element.hasJurisdiction()) { 27126 openArray("jurisdiction"); 27127 for (CodeableConcept e : element.getJurisdiction()) 27128 composeCodeableConcept(null, e); 27129 closeArray(); 27130 }; 27131 if (element.hasPurposeElement()) { 27132 composeMarkdownCore("purpose", element.getPurposeElement(), false); 27133 composeMarkdownExtras("purpose", element.getPurposeElement(), false); 27134 } 27135 if (element.hasCopyrightElement()) { 27136 composeMarkdownCore("copyright", element.getCopyrightElement(), false); 27137 composeMarkdownExtras("copyright", element.getCopyrightElement(), false); 27138 } 27139 if (element.hasSource()) { 27140 composeType("source", element.getSource()); 27141 } 27142 if (element.hasTarget()) { 27143 composeType("target", element.getTarget()); 27144 } 27145 if (element.hasGroup()) { 27146 openArray("group"); 27147 for (ConceptMap.ConceptMapGroupComponent e : element.getGroup()) 27148 composeConceptMapConceptMapGroupComponent(null, e); 27149 closeArray(); 27150 }; 27151 } 27152 27153 protected void composeConceptMapConceptMapGroupComponent(String name, ConceptMap.ConceptMapGroupComponent element) throws IOException { 27154 if (element != null) { 27155 open(name); 27156 composeConceptMapConceptMapGroupComponentInner(element); 27157 close(); 27158 } 27159 } 27160 27161 protected void composeConceptMapConceptMapGroupComponentInner(ConceptMap.ConceptMapGroupComponent element) throws IOException { 27162 composeBackbone(element); 27163 if (element.hasSourceElement()) { 27164 composeUriCore("source", element.getSourceElement(), false); 27165 composeUriExtras("source", element.getSourceElement(), false); 27166 } 27167 if (element.hasSourceVersionElement()) { 27168 composeStringCore("sourceVersion", element.getSourceVersionElement(), false); 27169 composeStringExtras("sourceVersion", element.getSourceVersionElement(), false); 27170 } 27171 if (element.hasTargetElement()) { 27172 composeUriCore("target", element.getTargetElement(), false); 27173 composeUriExtras("target", element.getTargetElement(), false); 27174 } 27175 if (element.hasTargetVersionElement()) { 27176 composeStringCore("targetVersion", element.getTargetVersionElement(), false); 27177 composeStringExtras("targetVersion", element.getTargetVersionElement(), false); 27178 } 27179 if (element.hasElement()) { 27180 openArray("element"); 27181 for (ConceptMap.SourceElementComponent e : element.getElement()) 27182 composeConceptMapSourceElementComponent(null, e); 27183 closeArray(); 27184 }; 27185 if (element.hasUnmapped()) { 27186 composeConceptMapConceptMapGroupUnmappedComponent("unmapped", element.getUnmapped()); 27187 } 27188 } 27189 27190 protected void composeConceptMapSourceElementComponent(String name, ConceptMap.SourceElementComponent element) throws IOException { 27191 if (element != null) { 27192 open(name); 27193 composeConceptMapSourceElementComponentInner(element); 27194 close(); 27195 } 27196 } 27197 27198 protected void composeConceptMapSourceElementComponentInner(ConceptMap.SourceElementComponent element) throws IOException { 27199 composeBackbone(element); 27200 if (element.hasCodeElement()) { 27201 composeCodeCore("code", element.getCodeElement(), false); 27202 composeCodeExtras("code", element.getCodeElement(), false); 27203 } 27204 if (element.hasDisplayElement()) { 27205 composeStringCore("display", element.getDisplayElement(), false); 27206 composeStringExtras("display", element.getDisplayElement(), false); 27207 } 27208 if (element.hasTarget()) { 27209 openArray("target"); 27210 for (ConceptMap.TargetElementComponent e : element.getTarget()) 27211 composeConceptMapTargetElementComponent(null, e); 27212 closeArray(); 27213 }; 27214 } 27215 27216 protected void composeConceptMapTargetElementComponent(String name, ConceptMap.TargetElementComponent element) throws IOException { 27217 if (element != null) { 27218 open(name); 27219 composeConceptMapTargetElementComponentInner(element); 27220 close(); 27221 } 27222 } 27223 27224 protected void composeConceptMapTargetElementComponentInner(ConceptMap.TargetElementComponent element) throws IOException { 27225 composeBackbone(element); 27226 if (element.hasCodeElement()) { 27227 composeCodeCore("code", element.getCodeElement(), false); 27228 composeCodeExtras("code", element.getCodeElement(), false); 27229 } 27230 if (element.hasDisplayElement()) { 27231 composeStringCore("display", element.getDisplayElement(), false); 27232 composeStringExtras("display", element.getDisplayElement(), false); 27233 } 27234 if (element.hasEquivalenceElement()) { 27235 composeEnumerationCore("equivalence", element.getEquivalenceElement(), new Enumerations.ConceptMapEquivalenceEnumFactory(), false); 27236 composeEnumerationExtras("equivalence", element.getEquivalenceElement(), new Enumerations.ConceptMapEquivalenceEnumFactory(), false); 27237 } 27238 if (element.hasCommentElement()) { 27239 composeStringCore("comment", element.getCommentElement(), false); 27240 composeStringExtras("comment", element.getCommentElement(), false); 27241 } 27242 if (element.hasDependsOn()) { 27243 openArray("dependsOn"); 27244 for (ConceptMap.OtherElementComponent e : element.getDependsOn()) 27245 composeConceptMapOtherElementComponent(null, e); 27246 closeArray(); 27247 }; 27248 if (element.hasProduct()) { 27249 openArray("product"); 27250 for (ConceptMap.OtherElementComponent e : element.getProduct()) 27251 composeConceptMapOtherElementComponent(null, e); 27252 closeArray(); 27253 }; 27254 } 27255 27256 protected void composeConceptMapOtherElementComponent(String name, ConceptMap.OtherElementComponent element) throws IOException { 27257 if (element != null) { 27258 open(name); 27259 composeConceptMapOtherElementComponentInner(element); 27260 close(); 27261 } 27262 } 27263 27264 protected void composeConceptMapOtherElementComponentInner(ConceptMap.OtherElementComponent element) throws IOException { 27265 composeBackbone(element); 27266 if (element.hasPropertyElement()) { 27267 composeUriCore("property", element.getPropertyElement(), false); 27268 composeUriExtras("property", element.getPropertyElement(), false); 27269 } 27270 if (element.hasSystemElement()) { 27271 composeUriCore("system", element.getSystemElement(), false); 27272 composeUriExtras("system", element.getSystemElement(), false); 27273 } 27274 if (element.hasCodeElement()) { 27275 composeStringCore("code", element.getCodeElement(), false); 27276 composeStringExtras("code", element.getCodeElement(), false); 27277 } 27278 if (element.hasDisplayElement()) { 27279 composeStringCore("display", element.getDisplayElement(), false); 27280 composeStringExtras("display", element.getDisplayElement(), false); 27281 } 27282 } 27283 27284 protected void composeConceptMapConceptMapGroupUnmappedComponent(String name, ConceptMap.ConceptMapGroupUnmappedComponent element) throws IOException { 27285 if (element != null) { 27286 open(name); 27287 composeConceptMapConceptMapGroupUnmappedComponentInner(element); 27288 close(); 27289 } 27290 } 27291 27292 protected void composeConceptMapConceptMapGroupUnmappedComponentInner(ConceptMap.ConceptMapGroupUnmappedComponent element) throws IOException { 27293 composeBackbone(element); 27294 if (element.hasModeElement()) { 27295 composeEnumerationCore("mode", element.getModeElement(), new ConceptMap.ConceptMapGroupUnmappedModeEnumFactory(), false); 27296 composeEnumerationExtras("mode", element.getModeElement(), new ConceptMap.ConceptMapGroupUnmappedModeEnumFactory(), false); 27297 } 27298 if (element.hasCodeElement()) { 27299 composeCodeCore("code", element.getCodeElement(), false); 27300 composeCodeExtras("code", element.getCodeElement(), false); 27301 } 27302 if (element.hasDisplayElement()) { 27303 composeStringCore("display", element.getDisplayElement(), false); 27304 composeStringExtras("display", element.getDisplayElement(), false); 27305 } 27306 if (element.hasUrlElement()) { 27307 composeUriCore("url", element.getUrlElement(), false); 27308 composeUriExtras("url", element.getUrlElement(), false); 27309 } 27310 } 27311 27312 protected void composeCondition(String name, Condition element) throws IOException { 27313 if (element != null) { 27314 prop("resourceType", name); 27315 composeConditionInner(element); 27316 } 27317 } 27318 27319 protected void composeConditionInner(Condition element) throws IOException { 27320 composeDomainResourceElements(element); 27321 if (element.hasIdentifier()) { 27322 openArray("identifier"); 27323 for (Identifier e : element.getIdentifier()) 27324 composeIdentifier(null, e); 27325 closeArray(); 27326 }; 27327 if (element.hasClinicalStatusElement()) { 27328 composeEnumerationCore("clinicalStatus", element.getClinicalStatusElement(), new Condition.ConditionClinicalStatusEnumFactory(), false); 27329 composeEnumerationExtras("clinicalStatus", element.getClinicalStatusElement(), new Condition.ConditionClinicalStatusEnumFactory(), false); 27330 } 27331 if (element.hasVerificationStatusElement()) { 27332 composeEnumerationCore("verificationStatus", element.getVerificationStatusElement(), new Condition.ConditionVerificationStatusEnumFactory(), false); 27333 composeEnumerationExtras("verificationStatus", element.getVerificationStatusElement(), new Condition.ConditionVerificationStatusEnumFactory(), false); 27334 } 27335 if (element.hasCategory()) { 27336 openArray("category"); 27337 for (CodeableConcept e : element.getCategory()) 27338 composeCodeableConcept(null, e); 27339 closeArray(); 27340 }; 27341 if (element.hasSeverity()) { 27342 composeCodeableConcept("severity", element.getSeverity()); 27343 } 27344 if (element.hasCode()) { 27345 composeCodeableConcept("code", element.getCode()); 27346 } 27347 if (element.hasBodySite()) { 27348 openArray("bodySite"); 27349 for (CodeableConcept e : element.getBodySite()) 27350 composeCodeableConcept(null, e); 27351 closeArray(); 27352 }; 27353 if (element.hasSubject()) { 27354 composeReference("subject", element.getSubject()); 27355 } 27356 if (element.hasContext()) { 27357 composeReference("context", element.getContext()); 27358 } 27359 if (element.hasOnset()) { 27360 composeType("onset", element.getOnset()); 27361 } 27362 if (element.hasAbatement()) { 27363 composeType("abatement", element.getAbatement()); 27364 } 27365 if (element.hasAssertedDateElement()) { 27366 composeDateTimeCore("assertedDate", element.getAssertedDateElement(), false); 27367 composeDateTimeExtras("assertedDate", element.getAssertedDateElement(), false); 27368 } 27369 if (element.hasAsserter()) { 27370 composeReference("asserter", element.getAsserter()); 27371 } 27372 if (element.hasStage()) { 27373 composeConditionConditionStageComponent("stage", element.getStage()); 27374 } 27375 if (element.hasEvidence()) { 27376 openArray("evidence"); 27377 for (Condition.ConditionEvidenceComponent e : element.getEvidence()) 27378 composeConditionConditionEvidenceComponent(null, e); 27379 closeArray(); 27380 }; 27381 if (element.hasNote()) { 27382 openArray("note"); 27383 for (Annotation e : element.getNote()) 27384 composeAnnotation(null, e); 27385 closeArray(); 27386 }; 27387 } 27388 27389 protected void composeConditionConditionStageComponent(String name, Condition.ConditionStageComponent element) throws IOException { 27390 if (element != null) { 27391 open(name); 27392 composeConditionConditionStageComponentInner(element); 27393 close(); 27394 } 27395 } 27396 27397 protected void composeConditionConditionStageComponentInner(Condition.ConditionStageComponent element) throws IOException { 27398 composeBackbone(element); 27399 if (element.hasSummary()) { 27400 composeCodeableConcept("summary", element.getSummary()); 27401 } 27402 if (element.hasAssessment()) { 27403 openArray("assessment"); 27404 for (Reference e : element.getAssessment()) 27405 composeReference(null, e); 27406 closeArray(); 27407 }; 27408 } 27409 27410 protected void composeConditionConditionEvidenceComponent(String name, Condition.ConditionEvidenceComponent element) throws IOException { 27411 if (element != null) { 27412 open(name); 27413 composeConditionConditionEvidenceComponentInner(element); 27414 close(); 27415 } 27416 } 27417 27418 protected void composeConditionConditionEvidenceComponentInner(Condition.ConditionEvidenceComponent element) throws IOException { 27419 composeBackbone(element); 27420 if (element.hasCode()) { 27421 openArray("code"); 27422 for (CodeableConcept e : element.getCode()) 27423 composeCodeableConcept(null, e); 27424 closeArray(); 27425 }; 27426 if (element.hasDetail()) { 27427 openArray("detail"); 27428 for (Reference e : element.getDetail()) 27429 composeReference(null, e); 27430 closeArray(); 27431 }; 27432 } 27433 27434 protected void composeConsent(String name, Consent element) throws IOException { 27435 if (element != null) { 27436 prop("resourceType", name); 27437 composeConsentInner(element); 27438 } 27439 } 27440 27441 protected void composeConsentInner(Consent element) throws IOException { 27442 composeDomainResourceElements(element); 27443 if (element.hasIdentifier()) { 27444 composeIdentifier("identifier", element.getIdentifier()); 27445 } 27446 if (element.hasStatusElement()) { 27447 composeEnumerationCore("status", element.getStatusElement(), new Consent.ConsentStateEnumFactory(), false); 27448 composeEnumerationExtras("status", element.getStatusElement(), new Consent.ConsentStateEnumFactory(), false); 27449 } 27450 if (element.hasCategory()) { 27451 openArray("category"); 27452 for (CodeableConcept e : element.getCategory()) 27453 composeCodeableConcept(null, e); 27454 closeArray(); 27455 }; 27456 if (element.hasPatient()) { 27457 composeReference("patient", element.getPatient()); 27458 } 27459 if (element.hasPeriod()) { 27460 composePeriod("period", element.getPeriod()); 27461 } 27462 if (element.hasDateTimeElement()) { 27463 composeDateTimeCore("dateTime", element.getDateTimeElement(), false); 27464 composeDateTimeExtras("dateTime", element.getDateTimeElement(), false); 27465 } 27466 if (element.hasConsentingParty()) { 27467 openArray("consentingParty"); 27468 for (Reference e : element.getConsentingParty()) 27469 composeReference(null, e); 27470 closeArray(); 27471 }; 27472 if (element.hasActor()) { 27473 openArray("actor"); 27474 for (Consent.ConsentActorComponent e : element.getActor()) 27475 composeConsentConsentActorComponent(null, e); 27476 closeArray(); 27477 }; 27478 if (element.hasAction()) { 27479 openArray("action"); 27480 for (CodeableConcept e : element.getAction()) 27481 composeCodeableConcept(null, e); 27482 closeArray(); 27483 }; 27484 if (element.hasOrganization()) { 27485 openArray("organization"); 27486 for (Reference e : element.getOrganization()) 27487 composeReference(null, e); 27488 closeArray(); 27489 }; 27490 if (element.hasSource()) { 27491 composeType("source", element.getSource()); 27492 } 27493 if (element.hasPolicy()) { 27494 openArray("policy"); 27495 for (Consent.ConsentPolicyComponent e : element.getPolicy()) 27496 composeConsentConsentPolicyComponent(null, e); 27497 closeArray(); 27498 }; 27499 if (element.hasPolicyRuleElement()) { 27500 composeUriCore("policyRule", element.getPolicyRuleElement(), false); 27501 composeUriExtras("policyRule", element.getPolicyRuleElement(), false); 27502 } 27503 if (element.hasSecurityLabel()) { 27504 openArray("securityLabel"); 27505 for (Coding e : element.getSecurityLabel()) 27506 composeCoding(null, e); 27507 closeArray(); 27508 }; 27509 if (element.hasPurpose()) { 27510 openArray("purpose"); 27511 for (Coding e : element.getPurpose()) 27512 composeCoding(null, e); 27513 closeArray(); 27514 }; 27515 if (element.hasDataPeriod()) { 27516 composePeriod("dataPeriod", element.getDataPeriod()); 27517 } 27518 if (element.hasData()) { 27519 openArray("data"); 27520 for (Consent.ConsentDataComponent e : element.getData()) 27521 composeConsentConsentDataComponent(null, e); 27522 closeArray(); 27523 }; 27524 if (element.hasExcept()) { 27525 openArray("except"); 27526 for (Consent.ExceptComponent e : element.getExcept()) 27527 composeConsentExceptComponent(null, e); 27528 closeArray(); 27529 }; 27530 } 27531 27532 protected void composeConsentConsentActorComponent(String name, Consent.ConsentActorComponent element) throws IOException { 27533 if (element != null) { 27534 open(name); 27535 composeConsentConsentActorComponentInner(element); 27536 close(); 27537 } 27538 } 27539 27540 protected void composeConsentConsentActorComponentInner(Consent.ConsentActorComponent element) throws IOException { 27541 composeBackbone(element); 27542 if (element.hasRole()) { 27543 composeCodeableConcept("role", element.getRole()); 27544 } 27545 if (element.hasReference()) { 27546 composeReference("reference", element.getReference()); 27547 } 27548 } 27549 27550 protected void composeConsentConsentPolicyComponent(String name, Consent.ConsentPolicyComponent element) throws IOException { 27551 if (element != null) { 27552 open(name); 27553 composeConsentConsentPolicyComponentInner(element); 27554 close(); 27555 } 27556 } 27557 27558 protected void composeConsentConsentPolicyComponentInner(Consent.ConsentPolicyComponent element) throws IOException { 27559 composeBackbone(element); 27560 if (element.hasAuthorityElement()) { 27561 composeUriCore("authority", element.getAuthorityElement(), false); 27562 composeUriExtras("authority", element.getAuthorityElement(), false); 27563 } 27564 if (element.hasUriElement()) { 27565 composeUriCore("uri", element.getUriElement(), false); 27566 composeUriExtras("uri", element.getUriElement(), false); 27567 } 27568 } 27569 27570 protected void composeConsentConsentDataComponent(String name, Consent.ConsentDataComponent element) throws IOException { 27571 if (element != null) { 27572 open(name); 27573 composeConsentConsentDataComponentInner(element); 27574 close(); 27575 } 27576 } 27577 27578 protected void composeConsentConsentDataComponentInner(Consent.ConsentDataComponent element) throws IOException { 27579 composeBackbone(element); 27580 if (element.hasMeaningElement()) { 27581 composeEnumerationCore("meaning", element.getMeaningElement(), new Consent.ConsentDataMeaningEnumFactory(), false); 27582 composeEnumerationExtras("meaning", element.getMeaningElement(), new Consent.ConsentDataMeaningEnumFactory(), false); 27583 } 27584 if (element.hasReference()) { 27585 composeReference("reference", element.getReference()); 27586 } 27587 } 27588 27589 protected void composeConsentExceptComponent(String name, Consent.ExceptComponent element) throws IOException { 27590 if (element != null) { 27591 open(name); 27592 composeConsentExceptComponentInner(element); 27593 close(); 27594 } 27595 } 27596 27597 protected void composeConsentExceptComponentInner(Consent.ExceptComponent element) throws IOException { 27598 composeBackbone(element); 27599 if (element.hasTypeElement()) { 27600 composeEnumerationCore("type", element.getTypeElement(), new Consent.ConsentExceptTypeEnumFactory(), false); 27601 composeEnumerationExtras("type", element.getTypeElement(), new Consent.ConsentExceptTypeEnumFactory(), false); 27602 } 27603 if (element.hasPeriod()) { 27604 composePeriod("period", element.getPeriod()); 27605 } 27606 if (element.hasActor()) { 27607 openArray("actor"); 27608 for (Consent.ExceptActorComponent e : element.getActor()) 27609 composeConsentExceptActorComponent(null, e); 27610 closeArray(); 27611 }; 27612 if (element.hasAction()) { 27613 openArray("action"); 27614 for (CodeableConcept e : element.getAction()) 27615 composeCodeableConcept(null, e); 27616 closeArray(); 27617 }; 27618 if (element.hasSecurityLabel()) { 27619 openArray("securityLabel"); 27620 for (Coding e : element.getSecurityLabel()) 27621 composeCoding(null, e); 27622 closeArray(); 27623 }; 27624 if (element.hasPurpose()) { 27625 openArray("purpose"); 27626 for (Coding e : element.getPurpose()) 27627 composeCoding(null, e); 27628 closeArray(); 27629 }; 27630 if (element.hasClass_()) { 27631 openArray("class"); 27632 for (Coding e : element.getClass_()) 27633 composeCoding(null, e); 27634 closeArray(); 27635 }; 27636 if (element.hasCode()) { 27637 openArray("code"); 27638 for (Coding e : element.getCode()) 27639 composeCoding(null, e); 27640 closeArray(); 27641 }; 27642 if (element.hasDataPeriod()) { 27643 composePeriod("dataPeriod", element.getDataPeriod()); 27644 } 27645 if (element.hasData()) { 27646 openArray("data"); 27647 for (Consent.ExceptDataComponent e : element.getData()) 27648 composeConsentExceptDataComponent(null, e); 27649 closeArray(); 27650 }; 27651 } 27652 27653 protected void composeConsentExceptActorComponent(String name, Consent.ExceptActorComponent element) throws IOException { 27654 if (element != null) { 27655 open(name); 27656 composeConsentExceptActorComponentInner(element); 27657 close(); 27658 } 27659 } 27660 27661 protected void composeConsentExceptActorComponentInner(Consent.ExceptActorComponent element) throws IOException { 27662 composeBackbone(element); 27663 if (element.hasRole()) { 27664 composeCodeableConcept("role", element.getRole()); 27665 } 27666 if (element.hasReference()) { 27667 composeReference("reference", element.getReference()); 27668 } 27669 } 27670 27671 protected void composeConsentExceptDataComponent(String name, Consent.ExceptDataComponent element) throws IOException { 27672 if (element != null) { 27673 open(name); 27674 composeConsentExceptDataComponentInner(element); 27675 close(); 27676 } 27677 } 27678 27679 protected void composeConsentExceptDataComponentInner(Consent.ExceptDataComponent element) throws IOException { 27680 composeBackbone(element); 27681 if (element.hasMeaningElement()) { 27682 composeEnumerationCore("meaning", element.getMeaningElement(), new Consent.ConsentDataMeaningEnumFactory(), false); 27683 composeEnumerationExtras("meaning", element.getMeaningElement(), new Consent.ConsentDataMeaningEnumFactory(), false); 27684 } 27685 if (element.hasReference()) { 27686 composeReference("reference", element.getReference()); 27687 } 27688 } 27689 27690 protected void composeContract(String name, Contract element) throws IOException { 27691 if (element != null) { 27692 prop("resourceType", name); 27693 composeContractInner(element); 27694 } 27695 } 27696 27697 protected void composeContractInner(Contract element) throws IOException { 27698 composeDomainResourceElements(element); 27699 if (element.hasIdentifier()) { 27700 composeIdentifier("identifier", element.getIdentifier()); 27701 } 27702 if (element.hasStatusElement()) { 27703 composeEnumerationCore("status", element.getStatusElement(), new Contract.ContractStatusEnumFactory(), false); 27704 composeEnumerationExtras("status", element.getStatusElement(), new Contract.ContractStatusEnumFactory(), false); 27705 } 27706 if (element.hasIssuedElement()) { 27707 composeDateTimeCore("issued", element.getIssuedElement(), false); 27708 composeDateTimeExtras("issued", element.getIssuedElement(), false); 27709 } 27710 if (element.hasApplies()) { 27711 composePeriod("applies", element.getApplies()); 27712 } 27713 if (element.hasSubject()) { 27714 openArray("subject"); 27715 for (Reference e : element.getSubject()) 27716 composeReference(null, e); 27717 closeArray(); 27718 }; 27719 if (element.hasTopic()) { 27720 openArray("topic"); 27721 for (Reference e : element.getTopic()) 27722 composeReference(null, e); 27723 closeArray(); 27724 }; 27725 if (element.hasAuthority()) { 27726 openArray("authority"); 27727 for (Reference e : element.getAuthority()) 27728 composeReference(null, e); 27729 closeArray(); 27730 }; 27731 if (element.hasDomain()) { 27732 openArray("domain"); 27733 for (Reference e : element.getDomain()) 27734 composeReference(null, e); 27735 closeArray(); 27736 }; 27737 if (element.hasType()) { 27738 composeCodeableConcept("type", element.getType()); 27739 } 27740 if (element.hasSubType()) { 27741 openArray("subType"); 27742 for (CodeableConcept e : element.getSubType()) 27743 composeCodeableConcept(null, e); 27744 closeArray(); 27745 }; 27746 if (element.hasAction()) { 27747 openArray("action"); 27748 for (CodeableConcept e : element.getAction()) 27749 composeCodeableConcept(null, e); 27750 closeArray(); 27751 }; 27752 if (element.hasActionReason()) { 27753 openArray("actionReason"); 27754 for (CodeableConcept e : element.getActionReason()) 27755 composeCodeableConcept(null, e); 27756 closeArray(); 27757 }; 27758 if (element.hasDecisionType()) { 27759 composeCodeableConcept("decisionType", element.getDecisionType()); 27760 } 27761 if (element.hasContentDerivative()) { 27762 composeCodeableConcept("contentDerivative", element.getContentDerivative()); 27763 } 27764 if (element.hasSecurityLabel()) { 27765 openArray("securityLabel"); 27766 for (Coding e : element.getSecurityLabel()) 27767 composeCoding(null, e); 27768 closeArray(); 27769 }; 27770 if (element.hasAgent()) { 27771 openArray("agent"); 27772 for (Contract.AgentComponent e : element.getAgent()) 27773 composeContractAgentComponent(null, e); 27774 closeArray(); 27775 }; 27776 if (element.hasSigner()) { 27777 openArray("signer"); 27778 for (Contract.SignatoryComponent e : element.getSigner()) 27779 composeContractSignatoryComponent(null, e); 27780 closeArray(); 27781 }; 27782 if (element.hasValuedItem()) { 27783 openArray("valuedItem"); 27784 for (Contract.ValuedItemComponent e : element.getValuedItem()) 27785 composeContractValuedItemComponent(null, e); 27786 closeArray(); 27787 }; 27788 if (element.hasTerm()) { 27789 openArray("term"); 27790 for (Contract.TermComponent e : element.getTerm()) 27791 composeContractTermComponent(null, e); 27792 closeArray(); 27793 }; 27794 if (element.hasBinding()) { 27795 composeType("binding", element.getBinding()); 27796 } 27797 if (element.hasFriendly()) { 27798 openArray("friendly"); 27799 for (Contract.FriendlyLanguageComponent e : element.getFriendly()) 27800 composeContractFriendlyLanguageComponent(null, e); 27801 closeArray(); 27802 }; 27803 if (element.hasLegal()) { 27804 openArray("legal"); 27805 for (Contract.LegalLanguageComponent e : element.getLegal()) 27806 composeContractLegalLanguageComponent(null, e); 27807 closeArray(); 27808 }; 27809 if (element.hasRule()) { 27810 openArray("rule"); 27811 for (Contract.ComputableLanguageComponent e : element.getRule()) 27812 composeContractComputableLanguageComponent(null, e); 27813 closeArray(); 27814 }; 27815 } 27816 27817 protected void composeContractAgentComponent(String name, Contract.AgentComponent element) throws IOException { 27818 if (element != null) { 27819 open(name); 27820 composeContractAgentComponentInner(element); 27821 close(); 27822 } 27823 } 27824 27825 protected void composeContractAgentComponentInner(Contract.AgentComponent element) throws IOException { 27826 composeBackbone(element); 27827 if (element.hasActor()) { 27828 composeReference("actor", element.getActor()); 27829 } 27830 if (element.hasRole()) { 27831 openArray("role"); 27832 for (CodeableConcept e : element.getRole()) 27833 composeCodeableConcept(null, e); 27834 closeArray(); 27835 }; 27836 } 27837 27838 protected void composeContractSignatoryComponent(String name, Contract.SignatoryComponent element) throws IOException { 27839 if (element != null) { 27840 open(name); 27841 composeContractSignatoryComponentInner(element); 27842 close(); 27843 } 27844 } 27845 27846 protected void composeContractSignatoryComponentInner(Contract.SignatoryComponent element) throws IOException { 27847 composeBackbone(element); 27848 if (element.hasType()) { 27849 composeCoding("type", element.getType()); 27850 } 27851 if (element.hasParty()) { 27852 composeReference("party", element.getParty()); 27853 } 27854 if (element.hasSignature()) { 27855 openArray("signature"); 27856 for (Signature e : element.getSignature()) 27857 composeSignature(null, e); 27858 closeArray(); 27859 }; 27860 } 27861 27862 protected void composeContractValuedItemComponent(String name, Contract.ValuedItemComponent element) throws IOException { 27863 if (element != null) { 27864 open(name); 27865 composeContractValuedItemComponentInner(element); 27866 close(); 27867 } 27868 } 27869 27870 protected void composeContractValuedItemComponentInner(Contract.ValuedItemComponent element) throws IOException { 27871 composeBackbone(element); 27872 if (element.hasEntity()) { 27873 composeType("entity", element.getEntity()); 27874 } 27875 if (element.hasIdentifier()) { 27876 composeIdentifier("identifier", element.getIdentifier()); 27877 } 27878 if (element.hasEffectiveTimeElement()) { 27879 composeDateTimeCore("effectiveTime", element.getEffectiveTimeElement(), false); 27880 composeDateTimeExtras("effectiveTime", element.getEffectiveTimeElement(), false); 27881 } 27882 if (element.hasQuantity()) { 27883 composeSimpleQuantity("quantity", element.getQuantity()); 27884 } 27885 if (element.hasUnitPrice()) { 27886 composeMoney("unitPrice", element.getUnitPrice()); 27887 } 27888 if (element.hasFactorElement()) { 27889 composeDecimalCore("factor", element.getFactorElement(), false); 27890 composeDecimalExtras("factor", element.getFactorElement(), false); 27891 } 27892 if (element.hasPointsElement()) { 27893 composeDecimalCore("points", element.getPointsElement(), false); 27894 composeDecimalExtras("points", element.getPointsElement(), false); 27895 } 27896 if (element.hasNet()) { 27897 composeMoney("net", element.getNet()); 27898 } 27899 } 27900 27901 protected void composeContractTermComponent(String name, Contract.TermComponent element) throws IOException { 27902 if (element != null) { 27903 open(name); 27904 composeContractTermComponentInner(element); 27905 close(); 27906 } 27907 } 27908 27909 protected void composeContractTermComponentInner(Contract.TermComponent element) throws IOException { 27910 composeBackbone(element); 27911 if (element.hasIdentifier()) { 27912 composeIdentifier("identifier", element.getIdentifier()); 27913 } 27914 if (element.hasIssuedElement()) { 27915 composeDateTimeCore("issued", element.getIssuedElement(), false); 27916 composeDateTimeExtras("issued", element.getIssuedElement(), false); 27917 } 27918 if (element.hasApplies()) { 27919 composePeriod("applies", element.getApplies()); 27920 } 27921 if (element.hasType()) { 27922 composeCodeableConcept("type", element.getType()); 27923 } 27924 if (element.hasSubType()) { 27925 composeCodeableConcept("subType", element.getSubType()); 27926 } 27927 if (element.hasTopic()) { 27928 openArray("topic"); 27929 for (Reference e : element.getTopic()) 27930 composeReference(null, e); 27931 closeArray(); 27932 }; 27933 if (element.hasAction()) { 27934 openArray("action"); 27935 for (CodeableConcept e : element.getAction()) 27936 composeCodeableConcept(null, e); 27937 closeArray(); 27938 }; 27939 if (element.hasActionReason()) { 27940 openArray("actionReason"); 27941 for (CodeableConcept e : element.getActionReason()) 27942 composeCodeableConcept(null, e); 27943 closeArray(); 27944 }; 27945 if (element.hasSecurityLabel()) { 27946 openArray("securityLabel"); 27947 for (Coding e : element.getSecurityLabel()) 27948 composeCoding(null, e); 27949 closeArray(); 27950 }; 27951 if (element.hasAgent()) { 27952 openArray("agent"); 27953 for (Contract.TermAgentComponent e : element.getAgent()) 27954 composeContractTermAgentComponent(null, e); 27955 closeArray(); 27956 }; 27957 if (element.hasTextElement()) { 27958 composeStringCore("text", element.getTextElement(), false); 27959 composeStringExtras("text", element.getTextElement(), false); 27960 } 27961 if (element.hasValuedItem()) { 27962 openArray("valuedItem"); 27963 for (Contract.TermValuedItemComponent e : element.getValuedItem()) 27964 composeContractTermValuedItemComponent(null, e); 27965 closeArray(); 27966 }; 27967 if (element.hasGroup()) { 27968 openArray("group"); 27969 for (Contract.TermComponent e : element.getGroup()) 27970 composeContractTermComponent(null, e); 27971 closeArray(); 27972 }; 27973 } 27974 27975 protected void composeContractTermAgentComponent(String name, Contract.TermAgentComponent element) throws IOException { 27976 if (element != null) { 27977 open(name); 27978 composeContractTermAgentComponentInner(element); 27979 close(); 27980 } 27981 } 27982 27983 protected void composeContractTermAgentComponentInner(Contract.TermAgentComponent element) throws IOException { 27984 composeBackbone(element); 27985 if (element.hasActor()) { 27986 composeReference("actor", element.getActor()); 27987 } 27988 if (element.hasRole()) { 27989 openArray("role"); 27990 for (CodeableConcept e : element.getRole()) 27991 composeCodeableConcept(null, e); 27992 closeArray(); 27993 }; 27994 } 27995 27996 protected void composeContractTermValuedItemComponent(String name, Contract.TermValuedItemComponent element) throws IOException { 27997 if (element != null) { 27998 open(name); 27999 composeContractTermValuedItemComponentInner(element); 28000 close(); 28001 } 28002 } 28003 28004 protected void composeContractTermValuedItemComponentInner(Contract.TermValuedItemComponent element) throws IOException { 28005 composeBackbone(element); 28006 if (element.hasEntity()) { 28007 composeType("entity", element.getEntity()); 28008 } 28009 if (element.hasIdentifier()) { 28010 composeIdentifier("identifier", element.getIdentifier()); 28011 } 28012 if (element.hasEffectiveTimeElement()) { 28013 composeDateTimeCore("effectiveTime", element.getEffectiveTimeElement(), false); 28014 composeDateTimeExtras("effectiveTime", element.getEffectiveTimeElement(), false); 28015 } 28016 if (element.hasQuantity()) { 28017 composeSimpleQuantity("quantity", element.getQuantity()); 28018 } 28019 if (element.hasUnitPrice()) { 28020 composeMoney("unitPrice", element.getUnitPrice()); 28021 } 28022 if (element.hasFactorElement()) { 28023 composeDecimalCore("factor", element.getFactorElement(), false); 28024 composeDecimalExtras("factor", element.getFactorElement(), false); 28025 } 28026 if (element.hasPointsElement()) { 28027 composeDecimalCore("points", element.getPointsElement(), false); 28028 composeDecimalExtras("points", element.getPointsElement(), false); 28029 } 28030 if (element.hasNet()) { 28031 composeMoney("net", element.getNet()); 28032 } 28033 } 28034 28035 protected void composeContractFriendlyLanguageComponent(String name, Contract.FriendlyLanguageComponent element) throws IOException { 28036 if (element != null) { 28037 open(name); 28038 composeContractFriendlyLanguageComponentInner(element); 28039 close(); 28040 } 28041 } 28042 28043 protected void composeContractFriendlyLanguageComponentInner(Contract.FriendlyLanguageComponent element) throws IOException { 28044 composeBackbone(element); 28045 if (element.hasContent()) { 28046 composeType("content", element.getContent()); 28047 } 28048 } 28049 28050 protected void composeContractLegalLanguageComponent(String name, Contract.LegalLanguageComponent element) throws IOException { 28051 if (element != null) { 28052 open(name); 28053 composeContractLegalLanguageComponentInner(element); 28054 close(); 28055 } 28056 } 28057 28058 protected void composeContractLegalLanguageComponentInner(Contract.LegalLanguageComponent element) throws IOException { 28059 composeBackbone(element); 28060 if (element.hasContent()) { 28061 composeType("content", element.getContent()); 28062 } 28063 } 28064 28065 protected void composeContractComputableLanguageComponent(String name, Contract.ComputableLanguageComponent element) throws IOException { 28066 if (element != null) { 28067 open(name); 28068 composeContractComputableLanguageComponentInner(element); 28069 close(); 28070 } 28071 } 28072 28073 protected void composeContractComputableLanguageComponentInner(Contract.ComputableLanguageComponent element) throws IOException { 28074 composeBackbone(element); 28075 if (element.hasContent()) { 28076 composeType("content", element.getContent()); 28077 } 28078 } 28079 28080 protected void composeCoverage(String name, Coverage element) throws IOException { 28081 if (element != null) { 28082 prop("resourceType", name); 28083 composeCoverageInner(element); 28084 } 28085 } 28086 28087 protected void composeCoverageInner(Coverage element) throws IOException { 28088 composeDomainResourceElements(element); 28089 if (element.hasIdentifier()) { 28090 openArray("identifier"); 28091 for (Identifier e : element.getIdentifier()) 28092 composeIdentifier(null, e); 28093 closeArray(); 28094 }; 28095 if (element.hasStatusElement()) { 28096 composeEnumerationCore("status", element.getStatusElement(), new Coverage.CoverageStatusEnumFactory(), false); 28097 composeEnumerationExtras("status", element.getStatusElement(), new Coverage.CoverageStatusEnumFactory(), false); 28098 } 28099 if (element.hasType()) { 28100 composeCodeableConcept("type", element.getType()); 28101 } 28102 if (element.hasPolicyHolder()) { 28103 composeReference("policyHolder", element.getPolicyHolder()); 28104 } 28105 if (element.hasSubscriber()) { 28106 composeReference("subscriber", element.getSubscriber()); 28107 } 28108 if (element.hasSubscriberIdElement()) { 28109 composeStringCore("subscriberId", element.getSubscriberIdElement(), false); 28110 composeStringExtras("subscriberId", element.getSubscriberIdElement(), false); 28111 } 28112 if (element.hasBeneficiary()) { 28113 composeReference("beneficiary", element.getBeneficiary()); 28114 } 28115 if (element.hasRelationship()) { 28116 composeCodeableConcept("relationship", element.getRelationship()); 28117 } 28118 if (element.hasPeriod()) { 28119 composePeriod("period", element.getPeriod()); 28120 } 28121 if (element.hasPayor()) { 28122 openArray("payor"); 28123 for (Reference e : element.getPayor()) 28124 composeReference(null, e); 28125 closeArray(); 28126 }; 28127 if (element.hasGrouping()) { 28128 composeCoverageGroupComponent("grouping", element.getGrouping()); 28129 } 28130 if (element.hasDependentElement()) { 28131 composeStringCore("dependent", element.getDependentElement(), false); 28132 composeStringExtras("dependent", element.getDependentElement(), false); 28133 } 28134 if (element.hasSequenceElement()) { 28135 composeStringCore("sequence", element.getSequenceElement(), false); 28136 composeStringExtras("sequence", element.getSequenceElement(), false); 28137 } 28138 if (element.hasOrderElement()) { 28139 composePositiveIntCore("order", element.getOrderElement(), false); 28140 composePositiveIntExtras("order", element.getOrderElement(), false); 28141 } 28142 if (element.hasNetworkElement()) { 28143 composeStringCore("network", element.getNetworkElement(), false); 28144 composeStringExtras("network", element.getNetworkElement(), false); 28145 } 28146 if (element.hasContract()) { 28147 openArray("contract"); 28148 for (Reference e : element.getContract()) 28149 composeReference(null, e); 28150 closeArray(); 28151 }; 28152 } 28153 28154 protected void composeCoverageGroupComponent(String name, Coverage.GroupComponent element) throws IOException { 28155 if (element != null) { 28156 open(name); 28157 composeCoverageGroupComponentInner(element); 28158 close(); 28159 } 28160 } 28161 28162 protected void composeCoverageGroupComponentInner(Coverage.GroupComponent element) throws IOException { 28163 composeBackbone(element); 28164 if (element.hasGroupElement()) { 28165 composeStringCore("group", element.getGroupElement(), false); 28166 composeStringExtras("group", element.getGroupElement(), false); 28167 } 28168 if (element.hasGroupDisplayElement()) { 28169 composeStringCore("groupDisplay", element.getGroupDisplayElement(), false); 28170 composeStringExtras("groupDisplay", element.getGroupDisplayElement(), false); 28171 } 28172 if (element.hasSubGroupElement()) { 28173 composeStringCore("subGroup", element.getSubGroupElement(), false); 28174 composeStringExtras("subGroup", element.getSubGroupElement(), false); 28175 } 28176 if (element.hasSubGroupDisplayElement()) { 28177 composeStringCore("subGroupDisplay", element.getSubGroupDisplayElement(), false); 28178 composeStringExtras("subGroupDisplay", element.getSubGroupDisplayElement(), false); 28179 } 28180 if (element.hasPlanElement()) { 28181 composeStringCore("plan", element.getPlanElement(), false); 28182 composeStringExtras("plan", element.getPlanElement(), false); 28183 } 28184 if (element.hasPlanDisplayElement()) { 28185 composeStringCore("planDisplay", element.getPlanDisplayElement(), false); 28186 composeStringExtras("planDisplay", element.getPlanDisplayElement(), false); 28187 } 28188 if (element.hasSubPlanElement()) { 28189 composeStringCore("subPlan", element.getSubPlanElement(), false); 28190 composeStringExtras("subPlan", element.getSubPlanElement(), false); 28191 } 28192 if (element.hasSubPlanDisplayElement()) { 28193 composeStringCore("subPlanDisplay", element.getSubPlanDisplayElement(), false); 28194 composeStringExtras("subPlanDisplay", element.getSubPlanDisplayElement(), false); 28195 } 28196 if (element.hasClass_Element()) { 28197 composeStringCore("class", element.getClass_Element(), false); 28198 composeStringExtras("class", element.getClass_Element(), false); 28199 } 28200 if (element.hasClassDisplayElement()) { 28201 composeStringCore("classDisplay", element.getClassDisplayElement(), false); 28202 composeStringExtras("classDisplay", element.getClassDisplayElement(), false); 28203 } 28204 if (element.hasSubClassElement()) { 28205 composeStringCore("subClass", element.getSubClassElement(), false); 28206 composeStringExtras("subClass", element.getSubClassElement(), false); 28207 } 28208 if (element.hasSubClassDisplayElement()) { 28209 composeStringCore("subClassDisplay", element.getSubClassDisplayElement(), false); 28210 composeStringExtras("subClassDisplay", element.getSubClassDisplayElement(), false); 28211 } 28212 } 28213 28214 protected void composeDataElement(String name, DataElement element) throws IOException { 28215 if (element != null) { 28216 prop("resourceType", name); 28217 composeDataElementInner(element); 28218 } 28219 } 28220 28221 protected void composeDataElementInner(DataElement element) throws IOException { 28222 composeDomainResourceElements(element); 28223 if (element.hasUrlElement()) { 28224 composeUriCore("url", element.getUrlElement(), false); 28225 composeUriExtras("url", element.getUrlElement(), false); 28226 } 28227 if (element.hasIdentifier()) { 28228 openArray("identifier"); 28229 for (Identifier e : element.getIdentifier()) 28230 composeIdentifier(null, e); 28231 closeArray(); 28232 }; 28233 if (element.hasVersionElement()) { 28234 composeStringCore("version", element.getVersionElement(), false); 28235 composeStringExtras("version", element.getVersionElement(), false); 28236 } 28237 if (element.hasStatusElement()) { 28238 composeEnumerationCore("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 28239 composeEnumerationExtras("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 28240 } 28241 if (element.hasExperimentalElement()) { 28242 composeBooleanCore("experimental", element.getExperimentalElement(), false); 28243 composeBooleanExtras("experimental", element.getExperimentalElement(), false); 28244 } 28245 if (element.hasDateElement()) { 28246 composeDateTimeCore("date", element.getDateElement(), false); 28247 composeDateTimeExtras("date", element.getDateElement(), false); 28248 } 28249 if (element.hasPublisherElement()) { 28250 composeStringCore("publisher", element.getPublisherElement(), false); 28251 composeStringExtras("publisher", element.getPublisherElement(), false); 28252 } 28253 if (element.hasNameElement()) { 28254 composeStringCore("name", element.getNameElement(), false); 28255 composeStringExtras("name", element.getNameElement(), false); 28256 } 28257 if (element.hasTitleElement()) { 28258 composeStringCore("title", element.getTitleElement(), false); 28259 composeStringExtras("title", element.getTitleElement(), false); 28260 } 28261 if (element.hasContact()) { 28262 openArray("contact"); 28263 for (ContactDetail e : element.getContact()) 28264 composeContactDetail(null, e); 28265 closeArray(); 28266 }; 28267 if (element.hasUseContext()) { 28268 openArray("useContext"); 28269 for (UsageContext e : element.getUseContext()) 28270 composeUsageContext(null, e); 28271 closeArray(); 28272 }; 28273 if (element.hasJurisdiction()) { 28274 openArray("jurisdiction"); 28275 for (CodeableConcept e : element.getJurisdiction()) 28276 composeCodeableConcept(null, e); 28277 closeArray(); 28278 }; 28279 if (element.hasCopyrightElement()) { 28280 composeMarkdownCore("copyright", element.getCopyrightElement(), false); 28281 composeMarkdownExtras("copyright", element.getCopyrightElement(), false); 28282 } 28283 if (element.hasStringencyElement()) { 28284 composeEnumerationCore("stringency", element.getStringencyElement(), new DataElement.DataElementStringencyEnumFactory(), false); 28285 composeEnumerationExtras("stringency", element.getStringencyElement(), new DataElement.DataElementStringencyEnumFactory(), false); 28286 } 28287 if (element.hasMapping()) { 28288 openArray("mapping"); 28289 for (DataElement.DataElementMappingComponent e : element.getMapping()) 28290 composeDataElementDataElementMappingComponent(null, e); 28291 closeArray(); 28292 }; 28293 if (element.hasElement()) { 28294 openArray("element"); 28295 for (ElementDefinition e : element.getElement()) 28296 composeElementDefinition(null, e); 28297 closeArray(); 28298 }; 28299 } 28300 28301 protected void composeDataElementDataElementMappingComponent(String name, DataElement.DataElementMappingComponent element) throws IOException { 28302 if (element != null) { 28303 open(name); 28304 composeDataElementDataElementMappingComponentInner(element); 28305 close(); 28306 } 28307 } 28308 28309 protected void composeDataElementDataElementMappingComponentInner(DataElement.DataElementMappingComponent element) throws IOException { 28310 composeBackbone(element); 28311 if (element.hasIdentityElement()) { 28312 composeIdCore("identity", element.getIdentityElement(), false); 28313 composeIdExtras("identity", element.getIdentityElement(), false); 28314 } 28315 if (element.hasUriElement()) { 28316 composeUriCore("uri", element.getUriElement(), false); 28317 composeUriExtras("uri", element.getUriElement(), false); 28318 } 28319 if (element.hasNameElement()) { 28320 composeStringCore("name", element.getNameElement(), false); 28321 composeStringExtras("name", element.getNameElement(), false); 28322 } 28323 if (element.hasCommentElement()) { 28324 composeStringCore("comment", element.getCommentElement(), false); 28325 composeStringExtras("comment", element.getCommentElement(), false); 28326 } 28327 } 28328 28329 protected void composeDetectedIssue(String name, DetectedIssue element) throws IOException { 28330 if (element != null) { 28331 prop("resourceType", name); 28332 composeDetectedIssueInner(element); 28333 } 28334 } 28335 28336 protected void composeDetectedIssueInner(DetectedIssue element) throws IOException { 28337 composeDomainResourceElements(element); 28338 if (element.hasIdentifier()) { 28339 composeIdentifier("identifier", element.getIdentifier()); 28340 } 28341 if (element.hasStatusElement()) { 28342 composeEnumerationCore("status", element.getStatusElement(), new DetectedIssue.DetectedIssueStatusEnumFactory(), false); 28343 composeEnumerationExtras("status", element.getStatusElement(), new DetectedIssue.DetectedIssueStatusEnumFactory(), false); 28344 } 28345 if (element.hasCategory()) { 28346 composeCodeableConcept("category", element.getCategory()); 28347 } 28348 if (element.hasSeverityElement()) { 28349 composeEnumerationCore("severity", element.getSeverityElement(), new DetectedIssue.DetectedIssueSeverityEnumFactory(), false); 28350 composeEnumerationExtras("severity", element.getSeverityElement(), new DetectedIssue.DetectedIssueSeverityEnumFactory(), false); 28351 } 28352 if (element.hasPatient()) { 28353 composeReference("patient", element.getPatient()); 28354 } 28355 if (element.hasDateElement()) { 28356 composeDateTimeCore("date", element.getDateElement(), false); 28357 composeDateTimeExtras("date", element.getDateElement(), false); 28358 } 28359 if (element.hasAuthor()) { 28360 composeReference("author", element.getAuthor()); 28361 } 28362 if (element.hasImplicated()) { 28363 openArray("implicated"); 28364 for (Reference e : element.getImplicated()) 28365 composeReference(null, e); 28366 closeArray(); 28367 }; 28368 if (element.hasDetailElement()) { 28369 composeStringCore("detail", element.getDetailElement(), false); 28370 composeStringExtras("detail", element.getDetailElement(), false); 28371 } 28372 if (element.hasReferenceElement()) { 28373 composeUriCore("reference", element.getReferenceElement(), false); 28374 composeUriExtras("reference", element.getReferenceElement(), false); 28375 } 28376 if (element.hasMitigation()) { 28377 openArray("mitigation"); 28378 for (DetectedIssue.DetectedIssueMitigationComponent e : element.getMitigation()) 28379 composeDetectedIssueDetectedIssueMitigationComponent(null, e); 28380 closeArray(); 28381 }; 28382 } 28383 28384 protected void composeDetectedIssueDetectedIssueMitigationComponent(String name, DetectedIssue.DetectedIssueMitigationComponent element) throws IOException { 28385 if (element != null) { 28386 open(name); 28387 composeDetectedIssueDetectedIssueMitigationComponentInner(element); 28388 close(); 28389 } 28390 } 28391 28392 protected void composeDetectedIssueDetectedIssueMitigationComponentInner(DetectedIssue.DetectedIssueMitigationComponent element) throws IOException { 28393 composeBackbone(element); 28394 if (element.hasAction()) { 28395 composeCodeableConcept("action", element.getAction()); 28396 } 28397 if (element.hasDateElement()) { 28398 composeDateTimeCore("date", element.getDateElement(), false); 28399 composeDateTimeExtras("date", element.getDateElement(), false); 28400 } 28401 if (element.hasAuthor()) { 28402 composeReference("author", element.getAuthor()); 28403 } 28404 } 28405 28406 protected void composeDevice(String name, Device element) throws IOException { 28407 if (element != null) { 28408 prop("resourceType", name); 28409 composeDeviceInner(element); 28410 } 28411 } 28412 28413 protected void composeDeviceInner(Device element) throws IOException { 28414 composeDomainResourceElements(element); 28415 if (element.hasIdentifier()) { 28416 openArray("identifier"); 28417 for (Identifier e : element.getIdentifier()) 28418 composeIdentifier(null, e); 28419 closeArray(); 28420 }; 28421 if (element.hasUdi()) { 28422 composeDeviceDeviceUdiComponent("udi", element.getUdi()); 28423 } 28424 if (element.hasStatusElement()) { 28425 composeEnumerationCore("status", element.getStatusElement(), new Device.FHIRDeviceStatusEnumFactory(), false); 28426 composeEnumerationExtras("status", element.getStatusElement(), new Device.FHIRDeviceStatusEnumFactory(), false); 28427 } 28428 if (element.hasType()) { 28429 composeCodeableConcept("type", element.getType()); 28430 } 28431 if (element.hasLotNumberElement()) { 28432 composeStringCore("lotNumber", element.getLotNumberElement(), false); 28433 composeStringExtras("lotNumber", element.getLotNumberElement(), false); 28434 } 28435 if (element.hasManufacturerElement()) { 28436 composeStringCore("manufacturer", element.getManufacturerElement(), false); 28437 composeStringExtras("manufacturer", element.getManufacturerElement(), false); 28438 } 28439 if (element.hasManufactureDateElement()) { 28440 composeDateTimeCore("manufactureDate", element.getManufactureDateElement(), false); 28441 composeDateTimeExtras("manufactureDate", element.getManufactureDateElement(), false); 28442 } 28443 if (element.hasExpirationDateElement()) { 28444 composeDateTimeCore("expirationDate", element.getExpirationDateElement(), false); 28445 composeDateTimeExtras("expirationDate", element.getExpirationDateElement(), false); 28446 } 28447 if (element.hasModelElement()) { 28448 composeStringCore("model", element.getModelElement(), false); 28449 composeStringExtras("model", element.getModelElement(), false); 28450 } 28451 if (element.hasVersionElement()) { 28452 composeStringCore("version", element.getVersionElement(), false); 28453 composeStringExtras("version", element.getVersionElement(), false); 28454 } 28455 if (element.hasPatient()) { 28456 composeReference("patient", element.getPatient()); 28457 } 28458 if (element.hasOwner()) { 28459 composeReference("owner", element.getOwner()); 28460 } 28461 if (element.hasContact()) { 28462 openArray("contact"); 28463 for (ContactPoint e : element.getContact()) 28464 composeContactPoint(null, e); 28465 closeArray(); 28466 }; 28467 if (element.hasLocation()) { 28468 composeReference("location", element.getLocation()); 28469 } 28470 if (element.hasUrlElement()) { 28471 composeUriCore("url", element.getUrlElement(), false); 28472 composeUriExtras("url", element.getUrlElement(), false); 28473 } 28474 if (element.hasNote()) { 28475 openArray("note"); 28476 for (Annotation e : element.getNote()) 28477 composeAnnotation(null, e); 28478 closeArray(); 28479 }; 28480 if (element.hasSafety()) { 28481 openArray("safety"); 28482 for (CodeableConcept e : element.getSafety()) 28483 composeCodeableConcept(null, e); 28484 closeArray(); 28485 }; 28486 } 28487 28488 protected void composeDeviceDeviceUdiComponent(String name, Device.DeviceUdiComponent element) throws IOException { 28489 if (element != null) { 28490 open(name); 28491 composeDeviceDeviceUdiComponentInner(element); 28492 close(); 28493 } 28494 } 28495 28496 protected void composeDeviceDeviceUdiComponentInner(Device.DeviceUdiComponent element) throws IOException { 28497 composeBackbone(element); 28498 if (element.hasDeviceIdentifierElement()) { 28499 composeStringCore("deviceIdentifier", element.getDeviceIdentifierElement(), false); 28500 composeStringExtras("deviceIdentifier", element.getDeviceIdentifierElement(), false); 28501 } 28502 if (element.hasNameElement()) { 28503 composeStringCore("name", element.getNameElement(), false); 28504 composeStringExtras("name", element.getNameElement(), false); 28505 } 28506 if (element.hasJurisdictionElement()) { 28507 composeUriCore("jurisdiction", element.getJurisdictionElement(), false); 28508 composeUriExtras("jurisdiction", element.getJurisdictionElement(), false); 28509 } 28510 if (element.hasCarrierHRFElement()) { 28511 composeStringCore("carrierHRF", element.getCarrierHRFElement(), false); 28512 composeStringExtras("carrierHRF", element.getCarrierHRFElement(), false); 28513 } 28514 if (element.hasCarrierAIDCElement()) { 28515 composeBase64BinaryCore("carrierAIDC", element.getCarrierAIDCElement(), false); 28516 composeBase64BinaryExtras("carrierAIDC", element.getCarrierAIDCElement(), false); 28517 } 28518 if (element.hasIssuerElement()) { 28519 composeUriCore("issuer", element.getIssuerElement(), false); 28520 composeUriExtras("issuer", element.getIssuerElement(), false); 28521 } 28522 if (element.hasEntryTypeElement()) { 28523 composeEnumerationCore("entryType", element.getEntryTypeElement(), new Device.UDIEntryTypeEnumFactory(), false); 28524 composeEnumerationExtras("entryType", element.getEntryTypeElement(), new Device.UDIEntryTypeEnumFactory(), false); 28525 } 28526 } 28527 28528 protected void composeDeviceComponent(String name, DeviceComponent element) throws IOException { 28529 if (element != null) { 28530 prop("resourceType", name); 28531 composeDeviceComponentInner(element); 28532 } 28533 } 28534 28535 protected void composeDeviceComponentInner(DeviceComponent element) throws IOException { 28536 composeDomainResourceElements(element); 28537 if (element.hasIdentifier()) { 28538 composeIdentifier("identifier", element.getIdentifier()); 28539 } 28540 if (element.hasType()) { 28541 composeCodeableConcept("type", element.getType()); 28542 } 28543 if (element.hasLastSystemChangeElement()) { 28544 composeInstantCore("lastSystemChange", element.getLastSystemChangeElement(), false); 28545 composeInstantExtras("lastSystemChange", element.getLastSystemChangeElement(), false); 28546 } 28547 if (element.hasSource()) { 28548 composeReference("source", element.getSource()); 28549 } 28550 if (element.hasParent()) { 28551 composeReference("parent", element.getParent()); 28552 } 28553 if (element.hasOperationalStatus()) { 28554 openArray("operationalStatus"); 28555 for (CodeableConcept e : element.getOperationalStatus()) 28556 composeCodeableConcept(null, e); 28557 closeArray(); 28558 }; 28559 if (element.hasParameterGroup()) { 28560 composeCodeableConcept("parameterGroup", element.getParameterGroup()); 28561 } 28562 if (element.hasMeasurementPrincipleElement()) { 28563 composeEnumerationCore("measurementPrinciple", element.getMeasurementPrincipleElement(), new DeviceComponent.MeasmntPrincipleEnumFactory(), false); 28564 composeEnumerationExtras("measurementPrinciple", element.getMeasurementPrincipleElement(), new DeviceComponent.MeasmntPrincipleEnumFactory(), false); 28565 } 28566 if (element.hasProductionSpecification()) { 28567 openArray("productionSpecification"); 28568 for (DeviceComponent.DeviceComponentProductionSpecificationComponent e : element.getProductionSpecification()) 28569 composeDeviceComponentDeviceComponentProductionSpecificationComponent(null, e); 28570 closeArray(); 28571 }; 28572 if (element.hasLanguageCode()) { 28573 composeCodeableConcept("languageCode", element.getLanguageCode()); 28574 } 28575 } 28576 28577 protected void composeDeviceComponentDeviceComponentProductionSpecificationComponent(String name, DeviceComponent.DeviceComponentProductionSpecificationComponent element) throws IOException { 28578 if (element != null) { 28579 open(name); 28580 composeDeviceComponentDeviceComponentProductionSpecificationComponentInner(element); 28581 close(); 28582 } 28583 } 28584 28585 protected void composeDeviceComponentDeviceComponentProductionSpecificationComponentInner(DeviceComponent.DeviceComponentProductionSpecificationComponent element) throws IOException { 28586 composeBackbone(element); 28587 if (element.hasSpecType()) { 28588 composeCodeableConcept("specType", element.getSpecType()); 28589 } 28590 if (element.hasComponentId()) { 28591 composeIdentifier("componentId", element.getComponentId()); 28592 } 28593 if (element.hasProductionSpecElement()) { 28594 composeStringCore("productionSpec", element.getProductionSpecElement(), false); 28595 composeStringExtras("productionSpec", element.getProductionSpecElement(), false); 28596 } 28597 } 28598 28599 protected void composeDeviceMetric(String name, DeviceMetric element) throws IOException { 28600 if (element != null) { 28601 prop("resourceType", name); 28602 composeDeviceMetricInner(element); 28603 } 28604 } 28605 28606 protected void composeDeviceMetricInner(DeviceMetric element) throws IOException { 28607 composeDomainResourceElements(element); 28608 if (element.hasIdentifier()) { 28609 composeIdentifier("identifier", element.getIdentifier()); 28610 } 28611 if (element.hasType()) { 28612 composeCodeableConcept("type", element.getType()); 28613 } 28614 if (element.hasUnit()) { 28615 composeCodeableConcept("unit", element.getUnit()); 28616 } 28617 if (element.hasSource()) { 28618 composeReference("source", element.getSource()); 28619 } 28620 if (element.hasParent()) { 28621 composeReference("parent", element.getParent()); 28622 } 28623 if (element.hasOperationalStatusElement()) { 28624 composeEnumerationCore("operationalStatus", element.getOperationalStatusElement(), new DeviceMetric.DeviceMetricOperationalStatusEnumFactory(), false); 28625 composeEnumerationExtras("operationalStatus", element.getOperationalStatusElement(), new DeviceMetric.DeviceMetricOperationalStatusEnumFactory(), false); 28626 } 28627 if (element.hasColorElement()) { 28628 composeEnumerationCore("color", element.getColorElement(), new DeviceMetric.DeviceMetricColorEnumFactory(), false); 28629 composeEnumerationExtras("color", element.getColorElement(), new DeviceMetric.DeviceMetricColorEnumFactory(), false); 28630 } 28631 if (element.hasCategoryElement()) { 28632 composeEnumerationCore("category", element.getCategoryElement(), new DeviceMetric.DeviceMetricCategoryEnumFactory(), false); 28633 composeEnumerationExtras("category", element.getCategoryElement(), new DeviceMetric.DeviceMetricCategoryEnumFactory(), false); 28634 } 28635 if (element.hasMeasurementPeriod()) { 28636 composeTiming("measurementPeriod", element.getMeasurementPeriod()); 28637 } 28638 if (element.hasCalibration()) { 28639 openArray("calibration"); 28640 for (DeviceMetric.DeviceMetricCalibrationComponent e : element.getCalibration()) 28641 composeDeviceMetricDeviceMetricCalibrationComponent(null, e); 28642 closeArray(); 28643 }; 28644 } 28645 28646 protected void composeDeviceMetricDeviceMetricCalibrationComponent(String name, DeviceMetric.DeviceMetricCalibrationComponent element) throws IOException { 28647 if (element != null) { 28648 open(name); 28649 composeDeviceMetricDeviceMetricCalibrationComponentInner(element); 28650 close(); 28651 } 28652 } 28653 28654 protected void composeDeviceMetricDeviceMetricCalibrationComponentInner(DeviceMetric.DeviceMetricCalibrationComponent element) throws IOException { 28655 composeBackbone(element); 28656 if (element.hasTypeElement()) { 28657 composeEnumerationCore("type", element.getTypeElement(), new DeviceMetric.DeviceMetricCalibrationTypeEnumFactory(), false); 28658 composeEnumerationExtras("type", element.getTypeElement(), new DeviceMetric.DeviceMetricCalibrationTypeEnumFactory(), false); 28659 } 28660 if (element.hasStateElement()) { 28661 composeEnumerationCore("state", element.getStateElement(), new DeviceMetric.DeviceMetricCalibrationStateEnumFactory(), false); 28662 composeEnumerationExtras("state", element.getStateElement(), new DeviceMetric.DeviceMetricCalibrationStateEnumFactory(), false); 28663 } 28664 if (element.hasTimeElement()) { 28665 composeInstantCore("time", element.getTimeElement(), false); 28666 composeInstantExtras("time", element.getTimeElement(), false); 28667 } 28668 } 28669 28670 protected void composeDeviceRequest(String name, DeviceRequest element) throws IOException { 28671 if (element != null) { 28672 prop("resourceType", name); 28673 composeDeviceRequestInner(element); 28674 } 28675 } 28676 28677 protected void composeDeviceRequestInner(DeviceRequest element) throws IOException { 28678 composeDomainResourceElements(element); 28679 if (element.hasIdentifier()) { 28680 openArray("identifier"); 28681 for (Identifier e : element.getIdentifier()) 28682 composeIdentifier(null, e); 28683 closeArray(); 28684 }; 28685 if (element.hasDefinition()) { 28686 openArray("definition"); 28687 for (Reference e : element.getDefinition()) 28688 composeReference(null, e); 28689 closeArray(); 28690 }; 28691 if (element.hasBasedOn()) { 28692 openArray("basedOn"); 28693 for (Reference e : element.getBasedOn()) 28694 composeReference(null, e); 28695 closeArray(); 28696 }; 28697 if (element.hasPriorRequest()) { 28698 openArray("priorRequest"); 28699 for (Reference e : element.getPriorRequest()) 28700 composeReference(null, e); 28701 closeArray(); 28702 }; 28703 if (element.hasGroupIdentifier()) { 28704 composeIdentifier("groupIdentifier", element.getGroupIdentifier()); 28705 } 28706 if (element.hasStatusElement()) { 28707 composeEnumerationCore("status", element.getStatusElement(), new DeviceRequest.DeviceRequestStatusEnumFactory(), false); 28708 composeEnumerationExtras("status", element.getStatusElement(), new DeviceRequest.DeviceRequestStatusEnumFactory(), false); 28709 } 28710 if (element.hasIntent()) { 28711 composeCodeableConcept("intent", element.getIntent()); 28712 } 28713 if (element.hasPriorityElement()) { 28714 composeEnumerationCore("priority", element.getPriorityElement(), new DeviceRequest.RequestPriorityEnumFactory(), false); 28715 composeEnumerationExtras("priority", element.getPriorityElement(), new DeviceRequest.RequestPriorityEnumFactory(), false); 28716 } 28717 if (element.hasCode()) { 28718 composeType("code", element.getCode()); 28719 } 28720 if (element.hasSubject()) { 28721 composeReference("subject", element.getSubject()); 28722 } 28723 if (element.hasContext()) { 28724 composeReference("context", element.getContext()); 28725 } 28726 if (element.hasOccurrence()) { 28727 composeType("occurrence", element.getOccurrence()); 28728 } 28729 if (element.hasAuthoredOnElement()) { 28730 composeDateTimeCore("authoredOn", element.getAuthoredOnElement(), false); 28731 composeDateTimeExtras("authoredOn", element.getAuthoredOnElement(), false); 28732 } 28733 if (element.hasRequester()) { 28734 composeDeviceRequestDeviceRequestRequesterComponent("requester", element.getRequester()); 28735 } 28736 if (element.hasPerformerType()) { 28737 composeCodeableConcept("performerType", element.getPerformerType()); 28738 } 28739 if (element.hasPerformer()) { 28740 composeReference("performer", element.getPerformer()); 28741 } 28742 if (element.hasReasonCode()) { 28743 openArray("reasonCode"); 28744 for (CodeableConcept e : element.getReasonCode()) 28745 composeCodeableConcept(null, e); 28746 closeArray(); 28747 }; 28748 if (element.hasReasonReference()) { 28749 openArray("reasonReference"); 28750 for (Reference e : element.getReasonReference()) 28751 composeReference(null, e); 28752 closeArray(); 28753 }; 28754 if (element.hasSupportingInfo()) { 28755 openArray("supportingInfo"); 28756 for (Reference e : element.getSupportingInfo()) 28757 composeReference(null, e); 28758 closeArray(); 28759 }; 28760 if (element.hasNote()) { 28761 openArray("note"); 28762 for (Annotation e : element.getNote()) 28763 composeAnnotation(null, e); 28764 closeArray(); 28765 }; 28766 if (element.hasRelevantHistory()) { 28767 openArray("relevantHistory"); 28768 for (Reference e : element.getRelevantHistory()) 28769 composeReference(null, e); 28770 closeArray(); 28771 }; 28772 } 28773 28774 protected void composeDeviceRequestDeviceRequestRequesterComponent(String name, DeviceRequest.DeviceRequestRequesterComponent element) throws IOException { 28775 if (element != null) { 28776 open(name); 28777 composeDeviceRequestDeviceRequestRequesterComponentInner(element); 28778 close(); 28779 } 28780 } 28781 28782 protected void composeDeviceRequestDeviceRequestRequesterComponentInner(DeviceRequest.DeviceRequestRequesterComponent element) throws IOException { 28783 composeBackbone(element); 28784 if (element.hasAgent()) { 28785 composeReference("agent", element.getAgent()); 28786 } 28787 if (element.hasOnBehalfOf()) { 28788 composeReference("onBehalfOf", element.getOnBehalfOf()); 28789 } 28790 } 28791 28792 protected void composeDeviceUseStatement(String name, DeviceUseStatement element) throws IOException { 28793 if (element != null) { 28794 prop("resourceType", name); 28795 composeDeviceUseStatementInner(element); 28796 } 28797 } 28798 28799 protected void composeDeviceUseStatementInner(DeviceUseStatement element) throws IOException { 28800 composeDomainResourceElements(element); 28801 if (element.hasIdentifier()) { 28802 openArray("identifier"); 28803 for (Identifier e : element.getIdentifier()) 28804 composeIdentifier(null, e); 28805 closeArray(); 28806 }; 28807 if (element.hasStatusElement()) { 28808 composeEnumerationCore("status", element.getStatusElement(), new DeviceUseStatement.DeviceUseStatementStatusEnumFactory(), false); 28809 composeEnumerationExtras("status", element.getStatusElement(), new DeviceUseStatement.DeviceUseStatementStatusEnumFactory(), false); 28810 } 28811 if (element.hasSubject()) { 28812 composeReference("subject", element.getSubject()); 28813 } 28814 if (element.hasWhenUsed()) { 28815 composePeriod("whenUsed", element.getWhenUsed()); 28816 } 28817 if (element.hasTiming()) { 28818 composeType("timing", element.getTiming()); 28819 } 28820 if (element.hasRecordedOnElement()) { 28821 composeDateTimeCore("recordedOn", element.getRecordedOnElement(), false); 28822 composeDateTimeExtras("recordedOn", element.getRecordedOnElement(), false); 28823 } 28824 if (element.hasSource()) { 28825 composeReference("source", element.getSource()); 28826 } 28827 if (element.hasDevice()) { 28828 composeReference("device", element.getDevice()); 28829 } 28830 if (element.hasIndication()) { 28831 openArray("indication"); 28832 for (CodeableConcept e : element.getIndication()) 28833 composeCodeableConcept(null, e); 28834 closeArray(); 28835 }; 28836 if (element.hasBodySite()) { 28837 composeCodeableConcept("bodySite", element.getBodySite()); 28838 } 28839 if (element.hasNote()) { 28840 openArray("note"); 28841 for (Annotation e : element.getNote()) 28842 composeAnnotation(null, e); 28843 closeArray(); 28844 }; 28845 } 28846 28847 protected void composeDiagnosticReport(String name, DiagnosticReport element) throws IOException { 28848 if (element != null) { 28849 prop("resourceType", name); 28850 composeDiagnosticReportInner(element); 28851 } 28852 } 28853 28854 protected void composeDiagnosticReportInner(DiagnosticReport element) throws IOException { 28855 composeDomainResourceElements(element); 28856 if (element.hasIdentifier()) { 28857 openArray("identifier"); 28858 for (Identifier e : element.getIdentifier()) 28859 composeIdentifier(null, e); 28860 closeArray(); 28861 }; 28862 if (element.hasBasedOn()) { 28863 openArray("basedOn"); 28864 for (Reference e : element.getBasedOn()) 28865 composeReference(null, e); 28866 closeArray(); 28867 }; 28868 if (element.hasStatusElement()) { 28869 composeEnumerationCore("status", element.getStatusElement(), new DiagnosticReport.DiagnosticReportStatusEnumFactory(), false); 28870 composeEnumerationExtras("status", element.getStatusElement(), new DiagnosticReport.DiagnosticReportStatusEnumFactory(), false); 28871 } 28872 if (element.hasCategory()) { 28873 composeCodeableConcept("category", element.getCategory()); 28874 } 28875 if (element.hasCode()) { 28876 composeCodeableConcept("code", element.getCode()); 28877 } 28878 if (element.hasSubject()) { 28879 composeReference("subject", element.getSubject()); 28880 } 28881 if (element.hasContext()) { 28882 composeReference("context", element.getContext()); 28883 } 28884 if (element.hasEffective()) { 28885 composeType("effective", element.getEffective()); 28886 } 28887 if (element.hasIssuedElement()) { 28888 composeInstantCore("issued", element.getIssuedElement(), false); 28889 composeInstantExtras("issued", element.getIssuedElement(), false); 28890 } 28891 if (element.hasPerformer()) { 28892 openArray("performer"); 28893 for (DiagnosticReport.DiagnosticReportPerformerComponent e : element.getPerformer()) 28894 composeDiagnosticReportDiagnosticReportPerformerComponent(null, e); 28895 closeArray(); 28896 }; 28897 if (element.hasSpecimen()) { 28898 openArray("specimen"); 28899 for (Reference e : element.getSpecimen()) 28900 composeReference(null, e); 28901 closeArray(); 28902 }; 28903 if (element.hasResult()) { 28904 openArray("result"); 28905 for (Reference e : element.getResult()) 28906 composeReference(null, e); 28907 closeArray(); 28908 }; 28909 if (element.hasImagingStudy()) { 28910 openArray("imagingStudy"); 28911 for (Reference e : element.getImagingStudy()) 28912 composeReference(null, e); 28913 closeArray(); 28914 }; 28915 if (element.hasImage()) { 28916 openArray("image"); 28917 for (DiagnosticReport.DiagnosticReportImageComponent e : element.getImage()) 28918 composeDiagnosticReportDiagnosticReportImageComponent(null, e); 28919 closeArray(); 28920 }; 28921 if (element.hasConclusionElement()) { 28922 composeStringCore("conclusion", element.getConclusionElement(), false); 28923 composeStringExtras("conclusion", element.getConclusionElement(), false); 28924 } 28925 if (element.hasCodedDiagnosis()) { 28926 openArray("codedDiagnosis"); 28927 for (CodeableConcept e : element.getCodedDiagnosis()) 28928 composeCodeableConcept(null, e); 28929 closeArray(); 28930 }; 28931 if (element.hasPresentedForm()) { 28932 openArray("presentedForm"); 28933 for (Attachment e : element.getPresentedForm()) 28934 composeAttachment(null, e); 28935 closeArray(); 28936 }; 28937 } 28938 28939 protected void composeDiagnosticReportDiagnosticReportPerformerComponent(String name, DiagnosticReport.DiagnosticReportPerformerComponent element) throws IOException { 28940 if (element != null) { 28941 open(name); 28942 composeDiagnosticReportDiagnosticReportPerformerComponentInner(element); 28943 close(); 28944 } 28945 } 28946 28947 protected void composeDiagnosticReportDiagnosticReportPerformerComponentInner(DiagnosticReport.DiagnosticReportPerformerComponent element) throws IOException { 28948 composeBackbone(element); 28949 if (element.hasRole()) { 28950 composeCodeableConcept("role", element.getRole()); 28951 } 28952 if (element.hasActor()) { 28953 composeReference("actor", element.getActor()); 28954 } 28955 } 28956 28957 protected void composeDiagnosticReportDiagnosticReportImageComponent(String name, DiagnosticReport.DiagnosticReportImageComponent element) throws IOException { 28958 if (element != null) { 28959 open(name); 28960 composeDiagnosticReportDiagnosticReportImageComponentInner(element); 28961 close(); 28962 } 28963 } 28964 28965 protected void composeDiagnosticReportDiagnosticReportImageComponentInner(DiagnosticReport.DiagnosticReportImageComponent element) throws IOException { 28966 composeBackbone(element); 28967 if (element.hasCommentElement()) { 28968 composeStringCore("comment", element.getCommentElement(), false); 28969 composeStringExtras("comment", element.getCommentElement(), false); 28970 } 28971 if (element.hasLink()) { 28972 composeReference("link", element.getLink()); 28973 } 28974 } 28975 28976 protected void composeDocumentManifest(String name, DocumentManifest element) throws IOException { 28977 if (element != null) { 28978 prop("resourceType", name); 28979 composeDocumentManifestInner(element); 28980 } 28981 } 28982 28983 protected void composeDocumentManifestInner(DocumentManifest element) throws IOException { 28984 composeDomainResourceElements(element); 28985 if (element.hasMasterIdentifier()) { 28986 composeIdentifier("masterIdentifier", element.getMasterIdentifier()); 28987 } 28988 if (element.hasIdentifier()) { 28989 openArray("identifier"); 28990 for (Identifier e : element.getIdentifier()) 28991 composeIdentifier(null, e); 28992 closeArray(); 28993 }; 28994 if (element.hasStatusElement()) { 28995 composeEnumerationCore("status", element.getStatusElement(), new Enumerations.DocumentReferenceStatusEnumFactory(), false); 28996 composeEnumerationExtras("status", element.getStatusElement(), new Enumerations.DocumentReferenceStatusEnumFactory(), false); 28997 } 28998 if (element.hasType()) { 28999 composeCodeableConcept("type", element.getType()); 29000 } 29001 if (element.hasSubject()) { 29002 composeReference("subject", element.getSubject()); 29003 } 29004 if (element.hasCreatedElement()) { 29005 composeDateTimeCore("created", element.getCreatedElement(), false); 29006 composeDateTimeExtras("created", element.getCreatedElement(), false); 29007 } 29008 if (element.hasAuthor()) { 29009 openArray("author"); 29010 for (Reference e : element.getAuthor()) 29011 composeReference(null, e); 29012 closeArray(); 29013 }; 29014 if (element.hasRecipient()) { 29015 openArray("recipient"); 29016 for (Reference e : element.getRecipient()) 29017 composeReference(null, e); 29018 closeArray(); 29019 }; 29020 if (element.hasSourceElement()) { 29021 composeUriCore("source", element.getSourceElement(), false); 29022 composeUriExtras("source", element.getSourceElement(), false); 29023 } 29024 if (element.hasDescriptionElement()) { 29025 composeStringCore("description", element.getDescriptionElement(), false); 29026 composeStringExtras("description", element.getDescriptionElement(), false); 29027 } 29028 if (element.hasContent()) { 29029 openArray("content"); 29030 for (DocumentManifest.DocumentManifestContentComponent e : element.getContent()) 29031 composeDocumentManifestDocumentManifestContentComponent(null, e); 29032 closeArray(); 29033 }; 29034 if (element.hasRelated()) { 29035 openArray("related"); 29036 for (DocumentManifest.DocumentManifestRelatedComponent e : element.getRelated()) 29037 composeDocumentManifestDocumentManifestRelatedComponent(null, e); 29038 closeArray(); 29039 }; 29040 } 29041 29042 protected void composeDocumentManifestDocumentManifestContentComponent(String name, DocumentManifest.DocumentManifestContentComponent element) throws IOException { 29043 if (element != null) { 29044 open(name); 29045 composeDocumentManifestDocumentManifestContentComponentInner(element); 29046 close(); 29047 } 29048 } 29049 29050 protected void composeDocumentManifestDocumentManifestContentComponentInner(DocumentManifest.DocumentManifestContentComponent element) throws IOException { 29051 composeBackbone(element); 29052 if (element.hasP()) { 29053 composeType("p", element.getP()); 29054 } 29055 } 29056 29057 protected void composeDocumentManifestDocumentManifestRelatedComponent(String name, DocumentManifest.DocumentManifestRelatedComponent element) throws IOException { 29058 if (element != null) { 29059 open(name); 29060 composeDocumentManifestDocumentManifestRelatedComponentInner(element); 29061 close(); 29062 } 29063 } 29064 29065 protected void composeDocumentManifestDocumentManifestRelatedComponentInner(DocumentManifest.DocumentManifestRelatedComponent element) throws IOException { 29066 composeBackbone(element); 29067 if (element.hasIdentifier()) { 29068 composeIdentifier("identifier", element.getIdentifier()); 29069 } 29070 if (element.hasRef()) { 29071 composeReference("ref", element.getRef()); 29072 } 29073 } 29074 29075 protected void composeDocumentReference(String name, DocumentReference element) throws IOException { 29076 if (element != null) { 29077 prop("resourceType", name); 29078 composeDocumentReferenceInner(element); 29079 } 29080 } 29081 29082 protected void composeDocumentReferenceInner(DocumentReference element) throws IOException { 29083 composeDomainResourceElements(element); 29084 if (element.hasMasterIdentifier()) { 29085 composeIdentifier("masterIdentifier", element.getMasterIdentifier()); 29086 } 29087 if (element.hasIdentifier()) { 29088 openArray("identifier"); 29089 for (Identifier e : element.getIdentifier()) 29090 composeIdentifier(null, e); 29091 closeArray(); 29092 }; 29093 if (element.hasStatusElement()) { 29094 composeEnumerationCore("status", element.getStatusElement(), new Enumerations.DocumentReferenceStatusEnumFactory(), false); 29095 composeEnumerationExtras("status", element.getStatusElement(), new Enumerations.DocumentReferenceStatusEnumFactory(), false); 29096 } 29097 if (element.hasDocStatusElement()) { 29098 composeEnumerationCore("docStatus", element.getDocStatusElement(), new DocumentReference.ReferredDocumentStatusEnumFactory(), false); 29099 composeEnumerationExtras("docStatus", element.getDocStatusElement(), new DocumentReference.ReferredDocumentStatusEnumFactory(), false); 29100 } 29101 if (element.hasType()) { 29102 composeCodeableConcept("type", element.getType()); 29103 } 29104 if (element.hasClass_()) { 29105 composeCodeableConcept("class", element.getClass_()); 29106 } 29107 if (element.hasSubject()) { 29108 composeReference("subject", element.getSubject()); 29109 } 29110 if (element.hasCreatedElement()) { 29111 composeDateTimeCore("created", element.getCreatedElement(), false); 29112 composeDateTimeExtras("created", element.getCreatedElement(), false); 29113 } 29114 if (element.hasIndexedElement()) { 29115 composeInstantCore("indexed", element.getIndexedElement(), false); 29116 composeInstantExtras("indexed", element.getIndexedElement(), false); 29117 } 29118 if (element.hasAuthor()) { 29119 openArray("author"); 29120 for (Reference e : element.getAuthor()) 29121 composeReference(null, e); 29122 closeArray(); 29123 }; 29124 if (element.hasAuthenticator()) { 29125 composeReference("authenticator", element.getAuthenticator()); 29126 } 29127 if (element.hasCustodian()) { 29128 composeReference("custodian", element.getCustodian()); 29129 } 29130 if (element.hasRelatesTo()) { 29131 openArray("relatesTo"); 29132 for (DocumentReference.DocumentReferenceRelatesToComponent e : element.getRelatesTo()) 29133 composeDocumentReferenceDocumentReferenceRelatesToComponent(null, e); 29134 closeArray(); 29135 }; 29136 if (element.hasDescriptionElement()) { 29137 composeStringCore("description", element.getDescriptionElement(), false); 29138 composeStringExtras("description", element.getDescriptionElement(), false); 29139 } 29140 if (element.hasSecurityLabel()) { 29141 openArray("securityLabel"); 29142 for (CodeableConcept e : element.getSecurityLabel()) 29143 composeCodeableConcept(null, e); 29144 closeArray(); 29145 }; 29146 if (element.hasContent()) { 29147 openArray("content"); 29148 for (DocumentReference.DocumentReferenceContentComponent e : element.getContent()) 29149 composeDocumentReferenceDocumentReferenceContentComponent(null, e); 29150 closeArray(); 29151 }; 29152 if (element.hasContext()) { 29153 composeDocumentReferenceDocumentReferenceContextComponent("context", element.getContext()); 29154 } 29155 } 29156 29157 protected void composeDocumentReferenceDocumentReferenceRelatesToComponent(String