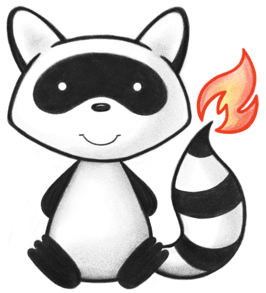
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.utilities.Utilities; 041 042import ca.uhn.fhir.model.api.annotation.Block; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 047/** 048 * A financial tool for tracking value accrued for a particular purpose. In the healthcare field, used to track charges for a patient, cost centers, etc. 049 */ 050@ResourceDef(name="Account", profile="http://hl7.org/fhir/Profile/Account") 051public class Account extends DomainResource { 052 053 public enum AccountStatus { 054 /** 055 * This account is active and may be used. 056 */ 057 ACTIVE, 058 /** 059 * This account is inactive and should not be used to track financial information. 060 */ 061 INACTIVE, 062 /** 063 * This instance should not have been part of this patient's medical record. 064 */ 065 ENTEREDINERROR, 066 /** 067 * added to help the parsers with the generic types 068 */ 069 NULL; 070 public static AccountStatus fromCode(String codeString) throws FHIRException { 071 if (codeString == null || "".equals(codeString)) 072 return null; 073 if ("active".equals(codeString)) 074 return ACTIVE; 075 if ("inactive".equals(codeString)) 076 return INACTIVE; 077 if ("entered-in-error".equals(codeString)) 078 return ENTEREDINERROR; 079 if (Configuration.isAcceptInvalidEnums()) 080 return null; 081 else 082 throw new FHIRException("Unknown AccountStatus code '"+codeString+"'"); 083 } 084 public String toCode() { 085 switch (this) { 086 case ACTIVE: return "active"; 087 case INACTIVE: return "inactive"; 088 case ENTEREDINERROR: return "entered-in-error"; 089 case NULL: return null; 090 default: return "?"; 091 } 092 } 093 public String getSystem() { 094 switch (this) { 095 case ACTIVE: return "http://hl7.org/fhir/account-status"; 096 case INACTIVE: return "http://hl7.org/fhir/account-status"; 097 case ENTEREDINERROR: return "http://hl7.org/fhir/account-status"; 098 case NULL: return null; 099 default: return "?"; 100 } 101 } 102 public String getDefinition() { 103 switch (this) { 104 case ACTIVE: return "This account is active and may be used."; 105 case INACTIVE: return "This account is inactive and should not be used to track financial information."; 106 case ENTEREDINERROR: return "This instance should not have been part of this patient's medical record."; 107 case NULL: return null; 108 default: return "?"; 109 } 110 } 111 public String getDisplay() { 112 switch (this) { 113 case ACTIVE: return "Active"; 114 case INACTIVE: return "Inactive"; 115 case ENTEREDINERROR: return "Entered in error"; 116 case NULL: return null; 117 default: return "?"; 118 } 119 } 120 } 121 122 public static class AccountStatusEnumFactory implements EnumFactory<AccountStatus> { 123 public AccountStatus fromCode(String codeString) throws IllegalArgumentException { 124 if (codeString == null || "".equals(codeString)) 125 if (codeString == null || "".equals(codeString)) 126 return null; 127 if ("active".equals(codeString)) 128 return AccountStatus.ACTIVE; 129 if ("inactive".equals(codeString)) 130 return AccountStatus.INACTIVE; 131 if ("entered-in-error".equals(codeString)) 132 return AccountStatus.ENTEREDINERROR; 133 throw new IllegalArgumentException("Unknown AccountStatus code '"+codeString+"'"); 134 } 135 public Enumeration<AccountStatus> fromType(PrimitiveType<?> code) throws FHIRException { 136 if (code == null) 137 return null; 138 if (code.isEmpty()) 139 return new Enumeration<AccountStatus>(this); 140 String codeString = code.asStringValue(); 141 if (codeString == null || "".equals(codeString)) 142 return null; 143 if ("active".equals(codeString)) 144 return new Enumeration<AccountStatus>(this, AccountStatus.ACTIVE); 145 if ("inactive".equals(codeString)) 146 return new Enumeration<AccountStatus>(this, AccountStatus.INACTIVE); 147 if ("entered-in-error".equals(codeString)) 148 return new Enumeration<AccountStatus>(this, AccountStatus.ENTEREDINERROR); 149 throw new FHIRException("Unknown AccountStatus code '"+codeString+"'"); 150 } 151 public String toCode(AccountStatus code) { 152 if (code == AccountStatus.NULL) 153 return null; 154 if (code == AccountStatus.ACTIVE) 155 return "active"; 156 if (code == AccountStatus.INACTIVE) 157 return "inactive"; 158 if (code == AccountStatus.ENTEREDINERROR) 159 return "entered-in-error"; 160 return "?"; 161 } 162 public String toSystem(AccountStatus code) { 163 return code.getSystem(); 164 } 165 } 166 167 @Block() 168 public static class CoverageComponent extends BackboneElement implements IBaseBackboneElement { 169 /** 170 * The party(s) that are responsible for payment (or part of) of charges applied to this account (including self-pay). 171 172A coverage may only be resposible for specific types of charges, and the sequence of the coverages in the account could be important when processing billing. 173 */ 174 @Child(name = "coverage", type = {Coverage.class}, order=1, min=1, max=1, modifier=false, summary=true) 175 @Description(shortDefinition="The party(s) that are responsible for covering the payment of this account", formalDefinition="The party(s) that are responsible for payment (or part of) of charges applied to this account (including self-pay).\n\nA coverage may only be resposible for specific types of charges, and the sequence of the coverages in the account could be important when processing billing." ) 176 protected Reference coverage; 177 178 /** 179 * The actual object that is the target of the reference (The party(s) that are responsible for payment (or part of) of charges applied to this account (including self-pay). 180 181A coverage may only be resposible for specific types of charges, and the sequence of the coverages in the account could be important when processing billing.) 182 */ 183 protected Coverage coverageTarget; 184 185 /** 186 * The priority of the coverage in the context of this account. 187 */ 188 @Child(name = "priority", type = {PositiveIntType.class}, order=2, min=0, max=1, modifier=false, summary=true) 189 @Description(shortDefinition="The priority of the coverage in the context of this account", formalDefinition="The priority of the coverage in the context of this account." ) 190 protected PositiveIntType priority; 191 192 private static final long serialVersionUID = -1046265008L; 193 194 /** 195 * Constructor 196 */ 197 public CoverageComponent() { 198 super(); 199 } 200 201 /** 202 * Constructor 203 */ 204 public CoverageComponent(Reference coverage) { 205 super(); 206 this.coverage = coverage; 207 } 208 209 /** 210 * @return {@link #coverage} (The party(s) that are responsible for payment (or part of) of charges applied to this account (including self-pay). 211 212A coverage may only be resposible for specific types of charges, and the sequence of the coverages in the account could be important when processing billing.) 213 */ 214 public Reference getCoverage() { 215 if (this.coverage == null) 216 if (Configuration.errorOnAutoCreate()) 217 throw new Error("Attempt to auto-create CoverageComponent.coverage"); 218 else if (Configuration.doAutoCreate()) 219 this.coverage = new Reference(); // cc 220 return this.coverage; 221 } 222 223 public boolean hasCoverage() { 224 return this.coverage != null && !this.coverage.isEmpty(); 225 } 226 227 /** 228 * @param value {@link #coverage} (The party(s) that are responsible for payment (or part of) of charges applied to this account (including self-pay). 229 230A coverage may only be resposible for specific types of charges, and the sequence of the coverages in the account could be important when processing billing.) 231 */ 232 public CoverageComponent setCoverage(Reference value) { 233 this.coverage = value; 234 return this; 235 } 236 237 /** 238 * @return {@link #coverage} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The party(s) that are responsible for payment (or part of) of charges applied to this account (including self-pay). 239 240A coverage may only be resposible for specific types of charges, and the sequence of the coverages in the account could be important when processing billing.) 241 */ 242 public Coverage getCoverageTarget() { 243 if (this.coverageTarget == null) 244 if (Configuration.errorOnAutoCreate()) 245 throw new Error("Attempt to auto-create CoverageComponent.coverage"); 246 else if (Configuration.doAutoCreate()) 247 this.coverageTarget = new Coverage(); // aa 248 return this.coverageTarget; 249 } 250 251 /** 252 * @param value {@link #coverage} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The party(s) that are responsible for payment (or part of) of charges applied to this account (including self-pay). 