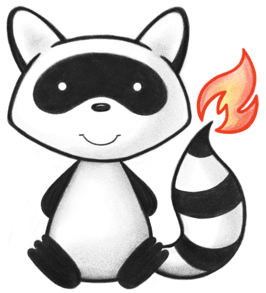
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.ArrayList; 037import java.util.Date; 038import java.util.List; 039 040import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus; 041import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatusEnumFactory; 042import org.hl7.fhir.exceptions.FHIRException; 043import org.hl7.fhir.exceptions.FHIRFormatError; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import org.hl7.fhir.utilities.Utilities; 046 047import ca.uhn.fhir.model.api.annotation.Block; 048import ca.uhn.fhir.model.api.annotation.Child; 049import ca.uhn.fhir.model.api.annotation.ChildOrder; 050import ca.uhn.fhir.model.api.annotation.Description; 051import ca.uhn.fhir.model.api.annotation.ResourceDef; 052import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 053/** 054 * This resource allows for the definition of some activity to be performed, independent of a particular patient, practitioner, or other performance context. 055 */ 056@ResourceDef(name="ActivityDefinition", profile="http://hl7.org/fhir/Profile/ActivityDefinition") 057@ChildOrder(names={"url", "identifier", "version", "name", "title", "status", "experimental", "date", "publisher", "description", "purpose", "usage", "approvalDate", "lastReviewDate", "effectivePeriod", "useContext", "jurisdiction", "topic", "contributor", "contact", "copyright", "relatedArtifact", "library", "kind", "code", "timing[x]", "location", "participant", "product[x]", "quantity", "dosage", "bodySite", "transform", "dynamicValue"}) 058public class ActivityDefinition extends MetadataResource { 059 060 public enum ActivityDefinitionKind { 061 /** 062 * A financial tool for tracking value accrued for a particular purpose. In the healthcare field, used to track charges for a patient, cost centers, etc. 063 */ 064 ACCOUNT, 065 /** 066 * This resource allows for the definition of some activity to be performed, independent of a particular patient, practitioner, or other performance context. 067 */ 068 ACTIVITYDEFINITION, 069 /** 070 * Actual or potential/avoided event causing unintended physical injury resulting from or contributed to by medical care, a research study or other healthcare setting factors that requires additional monitoring, treatment, or hospitalization, or that results in death. 071 */ 072 ADVERSEEVENT, 073 /** 074 * Risk of harmful or undesirable, physiological response which is unique to an individual and associated with exposure to a substance. 075 */ 076 ALLERGYINTOLERANCE, 077 /** 078 * A booking of a healthcare event among patient(s), practitioner(s), related person(s) and/or device(s) for a specific date/time. This may result in one or more Encounter(s). 079 */ 080 APPOINTMENT, 081 /** 082 * A reply to an appointment request for a patient and/or practitioner(s), such as a confirmation or rejection. 083 */ 084 APPOINTMENTRESPONSE, 085 /** 086 * A record of an event made for purposes of maintaining a security log. Typical uses include detection of intrusion attempts and monitoring for inappropriate usage. 087 */ 088 AUDITEVENT, 089 /** 090 * Basic is used for handling concepts not yet defined in FHIR, narrative-only resources that don't map to an existing resource, and custom resources not appropriate for inclusion in the FHIR specification. 091 */ 092 BASIC, 093 /** 094 * A binary resource can contain any content, whether text, image, pdf, zip archive, etc. 095 */ 096 BINARY, 097 /** 098 * Record details about the anatomical location of a specimen or body part. This resource may be used when a coded concept does not provide the necessary detail needed for the use case. 099 */ 100 BODYSITE, 101 /** 102 * A container for a collection of resources. 103 */ 104 BUNDLE, 105 /** 106 * A Capability Statement documents a set of capabilities (behaviors) of a FHIR Server that may be used as a statement of actual server functionality or a statement of required or desired server implementation. 107 */ 108 CAPABILITYSTATEMENT, 109 /** 110 * Describes the intention of how one or more practitioners intend to deliver care for a particular patient, group or community for a period of time, possibly limited to care for a specific condition or set of conditions. 111 */ 112 CAREPLAN, 113 /** 114 * The Care Team includes all the people and organizations who plan to participate in the coordination and delivery of care for a patient. 115 */ 116 CARETEAM, 117 /** 118 * The resource ChargeItem describes the provision of healthcare provider products for a certain patient, therefore referring not only to the product, but containing in addition details of the provision, like date, time, amounts and participating organizations and persons. Main Usage of the ChargeItem is to enable the billing process and internal cost allocation. 119 */ 120 CHARGEITEM, 121 /** 122 * A provider issued list of services and products provided, or to be provided, to a patient which is provided to an insurer for payment recovery. 123 */ 124 CLAIM, 125 /** 126 * This resource provides the adjudication details from the processing of a Claim resource. 127 */ 128 CLAIMRESPONSE, 129 /** 130 * A record of a clinical assessment performed to determine what problem(s) may affect the patient and before planning the treatments or management strategies that are best to manage a patient's condition. Assessments are often 1:1 with a clinical consultation / encounter, but this varies greatly depending on the clinical workflow. This resource is called "ClinicalImpression" rather than "ClinicalAssessment" to avoid confusion with the recording of assessment tools such as Apgar score. 131 */ 132 CLINICALIMPRESSION, 133 /** 134 * A code system resource specifies a set of codes drawn from one or more code systems. 135 */ 136 CODESYSTEM, 137 /** 138 * An occurrence of information being transmitted; e.g. an alert that was sent to a responsible provider, a public health agency was notified about a reportable condition. 139 */ 140 COMMUNICATION, 141 /** 142 * A request to convey information; e.g. the CDS system proposes that an alert be sent to a responsible provider, the CDS system proposes that the public health agency be notified about a reportable condition. 143 */ 144 COMMUNICATIONREQUEST, 145 /** 146 * A compartment definition that defines how resources are accessed on a server. 147 */ 148 COMPARTMENTDEFINITION, 149 /** 150 * A set of healthcare-related information that is assembled together into a single logical document that provides a single coherent statement of meaning, establishes its own context and that has clinical attestation with regard to who is making the statement. While a Composition defines the structure, it does not actually contain the content: rather the full content of a document is contained in a Bundle, of which the Composition is the first resource contained. 151 */ 152 COMPOSITION, 153 /** 154 * A statement of relationships from one set of concepts to one or more other concepts - either code systems or data elements, or classes in class models. 155 */ 156 CONCEPTMAP, 157 /** 158 * A clinical condition, problem, diagnosis, or other event, situation, issue, or clinical concept that has risen to a level of concern. 159 */ 160 CONDITION, 161 /** 162 * A record of a healthcare consumer?s policy choices, which permits or denies identified recipient(s) or recipient role(s) to perform one or more actions within a given policy context, for specific purposes and periods of time. 163 */ 164 CONSENT, 165 /** 166 * A formal agreement between parties regarding the conduct of business, exchange of information or other matters. 167 */ 168 CONTRACT, 169 /** 170 * Financial instrument which may be used to reimburse or pay for health care products and services. 171 */ 172 COVERAGE, 173 /** 174 * The formal description of a single piece of information that can be gathered and reported. 175 */ 176 DATAELEMENT, 177 /** 178 * Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, Ineffective treatment frequency, Procedure-condition conflict, etc. 179 */ 180 DETECTEDISSUE, 181 /** 182 * This resource identifies an instance or a type of a manufactured item that is used in the provision of healthcare without being substantially changed through that activity. The device may be a medical or non-medical device. Medical devices include durable (reusable) medical equipment, implantable devices, as well as disposable equipment used for diagnostic, treatment, and research for healthcare and public health. Non-medical devices may include items such as a machine, cellphone, computer, application, etc. 183 */ 184 DEVICE, 185 /** 186 * The characteristics, operational status and capabilities of a medical-related component of a medical device. 187 */ 188 DEVICECOMPONENT, 189 /** 190 * Describes a measurement, calculation or setting capability of a medical device. 191 */ 192 DEVICEMETRIC, 193 /** 194 * Represents a request for a patient to employ a medical device. The device may be an implantable device, or an external assistive device, such as a walker. 195 */ 196 DEVICEREQUEST, 197 /** 198 * A record of a device being used by a patient where the record is the result of a report from the patient or another clinician. 199 */ 200 DEVICEUSESTATEMENT, 201 /** 202 * The findings and interpretation of diagnostic tests performed on patients, groups of patients, devices, and locations, and/or specimens derived from these. The report includes clinical context such as requesting and provider information, and some mix of atomic results, images, textual and coded interpretations, and formatted representation of diagnostic reports. 203 */ 204 DIAGNOSTICREPORT, 205 /** 206 * A collection of documents compiled for a purpose together with metadata that applies to the collection. 207 */ 208 DOCUMENTMANIFEST, 209 /** 210 * A reference to a document. 211 */ 212 DOCUMENTREFERENCE, 213 /** 214 * A resource that includes narrative, extensions, and contained resources. 215 */ 216 DOMAINRESOURCE, 217 /** 218 * The EligibilityRequest provides patient and insurance coverage information to an insurer for them to respond, in the form of an EligibilityResponse, with information regarding whether the stated coverage is valid and in-force and optionally to provide the insurance details of the policy. 219 */ 220 ELIGIBILITYREQUEST, 221 /** 222 * This resource provides eligibility and plan details from the processing of an Eligibility resource. 223 */ 224 ELIGIBILITYRESPONSE, 225 /** 226 * An interaction between a patient and healthcare provider(s) for the purpose of providing healthcare service(s) or assessing the health status of a patient. 227 */ 228 ENCOUNTER, 229 /** 230 * The technical details of an endpoint that can be used for electronic services, such as for web services providing XDS.b or a REST endpoint for another FHIR server. This may include any security context information. 231 */ 232 ENDPOINT, 233 /** 234 * This resource provides the insurance enrollment details to the insurer regarding a specified coverage. 235 */ 236 ENROLLMENTREQUEST, 237 /** 238 * This resource provides enrollment and plan details from the processing of an Enrollment resource. 239 */ 240 ENROLLMENTRESPONSE, 241 /** 242 * An association between a patient and an organization / healthcare provider(s) during which time encounters may occur. The managing organization assumes a level of responsibility for the patient during this time. 243 */ 244 EPISODEOFCARE, 245 /** 246 * Resource to define constraints on the Expansion of a FHIR ValueSet. 247 */ 248 EXPANSIONPROFILE, 249 /** 250 * This resource provides: the claim details; adjudication details from the processing of a Claim; and optionally account balance information, for informing the subscriber of the benefits provided. 251 */ 252 EXPLANATIONOFBENEFIT, 253 /** 254 * Significant health events and conditions for a person related to the patient relevant in the context of care for the patient. 255 */ 256 FAMILYMEMBERHISTORY, 257 /** 258 * Prospective warnings of potential issues when providing care to the patient. 259 */ 260 FLAG, 261 /** 262 * Describes the intended objective(s) for a patient, group or organization care, for example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc. 263 */ 264 GOAL, 265 /** 266 * A formal computable definition of a graph of resources - that is, a coherent set of resources that form a graph by following references. The Graph Definition resource defines a set and makes rules about the set. 267 */ 268 GRAPHDEFINITION, 269 /** 270 * Represents a defined collection of entities that may be discussed or acted upon collectively but which are not expected to act collectively and are not formally or legally recognized; i.e. a collection of entities that isn't an Organization. 271 */ 272 GROUP, 273 /** 274 * A guidance response is the formal response to a guidance request, including any output parameters returned by the evaluation, as well as the description of any proposed actions to be taken. 275 */ 276 GUIDANCERESPONSE, 277 /** 278 * The details of a healthcare service available at a location. 279 */ 280 HEALTHCARESERVICE, 281 /** 282 * A text description of the DICOM SOP instances selected in the ImagingManifest; or the reason for, or significance of, the selection. 283 */ 284 IMAGINGMANIFEST, 285 /** 286 * Representation of the content produced in a DICOM imaging study. A study comprises a set of series, each of which includes a set of Service-Object Pair Instances (SOP Instances - images or other data) acquired or produced in a common context. A series is of only one modality (e.g. X-ray, CT, MR, ultrasound), but a study may have multiple series of different modalities. 287 */ 288 IMAGINGSTUDY, 289 /** 290 * Describes the event of a patient being administered a vaccination or a record of a vaccination as reported by a patient, a clinician or another party and may include vaccine reaction information and what vaccination protocol was followed. 291 */ 292 IMMUNIZATION, 293 /** 294 * A patient's point-in-time immunization and recommendation (i.e. forecasting a patient's immunization eligibility according to a published schedule) with optional supporting justification. 295 */ 296 IMMUNIZATIONRECOMMENDATION, 297 /** 298 * A set of rules of how FHIR is used to solve a particular problem. This resource is used to gather all the parts of an implementation guide into a logical whole and to publish a computable definition of all the parts. 299 */ 300 IMPLEMENTATIONGUIDE, 301 /** 302 * The Library resource is a general-purpose container for knowledge asset definitions. It can be used to describe and expose existing knowledge assets such as logic libraries and information model descriptions, as well as to describe a collection of knowledge assets. 303 */ 304 LIBRARY, 305 /** 306 * Identifies two or more records (resource instances) that are referring to the same real-world "occurrence". 307 */ 308 LINKAGE, 309 /** 310 * A set of information summarized from a list of other resources. 311 */ 312 LIST, 313 /** 314 * Details and position information for a physical place where services are provided and resources and participants may be stored, found, contained or accommodated. 315 */ 316 LOCATION, 317 /** 318 * The Measure resource provides the definition of a quality measure. 319 */ 320 MEASURE, 321 /** 322 * The MeasureReport resource contains the results of evaluating a measure. 323 */ 324 MEASUREREPORT, 325 /** 326 * A photo, video, or audio recording acquired or used in healthcare. The actual content may be inline or provided by direct reference. 327 */ 328 MEDIA, 329 /** 330 * This resource is primarily used for the identification and definition of a medication. It covers the ingredients and the packaging for a medication. 331 */ 332 MEDICATION, 333 /** 334 * Describes the event of a patient consuming or otherwise being administered a medication. This may be as simple as swallowing a tablet or it may be a long running infusion. Related resources tie this event to the authorizing prescription, and the specific encounter between patient and health care practitioner. 335 */ 336 MEDICATIONADMINISTRATION, 337 /** 338 * Indicates that a medication product is to be or has been dispensed for a named person/patient. This includes a description of the medication product (supply) provided and the instructions for administering the medication. The medication dispense is the result of a pharmacy system responding to a medication order. 339 */ 340 MEDICATIONDISPENSE, 341 /** 342 * An order or request for both supply of the medication and the instructions for administration of the medication to a patient. The resource is called "MedicationRequest" rather than "MedicationPrescription" or "MedicationOrder" to generalize the use across inpatient and outpatient settings, including care plans, etc., and to harmonize with workflow patterns. 343 */ 344 MEDICATIONREQUEST, 345 /** 346 * A record of a medication that is being consumed by a patient. A MedicationStatement may indicate that the patient may be taking the medication now, or has taken the medication in the past or will be taking the medication in the future. The source of this information can be the patient, significant other (such as a family member or spouse), or a clinician. A common scenario where this information is captured is during the history taking process during a patient visit or stay. The medication information may come from sources such as the patient's memory, from a prescription bottle, or from a list of medications the patient, clinician or other party maintains 347 348The primary difference between a medication statement and a medication administration is that the medication administration has complete administration information and is based on actual administration information from the person who administered the medication. A medication statement is often, if not always, less specific. There is no required date/time when the medication was administered, in fact we only know that a source has reported the patient is taking this medication, where details such as time, quantity, or rate or even medication product may be incomplete or missing or less precise. As stated earlier, the medication statement information may come from the patient's memory, from a prescription bottle or from a list of medications the patient, clinician or other party maintains. Medication administration is more formal and is not missing detailed information. 349 */ 350 MEDICATIONSTATEMENT, 351 /** 352 * Defines the characteristics of a message that can be shared between systems, including the type of event that initiates the message, the content to be transmitted and what response(s), if any, are permitted. 353 */ 354 MESSAGEDEFINITION, 355 /** 356 * The header for a message exchange that is either requesting or responding to an action. The reference(s) that are the subject of the action as well as other information related to the action are typically transmitted in a bundle in which the MessageHeader resource instance is the first resource in the bundle. 357 */ 358 MESSAGEHEADER, 359 /** 360 * A curated namespace that issues unique symbols within that namespace for the identification of concepts, people, devices, etc. Represents a "System" used within the Identifier and Coding data types. 361 */ 362 NAMINGSYSTEM, 363 /** 364 * A request to supply a diet, formula feeding (enteral) or oral nutritional supplement to a patient/resident. 365 */ 366 NUTRITIONORDER, 367 /** 368 * Measurements and simple assertions made about a patient, device or other subject. 369 */ 370 OBSERVATION, 371 /** 372 * A formal computable definition of an operation (on the RESTful interface) or a named query (using the search interaction). 373 */ 374 OPERATIONDEFINITION, 375 /** 376 * A collection of error, warning or information messages that result from a system action. 377 */ 378 OPERATIONOUTCOME, 379 /** 380 * A formally or informally recognized grouping of people or organizations formed for the purpose of achieving some form of collective action. Includes companies, institutions, corporations, departments, community groups, healthcare practice groups, etc. 381 */ 382 ORGANIZATION, 383 /** 384 * This special resource type is used to represent an operation request and response (operations.html). It has no other use, and there is no RESTful endpoint associated with it. 385 */ 386 PARAMETERS, 387 /** 388 * Demographics and other administrative information about an individual or animal receiving care or other health-related services. 389 */ 390 PATIENT, 391 /** 392 * This resource provides the status of the payment for goods and services rendered, and the request and response resource references. 393 */ 394 PAYMENTNOTICE, 395 /** 396 * This resource provides payment details and claim references supporting a bulk payment. 397 */ 398 PAYMENTRECONCILIATION, 399 /** 400 * Demographics and administrative information about a person independent of a specific health-related context. 401 */ 402 PERSON, 403 /** 404 * This resource allows for the definition of various types of plans as a sharable, consumable, and executable artifact. The resource is general enough to support the description of a broad range of clinical artifacts such as clinical decision support rules, order sets and protocols. 