name, DocumentReference.DocumentReferenceRelatesToComponent element) throws IOException { 29158 if (element != null) { 29159 open(name); 29160 composeDocumentReferenceDocumentReferenceRelatesToComponentInner(element); 29161 close(); 29162 } 29163 } 29164 29165 protected void composeDocumentReferenceDocumentReferenceRelatesToComponentInner(DocumentReference.DocumentReferenceRelatesToComponent element) throws IOException { 29166 composeBackbone(element); 29167 if (element.hasCodeElement()) { 29168 composeEnumerationCore("code", element.getCodeElement(), new DocumentReference.DocumentRelationshipTypeEnumFactory(), false); 29169 composeEnumerationExtras("code", element.getCodeElement(), new DocumentReference.DocumentRelationshipTypeEnumFactory(), false); 29170 } 29171 if (element.hasTarget()) { 29172 composeReference("target", element.getTarget()); 29173 } 29174 } 29175 29176 protected void composeDocumentReferenceDocumentReferenceContentComponent(String name, DocumentReference.DocumentReferenceContentComponent element) throws IOException { 29177 if (element != null) { 29178 open(name); 29179 composeDocumentReferenceDocumentReferenceContentComponentInner(element); 29180 close(); 29181 } 29182 } 29183 29184 protected void composeDocumentReferenceDocumentReferenceContentComponentInner(DocumentReference.DocumentReferenceContentComponent element) throws IOException { 29185 composeBackbone(element); 29186 if (element.hasAttachment()) { 29187 composeAttachment("attachment", element.getAttachment()); 29188 } 29189 if (element.hasFormat()) { 29190 composeCoding("format", element.getFormat()); 29191 } 29192 } 29193 29194 protected void composeDocumentReferenceDocumentReferenceContextComponent(String name, DocumentReference.DocumentReferenceContextComponent element) throws IOException { 29195 if (element != null) { 29196 open(name); 29197 composeDocumentReferenceDocumentReferenceContextComponentInner(element); 29198 close(); 29199 } 29200 } 29201 29202 protected void composeDocumentReferenceDocumentReferenceContextComponentInner(DocumentReference.DocumentReferenceContextComponent element) throws IOException { 29203 composeBackbone(element); 29204 if (element.hasEncounter()) { 29205 composeReference("encounter", element.getEncounter()); 29206 } 29207 if (element.hasEvent()) { 29208 openArray("event"); 29209 for (CodeableConcept e : element.getEvent()) 29210 composeCodeableConcept(null, e); 29211 closeArray(); 29212 }; 29213 if (element.hasPeriod()) { 29214 composePeriod("period", element.getPeriod()); 29215 } 29216 if (element.hasFacilityType()) { 29217 composeCodeableConcept("facilityType", element.getFacilityType()); 29218 } 29219 if (element.hasPracticeSetting()) { 29220 composeCodeableConcept("practiceSetting", element.getPracticeSetting()); 29221 } 29222 if (element.hasSourcePatientInfo()) { 29223 composeReference("sourcePatientInfo", element.getSourcePatientInfo()); 29224 } 29225 if (element.hasRelated()) { 29226 openArray("related"); 29227 for (DocumentReference.DocumentReferenceContextRelatedComponent e : element.getRelated()) 29228 composeDocumentReferenceDocumentReferenceContextRelatedComponent(null, e); 29229 closeArray(); 29230 }; 29231 } 29232 29233 protected void composeDocumentReferenceDocumentReferenceContextRelatedComponent(String name, DocumentReference.DocumentReferenceContextRelatedComponent element) throws IOException { 29234 if (element != null) { 29235 open(name); 29236 composeDocumentReferenceDocumentReferenceContextRelatedComponentInner(element); 29237 close(); 29238 } 29239 } 29240 29241 protected void composeDocumentReferenceDocumentReferenceContextRelatedComponentInner(DocumentReference.DocumentReferenceContextRelatedComponent element) throws IOException { 29242 composeBackbone(element); 29243 if (element.hasIdentifier()) { 29244 composeIdentifier("identifier", element.getIdentifier()); 29245 } 29246 if (element.hasRef()) { 29247 composeReference("ref", element.getRef()); 29248 } 29249 } 29250 29251 protected void composeEligibilityRequest(String name, EligibilityRequest element) throws IOException { 29252 if (element != null) { 29253 prop("resourceType", name); 29254 composeEligibilityRequestInner(element); 29255 } 29256 } 29257 29258 protected void composeEligibilityRequestInner(EligibilityRequest element) throws IOException { 29259 composeDomainResourceElements(element); 29260 if (element.hasIdentifier()) { 29261 openArray("identifier"); 29262 for (Identifier e : element.getIdentifier()) 29263 composeIdentifier(null, e); 29264 closeArray(); 29265 }; 29266 if (element.hasStatusElement()) { 29267 composeEnumerationCore("status", element.getStatusElement(), new EligibilityRequest.EligibilityRequestStatusEnumFactory(), false); 29268 composeEnumerationExtras("status", element.getStatusElement(), new EligibilityRequest.EligibilityRequestStatusEnumFactory(), false); 29269 } 29270 if (element.hasPriority()) { 29271 composeCodeableConcept("priority", element.getPriority()); 29272 } 29273 if (element.hasPatient()) { 29274 composeReference("patient", element.getPatient()); 29275 } 29276 if (element.hasServiced()) { 29277 composeType("serviced", element.getServiced()); 29278 } 29279 if (element.hasCreatedElement()) { 29280 composeDateTimeCore("created", element.getCreatedElement(), false); 29281 composeDateTimeExtras("created", element.getCreatedElement(), false); 29282 } 29283 if (element.hasEnterer()) { 29284 composeReference("enterer", element.getEnterer()); 29285 } 29286 if (element.hasProvider()) { 29287 composeReference("provider", element.getProvider()); 29288 } 29289 if (element.hasOrganization()) { 29290 composeReference("organization", element.getOrganization()); 29291 } 29292 if (element.hasInsurer()) { 29293 composeReference("insurer", element.getInsurer()); 29294 } 29295 if (element.hasFacility()) { 29296 composeReference("facility", element.getFacility()); 29297 } 29298 if (element.hasCoverage()) { 29299 composeReference("coverage", element.getCoverage()); 29300 } 29301 if (element.hasBusinessArrangementElement()) { 29302 composeStringCore("businessArrangement", element.getBusinessArrangementElement(), false); 29303 composeStringExtras("businessArrangement", element.getBusinessArrangementElement(), false); 29304 } 29305 if (element.hasBenefitCategory()) { 29306 composeCodeableConcept("benefitCategory", element.getBenefitCategory()); 29307 } 29308 if (element.hasBenefitSubCategory()) { 29309 composeCodeableConcept("benefitSubCategory", element.getBenefitSubCategory()); 29310 } 29311 } 29312 29313 protected void composeEligibilityResponse(String name, EligibilityResponse element) throws IOException { 29314 if (element != null) { 29315 prop("resourceType", name); 29316 composeEligibilityResponseInner(element); 29317 } 29318 } 29319 29320 protected void composeEligibilityResponseInner(EligibilityResponse element) throws IOException { 29321 composeDomainResourceElements(element); 29322 if (element.hasIdentifier()) { 29323 openArray("identifier"); 29324 for (Identifier e : element.getIdentifier()) 29325 composeIdentifier(null, e); 29326 closeArray(); 29327 }; 29328 if (element.hasStatusElement()) { 29329 composeEnumerationCore("status", element.getStatusElement(), new EligibilityResponse.EligibilityResponseStatusEnumFactory(), false); 29330 composeEnumerationExtras("status", element.getStatusElement(), new EligibilityResponse.EligibilityResponseStatusEnumFactory(), false); 29331 } 29332 if (element.hasCreatedElement()) { 29333 composeDateTimeCore("created", element.getCreatedElement(), false); 29334 composeDateTimeExtras("created", element.getCreatedElement(), false); 29335 } 29336 if (element.hasRequestProvider()) { 29337 composeReference("requestProvider", element.getRequestProvider()); 29338 } 29339 if (element.hasRequestOrganization()) { 29340 composeReference("requestOrganization", element.getRequestOrganization()); 29341 } 29342 if (element.hasRequest()) { 29343 composeReference("request", element.getRequest()); 29344 } 29345 if (element.hasOutcome()) { 29346 composeCodeableConcept("outcome", element.getOutcome()); 29347 } 29348 if (element.hasDispositionElement()) { 29349 composeStringCore("disposition", element.getDispositionElement(), false); 29350 composeStringExtras("disposition", element.getDispositionElement(), false); 29351 } 29352 if (element.hasInsurer()) { 29353 composeReference("insurer", element.getInsurer()); 29354 } 29355 if (element.hasInforceElement()) { 29356 composeBooleanCore("inforce", element.getInforceElement(), false); 29357 composeBooleanExtras("inforce", element.getInforceElement(), false); 29358 } 29359 if (element.hasInsurance()) { 29360 openArray("insurance"); 29361 for (EligibilityResponse.InsuranceComponent e : element.getInsurance()) 29362 composeEligibilityResponseInsuranceComponent(null, e); 29363 closeArray(); 29364 }; 29365 if (element.hasForm()) { 29366 composeCodeableConcept("form", element.getForm()); 29367 } 29368 if (element.hasError()) { 29369 openArray("error"); 29370 for (EligibilityResponse.ErrorsComponent e : element.getError()) 29371 composeEligibilityResponseErrorsComponent(null, e); 29372 closeArray(); 29373 }; 29374 } 29375 29376 protected void composeEligibilityResponseInsuranceComponent(String name, EligibilityResponse.InsuranceComponent element) throws IOException { 29377 if (element != null) { 29378 open(name); 29379 composeEligibilityResponseInsuranceComponentInner(element); 29380 close(); 29381 } 29382 } 29383 29384 protected void composeEligibilityResponseInsuranceComponentInner(EligibilityResponse.InsuranceComponent element) throws IOException { 29385 composeBackbone(element); 29386 if (element.hasCoverage()) { 29387 composeReference("coverage", element.getCoverage()); 29388 } 29389 if (element.hasContract()) { 29390 composeReference("contract", element.getContract()); 29391 } 29392 if (element.hasBenefitBalance()) { 29393 openArray("benefitBalance"); 29394 for (EligibilityResponse.BenefitsComponent e : element.getBenefitBalance()) 29395 composeEligibilityResponseBenefitsComponent(null, e); 29396 closeArray(); 29397 }; 29398 } 29399 29400 protected void composeEligibilityResponseBenefitsComponent(String name, EligibilityResponse.BenefitsComponent element) throws IOException { 29401 if (element != null) { 29402 open(name); 29403 composeEligibilityResponseBenefitsComponentInner(element); 29404 close(); 29405 } 29406 } 29407 29408 protected void composeEligibilityResponseBenefitsComponentInner(EligibilityResponse.BenefitsComponent element) throws IOException { 29409 composeBackbone(element); 29410 if (element.hasCategory()) { 29411 composeCodeableConcept("category", element.getCategory()); 29412 } 29413 if (element.hasSubCategory()) { 29414 composeCodeableConcept("subCategory", element.getSubCategory()); 29415 } 29416 if (element.hasExcludedElement()) { 29417 composeBooleanCore("excluded", element.getExcludedElement(), false); 29418 composeBooleanExtras("excluded", element.getExcludedElement(), false); 29419 } 29420 if (element.hasNameElement()) { 29421 composeStringCore("name", element.getNameElement(), false); 29422 composeStringExtras("name", element.getNameElement(), false); 29423 } 29424 if (element.hasDescriptionElement()) { 29425 composeStringCore("description", element.getDescriptionElement(), false); 29426 composeStringExtras("description", element.getDescriptionElement(), false); 29427 } 29428 if (element.hasNetwork()) { 29429 composeCodeableConcept("network", element.getNetwork()); 29430 } 29431 if (element.hasUnit()) { 29432 composeCodeableConcept("unit", element.getUnit()); 29433 } 29434 if (element.hasTerm()) { 29435 composeCodeableConcept("term", element.getTerm()); 29436 } 29437 if (element.hasFinancial()) { 29438 openArray("financial"); 29439 for (EligibilityResponse.BenefitComponent e : element.getFinancial()) 29440 composeEligibilityResponseBenefitComponent(null, e); 29441 closeArray(); 29442 }; 29443 } 29444 29445 protected void composeEligibilityResponseBenefitComponent(String name, EligibilityResponse.BenefitComponent element) throws IOException { 29446 if (element != null) { 29447 open(name); 29448 composeEligibilityResponseBenefitComponentInner(element); 29449 close(); 29450 } 29451 } 29452 29453 protected void composeEligibilityResponseBenefitComponentInner(EligibilityResponse.BenefitComponent element) throws IOException { 29454 composeBackbone(element); 29455 if (element.hasType()) { 29456 composeCodeableConcept("type", element.getType()); 29457 } 29458 if (element.hasAllowed()) { 29459 composeType("allowed", element.getAllowed()); 29460 } 29461 if (element.hasUsed()) { 29462 composeType("used", element.getUsed()); 29463 } 29464 } 29465 29466 protected void composeEligibilityResponseErrorsComponent(String name, EligibilityResponse.ErrorsComponent element) throws IOException { 29467 if (element != null) { 29468 open(name); 29469 composeEligibilityResponseErrorsComponentInner(element); 29470 close(); 29471 } 29472 } 29473 29474 protected void composeEligibilityResponseErrorsComponentInner(EligibilityResponse.ErrorsComponent element) throws IOException { 29475 composeBackbone(element); 29476 if (element.hasCode()) { 29477 composeCodeableConcept("code", element.getCode()); 29478 } 29479 } 29480 29481 protected void composeEncounter(String name, Encounter element) throws IOException { 29482 if (element != null) { 29483 prop("resourceType", name); 29484 composeEncounterInner(element); 29485 } 29486 } 29487 29488 protected void composeEncounterInner(Encounter element) throws IOException { 29489 composeDomainResourceElements(element); 29490 if (element.hasIdentifier()) { 29491 openArray("identifier"); 29492 for (Identifier e : element.getIdentifier()) 29493 composeIdentifier(null, e); 29494 closeArray(); 29495 }; 29496 if (element.hasStatusElement()) { 29497 composeEnumerationCore("status", element.getStatusElement(), new Encounter.EncounterStatusEnumFactory(), false); 29498 composeEnumerationExtras("status", element.getStatusElement(), new Encounter.EncounterStatusEnumFactory(), false); 29499 } 29500 if (element.hasStatusHistory()) { 29501 openArray("statusHistory"); 29502 for (Encounter.StatusHistoryComponent e : element.getStatusHistory()) 29503 composeEncounterStatusHistoryComponent(null, e); 29504 closeArray(); 29505 }; 29506 if (element.hasClass_()) { 29507 composeCoding("class", element.getClass_()); 29508 } 29509 if (element.hasClassHistory()) { 29510 openArray("classHistory"); 29511 for (Encounter.ClassHistoryComponent e : element.getClassHistory()) 29512 composeEncounterClassHistoryComponent(null, e); 29513 closeArray(); 29514 }; 29515 if (element.hasType()) { 29516 openArray("type"); 29517 for (CodeableConcept e : element.getType()) 29518 composeCodeableConcept(null, e); 29519 closeArray(); 29520 }; 29521 if (element.hasPriority()) { 29522 composeCodeableConcept("priority", element.getPriority()); 29523 } 29524 if (element.hasSubject()) { 29525 composeReference("subject", element.getSubject()); 29526 } 29527 if (element.hasEpisodeOfCare()) { 29528 openArray("episodeOfCare"); 29529 for (Reference e : element.getEpisodeOfCare()) 29530 composeReference(null, e); 29531 closeArray(); 29532 }; 29533 if (element.hasIncomingReferral()) { 29534 openArray("incomingReferral"); 29535 for (Reference e : element.getIncomingReferral()) 29536 composeReference(null, e); 29537 closeArray(); 29538 }; 29539 if (element.hasParticipant()) { 29540 openArray("participant"); 29541 for (Encounter.EncounterParticipantComponent e : element.getParticipant()) 29542 composeEncounterEncounterParticipantComponent(null, e); 29543 closeArray(); 29544 }; 29545 if (element.hasAppointment()) { 29546 composeReference("appointment", element.getAppointment()); 29547 } 29548 if (element.hasPeriod()) { 29549 composePeriod("period", element.getPeriod()); 29550 } 29551 if (element.hasLength()) { 29552 composeDuration("length", element.getLength()); 29553 } 29554 if (element.hasReason()) { 29555 openArray("reason"); 29556 for (CodeableConcept e : element.getReason()) 29557 composeCodeableConcept(null, e); 29558 closeArray(); 29559 }; 29560 if (element.hasDiagnosis()) { 29561 openArray("diagnosis"); 29562 for (Encounter.DiagnosisComponent e : element.getDiagnosis()) 29563 composeEncounterDiagnosisComponent(null, e); 29564 closeArray(); 29565 }; 29566 if (element.hasAccount()) { 29567 openArray("account"); 29568 for (Reference e : element.getAccount()) 29569 composeReference(null, e); 29570 closeArray(); 29571 }; 29572 if (element.hasHospitalization()) { 29573 composeEncounterEncounterHospitalizationComponent("hospitalization", element.getHospitalization()); 29574 } 29575 if (element.hasLocation()) { 29576 openArray("location"); 29577 for (Encounter.EncounterLocationComponent e : element.getLocation()) 29578 composeEncounterEncounterLocationComponent(null, e); 29579 closeArray(); 29580 }; 29581 if (element.hasServiceProvider()) { 29582 composeReference("serviceProvider", element.getServiceProvider()); 29583 } 29584 if (element.hasPartOf()) { 29585 composeReference("partOf", element.getPartOf()); 29586 } 29587 } 29588 29589 protected void composeEncounterStatusHistoryComponent(String name, Encounter.StatusHistoryComponent element) throws IOException { 29590 if (element != null) { 29591 open(name); 29592 composeEncounterStatusHistoryComponentInner(element); 29593 close(); 29594 } 29595 } 29596 29597 protected void composeEncounterStatusHistoryComponentInner(Encounter.StatusHistoryComponent element) throws IOException { 29598 composeBackbone(element); 29599 if (element.hasStatusElement()) { 29600 composeEnumerationCore("status", element.getStatusElement(), new Encounter.EncounterStatusEnumFactory(), false); 29601 composeEnumerationExtras("status", element.getStatusElement(), new Encounter.EncounterStatusEnumFactory(), false); 29602 } 29603 if (element.hasPeriod()) { 29604 composePeriod("period", element.getPeriod()); 29605 } 29606 } 29607 29608 protected void composeEncounterClassHistoryComponent(String name, Encounter.ClassHistoryComponent element) throws IOException { 29609 if (element != null) { 29610 open(name); 29611 composeEncounterClassHistoryComponentInner(element); 29612 close(); 29613 } 29614 } 29615 29616 protected void composeEncounterClassHistoryComponentInner(Encounter.ClassHistoryComponent element) throws IOException { 29617 composeBackbone(element); 29618 if (element.hasClass_()) { 29619 composeCoding("class", element.getClass_()); 29620 } 29621 if (element.hasPeriod()) { 29622 composePeriod("period", element.getPeriod()); 29623 } 29624 } 29625 29626 protected void composeEncounterEncounterParticipantComponent(String name, Encounter.EncounterParticipantComponent element) throws IOException { 29627 if (element != null) { 29628 open(name); 29629 composeEncounterEncounterParticipantComponentInner(element); 29630 close(); 29631 } 29632 } 29633 29634 protected void composeEncounterEncounterParticipantComponentInner(Encounter.EncounterParticipantComponent element) throws IOException { 29635 composeBackbone(element); 29636 if (element.hasType()) { 29637 openArray("type"); 29638 for (CodeableConcept e : element.getType()) 29639 composeCodeableConcept(null, e); 29640 closeArray(); 29641 }; 29642 if (element.hasPeriod()) { 29643 composePeriod("period", element.getPeriod()); 29644 } 29645 if (element.hasIndividual()) { 29646 composeReference("individual", element.getIndividual()); 29647 } 29648 } 29649 29650 protected void composeEncounterDiagnosisComponent(String name, Encounter.DiagnosisComponent element) throws IOException { 29651 if (element != null) { 29652 open(name); 29653 composeEncounterDiagnosisComponentInner(element); 29654 close(); 29655 } 29656 } 29657 29658 protected void composeEncounterDiagnosisComponentInner(Encounter.DiagnosisComponent element) throws IOException { 29659 composeBackbone(element); 29660 if (element.hasCondition()) { 29661 composeReference("condition", element.getCondition()); 29662 } 29663 if (element.hasRole()) { 29664 composeCodeableConcept("role", element.getRole()); 29665 } 29666 if (element.hasRankElement()) { 29667 composePositiveIntCore("rank", element.getRankElement(), false); 29668 composePositiveIntExtras("rank", element.getRankElement(), false); 29669 } 29670 } 29671 29672 protected void composeEncounterEncounterHospitalizationComponent(String name, Encounter.EncounterHospitalizationComponent element) throws IOException { 29673 if (element != null) { 29674 open(name); 29675 composeEncounterEncounterHospitalizationComponentInner(element); 29676 close(); 29677 } 29678 } 29679 29680 protected void composeEncounterEncounterHospitalizationComponentInner(Encounter.EncounterHospitalizationComponent element) throws IOException { 29681 composeBackbone(element); 29682 if (element.hasPreAdmissionIdentifier()) { 29683 composeIdentifier("preAdmissionIdentifier", element.getPreAdmissionIdentifier()); 29684 } 29685 if (element.hasOrigin()) { 29686 composeReference("origin", element.getOrigin()); 29687 } 29688 if (element.hasAdmitSource()) { 29689 composeCodeableConcept("admitSource", element.getAdmitSource()); 29690 } 29691 if (element.hasReAdmission()) { 29692 composeCodeableConcept("reAdmission", element.getReAdmission()); 29693 } 29694 if (element.hasDietPreference()) { 29695 openArray("dietPreference"); 29696 for (CodeableConcept e : element.getDietPreference()) 29697 composeCodeableConcept(null, e); 29698 closeArray(); 29699 }; 29700 if (element.hasSpecialCourtesy()) { 29701 openArray("specialCourtesy"); 29702 for (CodeableConcept e : element.getSpecialCourtesy()) 29703 composeCodeableConcept(null, e); 29704 closeArray(); 29705 }; 29706 if (element.hasSpecialArrangement()) { 29707 openArray("specialArrangement"); 29708 for (CodeableConcept e : element.getSpecialArrangement()) 29709 composeCodeableConcept(null, e); 29710 closeArray(); 29711 }; 29712 if (element.hasDestination()) { 29713 composeReference("destination", element.getDestination()); 29714 } 29715 if (element.hasDischargeDisposition()) { 29716 composeCodeableConcept("dischargeDisposition", element.getDischargeDisposition()); 29717 } 29718 } 29719 29720 protected void composeEncounterEncounterLocationComponent(String name, Encounter.EncounterLocationComponent element) throws IOException { 29721 if (element != null) { 29722 open(name); 29723 composeEncounterEncounterLocationComponentInner(element); 29724 close(); 29725 } 29726 } 29727 29728 protected void composeEncounterEncounterLocationComponentInner(Encounter.EncounterLocationComponent element) throws IOException { 29729 composeBackbone(element); 29730 if (element.hasLocation()) { 29731 composeReference("location", element.getLocation()); 29732 } 29733 if (element.hasStatusElement()) { 29734 composeEnumerationCore("status", element.getStatusElement(), new Encounter.EncounterLocationStatusEnumFactory(), false); 29735 composeEnumerationExtras("status", element.getStatusElement(), new Encounter.EncounterLocationStatusEnumFactory(), false); 29736 } 29737 if (element.hasPeriod()) { 29738 composePeriod("period", element.getPeriod()); 29739 } 29740 } 29741 29742 protected void composeEndpoint(String name, Endpoint element) throws IOException { 29743 if (element != null) { 29744 prop("resourceType", name); 29745 composeEndpointInner(element); 29746 } 29747 } 29748 29749 protected void composeEndpointInner(Endpoint element) throws IOException { 29750 composeDomainResourceElements(element); 29751 if (element.hasIdentifier()) { 29752 openArray("identifier"); 29753 for (Identifier e : element.getIdentifier()) 29754 composeIdentifier(null, e); 29755 closeArray(); 29756 }; 29757 if (element.hasStatusElement()) { 29758 composeEnumerationCore("status", element.getStatusElement(), new Endpoint.EndpointStatusEnumFactory(), false); 29759 composeEnumerationExtras("status", element.getStatusElement(), new Endpoint.EndpointStatusEnumFactory(), false); 29760 } 29761 if (element.hasConnectionType()) { 29762 composeCoding("connectionType", element.getConnectionType()); 29763 } 29764 if (element.hasNameElement()) { 29765 composeStringCore("name", element.getNameElement(), false); 29766 composeStringExtras("name", element.getNameElement(), false); 29767 } 29768 if (element.hasManagingOrganization()) { 29769 composeReference("managingOrganization", element.getManagingOrganization()); 29770 } 29771 if (element.hasContact()) { 29772 openArray("contact"); 29773 for (ContactPoint e : element.getContact()) 29774 composeContactPoint(null, e); 29775 closeArray(); 29776 }; 29777 if (element.hasPeriod()) { 29778 composePeriod("period", element.getPeriod()); 29779 } 29780 if (element.hasPayloadType()) { 29781 openArray("payloadType"); 29782 for (CodeableConcept e : element.getPayloadType()) 29783 composeCodeableConcept(null, e); 29784 closeArray(); 29785 }; 29786 if (element.hasPayloadMimeType()) { 29787 openArray("payloadMimeType"); 29788 for (CodeType e : element.getPayloadMimeType()) 29789 composeCodeCore(null, e, true); 29790 closeArray(); 29791 if (anyHasExtras(element.getPayloadMimeType())) { 29792 openArray("_payloadMimeType"); 29793 for (CodeType e : element.getPayloadMimeType()) 29794 composeCodeExtras(null, e, true); 29795 closeArray(); 29796 } 29797 }; 29798 if (element.hasAddressElement()) { 29799 composeUriCore("address", element.getAddressElement(), false); 29800 composeUriExtras("address", element.getAddressElement(), false); 29801 } 29802 if (element.hasHeader()) { 29803 openArray("header"); 29804 for (StringType e : element.getHeader()) 29805 composeStringCore(null, e, true); 29806 closeArray(); 29807 if (anyHasExtras(element.getHeader())) { 29808 openArray("_header"); 29809 for (StringType e : element.getHeader()) 29810 composeStringExtras(null, e, true); 29811 closeArray(); 29812 } 29813 }; 29814 } 29815 29816 protected void composeEnrollmentRequest(String name, EnrollmentRequest element) throws IOException { 29817 if (element != null) { 29818 prop("resourceType", name); 29819 composeEnrollmentRequestInner(element); 29820 } 29821 } 29822 29823 protected void composeEnrollmentRequestInner(EnrollmentRequest element) throws IOException { 29824 composeDomainResourceElements(element); 29825 if (element.hasIdentifier()) { 29826 openArray("identifier"); 29827 for (Identifier e : element.getIdentifier()) 29828 composeIdentifier(null, e); 29829 closeArray(); 29830 }; 29831 if (element.hasStatusElement()) { 29832 composeEnumerationCore("status", element.getStatusElement(), new EnrollmentRequest.EnrollmentRequestStatusEnumFactory(), false); 29833 composeEnumerationExtras("status", element.getStatusElement(), new EnrollmentRequest.EnrollmentRequestStatusEnumFactory(), false); 29834 } 29835 if (element.hasCreatedElement()) { 29836 composeDateTimeCore("created", element.getCreatedElement(), false); 29837 composeDateTimeExtras("created", element.getCreatedElement(), false); 29838 } 29839 if (element.hasInsurer()) { 29840 composeReference("insurer", element.getInsurer()); 29841 } 29842 if (element.hasProvider()) { 29843 composeReference("provider", element.getProvider()); 29844 } 29845 if (element.hasOrganization()) { 29846 composeReference("organization", element.getOrganization()); 29847 } 29848 if (element.hasSubject()) { 29849 composeReference("subject", element.getSubject()); 29850 } 29851 if (element.hasCoverage()) { 29852 composeReference("coverage", element.getCoverage()); 29853 } 29854 } 29855 29856 protected void composeEnrollmentResponse(String name, EnrollmentResponse element) throws IOException { 29857 if (element != null) { 29858 prop("resourceType", name); 29859 composeEnrollmentResponseInner(element); 29860 } 29861 } 29862 29863 protected void composeEnrollmentResponseInner(EnrollmentResponse element) throws IOException { 29864 composeDomainResourceElements(element); 29865 if (element.hasIdentifier()) { 29866 openArray("identifier"); 29867 for (Identifier e : element.getIdentifier()) 29868 composeIdentifier(null, e); 29869 closeArray(); 29870 }; 29871 if (element.hasStatusElement()) { 29872 composeEnumerationCore("status", element.getStatusElement(), new EnrollmentResponse.EnrollmentResponseStatusEnumFactory(), false); 29873 composeEnumerationExtras("status", element.getStatusElement(), new EnrollmentResponse.EnrollmentResponseStatusEnumFactory(), false); 29874 } 29875 if (element.hasRequest()) { 29876 composeReference("request", element.getRequest()); 29877 } 29878 if (element.hasOutcome()) { 29879 composeCodeableConcept("outcome", element.getOutcome()); 29880 } 29881 if (element.hasDispositionElement()) { 29882 composeStringCore("disposition", element.getDispositionElement(), false); 29883 composeStringExtras("disposition", element.getDispositionElement(), false); 29884 } 29885 if (element.hasCreatedElement()) { 29886 composeDateTimeCore("created", element.getCreatedElement(), false); 29887 composeDateTimeExtras("created", element.getCreatedElement(), false); 29888 } 29889 if (element.hasOrganization()) { 29890 composeReference("organization", element.getOrganization()); 29891 } 29892 if (element.hasRequestProvider()) { 29893 composeReference("requestProvider", element.getRequestProvider()); 29894 } 29895 if (element.hasRequestOrganization()) { 29896 composeReference("requestOrganization", element.getRequestOrganization()); 29897 } 29898 } 29899 29900 protected void composeEpisodeOfCare(String name, EpisodeOfCare element) throws IOException { 29901 if (element != null) { 29902 prop("resourceType", name); 29903 composeEpisodeOfCareInner(element); 29904 } 29905 } 29906 29907 protected void composeEpisodeOfCareInner(EpisodeOfCare element) throws IOException { 29908 composeDomainResourceElements(element); 29909 if (element.hasIdentifier()) { 29910 openArray("identifier"); 29911 for (Identifier e : element.getIdentifier()) 29912 composeIdentifier(null, e); 29913 closeArray(); 29914 }; 29915 if (element.hasStatusElement()) { 29916 composeEnumerationCore("status", element.getStatusElement(), new EpisodeOfCare.EpisodeOfCareStatusEnumFactory(), false); 29917 composeEnumerationExtras("status", element.getStatusElement(), new EpisodeOfCare.EpisodeOfCareStatusEnumFactory(), false); 29918 } 29919 if (element.hasStatusHistory()) { 29920 openArray("statusHistory"); 29921 for (EpisodeOfCare.EpisodeOfCareStatusHistoryComponent e : element.getStatusHistory()) 29922 composeEpisodeOfCareEpisodeOfCareStatusHistoryComponent(null, e); 29923 closeArray(); 29924 }; 29925 if (element.hasType()) { 29926 openArray("type"); 29927 for (CodeableConcept e : element.getType()) 29928 composeCodeableConcept(null, e); 29929 closeArray(); 29930 }; 29931 if (element.hasDiagnosis()) { 29932 openArray("diagnosis"); 29933 for (EpisodeOfCare.DiagnosisComponent e : element.getDiagnosis()) 29934 composeEpisodeOfCareDiagnosisComponent(null, e); 29935 closeArray(); 29936 }; 29937 if (element.hasPatient()) { 29938 composeReference("patient", element.getPatient()); 29939 } 29940 if (element.hasManagingOrganization()) { 29941 composeReference("managingOrganization", element.getManagingOrganization()); 29942 } 29943 if (element.hasPeriod()) { 29944 composePeriod("period", element.getPeriod()); 29945 } 29946 if (element.hasReferralRequest()) { 29947 openArray("referralRequest"); 29948 for (Reference e : element.getReferralRequest()) 29949 composeReference(null, e); 29950 closeArray(); 29951 }; 29952 if (element.hasCareManager()) { 29953 composeReference("careManager", element.getCareManager()); 29954 } 29955 if (element.hasTeam()) { 29956 openArray("team"); 29957 for (Reference e : element.getTeam()) 29958 composeReference(null, e); 29959 closeArray(); 29960 }; 29961 if (element.hasAccount()) { 29962 openArray("account"); 29963 for (Reference e : element.getAccount()) 29964 composeReference(null, e); 29965 closeArray(); 29966 }; 29967 } 29968 29969 protected void composeEpisodeOfCareEpisodeOfCareStatusHistoryComponent(String name, EpisodeOfCare.EpisodeOfCareStatusHistoryComponent element) throws IOException { 29970 if (element != null) { 29971 open(name); 29972 composeEpisodeOfCareEpisodeOfCareStatusHistoryComponentInner(element); 29973 close(); 29974 } 29975 } 29976 29977 protected void composeEpisodeOfCareEpisodeOfCareStatusHistoryComponentInner(EpisodeOfCare.EpisodeOfCareStatusHistoryComponent element) throws IOException { 29978 composeBackbone(element); 29979 if (element.hasStatusElement()) { 29980 composeEnumerationCore("status", element.getStatusElement(), new EpisodeOfCare.EpisodeOfCareStatusEnumFactory(), false); 29981 composeEnumerationExtras("status", element.getStatusElement(), new EpisodeOfCare.EpisodeOfCareStatusEnumFactory(), false); 29982 } 29983 if (element.hasPeriod()) { 29984 composePeriod("period", element.getPeriod()); 29985 } 29986 } 29987 29988 protected void composeEpisodeOfCareDiagnosisComponent(String name, EpisodeOfCare.DiagnosisComponent element) throws IOException { 29989 if (element != null) { 29990 open(name); 29991 composeEpisodeOfCareDiagnosisComponentInner(element); 29992 close(); 29993 } 29994 } 29995 29996 protected void composeEpisodeOfCareDiagnosisComponentInner(EpisodeOfCare.DiagnosisComponent element) throws IOException { 29997 composeBackbone(element); 29998 if (element.hasCondition()) { 29999 composeReference("condition", element.getCondition()); 30000 } 30001 if (element.hasRole()) { 30002 composeCodeableConcept("role", element.getRole()); 30003 } 30004 if (element.hasRankElement()) { 30005 composePositiveIntCore("rank", element.getRankElement(), false); 30006 composePositiveIntExtras("rank", element.getRankElement(), false); 30007 } 30008 } 30009 30010 protected void composeExpansionProfile(String name, ExpansionProfile element) throws IOException { 30011 if (element != null) { 30012 prop("resourceType", name); 30013 composeExpansionProfileInner(element); 30014 } 30015 } 30016 30017 protected void composeExpansionProfileInner(ExpansionProfile element) throws IOException { 30018 composeDomainResourceElements(element); 30019 if (element.hasUrlElement()) { 30020 composeUriCore("url", element.getUrlElement(), false); 30021 composeUriExtras("url", element.getUrlElement(), false); 30022 } 30023 if (element.hasIdentifier()) { 30024 composeIdentifier("identifier", element.getIdentifier()); 30025 } 30026 if (element.hasVersionElement()) { 30027 composeStringCore("version", element.getVersionElement(), false); 30028 composeStringExtras("version", element.getVersionElement(), false); 30029 } 30030 if (element.hasNameElement()) { 30031 composeStringCore("name", element.getNameElement(), false); 30032 composeStringExtras("name", element.getNameElement(), false); 30033 } 30034 if (element.hasStatusElement()) { 30035 composeEnumerationCore("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 30036 composeEnumerationExtras("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 30037 } 30038 if (element.hasExperimentalElement()) { 30039 composeBooleanCore("experimental", element.getExperimentalElement(), false); 30040 composeBooleanExtras("experimental", element.getExperimentalElement(), false); 30041 } 30042 if (element.hasDateElement()) { 30043 composeDateTimeCore("date", element.getDateElement(), false); 30044 composeDateTimeExtras("date", element.getDateElement(), false); 30045 } 30046 if (element.hasPublisherElement()) { 30047 composeStringCore("publisher", element.getPublisherElement(), false); 30048 composeStringExtras("publisher", element.getPublisherElement(), false); 30049 } 30050 if (element.hasContact()) { 30051 openArray("contact"); 30052 for (ContactDetail e : element.getContact()) 30053 composeContactDetail(null, e); 30054 closeArray(); 30055 }; 30056 if (element.hasDescriptionElement()) { 30057 composeMarkdownCore("description", element.getDescriptionElement(), false); 30058 composeMarkdownExtras("description", element.getDescriptionElement(), false); 30059 } 30060 if (element.hasUseContext()) { 30061 openArray("useContext"); 30062 for (UsageContext e : element.getUseContext()) 30063 composeUsageContext(null, e); 30064 closeArray(); 30065 }; 30066 if (element.hasJurisdiction()) { 30067 openArray("jurisdiction"); 30068 for (CodeableConcept e : element.getJurisdiction()) 30069 composeCodeableConcept(null, e); 30070 closeArray(); 30071 }; 30072 if (element.hasFixedVersion()) { 30073 openArray("fixedVersion"); 30074 for (ExpansionProfile.ExpansionProfileFixedVersionComponent e : element.getFixedVersion()) 30075 composeExpansionProfileExpansionProfileFixedVersionComponent(null, e); 30076 closeArray(); 30077 }; 30078 if (element.hasExcludedSystem()) { 30079 composeExpansionProfileExpansionProfileExcludedSystemComponent("excludedSystem", element.getExcludedSystem()); 30080 } 30081 if (element.hasIncludeDesignationsElement()) { 30082 composeBooleanCore("includeDesignations", element.getIncludeDesignationsElement(), false); 30083 composeBooleanExtras("includeDesignations", element.getIncludeDesignationsElement(), false); 30084 } 30085 if (element.hasDesignation()) { 30086 composeExpansionProfileExpansionProfileDesignationComponent("designation", element.getDesignation()); 30087 } 30088 if (element.hasIncludeDefinitionElement()) { 30089 composeBooleanCore("includeDefinition", element.getIncludeDefinitionElement(), false); 30090 composeBooleanExtras("includeDefinition", element.getIncludeDefinitionElement(), false); 30091 } 30092 if (element.hasActiveOnlyElement()) { 30093 composeBooleanCore("activeOnly", element.getActiveOnlyElement(), false); 30094 composeBooleanExtras("activeOnly", element.getActiveOnlyElement(), false); 30095 } 30096 if (element.hasExcludeNestedElement()) { 30097 composeBooleanCore("excludeNested", element.getExcludeNestedElement(), false); 30098 composeBooleanExtras("excludeNested", element.getExcludeNestedElement(), false); 30099 } 30100 if (element.hasExcludeNotForUIElement()) { 30101 composeBooleanCore("excludeNotForUI", element.getExcludeNotForUIElement(), false); 30102 composeBooleanExtras("excludeNotForUI", element.getExcludeNotForUIElement(), false); 30103 } 30104 if (element.hasExcludePostCoordinatedElement()) { 30105 composeBooleanCore("excludePostCoordinated", element.getExcludePostCoordinatedElement(), false); 30106 composeBooleanExtras("excludePostCoordinated", element.getExcludePostCoordinatedElement(), false); 30107 } 30108 if (element.hasDisplayLanguageElement()) { 30109 composeCodeCore("displayLanguage", element.getDisplayLanguageElement(), false); 30110 composeCodeExtras("displayLanguage", element.getDisplayLanguageElement(), false); 30111 } 30112 if (element.hasLimitedExpansionElement()) { 30113 composeBooleanCore("limitedExpansion", element.getLimitedExpansionElement(), false); 30114 composeBooleanExtras("limitedExpansion", element.getLimitedExpansionElement(), false); 30115 } 30116 } 30117 30118 protected void composeExpansionProfileExpansionProfileFixedVersionComponent(String name, ExpansionProfile.ExpansionProfileFixedVersionComponent element) throws IOException { 30119 if (element != null) { 30120 open(name); 30121 composeExpansionProfileExpansionProfileFixedVersionComponentInner(element); 30122 close(); 30123 } 30124 } 30125 30126 protected void composeExpansionProfileExpansionProfileFixedVersionComponentInner(ExpansionProfile.ExpansionProfileFixedVersionComponent element) throws IOException { 30127 composeBackbone(element); 30128 if (element.hasSystemElement()) { 30129 composeUriCore("system", element.getSystemElement(), false); 30130 composeUriExtras("system", element.getSystemElement(), false); 30131 } 30132 if (element.hasVersionElement()) { 30133 composeStringCore("version", element.getVersionElement(), false); 30134 composeStringExtras("version", element.getVersionElement(), false); 30135 } 30136 if (element.hasModeElement()) { 30137 composeEnumerationCore("mode", element.getModeElement(), new ExpansionProfile.SystemVersionProcessingModeEnumFactory(), false); 30138 composeEnumerationExtras("mode", element.getModeElement(), new ExpansionProfile.SystemVersionProcessingModeEnumFactory(), false); 30139 } 30140 } 30141 30142 protected void composeExpansionProfileExpansionProfileExcludedSystemComponent(String name, ExpansionProfile.ExpansionProfileExcludedSystemComponent element) throws IOException { 30143 if (element != null) { 30144 open(name); 30145 composeExpansionProfileExpansionProfileExcludedSystemComponentInner(element); 30146 close(); 30147 } 30148 } 30149 30150 protected void composeExpansionProfileExpansionProfileExcludedSystemComponentInner(ExpansionProfile.ExpansionProfileExcludedSystemComponent element) throws IOException { 30151 composeBackbone(element); 30152 if (element.hasSystemElement()) { 30153 composeUriCore("system", element.getSystemElement(), false); 30154 composeUriExtras("system", element.getSystemElement(), false); 30155 } 30156 if (element.hasVersionElement()) { 30157 composeStringCore("version", element.getVersionElement(), false); 30158 composeStringExtras("version", element.getVersionElement(), false); 30159 } 30160 } 30161 30162 protected void composeExpansionProfileExpansionProfileDesignationComponent(String name, ExpansionProfile.ExpansionProfileDesignationComponent element) throws IOException { 30163 if (element != null) { 30164 open(name); 30165 composeExpansionProfileExpansionProfileDesignationComponentInner(element); 30166 close(); 30167 } 30168 } 30169 30170 protected void composeExpansionProfileExpansionProfileDesignationComponentInner(ExpansionProfile.ExpansionProfileDesignationComponent element) throws IOException { 30171 composeBackbone(element); 30172 if (element.hasInclude()) { 30173 composeExpansionProfileDesignationIncludeComponent("include", element.getInclude()); 30174 } 30175 if (element.hasExclude()) { 30176 composeExpansionProfileDesignationExcludeComponent("exclude", element.getExclude()); 30177 } 30178 } 30179 30180 protected void composeExpansionProfileDesignationIncludeComponent(String name, ExpansionProfile.DesignationIncludeComponent element) throws IOException { 30181 if (element != null) { 30182 open(name); 30183 composeExpansionProfileDesignationIncludeComponentInner(element); 30184 close(); 30185 } 30186 } 30187 30188 protected void composeExpansionProfileDesignationIncludeComponentInner(ExpansionProfile.DesignationIncludeComponent element) throws IOException { 30189 composeBackbone(element); 30190 if (element.hasDesignation()) { 30191 openArray("designation"); 30192 for (ExpansionProfile.DesignationIncludeDesignationComponent e : element.getDesignation()) 30193 composeExpansionProfileDesignationIncludeDesignationComponent(null, e); 30194 closeArray(); 30195 }; 30196 } 30197 30198 protected void composeExpansionProfileDesignationIncludeDesignationComponent(String name, ExpansionProfile.DesignationIncludeDesignationComponent element) throws IOException { 30199 if (element != null) { 30200 open(name); 30201 composeExpansionProfileDesignationIncludeDesignationComponentInner(element); 30202 close(); 30203 } 30204 } 30205 30206 protected void composeExpansionProfileDesignationIncludeDesignationComponentInner(ExpansionProfile.DesignationIncludeDesignationComponent element) throws IOException { 30207 composeBackbone(element); 30208 if (element.hasLanguageElement()) { 30209 composeCodeCore("language", element.getLanguageElement(), false); 30210 composeCodeExtras("language", element.getLanguageElement(), false); 30211 } 30212 if (element.hasUse()) { 30213 composeCoding("use", element.getUse()); 30214 } 30215 } 30216 30217 protected void composeExpansionProfileDesignationExcludeComponent(String name, ExpansionProfile.DesignationExcludeComponent element) throws IOException { 30218 if (element != null) { 30219 open(name); 30220 composeExpansionProfileDesignationExcludeComponentInner(element); 30221 close(); 30222 } 30223 } 30224 30225 protected void composeExpansionProfileDesignationExcludeComponentInner(ExpansionProfile.DesignationExcludeComponent element) throws IOException { 30226 composeBackbone(element); 30227 if (element.hasDesignation()) { 30228 openArray("designation"); 30229 for (ExpansionProfile.DesignationExcludeDesignationComponent e : element.getDesignation()) 30230 composeExpansionProfileDesignationExcludeDesignationComponent(null, e); 30231 closeArray(); 30232 }; 30233 } 30234 30235 protected void composeExpansionProfileDesignationExcludeDesignationComponent(String name, ExpansionProfile.DesignationExcludeDesignationComponent element) throws IOException { 30236 if (element != null) { 30237 open(name); 30238 composeExpansionProfileDesignationExcludeDesignationComponentInner(element); 30239 close(); 30240 } 30241 } 30242 30243 protected void composeExpansionProfileDesignationExcludeDesignationComponentInner(ExpansionProfile.DesignationExcludeDesignationComponent element) throws IOException { 30244 composeBackbone(element); 30245 if (element.hasLanguageElement()) { 30246 composeCodeCore("language", element.getLanguageElement(), false); 30247 composeCodeExtras("language", element.getLanguageElement(), false); 30248 } 30249 if (element.hasUse()) { 30250 composeCoding("use", element.getUse()); 30251 } 30252 } 30253 30254 protected void composeExplanationOfBenefit(String name, ExplanationOfBenefit element) throws IOException { 30255 if (element != null) { 30256 prop("resourceType", name); 30257 composeExplanationOfBenefitInner(element); 30258 } 30259 } 30260 30261 protected void composeExplanationOfBenefitInner(ExplanationOfBenefit element) throws IOException { 30262 composeDomainResourceElements(element); 30263 if (element.hasIdentifier()) { 30264 openArray("identifier"); 30265 for (Identifier e : element.getIdentifier()) 30266 composeIdentifier(null, e); 30267 closeArray(); 30268 }; 30269 if (element.hasStatusElement()) { 30270 composeEnumerationCore("status", element.getStatusElement(), new ExplanationOfBenefit.ExplanationOfBenefitStatusEnumFactory(), false); 30271 composeEnumerationExtras("status", element.getStatusElement(), new ExplanationOfBenefit.ExplanationOfBenefitStatusEnumFactory(), false); 30272 } 30273 if (element.hasType()) { 30274 composeCodeableConcept("type", element.getType()); 30275 } 30276 if (element.hasSubType()) { 30277 openArray("subType"); 30278 for (CodeableConcept e : element.getSubType()) 30279 composeCodeableConcept(null, e); 30280 closeArray(); 30281 }; 30282 if (element.hasPatient()) { 30283 composeReference("patient", element.getPatient()); 30284 } 30285 if (element.hasBillablePeriod()) { 30286 composePeriod("billablePeriod", element.getBillablePeriod()); 30287 } 30288 if (element.hasCreatedElement()) { 30289 composeDateTimeCore("created", element.getCreatedElement(), false); 30290 composeDateTimeExtras("created", element.getCreatedElement(), false); 30291 } 30292 if (element.hasEnterer()) { 30293 composeReference("enterer", element.getEnterer()); 30294 } 30295 if (element.hasInsurer()) { 30296 composeReference("insurer", element.getInsurer()); 30297 } 30298 if (element.hasProvider()) { 30299 composeReference("provider", element.getProvider()); 30300 } 30301 if (element.hasOrganization()) { 30302 composeReference("organization", element.getOrganization()); 30303 } 30304 if (element.hasReferral()) { 30305 composeReference("referral", element.getReferral()); 30306 } 30307 if (element.hasFacility()) { 30308 composeReference("facility", element.getFacility()); 30309 } 30310 if (element.hasClaim()) { 30311 composeReference("claim", element.getClaim()); 30312 } 30313 if (element.hasClaimResponse()) { 30314 composeReference("claimResponse", element.getClaimResponse()); 30315 } 30316 if (element.hasOutcome()) { 30317 composeCodeableConcept("outcome", element.getOutcome()); 30318 } 30319 if (element.hasDispositionElement()) { 30320 composeStringCore("disposition", element.getDispositionElement(), false); 30321 composeStringExtras("disposition", element.getDispositionElement(), false); 30322 } 30323 if (element.hasRelated()) { 30324 openArray("related"); 30325 for (ExplanationOfBenefit.RelatedClaimComponent e : element.getRelated()) 30326 composeExplanationOfBenefitRelatedClaimComponent(null, e); 30327 closeArray(); 30328 }; 30329 if (element.hasPrescription()) { 30330 composeReference("prescription", element.getPrescription()); 30331 } 30332 if (element.hasOriginalPrescription()) { 30333 composeReference("originalPrescription", element.getOriginalPrescription()); 30334 } 30335 if (element.hasPayee()) { 30336 composeExplanationOfBenefitPayeeComponent("payee", element.getPayee()); 30337 } 30338 if (element.hasInformation()) { 30339 openArray("information"); 30340 for (ExplanationOfBenefit.SupportingInformationComponent e : element.getInformation()) 30341 composeExplanationOfBenefitSupportingInformationComponent(null, e); 30342 closeArray(); 30343 }; 30344 if (element.hasCareTeam()) { 30345 openArray("careTeam"); 30346 for (ExplanationOfBenefit.CareTeamComponent e : element.getCareTeam()) 30347 composeExplanationOfBenefitCareTeamComponent(null, e); 30348 closeArray(); 30349 }; 30350 if (element.hasDiagnosis()) { 30351 openArray("diagnosis"); 30352 for (ExplanationOfBenefit.DiagnosisComponent e : element.getDiagnosis()) 30353 composeExplanationOfBenefitDiagnosisComponent(null, e); 30354 closeArray(); 30355 }; 30356 if (element.hasProcedure()) { 30357 openArray("procedure"); 30358 for (ExplanationOfBenefit.ProcedureComponent e : element.getProcedure()) 30359 composeExplanationOfBenefitProcedureComponent(null, e); 30360 closeArray(); 30361 }; 30362 if (element.hasPrecedenceElement()) { 30363 composePositiveIntCore("precedence", element.getPrecedenceElement(), false); 30364 composePositiveIntExtras("precedence", element.getPrecedenceElement(), false); 30365 } 30366 if (element.hasInsurance()) { 30367 composeExplanationOfBenefitInsuranceComponent("insurance", element.getInsurance()); 30368 } 30369 if (element.hasAccident()) { 30370 composeExplanationOfBenefitAccidentComponent("accident", element.getAccident()); 30371 } 30372 if (element.hasEmploymentImpacted()) { 30373 composePeriod("employmentImpacted", element.getEmploymentImpacted()); 30374 } 30375 if (element.hasHospitalization()) { 30376 composePeriod("hospitalization", element.getHospitalization()); 30377 } 30378 if (element.hasItem()) { 30379 openArray("item"); 30380 for (ExplanationOfBenefit.ItemComponent e : element.getItem()) 30381 composeExplanationOfBenefitItemComponent(null, e); 30382 closeArray(); 30383 }; 30384 if (element.hasAddItem()) { 30385 openArray("addItem"); 30386 for (ExplanationOfBenefit.AddedItemComponent e : element.getAddItem()) 30387 composeExplanationOfBenefitAddedItemComponent(null, e); 30388 closeArray(); 30389 }; 30390 if (element.hasTotalCost()) { 30391 composeMoney("totalCost", element.getTotalCost()); 30392 } 30393 if (element.hasUnallocDeductable()) { 30394 composeMoney("unallocDeductable", element.getUnallocDeductable()); 30395 } 30396 if (element.hasTotalBenefit()) { 30397 composeMoney("totalBenefit", element.getTotalBenefit()); 30398 } 30399 if (element.hasPayment()) { 30400 composeExplanationOfBenefitPaymentComponent("payment", element.getPayment()); 30401 } 30402 if (element.hasForm()) { 30403 composeCodeableConcept("form", element.getForm()); 30404 } 30405 if (element.hasProcessNote()) { 30406 openArray("processNote"); 30407 for (ExplanationOfBenefit.NoteComponent e : element.getProcessNote()) 30408 composeExplanationOfBenefitNoteComponent(null, e); 30409 closeArray(); 30410 }; 30411 if (element.hasBenefitBalance()) { 30412 openArray("benefitBalance"); 30413 for (ExplanationOfBenefit.BenefitBalanceComponent e : element.getBenefitBalance()) 30414 composeExplanationOfBenefitBenefitBalanceComponent(null, e); 30415 closeArray(); 30416 }; 30417 } 30418 30419 protected void composeExplanationOfBenefitRelatedClaimComponent(String name, ExplanationOfBenefit.RelatedClaimComponent element) throws IOException { 30420 if (element != null) { 30421 open(name); 30422 composeExplanationOfBenefitRelatedClaimComponentInner(element); 30423 close(); 30424 } 30425 } 30426 30427 protected void composeExplanationOfBenefitRelatedClaimComponentInner(ExplanationOfBenefit.RelatedClaimComponent element) throws IOException { 30428 composeBackbone(element); 30429 if (element.hasClaim()) { 30430 composeReference("claim", element.getClaim()); 30431 } 30432 if (element.hasRelationship()) { 30433 composeCodeableConcept("relationship", element.getRelationship()); 30434 } 30435 if (element.hasReference()) { 30436 composeIdentifier("reference", element.getReference()); 30437 } 30438 } 30439 30440 protected void composeExplanationOfBenefitPayeeComponent(String name, ExplanationOfBenefit.PayeeComponent element) throws IOException { 30441 if (element != null) { 30442 open(name); 30443 composeExplanationOfBenefitPayeeComponentInner(element); 30444 close(); 30445 } 30446 } 30447 30448 protected void composeExplanationOfBenefitPayeeComponentInner(ExplanationOfBenefit.PayeeComponent element) throws IOException { 30449 composeBackbone(element); 30450 if (element.hasType()) { 30451 composeCodeableConcept("type", element.getType()); 30452 } 30453 if (element.hasResourceType()) { 30454 composeCodeableConcept("resourceType", element.getResourceType()); 30455 } 30456 if (element.hasParty()) { 30457 composeReference("party", element.getParty()); 30458 } 30459 } 30460 30461 protected void composeExplanationOfBenefitSupportingInformationComponent(String name, ExplanationOfBenefit.SupportingInformationComponent element) throws IOException { 30462 if (element != null) { 30463 open(name); 30464 composeExplanationOfBenefitSupportingInformationComponentInner(element); 30465 close(); 30466 } 30467 } 30468 30469 protected void composeExplanationOfBenefitSupportingInformationComponentInner(ExplanationOfBenefit.SupportingInformationComponent element) throws IOException { 30470 composeBackbone(element); 30471 if (element.hasSequenceElement()) { 30472 composePositiveIntCore("sequence", element.getSequenceElement(), false); 30473 composePositiveIntExtras("sequence", element.getSequenceElement(), false); 30474 } 30475 if (element.hasCategory()) { 30476 composeCodeableConcept("category", element.getCategory()); 30477 } 30478 if (element.hasCode()) { 30479 composeCodeableConcept("code", element.getCode()); 30480 } 30481 if (element.hasTiming()) { 30482 composeType("timing", element.getTiming()); 30483 } 30484 if (element.hasValue()) { 30485 composeType("value", element.getValue()); 30486 } 30487 if (element.hasReason()) { 30488 composeCoding("reason", element.getReason()); 30489 } 30490 } 30491 30492 protected void composeExplanationOfBenefitCareTeamComponent(String name, ExplanationOfBenefit.CareTeamComponent element) throws IOException { 30493 if (element != null) { 30494 open(name); 30495 composeExplanationOfBenefitCareTeamComponentInner(element); 30496 close(); 30497 } 30498 } 30499 30500 protected void composeExplanationOfBenefitCareTeamComponentInner(ExplanationOfBenefit.CareTeamComponent element) throws IOException { 30501 composeBackbone(element); 30502 if (element.hasSequenceElement()) { 30503 composePositiveIntCore("sequence", element.getSequenceElement(), false); 30504 composePositiveIntExtras("sequence", element.getSequenceElement(), false); 30505 } 30506 if (element.hasProvider()) { 30507 composeReference("provider", element.getProvider()); 30508 } 30509 if (element.hasResponsibleElement()) { 30510 composeBooleanCore("responsible", element.getResponsibleElement(), false); 30511 composeBooleanExtras("responsible", element.getResponsibleElement(), false); 30512 } 30513 if (element.hasRole()) { 30514 composeCodeableConcept("role", element.getRole()); 30515 } 30516 if (element.hasQualification()) { 30517 composeCodeableConcept("qualification", element.getQualification()); 30518 } 30519 } 30520 30521 protected void composeExplanationOfBenefitDiagnosisComponent(String name, ExplanationOfBenefit.DiagnosisComponent element) throws IOException { 30522 if (element != null) { 30523 open(name); 30524 composeExplanationOfBenefitDiagnosisComponentInner(element); 30525 close(); 30526 } 30527 } 30528 30529 protected void composeExplanationOfBenefitDiagnosisComponentInner(ExplanationOfBenefit.DiagnosisComponent element) throws IOException { 30530 composeBackbone(element); 30531 if (element.hasSequenceElement()) { 30532 composePositiveIntCore("sequence", element.getSequenceElement(), false); 30533 composePositiveIntExtras("sequence", element.getSequenceElement(), false); 30534 } 30535 if (element.hasDiagnosis()) { 30536 composeType("diagnosis", element.getDiagnosis()); 30537 } 30538 if (element.hasType()) { 30539 openArray("type"); 30540 for (CodeableConcept e : element.getType()) 30541 composeCodeableConcept(null, e); 30542 closeArray(); 30543 }; 30544 if (element.hasPackageCode()) { 30545 composeCodeableConcept("packageCode", element.getPackageCode()); 30546 } 30547 } 30548 30549 protected void composeExplanationOfBenefitProcedureComponent(String name, ExplanationOfBenefit.ProcedureComponent element) throws IOException { 30550 if (element != null) { 30551 open(name); 30552 composeExplanationOfBenefitProcedureComponentInner(element); 30553 close(); 30554 } 30555 } 30556 30557 protected void composeExplanationOfBenefitProcedureComponentInner(ExplanationOfBenefit.ProcedureComponent element) throws IOException { 30558 composeBackbone(element); 30559 if (element.hasSequenceElement()) { 30560 composePositiveIntCore("sequence", element.getSequenceElement(), false); 30561 composePositiveIntExtras("sequence", element.getSequenceElement(), false); 30562 } 30563 if (element.hasDateElement()) { 30564 composeDateTimeCore("date", element.getDateElement(), false); 30565 composeDateTimeExtras("date", element.getDateElement(), false); 30566 } 30567 if (element.hasProcedure()) { 30568 composeType("procedure", element.getProcedure()); 30569 } 30570 } 30571 30572 protected void composeExplanationOfBenefitInsuranceComponent(String name, ExplanationOfBenefit.InsuranceComponent element) throws IOException { 30573 if (element != null) { 30574 open(name); 30575 composeExplanationOfBenefitInsuranceComponentInner(element); 30576 close(); 30577 } 30578 } 30579 30580 protected void composeExplanationOfBenefitInsuranceComponentInner(ExplanationOfBenefit.InsuranceComponent element) throws IOException { 30581 composeBackbone(element); 30582 if (element.hasCoverage()) { 30583 composeReference("coverage", element.getCoverage()); 30584 } 30585 if (element.hasPreAuthRef()) { 30586 openArray("preAuthRef"); 30587 for (StringType e : element.getPreAuthRef()) 30588 composeStringCore(null, e, true); 30589 closeArray(); 30590 if (anyHasExtras(element.getPreAuthRef())) { 30591 openArray("_preAuthRef"); 30592 for (StringType e : element.getPreAuthRef()) 30593 composeStringExtras(null, e, true); 30594 closeArray(); 30595 } 30596 }; 30597 } 30598 30599 protected void composeExplanationOfBenefitAccidentComponent(String name, ExplanationOfBenefit.AccidentComponent element) throws IOException { 30600 if (element != null) { 30601 open(name); 30602 composeExplanationOfBenefitAccidentComponentInner(element); 30603 close(); 30604 } 30605 } 30606 30607 protected void composeExplanationOfBenefitAccidentComponentInner(ExplanationOfBenefit.AccidentComponent element) throws IOException { 30608 composeBackbone(element); 30609 if (element.hasDateElement()) { 30610 composeDateCore("date", element.getDateElement(), false); 30611 composeDateExtras("date", element.getDateElement(), false); 30612 } 30613 if (element.hasType()) { 30614 composeCodeableConcept("type", element.getType()); 30615 } 30616 if (element.hasLocation()) { 30617 composeType("location", element.getLocation()); 30618 } 30619 } 30620 30621 protected void composeExplanationOfBenefitItemComponent(String name, ExplanationOfBenefit.ItemComponent element) throws IOException { 30622 if (element != null) { 30623 open(name); 30624 composeExplanationOfBenefitItemComponentInner(element); 30625 close(); 30626 } 30627 } 30628 30629 protected void composeExplanationOfBenefitItemComponentInner(ExplanationOfBenefit.ItemComponent element) throws IOException { 30630 composeBackbone(element); 30631 if (element.hasSequenceElement()) { 30632 composePositiveIntCore("sequence", element.getSequenceElement(), false); 30633 composePositiveIntExtras("sequence", element.getSequenceElement(), false); 30634 } 30635 if (element.hasCareTeamLinkId()) { 30636 openArray("careTeamLinkId"); 30637 for (PositiveIntType e : element.getCareTeamLinkId()) 30638 composePositiveIntCore(null, e, true); 30639 closeArray(); 30640 if (anyHasExtras(element.getCareTeamLinkId())) { 30641 openArray("_careTeamLinkId"); 30642 for (PositiveIntType e : element.getCareTeamLinkId()) 30643 composePositiveIntExtras(null, e, true); 30644 closeArray(); 30645 } 30646 }; 30647 if (element.hasDiagnosisLinkId()) { 30648 openArray("diagnosisLinkId"); 30649 for (PositiveIntType e : element.getDiagnosisLinkId()) 30650 composePositiveIntCore(null, e, true); 30651 closeArray(); 30652 if (anyHasExtras(element.getDiagnosisLinkId())) { 30653 openArray("_diagnosisLinkId"); 30654 for (PositiveIntType e : element.getDiagnosisLinkId()) 30655 composePositiveIntExtras(null, e, true); 30656 closeArray(); 30657 } 30658 }; 30659 if (element.hasProcedureLinkId()) { 30660 openArray("procedureLinkId"); 30661 for (PositiveIntType e : element.getProcedureLinkId()) 30662 composePositiveIntCore(null, e, true); 30663 closeArray(); 30664 if (anyHasExtras(element.getProcedureLinkId())) { 30665 openArray("_procedureLinkId"); 30666 for (PositiveIntType e : element.getProcedureLinkId()) 30667 composePositiveIntExtras(null, e, true); 30668 closeArray(); 30669 } 30670 }; 30671 if (element.hasInformationLinkId()) { 30672 openArray("informationLinkId"); 30673 for (PositiveIntType e : element.getInformationLinkId()) 30674 composePositiveIntCore(null, e, true); 30675 closeArray(); 30676 if (anyHasExtras(element.getInformationLinkId())) { 30677 openArray("_informationLinkId"); 30678 for (PositiveIntType e : element.getInformationLinkId()) 30679 composePositiveIntExtras(null, e, true); 30680 closeArray(); 30681 } 30682 }; 30683 if (element.hasRevenue()) { 30684 composeCodeableConcept("revenue", element.getRevenue()); 30685 } 30686 if (element.hasCategory()) { 30687 composeCodeableConcept("category", element.getCategory()); 30688 } 30689 if (element.hasService()) { 30690 composeCodeableConcept("service", element.getService()); 30691 } 30692 if (element.hasModifier()) { 30693 openArray("modifier"); 30694 for (CodeableConcept e : element.getModifier()) 30695 composeCodeableConcept(null, e); 30696 closeArray(); 30697 }; 30698 if (element.hasProgramCode()) { 30699 openArray("programCode"); 30700 for (CodeableConcept e : element.getProgramCode()) 30701 composeCodeableConcept(null, e); 30702 closeArray(); 30703 }; 30704 if (element.hasServiced()) { 30705 composeType("serviced", element.getServiced()); 30706 } 30707 if (element.hasLocation()) { 30708 composeType("location", element.getLocation()); 30709 } 30710 if (element.hasQuantity()) { 30711 composeSimpleQuantity("quantity", element.getQuantity()); 30712 } 30713 if (element.hasUnitPrice()) { 30714 composeMoney("unitPrice", element.getUnitPrice()); 30715 } 30716 if (element.hasFactorElement()) { 30717 composeDecimalCore("factor", element.getFactorElement(), false); 30718 composeDecimalExtras("factor", element.getFactorElement(), false); 30719 } 30720 if (element.hasNet()) { 30721 composeMoney("net", element.getNet()); 30722 } 30723 if (element.hasUdi()) { 30724 openArray("udi"); 30725 for (Reference e : element.getUdi()) 30726 composeReference(null, e); 30727 closeArray(); 30728 }; 30729 if (element.hasBodySite()) { 30730 composeCodeableConcept("bodySite", element.getBodySite()); 30731 } 30732 if (element.hasSubSite()) { 30733 openArray("subSite"); 30734 for (CodeableConcept e : element.getSubSite()) 30735 composeCodeableConcept(null, e); 30736 closeArray(); 30737 }; 30738 if (element.hasEncounter()) { 30739 openArray("encounter"); 30740 for (Reference e : element.getEncounter()) 30741 composeReference(null, e); 30742 closeArray(); 30743 }; 30744 if (element.hasNoteNumber()) { 30745 openArray("noteNumber"); 30746 for (PositiveIntType e : element.getNoteNumber()) 30747 composePositiveIntCore(null, e, true); 30748 closeArray(); 30749 if (anyHasExtras(element.getNoteNumber())) { 30750 openArray("_noteNumber"); 30751 for (PositiveIntType e : element.getNoteNumber()) 30752 composePositiveIntExtras(null, e, true); 30753 closeArray(); 30754 } 30755 }; 30756 if (element.hasAdjudication()) { 30757 openArray("adjudication"); 30758 for (ExplanationOfBenefit.AdjudicationComponent e : element.getAdjudication()) 30759 composeExplanationOfBenefitAdjudicationComponent(null, e); 30760 closeArray(); 30761 }; 30762 if (element.hasDetail()) { 30763 openArray("detail"); 30764 for (ExplanationOfBenefit.DetailComponent e : element.getDetail()) 30765 composeExplanationOfBenefitDetailComponent(null, e); 30766 closeArray(); 30767 }; 30768 } 30769 30770 protected void composeExplanationOfBenefitAdjudicationComponent(String name, ExplanationOfBenefit.AdjudicationComponent element) throws IOException { 30771 if (element != null) { 30772 open(name); 30773 composeExplanationOfBenefitAdjudicationComponentInner(element); 30774 close(); 30775 } 30776 } 30777 30778 protected void composeExplanationOfBenefitAdjudicationComponentInner(ExplanationOfBenefit.AdjudicationComponent element) throws IOException { 30779 composeBackbone(element); 30780 if (element.hasCategory()) { 30781 composeCodeableConcept("category", element.getCategory()); 30782 } 30783 if (element.hasReason()) { 30784 composeCodeableConcept("reason", element.getReason()); 30785 } 30786 if (element.hasAmount()) { 30787 composeMoney("amount", element.getAmount()); 30788 } 30789 if (element.hasValueElement()) { 30790 composeDecimalCore("value", element.getValueElement(), false); 30791 composeDecimalExtras("value", element.getValueElement(), false); 30792 } 30793 } 30794 30795 protected void composeExplanationOfBenefitDetailComponent(String name, ExplanationOfBenefit.DetailComponent element) throws IOException { 30796 if (element != null) { 30797 open(name); 30798 composeExplanationOfBenefitDetailComponentInner(element); 30799 close(); 30800 } 30801 } 30802 30803 protected void composeExplanationOfBenefitDetailComponentInner(ExplanationOfBenefit.DetailComponent element) throws IOException { 30804 composeBackbone(element); 30805 if (element.hasSequenceElement()) { 30806 composePositiveIntCore("sequence", element.getSequenceElement(), false); 30807 composePositiveIntExtras("sequence", element.getSequenceElement(), false); 30808 } 30809 if (element.hasType()) { 30810 composeCodeableConcept("type", element.getType()); 30811 } 30812 if (element.hasRevenue()) { 30813 composeCodeableConcept("revenue", element.getRevenue()); 30814 } 30815 if (element.hasCategory()) { 30816 composeCodeableConcept("category", element.getCategory()); 30817 } 30818 if (element.hasService()) { 30819 composeCodeableConcept("service", element.getService()); 30820 } 30821 if (element.hasModifier()) { 30822 openArray("modifier"); 30823 for (CodeableConcept e : element.getModifier()) 30824 composeCodeableConcept(null, e); 30825 closeArray(); 30826 }; 30827 if (element.hasProgramCode()) { 30828 openArray("programCode"); 30829 for (CodeableConcept e : element.getProgramCode()) 30830 composeCodeableConcept(null, e); 30831 closeArray(); 30832 }; 30833 if (element.hasQuantity()) { 30834 composeSimpleQuantity("quantity", element.getQuantity()); 30835 } 30836 if (element.hasUnitPrice()) { 30837 composeMoney("unitPrice", element.getUnitPrice()); 30838 } 30839 if (element.hasFactorElement()) { 30840 composeDecimalCore("factor", element.getFactorElement(), false); 30841 composeDecimalExtras("factor", element.getFactorElement(), false); 30842 } 30843 if (element.hasNet()) { 30844 composeMoney("net", element.getNet()); 30845 } 30846 if (element.hasUdi()) { 30847 openArray("udi"); 30848 for (Reference e : element.getUdi()) 30849 composeReference(null, e); 30850 closeArray(); 30851 }; 30852 if (element.hasNoteNumber()) { 30853 openArray("noteNumber"); 30854 for (PositiveIntType e : element.getNoteNumber()) 30855 composePositiveIntCore(null, e, true); 30856 closeArray(); 30857 if (anyHasExtras(element.getNoteNumber())) { 30858 openArray("_noteNumber"); 30859 for (PositiveIntType e : element.getNoteNumber()) 30860 composePositiveIntExtras(null, e, true); 30861 closeArray(); 30862 } 30863 }; 30864 if (element.hasAdjudication()) { 30865 openArray("adjudication"); 30866 for (ExplanationOfBenefit.AdjudicationComponent e : element.getAdjudication()) 30867 composeExplanationOfBenefitAdjudicationComponent(null, e); 30868 closeArray(); 30869 }; 30870 if (element.hasSubDetail()) { 30871 openArray("subDetail"); 30872 for (ExplanationOfBenefit.SubDetailComponent e : element.getSubDetail()) 30873 composeExplanationOfBenefitSubDetailComponent(null, e); 30874 closeArray(); 30875 }; 30876 } 30877 30878 protected void composeExplanationOfBenefitSubDetailComponent(String name, ExplanationOfBenefit.SubDetailComponent element) throws IOException { 30879 if (element != null) { 30880 open(name); 30881 composeExplanationOfBenefitSubDetailComponentInner(element); 30882 close(); 30883 } 30884 } 30885 30886 protected void composeExplanationOfBenefitSubDetailComponentInner(ExplanationOfBenefit.SubDetailComponent element) throws IOException { 30887 composeBackbone(element); 30888 if (element.hasSequenceElement()) { 30889 composePositiveIntCore("sequence", element.getSequenceElement(), false); 30890 composePositiveIntExtras("sequence", element.getSequenceElement(), false); 30891 } 30892 if (element.hasType()) { 30893 composeCodeableConcept("type", element.getType()); 30894 } 30895 if (element.hasRevenue()) { 30896 composeCodeableConcept("revenue", element.getRevenue()); 30897 } 30898 if (element.hasCategory()) { 30899 composeCodeableConcept("category", element.getCategory()); 30900 } 30901 if (element.hasService()) { 30902 composeCodeableConcept("service", element.getService()); 30903 } 30904 if (element.hasModifier()) { 30905 openArray("modifier"); 30906 for (CodeableConcept e : element.getModifier()) 30907 composeCodeableConcept(null, e); 30908 closeArray(); 30909 }; 30910 if (element.hasProgramCode()) { 30911 openArray("programCode"); 30912 for (CodeableConcept e : element.getProgramCode()) 30913 composeCodeableConcept(null, e); 30914 closeArray(); 30915 }; 30916 if (element.hasQuantity()) { 30917 composeSimpleQuantity("quantity", element.getQuantity()); 30918 } 30919 if (element.hasUnitPrice()) { 30920 composeMoney("unitPrice", element.getUnitPrice()); 30921 } 30922 if (element.hasFactorElement()) { 30923 composeDecimalCore("factor", element.getFactorElement(), false); 30924 composeDecimalExtras("factor", element.getFactorElement(), false); 30925 } 30926 if (element.hasNet()) { 30927 composeMoney("net", element.getNet()); 30928 } 30929 if (element.hasUdi()) { 30930 openArray("udi"); 30931 for (Reference e : element.getUdi()) 30932 composeReference(null, e); 30933 closeArray(); 30934 }; 30935 if (element.hasNoteNumber()) { 30936 openArray("noteNumber"); 30937 for (PositiveIntType e : element.getNoteNumber()) 30938 composePositiveIntCore(null, e, true); 30939 closeArray(); 30940 if (anyHasExtras(element.getNoteNumber())) { 30941 openArray("_noteNumber"); 30942 for (PositiveIntType e : element.getNoteNumber()) 30943 composePositiveIntExtras(null, e, true); 30944 closeArray(); 30945 } 30946 }; 30947 if (element.hasAdjudication()) { 30948 openArray("adjudication"); 30949 for (ExplanationOfBenefit.AdjudicationComponent e : element.getAdjudication()) 30950 composeExplanationOfBenefitAdjudicationComponent(null, e); 30951 closeArray(); 30952 }; 30953 } 30954 30955 protected void composeExplanationOfBenefitAddedItemComponent(String name, ExplanationOfBenefit.AddedItemComponent element) throws IOException { 30956 if (element != null) { 30957 open(name); 30958 composeExplanationOfBenefitAddedItemComponentInner(element); 30959 close(); 30960 } 30961 } 30962 30963 protected void composeExplanationOfBenefitAddedItemComponentInner(ExplanationOfBenefit.AddedItemComponent element) throws IOException { 30964 composeBackbone(element); 30965 if (element.hasSequenceLinkId()) { 30966 openArray("sequenceLinkId"); 30967 for (PositiveIntType e : element.getSequenceLinkId()) 30968 composePositiveIntCore(null, e, true); 30969 closeArray(); 30970 if (anyHasExtras(element.getSequenceLinkId())) { 30971 openArray("_sequenceLinkId"); 30972 for (PositiveIntType e : element.getSequenceLinkId()) 30973 composePositiveIntExtras(null, e, true); 30974 closeArray(); 30975 } 30976 }; 30977 if (element.hasRevenue()) { 30978 composeCodeableConcept("revenue", element.getRevenue()); 30979 } 30980 if (element.hasCategory()) { 30981 composeCodeableConcept("category", element.getCategory()); 30982 } 30983 if (element.hasService()) { 30984 composeCodeableConcept("service", element.getService()); 30985 } 30986 if (element.hasModifier()) { 30987 openArray("modifier"); 30988 for (CodeableConcept e : element.getModifier()) 30989 composeCodeableConcept(null, e); 30990 closeArray(); 30991 }; 30992 if (element.hasFee()) { 30993 composeMoney("fee", element.getFee()); 30994 } 30995 if (element.hasNoteNumber()) { 30996 openArray("noteNumber"); 30997 for (PositiveIntType e : element.getNoteNumber()) 30998 composePositiveIntCore(null, e, true); 30999 closeArray(); 31000 if (anyHasExtras(element.getNoteNumber())) { 31001 openArray("_noteNumber"); 31002 for (PositiveIntType e : element.getNoteNumber()) 31003 composePositiveIntExtras(null, e, true); 31004 closeArray(); 31005 } 31006 }; 31007 if (element.hasAdjudication()) { 31008 openArray("adjudication"); 31009 for (ExplanationOfBenefit.AdjudicationComponent e : element.getAdjudication()) 31010 composeExplanationOfBenefitAdjudicationComponent(null, e); 31011 closeArray(); 31012 }; 31013 if (element.hasDetail()) { 31014 openArray("detail"); 31015 for (ExplanationOfBenefit.AddedItemsDetailComponent e : element.getDetail()) 31016 composeExplanationOfBenefitAddedItemsDetailComponent(null, e); 31017 closeArray(); 31018 }; 31019 } 31020 31021 protected void composeExplanationOfBenefitAddedItemsDetailComponent(String name, ExplanationOfBenefit.AddedItemsDetailComponent element) throws IOException { 31022 if (element != null) { 31023 open(name); 31024 composeExplanationOfBenefitAddedItemsDetailComponentInner(element); 31025 close(); 31026 } 31027 } 31028 31029 protected void composeExplanationOfBenefitAddedItemsDetailComponentInner(ExplanationOfBenefit.AddedItemsDetailComponent element) throws IOException { 31030 composeBackbone(element); 31031 if (element.hasRevenue()) { 31032 composeCodeableConcept("revenue", element.getRevenue()); 31033 } 31034 if (element.hasCategory()) { 31035 composeCodeableConcept("category", element.getCategory()); 31036 } 31037 if (element.hasService()) { 31038 composeCodeableConcept("service", element.getService()); 31039 } 31040 if (element.hasModifier()) { 31041 openArray("modifier"); 31042 for (CodeableConcept e : element.getModifier()) 31043 composeCodeableConcept(null, e); 31044 closeArray(); 31045 }; 31046 if (element.hasFee()) { 31047 composeMoney("fee", element.getFee()); 31048 } 31049 if (element.hasNoteNumber()) { 31050 openArray("noteNumber"); 31051 for (PositiveIntType e : element.getNoteNumber()) 31052 composePositiveIntCore(null, e, true); 31053 closeArray(); 31054 if (anyHasExtras(element.getNoteNumber())) { 31055 openArray("_noteNumber"); 31056 for (PositiveIntType e : element.getNoteNumber()) 31057 composePositiveIntExtras(null, e, true); 31058 closeArray(); 31059 } 31060 }; 31061 if (element.hasAdjudication()) { 31062 openArray("adjudication"); 31063 for (ExplanationOfBenefit.AdjudicationComponent e : element.getAdjudication()) 31064 composeExplanationOfBenefitAdjudicationComponent(null, e); 31065 closeArray(); 31066 }; 31067 } 31068 31069 protected void composeExplanationOfBenefitPaymentComponent(String name, ExplanationOfBenefit.PaymentComponent element) throws IOException { 31070 if (element != null) { 31071 open(name); 31072 composeExplanationOfBenefitPaymentComponentInner(element); 31073 close(); 31074 } 31075 } 31076 31077 protected void composeExplanationOfBenefitPaymentComponentInner(ExplanationOfBenefit.PaymentComponent element) throws IOException { 31078 composeBackbone(element); 31079 if (element.hasType()) { 31080 composeCodeableConcept("type", element.getType()); 31081 } 31082 if (element.hasAdjustment()) { 31083 composeMoney("adjustment", element.getAdjustment()); 31084 } 31085 if (element.hasAdjustmentReason()) { 31086 composeCodeableConcept("adjustmentReason", element.getAdjustmentReason()); 31087 } 31088 if (element.hasDateElement()) { 31089 composeDateCore("date", element.getDateElement(), false); 31090 composeDateExtras("date", element.getDateElement(), false); 31091 } 31092 if (element.hasAmount()) { 31093 composeMoney("amount", element.getAmount()); 31094 } 31095 if (element.hasIdentifier()) { 31096 composeIdentifier("identifier", element.getIdentifier()); 31097 } 31098 } 31099 31100 protected void composeExplanationOfBenefitNoteComponent(String name, ExplanationOfBenefit.NoteComponent element) throws IOException { 31101 if (element != null) { 31102 open(name); 31103 composeExplanationOfBenefitNoteComponentInner(element); 31104 close(); 31105 } 31106 } 31107 31108 protected void composeExplanationOfBenefitNoteComponentInner(ExplanationOfBenefit.NoteComponent element) throws IOException { 31109 composeBackbone(element); 31110 if (element.hasNumberElement()) { 31111 composePositiveIntCore("number", element.getNumberElement(), false); 31112 composePositiveIntExtras("number", element.getNumberElement(), false); 31113 } 31114 if (element.hasType()) { 31115 composeCodeableConcept("type", element.getType()); 31116 } 31117 if (element.hasTextElement()) { 31118 composeStringCore("text", element.getTextElement(), false); 31119 composeStringExtras("text", element.getTextElement(), false); 31120 } 31121 if (element.hasLanguage()) { 31122 composeCodeableConcept("language", element.getLanguage()); 31123 } 31124 } 31125 31126 protected void composeExplanationOfBenefitBenefitBalanceComponent(String name, ExplanationOfBenefit.BenefitBalanceComponent element) throws IOException { 31127 if (element != null) { 31128 open(name); 31129 composeExplanationOfBenefitBenefitBalanceComponentInner(element); 31130 close(); 31131 } 31132 } 31133 31134 protected void composeExplanationOfBenefitBenefitBalanceComponentInner(ExplanationOfBenefit.BenefitBalanceComponent element) throws IOException { 31135 composeBackbone(element); 31136 if (element.hasCategory()) { 31137 composeCodeableConcept("category", element.getCategory()); 31138 } 31139 if (element.hasSubCategory()) { 31140 composeCodeableConcept("subCategory", element.getSubCategory()); 31141 } 31142 if (element.hasExcludedElement()) { 31143 composeBooleanCore("excluded", element.getExcludedElement(), false); 31144 composeBooleanExtras("excluded", element.getExcludedElement(), false); 31145 } 31146 if (element.hasNameElement()) { 31147 composeStringCore("name", element.getNameElement(), false); 31148 composeStringExtras("name", element.getNameElement(), false); 31149 } 31150 if (element.hasDescriptionElement()) { 31151 composeStringCore("description", element.getDescriptionElement(), false); 31152 composeStringExtras("description", element.getDescriptionElement(), false); 31153 } 31154 if (element.hasNetwork()) { 31155 composeCodeableConcept("network", element.getNetwork()); 31156 } 31157 if (element.hasUnit()) { 31158 composeCodeableConcept("unit", element.getUnit()); 31159 } 31160 if (element.hasTerm()) { 31161 composeCodeableConcept("term", element.getTerm()); 31162 } 31163 if (element.hasFinancial()) { 31164 openArray("financial"); 31165 for (ExplanationOfBenefit.BenefitComponent e : element.getFinancial()) 31166 composeExplanationOfBenefitBenefitComponent(null, e); 31167 closeArray(); 31168 }; 31169 } 31170 31171 protected void composeExplanationOfBenefitBenefitComponent(String name, ExplanationOfBenefit.BenefitComponent element) throws IOException { 31172 if (element != null) { 31173 open(name); 31174 composeExplanationOfBenefitBenefitComponentInner(element); 31175 close(); 31176 } 31177 } 31178 31179 protected void composeExplanationOfBenefitBenefitComponentInner(ExplanationOfBenefit.BenefitComponent element) throws IOException { 31180 composeBackbone(element); 31181 if (element.hasType()) { 31182 composeCodeableConcept("type", element.getType()); 31183 } 31184 if (element.hasAllowed()) { 31185 composeType("allowed", element.getAllowed()); 31186 } 31187 if (element.hasUsed()) { 31188 composeType("used", element.getUsed()); 31189 } 31190 } 31191 31192 protected void composeFamilyMemberHistory(String name, FamilyMemberHistory element) throws IOException { 31193 if (element != null) { 31194 prop("resourceType", name); 