253 254A coverage may only be resposible for specific types of charges, and the sequence of the coverages in the account could be important when processing billing.) 255 */ 256 public CoverageComponent setCoverageTarget(Coverage value) { 257 this.coverageTarget = value; 258 return this; 259 } 260 261 /** 262 * @return {@link #priority} (The priority of the coverage in the context of this account.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 263 */ 264 public PositiveIntType getPriorityElement() { 265 if (this.priority == null) 266 if (Configuration.errorOnAutoCreate()) 267 throw new Error("Attempt to auto-create CoverageComponent.priority"); 268 else if (Configuration.doAutoCreate()) 269 this.priority = new PositiveIntType(); // bb 270 return this.priority; 271 } 272 273 public boolean hasPriorityElement() { 274 return this.priority != null && !this.priority.isEmpty(); 275 } 276 277 public boolean hasPriority() { 278 return this.priority != null && !this.priority.isEmpty(); 279 } 280 281 /** 282 * @param value {@link #priority} (The priority of the coverage in the context of this account.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 283 */ 284 public CoverageComponent setPriorityElement(PositiveIntType value) { 285 this.priority = value; 286 return this; 287 } 288 289 /** 290 * @return The priority of the coverage in the context of this account. 291 */ 292 public int getPriority() { 293 return this.priority == null || this.priority.isEmpty() ? 0 : this.priority.getValue(); 294 } 295 296 /** 297 * @param value The priority of the coverage in the context of this account. 298 */ 299 public CoverageComponent setPriority(int value) { 300 if (this.priority == null) 301 this.priority = new PositiveIntType(); 302 this.priority.setValue(value); 303 return this; 304 } 305 306 protected void listChildren(List<Property> children) { 307 super.listChildren(children); 308 children.add(new Property("coverage", "Reference(Coverage)", "The party(s) that are responsible for payment (or part of) of charges applied to this account (including self-pay).\n\nA coverage may only be resposible for specific types of charges, and the sequence of the coverages in the account could be important when processing billing.", 0, 1, coverage)); 309 children.add(new Property("priority", "positiveInt", "The priority of the coverage in the context of this account.", 0, 1, priority)); 310 } 311 312 @Override 313 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 314 switch (_hash) { 315 case -351767064: /*coverage*/ return new Property("coverage", "Reference(Coverage)", "The party(s) that are responsible for payment (or part of) of charges applied to this account (including self-pay).\n\nA coverage may only be resposible for specific types of charges, and the sequence of the coverages in the account could be important when processing billing.", 0, 1, coverage); 316 case -1165461084: /*priority*/ return new Property("priority", "positiveInt", "The priority of the coverage in the context of this account.", 0, 1, priority); 317 default: return super.getNamedProperty(_hash, _name, _checkValid); 318 } 319 320 } 321 322 @Override 323 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 324 switch (hash) { 325 case -351767064: /*coverage*/ return this.coverage == null ? new Base[0] : new Base[] {this.coverage}; // Reference 326 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // PositiveIntType 327 default: return super.getProperty(hash, name, checkValid); 328 } 329 330 } 331 332 @Override 333 public Base setProperty(int hash, String name, Base value) throws FHIRException { 334 switch (hash) { 335 case -351767064: // coverage 336 this.coverage = castToReference(value); // Reference 337 return value; 338 case -1165461084: // priority 339 this.priority = castToPositiveInt(value); // PositiveIntType 340 return value; 341 default: return super.setProperty(hash, name, value); 342 } 343 344 } 345 346 @Override 347 public Base setProperty(String name, Base value) throws FHIRException { 348 if (name.equals("coverage")) { 349 this.coverage = castToReference(value); // Reference 350 } else if (name.equals("priority")) { 351 this.priority = castToPositiveInt(value); // PositiveIntType 352 } else 353 return super.setProperty(name, value); 354 return value; 355 } 356 357 @Override 358 public Base makeProperty(int hash, String name) throws FHIRException { 359 switch (hash) { 360 case -351767064: return getCoverage(); 361 case -1165461084: return getPriorityElement(); 362 default: return super.makeProperty(hash, name); 363 } 364 365 } 366 367 @Override 368 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 369 switch (hash) { 370 case -351767064: /*coverage*/ return new String[] {"Reference"}; 371 case -1165461084: /*priority*/ return new String[] {"positiveInt"}; 372 default: return super.getTypesForProperty(hash, name); 373 } 374 375 } 376 377 @Override 378 public Base addChild(String name) throws FHIRException { 379 if (name.equals("coverage")) { 380 this.coverage = new Reference(); 381 return this.coverage; 382 } 383 else if (name.equals("priority")) { 384 throw new FHIRException("Cannot call addChild on a singleton property Account.priority"); 385 } 386 else 387 return super.addChild(name); 388 } 389 390 public CoverageComponent copy() { 391 CoverageComponent dst = new CoverageComponent(); 392 copyValues(dst); 393 dst.coverage = coverage == null ? null : coverage.copy(); 394 dst.priority = priority == null ? null : priority.copy(); 395 return dst; 396 } 397 398 @Override 399 public boolean equalsDeep(Base other_) { 400 if (!super.equalsDeep(other_)) 401 return false; 402 if (!(other_ instanceof CoverageComponent)) 403 return false; 404 CoverageComponent o = (CoverageComponent) other_; 405 return compareDeep(coverage, o.coverage, true) && compareDeep(priority, o.priority, true); 406 } 407 408 @Override 409 public boolean equalsShallow(Base other_) { 410 if (!super.equalsShallow(other_)) 411 return false; 412 if (!(other_ instanceof CoverageComponent)) 413 return false; 414 CoverageComponent o = (CoverageComponent) other_; 415 return compareValues(priority, o.priority, true); 416 } 417 418 public boolean isEmpty() { 419 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(coverage, priority); 420 } 421 422 public String fhirType() { 423 return "Account.coverage"; 424 425 } 426 427 } 428 429 @Block() 430 public static class GuarantorComponent extends BackboneElement implements IBaseBackboneElement { 431 /** 432 * The entity who is responsible. 433 */ 434 @Child(name = "party", type = {Patient.class, RelatedPerson.class, Organization.class}, order=1, min=1, max=1, modifier=false, summary=false) 435 @Description(shortDefinition="Responsible entity", formalDefinition="The entity who is responsible." ) 436 protected Reference party; 437 438 /** 439 * The actual object that is the target of the reference (The entity who is responsible.) 440 */ 441 protected Resource partyTarget; 442 443 /** 444 * A guarantor may be placed on credit hold or otherwise have their role temporarily suspended. 445 */ 446 @Child(name = "onHold", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=false) 447 @Description(shortDefinition="Credit or other hold applied", formalDefinition="A guarantor may be placed on credit hold or otherwise have their role temporarily suspended." ) 448 protected BooleanType onHold; 449 450 /** 451 * The timeframe during which the guarantor accepts responsibility for the account. 452 */ 453 @Child(name = "period", type = {Period.class}, order=3, min=0, max=1, modifier=false, summary=false) 454 @Description(shortDefinition="Guarrantee account during", formalDefinition="The timeframe during which the guarantor accepts responsibility for the account." ) 455 protected Period period; 456 457 private static final long serialVersionUID = -1012345396L; 458 459 /** 460 * Constructor 461 */ 462 public GuarantorComponent() { 463 super(); 464 } 465 466 /** 467 * Constructor 468 */ 469 public GuarantorComponent(Reference party) { 470 super(); 471 this.party = party; 472 } 473 474 /** 475 * @return {@link #party} (The entity who is responsible.) 476 */ 477 public Reference getParty() { 478 if (this.party == null) 479 if (Configuration.errorOnAutoCreate()) 480 throw new Error("Attempt to auto-create GuarantorComponent.party"); 481 else if (Configuration.doAutoCreate()) 482 this.party = new Reference(); // cc 483 return this.party; 484 } 485 486 public boolean hasParty() { 487 return this.party != null && !this.party.isEmpty(); 488 } 489 490 /** 491 * @param value {@link #party} (The entity who is responsible.) 