405 */ 406 PLANDEFINITION, 407 /** 408 * A person who is directly or indirectly involved in the provisioning of healthcare. 409 */ 410 PRACTITIONER, 411 /** 412 * A specific set of Roles/Locations/specialties/services that a practitioner may perform at an organization for a period of time. 413 */ 414 PRACTITIONERROLE, 415 /** 416 * An action that is or was performed on a patient. This can be a physical intervention like an operation, or less invasive like counseling or hypnotherapy. 417 */ 418 PROCEDURE, 419 /** 420 * A record of a request for diagnostic investigations, treatments, or operations to be performed. 421 */ 422 PROCEDUREREQUEST, 423 /** 424 * This resource provides the target, request and response, and action details for an action to be performed by the target on or about existing resources. 425 */ 426 PROCESSREQUEST, 427 /** 428 * This resource provides processing status, errors and notes from the processing of a resource. 429 */ 430 PROCESSRESPONSE, 431 /** 432 * Provenance of a resource is a record that describes entities and processes involved in producing and delivering or otherwise influencing that resource. Provenance provides a critical foundation for assessing authenticity, enabling trust, and allowing reproducibility. Provenance assertions are a form of contextual metadata and can themselves become important records with their own provenance. Provenance statement indicates clinical significance in terms of confidence in authenticity, reliability, and trustworthiness, integrity, and stage in lifecycle (e.g. Document Completion - has the artifact been legally authenticated), all of which may impact security, privacy, and trust policies. 433 */ 434 PROVENANCE, 435 /** 436 * A structured set of questions intended to guide the collection of answers from end-users. Questionnaires provide detailed control over order, presentation, phraseology and grouping to allow coherent, consistent data collection. 437 */ 438 QUESTIONNAIRE, 439 /** 440 * A structured set of questions and their answers. The questions are ordered and grouped into coherent subsets, corresponding to the structure of the grouping of the questionnaire being responded to. 441 */ 442 QUESTIONNAIRERESPONSE, 443 /** 444 * Used to record and send details about a request for referral service or transfer of a patient to the care of another provider or provider organization. 445 */ 446 REFERRALREQUEST, 447 /** 448 * Information about a person that is involved in the care for a patient, but who is not the target of healthcare, nor has a formal responsibility in the care process. 449 */ 450 RELATEDPERSON, 451 /** 452 * A group of related requests that can be used to capture intended activities that have inter-dependencies such as "give this medication after that one". 453 */ 454 REQUESTGROUP, 455 /** 456 * A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects. 457 */ 458 RESEARCHSTUDY, 459 /** 460 * A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects. 461 */ 462 RESEARCHSUBJECT, 463 /** 464 * This is the base resource type for everything. 465 */ 466 RESOURCE, 467 /** 468 * An assessment of the likely outcome(s) for a patient or other subject as well as the likelihood of each outcome. 469 */ 470 RISKASSESSMENT, 471 /** 472 * A container for slots of time that may be available for booking appointments. 473 */ 474 SCHEDULE, 475 /** 476 * A search parameter that defines a named search item that can be used to search/filter on a resource. 477 */ 478 SEARCHPARAMETER, 479 /** 480 * Raw data describing a biological sequence. 481 */ 482 SEQUENCE, 483 /** 484 * The ServiceDefinition describes a unit of decision support functionality that is made available as a service, such as immunization modules or drug-drug interaction checking. 485 */ 486 SERVICEDEFINITION, 487 /** 488 * A slot of time on a schedule that may be available for booking appointments. 489 */ 490 SLOT, 491 /** 492 * A sample to be used for analysis. 493 */ 494 SPECIMEN, 495 /** 496 * A definition of a FHIR structure. This resource is used to describe the underlying resources, data types defined in FHIR, and also for describing extensions and constraints on resources and data types. 497 */ 498 STRUCTUREDEFINITION, 499 /** 500 * A Map of relationships between 2 structures that can be used to transform data. 501 */ 502 STRUCTUREMAP, 503 /** 504 * The subscription resource is used to define a push based subscription from a server to another system. Once a subscription is registered with the server, the server checks every resource that is created or updated, and if the resource matches the given criteria, it sends a message on the defined "channel" so that another system is able to take an appropriate action. 505 */ 506 SUBSCRIPTION, 507 /** 508 * A homogeneous material with a definite composition. 509 */ 510 SUBSTANCE, 511 /** 512 * Record of delivery of what is supplied. 513 */ 514 SUPPLYDELIVERY, 515 /** 516 * A record of a request for a medication, substance or device used in the healthcare setting. 517 */ 518 SUPPLYREQUEST, 519 /** 520 * A task to be performed. 521 */ 522 TASK, 523 /** 524 * A summary of information based on the results of executing a TestScript. 525 */ 526 TESTREPORT, 527 /** 528 * A structured set of tests against a FHIR server implementation to determine compliance against the FHIR specification. 529 */ 530 TESTSCRIPT, 531 /** 532 * A value set specifies a set of codes drawn from one or more code systems. 533 */ 534 VALUESET, 535 /** 536 * An authorization for the supply of glasses and/or contact lenses to a patient. 537 */ 538 VISIONPRESCRIPTION, 539 /** 540 * added to help the parsers with the generic types 541 */ 542 NULL; 543 public static ActivityDefinitionKind fromCode(String codeString) throws FHIRException { 544 if (codeString == null || "".equals(codeString)) 545 return null; 546 if ("Account".equals(codeString)) 547 return ACCOUNT; 548 if ("ActivityDefinition".equals(codeString)) 549 return ACTIVITYDEFINITION; 550 if ("AdverseEvent".equals(codeString)) 551 return ADVERSEEVENT; 552 if ("AllergyIntolerance".equals(codeString)) 553 return ALLERGYINTOLERANCE; 554 if ("Appointment".equals(codeString)) 555 return APPOINTMENT; 556 if ("AppointmentResponse".equals(codeString)) 557 return APPOINTMENTRESPONSE; 558 if ("AuditEvent".equals(codeString)) 559 return AUDITEVENT; 560 if ("Basic".equals(codeString)) 561 return BASIC; 562 if ("Binary".equals(codeString)) 563 return BINARY; 564 if ("BodySite".equals(codeString)) 565 return BODYSITE; 566 if ("Bundle".equals(codeString)) 567 return BUNDLE; 568 if ("CapabilityStatement".equals(codeString)) 569 return CAPABILITYSTATEMENT; 570 if ("CarePlan".equals(codeString)) 571 return CAREPLAN; 572 if ("CareTeam".equals(codeString)) 573 return CARETEAM; 574 if ("ChargeItem".equals(codeString)) 575 return CHARGEITEM; 576 if ("Claim".equals(codeString)) 577 return CLAIM; 578 if ("ClaimResponse".equals(codeString)) 579 return CLAIMRESPONSE; 580 if ("ClinicalImpression".equals(codeString)) 581 return CLINICALIMPRESSION; 582 if ("CodeSystem".equals(codeString)) 583 return CODESYSTEM; 584 if ("Communication".equals(codeString)) 585 return COMMUNICATION; 586 if ("CommunicationRequest".equals(codeString)) 587 return COMMUNICATIONREQUEST; 588 if ("CompartmentDefinition".equals(codeString)) 589 return COMPARTMENTDEFINITION; 590 if ("Composition".equals(codeString)) 591 return COMPOSITION; 592 if ("ConceptMap".equals(codeString)) 593 return CONCEPTMAP; 594 if ("Condition".equals(codeString)) 595 return CONDITION; 596 if ("Consent".equals(codeString)) 597 return CONSENT; 598 if ("Contract".equals(codeString)) 599 return CONTRACT; 600 if ("Coverage".equals(codeString)) 601 return COVERAGE; 602 if ("DataElement".equals(codeString)) 603 return DATAELEMENT; 604 if ("DetectedIssue".equals(codeString)) 605 return DETECTEDISSUE; 606 if ("Device".equals(codeString)) 607 return DEVICE; 608 if ("DeviceComponent".equals(codeString)) 609 return DEVICECOMPONENT; 610 if ("DeviceMetric".equals(codeString)) 611 return DEVICEMETRIC; 612 if ("DeviceRequest".equals(codeString)) 613 return DEVICEREQUEST; 614 if ("DeviceUseStatement".equals(codeString)) 615 return DEVICEUSESTATEMENT; 616 if ("DiagnosticReport".equals(codeString)) 617 return DIAGNOSTICREPORT; 618 if ("DocumentManifest".equals(codeString)) 619 return DOCUMENTMANIFEST; 620 if ("DocumentReference".equals(codeString)) 621 return DOCUMENTREFERENCE; 622 if ("DomainResource".equals(codeString)) 623 return DOMAINRESOURCE; 624 if ("EligibilityRequest".equals(codeString)) 625 return ELIGIBILITYREQUEST; 626 if ("EligibilityResponse".equals(codeString)) 627 return ELIGIBILITYRESPONSE; 628 if ("Encounter".equals(codeString)) 629 return ENCOUNTER; 630 if ("Endpoint".equals(codeString)) 631 return ENDPOINT; 632 if ("EnrollmentRequest".equals(codeString)) 633 return ENROLLMENTREQUEST; 634 if ("EnrollmentResponse".equals(codeString)) 635 return ENROLLMENTRESPONSE; 636 if ("EpisodeOfCare".equals(codeString)) 637 return EPISODEOFCARE; 638 if ("ExpansionProfile".equals(codeString)) 639 return EXPANSIONPROFILE; 640 if ("ExplanationOfBenefit".equals(codeString)) 641 return EXPLANATIONOFBENEFIT; 642 if ("FamilyMemberHistory".equals(codeString)) 643 return FAMILYMEMBERHISTORY; 644 if ("Flag".equals(codeString)) 645 return FLAG; 646 if ("Goal".equals(codeString)) 647 return GOAL; 648 if ("GraphDefinition".equals(codeString)) 649 return GRAPHDEFINITION; 650 if ("Group".equals(codeString)) 651 return GROUP; 652 if ("GuidanceResponse".equals(codeString)) 653 return GUIDANCERESPONSE; 654 if ("HealthcareService".equals(codeString)) 655 return HEALTHCARESERVICE; 656 if ("ImagingManifest".equals(codeString)) 657 return IMAGINGMANIFEST; 658 if ("ImagingStudy".equals(codeString)) 659 return IMAGINGSTUDY; 660 if ("Immunization".equals(codeString)) 661 return IMMUNIZATION; 662 if ("ImmunizationRecommendation".equals(codeString)) 663 return IMMUNIZATIONRECOMMENDATION; 664 if ("ImplementationGuide".equals(codeString)) 665 return IMPLEMENTATIONGUIDE; 666 if ("Library".equals(codeString)) 667 return LIBRARY; 668 if ("Linkage".equals(codeString)) 669 return LINKAGE; 670 if ("List".equals(codeString)) 671 return LIST; 672 if ("Location".equals(codeString)) 673 return LOCATION; 674 if ("Measure".equals(codeString)) 675 return MEASURE; 676 if ("MeasureReport".equals(codeString)) 677 return MEASUREREPORT; 678 if ("Media".equals(codeString)) 679 return MEDIA; 680 if ("Medication".equals(codeString)) 681 return MEDICATION; 682 if ("MedicationAdministration".equals(codeString)) 683 return MEDICATIONADMINISTRATION; 684 if ("MedicationDispense".equals(codeString)) 685 return MEDICATIONDISPENSE; 686 if ("MedicationRequest".equals(codeString)) 687 return MEDICATIONREQUEST; 688 if ("MedicationStatement".equals(codeString)) 689 return MEDICATIONSTATEMENT; 690 if ("MessageDefinition".equals(codeString)) 691 return MESSAGEDEFINITION; 692 if ("MessageHeader".equals(codeString)) 693 return MESSAGEHEADER; 694 if ("NamingSystem".equals(codeString)) 695 return NAMINGSYSTEM; 696 if ("NutritionOrder".equals(codeString)) 697 return NUTRITIONORDER; 698 if ("Observation".equals(codeString)) 699 return OBSERVATION; 700 if ("OperationDefinition".equals(codeString)) 701 return OPERATIONDEFINITION; 702 if ("OperationOutcome".equals(codeString)) 703 return OPERATIONOUTCOME; 704 if ("Organization".equals(codeString)) 705 return ORGANIZATION; 706 if ("Parameters".equals(codeString)) 707 return PARAMETERS; 708 if ("Patient".equals(codeString)) 709 return PATIENT; 710 if ("PaymentNotice".equals(codeString)) 711 return PAYMENTNOTICE; 712 if ("PaymentReconciliation".equals(codeString)) 713 return PAYMENTRECONCILIATION; 714 if ("Person".equals(codeString)) 715 return PERSON; 716 if ("PlanDefinition".equals(codeString)) 717 return PLANDEFINITION; 718 if ("Practitioner".equals(codeString)) 719 return PRACTITIONER; 720 if ("PractitionerRole".equals(codeString)) 721 return PRACTITIONERROLE; 722 if ("Procedure".equals(codeString)) 723 return PROCEDURE; 724 if ("ProcedureRequest".equals(codeString)) 725 return PROCEDUREREQUEST; 726 if ("ProcessRequest".equals(codeString)) 727 return PROCESSREQUEST; 728 if ("ProcessResponse".equals(codeString)) 729 return PROCESSRESPONSE; 730 if ("Provenance".equals(codeString)) 731 return PROVENANCE; 732 if ("Questionnaire".equals(codeString)) 733 return QUESTIONNAIRE; 734 if ("QuestionnaireResponse".equals(codeString)) 735 return QUESTIONNAIRERESPONSE; 736 if ("ReferralRequest".equals(codeString)) 737 return REFERRALREQUEST; 738 if ("RelatedPerson".equals(codeString)) 739 return RELATEDPERSON; 740 if ("RequestGroup".equals(codeString)) 741 return REQUESTGROUP; 742 if ("ResearchStudy".equals(codeString)) 743 return RESEARCHSTUDY; 744 if ("ResearchSubject".equals(codeString)) 745 return RESEARCHSUBJECT; 746 if ("Resource".equals(codeString)) 747 return RESOURCE; 748 if ("RiskAssessment".equals(codeString)) 749 return RISKASSESSMENT; 750 if ("Schedule".equals(codeString)) 751 return SCHEDULE; 752 if ("SearchParameter".equals(codeString)) 753 return SEARCHPARAMETER; 754 if ("Sequence".equals(codeString)) 755 return SEQUENCE; 756 if ("ServiceDefinition".equals(codeString)) 757 return SERVICEDEFINITION; 758 if ("Slot".equals(codeString)) 759 return SLOT; 760 if ("Specimen".equals(codeString)) 761 return SPECIMEN; 762 if ("StructureDefinition".equals(codeString)) 763 return STRUCTUREDEFINITION; 764 if ("StructureMap".equals(codeString)) 765 return STRUCTUREMAP; 766 if ("Subscription".equals(codeString)) 767 return SUBSCRIPTION; 768 if ("Substance".equals(codeString)) 769 return SUBSTANCE; 770 if ("SupplyDelivery".equals(codeString)) 771 return SUPPLYDELIVERY; 772 if ("SupplyRequest".equals(codeString)) 773 return SUPPLYREQUEST; 774 if ("Task".equals(codeString)) 775 return TASK; 776 if ("TestReport".equals(codeString)) 777 return TESTREPORT; 778 if ("TestScript".equals(codeString)) 779 return TESTSCRIPT; 780 if ("ValueSet".equals(codeString)) 781 return VALUESET; 782 if ("VisionPrescription".equals(codeString)) 783 return VISIONPRESCRIPTION; 784 if (Configuration.isAcceptInvalidEnums()) 785 return null; 786 else 787 throw new FHIRException("Unknown ActivityDefinitionKind code '"+codeString+"'"); 788 } 789 public String toCode() { 790 switch (this) { 791 case ACCOUNT: return "Account"; 792 case ACTIVITYDEFINITION: return "ActivityDefinition"; 793 case ADVERSEEVENT: return "AdverseEvent"; 794 case ALLERGYINTOLERANCE: return "AllergyIntolerance"; 795 case APPOINTMENT: return "Appointment"; 796 case APPOINTMENTRESPONSE: return "AppointmentResponse"; 797 case AUDITEVENT: return "AuditEvent"; 798 case BASIC: return "Basic"; 799 case BINARY: return "Binary"; 800 case BODYSITE: return "BodySite"; 801 case BUNDLE: return "Bundle"; 802 case CAPABILITYSTATEMENT: return "CapabilityStatement"; 803 case CAREPLAN: return "CarePlan"; 804 case CARETEAM: return "CareTeam"; 805 case CHARGEITEM: return "ChargeItem"; 806 case CLAIM: return "Claim"; 807 case CLAIMRESPONSE: return "ClaimResponse"; 808 case CLINICALIMPRESSION: return "ClinicalImpression"; 809 case CODESYSTEM: return "CodeSystem"; 810 case COMMUNICATION: return "Communication"; 811 case COMMUNICATIONREQUEST: return "CommunicationRequest"; 812 case COMPARTMENTDEFINITION: return "CompartmentDefinition"; 813 case COMPOSITION: return "Composition"; 814 case CONCEPTMAP: return "ConceptMap"; 815 case CONDITION: return "Condition"; 816 case CONSENT: return "Consent"; 817 case CONTRACT: return "Contract"; 818 case COVERAGE: return "Coverage"; 819 case DATAELEMENT: return "DataElement"; 820 case DETECTEDISSUE: return "DetectedIssue"; 821 case DEVICE: return "Device"; 822 case DEVICECOMPONENT: return "DeviceComponent"; 823 case DEVICEMETRIC: return "DeviceMetric"; 824 case DEVICEREQUEST: return "DeviceRequest"; 825 case DEVICEUSESTATEMENT: return "DeviceUseStatement"; 826 case DIAGNOSTICREPORT: return "DiagnosticReport"; 827 case DOCUMENTMANIFEST: return "DocumentManifest"; 828 case DOCUMENTREFERENCE: return "DocumentReference"; 829 case DOMAINRESOURCE: return "DomainResource"; 830 case ELIGIBILITYREQUEST: return "EligibilityRequest"; 831 case ELIGIBILITYRESPONSE: return "EligibilityResponse"; 832 case ENCOUNTER: return "Encounter"; 833 case ENDPOINT: return "Endpoint"; 834 case ENROLLMENTREQUEST: return "EnrollmentRequest"; 835 case ENROLLMENTRESPONSE: return "EnrollmentResponse"; 836 case EPISODEOFCARE: return "EpisodeOfCare"; 837 case EXPANSIONPROFILE: return "ExpansionProfile"; 838 case EXPLANATIONOFBENEFIT: return "ExplanationOfBenefit"; 839 case FAMILYMEMBERHISTORY: return "FamilyMemberHistory"; 840 case FLAG: return "Flag"; 841 case GOAL: return "Goal"; 842 case GRAPHDEFINITION: return "GraphDefinition"; 843 case GROUP: return "Group"; 844 case GUIDANCERESPONSE: return "GuidanceResponse"; 845 case HEALTHCARESERVICE: return "HealthcareService"; 846 case IMAGINGMANIFEST: return "ImagingManifest"; 847 case IMAGINGSTUDY: return "ImagingStudy"; 848 case IMMUNIZATION: return "Immunization"; 849 case IMMUNIZATIONRECOMMENDATION: return "ImmunizationRecommendation"; 850 case IMPLEMENTATIONGUIDE: return "ImplementationGuide"; 851 case LIBRARY: return "Library"; 852 case LINKAGE: return "Linkage"; 853 case LIST: return "List"; 854 case LOCATION: return "Location"; 855 case MEASURE: return "Measure"; 856 case MEASUREREPORT: return "MeasureReport"; 857 case MEDIA: return "Media"; 858 case MEDICATION: return "Medication"; 859 case MEDICATIONADMINISTRATION: return "MedicationAdministration"; 860 case MEDICATIONDISPENSE: return "MedicationDispense"; 861 case MEDICATIONREQUEST: return "MedicationRequest"; 862 case MEDICATIONSTATEMENT: return "MedicationStatement"; 863 case MESSAGEDEFINITION: return "MessageDefinition"; 864 case MESSAGEHEADER: return "MessageHeader"; 865 case NAMINGSYSTEM: return "NamingSystem"; 866 case NUTRITIONORDER: return "NutritionOrder"; 867 case OBSERVATION: return "Observation"; 868 case OPERATIONDEFINITION: return "OperationDefinition"; 869 case OPERATIONOUTCOME: return "OperationOutcome"; 870 case ORGANIZATION: return "Organization"; 871 case PARAMETERS: return "Parameters"; 872 case PATIENT: return "Patient"; 873 case PAYMENTNOTICE: return "PaymentNotice"; 874 case PAYMENTRECONCILIATION: return "PaymentReconciliation"; 875 case PERSON: return "Person"; 876 case PLANDEFINITION: return "PlanDefinition"; 877 case PRACTITIONER: return "Practitioner"; 878 case PRACTITIONERROLE: return "PractitionerRole"; 879 case PROCEDURE: return "Procedure"; 880 case PROCEDUREREQUEST: return "ProcedureRequest"; 881 case PROCESSREQUEST: return "ProcessRequest"; 882 case PROCESSRESPONSE: return "ProcessResponse"; 883 case PROVENANCE: return "Provenance"; 884 case QUESTIONNAIRE: return "Questionnaire"; 885 case QUESTIONNAIRERESPONSE: return "QuestionnaireResponse"; 886 case REFERRALREQUEST: return "ReferralRequest"; 887 case RELATEDPERSON: return "RelatedPerson"; 888 case REQUESTGROUP: return "RequestGroup"; 889 case RESEARCHSTUDY: return "ResearchStudy"; 890 case RESEARCHSUBJECT: return "ResearchSubject"; 891 case RESOURCE: return "Resource"; 892 case RISKASSESSMENT: return "RiskAssessment"; 893 case SCHEDULE: return "Schedule"; 894 case SEARCHPARAMETER: return "SearchParameter"; 895 case SEQUENCE: return "Sequence"; 896 case SERVICEDEFINITION: return "ServiceDefinition"; 897 case SLOT: return "Slot"; 898 case SPECIMEN: return "Specimen"; 899 case STRUCTUREDEFINITION: return "StructureDefinition"; 900 case STRUCTUREMAP: return "StructureMap"; 901 case SUBSCRIPTION: return "Subscription"; 902 case SUBSTANCE: return "Substance"; 903 case SUPPLYDELIVERY: return "SupplyDelivery"; 904 case SUPPLYREQUEST: return "SupplyRequest"; 905 case TASK: return "Task"; 906 case TESTREPORT: return "TestReport"; 907 case TESTSCRIPT: return "TestScript"; 908 case VALUESET: return "ValueSet"; 909 case VISIONPRESCRIPTION: return "VisionPrescription"; 910 case NULL: return null; 911 default: return "?"; 912 } 913 } 914 public String getSystem() { 915 switch (this) { 916 case ACCOUNT: return "http://hl7.org/fhir/resource-types"; 917 case ACTIVITYDEFINITION: return "http://hl7.org/fhir/resource-types"; 918 case ADVERSEEVENT: return "http://hl7.org/fhir/resource-types"; 919 case ALLERGYINTOLERANCE: return "http://hl7.org/fhir/resource-types"; 920 case APPOINTMENT: return "http://hl7.org/fhir/resource-types"; 921 case APPOINTMENTRESPONSE: return "http://hl7.org/fhir/resource-types"; 922 case AUDITEVENT: return "http://hl7.org/fhir/resource-types"; 923 case BASIC: return "http://hl7.org/fhir/resource-types"; 924 case BINARY: return "http://hl7.org/fhir/resource-types"; 925 case BODYSITE: return "http://hl7.org/fhir/resource-types"; 926 case BUNDLE: return "http://hl7.org/fhir/resource-types"; 927 case CAPABILITYSTATEMENT: return "http://hl7.org/fhir/resource-types"; 928 case CAREPLAN: return "http://hl7.org/fhir/resource-types"; 929 case CARETEAM: return "http://hl7.org/fhir/resource-types"; 930 case CHARGEITEM: return "http://hl7.org/fhir/resource-types"; 931 case CLAIM: return "http://hl7.org/fhir/resource-types"; 932 case CLAIMRESPONSE: return "http://hl7.org/fhir/resource-types"; 933 case CLINICALIMPRESSION: return "http://hl7.org/fhir/resource-types"; 934 case CODESYSTEM: return "http://hl7.org/fhir/resource-types"; 935 case COMMUNICATION: return "http://hl7.org/fhir/resource-types"; 936 case COMMUNICATIONREQUEST: return "http://hl7.org/fhir/resource-types"; 937 case COMPARTMENTDEFINITION: return "http://hl7.org/fhir/resource-types"; 938 case COMPOSITION: return "http://hl7.org/fhir/resource-types"; 939 case CONCEPTMAP: return "http://hl7.org/fhir/resource-types"; 940 case CONDITION: return "http://hl7.org/fhir/resource-types"; 941 case CONSENT: return "http://hl7.org/fhir/resource-types"; 942 case CONTRACT: return "http://hl7.org/fhir/resource-types"; 943 case COVERAGE: return "http://hl7.org/fhir/resource-types"; 944 case DATAELEMENT: return "http://hl7.org/fhir/resource-types"; 945 case DETECTEDISSUE: return "http://hl7.org/fhir/resource-types"; 946 case DEVICE: return "http://hl7.org/fhir/resource-types"; 947 case DEVICECOMPONENT: return "http://hl7.org/fhir/resource-types"; 948 case DEVICEMETRIC: return "http://hl7.org/fhir/resource-types"; 949 case DEVICEREQUEST: return "http://hl7.org/fhir/resource-types"; 950 case DEVICEUSESTATEMENT: return "http://hl7.org/fhir/resource-types"; 951 case DIAGNOSTICREPORT: return "http://hl7.org/fhir/resource-types"; 952 case DOCUMENTMANIFEST: return "http://hl7.org/fhir/resource-types"; 953 case DOCUMENTREFERENCE: return "http://hl7.org/fhir/resource-types"; 954 case DOMAINRESOURCE: return "http://hl7.org/fhir/resource-types"; 955 case ELIGIBILITYREQUEST: return "http://hl7.org/fhir/resource-types"; 956 case ELIGIBILITYRESPONSE: return "http://hl7.org/fhir/resource-types"; 957 case ENCOUNTER: return "http://hl7.org/fhir/resource-types"; 958 case ENDPOINT: return "http://hl7.org/fhir/resource-types"; 959 case ENROLLMENTREQUEST: return "http://hl7.org/fhir/resource-types"; 960 case ENROLLMENTRESPONSE: return "http://hl7.org/fhir/resource-types"; 961 case EPISODEOFCARE: return "http://hl7.org/fhir/resource-types"; 962 case EXPANSIONPROFILE: return "http://hl7.org/fhir/resource-types"; 963 case EXPLANATIONOFBENEFIT: return "http://hl7.org/fhir/resource-types"; 964 case FAMILYMEMBERHISTORY: return "http://hl7.org/fhir/resource-types"; 965 case FLAG: return "http://hl7.org/fhir/resource-types"; 966 case GOAL: return "http://hl7.org/fhir/resource-types"; 967 case GRAPHDEFINITION: return "http://hl7.org/fhir/resource-types"; 968 case GROUP: return "http://hl7.org/fhir/resource-types"; 969 case GUIDANCERESPONSE: return "http://hl7.org/fhir/resource-types"; 970 case HEALTHCARESERVICE: return "http://hl7.org/fhir/resource-types"; 971 case IMAGINGMANIFEST: return "http://hl7.org/fhir/resource-types"; 972 case IMAGINGSTUDY: return "http://hl7.org/fhir/resource-types"; 973 case IMMUNIZATION: return "http://hl7.org/fhir/resource-types"; 974 case IMMUNIZATIONRECOMMENDATION: return "http://hl7.org/fhir/resource-types"; 975 case IMPLEMENTATIONGUIDE: return "http://hl7.org/fhir/resource-types"; 976 case LIBRARY: return "http://hl7.org/fhir/resource-types"; 977 case LINKAGE: return "http://hl7.org/fhir/resource-types"; 978 case LIST: return "http://hl7.org/fhir/resource-types"; 979 case LOCATION: return "http://hl7.org/fhir/resource-types"; 980 case MEASURE: return "http://hl7.org/fhir/resource-types"; 981 case MEASUREREPORT: return "http://hl7.org/fhir/resource-types"; 982 case MEDIA: return "http://hl7.org/fhir/resource-types"; 983 case MEDICATION: return "http://hl7.org/fhir/resource-types"; 984 case MEDICATIONADMINISTRATION: return "http://hl7.org/fhir/resource-types"; 985 case MEDICATIONDISPENSE: return "http://hl7.org/fhir/resource-types"; 986 case MEDICATIONREQUEST: return "http://hl7.org/fhir/resource-types"; 987 case MEDICATIONSTATEMENT: return "http://hl7.org/fhir/resource-types"; 988 case MESSAGEDEFINITION: return "http://hl7.org/fhir/resource-types"; 989 case MESSAGEHEADER: return "http://hl7.org/fhir/resource-types"; 990 case NAMINGSYSTEM: return "http://hl7.org/fhir/resource-types"; 991 case NUTRITIONORDER: return "http://hl7.org/fhir/resource-types"; 992 case OBSERVATION: return "http://hl7.org/fhir/resource-types"; 993 case OPERATIONDEFINITION: return "http://hl7.org/fhir/resource-types"; 994 case OPERATIONOUTCOME: return "http://hl7.org/fhir/resource-types"; 995 case ORGANIZATION: return "http://hl7.org/fhir/resource-types"; 996 case PARAMETERS: return "http://hl7.org/fhir/resource-types"; 997 case PATIENT: return "http://hl7.org/fhir/resource-types"; 998 case PAYMENTNOTICE: return "http://hl7.org/fhir/resource-types"; 999 case PAYMENTRECONCILIATION: return "http://hl7.org/fhir/resource-types"; 1000 case PERSON: return "http://hl7.org/fhir/resource-types"; 1001 case PLANDEFINITION: return "http://hl7.org/fhir/resource-types"; 1002 case PRACTITIONER: return "http://hl7.org/fhir/resource-types"; 1003 case PRACTITIONERROLE: return "http://hl7.org/fhir/resource-types"; 1004 case PROCEDURE: return "http://hl7.org/fhir/resource-types"; 1005 case PROCEDUREREQUEST: return "http://hl7.org/fhir/resource-types"; 1006 case PROCESSREQUEST: return "http://hl7.org/fhir/resource-types"; 1007 case PROCESSRESPONSE: return "http://hl7.org/fhir/resource-types"; 1008 case PROVENANCE: return "http://hl7.org/fhir/resource-types"; 1009 case QUESTIONNAIRE: return "http://hl7.org/fhir/resource-types"; 1010 case QUESTIONNAIRERESPONSE: return "http://hl7.org/fhir/resource-types"; 1011 case REFERRALREQUEST: return "http://hl7.org/fhir/resource-types"; 1012 case RELATEDPERSON: return "http://hl7.org/fhir/resource-types"; 1013 case REQUESTGROUP: return "http://hl7.org/fhir/resource-types"; 1014 case RESEARCHSTUDY: return "http://hl7.org/fhir/resource-types"; 1015 case RESEARCHSUBJECT: return "http://hl7.org/fhir/resource-types"; 1016 case RESOURCE: return "http://hl7.org/fhir/resource-types"; 1017 case RISKASSESSMENT: return "http://hl7.org/fhir/resource-types"; 1018 case SCHEDULE: return "http://hl7.org/fhir/resource-types"; 1019 case SEARCHPARAMETER: return "http://hl7.org/fhir/resource-types"; 1020 case SEQUENCE: return "http://hl7.org/fhir/resource-types"; 1021 case SERVICEDEFINITION: return "http://hl7.org/fhir/resource-types"; 1022 case SLOT: return "http://hl7.org/fhir/resource-types"; 1023 case SPECIMEN: return "http://hl7.org/fhir/resource-types"; 1024 case STRUCTUREDEFINITION: return "http://hl7.org/fhir/resource-types"; 1025 case STRUCTUREMAP: return "http://hl7.org/fhir/resource-types"; 1026 case SUBSCRIPTION: return "http://hl7.org/fhir/resource-types"; 1027 case SUBSTANCE: return "http://hl7.org/fhir/resource-types"; 1028 case SUPPLYDELIVERY: return "http://hl7.org/fhir/resource-types"; 1029 case SUPPLYREQUEST: return "http://hl7.org/fhir/resource-types"; 1030 case TASK: return "http://hl7.org/fhir/resource-types"; 1031 case TESTREPORT: return "http://hl7.org/fhir/resource-types"; 1032 case TESTSCRIPT: return "http://hl7.org/fhir/resource-types"; 1033 case VALUESET: return "http://hl7.org/fhir/resource-types"; 1034 case VISIONPRESCRIPTION: return "http://hl7.org/fhir/resource-types"; 1035 case NULL: return null; 1036 default: return "?"; 1037 } 1038 } 1039 public String getDefinition() { 1040 switch (this) { 1041 case ACCOUNT: return "A financial tool for tracking value accrued for a particular purpose. In the healthcare field, used to track charges for a patient, cost centers, etc."; 1042 case ACTIVITYDEFINITION: return "This resource allows for the definition of some activity to be performed, independent of a particular patient, practitioner, or other performance context."; 1043 case ADVERSEEVENT: return "Actual or potential/avoided event causing unintended physical injury resulting from or contributed to by medical care, a research study or other healthcare setting factors that requires additional monitoring, treatment, or hospitalization, or that results in death."; 1044 case ALLERGYINTOLERANCE: return "Risk of harmful or undesirable, physiological response which is unique to an individual and associated with exposure to a substance."; 1045 case APPOINTMENT: return "A booking of a healthcare event among patient(s), practitioner(s), related person(s) and/or device(s) for a specific date/time. This may result in one or more Encounter(s)."; 1046 case APPOINTMENTRESPONSE: return "A reply to an appointment request for a patient and/or practitioner(s), such as a confirmation or rejection."; 1047 case AUDITEVENT: return "A record of an event made for purposes of maintaining a security log. Typical uses include detection of intrusion attempts and monitoring for inappropriate usage."; 1048 case BASIC: return "Basic is used for handling concepts not yet defined in FHIR, narrative-only resources that don't map to an existing resource, and custom resources not appropriate for inclusion in the FHIR specification."; 1049 case BINARY: return "A binary resource can contain any content, whether text, image, pdf, zip archive, etc."; 1050 case BODYSITE: return "Record details about the anatomical location of a specimen or body part. This resource may be used when a coded concept does not provide the necessary detail needed for the use case."; 1051 case BUNDLE: return "A container for a collection of resources."; 1052 case CAPABILITYSTATEMENT: return "A Capability Statement documents a set of capabilities (behaviors) of a FHIR Server that may be used as a statement of actual server functionality or a statement of required or desired server implementation."; 1053 case CAREPLAN: return "Describes the intention of how one or more practitioners intend to deliver care for a particular patient, group or community for a period of time, possibly limited to care for a specific condition or set of conditions."; 1054 case CARETEAM: return "The Care Team includes all the people and organizations who plan to participate in the coordination and delivery of care for a patient."; 1055 case CHARGEITEM: return "The resource ChargeItem describes the provision of healthcare provider products for a certain patient, therefore referring not only to the product, but containing in addition details of the provision, like date, time, amounts and participating organizations and persons. Main Usage of the ChargeItem is to enable the billing process and internal cost allocation."; 1056 case CLAIM: return "A provider issued list of services and products provided, or to be provided, to a patient which is provided to an insurer for payment recovery."; 1057 case CLAIMRESPONSE: return "This resource provides the adjudication details from the processing of a Claim resource."; 1058 case CLINICALIMPRESSION: return "A record of a clinical assessment performed to determine what problem(s) may affect the patient and before planning the treatments or management strategies that are best to manage a patient's condition. Assessments are often 1:1 with a clinical consultation / encounter, but this varies greatly depending on the clinical workflow. This resource is called \"ClinicalImpression\" rather than \"ClinicalAssessment\" to avoid confusion with the recording of assessment tools such as Apgar score."; 1059 case CODESYSTEM: return "A code system resource specifies a set of codes drawn from one or more code systems."; 1060 case COMMUNICATION: return "An occurrence of information being transmitted; e.g. an alert that was sent to a responsible provider, a public health agency was notified about a reportable condition."; 1061 case COMMUNICATIONREQUEST: return "A request to convey information; e.g. the CDS system proposes that an alert be sent to a responsible provider, the CDS system proposes that the public health agency be notified about a reportable condition."; 1062 case COMPARTMENTDEFINITION: return "A compartment definition that defines how resources are accessed on a server."; 1063 case COMPOSITION: return "A set of healthcare-related information that is assembled together into a single logical document that provides a single coherent statement of meaning, establishes its own context and that has clinical attestation with regard to who is making the statement. While a Composition defines the structure, it does not actually contain the content: rather the full content of a document is contained in a Bundle, of which the Composition is the first resource contained."; 1064 case CONCEPTMAP: return "A statement of relationships from one set of concepts to one or more other concepts - either code systems or data elements, or classes in class models."; 1065 case CONDITION: return "A clinical condition, problem, diagnosis, or other event, situation, issue, or clinical concept that has risen to a level of concern."; 1066 case CONSENT: return "A record of a healthcare consumer?s policy choices, which permits or denies identified recipient(s) or recipient role(s) to perform one or more actions within a given policy context, for specific purposes and periods of time."; 1067 case CONTRACT: return "A formal agreement between parties regarding the conduct of business, exchange of information or other matters."; 1068 case COVERAGE: return "Financial instrument which may be used to reimburse or pay for health care products and services."; 1069 case DATAELEMENT: return "The formal description of a single piece of information that can be gathered and reported."; 1070 case DETECTEDISSUE: return "Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, Ineffective treatment frequency, Procedure-condition conflict, etc."; 1071 case DEVICE: return "This resource identifies an instance or a type of a manufactured item that is used in the provision of healthcare without being substantially changed through that activity. The device may be a medical or non-medical device. Medical devices include durable (reusable) medical equipment, implantable devices, as well as disposable equipment used for diagnostic, treatment, and research for healthcare and public health. Non-medical devices may include items such as a machine, cellphone, computer, application, etc."; 1072 case DEVICECOMPONENT: return "The characteristics, operational status and capabilities of a medical-related component of a medical device."; 1073 case DEVICEMETRIC: return "Describes a measurement, calculation or setting capability of a medical device."; 1074 case DEVICEREQUEST: return "Represents a request for a patient to employ a medical device. The device may be an implantable device, or an external assistive device, such as a walker."; 1075 case DEVICEUSESTATEMENT: return "A record of a device being used by a patient where the record is the result of a report from the patient or another clinician."; 1076 case DIAGNOSTICREPORT: return "The findings and interpretation of diagnostic tests performed on patients, groups of patients, devices, and locations, and/or specimens derived from these. The report includes clinical context such as requesting and provider information, and some mix of atomic results, images, textual and coded interpretations, and formatted representation of diagnostic reports."; 1077 case DOCUMENTMANIFEST: return "A collection of documents compiled for a purpose together with metadata that applies to the collection."; 1078 case DOCUMENTREFERENCE: return "A reference to a document."; 1079 case DOMAINRESOURCE: return "A resource that includes narrative, extensions, and contained resources."; 1080 case ELIGIBILITYREQUEST: return "The EligibilityRequest provides patient and insurance coverage information to an insurer for them to respond, in the form of an EligibilityResponse, with information regarding whether the stated coverage is valid and in-force and optionally to provide the insurance details of the policy."; 1081 case ELIGIBILITYRESPONSE: return "This resource provides eligibility and plan details from the processing of an Eligibility resource."; 1082 case ENCOUNTER: return "An interaction between a patient and healthcare provider(s) for the purpose of providing healthcare service(s) or assessing the health status of a patient."; 1083 case ENDPOINT: return "The technical details of an endpoint that can be used for electronic services, such as for web services providing XDS.b or a REST endpoint for another FHIR server. This may include any security context information."; 1084 case ENROLLMENTREQUEST: return "This resource provides the insurance enrollment details to the insurer regarding a specified coverage."; 1085 case ENROLLMENTRESPONSE: return "This resource provides enrollment and plan details from the processing of an Enrollment resource."; 1086 case EPISODEOFCARE: return "An association between a patient and an organization / healthcare provider(s) during which time encounters may occur. The managing organization assumes a level of responsibility for the patient during this time."; 1087 case EXPANSIONPROFILE: return "Resource to define constraints on the Expansion of a FHIR ValueSet."; 1088 case EXPLANATIONOFBENEFIT: return "This resource provides: the claim details; adjudication details from the processing of a Claim; and optionally account balance information, for informing the subscriber of the benefits provided."; 1089 case FAMILYMEMBERHISTORY: return "Significant health events and conditions for a person related to the patient relevant in the context of care for the patient."; 1090 case FLAG: return "Prospective warnings of potential issues when providing care to the patient."; 1091 case GOAL: return "Describes the intended objective(s) for a patient, group or organization care, for example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc."; 1092 case GRAPHDEFINITION: return "A formal computable definition of a graph of resources - that is, a coherent set of resources that form a graph by following references. The Graph Definition resource defines a set and makes rules about the set."; 1093 case GROUP: return "Represents a defined collection of entities that may be discussed or acted upon collectively but which are not expected to act collectively and are not formally or legally recognized; i.e. a collection of entities that isn't an Organization."; 1094 case GUIDANCERESPONSE: return "A guidance response is the formal response to a guidance request, including any output parameters returned by the evaluation, as well as the description of any proposed actions to be taken."; 1095 case HEALTHCARESERVICE: return "The details of a healthcare service available at a location."; 1096 case IMAGINGMANIFEST: return "A text description of the DICOM SOP instances selected in the ImagingManifest; or the reason for, or significance of, the selection."; 1097 case IMAGINGSTUDY: return "Representation of the content produced in a DICOM imaging study. A study comprises a set of series, each of which includes a set of Service-Object Pair Instances (SOP Instances - images or other data) acquired or produced in a common context. A series is of only one modality (e.g. X-ray, CT, MR, ultrasound), but a study may have multiple series of different modalities."; 1098 case IMMUNIZATION: return "Describes the event of a patient being administered a vaccination or a record of a vaccination as reported by a patient, a clinician or another party and may include vaccine reaction information and what vaccination protocol was followed."; 1099 case IMMUNIZATIONRECOMMENDATION: return "A patient's point-in-time immunization and recommendation (i.e. forecasting a patient's immunization eligibility according to a published schedule) with optional supporting justification."; 1100 case IMPLEMENTATIONGUIDE: return "A set of rules of how FHIR is used to solve a particular problem. This resource is used to gather all the parts of an implementation guide into a logical whole and to publish a computable definition of all the parts."; 1101 case LIBRARY: return "The Library resource is a general-purpose container for knowledge asset definitions. It can be used to describe and expose existing knowledge assets such as logic libraries and information model descriptions, as well as to describe a collection of knowledge assets."; 1102 case LINKAGE: return "Identifies two or more records (resource instances) that are referring to the same real-world \"occurrence\"."; 1103 case LIST: return "A set of information summarized from a list of other resources."; 1104 case LOCATION: return "Details and position information for a physical place where services are provided and resources and participants may be stored, found, contained or accommodated."; 1105 case MEASURE: return "The Measure resource provides the definition of a quality measure."; 1106 case MEASUREREPORT: return "The MeasureReport resource contains the results of evaluating a measure."; 1107 case MEDIA: return "A photo, video, or audio recording acquired or used in healthcare. The actual content may be inline or provided by direct reference."; 1108 case MEDICATION: return "This resource is primarily used for the identification and definition of a medication. It covers the ingredients and the packaging for a medication."; 1109 case MEDICATIONADMINISTRATION: return "Describes the event of a patient consuming or otherwise being administered a medication. This may be as simple as swallowing a tablet or it may be a long running infusion. Related resources tie this event to the authorizing prescription, and the specific encounter between patient and health care practitioner."; 1110 case MEDICATIONDISPENSE: return "Indicates that a medication product is to be or has been dispensed for a named person/patient. This includes a description of the medication product (supply) provided and the instructions for administering the medication. The medication