31195 composeFamilyMemberHistoryInner(element); 31196 } 31197 } 31198 31199 protected void composeFamilyMemberHistoryInner(FamilyMemberHistory element) throws IOException { 31200 composeDomainResourceElements(element); 31201 if (element.hasIdentifier()) { 31202 openArray("identifier"); 31203 for (Identifier e : element.getIdentifier()) 31204 composeIdentifier(null, e); 31205 closeArray(); 31206 }; 31207 if (element.hasDefinition()) { 31208 openArray("definition"); 31209 for (Reference e : element.getDefinition()) 31210 composeReference(null, e); 31211 closeArray(); 31212 }; 31213 if (element.hasStatusElement()) { 31214 composeEnumerationCore("status", element.getStatusElement(), new FamilyMemberHistory.FamilyHistoryStatusEnumFactory(), false); 31215 composeEnumerationExtras("status", element.getStatusElement(), new FamilyMemberHistory.FamilyHistoryStatusEnumFactory(), false); 31216 } 31217 if (element.hasNotDoneElement()) { 31218 composeBooleanCore("notDone", element.getNotDoneElement(), false); 31219 composeBooleanExtras("notDone", element.getNotDoneElement(), false); 31220 } 31221 if (element.hasNotDoneReason()) { 31222 composeCodeableConcept("notDoneReason", element.getNotDoneReason()); 31223 } 31224 if (element.hasPatient()) { 31225 composeReference("patient", element.getPatient()); 31226 } 31227 if (element.hasDateElement()) { 31228 composeDateTimeCore("date", element.getDateElement(), false); 31229 composeDateTimeExtras("date", element.getDateElement(), false); 31230 } 31231 if (element.hasNameElement()) { 31232 composeStringCore("name", element.getNameElement(), false); 31233 composeStringExtras("name", element.getNameElement(), false); 31234 } 31235 if (element.hasRelationship()) { 31236 composeCodeableConcept("relationship", element.getRelationship()); 31237 } 31238 if (element.hasGenderElement()) { 31239 composeEnumerationCore("gender", element.getGenderElement(), new Enumerations.AdministrativeGenderEnumFactory(), false); 31240 composeEnumerationExtras("gender", element.getGenderElement(), new Enumerations.AdministrativeGenderEnumFactory(), false); 31241 } 31242 if (element.hasBorn()) { 31243 composeType("born", element.getBorn()); 31244 } 31245 if (element.hasAge()) { 31246 composeType("age", element.getAge()); 31247 } 31248 if (element.hasEstimatedAgeElement()) { 31249 composeBooleanCore("estimatedAge", element.getEstimatedAgeElement(), false); 31250 composeBooleanExtras("estimatedAge", element.getEstimatedAgeElement(), false); 31251 } 31252 if (element.hasDeceased()) { 31253 composeType("deceased", element.getDeceased()); 31254 } 31255 if (element.hasReasonCode()) { 31256 openArray("reasonCode"); 31257 for (CodeableConcept e : element.getReasonCode()) 31258 composeCodeableConcept(null, e); 31259 closeArray(); 31260 }; 31261 if (element.hasReasonReference()) { 31262 openArray("reasonReference"); 31263 for (Reference e : element.getReasonReference()) 31264 composeReference(null, e); 31265 closeArray(); 31266 }; 31267 if (element.hasNote()) { 31268 openArray("note"); 31269 for (Annotation e : element.getNote()) 31270 composeAnnotation(null, e); 31271 closeArray(); 31272 }; 31273 if (element.hasCondition()) { 31274 openArray("condition"); 31275 for (FamilyMemberHistory.FamilyMemberHistoryConditionComponent e : element.getCondition()) 31276 composeFamilyMemberHistoryFamilyMemberHistoryConditionComponent(null, e); 31277 closeArray(); 31278 }; 31279 } 31280 31281 protected void composeFamilyMemberHistoryFamilyMemberHistoryConditionComponent(String name, FamilyMemberHistory.FamilyMemberHistoryConditionComponent element) throws IOException { 31282 if (element != null) { 31283 open(name); 31284 composeFamilyMemberHistoryFamilyMemberHistoryConditionComponentInner(element); 31285 close(); 31286 } 31287 } 31288 31289 protected void composeFamilyMemberHistoryFamilyMemberHistoryConditionComponentInner(FamilyMemberHistory.FamilyMemberHistoryConditionComponent element) throws IOException { 31290 composeBackbone(element); 31291 if (element.hasCode()) { 31292 composeCodeableConcept("code", element.getCode()); 31293 } 31294 if (element.hasOutcome()) { 31295 composeCodeableConcept("outcome", element.getOutcome()); 31296 } 31297 if (element.hasOnset()) { 31298 composeType("onset", element.getOnset()); 31299 } 31300 if (element.hasNote()) { 31301 openArray("note"); 31302 for (Annotation e : element.getNote()) 31303 composeAnnotation(null, e); 31304 closeArray(); 31305 }; 31306 } 31307 31308 protected void composeFlag(String name, Flag element) throws IOException { 31309 if (element != null) { 31310 prop("resourceType", name); 31311 composeFlagInner(element); 31312 } 31313 } 31314 31315 protected void composeFlagInner(Flag element) throws IOException { 31316 composeDomainResourceElements(element); 31317 if (element.hasIdentifier()) { 31318 openArray("identifier"); 31319 for (Identifier e : element.getIdentifier()) 31320 composeIdentifier(null, e); 31321 closeArray(); 31322 }; 31323 if (element.hasStatusElement()) { 31324 composeEnumerationCore("status", element.getStatusElement(), new Flag.FlagStatusEnumFactory(), false); 31325 composeEnumerationExtras("status", element.getStatusElement(), new Flag.FlagStatusEnumFactory(), false); 31326 } 31327 if (element.hasCategory()) { 31328 composeCodeableConcept("category", element.getCategory()); 31329 } 31330 if (element.hasCode()) { 31331 composeCodeableConcept("code", element.getCode()); 31332 } 31333 if (element.hasSubject()) { 31334 composeReference("subject", element.getSubject()); 31335 } 31336 if (element.hasPeriod()) { 31337 composePeriod("period", element.getPeriod()); 31338 } 31339 if (element.hasEncounter()) { 31340 composeReference("encounter", element.getEncounter()); 31341 } 31342 if (element.hasAuthor()) { 31343 composeReference("author", element.getAuthor()); 31344 } 31345 } 31346 31347 protected void composeGoal(String name, Goal element) throws IOException { 31348 if (element != null) { 31349 prop("resourceType", name); 31350 composeGoalInner(element); 31351 } 31352 } 31353 31354 protected void composeGoalInner(Goal element) throws IOException { 31355 composeDomainResourceElements(element); 31356 if (element.hasIdentifier()) { 31357 openArray("identifier"); 31358 for (Identifier e : element.getIdentifier()) 31359 composeIdentifier(null, e); 31360 closeArray(); 31361 }; 31362 if (element.hasStatusElement()) { 31363 composeEnumerationCore("status", element.getStatusElement(), new Goal.GoalStatusEnumFactory(), false); 31364 composeEnumerationExtras("status", element.getStatusElement(), new Goal.GoalStatusEnumFactory(), false); 31365 } 31366 if (element.hasCategory()) { 31367 openArray("category"); 31368 for (CodeableConcept e : element.getCategory()) 31369 composeCodeableConcept(null, e); 31370 closeArray(); 31371 }; 31372 if (element.hasPriority()) { 31373 composeCodeableConcept("priority", element.getPriority()); 31374 } 31375 if (element.hasDescription()) { 31376 composeCodeableConcept("description", element.getDescription()); 31377 } 31378 if (element.hasSubject()) { 31379 composeReference("subject", element.getSubject()); 31380 } 31381 if (element.hasStart()) { 31382 composeType("start", element.getStart()); 31383 } 31384 if (element.hasTarget()) { 31385 composeGoalGoalTargetComponent("target", element.getTarget()); 31386 } 31387 if (element.hasStatusDateElement()) { 31388 composeDateCore("statusDate", element.getStatusDateElement(), false); 31389 composeDateExtras("statusDate", element.getStatusDateElement(), false); 31390 } 31391 if (element.hasStatusReasonElement()) { 31392 composeStringCore("statusReason", element.getStatusReasonElement(), false); 31393 composeStringExtras("statusReason", element.getStatusReasonElement(), false); 31394 } 31395 if (element.hasExpressedBy()) { 31396 composeReference("expressedBy", element.getExpressedBy()); 31397 } 31398 if (element.hasAddresses()) { 31399 openArray("addresses"); 31400 for (Reference e : element.getAddresses()) 31401 composeReference(null, e); 31402 closeArray(); 31403 }; 31404 if (element.hasNote()) { 31405 openArray("note"); 31406 for (Annotation e : element.getNote()) 31407 composeAnnotation(null, e); 31408 closeArray(); 31409 }; 31410 if (element.hasOutcomeCode()) { 31411 openArray("outcomeCode"); 31412 for (CodeableConcept e : element.getOutcomeCode()) 31413 composeCodeableConcept(null, e); 31414 closeArray(); 31415 }; 31416 if (element.hasOutcomeReference()) { 31417 openArray("outcomeReference"); 31418 for (Reference e : element.getOutcomeReference()) 31419 composeReference(null, e); 31420 closeArray(); 31421 }; 31422 } 31423 31424 protected void composeGoalGoalTargetComponent(String name, Goal.GoalTargetComponent element) throws IOException { 31425 if (element != null) { 31426 open(name); 31427 composeGoalGoalTargetComponentInner(element); 31428 close(); 31429 } 31430 } 31431 31432 protected void composeGoalGoalTargetComponentInner(Goal.GoalTargetComponent element) throws IOException { 31433 composeBackbone(element); 31434 if (element.hasMeasure()) { 31435 composeCodeableConcept("measure", element.getMeasure()); 31436 } 31437 if (element.hasDetail()) { 31438 composeType("detail", element.getDetail()); 31439 } 31440 if (element.hasDue()) { 31441 composeType("due", element.getDue()); 31442 } 31443 } 31444 31445 protected void composeGraphDefinition(String name, GraphDefinition element) throws IOException { 31446 if (element != null) { 31447 prop("resourceType", name); 31448 composeGraphDefinitionInner(element); 31449 } 31450 } 31451 31452 protected void composeGraphDefinitionInner(GraphDefinition element) throws IOException { 31453 composeDomainResourceElements(element); 31454 if (element.hasUrlElement()) { 31455 composeUriCore("url", element.getUrlElement(), false); 31456 composeUriExtras("url", element.getUrlElement(), false); 31457 } 31458 if (element.hasVersionElement()) { 31459 composeStringCore("version", element.getVersionElement(), false); 31460 composeStringExtras("version", element.getVersionElement(), false); 31461 } 31462 if (element.hasNameElement()) { 31463 composeStringCore("name", element.getNameElement(), false); 31464 composeStringExtras("name", element.getNameElement(), false); 31465 } 31466 if (element.hasStatusElement()) { 31467 composeEnumerationCore("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 31468 composeEnumerationExtras("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 31469 } 31470 if (element.hasExperimentalElement()) { 31471 composeBooleanCore("experimental", element.getExperimentalElement(), false); 31472 composeBooleanExtras("experimental", element.getExperimentalElement(), false); 31473 } 31474 if (element.hasDateElement()) { 31475 composeDateTimeCore("date", element.getDateElement(), false); 31476 composeDateTimeExtras("date", element.getDateElement(), false); 31477 } 31478 if (element.hasPublisherElement()) { 31479 composeStringCore("publisher", element.getPublisherElement(), false); 31480 composeStringExtras("publisher", element.getPublisherElement(), false); 31481 } 31482 if (element.hasContact()) { 31483 openArray("contact"); 31484 for (ContactDetail e : element.getContact()) 31485 composeContactDetail(null, e); 31486 closeArray(); 31487 }; 31488 if (element.hasDescriptionElement()) { 31489 composeMarkdownCore("description", element.getDescriptionElement(), false); 31490 composeMarkdownExtras("description", element.getDescriptionElement(), false); 31491 } 31492 if (element.hasUseContext()) { 31493 openArray("useContext"); 31494 for (UsageContext e : element.getUseContext()) 31495 composeUsageContext(null, e); 31496 closeArray(); 31497 }; 31498 if (element.hasJurisdiction()) { 31499 openArray("jurisdiction"); 31500 for (CodeableConcept e : element.getJurisdiction()) 31501 composeCodeableConcept(null, e); 31502 closeArray(); 31503 }; 31504 if (element.hasPurposeElement()) { 31505 composeMarkdownCore("purpose", element.getPurposeElement(), false); 31506 composeMarkdownExtras("purpose", element.getPurposeElement(), false); 31507 } 31508 if (element.hasStartElement()) { 31509 composeCodeCore("start", element.getStartElement(), false); 31510 composeCodeExtras("start", element.getStartElement(), false); 31511 } 31512 if (element.hasProfileElement()) { 31513 composeUriCore("profile", element.getProfileElement(), false); 31514 composeUriExtras("profile", element.getProfileElement(), false); 31515 } 31516 if (element.hasLink()) { 31517 openArray("link"); 31518 for (GraphDefinition.GraphDefinitionLinkComponent e : element.getLink()) 31519 composeGraphDefinitionGraphDefinitionLinkComponent(null, e); 31520 closeArray(); 31521 }; 31522 } 31523 31524 protected void composeGraphDefinitionGraphDefinitionLinkComponent(String name, GraphDefinition.GraphDefinitionLinkComponent element) throws IOException { 31525 if (element != null) { 31526 open(name); 31527 composeGraphDefinitionGraphDefinitionLinkComponentInner(element); 31528 close(); 31529 } 31530 } 31531 31532 protected void composeGraphDefinitionGraphDefinitionLinkComponentInner(GraphDefinition.GraphDefinitionLinkComponent element) throws IOException { 31533 composeBackbone(element); 31534 if (element.hasPathElement()) { 31535 composeStringCore("path", element.getPathElement(), false); 31536 composeStringExtras("path", element.getPathElement(), false); 31537 } 31538 if (element.hasSliceNameElement()) { 31539 composeStringCore("sliceName", element.getSliceNameElement(), false); 31540 composeStringExtras("sliceName", element.getSliceNameElement(), false); 31541 } 31542 if (element.hasMinElement()) { 31543 composeIntegerCore("min", element.getMinElement(), false); 31544 composeIntegerExtras("min", element.getMinElement(), false); 31545 } 31546 if (element.hasMaxElement()) { 31547 composeStringCore("max", element.getMaxElement(), false); 31548 composeStringExtras("max", element.getMaxElement(), false); 31549 } 31550 if (element.hasDescriptionElement()) { 31551 composeStringCore("description", element.getDescriptionElement(), false); 31552 composeStringExtras("description", element.getDescriptionElement(), false); 31553 } 31554 if (element.hasTarget()) { 31555 openArray("target"); 31556 for (GraphDefinition.GraphDefinitionLinkTargetComponent e : element.getTarget()) 31557 composeGraphDefinitionGraphDefinitionLinkTargetComponent(null, e); 31558 closeArray(); 31559 }; 31560 } 31561 31562 protected void composeGraphDefinitionGraphDefinitionLinkTargetComponent(String name, GraphDefinition.GraphDefinitionLinkTargetComponent element) throws IOException { 31563 if (element != null) { 31564 open(name); 31565 composeGraphDefinitionGraphDefinitionLinkTargetComponentInner(element); 31566 close(); 31567 } 31568 } 31569 31570 protected void composeGraphDefinitionGraphDefinitionLinkTargetComponentInner(GraphDefinition.GraphDefinitionLinkTargetComponent element) throws IOException { 31571 composeBackbone(element); 31572 if (element.hasTypeElement()) { 31573 composeCodeCore("type", element.getTypeElement(), false); 31574 composeCodeExtras("type", element.getTypeElement(), false); 31575 } 31576 if (element.hasProfileElement()) { 31577 composeUriCore("profile", element.getProfileElement(), false); 31578 composeUriExtras("profile", element.getProfileElement(), false); 31579 } 31580 if (element.hasCompartment()) { 31581 openArray("compartment"); 31582 for (GraphDefinition.GraphDefinitionLinkTargetCompartmentComponent e : element.getCompartment()) 31583 composeGraphDefinitionGraphDefinitionLinkTargetCompartmentComponent(null, e); 31584 closeArray(); 31585 }; 31586 if (element.hasLink()) { 31587 openArray("link"); 31588 for (GraphDefinition.GraphDefinitionLinkComponent e : element.getLink()) 31589 composeGraphDefinitionGraphDefinitionLinkComponent(null, e); 31590 closeArray(); 31591 }; 31592 } 31593 31594 protected void composeGraphDefinitionGraphDefinitionLinkTargetCompartmentComponent(String name, GraphDefinition.GraphDefinitionLinkTargetCompartmentComponent element) throws IOException { 31595 if (element != null) { 31596 open(name); 31597 composeGraphDefinitionGraphDefinitionLinkTargetCompartmentComponentInner(element); 31598 close(); 31599 } 31600 } 31601 31602 protected void composeGraphDefinitionGraphDefinitionLinkTargetCompartmentComponentInner(GraphDefinition.GraphDefinitionLinkTargetCompartmentComponent element) throws IOException { 31603 composeBackbone(element); 31604 if (element.hasCodeElement()) { 31605 composeEnumerationCore("code", element.getCodeElement(), new GraphDefinition.CompartmentCodeEnumFactory(), false); 31606 composeEnumerationExtras("code", element.getCodeElement(), new GraphDefinition.CompartmentCodeEnumFactory(), false); 31607 } 31608 if (element.hasRuleElement()) { 31609 composeEnumerationCore("rule", element.getRuleElement(), new GraphDefinition.GraphCompartmentRuleEnumFactory(), false); 31610 composeEnumerationExtras("rule", element.getRuleElement(), new GraphDefinition.GraphCompartmentRuleEnumFactory(), false); 31611 } 31612 if (element.hasExpressionElement()) { 31613 composeStringCore("expression", element.getExpressionElement(), false); 31614 composeStringExtras("expression", element.getExpressionElement(), false); 31615 } 31616 if (element.hasDescriptionElement()) { 31617 composeStringCore("description", element.getDescriptionElement(), false); 31618 composeStringExtras("description", element.getDescriptionElement(), false); 31619 } 31620 } 31621 31622 protected void composeGroup(String name, Group element) throws IOException { 31623 if (element != null) { 31624 prop("resourceType", name); 31625 composeGroupInner(element); 31626 } 31627 } 31628 31629 protected void composeGroupInner(Group element) throws IOException { 31630 composeDomainResourceElements(element); 31631 if (element.hasIdentifier()) { 31632 openArray("identifier"); 31633 for (Identifier e : element.getIdentifier()) 31634 composeIdentifier(null, e); 31635 closeArray(); 31636 }; 31637 if (element.hasActiveElement()) { 31638 composeBooleanCore("active", element.getActiveElement(), false); 31639 composeBooleanExtras("active", element.getActiveElement(), false); 31640 } 31641 if (element.hasTypeElement()) { 31642 composeEnumerationCore("type", element.getTypeElement(), new Group.GroupTypeEnumFactory(), false); 31643 composeEnumerationExtras("type", element.getTypeElement(), new Group.GroupTypeEnumFactory(), false); 31644 } 31645 if (element.hasActualElement()) { 31646 composeBooleanCore("actual", element.getActualElement(), false); 31647 composeBooleanExtras("actual", element.getActualElement(), false); 31648 } 31649 if (element.hasCode()) { 31650 composeCodeableConcept("code", element.getCode()); 31651 } 31652 if (element.hasNameElement()) { 31653 composeStringCore("name", element.getNameElement(), false); 31654 composeStringExtras("name", element.getNameElement(), false); 31655 } 31656 if (element.hasQuantityElement()) { 31657 composeUnsignedIntCore("quantity", element.getQuantityElement(), false); 31658 composeUnsignedIntExtras("quantity", element.getQuantityElement(), false); 31659 } 31660 if (element.hasCharacteristic()) { 31661 openArray("characteristic"); 31662 for (Group.GroupCharacteristicComponent e : element.getCharacteristic()) 31663 composeGroupGroupCharacteristicComponent(null, e); 31664 closeArray(); 31665 }; 31666 if (element.hasMember()) { 31667 openArray("member"); 31668 for (Group.GroupMemberComponent e : element.getMember()) 31669 composeGroupGroupMemberComponent(null, e); 31670 closeArray(); 31671 }; 31672 } 31673 31674 protected void composeGroupGroupCharacteristicComponent(String name, Group.GroupCharacteristicComponent element) throws IOException { 31675 if (element != null) { 31676 open(name); 31677 composeGroupGroupCharacteristicComponentInner(element); 31678 close(); 31679 } 31680 } 31681 31682 protected void composeGroupGroupCharacteristicComponentInner(Group.GroupCharacteristicComponent element) throws IOException { 31683 composeBackbone(element); 31684 if (element.hasCode()) { 31685 composeCodeableConcept("code", element.getCode()); 31686 } 31687 if (element.hasValue()) { 31688 composeType("value", element.getValue()); 31689 } 31690 if (element.hasExcludeElement()) { 31691 composeBooleanCore("exclude", element.getExcludeElement(), false); 31692 composeBooleanExtras("exclude", element.getExcludeElement(), false); 31693 } 31694 if (element.hasPeriod()) { 31695 composePeriod("period", element.getPeriod()); 31696 } 31697 } 31698 31699 protected void composeGroupGroupMemberComponent(String name, Group.GroupMemberComponent element) throws IOException { 31700 if (element != null) { 31701 open(name); 31702 composeGroupGroupMemberComponentInner(element); 31703 close(); 31704 } 31705 } 31706 31707 protected void composeGroupGroupMemberComponentInner(Group.GroupMemberComponent element) throws IOException { 31708 composeBackbone(element); 31709 if (element.hasEntity()) { 31710 composeReference("entity", element.getEntity()); 31711 } 31712 if (element.hasPeriod()) { 31713 composePeriod("period", element.getPeriod()); 31714 } 31715 if (element.hasInactiveElement()) { 31716 composeBooleanCore("inactive", element.getInactiveElement(), false); 31717 composeBooleanExtras("inactive", element.getInactiveElement(), false); 31718 } 31719 } 31720 31721 protected void composeGuidanceResponse(String name, GuidanceResponse element) throws IOException { 31722 if (element != null) { 31723 prop("resourceType", name); 31724 composeGuidanceResponseInner(element); 31725 } 31726 } 31727 31728 protected void composeGuidanceResponseInner(GuidanceResponse element) throws IOException { 31729 composeDomainResourceElements(element); 31730 if (element.hasRequestIdElement()) { 31731 composeIdCore("requestId", element.getRequestIdElement(), false); 31732 composeIdExtras("requestId", element.getRequestIdElement(), false); 31733 } 31734 if (element.hasIdentifier()) { 31735 composeIdentifier("identifier", element.getIdentifier()); 31736 } 31737 if (element.hasModule()) { 31738 composeReference("module", element.getModule()); 31739 } 31740 if (element.hasStatusElement()) { 31741 composeEnumerationCore("status", element.getStatusElement(), new GuidanceResponse.GuidanceResponseStatusEnumFactory(), false); 31742 composeEnumerationExtras("status", element.getStatusElement(), new GuidanceResponse.GuidanceResponseStatusEnumFactory(), false); 31743 } 31744 if (element.hasSubject()) { 31745 composeReference("subject", element.getSubject()); 31746 } 31747 if (element.hasContext()) { 31748 composeReference("context", element.getContext()); 31749 } 31750 if (element.hasOccurrenceDateTimeElement()) { 31751 composeDateTimeCore("occurrenceDateTime", element.getOccurrenceDateTimeElement(), false); 31752 composeDateTimeExtras("occurrenceDateTime", element.getOccurrenceDateTimeElement(), false); 31753 } 31754 if (element.hasPerformer()) { 31755 composeReference("performer", element.getPerformer()); 31756 } 31757 if (element.hasReason()) { 31758 composeType("reason", element.getReason()); 31759 } 31760 if (element.hasNote()) { 31761 openArray("note"); 31762 for (Annotation e : element.getNote()) 31763 composeAnnotation(null, e); 31764 closeArray(); 31765 }; 31766 if (element.hasEvaluationMessage()) { 31767 openArray("evaluationMessage"); 31768 for (Reference e : element.getEvaluationMessage()) 31769 composeReference(null, e); 31770 closeArray(); 31771 }; 31772 if (element.hasOutputParameters()) { 31773 composeReference("outputParameters", element.getOutputParameters()); 31774 } 31775 if (element.hasResult()) { 31776 composeReference("result", element.getResult()); 31777 } 31778 if (element.hasDataRequirement()) { 31779 openArray("dataRequirement"); 31780 for (DataRequirement e : element.getDataRequirement()) 31781 composeDataRequirement(null, e); 31782 closeArray(); 31783 }; 31784 } 31785 31786 protected void composeHealthcareService(String name, HealthcareService element) throws IOException { 31787 if (element != null) { 31788 prop("resourceType", name); 31789 composeHealthcareServiceInner(element); 31790 } 31791 } 31792 31793 protected void composeHealthcareServiceInner(HealthcareService element) throws IOException { 31794 composeDomainResourceElements(element); 31795 if (element.hasIdentifier()) { 31796 openArray("identifier"); 31797 for (Identifier e : element.getIdentifier()) 31798 composeIdentifier(null, e); 31799 closeArray(); 31800 }; 31801 if (element.hasActiveElement()) { 31802 composeBooleanCore("active", element.getActiveElement(), false); 31803 composeBooleanExtras("active", element.getActiveElement(), false); 31804 } 31805 if (element.hasProvidedBy()) { 31806 composeReference("providedBy", element.getProvidedBy()); 31807 } 31808 if (element.hasCategory()) { 31809 composeCodeableConcept("category", element.getCategory()); 31810 } 31811 if (element.hasType()) { 31812 openArray("type"); 31813 for (CodeableConcept e : element.getType()) 31814 composeCodeableConcept(null, e); 31815 closeArray(); 31816 }; 31817 if (element.hasSpecialty()) { 31818 openArray("specialty"); 31819 for (CodeableConcept e : element.getSpecialty()) 31820 composeCodeableConcept(null, e); 31821 closeArray(); 31822 }; 31823 if (element.hasLocation()) { 31824 openArray("location"); 31825 for (Reference e : element.getLocation()) 31826 composeReference(null, e); 31827 closeArray(); 31828 }; 31829 if (element.hasNameElement()) { 31830 composeStringCore("name", element.getNameElement(), false); 31831 composeStringExtras("name", element.getNameElement(), false); 31832 } 31833 if (element.hasCommentElement()) { 31834 composeStringCore("comment", element.getCommentElement(), false); 31835 composeStringExtras("comment", element.getCommentElement(), false); 31836 } 31837 if (element.hasExtraDetailsElement()) { 31838 composeStringCore("extraDetails", element.getExtraDetailsElement(), false); 31839 composeStringExtras("extraDetails", element.getExtraDetailsElement(), false); 31840 } 31841 if (element.hasPhoto()) { 31842 composeAttachment("photo", element.getPhoto()); 31843 } 31844 if (element.hasTelecom()) { 31845 openArray("telecom"); 31846 for (ContactPoint e : element.getTelecom()) 31847 composeContactPoint(null, e); 31848 closeArray(); 31849 }; 31850 if (element.hasCoverageArea()) { 31851 openArray("coverageArea"); 31852 for (Reference e : element.getCoverageArea()) 31853 composeReference(null, e); 31854 closeArray(); 31855 }; 31856 if (element.hasServiceProvisionCode()) { 31857 openArray("serviceProvisionCode"); 31858 for (CodeableConcept e : element.getServiceProvisionCode()) 31859 composeCodeableConcept(null, e); 31860 closeArray(); 31861 }; 31862 if (element.hasEligibility()) { 31863 composeCodeableConcept("eligibility", element.getEligibility()); 31864 } 31865 if (element.hasEligibilityNoteElement()) { 31866 composeStringCore("eligibilityNote", element.getEligibilityNoteElement(), false); 31867 composeStringExtras("eligibilityNote", element.getEligibilityNoteElement(), false); 31868 } 31869 if (element.hasProgramName()) { 31870 openArray("programName"); 31871 for (StringType e : element.getProgramName()) 31872 composeStringCore(null, e, true); 31873 closeArray(); 31874 if (anyHasExtras(element.getProgramName())) { 31875 openArray("_programName"); 31876 for (StringType e : element.getProgramName()) 31877 composeStringExtras(null, e, true); 31878 closeArray(); 31879 } 31880 }; 31881 if (element.hasCharacteristic()) { 31882 openArray("characteristic"); 31883 for (CodeableConcept e : element.getCharacteristic()) 31884 composeCodeableConcept(null, e); 31885 closeArray(); 31886 }; 31887 if (element.hasReferralMethod()) { 31888 openArray("referralMethod"); 31889 for (CodeableConcept e : element.getReferralMethod()) 31890 composeCodeableConcept(null, e); 31891 closeArray(); 31892 }; 31893 if (element.hasAppointmentRequiredElement()) { 31894 composeBooleanCore("appointmentRequired", element.getAppointmentRequiredElement(), false); 31895 composeBooleanExtras("appointmentRequired", element.getAppointmentRequiredElement(), false); 31896 } 31897 if (element.hasAvailableTime()) { 31898 openArray("availableTime"); 31899 for (HealthcareService.HealthcareServiceAvailableTimeComponent e : element.getAvailableTime()) 31900 composeHealthcareServiceHealthcareServiceAvailableTimeComponent(null, e); 31901 closeArray(); 31902 }; 31903 if (element.hasNotAvailable()) { 31904 openArray("notAvailable"); 31905 for (HealthcareService.HealthcareServiceNotAvailableComponent e : element.getNotAvailable()) 31906 composeHealthcareServiceHealthcareServiceNotAvailableComponent(null, e); 31907 closeArray(); 31908 }; 31909 if (element.hasAvailabilityExceptionsElement()) { 31910 composeStringCore("availabilityExceptions", element.getAvailabilityExceptionsElement(), false); 31911 composeStringExtras("availabilityExceptions", element.getAvailabilityExceptionsElement(), false); 31912 } 31913 if (element.hasEndpoint()) { 31914 openArray("endpoint"); 31915 for (Reference e : element.getEndpoint()) 31916 composeReference(null, e); 31917 closeArray(); 31918 }; 31919 } 31920 31921 protected void composeHealthcareServiceHealthcareServiceAvailableTimeComponent(String name, HealthcareService.HealthcareServiceAvailableTimeComponent element) throws IOException { 31922 if (element != null) { 31923 open(name); 31924 composeHealthcareServiceHealthcareServiceAvailableTimeComponentInner(element); 31925 close(); 31926 } 31927 } 31928 31929 protected void composeHealthcareServiceHealthcareServiceAvailableTimeComponentInner(HealthcareService.HealthcareServiceAvailableTimeComponent element) throws IOException { 31930 composeBackbone(element); 31931 if (element.hasDaysOfWeek()) { 31932 openArray("daysOfWeek"); 31933 for (Enumeration<HealthcareService.DaysOfWeek> e : element.getDaysOfWeek()) 31934 composeEnumerationCore(null, e, new HealthcareService.DaysOfWeekEnumFactory(), true); 31935 closeArray(); 31936 if (anyHasExtras(element.getDaysOfWeek())) { 31937 openArray("_daysOfWeek"); 31938 for (Enumeration<HealthcareService.DaysOfWeek> e : element.getDaysOfWeek()) 31939 composeEnumerationExtras(null, e, new HealthcareService.DaysOfWeekEnumFactory(), true); 31940 closeArray(); 31941 } 31942 }; 31943 if (element.hasAllDayElement()) { 31944 composeBooleanCore("allDay", element.getAllDayElement(), false); 31945 composeBooleanExtras("allDay", element.getAllDayElement(), false); 31946 } 31947 if (element.hasAvailableStartTimeElement()) { 31948 composeTimeCore("availableStartTime", element.getAvailableStartTimeElement(), false); 31949 composeTimeExtras("availableStartTime", element.getAvailableStartTimeElement(), false); 31950 } 31951 if (element.hasAvailableEndTimeElement()) { 31952 composeTimeCore("availableEndTime", element.getAvailableEndTimeElement(), false); 31953 composeTimeExtras("availableEndTime", element.getAvailableEndTimeElement(), false); 31954 } 31955 } 31956 31957 protected void composeHealthcareServiceHealthcareServiceNotAvailableComponent(String name, HealthcareService.HealthcareServiceNotAvailableComponent element) throws IOException { 31958 if (element != null) { 31959 open(name); 31960 composeHealthcareServiceHealthcareServiceNotAvailableComponentInner(element); 31961 close(); 31962 } 31963 } 31964 31965 protected void composeHealthcareServiceHealthcareServiceNotAvailableComponentInner(HealthcareService.HealthcareServiceNotAvailableComponent element) throws IOException { 31966 composeBackbone(element); 31967 if (element.hasDescriptionElement()) { 31968 composeStringCore("description", element.getDescriptionElement(), false); 31969 composeStringExtras("description", element.getDescriptionElement(), false); 31970 } 31971 if (element.hasDuring()) { 31972 composePeriod("during", element.getDuring()); 31973 } 31974 } 31975 31976 protected void composeImagingManifest(String name, ImagingManifest element) throws IOException { 31977 if (element != null) { 31978 prop("resourceType", name); 31979 composeImagingManifestInner(element); 31980 } 31981 } 31982 31983 protected void composeImagingManifestInner(ImagingManifest element) throws IOException { 31984 composeDomainResourceElements(element); 31985 if (element.hasIdentifier()) { 31986 composeIdentifier("identifier", element.getIdentifier()); 31987 } 31988 if (element.hasPatient()) { 31989 composeReference("patient", element.getPatient()); 31990 } 31991 if (element.hasAuthoringTimeElement()) { 31992 composeDateTimeCore("authoringTime", element.getAuthoringTimeElement(), false); 31993 composeDateTimeExtras("authoringTime", element.getAuthoringTimeElement(), false); 31994 } 31995 if (element.hasAuthor()) { 31996 composeReference("author", element.getAuthor()); 31997 } 31998 if (element.hasDescriptionElement()) { 31999 composeStringCore("description", element.getDescriptionElement(), false); 32000 composeStringExtras("description", element.getDescriptionElement(), false); 32001 } 32002 if (element.hasStudy()) { 32003 openArray("study"); 32004 for (ImagingManifest.StudyComponent e : element.getStudy()) 32005 composeImagingManifestStudyComponent(null, e); 32006 closeArray(); 32007 }; 32008 } 32009 32010 protected void composeImagingManifestStudyComponent(String name, ImagingManifest.StudyComponent element) throws IOException { 32011 if (element != null) { 32012 open(name); 32013 composeImagingManifestStudyComponentInner(element); 32014 close(); 32015 } 32016 } 32017 32018 protected void composeImagingManifestStudyComponentInner(ImagingManifest.StudyComponent element) throws IOException { 32019 composeBackbone(element); 32020 if (element.hasUidElement()) { 32021 composeOidCore("uid", element.getUidElement(), false); 32022 composeOidExtras("uid", element.getUidElement(), false); 32023 } 32024 if (element.hasImagingStudy()) { 32025 composeReference("imagingStudy", element.getImagingStudy()); 32026 } 32027 if (element.hasEndpoint()) { 32028 openArray("endpoint"); 32029 for (Reference e : element.getEndpoint()) 32030 composeReference(null, e); 32031 closeArray(); 32032 }; 32033 if (element.hasSeries()) { 32034 openArray("series"); 32035 for (ImagingManifest.SeriesComponent e : element.getSeries()) 32036 composeImagingManifestSeriesComponent(null, e); 32037 closeArray(); 32038 }; 32039 } 32040 32041 protected void composeImagingManifestSeriesComponent(String name, ImagingManifest.SeriesComponent element) throws IOException { 32042 if (element != null) { 32043 open(name); 32044 composeImagingManifestSeriesComponentInner(element); 32045 close(); 32046 } 32047 } 32048 32049 protected void composeImagingManifestSeriesComponentInner(ImagingManifest.SeriesComponent element) throws IOException { 32050 composeBackbone(element); 32051 if (element.hasUidElement()) { 32052 composeOidCore("uid", element.getUidElement(), false); 32053 composeOidExtras("uid", element.getUidElement(), false); 32054 } 32055 if (element.hasEndpoint()) { 32056 openArray("endpoint"); 32057 for (Reference e : element.getEndpoint()) 32058 composeReference(null, e); 32059 closeArray(); 32060 }; 32061 if (element.hasInstance()) { 32062 openArray("instance"); 32063 for (ImagingManifest.InstanceComponent e : element.getInstance()) 32064 composeImagingManifestInstanceComponent(null, e); 32065 closeArray(); 32066 }; 32067 } 32068 32069 protected void composeImagingManifestInstanceComponent(String name, ImagingManifest.InstanceComponent element) throws IOException { 32070 if (element != null) { 32071 open(name); 32072 composeImagingManifestInstanceComponentInner(element); 32073 close(); 32074 } 32075 } 32076 32077 protected void composeImagingManifestInstanceComponentInner(ImagingManifest.InstanceComponent element) throws IOException { 32078 composeBackbone(element); 32079 if (element.hasSopClassElement()) { 32080 composeOidCore("sopClass", element.getSopClassElement(), false); 32081 composeOidExtras("sopClass", element.getSopClassElement(), false); 32082 } 32083 if (element.hasUidElement()) { 32084 composeOidCore("uid", element.getUidElement(), false); 32085 composeOidExtras("uid", element.getUidElement(), false); 32086 } 32087 } 32088 32089 protected void composeImagingStudy(String name, ImagingStudy element) throws IOException { 32090 if (element != null) { 32091 prop("resourceType", name); 32092 composeImagingStudyInner(element); 32093 } 32094 } 32095 32096 protected void composeImagingStudyInner(ImagingStudy element) throws IOException { 32097 composeDomainResourceElements(element); 32098 if (element.hasUidElement()) { 32099 composeOidCore("uid", element.getUidElement(), false); 32100 composeOidExtras("uid", element.getUidElement(), false); 32101 } 32102 if (element.hasAccession()) { 32103 composeIdentifier("accession", element.getAccession()); 32104 } 32105 if (element.hasIdentifier()) { 32106 openArray("identifier"); 32107 for (Identifier e : element.getIdentifier()) 32108 composeIdentifier(null, e); 32109 closeArray(); 32110 }; 32111 if (element.hasAvailabilityElement()) { 32112 composeEnumerationCore("availability", element.getAvailabilityElement(), new ImagingStudy.InstanceAvailabilityEnumFactory(), false); 32113 composeEnumerationExtras("availability", element.getAvailabilityElement(), new ImagingStudy.InstanceAvailabilityEnumFactory(), false); 32114 } 32115 if (element.hasModalityList()) { 32116 openArray("modalityList"); 32117 for (Coding e : element.getModalityList()) 32118 composeCoding(null, e); 32119 closeArray(); 32120 }; 32121 if (element.hasPatient()) { 32122 composeReference("patient", element.getPatient()); 32123 } 32124 if (element.hasContext()) { 32125 composeReference("context", element.getContext()); 32126 } 32127 if (element.hasStartedElement()) { 32128 composeDateTimeCore("started", element.getStartedElement(), false); 32129 composeDateTimeExtras("started", element.getStartedElement(), false); 32130 } 32131 if (element.hasBasedOn()) { 32132 openArray("basedOn"); 32133 for (Reference e : element.getBasedOn()) 32134 composeReference(null, e); 32135 closeArray(); 32136 }; 32137 if (element.hasReferrer()) { 32138 composeReference("referrer", element.getReferrer()); 32139 } 32140 if (element.hasInterpreter()) { 32141 openArray("interpreter"); 32142 for (Reference e : element.getInterpreter()) 32143 composeReference(null, e); 32144 closeArray(); 32145 }; 32146 if (element.hasEndpoint()) { 32147 openArray("endpoint"); 32148 for (Reference e : element.getEndpoint()) 32149 composeReference(null, e); 32150 closeArray(); 32151 }; 32152 if (element.hasNumberOfSeriesElement()) { 32153 composeUnsignedIntCore("numberOfSeries", element.getNumberOfSeriesElement(), false); 32154 composeUnsignedIntExtras("numberOfSeries", element.getNumberOfSeriesElement(), false); 32155 } 32156 if (element.hasNumberOfInstancesElement()) { 32157 composeUnsignedIntCore("numberOfInstances", element.getNumberOfInstancesElement(), false); 32158 composeUnsignedIntExtras("numberOfInstances", element.getNumberOfInstancesElement(), false); 32159 } 32160 if (element.hasProcedureReference()) { 32161 openArray("procedureReference"); 32162 for (Reference e : element.getProcedureReference()) 32163 composeReference(null, e); 32164 closeArray(); 32165 }; 32166 if (element.hasProcedureCode()) { 32167 openArray("procedureCode"); 32168 for (CodeableConcept e : element.getProcedureCode()) 32169 composeCodeableConcept(null, e); 32170 closeArray(); 32171 }; 32172 if (element.hasReason()) { 32173 composeCodeableConcept("reason", element.getReason()); 32174 } 32175 if (element.hasDescriptionElement()) { 32176 composeStringCore("description", element.getDescriptionElement(), false); 32177 composeStringExtras("description", element.getDescriptionElement(), false); 32178 } 32179 if (element.hasSeries()) { 32180 openArray("series"); 32181 for (ImagingStudy.ImagingStudySeriesComponent e : element.getSeries()) 32182 composeImagingStudyImagingStudySeriesComponent(null, e); 32183 closeArray(); 32184 }; 32185 } 32186 32187 protected void composeImagingStudyImagingStudySeriesComponent(String name, ImagingStudy.ImagingStudySeriesComponent element) throws IOException { 32188 if (element != null) { 32189 open(name); 32190 composeImagingStudyImagingStudySeriesComponentInner(element); 32191 close(); 32192 } 32193 } 32194 32195 protected void composeImagingStudyImagingStudySeriesComponentInner(ImagingStudy.ImagingStudySeriesComponent element) throws IOException { 32196 composeBackbone(element); 32197 if (element.hasUidElement()) { 32198 composeOidCore("uid", element.getUidElement(), false); 32199 composeOidExtras("uid", element.getUidElement(), false); 32200 } 32201 if (element.hasNumberElement()) { 32202 composeUnsignedIntCore("number", element.getNumberElement(), false); 32203 composeUnsignedIntExtras("number", element.getNumberElement(), false); 32204 } 32205 if (element.hasModality()) { 32206 composeCoding("modality", element.getModality()); 32207 } 32208 if (element.hasDescriptionElement()) { 32209 composeStringCore("description", element.getDescriptionElement(), false); 32210 composeStringExtras("description", element.getDescriptionElement(), false); 32211 } 32212 if (element.hasNumberOfInstancesElement()) { 32213 composeUnsignedIntCore("numberOfInstances", element.getNumberOfInstancesElement(), false); 32214 composeUnsignedIntExtras("numberOfInstances", element.getNumberOfInstancesElement(), false); 32215 } 32216 if (element.hasAvailabilityElement()) { 32217 composeEnumerationCore("availability", element.getAvailabilityElement(), new ImagingStudy.InstanceAvailabilityEnumFactory(), false); 32218 composeEnumerationExtras("availability", element.getAvailabilityElement(), new ImagingStudy.InstanceAvailabilityEnumFactory(), false); 32219 } 32220 if (element.hasEndpoint()) { 32221 openArray("endpoint"); 32222 for (Reference e : element.getEndpoint()) 32223 composeReference(null, e); 32224 closeArray(); 32225 }; 32226 if (element.hasBodySite()) { 32227 composeCoding("bodySite", element.getBodySite()); 32228 } 32229 if (element.hasLaterality()) { 32230 composeCoding("laterality", element.getLaterality()); 32231 } 32232 if (element.hasStartedElement()) { 32233 composeDateTimeCore("started", element.getStartedElement(), false); 32234 composeDateTimeExtras("started", element.getStartedElement(), false); 32235 } 32236 if (element.hasPerformer()) { 32237 openArray("performer"); 32238 for (Reference e : element.getPerformer()) 32239 composeReference(null, e); 32240 closeArray(); 32241 }; 32242 if (element.hasInstance()) { 32243 openArray("instance"); 32244 for (ImagingStudy.ImagingStudySeriesInstanceComponent e : element.getInstance()) 32245 composeImagingStudyImagingStudySeriesInstanceComponent(null, e); 32246 closeArray(); 32247 }; 32248 } 32249 32250 protected void composeImagingStudyImagingStudySeriesInstanceComponent(String name, ImagingStudy.ImagingStudySeriesInstanceComponent element) throws IOException { 32251 if (element != null) { 32252 open(name); 32253 composeImagingStudyImagingStudySeriesInstanceComponentInner(element); 32254 close(); 32255 } 32256 } 32257 32258 protected void composeImagingStudyImagingStudySeriesInstanceComponentInner(ImagingStudy.ImagingStudySeriesInstanceComponent element) throws IOException { 32259 composeBackbone(element); 32260 if (element.hasUidElement()) { 32261 composeOidCore("uid", element.getUidElement(), false); 32262 composeOidExtras("uid", element.getUidElement(), false); 32263 } 32264 if (element.hasNumberElement()) { 32265 composeUnsignedIntCore("number", element.getNumberElement(), false); 32266 composeUnsignedIntExtras("number", element.getNumberElement(), false); 32267 } 32268 if (element.hasSopClassElement()) { 32269 composeOidCore("sopClass", element.getSopClassElement(), false); 32270 composeOidExtras("sopClass", element.getSopClassElement(), false); 32271 } 32272 if (element.hasTitleElement()) { 32273 composeStringCore("title", element.getTitleElement(), false); 32274 composeStringExtras("title", element.getTitleElement(), false); 32275 } 32276 } 32277 32278 protected void composeImmunization(String name, Immunization element) throws IOException { 32279 if (element != null) { 32280 prop("resourceType", name); 32281 composeImmunizationInner(element); 32282 } 32283 } 32284 32285 protected void composeImmunizationInner(Immunization element) throws IOException { 32286 composeDomainResourceElements(element); 32287 if (element.hasIdentifier()) { 32288 openArray("identifier"); 32289 for (Identifier e : element.getIdentifier()) 32290 composeIdentifier(null, e); 32291 closeArray(); 32292 }; 32293 if (element.hasStatusElement()) { 32294 composeEnumerationCore("status", element.getStatusElement(), new Immunization.ImmunizationStatusEnumFactory(), false); 32295 composeEnumerationExtras("status", element.getStatusElement(), new Immunization.ImmunizationStatusEnumFactory(), false); 32296 } 32297 if (element.hasNotGivenElement()) { 32298 composeBooleanCore("notGiven", element.getNotGivenElement(), false); 32299 composeBooleanExtras("notGiven", element.getNotGivenElement(), false); 32300 } 32301 if (element.hasVaccineCode()) { 32302 composeCodeableConcept("vaccineCode", element.getVaccineCode()); 32303 } 32304 if (element.hasPatient()) { 32305 composeReference("patient", element.getPatient()); 32306 } 32307 if (element.hasEncounter()) { 32308 composeReference("encounter", element.getEncounter()); 32309 } 32310 if (element.hasDateElement()) { 32311 composeDateTimeCore("date", element.getDateElement(), false); 32312 composeDateTimeExtras("date", element.getDateElement(), false); 32313 } 32314 if (element.hasPrimarySourceElement()) { 32315 composeBooleanCore("primarySource", element.getPrimarySourceElement(), false); 32316 composeBooleanExtras("primarySource", element.getPrimarySourceElement(), false); 32317 } 32318 if (element.hasReportOrigin()) { 32319 composeCodeableConcept("reportOrigin", element.getReportOrigin()); 32320 } 32321 if (element.hasLocation()) { 32322 composeReference("location", element.getLocation()); 32323 } 32324 if (element.hasManufacturer()) { 32325 composeReference("manufacturer", element.getManufacturer()); 32326 } 32327 if (element.hasLotNumberElement()) { 32328 composeStringCore("lotNumber", element.getLotNumberElement(), false); 32329 composeStringExtras("lotNumber", element.getLotNumberElement(), false); 32330 } 32331 if (element.hasExpirationDateElement()) { 32332 composeDateCore("expirationDate", element.getExpirationDateElement(), false); 32333 composeDateExtras("expirationDate", element.getExpirationDateElement(), false); 32334 } 32335 if (element.hasSite()) { 32336 composeCodeableConcept("site", element.getSite()); 32337 } 32338 if (element.hasRoute()) { 32339 composeCodeableConcept("route", element.getRoute()); 32340 } 32341 if (element.hasDoseQuantity()) { 32342 composeSimpleQuantity("doseQuantity", element.getDoseQuantity()); 32343 } 32344 if (element.hasPractitioner()) { 32345 openArray("practitioner"); 32346 for (Immunization.ImmunizationPractitionerComponent e : element.getPractitioner()) 32347 composeImmunizationImmunizationPractitionerComponent(null, e); 32348 closeArray(); 32349 }; 32350 if (element.hasNote()) { 32351 openArray("note"); 32352 for (Annotation e : element.getNote()) 32353 composeAnnotation(null, e); 32354 closeArray(); 32355 }; 32356 if (element.hasExplanation()) { 32357 composeImmunizationImmunizationExplanationComponent("explanation", element.getExplanation()); 32358 } 32359 if (element.hasReaction()) { 32360 openArray("reaction"); 32361 for (Immunization.ImmunizationReactionComponent e : element.getReaction()) 32362 composeImmunizationImmunizationReactionComponent(null, e); 32363 closeArray(); 32364 }; 32365 if (element.hasVaccinationProtocol()) { 32366 openArray("vaccinationProtocol"); 32367 for (Immunization.ImmunizationVaccinationProtocolComponent e : element.getVaccinationProtocol()) 32368 composeImmunizationImmunizationVaccinationProtocolComponent(null, e); 32369 closeArray(); 32370 }; 32371 } 32372 32373 protected void composeImmunizationImmunizationPractitionerComponent(String name, Immunization.ImmunizationPractitionerComponent element) throws IOException { 32374 if (element != null) { 32375 open(name); 32376 composeImmunizationImmunizationPractitionerComponentInner(element); 32377 close(); 32378 } 32379 } 32380 32381 protected void composeImmunizationImmunizationPractitionerComponentInner(Immunization.ImmunizationPractitionerComponent element) throws IOException { 32382 composeBackbone(element); 32383 if (element.hasRole()) { 32384 composeCodeableConcept("role", element.getRole()); 32385 } 32386 if (element.hasActor()) { 32387 composeReference("actor", element.getActor()); 32388 } 32389 } 32390 32391 protected void composeImmunizationImmunizationExplanationComponent(String name, Immunization.ImmunizationExplanationComponent element) throws IOException { 32392 if (element != null) { 32393 open(name); 32394 composeImmunizationImmunizationExplanationComponentInner(element); 32395 close(); 32396 } 32397 } 32398 32399 protected void composeImmunizationImmunizationExplanationComponentInner(Immunization.ImmunizationExplanationComponent element) throws IOException { 32400 composeBackbone(element); 32401 if (element.hasReason()) { 32402 openArray("reason"); 32403 for (CodeableConcept e : element.getReason()) 32404 composeCodeableConcept(null, e); 32405 closeArray(); 32406 }; 32407 if (element.hasReasonNotGiven()) { 32408 openArray("reasonNotGiven"); 32409 for (CodeableConcept e : element.getReasonNotGiven()) 32410 composeCodeableConcept(null, e); 32411 closeArray(); 32412 }; 32413 } 32414 32415 protected void composeImmunizationImmunizationReactionComponent(String name, Immunization.ImmunizationReactionComponent element) throws IOException { 32416 if (element != null) { 32417 open(name); 32418 composeImmunizationImmunizationReactionComponentInner(element); 32419 close(); 32420 } 32421 } 32422 32423 protected void composeImmunizationImmunizationReactionComponentInner(Immunization.ImmunizationReactionComponent element) throws IOException { 32424 composeBackbone(element); 32425 if (element.hasDateElement()) { 32426 composeDateTimeCore("date", element.getDateElement(), false); 32427 composeDateTimeExtras("date", element.getDateElement(), false); 32428 } 32429 if (element.hasDetail()) { 32430 composeReference("detail", element.getDetail()); 32431 } 32432 if (element.hasReportedElement()) { 32433 composeBooleanCore("reported", element.getReportedElement(), false); 32434 composeBooleanExtras("reported", element.getReportedElement(), false); 32435 } 32436 } 32437 32438 protected void composeImmunizationImmunizationVaccinationProtocolComponent(String name, Immunization.ImmunizationVaccinationProtocolComponent element) throws IOException { 32439 if (element != null) { 32440 open(name); 32441 composeImmunizationImmunizationVaccinationProtocolComponentInner(element); 32442 close(); 32443 } 32444 } 32445 32446 protected void composeImmunizationImmunizationVaccinationProtocolComponentInner(Immunization.ImmunizationVaccinationProtocolComponent element) throws IOException { 32447 composeBackbone(element); 32448 if (element.hasDoseSequenceElement()) { 32449 composePositiveIntCore("doseSequence", element.getDoseSequenceElement(), false); 32450 composePositiveIntExtras("doseSequence", element.getDoseSequenceElement(), false); 32451 } 32452 if (element.hasDescriptionElement()) { 32453 composeStringCore("description", element.getDescriptionElement(), false); 32454 composeStringExtras("description", element.getDescriptionElement(), false); 32455 } 32456 if (element.hasAuthority()) { 32457 composeReference("authority", element.getAuthority()); 32458 } 32459 if (element.hasSeriesElement()) { 32460 composeStringCore("series", element.getSeriesElement(), false); 32461 composeStringExtras("series", element.getSeriesElement(), false); 32462 } 32463 if (element.hasSeriesDosesElement()) { 32464 composePositiveIntCore("seriesDoses", element.getSeriesDosesElement(), false); 32465 composePositiveIntExtras("seriesDoses", element.getSeriesDosesElement(), false); 32466 } 32467 if (element.hasTargetDisease()) { 32468 openArray("targetDisease"); 32469 for (CodeableConcept e : element.getTargetDisease()) 32470 composeCodeableConcept(null, e); 32471 closeArray(); 32472 }; 32473 if (element.hasDoseStatus()) { 32474 composeCodeableConcept("doseStatus", element.getDoseStatus()); 32475 } 32476 if (element.hasDoseStatusReason()) { 32477 composeCodeableConcept("doseStatusReason", element.getDoseStatusReason()); 32478 } 32479 } 32480 32481 protected void composeImmunizationRecommendation(String name, ImmunizationRecommendation element) throws IOException { 32482 if (element != null) { 32483 prop("resourceType", name); 32484 composeImmunizationRecommendationInner(element); 32485 } 32486 } 32487 32488 protected void composeImmunizationRecommendationInner(ImmunizationRecommendation element) throws IOException { 32489 composeDomainResourceElements(element); 32490 if (element.hasIdentifier()) { 32491 openArray("identifier"); 32492 for (Identifier e : element.getIdentifier()) 32493 composeIdentifier(null, e); 32494 closeArray(); 32495 }; 32496 if (element.hasPatient()) { 32497 composeReference("patient", element.getPatient()); 32498 } 32499 if (element.hasRecommendation()) { 32500 openArray("recommendation"); 32501 for (ImmunizationRecommendation.ImmunizationRecommendationRecommendationComponent e : element.getRecommendation()) 32502 composeImmunizationRecommendationImmunizationRecommendationRecommendationComponent(null, e); 32503 closeArray(); 32504 }; 32505 } 32506 32507 protected void composeImmunizationRecommendationImmunizationRecommendationRecommendationComponent(String name, ImmunizationRecommendation.ImmunizationRecommendationRecommendationComponent element) throws IOException { 32508 if (element != null) { 32509 open(name); 32510 composeImmunizationRecommendationImmunizationRecommendationRecommendationComponentInner(element); 32511 close(); 32512 } 32513 } 32514 32515 protected void composeImmunizationRecommendationImmunizationRecommendationRecommendationComponentInner(ImmunizationRecommendation.ImmunizationRecommendationRecommendationComponent element) throws IOException { 32516 composeBackbone(element); 32517 if (element.hasDateElement()) { 32518 composeDateTimeCore("date", element.getDateElement(), false); 32519 composeDateTimeExtras("date", element.getDateElement(), false); 32520 } 32521 if (element.hasVaccineCode()) { 32522 composeCodeableConcept("vaccineCode", element.getVaccineCode()); 32523 } 32524 if (element.hasTargetDisease()) { 32525 composeCodeableConcept("targetDisease", element.getTargetDisease()); 32526 } 32527 if (element.hasDoseNumberElement()) { 32528 composePositiveIntCore("doseNumber", element.getDoseNumberElement(), false); 32529 composePositiveIntExtras("doseNumber", element.getDoseNumberElement(), false); 32530 } 32531 if (element.hasForecastStatus()) { 32532 composeCodeableConcept("forecastStatus", element.getForecastStatus()); 32533 } 32534 if (element.hasDateCriterion()) { 32535 openArray("dateCriterion"); 32536 for (ImmunizationRecommendation.ImmunizationRecommendationRecommendationDateCriterionComponent e : element.getDateCriterion()) 32537 composeImmunizationRecommendationImmunizationRecommendationRecommendationDateCriterionComponent(null, e); 32538 closeArray(); 32539 }; 32540 if (element.hasProtocol()) { 32541 composeImmunizationRecommendationImmunizationRecommendationRecommendationProtocolComponent("protocol", element.getProtocol()); 32542 } 32543 if (element.hasSupportingImmunization()) { 32544 openArray("supportingImmunization"); 32545 for (Reference e : element.getSupportingImmunization()) 32546 composeReference(null, e); 32547 closeArray(); 32548 }; 32549 if (element.hasSupportingPatientInformation()) { 32550 openArray("supportingPatientInformation"); 32551 for (Reference e : element.getSupportingPatientInformation()) 32552 composeReference(null, e); 32553 closeArray(); 32554 }; 32555 } 32556 32557 protected void composeImmunizationRecommendationImmunizationRecommendationRecommendationDateCriterionComponent(String name, ImmunizationRecommendation.ImmunizationRecommendationRecommendationDateCriterionComponent element) throws IOException { 32558 if (element != null) { 32559 open(name); 32560 composeImmunizationRecommendationImmunizationRecommendationRecommendationDateCriterionComponentInner(element); 32561 close(); 32562 } 32563 } 32564 32565 protected void composeImmunizationRecommendationImmunizationRecommendationRecommendationDateCriterionComponentInner(ImmunizationRecommendation.ImmunizationRecommendationRecommendationDateCriterionComponent element) throws IOException { 32566 composeBackbone(element); 32567 if (element.hasCode()) { 32568 composeCodeableConcept("code", element.getCode()); 32569 } 32570 if (element.hasValueElement()) { 32571 composeDateTimeCore("value", element.getValueElement(), false); 32572 composeDateTimeExtras("value", element.getValueElement(), false); 32573 } 32574 } 32575 32576 protected void composeImmunizationRecommendationImmunizationRecommendationRecommendationProtocolComponent(String name, ImmunizationRecommendation.ImmunizationRecommendationRecommendationProtocolComponent element) throws IOException { 32577 if (element != null) { 32578 open(name); 32579 composeImmunizationRecommendationImmunizationRecommendationRecommendationProtocolComponentInner(element); 32580 close(); 32581 } 32582 } 32583 32584 protected void composeImmunizationRecommendationImmunizationRecommendationRecommendationProtocolComponentInner(ImmunizationRecommendation.ImmunizationRecommendationRecommendationProtocolComponent element) throws IOException { 32585 composeBackbone(element); 32586 if (element.hasDoseSequenceElement()) { 32587 composePositiveIntCore("doseSequence", element.getDoseSequenceElement(), false); 32588 composePositiveIntExtras("doseSequence", element.getDoseSequenceElement(), false); 32589 } 32590 if (element.hasDescriptionElement()) { 32591 composeStringCore("description", element.getDescriptionElement(), false); 32592 composeStringExtras("description", element.getDescriptionElement(), false); 32593 } 32594 if (element.hasAuthority()) { 32595 composeReference("authority", element.getAuthority()); 32596 } 32597 if (element.hasSeriesElement()) { 32598 composeStringCore("series", element.getSeriesElement(), false); 32599 composeStringExtras("series", element.getSeriesElement(), false); 32600 } 32601 } 32602 32603 protected void composeImplementationGuide(String name, ImplementationGuide element) throws IOException { 32604 if (element != null) { 32605 prop("resourceType", name); 32606 composeImplementationGuideInner(element); 32607 } 32608 } 32609 32610 protected void composeImplementationGuideInner(ImplementationGuide element) throws IOException { 32611 composeDomainResourceElements(element); 32612 if (element.hasUrlElement()) { 32613 composeUriCore("url", element.getUrlElement(), false); 32614 composeUriExtras("url", element.getUrlElement(), false); 32615 } 32616 if (element.hasVersionElement()) { 32617 composeStringCore("version", element.getVersionElement(), false); 32618 composeStringExtras("version", element.getVersionElement(), false); 32619 } 32620 if (element.hasNameElement()) { 32621 composeStringCore("name", element.getNameElement(), false); 32622 composeStringExtras("name", element.getNameElement(), false); 32623 } 32624 if (element.hasStatusElement()) { 32625 composeEnumerationCore("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 32626 composeEnumerationExtras("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 32627 } 32628 if (element.hasExperimentalElement()) { 32629 composeBooleanCore("experimental", element.getExperimentalElement(), false); 32630 composeBooleanExtras("experimental", element.getExperimentalElement(), false); 32631 } 32632 if (element.hasDateElement()) { 32633 composeDateTimeCore("date", element.getDateElement(), false); 32634 composeDateTimeExtras("date", element.getDateElement(), false); 32635 } 32636 if (element.hasPublisherElement()) { 32637 composeStringCore("publisher", element.getPublisherElement(), false); 32638 composeStringExtras("publisher", element.getPublisherElement(), false); 32639 } 32640 if (element.hasContact()) { 32641 openArray("contact"); 32642 for (ContactDetail e : element.getContact()) 32643 composeContactDetail(null, e); 32644 closeArray(); 32645 }; 32646 if (element.hasDescriptionElement()) { 32647 composeMarkdownCore("description", element.getDescriptionElement(), false); 32648 composeMarkdownExtras("description", element.getDescriptionElement(), false); 32649 } 32650 if (element.hasUseContext()) { 32651 openArray("useContext"); 32652 for (UsageContext e : element.getUseContext()) 32653 composeUsageContext(null, e); 32654 closeArray(); 32655 }; 32656 if (element.hasJurisdiction()) { 32657 openArray("jurisdiction"); 32658 for (CodeableConcept e : element.getJurisdiction()) 32659 composeCodeableConcept(null, e); 32660 closeArray(); 32661 }; 32662 if (element.hasCopyrightElement()) { 32663 composeMarkdownCore("copyright", element.getCopyrightElement(), false); 32664 composeMarkdownExtras("copyright", element.getCopyrightElement(), false); 32665 } 32666 if (element.hasFhirVersionElement()) { 32667 composeIdCore("fhirVersion", element.getFhirVersionElement(), false); 32668 composeIdExtras("fhirVersion", element.getFhirVersionElement(), false); 32669 } 32670 if (element.hasDependency()) { 32671 openArray("dependency"); 32672 for (ImplementationGuide.ImplementationGuideDependencyComponent e : element.getDependency()) 32673 composeImplementationGuideImplementationGuideDependencyComponent(null, e); 32674 closeArray(); 32675 }; 32676 if (element.hasPackage()) { 32677 openArray("package"); 32678 for (ImplementationGuide.ImplementationGuidePackageComponent e : element.getPackage()) 32679 composeImplementationGuideImplementationGuidePackageComponent(null, e); 32680 closeArray(); 32681 }; 32682 if (element.hasGlobal()) { 32683 openArray("global"); 32684 for (ImplementationGuide.ImplementationGuideGlobalComponent e : element.getGlobal()) 32685 composeImplementationGuideImplementationGuideGlobalComponent(null, e); 32686 closeArray(); 32687 }; 32688 if (element.hasBinary()) { 32689 openArray("binary"); 32690 for (UriType e : element.getBinary()) 32691 composeUriCore(null, e, true); 32692 closeArray(); 32693 if (anyHasExtras(element.getBinary())) { 32694 openArray("_binary"); 32695 for (UriType e : element.getBinary()) 32696 composeUriExtras(null, e, true); 32697 closeArray(); 32698 } 32699 }; 32700 if (element.hasPage()) { 32701 composeImplementationGuideImplementationGuidePageComponent("page", element.getPage()); 32702 } 32703 } 32704 32705 protected void composeImplementationGuideImplementationGuideDependencyComponent(String name, ImplementationGuide.ImplementationGuideDependencyComponent element) throws IOException { 32706 if (element != null) { 32707 open(name); 32708 composeImplementationGuideImplementationGuideDependencyComponentInner(element); 32709 close(); 32710 } 32711 } 32712 32713 protected void composeImplementationGuideImplementationGuideDependencyComponentInner(ImplementationGuide.ImplementationGuideDependencyComponent element) throws IOException { 32714 composeBackbone(element); 32715 if (element.hasTypeElement()) { 32716 composeEnumerationCore("type", element.getTypeElement(), new ImplementationGuide.GuideDependencyTypeEnumFactory(), false); 32717 composeEnumerationExtras("type", element.getTypeElement(), new ImplementationGuide.GuideDependencyTypeEnumFactory(), false); 32718 } 32719 if (element.hasUriElement()) { 32720 composeUriCore("uri", element.getUriElement(), false); 32721 composeUriExtras("uri", element.getUriElement(), false); 32722 } 32723 } 32724 32725 protected void composeImplementationGuideImplementationGuidePackageComponent(String name, ImplementationGuide.ImplementationGuidePackageComponent element) throws IOException { 32726 if (element != null) { 32727 open(name); 32728 composeImplementationGuideImplementationGuidePackageComponentInner(element); 32729 close(); 32730 } 32731 } 32732 32733 protected void composeImplementationGuideImplementationGuidePackageComponentInner(ImplementationGuide.ImplementationGuidePackageComponent element) throws IOException { 32734 composeBackbone(element); 32735 if (element.hasNameElement()) { 32736 composeStringCore("name", element.getNameElement(), false); 32737 composeStringExtras("name", element.getNameElement(), false); 32738 } 32739 if (element.hasDescriptionElement()) { 32740 composeStringCore("description", element.getDescriptionElement(), false); 32741 composeStringExtras("description", element.getDescriptionElement(), false); 32742 } 32743 if (element.hasResource()) { 32744 openArray("resource"); 32745 for (ImplementationGuide.ImplementationGuidePackageResourceComponent e : element.getResource()) 32746 composeImplementationGuideImplementationGuidePackageResourceComponent(null, e); 32747 closeArray(); 32748 }; 32749 } 32750 32751 protected void composeImplementationGuideImplementationGuidePackageResourceComponent(String name, ImplementationGuide.ImplementationGuidePackageResourceComponent element) throws IOException { 32752 if (element != null) { 32753 open(name); 32754 composeImplementationGuideImplementationGuidePackageResourceComponentInner(element); 32755 close(); 32756 } 32757 } 32758 32759 protected void composeImplementationGuideImplementationGuidePackageResourceComponentInner(ImplementationGuide.ImplementationGuidePackageResourceComponent element) throws IOException { 32760 composeBackbone(element); 32761 if (element.hasExampleElement()) { 32762 composeBooleanCore("example", element.getExampleElement(), false); 32763 composeBooleanExtras("example", element.getExampleElement(), false); 32764 } 32765 if (element.hasNameElement()) { 32766 composeStringCore("name", element.getNameElement(), false); 32767 composeStringExtras("name", element.getNameElement(), false); 32768 } 32769 if (element.hasDescriptionElement()) { 32770 composeStringCore("description", element.getDescriptionElement(), false); 32771 composeStringExtras("description", element.getDescriptionElement(), false); 32772 } 32773 if (element.hasAcronymElement()) { 32774 composeStringCore("acronym", element.getAcronymElement(), false); 32775 composeStringExtras("acronym", element.getAcronymElement(), false); 32776 } 32777 if (element.hasSource()) { 32778 composeType("source", element.getSource()); 32779 } 32780 if (element.hasExampleFor()) { 32781 composeReference("exampleFor", element.getExampleFor()); 32782 } 32783 } 32784 32785 protected void composeImplementationGuideImplementationGuideGlobalComponent(String name, ImplementationGuide.ImplementationGuideGlobalComponent element) throws IOException { 32786 if (element != null) { 32787 open(name); 32788 composeImplementationGuideImplementationGuideGlobalComponentInner(element); 32789 close(); 32790 } 32791 } 32792 32793 protected void composeImplementationGuideImplementationGuideGlobalComponentInner(ImplementationGuide.ImplementationGuideGlobalComponent element) throws IOException { 32794 composeBackbone(element); 32795 if (element.hasTypeElement()) { 32796 composeCodeCore("type", element.getTypeElement(), false); 32797 composeCodeExtras("type", element.getTypeElement(), false); 32798 } 32799 if (element.hasProfile()) { 32800 composeReference("profile", element.getProfile()); 32801 } 32802 } 32803 32804 protected void composeImplementationGuideImplementationGuidePageComponent(String name, ImplementationGuide.ImplementationGuidePageComponent element) throws IOException { 32805 if (element != null) { 32806 open(name); 32807 composeImplementationGuideImplementationGuidePageComponentInner(element); 32808 close(); 32809 } 32810 } 32811 32812 protected void composeImplementationGuideImplementationGuidePageComponentInner(ImplementationGuide.ImplementationGuidePageComponent element) throws IOException { 32813 composeBackbone(element); 32814 if (element.hasSourceElement()) { 32815 composeUriCore("source", element.getSourceElement(), false); 32816 composeUriExtras("source", element.getSourceElement(), false); 32817 } 32818 if (element.hasTitleElement()) { 32819 composeStringCore("title", element.getTitleElement(), false); 32820 composeStringExtras("title", element.getTitleElement(), false); 32821 } 32822 if (element.hasKindElement()) { 32823 composeEnumerationCore("kind", element.getKindElement(), new ImplementationGuide.GuidePageKindEnumFactory(), false); 32824 composeEnumerationExtras("kind", element.getKindElement(), new ImplementationGuide.GuidePageKindEnumFactory(), false); 32825 } 32826 if (element.hasType()) { 32827 openArray("type"); 32828 for (CodeType e : element.getType()) 32829 composeCodeCore(null, e, true); 32830 closeArray(); 32831 if (anyHasExtras(element.getType())) { 32832 openArray("_type"); 32833 for (CodeType e : element.getType()) 32834 composeCodeExtras(null, e, true); 32835 closeArray(); 32836 } 32837 }; 32838 if (element.hasPackage()) { 32839 openArray("package"); 32840 for (StringType e : element.getPackage()) 32841 composeStringCore(null, e, true); 32842 closeArray(); 32843 if (anyHasExtras(element.getPackage())) { 32844 openArray("_package"); 32845 for (StringType e : element.getPackage()) 32846 composeStringExtras(null, e, true); 32847 closeArray(); 32848 } 32849 }; 32850 if (element.hasFormatElement()) { 32851 composeCodeCore("format", element.getFormatElement(), false); 32852 composeCodeExtras("format", element.getFormatElement(), false); 32853 } 32854 if (element.hasPage()) { 32855 openArray("page"); 32856 for (ImplementationGuide.ImplementationGuidePageComponent e : element.getPage()) 32857 composeImplementationGuideImplementationGuidePageComponent(null, e); 32858 closeArray(); 32859 }; 32860 } 32861 32862 protected void composeLibrary(String name, Library element) throws IOException { 32863 if (element != null) { 32864 prop("resourceType", name); 32865 composeLibraryInner(element); 32866 } 32867 } 32868 32869 protected void composeLibraryInner(Library element) throws IOException { 32870 composeDomainResourceElements(element); 32871 if (element.hasUrlElement()) { 32872 composeUriCore("url", element.getUrlElement(), false); 32873 composeUriExtras("url", element.getUrlElement(), false); 32874 } 32875 if (element.hasIdentifier()) { 32876 openArray("identifier"); 32877 for (Identifier e : element.getIdentifier()) 32878 composeIdentifier(null, e); 32879 closeArray(); 32880 }; 32881 if (element.hasVersionElement()) { 32882 composeStringCore("version", element.getVersionElement(), false); 32883 composeStringExtras("version", element.getVersionElement(), false); 32884 } 32885 if (element.hasNameElement()) { 32886 composeStringCore("name", element.getNameElement(), false); 32887 composeStringExtras("name", element.getNameElement(), false); 32888 } 32889 if (element.hasTitleElement()) { 32890 composeStringCore("title", element.getTitleElement(), false); 32891 composeStringExtras("title", element.getTitleElement(), false); 32892 } 32893 if (element.hasStatusElement()) { 32894 composeEnumerationCore("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 32895 composeEnumerationExtras("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 32896 } 32897 if (element.hasExperimentalElement()) { 32898 composeBooleanCore("experimental", element.getExperimentalElement(), false); 32899 composeBooleanExtras("experimental", element.getExperimentalElement(), false); 32900 } 32901 if (element.hasType()) { 32902 composeCodeableConcept("type", element.getType()); 32903 } 32904 if (element.hasDateElement()) { 32905 composeDateTimeCore("date", element.getDateElement(), false); 32906 composeDateTimeExtras("date", element.getDateElement(), false); 32907 } 32908 if (element.hasPublisherElement()) { 32909 composeStringCore("publisher", element.getPublisherElement(), false); 32910 composeStringExtras("publisher", element.getPublisherElement(), false); 32911 } 32912 if (element.hasDescriptionElement()) { 32913 composeMarkdownCore("description", element.getDescriptionElement(), false); 32914 composeMarkdownExtras("description", element.getDescriptionElement(), false); 32915 } 32916 if (element.hasPurposeElement()) { 32917 composeMarkdownCore("purpose", element.getPurposeElement(), false); 32918 composeMarkdownExtras("purpose", element.getPurposeElement(), false); 32919 } 32920 if (element.hasUsageElement()) { 32921 composeStringCore("usage", element.getUsageElement(), false); 32922 composeStringExtras("usage", element.getUsageElement(), false); 32923 } 32924 if (element.hasApprovalDateElement()) { 32925 composeDateCore("approvalDate", element.getApprovalDateElement(), false); 32926 composeDateExtras("approvalDate", element.getApprovalDateElement(), false); 32927 } 32928 if (element.hasLastReviewDateElement()) { 32929 composeDateCore("lastReviewDate", element.getLastReviewDateElement(), false); 32930 composeDateExtras("lastReviewDate", element.getLastReviewDateElement(), false); 32931 } 32932 if (element.hasEffectivePeriod()) { 32933 composePeriod("effectivePeriod", element.getEffectivePeriod()); 32934 } 32935 if (element.hasUseContext()) { 32936 openArray("useContext"); 32937 for (UsageContext e : element.getUseContext()) 32938 composeUsageContext(null, e); 32939 closeArray(); 32940 }; 32941 if (element.hasJurisdiction()) { 32942 openArray("jurisdiction"); 32943 for (CodeableConcept e : element.getJurisdiction()) 32944 composeCodeableConcept(null, e); 32945 closeArray(); 32946 }; 32947 if (element.hasTopic()) { 32948 openArray("topic"); 32949 for (CodeableConcept e : element.getTopic()) 32950 composeCodeableConcept(null, e); 32951 closeArray(); 32952 }; 32953 if (element.hasContributor()) { 32954 openArray("contributor"); 32955 for (Contributor e : element.getContributor()) 32956 composeContributor(null, e); 32957 closeArray(); 32958 }; 32959 if (element.hasContact()) { 32960 openArray("contact"); 32961 for (ContactDetail e : element.getContact()) 32962 composeContactDetail(null, e); 32963 closeArray(); 32964 }; 32965 if (element.hasCopyrightElement()) { 32966 composeMarkdownCore("copyright", element.getCopyrightElement(), false); 32967 composeMarkdownExtras("copyright", element.getCopyrightElement(), false); 32968 } 32969 if (element.hasRelatedArtifact()) { 32970 openArray("relatedArtifact"); 32971 for (RelatedArtifact e : element.getRelatedArtifact()) 32972 composeRelatedArtifact(null, e); 32973 closeArray(); 32974 }; 32975 if (element.hasParameter()) { 32976 openArray("parameter"); 32977 for (ParameterDefinition e : element.getParameter()) 32978 composeParameterDefinition(null, e); 32979 closeArray(); 32980 }; 32981 if (element.hasDataRequirement()) { 32982 openArray("dataRequirement"); 32983 for (DataRequirement e : element.getDataRequirement()) 32984 composeDataRequirement(null, e); 32985 closeArray(); 32986 }; 32987 if (element.hasContent()) { 32988 openArray("content"); 32989 for (Attachment e : element.getContent()) 32990 composeAttachment(null, e); 32991 closeArray(); 32992 }; 32993 } 32994 32995 protected void composeLinkage(String name, Linkage element) throws IOException { 32996 if (element != null) { 32997 prop("resourceType", name); 32998 composeLinkageInner(element); 32999 } 33000 } 33001 33002 protected void composeLinkageInner(Linkage element) throws IOException { 33003 composeDomainResourceElements(element); 33004 if (element.hasActiveElement()) { 33005 composeBooleanCore("active", element.getActiveElement(), false); 33006 composeBooleanExtras("active", element.getActiveElement(), false); 33007 } 33008 if (element.hasAuthor()) { 33009 composeReference("author", element.getAuthor()); 33010 } 33011 if (element.hasItem()) { 33012 openArray("item"); 33013 for (Linkage.LinkageItemComponent e : element.getItem()) 33014 composeLinkageLinkageItemComponent(null, e); 33015 closeArray(); 33016 }; 33017 } 33018 33019 protected void composeLinkageLinkageItemComponent(String name, Linkage.LinkageItemComponent element) throws IOException { 33020 if (element != null) { 33021 open(name); 33022 composeLinkageLinkageItemComponentInner(element); 33023 close(); 33024 } 33025 } 33026 33027 protected void composeLinkageLinkageItemComponentInner(Linkage.LinkageItemComponent element) throws IOException { 33028 composeBackbone(element); 33029 if (element.hasTypeElement()) { 33030 composeEnumerationCore("type", element.getTypeElement(), new Linkage.LinkageTypeEnumFactory(), false); 33031 composeEnumerationExtras("type", element.getTypeElement(), new Linkage.LinkageTypeEnumFactory(), false); 33032 } 33033 if (element.hasResource()) { 33034 composeReference("resource", element.getResource()); 33035 } 33036 } 33037 33038 protected void composeListResource(String name, ListResource element) throws IOException { 33039 if (element != null) { 33040 prop("resourceType", name); 33041 composeListResourceInner(element); 33042 } 33043 } 33044 33045 protected void composeListResourceInner(ListResource element) throws IOException { 33046 composeDomainResourceElements(element); 33047 if (element.hasIdentifier()) { 33048 openArray("identifier"); 33049 for (Identifier e : element.getIdentifier()) 33050 composeIdentifier(null, e); 33051 closeArray(); 33052 }; 33053 if (element.hasStatusElement()) { 33054 composeEnumerationCore("status", element.getStatusElement(), new ListResource.ListStatusEnumFactory(), false); 33055 composeEnumerationExtras("status", element.getStatusElement(), new ListResource.ListStatusEnumFactory(), false); 33056 } 33057 if (element.hasModeElement()) { 33058 composeEnumerationCore("mode", element.getModeElement(), new ListResource.ListModeEnumFactory(), false); 33059 composeEnumerationExtras("mode", element.getModeElement(), new ListResource.ListModeEnumFactory(), false); 33060 } 33061 if (element.hasTitleElement()) { 33062 composeStringCore("title", element.getTitleElement(), false); 33063 composeStringExtras("title", element.getTitleElement(), false); 33064 } 33065 if (element.hasCode()) { 33066 composeCodeableConcept("code", element.getCode()); 33067 } 33068 if (element.hasSubject()) { 33069 composeReference("subject", element.getSubject()); 33070 } 33071 if (element.hasEncounter()) { 33072 composeReference("encounter", element.getEncounter()); 33073 } 33074 if (element.hasDateElement()) { 33075 composeDateTimeCore("date", element.getDateElement(), false); 33076 composeDateTimeExtras("date", element.getDateElement(), false); 33077 } 33078 if (element.hasSource()) { 33079 composeReference("source", element.getSource()); 33080 } 33081 if (element.hasOrderedBy()) { 33082 composeCodeableConcept("orderedBy", element.getOrderedBy()); 33083 } 33084 if (element.hasNote()) { 33085 openArray("note"); 33086 for (Annotation e : element.getNote()) 33087 composeAnnotation(null, e); 33088 closeArray(); 33089 }; 33090 if (element.hasEntry()) { 33091 openArray("entry"); 33092 for (ListResource.ListEntryComponent e : element.getEntry()) 33093 composeListResourceListEntryComponent(null, e); 33094 closeArray(); 33095 }; 33096 if (element.hasEmptyReason()) { 33097 composeCodeableConcept("emptyReason", element.getEmptyReason()); 33098 } 33099 } 33100 33101 protected void composeListResourceListEntryComponent(String name, ListResource.ListEntryComponent element) throws IOException { 33102 if (element != null) { 33103 open(name); 33104 composeListResourceListEntryComponentInner(element); 33105 close(); 33106 } 33107 } 33108 33109 protected void composeListResourceListEntryComponentInner(ListResource.ListEntryComponent element) throws IOException { 33110 composeBackbone(element); 33111 if (element.hasFlag()) { 33112 composeCodeableConcept("flag", element.getFlag()); 33113 } 33114 if (element.hasDeletedElement()) { 33115 composeBooleanCore("deleted", element.getDeletedElement(), false); 33116 composeBooleanExtras("deleted", element.getDeletedElement(), false); 33117 } 33118 if (element.hasDateElement()) { 33119 composeDateTimeCore("date", element.getDateElement(), false); 33120 composeDateTimeExtras("date", element.getDateElement(), false); 33121 } 33122 if (element.hasItem()) { 33123 composeReference("item", element.getItem()); 33124 } 33125 } 33126 33127 protected void composeLocation(String name, Location element) throws IOException { 33128 if (element != null) { 33129 prop("resourceType", name); 33130 composeLocationInner(element); 33131 } 33132 } 33133 33134 protected void composeLocationInner(Location element) throws IOException { 33135 composeDomainResourceElements(element); 33136 if (element.hasIdentifier()) { 33137 openArray("identifier"); 33138 for (Identifier e : element.getIdentifier()) 33139 composeIdentifier(null, e); 33140 closeArray(); 33141 }; 33142 if (element.hasStatusElement()) { 33143 composeEnumerationCore("status", element.getStatusElement(), new Location.LocationStatusEnumFactory(), false); 33144 composeEnumerationExtras("status", element.getStatusElement(), new Location.LocationStatusEnumFactory(), false); 33145 } 33146 if (element.hasOperationalStatus()) { 33147 