492 */ 493 public GuarantorComponent setParty(Reference value) { 494 this.party = value; 495 return this; 496 } 497 498 /** 499 * @return {@link #party} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The entity who is responsible.) 500 */ 501 public Resource getPartyTarget() { 502 return this.partyTarget; 503 } 504 505 /** 506 * @param value {@link #party} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The entity who is responsible.) 507 */ 508 public GuarantorComponent setPartyTarget(Resource value) { 509 this.partyTarget = value; 510 return this; 511 } 512 513 /** 514 * @return {@link #onHold} (A guarantor may be placed on credit hold or otherwise have their role temporarily suspended.). This is the underlying object with id, value and extensions. The accessor "getOnHold" gives direct access to the value 515 */ 516 public BooleanType getOnHoldElement() { 517 if (this.onHold == null) 518 if (Configuration.errorOnAutoCreate()) 519 throw new Error("Attempt to auto-create GuarantorComponent.onHold"); 520 else if (Configuration.doAutoCreate()) 521 this.onHold = new BooleanType(); // bb 522 return this.onHold; 523 } 524 525 public boolean hasOnHoldElement() { 526 return this.onHold != null && !this.onHold.isEmpty(); 527 } 528 529 public boolean hasOnHold() { 530 return this.onHold != null && !this.onHold.isEmpty(); 531 } 532 533 /** 534 * @param value {@link #onHold} (A guarantor may be placed on credit hold or otherwise have their role temporarily suspended.). This is the underlying object with id, value and extensions. The accessor "getOnHold" gives direct access to the value 535 */ 536 public GuarantorComponent setOnHoldElement(BooleanType value) { 537 this.onHold = value; 538 return this; 539 } 540 541 /** 542 * @return A guarantor may be placed on credit hold or otherwise have their role temporarily suspended. 543 */ 544 public boolean getOnHold() { 545 return this.onHold == null || this.onHold.isEmpty() ? false : this.onHold.getValue(); 546 } 547 548 /** 549 * @param value A guarantor may be placed on credit hold or otherwise have their role temporarily suspended. 550 */ 551 public GuarantorComponent setOnHold(boolean value) { 552 if (this.onHold == null) 553 this.onHold = new BooleanType(); 554 this.onHold.setValue(value); 555 return this; 556 } 557 558 /** 559 * @return {@link #period} (The timeframe during which the guarantor accepts responsibility for the account.) 560 */ 561 public Period getPeriod() { 562 if (this.period == null) 563 if (Configuration.errorOnAutoCreate()) 564 throw new Error("Attempt to auto-create GuarantorComponent.period"); 565 else if (Configuration.doAutoCreate()) 566 this.period = new Period(); // cc 567 return this.period; 568 } 569 570 public boolean hasPeriod() { 571 return this.period != null && !this.period.isEmpty(); 572 } 573 574 /** 575 * @param value {@link #period} (The timeframe during which the guarantor accepts responsibility for the account.) 576 */ 577 public GuarantorComponent setPeriod(Period value) { 578 this.period = value; 579 return this; 580 } 581 582 protected void listChildren(List<Property> children) { 583 super.listChildren(children); 584 children.add(new Property("party", "Reference(Patient|RelatedPerson|Organization)", "The entity who is responsible.", 0, 1, party)); 585 children.add(new Property("onHold", "boolean", "A guarantor may be placed on credit hold or otherwise have their role temporarily suspended.", 0, 1, onHold)); 586 children.add(new Property("period", "Period", "The timeframe during which the guarantor accepts responsibility for the account.", 0, 1, period)); 587 } 588 589 @Override 590 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 591 switch (_hash) { 592 case 106437350: /*party*/ return new Property("party", "Reference(Patient|RelatedPerson|Organization)", "The entity who is responsible.", 0, 1, party); 593 case -1013289154: /*onHold*/ return new Property("onHold", "boolean", "A guarantor may be placed on credit hold or otherwise have their role temporarily suspended.", 0, 1, onHold); 594 case -991726143: /*period*/ return new Property("period", "Period", "The timeframe during which the guarantor accepts responsibility for the account.", 0, 1, period); 595 default: return super.getNamedProperty(_hash, _name, _checkValid); 596 } 597 598 } 599 600 @Override 601 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 602 switch (hash) { 603 case 106437350: /*party*/ return this.party == null ? new Base[0] : new Base[] {this.party}; // Reference 604 case -1013289154: /*onHold*/ return this.onHold == null ? new Base[0] : new Base[] {this.onHold}; // BooleanType 605 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 606 default: return super.getProperty(hash, name, checkValid); 607 } 608 609 } 610 611 @Override 612 public Base setProperty(int hash, String name, Base value) throws FHIRException { 613 switch (hash) { 614 case 106437350: // party 615 this.party = castToReference(value); // Reference 616 return value; 617 case -1013289154: // onHold 618 this.onHold = castToBoolean(value); // BooleanType 619 return value; 620 case -991726143: // period 621 this.period = castToPeriod(value); // Period 622 return value; 623 default: return super.setProperty(hash, name, value); 624 } 625 626 } 627 628 @Override 629 public Base setProperty(String name, Base value) throws FHIRException { 630 if (name.equals("party")) { 631 this.party = castToReference(value); // Reference 632 } else if (name.equals("onHold")) { 633 this.onHold = castToBoolean(value); // BooleanType 634 } else if (name.equals("period")) { 635 this.period = castToPeriod(value); // Period 636 } else 637 return super.setProperty(name, value); 638 return value; 639 } 640 641 @Override 642 public Base makeProperty(int hash, String name) throws FHIRException { 643 switch (hash) { 644 case 106437350: return getParty(); 645 case -1013289154: return getOnHoldElement(); 646 case -991726143: return getPeriod(); 647 default: return super.makeProperty(hash, name); 648 } 649 650 } 651 652 @Override 653 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 654 switch (hash) { 655 case 106437350: /*party*/ return new String[] {"Reference"}; 656 case -1013289154: /*onHold*/ return new String[] {"boolean"}; 657 case -991726143: /*period*/ return new String[] {"Period"}; 658 default: return super.getTypesForProperty(hash, name); 659 } 660 661 } 662 663 @Override 664 public Base addChild(String name) throws FHIRException { 665 if (name.equals("party")) { 666 this.party = new Reference(); 667 return this.party; 668 } 669 else if (name.equals("onHold")) { 670 throw new FHIRException("Cannot call addChild on a singleton property Account.onHold"); 671 } 672 else if (name.equals("period")) { 673 this.period = new Period(); 674 return this.period; 675 } 676 else 677 return super.addChild(name); 678 } 679 680 public GuarantorComponent copy() { 681 GuarantorComponent dst = new GuarantorComponent(); 682 copyValues(dst); 683 dst.party = party == null ? null : party.copy(); 684 dst.onHold = onHold == null ? null : onHold.copy(); 685 dst.period = period == null ? null : period.copy(); 686 return dst; 687 } 688 689 @Override 690 public boolean equalsDeep(Base other_) { 691 if (!super.equalsDeep(other_)) 692 return false; 693 if (!(other_ instanceof GuarantorComponent)) 694 return false; 695 GuarantorComponent o = (GuarantorComponent) other_; 696 return compareDeep(party, o.party, true) && compareDeep(onHold, o.onHold, true) && compareDeep(period, o.period, true) 697 ; 698 } 699 700 @Override 701 public boolean equalsShallow(Base other_) { 702 if (!super.equalsShallow(other_)) 703 return false; 704 if (!(other_ instanceof GuarantorComponent)) 705 return false; 706 GuarantorComponent o = (GuarantorComponent) other_; 707 return compareValues(onHold, o.onHold, true); 708 } 709 710 public boolean isEmpty() { 711 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(party, onHold, period); 712 } 713 714 public String fhirType() { 715 return "Account.guarantor"; 716 717 } 718 719 } 720 721 /** 722 * Unique identifier used to reference the account. May or may not be intended for human use (e.g. credit card number). 723 */ 724 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 725 @Description(shortDefinition="Account number", formalDefinition="Unique identifier used to reference the account. May or may not be intended for human use (e.g. credit card number)." ) 726 protected List<Identifier> identifier; 727 728 /** 729 * Indicates whether the account is presently used/usable or not. 730 */ 731 @Child(name = "status", type = {CodeType.class}, order=1, min=0, max=1, modifier=true, summary=true) 732 @Description(shortDefinition="active | inactive | entered-in-error", formalDefinition="Indicates whether the account is presently used/usable or not." ) 733 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/account-status") 734 protected Enumeration<AccountStatus> status; 735 736 /** 737 * Categorizes the account for reporting and searching purposes. 