dispense is the result of a pharmacy system responding to a medication order."; 1111 case MEDICATIONREQUEST: return "An order or request for both supply of the medication and the instructions for administration of the medication to a patient. The resource is called \"MedicationRequest\" rather than \"MedicationPrescription\" or \"MedicationOrder\" to generalize the use across inpatient and outpatient settings, including care plans, etc., and to harmonize with workflow patterns."; 1112 case MEDICATIONSTATEMENT: return "A record of a medication that is being consumed by a patient. A MedicationStatement may indicate that the patient may be taking the medication now, or has taken the medication in the past or will be taking the medication in the future. The source of this information can be the patient, significant other (such as a family member or spouse), or a clinician. A common scenario where this information is captured is during the history taking process during a patient visit or stay. The medication information may come from sources such as the patient's memory, from a prescription bottle, or from a list of medications the patient, clinician or other party maintains \r\rThe primary difference between a medication statement and a medication administration is that the medication administration has complete administration information and is based on actual administration information from the person who administered the medication. A medication statement is often, if not always, less specific. There is no required date/time when the medication was administered, in fact we only know that a source has reported the patient is taking this medication, where details such as time, quantity, or rate or even medication product may be incomplete or missing or less precise. As stated earlier, the medication statement information may come from the patient's memory, from a prescription bottle or from a list of medications the patient, clinician or other party maintains. Medication administration is more formal and is not missing detailed information."; 1113 case MESSAGEDEFINITION: return "Defines the characteristics of a message that can be shared between systems, including the type of event that initiates the message, the content to be transmitted and what response(s), if any, are permitted."; 1114 case MESSAGEHEADER: return "The header for a message exchange that is either requesting or responding to an action. The reference(s) that are the subject of the action as well as other information related to the action are typically transmitted in a bundle in which the MessageHeader resource instance is the first resource in the bundle."; 1115 case NAMINGSYSTEM: return "A curated namespace that issues unique symbols within that namespace for the identification of concepts, people, devices, etc. Represents a \"System\" used within the Identifier and Coding data types."; 1116 case NUTRITIONORDER: return "A request to supply a diet, formula feeding (enteral) or oral nutritional supplement to a patient/resident."; 1117 case OBSERVATION: return "Measurements and simple assertions made about a patient, device or other subject."; 1118 case OPERATIONDEFINITION: return "A formal computable definition of an operation (on the RESTful interface) or a named query (using the search interaction)."; 1119 case OPERATIONOUTCOME: return "A collection of error, warning or information messages that result from a system action."; 1120 case ORGANIZATION: return "A formally or informally recognized grouping of people or organizations formed for the purpose of achieving some form of collective action. Includes companies, institutions, corporations, departments, community groups, healthcare practice groups, etc."; 1121 case PARAMETERS: return "This special resource type is used to represent an operation request and response (operations.html). It has no other use, and there is no RESTful endpoint associated with it."; 1122 case PATIENT: return "Demographics and other administrative information about an individual or animal receiving care or other health-related services."; 1123 case PAYMENTNOTICE: return "This resource provides the status of the payment for goods and services rendered, and the request and response resource references."; 1124 case PAYMENTRECONCILIATION: return "This resource provides payment details and claim references supporting a bulk payment."; 1125 case PERSON: return "Demographics and administrative information about a person independent of a specific health-related context."; 1126 case PLANDEFINITION: return "This resource allows for the definition of various types of plans as a sharable, consumable, and executable artifact. The resource is general enough to support the description of a broad range of clinical artifacts such as clinical decision support rules, order sets and protocols."; 1127 case PRACTITIONER: return "A person who is directly or indirectly involved in the provisioning of healthcare."; 1128 case PRACTITIONERROLE: return "A specific set of Roles/Locations/specialties/services that a practitioner may perform at an organization for a period of time."; 1129 case PROCEDURE: return "An action that is or was performed on a patient. This can be a physical intervention like an operation, or less invasive like counseling or hypnotherapy."; 1130 case PROCEDUREREQUEST: return "A record of a request for diagnostic investigations, treatments, or operations to be performed."; 1131 case PROCESSREQUEST: return "This resource provides the target, request and response, and action details for an action to be performed by the target on or about existing resources."; 1132 case PROCESSRESPONSE: return "This resource provides processing status, errors and notes from the processing of a resource."; 1133 case PROVENANCE: return "Provenance of a resource is a record that describes entities and processes involved in producing and delivering or otherwise influencing that resource. Provenance provides a critical foundation for assessing authenticity, enabling trust, and allowing reproducibility. Provenance assertions are a form of contextual metadata and can themselves become important records with their own provenance. Provenance statement indicates clinical significance in terms of confidence in authenticity, reliability, and trustworthiness, integrity, and stage in lifecycle (e.g. Document Completion - has the artifact been legally authenticated), all of which may impact security, privacy, and trust policies."; 1134 case QUESTIONNAIRE: return "A structured set of questions intended to guide the collection of answers from end-users. Questionnaires provide detailed control over order, presentation, phraseology and grouping to allow coherent, consistent data collection."; 1135 case QUESTIONNAIRERESPONSE: return "A structured set of questions and their answers. The questions are ordered and grouped into coherent subsets, corresponding to the structure of the grouping of the questionnaire being responded to."; 1136 case REFERRALREQUEST: return "Used to record and send details about a request for referral service or transfer of a patient to the care of another provider or provider organization."; 1137 case RELATEDPERSON: return "Information about a person that is involved in the care for a patient, but who is not the target of healthcare, nor has a formal responsibility in the care process."; 1138 case REQUESTGROUP: return "A group of related requests that can be used to capture intended activities that have inter-dependencies such as \"give this medication after that one\"."; 1139 case RESEARCHSTUDY: return "A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects."; 1140 case RESEARCHSUBJECT: return "A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects."; 1141 case RESOURCE: return "This is the base resource type for everything."; 1142 case RISKASSESSMENT: return "An assessment of the likely outcome(s) for a patient or other subject as well as the likelihood of each outcome."; 1143 case SCHEDULE: return "A container for slots of time that may be available for booking appointments."; 1144 case SEARCHPARAMETER: return "A search parameter that defines a named search item that can be used to search/filter on a resource."; 1145 case SEQUENCE: return "Raw data describing a biological sequence."; 1146 case SERVICEDEFINITION: return "The ServiceDefinition describes a unit of decision support functionality that is made available as a service, such as immunization modules or drug-drug interaction checking."; 1147 case SLOT: return "A slot of time on a schedule that may be available for booking appointments."; 1148 case SPECIMEN: return "A sample to be used for analysis."; 1149 case STRUCTUREDEFINITION: return "A definition of a FHIR structure. This resource is used to describe the underlying resources, data types defined in FHIR, and also for describing extensions and constraints on resources and data types."; 1150 case STRUCTUREMAP: return "A Map of relationships between 2 structures that can be used to transform data."; 1151 case SUBSCRIPTION: return "The subscription resource is used to define a push based subscription from a server to another system. Once a subscription is registered with the server, the server checks every resource that is created or updated, and if the resource matches the given criteria, it sends a message on the defined \"channel\" so that another system is able to take an appropriate action."; 1152 case SUBSTANCE: return "A homogeneous material with a definite composition."; 1153 case SUPPLYDELIVERY: return "Record of delivery of what is supplied."; 1154 case SUPPLYREQUEST: return "A record of a request for a medication, substance or device used in the healthcare setting."; 1155 case TASK: return "A task to be performed."; 1156 case TESTREPORT: return "A summary of information based on the results of executing a TestScript."; 1157 case TESTSCRIPT: return "A structured set of tests against a FHIR server implementation to determine compliance against the FHIR specification."; 1158 case VALUESET: return "A value set specifies a set of codes drawn from one or more code systems."; 1159 case VISIONPRESCRIPTION: return "An authorization for the supply of glasses and/or contact lenses to a patient."; 1160 case NULL: return null; 1161 default: return "?"; 1162 } 1163 } 1164 public String getDisplay() { 1165 switch (this) { 1166 case ACCOUNT: return "Account"; 1167 case ACTIVITYDEFINITION: return "ActivityDefinition"; 1168 case ADVERSEEVENT: return "AdverseEvent"; 1169 case ALLERGYINTOLERANCE: return "AllergyIntolerance"; 1170 case APPOINTMENT: return "Appointment"; 1171 case APPOINTMENTRESPONSE: return "AppointmentResponse"; 1172 case AUDITEVENT: return "AuditEvent"; 1173 case BASIC: return "Basic"; 1174 case BINARY: return "Binary"; 1175 case BODYSITE: return "BodySite"; 1176 case BUNDLE: return "Bundle"; 1177 case CAPABILITYSTATEMENT: return "CapabilityStatement"; 1178 case CAREPLAN: return "CarePlan"; 1179 case CARETEAM: return "CareTeam"; 1180 case CHARGEITEM: return "ChargeItem"; 1181 case CLAIM: return "Claim"; 1182 case CLAIMRESPONSE: return "ClaimResponse"; 1183 case CLINICALIMPRESSION: return "ClinicalImpression"; 1184 case CODESYSTEM: return "CodeSystem"; 1185 case COMMUNICATION: return "Communication"; 1186 case COMMUNICATIONREQUEST: return "CommunicationRequest"; 1187 case COMPARTMENTDEFINITION: return "CompartmentDefinition"; 1188 case COMPOSITION: return "Composition"; 1189 case CONCEPTMAP: return "ConceptMap"; 1190 case CONDITION: return "Condition"; 1191 case CONSENT: return "Consent"; 1192 case CONTRACT: return "Contract"; 1193 case COVERAGE: return "Coverage"; 1194 case DATAELEMENT: return "DataElement"; 1195 case DETECTEDISSUE: return "DetectedIssue"; 1196 case DEVICE: return "Device"; 1197 case DEVICECOMPONENT: return "DeviceComponent"; 1198 case DEVICEMETRIC: return "DeviceMetric"; 1199 case DEVICEREQUEST: return "DeviceRequest"; 1200 case DEVICEUSESTATEMENT: return "DeviceUseStatement"; 1201 case DIAGNOSTICREPORT: return "DiagnosticReport"; 1202 case DOCUMENTMANIFEST: return "DocumentManifest"; 1203 case DOCUMENTREFERENCE: return "DocumentReference"; 1204 case DOMAINRESOURCE: return "DomainResource"; 1205 case ELIGIBILITYREQUEST: return "EligibilityRequest"; 1206 case ELIGIBILITYRESPONSE: return "EligibilityResponse"; 1207 case ENCOUNTER: return "Encounter"; 1208 case ENDPOINT: return "Endpoint"; 1209 case ENROLLMENTREQUEST: return "EnrollmentRequest"; 1210 case ENROLLMENTRESPONSE: return "EnrollmentResponse"; 1211 case EPISODEOFCARE: return "EpisodeOfCare"; 1212 case EXPANSIONPROFILE: return "ExpansionProfile"; 1213 case EXPLANATIONOFBENEFIT: return "ExplanationOfBenefit"; 1214 case FAMILYMEMBERHISTORY: return "FamilyMemberHistory"; 1215 case FLAG: return "Flag"; 1216 case GOAL: return "Goal"; 1217 case GRAPHDEFINITION: return "GraphDefinition"; 1218 case GROUP: return "Group"; 1219 case GUIDANCERESPONSE: return "GuidanceResponse"; 1220 case HEALTHCARESERVICE: return "HealthcareService"; 1221 case IMAGINGMANIFEST: return "ImagingManifest"; 1222 case IMAGINGSTUDY: return "ImagingStudy"; 1223 case IMMUNIZATION: return "Immunization"; 1224 case IMMUNIZATIONRECOMMENDATION: return "ImmunizationRecommendation"; 1225 case IMPLEMENTATIONGUIDE: return "ImplementationGuide"; 1226 case LIBRARY: return "Library"; 1227 case LINKAGE: return "Linkage"; 1228 case LIST: return "List"; 1229 case LOCATION: return "Location"; 1230 case MEASURE: return "Measure"; 1231 case MEASUREREPORT: return "MeasureReport"; 1232 case MEDIA: return "Media"; 1233 case MEDICATION: return "Medication"; 1234 case MEDICATIONADMINISTRATION: return "MedicationAdministration"; 1235 case MEDICATIONDISPENSE: return "MedicationDispense"; 1236 case MEDICATIONREQUEST: return "MedicationRequest"; 1237 case MEDICATIONSTATEMENT: return "MedicationStatement"; 1238 case MESSAGEDEFINITION: return "MessageDefinition"; 1239 case MESSAGEHEADER: return "MessageHeader"; 1240 case NAMINGSYSTEM: return "NamingSystem"; 1241 case NUTRITIONORDER: return "NutritionOrder"; 1242 case OBSERVATION: return "Observation"; 1243 case OPERATIONDEFINITION: return "OperationDefinition"; 1244 case OPERATIONOUTCOME: return "OperationOutcome"; 1245 case ORGANIZATION: return "Organization"; 1246 case PARAMETERS: return "Parameters"; 1247 case PATIENT: return "Patient"; 1248 case PAYMENTNOTICE: return "PaymentNotice"; 1249 case PAYMENTRECONCILIATION: return "PaymentReconciliation"; 1250 case PERSON: return "Person"; 1251 case PLANDEFINITION: return "PlanDefinition"; 1252 case PRACTITIONER: return "Practitioner"; 1253 case PRACTITIONERROLE: return "PractitionerRole"; 1254 case PROCEDURE: return "Procedure"; 1255 case PROCEDUREREQUEST: return "ProcedureRequest"; 1256 case PROCESSREQUEST: return "ProcessRequest"; 1257 case PROCESSRESPONSE: return "ProcessResponse"; 1258 case PROVENANCE: return "Provenance"; 1259 case QUESTIONNAIRE: return "Questionnaire"; 1260 case QUESTIONNAIRERESPONSE: return "QuestionnaireResponse"; 1261 case REFERRALREQUEST: return "ReferralRequest"; 1262 case RELATEDPERSON: return "RelatedPerson"; 1263 case REQUESTGROUP: return "RequestGroup"; 1264 case RESEARCHSTUDY: return "ResearchStudy"; 1265 case RESEARCHSUBJECT: return "ResearchSubject"; 1266 case RESOURCE: return "Resource"; 1267 case RISKASSESSMENT: return "RiskAssessment"; 1268 case SCHEDULE: return "Schedule"; 1269 case SEARCHPARAMETER: return "SearchParameter"; 1270 case SEQUENCE: return "Sequence"; 1271 case SERVICEDEFINITION: return "ServiceDefinition"; 1272 case SLOT: return "Slot"; 1273 case SPECIMEN: return "Specimen"; 1274 case STRUCTUREDEFINITION: return "StructureDefinition"; 1275 case STRUCTUREMAP: return "StructureMap"; 1276 case SUBSCRIPTION: return "Subscription"; 1277 case SUBSTANCE: return "Substance"; 1278 case SUPPLYDELIVERY: return "SupplyDelivery"; 1279 case SUPPLYREQUEST: return "SupplyRequest"; 1280 case TASK: return "Task"; 1281 case TESTREPORT: return "TestReport"; 1282 case TESTSCRIPT: return "TestScript"; 1283 case VALUESET: return "ValueSet"; 1284 case VISIONPRESCRIPTION: return "VisionPrescription"; 1285 case NULL: return null; 1286 default: return "?"; 1287 } 1288 } 1289 } 1290 1291 public static class ActivityDefinitionKindEnumFactory implements EnumFactory<ActivityDefinitionKind> { 1292 public ActivityDefinitionKind fromCode(String codeString) throws IllegalArgumentException { 1293 if (codeString == null || "".equals(codeString)) 1294 if (codeString == null || "".equals(codeString)) 1295 return null; 1296 if ("Account".equals(codeString)) 1297 return ActivityDefinitionKind.ACCOUNT; 1298 if ("ActivityDefinition".equals(codeString)) 1299 return ActivityDefinitionKind.ACTIVITYDEFINITION; 1300 if ("AdverseEvent".equals(codeString)) 1301 return ActivityDefinitionKind.ADVERSEEVENT; 1302 if ("AllergyIntolerance".equals(codeString)) 1303 return ActivityDefinitionKind.ALLERGYINTOLERANCE; 1304 if ("Appointment".equals(codeString)) 1305 return ActivityDefinitionKind.APPOINTMENT; 1306 if ("AppointmentResponse".equals(codeString)) 1307 return ActivityDefinitionKind.APPOINTMENTRESPONSE; 1308 if ("AuditEvent".equals(codeString)) 1309 return ActivityDefinitionKind.AUDITEVENT; 1310 if ("Basic".equals(codeString)) 1311 return ActivityDefinitionKind.BASIC; 1312 if ("Binary".equals(codeString)) 1313 return ActivityDefinitionKind.BINARY; 1314 if ("BodySite".equals(codeString)) 1315 return ActivityDefinitionKind.BODYSITE; 1316 if ("Bundle".equals(codeString)) 1317 return ActivityDefinitionKind.BUNDLE; 1318 if ("CapabilityStatement".equals(codeString)) 1319 return ActivityDefinitionKind.CAPABILITYSTATEMENT; 1320 if ("CarePlan".equals(codeString)) 1321 return ActivityDefinitionKind.CAREPLAN; 1322 if ("CareTeam".equals(codeString)) 1323 return ActivityDefinitionKind.CARETEAM; 1324 if ("ChargeItem".equals(codeString)) 1325 return ActivityDefinitionKind.CHARGEITEM; 1326 if ("Claim".equals(codeString)) 1327 return ActivityDefinitionKind.CLAIM; 1328 if ("ClaimResponse".equals(codeString)) 1329 return ActivityDefinitionKind.CLAIMRESPONSE; 1330 if ("ClinicalImpression".equals(codeString)) 1331 return ActivityDefinitionKind.CLINICALIMPRESSION; 1332 if ("CodeSystem".equals(codeString)) 1333 return ActivityDefinitionKind.CODESYSTEM; 1334 if ("Communication".equals(codeString)) 1335 return ActivityDefinitionKind.COMMUNICATION; 1336 if ("CommunicationRequest".equals(codeString)) 1337 return ActivityDefinitionKind.COMMUNICATIONREQUEST; 1338 if ("CompartmentDefinition".equals(codeString)) 1339 return ActivityDefinitionKind.COMPARTMENTDEFINITION; 1340 if ("Composition".equals(codeString)) 1341 return ActivityDefinitionKind.COMPOSITION; 1342 if ("ConceptMap".equals(codeString)) 1343 return ActivityDefinitionKind.CONCEPTMAP; 1344 if ("Condition".equals(codeString)) 1345 return ActivityDefinitionKind.CONDITION; 1346 if ("Consent".equals(codeString)) 1347 return ActivityDefinitionKind.CONSENT; 1348 if ("Contract".equals(codeString)) 1349 return ActivityDefinitionKind.CONTRACT; 1350 if ("Coverage".equals(codeString)) 1351 return ActivityDefinitionKind.COVERAGE; 1352 if ("DataElement".equals(codeString)) 1353 return ActivityDefinitionKind.DATAELEMENT; 1354 if ("DetectedIssue".equals(codeString)) 1355 return ActivityDefinitionKind.DETECTEDISSUE; 1356 if ("Device".equals(codeString)) 1357 return ActivityDefinitionKind.DEVICE; 1358 if ("DeviceComponent".equals(codeString)) 1359 return ActivityDefinitionKind.DEVICECOMPONENT; 1360 if ("DeviceMetric".equals(codeString)) 1361 return ActivityDefinitionKind.DEVICEMETRIC; 1362 if ("DeviceRequest".equals(codeString)) 1363 return ActivityDefinitionKind.DEVICEREQUEST; 1364 if ("DeviceUseStatement".equals(codeString)) 1365 return ActivityDefinitionKind.DEVICEUSESTATEMENT; 1366 if ("DiagnosticReport".equals(codeString)) 1367 return ActivityDefinitionKind.DIAGNOSTICREPORT; 1368 if ("DocumentManifest".equals(codeString)) 1369 return ActivityDefinitionKind.DOCUMENTMANIFEST; 1370 if ("DocumentReference".equals(codeString)) 1371 return ActivityDefinitionKind.DOCUMENTREFERENCE; 1372 if ("DomainResource".equals(codeString)) 1373 return ActivityDefinitionKind.DOMAINRESOURCE; 1374 if ("EligibilityRequest".equals(codeString)) 1375 return ActivityDefinitionKind.ELIGIBILITYREQUEST; 1376 if ("EligibilityResponse".equals(codeString)) 1377 return ActivityDefinitionKind.ELIGIBILITYRESPONSE; 1378 if ("Encounter".equals(codeString)) 1379 return ActivityDefinitionKind.ENCOUNTER; 1380 if ("Endpoint".equals(codeString)) 1381 return ActivityDefinitionKind.ENDPOINT; 1382 if ("EnrollmentRequest".equals(codeString)) 1383 return ActivityDefinitionKind.ENROLLMENTREQUEST; 1384 if ("EnrollmentResponse".equals(codeString)) 1385 return ActivityDefinitionKind.ENROLLMENTRESPONSE; 1386 if ("EpisodeOfCare".equals(codeString)) 1387 return ActivityDefinitionKind.EPISODEOFCARE; 1388 if ("ExpansionProfile".equals(codeString)) 1389 return ActivityDefinitionKind.EXPANSIONPROFILE; 1390 if ("ExplanationOfBenefit".equals(codeString)) 1391 return ActivityDefinitionKind.EXPLANATIONOFBENEFIT; 1392 if ("FamilyMemberHistory".equals(codeString)) 1393 return ActivityDefinitionKind.FAMILYMEMBERHISTORY; 1394 if ("Flag".equals(codeString)) 1395 return ActivityDefinitionKind.FLAG; 1396 if ("Goal".equals(codeString)) 1397 return ActivityDefinitionKind.GOAL; 1398 if ("GraphDefinition".equals(codeString)) 