composeCoding("operationalStatus", element.getOperationalStatus()); 33148 } 33149 if (element.hasNameElement()) { 33150 composeStringCore("name", element.getNameElement(), false); 33151 composeStringExtras("name", element.getNameElement(), false); 33152 } 33153 if (element.hasAlias()) { 33154 openArray("alias"); 33155 for (StringType e : element.getAlias()) 33156 composeStringCore(null, e, true); 33157 closeArray(); 33158 if (anyHasExtras(element.getAlias())) { 33159 openArray("_alias"); 33160 for (StringType e : element.getAlias()) 33161 composeStringExtras(null, e, true); 33162 closeArray(); 33163 } 33164 }; 33165 if (element.hasDescriptionElement()) { 33166 composeStringCore("description", element.getDescriptionElement(), false); 33167 composeStringExtras("description", element.getDescriptionElement(), false); 33168 } 33169 if (element.hasModeElement()) { 33170 composeEnumerationCore("mode", element.getModeElement(), new Location.LocationModeEnumFactory(), false); 33171 composeEnumerationExtras("mode", element.getModeElement(), new Location.LocationModeEnumFactory(), false); 33172 } 33173 if (element.hasType()) { 33174 composeCodeableConcept("type", element.getType()); 33175 } 33176 if (element.hasTelecom()) { 33177 openArray("telecom"); 33178 for (ContactPoint e : element.getTelecom()) 33179 composeContactPoint(null, e); 33180 closeArray(); 33181 }; 33182 if (element.hasAddress()) { 33183 composeAddress("address", element.getAddress()); 33184 } 33185 if (element.hasPhysicalType()) { 33186 composeCodeableConcept("physicalType", element.getPhysicalType()); 33187 } 33188 if (element.hasPosition()) { 33189 composeLocationLocationPositionComponent("position", element.getPosition()); 33190 } 33191 if (element.hasManagingOrganization()) { 33192 composeReference("managingOrganization", element.getManagingOrganization()); 33193 } 33194 if (element.hasPartOf()) { 33195 composeReference("partOf", element.getPartOf()); 33196 } 33197 if (element.hasEndpoint()) { 33198 openArray("endpoint"); 33199 for (Reference e : element.getEndpoint()) 33200 composeReference(null, e); 33201 closeArray(); 33202 }; 33203 } 33204 33205 protected void composeLocationLocationPositionComponent(String name, Location.LocationPositionComponent element) throws IOException { 33206 if (element != null) { 33207 open(name); 33208 composeLocationLocationPositionComponentInner(element); 33209 close(); 33210 } 33211 } 33212 33213 protected void composeLocationLocationPositionComponentInner(Location.LocationPositionComponent element) throws IOException { 33214 composeBackbone(element); 33215 if (element.hasLongitudeElement()) { 33216 composeDecimalCore("longitude", element.getLongitudeElement(), false); 33217 composeDecimalExtras("longitude", element.getLongitudeElement(), false); 33218 } 33219 if (element.hasLatitudeElement()) { 33220 composeDecimalCore("latitude", element.getLatitudeElement(), false); 33221 composeDecimalExtras("latitude", element.getLatitudeElement(), false); 33222 } 33223 if (element.hasAltitudeElement()) { 33224 composeDecimalCore("altitude", element.getAltitudeElement(), false); 33225 composeDecimalExtras("altitude", element.getAltitudeElement(), false); 33226 } 33227 } 33228 33229 protected void composeMeasure(String name, Measure element) throws IOException { 33230 if (element != null) { 33231 prop("resourceType", name); 33232 composeMeasureInner(element); 33233 } 33234 } 33235 33236 protected void composeMeasureInner(Measure element) throws IOException { 33237 composeDomainResourceElements(element); 33238 if (element.hasUrlElement()) { 33239 composeUriCore("url", element.getUrlElement(), false); 33240 composeUriExtras("url", element.getUrlElement(), false); 33241 } 33242 if (element.hasIdentifier()) { 33243 openArray("identifier"); 33244 for (Identifier e : element.getIdentifier()) 33245 composeIdentifier(null, e); 33246 closeArray(); 33247 }; 33248 if (element.hasVersionElement()) { 33249 composeStringCore("version", element.getVersionElement(), false); 33250 composeStringExtras("version", element.getVersionElement(), false); 33251 } 33252 if (element.hasNameElement()) { 33253 composeStringCore("name", element.getNameElement(), false); 33254 composeStringExtras("name", element.getNameElement(), false); 33255 } 33256 if (element.hasTitleElement()) { 33257 composeStringCore("title", element.getTitleElement(), false); 33258 composeStringExtras("title", element.getTitleElement(), false); 33259 } 33260 if (element.hasStatusElement()) { 33261 composeEnumerationCore("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 33262 composeEnumerationExtras("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 33263 } 33264 if (element.hasExperimentalElement()) { 33265 composeBooleanCore("experimental", element.getExperimentalElement(), false); 33266 composeBooleanExtras("experimental", element.getExperimentalElement(), false); 33267 } 33268 if (element.hasDateElement()) { 33269 composeDateTimeCore("date", element.getDateElement(), false); 33270 composeDateTimeExtras("date", element.getDateElement(), false); 33271 } 33272 if (element.hasPublisherElement()) { 33273 composeStringCore("publisher", element.getPublisherElement(), false); 33274 composeStringExtras("publisher", element.getPublisherElement(), false); 33275 } 33276 if (element.hasDescriptionElement()) { 33277 composeMarkdownCore("description", element.getDescriptionElement(), false); 33278 composeMarkdownExtras("description", element.getDescriptionElement(), false); 33279 } 33280 if (element.hasPurposeElement()) { 33281 composeMarkdownCore("purpose", element.getPurposeElement(), false); 33282 composeMarkdownExtras("purpose", element.getPurposeElement(), false); 33283 } 33284 if (element.hasUsageElement()) { 33285 composeStringCore("usage", element.getUsageElement(), false); 33286 composeStringExtras("usage", element.getUsageElement(), false); 33287 } 33288 if (element.hasApprovalDateElement()) { 33289 composeDateCore("approvalDate", element.getApprovalDateElement(), false); 33290 composeDateExtras("approvalDate", element.getApprovalDateElement(), false); 33291 } 33292 if (element.hasLastReviewDateElement()) { 33293 composeDateCore("lastReviewDate", element.getLastReviewDateElement(), false); 33294 composeDateExtras("lastReviewDate", element.getLastReviewDateElement(), false); 33295 } 33296 if (element.hasEffectivePeriod()) { 33297 composePeriod("effectivePeriod", element.getEffectivePeriod()); 33298 } 33299 if (element.hasUseContext()) { 33300 openArray("useContext"); 33301 for (UsageContext e : element.getUseContext()) 33302 composeUsageContext(null, e); 33303 closeArray(); 33304 }; 33305 if (element.hasJurisdiction()) { 33306 openArray("jurisdiction"); 33307 for (CodeableConcept e : element.getJurisdiction()) 33308 composeCodeableConcept(null, e); 33309 closeArray(); 33310 }; 33311 if (element.hasTopic()) { 33312 openArray("topic"); 33313 for (CodeableConcept e : element.getTopic()) 33314 composeCodeableConcept(null, e); 33315 closeArray(); 33316 }; 33317 if (element.hasContributor()) { 33318 openArray("contributor"); 33319 for (Contributor e : element.getContributor()) 33320 composeContributor(null, e); 33321 closeArray(); 33322 }; 33323 if (element.hasContact()) { 33324 openArray("contact"); 33325 for (ContactDetail e : element.getContact()) 33326 composeContactDetail(null, e); 33327 closeArray(); 33328 }; 33329 if (element.hasCopyrightElement()) { 33330 composeMarkdownCore("copyright", element.getCopyrightElement(), false); 33331 composeMarkdownExtras("copyright", element.getCopyrightElement(), false); 33332 } 33333 if (element.hasRelatedArtifact()) { 33334 openArray("relatedArtifact"); 33335 for (RelatedArtifact e : element.getRelatedArtifact()) 33336 composeRelatedArtifact(null, e); 33337 closeArray(); 33338 }; 33339 if (element.hasLibrary()) { 33340 openArray("library"); 33341 for (Reference e : element.getLibrary()) 33342 composeReference(null, e); 33343 closeArray(); 33344 }; 33345 if (element.hasDisclaimerElement()) { 33346 composeMarkdownCore("disclaimer", element.getDisclaimerElement(), false); 33347 composeMarkdownExtras("disclaimer", element.getDisclaimerElement(), false); 33348 } 33349 if (element.hasScoring()) { 33350 composeCodeableConcept("scoring", element.getScoring()); 33351 } 33352 if (element.hasCompositeScoring()) { 33353 composeCodeableConcept("compositeScoring", element.getCompositeScoring()); 33354 } 33355 if (element.hasType()) { 33356 openArray("type"); 33357 for (CodeableConcept e : element.getType()) 33358 composeCodeableConcept(null, e); 33359 closeArray(); 33360 }; 33361 if (element.hasRiskAdjustmentElement()) { 33362 composeStringCore("riskAdjustment", element.getRiskAdjustmentElement(), false); 33363 composeStringExtras("riskAdjustment", element.getRiskAdjustmentElement(), false); 33364 } 33365 if (element.hasRateAggregationElement()) { 33366 composeStringCore("rateAggregation", element.getRateAggregationElement(), false); 33367 composeStringExtras("rateAggregation", element.getRateAggregationElement(), false); 33368 } 33369 if (element.hasRationaleElement()) { 33370 composeMarkdownCore("rationale", element.getRationaleElement(), false); 33371 composeMarkdownExtras("rationale", element.getRationaleElement(), false); 33372 } 33373 if (element.hasClinicalRecommendationStatementElement()) { 33374 composeMarkdownCore("clinicalRecommendationStatement", element.getClinicalRecommendationStatementElement(), false); 33375 composeMarkdownExtras("clinicalRecommendationStatement", element.getClinicalRecommendationStatementElement(), false); 33376 } 33377 if (element.hasImprovementNotationElement()) { 33378 composeStringCore("improvementNotation", element.getImprovementNotationElement(), false); 33379 composeStringExtras("improvementNotation", element.getImprovementNotationElement(), false); 33380 } 33381 if (element.hasDefinition()) { 33382 openArray("definition"); 33383 for (MarkdownType e : element.getDefinition()) 33384 composeMarkdownCore(null, e, true); 33385 closeArray(); 33386 if (anyHasExtras(element.getDefinition())) { 33387 openArray("_definition"); 33388 for (MarkdownType e : element.getDefinition()) 33389 composeMarkdownExtras(null, e, true); 33390 closeArray(); 33391 } 33392 }; 33393 if (element.hasGuidanceElement()) { 33394 composeMarkdownCore("guidance", element.getGuidanceElement(), false); 33395 composeMarkdownExtras("guidance", element.getGuidanceElement(), false); 33396 } 33397 if (element.hasSetElement()) { 33398 composeStringCore("set", element.getSetElement(), false); 33399 composeStringExtras("set", element.getSetElement(), false); 33400 } 33401 if (element.hasGroup()) { 33402 openArray("group"); 33403 for (Measure.MeasureGroupComponent e : element.getGroup()) 33404 composeMeasureMeasureGroupComponent(null, e); 33405 closeArray(); 33406 }; 33407 if (element.hasSupplementalData()) { 33408 openArray("supplementalData"); 33409 for (Measure.MeasureSupplementalDataComponent e : element.getSupplementalData()) 33410 composeMeasureMeasureSupplementalDataComponent(null, e); 33411 closeArray(); 33412 }; 33413 } 33414 33415 protected void composeMeasureMeasureGroupComponent(String name, Measure.MeasureGroupComponent element) throws IOException { 33416 if (element != null) { 33417 open(name); 33418 composeMeasureMeasureGroupComponentInner(element); 33419 close(); 33420 } 33421 } 33422 33423 protected void composeMeasureMeasureGroupComponentInner(Measure.MeasureGroupComponent element) throws IOException { 33424 composeBackbone(element); 33425 if (element.hasIdentifier()) { 33426 composeIdentifier("identifier", element.getIdentifier()); 33427 } 33428 if (element.hasNameElement()) { 33429 composeStringCore("name", element.getNameElement(), false); 33430 composeStringExtras("name", element.getNameElement(), false); 33431 } 33432 if (element.hasDescriptionElement()) { 33433 composeStringCore("description", element.getDescriptionElement(), false); 33434 composeStringExtras("description", element.getDescriptionElement(), false); 33435 } 33436 if (element.hasPopulation()) { 33437 openArray("population"); 33438 for (Measure.MeasureGroupPopulationComponent e : element.getPopulation()) 33439 composeMeasureMeasureGroupPopulationComponent(null, e); 33440 closeArray(); 33441 }; 33442 if (element.hasStratifier()) { 33443 openArray("stratifier"); 33444 for (Measure.MeasureGroupStratifierComponent e : element.getStratifier()) 33445 composeMeasureMeasureGroupStratifierComponent(null, e); 33446 closeArray(); 33447 }; 33448 } 33449 33450 protected void composeMeasureMeasureGroupPopulationComponent(String name, Measure.MeasureGroupPopulationComponent element) throws IOException { 33451 if (element != null) { 33452 open(name); 33453 composeMeasureMeasureGroupPopulationComponentInner(element); 33454 close(); 33455 } 33456 } 33457 33458 protected void composeMeasureMeasureGroupPopulationComponentInner(Measure.MeasureGroupPopulationComponent element) throws IOException { 33459 composeBackbone(element); 33460 if (element.hasIdentifier()) { 33461 composeIdentifier("identifier", element.getIdentifier()); 33462 } 33463 if (element.hasCode()) { 33464 composeCodeableConcept("code", element.getCode()); 33465 } 33466 if (element.hasNameElement()) { 33467 composeStringCore("name", element.getNameElement(), false); 33468 composeStringExtras("name", element.getNameElement(), false); 33469 } 33470 if (element.hasDescriptionElement()) { 33471 composeStringCore("description", element.getDescriptionElement(), false); 33472 composeStringExtras("description", element.getDescriptionElement(), false); 33473 } 33474 if (element.hasCriteriaElement()) { 33475 composeStringCore("criteria", element.getCriteriaElement(), false); 33476 composeStringExtras("criteria", element.getCriteriaElement(), false); 33477 } 33478 } 33479 33480 protected void composeMeasureMeasureGroupStratifierComponent(String name, Measure.MeasureGroupStratifierComponent element) throws IOException { 33481 if (element != null) { 33482 open(name); 33483 composeMeasureMeasureGroupStratifierComponentInner(element); 33484 close(); 33485 } 33486 } 33487 33488 protected void composeMeasureMeasureGroupStratifierComponentInner(Measure.MeasureGroupStratifierComponent element) throws IOException { 33489 composeBackbone(element); 33490 if (element.hasIdentifier()) { 33491 composeIdentifier("identifier", element.getIdentifier()); 33492 } 33493 if (element.hasCriteriaElement()) { 33494 composeStringCore("criteria", element.getCriteriaElement(), false); 33495 composeStringExtras("criteria", element.getCriteriaElement(), false); 33496 } 33497 if (element.hasPathElement()) { 33498 composeStringCore("path", element.getPathElement(), false); 33499 composeStringExtras("path", element.getPathElement(), false); 33500 } 33501 } 33502 33503 protected void composeMeasureMeasureSupplementalDataComponent(String name, Measure.MeasureSupplementalDataComponent element) throws IOException { 33504 if (element != null) { 33505 open(name); 33506 composeMeasureMeasureSupplementalDataComponentInner(element); 33507 close(); 33508 } 33509 } 33510 33511 protected void composeMeasureMeasureSupplementalDataComponentInner(Measure.MeasureSupplementalDataComponent element) throws IOException { 33512 composeBackbone(element); 33513 if (element.hasIdentifier()) { 33514 composeIdentifier("identifier", element.getIdentifier()); 33515 } 33516 if (element.hasUsage()) { 33517 openArray("usage"); 33518 for (CodeableConcept e : element.getUsage()) 33519 composeCodeableConcept(null, e); 33520 closeArray(); 33521 }; 33522 if (element.hasCriteriaElement()) { 33523 composeStringCore("criteria", element.getCriteriaElement(), false); 33524 composeStringExtras("criteria", element.getCriteriaElement(), false); 33525 } 33526 if (element.hasPathElement()) { 33527 composeStringCore("path", element.getPathElement(), false); 33528 composeStringExtras("path", element.getPathElement(), false); 33529 } 33530 } 33531 33532 protected void composeMeasureReport(String name, MeasureReport element) throws IOException { 33533 if (element != null) { 33534 prop("resourceType", name); 33535 composeMeasureReportInner(element); 33536 } 33537 } 33538 33539 protected void composeMeasureReportInner(MeasureReport element) throws IOException { 33540 composeDomainResourceElements(element); 33541 if (element.hasIdentifier()) { 33542 composeIdentifier("identifier", element.getIdentifier()); 33543 } 33544 if (element.hasStatusElement()) { 33545 composeEnumerationCore("status", element.getStatusElement(), new MeasureReport.MeasureReportStatusEnumFactory(), false); 33546 composeEnumerationExtras("status", element.getStatusElement(), new MeasureReport.MeasureReportStatusEnumFactory(), false); 33547 } 33548 if (element.hasTypeElement()) { 33549 composeEnumerationCore("type", element.getTypeElement(), new MeasureReport.MeasureReportTypeEnumFactory(), false); 33550 composeEnumerationExtras("type", element.getTypeElement(), new MeasureReport.MeasureReportTypeEnumFactory(), false); 33551 } 33552 if (element.hasMeasure()) { 33553 composeReference("measure", element.getMeasure()); 33554 } 33555 if (element.hasPatient()) { 33556 composeReference("patient", element.getPatient()); 33557 } 33558 if (element.hasDateElement()) { 33559 composeDateTimeCore("date", element.getDateElement(), false); 33560 composeDateTimeExtras("date", element.getDateElement(), false); 33561 } 33562 if (element.hasReportingOrganization()) { 33563 composeReference("reportingOrganization", element.getReportingOrganization()); 33564 } 33565 if (element.hasPeriod()) { 33566 composePeriod("period", element.getPeriod()); 33567 } 33568 if (element.hasGroup()) { 33569 openArray("group"); 33570 for (MeasureReport.MeasureReportGroupComponent e : element.getGroup()) 33571 composeMeasureReportMeasureReportGroupComponent(null, e); 33572 closeArray(); 33573 }; 33574 if (element.hasEvaluatedResources()) { 33575 composeReference("evaluatedResources", element.getEvaluatedResources()); 33576 } 33577 } 33578 33579 protected void composeMeasureReportMeasureReportGroupComponent(String name, MeasureReport.MeasureReportGroupComponent element) throws IOException { 33580 if (element != null) { 33581 open(name); 33582 composeMeasureReportMeasureReportGroupComponentInner(element); 33583 close(); 33584 } 33585 } 33586 33587 protected void composeMeasureReportMeasureReportGroupComponentInner(MeasureReport.MeasureReportGroupComponent element) throws IOException { 33588 composeBackbone(element); 33589 if (element.hasIdentifier()) { 33590 composeIdentifier("identifier", element.getIdentifier()); 33591 } 33592 if (element.hasPopulation()) { 33593 openArray("population"); 33594 for (MeasureReport.MeasureReportGroupPopulationComponent e : element.getPopulation()) 33595 composeMeasureReportMeasureReportGroupPopulationComponent(null, e); 33596 closeArray(); 33597 }; 33598 if (element.hasMeasureScoreElement()) { 33599 composeDecimalCore("measureScore", element.getMeasureScoreElement(), false); 33600 composeDecimalExtras("measureScore", element.getMeasureScoreElement(), false); 33601 } 33602 if (element.hasStratifier()) { 33603 openArray("stratifier"); 33604 for (MeasureReport.MeasureReportGroupStratifierComponent e : element.getStratifier()) 33605 composeMeasureReportMeasureReportGroupStratifierComponent(null, e); 33606 closeArray(); 33607 }; 33608 } 33609 33610 protected void composeMeasureReportMeasureReportGroupPopulationComponent(String name, MeasureReport.MeasureReportGroupPopulationComponent element) throws IOException { 33611 if (element != null) { 33612 open(name); 33613 composeMeasureReportMeasureReportGroupPopulationComponentInner(element); 33614 close(); 33615 } 33616 } 33617 33618 protected void composeMeasureReportMeasureReportGroupPopulationComponentInner(MeasureReport.MeasureReportGroupPopulationComponent element) throws IOException { 33619 composeBackbone(element); 33620 if (element.hasIdentifier()) { 33621 composeIdentifier("identifier", element.getIdentifier()); 33622 } 33623 if (element.hasCode()) { 33624 composeCodeableConcept("code", element.getCode()); 33625 } 33626 if (element.hasCountElement()) { 33627 composeIntegerCore("count", element.getCountElement(), false); 33628 composeIntegerExtras("count", element.getCountElement(), false); 33629 } 33630 if (element.hasPatients()) { 33631 composeReference("patients", element.getPatients()); 33632 } 33633 } 33634 33635 protected void composeMeasureReportMeasureReportGroupStratifierComponent(String name, MeasureReport.MeasureReportGroupStratifierComponent element) throws IOException { 33636 if (element != null) { 33637 open(name); 33638 composeMeasureReportMeasureReportGroupStratifierComponentInner(element); 33639 close(); 33640 } 33641 } 33642 33643 protected void composeMeasureReportMeasureReportGroupStratifierComponentInner(MeasureReport.MeasureReportGroupStratifierComponent element) throws IOException { 33644 composeBackbone(element); 33645 if (element.hasIdentifier()) { 33646 composeIdentifier("identifier", element.getIdentifier()); 33647 } 33648 if (element.hasStratum()) { 33649 openArray("stratum"); 33650 for (MeasureReport.StratifierGroupComponent e : element.getStratum()) 33651 composeMeasureReportStratifierGroupComponent(null, e); 33652 closeArray(); 33653 }; 33654 } 33655 33656 protected void composeMeasureReportStratifierGroupComponent(String name, MeasureReport.StratifierGroupComponent element) throws IOException { 33657 if (element != null) { 33658 open(name); 33659 composeMeasureReportStratifierGroupComponentInner(element); 33660 close(); 33661 } 33662 } 33663 33664 protected void composeMeasureReportStratifierGroupComponentInner(MeasureReport.StratifierGroupComponent element) throws IOException { 33665 composeBackbone(element); 33666 if (element.hasValueElement()) { 33667 composeStringCore("value", element.getValueElement(), false); 33668 composeStringExtras("value", element.getValueElement(), false); 33669 } 33670 if (element.hasPopulation()) { 33671 openArray("population"); 33672 for (MeasureReport.StratifierGroupPopulationComponent e : element.getPopulation()) 33673 composeMeasureReportStratifierGroupPopulationComponent(null, e); 33674 closeArray(); 33675 }; 33676 if (element.hasMeasureScoreElement()) { 33677 composeDecimalCore("measureScore", element.getMeasureScoreElement(), false); 33678 composeDecimalExtras("measureScore", element.getMeasureScoreElement(), false); 33679 } 33680 } 33681 33682 protected void composeMeasureReportStratifierGroupPopulationComponent(String name, MeasureReport.StratifierGroupPopulationComponent element) throws IOException { 33683 if (element != null) { 33684 open(name); 33685 composeMeasureReportStratifierGroupPopulationComponentInner(element); 33686 close(); 33687 } 33688 } 33689 33690 protected void composeMeasureReportStratifierGroupPopulationComponentInner(MeasureReport.StratifierGroupPopulationComponent element) throws IOException { 33691 composeBackbone(element); 33692 if (element.hasIdentifier()) { 33693 composeIdentifier("identifier", element.getIdentifier()); 33694 } 33695 if (element.hasCode()) { 33696 composeCodeableConcept("code", element.getCode()); 33697 } 33698 if (element.hasCountElement()) { 33699 composeIntegerCore("count", element.getCountElement(), false); 33700 composeIntegerExtras("count", element.getCountElement(), false); 33701 } 33702 if (element.hasPatients()) { 33703 composeReference("patients", element.getPatients()); 33704 } 33705 } 33706 33707 protected void composeMedia(String name, Media element) throws IOException { 33708 if (element != null) { 33709 prop("resourceType", name); 33710 composeMediaInner(element); 33711 } 33712 } 33713 33714 protected void composeMediaInner(Media element) throws IOException { 33715 composeDomainResourceElements(element); 33716 if (element.hasIdentifier()) { 33717 openArray("identifier"); 33718 for (Identifier e : element.getIdentifier()) 33719 composeIdentifier(null, e); 33720 closeArray(); 33721 }; 33722 if (element.hasBasedOn()) { 33723 openArray("basedOn"); 33724 for (Reference e : element.getBasedOn()) 33725 composeReference(null, e); 33726 closeArray(); 33727 }; 33728 if (element.hasTypeElement()) { 33729 composeEnumerationCore("type", element.getTypeElement(), new Media.DigitalMediaTypeEnumFactory(), false); 33730 composeEnumerationExtras("type", element.getTypeElement(), new Media.DigitalMediaTypeEnumFactory(), false); 33731 } 33732 if (element.hasSubtype()) { 33733 composeCodeableConcept("subtype", element.getSubtype()); 33734 } 33735 if (element.hasView()) { 33736 composeCodeableConcept("view", element.getView()); 33737 } 33738 if (element.hasSubject()) { 33739 composeReference("subject", element.getSubject()); 33740 } 33741 if (element.hasContext()) { 33742 composeReference("context", element.getContext()); 33743 } 33744 if (element.hasOccurrence()) { 33745 composeType("occurrence", element.getOccurrence()); 33746 } 33747 if (element.hasOperator()) { 33748 composeReference("operator", element.getOperator()); 33749 } 33750 if (element.hasReasonCode()) { 33751 openArray("reasonCode"); 33752 for (CodeableConcept e : element.getReasonCode()) 33753 composeCodeableConcept(null, e); 33754 closeArray(); 33755 }; 33756 if (element.hasBodySite()) { 33757 composeCodeableConcept("bodySite", element.getBodySite()); 33758 } 33759 if (element.hasDevice()) { 33760 composeReference("device", element.getDevice()); 33761 } 33762 if (element.hasHeightElement()) { 33763 composePositiveIntCore("height", element.getHeightElement(), false); 33764 composePositiveIntExtras("height", element.getHeightElement(), false); 33765 } 33766 if (element.hasWidthElement()) { 33767 composePositiveIntCore("width", element.getWidthElement(), false); 33768 composePositiveIntExtras("width", element.getWidthElement(), false); 33769 } 33770 if (element.hasFramesElement()) { 33771 composePositiveIntCore("frames", element.getFramesElement(), false); 33772 composePositiveIntExtras("frames", element.getFramesElement(), false); 33773 } 33774 if (element.hasDurationElement()) { 33775 composeUnsignedIntCore("duration", element.getDurationElement(), false); 33776 composeUnsignedIntExtras("duration", element.getDurationElement(), false); 33777 } 33778 if (element.hasContent()) { 33779 composeAttachment("content", element.getContent()); 33780 } 33781 if (element.hasNote()) { 33782 openArray("note"); 33783 for (Annotation e : element.getNote()) 33784 composeAnnotation(null, e); 33785 closeArray(); 33786 }; 33787 } 33788 33789 protected void composeMedication(String name, Medication element) throws IOException { 33790 if (element != null) { 33791 prop("resourceType", name); 33792 composeMedicationInner(element); 33793 } 33794 } 33795 33796 protected void composeMedicationInner(Medication element) throws IOException { 33797 composeDomainResourceElements(element); 33798 if (element.hasCode()) { 33799 composeCodeableConcept("code", element.getCode()); 33800 } 33801 if (element.hasStatusElement()) { 33802 composeEnumerationCore("status", element.getStatusElement(), new Medication.MedicationStatusEnumFactory(), false); 33803 composeEnumerationExtras("status", element.getStatusElement(), new Medication.MedicationStatusEnumFactory(), false); 33804 } 33805 if (element.hasIsBrandElement()) { 33806 composeBooleanCore("isBrand", element.getIsBrandElement(), false); 33807 composeBooleanExtras("isBrand", element.getIsBrandElement(), false); 33808 } 33809 if (element.hasIsOverTheCounterElement()) { 33810 composeBooleanCore("isOverTheCounter", element.getIsOverTheCounterElement(), false); 33811 composeBooleanExtras("isOverTheCounter", element.getIsOverTheCounterElement(), false); 33812 } 33813 if (element.hasManufacturer()) { 33814 composeReference("manufacturer", element.getManufacturer()); 33815 } 33816 if (element.hasForm()) { 33817 composeCodeableConcept("form", element.getForm()); 33818 } 33819 if (element.hasIngredient()) { 33820 openArray("ingredient"); 33821 for (Medication.MedicationIngredientComponent e : element.getIngredient()) 33822 composeMedicationMedicationIngredientComponent(null, e); 33823 closeArray(); 33824 }; 33825 if (element.hasPackage()) { 33826 composeMedicationMedicationPackageComponent("package", element.getPackage()); 33827 } 33828 if (element.hasImage()) { 33829 openArray("image"); 33830 for (Attachment e : element.getImage()) 33831 composeAttachment(null, e); 33832 closeArray(); 33833 }; 33834 } 33835 33836 protected void composeMedicationMedicationIngredientComponent(String name, Medication.MedicationIngredientComponent element) throws IOException { 33837 if (element != null) { 33838 open(name); 33839 composeMedicationMedicationIngredientComponentInner(element); 33840 close(); 33841 } 33842 } 33843 33844 protected void composeMedicationMedicationIngredientComponentInner(Medication.MedicationIngredientComponent element) throws IOException { 33845 composeBackbone(element); 33846 if (element.hasItem()) { 33847 composeType("item", element.getItem()); 33848 } 33849 if (element.hasIsActiveElement()) { 33850 composeBooleanCore("isActive", element.getIsActiveElement(), false); 33851 composeBooleanExtras("isActive", element.getIsActiveElement(), false); 33852 } 33853 if (element.hasAmount()) { 33854 composeRatio("amount", element.getAmount()); 33855 } 33856 } 33857 33858 protected void composeMedicationMedicationPackageComponent(String name, Medication.MedicationPackageComponent element) throws IOException { 33859 if (element != null) { 33860 open(name); 33861 composeMedicationMedicationPackageComponentInner(element); 33862 close(); 33863 } 33864 } 33865 33866 protected void composeMedicationMedicationPackageComponentInner(Medication.MedicationPackageComponent element) throws IOException { 33867 composeBackbone(element); 33868 if (element.hasContainer()) { 33869 composeCodeableConcept("container", element.getContainer()); 33870 } 33871 if (element.hasContent()) { 33872 openArray("content"); 33873 for (Medication.MedicationPackageContentComponent e : element.getContent()) 33874 composeMedicationMedicationPackageContentComponent(null, e); 33875 closeArray(); 33876 }; 33877 if (element.hasBatch()) { 33878 openArray("batch"); 33879 for (Medication.MedicationPackageBatchComponent e : element.getBatch()) 33880 composeMedicationMedicationPackageBatchComponent(null, e); 33881 closeArray(); 33882 }; 33883 } 33884 33885 protected void composeMedicationMedicationPackageContentComponent(String name, Medication.MedicationPackageContentComponent element) throws IOException { 33886 if (element != null) { 33887 open(name); 33888 composeMedicationMedicationPackageContentComponentInner(element); 33889 close(); 33890 } 33891 } 33892 33893 protected void composeMedicationMedicationPackageContentComponentInner(Medication.MedicationPackageContentComponent element) throws IOException { 33894 composeBackbone(element); 33895 if (element.hasItem()) { 33896 composeType("item", element.getItem()); 33897 } 33898 if (element.hasAmount()) { 33899 composeSimpleQuantity("amount", element.getAmount()); 33900 } 33901 } 33902 33903 protected void composeMedicationMedicationPackageBatchComponent(String name, Medication.MedicationPackageBatchComponent element) throws IOException { 33904 if (element != null) { 33905 open(name); 33906 composeMedicationMedicationPackageBatchComponentInner(element); 33907 close(); 33908 } 33909 } 33910 33911 protected void composeMedicationMedicationPackageBatchComponentInner(Medication.MedicationPackageBatchComponent element) throws IOException { 33912 composeBackbone(element); 33913 if (element.hasLotNumberElement()) { 33914 composeStringCore("lotNumber", element.getLotNumberElement(), false); 33915 composeStringExtras("lotNumber", element.getLotNumberElement(), false); 33916 } 33917 if (element.hasExpirationDateElement()) { 33918 composeDateTimeCore("expirationDate", element.getExpirationDateElement(), false); 33919 composeDateTimeExtras("expirationDate", element.getExpirationDateElement(), false); 33920 } 33921 } 33922 33923 protected void composeMedicationAdministration(String name, MedicationAdministration element) throws IOException { 33924 if (element != null) { 33925 prop("resourceType", name); 33926 composeMedicationAdministrationInner(element); 33927 } 33928 } 33929 33930 protected void composeMedicationAdministrationInner(MedicationAdministration element) throws IOException { 33931 composeDomainResourceElements(element); 33932 if (element.hasIdentifier()) { 33933 openArray("identifier"); 33934 for (Identifier e : element.getIdentifier()) 33935 composeIdentifier(null, e); 33936 closeArray(); 33937 }; 33938 if (element.hasDefinition()) { 33939 openArray("definition"); 33940 for (Reference e : element.getDefinition()) 33941 composeReference(null, e); 33942 closeArray(); 33943 }; 33944 if (element.hasPartOf()) { 33945 openArray("partOf"); 33946 for (Reference e : element.getPartOf()) 33947 composeReference(null, e); 33948 closeArray(); 33949 }; 33950 if (element.hasStatusElement()) { 33951 composeEnumerationCore("status", element.getStatusElement(), new MedicationAdministration.MedicationAdministrationStatusEnumFactory(), false); 33952 composeEnumerationExtras("status", element.getStatusElement(), new MedicationAdministration.MedicationAdministrationStatusEnumFactory(), false); 33953 } 33954 if (element.hasCategory()) { 33955 composeCodeableConcept("category", element.getCategory()); 33956 } 33957 if (element.hasMedication()) { 33958 composeType("medication", element.getMedication()); 33959 } 33960 if (element.hasSubject()) { 33961 composeReference("subject", element.getSubject()); 33962 } 33963 if (element.hasContext()) { 33964 composeReference("context", element.getContext()); 33965 } 33966 if (element.hasSupportingInformation()) { 33967 openArray("supportingInformation"); 33968 for (Reference e : element.getSupportingInformation()) 33969 composeReference(null, e); 33970 closeArray(); 33971 }; 33972 if (element.hasEffective()) { 33973 composeType("effective", element.getEffective()); 33974 } 33975 if (element.hasPerformer()) { 33976 openArray("performer"); 33977 for (MedicationAdministration.MedicationAdministrationPerformerComponent e : element.getPerformer()) 33978 composeMedicationAdministrationMedicationAdministrationPerformerComponent(null, e); 33979 closeArray(); 33980 }; 33981 if (element.hasNotGivenElement()) { 33982 composeBooleanCore("notGiven", element.getNotGivenElement(), false); 33983 composeBooleanExtras("notGiven", element.getNotGivenElement(), false); 33984 } 33985 if (element.hasReasonNotGiven()) { 33986 openArray("reasonNotGiven"); 33987 for (CodeableConcept e : element.getReasonNotGiven()) 33988 composeCodeableConcept(null, e); 33989 closeArray(); 33990 }; 33991 if (element.hasReasonCode()) { 33992 openArray("reasonCode"); 33993 for (CodeableConcept e : element.getReasonCode()) 33994 composeCodeableConcept(null, e); 33995 closeArray(); 33996 }; 33997 if (element.hasReasonReference()) { 33998 openArray("reasonReference"); 33999 for (Reference e : element.getReasonReference()) 34000 composeReference(null, e); 34001 closeArray(); 34002 }; 34003 if (element.hasPrescription()) { 34004 composeReference("prescription", element.getPrescription()); 34005 } 34006 if (element.hasDevice()) { 34007 openArray("device"); 34008 for (Reference e : element.getDevice()) 34009 composeReference(null, e); 34010 closeArray(); 34011 }; 34012 if (element.hasNote()) { 34013 openArray("note"); 34014 for (Annotation e : element.getNote()) 34015 composeAnnotation(null, e); 34016 closeArray(); 34017 }; 34018 if (element.hasDosage()) { 34019 composeMedicationAdministrationMedicationAdministrationDosageComponent("dosage", element.getDosage()); 34020 } 34021 if (element.hasEventHistory()) { 34022 openArray("eventHistory"); 34023 for (Reference e : element.getEventHistory()) 34024 composeReference(null, e); 34025 closeArray(); 34026 }; 34027 } 34028 34029 protected void composeMedicationAdministrationMedicationAdministrationPerformerComponent(String name, MedicationAdministration.MedicationAdministrationPerformerComponent element) throws IOException { 34030 if (element != null) { 34031 open(name); 34032 composeMedicationAdministrationMedicationAdministrationPerformerComponentInner(element); 34033 close(); 34034 } 34035 } 34036 34037 protected void composeMedicationAdministrationMedicationAdministrationPerformerComponentInner(MedicationAdministration.MedicationAdministrationPerformerComponent element) throws IOException { 34038 composeBackbone(element); 34039 if (element.hasActor()) { 34040 composeReference("actor", element.getActor()); 34041 } 34042 if (element.hasOnBehalfOf()) { 34043 composeReference("onBehalfOf", element.getOnBehalfOf()); 34044 } 34045 } 34046 34047 protected void composeMedicationAdministrationMedicationAdministrationDosageComponent(String name, MedicationAdministration.MedicationAdministrationDosageComponent element) throws IOException { 34048 if (element != null) { 34049 open(name); 34050 composeMedicationAdministrationMedicationAdministrationDosageComponentInner(element); 34051 close(); 34052 } 34053 } 34054 34055 protected void composeMedicationAdministrationMedicationAdministrationDosageComponentInner(MedicationAdministration.MedicationAdministrationDosageComponent element) throws IOException { 34056 composeBackbone(element); 34057 if (element.hasTextElement()) { 34058 composeStringCore("text", element.getTextElement(), false); 34059 composeStringExtras("text", element.getTextElement(), false); 34060 } 34061 if (element.hasSite()) { 34062 composeCodeableConcept("site", element.getSite()); 34063 } 34064 if (element.hasRoute()) { 34065 composeCodeableConcept("route", element.getRoute()); 34066 } 34067 if (element.hasMethod()) { 34068 composeCodeableConcept("method", element.getMethod()); 34069 } 34070 if (element.hasDose()) { 34071 composeSimpleQuantity("dose", element.getDose()); 34072 } 34073 if (element.hasRate()) { 34074 composeType("rate", element.getRate()); 34075 } 34076 } 34077 34078 protected void composeMedicationDispense(String name, MedicationDispense element) throws IOException { 34079 if (element != null) { 34080 prop("resourceType", name); 34081 composeMedicationDispenseInner(element); 34082 } 34083 } 34084 34085 protected void composeMedicationDispenseInner(MedicationDispense element) throws IOException { 34086 composeDomainResourceElements(element); 34087 if (element.hasIdentifier()) { 34088 openArray("identifier"); 34089 for (Identifier e : element.getIdentifier()) 34090 composeIdentifier(null, e); 34091 closeArray(); 34092 }; 34093 if (element.hasPartOf()) { 34094 openArray("partOf"); 34095 for (Reference e : element.getPartOf()) 34096 composeReference(null, e); 34097 closeArray(); 34098 }; 34099 if (element.hasStatusElement()) { 34100 composeEnumerationCore("status", element.getStatusElement(), new MedicationDispense.MedicationDispenseStatusEnumFactory(), false); 34101 composeEnumerationExtras("status", element.getStatusElement(), new MedicationDispense.MedicationDispenseStatusEnumFactory(), false); 34102 } 34103 if (element.hasCategory()) { 34104 composeCodeableConcept("category", element.getCategory()); 34105 } 34106 if (element.hasMedication()) { 34107 composeType("medication", element.getMedication()); 34108 } 34109 if (element.hasSubject()) { 34110 composeReference("subject", element.getSubject()); 34111 } 34112 if (element.hasContext()) { 34113 composeReference("context", element.getContext()); 34114 } 34115 if (element.hasSupportingInformation()) { 34116 openArray("supportingInformation"); 34117 for (Reference e : element.getSupportingInformation()) 34118 composeReference(null, e); 34119 closeArray(); 34120 }; 34121 if (element.hasPerformer()) { 34122 openArray("performer"); 34123 for (MedicationDispense.MedicationDispensePerformerComponent e : element.getPerformer()) 34124 composeMedicationDispenseMedicationDispensePerformerComponent(null, e); 34125 closeArray(); 34126 }; 34127 if (element.hasAuthorizingPrescription()) { 34128 openArray("authorizingPrescription"); 34129 for (Reference e : element.getAuthorizingPrescription()) 34130 composeReference(null, e); 34131 closeArray(); 34132 }; 34133 if (element.hasType()) { 34134 composeCodeableConcept("type", element.getType()); 34135 } 34136 if (element.hasQuantity()) { 34137 composeSimpleQuantity("quantity", element.getQuantity()); 34138 } 34139 if (element.hasDaysSupply()) { 34140 composeSimpleQuantity("daysSupply", element.getDaysSupply()); 34141 } 34142 if (element.hasWhenPreparedElement()) { 34143 composeDateTimeCore("whenPrepared", element.getWhenPreparedElement(), false); 34144 composeDateTimeExtras("whenPrepared", element.getWhenPreparedElement(), false); 34145 } 34146 if (element.hasWhenHandedOverElement()) { 34147 composeDateTimeCore("whenHandedOver", element.getWhenHandedOverElement(), false); 34148 composeDateTimeExtras("whenHandedOver", element.getWhenHandedOverElement(), false); 34149 } 34150 if (element.hasDestination()) { 34151 composeReference("destination", element.getDestination()); 34152 } 34153 if (element.hasReceiver()) { 34154 openArray("receiver"); 34155 for (Reference e : element.getReceiver()) 34156 composeReference(null, e); 34157 closeArray(); 34158 }; 34159 if (element.hasNote()) { 34160 openArray("note"); 34161 for (Annotation e : element.getNote()) 34162 composeAnnotation(null, e); 34163 closeArray(); 34164 }; 34165 if (element.hasDosageInstruction()) { 34166 openArray("dosageInstruction"); 34167 for (Dosage e : element.getDosageInstruction()) 34168 composeDosage(null, e); 34169 closeArray(); 34170 }; 34171 if (element.hasSubstitution()) { 34172 composeMedicationDispenseMedicationDispenseSubstitutionComponent("substitution", element.getSubstitution()); 34173 } 34174 if (element.hasDetectedIssue()) { 34175 openArray("detectedIssue"); 34176 for (Reference e : element.getDetectedIssue()) 34177 composeReference(null, e); 34178 closeArray(); 34179 }; 34180 if (element.hasNotDoneElement()) { 34181 composeBooleanCore("notDone", element.getNotDoneElement(), false); 34182 composeBooleanExtras("notDone", element.getNotDoneElement(), false); 34183 } 34184 if (element.hasNotDoneReason()) { 34185 composeType("notDoneReason", element.getNotDoneReason()); 34186 } 34187 if (element.hasEventHistory()) { 34188 openArray("eventHistory"); 34189 for (Reference e : element.getEventHistory()) 34190 composeReference(null, e); 34191 closeArray(); 34192 }; 34193 } 34194 34195 protected void composeMedicationDispenseMedicationDispensePerformerComponent(String name, MedicationDispense.MedicationDispensePerformerComponent element) throws IOException { 34196 if (element != null) { 34197 open(name); 34198 composeMedicationDispenseMedicationDispensePerformerComponentInner(element); 34199 close(); 34200 } 34201 } 34202 34203 protected void composeMedicationDispenseMedicationDispensePerformerComponentInner(MedicationDispense.MedicationDispensePerformerComponent element) throws IOException { 34204 composeBackbone(element); 34205 if (element.hasActor()) { 34206 composeReference("actor", element.getActor()); 34207 } 34208 if (element.hasOnBehalfOf()) { 34209 composeReference("onBehalfOf", element.getOnBehalfOf()); 34210 } 34211 } 34212 34213 protected void composeMedicationDispenseMedicationDispenseSubstitutionComponent(String name, MedicationDispense.MedicationDispenseSubstitutionComponent element) throws IOException { 34214 if (element != null) { 34215 open(name); 34216 composeMedicationDispenseMedicationDispenseSubstitutionComponentInner(element); 34217 close(); 34218 } 34219 } 34220 34221 protected void composeMedicationDispenseMedicationDispenseSubstitutionComponentInner(MedicationDispense.MedicationDispenseSubstitutionComponent element) throws IOException { 34222 composeBackbone(element); 34223 if (element.hasWasSubstitutedElement()) { 34224 composeBooleanCore("wasSubstituted", element.getWasSubstitutedElement(), false); 34225 composeBooleanExtras("wasSubstituted", element.getWasSubstitutedElement(), false); 34226 } 34227 if (element.hasType()) { 34228 composeCodeableConcept("type", element.getType()); 34229 } 34230 if (element.hasReason()) { 34231 openArray("reason"); 34232 for (CodeableConcept e : element.getReason()) 34233 composeCodeableConcept(null, e); 34234 closeArray(); 34235 }; 34236 if (element.hasResponsibleParty()) { 34237 openArray("responsibleParty"); 34238 for (Reference e : element.getResponsibleParty()) 34239 composeReference(null, e); 34240 closeArray(); 34241 }; 34242 } 34243 34244 protected void composeMedicationRequest(String name, MedicationRequest element) throws IOException { 34245 if (element != null) { 34246 prop("resourceType", name); 34247 composeMedicationRequestInner(element); 34248 } 34249 } 34250 34251 protected void composeMedicationRequestInner(MedicationRequest element) throws IOException { 34252 composeDomainResourceElements(element); 34253 if (element.hasIdentifier()) { 34254 openArray("identifier"); 34255 for (Identifier e : element.getIdentifier()) 34256 composeIdentifier(null, e); 34257 closeArray(); 34258 }; 34259 if (element.hasDefinition()) { 34260 openArray("definition"); 34261 for (Reference e : element.getDefinition()) 34262 composeReference(null, e); 34263 closeArray(); 34264 }; 34265 if (element.hasBasedOn()) { 34266 openArray("basedOn"); 34267 for (Reference e : element.getBasedOn()) 34268 composeReference(null, e); 34269 closeArray(); 34270 }; 34271 if (element.hasGroupIdentifier()) { 34272 composeIdentifier("groupIdentifier", element.getGroupIdentifier()); 34273 } 34274 if (element.hasStatusElement()) { 34275 composeEnumerationCore("status", element.getStatusElement(), new MedicationRequest.MedicationRequestStatusEnumFactory(), false); 34276 composeEnumerationExtras("status", element.getStatusElement(), new MedicationRequest.MedicationRequestStatusEnumFactory(), false); 34277 } 34278 if (element.hasIntentElement()) { 34279 composeEnumerationCore("intent", element.getIntentElement(), new MedicationRequest.MedicationRequestIntentEnumFactory(), false); 34280 composeEnumerationExtras("intent", element.getIntentElement(), new MedicationRequest.MedicationRequestIntentEnumFactory(), false); 34281 } 34282 if (element.hasCategory()) { 34283 composeCodeableConcept("category", element.getCategory()); 34284 } 34285 if (element.hasPriorityElement()) { 34286 composeEnumerationCore("priority", element.getPriorityElement(), new MedicationRequest.MedicationRequestPriorityEnumFactory(), false); 34287 composeEnumerationExtras("priority", element.getPriorityElement(), new MedicationRequest.MedicationRequestPriorityEnumFactory(), false); 34288 } 34289 if (element.hasMedication()) { 34290 composeType("medication", element.getMedication()); 34291 } 34292 if (element.hasSubject()) { 34293 composeReference("subject", element.getSubject()); 34294 } 34295 if (element.hasContext()) { 34296 composeReference("context", element.getContext()); 34297 } 34298 if (element.hasSupportingInformation()) { 34299 openArray("supportingInformation"); 34300 for (Reference e : element.getSupportingInformation()) 34301 composeReference(null, e); 34302 closeArray(); 34303 }; 34304 if (element.hasAuthoredOnElement()) { 34305 composeDateTimeCore("authoredOn", element.getAuthoredOnElement(), false); 34306 composeDateTimeExtras("authoredOn", element.getAuthoredOnElement(), false); 34307 } 34308 if (element.hasRequester()) { 34309 composeMedicationRequestMedicationRequestRequesterComponent("requester", element.getRequester()); 34310 } 34311 if (element.hasRecorder()) { 34312 composeReference("recorder", element.getRecorder()); 34313 } 34314 if (element.hasReasonCode()) { 34315 openArray("reasonCode"); 34316 for (CodeableConcept e : element.getReasonCode()) 34317 composeCodeableConcept(null, e); 34318 closeArray(); 34319 }; 34320 if (element.hasReasonReference()) { 34321 openArray("reasonReference"); 34322 for (Reference e : element.getReasonReference()) 34323 composeReference(null, e); 34324 closeArray(); 34325 }; 34326 if (element.hasNote()) { 34327 openArray("note"); 34328 for (Annotation e : element.getNote()) 34329 composeAnnotation(null, e); 34330 closeArray(); 34331 }; 34332 if (element.hasDosageInstruction()) { 34333 openArray("dosageInstruction"); 34334 for (Dosage e : element.getDosageInstruction()) 34335 composeDosage(null, e); 34336 closeArray(); 34337 }; 34338 if (element.hasDispenseRequest()) { 34339 composeMedicationRequestMedicationRequestDispenseRequestComponent("dispenseRequest", element.getDispenseRequest()); 34340 } 34341 if (element.hasSubstitution()) { 34342 composeMedicationRequestMedicationRequestSubstitutionComponent("substitution", element.getSubstitution()); 34343 } 34344 if (element.hasPriorPrescription()) { 34345 composeReference("priorPrescription", element.getPriorPrescription()); 34346 } 34347 if (element.hasDetectedIssue()) { 34348 openArray("detectedIssue"); 34349 for (Reference e : element.getDetectedIssue()) 34350 composeReference(null, e); 34351 closeArray(); 34352 }; 34353 if (element.hasEventHistory()) { 34354 openArray("eventHistory"); 34355 for (Reference e : element.getEventHistory()) 34356 composeReference(null, e); 34357 closeArray(); 34358 }; 34359 } 34360 34361 protected void composeMedicationRequestMedicationRequestRequesterComponent(String name, MedicationRequest.MedicationRequestRequesterComponent element) throws IOException { 34362 if (element != null) { 34363 open(name); 34364 composeMedicationRequestMedicationRequestRequesterComponentInner(element); 34365 close(); 34366 } 34367 } 34368 34369 protected void composeMedicationRequestMedicationRequestRequesterComponentInner(MedicationRequest.MedicationRequestRequesterComponent element) throws IOException { 34370 composeBackbone(element); 34371 if (element.hasAgent()) { 34372 composeReference("agent", element.getAgent()); 34373 } 34374 if (element.hasOnBehalfOf()) { 34375 composeReference("onBehalfOf", element.getOnBehalfOf()); 34376 } 34377 } 34378 34379 protected void composeMedicationRequestMedicationRequestDispenseRequestComponent(String name, MedicationRequest.MedicationRequestDispenseRequestComponent element) throws IOException { 34380 if (element != null) { 34381 open(name); 34382 composeMedicationRequestMedicationRequestDispenseRequestComponentInner(element); 34383 close(); 34384 } 34385 } 34386 34387 protected void composeMedicationRequestMedicationRequestDispenseRequestComponentInner(MedicationRequest.MedicationRequestDispenseRequestComponent element) throws IOException { 34388 composeBackbone(element); 34389 if (element.hasValidityPeriod()) { 34390 composePeriod("validityPeriod", element.getValidityPeriod()); 34391 } 34392 if (element.hasNumberOfRepeatsAllowedElement()) { 34393 composePositiveIntCore("numberOfRepeatsAllowed", element.getNumberOfRepeatsAllowedElement(), false); 34394 composePositiveIntExtras("numberOfRepeatsAllowed", element.getNumberOfRepeatsAllowedElement(), false); 34395 } 34396 if (element.hasQuantity()) { 34397 composeSimpleQuantity("quantity", element.getQuantity()); 34398 } 34399 if (element.hasExpectedSupplyDuration()) { 34400 composeDuration("expectedSupplyDuration", element.getExpectedSupplyDuration()); 34401 } 34402 if (element.hasPerformer()) { 34403 composeReference("performer", element.getPerformer()); 34404 } 34405 } 34406 34407 protected void composeMedicationRequestMedicationRequestSubstitutionComponent(String name, MedicationRequest.MedicationRequestSubstitutionComponent element) throws IOException { 34408 if (element != null) { 34409 open(name); 34410 composeMedicationRequestMedicationRequestSubstitutionComponentInner(element); 34411 close(); 34412 } 34413 } 34414 34415 protected void composeMedicationRequestMedicationRequestSubstitutionComponentInner(MedicationRequest.MedicationRequestSubstitutionComponent element) throws IOException { 34416 composeBackbone(element); 34417 if (element.hasAllowedElement()) { 34418 composeBooleanCore("allowed", element.getAllowedElement(), false); 34419 composeBooleanExtras("allowed", element.getAllowedElement(), false); 34420 } 34421 if (element.hasReason()) { 34422 composeCodeableConcept("reason", element.getReason()); 34423 } 34424 } 34425 34426 protected void composeMedicationStatement(String name, MedicationStatement element) throws IOException { 34427 if (element != null) { 34428 prop("resourceType", name); 34429 composeMedicationStatementInner(element); 34430 } 34431 } 34432 34433 protected void composeMedicationStatementInner(MedicationStatement element) throws IOException { 34434 composeDomainResourceElements(element); 34435 if (element.hasIdentifier()) { 34436 openArray("identifier"); 34437 for (Identifier e : element.getIdentifier()) 34438 composeIdentifier(null, e); 34439 closeArray(); 34440 }; 34441 if (element.hasBasedOn()) { 34442 openArray("basedOn"); 34443 for (Reference e : element.getBasedOn()) 34444 composeReference(null, e); 34445 closeArray(); 34446 }; 34447 if (element.hasPartOf()) { 34448 openArray("partOf"); 34449 for (Reference e : element.getPartOf()) 34450 composeReference(null, e); 34451 closeArray(); 34452 }; 34453 if (element.hasContext()) { 34454 composeReference("context", element.getContext()); 34455 } 34456 if (element.hasStatusElement()) { 34457 composeEnumerationCore("status", element.getStatusElement(), new MedicationStatement.MedicationStatementStatusEnumFactory(), false); 34458 composeEnumerationExtras("status", element.getStatusElement(), new MedicationStatement.MedicationStatementStatusEnumFactory(), false); 34459 } 34460 if (element.hasCategory()) { 34461 composeCodeableConcept("category", element.getCategory()); 34462 } 34463 if (element.hasMedication()) { 34464 composeType("medication", element.getMedication()); 34465 } 34466 if (element.hasEffective()) { 34467 composeType("effective", element.getEffective()); 34468 } 34469 if (element.hasDateAssertedElement()) { 34470 composeDateTimeCore("dateAsserted", element.getDateAssertedElement(), false); 34471 composeDateTimeExtras("dateAsserted", element.getDateAssertedElement(), false); 34472 } 34473 if (element.hasInformationSource()) { 34474 composeReference("informationSource", element.getInformationSource()); 34475 } 34476 if (element.hasSubject()) { 34477 composeReference("subject", element.getSubject()); 34478 } 34479 if (element.hasDerivedFrom()) { 34480 openArray("derivedFrom"); 34481 for (Reference e : element.getDerivedFrom()) 34482 composeReference(null, e); 34483 closeArray(); 34484 }; 34485 if (element.hasTakenElement()) { 34486 composeEnumerationCore("taken", element.getTakenElement(), new MedicationStatement.MedicationStatementTakenEnumFactory(), false); 34487 composeEnumerationExtras("taken", element.getTakenElement(), new MedicationStatement.MedicationStatementTakenEnumFactory(), false); 34488 } 34489 if (element.hasReasonNotTaken()) { 34490 openArray("reasonNotTaken"); 34491 for (CodeableConcept e : element.getReasonNotTaken()) 34492 composeCodeableConcept(null, e); 34493 closeArray(); 34494 }; 34495 if (element.hasReasonCode()) { 34496 openArray("reasonCode"); 34497 for (CodeableConcept e : element.getReasonCode()) 34498 composeCodeableConcept(null, e); 34499 closeArray(); 34500 }; 34501 if (element.hasReasonReference()) { 34502 openArray("reasonReference"); 34503 for (Reference e : element.getReasonReference()) 34504 composeReference(null, e); 34505 closeArray(); 34506 }; 34507 if (element.hasNote()) { 34508 openArray("note"); 34509 for (Annotation e : element.getNote()) 34510 composeAnnotation(null, e); 34511 closeArray(); 34512 }; 34513 if (element.hasDosage()) { 34514 openArray("dosage"); 34515 for (Dosage e : element.getDosage()) 34516 composeDosage(null, e); 34517 closeArray(); 34518 }; 34519 } 34520 34521 protected void composeMessageDefinition(String name, MessageDefinition element) throws IOException { 34522 if (element != null) { 34523 prop("resourceType", name); 34524 composeMessageDefinitionInner(element); 34525 } 34526 } 34527 34528 protected void composeMessageDefinitionInner(MessageDefinition element) throws IOException { 34529 composeDomainResourceElements(element); 34530 if (element.hasUrlElement()) { 34531 composeUriCore("url", element.getUrlElement(), false); 34532 composeUriExtras("url", element.getUrlElement(), false); 34533 } 34534 if (element.hasIdentifier()) { 34535 composeIdentifier("identifier", element.getIdentifier()); 34536 } 34537 if (element.hasVersionElement()) { 34538 composeStringCore("version", element.getVersionElement(), false); 34539 composeStringExtras("version", element.getVersionElement(), false); 34540 } 34541 if (element.hasNameElement()) { 34542 composeStringCore("name", element.getNameElement(), false); 34543 composeStringExtras("name", element.getNameElement(), false); 34544 } 34545 if (element.hasTitleElement()) { 34546 composeStringCore("title", element.getTitleElement(), false); 34547 composeStringExtras("title", element.getTitleElement(), false); 34548 } 34549 if (element.hasStatusElement()) { 34550 composeEnumerationCore("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 34551 composeEnumerationExtras("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 34552 } 34553 if (element.hasExperimentalElement()) { 34554 composeBooleanCore("experimental", element.getExperimentalElement(), false); 34555 composeBooleanExtras("experimental", element.getExperimentalElement(), false); 34556 } 34557 if (element.hasDateElement()) { 34558 composeDateTimeCore("date", element.getDateElement(), false); 34559 composeDateTimeExtras("date", element.getDateElement(), false); 34560 } 34561 if (element.hasPublisherElement()) { 34562 composeStringCore("publisher", element.getPublisherElement(), false); 34563 composeStringExtras("publisher", element.getPublisherElement(), false); 34564 } 34565 if (element.hasContact()) { 34566 openArray("contact"); 34567 for (ContactDetail e : element.getContact()) 34568 composeContactDetail(null, e); 34569 closeArray(); 34570 }; 34571 if (element.hasDescriptionElement()) { 34572 composeMarkdownCore("description", element.getDescriptionElement(), false); 34573 composeMarkdownExtras("description", element.getDescriptionElement(), false); 34574 } 34575 if (element.hasUseContext()) { 34576 openArray("useContext"); 34577 for (UsageContext e : element.getUseContext()) 34578 composeUsageContext(null, e); 34579 closeArray(); 34580 }; 34581 if (element.hasJurisdiction()) { 34582 openArray("jurisdiction"); 34583 for (CodeableConcept e : element.getJurisdiction()) 34584 composeCodeableConcept(null, e); 34585 closeArray(); 34586 }; 34587 if (element.hasPurposeElement()) { 34588 composeMarkdownCore("purpose", element.getPurposeElement(), false); 34589 composeMarkdownExtras("purpose", element.getPurposeElement(), false); 34590 } 34591 if (element.hasCopyrightElement()) { 34592 composeMarkdownCore("copyright", element.getCopyrightElement(), false); 34593 composeMarkdownExtras("copyright", element.getCopyrightElement(), false); 34594 } 34595 if (element.hasBase()) { 34596 composeReference("base", element.getBase()); 34597 } 34598 if (element.hasParent()) { 34599 openArray("parent"); 34600 for (Reference e : element.getParent()) 34601 composeReference(null, e); 34602 closeArray(); 34603 }; 34604 if (element.hasReplaces()) { 34605 openArray("replaces"); 34606 for (Reference e : element.getReplaces()) 34607 composeReference(null, e); 34608 closeArray(); 34609 }; 34610 if (element.hasEvent()) { 34611 composeCoding("event", element.getEvent()); 34612 } 34613 if (element.hasCategoryElement()) { 34614 composeEnumerationCore("category", element.getCategoryElement(), new MessageDefinition.MessageSignificanceCategoryEnumFactory(), false); 34615 composeEnumerationExtras("category", element.getCategoryElement(), new MessageDefinition.MessageSignificanceCategoryEnumFactory(), false); 34616 } 34617 if (element.hasFocus()) { 34618 openArray("focus"); 34619 for (MessageDefinition.MessageDefinitionFocusComponent e : element.getFocus()) 34620 composeMessageDefinitionMessageDefinitionFocusComponent(null, e); 34621 closeArray(); 34622 }; 34623 if (element.hasResponseRequiredElement()) { 34624 composeBooleanCore("responseRequired", element.getResponseRequiredElement(), false); 34625 composeBooleanExtras("responseRequired", element.getResponseRequiredElement(), false); 34626 } 34627 if (element.hasAllowedResponse()) { 34628 openArray("allowedResponse"); 34629 for (MessageDefinition.MessageDefinitionAllowedResponseComponent e : element.getAllowedResponse()) 34630 composeMessageDefinitionMessageDefinitionAllowedResponseComponent(null, e); 34631 closeArray(); 34632 }; 34633 } 34634 34635 protected void composeMessageDefinitionMessageDefinitionFocusComponent(String name, MessageDefinition.MessageDefinitionFocusComponent element) throws IOException { 34636 if (element != null) { 34637 open(name); 34638 composeMessageDefinitionMessageDefinitionFocusComponentInner(element); 34639 close(); 34640 } 34641 } 34642 34643 protected void composeMessageDefinitionMessageDefinitionFocusComponentInner(MessageDefinition.MessageDefinitionFocusComponent element) throws IOException { 34644 composeBackbone(element); 34645 if (element.hasCodeElement()) { 34646 composeCodeCore("code", element.getCodeElement(), false); 34647 composeCodeExtras("code", element.getCodeElement(), false); 34648 } 34649 if (element.hasProfile()) { 34650 composeReference("profile", element.getProfile()); 34651 } 34652 if (element.hasMinElement()) { 34653 composeUnsignedIntCore("min", element.getMinElement(), false); 34654 composeUnsignedIntExtras("min", element.getMinElement(), false); 34655 } 34656 if (element.hasMaxElement()) { 34657 composeStringCore("max", element.getMaxElement(), false); 34658 composeStringExtras("max", element.getMaxElement(), false); 34659 } 34660 } 34661 34662 protected void composeMessageDefinitionMessageDefinitionAllowedResponseComponent(String name, MessageDefinition.MessageDefinitionAllowedResponseComponent element) throws IOException { 34663 if (element != null) { 34664 open(name); 34665 composeMessageDefinitionMessageDefinitionAllowedResponseComponentInner(element); 34666 close(); 34667 } 34668 } 34669 34670 protected void composeMessageDefinitionMessageDefinitionAllowedResponseComponentInner(MessageDefinition.MessageDefinitionAllowedResponseComponent element) throws IOException { 34671 composeBackbone(element); 34672 if (element.hasMessage()) { 34673 composeReference("message", element.getMessage()); 34674 } 34675 if (element.hasSituationElement()) { 34676 composeMarkdownCore("situation", element.getSituationElement(), false); 34677 composeMarkdownExtras("situation", element.getSituationElement(), false); 34678 } 34679 } 34680 34681 protected void composeMessageHeader(String name, MessageHeader element) throws IOException { 34682 if (element != null) { 34683 prop("resourceType", name); 34684 composeMessageHeaderInner(element); 34685 } 34686 } 34687 34688 protected void composeMessageHeaderInner(MessageHeader element) throws IOException { 34689 composeDomainResourceElements(element); 34690 if (element.hasEvent()) { 34691 composeCoding("event", element.getEvent()); 34692 } 34693 if (element.hasDestination()) { 34694 openArray("destination"); 34695 for (MessageHeader.MessageDestinationComponent e : element.getDestination()) 34696 composeMessageHeaderMessageDestinationComponent(null, e); 34697 closeArray(); 34698 }; 34699 if (element.hasReceiver()) { 34700 composeReference("receiver", element.getReceiver()); 34701 } 34702 if (element.hasSender()) { 34703 composeReference("sender", element.getSender()); 34704 } 34705 if (element.hasTimestampElement()) { 34706 composeInstantCore("timestamp", element.getTimestampElement(), false); 34707 composeInstantExtras("timestamp", element.getTimestampElement(), false); 34708 } 34709 if (element.hasEnterer()) { 34710 composeReference("enterer", element.getEnterer()); 34711 } 34712 if (element.hasAuthor()) { 34713 composeReference("author", element.getAuthor()); 34714 } 34715 if (element.hasSource()) { 34716 composeMessageHeaderMessageSourceComponent("source", element.getSource()); 34717 } 34718 if (element.hasResponsible()) { 34719 composeReference("responsible", element.getResponsible()); 34720 } 34721 if (element.hasReason()) { 34722 composeCodeableConcept("reason", element.getReason()); 34723 } 34724 if (element.hasResponse()) { 34725 composeMessageHeaderMessageHeaderResponseComponent("response", element.getResponse()); 34726 } 34727 if (element.hasFocus()) { 34728 openArray("focus"); 34729 for (Reference e : element.getFocus()) 34730 composeReference(null, e); 34731 closeArray(); 34732 }; 34733 } 34734 34735 protected void composeMessageHeaderMessageDestinationComponent(String name, MessageHeader.MessageDestinationComponent element) throws IOException { 34736 if (element != null) { 34737 open(name); 34738 composeMessageHeaderMessageDestinationComponentInner(element); 34739 close(); 34740 } 34741 } 34742 34743 protected void composeMessageHeaderMessageDestinationComponentInner(MessageHeader.MessageDestinationComponent element) throws IOException { 34744 composeBackbone(element); 34745 if (element.hasNameElement()) { 34746 composeStringCore("name", element.getNameElement(), false); 34747 composeStringExtras("name", element.getNameElement(), false); 34748 } 34749 if (element.hasTarget()) { 34750 composeReference("target", element.getTarget()); 34751 } 34752 if (element.hasEndpointElement()) { 34753 composeUriCore("endpoint", element.getEndpointElement(), false); 34754 composeUriExtras("endpoint", element.getEndpointElement(), false); 34755 } 34756 } 34757 34758 protected void composeMessageHeaderMessageSourceComponent(String name, MessageHeader.MessageSourceComponent element) throws IOException { 34759 if (element != null) { 34760 open(name); 34761 composeMessageHeaderMessageSourceComponentInner(element); 34762 close(); 34763 } 34764 } 34765 34766 protected void composeMessageHeaderMessageSourceComponentInner(MessageHeader.MessageSourceComponent element) throws IOException { 34767 composeBackbone(element); 34768 if (element.hasNameElement()) { 34769 composeStringCore("name", element.getNameElement(), false); 34770 composeStringExtras("name", element.getNameElement(), false); 34771 } 34772 if (element.hasSoftwareElement()) { 34773 composeStringCore("software", element.getSoftwareElement(), false); 34774 composeStringExtras("software", element.getSoftwareElement(), false); 34775 } 34776 if (element.hasVersionElement()) { 34777 composeStringCore("version", element.getVersionElement(), false); 34778 composeStringExtras("version", element.getVersionElement(), false); 34779 } 34780 if (element.hasContact()) { 34781 composeContactPoint("contact", element.getContact()); 34782 } 34783 if (element.hasEndpointElement()) { 34784 composeUriCore("endpoint", element.getEndpointElement(), false); 34785 composeUriExtras("endpoint", element.getEndpointElement(), false); 34786 } 34787 } 34788 34789 protected void composeMessageHeaderMessageHeaderResponseComponent(String name, MessageHeader.MessageHeaderResponseComponent element) throws IOException { 34790 if (element != null) { 34791 open(name); 34792 composeMessageHeaderMessageHeaderResponseComponentInner(element); 34793 close(); 34794 } 34795 } 34796 34797 protected void composeMessageHeaderMessageHeaderResponseComponentInner(MessageHeader.MessageHeaderResponseComponent element) throws IOException { 34798 composeBackbone(element); 34799 if (element.hasIdentifierElement()) { 34800 composeIdCore("identifier", element.getIdentifierElement(), false); 34801 composeIdExtras("identifier", element.getIdentifierElement(), false); 34802 } 34803 if (element.hasCodeElement()) { 34804 composeEnumerationCore("code", element.getCodeElement(), new MessageHeader.ResponseTypeEnumFactory(), false); 34805 composeEnumerationExtras("code", element.getCodeElement(), new MessageHeader.ResponseTypeEnumFactory(), false); 34806 } 34807 if (element.hasDetails()) { 34808 composeReference("details", element.getDetails()); 34809 } 34810 } 34811 34812 protected void composeNamingSystem(String name, NamingSystem element) throws IOException { 34813 if (element != null) { 34814 prop("resourceType", name); 34815 composeNamingSystemInner(element); 34816 } 34817 } 34818 34819 protected void composeNamingSystemInner(NamingSystem element) throws IOException { 34820 composeDomainResourceElements(element); 34821 if (element.hasNameElement()) { 34822 composeStringCore("name", element.getNameElement(), false); 34823 composeStringExtras("name", element.getNameElement(), false); 34824 } 34825 if (element.hasStatusElement()) { 34826 composeEnumerationCore("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 34827 composeEnumerationExtras("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 34828 } 34829 if (element.hasKindElement()) { 34830 composeEnumerationCore("kind", element.getKindElement(), new NamingSystem.NamingSystemTypeEnumFactory(), false); 34831 composeEnumerationExtras("kind", element.getKindElement(), new NamingSystem.NamingSystemTypeEnumFactory(), false); 34832 } 34833 if (element.hasDateElement()) { 34834 composeDateTimeCore("date", element.getDateElement(), false); 34835 composeDateTimeExtras("date", element.getDateElement(), false); 34836 } 34837 if (element.hasPublisherElement()) { 34838 composeStringCore("publisher", element.getPublisherElement(), false); 34839 composeStringExtras("publisher", element.getPublisherElement(), false); 34840 } 34841 if (element.hasContact()) { 34842 openArray("contact"); 34843 for (ContactDetail e : element.getContact()) 34844 composeContactDetail(null, e); 34845 closeArray(); 34846 }; 34847 if (element.hasResponsibleElement()) { 34848 composeStringCore("responsible", element.getResponsibleElement(), false); 34849 composeStringExtras("responsible", element.getResponsibleElement(), false); 34850 } 34851 if (element.hasType()) { 34852 composeCodeableConcept("type", element.getType()); 34853 } 34854 if (element.hasDescriptionElement()) { 34855 composeMarkdownCore("description", element.getDescriptionElement(), false); 34856 composeMarkdownExtras("description", element.getDescriptionElement(), false); 34857 } 34858 if (element.hasUseContext()) { 34859 openArray("useContext"); 34860 for (UsageContext e : element.getUseContext()) 34861 composeUsageContext(null, e); 34862 closeArray(); 34863 }; 34864 if (element.hasJurisdiction()) { 34865 openArray("jurisdiction"); 34866 for (CodeableConcept e : element.getJurisdiction()) 34867 composeCodeableConcept(null, e); 34868 closeArray(); 34869 }; 34870 if (element.hasUsageElement()) { 34871 composeStringCore("usage", element.getUsageElement(), false); 34872 composeStringExtras("usage", element.getUsageElement(), false); 34873 } 34874 if (element.hasUniqueId()) { 34875 openArray("uniqueId"); 34876 for (NamingSystem.NamingSystemUniqueIdComponent e : element.getUniqueId()) 34877 composeNamingSystemNamingSystemUniqueIdComponent(null, e); 34878 closeArray(); 34879 }; 34880 if (element.hasReplacedBy()) { 34881 composeReference("replacedBy", element.getReplacedBy()); 34882 } 34883 } 34884 34885 protected void composeNamingSystemNamingSystemUniqueIdComponent(String name, NamingSystem.NamingSystemUniqueIdComponent element) throws IOException { 34886 if (element != null) { 34887 open(name); 34888 composeNamingSystemNamingSystemUniqueIdComponentInner(element); 34889 close(); 34890 } 34891 } 34892 34893 protected void composeNamingSystemNamingSystemUniqueIdComponentInner(NamingSystem.NamingSystemUniqueIdComponent element) throws IOException { 34894 composeBackbone(element); 34895 if (element.hasTypeElement()) { 34896 composeEnumerationCore("type", element.getTypeElement(), new NamingSystem.NamingSystemIdentifierTypeEnumFactory(), false); 34897 composeEnumerationExtras("type", element.getTypeElement(), new NamingSystem.NamingSystemIdentifierTypeEnumFactory(), false); 34898 } 34899 if (element.hasValueElement()) { 34900 composeStringCore("value", element.getValueElement(), false); 34901 composeStringExtras("value", element.getValueElement(), false); 34902 } 34903 if (element.hasPreferredElement()) { 34904 composeBooleanCore("preferred", element.getPreferredElement(), false); 34905 composeBooleanExtras("preferred", element.getPreferredElement(), false); 34906 } 34907 if (element.hasCommentElement()) { 34908 composeStringCore("comment", element.getCommentElement(), false); 34909 composeStringExtras("comment", element.getCommentElement(), false); 34910 } 34911 if (element.hasPeriod()) { 34912 composePeriod("period", element.getPeriod()); 34913 } 34914 } 34915 34916 protected void composeNutritionOrder(String name, NutritionOrder element) throws IOException { 34917 if (element != null) { 34918 prop("resourceType", name); 34919 composeNutritionOrderInner(element); 34920 } 34921 } 34922 34923 protected void composeNutritionOrderInner(NutritionOrder element) throws IOException { 34924 composeDomainResourceElements(element); 34925 if (element.hasIdentifier()) { 34926 openArray("identifier"); 34927 for (Identifier e : element.getIdentifier()) 34928 composeIdentifier(null, e); 34929 closeArray(); 34930 }; 34931 if (element.hasStatusElement()) { 34932 composeEnumerationCore("status", element.getStatusElement(), new NutritionOrder.NutritionOrderStatusEnumFactory(), false); 34933 composeEnumerationExtras("status", element.getStatusElement(), new NutritionOrder.NutritionOrderStatusEnumFactory(), false); 34934 } 34935 if (element.hasPatient()) { 34936 composeReference("patient", element.getPatient()); 34937 } 34938 if (element.hasEncounter()) { 34939 composeReference("encounter", element.getEncounter()); 34940 } 34941 if (element.hasDateTimeElement()) { 34942 composeDateTimeCore("dateTime", element.getDateTimeElement(), false); 34943 composeDateTimeExtras("dateTime", element.getDateTimeElement(), false); 34944 } 34945 if (element.hasOrderer()) { 34946 composeReference("orderer", element.getOrderer()); 34947 } 34948 if (element.hasAllergyIntolerance()) { 34949 openArray("allergyIntolerance"); 34950 for (Reference e : element.getAllergyIntolerance()) 34951 composeReference(null, e); 34952 closeArray(); 34953 }; 34954 if (element.hasFoodPreferenceModifier()) { 34955 openArray("foodPreferenceModifier"); 34956 for (CodeableConcept e : element.getFoodPreferenceModifier()) 34957 composeCodeableConcept(null, e); 34958 closeArray(); 34959 }; 34960 if (element.hasExcludeFoodModifier()) { 34961 openArray("excludeFoodModifier"); 34962 for (CodeableConcept e : element.getExcludeFoodModifier()) 34963 composeCodeableConcept(null, e); 34964 closeArray(); 34965 }; 34966 if (element.hasOralDiet()) { 34967 composeNutritionOrderNutritionOrderOralDietComponent("oralDiet", element.getOralDiet()); 34968 } 34969 if (element.hasSupplement()) { 34970 openArray("supplement"); 34971 for (NutritionOrder.NutritionOrderSupplementComponent e : element.getSupplement()) 34972 composeNutritionOrderNutritionOrderSupplementComponent(null, e); 34973 closeArray(); 34974 }; 34975 if (element.hasEnteralFormula()) { 34976 composeNutritionOrderNutritionOrderEnteralFormulaComponent("enteralFormula", element.getEnteralFormula()); 34977 } 34978 } 34979 34980 protected void composeNutritionOrderNutritionOrderOralDietComponent(String name, NutritionOrder.NutritionOrderOralDietComponent element) throws IOException { 34981 if (element != null) { 34982 open(name); 34983 composeNutritionOrderNutritionOrderOralDietComponentInner(element); 34984 close(); 34985 } 34986 } 34987 34988 protected void composeNutritionOrderNutritionOrderOralDietComponentInner(NutritionOrder.NutritionOrderOralDietComponent element) throws IOException { 34989 composeBackbone(element); 34990 if (element.hasType()) { 34991 openArray("type"); 34992 for (CodeableConcept e : element.getType()) 34993 composeCodeableConcept(null, e); 34994 closeArray(); 34995 }; 34996 if (element.hasSchedule()) { 34997 openArray("schedule"); 34998 for (Timing e : element.getSchedule()) 34999 composeTiming(null, e); 35000 closeArray(); 35001 }; 35002 if (element.hasNutrient()) { 35003 openArray("nutrient"); 35004 for (NutritionOrder.NutritionOrderOralDietNutrientComponent e : element.getNutrient()) 35005 composeNutritionOrderNutritionOrderOralDietNutrientComponent(null, e); 35006 closeArray(); 35007 }; 35008 if (element.hasTexture()) { 35009 openArray("texture"); 35010 for (NutritionOrder.NutritionOrderOralDietTextureComponent e : element.getTexture()) 35011 composeNutritionOrderNutritionOrderOralDietTextureComponent(null, e); 35012 closeArray(); 35013 }; 35014 if (element.hasFluidConsistencyType()) { 35015 openArray("fluidConsistencyType"); 35016 for (CodeableConcept e : element.getFluidConsistencyType()) 35017 composeCodeableConcept(null, e); 35018 closeArray(); 35019 }; 35020 if (element.hasInstructionElement()) { 35021 composeStringCore("instruction", element.getInstructionElement(), false); 35022 composeStringExtras("instruction", element.getInstructionElement(), false); 35023 } 35024 } 35025 35026 protected void composeNutritionOrderNutritionOrderOralDietNutrientComponent(String name, NutritionOrder.NutritionOrderOralDietNutrientComponent element) throws IOException { 35027 if (element != null) { 35028 open(name); 35029 composeNutritionOrderNutritionOrderOralDietNutrientComponentInner(element); 35030 close(); 35031 } 35032 } 35033 35034 protected void composeNutritionOrderNutritionOrderOralDietNutrientComponentInner(NutritionOrder.NutritionOrderOralDietNutrientComponent element) throws IOException { 35035 composeBackbone(element); 35036 if (element.hasModifier()) { 35037 composeCodeableConcept("modifier", element.getModifier()); 35038 } 35039 if (element.hasAmount()) { 35040 composeSimpleQuantity("amount", element.getAmount()); 35041 } 35042 } 35043 35044 protected void composeNutritionOrderNutritionOrderOralDietTextureComponent(String name, NutritionOrder.NutritionOrderOralDietTextureComponent element) throws IOException { 35045 if (element != null) { 35046 open(name); 35047 composeNutritionOrderNutritionOrderOralDietTextureComponentInner(element); 35048 close(); 35049 } 35050 } 35051 35052 protected void composeNutritionOrderNutritionOrderOralDietTextureComponentInner(NutritionOrder.NutritionOrderOralDietTextureComponent element) throws IOException { 35053 composeBackbone(element); 35054 if (element.hasModifier()) { 35055 composeCodeableConcept("modifier", element.getModifier()); 35056 } 35057 if (element.hasFoodType()) { 35058 composeCodeableConcept("foodType", element.getFoodType()); 35059 } 35060 } 35061 35062 protected void composeNutritionOrderNutritionOrderSupplementComponent(String name, NutritionOrder.NutritionOrderSupplementComponent element) throws IOException { 35063 if (element != null) { 35064 open(name); 35065 composeNutritionOrderNutritionOrderSupplementComponentInner(element); 35066 close(); 35067 } 35068 } 35069 35070 protected void composeNutritionOrderNutritionOrderSupplementComponentInner(NutritionOrder.NutritionOrderSupplementComponent element) throws IOException { 35071 composeBackbone(element); 35072 if (element.hasType()) { 35073 composeCodeableConcept("type", element.getType()); 35074 } 35075 if (element.hasProductNameElement()) { 35076 composeStringCore("productName", element.getProductNameElement(), false); 35077 composeStringExtras("productName", element.getProductNameElement(), false); 35078 } 35079 if (element.hasSchedule()) { 35080 openArray("schedule"); 35081 for (Timing e : element.getSchedule()) 35082 composeTiming(null, e); 35083 closeArray(); 35084 }; 35085 if (element.hasQuantity()) { 35086 composeSimpleQuantity("quantity", element.getQuantity()); 35087 } 35088 if (element.hasInstructionElement()) { 35089 composeStringCore("instruction", element.getInstructionElement(), false); 35090 composeStringExtras("instruction", element.getInstructionElement(), false); 35091 } 35092 } 35093 35094 protected void composeNutritionOrderNutritionOrderEnteralFormulaComponent(String name, NutritionOrder.NutritionOrderEnteralFormulaComponent element) throws IOException { 35095 if (element != null) { 35096 open(name); 35097 composeNutritionOrderNutritionOrderEnteralFormulaComponentInner(element); 35098 close(); 35099 } 35100 } 35101 35102 protected void composeNutritionOrderNutritionOrderEnteralFormulaComponentInner(NutritionOrder.NutritionOrderEnteralFormulaComponent element) throws IOException { 35103 composeBackbone(element); 35104 if (element.hasBaseFormulaType()) { 35105 composeCodeableConcept("baseFormulaType", element.getBaseFormulaType()); 35106 } 35107 if (element.hasBaseFormulaProductNameElement()) { 35108 composeStringCore("baseFormulaProductName", element.getBaseFormulaProductNameElement(), false); 35109 composeStringExtras("baseFormulaProductName", element.getBaseFormulaProductNameElement(), false); 35110 } 35111 if (element.hasAdditiveType()) { 35112 composeCodeableConcept("additiveType", element.getAdditiveType()); 35113 } 35114 if (element.hasAdditiveProductNameElement()) { 35115 composeStringCore("additiveProductName", element.getAdditiveProductNameElement(), false); 35116 composeStringExtras("additiveProductName", element.getAdditiveProductNameElement(), false); 35117 } 35118 if (element.hasCaloricDensity()) { 35119 composeSimpleQuantity("caloricDensity", element.getCaloricDensity()); 35120 } 35121 if (element.hasRouteofAdministration()) { 35122 composeCodeableConcept("routeofAdministration", element.getRouteofAdministration()); 35123 } 35124 if (element.hasAdministration()) { 35125 openArray("administration"); 35126 for (NutritionOrder.NutritionOrderEnteralFormulaAdministrationComponent e : element.getAdministration()) 35127 composeNutritionOrderNutritionOrderEnteralFormulaAdministrationComponent(null, e); 35128 closeArray(); 35129 }; 35130 if (element.hasMaxVolumeToDeliver()) { 35131 composeSimpleQuantity("maxVolumeToDeliver", element.getMaxVolumeToDeliver()); 35132 } 35133 if (element.hasAdministrationInstructionElement()) { 35134 composeStringCore("administrationInstruction", element.getAdministrationInstructionElement(), false); 35135 composeStringExtras("administrationInstruction", element.getAdministrationInstructionElement(), false); 35136 } 35137 } 35138 35139 protected void composeNutritionOrderNutritionOrderEnteralFormulaAdministrationComponent(String name, NutritionOrder.NutritionOrderEnteralFormulaAdministrationComponent element) throws IOException { 35140 if (element != null) { 35141 open(name); 35142 composeNutritionOrderNutritionOrderEnteralFormulaAdministrationComponentInner(element); 35143 close(); 35144 } 35145 } 35146 35147 protected void composeNutritionOrderNutritionOrderEnteralFormulaAdministrationComponentInner(NutritionOrder.NutritionOrderEnteralFormulaAdministrationComponent element) throws IOException { 35148 composeBackbone(element); 35149 if (element.hasSchedule()) { 35150 composeTiming("schedule", element.getSchedule()); 35151 } 35152 if (element.hasQuantity()) { 35153 composeSimpleQuantity("quantity", element.getQuantity()); 35154 } 35155 if (element.hasRate()) { 35156 composeType("rate", element.getRate()); 35157 } 35158 } 35159 35160 protected void composeObservation(String name, Observation element) throws IOException { 35161 if (element != null) { 35162 prop("resourceType", name); 35163 composeObservationInner(element); 35164 } 35165 } 35166 35167 protected void composeObservationInner(Observation element) throws IOException { 35168 composeDomainResourceElements(element); 35169 if (element.hasIdentifier()) { 35170 openArray("identifier"); 35171 for (Identifier e : element.getIdentifier()) 35172 composeIdentifier(null, e); 35173 closeArray(); 35174 }; 35175 if (element.hasBasedOn()) { 35176 openArray("basedOn"); 35177 for (Reference e : element.getBasedOn()) 35178 composeReference(null, e); 35179 closeArray(); 35180 }; 35181 if (element.hasStatusElement()) { 35182 composeEnumerationCore("status", element.getStatusElement(), new Observation.ObservationStatusEnumFactory(), false); 35183 composeEnumerationExtras("status", element.getStatusElement(), new Observation.ObservationStatusEnumFactory(), false); 35184 } 35185 if (element.hasCategory()) { 35186 openArray("category"); 35187 for (CodeableConcept e : element.getCategory()) 35188 composeCodeableConcept(null, e); 35189 closeArray(); 35190 }; 35191 if (element.hasCode()) { 35192 composeCodeableConcept("code", element.getCode()); 35193 } 35194 if (element.hasSubject()) { 35195 composeReference("subject", element.getSubject()); 35196 } 35197 if (element.hasContext()) { 35198 composeReference("context", element.getContext()); 35199 } 35200 if (element.hasEffective()) { 35201 composeType("effective", element.getEffective()); 35202 } 35203 if (element.hasIssuedElement()) { 35204 composeInstantCore("issued", element.getIssuedElement(), false); 35205 composeInstantExtras("issued", element.getIssuedElement(), false); 35206 } 35207 if (element.hasPerformer()) { 35208 openArray("performer"); 35209 for (Reference e : element.getPerformer()) 35210 composeReference(null, e); 35211 closeArray(); 35212 }; 35213 if (element.hasValue()) { 35214 composeType("value", element.getValue()); 35215 } 35216 if (element.hasDataAbsentReason()) { 35217 composeCodeableConcept("dataAbsentReason", element.getDataAbsentReason()); 35218 } 35219 if (element.hasInterpretation()) { 35220 composeCodeableConcept("interpretation", element.getInterpretation()); 35221 } 35222 if (element.hasCommentElement()) { 35223 composeStringCore("comment", element.getCommentElement(), false); 35224 composeStringExtras("comment", element.getCommentElement(), false); 35225 } 35226 if (element.hasBodySite()) { 35227 composeCodeableConcept("bodySite", element.getBodySite()); 35228 } 35229 if (element.hasMethod()) { 35230 composeCodeableConcept("method", element.getMethod()); 35231 } 35232 if (element.hasSpecimen()) { 35233 composeReference("specimen", element.getSpecimen()); 35234 } 35235 if (element.hasDevice()) { 35236 composeReference("device", element.getDevice()); 35237 } 35238 if (element.hasReferenceRange()) { 35239 openArray("referenceRange"); 35240 for (Observation.ObservationReferenceRangeComponent e : element.getReferenceRange()) 35241 composeObservationObservationReferenceRangeComponent(null, e); 35242 closeArray(); 35243 }; 35244 if (element.hasRelated()) { 35245 openArray("related"); 35246 for (Observation.ObservationRelatedComponent e : element.getRelated()) 35247 composeObservationObservationRelatedComponent(null, e); 35248 closeArray(); 35249 }; 35250 if (element.hasComponent()) { 35251 openArray("component"); 35252 for (Observation.ObservationComponentComponent e : element.getComponent()) 35253 composeObservationObservationComponentComponent(null, e); 35254 closeArray(); 35255 }; 35256 } 35257 35258 protected void composeObservationObservationReferenceRangeComponent(String name, Observation.ObservationReferenceRangeComponent element) throws IOException { 35259 if (element != null) { 35260 open(name); 35261 composeObservationObservationReferenceRangeComponentInner(element); 35262 close(); 35263 } 35264 } 35265 35266 protected void composeObservationObservationReferenceRangeComponentInner(Observation.ObservationReferenceRangeComponent element) throws IOException { 35267 composeBackbone(element); 35268 if (element.hasLow()) { 35269 composeSimpleQuantity("low", element.getLow()); 35270 } 35271 if (element.hasHigh()) { 35272 composeSimpleQuantity("high", element.getHigh()); 35273 } 35274 if (element.hasType()) { 35275 composeCodeableConcept("type", element.getType()); 35276 } 35277 if (element.hasAppliesTo()) { 35278 openArray("appliesTo"); 35279 for (CodeableConcept e : element.getAppliesTo()) 35280 composeCodeableConcept(null, e); 35281 closeArray(); 35282 }; 35283 if (element.hasAge()) { 35284 composeRange("age", element.getAge()); 35285 } 35286 if (element.hasTextElement()) { 35287 composeStringCore("text", element.getTextElement(), false); 35288 composeStringExtras("text", element.getTextElement(), false); 35289 } 35290 } 35291 35292 protected void composeObservationObservationRelatedComponent(String name, Observation.ObservationRelatedComponent element) throws IOException { 35293 if (element != null) { 35294 open(name); 35295 composeObservationObservationRelatedComponentInner(element); 35296 close(); 35297 } 35298 } 35299 35300 protected void composeObservationObservationRelatedComponentInner(Observation.ObservationRelatedComponent element) throws IOException { 35301 composeBackbone(element); 35302 if (element.hasTypeElement()) { 35303 composeEnumerationCore("type", element.getTypeElement(), new Observation.ObservationRelationshipTypeEnumFactory(), false); 35304 composeEnumerationExtras("type", element.getTypeElement(), new Observation.ObservationRelationshipTypeEnumFactory(), false); 35305 } 35306 if (element.hasTarget()) { 35307 composeReference("target", element.getTarget()); 35308 } 35309 } 35310 35311 protected void composeObservationObservationComponentComponent(String name, Observation.ObservationComponentComponent element) throws IOException { 35312 if (element != null) { 35313 open(name); 35314 composeObservationObservationComponentComponentInner(element); 35315 close(); 35316 } 35317 } 35318 35319 protected void composeObservationObservationComponentComponentInner(Observation.ObservationComponentComponent element) throws IOException { 35320 composeBackbone(element); 35321 if (element.hasCode()) { 35322 composeCodeableConcept("code", element.getCode()); 35323 } 35324 if (element.hasValue()) { 35325 composeType("value", element.getValue()); 35326 } 35327 if (element.hasDataAbsentReason()) { 35328 composeCodeableConcept("dataAbsentReason", element.getDataAbsentReason()); 35329 } 35330 if (element.hasInterpretation()) { 35331 composeCodeableConcept("interpretation", element.getInterpretation()); 35332 } 35333 if (element.hasReferenceRange()) { 35334 openArray("referenceRange"); 35335 for (Observation.ObservationReferenceRangeComponent e : element.getReferenceRange()) 35336 composeObservationObservationReferenceRangeComponent(null, e); 35337 closeArray(); 35338 }; 35339 } 35340 35341 protected void composeOperationDefinition(String name, OperationDefinition element) throws IOException { 35342 if (element != null) { 35343 prop("resourceType", name); 35344 composeOperationDefinitionInner(element); 35345 } 35346 } 35347 35348 protected void composeOperationDefinitionInner(OperationDefinition element) throws IOException { 35349 composeDomainResourceElements(element); 35350 if (element.hasUrlElement()) { 35351 composeUriCore("url", element.getUrlElement(), false); 35352 composeUriExtras("url", element.getUrlElement(), false); 35353 } 35354 if (element.hasVersionElement()) { 35355 composeStringCore("version", element.getVersionElement(), false); 35356 composeStringExtras("version", element.getVersionElement(), false); 35357 } 35358 if (element.hasNameElement()) { 35359 composeStringCore("name", element.getNameElement(), false); 35360 composeStringExtras("name", element.getNameElement(), false); 35361 } 35362 if (element.hasStatusElement()) { 35363 composeEnumerationCore("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 35364 composeEnumerationExtras("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 35365 } 35366 if (element.hasKindElement()) { 35367 composeEnumerationCore("kind", element.getKindElement(), new OperationDefinition.OperationKindEnumFactory(), false); 35368 composeEnumerationExtras("kind", element.getKindElement(), new OperationDefinition.OperationKindEnumFactory(), false); 35369 } 35370 if (element.hasExperimentalElement()) { 35371 composeBooleanCore("experimental", element.getExperimentalElement(), false); 35372 composeBooleanExtras("experimental", element.getExperimentalElement(), false); 35373 } 35374 if (element.hasDateElement()) { 35375 composeDateTimeCore("date", element.getDateElement(), false); 35376 composeDateTimeExtras("date", element.getDateElement(), false); 35377 } 35378 if (element.hasPublisherElement()) { 35379 composeStringCore("publisher", element.getPublisherElement(), false); 35380 composeStringExtras("publisher", element.getPublisherElement(), false); 35381 } 35382 if (element.hasContact()) { 35383 openArray("contact"); 35384 for (ContactDetail e : element.getContact()) 35385 composeContactDetail(null, e); 35386 closeArray(); 35387 }; 35388 if (element.hasDescriptionElement()) { 35389 composeMarkdownCore("description", element.getDescriptionElement(), false); 35390 composeMarkdownExtras("description", element.getDescriptionElement(), false); 35391 } 35392 if (element.hasUseContext()) { 35393 openArray("useContext"); 35394 for (UsageContext e : element.getUseContext()) 35395 composeUsageContext(null, e); 35396 closeArray(); 35397 }; 35398 if (element.hasJurisdiction()) { 35399 openArray("jurisdiction"); 35400 for (CodeableConcept e : element.getJurisdiction()) 35401 composeCodeableConcept(null, e); 35402 closeArray(); 35403 }; 35404 if (element.hasPurposeElement()) { 35405 composeMarkdownCore("purpose", element.getPurposeElement(), false); 35406 composeMarkdownExtras("purpose", element.getPurposeElement(), false); 35407 } 35408 if (element.hasIdempotentElement()) { 35409 composeBooleanCore("idempotent", element.getIdempotentElement(), false); 35410 composeBooleanExtras("idempotent", element.getIdempotentElement(), false); 35411 } 35412 if (element.hasCodeElement()) { 35413 composeCodeCore("code", element.getCodeElement(), false); 35414 composeCodeExtras("code", element.getCodeElement(), false); 35415 } 35416 if (element.hasCommentElement()) { 35417 composeStringCore("comment", element.getCommentElement(), false); 35418 composeStringExtras("comment", element.getCommentElement(), false); 35419 } 35420 if (element.hasBase()) { 35421 composeReference("base", element.getBase()); 35422 } 35423 if (element.hasResource()) { 35424 openArray("resource"); 35425 for (CodeType e : element.getResource()) 35426 composeCodeCore(null, e, true); 35427 closeArray(); 35428 if (anyHasExtras(element.getResource())) { 35429 openArray("_resource"); 35430 for (CodeType e : element.getResource()) 35431 composeCodeExtras(null, e, true); 35432 closeArray(); 35433 } 35434 }; 35435 if (element.hasSystemElement()) { 35436 composeBooleanCore("system", element.getSystemElement(), false); 35437 composeBooleanExtras("system", element.getSystemElement(), false); 35438 } 35439 if (element.hasTypeElement()) { 35440 composeBooleanCore("type", element.getTypeElement(), false); 35441 composeBooleanExtras("type", element.getTypeElement(), false); 35442 } 35443 if (element.hasInstanceElement()) { 35444 composeBooleanCore("instance", element.getInstanceElement(), false); 35445 composeBooleanExtras("instance", element.getInstanceElement(), false); 35446 } 35447 if (element.hasParameter()) { 35448 openArray("parameter"); 35449 for (OperationDefinition.OperationDefinitionParameterComponent e : element.getParameter()) 35450 composeOperationDefinitionOperationDefinitionParameterComponent(null, e); 35451 closeArray(); 35452 }; 35453 if (element.hasOverload()) { 35454 openArray("overload"); 35455 for (OperationDefinition.OperationDefinitionOverloadComponent e : element.getOverload()) 35456 composeOperationDefinitionOperationDefinitionOverloadComponent(null, e); 35457 closeArray(); 35458 }; 35459 } 35460 35461 protected void composeOperationDefinitionOperationDefinitionParameterComponent(String name, OperationDefinition.OperationDefinitionParameterComponent element) throws IOException { 35462 if (element != null) { 35463 open(name); 35464 composeOperationDefinitionOperationDefinitionParameterComponentInner(element); 35465 close(); 35466 } 35467 } 35468 35469 protected void composeOperationDefinitionOperationDefinitionParameterComponentInner(OperationDefinition.OperationDefinitionParameterComponent element) throws IOException { 35470 composeBackbone(element); 35471 if (element.hasNameElement()) { 35472 composeCodeCore("name", element.getNameElement(), false); 35473 composeCodeExtras("name", element.getNameElement(), false); 35474 } 35475 if (element.hasUseElement()) { 35476 composeEnumerationCore("use", element.getUseElement(), new OperationDefinition.OperationParameterUseEnumFactory(), false); 35477 composeEnumerationExtras("use", element.getUseElement(), new OperationDefinition.OperationParameterUseEnumFactory(), false); 35478 } 35479 if (element.hasMinElement()) { 35480 composeIntegerCore("min", element.getMinElement(), false); 35481 composeIntegerExtras("min", element.getMinElement(), false); 35482 } 35483 if (element.hasMaxElement()) { 35484 composeStringCore("max", element.getMaxElement(), false); 35485 composeStringExtras("max", element.getMaxElement(), false); 35486 } 35487 if (element.hasDocumentationElement()) { 35488 composeStringCore("documentation", element.getDocumentationElement(), false); 35489 composeStringExtras("documentation", element.getDocumentationElement(), false); 35490 } 35491 if (element.hasTypeElement()) { 35492 composeCodeCore("type", element.getTypeElement(), false); 35493 composeCodeExtras("type", element.getTypeElement(), false); 35494 } 35495 if (element.hasSearchTypeElement()) { 35496 composeEnumerationCore("searchType", element.getSearchTypeElement(), new Enumerations.SearchParamTypeEnumFactory(), false); 35497 composeEnumerationExtras("searchType", element.getSearchTypeElement(), new Enumerations.SearchParamTypeEnumFactory(), false); 35498 } 35499 if (element.hasProfile()) { 35500 composeReference("profile", element.getProfile()); 35501 } 35502 if (element.hasBinding()) { 35503 composeOperationDefinitionOperationDefinitionParameterBindingComponent("binding", element.getBinding()); 35504 } 35505 if (element.hasPart()) { 35506 openArray("part"); 35507 for (OperationDefinition.OperationDefinitionParameterComponent e : element.getPart()) 35508 composeOperationDefinitionOperationDefinitionParameterComponent(null, e); 35509 closeArray(); 35510 }; 35511 } 35512 35513 protected void composeOperationDefinitionOperationDefinitionParameterBindingComponent(String name, OperationDefinition.OperationDefinitionParameterBindingComponent element) throws IOException { 35514 if (element != null) { 35515 open(name); 35516 composeOperationDefinitionOperationDefinitionParameterBindingComponentInner(element); 35517 close(); 35518 } 35519 } 35520 35521 protected void composeOperationDefinitionOperationDefinitionParameterBindingComponentInner(OperationDefinition.OperationDefinitionParameterBindingComponent element) throws IOException { 35522 composeBackbone(element); 35523 if (element.hasStrengthElement()) { 35524 composeEnumerationCore("strength", element.getStrengthElement(), new Enumerations.BindingStrengthEnumFactory(), false); 35525 composeEnumerationExtras("strength", element.getStrengthElement(), new Enumerations.BindingStrengthEnumFactory(), false); 35526 } 35527 if (element.hasValueSet()) { 35528 composeType("valueSet", element.getValueSet()); 35529 } 35530 } 35531 35532 protected void composeOperationDefinitionOperationDefinitionOverloadComponent(String name, OperationDefinition.OperationDefinitionOverloadComponent element) throws IOException { 35533 if (element != null) { 35534 open(name); 35535 composeOperationDefinitionOperationDefinitionOverloadComponentInner(element); 35536 close(); 35537 } 35538 } 35539 35540 protected void composeOperationDefinitionOperationDefinitionOverloadComponentInner(OperationDefinition.OperationDefinitionOverloadComponent element) throws IOException { 35541 composeBackbone(element); 35542 if (element.hasParameterName()) { 35543 openArray("parameterName"); 35544 for (StringType e : element.getParameterName()) 35545 composeStringCore(null, e, true); 35546 closeArray(); 35547 if (anyHasExtras(element.getParameterName())) { 35548 openArray("_parameterName"); 35549 for (StringType e : element.getParameterName()) 35550 composeStringExtras(null, e, true); 35551 closeArray(); 35552 } 35553 }; 35554 if (element.hasCommentElement()) { 35555 composeStringCore("comment", element.getCommentElement(), false); 35556 composeStringExtras("comment", element.getCommentElement(), false); 35557 } 35558 } 35559 35560 protected void composeOperationOutcome(String name, OperationOutcome element) throws IOException { 35561 if (element != null) { 35562 prop("resourceType", name); 35563 composeOperationOutcomeInner(element); 35564 } 35565 } 35566 35567 protected void composeOperationOutcomeInner(OperationOutcome element) throws IOException { 35568 composeDomainResourceElements(element); 35569 if (element.hasIssue()) { 35570 openArray("issue"); 35571 for (OperationOutcome.OperationOutcomeIssueComponent e : element.getIssue()) 35572 composeOperationOutcomeOperationOutcomeIssueComponent(null, e); 35573 closeArray(); 35574 }; 35575 } 35576 35577 protected void composeOperationOutcomeOperationOutcomeIssueComponent(String name, OperationOutcome.OperationOutcomeIssueComponent element) throws IOException { 35578 if (element != null) { 35579 open(name); 35580 composeOperationOutcomeOperationOutcomeIssueComponentInner(element); 35581 close(); 35582 } 35583 } 35584 35585 protected void composeOperationOutcomeOperationOutcomeIssueComponentInner(OperationOutcome.OperationOutcomeIssueComponent element) throws IOException { 35586 composeBackbone(element); 35587 if (element.hasSeverityElement()) { 35588 composeEnumerationCore("severity", element.getSeverityElement(), new OperationOutcome.IssueSeverityEnumFactory(), false); 35589 composeEnumerationExtras("severity", element.getSeverityElement(), new OperationOutcome.IssueSeverityEnumFactory(), false); 35590 } 35591 if (element.hasCodeElement()) { 35592 composeEnumerationCore("code", element.getCodeElement(), new OperationOutcome.IssueTypeEnumFactory(), false); 35593 composeEnumerationExtras("code", element.getCodeElement(), new OperationOutcome.IssueTypeEnumFactory(), false); 35594 } 35595 if (element.hasDetails()) { 35596 composeCodeableConcept("details", element.getDetails()); 35597 } 35598 if (element.hasDiagnosticsElement()) { 35599 composeStringCore("diagnostics", element.getDiagnosticsElement(), false); 35600 composeStringExtras("diagnostics", element.getDiagnosticsElement(), false); 35601 } 35602 if (element.hasLocation()) { 35603 openArray("location"); 35604 for (StringType e : element.getLocation()) 35605 composeStringCore(null, e, true); 35606 closeArray(); 35607 if (anyHasExtras(element.getLocation())) { 35608 openArray("_location"); 35609 for (StringType e : element.getLocation()) 35610 composeStringExtras(null, e, true); 35611 closeArray(); 35612 } 35613 }; 35614 if (element.hasExpression()) { 35615 openArray("expression"); 35616 for (StringType e : element.getExpression()) 35617 composeStringCore(null, e, true); 35618 closeArray(); 35619 if (anyHasExtras(element.getExpression())) { 35620 openArray("_expression"); 35621 for (StringType e : element.getExpression()) 35622 composeStringExtras(null, e, true); 35623 closeArray(); 35624 } 35625 }; 35626 } 35627 35628 protected void composeOrganization(String name, Organization element) throws IOException { 35629 if (element != null) { 35630 prop("resourceType", name); 35631 composeOrganizationInner(element); 35632 } 35633 } 35634 35635 protected void composeOrganizationInner(Organization element) throws IOException { 35636 composeDomainResourceElements(element); 35637 if (element.hasIdentifier()) { 35638 openArray("identifier"); 35639 for (Identifier e : element.getIdentifier()) 35640 composeIdentifier(null, e); 35641 closeArray(); 35642 }; 35643 if (element.hasActiveElement()) { 35644 composeBooleanCore("active", element.getActiveElement(), false); 35645 composeBooleanExtras("active", element.getActiveElement(), false); 35646 } 35647 if (element.hasType()) { 35648 openArray("type"); 35649 for (CodeableConcept e : element.getType()) 35650 composeCodeableConcept(null, e); 35651 closeArray(); 35652 }; 35653 if (element.hasNameElement()) { 35654 composeStringCore("name", element.getNameElement(), false); 35655 composeStringExtras("name", element.getNameElement(), false); 35656 } 35657 if (element.hasAlias()) { 35658 openArray("alias"); 35659 for (StringType e : element.getAlias()) 35660 composeStringCore(null, e, true); 35661 closeArray(); 35662 if (anyHasExtras(element.getAlias())) { 35663 openArray("_alias"); 35664 for (StringType e : element.getAlias()) 35665 composeStringExtras(null, e, true); 35666 closeArray(); 35667 } 35668 }; 35669 if (element.hasTelecom()) { 35670 openArray("telecom"); 35671 for (ContactPoint e : element.getTelecom()) 35672 composeContactPoint(null, e); 35673 closeArray(); 35674 }; 35675 if (element.hasAddress()) { 35676 openArray("address"); 35677 for (Address e : element.getAddress()) 35678 composeAddress(null, e); 35679 closeArray(); 35680 }; 35681 if (element.hasPartOf()) { 35682 composeReference("partOf", element.getPartOf()); 35683 } 35684 if (element.hasContact()) { 35685 openArray("contact"); 35686 for (Organization.OrganizationContactComponent e : element.getContact()) 35687 composeOrganizationOrganizationContactComponent(null, e); 35688 closeArray(); 35689 }; 35690 if (element.hasEndpoint()) { 35691 openArray("endpoint"); 35692 for (Reference e : element.getEndpoint()) 35693 composeReference(null, e); 35694 closeArray(); 35695 }; 35696 } 35697 35698 protected void composeOrganizationOrganizationContactComponent(String name, Organization.OrganizationContactComponent element) throws IOException { 35699 if (element != null) { 35700 open(name); 35701 composeOrganizationOrganizationContactComponentInner(element); 35702 close(); 35703 } 35704 } 35705 35706 protected void composeOrganizationOrganizationContactComponentInner(Organization.OrganizationContactComponent element) throws IOException { 35707 composeBackbone(element); 35708 if (element.hasPurpose()) { 35709 composeCodeableConcept("purpose", element.getPurpose()); 35710 } 35711 if (element.hasName()) { 35712 composeHumanName("name", element.getName()); 35713 } 35714 if (element.hasTelecom()) { 35715 openArray("telecom"); 35716 for (ContactPoint e : element.getTelecom()) 35717 composeContactPoint(null, e); 35718 closeArray(); 35719 }; 35720 if (element.hasAddress()) { 35721 composeAddress("address", element.getAddress()); 35722 } 35723 } 35724 35725 protected void composePatient(String name, Patient element) throws IOException { 35726 if (element != null) { 35727 prop("resourceType", name); 35728 composePatientInner(element); 35729 } 35730 } 35731 35732 protected void composePatientInner(Patient element) throws IOException { 35733 composeDomainResourceElements(element); 35734 if (element.hasIdentifier()) { 35735 openArray("identifier"); 35736 for (Identifier e : element.getIdentifier()) 35737 composeIdentifier(null, e); 35738 closeArray(); 35739 }; 35740 if (element.hasActiveElement()) { 35741 composeBooleanCore("active", element.getActiveElement(), false); 35742 composeBooleanExtras("active", element.getActiveElement(), false); 35743 } 35744 if (element.hasName()) { 35745 openArray("name"); 35746 for (HumanName e : element.getName()) 35747 composeHumanName(null, e); 35748 closeArray(); 35749 }; 35750 if (element.hasTelecom()) { 35751 openArray("telecom"); 35752 for (ContactPoint e : element.getTelecom()) 35753 composeContactPoint(null, e); 35754 closeArray(); 35755 }; 35756 if (element.hasGenderElement()) { 35757 composeEnumerationCore("gender", element.getGenderElement(), new Enumerations.AdministrativeGenderEnumFactory(), false); 35758 composeEnumerationExtras("gender", element.getGenderElement(), new Enumerations.AdministrativeGenderEnumFactory(), false); 35759 } 35760 if (element.hasBirthDateElement()) { 35761 composeDateCore("birthDate", element.getBirthDateElement(), false); 35762 composeDateExtras("birthDate", element.getBirthDateElement(), false); 35763 } 35764 if (element.hasDeceased()) { 35765 composeType("deceased", element.getDeceased()); 35766 } 35767 if (element.hasAddress()) { 35768 openArray("address"); 35769 for (Address e : element.getAddress()) 35770 composeAddress(null, e); 35771 closeArray(); 35772 }; 35773 if (element.hasMaritalStatus()) { 35774 composeCodeableConcept("maritalStatus", element.getMaritalStatus()); 35775 } 35776 if (element.hasMultipleBirth()) { 35777 composeType("multipleBirth", element.getMultipleBirth()); 35778 } 35779 if (element.hasPhoto()) { 35780 openArray("photo"); 35781 for (Attachment e : element.getPhoto()) 35782 composeAttachment(null, e); 35783 closeArray(); 35784 }; 35785 if (element.hasContact()) { 35786 openArray("contact"); 35787 for (Patient.ContactComponent e : element.getContact()) 35788 composePatientContactComponent(null, e); 35789 closeArray(); 35790 }; 35791 if (element.hasAnimal()) { 35792 composePatientAnimalComponent("animal", element.getAnimal()); 35793 } 35794 if (element.hasCommunication()) { 35795 openArray("communication"); 35796 for (Patient.PatientCommunicationComponent e : element.getCommunication()) 35797 composePatientPatientCommunicationComponent(null, e); 35798 closeArray(); 35799 }; 35800 if (element.hasGeneralPractitioner()) { 35801 openArray("generalPractitioner"); 35802 for (Reference e : element.getGeneralPractitioner()) 35803 composeReference(null, e); 35804 closeArray(); 35805 }; 35806 if (element.hasManagingOrganization()) { 35807 composeReference("managingOrganization", element.getManagingOrganization()); 35808 } 35809 if (element.hasLink()) { 35810 openArray("link"); 35811 for (Patient.PatientLinkComponent e : element.getLink()) 35812 composePatientPatientLinkComponent(null, e); 35813 closeArray(); 35814 }; 35815 } 35816 35817 protected void composePatientContactComponent(String name, Patient.ContactComponent element) throws IOException { 35818 if (element != null) { 35819 open(name); 35820 composePatientContactComponentInner(element); 35821 close(); 35822 } 35823 } 35824 35825 protected void composePatientContactComponentInner(Patient.ContactComponent element) throws IOException { 35826 composeBackbone(element); 35827 if (element.hasRelationship()) { 35828 openArray("relationship"); 35829 for (CodeableConcept e : element.getRelationship()) 35830 composeCodeableConcept(null, e); 35831 closeArray(); 35832 }; 35833 if (element.hasName()) { 35834 composeHumanName("name", element.getName()); 35835 } 35836 if (element.hasTelecom()) { 35837 openArray("telecom"); 35838 for (ContactPoint e : element.getTelecom()) 35839 composeContactPoint(null, e); 35840 closeArray(); 35841 }; 35842 if (element.hasAddress()) { 35843 composeAddress("address", element.getAddress()); 35844 } 35845 if (element.hasGenderElement()) { 35846 composeEnumerationCore("gender", element.getGenderElement(), new Enumerations.AdministrativeGenderEnumFactory(), false); 35847 composeEnumerationExtras("gender", element.getGenderElement(), new Enumerations.AdministrativeGenderEnumFactory(), false); 35848 } 35849 if (element.hasOrganization()) { 35850 composeReference("organization", element.getOrganization()); 35851 } 35852 if (element.hasPeriod()) { 35853 composePeriod("period", element.getPeriod()); 35854 } 35855 } 35856 35857 protected void composePatientAnimalComponent(String name, Patient.AnimalComponent element) throws IOException { 35858 if (element != null) { 35859 open(name); 35860 composePatientAnimalComponentInner(element); 35861 close(); 35862 } 35863 } 35864 35865 protected void composePatientAnimalComponentInner(Patient.AnimalComponent element) throws IOException { 35866 composeBackbone(element); 35867 if (element.hasSpecies()) { 35868 composeCodeableConcept("species", element.getSpecies()); 35869 } 35870 if (element.hasBreed()) { 35871 composeCodeableConcept("breed", element.getBreed()); 35872 } 35873 if (element.hasGenderStatus()) { 35874 composeCodeableConcept("genderStatus", element.getGenderStatus()); 35875 } 35876 } 35877 35878 protected void composePatientPatientCommunicationComponent(String name, Patient.PatientCommunicationComponent element) throws IOException { 35879 if (element != null) { 35880 open(name); 35881 composePatientPatientCommunicationComponentInner(element); 35882 close(); 35883 } 35884 } 35885 35886 protected void composePatientPatientCommunicationComponentInner(Patient.PatientCommunicationComponent element) throws IOException { 35887 composeBackbone(element); 35888 if (element.hasLanguage()) { 35889 composeCodeableConcept("language", element.getLanguage()); 35890 } 35891 if (element.hasPreferredElement()) { 35892 composeBooleanCore("preferred", element.getPreferredElement(), false); 35893 composeBooleanExtras("preferred", element.getPreferredElement(), false); 35894 } 35895 } 35896 35897 protected void composePatientPatientLinkComponent(String name, Patient.PatientLinkComponent element) throws IOException { 35898 if (element != null) { 35899 open(name); 35900 composePatientPatientLinkComponentInner(element); 35901 close(); 35902 } 35903 } 35904 35905 protected void composePatientPatientLinkComponentInner(Patient.PatientLinkComponent element) throws IOException { 35906 composeBackbone(element); 35907 if (element.hasOther()) { 35908 composeReference("other", element.getOther()); 35909 } 35910 if (element.hasTypeElement()) { 35911 composeEnumerationCore("type", element.getTypeElement(), new Patient.LinkTypeEnumFactory(), false); 35912 composeEnumerationExtras("type", element.getTypeElement(), new Patient.LinkTypeEnumFactory(), false); 35913 } 35914 } 35915 35916 protected void composePaymentNotice(String name, PaymentNotice element) throws IOException { 35917 if (element != null) { 35918 prop("resourceType", name); 35919 composePaymentNoticeInner(element); 35920 } 35921 } 35922 35923 protected void composePaymentNoticeInner(PaymentNotice element) throws IOException { 35924 composeDomainResourceElements(element); 35925 if (element.hasIdentifier()) { 35926 openArray("identifier"); 35927 for (Identifier e : element.getIdentifier()) 35928 composeIdentifier(null, e); 35929 closeArray(); 35930 }; 35931 if (element.hasStatusElement()) { 35932 composeEnumerationCore("status", element.getStatusElement(), new PaymentNotice.PaymentNoticeStatusEnumFactory(), false); 35933 composeEnumerationExtras("status", element.getStatusElement(), new PaymentNotice.PaymentNoticeStatusEnumFactory(), false); 35934 } 35935 if (element.hasRequest()) { 35936 composeReference("request", element.getRequest()); 35937 } 35938 if (element.hasResponse()) { 35939 composeReference("response", element.getResponse()); 35940 } 35941 if (element.hasStatusDateElement()) { 35942 composeDateCore("statusDate", element.getStatusDateElement(), false); 35943 composeDateExtras("statusDate", element.getStatusDateElement(), false); 35944 } 35945 if (element.hasCreatedElement()) { 35946 composeDateTimeCore("created", element.getCreatedElement(), false); 35947 composeDateTimeExtras("created", element.getCreatedElement(), false); 35948 } 35949 if (element.hasTarget()) { 35950 composeReference("target", element.getTarget()); 35951 } 35952 if (element.hasProvider()) { 35953 composeReference("provider", element.getProvider()); 35954 } 35955 if (element.hasOrganization()) { 35956 composeReference("organization", element.getOrganization()); 35957 } 35958 if (element.hasPaymentStatus()) { 35959 composeCodeableConcept("paymentStatus", element.getPaymentStatus()); 35960 } 35961 } 35962 35963 protected void composePaymentReconciliation(String name, PaymentReconciliation element) throws IOException { 35964 if (element != null) { 35965 prop("resourceType", name); 35966 composePaymentReconciliationInner(element); 35967 } 35968 } 35969 35970 protected void composePaymentReconciliationInner(PaymentReconciliation element) throws IOException { 35971 composeDomainResourceElements(element); 35972 if (element.hasIdentifier()) { 35973 openArray("identifier"); 35974 for (Identifier e : element.getIdentifier()) 35975 composeIdentifier(null, e); 35976 closeArray(); 35977 }; 35978 if (element.hasStatusElement()) { 35979 composeEnumerationCore("status", element.getStatusElement(), new PaymentReconciliation.PaymentReconciliationStatusEnumFactory(), false); 35980 composeEnumerationExtras("status", element.getStatusElement(), new PaymentReconciliation.PaymentReconciliationStatusEnumFactory(), false); 35981 } 35982 if (element.hasPeriod()) { 35983 composePeriod("period", element.getPeriod()); 35984 } 35985 if (element.hasCreatedElement()) { 35986 composeDateTimeCore("created", element.getCreatedElement(), false); 35987 composeDateTimeExtras("created", element.getCreatedElement(), false); 35988 } 35989 if (element.hasOrganization()) { 35990 composeReference("organization", element.getOrganization()); 35991 } 35992 if (element.hasRequest()) { 35993 composeReference("request", element.getRequest()); 35994 } 35995 if (element.hasOutcome()) { 35996 composeCodeableConcept("outcome", element.getOutcome()); 35997 } 35998 if (element.hasDispositionElement()) { 35999 composeStringCore("disposition", element.getDispositionElement(), false); 36000 composeStringExtras("disposition", element.getDispositionElement(), false); 36001 } 36002 if (element.hasRequestProvider()) { 36003 composeReference("requestProvider", element.getRequestProvider()); 36004 } 36005 if (element.hasRequestOrganization()) { 36006 composeReference("requestOrganization", element.getRequestOrganization()); 36007 } 36008 if (element.hasDetail()) { 36009 openArray("detail"); 36010 for (PaymentReconciliation.DetailsComponent e : element.getDetail()) 36011 composePaymentReconciliationDetailsComponent(null, e); 36012 closeArray(); 36013 }; 36014 if (element.hasForm()) { 36015 composeCodeableConcept("form", element.getForm()); 36016 } 36017 if (element.hasTotal()) { 36018 composeMoney("total", element.getTotal()); 36019 } 36020 if (element.hasProcessNote()) { 36021 openArray("processNote"); 36022 for (PaymentReconciliation.NotesComponent e : element.getProcessNote()) 36023 composePaymentReconciliationNotesComponent(null, e); 36024 closeArray(); 36025 }; 36026 } 36027 36028 protected void composePaymentReconciliationDetailsComponent(String name, PaymentReconciliation.DetailsComponent element) throws IOException { 36029 if (element != null) { 36030 open(name); 36031 composePaymentReconciliationDetailsComponentInner(element); 36032 close(); 36033 } 36034 } 36035 36036 protected void composePaymentReconciliationDetailsComponentInner(PaymentReconciliation.DetailsComponent element) throws IOException { 36037 composeBackbone(element); 36038 if (element.hasType()) { 36039 composeCodeableConcept("type", element.getType()); 36040 } 36041 if (element.hasRequest()) { 36042 composeReference("request", element.getRequest()); 36043 } 36044 if (element.hasResponse()) { 36045 composeReference("response", element.getResponse()); 36046 } 36047 if (element.hasSubmitter()) { 36048 composeReference("submitter", element.getSubmitter()); 36049 } 36050 if (element.hasPayee()) { 36051 composeReference("payee", element.getPayee()); 36052 } 36053 if (element.hasDateElement()) { 36054 composeDateCore("date", element.getDateElement(), false); 36055 composeDateExtras("date", element.getDateElement(), false); 36056 } 36057 if (element.hasAmount()) { 36058 composeMoney("amount", element.getAmount()); 36059 } 36060 } 36061 36062 protected void composePaymentReconciliationNotesComponent(String name, PaymentReconciliation.NotesComponent element) throws IOException { 36063 if (element != null) { 36064 open(name); 36065 composePaymentReconciliationNotesComponentInner(element); 36066 close(); 36067 } 36068 } 36069 36070 protected void composePaymentReconciliationNotesComponentInner(PaymentReconciliation.NotesComponent element) throws IOException { 36071 composeBackbone(element); 36072 if (element.hasType()) { 36073 composeCodeableConcept("type", element.getType()); 36074 } 36075 if (element.hasTextElement()) { 36076 composeStringCore("text", element.getTextElement(), false); 36077 composeStringExtras("text", element.getTextElement(), false); 36078 } 36079 } 36080 36081 protected void composePerson(String name, Person element) throws IOException { 36082 if (element != null) { 36083 prop("resourceType", name); 36084 composePersonInner(element); 36085 } 36086 } 36087 36088 protected void composePersonInner(Person element) throws IOException { 36089 composeDomainResourceElements(element); 36090 if (element.hasIdentifier()) { 36091 openArray("identifier"); 36092 for (Identifier e : element.getIdentifier()) 36093 composeIdentifier(null, e); 36094 closeArray(); 36095 }; 36096 if (element.hasName()) { 36097 openArray("name"); 36098 for (HumanName e : element.getName()) 36099 composeHumanName(null, e); 36100 closeArray(); 36101 }; 36102 if (element.hasTelecom()) { 36103 openArray("telecom"); 36104 for (ContactPoint e : element.getTelecom()) 36105 composeContactPoint(null, e); 36106 closeArray(); 36107 }; 36108 if (element.hasGenderElement()) { 36109 composeEnumerationCore("gender", element.getGenderElement(), new Enumerations.AdministrativeGenderEnumFactory(), false); 36110 composeEnumerationExtras("gender", element.getGenderElement(), new Enumerations.AdministrativeGenderEnumFactory(), false); 36111 } 36112 if (element.hasBirthDateElement()) { 36113 composeDateCore("birthDate", element.getBirthDateElement(), false); 36114 composeDateExtras("birthDate", element.getBirthDateElement(), false); 36115 } 36116 if (element.hasAddress()) { 36117 openArray("address"); 36118 for (Address e : element.getAddress()) 36119 composeAddress(null, e); 36120 closeArray(); 36121 }; 36122 if (element.hasPhoto()) { 36123 composeAttachment("photo", element.getPhoto()); 36124 } 36125 if (element.hasManagingOrganization()) { 36126 composeReference("managingOrganization", element.getManagingOrganization()); 36127 } 36128 if (element.hasActiveElement()) { 36129 composeBooleanCore("active", element.getActiveElement(), false); 36130 composeBooleanExtras("active", element.getActiveElement(), false); 36131 } 36132 if (element.hasLink()) { 36133 openArray("link"); 36134 for (Person.PersonLinkComponent e : element.getLink()) 36135 composePersonPersonLinkComponent(null, e); 36136 closeArray(); 36137 }; 36138 } 36139 36140 protected void composePersonPersonLinkComponent(String name, Person.PersonLinkComponent element) throws IOException { 36141 if (element != null) { 36142 open(name); 36143 composePersonPersonLinkComponentInner(element); 36144 close(); 36145 } 36146 } 36147 36148 protected void composePersonPersonLinkComponentInner(Person.PersonLinkComponent element) throws IOException { 36149 composeBackbone(element); 36150 if (element.hasTarget()) { 36151 composeReference("target", element.getTarget()); 36152 } 36153 if (element.hasAssuranceElement()) { 36154 composeEnumerationCore("assurance", element.getAssuranceElement(), new Person.IdentityAssuranceLevelEnumFactory(), false); 36155 composeEnumerationExtras("assurance", element.getAssuranceElement(), new Person.IdentityAssuranceLevelEnumFactory(), false); 36156 } 36157 } 36158 36159 protected void composePlanDefinition(String name, PlanDefinition element) throws IOException { 36160 if (element != null) { 36161 prop("resourceType", name); 36162 composePlanDefinitionInner(element); 36163 } 36164 } 36165 36166 protected void composePlanDefinitionInner(PlanDefinition element) throws IOException { 36167 composeDomainResourceElements(element); 36168 if (element.hasUrlElement()) { 36169 composeUriCore("url", element.getUrlElement(), false); 36170 composeUriExtras("url", element.getUrlElement(), false); 36171 } 36172 if (element.hasIdentifier()) { 36173 openArray("identifier"); 36174 for (Identifier e : element.getIdentifier()) 36175 composeIdentifier(null, e); 36176 closeArray(); 36177 }; 36178 if (element.hasVersionElement()) { 36179 composeStringCore("version", element.getVersionElement(), false); 36180 composeStringExtras("version", element.getVersionElement(), false); 36181 } 36182 if (element.hasNameElement()) { 36183 composeStringCore("name", element.getNameElement(), false); 36184 composeStringExtras("name", element.getNameElement(), false); 36185 } 36186 if (element.hasTitleElement()) { 36187 composeStringCore("title", element.getTitleElement(), false); 36188 composeStringExtras("title", element.getTitleElement(), false); 36189 } 36190 if (element.hasType()) { 36191 composeCodeableConcept("type", element.getType()); 36192 } 36193 if (element.hasStatusElement()) { 36194 composeEnumerationCore("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 36195 composeEnumerationExtras("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 36196 } 36197 if (element.hasExperimentalElement()) { 36198 composeBooleanCore("experimental", element.getExperimentalElement(), false); 36199 composeBooleanExtras("experimental", element.getExperimentalElement(), false); 36200 } 36201 if (element.hasDateElement()) { 36202 composeDateTimeCore("date", element.getDateElement(), false); 36203 composeDateTimeExtras("date", element.getDateElement(), false); 36204 } 36205 if (element.hasPublisherElement()) { 36206 composeStringCore("publisher", element.getPublisherElement(), false); 36207 composeStringExtras("publisher", element.getPublisherElement(), false); 36208 } 36209 if (element.hasDescriptionElement()) { 36210 composeMarkdownCore("description", element.getDescriptionElement(), false); 36211 composeMarkdownExtras("description", element.getDescriptionElement(), false); 36212 } 36213 if (element.hasPurposeElement()) { 36214 composeMarkdownCore("purpose", element.getPurposeElement(), false); 36215 composeMarkdownExtras("purpose", element.getPurposeElement(), false); 36216 } 36217 if (element.hasUsageElement()) { 36218 composeStringCore("usage", element.getUsageElement(), false); 36219 composeStringExtras("usage", element.getUsageElement(), false); 36220 } 36221 if (element.hasApprovalDateElement()) { 36222 composeDateCore("approvalDate", element.getApprovalDateElement(), false); 36223 composeDateExtras("approvalDate", element.getApprovalDateElement(), false); 36224 } 36225 if (element.hasLastReviewDateElement()) { 36226 composeDateCore("lastReviewDate", element.getLastReviewDateElement(), false); 36227 composeDateExtras("lastReviewDate", element.getLastReviewDateElement(), false); 36228 } 36229 if (element.hasEffectivePeriod()) { 36230 composePeriod("effectivePeriod", element.getEffectivePeriod()); 36231 } 36232 if (element.hasUseContext()) { 36233 openArray("useContext"); 36234 for (UsageContext e : element.getUseContext()) 36235 composeUsageContext(null, e); 36236 closeArray(); 36237 }; 36238 if (element.hasJurisdiction()) { 36239 openArray("jurisdiction"); 36240 for (CodeableConcept e : element.getJurisdiction()) 36241 composeCodeableConcept(null, e); 36242 closeArray(); 36243 }; 36244 if (element.hasTopic()) { 36245 openArray("topic"); 36246 for (CodeableConcept e : element.getTopic()) 36247 composeCodeableConcept(null, e); 36248 closeArray(); 36249 }; 36250 if (element.hasContributor()) { 36251 openArray("contributor"); 36252 for (Contributor e : element.getContributor()) 36253 composeContributor(null, e); 36254 closeArray(); 36255 }; 36256 if (element.hasContact()) { 36257 openArray("contact"); 36258 for (ContactDetail e : element.getContact()) 36259 composeContactDetail(null, e); 36260 closeArray(); 36261 }; 36262 if (element.hasCopyrightElement()) { 36263 composeMarkdownCore("copyright", element.getCopyrightElement(), false); 36264 composeMarkdownExtras("copyright", element.getCopyrightElement(), false); 36265 } 36266 if (element.hasRelatedArtifact()) { 36267 openArray("relatedArtifact"); 36268 for (RelatedArtifact e : element.getRelatedArtifact()) 36269 composeRelatedArtifact(null, e); 36270 closeArray(); 36271 }; 36272 if (element.hasLibrary()) { 36273 openArray("library"); 36274 for (Reference e : element.getLibrary()) 36275 composeReference(null, e); 36276 closeArray(); 36277 }; 36278 if (element.hasGoal()) { 36279 openArray("goal"); 36280 for (PlanDefinition.PlanDefinitionGoalComponent e : element.getGoal()) 36281 composePlanDefinitionPlanDefinitionGoalComponent(null, e); 36282 closeArray(); 36283 }; 36284 if (element.hasAction()) { 36285 openArray("action"); 36286 for (PlanDefinition.PlanDefinitionActionComponent e : element.getAction()) 36287 composePlanDefinitionPlanDefinitionActionComponent(null, e); 36288 closeArray(); 36289 }; 36290 } 36291 36292 protected void composePlanDefinitionPlanDefinitionGoalComponent(String name, PlanDefinition.PlanDefinitionGoalComponent element) throws IOException { 36293 if (element != null) { 36294 open(name); 36295 composePlanDefinitionPlanDefinitionGoalComponentInner(element); 36296 close(); 36297 } 36298 } 36299 36300 protected void composePlanDefinitionPlanDefinitionGoalComponentInner(PlanDefinition.PlanDefinitionGoalComponent element) throws IOException { 36301 composeBackbone(element); 36302 if (element.hasCategory()) { 36303 composeCodeableConcept("category", element.getCategory()); 36304 } 36305 if (element.hasDescription()) { 36306 composeCodeableConcept("description", element.getDescription()); 36307 } 36308 if (element.hasPriority()) { 36309 composeCodeableConcept("priority", element.getPriority()); 36310 } 36311 if (element.hasStart()) { 36312 composeCodeableConcept("start", element.getStart()); 36313 } 36314 if (element.hasAddresses()) { 36315 openArray("addresses"); 36316 for (CodeableConcept e : element.getAddresses()) 36317 composeCodeableConcept(null, e); 36318 closeArray(); 36319 }; 36320 if (element.hasDocumentation()) { 36321 openArray("documentation"); 36322 for (RelatedArtifact e : element.getDocumentation()) 36323 composeRelatedArtifact(null, e); 36324 closeArray(); 36325 }; 36326 if (element.hasTarget()) { 36327 openArray("target"); 36328 for (PlanDefinition.PlanDefinitionGoalTargetComponent e : element.getTarget()) 36329 composePlanDefinitionPlanDefinitionGoalTargetComponent(null, e); 36330 closeArray(); 36331 }; 36332 } 36333 36334 protected void composePlanDefinitionPlanDefinitionGoalTargetComponent(String name, PlanDefinition.PlanDefinitionGoalTargetComponent element) throws IOException { 36335 if (element != null) { 36336 open(name); 36337 composePlanDefinitionPlanDefinitionGoalTargetComponentInner(element); 36338 close(); 36339 } 36340 } 36341 36342 protected void composePlanDefinitionPlanDefinitionGoalTargetComponentInner(PlanDefinition.PlanDefinitionGoalTargetComponent element) throws IOException { 36343 composeBackbone(element); 36344 if (element.hasMeasure()) { 36345 composeCodeableConcept("measure", element.getMeasure()); 36346 } 36347 if (element.hasDetail()) { 36348 composeType("detail", element.getDetail()); 36349 } 36350 if (element.hasDue()) { 36351 composeDuration("due", element.getDue()); 36352 } 36353 } 36354 36355 protected void composePlanDefinitionPlanDefinitionActionComponent(String name, PlanDefinition.PlanDefinitionActionComponent element) throws IOException { 36356 if (element != null) { 36357 open(name); 36358 composePlanDefinitionPlanDefinitionActionComponentInner(element); 36359 close(); 36360 } 36361 } 36362 36363 protected void composePlanDefinitionPlanDefinitionActionComponentInner(PlanDefinition.PlanDefinitionActionComponent element) throws IOException { 36364 composeBackbone(element); 36365 if (element.hasLabelElement()) { 36366 composeStringCore("label", element.getLabelElement(), false); 36367 composeStringExtras("label", element.getLabelElement(), false); 36368 } 36369 if (element.hasTitleElement()) { 36370 composeStringCore("title", element.getTitleElement(), false); 36371 composeStringExtras("title", element.getTitleElement(), false); 36372 } 36373 if (element.hasDescriptionElement()) { 36374 composeStringCore("description", element.getDescriptionElement(), false); 36375 composeStringExtras("description", element.getDescriptionElement(), false); 36376 } 36377 if (element.hasTextEquivalentElement()) { 36378 composeStringCore("textEquivalent", element.getTextEquivalentElement(), false); 36379 composeStringExtras("textEquivalent", element.getTextEquivalentElement(), false); 36380 } 36381 if (element.hasCode()) { 36382 openArray("code"); 36383 for (CodeableConcept e : element.getCode()) 36384 composeCodeableConcept(null, e); 36385 closeArray(); 36386 }; 36387 if (element.hasReason()) { 36388 openArray("reason"); 36389 for (CodeableConcept e : element.getReason()) 36390 composeCodeableConcept(null, e); 36391 closeArray(); 36392 }; 36393 if (element.hasDocumentation()) { 36394 openArray("documentation"); 36395 for (RelatedArtifact e : element.getDocumentation()) 36396 composeRelatedArtifact(null, e); 36397 closeArray(); 36398 }; 36399 if (element.hasGoalId()) { 36400 openArray("goalId"); 36401 for (IdType e : element.getGoalId()) 36402 composeIdCore(null, e, true); 36403 closeArray(); 36404 if (anyHasExtras(element.getGoalId())) { 36405 openArray("_goalId"); 36406 for (IdType e : element.getGoalId()) 36407 composeIdExtras(null, e, true); 36408 closeArray(); 36409 } 36410 }; 36411 if (element.hasTriggerDefinition()) { 36412 openArray("triggerDefinition"); 36413 for (TriggerDefinition e : element.getTriggerDefinition()) 36414 composeTriggerDefinition(null, e); 36415 closeArray(); 36416 }; 36417 if (element.hasCondition()) { 36418 openArray("condition"); 36419 for (PlanDefinition.PlanDefinitionActionConditionComponent e : element.getCondition()) 36420 composePlanDefinitionPlanDefinitionActionConditionComponent(null, e); 36421 closeArray(); 36422 }; 36423 if (element.hasInput()) { 36424 openArray("input"); 36425 for (DataRequirement e : element.getInput()) 36426 composeDataRequirement(null, e); 36427 closeArray(); 36428 }; 36429 if (element.hasOutput()) { 36430 openArray("output"); 36431 for (DataRequirement e : element.getOutput()) 36432 composeDataRequirement(null, e); 36433 closeArray(); 36434 }; 36435 if (element.hasRelatedAction()) { 36436 openArray("relatedAction"); 36437 for (PlanDefinition.PlanDefinitionActionRelatedActionComponent e : element.getRelatedAction()) 36438 composePlanDefinitionPlanDefinitionActionRelatedActionComponent(null, e); 36439 closeArray(); 36440 }; 36441 if (element.hasTiming()) { 36442 composeType("timing", element.getTiming()); 36443 } 36444 if (element.hasParticipant()) { 36445 openArray("participant"); 36446 for (PlanDefinition.PlanDefinitionActionParticipantComponent e : element.getParticipant()) 36447 composePlanDefinitionPlanDefinitionActionParticipantComponent(null, e); 36448 closeArray(); 36449 }; 36450 if (element.hasType()) { 36451 composeCoding("type", element.getType()); 36452 } 36453 if (element.hasGroupingBehaviorElement()) { 36454 composeEnumerationCore("groupingBehavior", element.getGroupingBehaviorElement(), new PlanDefinition.ActionGroupingBehaviorEnumFactory(), false); 36455 composeEnumerationExtras("groupingBehavior", element.getGroupingBehaviorElement(), new PlanDefinition.ActionGroupingBehaviorEnumFactory(), false); 36456 } 36457 if (element.hasSelectionBehaviorElement()) { 36458 composeEnumerationCore("selectionBehavior", element.getSelectionBehaviorElement(), new PlanDefinition.ActionSelectionBehaviorEnumFactory(), false); 36459 composeEnumerationExtras("selectionBehavior", element.getSelectionBehaviorElement(), new PlanDefinition.ActionSelectionBehaviorEnumFactory(), false); 36460 } 36461 if (element.hasRequiredBehaviorElement()) { 36462 composeEnumerationCore("requiredBehavior", element.getRequiredBehaviorElement(), new PlanDefinition.ActionRequiredBehaviorEnumFactory(), false); 36463 composeEnumerationExtras("requiredBehavior", element.getRequiredBehaviorElement(), new PlanDefinition.ActionRequiredBehaviorEnumFactory(), false); 36464 } 36465 if (element.hasPrecheckBehaviorElement()) { 36466 composeEnumerationCore("precheckBehavior", element.getPrecheckBehaviorElement(), new PlanDefinition.ActionPrecheckBehaviorEnumFactory(), false); 36467 composeEnumerationExtras("precheckBehavior", element.getPrecheckBehaviorElement(), new PlanDefinition.ActionPrecheckBehaviorEnumFactory(), false); 36468 } 36469 if (element.hasCardinalityBehaviorElement()) { 36470 composeEnumerationCore("cardinalityBehavior", element.getCardinalityBehaviorElement(), new PlanDefinition.ActionCardinalityBehaviorEnumFactory(), false); 36471 composeEnumerationExtras("cardinalityBehavior", element.getCardinalityBehaviorElement(), new PlanDefinition.ActionCardinalityBehaviorEnumFactory(), false); 36472 } 36473 if (element.hasDefinition()) { 36474 composeReference("definition", element.getDefinition()); 36475 } 36476 if (element.hasTransform()) { 36477 composeReference("transform", element.getTransform()); 36478 } 36479 if (element.hasDynamicValue()) { 36480 openArray("dynamicValue"); 36481 for (PlanDefinition.PlanDefinitionActionDynamicValueComponent e : element.getDynamicValue()) 36482 composePlanDefinitionPlanDefinitionActionDynamicValueComponent(null, e); 36483 closeArray(); 36484 }; 36485 if (element.hasAction()) { 36486 openArray("action"); 36487 for (PlanDefinition.PlanDefinitionActionComponent e : element.getAction()) 36488 composePlanDefinitionPlanDefinitionActionComponent(null, e); 36489 closeArray(); 36490 }; 36491 } 36492 36493 protected void composePlanDefinitionPlanDefinitionActionConditionComponent(String name, PlanDefinition.PlanDefinitionActionConditionComponent element) throws IOException { 36494 if (element != null) { 36495 open(name); 36496 composePlanDefinitionPlanDefinitionActionConditionComponentInner(element); 36497 close(); 36498 } 36499 } 36500 36501 protected void composePlanDefinitionPlanDefinitionActionConditionComponentInner(PlanDefinition.PlanDefinitionActionConditionComponent element) throws IOException { 36502 composeBackbone(element); 36503 if (element.hasKindElement()) { 36504 composeEnumerationCore("kind", element.getKindElement(), new PlanDefinition.ActionConditionKindEnumFactory(), false); 36505 composeEnumerationExtras("kind", element.getKindElement(), new PlanDefinition.ActionConditionKindEnumFactory(), false); 36506 } 36507 if (element.hasDescriptionElement()) { 36508 composeStringCore("description", element.getDescriptionElement(), false); 36509 composeStringExtras("description", element.getDescriptionElement(), false); 36510 } 36511 if (element.hasLanguageElement()) { 36512 composeStringCore("language", element.getLanguageElement(), false); 36513 composeStringExtras("language", element.getLanguageElement(), false); 36514 } 36515 if (element.hasExpressionElement()) { 36516 composeStringCore("expression", element.getExpressionElement(), false); 36517 composeStringExtras("expression", element.getExpressionElement(), false); 36518 } 36519 } 36520 36521 protected void composePlanDefinitionPlanDefinitionActionRelatedActionComponent(String name, PlanDefinition.PlanDefinitionActionRelatedActionComponent element) throws IOException { 36522 if (element != null) { 36523 open(name); 36524 composePlanDefinitionPlanDefinitionActionRelatedActionComponentInner(element); 36525 close(); 36526 } 36527 } 36528 36529 protected void composePlanDefinitionPlanDefinitionActionRelatedActionComponentInner(PlanDefinition.PlanDefinitionActionRelatedActionComponent element) throws IOException { 36530 composeBackbone(element); 36531 if (element.hasActionIdElement()) { 36532 composeIdCore("actionId", element.getActionIdElement(), false); 36533 composeIdExtras("actionId", element.getActionIdElement(), false); 36534 } 36535 if (element.hasRelationshipElement()) { 36536 composeEnumerationCore("relationship", element.getRelationshipElement(), new PlanDefinition.ActionRelationshipTypeEnumFactory(), false); 36537 composeEnumerationExtras("relationship", element.getRelationshipElement(), new PlanDefinition.ActionRelationshipTypeEnumFactory(), false); 36538 } 36539 if (element.hasOffset()) { 36540 composeType("offset", element.getOffset()); 36541 } 36542 } 36543 36544 protected void composePlanDefinitionPlanDefinitionActionParticipantComponent(String name, PlanDefinition.PlanDefinitionActionParticipantComponent element) throws IOException { 36545 if (element != null) { 36546 open(name); 36547 composePlanDefinitionPlanDefinitionActionParticipantComponentInner(element); 36548 close(); 36549 } 36550 } 36551 36552 protected void composePlanDefinitionPlanDefinitionActionParticipantComponentInner(PlanDefinition.PlanDefinitionActionParticipantComponent element) throws IOException { 36553 composeBackbone(element); 36554 if (element.hasTypeElement()) { 36555 composeEnumerationCore("type", element.getTypeElement(), new PlanDefinition.ActionParticipantTypeEnumFactory(), false); 36556 composeEnumerationExtras("type", element.getTypeElement(), new PlanDefinition.ActionParticipantTypeEnumFactory(), false); 36557 } 36558 if (element.hasRole()) { 36559 composeCodeableConcept("role", element.getRole()); 36560 } 36561 } 36562 36563 protected void composePlanDefinitionPlanDefinitionActionDynamicValueComponent(String name, PlanDefinition.PlanDefinitionActionDynamicValueComponent element) throws IOException { 36564 if (element != null) { 36565 open(name); 36566 composePlanDefinitionPlanDefinitionActionDynamicValueComponentInner(element); 36567 close(); 36568 } 36569 } 36570 36571 protected void composePlanDefinitionPlanDefinitionActionDynamicValueComponentInner(PlanDefinition.PlanDefinitionActionDynamicValueComponent element) throws IOException { 36572 composeBackbone(element); 36573 if (element.hasDescriptionElement()) { 36574 composeStringCore("description", element.getDescriptionElement(), false); 36575 composeStringExtras("description", element.getDescriptionElement(), false); 36576 } 36577 if (element.hasPathElement()) { 36578 composeStringCore("path", element.getPathElement(), false); 36579 composeStringExtras("path", element.getPathElement(), false); 36580 } 36581 if (element.hasLanguageElement()) { 36582 composeStringCore("language", element.getLanguageElement(), false); 36583 composeStringExtras("language", element.getLanguageElement(), false); 36584 } 36585 if (element.hasExpressionElement()) { 36586 composeStringCore("expression", element.getExpressionElement(), false); 36587 composeStringExtras("expression", element.getExpressionElement(), false); 36588 } 36589 } 36590 36591 protected void composePractitioner(String name, Practitioner element) throws IOException { 36592 if (element != null) { 36593 prop("resourceType", name); 36594 composePractitionerInner(element); 36595 } 36596 } 36597 36598 protected void composePractitionerInner(Practitioner element) throws IOException { 36599 composeDomainResourceElements(element); 36600 if (element.hasIdentifier()) { 36601 openArray("identifier"); 36602 for (Identifier e : element.getIdentifier()) 36603 composeIdentifier(null, e); 36604 closeArray(); 36605 }; 36606 if (element.hasActiveElement()) { 36607 composeBooleanCore("active", element.getActiveElement(), false); 36608 composeBooleanExtras("active", element.getActiveElement(), false); 36609 } 36610 if (element.hasName()) { 36611 openArray("name"); 36612 for (HumanName e : element.getName()) 36613 composeHumanName(null, e); 36614 closeArray(); 36615 }; 36616 if (element.hasTelecom()) { 36617 openArray("telecom"); 36618 for (ContactPoint e : element.getTelecom()) 36619 composeContactPoint(null, e); 36620 closeArray(); 36621 }; 36622 if (element.hasAddress()) { 36623 openArray("address"); 36624 for (Address e : element.getAddress()) 36625 composeAddress(null, e); 36626 closeArray(); 36627 }; 36628 if (element.hasGenderElement()) { 36629 composeEnumerationCore("gender", element.getGenderElement(), new Enumerations.AdministrativeGenderEnumFactory(), false); 36630 composeEnumerationExtras("gender", element.getGenderElement(), new Enumerations.AdministrativeGenderEnumFactory(), false); 36631 } 36632 if (element.hasBirthDateElement()) { 36633 composeDateCore("birthDate", element.getBirthDateElement(), false); 36634 composeDateExtras("birthDate", element.getBirthDateElement(), false); 36635 } 36636 if (element.hasPhoto()) { 36637 openArray("photo"); 36638 for (Attachment e : element.getPhoto()) 36639 composeAttachment(null, e); 36640 closeArray(); 36641 }; 36642 if (element.hasQualification()) { 36643 openArray("qualification"); 36644 for (Practitioner.PractitionerQualificationComponent e : element.getQualification()) 36645 composePractitionerPractitionerQualificationComponent(null, e); 36646 closeArray(); 36647 }; 36648 if (element.hasCommunication()) { 36649 openArray("communication"); 36650 for (CodeableConcept e : element.getCommunication()) 36651 composeCodeableConcept(null, e); 36652 closeArray(); 36653 }; 36654 } 36655 36656 protected void composePractitionerPractitionerQualificationComponent(String name, Practitioner.PractitionerQualificationComponent element) throws IOException { 36657 if (element != null) { 36658 open(name); 36659 composePractitionerPractitionerQualificationComponentInner(element); 36660 close(); 36661 } 36662 } 36663 36664 protected void composePractitionerPractitionerQualificationComponentInner(Practitioner.PractitionerQualificationComponent element) throws IOException { 36665 composeBackbone(element); 36666 if (element.hasIdentifier()) { 36667 openArray("identifier"); 36668 for (Identifier e : element.getIdentifier()) 36669 composeIdentifier(null, e); 36670 closeArray(); 36671 }; 36672 if (element.hasCode()) { 36673 composeCodeableConcept("code", element.getCode()); 36674 } 36675 if (element.hasPeriod()) { 36676 composePeriod("period", element.getPeriod()); 36677 } 36678 if (element.hasIssuer()) { 36679 composeReference("issuer", element.getIssuer()); 36680 } 36681 } 36682 36683 protected void composePractitionerRole(String name, PractitionerRole element) throws IOException { 36684 if (element != null) { 36685 prop("resourceType", name); 36686 composePractitionerRoleInner(element); 36687 } 36688 } 36689 36690 protected void composePractitionerRoleInner(PractitionerRole element) throws IOException { 36691 composeDomainResourceElements(element); 36692 if (element.hasIdentifier()) { 36693 openArray("identifier"); 36694 for (Identifier e : element.getIdentifier()) 36695 composeIdentifier(null, e); 36696 closeArray(); 36697 }; 36698 if (element.hasActiveElement()) { 36699 composeBooleanCore("active", element.getActiveElement(), false); 36700 composeBooleanExtras("active", element.getActiveElement(), false); 36701 } 36702 if (element.hasPeriod()) { 36703 composePeriod("period", element.getPeriod()); 36704 } 36705 if (element.hasPractitioner()) { 36706 composeReference("practitioner", element.getPractitioner()); 36707 } 36708 if (element.hasOrganization()) { 36709 composeReference("organization", element.getOrganization()); 36710 } 36711 if (element.hasCode()) { 36712 openArray("code"); 36713 for (CodeableConcept e : element.getCode()) 36714 composeCodeableConcept(null, e); 36715 closeArray(); 36716 }; 36717 if (element.hasSpecialty()) { 36718 openArray("specialty"); 36719 for (CodeableConcept e : element.getSpecialty()) 36720 composeCodeableConcept(null, e); 36721 closeArray(); 36722 }; 36723 if (element.hasLocation()) { 36724 openArray("location"); 36725 for (Reference e : element.getLocation()) 36726 composeReference(null, e); 36727 closeArray(); 36728 }; 36729 if (element.hasHealthcareService()) { 36730 openArray("healthcareService"); 36731 for (Reference e : element.getHealthcareService()) 36732 composeReference(null, e); 36733 closeArray(); 36734 }; 36735 if (element.hasTelecom()) { 36736 openArray("telecom"); 36737 for (ContactPoint e : element.getTelecom()) 36738 composeContactPoint(null, e); 36739 closeArray(); 36740 }; 36741 if (element.hasAvailableTime()) { 36742 openArray("availableTime"); 36743 for (PractitionerRole.PractitionerRoleAvailableTimeComponent e : element.getAvailableTime()) 36744 composePractitionerRolePractitionerRoleAvailableTimeComponent(null, e); 36745 closeArray(); 36746 }; 36747 if (element.hasNotAvailable()) { 36748 openArray("notAvailable"); 36749 for (PractitionerRole.PractitionerRoleNotAvailableComponent e : element.getNotAvailable()) 36750 composePractitionerRolePractitionerRoleNotAvailableComponent(null, e); 36751 closeArray(); 36752 }; 36753 if (element.hasAvailabilityExceptionsElement()) { 36754 composeStringCore("availabilityExceptions", element.getAvailabilityExceptionsElement(), false); 36755 composeStringExtras("availabilityExceptions", element.getAvailabilityExceptionsElement(), false); 36756 } 36757 if (element.hasEndpoint()) { 36758 openArray("endpoint"); 36759 for (Reference e : element.getEndpoint()) 36760 composeReference(null, e); 36761 closeArray(); 36762 }; 36763 } 36764 36765 protected void composePractitionerRolePractitionerRoleAvailableTimeComponent(String name, PractitionerRole.PractitionerRoleAvailableTimeComponent element) throws IOException { 36766 if (element != null) { 36767 open(name); 36768 composePractitionerRolePractitionerRoleAvailableTimeComponentInner(element); 36769 close(); 36770 } 36771 } 36772 36773 protected void composePractitionerRolePractitionerRoleAvailableTimeComponentInner(PractitionerRole.PractitionerRoleAvailableTimeComponent element) throws IOException { 36774 composeBackbone(element); 36775 if (element.hasDaysOfWeek()) { 36776 openArray("daysOfWeek"); 36777 for (Enumeration<PractitionerRole.DaysOfWeek> e : element.getDaysOfWeek()) 36778 composeEnumerationCore(null, e, new PractitionerRole.DaysOfWeekEnumFactory(), true); 36779 closeArray(); 36780 if (anyHasExtras(element.getDaysOfWeek())) { 36781 openArray("_daysOfWeek"); 36782 for (Enumeration<PractitionerRole.DaysOfWeek> e : element.getDaysOfWeek()) 36783 composeEnumerationExtras(null, e, new PractitionerRole.DaysOfWeekEnumFactory(), true); 36784 closeArray(); 36785 } 36786 }; 36787 if (element.hasAllDayElement()) { 36788 composeBooleanCore("allDay", element.getAllDayElement(), false); 36789 composeBooleanExtras("allDay", element.getAllDayElement(), false); 36790 } 36791 if (element.hasAvailableStartTimeElement()) { 36792 composeTimeCore("availableStartTime", element.getAvailableStartTimeElement(), false); 36793 composeTimeExtras("availableStartTime", element.getAvailableStartTimeElement(), false); 36794 } 36795 if (element.hasAvailableEndTimeElement()) { 36796 composeTimeCore("availableEndTime", element.getAvailableEndTimeElement(), false); 36797 composeTimeExtras("availableEndTime", element.getAvailableEndTimeElement(), false); 36798 } 36799 } 36800 36801 protected void composePractitionerRolePractitionerRoleNotAvailableComponent(String name, PractitionerRole.PractitionerRoleNotAvailableComponent element) throws IOException { 36802 if (element != null) { 36803 open(name); 36804 composePractitionerRolePractitionerRoleNotAvailableComponentInner(element); 36805 close(); 36806 } 36807 } 36808 36809 protected void composePractitionerRolePractitionerRoleNotAvailableComponentInner(PractitionerRole.PractitionerRoleNotAvailableComponent element) throws IOException { 36810 composeBackbone(element); 36811 if (element.hasDescriptionElement()) { 36812 composeStringCore("description", element.getDescriptionElement(), false); 36813 composeStringExtras("description", element.getDescriptionElement(), false); 36814 } 36815 if (element.hasDuring()) { 36816 composePeriod("during", element.getDuring()); 36817 } 36818 } 36819 36820 protected void composeProcedure(String name, Procedure element) throws IOException { 36821 if (element != null) { 36822 prop("resourceType", name); 36823 composeProcedureInner(element); 36824 } 36825 } 36826 36827 protected void composeProcedureInner(Procedure element) throws IOException { 36828 composeDomainResourceElements(element); 36829 if (element.hasIdentifier()) { 36830 openArray("identifier"); 36831 for (Identifier e : element.getIdentifier()) 36832 composeIdentifier(null, e); 36833 closeArray(); 36834 }; 36835 if (element.hasDefinition()) { 36836 openArray("definition"); 36837 for (Reference e : element.getDefinition()) 36838 composeReference(null, e); 36839 closeArray(); 36840 }; 36841 if (element.hasBasedOn()) { 36842 openArray("basedOn"); 36843 for (Reference e : element.getBasedOn()) 36844 composeReference(null, e); 36845 closeArray(); 36846 }; 36847 if (element.hasPartOf()) { 36848 openArray("partOf"); 36849 for (Reference e : element.getPartOf()) 36850 composeReference(null, e); 36851 closeArray(); 36852 }; 36853 if (element.hasStatusElement()) { 36854 composeEnumerationCore("status", element.getStatusElement(), new Procedure.ProcedureStatusEnumFactory(), false); 36855 composeEnumerationExtras("status", element.getStatusElement(), new Procedure.ProcedureStatusEnumFactory(), false); 36856 } 36857 if (element.hasNotDoneElement()) { 36858 composeBooleanCore("notDone", element.getNotDoneElement(), false); 36859 composeBooleanExtras("notDone", element.getNotDoneElement(), false); 36860 } 36861 if (element.hasNotDoneReason()) { 36862 composeCodeableConcept("notDoneReason", element.getNotDoneReason()); 36863 } 36864 if (element.hasCategory()) { 36865 composeCodeableConcept("category", element.getCategory()); 36866 } 36867 if (element.hasCode()) { 36868 composeCodeableConcept("code", element.getCode()); 36869 } 36870 if (element.hasSubject()) { 36871 composeReference("subject", element.getSubject()); 36872 } 36873 if (element.hasContext()) { 36874 composeReference("context", element.getContext()); 36875 } 36876 if (element.hasPerformed()) { 36877 composeType("performed", element.getPerformed()); 36878 } 36879 if (element.hasPerformer()) { 36880 openArray("performer"); 36881 for (Procedure.ProcedurePerformerComponent e : element.getPerformer()) 36882 composeProcedureProcedurePerformerComponent(null, e); 36883 closeArray(); 36884 }; 36885 if (element.hasLocation()) { 36886 composeReference("location", element.getLocation()); 36887 } 36888 if (element.hasReasonCode()) { 36889 openArray("reasonCode"); 36890 for (CodeableConcept e : element.getReasonCode()) 36891 composeCodeableConcept(null, e); 36892 closeArray(); 36893 }; 36894 if (element.hasReasonReference()) { 36895 openArray("reasonReference"); 36896 for (Reference e : element.getReasonReference()) 36897 composeReference(null, e); 36898 closeArray(); 36899 }; 36900 if (element.hasBodySite()) { 36901 openArray("bodySite"); 36902 for (CodeableConcept e : element.getBodySite()) 36903 composeCodeableConcept(null, e); 36904 closeArray(); 36905 }; 36906 if (element.hasOutcome()) { 36907 composeCodeableConcept("outcome", element.getOutcome()); 36908 } 36909 if (element.hasReport()) { 36910 openArray("report"); 36911 for (Reference e : element.getReport()) 36912 composeReference(null, e); 36913 closeArray(); 36914 }; 36915 if (element.hasComplication()) { 36916 openArray("complication"); 36917 for (CodeableConcept e : element.getComplication()) 36918 composeCodeableConcept(null, e); 36919 closeArray(); 36920 }; 36921 if (element.hasComplicationDetail()) { 36922 openArray("complicationDetail"); 36923 for (Reference e : element.getComplicationDetail()) 36924 composeReference(null, e); 36925 closeArray(); 36926 }; 36927 if (element.hasFollowUp()) { 36928 openArray("followUp"); 36929 for (CodeableConcept e : element.getFollowUp()) 36930 composeCodeableConcept(null, e); 36931 closeArray(); 36932 }; 36933 if (element.hasNote()) { 36934 openArray("note"); 36935 for (Annotation e : element.getNote()) 36936 composeAnnotation(null, e); 36937 closeArray(); 36938 }; 36939 if (element.hasFocalDevice()) { 36940 openArray("focalDevice"); 36941 for (Procedure.ProcedureFocalDeviceComponent e : element.getFocalDevice()) 36942 composeProcedureProcedureFocalDeviceComponent(null, e); 36943 closeArray(); 36944 }; 36945 if (element.hasUsedReference()) { 36946 openArray("usedReference"); 36947 for (Reference e : element.getUsedReference()) 36948 composeReference(null, e); 36949 closeArray(); 36950 }; 36951 if (element.hasUsedCode()) { 36952 openArray("usedCode"); 36953 for (CodeableConcept e : element.getUsedCode()) 36954 composeCodeableConcept(null, e); 36955 closeArray(); 36956 }; 36957 } 36958 36959 protected void composeProcedureProcedurePerformerComponent(String name, Procedure.ProcedurePerformerComponent element) throws IOException { 36960 if (element != null) { 36961 open(name); 36962 composeProcedureProcedurePerformerComponentInner(element); 36963 close(); 36964 } 36965 } 36966 36967 protected void composeProcedureProcedurePerformerComponentInner(Procedure.ProcedurePerformerComponent element) throws IOException { 36968 composeBackbone(element); 36969 if (element.hasRole()) { 36970 composeCodeableConcept("role", element.getRole()); 36971 } 36972 if (element.hasActor()) { 36973 composeReference("actor", element.getActor()); 36974 } 36975 if (element.hasOnBehalfOf()) { 36976 composeReference("onBehalfOf", element.getOnBehalfOf()); 36977 } 36978 } 36979 36980 protected void composeProcedureProcedureFocalDeviceComponent(String name, Procedure.ProcedureFocalDeviceComponent element) throws IOException { 36981 if (element != null) { 36982 open(name); 36983 composeProcedureProcedureFocalDeviceComponentInner(element); 36984 close(); 36985 } 36986 } 36987 36988 protected void composeProcedureProcedureFocalDeviceComponentInner(Procedure.ProcedureFocalDeviceComponent element) throws IOException { 36989 composeBackbone(element); 36990 if (element.hasAction()) { 36991 composeCodeableConcept("action", element.getAction()); 36992 } 36993 if (element.hasManipulated()) { 36994 composeReference("manipulated", element.getManipulated()); 36995 } 36996 } 36997 36998 protected void composeProcedureRequest(String name, ProcedureRequest element) throws IOException { 36999 if (element != null) { 37000 prop("resourceType", name); 37001 composeProcedureRequestInner(element); 37002 } 37003 } 37004 37005 protected void composeProcedureRequestInner(ProcedureRequest element) throws IOException { 37006 composeDomainResourceElements(element); 37007 if (element.hasIdentifier()) { 37008 openArray("identifier"); 37009 for (Identifier e : element.getIdentifier()) 37010 composeIdentifier(null, e); 37011 closeArray(); 37012 }; 37013 if (element.hasDefinition()) { 37014 openArray("definition"); 37015 for (Reference e : element.getDefinition()) 37016 composeReference(null, e); 37017 closeArray(); 37018 }; 37019 if (element.hasBasedOn()) { 37020 openArray("basedOn"); 37021 for (Reference e : element.getBasedOn()) 37022 composeReference(null, e); 37023 closeArray(); 37024 }; 37025 if (element.hasReplaces()) { 37026 openArray("replaces"); 37027 for (Reference e : element.getReplaces()) 37028 composeReference(null, e); 37029 closeArray(); 37030 }; 37031 if (element.hasRequisition()) { 37032 composeIdentifier("requisition", element.getRequisition()); 37033 } 37034 if (element.hasStatusElement()) { 37035 composeEnumerationCore("status", element.getStatusElement(), new ProcedureRequest.ProcedureRequestStatusEnumFactory(), false); 37036 composeEnumerationExtras("status", element.getStatusElement(), new ProcedureRequest.ProcedureRequestStatusEnumFactory(), false); 37037 } 37038 if (element.hasIntentElement()) { 37039 composeEnumerationCore("intent", element.getIntentElement(), new ProcedureRequest.ProcedureRequestIntentEnumFactory(), false); 37040 composeEnumerationExtras("intent", element.getIntentElement(), new ProcedureRequest.ProcedureRequestIntentEnumFactory(), false); 37041 } 37042 if (element.hasPriorityElement()) { 37043 composeEnumerationCore("priority", element.getPriorityElement(), new ProcedureRequest.ProcedureRequestPriorityEnumFactory(), false); 37044 composeEnumerationExtras("priority", element.getPriorityElement(), new ProcedureRequest.ProcedureRequestPriorityEnumFactory(), false); 37045 } 37046 if (element.hasDoNotPerformElement()) { 37047 composeBooleanCore("doNotPerform", element.getDoNotPerformElement(), false); 37048 composeBooleanExtras("doNotPerform", element.getDoNotPerformElement(), false); 37049 } 37050 if (element.hasCategory()) { 37051 openArray("category"); 37052 for (CodeableConcept e : element.getCategory()) 37053 composeCodeableConcept(null, e); 37054 closeArray(); 37055 }; 37056 if (element.hasCode()) { 37057 composeCodeableConcept("code", element.getCode()); 37058 } 37059 if (element.hasSubject()) { 37060 composeReference("subject", element.getSubject()); 37061 } 37062 if (element.hasContext()) { 37063 composeReference("context", element.getContext()); 37064 } 37065 if (element.hasOccurrence()) { 37066 composeType("occurrence", element.getOccurrence()); 37067 } 37068 if (element.hasAsNeeded()) { 37069 composeType("asNeeded", element.getAsNeeded()); 37070 } 37071 if (element.hasAuthoredOnElement()) { 37072 composeDateTimeCore("authoredOn", element.getAuthoredOnElement(), false); 37073 composeDateTimeExtras("authoredOn", element.getAuthoredOnElement(), false); 37074 } 37075 if (element.hasRequester()) { 37076 composeProcedureRequestProcedureRequestRequesterComponent("requester", element.getRequester()); 37077 } 37078 if (element.hasPerformerType()) { 37079 composeCodeableConcept("performerType", element.getPerformerType()); 37080 } 37081 if (element.hasPerformer()) { 37082 composeReference("performer", element.getPerformer()); 37083 } 37084 if (element.hasReasonCode()) { 37085 openArray("reasonCode"); 37086 for (CodeableConcept e : element.getReasonCode()) 37087 composeCodeableConcept(null, e); 37088 closeArray(); 37089 }; 37090 if (element.hasReasonReference()) { 37091 openArray("reasonReference"); 37092 for (Reference e : element.getReasonReference()) 37093 composeReference(null, e); 37094 closeArray(); 37095 }; 37096 if (element.hasSupportingInfo()) { 37097 openArray("supportingInfo"); 37098 for (Reference e : element.getSupportingInfo()) 37099 composeReference(null, e); 37100 closeArray(); 37101 }; 37102 if (element.hasSpecimen()) { 37103 openArray("specimen"); 37104 for (Reference e : element.getSpecimen()) 37105 composeReference(null, e); 37106 closeArray(); 37107 }; 37108 if (element.hasBodySite()) { 37109 openArray("bodySite"); 37110 for (CodeableConcept e : element.getBodySite()) 37111 composeCodeableConcept(null, e); 