738 */ 739 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 740 @Description(shortDefinition="E.g. patient, expense, depreciation", formalDefinition="Categorizes the account for reporting and searching purposes." ) 741 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/account-type") 742 protected CodeableConcept type; 743 744 /** 745 * Name used for the account when displaying it to humans in reports, etc. 746 */ 747 @Child(name = "name", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 748 @Description(shortDefinition="Human-readable label", formalDefinition="Name used for the account when displaying it to humans in reports, etc." ) 749 protected StringType name; 750 751 /** 752 * Identifies the patient, device, practitioner, location or other object the account is associated with. 753 */ 754 @Child(name = "subject", type = {Patient.class, Device.class, Practitioner.class, Location.class, HealthcareService.class, Organization.class}, order=4, min=0, max=1, modifier=false, summary=true) 755 @Description(shortDefinition="What is account tied to?", formalDefinition="Identifies the patient, device, practitioner, location or other object the account is associated with." ) 756 protected Reference subject; 757 758 /** 759 * The actual object that is the target of the reference (Identifies the patient, device, practitioner, location or other object the account is associated with.) 760 */ 761 protected Resource subjectTarget; 762 763 /** 764 * Identifies the period of time the account applies to; e.g. accounts created per fiscal year, quarter, etc. 765 */ 766 @Child(name = "period", type = {Period.class}, order=5, min=0, max=1, modifier=false, summary=true) 767 @Description(shortDefinition="Transaction window", formalDefinition="Identifies the period of time the account applies to; e.g. accounts created per fiscal year, quarter, etc." ) 768 protected Period period; 769 770 /** 771 * Indicates the period of time over which the account is allowed to have transactions posted to it. 772This period may be different to the coveragePeriod which is the duration of time that services may occur. 773 */ 774 @Child(name = "active", type = {Period.class}, order=6, min=0, max=1, modifier=false, summary=true) 775 @Description(shortDefinition="Time window that transactions may be posted to this account", formalDefinition="Indicates the period of time over which the account is allowed to have transactions posted to it.\nThis period may be different to the coveragePeriod which is the duration of time that services may occur." ) 776 protected Period active; 777 778 /** 779 * Represents the sum of all credits less all debits associated with the account. Might be positive, zero or negative. 780 */ 781 @Child(name = "balance", type = {Money.class}, order=7, min=0, max=1, modifier=false, summary=false) 782 @Description(shortDefinition="How much is in account?", formalDefinition="Represents the sum of all credits less all debits associated with the account. Might be positive, zero or negative." ) 783 protected Money balance; 784 785 /** 786 * The party(s) that are responsible for covering the payment of this account, and what order should they be applied to the account. 787 */ 788 @Child(name = "coverage", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 789 @Description(shortDefinition="The party(s) that are responsible for covering the payment of this account, and what order should they be applied to the account", formalDefinition="The party(s) that are responsible for covering the payment of this account, and what order should they be applied to the account." ) 790 protected List<CoverageComponent> coverage; 791 792 /** 793 * Indicates the organization, department, etc. with responsibility for the account. 794 */ 795 @Child(name = "owner", type = {Organization.class}, order=9, min=0, max=1, modifier=false, summary=true) 796 @Description(shortDefinition="Who is responsible?", formalDefinition="Indicates the organization, department, etc. with responsibility for the account." ) 797 protected Reference owner; 798 799 /** 800 * The actual object that is the target of the reference (Indicates the organization, department, etc. with responsibility for the account.) 801 */ 802 protected Organization ownerTarget; 803 804 /** 805 * Provides additional information about what the account tracks and how it is used. 806 */ 807 @Child(name = "description", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=true) 808 @Description(shortDefinition="Explanation of purpose/use", formalDefinition="Provides additional information about what the account tracks and how it is used." ) 809 protected StringType description; 810 811 /** 812 * Parties financially responsible for the account. 813 */ 814 @Child(name = "guarantor", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 815 @Description(shortDefinition="Responsible for the account", formalDefinition="Parties financially responsible for the account." ) 816 protected List<GuarantorComponent> guarantor; 817 818 private static final long serialVersionUID = 1653702558L; 819 820 /** 821 * Constructor 822 */ 823 public Account() { 824 super(); 825 } 826 827 /** 828 * @return {@link #identifier} (Unique identifier used to reference the account. May or may not be intended for human use (e.g. credit card number).) 829 */ 830 public List<Identifier> getIdentifier() { 831 if (this.identifier == null) 832 this.identifier = new ArrayList<Identifier>(); 833 return this.identifier; 834 } 835 836 /** 837 * @return Returns a reference to <code>this</code> for easy method chaining 838 */ 839 public Account setIdentifier(List<Identifier> theIdentifier) { 840 this.identifier = theIdentifier; 841 return this; 842 } 843 844 public boolean hasIdentifier() { 845 if (this.identifier == null) 846 return false; 847 for (Identifier item : this.identifier) 848 if (!item.isEmpty()) 849 return true; 850 return false; 851 } 852 853 public Identifier addIdentifier() { //3 854 Identifier t = new Identifier(); 855 if (this.identifier == null) 856 this.identifier = new ArrayList<Identifier>(); 857 this.identifier.add(t); 858 return t; 859 } 860 861 public Account addIdentifier(Identifier t) { //3 862 if (t == null) 863 return this; 864 if (this.identifier == null) 865 this.identifier = new ArrayList<Identifier>(); 866 this.identifier.add(t); 867 return this; 868 } 869 870 /** 871 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 872 */ 873 public Identifier getIdentifierFirstRep() { 874 if (getIdentifier().isEmpty()) { 875 addIdentifier(); 876 } 877 return getIdentifier().get(0); 878 } 879 880 /** 881 * @return {@link #status} (Indicates whether the account is presently used/usable or not.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 882 */ 883 public Enumeration<AccountStatus> getStatusElement() { 884 if (this.status == null) 885 if (Configuration.errorOnAutoCreate()) 886 throw new Error("Attempt to auto-create Account.status"); 887 else if (Configuration.doAutoCreate()) 888 this.status = new Enumeration<AccountStatus>(new AccountStatusEnumFactory()); // bb 889 return this.status; 890 } 891 892 public boolean hasStatusElement() { 893 return this.status != null && !this.status.isEmpty(); 894 } 895 896 public boolean hasStatus() { 897 return this.status != null && !this.status.isEmpty(); 898 } 899 900 /** 901 * @param value {@link #status} (Indicates whether the account is presently used/usable or not.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 902 */ 903 public Account setStatusElement(Enumeration<AccountStatus> value) { 904 this.status = value; 905 return this; 906 } 907 908 /** 909 * @return Indicates whether the account is presently used/usable or not. 910 */ 911 public AccountStatus getStatus() { 912 return this.status == null ? null : this.status.getValue(); 913 } 914 915 /** 916 * @param value Indicates whether the account is presently used/usable or not. 917 */ 918 public Account setStatus(AccountStatus value) { 919 if (value == null) 920 this.status = null; 921 else { 922 if (this.status == null) 923 this.status = new Enumeration<AccountStatus>(new AccountStatusEnumFactory()); 924 this.status.setValue(value); 925 } 926 return this; 927 } 928 929 /** 930 * @return {@link #type} (Categorizes the account for reporting and searching purposes.) 