1399 return ActivityDefinitionKind.GRAPHDEFINITION; 1400 if ("Group".equals(codeString)) 1401 return ActivityDefinitionKind.GROUP; 1402 if ("GuidanceResponse".equals(codeString)) 1403 return ActivityDefinitionKind.GUIDANCERESPONSE; 1404 if ("HealthcareService".equals(codeString)) 1405 return ActivityDefinitionKind.HEALTHCARESERVICE; 1406 if ("ImagingManifest".equals(codeString)) 1407 return ActivityDefinitionKind.IMAGINGMANIFEST; 1408 if ("ImagingStudy".equals(codeString)) 1409 return ActivityDefinitionKind.IMAGINGSTUDY; 1410 if ("Immunization".equals(codeString)) 1411 return ActivityDefinitionKind.IMMUNIZATION; 1412 if ("ImmunizationRecommendation".equals(codeString)) 1413 return ActivityDefinitionKind.IMMUNIZATIONRECOMMENDATION; 1414 if ("ImplementationGuide".equals(codeString)) 1415 return ActivityDefinitionKind.IMPLEMENTATIONGUIDE; 1416 if ("Library".equals(codeString)) 1417 return ActivityDefinitionKind.LIBRARY; 1418 if ("Linkage".equals(codeString)) 1419 return ActivityDefinitionKind.LINKAGE; 1420 if ("List".equals(codeString)) 1421 return ActivityDefinitionKind.LIST; 1422 if ("Location".equals(codeString)) 1423 return ActivityDefinitionKind.LOCATION; 1424 if ("Measure".equals(codeString)) 1425 return ActivityDefinitionKind.MEASURE; 1426 if ("MeasureReport".equals(codeString)) 1427 return ActivityDefinitionKind.MEASUREREPORT; 1428 if ("Media".equals(codeString)) 1429 return ActivityDefinitionKind.MEDIA; 1430 if ("Medication".equals(codeString)) 1431 return ActivityDefinitionKind.MEDICATION; 1432 if ("MedicationAdministration".equals(codeString)) 1433 return ActivityDefinitionKind.MEDICATIONADMINISTRATION; 1434 if ("MedicationDispense".equals(codeString)) 1435 return ActivityDefinitionKind.MEDICATIONDISPENSE; 1436 if ("MedicationRequest".equals(codeString)) 1437 return ActivityDefinitionKind.MEDICATIONREQUEST; 1438 if ("MedicationStatement".equals(codeString)) 1439 return ActivityDefinitionKind.MEDICATIONSTATEMENT; 1440 if ("MessageDefinition".equals(codeString)) 1441 return ActivityDefinitionKind.MESSAGEDEFINITION; 1442 if ("MessageHeader".equals(codeString)) 1443 return ActivityDefinitionKind.MESSAGEHEADER; 1444 if ("NamingSystem".equals(codeString)) 1445 return ActivityDefinitionKind.NAMINGSYSTEM; 1446 if ("NutritionOrder".equals(codeString)) 1447 return ActivityDefinitionKind.NUTRITIONORDER; 1448 if ("Observation".equals(codeString)) 1449 return ActivityDefinitionKind.OBSERVATION; 1450 if ("OperationDefinition".equals(codeString)) 1451 return ActivityDefinitionKind.OPERATIONDEFINITION; 1452 if ("OperationOutcome".equals(codeString)) 1453 return ActivityDefinitionKind.OPERATIONOUTCOME; 1454 if ("Organization".equals(codeString)) 1455 return ActivityDefinitionKind.ORGANIZATION; 1456 if ("Parameters".equals(codeString)) 1457 return ActivityDefinitionKind.PARAMETERS; 1458 if ("Patient".equals(codeString)) 1459 return ActivityDefinitionKind.PATIENT; 1460 if ("PaymentNotice".equals(codeString)) 1461 return ActivityDefinitionKind.PAYMENTNOTICE; 1462 if ("PaymentReconciliation".equals(codeString)) 1463 return ActivityDefinitionKind.PAYMENTRECONCILIATION; 1464 if ("Person".equals(codeString)) 1465 return ActivityDefinitionKind.PERSON; 1466 if ("PlanDefinition".equals(codeString)) 1467 return ActivityDefinitionKind.PLANDEFINITION; 1468 if ("Practitioner".equals(codeString)) 1469 return ActivityDefinitionKind.PRACTITIONER; 1470 if ("PractitionerRole".equals(codeString)) 1471 return ActivityDefinitionKind.PRACTITIONERROLE; 1472 if ("Procedure".equals(codeString)) 1473 return ActivityDefinitionKind.PROCEDURE; 1474 if ("ProcedureRequest".equals(codeString)) 1475 return ActivityDefinitionKind.PROCEDUREREQUEST; 1476 if ("ProcessRequest".equals(codeString)) 1477 return ActivityDefinitionKind.PROCESSREQUEST; 1478 if ("ProcessResponse".equals(codeString)) 1479 return ActivityDefinitionKind.PROCESSRESPONSE; 1480 if ("Provenance".equals(codeString)) 1481 return ActivityDefinitionKind.PROVENANCE; 1482 if ("Questionnaire".equals(codeString)) 1483 return ActivityDefinitionKind.QUESTIONNAIRE; 1484 if ("QuestionnaireResponse".equals(codeString)) 1485 return ActivityDefinitionKind.QUESTIONNAIRERESPONSE; 1486 if ("ReferralRequest".equals(codeString)) 1487 return ActivityDefinitionKind.REFERRALREQUEST; 1488 if ("RelatedPerson".equals(codeString)) 1489 return ActivityDefinitionKind.RELATEDPERSON; 1490 if ("RequestGroup".equals(codeString)) 1491 return ActivityDefinitionKind.REQUESTGROUP; 1492 if ("ResearchStudy".equals(codeString)) 1493 return ActivityDefinitionKind.RESEARCHSTUDY; 1494 if ("ResearchSubject".equals(codeString)) 1495 return ActivityDefinitionKind.RESEARCHSUBJECT; 1496 if ("Resource".equals(codeString)) 1497 return ActivityDefinitionKind.RESOURCE; 1498 if ("RiskAssessment".equals(codeString)) 1499 return ActivityDefinitionKind.RISKASSESSMENT; 1500 if ("Schedule".equals(codeString)) 1501 return ActivityDefinitionKind.SCHEDULE; 1502 if ("SearchParameter".equals(codeString)) 1503 return ActivityDefinitionKind.SEARCHPARAMETER; 1504 if ("Sequence".equals(codeString)) 1505 return ActivityDefinitionKind.SEQUENCE; 1506 if ("ServiceDefinition".equals(codeString)) 1507 return ActivityDefinitionKind.SERVICEDEFINITION; 1508 if ("Slot".equals(codeString)) 1509 return ActivityDefinitionKind.SLOT; 1510 if ("Specimen".equals(codeString)) 1511 return ActivityDefinitionKind.SPECIMEN; 1512 if ("StructureDefinition".equals(codeString)) 1513 return ActivityDefinitionKind.STRUCTUREDEFINITION; 1514 if ("StructureMap".equals(codeString)) 1515 return ActivityDefinitionKind.STRUCTUREMAP; 1516 if ("Subscription".equals(codeString)) 1517 return ActivityDefinitionKind.SUBSCRIPTION; 1518 if ("Substance".equals(codeString)) 1519 return ActivityDefinitionKind.SUBSTANCE; 1520 if ("SupplyDelivery".equals(codeString)) 1521 return ActivityDefinitionKind.SUPPLYDELIVERY; 1522 if ("SupplyRequest".equals(codeString)) 1523 return ActivityDefinitionKind.SUPPLYREQUEST; 1524 if ("Task".equals(codeString)) 1525 return ActivityDefinitionKind.TASK; 1526 if ("TestReport".equals(codeString)) 1527 return ActivityDefinitionKind.TESTREPORT; 1528 if ("TestScript".equals(codeString)) 1529 return ActivityDefinitionKind.TESTSCRIPT; 1530 if ("ValueSet".equals(codeString)) 1531 return ActivityDefinitionKind.VALUESET; 1532 if ("VisionPrescription".equals(codeString)) 1533 return ActivityDefinitionKind.VISIONPRESCRIPTION; 1534 throw new IllegalArgumentException("Unknown ActivityDefinitionKind code '"+codeString+"'"); 1535 } 1536 public Enumeration<ActivityDefinitionKind> fromType(PrimitiveType<?> code) throws FHIRException { 1537 if (code == null) 1538 return null; 1539 if (code.isEmpty()) 1540 return new Enumeration<ActivityDefinitionKind>(this); 1541 String codeString = code.asStringValue(); 1542 if (codeString == null || "".equals(codeString)) 1543 return null; 1544 if ("Account".equals(codeString)) 1545 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.ACCOUNT); 1546 if ("ActivityDefinition".equals(codeString)) 1547 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.ACTIVITYDEFINITION); 1548 if ("AdverseEvent".equals(codeString)) 1549 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.ADVERSEEVENT); 1550 if ("AllergyIntolerance".equals(codeString)) 1551 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.ALLERGYINTOLERANCE); 1552 if ("Appointment".equals(codeString)) 1553 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.APPOINTMENT); 1554 if ("AppointmentResponse".equals(codeString)) 1555 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.APPOINTMENTRESPONSE); 1556 if ("AuditEvent".equals(codeString)) 1557 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.AUDITEVENT); 1558 if ("Basic".equals(codeString)) 1559 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.BASIC); 1560 if ("Binary".equals(codeString)) 1561 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.BINARY); 1562 if ("BodySite".equals(codeString)) 1563 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.BODYSITE); 1564 if ("Bundle".equals(codeString)) 1565 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.BUNDLE); 1566 if ("CapabilityStatement".equals(codeString)) 1567 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.CAPABILITYSTATEMENT); 1568 if ("CarePlan".equals(codeString)) 1569 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.CAREPLAN); 1570 if ("CareTeam".equals(codeString)) 1571 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.CARETEAM); 1572 if ("ChargeItem".equals(codeString)) 1573 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.CHARGEITEM); 1574 if ("Claim".equals(codeString)) 1575 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.CLAIM); 1576 if ("ClaimResponse".equals(codeString)) 1577 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.CLAIMRESPONSE); 1578 if ("ClinicalImpression".equals(codeString)) 1579 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.CLINICALIMPRESSION); 1580 if ("CodeSystem".equals(codeString)) 1581 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.CODESYSTEM); 1582 if ("Communication".equals(codeString)) 1583 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.COMMUNICATION); 1584 if ("CommunicationRequest".equals(codeString)) 1585 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.COMMUNICATIONREQUEST); 1586 if ("CompartmentDefinition".equals(codeString)) 1587 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.COMPARTMENTDEFINITION); 1588 if ("Composition".equals(codeString)) 1589 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.COMPOSITION); 1590 if ("ConceptMap".equals(codeString)) 1591 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.CONCEPTMAP); 1592 if ("Condition".equals(codeString)) 1593 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.CONDITION); 1594 if ("Consent".equals(codeString)) 1595 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.CONSENT); 1596 if ("Contract".equals(codeString)) 1597 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.CONTRACT); 1598 if ("Coverage".equals(codeString)) 1599 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.COVERAGE); 1600 if ("DataElement".equals(codeString)) 1601 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.DATAELEMENT); 1602 if ("DetectedIssue".equals(codeString)) 1603 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.DETECTEDISSUE); 1604 if ("Device".equals(codeString)) 1605 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.DEVICE); 1606 if ("DeviceComponent".equals(codeString)) 1607 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.DEVICECOMPONENT); 1608 if ("DeviceMetric".equals(codeString)) 1609 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.DEVICEMETRIC); 1610 if ("DeviceRequest".equals(codeString)) 1611 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.DEVICEREQUEST); 1612 if ("DeviceUseStatement".equals(codeString)) 1613 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.DEVICEUSESTATEMENT); 1614 if ("DiagnosticReport".equals(codeString)) 1615 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.DIAGNOSTICREPORT); 1616 if ("DocumentManifest".equals(codeString)) 1617 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.DOCUMENTMANIFEST); 1618 if ("DocumentReference".equals(codeString)) 1619 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.DOCUMENTREFERENCE); 1620 if ("DomainResource".equals(codeString)) 1621 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.DOMAINRESOURCE); 1622 if ("EligibilityRequest".equals(codeString)) 1623 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.ELIGIBILITYREQUEST); 1624 if ("EligibilityResponse".equals(codeString)) 1625 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.ELIGIBILITYRESPONSE); 1626 if ("Encounter".equals(codeString)) 1627 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.ENCOUNTER); 1628 if ("Endpoint".equals(codeString)) 1629 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.ENDPOINT); 1630 if ("EnrollmentRequest".equals(codeString)) 1631 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.ENROLLMENTREQUEST); 1632 if ("EnrollmentResponse".equals(codeString)) 1633 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.ENROLLMENTRESPONSE); 1634 if ("EpisodeOfCare".equals(codeString)) 1635 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.EPISODEOFCARE); 1636 if ("ExpansionProfile".equals(codeString)) 1637 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.EXPANSIONPROFILE); 1638 if ("ExplanationOfBenefit".equals(codeString)) 1639 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.EXPLANATIONOFBENEFIT); 1640 if ("FamilyMemberHistory".equals(codeString)) 1641 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.FAMILYMEMBERHISTORY); 1642 if ("Flag".equals(codeString)) 1643 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.FLAG); 1644 if ("Goal".equals(codeString)) 1645 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.GOAL); 1646 if ("GraphDefinition".equals(codeString)) 1647 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.GRAPHDEFINITION); 1648 if ("Group".equals(codeString)) 1649 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.GROUP); 1650 if ("GuidanceResponse".equals(codeString)) 1651 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.GUIDANCERESPONSE); 1652 if ("HealthcareService".equals(codeString)) 1653 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.HEALTHCARESERVICE); 1654 if ("ImagingManifest".equals(codeString)) 1655 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.IMAGINGMANIFEST); 1656 if ("ImagingStudy".equals(codeString)) 1657 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.IMAGINGSTUDY); 1658 if ("Immunization".equals(codeString)) 1659 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.IMMUNIZATION); 1660 if ("ImmunizationRecommendation".equals(codeString)) 1661 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.IMMUNIZATIONRECOMMENDATION); 1662 if ("ImplementationGuide".equals(codeString)) 1663 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.IMPLEMENTATIONGUIDE); 1664 if ("Library".equals(codeString)) 1665 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.LIBRARY); 1666 if ("Linkage".equals(codeString)) 1667 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.LINKAGE); 1668 if ("List".equals(codeString)) 1669 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.LIST); 1670 if ("Location".equals(codeString)) 1671 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.LOCATION); 1672 if ("Measure".equals(codeString)) 1673 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.MEASURE); 1674 if ("MeasureReport".equals(codeString)) 1675 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.MEASUREREPORT); 1676 if ("Media".equals(codeString)) 1677 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.MEDIA); 1678 if ("Medication".equals(codeString)) 1679 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.MEDICATION); 1680 if ("MedicationAdministration".equals(codeString)) 1681 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.MEDICATIONADMINISTRATION); 1682 if ("MedicationDispense".equals(codeString)) 1683 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.MEDICATIONDISPENSE); 1684 if ("MedicationRequest".equals(codeString)) 1685 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.MEDICATIONREQUEST); 1686 if ("MedicationStatement".equals(codeString)) 1687 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.MEDICATIONSTATEMENT); 1688 if ("MessageDefinition".equals(codeString)) 1689 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.MESSAGEDEFINITION); 1690 if ("MessageHeader".equals(codeString)) 1691 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.MESSAGEHEADER); 1692 if ("NamingSystem".equals(codeString)) 1693 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.NAMINGSYSTEM); 1694 if ("NutritionOrder".equals(codeString)) 1695 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.NUTRITIONORDER); 1696 if ("Observation".equals(codeString)) 1697 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.OBSERVATION); 1698 if ("OperationDefinition".equals(codeString)) 1699 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.OPERATIONDEFINITION); 1700 if ("OperationOutcome".equals(codeString)) 1701 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.OPERATIONOUTCOME); 1702 if ("Organization".equals(codeString)) 1703 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.ORGANIZATION); 1704 if ("Parameters".equals(codeString)) 1705 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.PARAMETERS); 1706 if ("Patient".equals(codeString)) 1707 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.PATIENT); 1708 if ("PaymentNotice".equals(codeString)) 1709 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.PAYMENTNOTICE); 1710 if ("PaymentReconciliation".equals(codeString)) 1711 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.PAYMENTRECONCILIATION); 1712 if ("Person".equals(codeString)) 1713 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.PERSON); 1714 if ("PlanDefinition".equals(codeString)) 1715 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.PLANDEFINITION); 1716 if ("Practitioner".equals(codeString)) 1717 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.PRACTITIONER); 1718 if ("PractitionerRole".equals(codeString)) 1719 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.PRACTITIONERROLE); 1720 if ("Procedure".equals(codeString)) 1721 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.PROCEDURE); 1722 if ("ProcedureRequest".equals(codeString)) 1723 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.PROCEDUREREQUEST); 1724 if ("ProcessRequest".equals(codeString)) 1725 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.PROCESSREQUEST); 1726 if ("ProcessResponse".equals(codeString)) 1727 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.PROCESSRESPONSE); 1728 if ("Provenance".equals(codeString)) 1729 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.PROVENANCE); 1730 if ("Questionnaire".equals(codeString)) 1731 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.QUESTIONNAIRE); 1732 if ("QuestionnaireResponse".equals(codeString)) 1733 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.QUESTIONNAIRERESPONSE); 1734 if ("ReferralRequest".equals(codeString)) 1735 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.REFERRALREQUEST); 1736 if ("RelatedPerson".equals(codeString)) 1737 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.RELATEDPERSON); 1738 if ("RequestGroup".equals(codeString)) 1739 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.REQUESTGROUP); 1740 if ("ResearchStudy".equals(codeString)) 1741 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.RESEARCHSTUDY); 1742 if ("ResearchSubject".equals(codeString)) 1743 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.RESEARCHSUBJECT); 1744 if ("Resource".equals(codeString)) 1745 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.RESOURCE); 1746 if ("RiskAssessment".equals(codeString)) 1747 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.RISKASSESSMENT); 1748 if ("Schedule".equals(codeString)) 1749 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.SCHEDULE); 1750 if ("SearchParameter".equals(codeString)) 1751 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.SEARCHPARAMETER); 1752 if ("Sequence".equals(codeString)) 1753 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.SEQUENCE); 1754 if ("ServiceDefinition".equals(codeString)) 1755 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.SERVICEDEFINITION); 1756 if ("Slot".equals(codeString)) 1757 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.SLOT); 1758 if ("Specimen".equals(codeString)) 1759 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.SPECIMEN); 1760 if ("StructureDefinition".equals(codeString)) 1761 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.STRUCTUREDEFINITION); 1762 if ("StructureMap".equals(codeString)) 1763 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.STRUCTUREMAP); 1764 if ("Subscription".equals(codeString)) 1765 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.SUBSCRIPTION); 1766 if ("Substance".equals(codeString)) 1767 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.SUBSTANCE); 1768 if ("SupplyDelivery".equals(codeString)) 1769 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.SUPPLYDELIVERY); 1770 if ("SupplyRequest".equals(codeString)) 1771 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.SUPPLYREQUEST); 1772 if ("Task".equals(codeString)) 1773 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.TASK); 1774 if ("TestReport".equals(codeString)) 1775 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.TESTREPORT); 1776 if ("TestScript".equals(codeString)) 1777 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.TESTSCRIPT); 1778 if ("ValueSet".equals(codeString)) 1779 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.VALUESET); 1780 if ("VisionPrescription".equals(codeString)) 1781 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.VISIONPRESCRIPTION); 1782 throw new FHIRException("Unknown ActivityDefinitionKind code '"+codeString+"'"); 1783 } 1784 public String toCode(ActivityDefinitionKind code) { 1785 if (code == ActivityDefinitionKind.NULL) 1786 return null; 1787 if (code == ActivityDefinitionKind.ACCOUNT) 1788 return "Account"; 1789 if (code == ActivityDefinitionKind.ACTIVITYDEFINITION) 1790 return "ActivityDefinition"; 1791 if (code == ActivityDefinitionKind.ADVERSEEVENT) 1792 return "AdverseEvent"; 1793 if (code == ActivityDefinitionKind.ALLERGYINTOLERANCE) 1794 return "AllergyIntolerance"; 1795 if (code == ActivityDefinitionKind.APPOINTMENT) 1796 return "Appointment"; 1797 if (code == ActivityDefinitionKind.APPOINTMENTRESPONSE) 1798 return "AppointmentResponse"; 1799 if (code == ActivityDefinitionKind.AUDITEVENT) 1800 return "AuditEvent"; 1801 if (code == ActivityDefinitionKind.BASIC) 1802 return "Basic"; 1803 if (code == ActivityDefinitionKind.BINARY) 1804 return "Binary"; 1805 if (code == ActivityDefinitionKind.BODYSITE) 1806 return "BodySite"; 1807 if (code == ActivityDefinitionKind.BUNDLE) 1808 return "Bundle"; 1809 if (code == ActivityDefinitionKind.CAPABILITYSTATEMENT) 1810 return "CapabilityStatement"; 1811 if (code == ActivityDefinitionKind.CAREPLAN) 1812 return "CarePlan"; 1813 if (code == ActivityDefinitionKind.CARETEAM) 1814 return "CareTeam"; 1815 if (code == ActivityDefinitionKind.CHARGEITEM) 1816 return "ChargeItem"; 1817 if (code == ActivityDefinitionKind.CLAIM) 1818 return "Claim"; 1819 if (code == ActivityDefinitionKind.CLAIMRESPONSE) 1820 return "ClaimResponse"; 1821 if (code == ActivityDefinitionKind.CLINICALIMPRESSION) 1822 return "ClinicalImpression"; 1823 if (code == ActivityDefinitionKind.CODESYSTEM) 1824 return "CodeSystem"; 1825 if (code == ActivityDefinitionKind.COMMUNICATION) 1826 return "Communication"; 1827 if (code == ActivityDefinitionKind.COMMUNICATIONREQUEST) 1828 return "CommunicationRequest"; 1829 if (code == ActivityDefinitionKind.COMPARTMENTDEFINITION) 1830 return "CompartmentDefinition"; 1831 if (code == ActivityDefinitionKind.COMPOSITION) 1832 return "Composition"; 1833 if (code == ActivityDefinitionKind.CONCEPTMAP) 1834 return "ConceptMap"; 1835 if (code == ActivityDefinitionKind.CONDITION) 1836 return "Condition"; 1837 if (code == ActivityDefinitionKind.CONSENT) 1838 return "Consent"; 1839 if (code == ActivityDefinitionKind.CONTRACT) 1840 return "Contract"; 1841 if (code == ActivityDefinitionKind.COVERAGE) 1842 return "Coverage"; 1843 if (code == ActivityDefinitionKind.DATAELEMENT) 1844 return "DataElement"; 1845 if (code == ActivityDefinitionKind.DETECTEDISSUE) 1846 return "DetectedIssue"; 1847 if (code == ActivityDefinitionKind.DEVICE) 1848 return "Device"; 1849 if (code == ActivityDefinitionKind.DEVICECOMPONENT) 1850 return "DeviceComponent"; 1851 if (code == ActivityDefinitionKind.DEVICEMETRIC) 1852 return "DeviceMetric"; 1853 if (code == ActivityDefinitionKind.DEVICEREQUEST) 1854 return "DeviceRequest"; 1855 if (code == ActivityDefinitionKind.DEVICEUSESTATEMENT) 1856 return "DeviceUseStatement"; 1857 if (code == ActivityDefinitionKind.DIAGNOSTICREPORT) 1858 return "DiagnosticReport"; 1859 if (code == ActivityDefinitionKind.DOCUMENTMANIFEST) 1860 return "DocumentManifest"; 1861 if (code == ActivityDefinitionKind.DOCUMENTREFERENCE) 1862 return "DocumentReference"; 1863 if (code == ActivityDefinitionKind.DOMAINRESOURCE) 1864 return "DomainResource"; 1865 if (code == ActivityDefinitionKind.ELIGIBILITYREQUEST) 1866 return "EligibilityRequest"; 1867 if (code == ActivityDefinitionKind.ELIGIBILITYRESPONSE) 1868 return "EligibilityResponse"; 1869 if (code == ActivityDefinitionKind.ENCOUNTER) 1870 return "Encounter"; 1871 if (code == ActivityDefinitionKind.ENDPOINT) 1872 return "Endpoint"; 1873 if (code == ActivityDefinitionKind.ENROLLMENTREQUEST) 1874 return "EnrollmentRequest"; 1875 if (code == ActivityDefinitionKind.ENROLLMENTRESPONSE) 1876 return "EnrollmentResponse"; 1877 if (code == ActivityDefinitionKind.EPISODEOFCARE) 1878 return "EpisodeOfCare"; 1879 if (code == ActivityDefinitionKind.EXPANSIONPROFILE) 1880 return "ExpansionProfile"; 1881 if (code == ActivityDefinitionKind.EXPLANATIONOFBENEFIT) 1882 return "ExplanationOfBenefit"; 1883 if (code == ActivityDefinitionKind.FAMILYMEMBERHISTORY) 1884 return "FamilyMemberHistory"; 1885 if (code == ActivityDefinitionKind.FLAG) 1886 return "Flag"; 1887 if (code == ActivityDefinitionKind.GOAL) 1888 return "Goal"; 1889 if (code == ActivityDefinitionKind.GRAPHDEFINITION) 1890 return "GraphDefinition"; 1891 if (code == ActivityDefinitionKind.GROUP) 1892 return "Group"; 1893 if (code == ActivityDefinitionKind.GUIDANCERESPONSE) 1894 return "GuidanceResponse"; 1895 if (code == ActivityDefinitionKind.HEALTHCARESERVICE) 1896 return "HealthcareService"; 1897 if (code == ActivityDefinitionKind.IMAGINGMANIFEST) 1898 return "ImagingManifest"; 1899 if (code == ActivityDefinitionKind.IMAGINGSTUDY) 1900 return "ImagingStudy"; 1901 if (code == ActivityDefinitionKind.IMMUNIZATION) 1902 return "Immunization"; 1903 if (code == ActivityDefinitionKind.IMMUNIZATIONRECOMMENDATION) 1904 return "ImmunizationRecommendation"; 1905 if (code == ActivityDefinitionKind.IMPLEMENTATIONGUIDE) 1906 return "ImplementationGuide"; 1907 if (code == ActivityDefinitionKind.LIBRARY) 1908 return "Library"; 1909 if (code == ActivityDefinitionKind.LINKAGE) 1910 return "Linkage"; 1911 if (code == ActivityDefinitionKind.LIST) 1912 return "List"; 1913 if (code == ActivityDefinitionKind.LOCATION) 1914 return "Location"; 1915 if (code == ActivityDefinitionKind.MEASURE) 1916 return "Measure"; 1917 if (code == ActivityDefinitionKind.MEASUREREPORT) 1918 return "MeasureReport"; 1919 if (code == ActivityDefinitionKind.MEDIA) 1920 return "Media"; 1921 if (code == ActivityDefinitionKind.MEDICATION) 1922 return "Medication"; 1923 if (code == ActivityDefinitionKind.MEDICATIONADMINISTRATION) 1924 return "MedicationAdministration"; 1925 if (code == ActivityDefinitionKind.MEDICATIONDISPENSE) 1926 return "MedicationDispense"; 1927 if (code == ActivityDefinitionKind.MEDICATIONREQUEST) 1928 return "MedicationRequest"; 1929 if (code == ActivityDefinitionKind.MEDICATIONSTATEMENT) 1930 return "MedicationStatement"; 1931 if (code == ActivityDefinitionKind.MESSAGEDEFINITION) 1932 return "MessageDefinition"; 1933 if (code == ActivityDefinitionKind.MESSAGEHEADER) 1934 return "MessageHeader"; 1935 if (code == ActivityDefinitionKind.NAMINGSYSTEM) 1936 return "NamingSystem"; 1937 if (code == ActivityDefinitionKind.NUTRITIONORDER) 1938 return "NutritionOrder"; 1939 if (code == ActivityDefinitionKind.OBSERVATION) 1940 return "Observation"; 1941 if (code == ActivityDefinitionKind.OPERATIONDEFINITION) 1942 return "OperationDefinition"; 1943 if (code == ActivityDefinitionKind.OPERATIONOUTCOME) 1944 return "OperationOutcome"; 1945 if (code == ActivityDefinitionKind.ORGANIZATION) 1946 return "Organization"; 1947 if (code == ActivityDefinitionKind.PARAMETERS) 1948 return "Parameters"; 1949 if (code == ActivityDefinitionKind.PATIENT) 1950 return "Patient"; 1951 if (code == ActivityDefinitionKind.PAYMENTNOTICE) 1952 return "PaymentNotice"; 1953 if (code == ActivityDefinitionKind.PAYMENTRECONCILIATION) 1954 return "PaymentReconciliation"; 1955 if (code == ActivityDefinitionKind.PERSON) 1956 return "Person"; 1957 if (code == ActivityDefinitionKind.PLANDEFINITION) 1958 return "PlanDefinition"; 1959 if (code == ActivityDefinitionKind.PRACTITIONER) 1960 return "Practitioner"; 1961 if (code == ActivityDefinitionKind.PRACTITIONERROLE) 1962 return "PractitionerRole"; 1963 if (code == ActivityDefinitionKind.PROCEDURE) 1964 return "Procedure"; 1965 if (code == ActivityDefinitionKind.PROCEDUREREQUEST) 1966 return "ProcedureRequest"; 1967 if (code == ActivityDefinitionKind.PROCESSREQUEST) 1968 return "ProcessRequest"; 1969 if (code == ActivityDefinitionKind.PROCESSRESPONSE) 1970 return "ProcessResponse"; 1971 if (code == ActivityDefinitionKind.PROVENANCE) 1972 return "Provenance"; 1973 if (code == ActivityDefinitionKind.QUESTIONNAIRE) 1974 return "Questionnaire"; 1975 if (code == ActivityDefinitionKind.QUESTIONNAIRERESPONSE) 1976 return "QuestionnaireResponse"; 1977 if (code == ActivityDefinitionKind.REFERRALREQUEST) 1978 return "ReferralRequest"; 1979 if (code == ActivityDefinitionKind.RELATEDPERSON) 1980 return "RelatedPerson"; 1981 if (code == ActivityDefinitionKind.REQUESTGROUP) 1982 return "RequestGroup"; 1983 if (code == ActivityDefinitionKind.RESEARCHSTUDY) 1984 return "ResearchStudy"; 1985 if (code == ActivityDefinitionKind.RESEARCHSUBJECT) 1986 return "ResearchSubject"; 1987 if (code == ActivityDefinitionKind.RESOURCE) 1988 return "Resource"; 1989 if (code == ActivityDefinitionKind.RISKASSESSMENT) 1990 return "RiskAssessment"; 1991 if (code == ActivityDefinitionKind.SCHEDULE) 1992 return "Schedule"; 1993 if (code == ActivityDefinitionKind.SEARCHPARAMETER) 1994 return "SearchParameter"; 1995 if (code == ActivityDefinitionKind.SEQUENCE) 1996 return "Sequence"; 1997 if (code == ActivityDefinitionKind.SERVICEDEFINITION) 1998 return "ServiceDefinition"; 1999 if (code == ActivityDefinitionKind.SLOT) 2000 return "Slot"; 2001 if (code == ActivityDefinitionKind.SPECIMEN) 2002 return "Specimen"; 2003 if (code == ActivityDefinitionKind.STRUCTUREDEFINITION) 2004 return "StructureDefinition"; 2005 if (code == ActivityDefinitionKind.STRUCTUREMAP) 2006 return "StructureMap"; 2007 if (code == ActivityDefinitionKind.SUBSCRIPTION) 2008 return "Subscription"; 2009 if (code == ActivityDefinitionKind.SUBSTANCE) 2010 return "Substance"; 2011 if (code == ActivityDefinitionKind.SUPPLYDELIVERY) 2012 return "SupplyDelivery"; 2013 if (code == ActivityDefinitionKind.SUPPLYREQUEST) 2014 return "SupplyRequest"; 2015 if (code == ActivityDefinitionKind.TASK) 2016 return "Task"; 2017 if (code == ActivityDefinitionKind.TESTREPORT) 2018 return "TestReport"; 2019 if (code == ActivityDefinitionKind.TESTSCRIPT) 2020 return "TestScript"; 2021 if (code == ActivityDefinitionKind.VALUESET) 2022 return "ValueSet"; 2023 if (code == ActivityDefinitionKind.VISIONPRESCRIPTION) 2024 return "VisionPrescription"; 2025 return "?"; 2026 } 2027 public String toSystem(ActivityDefinitionKind code) { 2028 return code.getSystem(); 2029 } 2030 } 2031 2032 public enum ActivityParticipantType { 2033 /** 2034 * The participant is the patient under evaluation 2035 */ 2036 PATIENT, 2037 /** 2038 * The participant is a practitioner involved in the patient's care 2039 */ 2040 PRACTITIONER, 2041 /** 2042 * The participant is a person related to the patient 2043 */ 2044 RELATEDPERSON, 2045 /** 2046 * added to help the parsers with the generic types 2047 */ 2048 NULL; 2049 public static ActivityParticipantType fromCode(String codeString) throws FHIRException { 2050 if (codeString == null || "".equals(codeString)) 2051 return null; 2052 if ("patient".equals(codeString)) 2053 return PATIENT; 2054 if ("practitioner".equals(codeString)) 2055 return PRACTITIONER; 2056 if ("related-person".equals(codeString)) 2057 return RELATEDPERSON; 2058 if (Configuration.isAcceptInvalidEnums()) 2059 return null; 2060 else 2061 throw new FHIRException("Unknown ActivityParticipantType code '"+codeString+"'"); 2062 } 2063 public String toCode() { 2064 switch (this) { 2065 case PATIENT: return "patient"; 2066 case PRACTITIONER: return "practitioner"; 2067 case RELATEDPERSON: return "related-person"; 2068 case NULL: return null; 2069 default: return "?"; 2070 } 2071 } 2072 public String getSystem() { 2073 switch (this) { 2074 case PATIENT: return "http://hl7.org/fhir/action-participant-type"; 2075 case PRACTITIONER: return "http://hl7.org/fhir/action-participant-type"; 2076 case RELATEDPERSON: return "http://hl7.org/fhir/action-participant-type"; 2077 case NULL: return null; 2078 default: return "?"; 2079 } 2080 } 2081 public String getDefinition() { 2082 switch (this) { 2083 case PATIENT: return "The participant is the patient under evaluation"; 2084 case PRACTITIONER: return "The participant is a practitioner involved in the patient's care"; 2085 case RELATEDPERSON: return "The participant is a person related to the patient"; 2086 case NULL: return null; 2087 default: return "?"; 2088 } 2089 } 2090 public String getDisplay() { 2091 switch (this) { 2092 case PATIENT: return "Patient"; 2093 case PRACTITIONER: return "Practitioner"; 2094 case RELATEDPERSON: return "Related Person"; 2095 case NULL: return null; 2096 default: return "?"; 2097 } 2098 } 2099 } 2100 2101 public static class ActivityParticipantTypeEnumFactory implements EnumFactory<ActivityParticipantType> { 2102 public ActivityParticipantType fromCode(String codeString) throws IllegalArgumentException { 2103 if (codeString == null || "".equals(codeString)) 2104 if (codeString == null || "".equals(codeString)) 2105 return null; 2106 if ("patient".equals(codeString)) 2107 return ActivityParticipantType.PATIENT; 2108 if ("practitioner".equals(codeString)) 2109 return ActivityParticipantType.PRACTITIONER; 2110 if ("related-person".equals(codeString)) 2111 return ActivityParticipantType.RELATEDPERSON; 2112 throw new IllegalArgumentException("Unknown ActivityParticipantType code '"+codeString+"'"); 2113 } 2114 public Enumeration<ActivityParticipantType> fromType(PrimitiveType<?> code) throws FHIRException { 2115 if (code == null) 2116 return null; 2117 if (code.isEmpty()) 2118 return new Enumeration<ActivityParticipantType>(this); 2119 String codeString = code.asStringValue(); 2120 if (codeString == null || "".equals(codeString)) 2121 return null; 2122 if ("patient".equals(codeString)) 2123 return new Enumeration<ActivityParticipantType>(this, ActivityParticipantType.PATIENT); 2124 if ("practitioner".equals(codeString)) 2125 return new Enumeration<ActivityParticipantType>(this, ActivityParticipantType.PRACTITIONER); 2126 if ("related-person".equals(codeString)) 2127 return new Enumeration<ActivityParticipantType>(this, ActivityParticipantType.RELATEDPERSON); 2128 throw new FHIRException("Unknown ActivityParticipantType code '"+codeString+"'"); 2129 } 2130 public String toCode(ActivityParticipantType code) { 2131 if (code == ActivityParticipantType.NULL) 2132 return null; 2133 if (code == ActivityParticipantType.PATIENT) 2134 return "patient"; 2135 if (code == ActivityParticipantType.PRACTITIONER) 2136 return "practitioner"; 2137 if (code == ActivityParticipantType.RELATEDPERSON) 2138 return "related-person"; 2139 return "?"; 2140 } 2141 public String toSystem(ActivityParticipantType code) { 2142 return code.getSystem(); 2143 } 2144 } 2145 2146 @Block() 2147 public static class ActivityDefinitionParticipantComponent extends BackboneElement implements IBaseBackboneElement { 2148 /** 2149 * The type of participant in the action. 2150 */ 2151 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 2152 @Description(shortDefinition="patient | practitioner | related-person", formalDefinition="The type of participant in the action." ) 2153 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-participant-type") 2154 protected Enumeration<ActivityParticipantType> type; 2155 2156 /** 2157 * The role the participant should play in performing the described action. 2158 */ 2159 @Child(name = "role", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 2160 @Description(shortDefinition="E.g. Nurse, Surgeon, Parent, etc", formalDefinition="The role the participant should play in performing the described action." ) 2161 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-participant-role") 2162 protected CodeableConcept role; 2163 2164 private static final long serialVersionUID = -1450932564L; 2165 2166 /** 2167 * Constructor 2168 */ 2169 public ActivityDefinitionParticipantComponent() { 2170 super(); 2171 } 2172 2173 /** 2174 * Constructor 2175 */ 2176 public ActivityDefinitionParticipantComponent(Enumeration<ActivityParticipantType> type) { 2177 super(); 2178 this.type = type; 2179 } 2180 2181 /** 2182 * @return {@link #type} (The type of participant in the action.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 2183 */ 2184 public Enumeration<ActivityParticipantType> getTypeElement() { 2185 if (this.type == null) 2186 if (Configuration.errorOnAutoCreate()) 2187 throw new Error("Attempt to auto-create ActivityDefinitionParticipantComponent.type"); 2188 else if (Configuration.doAutoCreate()) 2189 this.type = new Enumeration<ActivityParticipantType>(new ActivityParticipantTypeEnumFactory()); // bb 2190 return this.type; 2191 } 2192 2193 public boolean hasTypeElement() { 2194 return this.type != null && !this.type.isEmpty(); 2195 } 2196 2197 public boolean hasType() { 2198 return this.type != null && !this.type.isEmpty(); 2199 } 2200 2201 /** 2202 * @param value {@link #type} (The type of participant in the action.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 2203 */ 2204 public ActivityDefinitionParticipantComponent setTypeElement(Enumeration<ActivityParticipantType> value) { 2205 this.type = value; 2206 return this; 2207 } 2208 2209 /** 2210 * @return The type of participant in the action. 2211 */ 2212 public ActivityParticipantType getType() { 2213 return this.type == null ? null : this.type.getValue(); 2214 } 2215 2216 /** 2217 * @param value The type of participant in the action. 2218 */ 2219 public ActivityDefinitionParticipantComponent setType(ActivityParticipantType value) { 2220 if (this.type == null) 2221 this.type = new Enumeration<ActivityParticipantType>(new ActivityParticipantTypeEnumFactory()); 2222 this.type.setValue(value); 2223 return this; 2224 } 2225 2226 /** 2227 * @return {@link #role} (The role the participant should play in performing the described action.) 2228 */ 2229 public CodeableConcept getRole() { 2230 if (this.role == null) 2231 if (Configuration.errorOnAutoCreate()) 2232 throw new Error("Attempt to auto-create ActivityDefinitionParticipantComponent.role"); 2233 else if (Configuration.doAutoCreate()) 2234 this.role = new CodeableConcept(); // cc 2235 return this.role; 2236 } 2237 2238 public boolean hasRole() { 2239 return this.role != null && !this.role.isEmpty(); 2240 } 2241 2242 /** 2243 * @param value {@link #role} (The role the participant should play in performing the described action.) 2244 */ 2245 public ActivityDefinitionParticipantComponent setRole(CodeableConcept value) { 2246 this.role = value; 2247 return this; 2248 } 2249 2250 protected void listChildren(List<Property> children) { 2251 super.listChildren(children); 2252 children.add(new Property("type", "code", "The type of participant in the action.", 0, 1, type)); 2253 children.add(new Property("role", "CodeableConcept", "The role the participant should play in performing the described action.", 0, 1, role)); 2254 } 2255 2256 @Override 2257 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2258 switch (_hash) { 2259 case 3575610: /*type*/ return new Property("type", "code", "The type of participant in the action.", 0, 1, type); 2260 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "The role the participant should play in performing the described action.", 0, 1, role); 2261 default: return super.getNamedProperty(_hash, _name, _checkValid); 2262 } 2263 2264 } 2265 2266 @Override 2267 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2268 switch (hash) { 2269 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<ActivityParticipantType> 2270 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 2271 default: return super.getProperty(hash, name, checkValid); 2272 } 2273 2274 } 2275 2276 @Override 2277 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2278 switch (hash) { 2279 case 3575610: // type 2280 value = new ActivityParticipantTypeEnumFactory().fromType(castToCode(value)); 2281 this.type = (Enumeration) value; // Enumeration<ActivityParticipantType> 2282 return value; 2283 case 3506294: // role 2284 this.role = castToCodeableConcept(value); // CodeableConcept 2285 return value; 2286 default: return super.setProperty(hash, name, value); 2287 } 2288 2289 } 2290 2291 @Override 2292 public Base setProperty(String name, Base value) throws FHIRException { 2293 if (name.equals("type")) { 2294 value = new ActivityParticipantTypeEnumFactory().fromType(castToCode(value)); 2295 this.type = (Enumeration) value; // Enumeration<ActivityParticipantType> 2296 } else if (name.equals("role")) { 2297 this.role = castToCodeableConcept(value); // CodeableConcept 2298 } else 2299 return super.setProperty(name, value); 2300 return value; 2301 } 2302 2303 @Override 2304 public Base makeProperty(int hash, String name) throws FHIRException { 2305 switch (hash) { 2306 case 3575610: return getTypeElement(); 2307 case 3506294: return getRole(); 2308 default: return super.makeProperty(hash, name); 2309 } 2310 2311 } 2312 2313 @Override 2314 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2315 switch (hash) { 2316 case 3575610: /*type*/ return new String[] {"code"}; 2317 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 2318 default: return super.getTypesForProperty(hash, name); 2319 } 2320 2321 } 2322 2323 @Override 2324 public Base addChild(String name) throws FHIRException { 2325 if (name.equals("type")) { 2326 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.type"); 2327 } 2328 else if (name.equals("role")) { 2329 this.role = new CodeableConcept(); 2330 return this.role; 2331 } 2332 else 2333 return super.addChild(name); 2334 } 2335 2336 public ActivityDefinitionParticipantComponent copy() { 2337 ActivityDefinitionParticipantComponent dst = new ActivityDefinitionParticipantComponent(); 2338 copyValues(dst); 2339 dst.type = type == null ? null : type.copy(); 2340 dst.role = role == null ? null : role.copy(); 2341 return dst; 2342 } 2343 2344 @Override 2345 public boolean equalsDeep(Base other_) { 2346 if (!super.equalsDeep(other_)) 2347 return false; 2348 if (!