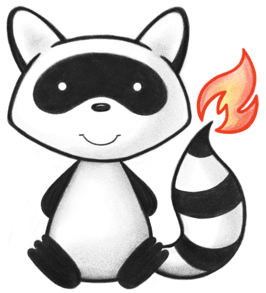
001/* 002 * #%L 003 * HAPI FHIR Structures - DSTU2 (FHIR v1.0.0) 004 * %% 005 * Copyright (C) 2014 - 2015 University Health Network 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package org.hl7.fhir.dstu3.hapi.ctx; 021 022import ca.uhn.fhir.context.ConfigurationException; 023import ca.uhn.fhir.context.FhirContext; 024import ca.uhn.fhir.context.FhirVersionEnum; 025import ca.uhn.fhir.context.RuntimeResourceDefinition; 026import ca.uhn.fhir.fhirpath.IFhirPath; 027import ca.uhn.fhir.i18n.Msg; 028import ca.uhn.fhir.model.api.IFhirVersion; 029import ca.uhn.fhir.model.primitive.IdDt; 030import ca.uhn.fhir.rest.api.IVersionSpecificBundleFactory; 031import ca.uhn.fhir.util.ReflectionUtil; 032import org.apache.commons.lang3.StringUtils; 033import org.hl7.fhir.dstu3.hapi.fluentpath.FhirPathDstu3; 034import org.hl7.fhir.dstu3.hapi.rest.server.Dstu3BundleFactory; 035import org.hl7.fhir.dstu3.model.*; 036import org.hl7.fhir.instance.model.api.*; 037 038import java.io.InputStream; 039import java.util.Date; 040import java.util.List; 041 042public class FhirDstu3 implements IFhirVersion { 043 044 private String myId; 045 046 @Override 047 public IFhirPath createFhirPathExecutor(FhirContext theFhirContext) { 048 return new FhirPathDstu3(theFhirContext); 049 } 050 051 @Override 052 public IBaseResource generateProfile(RuntimeResourceDefinition theRuntimeResourceDefinition, String theServerBase) { 053 StructureDefinition retVal = new StructureDefinition(); 054 055 RuntimeResourceDefinition def = theRuntimeResourceDefinition; 056 057 myId = def.getId(); 058 if (StringUtils.isBlank(myId)) { 059 myId = theRuntimeResourceDefinition.getName().toLowerCase(); 060 } 061 062 retVal.setId(new IdDt(myId)); 063 return retVal; 064 } 065 066 @SuppressWarnings("rawtypes") 067 @Override 068 public Class<List> getContainedType() { 069 return List.class; 070 } 071 072 @Override 073 public InputStream getFhirVersionPropertiesFile() { 074 InputStream str = FhirDstu3.class.getResourceAsStream("/org/hl7/fhir/dstu3/hapi/model/fhirversion.properties"); 075 if (str == null) { 076 str = FhirDstu3.class.getResourceAsStream("/org/hl7/fhir/dstu3/hapi/model/fhirversion.properties"); 077 } 078 if (str == null) { 079 throw new ConfigurationException(Msg.code(609) + "Can not find model property file on classpath: " 080 + "/org/hl7/fhir/dstu3/hapi/model/fhirversion.properties"); 081 } 082 return str; 083 } 084 085 @Override 086 public IPrimitiveType<Date> getLastUpdated(IBaseResource theResource) { 087 return ((Resource) theResource).getMeta().getLastUpdatedElement(); 088 } 089 090 @Override 091 public String getPathToSchemaDefinitions() { 092 return "/org/hl7/fhir/dstu3/model/schema"; 093 } 094 095 @Override 096 public Class<? extends IBaseReference> getResourceReferenceType() { 097 return Reference.class; 098 } 099 100 @Override 101 public Object getServerVersion() { 102 return ReflectionUtil.newInstanceOfFhirServerType("org.hl7.fhir.dstu3.hapi.ctx.FhirServerDstu3"); 103 } 104 105 @Override 106 public FhirVersionEnum getVersion() { 107 return FhirVersionEnum.DSTU3; 108 } 109 110 @Override 111 public IVersionSpecificBundleFactory newBundleFactory(FhirContext theContext) { 112 return new Dstu3BundleFactory(theContext); 113 } 114 115 @Override 116 public IBaseCoding newCodingDt() { 117 return new Coding(); 118 } 119 120 @Override 121 public IIdType newIdType() { 122 return new IdType(); 123 } 124}