931 */ 932 public CodeableConcept getType() { 933 if (this.type == null) 934 if (Configuration.errorOnAutoCreate()) 935 throw new Error("Attempt to auto-create Account.type"); 936 else if (Configuration.doAutoCreate()) 937 this.type = new CodeableConcept(); // cc 938 return this.type; 939 } 940 941 public boolean hasType() { 942 return this.type != null && !this.type.isEmpty(); 943 } 944 945 /** 946 * @param value {@link #type} (Categorizes the account for reporting and searching purposes.) 947 */ 948 public Account setType(CodeableConcept value) { 949 this.type = value; 950 return this; 951 } 952 953 /** 954 * @return {@link #name} (Name used for the account when displaying it to humans in reports, etc.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 955 */ 956 public StringType getNameElement() { 957 if (this.name == null) 958 if (Configuration.errorOnAutoCreate()) 959 throw new Error("Attempt to auto-create Account.name"); 960 else if (Configuration.doAutoCreate()) 961 this.name = new StringType(); // bb 962 return this.name; 963 } 964 965 public boolean hasNameElement() { 966 return this.name != null && !this.name.isEmpty(); 967 } 968 969 public boolean hasName() { 970 return this.name != null && !this.name.isEmpty(); 971 } 972 973 /** 974 * @param value {@link #name} (Name used for the account when displaying it to humans in reports, etc.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 975 */ 976 public Account setNameElement(StringType value) { 977 this.name = value; 978 return this; 979 } 980 981 /** 982 * @return Name used for the account when displaying it to humans in reports, etc. 983 */ 984 public String getName() { 985 return this.name == null ? null : this.name.getValue(); 986 } 987 988 /** 989 * @param value Name used for the account when displaying it to humans in reports, etc. 990 */ 991 public Account setName(String value) { 992 if (Utilities.noString(value)) 993 this.name = null; 994 else { 995 if (this.name == null) 996 this.name = new StringType(); 997 this.name.setValue(value); 998 } 999 return this; 1000 } 1001 1002 /** 1003 * @return {@link #subject} (Identifies the patient, device, practitioner, location or other object the account is associated with.) 1004 */ 1005 public Reference getSubject() { 1006 if (this.subject == null) 1007 if (Configuration.errorOnAutoCreate()) 1008 throw new Error("Attempt to auto-create Account.subject"); 1009 else if (Configuration.doAutoCreate()) 1010 this.subject = new Reference(); // cc 1011 return this.subject; 1012 } 1013 1014 public boolean hasSubject() { 1015 return this.subject != null && !this.subject.isEmpty(); 1016 } 1017 1018 /** 1019 * @param value {@link #subject} (Identifies the patient, device, practitioner, location or other object the account is associated with.) 1020 */ 1021 public Account setSubject(Reference value) { 1022 this.subject = value; 1023 return this; 1024 } 1025 1026 /** 1027 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Identifies the patient, device, practitioner, location or other object the account is associated with.) 1028 */ 1029 public Resource getSubjectTarget() { 1030 return this.subjectTarget; 1031 } 1032 1033 /** 1034 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Identifies the patient, device, practitioner, location or other object the account is associated with.) 1035 */ 1036 public Account setSubjectTarget(Resource value) { 1037 this.subjectTarget = value; 1038 return this; 1039 } 1040 1041 /** 1042 * @return {@link #period} (Identifies the period of time the account applies to; e.g. accounts created per fiscal year, quarter, etc.) 1043 */ 1044 public Period getPeriod() { 1045 if (this.period == null) 1046 if (Configuration.errorOnAutoCreate()) 1047 throw new Error("Attempt to auto-create Account.period"); 1048 else if (Configuration.doAutoCreate()) 1049 this.period = new Period(); // cc 1050 return this.period; 1051 } 1052 1053 public boolean hasPeriod() { 1054 return this.period != null && !this.period.isEmpty(); 1055 } 1056 1057 /** 1058 * @param value {@link #period} (Identifies the period of time the account applies to; e.g. accounts created per fiscal year, quarter, etc.) 1059 */ 1060 public Account setPeriod(Period value) { 1061 this.period = value; 1062 return this; 1063 } 1064 1065 /** 1066 * @return {@link #active} (Indicates the period of time over which the account is allowed to have transactions posted to it. 1067This period may be different to the coveragePeriod which is the duration of time that services may occur.) 1068 */ 1069 public Period getActive() { 1070 if (this.active == null) 1071 if (Configuration.errorOnAutoCreate()) 1072 throw new Error("Attempt to auto-create Account.active"); 1073 else if (Configuration.doAutoCreate()) 1074 this.active = new Period(); // cc 1075 return this.active; 1076 } 1077 1078 public boolean hasActive() { 1079 return this.active != null && !this.active.isEmpty(); 1080 } 1081 1082 /** 1083 * @param value {@link #active} (Indicates the period of time over which the account is allowed to have transactions posted to it. 1084This period may be different to the coveragePeriod which is the duration of time that services may occur.) 1085 */ 1086 public Account setActive(Period value) { 1087 this.active = value; 1088 return this; 1089 } 1090 1091 /** 1092 * @return {@link #balance} (Represents the sum of all credits less all debits associated with the account. Might be positive, zero or negative.) 1093 */ 1094 public Money getBalance() { 1095 if (this.balance == null) 1096 if (Configuration.errorOnAutoCreate()) 1097 throw new Error("Attempt to auto-create Account.balance"); 1098 else if (Configuration.doAutoCreate()) 1099 this.balance = new Money(); // cc 1100 return this.balance; 1101 } 1102 1103 public boolean hasBalance() { 1104 return this.balance != null && !this.balance.isEmpty(); 1105 } 1106 1107 /** 1108 * @param value {@link #balance} (Represents the sum of all credits less all debits associated with the account. Might be positive, zero or negative.) 1109 */ 1110 public Account setBalance(Money value) { 1111 this.balance = value; 1112 return this; 1113 } 1114 1115 /** 1116 * @return {@link #coverage} (The party(s) that are responsible for covering the payment of this account, and what order should they be applied to the account.) 1117 */ 1118 public List<CoverageComponent> getCoverage() { 1119 if (this.coverage == null) 1120 this.coverage = new ArrayList<CoverageComponent>(); 1121 return this.coverage; 1122 } 1123 1124 /** 1125 * @return Returns a reference to <code>this</code> for easy method chaining 1126 */ 1127 public Account setCoverage(List<CoverageComponent> theCoverage) { 1128 this.coverage = theCoverage; 1129 return this; 1130 } 1131 1132 public boolean hasCoverage() { 1133 if (this.coverage == null) 1134 return false; 1135 for (CoverageComponent item : this.coverage) 1136 if (!item.isEmpty()) 1137 return true; 1138 return false; 1139 } 1140 1141 public CoverageComponent addCoverage() { //3 1142 CoverageComponent t = new CoverageComponent(); 1143 if (this.coverage == null) 1144 this.coverage = new ArrayList<CoverageComponent>(); 1145 this.coverage.add(t); 1146 return t; 1147 } 1148 1149 public Account addCoverage(CoverageComponent t) { //3 1150 if (t == null) 1151 return this; 1152 if (this.coverage == null) 1153 this.coverage = new ArrayList<CoverageComponent>(); 1154 this.coverage.add(t); 1155 return this; 1156 } 1157 1158 /** 1159 * @return The first repetition of repeating field {@link #coverage}, creating it if it does not already exist 1160 */ 1161 public CoverageComponent getCoverageFirstRep() { 1162 if (getCoverage().isEmpty()) { 1163 addCoverage(); 1164 } 1165 return getCoverage().get(0); 1166 } 1167 1168 /** 1169 * @return {@link #owner} (Indicates the organization, department, etc. with responsibility for the account.) 1170 */ 1171 public Reference getOwner() { 1172 if (this.owner == null) 1173 if (Configuration.errorOnAutoCreate()) 1174 throw new Error("Attempt to auto-create Account.owner"); 1175 else if (Configuration.doAutoCreate()) 1176 this.owner = new Reference(); // cc 1177 return this.owner; 1178 } 1179 1180 public boolean hasOwner() { 1181 return this.owner != null && !this.owner.isEmpty(); 1182 } 1183 1184 /** 1185 * @param value {@link #owner} (Indicates the organization, department, etc. with responsibility for the account.) 1186 */ 1187 public Account setOwner(Reference value) { 1188 this.owner = value; 1189 return this; 1190 } 1191 1192 /** 1193 * @return {@link #owner} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Indicates the organization, department, etc. with responsibility for the account.) 