(other_ instanceof ActivityDefinitionParticipantComponent)) 2349 return false; 2350 ActivityDefinitionParticipantComponent o = (ActivityDefinitionParticipantComponent) other_; 2351 return compareDeep(type, o.type, true) && compareDeep(role, o.role, true); 2352 } 2353 2354 @Override 2355 public boolean equalsShallow(Base other_) { 2356 if (!super.equalsShallow(other_)) 2357 return false; 2358 if (!(other_ instanceof ActivityDefinitionParticipantComponent)) 2359 return false; 2360 ActivityDefinitionParticipantComponent o = (ActivityDefinitionParticipantComponent) other_; 2361 return compareValues(type, o.type, true); 2362 } 2363 2364 public boolean isEmpty() { 2365 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, role); 2366 } 2367 2368 public String fhirType() { 2369 return "ActivityDefinition.participant"; 2370 2371 } 2372 2373 } 2374 2375 @Block() 2376 public static class ActivityDefinitionDynamicValueComponent extends BackboneElement implements IBaseBackboneElement { 2377 /** 2378 * A brief, natural language description of the intended semantics of the dynamic value. 2379 */ 2380 @Child(name = "description", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 2381 @Description(shortDefinition="Natural language description of the dynamic value", formalDefinition="A brief, natural language description of the intended semantics of the dynamic value." ) 2382 protected StringType description; 2383 2384 /** 2385 * The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. 2386 */ 2387 @Child(name = "path", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2388 @Description(shortDefinition="The path to the element to be set dynamically", formalDefinition="The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression." ) 2389 protected StringType path; 2390 2391 /** 2392 * The media type of the language for the expression. 2393 */ 2394 @Child(name = "language", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 2395 @Description(shortDefinition="Language of the expression", formalDefinition="The media type of the language for the expression." ) 2396 protected StringType language; 2397 2398 /** 2399 * An expression specifying the value of the customized element. 2400 */ 2401 @Child(name = "expression", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 2402 @Description(shortDefinition="An expression that provides the dynamic value for the customization", formalDefinition="An expression specifying the value of the customized element." ) 2403 protected StringType expression; 2404 2405 private static final long serialVersionUID = 448404361L; 2406 2407 /** 2408 * Constructor 2409 */ 2410 public ActivityDefinitionDynamicValueComponent() { 2411 super(); 2412 } 2413 2414 /** 2415 * @return {@link #description} (A brief, natural language description of the intended semantics of the dynamic value.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2416 */ 2417 public StringType getDescriptionElement() { 2418 if (this.description == null) 2419 if (Configuration.errorOnAutoCreate()) 2420 throw new Error("Attempt to auto-create ActivityDefinitionDynamicValueComponent.description"); 2421 else if (Configuration.doAutoCreate()) 2422 this.description = new StringType(); // bb 2423 return this.description; 2424 } 2425 2426 public boolean hasDescriptionElement() { 2427 return this.description != null && !this.description.isEmpty(); 2428 } 2429 2430 public boolean hasDescription() { 2431 return this.description != null && !this.description.isEmpty(); 2432 } 2433 2434 /** 2435 * @param value {@link #description} (A brief, natural language description of the intended semantics of the dynamic value.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2436 */ 2437 public ActivityDefinitionDynamicValueComponent setDescriptionElement(StringType value) { 2438 this.description = value; 2439 return this; 2440 } 2441 2442 /** 2443 * @return A brief, natural language description of the intended semantics of the dynamic value. 2444 */ 2445 public String getDescription() { 2446 return this.description == null ? null : this.description.getValue(); 2447 } 2448 2449 /** 2450 * @param value A brief, natural language description of the intended semantics of the dynamic value. 2451 */ 2452 public ActivityDefinitionDynamicValueComponent setDescription(String value) { 2453 if (Utilities.noString(value)) 2454 this.description = null; 2455 else { 2456 if (this.description == null) 2457 this.description = new StringType(); 2458 this.description.setValue(value); 2459 } 2460 return this; 2461 } 2462 2463 /** 2464 * @return {@link #path} (The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 2465 */ 2466 public StringType getPathElement() { 2467 if (this.path == null) 2468 if (Configuration.errorOnAutoCreate()) 2469 throw new Error("Attempt to auto-create ActivityDefinitionDynamicValueComponent.path"); 2470 else if (Configuration.doAutoCreate()) 2471 this.path = new StringType(); // bb 2472 return this.path; 2473 } 2474 2475 public boolean hasPathElement() { 2476 return this.path != null && !this.path.isEmpty(); 2477 } 2478 2479 public boolean hasPath() { 2480 return this.path != null && !this.path.isEmpty(); 2481 } 2482 2483 /** 2484 * @param value {@link #path} (The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 2485 */ 2486 public ActivityDefinitionDynamicValueComponent setPathElement(StringType value) { 2487 this.path = value; 2488 return this; 2489 } 2490 2491 /** 2492 * @return The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. 2493 */ 2494 public String getPath() { 2495 return this.path == null ? null : this.path.getValue(); 2496 } 2497 2498 /** 2499 * @param value The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. 2500 */ 2501 public ActivityDefinitionDynamicValueComponent setPath(String value) { 2502 if (Utilities.noString(value)) 2503 this.path = null; 2504 else { 2505 if (this.path == null) 2506 this.path = new StringType(); 2507 this.path.setValue(value); 2508 } 2509 return this; 2510 } 2511 2512 /** 2513 * @return {@link #language} (The media type of the language for the expression.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 2514 */ 2515 public StringType getLanguageElement() { 2516 if (this.language == null) 2517 if (Configuration.errorOnAutoCreate()) 2518 throw new Error("Attempt to auto-create ActivityDefinitionDynamicValueComponent.language"); 2519 else if (Configuration.doAutoCreate()) 2520 this.language = new StringType(); // bb 2521 return this.language; 2522 } 2523 2524 public boolean hasLanguageElement() { 2525 return this.language != null && !this.language.isEmpty(); 2526 } 2527 2528 public boolean hasLanguage() { 2529 return this.language != null && !this.language.isEmpty(); 2530 } 2531 2532 /** 2533 * @param value {@link #language} (The media type of the language for the expression.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 2534 */ 2535 public ActivityDefinitionDynamicValueComponent setLanguageElement(StringType value) { 2536 this.language = value; 2537 return this; 2538 } 2539 2540 /** 2541 * @return The media type of the language for the expression. 2542 */ 2543 public String getLanguage() { 2544 return this.language == null ? null : this.language.getValue(); 2545 } 2546 2547 /** 2548 * @param value The media type of the language for the expression. 2549 */ 2550 public ActivityDefinitionDynamicValueComponent setLanguage(String value) { 2551 if (Utilities.noString(value)) 2552 this.language = null; 2553 else { 2554 if (this.language == null) 2555 this.language = new StringType(); 2556 this.language.setValue(value); 2557 } 2558 return this; 2559 } 2560 2561 /** 2562 * @return {@link #expression} (An expression specifying the value of the customized element.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 2563 */ 2564 public StringType getExpressionElement() { 2565 if (this.expression == null) 2566 if (Configuration.errorOnAutoCreate()) 2567 throw new Error("Attempt to auto-create ActivityDefinitionDynamicValueComponent.expression"); 2568 else if (Configuration.doAutoCreate()) 2569 this.expression = new StringType(); // bb 2570 return this.expression; 2571 } 2572 2573 public boolean hasExpressionElement() { 2574 return this.expression != null && !this.expression.isEmpty(); 2575 } 2576 2577 public boolean hasExpression() { 2578 return this.expression != null && !this.expression.isEmpty(); 2579 } 2580 2581 /** 2582 * @param value {@link #expression} (An expression specifying the value of the customized element.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 2583 */ 2584 public ActivityDefinitionDynamicValueComponent setExpressionElement(StringType value) { 2585 this.expression = value; 2586 return this; 2587 } 2588 2589 /** 2590 * @return An expression specifying the value of the customized element. 2591 */ 2592 public String getExpression() { 2593 return this.expression == null ? null : this.expression.getValue(); 2594 } 2595 2596 /** 2597 * @param value An expression specifying the value of the customized element. 2598 */ 2599 public ActivityDefinitionDynamicValueComponent setExpression(String value) { 2600 if (Utilities.noString(value)) 2601 this.expression = null; 2602 else { 2603 if (this.expression == null) 2604 this.expression = new StringType(); 2605 this.expression.setValue(value); 2606 } 2607 return this; 2608 } 2609 2610 protected void listChildren(List<Property> children) { 2611 super.listChildren(children); 2612 children.add(new Property("description", "string", "A brief, natural language description of the intended semantics of the dynamic value.", 0, 1, description)); 2613 children.add(new Property("path", "string", "The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression.", 0, 1, path)); 2614 children.add(new Property("language", "string", "The media type of the language for the expression.", 0, 1, language)); 2615 children.add(new Property("expression", "string", "An expression specifying the value of the customized element.", 0, 1, expression)); 2616 } 2617 2618 @Override 2619 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2620 switch (_hash) { 2621 case -1724546052: /*description*/ return new Property("description", "string", "A brief, natural language description of the intended semantics of the dynamic value.", 0, 1, description); 2622 case 3433509: /*path*/ return new Property("path", "string", "The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression.", 0, 1, path); 2623 case -1613589672: /*language*/ return new Property("language", "string", "The media type of the language for the expression.", 0, 1, language); 2624 case -1795452264: /*expression*/ return new Property("expression", "string", "An expression specifying the value of the customized element.", 0, 1, expression); 2625 default: return super.getNamedProperty(_hash, _name, _checkValid); 2626 } 2627 2628 } 2629 2630 @Override 2631 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2632 switch (hash) { 2633 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 2634 case 3433509: /*path*/ return this.path == null ? new Base[0] : new Base[] {this.path}; // StringType 2635 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // StringType 2636 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : new Base[] {this.expression}; // StringType 2637 default: return super.getProperty(hash, name, checkValid); 2638 } 2639 2640 } 2641 2642 @Override 2643 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2644 switch (hash) { 2645 case -1724546052: // description 2646 this.description = castToString(value); // StringType 2647 return value; 2648 case 3433509: // path 2649 this.path = castToString(value); // StringType 2650 return value; 2651 case -1613589672: // language 2652 this.language = castToString(value); // StringType 2653 return value; 2654 case -1795452264: // expression 2655 this.expression = castToString(value); // StringType 2656 return value; 2657 default: return super.setProperty(hash, name, value); 2658 } 2659 2660 } 2661 2662 @Override 2663 public Base setProperty(String name, Base value) throws FHIRException { 2664 if (name.equals("description")) { 2665 this.description = castToString(value); // StringType 2666 } else if (name.equals("path")) { 2667 this.path = castToString(value); // StringType 2668 } else if (name.equals("language")) { 2669 this.language = castToString(value); // StringType 2670 } else if (name.equals("expression")) { 2671 this.expression = castToString(value); // StringType 2672 } else 2673 return super.setProperty(name, value); 2674 return value; 2675 } 2676 2677 @Override 2678 public Base makeProperty(int hash, String name) throws FHIRException { 2679 switch (hash) { 2680 case -1724546052: return getDescriptionElement(); 2681 case 3433509: return getPathElement(); 2682 case -1613589672: return getLanguageElement(); 2683 case -1795452264: return getExpressionElement(); 2684 default: return super.makeProperty(hash, name); 2685 } 2686 2687 } 2688 2689 @Override 2690 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2691 switch (hash) { 2692 case -1724546052: /*description*/ return new String[] {"string"}; 2693 case 3433509: /*path*/ return new String[] {"string"}; 2694 case -1613589672: /*language*/ return new String[] {"string"}; 2695 case -1795452264: /*expression*/ return new String[] {"string"}; 2696 default: return super.getTypesForProperty(hash, name); 2697 } 2698 2699 } 2700 2701 @Override 2702 public Base addChild(String name) throws FHIRException { 2703 if (name.equals("description")) { 2704 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.description"); 2705 } 2706 else if (name.equals("path")) { 2707 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.path"); 2708 } 2709 else if (name.equals("language")) { 2710 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.language"); 2711 } 2712 else if (name.equals("expression")) { 2713 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.expression"); 2714 } 2715 else 2716 return super.addChild(name); 2717 } 2718 2719 public ActivityDefinitionDynamicValueComponent copy() { 2720 ActivityDefinitionDynamicValueComponent dst = new ActivityDefinitionDynamicValueComponent(); 2721 copyValues(dst); 2722 dst.description = description == null ? null : description.copy(); 2723 dst.path = path == null ? null : path.copy(); 2724 dst.language = language == null ? null : language.copy(); 2725 dst.expression = expression == null ? null : expression.copy(); 2726 return dst; 2727 } 2728 2729 @Override 2730 public boolean equalsDeep(Base other_) { 2731 if (!super.equalsDeep(other_)) 2732 return false; 2733 if (!(other_ instanceof ActivityDefinitionDynamicValueComponent)) 2734 return false; 2735 ActivityDefinitionDynamicValueComponent o = (ActivityDefinitionDynamicValueComponent) other_; 2736 return compareDeep(description, o.description, true) && compareDeep(path, o.path, true) && compareDeep(language, o.language, true) 2737 && compareDeep(expression, o.expression, true); 2738 } 2739 2740 @Override 2741 public boolean equalsShallow(Base other_) { 2742 if (!super.equalsShallow(other_)) 2743 return false; 2744 if (!(other_ instanceof ActivityDefinitionDynamicValueComponent)) 2745 return false; 2746 ActivityDefinitionDynamicValueComponent o = (ActivityDefinitionDynamicValueComponent) other_; 2747 return compareValues(description, o.description, true) && compareValues(path, o.path, true) && compareValues(language, o.language, true) 2748 && compareValues(expression, o.expression, true); 2749 } 2750 2751 public boolean isEmpty() { 2752 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, path, language 2753 , expression); 2754 } 2755 2756 public String fhirType() { 2757 return "ActivityDefinition.dynamicValue"; 2758 2759 } 2760 2761 } 2762 2763 /** 2764 * A formal identifier that is used to identify this activity definition when it is represented in other formats, or referenced in a specification, model, design or an instance. 2765 */ 2766 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2767 @Description(shortDefinition="Additional identifier for the activity definition", formalDefinition="A formal identifier that is used to identify this activity definition when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 2768 protected List<Identifier> identifier; 2769 2770 /** 2771 * Explaination of why this activity definition is needed and why it has been designed as it has. 2772 */ 2773 @Child(name = "purpose", type = {MarkdownType.class}, order=1, min=0, max=1, modifier=false, summary=false) 2774 @Description(shortDefinition="Why this activity definition is defined", formalDefinition="Explaination of why this activity definition is needed and why it has been designed as it has." ) 2775 protected MarkdownType purpose; 2776 2777 /** 2778 * A detailed description of how the asset is used from a clinical perspective. 2779 */ 2780 @Child(name = "usage", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2781 @Description(shortDefinition="Describes the clinical usage of the asset", formalDefinition="A detailed description of how the asset is used from a clinical perspective." ) 2782 protected StringType usage; 2783 2784 /** 2785 * The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 2786 */ 2787 @Child(name = "approvalDate", type = {DateType.class}, order=3, min=0, max=1, modifier=false, summary=false) 2788 @Description(shortDefinition="When the activity definition was approved by publisher", formalDefinition="The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage." ) 2789 protected DateType approvalDate; 2790 2791 /** 2792 * The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date. 2793 */ 2794 @Child(name = "lastReviewDate", type = {DateType.class}, order=4, min=0, max=1, modifier=false, summary=false) 2795 @Description(shortDefinition="When the activity definition was last reviewed", formalDefinition="The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date." ) 2796 protected DateType lastReviewDate; 2797 2798 /** 2799 * The period during which the activity definition content was or is planned to be in active use. 2800 */ 2801 @Child(name = "effectivePeriod", type = {Period.class}, order=5, min=0, max=1, modifier=false, summary=true) 2802 @Description(shortDefinition="When the activity definition is expected to be used", formalDefinition="The period during which the activity definition content was or is planned to be in active use." ) 2803 protected Period effectivePeriod; 2804 2805 /** 2806 * Descriptive topics related to the content of the activity. Topics provide a high-level categorization of the activity that can be useful for filtering and searching. 2807 */ 2808 @Child(name = "topic", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2809 @Description(shortDefinition="E.g. Education, Treatment, Assessment, etc", formalDefinition="Descriptive topics related to the content of the activity. Topics provide a high-level categorization of the activity that can be useful for filtering and searching." ) 2810 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/definition-topic") 2811 protected List<CodeableConcept> topic; 2812 2813 /** 2814 * A contributor to the content of the asset, including authors, editors, reviewers, and endorsers. 2815 */ 2816 @Child(name = "contributor", type = {Contributor.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2817 @Description(shortDefinition="A content contributor", formalDefinition="A contributor to the content of the asset, including authors, editors, reviewers, and endorsers." ) 2818 protected List<Contributor> contributor; 2819 2820 /** 2821 * A copyright statement relating to the activity definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the activity definition. 2822 */ 2823 @Child(name = "copyright", type = {MarkdownType.class}, order=8, min=0, max=1, modifier=false, summary=false) 2824 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the activity definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the activity definition." ) 2825 protected MarkdownType copyright; 2826 2827 /** 2828 * Related artifacts such as additional documentation, justification, or bibliographic references. 2829 */ 2830 @Child(name = "relatedArtifact", type = {RelatedArtifact.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2831 @Description(shortDefinition="Additional documentation, citations, etc", formalDefinition="Related artifacts such as additional documentation, justification, or bibliographic references." ) 2832 protected List<RelatedArtifact> relatedArtifact; 2833 2834 /** 2835 * A reference to a Library resource containing any formal logic used by the asset. 2836 */ 2837 @Child(name = "library", type = {Library.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2838 @Description(shortDefinition="Logic used by the asset", formalDefinition="A reference to a Library resource containing any formal logic used by the asset." ) 2839 protected List<Reference> library; 2840 /** 2841 * The actual objects that are the target of the reference (A reference to a Library resource containing any formal logic used by the asset.) 2842 */ 2843 protected List<Library> libraryTarget; 2844 2845 2846 /** 2847 * A description of the kind of resource the activity definition is representing. For example, a MedicationRequest, a ProcedureRequest, or a CommunicationRequest. Typically, but not always, this is a Request resource. 