37112 closeArray(); 37113 }; 37114 if (element.hasNote()) { 37115 openArray("note"); 37116 for (Annotation e : element.getNote()) 37117 composeAnnotation(null, e); 37118 closeArray(); 37119 }; 37120 if (element.hasRelevantHistory()) { 37121 openArray("relevantHistory"); 37122 for (Reference e : element.getRelevantHistory()) 37123 composeReference(null, e); 37124 closeArray(); 37125 }; 37126 } 37127 37128 protected void composeProcedureRequestProcedureRequestRequesterComponent(String name, ProcedureRequest.ProcedureRequestRequesterComponent element) throws IOException { 37129 if (element != null) { 37130 open(name); 37131 composeProcedureRequestProcedureRequestRequesterComponentInner(element); 37132 close(); 37133 } 37134 } 37135 37136 protected void composeProcedureRequestProcedureRequestRequesterComponentInner(ProcedureRequest.ProcedureRequestRequesterComponent element) throws IOException { 37137 composeBackbone(element); 37138 if (element.hasAgent()) { 37139 composeReference("agent", element.getAgent()); 37140 } 37141 if (element.hasOnBehalfOf()) { 37142 composeReference("onBehalfOf", element.getOnBehalfOf()); 37143 } 37144 } 37145 37146 protected void composeProcessRequest(String name, ProcessRequest element) throws IOException { 37147 if (element != null) { 37148 prop("resourceType", name); 37149 composeProcessRequestInner(element); 37150 } 37151 } 37152 37153 protected void composeProcessRequestInner(ProcessRequest element) throws IOException { 37154 composeDomainResourceElements(element); 37155 if (element.hasIdentifier()) { 37156 openArray("identifier"); 37157 for (Identifier e : element.getIdentifier()) 37158 composeIdentifier(null, e); 37159 closeArray(); 37160 }; 37161 if (element.hasStatusElement()) { 37162 composeEnumerationCore("status", element.getStatusElement(), new ProcessRequest.ProcessRequestStatusEnumFactory(), false); 37163 composeEnumerationExtras("status", element.getStatusElement(), new ProcessRequest.ProcessRequestStatusEnumFactory(), false); 37164 } 37165 if (element.hasActionElement()) { 37166 composeEnumerationCore("action", element.getActionElement(), new ProcessRequest.ActionListEnumFactory(), false); 37167 composeEnumerationExtras("action", element.getActionElement(), new ProcessRequest.ActionListEnumFactory(), false); 37168 } 37169 if (element.hasTarget()) { 37170 composeReference("target", element.getTarget()); 37171 } 37172 if (element.hasCreatedElement()) { 37173 composeDateTimeCore("created", element.getCreatedElement(), false); 37174 composeDateTimeExtras("created", element.getCreatedElement(), false); 37175 } 37176 if (element.hasProvider()) { 37177 composeReference("provider", element.getProvider()); 37178 } 37179 if (element.hasOrganization()) { 37180 composeReference("organization", element.getOrganization()); 37181 } 37182 if (element.hasRequest()) { 37183 composeReference("request", element.getRequest()); 37184 } 37185 if (element.hasResponse()) { 37186 composeReference("response", element.getResponse()); 37187 } 37188 if (element.hasNullifyElement()) { 37189 composeBooleanCore("nullify", element.getNullifyElement(), false); 37190 composeBooleanExtras("nullify", element.getNullifyElement(), false); 37191 } 37192 if (element.hasReferenceElement()) { 37193 composeStringCore("reference", element.getReferenceElement(), false); 37194 composeStringExtras("reference", element.getReferenceElement(), false); 37195 } 37196 if (element.hasItem()) { 37197 openArray("item"); 37198 for (ProcessRequest.ItemsComponent e : element.getItem()) 37199 composeProcessRequestItemsComponent(null, e); 37200 closeArray(); 37201 }; 37202 if (element.hasInclude()) { 37203 openArray("include"); 37204 for (StringType e : element.getInclude()) 37205 composeStringCore(null, e, true); 37206 closeArray(); 37207 if (anyHasExtras(element.getInclude())) { 37208 openArray("_include"); 37209 for (StringType e : element.getInclude()) 37210 composeStringExtras(null, e, true); 37211 closeArray(); 37212 } 37213 }; 37214 if (element.hasExclude()) { 37215 openArray("exclude"); 37216 for (StringType e : element.getExclude()) 37217 composeStringCore(null, e, true); 37218 closeArray(); 37219 if (anyHasExtras(element.getExclude())) { 37220 openArray("_exclude"); 37221 for (StringType e : element.getExclude()) 37222 composeStringExtras(null, e, true); 37223 closeArray(); 37224 } 37225 }; 37226 if (element.hasPeriod()) { 37227 composePeriod("period", element.getPeriod()); 37228 } 37229 } 37230 37231 protected void composeProcessRequestItemsComponent(String name, ProcessRequest.ItemsComponent element) throws IOException { 37232 if (element != null) { 37233 open(name); 37234 composeProcessRequestItemsComponentInner(element); 37235 close(); 37236 } 37237 } 37238 37239 protected void composeProcessRequestItemsComponentInner(ProcessRequest.ItemsComponent element) throws IOException { 37240 composeBackbone(element); 37241 if (element.hasSequenceLinkIdElement()) { 37242 composeIntegerCore("sequenceLinkId", element.getSequenceLinkIdElement(), false); 37243 composeIntegerExtras("sequenceLinkId", element.getSequenceLinkIdElement(), false); 37244 } 37245 } 37246 37247 protected void composeProcessResponse(String name, ProcessResponse element) throws IOException { 37248 if (element != null) { 37249 prop("resourceType", name); 37250 composeProcessResponseInner(element); 37251 } 37252 } 37253 37254 protected void composeProcessResponseInner(ProcessResponse element) throws IOException { 37255 composeDomainResourceElements(element); 37256 if (element.hasIdentifier()) { 37257 openArray("identifier"); 37258 for (Identifier e : element.getIdentifier()) 37259 composeIdentifier(null, e); 37260 closeArray(); 37261 }; 37262 if (element.hasStatusElement()) { 37263 composeEnumerationCore("status", element.getStatusElement(), new ProcessResponse.ProcessResponseStatusEnumFactory(), false); 37264 composeEnumerationExtras("status", element.getStatusElement(), new ProcessResponse.ProcessResponseStatusEnumFactory(), false); 37265 } 37266 if (element.hasCreatedElement()) { 37267 composeDateTimeCore("created", element.getCreatedElement(), false); 37268 composeDateTimeExtras("created", element.getCreatedElement(), false); 37269 } 37270 if (element.hasOrganization()) { 37271 composeReference("organization", element.getOrganization()); 37272 } 37273 if (element.hasRequest()) { 37274 composeReference("request", element.getRequest()); 37275 } 37276 if (element.hasOutcome()) { 37277 composeCodeableConcept("outcome", element.getOutcome()); 37278 } 37279 if (element.hasDispositionElement()) { 37280 composeStringCore("disposition", element.getDispositionElement(), false); 37281 composeStringExtras("disposition", element.getDispositionElement(), false); 37282 } 37283 if (element.hasRequestProvider()) { 37284 composeReference("requestProvider", element.getRequestProvider()); 37285 } 37286 if (element.hasRequestOrganization()) { 37287 composeReference("requestOrganization", element.getRequestOrganization()); 37288 } 37289 if (element.hasForm()) { 37290 composeCodeableConcept("form", element.getForm()); 37291 } 37292 if (element.hasProcessNote()) { 37293 openArray("processNote"); 37294 for (ProcessResponse.ProcessResponseProcessNoteComponent e : element.getProcessNote()) 37295 composeProcessResponseProcessResponseProcessNoteComponent(null, e); 37296 closeArray(); 37297 }; 37298 if (element.hasError()) { 37299 openArray("error"); 37300 for (CodeableConcept e : element.getError()) 37301 composeCodeableConcept(null, e); 37302 closeArray(); 37303 }; 37304 if (element.hasCommunicationRequest()) { 37305 openArray("communicationRequest"); 37306 for (Reference e : element.getCommunicationRequest()) 37307 composeReference(null, e); 37308 closeArray(); 37309 }; 37310 } 37311 37312 protected void composeProcessResponseProcessResponseProcessNoteComponent(String name, ProcessResponse.ProcessResponseProcessNoteComponent element) throws IOException { 37313 if (element != null) { 37314 open(name); 37315 composeProcessResponseProcessResponseProcessNoteComponentInner(element); 37316 close(); 37317 } 37318 } 37319 37320 protected void composeProcessResponseProcessResponseProcessNoteComponentInner(ProcessResponse.ProcessResponseProcessNoteComponent element) throws IOException { 37321 composeBackbone(element); 37322 if (element.hasType()) { 37323 composeCodeableConcept("type", element.getType()); 37324 } 37325 if (element.hasTextElement()) { 37326 composeStringCore("text", element.getTextElement(), false); 37327 composeStringExtras("text", element.getTextElement(), false); 37328 } 37329 } 37330 37331 protected void composeProvenance(String name, Provenance element) throws IOException { 37332 if (element != null) { 37333 prop("resourceType", name); 37334 composeProvenanceInner(element); 37335 } 37336 } 37337 37338 protected void composeProvenanceInner(Provenance element) throws IOException { 37339 composeDomainResourceElements(element); 37340 if (element.hasTarget()) { 37341 openArray("target"); 37342 for (Reference e : element.getTarget()) 37343 composeReference(null, e); 37344 closeArray(); 37345 }; 37346 if (element.hasPeriod()) { 37347 composePeriod("period", element.getPeriod()); 37348 } 37349 if (element.hasRecordedElement()) { 37350 composeInstantCore("recorded", element.getRecordedElement(), false); 37351 composeInstantExtras("recorded", element.getRecordedElement(), false); 37352 } 37353 if (element.hasPolicy()) { 37354 openArray("policy"); 37355 for (UriType e : element.getPolicy()) 37356 composeUriCore(null, e, true); 37357 closeArray(); 37358 if (anyHasExtras(element.getPolicy())) { 37359 openArray("_policy"); 37360 for (UriType e : element.getPolicy()) 37361 composeUriExtras(null, e, true); 37362 closeArray(); 37363 } 37364 }; 37365 if (element.hasLocation()) { 37366 composeReference("location", element.getLocation()); 37367 } 37368 if (element.hasReason()) { 37369 openArray("reason"); 37370 for (Coding e : element.getReason()) 37371 composeCoding(null, e); 37372 closeArray(); 37373 }; 37374 if (element.hasActivity()) { 37375 composeCoding("activity", element.getActivity()); 37376 } 37377 if (element.hasAgent()) { 37378 openArray("agent"); 37379 for (Provenance.ProvenanceAgentComponent e : element.getAgent()) 37380 composeProvenanceProvenanceAgentComponent(null, e); 37381 closeArray(); 37382 }; 37383 if (element.hasEntity()) { 37384 openArray("entity"); 37385 for (Provenance.ProvenanceEntityComponent e : element.getEntity()) 37386 composeProvenanceProvenanceEntityComponent(null, e); 37387 closeArray(); 37388 }; 37389 if (element.hasSignature()) { 37390 openArray("signature"); 37391 for (Signature e : element.getSignature()) 37392 composeSignature(null, e); 37393 closeArray(); 37394 }; 37395 } 37396 37397 protected void composeProvenanceProvenanceAgentComponent(String name, Provenance.ProvenanceAgentComponent element) throws IOException { 37398 if (element != null) { 37399 open(name); 37400 composeProvenanceProvenanceAgentComponentInner(element); 37401 close(); 37402 } 37403 } 37404 37405 protected void composeProvenanceProvenanceAgentComponentInner(Provenance.ProvenanceAgentComponent element) throws IOException { 37406 composeBackbone(element); 37407 if (element.hasRole()) { 37408 openArray("role"); 37409 for (CodeableConcept e : element.getRole()) 37410 composeCodeableConcept(null, e); 37411 closeArray(); 37412 }; 37413 if (element.hasWho()) { 37414 composeType("who", element.getWho()); 37415 } 37416 if (element.hasOnBehalfOf()) { 37417 composeType("onBehalfOf", element.getOnBehalfOf()); 37418 } 37419 if (element.hasRelatedAgentType()) { 37420 composeCodeableConcept("relatedAgentType", element.getRelatedAgentType()); 37421 } 37422 } 37423 37424 protected void composeProvenanceProvenanceEntityComponent(String name, Provenance.ProvenanceEntityComponent element) throws IOException { 37425 if (element != null) { 37426 open(name); 37427 composeProvenanceProvenanceEntityComponentInner(element); 37428 close(); 37429 } 37430 } 37431 37432 protected void composeProvenanceProvenanceEntityComponentInner(Provenance.ProvenanceEntityComponent element) throws IOException { 37433 composeBackbone(element); 37434 if (element.hasRoleElement()) { 37435 composeEnumerationCore("role", element.getRoleElement(), new Provenance.ProvenanceEntityRoleEnumFactory(), false); 37436 composeEnumerationExtras("role", element.getRoleElement(), new Provenance.ProvenanceEntityRoleEnumFactory(), false); 37437 } 37438 if (element.hasWhat()) { 37439 composeType("what", element.getWhat()); 37440 } 37441 if (element.hasAgent()) { 37442 openArray("agent"); 37443 for (Provenance.ProvenanceAgentComponent e : element.getAgent()) 37444 composeProvenanceProvenanceAgentComponent(null, e); 37445 closeArray(); 37446 }; 37447 } 37448 37449 protected void composeQuestionnaire(String name, Questionnaire element) throws IOException { 37450 if (element != null) { 37451 prop("resourceType", name); 37452 composeQuestionnaireInner(element); 37453 } 37454 } 37455 37456 protected void composeQuestionnaireInner(Questionnaire element) throws IOException { 37457 composeDomainResourceElements(element); 37458 if (element.hasUrlElement()) { 37459 composeUriCore("url", element.getUrlElement(), false); 37460 composeUriExtras("url", element.getUrlElement(), false); 37461 } 37462 if (element.hasIdentifier()) { 37463 openArray("identifier"); 37464 for (Identifier e : element.getIdentifier()) 37465 composeIdentifier(null, e); 37466 closeArray(); 37467 }; 37468 if (element.hasVersionElement()) { 37469 composeStringCore("version", element.getVersionElement(), false); 37470 composeStringExtras("version", element.getVersionElement(), false); 37471 } 37472 if (element.hasNameElement()) { 37473 composeStringCore("name", element.getNameElement(), false); 37474 composeStringExtras("name", element.getNameElement(), false); 37475 } 37476 if (element.hasTitleElement()) { 37477 composeStringCore("title", element.getTitleElement(), false); 37478 composeStringExtras("title", element.getTitleElement(), false); 37479 } 37480 if (element.hasStatusElement()) { 37481 composeEnumerationCore("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 37482 composeEnumerationExtras("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 37483 } 37484 if (element.hasExperimentalElement()) { 37485 composeBooleanCore("experimental", element.getExperimentalElement(), false); 37486 composeBooleanExtras("experimental", element.getExperimentalElement(), false); 37487 } 37488 if (element.hasDateElement()) { 37489 composeDateTimeCore("date", element.getDateElement(), false); 37490 composeDateTimeExtras("date", element.getDateElement(), false); 37491 } 37492 if (element.hasPublisherElement()) { 37493 composeStringCore("publisher", element.getPublisherElement(), false); 37494 composeStringExtras("publisher", element.getPublisherElement(), false); 37495 } 37496 if (element.hasDescriptionElement()) { 37497 composeMarkdownCore("description", element.getDescriptionElement(), false); 37498 composeMarkdownExtras("description", element.getDescriptionElement(), false); 37499 } 37500 if (element.hasPurposeElement()) { 37501 composeMarkdownCore("purpose", element.getPurposeElement(), false); 37502 composeMarkdownExtras("purpose", element.getPurposeElement(), false); 37503 } 37504 if (element.hasApprovalDateElement()) { 37505 composeDateCore("approvalDate", element.getApprovalDateElement(), false); 37506 composeDateExtras("approvalDate", element.getApprovalDateElement(), false); 37507 } 37508 if (element.hasLastReviewDateElement()) { 37509 composeDateCore("lastReviewDate", element.getLastReviewDateElement(), false); 37510 composeDateExtras("lastReviewDate", element.getLastReviewDateElement(), false); 37511 } 37512 if (element.hasEffectivePeriod()) { 37513 composePeriod("effectivePeriod", element.getEffectivePeriod()); 37514 } 37515 if (element.hasUseContext()) { 37516 openArray("useContext"); 37517 for (UsageContext e : element.getUseContext()) 37518 composeUsageContext(null, e); 37519 closeArray(); 37520 }; 37521 if (element.hasJurisdiction()) { 37522 openArray("jurisdiction"); 37523 for (CodeableConcept e : element.getJurisdiction()) 37524 composeCodeableConcept(null, e); 37525 closeArray(); 37526 }; 37527 if (element.hasContact()) { 37528 openArray("contact"); 37529 for (ContactDetail e : element.getContact()) 37530 composeContactDetail(null, e); 37531 closeArray(); 37532 }; 37533 if (element.hasCopyrightElement()) { 37534 composeMarkdownCore("copyright", element.getCopyrightElement(), false); 37535 composeMarkdownExtras("copyright", element.getCopyrightElement(), false); 37536 } 37537 if (element.hasCode()) { 37538 openArray("code"); 37539 for (Coding e : element.getCode()) 37540 composeCoding(null, e); 37541 closeArray(); 37542 }; 37543 if (element.hasSubjectType()) { 37544 openArray("subjectType"); 37545 for (CodeType e : element.getSubjectType()) 37546 composeCodeCore(null, e, true); 37547 closeArray(); 37548 if (anyHasExtras(element.getSubjectType())) { 37549 openArray("_subjectType"); 37550 for (CodeType e : element.getSubjectType()) 37551 composeCodeExtras(null, e, true); 37552 closeArray(); 37553 } 37554 }; 37555 if (element.hasItem()) { 37556 openArray("item"); 37557 for (Questionnaire.QuestionnaireItemComponent e : element.getItem()) 37558 composeQuestionnaireQuestionnaireItemComponent(null, e); 37559 closeArray(); 37560 }; 37561 } 37562 37563 protected void composeQuestionnaireQuestionnaireItemComponent(String name, Questionnaire.QuestionnaireItemComponent element) throws IOException { 37564 if (element != null) { 37565 open(name); 37566 composeQuestionnaireQuestionnaireItemComponentInner(element); 37567 close(); 37568 } 37569 } 37570 37571 protected void composeQuestionnaireQuestionnaireItemComponentInner(Questionnaire.QuestionnaireItemComponent element) throws IOException { 37572 composeBackbone(element); 37573 if (element.hasLinkIdElement()) { 37574 composeStringCore("linkId", element.getLinkIdElement(), false); 37575 composeStringExtras("linkId", element.getLinkIdElement(), false); 37576 } 37577 if (element.hasDefinitionElement()) { 37578 composeUriCore("definition", element.getDefinitionElement(), false); 37579 composeUriExtras("definition", element.getDefinitionElement(), false); 37580 } 37581 if (element.hasCode()) { 37582 openArray("code"); 37583 for (Coding e : element.getCode()) 37584 composeCoding(null, e); 37585 closeArray(); 37586 }; 37587 if (element.hasPrefixElement()) { 37588 composeStringCore("prefix", element.getPrefixElement(), false); 37589 composeStringExtras("prefix", element.getPrefixElement(), false); 37590 } 37591 if (element.hasTextElement()) { 37592 composeStringCore("text", element.getTextElement(), false); 37593 composeStringExtras("text", element.getTextElement(), false); 37594 } 37595 if (element.hasTypeElement()) { 37596 composeEnumerationCore("type", element.getTypeElement(), new Questionnaire.QuestionnaireItemTypeEnumFactory(), false); 37597 composeEnumerationExtras("type", element.getTypeElement(), new Questionnaire.QuestionnaireItemTypeEnumFactory(), false); 37598 } 37599 if (element.hasEnableWhen()) { 37600 openArray("enableWhen"); 37601 for (Questionnaire.QuestionnaireItemEnableWhenComponent e : element.getEnableWhen()) 37602 composeQuestionnaireQuestionnaireItemEnableWhenComponent(null, e); 37603 closeArray(); 37604 }; 37605 if (element.hasRequiredElement()) { 37606 composeBooleanCore("required", element.getRequiredElement(), false); 37607 composeBooleanExtras("required", element.getRequiredElement(), false); 37608 } 37609 if (element.hasRepeatsElement()) { 37610 composeBooleanCore("repeats", element.getRepeatsElement(), false); 37611 composeBooleanExtras("repeats", element.getRepeatsElement(), false); 37612 } 37613 if (element.hasReadOnlyElement()) { 37614 composeBooleanCore("readOnly", element.getReadOnlyElement(), false); 37615 composeBooleanExtras("readOnly", element.getReadOnlyElement(), false); 37616 } 37617 if (element.hasMaxLengthElement()) { 37618 composeIntegerCore("maxLength", element.getMaxLengthElement(), false); 37619 composeIntegerExtras("maxLength", element.getMaxLengthElement(), false); 37620 } 37621 if (element.hasOptions()) { 37622 composeReference("options", element.getOptions()); 37623 } 37624 if (element.hasOption()) { 37625 openArray("option"); 37626 for (Questionnaire.QuestionnaireItemOptionComponent e : element.getOption()) 37627 composeQuestionnaireQuestionnaireItemOptionComponent(null, e); 37628 closeArray(); 37629 }; 37630 if (element.hasInitial()) { 37631 composeType("initial", element.getInitial()); 37632 } 37633 if (element.hasItem()) { 37634 openArray("item"); 37635 for (Questionnaire.QuestionnaireItemComponent e : element.getItem()) 37636 composeQuestionnaireQuestionnaireItemComponent(null, e); 37637 closeArray(); 37638 }; 37639 } 37640 37641 protected void composeQuestionnaireQuestionnaireItemEnableWhenComponent(String name, Questionnaire.QuestionnaireItemEnableWhenComponent element) throws IOException { 37642 if (element != null) { 37643 open(name); 37644 composeQuestionnaireQuestionnaireItemEnableWhenComponentInner(element); 37645 close(); 37646 } 37647 } 37648 37649 protected void composeQuestionnaireQuestionnaireItemEnableWhenComponentInner(Questionnaire.QuestionnaireItemEnableWhenComponent element) throws IOException { 37650 composeBackbone(element); 37651 if (element.hasQuestionElement()) { 37652 composeStringCore("question", element.getQuestionElement(), false); 37653 composeStringExtras("question", element.getQuestionElement(), false); 37654 } 37655 if (element.hasHasAnswerElement()) { 37656 composeBooleanCore("hasAnswer", element.getHasAnswerElement(), false); 37657 composeBooleanExtras("hasAnswer", element.getHasAnswerElement(), false); 37658 } 37659 if (element.hasAnswer()) { 37660 composeType("answer", element.getAnswer()); 37661 } 37662 } 37663 37664 protected void composeQuestionnaireQuestionnaireItemOptionComponent(String name, Questionnaire.QuestionnaireItemOptionComponent element) throws IOException { 37665 if (element != null) { 37666 open(name); 37667 composeQuestionnaireQuestionnaireItemOptionComponentInner(element); 37668 close(); 37669 } 37670 } 37671 37672 protected void composeQuestionnaireQuestionnaireItemOptionComponentInner(Questionnaire.QuestionnaireItemOptionComponent element) throws IOException { 37673 composeBackbone(element); 37674 if (element.hasValue()) { 37675 composeType("value", element.getValue()); 37676 } 37677 } 37678 37679 protected void composeQuestionnaireResponse(String name, QuestionnaireResponse element) throws IOException { 37680 if (element != null) { 37681 prop("resourceType", name); 37682 composeQuestionnaireResponseInner(element); 37683 } 37684 } 37685 37686 protected void composeQuestionnaireResponseInner(QuestionnaireResponse element) throws IOException { 37687 composeDomainResourceElements(element); 37688 if (element.hasIdentifier()) { 37689 composeIdentifier("identifier", element.getIdentifier()); 37690 } 37691 if (element.hasBasedOn()) { 37692 openArray("basedOn"); 37693 for (Reference e : element.getBasedOn()) 37694 composeReference(null, e); 37695 closeArray(); 37696 }; 37697 if (element.hasParent()) { 37698 openArray("parent"); 37699 for (Reference e : element.getParent()) 37700 composeReference(null, e); 37701 closeArray(); 37702 }; 37703 if (element.hasQuestionnaire()) { 37704 composeReference("questionnaire", element.getQuestionnaire()); 37705 } 37706 if (element.hasStatusElement()) { 37707 composeEnumerationCore("status", element.getStatusElement(), new QuestionnaireResponse.QuestionnaireResponseStatusEnumFactory(), false); 37708 composeEnumerationExtras("status", element.getStatusElement(), new QuestionnaireResponse.QuestionnaireResponseStatusEnumFactory(), false); 37709 } 37710 if (element.hasSubject()) { 37711 composeReference("subject", element.getSubject()); 37712 } 37713 if (element.hasContext()) { 37714 composeReference("context", element.getContext()); 37715 } 37716 if (element.hasAuthoredElement()) { 37717 composeDateTimeCore("authored", element.getAuthoredElement(), false); 37718 composeDateTimeExtras("authored", element.getAuthoredElement(), false); 37719 } 37720 if (element.hasAuthor()) { 37721 composeReference("author", element.getAuthor()); 37722 } 37723 if (element.hasSource()) { 37724 composeReference("source", element.getSource()); 37725 } 37726 if (element.hasItem()) { 37727 openArray("item"); 37728 for (QuestionnaireResponse.QuestionnaireResponseItemComponent e : element.getItem()) 37729 composeQuestionnaireResponseQuestionnaireResponseItemComponent(null, e); 37730 closeArray(); 37731 }; 37732 } 37733 37734 protected void composeQuestionnaireResponseQuestionnaireResponseItemComponent(String name, QuestionnaireResponse.QuestionnaireResponseItemComponent element) throws IOException { 37735 if (element != null) { 37736 open(name); 37737 composeQuestionnaireResponseQuestionnaireResponseItemComponentInner(element); 37738 close(); 37739 } 37740 } 37741 37742 protected void composeQuestionnaireResponseQuestionnaireResponseItemComponentInner(QuestionnaireResponse.QuestionnaireResponseItemComponent element) throws IOException { 37743 composeBackbone(element); 37744 if (element.hasLinkIdElement()) { 37745 composeStringCore("linkId", element.getLinkIdElement(), false); 37746 composeStringExtras("linkId", element.getLinkIdElement(), false); 37747 } 37748 if (element.hasDefinitionElement()) { 37749 composeUriCore("definition", element.getDefinitionElement(), false); 37750 composeUriExtras("definition", element.getDefinitionElement(), false); 37751 } 37752 if (element.hasTextElement()) { 37753 composeStringCore("text", element.getTextElement(), false); 37754 composeStringExtras("text", element.getTextElement(), false); 37755 } 37756 if (element.hasSubject()) { 37757 composeReference("subject", element.getSubject()); 37758 } 37759 if (element.hasAnswer()) { 37760 openArray("answer"); 37761 for (QuestionnaireResponse.QuestionnaireResponseItemAnswerComponent e : element.getAnswer()) 37762 composeQuestionnaireResponseQuestionnaireResponseItemAnswerComponent(null, e); 37763 closeArray(); 37764 }; 37765 if (element.hasItem()) { 37766 openArray("item"); 37767 for (QuestionnaireResponse.QuestionnaireResponseItemComponent e : element.getItem()) 37768 composeQuestionnaireResponseQuestionnaireResponseItemComponent(null, e); 37769 closeArray(); 37770 }; 37771 } 37772 37773 protected void composeQuestionnaireResponseQuestionnaireResponseItemAnswerComponent(String name, QuestionnaireResponse.QuestionnaireResponseItemAnswerComponent element) throws IOException { 37774 if (element != null) { 37775 open(name); 37776 composeQuestionnaireResponseQuestionnaireResponseItemAnswerComponentInner(element); 37777 close(); 37778 } 37779 } 37780 37781 protected void composeQuestionnaireResponseQuestionnaireResponseItemAnswerComponentInner(QuestionnaireResponse.QuestionnaireResponseItemAnswerComponent element) throws IOException { 37782 composeBackbone(element); 37783 if (element.hasValue()) { 37784 composeType("value", element.getValue()); 37785 } 37786 if (element.hasItem()) { 37787 openArray("item"); 37788 for (QuestionnaireResponse.QuestionnaireResponseItemComponent e : element.getItem()) 37789 composeQuestionnaireResponseQuestionnaireResponseItemComponent(null, e); 37790 closeArray(); 37791 }; 37792 } 37793 37794 protected void composeReferralRequest(String name, ReferralRequest element) throws IOException { 37795 if (element != null) { 37796 prop("resourceType", name); 37797 composeReferralRequestInner(element); 37798 } 37799 } 37800 37801 protected void composeReferralRequestInner(ReferralRequest element) throws IOException { 37802 composeDomainResourceElements(element); 37803 if (element.hasIdentifier()) { 37804 openArray("identifier"); 37805 for (Identifier e : element.getIdentifier()) 37806 composeIdentifier(null, e); 37807 closeArray(); 37808 }; 37809 if (element.hasDefinition()) { 37810 openArray("definition"); 37811 for (Reference e : element.getDefinition()) 37812 composeReference(null, e); 37813 closeArray(); 37814 }; 37815 if (element.hasBasedOn()) { 37816 openArray("basedOn"); 37817 for (Reference e : element.getBasedOn()) 37818 composeReference(null, e); 37819 closeArray(); 37820 }; 37821 if (element.hasReplaces()) { 37822 openArray("replaces"); 37823 for (Reference e : element.getReplaces()) 37824 composeReference(null, e); 37825 closeArray(); 37826 }; 37827 if (element.hasGroupIdentifier()) { 37828 composeIdentifier("groupIdentifier", element.getGroupIdentifier()); 37829 } 37830 if (element.hasStatusElement()) { 37831 composeEnumerationCore("status", element.getStatusElement(), new ReferralRequest.ReferralRequestStatusEnumFactory(), false); 37832 composeEnumerationExtras("status", element.getStatusElement(), new ReferralRequest.ReferralRequestStatusEnumFactory(), false); 37833 } 37834 if (element.hasIntentElement()) { 37835 composeEnumerationCore("intent", element.getIntentElement(), new ReferralRequest.ReferralCategoryEnumFactory(), false); 37836 composeEnumerationExtras("intent", element.getIntentElement(), new ReferralRequest.ReferralCategoryEnumFactory(), false); 37837 } 37838 if (element.hasType()) { 37839 composeCodeableConcept("type", element.getType()); 37840 } 37841 if (element.hasPriorityElement()) { 37842 composeEnumerationCore("priority", element.getPriorityElement(), new ReferralRequest.ReferralPriorityEnumFactory(), false); 37843 composeEnumerationExtras("priority", element.getPriorityElement(), new ReferralRequest.ReferralPriorityEnumFactory(), false); 37844 } 37845 if (element.hasServiceRequested()) { 37846 openArray("serviceRequested"); 37847 for (CodeableConcept e : element.getServiceRequested()) 37848 composeCodeableConcept(null, e); 37849 closeArray(); 37850 }; 37851 if (element.hasSubject()) { 37852 composeReference("subject", element.getSubject()); 37853 } 37854 if (element.hasContext()) { 37855 composeReference("context", element.getContext()); 37856 } 37857 if (element.hasOccurrence()) { 37858 composeType("occurrence", element.getOccurrence()); 37859 } 37860 if (element.hasAuthoredOnElement()) { 37861 composeDateTimeCore("authoredOn", element.getAuthoredOnElement(), false); 37862 composeDateTimeExtras("authoredOn", element.getAuthoredOnElement(), false); 37863 } 37864 if (element.hasRequester()) { 37865 composeReferralRequestReferralRequestRequesterComponent("requester", element.getRequester()); 37866 } 37867 if (element.hasSpecialty()) { 37868 composeCodeableConcept("specialty", element.getSpecialty()); 37869 } 37870 if (element.hasRecipient()) { 37871 openArray("recipient"); 37872 for (Reference e : element.getRecipient()) 37873 composeReference(null, e); 37874 closeArray(); 37875 }; 37876 if (element.hasReasonCode()) { 37877 openArray("reasonCode"); 37878 for (CodeableConcept e : element.getReasonCode()) 37879 composeCodeableConcept(null, e); 37880 closeArray(); 37881 }; 37882 if (element.hasReasonReference()) { 37883 openArray("reasonReference"); 37884 for (Reference e : element.getReasonReference()) 37885 composeReference(null, e); 37886 closeArray(); 37887 }; 37888 if (element.hasDescriptionElement()) { 37889 composeStringCore("description", element.getDescriptionElement(), false); 37890 composeStringExtras("description", element.getDescriptionElement(), false); 37891 } 37892 if (element.hasSupportingInfo()) { 37893 openArray("supportingInfo"); 37894 for (Reference e : element.getSupportingInfo()) 37895 composeReference(null, e); 37896 closeArray(); 37897 }; 37898 if (element.hasNote()) { 37899 openArray("note"); 37900 for (Annotation e : element.getNote()) 37901 composeAnnotation(null, e); 37902 closeArray(); 37903 }; 37904 if (element.hasRelevantHistory()) { 37905 openArray("relevantHistory"); 37906 for (Reference e : element.getRelevantHistory()) 37907 composeReference(null, e); 37908 closeArray(); 37909 }; 37910 } 37911 37912 protected void composeReferralRequestReferralRequestRequesterComponent(String name, ReferralRequest.ReferralRequestRequesterComponent element) throws IOException { 37913 if (element != null) { 37914 open(name); 37915 composeReferralRequestReferralRequestRequesterComponentInner(element); 37916 close(); 37917 } 37918 } 37919 37920 protected void composeReferralRequestReferralRequestRequesterComponentInner(ReferralRequest.ReferralRequestRequesterComponent element) throws IOException { 37921 composeBackbone(element); 37922 if (element.hasAgent()) { 37923 composeReference("agent", element.getAgent()); 37924 } 37925 if (element.hasOnBehalfOf()) { 37926 composeReference("onBehalfOf", element.getOnBehalfOf()); 37927 } 37928 } 37929 37930 protected void composeRelatedPerson(String name, RelatedPerson element) throws IOException { 37931 if (element != null) { 37932 prop("resourceType", name); 37933 composeRelatedPersonInner(element); 37934 } 37935 } 37936 37937 protected void composeRelatedPersonInner(RelatedPerson element) throws IOException { 37938 composeDomainResourceElements(element); 37939 if (element.hasIdentifier()) { 37940 openArray("identifier"); 37941 for (Identifier e : element.getIdentifier()) 37942 composeIdentifier(null, e); 37943 closeArray(); 37944 }; 37945 if (element.hasActiveElement()) { 37946 composeBooleanCore("active", element.getActiveElement(), false); 37947 composeBooleanExtras("active", element.getActiveElement(), false); 37948 } 37949 if (element.hasPatient()) { 37950 composeReference("patient", element.getPatient()); 37951 } 37952 if (element.hasRelationship()) { 37953 composeCodeableConcept("relationship", element.getRelationship()); 37954 } 37955 if (element.hasName()) { 37956 openArray("name"); 37957 for (HumanName e : element.getName()) 37958 composeHumanName(null, e); 37959 closeArray(); 37960 }; 37961 if (element.hasTelecom()) { 37962 openArray("telecom"); 37963 for (ContactPoint e : element.getTelecom()) 37964 composeContactPoint(null, e); 37965 closeArray(); 37966 }; 37967 if (element.hasGenderElement()) { 37968 composeEnumerationCore("gender", element.getGenderElement(), new Enumerations.AdministrativeGenderEnumFactory(), false); 37969 composeEnumerationExtras("gender", element.getGenderElement(), new Enumerations.AdministrativeGenderEnumFactory(), false); 37970 } 37971 if (element.hasBirthDateElement()) { 37972 composeDateCore("birthDate", element.getBirthDateElement(), false); 37973 composeDateExtras("birthDate", element.getBirthDateElement(), false); 37974 } 37975 if (element.hasAddress()) { 37976 openArray("address"); 37977 for (Address e : element.getAddress()) 37978 composeAddress(null, e); 37979 closeArray(); 37980 }; 37981 if (element.hasPhoto()) { 37982 openArray("photo"); 37983 for (Attachment e : element.getPhoto()) 37984 composeAttachment(null, e); 37985 closeArray(); 37986 }; 37987 if (element.hasPeriod()) { 37988 composePeriod("period", element.getPeriod()); 37989 } 37990 } 37991 37992 protected void composeRequestGroup(String name, RequestGroup element) throws IOException { 37993 if (element != null) { 37994 prop("resourceType", name); 37995 composeRequestGroupInner(element); 37996 } 37997 } 37998 37999 protected void composeRequestGroupInner(RequestGroup element) throws IOException { 38000 composeDomainResourceElements(element); 38001 if (element.hasIdentifier()) { 38002 openArray("identifier"); 38003 for (Identifier e : element.getIdentifier()) 38004 composeIdentifier(null, e); 38005 closeArray(); 38006 }; 38007 if (element.hasDefinition()) { 38008 openArray("definition"); 38009 for (Reference e : element.getDefinition()) 38010 composeReference(null, e); 38011 closeArray(); 38012 }; 38013 if (element.hasBasedOn()) { 38014 openArray("basedOn"); 38015 for (Reference e : element.getBasedOn()) 38016 composeReference(null, e); 38017 closeArray(); 38018 }; 38019 if (element.hasReplaces()) { 38020 openArray("replaces"); 38021 for (Reference e : element.getReplaces()) 38022 composeReference(null, e); 38023 closeArray(); 38024 }; 38025 if (element.hasGroupIdentifier()) { 38026 composeIdentifier("groupIdentifier", element.getGroupIdentifier()); 38027 } 38028 if (element.hasStatusElement()) { 38029 composeEnumerationCore("status", element.getStatusElement(), new RequestGroup.RequestStatusEnumFactory(), false); 38030 composeEnumerationExtras("status", element.getStatusElement(), new RequestGroup.RequestStatusEnumFactory(), false); 38031 } 38032 if (element.hasIntentElement()) { 38033 composeEnumerationCore("intent", element.getIntentElement(), new RequestGroup.RequestIntentEnumFactory(), false); 38034 composeEnumerationExtras("intent", element.getIntentElement(), new RequestGroup.RequestIntentEnumFactory(), false); 38035 } 38036 if (element.hasPriorityElement()) { 38037 composeEnumerationCore("priority", element.getPriorityElement(), new RequestGroup.RequestPriorityEnumFactory(), false); 38038 composeEnumerationExtras("priority", element.getPriorityElement(), new RequestGroup.RequestPriorityEnumFactory(), false); 38039 } 38040 if (element.hasSubject()) { 38041 composeReference("subject", element.getSubject()); 38042 } 38043 if (element.hasContext()) { 38044 composeReference("context", element.getContext()); 38045 } 38046 if (element.hasAuthoredOnElement()) { 38047 composeDateTimeCore("authoredOn", element.getAuthoredOnElement(), false); 38048 composeDateTimeExtras("authoredOn", element.getAuthoredOnElement(), false); 38049 } 38050 if (element.hasAuthor()) { 38051 composeReference("author", element.getAuthor()); 38052 } 38053 if (element.hasReason()) { 38054 composeType("reason", element.getReason()); 38055 } 38056 if (element.hasNote()) { 38057 openArray("note"); 38058 for (Annotation e : element.getNote()) 38059 composeAnnotation(null, e); 38060 closeArray(); 38061 }; 38062 if (element.hasAction()) { 38063 openArray("action"); 38064 for (RequestGroup.RequestGroupActionComponent e : element.getAction()) 38065 composeRequestGroupRequestGroupActionComponent(null, e); 38066 closeArray(); 38067 }; 38068 } 38069 38070 protected void composeRequestGroupRequestGroupActionComponent(String name, RequestGroup.RequestGroupActionComponent element) throws IOException { 38071 if (element != null) { 38072 open(name); 38073 composeRequestGroupRequestGroupActionComponentInner(element); 38074 close(); 38075 } 38076 } 38077 38078 protected void composeRequestGroupRequestGroupActionComponentInner(RequestGroup.RequestGroupActionComponent element) throws IOException { 38079 composeBackbone(element); 38080 if (element.hasLabelElement()) { 38081 composeStringCore("label", element.getLabelElement(), false); 38082 composeStringExtras("label", element.getLabelElement(), false); 38083 } 38084 if (element.hasTitleElement()) { 38085 composeStringCore("title", element.getTitleElement(), false); 38086 composeStringExtras("title", element.getTitleElement(), false); 38087 } 38088 if (element.hasDescriptionElement()) { 38089 composeStringCore("description", element.getDescriptionElement(), false); 38090 composeStringExtras("description", element.getDescriptionElement(), false); 38091 } 38092 if (element.hasTextEquivalentElement()) { 38093 composeStringCore("textEquivalent", element.getTextEquivalentElement(), false); 38094 composeStringExtras("textEquivalent", element.getTextEquivalentElement(), false); 38095 } 38096 if (element.hasCode()) { 38097 openArray("code"); 38098 for (CodeableConcept e : element.getCode()) 38099 composeCodeableConcept(null, e); 38100 closeArray(); 38101 }; 38102 if (element.hasDocumentation()) { 38103 openArray("documentation"); 38104 for (RelatedArtifact e : element.getDocumentation()) 38105 composeRelatedArtifact(null, e); 38106 closeArray(); 38107 }; 38108 if (element.hasCondition()) { 38109 openArray("condition"); 38110 for (RequestGroup.RequestGroupActionConditionComponent e : element.getCondition()) 38111 composeRequestGroupRequestGroupActionConditionComponent(null, e); 38112 closeArray(); 38113 }; 38114 if (element.hasRelatedAction()) { 38115 openArray("relatedAction"); 38116 for (RequestGroup.RequestGroupActionRelatedActionComponent e : element.getRelatedAction()) 38117 composeRequestGroupRequestGroupActionRelatedActionComponent(null, e); 38118 closeArray(); 38119 }; 38120 if (element.hasTiming()) { 38121 composeType("timing", element.getTiming()); 38122 } 38123 if (element.hasParticipant()) { 38124 openArray("participant"); 38125 for (Reference e : element.getParticipant()) 38126 composeReference(null, e); 38127 closeArray(); 38128 }; 38129 if (element.hasType()) { 38130 composeCoding("type", element.getType()); 38131 } 38132 if (element.hasGroupingBehaviorElement()) { 38133 composeEnumerationCore("groupingBehavior", element.getGroupingBehaviorElement(), new RequestGroup.ActionGroupingBehaviorEnumFactory(), false); 38134 composeEnumerationExtras("groupingBehavior", element.getGroupingBehaviorElement(), new RequestGroup.ActionGroupingBehaviorEnumFactory(), false); 38135 } 38136 if (element.hasSelectionBehaviorElement()) { 38137 composeEnumerationCore("selectionBehavior", element.getSelectionBehaviorElement(), new RequestGroup.ActionSelectionBehaviorEnumFactory(), false); 38138 composeEnumerationExtras("selectionBehavior", element.getSelectionBehaviorElement(), new RequestGroup.ActionSelectionBehaviorEnumFactory(), false); 38139 } 38140 if (element.hasRequiredBehaviorElement()) { 38141 composeEnumerationCore("requiredBehavior", element.getRequiredBehaviorElement(), new RequestGroup.ActionRequiredBehaviorEnumFactory(), false); 38142 composeEnumerationExtras("requiredBehavior", element.getRequiredBehaviorElement(), new RequestGroup.ActionRequiredBehaviorEnumFactory(), false); 38143 } 38144 if (element.hasPrecheckBehaviorElement()) { 38145 composeEnumerationCore("precheckBehavior", element.getPrecheckBehaviorElement(), new RequestGroup.ActionPrecheckBehaviorEnumFactory(), false); 38146 composeEnumerationExtras("precheckBehavior", element.getPrecheckBehaviorElement(), new RequestGroup.ActionPrecheckBehaviorEnumFactory(), false); 38147 } 38148 if (element.hasCardinalityBehaviorElement()) { 38149 composeEnumerationCore("cardinalityBehavior", element.getCardinalityBehaviorElement(), new RequestGroup.ActionCardinalityBehaviorEnumFactory(), false); 38150 composeEnumerationExtras("cardinalityBehavior", element.getCardinalityBehaviorElement(), new RequestGroup.ActionCardinalityBehaviorEnumFactory(), false); 38151 } 38152 if (element.hasResource()) { 38153 composeReference("resource", element.getResource()); 38154 } 38155 if (element.hasAction()) { 38156 openArray("action"); 38157 for (RequestGroup.RequestGroupActionComponent e : element.getAction()) 38158 composeRequestGroupRequestGroupActionComponent(null, e); 38159 closeArray(); 38160 }; 38161 } 38162 38163 protected void composeRequestGroupRequestGroupActionConditionComponent(String name, RequestGroup.RequestGroupActionConditionComponent element) throws IOException { 38164 if (element != null) { 38165 open(name); 38166 composeRequestGroupRequestGroupActionConditionComponentInner(element); 38167 close(); 38168 } 38169 } 38170 38171 protected void composeRequestGroupRequestGroupActionConditionComponentInner(RequestGroup.RequestGroupActionConditionComponent element) throws IOException { 38172 composeBackbone(element); 38173 if (element.hasKindElement()) { 38174 composeEnumerationCore("kind", element.getKindElement(), new RequestGroup.ActionConditionKindEnumFactory(), false); 38175 composeEnumerationExtras("kind", element.getKindElement(), new RequestGroup.ActionConditionKindEnumFactory(), false); 38176 } 38177 if (element.hasDescriptionElement()) { 38178 composeStringCore("description", element.getDescriptionElement(), false); 38179 composeStringExtras("description", element.getDescriptionElement(), false); 38180 } 38181 if (element.hasLanguageElement()) { 38182 composeStringCore("language", element.getLanguageElement(), false); 38183 composeStringExtras("language", element.getLanguageElement(), false); 38184 } 38185 if (element.hasExpressionElement()) { 38186 composeStringCore("expression", element.getExpressionElement(), false); 38187 composeStringExtras("expression", element.getExpressionElement(), false); 38188 } 38189 } 38190 38191 protected void composeRequestGroupRequestGroupActionRelatedActionComponent(String name, RequestGroup.RequestGroupActionRelatedActionComponent element) throws IOException { 38192 if (element != null) { 38193 open(name); 38194 composeRequestGroupRequestGroupActionRelatedActionComponentInner(element); 38195 close(); 38196 } 38197 } 38198 38199 protected void composeRequestGroupRequestGroupActionRelatedActionComponentInner(RequestGroup.RequestGroupActionRelatedActionComponent element) throws IOException { 38200 composeBackbone(element); 38201 if (element.hasActionIdElement()) { 38202 composeIdCore("actionId", element.getActionIdElement(), false); 38203 composeIdExtras("actionId", element.getActionIdElement(), false); 38204 } 38205 if (element.hasRelationshipElement()) { 38206 composeEnumerationCore("relationship", element.getRelationshipElement(), new RequestGroup.ActionRelationshipTypeEnumFactory(), false); 38207 composeEnumerationExtras("relationship", element.getRelationshipElement(), new RequestGroup.ActionRelationshipTypeEnumFactory(), false); 38208 } 38209 if (element.hasOffset()) { 38210 composeType("offset", element.getOffset()); 38211 } 38212 } 38213 38214 protected void composeResearchStudy(String name, ResearchStudy element) throws IOException { 38215 if (element != null) { 38216 prop("resourceType", name); 38217 composeResearchStudyInner(element); 38218 } 38219 } 38220 38221 protected void composeResearchStudyInner(ResearchStudy element) throws IOException { 38222 composeDomainResourceElements(element); 38223 if (element.hasIdentifier()) { 38224 openArray("identifier"); 38225 for (Identifier e : element.getIdentifier()) 38226 composeIdentifier(null, e); 38227 closeArray(); 38228 }; 38229 if (element.hasTitleElement()) { 38230 composeStringCore("title", element.getTitleElement(), false); 38231 composeStringExtras("title", element.getTitleElement(), false); 38232 } 38233 if (element.hasProtocol()) { 38234 openArray("protocol"); 38235 for (Reference e : element.getProtocol()) 38236 composeReference(null, e); 38237 closeArray(); 38238 }; 38239 if (element.hasPartOf()) { 38240 openArray("partOf"); 38241 for (Reference e : element.getPartOf()) 38242 composeReference(null, e); 38243 closeArray(); 38244 }; 38245 if (element.hasStatusElement()) { 38246 composeEnumerationCore("status", element.getStatusElement(), new ResearchStudy.ResearchStudyStatusEnumFactory(), false); 38247 composeEnumerationExtras("status", element.getStatusElement(), new ResearchStudy.ResearchStudyStatusEnumFactory(), false); 38248 } 38249 if (element.hasCategory()) { 38250 openArray("category"); 38251 for (CodeableConcept e : element.getCategory()) 38252 composeCodeableConcept(null, e); 38253 closeArray(); 38254 }; 38255 if (element.hasFocus()) { 38256 openArray("focus"); 38257 for (CodeableConcept e : element.getFocus()) 38258 composeCodeableConcept(null, e); 38259 closeArray(); 38260 }; 38261 if (element.hasContact()) { 38262 openArray("contact"); 38263 for (ContactDetail e : element.getContact()) 38264 composeContactDetail(null, e); 38265 closeArray(); 38266 }; 38267 if (element.hasRelatedArtifact()) { 38268 openArray("relatedArtifact"); 38269 for (RelatedArtifact e : element.getRelatedArtifact()) 38270 composeRelatedArtifact(null, e); 38271 closeArray(); 38272 }; 38273 if (element.hasKeyword()) { 38274 openArray("keyword"); 38275 for (CodeableConcept e : element.getKeyword()) 38276 composeCodeableConcept(null, e); 38277 closeArray(); 38278 }; 38279 if (element.hasJurisdiction()) { 38280 openArray("jurisdiction"); 38281 for (CodeableConcept e : element.getJurisdiction()) 38282 composeCodeableConcept(null, e); 38283 closeArray(); 38284 }; 38285 if (element.hasDescriptionElement()) { 38286 composeMarkdownCore("description", element.getDescriptionElement(), false); 38287 composeMarkdownExtras("description", element.getDescriptionElement(), false); 38288 } 38289 if (element.hasEnrollment()) { 38290 openArray("enrollment"); 38291 for (Reference e : element.getEnrollment()) 38292 composeReference(null, e); 38293 closeArray(); 38294 }; 38295 if (element.hasPeriod()) { 38296 composePeriod("period", element.getPeriod()); 38297 } 38298 if (element.hasSponsor()) { 38299 composeReference("sponsor", element.getSponsor()); 38300 } 38301 if (element.hasPrincipalInvestigator()) { 38302 composeReference("principalInvestigator", element.getPrincipalInvestigator()); 38303 } 38304 if (element.hasSite()) { 38305 openArray("site"); 38306 for (Reference e : element.getSite()) 38307 composeReference(null, e); 38308 closeArray(); 38309 }; 38310 if (element.hasReasonStopped()) { 38311 composeCodeableConcept("reasonStopped", element.getReasonStopped()); 38312 } 38313 if (element.hasNote()) { 38314 openArray("note"); 38315 for (Annotation e : element.getNote()) 38316 composeAnnotation(null, e); 38317 closeArray(); 38318 }; 38319 if (element.hasArm()) { 38320 openArray("arm"); 38321 for (ResearchStudy.ResearchStudyArmComponent e : element.getArm()) 38322 composeResearchStudyResearchStudyArmComponent(null, e); 38323 closeArray(); 38324 }; 38325 } 38326 38327 protected void composeResearchStudyResearchStudyArmComponent(String name, ResearchStudy.ResearchStudyArmComponent element) throws IOException { 38328 if (element != null) { 38329 open(name); 38330 composeResearchStudyResearchStudyArmComponentInner(element); 38331 close(); 38332 } 38333 } 38334 38335 protected void composeResearchStudyResearchStudyArmComponentInner(ResearchStudy.ResearchStudyArmComponent element) throws IOException { 38336 composeBackbone(element); 38337 if (element.hasNameElement()) { 38338 composeStringCore("name", element.getNameElement(), false); 38339 composeStringExtras("name", element.getNameElement(), false); 38340 } 38341 if (element.hasCode()) { 38342 composeCodeableConcept("code", element.getCode()); 38343 } 38344 if (element.hasDescriptionElement()) { 38345 composeStringCore("description", element.getDescriptionElement(), false); 38346 composeStringExtras("description", element.getDescriptionElement(), false); 38347 } 38348 } 38349 38350 protected void composeResearchSubject(String name, ResearchSubject element) throws IOException { 38351 if (element != null) { 38352 prop("resourceType", name); 38353 composeResearchSubjectInner(element); 38354 } 38355 } 38356 38357 protected void composeResearchSubjectInner(ResearchSubject element) throws IOException { 38358 composeDomainResourceElements(element); 38359 if (element.hasIdentifier()) { 38360 composeIdentifier("identifier", element.getIdentifier()); 38361 } 38362 if (element.hasStatusElement()) { 38363 composeEnumerationCore("status", element.getStatusElement(), new ResearchSubject.ResearchSubjectStatusEnumFactory(), false); 38364 composeEnumerationExtras("status", element.getStatusElement(), new ResearchSubject.ResearchSubjectStatusEnumFactory(), false); 38365 } 38366 if (element.hasPeriod()) { 38367 composePeriod("period", element.getPeriod()); 38368 } 38369 if (element.hasStudy()) { 38370 composeReference("study", element.getStudy()); 38371 } 38372 if (element.hasIndividual()) { 38373 composeReference("individual", element.getIndividual()); 38374 } 38375 if (element.hasAssignedArmElement()) { 38376 composeStringCore("assignedArm", element.getAssignedArmElement(), false); 38377 composeStringExtras("assignedArm", element.getAssignedArmElement(), false); 38378 } 38379 if (element.hasActualArmElement()) { 38380 composeStringCore("actualArm", element.getActualArmElement(), false); 38381 composeStringExtras("actualArm", element.getActualArmElement(), false); 38382 } 38383 if (element.hasConsent()) { 38384 composeReference("consent", element.getConsent()); 38385 } 38386 } 38387 38388 protected void composeRiskAssessment(String name, RiskAssessment element) throws IOException { 38389 if (element != null) { 38390 prop("resourceType", name); 38391 composeRiskAssessmentInner(element); 38392 } 38393 } 38394 38395 protected void composeRiskAssessmentInner(RiskAssessment element) throws IOException { 38396 composeDomainResourceElements(element); 38397 if (element.hasIdentifier()) { 38398 composeIdentifier("identifier", element.getIdentifier()); 38399 } 38400 if (element.hasBasedOn()) { 38401 composeReference("basedOn", element.getBasedOn()); 38402 } 38403 if (element.hasParent()) { 38404 composeReference("parent", element.getParent()); 38405 } 38406 if (element.hasStatusElement()) { 38407 composeEnumerationCore("status", element.getStatusElement(), new RiskAssessment.RiskAssessmentStatusEnumFactory(), false); 38408 composeEnumerationExtras("status", element.getStatusElement(), new RiskAssessment.RiskAssessmentStatusEnumFactory(), false); 38409 } 38410 if (element.hasMethod()) { 38411 composeCodeableConcept("method", element.getMethod()); 38412 } 38413 if (element.hasCode()) { 38414 composeCodeableConcept("code", element.getCode()); 38415 } 38416 if (element.hasSubject()) { 38417 composeReference("subject", element.getSubject()); 38418 } 38419 if (element.hasContext()) { 38420 composeReference("context", element.getContext()); 38421 } 38422 if (element.hasOccurrence()) { 38423 composeType("occurrence", element.getOccurrence()); 38424 } 38425 if (element.hasCondition()) { 38426 composeReference("condition", element.getCondition()); 38427 } 38428 if (element.hasPerformer()) { 38429 composeReference("performer", element.getPerformer()); 38430 } 38431 if (element.hasReason()) { 38432 composeType("reason", element.getReason()); 38433 } 38434 if (element.hasBasis()) { 38435 openArray("basis"); 38436 for (Reference e : element.getBasis()) 38437 composeReference(null, e); 38438 closeArray(); 38439 }; 38440 if (element.hasPrediction()) { 38441 openArray("prediction"); 38442 for (RiskAssessment.RiskAssessmentPredictionComponent e : element.getPrediction()) 38443 composeRiskAssessmentRiskAssessmentPredictionComponent(null, e); 38444 closeArray(); 38445 }; 38446 if (element.hasMitigationElement()) { 38447 composeStringCore("mitigation", element.getMitigationElement(), false); 38448 composeStringExtras("mitigation", element.getMitigationElement(), false); 38449 } 38450 if (element.hasCommentElement()) { 38451 composeStringCore("comment", element.getCommentElement(), false); 38452 composeStringExtras("comment", element.getCommentElement(), false); 38453 } 38454 } 38455 38456 protected void composeRiskAssessmentRiskAssessmentPredictionComponent(String name, RiskAssessment.RiskAssessmentPredictionComponent element) throws IOException { 38457 if (element != null) { 38458 open(name); 38459 composeRiskAssessmentRiskAssessmentPredictionComponentInner(element); 38460 close(); 38461 } 38462 } 38463 38464 protected void composeRiskAssessmentRiskAssessmentPredictionComponentInner(RiskAssessment.RiskAssessmentPredictionComponent element) throws IOException { 38465 composeBackbone(element); 38466 if (element.hasOutcome()) { 38467 composeCodeableConcept("outcome", element.getOutcome()); 38468 } 38469 if (element.hasProbability()) { 38470 composeType("probability", element.getProbability()); 38471 } 38472 if (element.hasQualitativeRisk()) { 38473 composeCodeableConcept("qualitativeRisk", element.getQualitativeRisk()); 38474 } 38475 if (element.hasRelativeRiskElement()) { 38476 composeDecimalCore("relativeRisk", element.getRelativeRiskElement(), false); 38477 composeDecimalExtras("relativeRisk", element.getRelativeRiskElement(), false); 38478 } 38479 if (element.hasWhen()) { 38480 composeType("when", element.getWhen()); 38481 } 38482 if (element.hasRationaleElement()) { 38483 composeStringCore("rationale", element.getRationaleElement(), false); 38484 composeStringExtras("rationale", element.getRationaleElement(), false); 38485 } 38486 } 38487 38488 protected void composeSchedule(String name, Schedule element) throws IOException { 38489 if (element != null) { 38490 prop("resourceType", name); 38491 composeScheduleInner(element); 38492 } 38493 } 38494 38495 protected void composeScheduleInner(Schedule element) throws IOException { 38496 composeDomainResourceElements(element); 38497 if (element.hasIdentifier()) { 38498 openArray("identifier"); 38499 for (Identifier e : element.getIdentifier()) 38500 composeIdentifier(null, e); 38501 closeArray(); 38502 }; 38503 if (element.hasActiveElement()) { 38504 composeBooleanCore("active", element.getActiveElement(), false); 38505 composeBooleanExtras("active", element.getActiveElement(), false); 38506 } 38507 if (element.hasServiceCategory()) { 38508 composeCodeableConcept("serviceCategory", element.getServiceCategory()); 38509 } 38510 if (element.hasServiceType()) { 38511 openArray("serviceType"); 38512 for (CodeableConcept e : element.getServiceType()) 38513 composeCodeableConcept(null, e); 38514 closeArray(); 38515 }; 38516 if (element.hasSpecialty()) { 38517 openArray("specialty"); 38518 for (CodeableConcept e : element.getSpecialty()) 38519 composeCodeableConcept(null, e); 38520 closeArray(); 38521 }; 38522 if (element.hasActor()) { 38523 openArray("actor"); 38524 for (Reference e : element.getActor()) 38525 composeReference(null, e); 38526 closeArray(); 38527 }; 38528 if (element.hasPlanningHorizon()) { 38529 composePeriod("planningHorizon", element.getPlanningHorizon()); 38530 } 38531 if (element.hasCommentElement()) { 38532 composeStringCore("comment", element.getCommentElement(), false); 38533 composeStringExtras("comment", element.getCommentElement(), false); 38534 } 38535 } 38536 38537 protected void composeSearchParameter(String name, SearchParameter element) throws IOException { 38538 if (element != null) { 38539 prop("resourceType", name); 38540 composeSearchParameterInner(element); 38541 } 38542 } 38543 38544 protected void composeSearchParameterInner(SearchParameter element) throws IOException { 38545 composeDomainResourceElements(element); 38546 if (element.hasUrlElement()) { 38547 composeUriCore("url", element.getUrlElement(), false); 38548 composeUriExtras("url", element.getUrlElement(), false); 38549 } 38550 if (element.hasVersionElement()) { 38551 composeStringCore("version", element.getVersionElement(), false); 38552 composeStringExtras("version", element.getVersionElement(), false); 38553 } 38554 if (element.hasNameElement()) { 38555 composeStringCore("name", element.getNameElement(), false); 38556 composeStringExtras("name", element.getNameElement(), false); 38557 } 38558 if (element.hasStatusElement()) { 38559 composeEnumerationCore("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 38560 composeEnumerationExtras("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 38561 } 38562 if (element.hasExperimentalElement()) { 38563 composeBooleanCore("experimental", element.getExperimentalElement(), false); 38564 composeBooleanExtras("experimental", element.getExperimentalElement(), false); 38565 } 38566 if (element.hasDateElement()) { 38567 composeDateTimeCore("date", element.getDateElement(), false); 38568 composeDateTimeExtras("date", element.getDateElement(), false); 38569 } 38570 if (element.hasPublisherElement()) { 38571 composeStringCore("publisher", element.getPublisherElement(), false); 38572 composeStringExtras("publisher", element.getPublisherElement(), false); 38573 } 38574 if (element.hasContact()) { 38575 openArray("contact"); 38576 for (ContactDetail e : element.getContact()) 38577 composeContactDetail(null, e); 38578 closeArray(); 38579 }; 38580 if (element.hasUseContext()) { 38581 openArray("useContext"); 38582 for (UsageContext e : element.getUseContext()) 38583 composeUsageContext(null, e); 38584 closeArray(); 38585 }; 38586 if (element.hasJurisdiction()) { 38587 openArray("jurisdiction"); 38588 for (CodeableConcept e : element.getJurisdiction()) 38589 composeCodeableConcept(null, e); 38590 closeArray(); 38591 }; 38592 if (element.hasPurposeElement()) { 38593 composeMarkdownCore("purpose", element.getPurposeElement(), false); 38594 composeMarkdownExtras("purpose", element.getPurposeElement(), false); 38595 } 38596 if (element.hasCodeElement()) { 38597 composeCodeCore("code", element.getCodeElement(), false); 38598 composeCodeExtras("code", element.getCodeElement(), false); 38599 } 38600 if (element.hasBase()) { 38601 openArray("base"); 38602 for (CodeType e : element.getBase()) 38603 composeCodeCore(null, e, true); 38604 closeArray(); 38605 if (anyHasExtras(element.getBase())) { 38606 openArray("_base"); 38607 for (CodeType e : element.getBase()) 38608 composeCodeExtras(null, e, true); 38609 closeArray(); 38610 } 38611 }; 38612 if (element.hasTypeElement()) { 38613 composeEnumerationCore("type", element.getTypeElement(), new Enumerations.SearchParamTypeEnumFactory(), false); 38614 composeEnumerationExtras("type", element.getTypeElement(), new Enumerations.SearchParamTypeEnumFactory(), false); 38615 } 38616 if (element.hasDerivedFromElement()) { 38617 composeUriCore("derivedFrom", element.getDerivedFromElement(), false); 38618 composeUriExtras("derivedFrom", element.getDerivedFromElement(), false); 38619 } 38620 if (element.hasDescriptionElement()) { 38621 composeMarkdownCore("description", element.getDescriptionElement(), false); 38622 composeMarkdownExtras("description", element.getDescriptionElement(), false); 38623 } 38624 if (element.hasExpressionElement()) { 38625 composeStringCore("expression", element.getExpressionElement(), false); 38626 composeStringExtras("expression", element.getExpressionElement(), false); 38627 } 38628 if (element.hasXpathElement()) { 38629 composeStringCore("xpath", element.getXpathElement(), false); 38630 composeStringExtras("xpath", element.getXpathElement(), false); 38631 } 38632 if (element.hasXpathUsageElement()) { 38633 composeEnumerationCore("xpathUsage", element.getXpathUsageElement(), new SearchParameter.XPathUsageTypeEnumFactory(), false); 38634 composeEnumerationExtras("xpathUsage", element.getXpathUsageElement(), new SearchParameter.XPathUsageTypeEnumFactory(), false); 38635 } 38636 if (element.hasTarget()) { 38637 openArray("target"); 38638 for (CodeType e : element.getTarget()) 38639 composeCodeCore(null, e, true); 38640 closeArray(); 38641 if (anyHasExtras(element.getTarget())) { 38642 openArray("_target"); 38643 for (CodeType e : element.getTarget()) 38644 composeCodeExtras(null, e, true); 38645 closeArray(); 38646 } 38647 }; 38648 if (element.hasComparator()) { 38649 openArray("comparator"); 38650 for (Enumeration<SearchParameter.SearchComparator> e : element.getComparator()) 38651 composeEnumerationCore(null, e, new SearchParameter.SearchComparatorEnumFactory(), true); 38652 closeArray(); 38653 if (anyHasExtras(element.getComparator())) { 38654 openArray("_comparator"); 38655 for (Enumeration<SearchParameter.SearchComparator> e : element.getComparator()) 38656 composeEnumerationExtras(null, e, new SearchParameter.SearchComparatorEnumFactory(), true); 38657 closeArray(); 38658 } 38659 }; 38660 if (element.hasModifier()) { 38661 openArray("modifier"); 38662 for (Enumeration<SearchParameter.SearchModifierCode> e : element.getModifier()) 38663 composeEnumerationCore(null, e, new SearchParameter.SearchModifierCodeEnumFactory(), true); 38664 closeArray(); 38665 if (anyHasExtras(element.getModifier())) { 38666 openArray("_modifier"); 38667 for (Enumeration<SearchParameter.SearchModifierCode> e : element.getModifier()) 38668 composeEnumerationExtras(null, e, new SearchParameter.SearchModifierCodeEnumFactory(), true); 38669 closeArray(); 38670 } 38671 }; 38672 if (element.hasChain()) { 38673 openArray("chain"); 38674 for (StringType e : element.getChain()) 38675 composeStringCore(null, e, true); 38676 closeArray(); 38677 if (anyHasExtras(element.getChain())) { 38678 openArray("_chain"); 38679 for (StringType e : element.getChain()) 38680 composeStringExtras(null, e, true); 38681 closeArray(); 38682 } 38683 }; 38684 if (element.hasComponent()) { 38685 openArray("component"); 38686 for (SearchParameter.SearchParameterComponentComponent e : element.getComponent()) 38687 composeSearchParameterSearchParameterComponentComponent(null, e); 38688 closeArray(); 38689 }; 38690 } 38691 38692 protected void composeSearchParameterSearchParameterComponentComponent(String name, SearchParameter.SearchParameterComponentComponent element) throws IOException { 38693 if (element != null) { 38694 open(name); 38695 composeSearchParameterSearchParameterComponentComponentInner(element); 38696 close(); 38697 } 38698 } 38699 38700 protected void composeSearchParameterSearchParameterComponentComponentInner(SearchParameter.SearchParameterComponentComponent element) throws IOException { 38701 composeBackbone(element); 38702 if (element.hasDefinition()) { 38703 composeReference("definition", element.getDefinition()); 38704 } 38705 if (element.hasExpressionElement()) { 38706 composeStringCore("expression", element.getExpressionElement(), false); 38707 composeStringExtras("expression", element.getExpressionElement(), false); 38708 } 38709 } 38710 38711 protected void composeSequence(String name, Sequence element) throws IOException { 38712 if (element != null) { 38713 prop("resourceType", name); 38714 composeSequenceInner(element); 38715 } 38716 } 38717 38718 protected void composeSequenceInner(Sequence element) throws IOException { 38719 composeDomainResourceElements(element); 38720 if (element.hasIdentifier()) { 38721 openArray("identifier"); 38722 for (Identifier e : element.getIdentifier()) 38723 composeIdentifier(null, e); 38724 closeArray(); 38725 }; 38726 if (element.hasTypeElement()) { 38727 composeEnumerationCore("type", element.getTypeElement(), new Sequence.SequenceTypeEnumFactory(), false); 38728 composeEnumerationExtras("type", element.getTypeElement(), new Sequence.SequenceTypeEnumFactory(), false); 38729 } 38730 if (element.hasCoordinateSystemElement()) { 38731 composeIntegerCore("coordinateSystem", element.getCoordinateSystemElement(), false); 38732 composeIntegerExtras("coordinateSystem", element.getCoordinateSystemElement(), false); 38733 } 38734 if (element.hasPatient()) { 38735 composeReference("patient", element.getPatient()); 38736 } 38737 if (element.hasSpecimen()) { 38738 composeReference("specimen", element.getSpecimen()); 38739 } 38740 if (element.hasDevice()) { 38741 composeReference("device", element.getDevice()); 38742 } 38743 if (element.hasPerformer()) { 38744 composeReference("performer", element.getPerformer()); 38745 } 38746 if (element.hasQuantity()) { 38747 composeQuantity("quantity", element.getQuantity()); 38748 } 38749 if (element.hasReferenceSeq()) { 38750 composeSequenceSequenceReferenceSeqComponent("referenceSeq", element.getReferenceSeq()); 38751 } 38752 if (element.hasVariant()) { 38753 openArray("variant"); 38754 for (Sequence.SequenceVariantComponent e : element.getVariant()) 38755 composeSequenceSequenceVariantComponent(null, e); 38756 closeArray(); 38757 }; 38758 if (element.hasObservedSeqElement()) { 38759 composeStringCore("observedSeq", element.getObservedSeqElement(), false); 38760 composeStringExtras("observedSeq", element.getObservedSeqElement(), false); 38761 } 38762 if (element.hasQuality()) { 38763 openArray("quality"); 38764 for (Sequence.SequenceQualityComponent e : element.getQuality()) 38765 composeSequenceSequenceQualityComponent(null, e); 38766 closeArray(); 38767 }; 38768 if (element.hasReadCoverageElement()) { 38769 composeIntegerCore("readCoverage", element.getReadCoverageElement(), false); 38770 composeIntegerExtras("readCoverage", element.getReadCoverageElement(), false); 38771 } 38772 if (element.hasRepository()) { 38773 openArray("repository"); 38774 for (Sequence.SequenceRepositoryComponent e : element.getRepository()) 38775 composeSequenceSequenceRepositoryComponent(null, e); 38776 closeArray(); 38777 }; 38778 if (element.hasPointer()) { 38779 openArray("pointer"); 38780 for (Reference e : element.getPointer()) 38781 composeReference(null, e); 38782 closeArray(); 38783 }; 38784 } 38785 38786 protected void composeSequenceSequenceReferenceSeqComponent(String name, Sequence.SequenceReferenceSeqComponent element) throws IOException { 38787 if (element != null) { 38788 open(name); 38789 composeSequenceSequenceReferenceSeqComponentInner(element); 38790 close(); 38791 } 38792 } 38793 38794 protected void composeSequenceSequenceReferenceSeqComponentInner(Sequence.SequenceReferenceSeqComponent element) throws IOException { 38795 composeBackbone(element); 38796 if (element.hasChromosome()) { 38797 composeCodeableConcept("chromosome", element.getChromosome()); 38798 } 38799 if (element.hasGenomeBuildElement()) { 38800 composeStringCore("genomeBuild", element.getGenomeBuildElement(), false); 38801 composeStringExtras("genomeBuild", element.getGenomeBuildElement(), false); 38802 } 38803 if (element.hasReferenceSeqId()) { 38804 composeCodeableConcept("referenceSeqId", element.getReferenceSeqId()); 38805 } 38806 if (element.hasReferenceSeqPointer()) { 38807 composeReference("referenceSeqPointer", element.getReferenceSeqPointer()); 38808 } 38809 if (element.hasReferenceSeqStringElement()) { 38810 composeStringCore("referenceSeqString", element.getReferenceSeqStringElement(), false); 38811 composeStringExtras("referenceSeqString", element.getReferenceSeqStringElement(), false); 38812 } 38813 if (element.hasStrandElement()) { 38814 composeIntegerCore("strand", element.getStrandElement(), false); 38815 composeIntegerExtras("strand", element.getStrandElement(), false); 38816 } 38817 if (element.hasWindowStartElement()) { 38818 composeIntegerCore("windowStart", element.getWindowStartElement(), false); 38819 composeIntegerExtras("windowStart", element.getWindowStartElement(), false); 38820 } 38821 if (element.hasWindowEndElement()) { 38822 composeIntegerCore("windowEnd", element.getWindowEndElement(), false); 38823 composeIntegerExtras("windowEnd", element.getWindowEndElement(), false); 38824 } 38825 } 38826 38827 protected void composeSequenceSequenceVariantComponent(String name, Sequence.SequenceVariantComponent element) throws IOException { 38828 if (element != null) { 38829 open(name); 38830 composeSequenceSequenceVariantComponentInner(element); 38831 close(); 38832 } 38833 } 38834 38835 protected void composeSequenceSequenceVariantComponentInner(Sequence.SequenceVariantComponent element) throws IOException { 38836 composeBackbone(element); 38837 if (element.hasStartElement()) { 38838 composeIntegerCore("start", element.getStartElement(), false); 38839 composeIntegerExtras("start", element.getStartElement(), false); 38840 } 38841 if (element.hasEndElement()) { 38842 composeIntegerCore("end", element.getEndElement(), false); 38843 composeIntegerExtras("end", element.getEndElement(), false); 38844 } 38845 if (element.hasObservedAlleleElement()) { 38846 composeStringCore("observedAllele", element.getObservedAlleleElement(), false); 38847 composeStringExtras("observedAllele", element.getObservedAlleleElement(), false); 38848 } 38849 if (element.hasReferenceAlleleElement()) { 38850 composeStringCore("referenceAllele", element.getReferenceAlleleElement(), false); 38851 composeStringExtras("referenceAllele", element.getReferenceAlleleElement(), false); 38852 } 38853 if (element.hasCigarElement()) { 38854 composeStringCore("cigar", element.getCigarElement(), false); 38855 composeStringExtras("cigar", element.getCigarElement(), false); 38856 } 38857 if (element.hasVariantPointer()) { 38858 composeReference("variantPointer", element.getVariantPointer()); 38859 } 38860 } 38861 38862 protected void composeSequenceSequenceQualityComponent(String name, Sequence.SequenceQualityComponent element) throws IOException { 38863 if (element != null) { 38864 open(name); 38865 composeSequenceSequenceQualityComponentInner(element); 38866 close(); 38867 } 38868 } 38869 38870 protected void composeSequenceSequenceQualityComponentInner(Sequence.SequenceQualityComponent element) throws IOException { 38871 composeBackbone(element); 38872 if (element.hasTypeElement()) { 38873 composeEnumerationCore("type", element.getTypeElement(), new Sequence.QualityTypeEnumFactory(), false); 38874 composeEnumerationExtras("type", element.getTypeElement(), new Sequence.QualityTypeEnumFactory(), false); 38875 } 38876 if (element.hasStandardSequence()) { 38877 composeCodeableConcept("standardSequence", element.getStandardSequence()); 38878 } 38879 if (element.hasStartElement()) { 38880 composeIntegerCore("start", element.getStartElement(), false); 38881 composeIntegerExtras("start", element.getStartElement(), false); 38882 } 38883 if (element.hasEndElement()) { 38884 composeIntegerCore("end", element.getEndElement(), false); 38885 composeIntegerExtras("end", element.getEndElement(), false); 38886 } 38887 if (element.hasScore()) { 38888 composeQuantity("score", element.getScore()); 38889 } 38890 if (element.hasMethod()) { 38891 composeCodeableConcept("method", element.getMethod()); 38892 } 38893 if (element.hasTruthTPElement()) { 38894 composeDecimalCore("truthTP", element.getTruthTPElement(), false); 38895 composeDecimalExtras("truthTP", element.getTruthTPElement(), false); 38896 } 38897 if (element.hasQueryTPElement()) { 38898 composeDecimalCore("queryTP", element.getQueryTPElement(), false); 38899 composeDecimalExtras("queryTP", element.getQueryTPElement(), false); 38900 } 38901 if (element.hasTruthFNElement()) { 38902 composeDecimalCore("truthFN", element.getTruthFNElement(), false); 38903 composeDecimalExtras("truthFN", element.getTruthFNElement(), false); 38904 } 38905 if (element.hasQueryFPElement()) { 38906 composeDecimalCore("queryFP", element.getQueryFPElement(), false); 38907 composeDecimalExtras("queryFP", element.getQueryFPElement(), false); 38908 } 38909 if (element.hasGtFPElement()) { 38910 composeDecimalCore("gtFP", element.getGtFPElement(), false); 38911 composeDecimalExtras("gtFP", element.getGtFPElement(), false); 38912 } 38913 if (element.hasPrecisionElement()) { 38914 composeDecimalCore("precision", element.getPrecisionElement(), false); 38915 composeDecimalExtras("precision", element.getPrecisionElement(), false); 38916 } 38917 if (element.hasRecallElement()) { 38918 composeDecimalCore("recall", element.getRecallElement(), false); 38919 composeDecimalExtras("recall", element.getRecallElement(), false); 38920 } 38921 if (element.hasFScoreElement()) { 38922 composeDecimalCore("fScore", element.getFScoreElement(), false); 38923 composeDecimalExtras("fScore", element.getFScoreElement(), false); 38924 } 38925 } 38926 38927 protected void composeSequenceSequenceRepositoryComponent(String name, Sequence.SequenceRepositoryComponent element) throws IOException { 38928 if (element != null) { 38929 open(name); 38930 composeSequenceSequenceRepositoryComponentInner(element); 38931 close(); 38932 } 38933 } 38934 38935 protected void composeSequenceSequenceRepositoryComponentInner(Sequence.SequenceRepositoryComponent element) throws IOException { 38936 composeBackbone(element); 38937 if (element.hasTypeElement()) { 38938 composeEnumerationCore("type", element.getTypeElement(), new Sequence.RepositoryTypeEnumFactory(), false); 38939 composeEnumerationExtras("type", element.getTypeElement(), new Sequence.RepositoryTypeEnumFactory(), false); 38940 } 38941 if (element.hasUrlElement()) { 38942 composeUriCore("url", element.getUrlElement(), false); 38943 composeUriExtras("url", element.getUrlElement(), false); 38944 } 38945 if (element.hasNameElement()) { 38946 composeStringCore("name", element.getNameElement(), false); 38947 composeStringExtras("name", element.getNameElement(), false); 38948 } 38949 if (element.hasDatasetIdElement()) { 38950 composeStringCore("datasetId", element.getDatasetIdElement(), false); 38951 composeStringExtras("datasetId", element.getDatasetIdElement(), false); 38952 } 38953 if (element.hasVariantsetIdElement()) { 38954 composeStringCore("variantsetId", element.getVariantsetIdElement(), false); 38955 composeStringExtras("variantsetId", element.getVariantsetIdElement(), false); 38956 } 38957 if (element.hasReadsetIdElement()) { 38958 composeStringCore("readsetId", element.getReadsetIdElement(), false); 38959 composeStringExtras("readsetId", element.getReadsetIdElement(), false); 38960 } 38961 } 38962 38963 protected void composeServiceDefinition(String name, ServiceDefinition element) throws IOException { 38964 if (element != null) { 38965 prop("resourceType", name); 38966 composeServiceDefinitionInner(element); 38967 } 38968 } 38969 38970 protected void composeServiceDefinitionInner(ServiceDefinition element) throws IOException { 38971 composeDomainResourceElements(element); 38972 if (element.hasUrlElement()) { 38973 composeUriCore("url", element.getUrlElement(), false); 38974 composeUriExtras("url", element.getUrlElement(), false); 38975 } 38976 if (element.hasIdentifier()) { 38977 openArray("identifier"); 38978 for (Identifier e : element.getIdentifier()) 38979 composeIdentifier(null, e); 38980 closeArray(); 38981 }; 38982 if (element.hasVersionElement()) { 38983 composeStringCore("version", element.getVersionElement(), false); 38984 composeStringExtras("version", element.getVersionElement(), false); 38985 } 38986 if (element.hasNameElement()) { 38987 composeStringCore("name", element.getNameElement(), false); 38988 composeStringExtras("name", element.getNameElement(), false); 38989 } 38990 if (element.hasTitleElement()) { 38991 composeStringCore("title", element.getTitleElement(), false); 38992 composeStringExtras("title", element.getTitleElement(), false); 38993 } 38994 if (element.hasStatusElement()) { 38995 composeEnumerationCore("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 38996 composeEnumerationExtras("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 38997 } 38998 if (element.hasExperimentalElement()) { 38999 composeBooleanCore("experimental", element.getExperimentalElement(), false); 39000 composeBooleanExtras("experimental", element.getExperimentalElement(), false); 39001 } 39002 if (element.hasDateElement()) { 39003 composeDateTimeCore("date", element.getDateElement(), false); 39004 composeDateTimeExtras("date", element.getDateElement(), false); 39005 } 39006 if (element.hasPublisherElement()) { 39007 composeStringCore("publisher", element.getPublisherElement(), false); 39008 composeStringExtras("publisher", element.getPublisherElement(), false); 39009 } 39010 if (element.hasDescriptionElement()) { 39011 composeMarkdownCore("description", element.getDescriptionElement(), false); 39012 composeMarkdownExtras("description", element.getDescriptionElement(), false); 39013 } 39014 if (element.hasPurposeElement()) { 39015 composeMarkdownCore("purpose", element.getPurposeElement(), false); 39016 composeMarkdownExtras("purpose", element.getPurposeElement(), false); 39017 } 39018 if (element.hasUsageElement()) { 39019 composeStringCore("usage", element.getUsageElement(), false); 39020 composeStringExtras("usage", element.getUsageElement(), false); 39021 } 39022 if (element.hasApprovalDateElement()) { 39023 composeDateCore("approvalDate", element.getApprovalDateElement(), false); 39024 composeDateExtras("approvalDate", element.getApprovalDateElement(), false); 39025 } 39026 if (element.hasLastReviewDateElement()) { 39027 composeDateCore("lastReviewDate", element.getLastReviewDateElement(), false); 39028 composeDateExtras("lastReviewDate", element.getLastReviewDateElement(), false); 39029 } 39030 if (element.hasEffectivePeriod()) { 39031 composePeriod("effectivePeriod", element.getEffectivePeriod()); 39032 } 39033 if (element.hasUseContext()) { 39034 openArray("useContext"); 39035 for (UsageContext e : element.getUseContext()) 39036 composeUsageContext(null, e); 39037 closeArray(); 39038 }; 39039 if (element.hasJurisdiction()) { 39040 openArray("jurisdiction"); 39041 for (CodeableConcept e : element.getJurisdiction()) 39042 composeCodeableConcept(null, e); 39043 closeArray(); 39044 }; 39045 if (element.hasTopic()) { 39046 openArray("topic"); 39047 for (CodeableConcept e : element.getTopic()) 39048 composeCodeableConcept(null, e); 39049 closeArray(); 39050 }; 39051 if (element.hasContributor()) { 39052 openArray("contributor"); 39053 for (Contributor e : element.getContributor()) 39054 composeContributor(null, e); 39055 closeArray(); 39056 }; 39057 if (element.hasContact()) { 39058 openArray("contact"); 39059 for (ContactDetail e : element.getContact()) 39060 composeContactDetail(null, e); 39061 closeArray(); 39062 }; 39063 if (element.hasCopyrightElement()) { 39064 composeMarkdownCore("copyright", element.getCopyrightElement(), false); 39065 composeMarkdownExtras("copyright", element.getCopyrightElement(), false); 39066 } 39067 if (element.hasRelatedArtifact()) { 39068 openArray("relatedArtifact"); 39069 for (RelatedArtifact e : element.getRelatedArtifact()) 39070 composeRelatedArtifact(null, e); 39071 closeArray(); 39072 }; 39073 if (element.hasTrigger()) { 39074 openArray("trigger"); 39075 for (TriggerDefinition e : element.getTrigger()) 39076 composeTriggerDefinition(null, e); 39077 closeArray(); 39078 }; 39079 if (element.hasDataRequirement()) { 39080 openArray("dataRequirement"); 39081 for (DataRequirement e : element.getDataRequirement()) 39082 composeDataRequirement(null, e); 39083 closeArray(); 39084 }; 39085 if (element.hasOperationDefinition()) { 39086 composeReference("operationDefinition", element.getOperationDefinition()); 39087 } 39088 } 39089 39090 protected void composeSlot(String name, Slot element) throws IOException { 39091 if (element != null) { 39092 prop("resourceType", name); 39093 composeSlotInner(element); 39094 } 39095 } 39096 39097 protected void composeSlotInner(Slot element) throws IOException { 39098 composeDomainResourceElements(element); 39099 if (element.hasIdentifier()) { 39100 openArray("identifier"); 39101 for (Identifier e : element.getIdentifier()) 39102 composeIdentifier(null, e); 39103 closeArray(); 39104 }; 39105 if (element.hasServiceCategory()) { 39106 composeCodeableConcept("serviceCategory", element.getServiceCategory()); 39107 } 39108 if (element.hasServiceType()) { 39109 openArray("serviceType"); 39110 for (CodeableConcept e : element.getServiceType()) 39111 composeCodeableConcept(null, e); 39112 closeArray(); 39113 }; 39114 if (element.hasSpecialty()) { 39115 openArray("specialty"); 39116 for (CodeableConcept e : element.getSpecialty()) 39117 composeCodeableConcept(null, e); 39118 closeArray(); 39119 }; 39120 if (element.hasAppointmentType()) { 39121 composeCodeableConcept("appointmentType", element.getAppointmentType()); 39122 } 39123 if (element.hasSchedule()) { 39124 composeReference("schedule", element.getSchedule()); 39125 } 39126 if (element.hasStatusElement()) { 39127 composeEnumerationCore("status", element.getStatusElement(), new Slot.SlotStatusEnumFactory(), false); 39128 composeEnumerationExtras("status", element.getStatusElement(), new Slot.SlotStatusEnumFactory(), false); 39129 } 39130 if (element.hasStartElement()) { 39131 composeInstantCore("start", element.getStartElement(), false); 39132 composeInstantExtras("start", element.getStartElement(), false); 39133 } 39134 if (element.hasEndElement()) { 39135 composeInstantCore("end", element.getEndElement(), false); 39136 composeInstantExtras("end", element.getEndElement(), false); 39137 } 39138 if (element.hasOverbookedElement()) { 39139 composeBooleanCore("overbooked", element.getOverbookedElement(), false); 39140 composeBooleanExtras("overbooked", element.getOverbookedElement(), false); 39141 } 39142 if (element.hasCommentElement()) { 39143 composeStringCore("comment", element.getCommentElement(), false); 39144 composeStringExtras("comment", element.getCommentElement(), false); 39145 } 39146 } 39147 39148 protected void composeSpecimen(String name, Specimen element) throws IOException { 39149 if (element != null) { 39150 prop("resourceType", name); 39151 composeSpecimenInner(element); 39152 } 39153 } 39154 39155 protected void composeSpecimenInner(Specimen element) throws IOException { 39156 composeDomainResourceElements(element); 39157 if (element.hasIdentifier()) { 39158 openArray("identifier"); 39159 for (Identifier e : element.getIdentifier()) 39160 composeIdentifier(null, e); 39161 closeArray(); 39162 }; 39163 if (element.hasAccessionIdentifier()) { 39164 composeIdentifier("accessionIdentifier", element.getAccessionIdentifier()); 39165 } 39166 if (element.hasStatusElement()) { 39167 composeEnumerationCore("status", element.getStatusElement(), new Specimen.SpecimenStatusEnumFactory(), false); 39168 composeEnumerationExtras("status", element.getStatusElement(), new Specimen.SpecimenStatusEnumFactory(), false); 39169 } 39170 if (element.hasType()) { 39171 composeCodeableConcept("type", element.getType()); 39172 } 39173 if (element.hasSubject()) { 39174 composeReference("subject", element.getSubject()); 39175 } 39176 if (element.hasReceivedTimeElement()) { 39177 composeDateTimeCore("receivedTime", element.getReceivedTimeElement(), false); 39178 composeDateTimeExtras("receivedTime", element.getReceivedTimeElement(), false); 39179 } 39180 if (element.hasParent()) { 39181 openArray("parent"); 39182 for (Reference e : element.getParent()) 39183 composeReference(null, e); 39184 closeArray(); 39185 }; 39186 if (element.hasRequest()) { 39187 openArray("request"); 39188 for (Reference e : element.getRequest()) 39189 composeReference(null, e); 39190 closeArray(); 39191 }; 39192 if (element.hasCollection()) { 39193 composeSpecimenSpecimenCollectionComponent("collection", element.getCollection()); 39194 } 39195 if (element.hasProcessing()) { 39196 openArray("processing"); 39197 for (Specimen.SpecimenProcessingComponent e : element.getProcessing()) 39198 composeSpecimenSpecimenProcessingComponent(null, e); 39199 closeArray(); 39200 }; 39201 if (element.hasContainer()) { 39202 openArray("container"); 39203 for (Specimen.SpecimenContainerComponent e : element.getContainer()) 39204 composeSpecimenSpecimenContainerComponent(null, e); 39205 closeArray(); 39206 }; 39207 if (element.hasNote()) { 39208 openArray("note"); 39209 for (Annotation e : element.getNote()) 39210 composeAnnotation(null, e); 39211 closeArray(); 39212 }; 39213 } 39214 39215 protected void composeSpecimenSpecimenCollectionComponent(String name, Specimen.SpecimenCollectionComponent element) throws IOException { 39216 if (element != null) { 39217 open(name); 39218 composeSpecimenSpecimenCollectionComponentInner(element); 39219 close(); 39220 } 39221 } 39222 39223 protected void composeSpecimenSpecimenCollectionComponentInner(Specimen.SpecimenCollectionComponent element) throws IOException { 39224 composeBackbone(element); 39225 if (element.hasCollector()) { 39226 composeReference("collector", element.getCollector()); 39227 } 39228 if (element.hasCollected()) { 39229 composeType("collected", element.getCollected()); 39230 } 39231 if (element.hasQuantity()) { 39232 composeSimpleQuantity("quantity", element.getQuantity()); 39233 } 39234 if (element.hasMethod()) { 39235 composeCodeableConcept("method", element.getMethod()); 39236 } 39237 if (element.hasBodySite()) { 39238 composeCodeableConcept("bodySite", element.getBodySite()); 39239 } 39240 } 39241 39242 protected void composeSpecimenSpecimenProcessingComponent(String name, Specimen.SpecimenProcessingComponent element) throws IOException { 39243 if (element != null) { 39244 open(name); 39245 composeSpecimenSpecimenProcessingComponentInner(element); 39246 close(); 39247 } 39248 } 39249 39250 protected void composeSpecimenSpecimenProcessingComponentInner(Specimen.SpecimenProcessingComponent element) throws IOException { 39251 composeBackbone(element); 39252 if (element.hasDescriptionElement()) { 39253 composeStringCore("description", element.getDescriptionElement(), false); 39254 composeStringExtras("description", element.getDescriptionElement(), false); 39255 } 39256 if (element.hasProcedure()) { 39257 composeCodeableConcept("procedure", element.getProcedure()); 39258 } 39259 if (element.hasAdditive()) { 39260 openArray("additive"); 39261 for (Reference e : element.getAdditive()) 39262 composeReference(null, e); 39263 closeArray(); 39264 }; 39265 if (element.hasTime()) { 39266 composeType("time", element.getTime()); 39267 } 39268 } 39269 39270 protected void composeSpecimenSpecimenContainerComponent(String name, Specimen.SpecimenContainerComponent element) throws IOException { 39271 if (element != null) { 39272 open(name); 39273 composeSpecimenSpecimenContainerComponentInner(element); 39274 close(); 39275 } 39276 } 39277 39278 protected void composeSpecimenSpecimenContainerComponentInner(Specimen.SpecimenContainerComponent element) throws IOException { 39279 composeBackbone(element); 39280 if (element.hasIdentifier()) { 39281 openArray("identifier"); 39282 for (Identifier e : element.getIdentifier()) 39283 composeIdentifier(null, e); 39284 closeArray(); 39285 }; 39286 if (element.hasDescriptionElement()) { 39287 composeStringCore("description", element.getDescriptionElement(), false); 39288 composeStringExtras("description", element.getDescriptionElement(), false); 39289 } 39290 if (element.hasType()) { 39291 composeCodeableConcept("type", element.getType()); 39292 } 39293 if (element.hasCapacity()) { 39294 composeSimpleQuantity("capacity", element.getCapacity()); 39295 } 39296 if (element.hasSpecimenQuantity()) { 39297 composeSimpleQuantity("specimenQuantity", element.getSpecimenQuantity()); 39298 } 39299 if (element.hasAdditive()) { 39300 composeType("additive", element.getAdditive()); 39301 } 39302 } 39303 39304 protected void composeStructureDefinition(String name, StructureDefinition element) throws IOException { 39305 if (element != null) { 39306 prop("resourceType", name); 39307 composeStructureDefinitionInner(element); 39308 } 39309 } 39310 39311 protected void composeStructureDefinitionInner(StructureDefinition element) throws IOException { 39312 composeDomainResourceElements(element); 39313 if (element.hasUrlElement()) { 39314 composeUriCore("url", element.getUrlElement(), false); 39315 composeUriExtras("url", element.getUrlElement(), false); 39316 } 39317 if (element.hasIdentifier()) { 39318 openArray("identifier"); 39319 for (Identifier e : element.getIdentifier()) 39320 composeIdentifier(null, e); 39321 closeArray(); 39322 }; 39323 if (element.hasVersionElement()) { 39324 composeStringCore("version", element.getVersionElement(), false); 39325 composeStringExtras("version", element.getVersionElement(), false); 39326 } 39327 if (element.hasNameElement()) { 39328 composeStringCore("name", element.getNameElement(), false); 39329 composeStringExtras("name", element.getNameElement(), false); 39330 } 39331 if (element.hasTitleElement()) { 39332 composeStringCore("title", element.getTitleElement(), false); 39333 composeStringExtras("title", element.getTitleElement(), false); 39334 } 39335 if (element.hasStatusElement()) { 39336 composeEnumerationCore("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 39337 composeEnumerationExtras("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 39338 } 39339 if (element.hasExperimentalElement()) { 39340 composeBooleanCore("experimental", element.getExperimentalElement(), false); 39341 composeBooleanExtras("experimental", element.getExperimentalElement(), false); 39342 } 39343 if (element.hasDateElement()) { 39344 composeDateTimeCore("date", element.getDateElement(), false); 39345 composeDateTimeExtras("date", element.getDateElement(), false); 39346 } 39347 if (element.hasPublisherElement()) { 39348 composeStringCore("publisher", element.getPublisherElement(), false); 39349 composeStringExtras("publisher", element.getPublisherElement(), false); 39350 } 39351 if (element.hasContact()) { 39352 openArray("contact"); 39353 for (ContactDetail e : element.getContact()) 39354 composeContactDetail(null, e); 39355 closeArray(); 39356 }; 39357 if (element.hasDescriptionElement()) { 39358 composeMarkdownCore("description", element.getDescriptionElement(), false); 39359 composeMarkdownExtras("description", element.getDescriptionElement(), false); 39360 } 39361 if (element.hasUseContext()) { 39362 openArray("useContext"); 39363 for (UsageContext e : element.getUseContext()) 39364 composeUsageContext(null, e); 39365 closeArray(); 39366 }; 39367 if (element.hasJurisdiction()) { 39368 openArray("jurisdiction"); 39369 for (CodeableConcept e : element.getJurisdiction()) 39370 composeCodeableConcept(null, e); 39371 closeArray(); 39372 }; 39373 if (element.hasPurposeElement()) { 39374 composeMarkdownCore("purpose", element.getPurposeElement(), false); 39375 composeMarkdownExtras("purpose", element.getPurposeElement(), false); 39376 } 39377 if (element.hasCopyrightElement()) { 39378 composeMarkdownCore("copyright", element.getCopyrightElement(), false); 39379 composeMarkdownExtras("copyright", element.getCopyrightElement(), false); 39380 } 39381 if (element.hasKeyword()) { 39382 openArray("keyword"); 39383 for (Coding e : element.getKeyword()) 39384 composeCoding(null, e); 39385 closeArray(); 39386 }; 39387 if (element.hasFhirVersionElement()) { 39388 composeIdCore("fhirVersion", element.getFhirVersionElement(), false); 39389 composeIdExtras("fhirVersion", element.getFhirVersionElement(), false); 39390 } 39391 if (element.hasMapping()) { 39392 openArray("mapping"); 39393 for (StructureDefinition.StructureDefinitionMappingComponent e : element.getMapping()) 39394 composeStructureDefinitionStructureDefinitionMappingComponent(null, e); 39395 closeArray(); 39396 }; 39397 if (element.hasKindElement()) { 39398 composeEnumerationCore("kind", element.getKindElement(), new StructureDefinition.StructureDefinitionKindEnumFactory(), false); 39399 composeEnumerationExtras("kind", element.getKindElement(), new StructureDefinition.StructureDefinitionKindEnumFactory(), false); 39400 } 39401 if (element.hasAbstractElement()) { 39402 composeBooleanCore("abstract", element.getAbstractElement(), false); 39403 composeBooleanExtras("abstract", element.getAbstractElement(), false); 39404 } 39405 if (element.hasContextTypeElement()) { 39406 composeEnumerationCore("contextType", element.getContextTypeElement(), new StructureDefinition.ExtensionContextEnumFactory(), false); 39407 composeEnumerationExtras("contextType", element.getContextTypeElement(), new StructureDefinition.ExtensionContextEnumFactory(), false); 39408 } 39409 if (element.hasContext()) { 39410 openArray("context"); 39411 for (StringType e : element.getContext()) 39412 composeStringCore(null, e, true); 39413 closeArray(); 39414 if (anyHasExtras(element.getContext())) { 39415 openArray("_context"); 39416 for (StringType e : element.getContext()) 39417 composeStringExtras(null, e, true); 39418 closeArray(); 39419 } 39420 }; 39421 if (element.hasContextInvariant()) { 39422 openArray("contextInvariant"); 39423 for (StringType e : element.getContextInvariant()) 39424 composeStringCore(null, e, true); 39425 closeArray(); 39426 if (anyHasExtras(element.getContextInvariant())) { 39427 openArray("_contextInvariant"); 39428 for (StringType e : element.getContextInvariant()) 39429 composeStringExtras(null, e, true); 39430 closeArray(); 39431 } 39432 }; 39433 if (element.hasTypeElement()) { 39434 composeCodeCore("type", element.getTypeElement(), false); 39435 composeCodeExtras("type", element.getTypeElement(), false); 39436 } 39437 if (element.hasBaseDefinitionElement()) { 39438 composeUriCore("baseDefinition", element.getBaseDefinitionElement(), false); 39439 composeUriExtras("baseDefinition", element.getBaseDefinitionElement(), false); 39440 } 39441 if (element.hasDerivationElement()) { 39442 composeEnumerationCore("derivation", element.getDerivationElement(), new StructureDefinition.TypeDerivationRuleEnumFactory(), false); 39443 composeEnumerationExtras("derivation", element.getDerivationElement(), new StructureDefinition.TypeDerivationRuleEnumFactory(), false); 39444 } 39445 if (element.hasSnapshot()) { 39446 composeStructureDefinitionStructureDefinitionSnapshotComponent("snapshot", element.getSnapshot()); 39447 } 39448 if (element.hasDifferential()) { 39449 composeStructureDefinitionStructureDefinitionDifferentialComponent("differential", element.getDifferential()); 39450 } 39451 } 39452 39453 protected void composeStructureDefinitionStructureDefinitionMappingComponent(String name, StructureDefinition.StructureDefinitionMappingComponent element) throws IOException { 39454 if (element != null) { 39455 open(name); 39456 composeStructureDefinitionStructureDefinitionMappingComponentInner(element); 39457 close(); 39458 } 39459 } 39460 39461 protected void composeStructureDefinitionStructureDefinitionMappingComponentInner(StructureDefinition.StructureDefinitionMappingComponent element) throws IOException { 39462 composeBackbone(element); 39463 if (element.hasIdentityElement()) { 39464 composeIdCore("identity", element.getIdentityElement(), false); 39465 composeIdExtras("identity", element.getIdentityElement(), false); 39466 } 39467 if (element.hasUriElement()) { 39468 composeUriCore("uri", element.getUriElement(), false); 39469 composeUriExtras("uri", element.getUriElement(), false); 39470 } 39471 if (element.hasNameElement()) { 39472 composeStringCore("name", element.getNameElement(), false); 39473 composeStringExtras("name", element.getNameElement(), false); 39474 } 39475 if (element.hasCommentElement()) { 39476 composeStringCore("comment", element.getCommentElement(), false); 39477 composeStringExtras("comment", element.getCommentElement(), false); 39478 } 39479 } 39480 39481 protected void composeStructureDefinitionStructureDefinitionSnapshotComponent(String name, StructureDefinition.StructureDefinitionSnapshotComponent element) throws IOException { 39482 if (element != null) { 39483 open(name); 39484 composeStructureDefinitionStructureDefinitionSnapshotComponentInner(element); 39485 close(); 39486 } 39487 } 39488 39489 protected void composeStructureDefinitionStructureDefinitionSnapshotComponentInner(StructureDefinition.StructureDefinitionSnapshotComponent element) throws IOException { 39490 composeBackbone(element); 39491 if (element.hasElement()) { 39492 openArray("element"); 39493 for (ElementDefinition e : element.getElement()) 39494 composeElementDefinition(null, e); 39495 closeArray(); 39496 }; 39497 } 39498 39499 protected void composeStructureDefinitionStructureDefinitionDifferentialComponent(String name, StructureDefinition.StructureDefinitionDifferentialComponent element) throws IOException { 39500 if (element != null) { 39501 open(name); 39502 composeStructureDefinitionStructureDefinitionDifferentialComponentInner(element); 39503 close(); 39504 } 39505 } 39506 39507 protected void composeStructureDefinitionStructureDefinitionDifferentialComponentInner(StructureDefinition.StructureDefinitionDifferentialComponent element) throws IOException { 39508 composeBackbone(element); 39509 if (element.hasElement()) { 39510 openArray("element"); 39511 for (ElementDefinition e : element.getElement()) 39512 composeElementDefinition(null, e); 39513 closeArray(); 39514 }; 39515 } 39516 39517 protected void composeStructureMap(String name, StructureMap element) throws IOException { 39518 if (element != null) { 39519 prop("resourceType", name); 39520 composeStructureMapInner(element); 39521 } 39522 } 39523 39524 protected void composeStructureMapInner(StructureMap element) throws IOException { 39525 composeDomainResourceElements(element); 39526 if (element.hasUrlElement()) { 39527 composeUriCore("url", element.getUrlElement(), false); 39528 composeUriExtras("url", element.getUrlElement(), false); 39529 } 39530 if (element.hasIdentifier()) { 39531 openArray("identifier"); 39532 for (Identifier e : element.getIdentifier()) 39533 composeIdentifier(null, e); 39534 closeArray(); 39535 }; 39536 if (element.hasVersionElement()) { 39537 composeStringCore("version", element.getVersionElement(), false); 39538 composeStringExtras("version", element.getVersionElement(), false); 39539 } 39540 if (element.hasNameElement()) { 39541 composeStringCore("name", element.getNameElement(), false); 39542 composeStringExtras("name", element.getNameElement(), false); 39543 } 39544 if (element.hasTitleElement()) { 39545 composeStringCore("title", element.getTitleElement(), false); 39546 composeStringExtras("title", element.getTitleElement(), false); 39547 } 39548 if (element.hasStatusElement()) { 39549 composeEnumerationCore("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 39550 composeEnumerationExtras("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 39551 } 39552 if (element.hasExperimentalElement()) { 39553 composeBooleanCore("experimental", element.getExperimentalElement(), false); 39554 composeBooleanExtras("experimental", element.getExperimentalElement(), false); 39555 } 39556 if (element.hasDateElement()) { 39557 composeDateTimeCore("date", element.getDateElement(), false); 39558 composeDateTimeExtras("date", element.getDateElement(), false); 39559 } 39560 if (element.hasPublisherElement()) { 39561 composeStringCore("publisher", element.getPublisherElement(), false); 39562 composeStringExtras("publisher", element.getPublisherElement(), false); 39563 } 39564 if (element.hasContact()) { 39565 openArray("contact"); 39566 for (ContactDetail e : element.getContact()) 39567 composeContactDetail(null, e); 39568 closeArray(); 39569 }; 39570 if (element.hasDescriptionElement()) { 39571 composeMarkdownCore("description", element.getDescriptionElement(), false); 39572 composeMarkdownExtras("description", element.getDescriptionElement(), false); 39573 } 39574 if (element.hasUseContext()) { 39575 openArray("useContext"); 39576 for (UsageContext e : element.getUseContext()) 39577 composeUsageContext(null, e); 39578 closeArray(); 39579 }; 39580 if (element.hasJurisdiction()) { 39581 openArray("jurisdiction"); 39582 for (CodeableConcept e : element.getJurisdiction()) 39583 composeCodeableConcept(null, e); 39584 closeArray(); 39585 }; 39586 if (element.hasPurposeElement()) { 39587 composeMarkdownCore("purpose", element.getPurposeElement(), false); 39588 composeMarkdownExtras("purpose", element.getPurposeElement(), false); 39589 } 39590 if (element.hasCopyrightElement()) { 39591 composeMarkdownCore("copyright", element.getCopyrightElement(), false); 39592 composeMarkdownExtras("copyright", element.getCopyrightElement(), false); 39593 } 39594 if (element.hasStructure()) { 39595 openArray("structure"); 39596 for (StructureMap.StructureMapStructureComponent e : element.getStructure()) 39597 composeStructureMapStructureMapStructureComponent(null, e); 39598 closeArray(); 39599 }; 39600 if (element.hasImport()) { 39601 openArray("import"); 39602 for (UriType e : element.getImport()) 39603 composeUriCore(null, e, true); 39604 closeArray(); 39605 if (anyHasExtras(element.getImport())) { 39606 openArray("_import"); 39607 for (UriType e : element.getImport()) 39608 composeUriExtras(null, e, true); 39609 closeArray(); 39610 } 39611 }; 39612 if (element.hasGroup()) { 39613 openArray("group"); 39614 for (StructureMap.StructureMapGroupComponent e : element.getGroup()) 39615 composeStructureMapStructureMapGroupComponent(null, e); 39616 closeArray(); 39617 }; 39618 } 39619 39620 protected void composeStructureMapStructureMapStructureComponent(String name, StructureMap.StructureMapStructureComponent element) throws IOException { 39621 if (element != null) { 39622 open(name); 39623 composeStructureMapStructureMapStructureComponentInner(element); 39624 close(); 39625 } 39626 } 39627 39628 protected void composeStructureMapStructureMapStructureComponentInner(StructureMap.StructureMapStructureComponent element) throws IOException { 39629 composeBackbone(element); 39630 if (element.hasUrlElement()) { 39631 composeUriCore("url", element.getUrlElement(), false); 39632 composeUriExtras("url", element.getUrlElement(), false); 39633 } 39634 if (element.hasModeElement()) { 39635 composeEnumerationCore("mode", element.getModeElement(), new StructureMap.StructureMapModelModeEnumFactory(), false); 39636 composeEnumerationExtras("mode", element.getModeElement(), new StructureMap.StructureMapModelModeEnumFactory(), false); 39637 } 39638 if (element.hasAliasElement()) { 39639 composeStringCore("alias", element.getAliasElement(), false); 39640 composeStringExtras("alias", element.getAliasElement(), false); 39641 } 39642 if (element.hasDocumentationElement()) { 39643 composeStringCore("documentation", element.getDocumentationElement(), false); 39644 composeStringExtras("documentation", element.getDocumentationElement(), false); 39645 } 39646 } 39647 39648 protected void composeStructureMapStructureMapGroupComponent(String name, StructureMap.StructureMapGroupComponent element) throws IOException { 39649 if (element != null) { 39650 open(name); 39651 composeStructureMapStructureMapGroupComponentInner(element); 39652 close(); 39653 } 39654 } 39655 39656 protected void composeStructureMapStructureMapGroupComponentInner(StructureMap.StructureMapGroupComponent element) throws IOException { 39657 composeBackbone(element); 39658 if (element.hasNameElement()) { 39659 composeIdCore("name", element.getNameElement(), false); 39660 composeIdExtras("name", element.getNameElement(), false); 39661 } 39662 if (element.hasExtendsElement()) { 39663 composeIdCore("extends", element.getExtendsElement(), false); 39664 composeIdExtras("extends", element.getExtendsElement(), false); 39665 } 39666 if (element.hasTypeModeElement()) { 39667 composeEnumerationCore("typeMode", element.getTypeModeElement(), new StructureMap.StructureMapGroupTypeModeEnumFactory(), false); 39668 composeEnumerationExtras("typeMode", element.getTypeModeElement(), new StructureMap.StructureMapGroupTypeModeEnumFactory(), false); 39669 } 39670 if (element.hasDocumentationElement()) { 39671 composeStringCore("documentation", element.getDocumentationElement(), false); 39672 composeStringExtras("documentation", element.getDocumentationElement(), false); 39673 } 39674 if (element.hasInput()) { 39675 openArray("input"); 39676 for (StructureMap.StructureMapGroupInputComponent e : element.getInput()) 39677 composeStructureMapStructureMapGroupInputComponent(null, e); 39678 closeArray(); 39679 }; 39680 if (element.hasRule()) { 39681 openArray("rule"); 39682 for (StructureMap.StructureMapGroupRuleComponent e : element.getRule()) 39683 composeStructureMapStructureMapGroupRuleComponent(null, e); 39684 closeArray(); 39685 }; 39686 } 39687 39688 protected void composeStructureMapStructureMapGroupInputComponent(String name, StructureMap.StructureMapGroupInputComponent element) throws IOException { 39689 if (element != null) { 39690 open(name); 39691 composeStructureMapStructureMapGroupInputComponentInner(element); 39692 close(); 39693 } 39694 } 39695 39696 protected void composeStructureMapStructureMapGroupInputComponentInner(StructureMap.StructureMapGroupInputComponent element) throws IOException { 39697 composeBackbone(element); 39698 if (element.hasNameElement()) { 39699 composeIdCore("name", element.getNameElement(), false); 39700 composeIdExtras("name", element.getNameElement(), false); 39701 } 39702 if (element.hasTypeElement()) { 39703 composeStringCore("type", element.getTypeElement(), false); 39704 composeStringExtras("type", element.getTypeElement(), false); 39705 } 39706 if (element.hasModeElement()) { 39707 composeEnumerationCore("mode", element.getModeElement(), new StructureMap.StructureMapInputModeEnumFactory(), false); 39708 composeEnumerationExtras("mode", element.getModeElement(), new StructureMap.StructureMapInputModeEnumFactory(), false); 39709 } 39710 if (element.hasDocumentationElement()) { 39711 composeStringCore("documentation", element.getDocumentationElement(), false); 39712 composeStringExtras("documentation", element.getDocumentationElement(), false); 39713 } 39714 } 39715 39716 protected void composeStructureMapStructureMapGroupRuleComponent(String name, StructureMap.StructureMapGroupRuleComponent element) throws IOException { 39717 if (element != null) { 39718 open(name); 39719 composeStructureMapStructureMapGroupRuleComponentInner(element); 39720 close(); 39721 } 39722 } 39723 39724 protected void composeStructureMapStructureMapGroupRuleComponentInner(StructureMap.StructureMapGroupRuleComponent element) throws IOException { 39725 composeBackbone(element); 39726 if (element.hasNameElement()) { 39727 composeIdCore("name", element.getNameElement(), false); 39728 composeIdExtras("name", element.getNameElement(), false); 39729 } 39730 if (element.hasSource()) { 39731 openArray("source"); 39732 for (StructureMap.StructureMapGroupRuleSourceComponent e : element.getSource()) 39733 composeStructureMapStructureMapGroupRuleSourceComponent(null, e); 39734 closeArray(); 39735 }; 39736 if (element.hasTarget()) { 39737 openArray("target"); 39738 for (StructureMap.StructureMapGroupRuleTargetComponent e : element.getTarget()) 39739 composeStructureMapStructureMapGroupRuleTargetComponent(null, e); 39740 closeArray(); 39741 }; 39742 if (element.hasRule()) { 39743 openArray("rule"); 39744 for (StructureMap.StructureMapGroupRuleComponent e : element.getRule()) 39745 composeStructureMapStructureMapGroupRuleComponent(null, e); 39746 closeArray(); 39747 }; 39748 if (element.hasDependent()) { 39749 openArray("dependent"); 39750 for (StructureMap.StructureMapGroupRuleDependentComponent e : element.getDependent()) 39751 composeStructureMapStructureMapGroupRuleDependentComponent(null, e); 39752 closeArray(); 39753 }; 39754 if (element.hasDocumentationElement()) { 39755 composeStringCore("documentation", element.getDocumentationElement(), false); 39756 composeStringExtras("documentation", element.getDocumentationElement(), false); 39757 } 39758 } 39759 39760 protected void composeStructureMapStructureMapGroupRuleSourceComponent(String name, StructureMap.StructureMapGroupRuleSourceComponent element) throws IOException { 39761 if (element != null) { 39762 open(name); 39763 composeStructureMapStructureMapGroupRuleSourceComponentInner(element); 39764 close(); 39765 } 39766 } 39767 39768 protected void composeStructureMapStructureMapGroupRuleSourceComponentInner(StructureMap.StructureMapGroupRuleSourceComponent element) throws IOException { 39769 composeBackbone(element); 39770 if (element.hasContextElement()) { 39771 composeIdCore("context", element.getContextElement(), false); 39772 composeIdExtras("context", element.getContextElement(), false); 39773 } 39774 if (element.hasMinElement()) { 39775 composeIntegerCore("min", element.getMinElement(), false); 39776 composeIntegerExtras("min", element.getMinElement(), false); 39777 } 39778 if (element.hasMaxElement()) { 39779 composeStringCore("max", element.getMaxElement(), false); 39780 composeStringExtras("max", element.getMaxElement(), false); 39781 } 39782 if (element.hasTypeElement()) { 39783 composeStringCore("type", element.getTypeElement(), false); 39784 composeStringExtras("type", element.getTypeElement(), false); 39785 } 39786 if (element.hasDefaultValue()) { 39787 composeType("defaultValue", element.getDefaultValue()); 39788 } 39789 if (element.hasElementElement()) { 39790 composeStringCore("element", element.getElementElement(), false); 39791 composeStringExtras("element", element.getElementElement(), false); 39792 } 39793 if (element.hasListModeElement()) { 39794 composeEnumerationCore("listMode", element.getListModeElement(), new StructureMap.StructureMapSourceListModeEnumFactory(), false); 39795 composeEnumerationExtras("listMode", element.getListModeElement(), new StructureMap.StructureMapSourceListModeEnumFactory(), false); 39796 } 39797 if (element.hasVariableElement()) { 39798 composeIdCore("variable", element.getVariableElement(), false); 39799 composeIdExtras("variable", element.getVariableElement(), false); 39800 } 39801 if (element.hasConditionElement()) { 39802 composeStringCore("condition", element.getConditionElement(), false); 39803 composeStringExtras("condition", element.getConditionElement(), false); 39804 } 39805 if (element.hasCheckElement()) { 39806 composeStringCore("check", element.getCheckElement(), false); 39807 composeStringExtras("check", element.getCheckElement(), false); 39808 } 39809 } 39810 39811 protected void composeStructureMapStructureMapGroupRuleTargetComponent(String name, StructureMap.StructureMapGroupRuleTargetComponent element) throws IOException { 39812 if (element != null) { 39813 open(name); 39814 composeStructureMapStructureMapGroupRuleTargetComponentInner(element); 39815 close(); 39816 } 39817 } 39818 39819 protected void composeStructureMapStructureMapGroupRuleTargetComponentInner(StructureMap.StructureMapGroupRuleTargetComponent element) throws IOException { 39820 composeBackbone(element); 39821 if (element.hasContextElement()) { 39822 composeIdCore("context", element.getContextElement(), false); 39823 composeIdExtras("context", element.getContextElement(), false); 39824 } 39825 if (element.hasContextTypeElement()) { 39826 composeEnumerationCore("contextType", element.getContextTypeElement(), new StructureMap.StructureMapContextTypeEnumFactory(), false); 39827 composeEnumerationExtras("contextType", element.getContextTypeElement(), new StructureMap.StructureMapContextTypeEnumFactory(), false); 39828 } 39829 if (element.hasElementElement()) { 39830 composeStringCore("element", element.getElementElement(), false); 39831 composeStringExtras("element", element.getElementElement(), false); 39832 } 39833 if (element.hasVariableElement()) { 39834 composeIdCore("variable", element.getVariableElement(), false); 39835 composeIdExtras("variable", element.getVariableElement(), false); 39836 } 39837 if (element.hasListMode()) { 39838 openArray("listMode"); 39839 for (Enumeration<StructureMap.StructureMapTargetListMode> e : element.getListMode()) 39840 composeEnumerationCore(null, e, new StructureMap.StructureMapTargetListModeEnumFactory(), true); 39841 closeArray(); 39842 if (anyHasExtras(element.getListMode())) { 39843 openArray("_listMode"); 39844 for (Enumeration<StructureMap.StructureMapTargetListMode> e : element.getListMode()) 39845 composeEnumerationExtras(null, e, new StructureMap.StructureMapTargetListModeEnumFactory(), true); 39846 closeArray(); 39847 } 39848 }; 39849 if (element.hasListRuleIdElement()) { 39850 composeIdCore("listRuleId", element.getListRuleIdElement(), false); 39851 composeIdExtras("listRuleId", element.getListRuleIdElement(), false); 39852 } 39853 if (element.hasTransformElement()) { 39854 composeEnumerationCore("transform", element.getTransformElement(), new StructureMap.StructureMapTransformEnumFactory(), false); 39855 composeEnumerationExtras("transform", element.getTransformElement(), new StructureMap.StructureMapTransformEnumFactory(), false); 39856 } 39857 if (element.hasParameter()) { 39858 openArray("parameter"); 39859 for (StructureMap.StructureMapGroupRuleTargetParameterComponent e : element.getParameter()) 39860 composeStructureMapStructureMapGroupRuleTargetParameterComponent(null, e); 39861 closeArray(); 39862 }; 39863 } 39864 39865 protected void composeStructureMapStructureMapGroupRuleTargetParameterComponent(String name, StructureMap.StructureMapGroupRuleTargetParameterComponent element) throws IOException { 39866 if (element != null) { 39867 open(name); 39868 composeStructureMapStructureMapGroupRuleTargetParameterComponentInner(element); 39869 close(); 39870 } 39871 } 39872 39873 protected void composeStructureMapStructureMapGroupRuleTargetParameterComponentInner(StructureMap.StructureMapGroupRuleTargetParameterComponent element) throws IOException { 39874 composeBackbone(element); 39875 if (element.hasValue()) { 39876 composeType("value", element.getValue()); 39877 } 39878 } 39879 39880 protected void composeStructureMapStructureMapGroupRuleDependentComponent(String name, StructureMap.StructureMapGroupRuleDependentComponent element) throws IOException { 39881 if (element != null) { 39882 open(name); 39883 composeStructureMapStructureMapGroupRuleDependentComponentInner(element); 39884 close(); 39885 } 39886 } 39887 39888 protected void composeStructureMapStructureMapGroupRuleDependentComponentInner(StructureMap.StructureMapGroupRuleDependentComponent element) throws IOException { 39889 composeBackbone(element); 39890 if (element.hasNameElement()) { 39891 composeIdCore("name", element.getNameElement(), false); 39892 composeIdExtras("name", element.getNameElement(), false); 39893 } 39894 if (element.hasVariable()) { 39895 openArray("variable"); 39896 for (StringType e : element.getVariable()) 39897 composeStringCore(null, e, true); 39898 closeArray(); 39899 if (anyHasExtras(element.getVariable())) { 39900 openArray("_variable"); 39901 for (StringType e : element.getVariable()) 39902 composeStringExtras(null, e, true); 39903 closeArray(); 39904 } 39905 }; 39906 } 39907 39908 protected void composeSubscription(String name, Subscription element) throws IOException { 39909 if (element != null) { 39910 prop("resourceType", name); 39911 composeSubscriptionInner(element); 39912 } 39913 } 39914 39915 protected void composeSubscriptionInner(Subscription element) throws IOException { 39916 composeDomainResourceElements(element); 39917 if (element.hasStatusElement()) { 39918 composeEnumerationCore("status", element.getStatusElement(), new Subscription.SubscriptionStatusEnumFactory(), false); 39919 composeEnumerationExtras("status", element.getStatusElement(), new Subscription.SubscriptionStatusEnumFactory(), false); 39920 } 39921 if (element.hasContact()) { 39922 openArray("contact"); 39923 for (ContactPoint e : element.getContact()) 39924 composeContactPoint(null, e); 39925 closeArray(); 39926 }; 39927 if (element.hasEndElement()) { 39928 composeInstantCore("end", element.getEndElement(), false); 39929 composeInstantExtras("end", element.getEndElement(), false); 39930 } 39931 if (element.hasReasonElement()) { 39932 composeStringCore("reason", element.getReasonElement(), false); 39933 composeStringExtras("reason", element.getReasonElement(), false); 39934 } 39935 if (element.hasCriteriaElement()) { 39936 composeStringCore("criteria", element.getCriteriaElement(), false); 39937 composeStringExtras("criteria", element.getCriteriaElement(), false); 39938 } 39939 if (element.hasErrorElement()) { 39940 composeStringCore("error", element.getErrorElement(), false); 39941 composeStringExtras("error", element.getErrorElement(), false); 39942 } 39943 if (element.hasChannel()) { 39944 composeSubscriptionSubscriptionChannelComponent("channel", element.getChannel()); 39945 } 39946 if (element.hasTag()) { 39947 openArray("tag"); 39948 for (Coding e : element.getTag()) 39949 composeCoding(null, e); 39950 closeArray(); 39951 }; 39952 } 39953 39954 protected void composeSubscriptionSubscriptionChannelComponent(String name, Subscription.SubscriptionChannelComponent element) throws IOException { 39955 if (element != null) { 39956 open(name); 39957 composeSubscriptionSubscriptionChannelComponentInner(element); 39958 close(); 39959 } 39960 } 39961 39962 protected void composeSubscriptionSubscriptionChannelComponentInner(Subscription.SubscriptionChannelComponent element) throws IOException { 39963 composeBackbone(element); 39964 if (element.hasTypeElement()) { 39965 composeEnumerationCore("type", element.getTypeElement(), new Subscription.SubscriptionChannelTypeEnumFactory(), false); 39966 composeEnumerationExtras("type", element.getTypeElement(), new Subscription.SubscriptionChannelTypeEnumFactory(), false); 39967 } 39968 if (element.hasEndpointElement()) { 39969 composeUriCore("endpoint", element.getEndpointElement(), false); 39970 composeUriExtras("endpoint", element.getEndpointElement(), false); 39971 } 39972 if (element.hasPayloadElement()) { 39973 composeStringCore("payload", element.getPayloadElement(), false); 39974 composeStringExtras("payload", element.getPayloadElement(), false); 39975 } 39976 if (element.hasHeader()) { 39977 openArray("header"); 39978 for (StringType e : element.getHeader()) 39979 composeStringCore(null, e, true); 39980 closeArray(); 39981 if (anyHasExtras(element.getHeader())) { 39982 openArray("_header"); 39983 for (StringType e : element.getHeader()) 39984 composeStringExtras(null, e, true); 39985 closeArray(); 39986 } 39987 }; 39988 } 39989 39990 protected void composeSubstance(String name, Substance element) throws IOException { 39991 if (element != null) { 39992 prop("resourceType", name); 39993 composeSubstanceInner(element); 39994 } 39995 } 39996 39997 protected void composeSubstanceInner(Substance element) throws IOException { 39998 composeDomainResourceElements(element); 39999 if (element.hasIdentifier()) { 40000 openArray("identifier"); 40001 for (Identifier e : element.getIdentifier()) 40002 composeIdentifier(null, e); 40003 closeArray(); 40004 }; 40005 if (element.hasStatusElement()) { 40006 composeEnumerationCore("status", element.getStatusElement(), new Substance.FHIRSubstanceStatusEnumFactory(), false); 40007 composeEnumerationExtras("status", element.getStatusElement(), new Substance.FHIRSubstanceStatusEnumFactory(), false); 40008 } 40009 if (element.hasCategory()) { 40010 openArray("category"); 40011 for (CodeableConcept e : element.getCategory()) 40012 composeCodeableConcept(null, e); 40013 closeArray(); 40014 }; 40015 if (element.hasCode()) { 40016 composeCodeableConcept("code", element.getCode()); 40017 } 40018 if (element.hasDescriptionElement()) { 40019 composeStringCore("description", element.getDescriptionElement(), false); 40020 composeStringExtras("description", element.getDescriptionElement(), false); 40021 } 40022 if (element.hasInstance()) { 40023 openArray("instance"); 40024 for (Substance.SubstanceInstanceComponent e : element.getInstance()) 40025 composeSubstanceSubstanceInstanceComponent(null, e); 40026 closeArray(); 40027 }; 40028 if (element.hasIngredient()) { 40029 openArray("ingredient"); 40030 for (Substance.SubstanceIngredientComponent e : element.getIngredient()) 40031 composeSubstanceSubstanceIngredientComponent(null, e); 40032 closeArray(); 40033 }; 40034 } 40035 40036 protected void composeSubstanceSubstanceInstanceComponent(String name, Substance.SubstanceInstanceComponent element) throws IOException { 40037 if (element != null) { 40038 open(name); 40039 composeSubstanceSubstanceInstanceComponentInner(element); 40040 close(); 40041 } 40042 } 40043 40044 protected void composeSubstanceSubstanceInstanceComponentInner(Substance.SubstanceInstanceComponent element) throws IOException { 40045 composeBackbone(element); 40046 if (element.hasIdentifier()) { 40047 composeIdentifier("identifier", element.getIdentifier()); 40048 } 40049 if (element.hasExpiryElement()) { 40050 composeDateTimeCore("expiry", element.getExpiryElement(), false); 40051 composeDateTimeExtras("expiry", element.getExpiryElement(), false); 40052 } 40053 if (element.hasQuantity()) { 40054 composeSimpleQuantity("quantity", element.getQuantity()); 40055 } 40056 } 40057 40058 protected void composeSubstanceSubstanceIngredientComponent(String name, Substance.SubstanceIngredientComponent element) throws IOException { 40059 if (element != null) { 40060 open(name); 40061 composeSubstanceSubstanceIngredientComponentInner(element); 40062 close(); 40063 } 40064 } 40065 40066 protected void composeSubstanceSubstanceIngredientComponentInner(Substance.SubstanceIngredientComponent element) throws IOException { 40067 composeBackbone(element); 40068 if (element.hasQuantity()) { 40069 composeRatio("quantity", element.getQuantity()); 40070 } 40071 if (element.hasSubstance()) { 40072 composeType("substance", element.getSubstance()); 40073 } 40074 } 40075 40076 protected void composeSupplyDelivery(String name, SupplyDelivery element) throws IOException { 40077 if (element != null) { 40078 prop("resourceType", name); 40079 composeSupplyDeliveryInner(element); 40080 } 40081 } 40082 40083 protected void composeSupplyDeliveryInner(SupplyDelivery element) throws IOException { 40084 composeDomainResourceElements(element); 40085 if (element.hasIdentifier()) { 40086 composeIdentifier("identifier", element.getIdentifier()); 40087 } 40088 if (element.hasBasedOn()) { 40089 openArray("basedOn"); 40090 for (Reference e : element.getBasedOn()) 40091 composeReference(null, e); 40092 closeArray(); 40093 }; 40094 if (element.hasPartOf()) { 40095 openArray("partOf"); 40096 for (Reference e : element.getPartOf()) 40097 composeReference(null, e); 40098 closeArray(); 40099 }; 40100 if (element.hasStatusElement()) { 40101 composeEnumerationCore("status", element.getStatusElement(), new SupplyDelivery.SupplyDeliveryStatusEnumFactory(), false); 40102 composeEnumerationExtras("status", element.getStatusElement(), new SupplyDelivery.SupplyDeliveryStatusEnumFactory(), false); 40103 } 40104 if (element.hasPatient()) { 40105 composeReference("patient", element.getPatient()); 40106 } 40107 if (element.hasType()) { 40108 composeCodeableConcept("type", element.getType()); 40109 } 40110 if (element.hasSuppliedItem()) { 40111 composeSupplyDeliverySupplyDeliverySuppliedItemComponent("suppliedItem", element.getSuppliedItem()); 40112 } 40113 if (element.hasOccurrence()) { 40114 composeType("occurrence", element.getOccurrence()); 40115 } 40116 if (element.hasSupplier()) { 40117 composeReference("supplier", element.getSupplier()); 40118 } 40119 if (element.hasDestination()) { 40120 composeReference("destination", element.getDestination()); 40121 } 40122 if (element.hasReceiver()) { 40123 openArray("receiver"); 40124 for (Reference e : element.getReceiver()) 40125 composeReference(null, e); 40126 closeArray(); 40127 }; 40128 } 40129 40130 protected void composeSupplyDeliverySupplyDeliverySuppliedItemComponent(String name, SupplyDelivery.SupplyDeliverySuppliedItemComponent element) throws IOException { 40131 if (element != null) { 40132 open(name); 40133 composeSupplyDeliverySupplyDeliverySuppliedItemComponentInner(element); 40134 close(); 40135 } 40136 } 40137 40138 protected void composeSupplyDeliverySupplyDeliverySuppliedItemComponentInner(SupplyDelivery.SupplyDeliverySuppliedItemComponent element) throws IOException { 40139 composeBackbone(element); 40140 if (element.hasQuantity()) { 40141 composeSimpleQuantity("quantity", element.getQuantity()); 40142 } 40143 if (element.hasItem()) { 40144 composeType("item", element.getItem()); 40145 } 40146 } 40147 40148 protected void composeSupplyRequest(String name, SupplyRequest element) throws IOException { 40149 if (element != null) { 40150 prop("resourceType", name); 40151 composeSupplyRequestInner(element); 40152 } 40153 } 40154 40155 protected void composeSupplyRequestInner(SupplyRequest element) throws IOException { 40156 composeDomainResourceElements(element); 40157 if (element.hasIdentifier()) { 40158 composeIdentifier("identifier", element.getIdentifier()); 40159 } 40160 if (element.hasStatusElement()) { 40161 composeEnumerationCore("status", element.getStatusElement(), new SupplyRequest.SupplyRequestStatusEnumFactory(), false); 40162 composeEnumerationExtras("status", element.getStatusElement(), new SupplyRequest.SupplyRequestStatusEnumFactory(), false); 40163 } 40164 if (element.hasCategory()) { 40165 composeCodeableConcept("category", element.getCategory()); 40166 } 40167 if (element.hasPriorityElement()) { 40168 composeEnumerationCore("priority", element.getPriorityElement(), new SupplyRequest.RequestPriorityEnumFactory(), false); 40169 composeEnumerationExtras("priority", element.getPriorityElement(), new SupplyRequest.RequestPriorityEnumFactory(), false); 40170 } 40171 if (element.hasOrderedItem()) { 40172 composeSupplyRequestSupplyRequestOrderedItemComponent("orderedItem", element.getOrderedItem()); 40173 } 40174 if (element.hasOccurrence()) { 40175 composeType("occurrence", element.getOccurrence()); 40176 } 40177 if (element.hasAuthoredOnElement()) { 40178 composeDateTimeCore("authoredOn", element.getAuthoredOnElement(), false); 40179 composeDateTimeExtras("authoredOn", element.getAuthoredOnElement(), false); 40180 } 40181 if (element.hasRequester()) { 40182 composeSupplyRequestSupplyRequestRequesterComponent("requester", element.getRequester()); 40183 } 40184 if (element.hasSupplier()) { 40185 openArray("supplier"); 40186 for (Reference e : element.getSupplier()) 40187 composeReference(null, e); 40188 closeArray(); 40189 }; 40190 if (element.hasReason()) { 40191 composeType("reason", element.getReason()); 40192 } 40193 if (element.hasDeliverFrom()) { 40194 composeReference("deliverFrom", element.getDeliverFrom()); 40195 } 40196 if (element.hasDeliverTo()) { 40197 composeReference("deliverTo", element.getDeliverTo()); 40198 } 40199 } 40200 40201 protected void composeSupplyRequestSupplyRequestOrderedItemComponent(String name, SupplyRequest.SupplyRequestOrderedItemComponent element) throws IOException { 40202 if (element != null) { 40203 open(name); 40204 composeSupplyRequestSupplyRequestOrderedItemComponentInner(element); 40205 close(); 40206 } 40207 } 40208 40209 protected void composeSupplyRequestSupplyRequestOrderedItemComponentInner(SupplyRequest.SupplyRequestOrderedItemComponent element) throws IOException { 40210 composeBackbone(element); 40211 if (element.hasQuantity()) { 40212 composeQuantity("quantity", element.getQuantity()); 40213 } 40214 if (element.hasItem()) { 40215 composeType("item", element.getItem()); 40216 } 40217 } 40218 40219 protected void composeSupplyRequestSupplyRequestRequesterComponent(String name, SupplyRequest.SupplyRequestRequesterComponent element) throws IOException { 40220 if (element != null) { 40221 open(name); 40222 composeSupplyRequestSupplyRequestRequesterComponentInner(element); 40223 close(); 40224 } 40225 } 40226 40227 protected void composeSupplyRequestSupplyRequestRequesterComponentInner(SupplyRequest.SupplyRequestRequesterComponent element) throws IOException { 40228 composeBackbone(element); 40229 if (element.hasAgent()) { 40230 composeReference("agent", element.getAgent()); 40231 } 40232 if (element.hasOnBehalfOf()) { 40233 composeReference("onBehalfOf", element.getOnBehalfOf()); 40234 } 40235 } 40236 40237 protected void composeTask(String name, Task element) throws IOException { 40238 if (element != null) { 40239 prop("resourceType", name); 40240 composeTaskInner(element); 40241 } 40242 } 40243 40244 protected void composeTaskInner(Task element) throws IOException { 40245 composeDomainResourceElements(element); 40246 if (element.hasIdentifier()) { 40247 openArray("identifier"); 40248 for (Identifier e : element.getIdentifier()) 40249 composeIdentifier(null, e); 40250 closeArray(); 40251 }; 40252 if (element.hasDefinition()) { 40253 composeType("definition", element.getDefinition()); 40254 } 40255 if (element.hasBasedOn()) { 40256 openArray("basedOn"); 40257 for (Reference e : element.getBasedOn()) 40258 composeReference(null, e); 40259 closeArray(); 40260 }; 40261 if (element.hasGroupIdentifier()) { 40262 composeIdentifier("groupIdentifier", element.getGroupIdentifier()); 40263 } 40264 if (element.hasPartOf()) { 40265 openArray("partOf"); 40266 for (Reference e : element.getPartOf()) 40267 composeReference(null, e); 40268 closeArray(); 40269 }; 40270 if (element.hasStatusElement()) { 40271 composeEnumerationCore("status", element.getStatusElement(), new Task.TaskStatusEnumFactory(), false); 40272 composeEnumerationExtras("status", element.getStatusElement(), new Task.TaskStatusEnumFactory(), false); 40273 } 40274 if (element.hasStatusReason()) { 40275 composeCodeableConcept("statusReason", element.getStatusReason()); 40276 } 40277 if (element.hasBusinessStatus()) { 40278 composeCodeableConcept("businessStatus", element.getBusinessStatus()); 40279 } 40280 if (element.hasIntentElement()) { 40281 composeEnumerationCore("intent", element.getIntentElement(), new Task.TaskIntentEnumFactory(), false); 40282 composeEnumerationExtras("intent", element.getIntentElement(), new Task.TaskIntentEnumFactory(), false); 40283 } 40284 if (element.hasPriorityElement()) { 40285 composeEnumerationCore("priority", element.getPriorityElement(), new Task.TaskPriorityEnumFactory(), false); 40286 composeEnumerationExtras("priority", element.getPriorityElement(), new Task.TaskPriorityEnumFactory(), false); 40287 } 40288 if (element.hasCode()) { 40289 composeCodeableConcept("code", element.getCode()); 40290 } 40291 if (element.hasDescriptionElement()) { 40292 composeStringCore("description", element.getDescriptionElement(), false); 40293 composeStringExtras("description", element.getDescriptionElement(), false); 40294 } 40295 if (element.hasFocus()) { 40296 composeReference("focus", element.getFocus()); 40297 } 40298 if (element.hasFor()) { 40299 composeReference("for", element.getFor()); 40300 } 40301 if (element.hasContext()) { 40302 composeReference("context", element.getContext()); 40303 } 40304 if (element.hasExecutionPeriod()) { 40305 composePeriod("executionPeriod", element.getExecutionPeriod()); 40306 } 40307 if (element.hasAuthoredOnElement()) { 40308 composeDateTimeCore("authoredOn", element.getAuthoredOnElement(), false); 40309 composeDateTimeExtras("authoredOn", element.getAuthoredOnElement(), false); 40310 } 40311 if (element.hasLastModifiedElement()) { 40312 composeDateTimeCore("lastModified", element.getLastModifiedElement(), false); 40313 composeDateTimeExtras("lastModified", element.getLastModifiedElement(), false); 40314 } 40315 if (element.hasRequester()) { 40316 composeTaskTaskRequesterComponent("requester", element.getRequester()); 40317 } 40318 if (element.hasPerformerType()) { 40319 openArray("performerType"); 40320 for (CodeableConcept e : element.getPerformerType()) 40321 composeCodeableConcept(null, e); 40322 closeArray(); 40323 }; 40324 if (element.hasOwner()) { 40325 composeReference("owner", element.getOwner()); 40326 } 40327 if (element.hasReason()) { 40328 composeCodeableConcept("reason", element.getReason()); 40329 } 40330 if (element.hasNote()) { 40331 openArray("note"); 40332 for (Annotation e : element.getNote()) 40333 composeAnnotation(null, e); 40334 closeArray(); 40335 }; 40336 if (element.hasRelevantHistory()) { 40337 openArray("relevantHistory"); 40338 for (Reference e : element.getRelevantHistory()) 40339 composeReference(null, e); 40340 closeArray(); 40341 }; 40342 if (element.hasRestriction()) { 40343 composeTaskTaskRestrictionComponent("restriction", element.getRestriction()); 40344 } 40345 if (element.hasInput()) { 40346 openArray("input"); 40347 for (Task.ParameterComponent e : element.getInput()) 40348 composeTaskParameterComponent(null, e); 40349 closeArray(); 40350 }; 40351 if (element.hasOutput()) { 40352 openArray("output"); 40353 for (Task.TaskOutputComponent e : element.getOutput()) 40354 composeTaskTaskOutputComponent(null, e); 40355 closeArray(); 40356 }; 40357 } 40358 40359 protected void composeTaskTaskRequesterComponent(String name, Task.TaskRequesterComponent element) throws IOException { 40360 if (element != null) { 40361 open(name); 40362 composeTaskTaskRequesterComponentInner(element); 40363 close(); 40364 } 40365 } 40366 40367 protected void composeTaskTaskRequesterComponentInner(Task.TaskRequesterComponent element) throws IOException { 40368 composeBackbone(element); 40369 if (element.hasAgent()) { 40370 composeReference("agent", element.getAgent()); 40371 } 40372 if (element.hasOnBehalfOf()) { 40373 composeReference("onBehalfOf", element.getOnBehalfOf()); 40374 } 40375 } 40376 40377 protected void composeTaskTaskRestrictionComponent(String name, Task.TaskRestrictionComponent element) throws IOException { 40378 if (element != null) { 40379 open(name); 40380 composeTaskTaskRestrictionComponentInner(element); 40381 close(); 40382 } 40383 } 40384 40385 protected void composeTaskTaskRestrictionComponentInner(Task.TaskRestrictionComponent element) throws IOException { 40386 composeBackbone(element); 40387 if (element.hasRepetitionsElement()) { 40388 composePositiveIntCore("repetitions", element.getRepetitionsElement(), false); 40389 composePositiveIntExtras("repetitions", element.getRepetitionsElement(), false); 40390 } 40391 if (element.hasPeriod()) { 40392 composePeriod("period", element.getPeriod()); 40393 } 40394 if (element.hasRecipient()) { 40395 openArray("recipient"); 40396 for (Reference e : element.getRecipient()) 40397 composeReference(null, e); 40398 closeArray(); 40399 }; 40400 } 40401 40402 protected void composeTaskParameterComponent(String name, Task.ParameterComponent element) throws IOException { 40403 if (element != null) { 40404 open(name); 40405 composeTaskParameterComponentInner(element); 40406 close(); 40407 } 40408 } 40409 40410 protected void composeTaskParameterComponentInner(Task.ParameterComponent element) throws IOException { 40411 composeBackbone(element); 40412 if (element.hasType()) { 40413 composeCodeableConcept("type", element.getType()); 40414 } 40415 if (element.hasValue()) { 40416 composeType("value", element.getValue()); 40417 } 40418 } 40419 40420 protected void composeTaskTaskOutputComponent(String name, Task.TaskOutputComponent element) throws IOException { 40421 if (element != null) { 40422 open(name); 40423 composeTaskTaskOutputComponentInner(element); 40424 close(); 40425 } 40426 } 40427 40428 protected void composeTaskTaskOutputComponentInner(Task.TaskOutputComponent element) throws IOException { 40429 composeBackbone(element); 40430 if (element.hasType()) { 40431 composeCodeableConcept("type", element.getType()); 40432 } 40433 if (element.hasValue()) { 40434 composeType("value", element.getValue()); 40435 } 40436 } 40437 40438 protected void composeTestReport(String name, TestReport element) throws IOException { 40439 if (element != null) { 40440 prop("resourceType", name); 40441 composeTestReportInner(element); 40442 } 40443 } 40444 40445 protected void composeTestReportInner(TestReport element) throws IOException { 40446 composeDomainResourceElements(element); 40447 if (element.hasIdentifier()) { 40448 composeIdentifier("identifier", element.getIdentifier()); 40449 } 40450 if (element.hasNameElement()) { 40451 composeStringCore("name", element.getNameElement(), false); 40452 composeStringExtras("name", element.getNameElement(), false); 40453 } 40454 if (element.hasStatusElement()) { 40455 composeEnumerationCore("status", element.getStatusElement(), new TestReport.TestReportStatusEnumFactory(), false); 40456 composeEnumerationExtras("status", element.getStatusElement(), new TestReport.TestReportStatusEnumFactory(), false); 40457 } 40458 if (element.hasTestScript()) { 40459 composeReference("testScript", element.getTestScript()); 40460 } 40461 if (element.hasResultElement()) { 40462 composeEnumerationCore("result", element.getResultElement(), new TestReport.TestReportResultEnumFactory(), false); 40463 composeEnumerationExtras("result", element.getResultElement(), new TestReport.TestReportResultEnumFactory(), false); 40464 } 40465 if (element.hasScoreElement()) { 40466 composeDecimalCore("score", element.getScoreElement(), false); 40467 composeDecimalExtras("score", element.getScoreElement(), false); 40468 } 40469 if (element.hasTesterElement()) { 40470 composeStringCore("tester", element.getTesterElement(), false); 40471 composeStringExtras("tester", element.getTesterElement(), false); 40472 } 40473 if (element.hasIssuedElement()) { 40474 composeDateTimeCore("issued", element.getIssuedElement(), false); 40475 composeDateTimeExtras("issued", element.getIssuedElement(), false); 40476 } 40477 if (element.hasParticipant()) { 40478 openArray("participant"); 40479 for (TestReport.TestReportParticipantComponent e : element.getParticipant()) 40480 composeTestReportTestReportParticipantComponent(null, e); 40481 closeArray(); 40482 }; 40483 if (element.hasSetup()) { 40484 composeTestReportTestReportSetupComponent("setup", element.getSetup()); 40485 } 40486 if (element.hasTest()) { 40487 openArray("test"); 40488 for (TestReport.TestReportTestComponent e : element.getTest()) 40489 composeTestReportTestReportTestComponent(null, e); 40490 closeArray(); 40491 }; 40492 if (element.hasTeardown()) { 40493 composeTestReportTestReportTeardownComponent("teardown", element.getTeardown()); 40494 } 40495 } 40496 40497 protected void composeTestReportTestReportParticipantComponent(String name, TestReport.TestReportParticipantComponent element) throws IOException { 40498 if (element != null) { 40499 open(name); 40500 composeTestReportTestReportParticipantComponentInner(element); 40501 close(); 40502 } 40503 } 40504 40505 protected void composeTestReportTestReportParticipantComponentInner(TestReport.TestReportParticipantComponent element) throws IOException { 40506 composeBackbone(element); 40507 if (element.hasTypeElement()) { 40508 composeEnumerationCore("type", element.getTypeElement(), new TestReport.TestReportParticipantTypeEnumFactory(), false); 40509 composeEnumerationExtras("type", element.getTypeElement(), new TestReport.TestReportParticipantTypeEnumFactory(), false); 40510 } 40511 if (element.hasUriElement()) { 40512 composeUriCore("uri", element.getUriElement(), false); 40513 composeUriExtras("uri", element.getUriElement(), false); 40514 } 40515 if (element.hasDisplayElement()) { 40516 composeStringCore("display", element.getDisplayElement(), false); 40517 composeStringExtras("display", element.getDisplayElement(), false); 40518 } 40519 } 40520 40521 protected void composeTestReportTestReportSetupComponent(String name, TestReport.TestReportSetupComponent element) throws IOException { 40522 if (element != null) { 40523 open(name); 40524 composeTestReportTestReportSetupComponentInner(element); 40525 close(); 40526 } 40527 } 40528 40529 protected void composeTestReportTestReportSetupComponentInner(TestReport.TestReportSetupComponent element) throws IOException { 40530 composeBackbone(element); 40531 if (element.hasAction()) { 40532 openArray("action"); 40533 for (TestReport.SetupActionComponent e : element.getAction()) 40534 composeTestReportSetupActionComponent(null, e); 40535 closeArray(); 40536 }; 40537 } 40538 40539 protected void composeTestReportSetupActionComponent(String name, TestReport.SetupActionComponent element) throws IOException { 40540 if (element != null) { 40541 open(name); 40542 composeTestReportSetupActionComponentInner(element); 40543 close(); 40544 } 40545 } 40546 40547 protected void composeTestReportSetupActionComponentInner(TestReport.SetupActionComponent element) throws IOException { 40548 composeBackbone(element); 40549 if (element.hasOperation()) { 40550 composeTestReportSetupActionOperationComponent("operation", element.getOperation()); 40551 } 40552 if (element.hasAssert()) { 40553 composeTestReportSetupActionAssertComponent("assert", element.getAssert()); 40554 } 40555 } 40556 40557 protected void composeTestReportSetupActionOperationComponent(String name, TestReport.SetupActionOperationComponent element) throws IOException { 40558 if (element != null) { 40559 open(name); 40560 composeTestReportSetupActionOperationComponentInner(element); 40561 close(); 40562 } 40563 } 40564 40565 protected void composeTestReportSetupActionOperationComponentInner(TestReport.SetupActionOperationComponent element) throws IOException { 40566 composeBackbone(element); 40567 if (element.hasResultElement()) { 40568 composeEnumerationCore("result", element.getResultElement(), new TestReport.TestReportActionResultEnumFactory(), false); 40569 composeEnumerationExtras("result", element.getResultElement(), new TestReport.TestReportActionResultEnumFactory(), false); 40570 } 40571 if (element.hasMessageElement()) { 40572 composeMarkdownCore("message", element.getMessageElement(), false); 40573 composeMarkdownExtras("message", element.getMessageElement(), false); 40574 } 40575 if (element.hasDetailElement()) { 40576 composeUriCore("detail", element.getDetailElement(), false); 40577 composeUriExtras("detail", element.getDetailElement(), false); 40578 } 40579 } 40580 40581 protected void composeTestReportSetupActionAssertComponent(String name, TestReport.SetupActionAssertComponent element) throws IOException { 40582 if (element != null) { 40583 open(name); 40584 composeTestReportSetupActionAssertComponentInner(element); 40585 close(); 40586 } 40587 } 40588 40589 protected void composeTestReportSetupActionAssertComponentInner(TestReport.SetupActionAssertComponent element) throws IOException { 40590 composeBackbone(element); 40591 if (element.hasResultElement()) { 40592 composeEnumerationCore("result", element.getResultElement(), new TestReport.TestReportActionResultEnumFactory(), false); 40593 composeEnumerationExtras("result", element.getResultElement(), new TestReport.TestReportActionResultEnumFactory(), false); 40594 } 40595 if (element.hasMessageElement()) { 40596 composeMarkdownCore("message", element.getMessageElement(), false); 40597 composeMarkdownExtras("message", element.getMessageElement(), false); 40598 } 40599 if (element.hasDetailElement()) { 40600 composeStringCore("detail", element.getDetailElement(), false); 40601 composeStringExtras("detail", element.getDetailElement(), false); 40602 } 40603 } 40604 40605 protected void composeTestReportTestReportTestComponent(String name, TestReport.TestReportTestComponent element) throws IOException { 40606 if (element != null) { 40607 open(name); 40608 composeTestReportTestReportTestComponentInner(element); 40609 close(); 40610 } 40611 } 40612 40613 protected void composeTestReportTestReportTestComponentInner(TestReport.TestReportTestComponent element) throws IOException { 40614 composeBackbone(element); 40615 if (element.hasNameElement()) { 40616 composeStringCore("name", element.getNameElement(), false); 40617 composeStringExtras("name", element.getNameElement(), false); 40618 } 40619 if (element.hasDescriptionElement()) { 40620 composeStringCore("description", element.getDescriptionElement(), false); 40621 composeStringExtras("description", element.getDescriptionElement(), false); 40622 } 40623 if (element.hasAction()) { 40624 openArray("action"); 40625 for (TestReport.TestActionComponent e : element.getAction()) 40626 composeTestReportTestActionComponent(null, e); 40627 closeArray(); 40628 }; 40629 } 40630 40631 protected void composeTestReportTestActionComponent(String name, TestReport.TestActionComponent element) throws IOException { 40632 if (element != null) { 40633 open(name); 40634 composeTestReportTestActionComponentInner(element); 40635 close(); 40636 } 40637 } 40638 40639 protected void composeTestReportTestActionComponentInner(TestReport.TestActionComponent element) throws IOException { 40640 composeBackbone(element); 40641 if (element.hasOperation()) { 40642 composeTestReportSetupActionOperationComponent("operation", element.getOperation()); 40643 } 40644 if (element.hasAssert()) { 40645 composeTestReportSetupActionAssertComponent("assert", element.getAssert()); 40646 } 40647 } 40648 40649 protected void composeTestReportTestReportTeardownComponent(String name, TestReport.TestReportTeardownComponent element) throws IOException { 40650 if (element != null) { 40651 open(name); 40652 composeTestReportTestReportTeardownComponentInner(element); 40653 close(); 40654 } 40655 } 40656 40657 protected void composeTestReportTestReportTeardownComponentInner(TestReport.TestReportTeardownComponent element) throws IOException { 40658 composeBackbone(element); 40659 if (element.hasAction()) { 40660 openArray("action"); 40661 for (TestReport.TeardownActionComponent e : element.getAction()) 40662 composeTestReportTeardownActionComponent(null, e); 40663 closeArray(); 40664 }; 40665 } 40666 40667 protected void composeTestReportTeardownActionComponent(String name, TestReport.TeardownActionComponent element) throws IOException { 40668 if (element != null) { 40669 open(name); 40670 composeTestReportTeardownActionComponentInner(element); 40671 close(); 40672 } 40673 } 40674 40675 protected void composeTestReportTeardownActionComponentInner(TestReport.TeardownActionComponent element) throws IOException { 40676 composeBackbone(element); 40677 if (element.hasOperation()) { 40678 composeTestReportSetupActionOperationComponent("operation", element.getOperation()); 40679 } 40680 } 40681 40682 protected void composeTestScript(String name, TestScript element) throws IOException { 40683 if (element != null) { 40684 prop("resourceType", name); 40685 composeTestScriptInner(element); 40686 } 40687 } 40688 40689 protected void composeTestScriptInner(TestScript element) throws IOException { 40690 composeDomainResourceElements(element); 40691 if (element.hasUrlElement()) { 40692 composeUriCore("url", element.getUrlElement(), false); 40693 composeUriExtras("url", element.getUrlElement(), false); 40694 } 40695 if (element.hasIdentifier()) { 40696 composeIdentifier("identifier", element.getIdentifier()); 40697 } 40698 if (element.hasVersionElement()) { 40699 composeStringCore("version", element.getVersionElement(), false); 40700 composeStringExtras("version", element.getVersionElement(), false); 40701 } 40702 if (element.hasNameElement()) { 40703 composeStringCore("name", element.getNameElement(), false); 40704 composeStringExtras("name", element.getNameElement(), false); 40705 } 40706 if (element.hasTitleElement()) { 40707 composeStringCore("title", element.getTitleElement(), false); 40708 composeStringExtras("title", element.getTitleElement(), false); 40709 } 40710 if (element.hasStatusElement()) { 40711 composeEnumerationCore("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 40712 composeEnumerationExtras("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 40713 } 40714 if (element.hasExperimentalElement()) { 40715 composeBooleanCore("experimental", element.getExperimentalElement(), false); 40716 composeBooleanExtras("experimental", element.getExperimentalElement(), false); 40717 } 40718 if (element.hasDateElement()) { 40719 composeDateTimeCore("date", element.getDateElement(), false); 40720 composeDateTimeExtras("date", element.getDateElement(), false); 40721 } 40722 if (element.hasPublisherElement()) { 40723 composeStringCore("publisher", element.getPublisherElement(), false); 40724 composeStringExtras("publisher", element.getPublisherElement(), false); 40725 } 40726 if (element.hasContact()) { 40727 openArray("contact"); 40728 for (ContactDetail e : element.getContact()) 40729 composeContactDetail(null, e); 40730 closeArray(); 40731 }; 40732 if (element.hasDescriptionElement()) { 40733 composeMarkdownCore("description", element.getDescriptionElement(), false); 40734 composeMarkdownExtras("description", element.getDescriptionElement(), false); 40735 } 40736 if (element.hasUseContext()) { 40737 openArray("useContext"); 40738 for (UsageContext e : element.getUseContext()) 40739 composeUsageContext(null, e); 40740 closeArray(); 40741 }; 40742 if (element.hasJurisdiction()) { 40743 openArray("jurisdiction"); 40744 for (CodeableConcept e : element.getJurisdiction()) 40745 composeCodeableConcept(null, e); 40746 closeArray(); 40747 }; 40748 if (element.hasPurposeElement()) { 40749 composeMarkdownCore("purpose", element.getPurposeElement(), false); 40750 composeMarkdownExtras("purpose", element.getPurposeElement(), false); 40751 } 40752 if (element.hasCopyrightElement()) { 40753 composeMarkdownCore("copyright", element.getCopyrightElement(), false); 40754 composeMarkdownExtras("copyright", element.getCopyrightElement(), false); 40755 } 40756 if (element.hasOrigin()) { 40757 openArray("origin"); 40758 for (TestScript.TestScriptOriginComponent e : element.getOrigin()) 40759 composeTestScriptTestScriptOriginComponent(null, e); 40760 closeArray(); 40761 }; 40762 if (element.hasDestination()) { 40763 openArray("destination"); 40764 for (TestScript.TestScriptDestinationComponent e : element.getDestination()) 40765 composeTestScriptTestScriptDestinationComponent(null, e); 40766 closeArray(); 40767 }; 40768 if (element.hasMetadata()) { 40769 composeTestScriptTestScriptMetadataComponent("metadata", element.getMetadata()); 40770 } 40771 if (element.hasFixture()) { 40772 openArray("fixture"); 40773 for (TestScript.TestScriptFixtureComponent e : element.getFixture()) 40774 composeTestScriptTestScriptFixtureComponent(null, e); 40775 closeArray(); 40776 }; 40777 if (element.hasProfile()) { 40778 openArray("profile"); 40779 for (Reference e : element.getProfile()) 40780 composeReference(null, e); 40781 closeArray(); 40782 }; 40783 if (element.hasVariable()) { 40784 openArray("variable"); 40785 for (TestScript.TestScriptVariableComponent e : element.getVariable()) 40786 composeTestScriptTestScriptVariableComponent(null, e); 40787 closeArray(); 40788 }; 40789 if (element.hasRule()) { 40790 openArray("rule"); 40791 for (TestScript.TestScriptRuleComponent e : element.getRule()) 40792 composeTestScriptTestScriptRuleComponent(null, e); 40793 closeArray(); 40794 }; 40795 if (element.hasRuleset()) { 40796 openArray("ruleset"); 40797 for (TestScript.TestScriptRulesetComponent e : element.getRuleset()) 40798 composeTestScriptTestScriptRulesetComponent(null, e); 40799 closeArray(); 40800 }; 40801 if (element.hasSetup()) { 40802 composeTestScriptTestScriptSetupComponent("setup", element.getSetup()); 40803 } 40804 if (element.hasTest()) { 40805 openArray("test"); 40806 for (TestScript.TestScriptTestComponent e : element.getTest()) 40807 composeTestScriptTestScriptTestComponent(null, e); 40808 closeArray(); 40809 }; 40810 if (element.hasTeardown()) { 40811 composeTestScriptTestScriptTeardownComponent("teardown", element.getTeardown()); 40812 } 40813 } 40814 40815 protected void composeTestScriptTestScriptOriginComponent(String name, TestScript.TestScriptOriginComponent element) throws IOException { 40816 if (element != null) { 40817 open(name); 40818 composeTestScriptTestScriptOriginComponentInner(element); 40819 close(); 40820 } 40821 } 40822 40823 protected void composeTestScriptTestScriptOriginComponentInner(TestScript.TestScriptOriginComponent element) throws IOException { 40824 composeBackbone(element); 40825 if (element.hasIndexElement()) { 40826 composeIntegerCore("index", element.getIndexElement(), false); 40827 composeIntegerExtras("index", element.getIndexElement(), false); 40828 } 40829 if (element.hasProfile()) { 40830 composeCoding("profile", element.getProfile()); 40831 } 40832 } 40833 40834 protected void composeTestScriptTestScriptDestinationComponent(String name, TestScript.TestScriptDestinationComponent element) throws IOException { 40835 if (element != null) { 40836 open(name); 40837 composeTestScriptTestScriptDestinationComponentInner(element); 40838 close(); 40839 } 40840 } 40841 40842 protected void composeTestScriptTestScriptDestinationComponentInner(TestScript.TestScriptDestinationComponent element) throws IOException { 40843 composeBackbone(element); 40844 if (element.hasIndexElement()) { 40845 composeIntegerCore("index", element.getIndexElement(), false); 40846 composeIntegerExtras("index", element.getIndexElement(), false); 40847 } 40848 if (element.hasProfile()) { 40849 composeCoding("profile", element.getProfile()); 40850 } 40851 } 40852 40853 protected void composeTestScriptTestScriptMetadataComponent(String name, TestScript.TestScriptMetadataComponent element) throws IOException { 40854 if (element != null) { 40855 open(name); 40856 composeTestScriptTestScriptMetadataComponentInner(element); 40857 close(); 40858 } 40859 } 40860 40861 protected void composeTestScriptTestScriptMetadataComponentInner(TestScript.TestScriptMetadataComponent element) throws IOException { 40862 composeBackbone(element); 40863 if (element.hasLink()) { 40864 openArray("link"); 40865 for (TestScript.TestScriptMetadataLinkComponent e : element.getLink()) 40866 composeTestScriptTestScriptMetadataLinkComponent(null, e); 40867 closeArray(); 40868 }; 40869 if (element.hasCapability()) { 40870 openArray("capability"); 40871 for (TestScript.TestScriptMetadataCapabilityComponent e : element.getCapability()) 40872 composeTestScriptTestScriptMetadataCapabilityComponent(null, e); 40873 closeArray(); 40874 }; 40875 } 40876 40877 protected void composeTestScriptTestScriptMetadataLinkComponent(String name, TestScript.TestScriptMetadataLinkComponent element) throws IOException { 40878 if (element != null) { 40879 open(name); 40880 composeTestScriptTestScriptMetadataLinkComponentInner(element); 40881 close(); 40882 } 40883 } 40884 40885 protected void composeTestScriptTestScriptMetadataLinkComponentInner(TestScript.TestScriptMetadataLinkComponent element) throws IOException { 40886 composeBackbone(element); 40887 if (element.hasUrlElement()) { 40888 composeUriCore("url", element.getUrlElement(), false); 40889 composeUriExtras("url", element.getUrlElement(), false); 40890 } 40891 if (element.hasDescriptionElement()) { 40892 composeStringCore("description", element.getDescriptionElement(), false); 40893 composeStringExtras("description", element.getDescriptionElement(), false); 40894 } 40895 } 40896 40897 protected void composeTestScriptTestScriptMetadataCapabilityComponent(String name, TestScript.TestScriptMetadataCapabilityComponent element) throws IOException { 40898 if (element != null) { 40899 open(name); 40900 composeTestScriptTestScriptMetadataCapabilityComponentInner(element); 40901 close(); 40902 } 40903 } 40904 40905 protected void composeTestScriptTestScriptMetadataCapabilityComponentInner(TestScript.TestScriptMetadataCapabilityComponent element) throws IOException { 40906 composeBackbone(element); 40907 if (element.hasRequiredElement()) { 40908 composeBooleanCore("required", element.getRequiredElement(), false); 40909 composeBooleanExtras("required", element.getRequiredElement(), false); 40910 } 40911 if (element.hasValidatedElement()) { 40912 composeBooleanCore("validated", element.getValidatedElement(), false); 40913 composeBooleanExtras("validated", element.getValidatedElement(), false); 40914 } 40915 if (element.hasDescriptionElement()) { 40916 composeStringCore("description", element.getDescriptionElement(), false); 40917 composeStringExtras("description", element.getDescriptionElement(), false); 40918 } 40919 if (element.hasOrigin()) { 40920 openArray("origin"); 40921 for (IntegerType e : element.getOrigin()) 40922 composeIntegerCore(null, e, true); 40923 closeArray(); 40924 if (anyHasExtras(element.getOrigin())) { 40925 openArray("_origin"); 40926 for (IntegerType e : element.getOrigin()) 40927 composeIntegerExtras(null, e, true); 40928 closeArray(); 40929 } 40930 }; 40931 if (element.hasDestinationElement()) { 40932 composeIntegerCore("destination", element.getDestinationElement(), false); 40933 composeIntegerExtras("destination", element.getDestinationElement(), false); 40934 } 40935 if (element.hasLink()) { 40936 openArray("link"); 40937 for (UriType e : element.getLink()) 40938 composeUriCore(null, e, true); 40939 closeArray(); 40940 if (anyHasExtras(element.getLink())) { 40941 openArray("_link"); 40942 for (UriType e : element.getLink()) 40943 composeUriExtras(null, e, true); 40944 closeArray(); 40945 } 40946 }; 40947 if (element.hasCapabilities()) { 40948 composeReference("capabilities", element.getCapabilities()); 40949 } 40950 } 40951 40952 protected void composeTestScriptTestScriptFixtureComponent(String name, TestScript.TestScriptFixtureComponent element) throws IOException { 40953 if (element != null) { 40954 open(name); 40955 composeTestScriptTestScriptFixtureComponentInner(element); 40956 close(); 40957 } 40958 } 40959 40960 protected void composeTestScriptTestScriptFixtureComponentInner(TestScript.TestScriptFixtureComponent element) throws IOException { 40961 composeBackbone(element); 40962 if (element.hasAutocreateElement()) { 40963 composeBooleanCore("autocreate", element.getAutocreateElement(), false); 40964 composeBooleanExtras("autocreate", element.getAutocreateElement(), false); 40965 } 40966 if (element.hasAutodeleteElement()) { 40967 composeBooleanCore("autodelete", element.getAutodeleteElement(), false); 40968 composeBooleanExtras("autodelete", element.getAutodeleteElement(), false); 40969 } 40970 if (element.hasResource()) { 40971 composeReference("resource", element.getResource()); 40972 } 40973 } 40974 40975 protected void composeTestScriptTestScriptVariableComponent(String name, TestScript.TestScriptVariableComponent element) throws IOException { 40976 if (element != null) { 40977 open(name); 40978 composeTestScriptTestScriptVariableComponentInner(element); 40979 close(); 40980 } 40981 } 40982 40983 protected void composeTestScriptTestScriptVariableComponentInner(TestScript.TestScriptVariableComponent element) throws IOException { 40984 composeBackbone(element); 40985 if (element.hasNameElement()) { 40986 composeStringCore("name", element.getNameElement(), false); 40987 composeStringExtras("name", element.getNameElement(), false); 40988 } 40989 if (element.hasDefaultValueElement()) { 40990 composeStringCore("defaultValue", element.getDefaultValueElement(), false); 40991 composeStringExtras("defaultValue", element.getDefaultValueElement(), false); 40992 } 40993 if (element.hasDescriptionElement()) { 40994 composeStringCore("description", element.getDescriptionElement(), false); 40995 composeStringExtras("description", element.getDescriptionElement(), false); 40996 } 40997 if (element.hasExpressionElement()) { 40998 composeStringCore("expression", element.getExpressionElement(), false); 40999 composeStringExtras("expression", element.getExpressionElement(), false); 41000 } 41001 if (element.hasHeaderFieldElement()) { 41002 composeStringCore("headerField", element.getHeaderFieldElement(), false); 41003 composeStringExtras("headerField", element.getHeaderFieldElement(), false); 41004 } 41005 if (element.hasHintElement()) { 41006 composeStringCore("hint", element.getHintElement(), false); 41007 composeStringExtras("hint", element.getHintElement(), false); 41008 } 41009 if (element.hasPathElement()) { 41010 composeStringCore("path", element.getPathElement(), false); 41011 composeStringExtras("path", element.getPathElement(), false); 41012 } 41013 if (element.hasSourceIdElement()) { 