1194 */ 1195 public Organization getOwnerTarget() { 1196 if (this.ownerTarget == null) 1197 if (Configuration.errorOnAutoCreate()) 1198 throw new Error("Attempt to auto-create Account.owner"); 1199 else if (Configuration.doAutoCreate()) 1200 this.ownerTarget = new Organization(); // aa 1201 return this.ownerTarget; 1202 } 1203 1204 /** 1205 * @param value {@link #owner} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Indicates the organization, department, etc. with responsibility for the account.) 1206 */ 1207 public Account setOwnerTarget(Organization value) { 1208 this.ownerTarget = value; 1209 return this; 1210 } 1211 1212 /** 1213 * @return {@link #description} (Provides additional information about what the account tracks and how it is used.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1214 */ 1215 public StringType getDescriptionElement() { 1216 if (this.description == null) 1217 if (Configuration.errorOnAutoCreate()) 1218 throw new Error("Attempt to auto-create Account.description"); 1219 else if (Configuration.doAutoCreate()) 1220 this.description = new StringType(); // bb 1221 return this.description; 1222 } 1223 1224 public boolean hasDescriptionElement() { 1225 return this.description != null && !this.description.isEmpty(); 1226 } 1227 1228 public boolean hasDescription() { 1229 return this.description != null && !this.description.isEmpty(); 1230 } 1231 1232 /** 1233 * @param value {@link #description} (Provides additional information about what the account tracks and how it is used.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1234 */ 1235 public Account setDescriptionElement(StringType value) { 1236 this.description = value; 1237 return this; 1238 } 1239 1240 /** 1241 * @return Provides additional information about what the account tracks and how it is used. 1242 */ 1243 public String getDescription() { 1244 return this.description == null ? null : this.description.getValue(); 1245 } 1246 1247 /** 1248 * @param value Provides additional information about what the account tracks and how it is used. 1249 */ 1250 public Account setDescription(String value) { 1251 if (Utilities.noString(value)) 1252 this.description = null; 1253 else { 1254 if (this.description == null) 1255 this.description = new StringType(); 1256 this.description.setValue(value); 1257 } 1258 return this; 1259 } 1260 1261 /** 1262 * @return {@link #guarantor} (Parties financially responsible for the account.) 1263 */ 1264 public List<GuarantorComponent> getGuarantor() { 1265 if (this.guarantor == null) 1266 this.guarantor = new ArrayList<GuarantorComponent>(); 1267 return this.guarantor; 1268 } 1269 1270 /** 1271 * @return Returns a reference to <code>this</code> for easy method chaining 1272 */ 1273 public Account setGuarantor(List<GuarantorComponent> theGuarantor) { 1274 this.guarantor = theGuarantor; 1275 return this; 1276 } 1277 1278 public boolean hasGuarantor() { 1279 if (this.guarantor == null) 1280 return false; 1281 for (GuarantorComponent item : this.guarantor) 1282 if (!item.isEmpty()) 1283 return true; 1284 return false; 1285 } 1286 1287 public GuarantorComponent addGuarantor() { //3 1288 GuarantorComponent t = new GuarantorComponent(); 1289 if (this.guarantor == null) 1290 this.guarantor = new ArrayList<GuarantorComponent>(); 1291 this.guarantor.add(t); 1292 return t; 1293 } 1294 1295 public Account addGuarantor(GuarantorComponent t) { //3 1296 if (t == null) 1297 return this; 1298 if (this.guarantor == null) 1299 this.guarantor = new ArrayList<GuarantorComponent>(); 1300 this.guarantor.add(t); 1301 return this; 1302 } 1303 1304 /** 1305 * @return The first repetition of repeating field {@link #guarantor}, creating it if it does not already exist 1306 */ 1307 public GuarantorComponent getGuarantorFirstRep() { 1308 if (getGuarantor().isEmpty()) { 1309 addGuarantor(); 1310 } 1311 return getGuarantor().get(0); 1312 } 1313 1314 protected void listChildren(List<Property> children) { 1315 super.listChildren(children); 1316 children.add(new Property("identifier", "Identifier", "Unique identifier used to reference the account. May or may not be intended for human use (e.g. credit card number).", 0, java.lang.Integer.MAX_VALUE, identifier)); 1317 children.add(new Property("status", "code", "Indicates whether the account is presently used/usable or not.", 0, 1, status)); 1318 children.add(new Property("type", "CodeableConcept", "Categorizes the account for reporting and searching purposes.", 0, 1, type)); 1319 children.add(new Property("name", "string", "Name used for the account when displaying it to humans in reports, etc.", 0, 1, name)); 1320 children.add(new Property("subject", "Reference(Patient|Device|Practitioner|Location|HealthcareService|Organization)", "Identifies the patient, device, practitioner, location or other object the account is associated with.", 0, 1, subject)); 1321 children.add(new Property("period", "Period", "Identifies the period of time the account applies to; e.g. accounts created per fiscal year, quarter, etc.", 0, 1, period)); 1322 children.add(new Property("active", "Period", "Indicates the period of time over which the account is allowed to have transactions posted to it.\nThis period may be different to the coveragePeriod which is the duration of time that services may occur.", 0, 1, active)); 1323 children.add(new Property("balance", "Money", "Represents the sum of all credits less all debits associated with the account. Might be positive, zero or negative.", 0, 1, balance)); 1324 children.add(new Property("coverage", "", "The party(s) that are responsible for covering the payment of this account, and what order should they be applied to the account.", 0, java.lang.Integer.MAX_VALUE, coverage)); 1325 children.add(new Property("owner", "Reference(Organization)", "Indicates the organization, department, etc. with responsibility for the account.", 0, 1, owner)); 1326 children.add(new Property("description", "string", "Provides additional information about what the account tracks and how it is used.", 0, 1, description)); 1327 children.add(new Property("guarantor", "", "Parties financially responsible for the account.", 0, java.lang.Integer.MAX_VALUE, guarantor)); 1328 } 1329 1330 @Override 1331 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1332 switch (_hash) { 1333 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Unique identifier used to reference the account. May or may not be intended for human use (e.g. credit card number).", 0, java.lang.Integer.MAX_VALUE, identifier); 1334 case -892481550: /*status*/ return new Property("status", "code", "Indicates whether the account is presently used/usable or not.", 0, 1, status); 1335 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Categorizes the account for reporting and searching purposes.", 0, 1, type); 1336 case 3373707: /*name*/ return new Property("name", "string", "Name used for the account when displaying it to humans in reports, etc.", 0, 1, name); 1337 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Device|Practitioner|Location|HealthcareService|Organization)", "Identifies the patient, device, practitioner, location or other object the account is associated with.", 0, 1, subject); 1338 case -991726143: /*period*/ return new Property("period", "Period", "Identifies the period of time the account applies to; e.g. accounts created per fiscal year, quarter, etc.", 0, 1, period); 1339 case -1422950650: /*active*/ return new Property("active", "Period", "Indicates the period of time over which the account is allowed to have transactions posted to it.