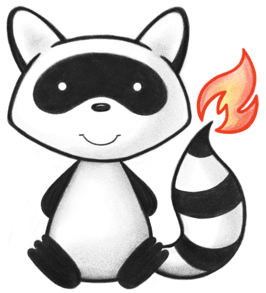
001package org.hl7.fhir.dstu3.formats; 002 003 004 005 006 007/* 008 Copyright (c) 2011+, HL7, Inc. 009 All rights reserved. 010 011 Redistribution and use in source and binary forms, with or without modification, 012 are permitted provided that the following conditions are met: 013 014 * Redistributions of source code must retain the above copyright notice, this 015 list of conditions and the following disclaimer. 016 * Redistributions in binary form must reproduce the above copyright notice, 017 this list of conditions and the following disclaimer in the documentation 018 and/or other materials provided with the distribution. 019 * Neither the name of HL7 nor the names of its contributors may be used to 020 endorse or promote products derived from this software without specific 021 prior written permission. 022 023 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 024 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 025 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 026 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 027 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 028 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 029 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 030 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 031 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 032 POSSIBILITY OF SUCH DAMAGE. 033 034*/ 035 036 037import java.io.ByteArrayInputStream; 038import java.io.ByteArrayOutputStream; 039import java.io.IOException; 040import java.math.BigDecimal; 041import java.nio.charset.StandardCharsets; 042import java.text.ParseException; 043import java.util.HashMap; 044import java.util.Map; 045 046import org.apache.commons.codec.binary.Base64; 047import org.hl7.fhir.dstu3.model.Resource; 048import org.hl7.fhir.dstu3.model.Type; 049import org.hl7.fhir.exceptions.FHIRFormatError; 050import org.hl7.fhir.utilities.Utilities; 051 052public abstract class ParserBase extends FormatUtilities implements IParser { 053 054 // -- implementation of variant type methods from the interface -------------------------------- 055 056 public Resource parse(String input) throws FHIRFormatError, IOException { 057 return parse(input.getBytes(StandardCharsets.UTF_8)); 058 } 059 060 public Resource parse(byte[] bytes) throws FHIRFormatError, IOException { 061 ByteArrayInputStream bi = new ByteArrayInputStream(bytes); 062 return parse(bi); 063 } 064 065 public Type parseType(String input, String typeName) throws FHIRFormatError, IOException { 066 return parseType(input.getBytes(StandardCharsets.UTF_8), typeName); 067 } 068 069 public Type parseType(byte[] bytes, String typeName) throws FHIRFormatError, IOException { 070 ByteArrayInputStream bi = new ByteArrayInputStream(bytes); 071 return parseType(bi, typeName); 072 } 073 074 public String composeString(Resource resource) throws IOException { 075 return new String(composeBytes(resource), StandardCharsets.UTF_8); 076 } 077 078 public byte[] composeBytes(Resource resource) throws IOException { 079 ByteArrayOutputStream bytes = new ByteArrayOutputStream(); 080 compose(bytes, resource); 081 bytes.close(); 082 return bytes.toByteArray(); 083 } 084 085 public String composeString(Type type, String typeName) throws IOException { 086 return new String(composeBytes(type, typeName), StandardCharsets.UTF_8); 087 } 088 089 public byte[] composeBytes(Type type, String typeName) throws IOException { 090 ByteArrayOutputStream bytes = new ByteArrayOutputStream(); 091 compose(bytes, type, typeName); 092 bytes.close(); 093 return bytes.toByteArray(); 094 } 095 096 // -- Parser Configuration -------------------------------- 097 098 protected String xhtmlMessage; 099 100 @Override 101 public IParser setSuppressXhtml(String message) { 102 xhtmlMessage = message; 103 return this; 104 } 105 106 protected boolean handleComments = false; 107 108 public boolean getHandleComments() { 109 return handleComments; 110 } 111 112 public IParser setHandleComments(boolean value) { 113 this.handleComments = value; 114 return this; 115 } 116 117 /** 118 * Whether to throw an exception if unknown content is found (or just skip it) 119 */ 120 protected boolean allowUnknownContent; 121 122 /** 123 * @return Whether to throw an exception if unknown content is found (or just skip it) 124 */ 125 public boolean isAllowUnknownContent() { 126 return allowUnknownContent; 127 } 128 /** 129 * @param allowUnknownContent Whether to throw an exception if unknown content is found (or just skip it) 130 */ 131 public IParser setAllowUnknownContent(boolean allowUnknownContent) { 132 this.allowUnknownContent = allowUnknownContent; 133 return this; 134 } 135 136 protected OutputStyle style = OutputStyle.NORMAL; 137 138 public OutputStyle getOutputStyle() { 139 return style; 140 } 141 142 public IParser setOutputStyle(OutputStyle style) { 143 this.style = style; 144 return this; 145 } 146 147 // -- Parser Utilities -------------------------------- 148 149 150 protected Map<String, Object> idMap = new HashMap<String, Object>(); 151 152 153 protected int parseIntegerPrimitive(String value) { 154 if (value.startsWith("+") && Utilities.isInteger(value.substring(1))) 155 value = value.substring(1); 156 return java.lang.Integer.parseInt(value); 157 } 158 protected int parseIntegerPrimitive(java.lang.Long value) { 159 if (value < java.lang.Integer.MIN_VALUE || value > java.lang.Integer.MAX_VALUE) { 160 throw new IllegalArgumentException 161 (value + " cannot be cast to int without changing its value."); 162 } 163 return value.intValue(); 164 } 165 166 167 protected String parseCodePrimitive(String value) { 168 return value; 169 } 170 171 protected String parseTimePrimitive(String value) throws ParseException { 172 return value; 173 } 174 175 protected BigDecimal parseDecimalPrimitive(BigDecimal value) { 176 return value; 177 } 178 179 protected BigDecimal parseDecimalPrimitive(String value) { 180 return new BigDecimal(value); 181 } 182 183 protected String parseUriPrimitive(String value) { 184 return value; 185 } 186 187 protected byte[] parseBase64BinaryPrimitive(String value) { 188 return Base64.decodeBase64(value.getBytes()); 189 } 190 191 protected String parseOidPrimitive(String value) { 192 return value; 193 } 194 195 protected Boolean parseBooleanPrimitive(String value) { 196 return java.lang.Boolean.valueOf(value); 197 } 198 199 protected Boolean parseBooleanPrimitive(Boolean value) { 200 return java.lang.Boolean.valueOf(value); 201 } 202 203 protected String parseIdPrimitive(String value) { 204 return value; 205 } 206 207 protected String parseStringPrimitive(String value) { 208 return value; 209 } 210 211 protected String parseUuidPrimitive(String value) { 212 return value; 213 } 214 215 216}