41014 composeIdCore("sourceId", element.getSourceIdElement(), false); 41015 composeIdExtras("sourceId", element.getSourceIdElement(), false); 41016 } 41017 } 41018 41019 protected void composeTestScriptTestScriptRuleComponent(String name, TestScript.TestScriptRuleComponent element) throws IOException { 41020 if (element != null) { 41021 open(name); 41022 composeTestScriptTestScriptRuleComponentInner(element); 41023 close(); 41024 } 41025 } 41026 41027 protected void composeTestScriptTestScriptRuleComponentInner(TestScript.TestScriptRuleComponent element) throws IOException { 41028 composeBackbone(element); 41029 if (element.hasResource()) { 41030 composeReference("resource", element.getResource()); 41031 } 41032 if (element.hasParam()) { 41033 openArray("param"); 41034 for (TestScript.RuleParamComponent e : element.getParam()) 41035 composeTestScriptRuleParamComponent(null, e); 41036 closeArray(); 41037 }; 41038 } 41039 41040 protected void composeTestScriptRuleParamComponent(String name, TestScript.RuleParamComponent element) throws IOException { 41041 if (element != null) { 41042 open(name); 41043 composeTestScriptRuleParamComponentInner(element); 41044 close(); 41045 } 41046 } 41047 41048 protected void composeTestScriptRuleParamComponentInner(TestScript.RuleParamComponent element) throws IOException { 41049 composeBackbone(element); 41050 if (element.hasNameElement()) { 41051 composeStringCore("name", element.getNameElement(), false); 41052 composeStringExtras("name", element.getNameElement(), false); 41053 } 41054 if (element.hasValueElement()) { 41055 composeStringCore("value", element.getValueElement(), false); 41056 composeStringExtras("value", element.getValueElement(), false); 41057 } 41058 } 41059 41060 protected void composeTestScriptTestScriptRulesetComponent(String name, TestScript.TestScriptRulesetComponent element) throws IOException { 41061 if (element != null) { 41062 open(name); 41063 composeTestScriptTestScriptRulesetComponentInner(element); 41064 close(); 41065 } 41066 } 41067 41068 protected void composeTestScriptTestScriptRulesetComponentInner(TestScript.TestScriptRulesetComponent element) throws IOException { 41069 composeBackbone(element); 41070 if (element.hasResource()) { 41071 composeReference("resource", element.getResource()); 41072 } 41073 if (element.hasRule()) { 41074 openArray("rule"); 41075 for (TestScript.RulesetRuleComponent e : element.getRule()) 41076 composeTestScriptRulesetRuleComponent(null, e); 41077 closeArray(); 41078 }; 41079 } 41080 41081 protected void composeTestScriptRulesetRuleComponent(String name, TestScript.RulesetRuleComponent element) throws IOException { 41082 if (element != null) { 41083 open(name); 41084 composeTestScriptRulesetRuleComponentInner(element); 41085 close(); 41086 } 41087 } 41088 41089 protected void composeTestScriptRulesetRuleComponentInner(TestScript.RulesetRuleComponent element) throws IOException { 41090 composeBackbone(element); 41091 if (element.hasRuleIdElement()) { 41092 composeIdCore("ruleId", element.getRuleIdElement(), false); 41093 composeIdExtras("ruleId", element.getRuleIdElement(), false); 41094 } 41095 if (element.hasParam()) { 41096 openArray("param"); 41097 for (TestScript.RulesetRuleParamComponent e : element.getParam()) 41098 composeTestScriptRulesetRuleParamComponent(null, e); 41099 closeArray(); 41100 }; 41101 } 41102 41103 protected void composeTestScriptRulesetRuleParamComponent(String name, TestScript.RulesetRuleParamComponent element) throws IOException { 41104 if (element != null) { 41105 open(name); 41106 composeTestScriptRulesetRuleParamComponentInner(element); 41107 close(); 41108 } 41109 } 41110 41111 protected void composeTestScriptRulesetRuleParamComponentInner(TestScript.RulesetRuleParamComponent element) throws IOException { 41112 composeBackbone(element); 41113 if (element.hasNameElement()) { 41114 composeStringCore("name", element.getNameElement(), false); 41115 composeStringExtras("name", element.getNameElement(), false); 41116 } 41117 if (element.hasValueElement()) { 41118 composeStringCore("value", element.getValueElement(), false); 41119 composeStringExtras("value", element.getValueElement(), false); 41120 } 41121 } 41122 41123 protected void composeTestScriptTestScriptSetupComponent(String name, TestScript.TestScriptSetupComponent element) throws IOException { 41124 if (element != null) { 41125 open(name); 41126 composeTestScriptTestScriptSetupComponentInner(element); 41127 close(); 41128 } 41129 } 41130 41131 protected void composeTestScriptTestScriptSetupComponentInner(TestScript.TestScriptSetupComponent element) throws IOException { 41132 composeBackbone(element); 41133 if (element.hasAction()) { 41134 openArray("action"); 41135 for (TestScript.SetupActionComponent e : element.getAction()) 41136 composeTestScriptSetupActionComponent(null, e); 41137 closeArray(); 41138 }; 41139 } 41140 41141 protected void composeTestScriptSetupActionComponent(String name, TestScript.SetupActionComponent element) throws IOException { 41142 if (element != null) { 41143 open(name); 41144 composeTestScriptSetupActionComponentInner(element); 41145 close(); 41146 } 41147 } 41148 41149 protected void composeTestScriptSetupActionComponentInner(TestScript.SetupActionComponent element) throws IOException { 41150 composeBackbone(element); 41151 if (element.hasOperation()) { 41152 composeTestScriptSetupActionOperationComponent("operation", element.getOperation()); 41153 } 41154 if (element.hasAssert()) { 41155 composeTestScriptSetupActionAssertComponent("assert", element.getAssert()); 41156 } 41157 } 41158 41159 protected void composeTestScriptSetupActionOperationComponent(String name, TestScript.SetupActionOperationComponent element) throws IOException { 41160 if (element != null) { 41161 open(name); 41162 composeTestScriptSetupActionOperationComponentInner(element); 41163 close(); 41164 } 41165 } 41166 41167 protected void composeTestScriptSetupActionOperationComponentInner(TestScript.SetupActionOperationComponent element) throws IOException { 41168 composeBackbone(element); 41169 if (element.hasType()) { 41170 composeCoding("type", element.getType()); 41171 } 41172 if (element.hasResourceElement()) { 41173 composeCodeCore("resource", element.getResourceElement(), false); 41174 composeCodeExtras("resource", element.getResourceElement(), false); 41175 } 41176 if (element.hasLabelElement()) { 41177 composeStringCore("label", element.getLabelElement(), false); 41178 composeStringExtras("label", element.getLabelElement(), false); 41179 } 41180 if (element.hasDescriptionElement()) { 41181 composeStringCore("description", element.getDescriptionElement(), false); 41182 composeStringExtras("description", element.getDescriptionElement(), false); 41183 } 41184 if (element.hasAcceptElement()) { 41185 composeEnumerationCore("accept", element.getAcceptElement(), new TestScript.ContentTypeEnumFactory(), false); 41186 composeEnumerationExtras("accept", element.getAcceptElement(), new TestScript.ContentTypeEnumFactory(), false); 41187 } 41188 if (element.hasContentTypeElement()) { 41189 composeEnumerationCore("contentType", element.getContentTypeElement(), new TestScript.ContentTypeEnumFactory(), false); 41190 composeEnumerationExtras("contentType", element.getContentTypeElement(), new TestScript.ContentTypeEnumFactory(), false); 41191 } 41192 if (element.hasDestinationElement()) { 41193 composeIntegerCore("destination", element.getDestinationElement(), false); 41194 composeIntegerExtras("destination", element.getDestinationElement(), false); 41195 } 41196 if (element.hasEncodeRequestUrlElement()) { 41197 composeBooleanCore("encodeRequestUrl", element.getEncodeRequestUrlElement(), false); 41198 composeBooleanExtras("encodeRequestUrl", element.getEncodeRequestUrlElement(), false); 41199 } 41200 if (element.hasOriginElement()) { 41201 composeIntegerCore("origin", element.getOriginElement(), false); 41202 composeIntegerExtras("origin", element.getOriginElement(), false); 41203 } 41204 if (element.hasParamsElement()) { 41205 composeStringCore("params", element.getParamsElement(), false); 41206 composeStringExtras("params", element.getParamsElement(), false); 41207 } 41208 if (element.hasRequestHeader()) { 41209 openArray("requestHeader"); 41210 for (TestScript.SetupActionOperationRequestHeaderComponent e : element.getRequestHeader()) 41211 composeTestScriptSetupActionOperationRequestHeaderComponent(null, e); 41212 closeArray(); 41213 }; 41214 if (element.hasRequestIdElement()) { 41215 composeIdCore("requestId", element.getRequestIdElement(), false); 41216 composeIdExtras("requestId", element.getRequestIdElement(), false); 41217 } 41218 if (element.hasResponseIdElement()) { 41219 composeIdCore("responseId", element.getResponseIdElement(), false); 41220 composeIdExtras("responseId", element.getResponseIdElement(), false); 41221 } 41222 if (element.hasSourceIdElement()) { 41223 composeIdCore("sourceId", element.getSourceIdElement(), false); 41224 composeIdExtras("sourceId", element.getSourceIdElement(), false); 41225 } 41226 if (element.hasTargetIdElement()) { 41227 composeIdCore("targetId", element.getTargetIdElement(), false); 41228 composeIdExtras("targetId", element.getTargetIdElement(), false); 41229 } 41230 if (element.hasUrlElement()) { 41231 composeStringCore("url", element.getUrlElement(), false); 41232 composeStringExtras("url", element.getUrlElement(), false); 41233 } 41234 } 41235 41236 protected void composeTestScriptSetupActionOperationRequestHeaderComponent(String name, TestScript.SetupActionOperationRequestHeaderComponent element) throws IOException { 41237 if (element != null) { 41238 open(name); 41239 composeTestScriptSetupActionOperationRequestHeaderComponentInner(element); 41240 close(); 41241 } 41242 } 41243 41244 protected void composeTestScriptSetupActionOperationRequestHeaderComponentInner(TestScript.SetupActionOperationRequestHeaderComponent element) throws IOException { 41245 composeBackbone(element); 41246 if (element.hasFieldElement()) { 41247 composeStringCore("field", element.getFieldElement(), false); 41248 composeStringExtras("field", element.getFieldElement(), false); 41249 } 41250 if (element.hasValueElement()) { 41251 composeStringCore("value", element.getValueElement(), false); 41252 composeStringExtras("value", element.getValueElement(), false); 41253 } 41254 } 41255 41256 protected void composeTestScriptSetupActionAssertComponent(String name, TestScript.SetupActionAssertComponent element) throws IOException { 41257 if (element != null) { 41258 open(name); 41259 composeTestScriptSetupActionAssertComponentInner(element); 41260 close(); 41261 } 41262 } 41263 41264 protected void composeTestScriptSetupActionAssertComponentInner(TestScript.SetupActionAssertComponent element) throws IOException { 41265 composeBackbone(element); 41266 if (element.hasLabelElement()) { 41267 composeStringCore("label", element.getLabelElement(), false); 41268 composeStringExtras("label", element.getLabelElement(), false); 41269 } 41270 if (element.hasDescriptionElement()) { 41271 composeStringCore("description", element.getDescriptionElement(), false); 41272 composeStringExtras("description", element.getDescriptionElement(), false); 41273 } 41274 if (element.hasDirectionElement()) { 41275 composeEnumerationCore("direction", element.getDirectionElement(), new TestScript.AssertionDirectionTypeEnumFactory(), false); 41276 composeEnumerationExtras("direction", element.getDirectionElement(), new TestScript.AssertionDirectionTypeEnumFactory(), false); 41277 } 41278 if (element.hasCompareToSourceIdElement()) { 41279 composeStringCore("compareToSourceId", element.getCompareToSourceIdElement(), false); 41280 composeStringExtras("compareToSourceId", element.getCompareToSourceIdElement(), false); 41281 } 41282 if (element.hasCompareToSourceExpressionElement()) { 41283 composeStringCore("compareToSourceExpression", element.getCompareToSourceExpressionElement(), false); 41284 composeStringExtras("compareToSourceExpression", element.getCompareToSourceExpressionElement(), false); 41285 } 41286 if (element.hasCompareToSourcePathElement()) { 41287 composeStringCore("compareToSourcePath", element.getCompareToSourcePathElement(), false); 41288 composeStringExtras("compareToSourcePath", element.getCompareToSourcePathElement(), false); 41289 } 41290 if (element.hasContentTypeElement()) { 41291 composeEnumerationCore("contentType", element.getContentTypeElement(), new TestScript.ContentTypeEnumFactory(), false); 41292 composeEnumerationExtras("contentType", element.getContentTypeElement(), new TestScript.ContentTypeEnumFactory(), false); 41293 } 41294 if (element.hasExpressionElement()) { 41295 composeStringCore("expression", element.getExpressionElement(), false); 41296 composeStringExtras("expression", element.getExpressionElement(), false); 41297 } 41298 if (element.hasHeaderFieldElement()) { 41299 composeStringCore("headerField", element.getHeaderFieldElement(), false); 41300 composeStringExtras("headerField", element.getHeaderFieldElement(), false); 41301 } 41302 if (element.hasMinimumIdElement()) { 41303 composeStringCore("minimumId", element.getMinimumIdElement(), false); 41304 composeStringExtras("minimumId", element.getMinimumIdElement(), false); 41305 } 41306 if (element.hasNavigationLinksElement()) { 41307 composeBooleanCore("navigationLinks", element.getNavigationLinksElement(), false); 41308 composeBooleanExtras("navigationLinks", element.getNavigationLinksElement(), false); 41309 } 41310 if (element.hasOperatorElement()) { 41311 composeEnumerationCore("operator", element.getOperatorElement(), new TestScript.AssertionOperatorTypeEnumFactory(), false); 41312 composeEnumerationExtras("operator", element.getOperatorElement(), new TestScript.AssertionOperatorTypeEnumFactory(), false); 41313 } 41314 if (element.hasPathElement()) { 41315 composeStringCore("path", element.getPathElement(), false); 41316 composeStringExtras("path", element.getPathElement(), false); 41317 } 41318 if (element.hasRequestMethodElement()) { 41319 composeEnumerationCore("requestMethod", element.getRequestMethodElement(), new TestScript.TestScriptRequestMethodCodeEnumFactory(), false); 41320 composeEnumerationExtras("requestMethod", element.getRequestMethodElement(), new TestScript.TestScriptRequestMethodCodeEnumFactory(), false); 41321 } 41322 if (element.hasRequestURLElement()) { 41323 composeStringCore("requestURL", element.getRequestURLElement(), false); 41324 composeStringExtras("requestURL", element.getRequestURLElement(), false); 41325 } 41326 if (element.hasResourceElement()) { 41327 composeCodeCore("resource", element.getResourceElement(), false); 41328 composeCodeExtras("resource", element.getResourceElement(), false); 41329 } 41330 if (element.hasResponseElement()) { 41331 composeEnumerationCore("response", element.getResponseElement(), new TestScript.AssertionResponseTypesEnumFactory(), false); 41332 composeEnumerationExtras("response", element.getResponseElement(), new TestScript.AssertionResponseTypesEnumFactory(), false); 41333 } 41334 if (element.hasResponseCodeElement()) { 41335 composeStringCore("responseCode", element.getResponseCodeElement(), false); 41336 composeStringExtras("responseCode", element.getResponseCodeElement(), false); 41337 } 41338 if (element.hasRule()) { 41339 composeTestScriptActionAssertRuleComponent("rule", element.getRule()); 41340 } 41341 if (element.hasRuleset()) { 41342 composeTestScriptActionAssertRulesetComponent("ruleset", element.getRuleset()); 41343 } 41344 if (element.hasSourceIdElement()) { 41345 composeIdCore("sourceId", element.getSourceIdElement(), false); 41346 composeIdExtras("sourceId", element.getSourceIdElement(), false); 41347 } 41348 if (element.hasValidateProfileIdElement()) { 41349 composeIdCore("validateProfileId", element.getValidateProfileIdElement(), false); 41350 composeIdExtras("validateProfileId", element.getValidateProfileIdElement(), false); 41351 } 41352 if (element.hasValueElement()) { 41353 composeStringCore("value", element.getValueElement(), false); 41354 composeStringExtras("value", element.getValueElement(), false); 41355 } 41356 if (element.hasWarningOnlyElement()) { 41357 composeBooleanCore("warningOnly", element.getWarningOnlyElement(), false); 41358 composeBooleanExtras("warningOnly", element.getWarningOnlyElement(), false); 41359 } 41360 } 41361 41362 protected void composeTestScriptActionAssertRuleComponent(String name, TestScript.ActionAssertRuleComponent element) throws IOException { 41363 if (element != null) { 41364 open(name); 41365 composeTestScriptActionAssertRuleComponentInner(element); 41366 close(); 41367 } 41368 } 41369 41370 protected void composeTestScriptActionAssertRuleComponentInner(TestScript.ActionAssertRuleComponent element) throws IOException { 41371 composeBackbone(element); 41372 if (element.hasRuleIdElement()) { 41373 composeIdCore("ruleId", element.getRuleIdElement(), false); 41374 composeIdExtras("ruleId", element.getRuleIdElement(), false); 41375 } 41376 if (element.hasParam()) { 41377 openArray("param"); 41378 for (TestScript.ActionAssertRuleParamComponent e : element.getParam()) 41379 composeTestScriptActionAssertRuleParamComponent(null, e); 41380 closeArray(); 41381 }; 41382 } 41383 41384 protected void composeTestScriptActionAssertRuleParamComponent(String name, TestScript.ActionAssertRuleParamComponent element) throws IOException { 41385 if (element != null) { 41386 open(name); 41387 composeTestScriptActionAssertRuleParamComponentInner(element); 41388 close(); 41389 } 41390 } 41391 41392 protected void composeTestScriptActionAssertRuleParamComponentInner(TestScript.ActionAssertRuleParamComponent element) throws IOException { 41393 composeBackbone(element); 41394 if (element.hasNameElement()) { 41395 composeStringCore("name", element.getNameElement(), false); 41396 composeStringExtras("name", element.getNameElement(), false); 41397 } 41398 if (element.hasValueElement()) { 41399 composeStringCore("value", element.getValueElement(), false); 41400 composeStringExtras("value", element.getValueElement(), false); 41401 } 41402 } 41403 41404 protected void composeTestScriptActionAssertRulesetComponent(String name, TestScript.ActionAssertRulesetComponent element) throws IOException { 41405 if (element != null) { 41406 open(name); 41407 composeTestScriptActionAssertRulesetComponentInner(element); 41408 close(); 41409 } 41410 } 41411 41412 protected void composeTestScriptActionAssertRulesetComponentInner(TestScript.ActionAssertRulesetComponent element) throws IOException { 41413 composeBackbone(element); 41414 if (element.hasRulesetIdElement()) { 41415 composeIdCore("rulesetId", element.getRulesetIdElement(), false); 41416 composeIdExtras("rulesetId", element.getRulesetIdElement(), false); 41417 } 41418 if (element.hasRule()) { 41419 openArray("rule"); 41420 for (TestScript.ActionAssertRulesetRuleComponent e : element.getRule()) 41421 composeTestScriptActionAssertRulesetRuleComponent(null, e); 41422 closeArray(); 41423 }; 41424 } 41425 41426 protected void composeTestScriptActionAssertRulesetRuleComponent(String name, TestScript.ActionAssertRulesetRuleComponent element) throws IOException { 41427 if (element != null) { 41428 open(name); 41429 composeTestScriptActionAssertRulesetRuleComponentInner(element); 41430 close(); 41431 } 41432 } 41433 41434 protected void composeTestScriptActionAssertRulesetRuleComponentInner(TestScript.ActionAssertRulesetRuleComponent element) throws IOException { 41435 composeBackbone(element); 41436 if (element.hasRuleIdElement()) { 41437 composeIdCore("ruleId", element.getRuleIdElement(), false); 41438 composeIdExtras("ruleId", element.getRuleIdElement(), false); 41439 } 41440 if (element.hasParam()) { 41441 openArray("param"); 41442 for (TestScript.ActionAssertRulesetRuleParamComponent e : element.getParam()) 41443 composeTestScriptActionAssertRulesetRuleParamComponent(null, e); 41444 closeArray(); 41445 }; 41446 } 41447 41448 protected void composeTestScriptActionAssertRulesetRuleParamComponent(String name, TestScript.ActionAssertRulesetRuleParamComponent element) throws IOException { 41449 if (element != null) { 41450 open(name); 41451 composeTestScriptActionAssertRulesetRuleParamComponentInner(element); 41452 close(); 41453 } 41454 } 41455 41456 protected void composeTestScriptActionAssertRulesetRuleParamComponentInner(TestScript.ActionAssertRulesetRuleParamComponent element) throws IOException { 41457 composeBackbone(element); 41458 if (element.hasNameElement()) { 41459 composeStringCore("name", element.getNameElement(), false); 41460 composeStringExtras("name", element.getNameElement(), false); 41461 } 41462 if (element.hasValueElement()) { 41463 composeStringCore("value", element.getValueElement(), false); 41464 composeStringExtras("value", element.getValueElement(), false); 41465 } 41466 } 41467 41468 protected void composeTestScriptTestScriptTestComponent(String name, TestScript.TestScriptTestComponent element) throws IOException { 41469 if (element != null) { 41470 open(name); 41471 composeTestScriptTestScriptTestComponentInner(element); 41472 close(); 41473 } 41474 } 41475 41476 protected void composeTestScriptTestScriptTestComponentInner(TestScript.TestScriptTestComponent element) throws IOException { 41477 composeBackbone(element); 41478 if (element.hasNameElement()) { 41479 composeStringCore("name", element.getNameElement(), false); 41480 composeStringExtras("name", element.getNameElement(), false); 41481 } 41482 if (element.hasDescriptionElement()) { 41483 composeStringCore("description", element.getDescriptionElement(), false); 41484 composeStringExtras("description", element.getDescriptionElement(), false); 41485 } 41486 if (element.hasAction()) { 41487 openArray("action"); 41488 for (TestScript.TestActionComponent e : element.getAction()) 41489 composeTestScriptTestActionComponent(null, e); 41490 closeArray(); 41491 }; 41492 } 41493 41494 protected void composeTestScriptTestActionComponent(String name, TestScript.TestActionComponent element) throws IOException { 41495 if (element != null) { 41496 open(name); 41497 composeTestScriptTestActionComponentInner(element); 41498 close(); 41499 } 41500 } 41501 41502 protected void composeTestScriptTestActionComponentInner(TestScript.TestActionComponent element) throws IOException { 41503 composeBackbone(element); 41504 if (element.hasOperation()) { 41505 composeTestScriptSetupActionOperationComponent("operation", element.getOperation()); 41506 } 41507 if (element.hasAssert()) { 41508 composeTestScriptSetupActionAssertComponent("assert", element.getAssert()); 41509 } 41510 } 41511 41512 protected void composeTestScriptTestScriptTeardownComponent(String name, TestScript.TestScriptTeardownComponent element) throws IOException { 41513 if (element != null) { 41514 open(name); 41515 composeTestScriptTestScriptTeardownComponentInner(element); 41516 close(); 41517 } 41518 } 41519 41520 protected void composeTestScriptTestScriptTeardownComponentInner(TestScript.TestScriptTeardownComponent element) throws IOException { 41521 composeBackbone(element); 41522 if (element.hasAction()) { 41523 openArray("action"); 41524 for (TestScript.TeardownActionComponent e : element.getAction()) 41525 composeTestScriptTeardownActionComponent(null, e); 41526 closeArray(); 41527 }; 41528 } 41529 41530 protected void composeTestScriptTeardownActionComponent(String name, TestScript.TeardownActionComponent element) throws IOException { 41531 if (element != null) { 41532 open(name); 41533 composeTestScriptTeardownActionComponentInner(element); 41534 close(); 41535 } 41536 } 41537 41538 protected void composeTestScriptTeardownActionComponentInner(TestScript.TeardownActionComponent element) throws IOException { 41539 composeBackbone(element); 41540 if (element.hasOperation()) { 41541 composeTestScriptSetupActionOperationComponent("operation", element.getOperation()); 41542 } 41543 } 41544 41545 protected void composeValueSet(String name, ValueSet element) throws IOException { 41546 if (element != null) { 41547 prop("resourceType", name); 41548 composeValueSetInner(element); 41549 } 41550 } 41551 41552 protected void composeValueSetInner(ValueSet element) throws IOException { 41553 composeDomainResourceElements(element); 41554 if (element.hasUrlElement()) { 41555 composeUriCore("url", element.getUrlElement(), false); 41556 composeUriExtras("url", element.getUrlElement(), false); 41557 } 41558 if (element.hasIdentifier()) { 41559 openArray("identifier"); 41560 for (Identifier e : element.getIdentifier()) 41561 composeIdentifier(null, e); 41562 closeArray(); 41563 }; 41564 if (element.hasVersionElement()) { 41565 composeStringCore("version", element.getVersionElement(), false); 41566 composeStringExtras("version", element.getVersionElement(), false); 41567 } 41568 if (element.hasNameElement()) { 41569 composeStringCore("name", element.getNameElement(), false); 41570 composeStringExtras("name", element.getNameElement(), false); 41571 } 41572 if (element.hasTitleElement()) { 41573 composeStringCore("title", element.getTitleElement(), false); 41574 composeStringExtras("title", element.getTitleElement(), false); 41575 } 41576 if (element.hasStatusElement()) { 41577 composeEnumerationCore("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 41578 composeEnumerationExtras("status", element.getStatusElement(), new Enumerations.PublicationStatusEnumFactory(), false); 41579 } 41580 if (element.hasExperimentalElement()) { 41581 composeBooleanCore("experimental", element.getExperimentalElement(), false); 41582 composeBooleanExtras("experimental", element.getExperimentalElement(), false); 41583 } 41584 if (element.hasDateElement()) { 41585 composeDateTimeCore("date", element.getDateElement(), false); 41586 composeDateTimeExtras("date", element.getDateElement(), false); 41587 } 41588 if (element.hasPublisherElement()) { 41589 composeStringCore("publisher", element.getPublisherElement(), false); 41590 composeStringExtras("publisher", element.getPublisherElement(), false); 41591 } 41592 if (element.hasContact()) { 41593 openArray("contact"); 41594 for (ContactDetail e : element.getContact()) 41595 composeContactDetail(null, e); 41596 closeArray(); 41597 }; 41598 if (element.hasDescriptionElement()) { 41599 composeMarkdownCore("description", element.getDescriptionElement(), false); 41600 composeMarkdownExtras("description", element.getDescriptionElement(), false); 41601 } 41602 if (element.hasUseContext()) { 41603 openArray("useContext"); 41604 for (UsageContext e : element.getUseContext()) 41605 composeUsageContext(null, e); 41606 closeArray(); 41607 }; 41608 if (element.hasJurisdiction()) { 41609 openArray("jurisdiction"); 41610 for (CodeableConcept e : element.getJurisdiction()) 41611 composeCodeableConcept(null, e); 41612 closeArray(); 41613 }; 41614 if (element.hasImmutableElement()) { 41615 composeBooleanCore("immutable", element.getImmutableElement(), false); 41616 composeBooleanExtras("immutable", element.getImmutableElement(), false); 41617 } 41618 if (element.hasPurposeElement()) { 41619 composeMarkdownCore("purpose", element.getPurposeElement(), false); 41620 composeMarkdownExtras("purpose", element.getPurposeElement(), false); 41621 } 41622 if (element.hasCopyrightElement()) { 41623 composeMarkdownCore("copyright", element.getCopyrightElement(), false); 41624 composeMarkdownExtras("copyright", element.getCopyrightElement(), false); 41625 } 41626 if (element.hasExtensibleElement()) { 41627 composeBooleanCore("extensible", element.getExtensibleElement(), false); 41628 composeBooleanExtras("extensible", element.getExtensibleElement(), false); 41629 } 41630 if (element.hasCompose()) { 41631 composeValueSetValueSetComposeComponent("compose", element.getCompose()); 41632 } 41633 if (element.hasExpansion()) { 41634 composeValueSetValueSetExpansionComponent("expansion", element.getExpansion()); 41635 } 41636 } 41637 41638 protected void composeValueSetValueSetComposeComponent(String name, ValueSet.ValueSetComposeComponent element) throws IOException { 41639 if (element != null) { 41640 open(name); 41641 composeValueSetValueSetComposeComponentInner(element); 41642 close(); 41643 } 41644 } 41645 41646 protected void composeValueSetValueSetComposeComponentInner(ValueSet.ValueSetComposeComponent element) throws IOException { 41647 composeBackbone(element); 41648 if (element.hasLockedDateElement()) { 41649 composeDateCore("lockedDate", element.getLockedDateElement(), false); 41650 composeDateExtras("lockedDate", element.getLockedDateElement(), false); 41651 } 41652 if (element.hasInactiveElement()) { 41653 composeBooleanCore("inactive", element.getInactiveElement(), false); 41654 composeBooleanExtras("inactive", element.getInactiveElement(), false); 41655 } 41656 if (element.hasInclude()) { 41657 openArray("include"); 41658 for (ValueSet.ConceptSetComponent e : element.getInclude()) 41659 composeValueSetConceptSetComponent(null, e); 41660 closeArray(); 41661 }; 41662 if (element.hasExclude()) { 41663 openArray("exclude"); 41664 for (ValueSet.ConceptSetComponent e : element.getExclude()) 41665 composeValueSetConceptSetComponent(null, e); 41666 closeArray(); 41667 }; 41668 } 41669 41670 protected void composeValueSetConceptSetComponent(String name, ValueSet.ConceptSetComponent element) throws IOException { 41671 if (element != null) { 41672 open(name); 41673 composeValueSetConceptSetComponentInner(element); 41674 close(); 41675 } 41676 } 41677 41678 protected void composeValueSetConceptSetComponentInner(ValueSet.ConceptSetComponent element) throws IOException { 41679 composeBackbone(element); 41680 if (element.hasSystemElement()) { 41681 composeUriCore("system", element.getSystemElement(), false); 41682 composeUriExtras("system", element.getSystemElement(), false); 41683 } 41684 if (element.hasVersionElement()) { 41685 composeStringCore("version", element.getVersionElement(), false); 41686 composeStringExtras("version", element.getVersionElement(), false); 41687 } 41688 if (element.hasConcept()) { 41689 openArray("concept"); 41690 for (ValueSet.ConceptReferenceComponent e : element.getConcept()) 41691 composeValueSetConceptReferenceComponent(null, e); 41692 closeArray(); 41693 }; 41694 if (element.hasFilter()) { 41695 openArray("filter"); 41696 for (ValueSet.ConceptSetFilterComponent e : element.getFilter()) 41697 composeValueSetConceptSetFilterComponent(null, e); 41698 closeArray(); 41699 }; 41700 if (element.hasValueSet()) { 41701 openArray("valueSet"); 41702 for (UriType e : element.getValueSet()) 41703 composeUriCore(null, e, true); 41704 closeArray(); 41705 if (anyHasExtras(element.getValueSet())) { 41706 openArray("_valueSet"); 41707 for (UriType e : element.getValueSet()) 41708 composeUriExtras(null, e, true); 41709 closeArray(); 41710 } 41711 }; 41712 } 41713 41714 protected void composeValueSetConceptReferenceComponent(String name, ValueSet.ConceptReferenceComponent element) throws IOException { 41715 if (element != null) { 41716 open(name); 41717 composeValueSetConceptReferenceComponentInner(element); 41718 close(); 41719 } 41720 } 41721 41722 protected void composeValueSetConceptReferenceComponentInner(ValueSet.ConceptReferenceComponent element) throws IOException { 41723 composeBackbone(element); 41724 if (element.hasCodeElement()) { 41725 composeCodeCore("code", element.getCodeElement(), false); 41726 composeCodeExtras("code", element.getCodeElement(), false); 41727 } 41728 if (element.hasDisplayElement()) { 41729 composeStringCore("display", element.getDisplayElement(), false); 41730 composeStringExtras("display", element.getDisplayElement(), false); 41731 } 41732 if (element.hasDesignation()) { 41733 openArray("designation"); 41734 for (ValueSet.ConceptReferenceDesignationComponent e : element.getDesignation()) 41735 composeValueSetConceptReferenceDesignationComponent(null, e); 41736 closeArray(); 41737 }; 41738 } 41739 41740 protected void composeValueSetConceptReferenceDesignationComponent(String name, ValueSet.ConceptReferenceDesignationComponent element) throws IOException { 41741 if (element != null) { 41742 open(name); 41743 composeValueSetConceptReferenceDesignationComponentInner(element); 41744 close(); 41745 } 41746 } 41747 41748 protected void composeValueSetConceptReferenceDesignationComponentInner(ValueSet.ConceptReferenceDesignationComponent element) throws IOException { 41749 composeBackbone(element); 41750 if (element.hasLanguageElement()) { 41751 composeCodeCore("language", element.getLanguageElement(), false); 41752 composeCodeExtras("language", element.getLanguageElement(), false); 41753 } 41754 if (element.hasUse()) { 41755 composeCoding("use", element.getUse()); 41756 } 41757 if (element.hasValueElement()) { 41758 composeStringCore("value", element.getValueElement(), false); 41759 composeStringExtras("value", element.getValueElement(), false); 41760 } 41761 } 41762 41763 protected void composeValueSetConceptSetFilterComponent(String name, ValueSet.ConceptSetFilterComponent element) throws IOException { 41764 if (element != null) { 41765 open(name); 41766 composeValueSetConceptSetFilterComponentInner(element); 41767 close(); 41768 } 41769 } 41770 41771 protected void composeValueSetConceptSetFilterComponentInner(ValueSet.ConceptSetFilterComponent element) throws IOException { 41772 composeBackbone(element); 41773 if (element.hasPropertyElement()) { 41774 composeCodeCore("property", element.getPropertyElement(), false); 41775 composeCodeExtras("property", element.getPropertyElement(), false); 41776 } 41777 if (element.hasOpElement()) { 41778 composeEnumerationCore("op", element.getOpElement(), new ValueSet.FilterOperatorEnumFactory(), false); 41779 composeEnumerationExtras("op", element.getOpElement(), new ValueSet.FilterOperatorEnumFactory(), false); 41780 } 41781 if (element.hasValueElement()) { 41782 composeCodeCore("value", element.getValueElement(), false); 41783 composeCodeExtras("value", element.getValueElement(), false); 41784 } 41785 } 41786 41787 protected void composeValueSetValueSetExpansionComponent(String name, ValueSet.ValueSetExpansionComponent element) throws IOException { 41788 if (element != null) { 41789 open(name); 41790 composeValueSetValueSetExpansionComponentInner(element); 41791 close(); 41792 } 41793 } 41794 41795 protected void composeValueSetValueSetExpansionComponentInner(ValueSet.ValueSetExpansionComponent element) throws IOException { 41796 composeBackbone(element); 41797 if (element.hasIdentifierElement()) { 41798 composeUriCore("identifier", element.getIdentifierElement(), false); 41799 composeUriExtras("identifier", element.getIdentifierElement(), false); 41800 } 41801 if (element.hasTimestampElement()) { 41802 composeDateTimeCore("timestamp", element.getTimestampElement(), false); 41803 composeDateTimeExtras("timestamp", element.getTimestampElement(), false); 41804 } 41805 if (element.hasTotalElement()) { 41806 composeIntegerCore("total", element.getTotalElement(), false); 41807 composeIntegerExtras("total", element.getTotalElement(), false); 41808 } 41809 if (element.hasOffsetElement()) { 41810 composeIntegerCore("offset", element.getOffsetElement(), false); 41811 composeIntegerExtras("offset", element.getOffsetElement(), false); 41812 } 41813 if (element.hasParameter()) { 41814 openArray("parameter"); 41815 for (ValueSet.ValueSetExpansionParameterComponent e : element.getParameter()) 41816 composeValueSetValueSetExpansionParameterComponent(null, e); 41817 closeArray(); 41818 }; 41819 if (element.hasContains()) { 41820 openArray("contains"); 41821 for (ValueSet.ValueSetExpansionContainsComponent e : element.getContains()) 41822 composeValueSetValueSetExpansionContainsComponent(null, e); 41823 closeArray(); 41824 }; 41825 } 41826 41827 protected void composeValueSetValueSetExpansionParameterComponent(String name, ValueSet.ValueSetExpansionParameterComponent element) throws IOException { 41828 if (element != null) { 41829 open(name); 41830 composeValueSetValueSetExpansionParameterComponentInner(element); 41831 close(); 41832 } 41833 } 41834 41835 protected void composeValueSetValueSetExpansionParameterComponentInner(ValueSet.ValueSetExpansionParameterComponent element) throws IOException { 41836 composeBackbone(element); 41837 if (element.hasNameElement()) { 41838 composeStringCore("name", element.getNameElement(), false); 41839 composeStringExtras("name", element.getNameElement(), false); 41840 } 41841 if (element.hasValue()) { 41842 composeType("value", element.getValue()); 41843 } 41844 } 41845 41846 protected void composeValueSetValueSetExpansionContainsComponent(String name, ValueSet.ValueSetExpansionContainsComponent element) throws IOException { 41847 if (element != null) { 41848 open(name); 41849 composeValueSetValueSetExpansionContainsComponentInner(element); 41850 close(); 41851 } 41852 } 41853 41854 protected void composeValueSetValueSetExpansionContainsComponentInner(ValueSet.ValueSetExpansionContainsComponent element) throws IOException { 41855 composeBackbone(element); 41856 if (element.hasSystemElement()) { 41857 composeUriCore("system", element.getSystemElement(), false); 41858 composeUriExtras("system", element.getSystemElement(), false); 41859 } 41860 if (element.hasAbstractElement()) { 41861 composeBooleanCore("abstract", element.getAbstractElement(), false); 41862 composeBooleanExtras("abstract", element.getAbstractElement(), false); 41863 } 41864 if (element.hasInactiveElement()) { 41865 composeBooleanCore("inactive", element.getInactiveElement(), false); 41866 composeBooleanExtras("inactive", element.getInactiveElement(), false); 41867 } 41868 if (element.hasVersionElement()) { 41869 composeStringCore("version", element.getVersionElement(), false); 41870 composeStringExtras("version", element.getVersionElement(), false); 41871 } 41872 if (element.hasCodeElement()) { 41873 composeCodeCore("code", element.getCodeElement(), false); 41874 composeCodeExtras("code", element.getCodeElement(), false); 41875 } 41876 if (element.hasDisplayElement()) { 41877 composeStringCore("display", element.getDisplayElement(), false); 41878 composeStringExtras("display", element.getDisplayElement(), false); 41879 } 41880 if (element.hasDesignation()) { 41881 openArray("designation"); 41882 for (ValueSet.ConceptReferenceDesignationComponent e : element.getDesignation()) 41883 composeValueSetConceptReferenceDesignationComponent(null, e); 41884 closeArray(); 41885 }; 41886 if (element.hasContains()) { 41887 openArray("contains"); 41888 for (ValueSet.ValueSetExpansionContainsComponent e : element.getContains()) 41889 composeValueSetValueSetExpansionContainsComponent(null, e); 41890 closeArray(); 41891 }; 41892 } 41893 41894 protected void composeVisionPrescription(String name, VisionPrescription element) throws IOException { 41895 if (element != null) { 41896 prop("resourceType", name); 41897 composeVisionPrescriptionInner(element); 41898 } 41899 } 41900 41901 protected void composeVisionPrescriptionInner(VisionPrescription element) throws IOException { 41902 composeDomainResourceElements(element); 41903 if (element.hasIdentifier()) { 41904 openArray("identifier"); 41905 for (Identifier e : element.getIdentifier()) 41906 composeIdentifier(null, e); 41907 closeArray(); 41908 }; 41909 if (element.hasStatusElement()) { 41910 composeEnumerationCore("status", element.getStatusElement(), new VisionPrescription.VisionStatusEnumFactory(), false); 41911 composeEnumerationExtras("status", element.getStatusElement(), new VisionPrescription.VisionStatusEnumFactory(), false); 41912 } 41913 if (element.hasPatient()) { 41914 composeReference("patient", element.getPatient()); 41915 } 41916 if (element.hasEncounter()) { 41917 composeReference("encounter", element.getEncounter()); 41918 } 41919 if (element.hasDateWrittenElement()) { 41920 composeDateTimeCore("dateWritten", element.getDateWrittenElement(), false); 41921 composeDateTimeExtras("dateWritten", element.getDateWrittenElement(), false); 41922 } 41923 if (element.hasPrescriber()) { 41924 composeReference("prescriber", element.getPrescriber()); 41925 } 41926 if (element.hasReason()) { 41927 composeType("reason", element.getReason()); 41928 } 41929 if (element.hasDispense()) { 41930 openArray("dispense"); 41931 for (VisionPrescription.VisionPrescriptionDispenseComponent e : element.getDispense()) 41932 composeVisionPrescriptionVisionPrescriptionDispenseComponent(null, e); 41933 closeArray(); 41934 }; 41935 } 41936 41937 protected void composeVisionPrescriptionVisionPrescriptionDispenseComponent(String name, VisionPrescription.VisionPrescriptionDispenseComponent element) throws IOException { 41938 if (element != null) { 41939 open(name); 41940 composeVisionPrescriptionVisionPrescriptionDispenseComponentInner(element); 41941 close(); 41942 } 41943 } 41944 41945 protected void composeVisionPrescriptionVisionPrescriptionDispenseComponentInner(VisionPrescription.VisionPrescriptionDispenseComponent element) throws IOException { 41946 composeBackbone(element); 41947 if (element.hasProduct()) { 41948 composeCodeableConcept("product", element.getProduct()); 41949 } 41950 if (element.hasEyeElement()) { 41951 composeEnumerationCore("eye", element.getEyeElement(), new VisionPrescription.VisionEyesEnumFactory(), false); 41952 composeEnumerationExtras("eye", element.getEyeElement(), new VisionPrescription.VisionEyesEnumFactory(), false); 41953 } 41954 if (element.hasSphereElement()) { 41955 composeDecimalCore("sphere", element.getSphereElement(), false); 41956 composeDecimalExtras("sphere", element.getSphereElement(), false); 41957 } 41958 if (element.hasCylinderElement()) { 41959 composeDecimalCore("cylinder", element.getCylinderElement(), false); 41960 composeDecimalExtras("cylinder", element.getCylinderElement(), false); 41961 } 41962 if (element.hasAxisElement()) { 41963 composeIntegerCore("axis", element.getAxisElement(), false); 41964 composeIntegerExtras("axis", element.getAxisElement(), false); 41965 } 41966 if (element.hasPrismElement()) { 41967 composeDecimalCore("prism", element.getPrismElement(), false); 41968 composeDecimalExtras("prism", element.getPrismElement(), false); 41969 } 41970 if (element.hasBaseElement()) { 41971 composeEnumerationCore("base", element.getBaseElement(), new VisionPrescription.VisionBaseEnumFactory(), false); 41972 composeEnumerationExtras("base", element.getBaseElement(), new VisionPrescription.VisionBaseEnumFactory(), false); 41973 } 41974 if (element.hasAddElement()) { 41975 composeDecimalCore("add", element.getAddElement(), false); 41976 composeDecimalExtras("add", element.getAddElement(), false); 41977 } 41978 if (element.hasPowerElement()) { 41979 composeDecimalCore("power", element.getPowerElement(), false); 41980 composeDecimalExtras("power", element.getPowerElement(), false); 41981 } 41982 if (element.hasBackCurveElement()) { 41983 composeDecimalCore("backCurve", element.getBackCurveElement(), false); 41984 composeDecimalExtras("backCurve", element.getBackCurveElement(), false); 41985 } 41986 if (element.hasDiameterElement()) { 41987 composeDecimalCore("diameter", element.getDiameterElement(), false); 41988 composeDecimalExtras("diameter", element.getDiameterElement(), false); 41989 } 41990 if (element.hasDuration()) { 41991 composeSimpleQuantity("duration", element.getDuration()); 41992 } 41993 if (element.hasColorElement()) { 41994 composeStringCore("color", element.getColorElement(), false); 41995 composeStringExtras("color", element.getColorElement(), false); 41996 } 41997 if (element.hasBrandElement()) { 41998 composeStringCore("brand", element.getBrandElement(), false); 41999 composeStringExtras("brand", element.getBrandElement(), false); 42000 } 42001 if (element.hasNote()) { 42002 openArray("note"); 42003 for (Annotation e : element.getNote()) 42004 composeAnnotation(null, e); 42005 closeArray(); 42006 }; 42007 } 42008 42009 @Override 42010 protected void composeResource(Resource resource) throws IOException { 42011 if (resource instanceof Parameters) 42012 composeParameters("Parameters", (Parameters)resource); 42013 else if (resource instanceof Account) 42014 composeAccount("Account", (Account)resource); 42015 else if (resource instanceof ActivityDefinition) 42016 composeActivityDefinition("ActivityDefinition", (ActivityDefinition)resource); 42017 else if (resource instanceof AdverseEvent) 42018 composeAdverseEvent("AdverseEvent", (AdverseEvent)resource); 42019 else if (resource instanceof AllergyIntolerance) 42020 composeAllergyIntolerance("AllergyIntolerance", (AllergyIntolerance)resource); 42021 else if (resource instanceof Appointment) 42022 composeAppointment("Appointment", (Appointment)resource); 42023 else if (resource instanceof AppointmentResponse) 42024 composeAppointmentResponse("AppointmentResponse", (AppointmentResponse)resource); 42025 else if (resource instanceof AuditEvent) 42026 composeAuditEvent("AuditEvent", (AuditEvent)resource); 42027 else if (resource instanceof Basic) 42028 composeBasic("Basic", (Basic)resource); 42029 else if (resource instanceof Binary) 42030 composeBinary("Binary", (Binary)resource); 42031 else if (resource instanceof BodySite) 42032 composeBodySite("BodySite", (BodySite)resource); 42033 else if (resource instanceof Bundle) 42034 composeBundle("Bundle", (Bundle)resource); 42035 else if (resource instanceof CapabilityStatement) 42036 composeCapabilityStatement("CapabilityStatement", (CapabilityStatement)resource); 42037 else if (resource instanceof CarePlan) 42038 composeCarePlan("CarePlan", (CarePlan)resource); 42039 else if (resource instanceof CareTeam) 42040 composeCareTeam("CareTeam", (CareTeam)resource); 42041 else if (resource instanceof ChargeItem) 42042 composeChargeItem("ChargeItem", (ChargeItem)resource); 42043 else if (resource instanceof Claim) 42044 composeClaim("Claim", (Claim)resource); 42045 else if (resource instanceof ClaimResponse) 42046 composeClaimResponse("ClaimResponse", (ClaimResponse)resource); 42047 else if (resource instanceof ClinicalImpression) 42048 composeClinicalImpression("ClinicalImpression", (ClinicalImpression)resource); 42049 else if (resource instanceof CodeSystem) 42050 composeCodeSystem("CodeSystem", (CodeSystem)resource); 42051 else if (resource instanceof Communication) 42052 composeCommunication("Communication", (Communication)resource); 42053 else if (resource instanceof CommunicationRequest) 42054 composeCommunicationRequest("CommunicationRequest", (CommunicationRequest)resource); 42055 else if (resource instanceof CompartmentDefinition) 42056 composeCompartmentDefinition("CompartmentDefinition", (CompartmentDefinition)resource); 42057 else if (resource instanceof Composition) 42058 composeComposition("Composition", (Composition)resource); 42059 else if (resource instanceof ConceptMap) 42060 composeConceptMap("ConceptMap", (ConceptMap)resource); 42061 else if (resource instanceof Condition) 42062 composeCondition("Condition", (Condition)resource); 42063 else if (resource instanceof Consent) 42064 composeConsent("Consent", (Consent)resource); 42065 else if (resource instanceof Contract) 42066 composeContract("Contract", (Contract)resource); 42067 else if (resource instanceof Coverage) 42068 composeCoverage("Coverage", (Coverage)resource); 42069 else if (resource instanceof DataElement) 42070 composeDataElement("DataElement", (DataElement)resource); 42071 else if (resource instanceof DetectedIssue) 42072 composeDetectedIssue("DetectedIssue", (DetectedIssue)resource); 42073 else if (resource instanceof Device) 42074 composeDevice("Device", (Device)resource); 42075 else if (resource instanceof DeviceComponent) 42076 composeDeviceComponent("DeviceComponent", (DeviceComponent)resource); 42077 else if (resource instanceof DeviceMetric) 42078 composeDeviceMetric("DeviceMetric", (DeviceMetric)resource); 42079 else if (resource instanceof DeviceRequest) 42080 composeDeviceRequest("DeviceRequest", (DeviceRequest)resource); 42081 else if (resource instanceof DeviceUseStatement) 42082 composeDeviceUseStatement("DeviceUseStatement", (DeviceUseStatement)resource); 42083 else if (resource instanceof DiagnosticReport) 42084 composeDiagnosticReport("DiagnosticReport", (DiagnosticReport)resource); 42085 else if (resource instanceof DocumentManifest) 42086 composeDocumentManifest("DocumentManifest", (DocumentManifest)resource); 42087 else if (resource instanceof DocumentReference) 42088 composeDocumentReference("DocumentReference", (DocumentReference)resource); 42089 else if (resource instanceof EligibilityRequest) 42090 composeEligibilityRequest("EligibilityRequest", (EligibilityRequest)resource); 42091 else if (resource instanceof EligibilityResponse) 42092 composeEligibilityResponse("EligibilityResponse", (EligibilityResponse)resource); 42093 else if (resource instanceof Encounter) 42094 composeEncounter("Encounter", (Encounter)resource); 42095 else if (resource instanceof Endpoint) 42096 composeEndpoint("Endpoint", (Endpoint)resource); 42097 else if (resource instanceof EnrollmentRequest) 42098 composeEnrollmentRequest("EnrollmentRequest", (EnrollmentRequest)resource); 42099 else if (resource instanceof EnrollmentResponse) 42100 composeEnrollmentResponse("EnrollmentResponse", (EnrollmentResponse)resource); 42101 else if (resource instanceof EpisodeOfCare) 42102 composeEpisodeOfCare("EpisodeOfCare", (EpisodeOfCare)resource); 42103 else if (resource instanceof ExpansionProfile) 42104 composeExpansionProfile("ExpansionProfile", (ExpansionProfile)resource); 42105 else if (resource instanceof ExplanationOfBenefit) 42106 composeExplanationOfBenefit("ExplanationOfBenefit", (ExplanationOfBenefit)resource); 42107 else if (resource instanceof FamilyMemberHistory) 42108 composeFamilyMemberHistory("FamilyMemberHistory", (FamilyMemberHistory)resource); 42109 else if (resource instanceof Flag) 42110 composeFlag("Flag", (Flag)resource); 42111 else if (resource instanceof Goal) 42112 composeGoal("Goal", (Goal)resource); 42113 else if (resource instanceof GraphDefinition) 42114 composeGraphDefinition("GraphDefinition", (GraphDefinition)resource); 42115 else if (resource instanceof Group) 42116 composeGroup("Group", (Group)resource); 42117 else if (resource instanceof GuidanceResponse) 42118 composeGuidanceResponse("GuidanceResponse", (GuidanceResponse)resource); 42119 else if (resource instanceof HealthcareService) 42120 composeHealthcareService("HealthcareService", (HealthcareService)resource); 42121 else if (resource instanceof ImagingManifest) 42122 composeImagingManifest("ImagingManifest", (ImagingManifest)resource); 42123 else if (resource instanceof ImagingStudy) 42124 composeImagingStudy("ImagingStudy", (ImagingStudy)resource); 42125 else if (resource instanceof Immunization) 42126 composeImmunization("Immunization", (Immunization)resource); 42127 else if (resource instanceof ImmunizationRecommendation) 42128 composeImmunizationRecommendation("ImmunizationRecommendation", (ImmunizationRecommendation)resource); 42129 else if (resource instanceof ImplementationGuide) 42130 composeImplementationGuide("ImplementationGuide", (ImplementationGuide)resource); 42131 else if (resource instanceof Library) 42132 composeLibrary("Library", (Library)resource); 42133 else if (resource instanceof Linkage) 42134 composeLinkage("Linkage", (Linkage)resource); 42135 else if (resource instanceof ListResource) 42136 composeListResource("List", (ListResource)resource); 42137 else if (resource instanceof Location) 42138 composeLocation("Location", (Location)resource); 42139 else if (resource instanceof Measure) 42140 composeMeasure("Measure", (Measure)resource); 42141 else if (resource instanceof MeasureReport) 42142 composeMeasureReport("MeasureReport", (MeasureReport)resource); 42143 else if (resource instanceof Media) 42144 composeMedia("Media", (Media)resource); 42145 else if (resource instanceof Medication) 42146 composeMedication("Medication", (Medication)resource); 42147 else if (resource instanceof MedicationAdministration) 42148 composeMedicationAdministration("MedicationAdministration", (MedicationAdministration)resource); 42149 else if (resource instanceof MedicationDispense) 42150 composeMedicationDispense("MedicationDispense", (MedicationDispense)resource); 42151 else if (resource instanceof MedicationRequest) 42152 composeMedicationRequest("MedicationRequest", (MedicationRequest)resource); 42153 else if (resource instanceof MedicationStatement) 42154 composeMedicationStatement("MedicationStatement", (MedicationStatement)resource); 42155 else if (resource instanceof MessageDefinition) 42156 composeMessageDefinition("MessageDefinition", (MessageDefinition)resource); 42157 else if (resource instanceof MessageHeader) 42158 composeMessageHeader("MessageHeader", (MessageHeader)resource); 42159 else if (resource instanceof NamingSystem) 42160 composeNamingSystem("NamingSystem", (NamingSystem)resource); 42161 else if (resource instanceof NutritionOrder) 42162 composeNutritionOrder("NutritionOrder", (NutritionOrder)resource); 42163 else if (resource instanceof Observation) 42164 composeObservation("Observation", (Observation)resource); 42165 else if (resource instanceof OperationDefinition) 42166 composeOperationDefinition("OperationDefinition", (OperationDefinition)resource); 42167 else if (resource instanceof OperationOutcome) 42168 composeOperationOutcome("OperationOutcome", (OperationOutcome)resource); 42169 else if (resource instanceof Organization) 42170 composeOrganization("Organization", (Organization)resource); 42171 else if (resource instanceof Patient) 42172 composePatient("Patient", (Patient)resource); 42173 else if (resource instanceof PaymentNotice) 42174 composePaymentNotice("PaymentNotice", (PaymentNotice)resource); 42175 else if (resource instanceof PaymentReconciliation) 42176 composePaymentReconciliation("PaymentReconciliation", (PaymentReconciliation)resource); 42177 else if (resource instanceof Person) 42178 composePerson("Person", (Person)resource); 42179 else if (resource instanceof PlanDefinition) 42180 composePlanDefinition("PlanDefinition", (PlanDefinition)resource); 42181 else if (resource instanceof Practitioner) 42182 composePractitioner("Practitioner", (Practitioner)resource); 42183 else if (resource instanceof PractitionerRole) 42184 composePractitionerRole("PractitionerRole", (PractitionerRole)resource); 42185 else if (resource instanceof Procedure) 42186 composeProcedure("Procedure", (Procedure)resource); 42187 else if (resource instanceof ProcedureRequest) 42188 composeProcedureRequest("ProcedureRequest", (ProcedureRequest)resource); 42189 else if (resource instanceof ProcessRequest) 42190 composeProcessRequest("ProcessRequest", (ProcessRequest)resource); 42191 else if (resource instanceof ProcessResponse) 42192 composeProcessResponse("ProcessResponse", (ProcessResponse)resource); 42193 else if (resource instanceof Provenance) 42194 composeProvenance("Provenance", (Provenance)resource); 42195 else if (resource instanceof Questionnaire) 42196 composeQuestionnaire("Questionnaire", (Questionnaire)resource); 42197 else if (resource instanceof QuestionnaireResponse) 42198 composeQuestionnaireResponse("QuestionnaireResponse", (QuestionnaireResponse)resource); 42199 else if (resource instanceof ReferralRequest) 42200 composeReferralRequest("ReferralRequest", (ReferralRequest)resource); 42201 else if (resource instanceof RelatedPerson) 42202 composeRelatedPerson("RelatedPerson", (RelatedPerson)resource); 42203 else if (resource instanceof RequestGroup) 42204 composeRequestGroup("RequestGroup", (RequestGroup)resource); 42205 else if (resource instanceof ResearchStudy) 42206 composeResearchStudy("ResearchStudy", (ResearchStudy)resource); 42207 else if (resource instanceof ResearchSubject) 42208 composeResearchSubject("ResearchSubject", (ResearchSubject)resource); 42209 else if (resource instanceof RiskAssessment) 42210 composeRiskAssessment("RiskAssessment", (RiskAssessment)resource); 42211 else if (resource instanceof Schedule) 42212 composeSchedule("Schedule", (Schedule)resource); 42213 else if (resource instanceof SearchParameter) 42214 composeSearchParameter("SearchParameter", (SearchParameter)resource); 42215 else if (resource instanceof Sequence) 42216 composeSequence("Sequence", (Sequence)resource); 42217 else if (resource instanceof ServiceDefinition) 42218 composeServiceDefinition("ServiceDefinition", (ServiceDefinition)resource); 42219 else if (resource instanceof Slot) 42220 composeSlot("Slot", (Slot)resource); 42221 else if (resource instanceof Specimen) 42222 composeSpecimen("Specimen", (Specimen)resource); 42223 else if (resource instanceof StructureDefinition) 42224 composeStructureDefinition("StructureDefinition", (StructureDefinition)resource); 42225 else if (resource instanceof StructureMap) 42226 composeStructureMap("StructureMap", (StructureMap)resource); 42227 else if (resource instanceof Subscription) 42228 composeSubscription("Subscription", (Subscription)resource); 42229 else if (resource instanceof Substance) 42230 composeSubstance("Substance", (Substance)resource); 42231 else if (resource instanceof SupplyDelivery) 42232 composeSupplyDelivery("SupplyDelivery", (SupplyDelivery)resource); 42233 else if (resource instanceof SupplyRequest) 42234 composeSupplyRequest("SupplyRequest", (SupplyRequest)resource); 42235 else if (resource instanceof Task) 42236 composeTask("Task", (Task)resource); 42237 else if (resource instanceof TestReport) 42238 composeTestReport("TestReport", (TestReport)resource); 42239 else if (resource instanceof TestScript) 42240 composeTestScript("TestScript", (TestScript)resource); 42241 else if (resource instanceof ValueSet) 42242 composeValueSet("ValueSet", (ValueSet)resource); 42243 else if (resource instanceof VisionPrescription) 42244 composeVisionPrescription("VisionPrescription", (VisionPrescription)resource); 42245 else if (resource instanceof Binary) 42246 composeBinary("Binary", (Binary)resource); 42247 else 42248 throw new Error("Unhandled resource type "+resource.getClass().getName()); 42249 } 42250 42251 protected void composeNamedReference(String name, Resource resource) throws IOException { 42252 if (resource instanceof Parameters) 42253 composeParameters(name, (Parameters)resource); 42254 else if (resource instanceof Account) 42255 composeAccount(name, (Account)resource); 42256 else if (resource instanceof ActivityDefinition) 42257 composeActivityDefinition(name, (ActivityDefinition)resource); 42258 else if (resource instanceof AdverseEvent) 42259 composeAdverseEvent(name, (AdverseEvent)resource); 42260 else if (resource instanceof AllergyIntolerance) 42261 composeAllergyIntolerance(name, (AllergyIntolerance)resource); 42262 else if (resource instanceof Appointment) 42263 composeAppointment(name, (Appointment)resource); 42264 else if (resource instanceof AppointmentResponse) 42265 composeAppointmentResponse(name, (AppointmentResponse)resource); 42266 else if (resource instanceof AuditEvent) 42267 composeAuditEvent(name, (AuditEvent)resource); 42268 else if (resource instanceof Basic) 42269 composeBasic(name, (Basic)resource); 42270 else if (resource instanceof Binary) 42271 composeBinary(name, (Binary)resource); 42272 else if (resource instanceof BodySite) 42273 composeBodySite(name, (BodySite)resource); 42274 else if (resource instanceof Bundle) 42275 composeBundle(name, (Bundle)resource); 42276 else if (resource instanceof CapabilityStatement) 42277 composeCapabilityStatement(name, (CapabilityStatement)resource); 42278 else if (resource instanceof CarePlan) 42279 composeCarePlan(name, (CarePlan)resource); 42280 else if (resource instanceof CareTeam) 42281 composeCareTeam(name, (CareTeam)resource); 42282 else if (resource instanceof ChargeItem) 42283 composeChargeItem(name, (ChargeItem)resource); 42284 else if (resource instanceof Claim) 42285 composeClaim(name, (Claim)resource); 42286 else if (resource instanceof ClaimResponse) 42287 composeClaimResponse(name, (ClaimResponse)resource); 42288 else if (resource instanceof ClinicalImpression) 42289 composeClinicalImpression(name, (ClinicalImpression)resource); 42290 else if (resource instanceof CodeSystem) 42291 composeCodeSystem(name, (CodeSystem)resource); 42292 else if (resource instanceof Communication) 42293 composeCommunication(name, (Communication)resource); 42294 else if (resource instanceof CommunicationRequest) 42295 composeCommunicationRequest(name, (CommunicationRequest)resource); 42296 else if (resource instanceof CompartmentDefinition) 42297 composeCompartmentDefinition(name, (CompartmentDefinition)resource); 42298 else if (resource instanceof Composition) 42299 composeComposition(name, (Composition)resource); 42300 else if (resource instanceof ConceptMap) 42301 composeConceptMap(name, (ConceptMap)resource); 42302 else if (resource instanceof Condition) 42303 composeCondition(name, (Condition)resource); 42304 else if (resource instanceof Consent) 42305 composeConsent(name, (Consent)resource); 42306 else if (resource instanceof Contract) 42307 composeContract(name, (Contract)resource); 42308 else if (resource instanceof Coverage) 42309 composeCoverage(name, (Coverage)resource); 42310 else if (resource instanceof DataElement) 42311 composeDataElement(name, (DataElement)resource); 42312 else if (resource instanceof DetectedIssue) 42313 composeDetectedIssue(name, (DetectedIssue)resource); 42314 else if (resource instanceof Device) 42315 composeDevice(name, (Device)resource); 42316 else if (resource instanceof DeviceComponent) 42317 composeDeviceComponent(name, (DeviceComponent)resource); 42318 else if (resource instanceof DeviceMetric) 42319 composeDeviceMetric(name, (DeviceMetric)resource); 42320 else if (resource instanceof DeviceRequest) 42321 composeDeviceRequest(name, (DeviceRequest)resource); 42322 else if (resource instanceof DeviceUseStatement) 42323 composeDeviceUseStatement(name, (DeviceUseStatement)resource); 42324 else if (resource instanceof DiagnosticReport) 42325 composeDiagnosticReport(name, (DiagnosticReport)resource); 42326 else if (resource instanceof DocumentManifest) 42327 composeDocumentManifest(name, (DocumentManifest)resource); 42328 else if (resource instanceof DocumentReference) 42329 composeDocumentReference(name, (DocumentReference)resource); 42330 else if (resource instanceof EligibilityRequest) 42331 composeEligibilityRequest(name, (EligibilityRequest)resource); 42332 else if (resource instanceof EligibilityResponse) 42333 composeEligibilityResponse(name, (EligibilityResponse)resource); 42334 else if (resource instanceof Encounter) 42335 composeEncounter(name, (Encounter)resource); 42336 else if (resource instanceof Endpoint) 42337 composeEndpoint(name, (Endpoint)resource); 42338 else if (resource instanceof EnrollmentRequest) 42339 composeEnrollmentRequest(name, (EnrollmentRequest)resource); 42340 else if (resource instanceof EnrollmentResponse) 42341 composeEnrollmentResponse(name, (EnrollmentResponse)resource); 42342 else if (resource instanceof EpisodeOfCare) 42343 composeEpisodeOfCare(name, (EpisodeOfCare)resource); 42344 else if (resource instanceof ExpansionProfile) 42345 composeExpansionProfile(name, (ExpansionProfile)resource); 42346 else if (resource instanceof ExplanationOfBenefit) 42347 composeExplanationOfBenefit(name, (ExplanationOfBenefit)resource); 42348 else if (resource instanceof FamilyMemberHistory) 42349 composeFamilyMemberHistory(name, (FamilyMemberHistory)resource); 42350 else if (resource instanceof Flag) 42351 composeFlag(name, (Flag)resource); 42352 else if (resource instanceof Goal) 42353 composeGoal(name, (Goal)resource); 42354 else if (resource instanceof GraphDefinition) 42355 composeGraphDefinition(name, (GraphDefinition)resource); 42356 else if (resource instanceof Group) 42357 composeGroup(name, (Group)resource); 42358 else if (resource instanceof GuidanceResponse) 42359 composeGuidanceResponse(name, (GuidanceResponse)resource); 42360 else if (resource instanceof HealthcareService) 42361 composeHealthcareService(name, (HealthcareService)resource); 42362 else if (resource instanceof ImagingManifest) 42363 composeImagingManifest(name, (ImagingManifest)resource); 42364 else if (resource instanceof ImagingStudy) 42365 composeImagingStudy(name, (ImagingStudy)resource); 42366 else if (resource instanceof Immunization) 42367 composeImmunization(name, (Immunization)resource); 42368 else if (resource instanceof ImmunizationRecommendation) 42369 composeImmunizationRecommendation(name, (ImmunizationRecommendation)resource); 42370 else if (resource instanceof ImplementationGuide) 42371 composeImplementationGuide(name, (ImplementationGuide)resource); 42372 else if (resource instanceof Library) 42373 composeLibrary(name, (Library)resource); 42374 else if (resource instanceof Linkage) 42375 composeLinkage(name, (Linkage)resource); 42376 else if (resource instanceof ListResource) 42377 composeListResource(name, (ListResource)resource); 42378 else if (resource instanceof Location) 42379 composeLocation(name, (Location)resource); 42380 else if (resource instanceof Measure) 42381 composeMeasure(name, (Measure)resource); 42382 else if (resource instanceof MeasureReport) 42383 composeMeasureReport(name, (MeasureReport)resource); 42384 else if (resource instanceof Media) 42385 composeMedia(name, (Media)resource); 42386 else if (resource instanceof Medication) 42387 composeMedication(name, (Medication)resource); 42388 else if (resource instanceof MedicationAdministration) 42389 composeMedicationAdministration(name, (MedicationAdministration)resource); 42390 else if (resource instanceof MedicationDispense) 42391 composeMedicationDispense(name, (MedicationDispense)resource); 42392 else if (resource instanceof MedicationRequest) 42393 composeMedicationRequest(name, (MedicationRequest)resource); 42394 else if (resource instanceof MedicationStatement) 42395 composeMedicationStatement(name, (MedicationStatement)resource); 42396 else if (resource instanceof MessageDefinition) 42397 composeMessageDefinition(name, (MessageDefinition)resource); 42398 else if (resource instanceof MessageHeader) 42399 composeMessageHeader(name, (MessageHeader)resource); 42400 else if (resource instanceof NamingSystem) 42401 composeNamingSystem(name, (NamingSystem)resource); 42402 else if (resource instanceof NutritionOrder) 42403 composeNutritionOrder(name, (NutritionOrder)resource); 42404 else if (resource instanceof Observation) 42405 composeObservation(name, (Observation)resource); 42406 else if (resource instanceof OperationDefinition) 42407 composeOperationDefinition(name, (OperationDefinition)resource); 42408 else if (resource instanceof OperationOutcome) 42409 composeOperationOutcome(name, (OperationOutcome)resource); 42410 else if (resource instanceof Organization) 42411 composeOrganization(name, (Organization)resource); 42412 else if (resource instanceof Patient) 42413 composePatient(name, (Patient)resource); 42414 else if (resource instanceof PaymentNotice) 42415 composePaymentNotice(name, (PaymentNotice)resource); 42416 else if (resource instanceof PaymentReconciliation) 42417 composePaymentReconciliation(name, (PaymentReconciliation)resource); 42418 else if (resource instanceof Person) 42419 composePerson(name, (Person)resource); 42420 else if (resource instanceof PlanDefinition) 42421 composePlanDefinition(name, (PlanDefinition)resource); 42422 else if (resource instanceof Practitioner) 42423 composePractitioner(name, (Practitioner)resource); 42424 else if (resource instanceof PractitionerRole) 42425 composePractitionerRole(name, (PractitionerRole)resource); 42426 else if (resource instanceof Procedure) 42427 composeProcedure(name, (Procedure)resource); 42428 else if (resource instanceof ProcedureRequest) 42429 composeProcedureRequest(name, (ProcedureRequest)resource); 42430 else if (resource instanceof ProcessRequest) 42431 composeProcessRequest(name, (ProcessRequest)resource); 42432 else if (resource instanceof ProcessResponse) 42433 composeProcessResponse(name, (ProcessResponse)resource); 42434 else if (resource instanceof Provenance) 42435 composeProvenance(name, (Provenance)resource); 42436 else if (resource instanceof Questionnaire) 42437 composeQuestionnaire(name, (Questionnaire)resource); 42438 else if (resource instanceof QuestionnaireResponse) 42439 composeQuestionnaireResponse(name, (QuestionnaireResponse)resource); 42440 else if (resource instanceof ReferralRequest) 42441 composeReferralRequest(name, (ReferralRequest)resource); 42442 else if (resource instanceof RelatedPerson) 42443 composeRelatedPerson(name, (RelatedPerson)resource); 42444 else if (resource instanceof RequestGroup) 42445 composeRequestGroup(name, (RequestGroup)resource); 42446 else if (resource instanceof ResearchStudy) 42447 composeResearchStudy(name, (ResearchStudy)resource); 42448 else if (resource instanceof ResearchSubject) 42449 composeResearchSubject(name, (ResearchSubject)resource); 42450 else if (resource instanceof RiskAssessment) 42451 composeRiskAssessment(name, (RiskAssessment)resource); 42452 else if (resource instanceof Schedule) 42453 composeSchedule(name, (Schedule)resource); 42454 else if (resource instanceof SearchParameter) 42455 composeSearchParameter(name, (SearchParameter)resource); 42456 else if (resource instanceof Sequence) 42457 composeSequence(name, (Sequence)resource); 42458 else if (resource instanceof ServiceDefinition) 42459 composeServiceDefinition(name, (ServiceDefinition)resource); 42460 else if (resource instanceof Slot) 42461 composeSlot(name, (Slot)resource); 42462 else if (resource instanceof Specimen) 42463 composeSpecimen(name, (Specimen)resource); 42464 else if (resource instanceof StructureDefinition) 42465 composeStructureDefinition(name, (StructureDefinition)resource); 42466 else if (resource instanceof StructureMap) 42467 composeStructureMap(name, (StructureMap)resource); 42468 else if (resource instanceof Subscription) 42469 composeSubscription(name, (Subscription)resource); 42470 else if (resource instanceof Substance) 42471 composeSubstance(name, (Substance)resource); 42472 else if (resource instanceof SupplyDelivery) 42473 composeSupplyDelivery(name, (SupplyDelivery)resource); 42474 else if (resource instanceof SupplyRequest) 42475 composeSupplyRequest(name, (SupplyRequest)resource); 42476 else if (resource instanceof Task) 42477 composeTask(name, (Task)resource); 42478 else if (resource instanceof TestReport) 42479 composeTestReport(name, (TestReport)resource); 42480 else if (resource instanceof TestScript) 42481 composeTestScript(name, (TestScript)resource); 42482 else if (resource instanceof ValueSet) 42483 composeValueSet(name, (ValueSet)resource); 42484 else if (resource instanceof VisionPrescription) 42485 composeVisionPrescription(name, (VisionPrescription)resource); 42486 else if (resource instanceof Binary) 42487 composeBinary(name, (Binary)resource); 42488 else 42489 throw new Error("Unhandled resource type "+resource.getClass().getName()); 42490 } 42491 42492 protected void composeType(String prefix, Type type) throws IOException { 42493 if (type == null) 42494 ; 42495 else if (type instanceof SimpleQuantity) 42496 composeSimpleQuantity(prefix+"SimpleQuantity", (SimpleQuantity) type); 42497 else if (type instanceof Duration) 42498 composeDuration(prefix+"Duration", (Duration) type); 42499 else if (type instanceof Count) 42500 composeCount(prefix+"Count", (Count) type); 42501 else if (type instanceof Money) 42502 composeMoney(prefix+"Money", (Money) type); 42503 else if (type instanceof Distance) 42504 composeDistance(prefix+"Distance", (Distance) type); 42505 else if (type instanceof Age) 42506 composeAge(prefix+"Age", (Age) type); 42507 else if (type instanceof Reference) 42508 composeReference(prefix+"Reference", (Reference) type); 42509 else if (type instanceof Quantity) 42510 composeQuantity(prefix+"Quantity", (Quantity) type); 42511 else if (type instanceof Period) 42512 composePeriod(prefix+"Period", (Period) type); 42513 else if (type instanceof Attachment) 42514 composeAttachment(prefix+"Attachment", (Attachment) type); 42515 else if (type instanceof Range) 42516 composeRange(prefix+"Range", (Range) type); 42517 else if (type instanceof Annotation) 42518 composeAnnotation(prefix+"Annotation", (Annotation) type); 42519 else if (type instanceof Identifier) 42520 composeIdentifier(prefix+"Identifier", (Identifier) type); 42521 else if (type instanceof Coding) 42522 composeCoding(prefix+"Coding", (Coding) type); 42523 else if (type instanceof Signature) 42524 composeSignature(prefix+"Signature", (Signature) type); 42525 else if (type instanceof SampledData) 42526 composeSampledData(prefix+"SampledData", (SampledData) type); 42527 else if (type instanceof Ratio) 42528 composeRatio(prefix+"Ratio", (Ratio) type); 42529 else if (type instanceof CodeableConcept) 42530 composeCodeableConcept(prefix+"CodeableConcept", (CodeableConcept) type); 42531 else if (type instanceof Meta) 42532 composeMeta(prefix+"Meta", (Meta) type); 42533 else if (type instanceof Address) 42534 composeAddress(prefix+"Address", (Address) type); 42535 else if (type instanceof TriggerDefinition) 42536 composeTriggerDefinition(prefix+"TriggerDefinition", (TriggerDefinition) type); 42537 else if (type instanceof Contributor) 42538 composeContributor(prefix+"Contributor", (Contributor) type); 42539 else if (type instanceof DataRequirement) 42540 composeDataRequirement(prefix+"DataRequirement", (DataRequirement) type); 42541 else if (type instanceof Dosage) 42542 composeDosage(prefix+"Dosage", (Dosage) type); 42543 else if (type instanceof RelatedArtifact) 42544 composeRelatedArtifact(prefix+"RelatedArtifact", (RelatedArtifact) type); 42545 else if (type instanceof ContactDetail) 42546 composeContactDetail(prefix+"ContactDetail", (ContactDetail) type); 42547 else if (type instanceof HumanName) 42548 composeHumanName(prefix+"HumanName", (HumanName) type); 42549 else if (type instanceof ContactPoint) 42550 composeContactPoint(prefix+"ContactPoint", (ContactPoint) type); 42551 else if (type instanceof UsageContext) 42552 composeUsageContext(prefix+"UsageContext", (UsageContext) type); 42553 else if (type instanceof Timing) 42554 composeTiming(prefix+"Timing", (Timing) type); 42555 else if (type instanceof ElementDefinition) 42556 composeElementDefinition(prefix+"ElementDefinition", (ElementDefinition) type); 42557 else if (type instanceof ParameterDefinition) 42558 composeParameterDefinition(prefix+"ParameterDefinition", (ParameterDefinition) type); 42559 else if (type instanceof CodeType) { 42560 composeCodeCore(prefix+"Code", (CodeType) type, false); 42561 composeCodeExtras(prefix+"Code", (CodeType) type, false); 42562 } 42563 else if (type instanceof OidType) { 42564 composeOidCore(prefix+"Oid", (OidType) type, false); 42565 composeOidExtras(prefix+"Oid", (OidType) type, false); 42566 } 42567 else if (type instanceof UuidType) { 42568 composeUuidCore(prefix+"Uuid", (UuidType) type, false); 42569 composeUuidExtras(prefix+"Uuid", (UuidType) type, false); 42570 } 42571 else if (type instanceof UnsignedIntType) { 42572 composeUnsignedIntCore(prefix+"UnsignedInt", (UnsignedIntType) type, false); 42573 composeUnsignedIntExtras(prefix+"UnsignedInt", (UnsignedIntType) type, false); 42574 } 42575 else if (type instanceof MarkdownType) { 42576 composeMarkdownCore(prefix+"Markdown", (MarkdownType) type, false); 42577 composeMarkdownExtras(prefix+"Markdown", (MarkdownType) type, false); 42578 } 42579 else if (type instanceof IdType) { 42580 composeIdCore(prefix+"Id", (IdType) type, false); 42581 composeIdExtras(prefix+"Id", (IdType) type, false); 42582 } 42583 else if (type instanceof PositiveIntType) { 42584 composePositiveIntCore(prefix+"PositiveInt", (PositiveIntType) type, false); 42585 composePositiveIntExtras(prefix+"PositiveInt", (PositiveIntType) type, false); 42586 } 42587 else if (type instanceof DateType) { 42588 composeDateCore(prefix+"Date", (DateType) type, false); 42589 composeDateExtras(prefix+"Date", (DateType) type, false); 42590 } 42591 else if (type instanceof DateTimeType) { 42592 composeDateTimeCore(prefix+"DateTime", (DateTimeType) type, false); 42593 composeDateTimeExtras(prefix+"DateTime", (DateTimeType) type, false); 42594 } 42595 else if (type instanceof StringType) { 42596 composeStringCore(prefix+"String", (StringType) type, false); 42597 composeStringExtras(prefix+"String", (StringType) type, false); 42598 } 42599 else if (type instanceof IntegerType) { 42600 composeIntegerCore(prefix+"Integer", (IntegerType) type, false); 42601 composeIntegerExtras(prefix+"Integer", (IntegerType) type, false); 42602 } 42603 else if (type instanceof UriType) { 42604 composeUriCore(prefix+"Uri", (UriType) type, false); 42605 composeUriExtras(prefix+"Uri", (UriType) type, false); 42606 } 42607 else if (type instanceof InstantType) { 42608 composeInstantCore(prefix+"Instant", (InstantType) type, false); 42609 composeInstantExtras(prefix+"Instant", (InstantType) type, false); 42610 } 42611 else if (type instanceof BooleanType) { 42612 composeBooleanCore(prefix+"Boolean", (BooleanType) type, false); 42613 composeBooleanExtras(prefix+"Boolean", (BooleanType) type, false); 42614 } 42615 else if (type instanceof Base64BinaryType) { 42616 composeBase64BinaryCore(prefix+"Base64Binary", (Base64BinaryType) type, false); 42617 composeBase64BinaryExtras(prefix+"Base64Binary", (Base64BinaryType) type, false); 42618 } 42619 else if (type instanceof TimeType) { 42620 composeTimeCore(prefix+"Time", (TimeType) type, false); 42621 composeTimeExtras(prefix+"Time", (TimeType) type, false); 42622 } 42623 else if (type instanceof DecimalType) { 42624 composeDecimalCore(prefix+"Decimal", (DecimalType) type, false); 42625 composeDecimalExtras(prefix+"Decimal", (DecimalType) type, false); 42626 } 42627 else 42628 throw new Error("Unhandled type"); 42629 } 42630 42631 protected void composeTypeInner(Type type) throws IOException { 42632 if (type == null) 42633 ; 42634 else if (type instanceof Duration) 42635 composeDurationInner((Duration) type); 42636 else if (type instanceof Count) 42637 composeCountInner((Count) type); 42638 else if (type instanceof Money) 42639 composeMoneyInner((Money) type); 42640 else if (type instanceof Distance) 42641 composeDistanceInner((Distance) type); 42642 else if (type instanceof Age) 42643 composeAgeInner((Age) type); 42644 else if (type instanceof Reference) 42645 composeReferenceInner((Reference) type); 42646 else if (type instanceof Quantity) 42647 composeQuantityInner((Quantity) type); 42648 else if (type instanceof Period) 42649 composePeriodInner((Period) type); 42650 else if (type instanceof Attachment) 42651 composeAttachmentInner((Attachment) type); 42652 else if (type instanceof Range) 42653 composeRangeInner((Range) type); 42654 else if (type instanceof Annotation) 42655 composeAnnotationInner((Annotation) type); 42656 else if (type instanceof Identifier) 42657 composeIdentifierInner((Identifier) type); 42658 else if (type instanceof Coding) 42659 composeCodingInner((Coding) type); 42660 else if (type instanceof Signature) 42661 composeSignatureInner((Signature) type); 42662 else if (type instanceof SampledData) 42663 composeSampledDataInner((SampledData) type); 42664 else if (type instanceof Ratio) 42665 composeRatioInner((Ratio) type); 42666 else if (type instanceof CodeableConcept) 42667 composeCodeableConceptInner((CodeableConcept) type); 42668 else if (type instanceof SimpleQuantity) 42669 composeSimpleQuantityInner((SimpleQuantity) type); 42670 else if (type instanceof Meta) 42671 composeMetaInner((Meta) type); 42672 else if (type instanceof Address) 42673 composeAddressInner((Address) type); 42674 else if (type instanceof TriggerDefinition) 42675 composeTriggerDefinitionInner((TriggerDefinition) type); 42676 else if (type instanceof Contributor) 42677 composeContributorInner((Contributor) type); 42678 else if (type instanceof DataRequirement) 42679 composeDataRequirementInner((DataRequirement) type); 42680 else if (type instanceof Dosage) 42681 composeDosageInner((Dosage) type); 42682 else if (type instanceof RelatedArtifact) 42683 composeRelatedArtifactInner((RelatedArtifact) type); 42684 else if (type instanceof ContactDetail) 42685 composeContactDetailInner((ContactDetail) type); 42686 else if (type instanceof HumanName) 42687 composeHumanNameInner((HumanName) type); 42688 else if (type instanceof ContactPoint) 42689 composeContactPointInner((ContactPoint) type); 42690 else if (type instanceof UsageContext) 42691 composeUsageContextInner((UsageContext) type); 42692 else if (type instanceof Timing) 42693 composeTimingInner((Timing) type); 42694 else if (type instanceof ElementDefinition) 42695 composeElementDefinitionInner((ElementDefinition) type); 42696 else if (type instanceof ParameterDefinition) 42697 composeParameterDefinitionInner((ParameterDefinition) type); 42698 else 42699 throw new Error("Unhandled type"); 42700 } 42701 42702}