\nThis period may be different to the coveragePeriod which is the duration of time that services may occur.", 0, 1, active); 1340 case -339185956: /*balance*/ return new Property("balance", "Money", "Represents the sum of all credits less all debits associated with the account. Might be positive, zero or negative.", 0, 1, balance); 1341 case -351767064: /*coverage*/ return new Property("coverage", "", "The party(s) that are responsible for covering the payment of this account, and what order should they be applied to the account.", 0, java.lang.Integer.MAX_VALUE, coverage); 1342 case 106164915: /*owner*/ return new Property("owner", "Reference(Organization)", "Indicates the organization, department, etc. with responsibility for the account.", 0, 1, owner); 1343 case -1724546052: /*description*/ return new Property("description", "string", "Provides additional information about what the account tracks and how it is used.", 0, 1, description); 1344 case -188629045: /*guarantor*/ return new Property("guarantor", "", "Parties financially responsible for the account.", 0, java.lang.Integer.MAX_VALUE, guarantor); 1345 default: return super.getNamedProperty(_hash, _name, _checkValid); 1346 } 1347 1348 } 1349 1350 @Override 1351 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1352 switch (hash) { 1353 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1354 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<AccountStatus> 1355 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1356 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1357 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1358 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 1359 case -1422950650: /*active*/ return this.active == null ? new Base[0] : new Base[] {this.active}; // Period 1360 case -339185956: /*balance*/ return this.balance == null ? new Base[0] : new Base[] {this.balance}; // Money 1361 case -351767064: /*coverage*/ return this.coverage == null ? new Base[0] : this.coverage.toArray(new Base[this.coverage.size()]); // CoverageComponent 1362 case 106164915: /*owner*/ return this.owner == null ? new Base[0] : new Base[] {this.owner}; // Reference 1363 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 1364 case -188629045: /*guarantor*/ return this.guarantor == null ? new Base[0] : this.guarantor.toArray(new Base[this.guarantor.size()]); // GuarantorComponent 1365 default: return super.getProperty(hash, name, checkValid); 1366 } 1367 1368 } 1369 1370 @Override 1371 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1372 switch (hash) { 1373 case -1618432855: // identifier 1374 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1375 return value; 1376 case -892481550: // status 1377 value = new AccountStatusEnumFactory().fromType(castToCode(value)); 1378 this.status = (Enumeration) value; // Enumeration<AccountStatus> 1379 return value; 1380 case 3575610: // type 1381 this.type = castToCodeableConcept(value); // CodeableConcept 1382 return value; 1383 case 3373707: // name 1384 this.name = castToString(value); // StringType 1385 return value; 1386 case -1867885268: // subject 1387 this.subject = castToReference(value); // Reference 1388 return value; 1389 case -991726143: // period 1390 this.period = castToPeriod(value); // Period 1391 return value; 1392 case -1422950650: // active 1393 this.active = castToPeriod(value); // Period 1394 return value; 1395 case -339185956: // balance 1396 this.balance = castToMoney(value); // Money 1397 return value; 1398 case -351767064: // coverage 1399 this.getCoverage().add((CoverageComponent) value); // CoverageComponent 1400 return value; 1401 case 106164915: // owner 1402 this.owner = castToReference(value); // Reference 1403 return value; 1404 case -1724546052: // description 1405 this.description = castToString(value); // StringType 1406 return value; 1407 case -188629045: // guarantor 1408 this.getGuarantor().add((GuarantorComponent) value); // GuarantorComponent 1409 return value; 1410 default: return super.setProperty(hash, name, value); 1411 } 1412 1413 } 1414 1415 @Override 1416 public Base setProperty(String name, Base value) throws FHIRException { 1417 if (name.equals("identifier")) { 1418 this.getIdentifier().add(castToIdentifier(value)); 1419 } else if (name.equals("status")) { 1420 value = new AccountStatusEnumFactory().fromType(castToCode(value)); 1421 this.status = (Enumeration) value; // Enumeration<AccountStatus> 1422 } else if (name.equals("type")) { 1423 this.type = castToCodeableConcept(value); // CodeableConcept 1424 } else if (name.equals("name")) { 1425 this.name = castToString(value); // StringType 1426 } else if (name.equals("subject")) { 1427 this.subject = castToReference(value); // Reference 1428 } else if (name.equals("period")) { 1429 this.period = castToPeriod(value); // Period 1430 } else if (name.equals("active")) { 1431 this.active = castToPeriod(value); // Period 1432 } else if (name.equals("balance")) { 1433 this.balance = castToMoney(value); // Money 1434 } else if (name.equals("coverage")) { 1435 this.getCoverage().add((CoverageComponent) value); 1436 } else if (name.equals("owner")) { 1437 this.owner = castToReference(value); // Reference 1438 } else if (name.equals("description")) { 1439 this.description = castToString(value); // StringType 1440 } else if (name.equals("guarantor")) { 1441 this.getGuarantor().add((GuarantorComponent) value); 1442 } else 1443 return super.setProperty(name, value); 1444 return value; 1445 } 1446 1447 @Override 1448 public Base makeProperty(int hash, String name) throws FHIRException { 1449 switch (hash) { 1450 case -1618432855: return addIdentifier(); 1451 case -892481550: return getStatusElement(); 1452 case 3575610: return getType(); 1453 case 3373707: return getNameElement(); 1454 case -1867885268: return getSubject(); 1455 case -991726143: return getPeriod(); 1456 case -1422950650: return getActive(); 1457 case -339185956: return getBalance(); 1458 case -351767064: return addCoverage(); 1459 case 106164915: return getOwner(); 1460 case -1724546052: return getDescriptionElement(); 1461 case -188629045: return addGuarantor(); 1462 default: return super.makeProperty(hash, name); 1463 } 1464 1465 } 1466 1467 @Override 1468 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1469 switch (hash) { 1470 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1471 case -892481550: /*status*/ return new String[] {"code"}; 1472 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1473 case 3373707: /*name*/ return new String[] {"string"}; 1474 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1475 case -991726143: /*period*/ return new String[] {"Period"}; 1476 case -1422950650: /*active*/ return new String[] {"Period"}; 1477 case -339185956: /*balance*/ return new String[] {"Money"}; 1478 case -351767064: /*coverage*/ return new String[] {}; 1479 case 106164915: /*owner*/ return new String[] {"Reference"}; 1480 case -1724546052: /*description*/ return new String[] {"string"}; 1481 case -188629045: /*guarantor*/ return new String[] {}; 1482 default: return super.getTypesForProperty(hash, name); 1483 } 1484 1485 } 1486 1487 @Override 1488 public Base addChild(String name) throws FHIRException { 1489 if (name.equals("identifier")) { 1490 return addIdentifier(); 1491 } 1492 else if (name.equals("status")) { 1493 throw new FHIRException("Cannot call addChild on a singleton property Account.status"); 1494 } 1495 else if (name.equals("type")) { 1496 this.type = new CodeableConcept(); 1497 return this.type; 1498 } 1499 else if (name.equals("name")) { 1500 throw new FHIRException("Cannot call addChild on a singleton property Account.name"); 1501 } 1502 else if (name.equals("subject")) { 1503 this.subject = new Reference(); 1504 return this.subject; 1505 } 1506 else if (name.equals("period")) { 1507 this.period = new Period(); 1508 return this.period; 1509 } 1510 else if (name.equals("active")) { 1511 this.active = new Period(); 1512 return this.active; 1513 } 1514 else if (name.equals("balance")) { 1515 this.balance = new Money(); 1516 return this.balance; 1517 } 1518 else if (name.equals("coverage")) { 1519 return addCoverage(); 1520 } 1521 else if (name.equals("owner")) { 1522 this.owner = new Reference(); 1523 return this.owner; 1524 } 1525 else if (name.equals("description")) { 1526 throw new FHIRException("Cannot call addChild on a singleton property Account.description"); 1527 } 1528 else if (name.equals("guarantor")) { 1529 return addGuarantor(); 1530 } 1531 else 1532 return super.addChild(name); 1533 } 1534 1535 public String fhirType() { 1536 return "Account"; 1537 1538 } 1539 1540 public Account copy() { 1541 Account dst = new Account(); 1542 copyValues(dst); 1543 if (identifier != null) { 1544 dst.identifier = new ArrayList<Identifier>(); 1545 for (Identifier i : identifier) 1546 dst.identifier.add(i.copy()); 1547 }; 1548 dst.status = status == null ? null : status.copy(); 1549 dst.type = type == null ? null : type.copy(); 1550 dst.name = name == null ? null : name.copy(); 1551 dst.subject = subject == null ? null : subject.copy(); 1552 dst.period = period == null ? null : period.copy(); 1553 dst.active = active == null ? null : active.copy(); 1554 dst.balance = balance == null ? null : balance.copy(); 1555 if (coverage != null) { 1556 dst.coverage = new ArrayList<CoverageComponent>(); 1557 for (CoverageComponent i : coverage) 1558 dst.coverage.add(i.copy()); 1559 }; 1560 dst.owner = owner == null ? null : owner.copy(); 1561 dst.description = description == null ? null : description.copy(); 1562 if (guarantor != null) { 1563 dst.guarantor = new ArrayList<GuarantorComponent>(); 1564 for (GuarantorComponent i : guarantor) 1565 dst.guarantor.add(i.copy()); 1566 }; 1567 return dst; 1568 } 1569 1570 protected Account typedCopy() { 1571 return copy(); 1572 } 1573 1574 @Override 1575 public boolean equalsDeep(Base other_) { 1576 if (!super.equalsDeep(other_)) 1577 return false; 1578 if (!(other_ instanceof Account)) 1579 return false; 1580 Account o = (Account) other_; 1581 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(type, o.type, true) 1582 && compareDeep(name, o.name, true) && compareDeep(subject, o.subject, true) && compareDeep(period, o.period, true) 1583 && compareDeep(active, o.active, true) && compareDeep(balance, o.balance, true) && compareDeep(coverage, o.coverage, true) 1584 && compareDeep(owner, o.owner, true) && compareDeep(description, o.description, true) && compareDeep(guarantor, o.guarantor, true) 1585 ; 1586 } 1587 1588 @Override 1589 public boolean equalsShallow(Base other_) { 1590 if (!super.equalsShallow(other_)) 1591 return false; 1592 if (!(other_ instanceof Account)) 1593 return false; 1594 Account o = (Account) other_; 1595 return compareValues(status, o.status, true) && compareValues(name, o.name, true) && compareValues(description, o.description, true) 1596 ; 1597 } 1598 1599 public boolean isEmpty() { 1600 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, type 1601 , name, subject, period, active, balance, coverage, owner, description, guarantor 1602 ); 1603 } 1604 1605 @Override 1606 public ResourceType getResourceType() { 1607 return ResourceType.Account; 1608 } 1609 1610 /** 1611 * Search parameter: <b>owner</b> 1612 * <p> 1613 * Description: <b>Who is responsible?</b><br> 1614 * Type: <b>reference</b><br> 1615 * Path: <b>Account.owner</b><br> 1616 * </p> 1617 */ 1618 @SearchParamDefinition(name="owner", path="Account.owner", description="Who is responsible?", type="reference", target={Organization.class } ) 1619 public static final String SP_OWNER = "owner"; 1620 /** 1621 * <b>Fluent Client</b> search parameter constant for <b>owner</b> 1622 * <p> 1623 * Description: <b>Who is responsible?</b><br> 1624 * Type: <b>reference</b><br> 1625 * Path: <b>Account.owner</b><br> 1626 * </p> 1627 */ 1628 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam OWNER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_OWNER); 1629 1630/** 1631 * Constant for fluent queries to be used to add include statements. Specifies 1632 * the path value of "<b>Account:owner</b>". 1633 */ 1634 public static final ca.uhn.fhir.model.api.Include INCLUDE_OWNER = new ca.uhn.fhir.model.api.Include("Account:owner").toLocked(); 1635 1636 /** 1637 * Search parameter: <b>identifier</b> 1638 * <p> 1639 * Description: <b>Account number</b><br> 1640 * Type: <b>token</b><br> 1641 * Path: <b>Account.identifier</b><br> 1642 * </p> 1643 */ 1644 @SearchParamDefinition(name="identifier", path="Account.identifier", description="Account number", type="token" ) 1645 public static final String SP_IDENTIFIER = "identifier"; 1646 /** 1647 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1648 * <p> 1649 * Description: <b>Account number</b><br> 1650 * Type: <b>token</b><br> 1651 * Path: <b>Account.identifier</b><br> 1652 * </p> 1653 */ 1654 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1655 1656 /** 1657 * Search parameter: <b>period</b> 1658 * <p> 1659 * Description: <b>Transaction window</b><br> 1660 * Type: <b>date</b><br> 1661 * Path: <b>Account.period</b><br> 1662 * </p> 1663 */ 1664 @SearchParamDefinition(name="period", path="Account.period", description="Transaction window", type="date" ) 1665 public static final String SP_PERIOD = "period"; 1666 /** 1667 * <b>Fluent Client</b> search parameter constant for <b>period</b> 1668 * <p> 1669 * Description: <b>Transaction window</b><br> 1670 * Type: <b>date</b><br> 1671 * Path: <b>Account.period</b><br> 1672 * </p> 1673 */ 1674 public static final ca.uhn.fhir.rest.gclient.DateClientParam PERIOD = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_PERIOD); 1675 1676 /** 1677 * Search parameter: <b>balance</b> 1678 * <p> 1679 * Description: <b>How much is in account?</b><br> 1680 * Type: <b>quantity</b><br> 1681 * Path: <b>Account.balance</b><br> 1682 * </p> 1683 */ 1684 @SearchParamDefinition(name="balance", path="Account.balance", description="How much is in account?", type="quantity" ) 1685 public static final String SP_BALANCE = "balance"; 1686 /** 1687 * <b>Fluent Client</b> search parameter constant for <b>balance</b> 1688 * <p> 1689 * Description: <b>How much is in account?</b><br> 1690 * Type: <b>quantity</b><br> 1691 * Path: <b>Account.balance</b><br> 1692 * </p> 1693 */ 1694 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam BALANCE = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_BALANCE); 1695 1696 /** 1697 * Search parameter: <b>subject</b> 1698 * <p> 1699 * Description: <b>What is account tied to?</b><br> 1700 * Type: <b>reference</b><br> 1701 * Path: <b>Account.subject</b><br> 1702 * </p> 1703 */ 1704 @SearchParamDefinition(name="subject", path="Account.subject", description="What is account tied to?", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Device.class, HealthcareService.class, Location.class, Organization.class, Patient.class, Practitioner.class } ) 1705 public static final String SP_SUBJECT = "subject"; 1706 /** 1707 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 1708 * <p> 1709 * Description: <b>What is account tied to?</b><br> 1710 * Type: <b>reference</b><br> 1711 * Path: <b>Account.subject</b><br> 1712 * </p> 1713 */ 1714 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 1715 1716/** 1717 * Constant for fluent queries to be used to add include statements. Specifies 1718 * the path value of "<b>Account:subject</b>". 1719 */ 1720 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Account:subject").toLocked(); 1721 1722 /** 1723 * Search parameter: <b>patient</b> 1724 * <p> 1725 * Description: <b>What is account tied to?</b><br> 1726 * Type: <b>reference</b><br> 1727 * Path: <b>Account.subject</b><br> 1728 * </p> 1729 */ 1730 @SearchParamDefinition(name="patient", path="Account.subject", description="What is account tied to?", type="reference", target={Patient.class } ) 1731 public static final String SP_PATIENT = "patient"; 1732 /** 1733 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1734 * <p> 1735 * Description: <b>What is account tied to?</b><br> 1736 * Type: <b>reference</b><br> 1737 * Path: <b>Account.subject</b><br> 1738 * </p> 1739 */ 1740 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 1741 1742/** 1743 * Constant for fluent queries to be used to add include statements. Specifies 1744 * the path value of "<b>Account:patient</b>". 1745 */ 1746 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Account:patient").toLocked(); 1747 1748 /** 1749 * Search parameter: <b>name</b> 1750 * <p> 1751 * Description: <b>Human-readable label</b><br> 1752 * Type: <b>string</b><br> 1753 * Path: <b>Account.name</b><br> 1754 * </p> 1755 */ 1756 @SearchParamDefinition(name="name", path="Account.name", description="Human-readable label", type="string" ) 1757 public static final String SP_NAME = "name"; 1758 /** 1759 * <b>Fluent Client</b> search parameter constant for <b>name</b> 1760 * <p> 1761 * Description: <b>Human-readable label</b><br> 1762 * Type: <b>string</b><br> 1763 * Path: <b>Account.name</b><br> 1764 * </p> 1765 */ 1766 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 1767 1768 /** 1769 * Search parameter: <b>type</b> 1770 * <p> 1771 * Description: <b>E.g. patient, expense, depreciation</b><br> 1772 * Type: <b>token</b><br> 1773 * Path: <b>Account.type</b><br> 1774 * </p> 1775 */ 1776 @SearchParamDefinition(name="type", path="Account.type", description="E.g. patient, expense, depreciation", type="token" ) 1777 public static final String SP_TYPE = "type"; 1778 /** 1779 * <b>Fluent Client</b> search parameter constant for <b>type</b> 1780 * <p> 1781 * Description: <b>E.g. patient, expense, depreciation</b><br> 1782 * Type: <b>token</b><br> 1783 * Path: <b>Account.type</b><br> 1784 * </p> 1785 */ 1786 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 1787 1788 /** 1789 * Search parameter: <b>status</b> 1790 * <p> 1791 * Description: <b>active | inactive | entered-in-error</b><br> 1792 * Type: <b>token</b><br> 1793 * Path: <b>Account.status</b><br> 1794 * </p> 1795 */ 1796 @SearchParamDefinition(name="status", path="Account.status", description="active | inactive | entered-in-error", type="token" ) 1797 public static final String SP_STATUS = "status"; 1798 /** 1799 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1800 * <p> 1801 * Description: <b>active | inactive | entered-in-error</b><br> 1802 * Type: <b>token</b><br> 1803 * Path: <b>Account.status</b><br> 1804 * </p> 1805 */ 1806 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1807 1808 1809}