2848 */ 2849 @Child(name = "kind", type = {CodeType.class}, order=11, min=0, max=1, modifier=false, summary=false) 2850 @Description(shortDefinition="Kind of resource", formalDefinition="A description of the kind of resource the activity definition is representing. For example, a MedicationRequest, a ProcedureRequest, or a CommunicationRequest. Typically, but not always, this is a Request resource." ) 2851 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/resource-types") 2852 protected Enumeration<ActivityDefinitionKind> kind; 2853 2854 /** 2855 * Detailed description of the type of activity; e.g. What lab test, what procedure, what kind of encounter. 2856 */ 2857 @Child(name = "code", type = {CodeableConcept.class}, order=12, min=0, max=1, modifier=false, summary=false) 2858 @Description(shortDefinition="Detail type of activity", formalDefinition="Detailed description of the type of activity; e.g. What lab test, what procedure, what kind of encounter." ) 2859 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/procedure-code") 2860 protected CodeableConcept code; 2861 2862 /** 2863 * The period, timing or frequency upon which the described activity is to occur. 2864 */ 2865 @Child(name = "timing", type = {Timing.class, DateTimeType.class, Period.class, Range.class}, order=13, min=0, max=1, modifier=false, summary=false) 2866 @Description(shortDefinition="When activity is to occur", formalDefinition="The period, timing or frequency upon which the described activity is to occur." ) 2867 protected Type timing; 2868 2869 /** 2870 * Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc. 2871 */ 2872 @Child(name = "location", type = {Location.class}, order=14, min=0, max=1, modifier=false, summary=false) 2873 @Description(shortDefinition="Where it should happen", formalDefinition="Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc." ) 2874 protected Reference location; 2875 2876 /** 2877 * The actual object that is the target of the reference (Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc.) 2878 */ 2879 protected Location locationTarget; 2880 2881 /** 2882 * Indicates who should participate in performing the action described. 2883 */ 2884 @Child(name = "participant", type = {}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2885 @Description(shortDefinition="Who should participate in the action", formalDefinition="Indicates who should participate in performing the action described." ) 2886 protected List<ActivityDefinitionParticipantComponent> participant; 2887 2888 /** 2889 * Identifies the food, drug or other product being consumed or supplied in the activity. 2890 */ 2891 @Child(name = "product", type = {Medication.class, Substance.class, CodeableConcept.class}, order=16, min=0, max=1, modifier=false, summary=false) 2892 @Description(shortDefinition="What's administered/supplied", formalDefinition="Identifies the food, drug or other product being consumed or supplied in the activity." ) 2893 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-codes") 2894 protected Type product; 2895 2896 /** 2897 * Identifies the quantity expected to be consumed at once (per dose, per meal, etc.). 2898 */ 2899 @Child(name = "quantity", type = {SimpleQuantity.class}, order=17, min=0, max=1, modifier=false, summary=false) 2900 @Description(shortDefinition="How much is administered/consumed/supplied", formalDefinition="Identifies the quantity expected to be consumed at once (per dose, per meal, etc.)." ) 2901 protected SimpleQuantity quantity; 2902 2903 /** 2904 * Provides detailed dosage instructions in the same way that they are described for MedicationRequest resources. 2905 */ 2906 @Child(name = "dosage", type = {Dosage.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2907 @Description(shortDefinition="Detailed dosage instructions", formalDefinition="Provides detailed dosage instructions in the same way that they are described for MedicationRequest resources." ) 2908 protected List<Dosage> dosage; 2909 2910 /** 2911 * Indicates the sites on the subject's body where the procedure should be performed (I.e. the target sites). 2912 */ 2913 @Child(name = "bodySite", type = {CodeableConcept.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2914 @Description(shortDefinition="What part of body to perform on", formalDefinition="Indicates the sites on the subject's body where the procedure should be performed (I.e. the target sites)." ) 2915 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/body-site") 2916 protected List<CodeableConcept> bodySite; 2917 2918 /** 2919 * A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input. 2920 */ 2921 @Child(name = "transform", type = {StructureMap.class}, order=20, min=0, max=1, modifier=false, summary=false) 2922 @Description(shortDefinition="Transform to apply the template", formalDefinition="A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input." ) 2923 protected Reference transform; 2924 2925 /** 2926 * The actual object that is the target of the reference (A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.) 2927 */ 2928 protected StructureMap transformTarget; 2929 2930 /** 2931 * Dynamic values that will be evaluated to produce values for elements of the resulting resource. For example, if the dosage of a medication must be computed based on the patient's weight, a dynamic value would be used to specify an expression that calculated the weight, and the path on the intent resource that would contain the result. 2932 */ 2933 @Child(name = "dynamicValue", type = {}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2934 @Description(shortDefinition="Dynamic aspects of the definition", formalDefinition="Dynamic values that will be evaluated to produce values for elements of the resulting resource. For example, if the dosage of a medication must be computed based on the patient's weight, a dynamic value would be used to specify an expression that calculated the weight, and the path on the intent resource that would contain the result." ) 2935 protected List<ActivityDefinitionDynamicValueComponent> dynamicValue; 2936 2937 private static final long serialVersionUID = 1741931476L; 2938 2939 /** 2940 * Constructor 2941 */ 2942 public ActivityDefinition() { 2943 super(); 2944 } 2945 2946 /** 2947 * Constructor 2948 */ 2949 public ActivityDefinition(Enumeration<PublicationStatus> status) { 2950 super(); 2951 this.status = status; 2952 } 2953 2954 /** 2955 * @return {@link #url} (An absolute URI that is used to identify this activity definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this activity definition is (or will be) published. The URL SHOULD include the major version of the activity definition. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2956 */ 2957 public UriType getUrlElement() { 2958 if (this.url == null) 2959 if (Configuration.errorOnAutoCreate()) 2960 throw new Error("Attempt to auto-create ActivityDefinition.url"); 2961 else if (Configuration.doAutoCreate()) 2962 this.url = new UriType(); // bb 2963 return this.url; 2964 } 2965 2966 public boolean hasUrlElement() { 2967 return this.url != null && !this.url.isEmpty(); 2968 } 2969 2970 public boolean hasUrl() { 2971 return this.url != null && !this.url.isEmpty(); 2972 } 2973 2974 /** 2975 * @param value {@link #url} (An absolute URI that is used to identify this activity definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this activity definition is (or will be) published. The URL SHOULD include the major version of the activity definition. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2976 */ 2977 public ActivityDefinition setUrlElement(UriType value) { 2978 this.url = value; 2979 return this; 2980 } 2981 2982 /** 2983 * @return An absolute URI that is used to identify this activity definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this activity definition is (or will be) published. The URL SHOULD include the major version of the activity definition. For more information see [Technical and Business Versions](resource.html#versions). 2984 */ 2985 public String getUrl() { 2986 return this.url == null ? null : this.url.getValue(); 2987 } 2988 2989 /** 2990 * @param value An absolute URI that is used to identify this activity definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this activity definition is (or will be) published. The URL SHOULD include the major version of the activity definition. For more information see [Technical and Business Versions](resource.html#versions). 2991 */ 2992 public ActivityDefinition setUrl(String value) { 2993 if (Utilities.noString(value)) 2994 this.url = null; 2995 else { 2996 if (this.url == null) 2997 this.url = new UriType(); 2998 this.url.setValue(value); 2999 } 3000 return this; 3001 } 3002 3003 /** 3004 * @return {@link #identifier} (A formal identifier that is used to identify this activity definition when it is represented in other formats, or referenced in a specification, model, design or an instance.) 3005 */ 3006 public List<Identifier> getIdentifier() { 3007 if (this.identifier == null) 3008 this.identifier = new ArrayList<Identifier>(); 3009 return this.identifier; 3010 } 3011 3012 /** 3013 * @return Returns a reference to <code>this</code> for easy method chaining 3014 */ 3015 public ActivityDefinition setIdentifier(List<Identifier> theIdentifier) { 3016 this.identifier = theIdentifier; 3017 return this; 3018 } 3019 3020 public boolean hasIdentifier() { 3021 if (this.identifier == null) 3022 return false; 3023 for (Identifier item : this.identifier) 3024 if (!item.isEmpty()) 3025 return true; 3026 return false; 3027 } 3028 3029 public Identifier addIdentifier() { //3 3030 Identifier t = new Identifier(); 3031 if (this.identifier == null) 3032 this.identifier = new ArrayList<Identifier>(); 3033 this.identifier.add(t); 3034 return t; 3035 } 3036 3037 public ActivityDefinition addIdentifier(Identifier t) { //3 3038 if (t == null) 3039 return this; 3040 if (this.identifier == null) 3041 this.identifier = new ArrayList<Identifier>(); 3042 this.identifier.add(t); 3043 return this; 3044 } 3045 3046 /** 3047 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 3048 */ 3049 public Identifier getIdentifierFirstRep() { 3050 if (getIdentifier().isEmpty()) { 3051 addIdentifier(); 3052 } 3053 return getIdentifier().get(0); 3054 } 3055 3056 /** 3057 * @return {@link #version} (The identifier that is used to identify this version of the activity definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the activity definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 3058 */ 3059 public StringType getVersionElement() { 3060 if (this.version == null) 3061 if (Configuration.errorOnAutoCreate()) 3062 throw new Error("Attempt to auto-create ActivityDefinition.version"); 3063 else if (Configuration.doAutoCreate()) 3064 this.version = new StringType(); // bb 3065 return this.version; 3066 } 3067 3068 public boolean hasVersionElement() { 3069 return this.version != null && !this.version.isEmpty(); 3070 } 3071 3072 public boolean hasVersion() { 3073 return this.version != null && !this.version.isEmpty(); 3074 } 3075 3076 /** 3077 * @param value {@link #version} (The identifier that is used to identify this version of the activity definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the activity definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 3078 */ 3079 public ActivityDefinition setVersionElement(StringType value) { 3080 this.version = value; 3081 return this; 3082 } 3083 3084 /** 3085 * @return The identifier that is used to identify this version of the activity definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the activity definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets. 3086 */ 3087 public String getVersion() { 3088 return this.version == null ? null : this.version.getValue(); 3089 } 3090 3091 /** 3092 * @param value The identifier that is used to identify this version of the activity definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the activity definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets. 3093 */ 3094 public ActivityDefinition setVersion(String value) { 3095 if (Utilities.noString(value)) 3096 this.version = null; 3097 else { 3098 if (this.version == null) 3099 this.version = new StringType(); 3100 this.version.setValue(value); 3101 } 3102 return this; 3103 } 3104 3105 /** 3106 * @return {@link #name} (A natural language name identifying the activity definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 3107 */ 3108 public StringType getNameElement() { 3109 if (this.name == null) 3110 if (Configuration.errorOnAutoCreate()) 3111 throw new Error("Attempt to auto-create ActivityDefinition.name"); 3112 else if (Configuration.doAutoCreate()) 3113 this.name = new StringType(); // bb 3114 return this.name; 3115 } 3116 3117 public boolean hasNameElement() { 3118 return this.name != null && !this.name.isEmpty(); 3119 } 3120 3121 public boolean hasName() { 3122 return this.name != null && !this.name.isEmpty(); 3123 } 3124 3125 /** 3126 * @param value {@link #name} (A natural language name identifying the activity definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 3127 */ 3128 public ActivityDefinition setNameElement(StringType value) { 3129 this.name = value; 3130 return this; 3131 } 3132 3133 /** 3134 * @return A natural language name identifying the activity definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 3135 */ 3136 public String getName() { 3137 return this.name == null ? null : this.name.getValue(); 3138 } 3139 3140 /** 3141 * @param value A natural language name identifying the activity definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 3142 */ 3143 public ActivityDefinition setName(String value) { 3144 if (Utilities.noString(value)) 3145 this.name = null; 3146 else { 3147 if (this.name == null) 3148 this.name = new StringType(); 3149 this.name.setValue(value); 3150 } 3151 return this; 3152 } 3153 3154 /** 3155 * @return {@link #title} (A short, descriptive, user-friendly title for the activity definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 3156 */ 3157 public StringType getTitleElement() { 3158 if (this.title == null) 3159 if (Configuration.errorOnAutoCreate()) 3160 throw new Error("Attempt to auto-create ActivityDefinition.title"); 3161 else if (Configuration.doAutoCreate()) 3162 this.title = new StringType(); // bb 3163 return this.title; 3164 } 3165 3166 public boolean hasTitleElement() { 3167 return this.title != null && !this.title.isEmpty(); 3168 } 3169 3170 public boolean hasTitle() { 3171 return this.title != null && !this.title.isEmpty(); 3172 } 3173 3174 /** 3175 * @param value {@link #title} (A short, descriptive, user-friendly title for the activity definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 3176 */ 3177 public ActivityDefinition setTitleElement(StringType value) { 3178 this.title = value; 3179 return this; 3180 } 3181 3182 /** 3183 * @return A short, descriptive, user-friendly title for the activity definition. 3184 */ 3185 public String getTitle() { 3186 return this.title == null ? null : this.title.getValue(); 3187 } 3188 3189 /** 3190 * @param value A short, descriptive, user-friendly title for the activity definition. 3191 */ 3192 public ActivityDefinition setTitle(String value) { 3193 if (Utilities.noString(value)) 3194 this.title = null; 3195 else { 3196 if (this.title == null) 3197 this.title = new StringType(); 3198 this.title.setValue(value); 3199 } 3200 return this; 3201 } 3202 3203 /** 3204 * @return {@link #status} (The status of this activity definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 3205 */ 3206 public Enumeration<PublicationStatus> getStatusElement() { 3207 if (this.status == null) 3208 if (Configuration.errorOnAutoCreate()) 3209 throw new Error("Attempt to auto-create ActivityDefinition.status"); 3210 else if (Configuration.doAutoCreate()) 3211 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 3212 return this.status; 3213 } 3214 3215 public boolean hasStatusElement() { 3216 return this.status != null && !this.status.isEmpty(); 3217 } 3218 3219 public boolean hasStatus() { 3220 return this.status != null && !this.status.isEmpty(); 3221 } 3222 3223 /** 3224 * @param value {@link #status} (The status of this activity definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 3225 */ 3226 public ActivityDefinition setStatusElement(Enumeration<PublicationStatus> value) { 3227 this.status = value; 3228 return this; 3229 } 3230 3231 /** 3232 * @return The status of this activity definition. Enables tracking the life-cycle of the content. 3233 */ 3234 public PublicationStatus getStatus() { 3235 return this.status == null ? null : this.status.getValue(); 3236 } 3237 3238 /** 3239 * @param value The status of this activity definition. Enables tracking the life-cycle of the content. 3240 */ 3241 public ActivityDefinition setStatus(PublicationStatus value) { 3242 if (this.status == null) 3243 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 3244 this.status.setValue(value); 3245 return this; 3246 } 3247 3248 /** 3249 * @return {@link #experimental} (A boolean value to indicate that this activity definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 3250 */ 3251 public BooleanType getExperimentalElement() { 3252 if (this.experimental == null) 3253 if (Configuration.errorOnAutoCreate()) 3254 throw new Error("Attempt to auto-create ActivityDefinition.experimental"); 3255 else if (Configuration.doAutoCreate()) 3256 this.experimental = new BooleanType(); // bb 3257 return this.experimental; 3258 } 3259 3260 public boolean hasExperimentalElement() { 3261 return this.experimental != null && !this.experimental.isEmpty(); 3262 } 3263 3264 public boolean hasExperimental() { 3265 return this.experimental != null && !this.experimental.isEmpty(); 3266 } 3267 3268 /** 3269 * @param value {@link #experimental} (A boolean value to indicate that this activity definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 3270 */ 3271 public ActivityDefinition setExperimentalElement(BooleanType value) { 3272 this.experimental = value; 3273 return this; 3274 } 3275 3276 /** 3277 * @return A boolean value to indicate that this activity definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 3278 */ 3279 public boolean getExperimental() { 3280 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 3281 } 3282 3283 /** 3284 * @param value A boolean value to indicate that this activity definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 3285 */ 3286 public ActivityDefinition setExperimental(boolean value) { 3287 if (this.experimental == null) 3288 this.experimental = new BooleanType(); 3289 this.experimental.setValue(value); 3290 return this; 3291 } 3292 3293 /** 3294 * @return {@link #date} (The date (and optionally time) when the activity definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the activity definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3295 */ 3296 public DateTimeType getDateElement() { 3297 if (this.date == null) 3298 if (Configuration.errorOnAutoCreate()) 3299 throw new Error("Attempt to auto-create ActivityDefinition.date"); 3300 else if (Configuration.doAutoCreate()) 3301 this.date = new DateTimeType(); // bb 3302 return this.date; 3303 } 3304 3305 public boolean hasDateElement() { 3306 return this.date != null && !this.date.isEmpty(); 3307 } 3308 3309 public boolean hasDate() { 3310 return this.date != null && !this.date.isEmpty(); 3311 } 3312 3313 /** 3314 * @param value {@link #date} (The date (and optionally time) when the activity definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the activity definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3315 */ 3316 public ActivityDefinition setDateElement(DateTimeType value) { 3317 this.date = value; 3318 return this; 3319 } 3320 3321 /** 3322 * @return The date (and optionally time) when the activity definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the activity definition changes. 3323 */ 3324 public Date getDate() { 3325 return this.date == null ? null : this.date.getValue(); 3326 } 3327 3328 /** 3329 * @param value The date (and optionally time) when the activity definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the activity definition changes. 3330 */ 3331 public ActivityDefinition setDate(Date value) { 3332 if (value == null) 3333 this.date = null; 3334 else { 3335 if (this.date == null) 3336 this.date = new DateTimeType(); 3337 this.date.setValue(value); 3338 } 3339 return this; 3340 } 3341 3342 /** 3343 * @return {@link #publisher} (The name of the individual or organization that published the activity definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 3344 */ 3345 public StringType getPublisherElement() { 3346 if (this.publisher == null) 3347 if (Configuration.errorOnAutoCreate()) 3348 throw new Error("Attempt to auto-create ActivityDefinition.publisher"); 3349 else if (Configuration.doAutoCreate()) 3350 this.publisher = new StringType(); // bb 3351 return this.publisher; 3352 } 3353 3354 public boolean hasPublisherElement() { 3355 return this.publisher != null && !this.publisher.isEmpty(); 3356 } 3357 3358 public boolean hasPublisher() { 3359 return this.publisher != null && !this.publisher.isEmpty(); 3360 } 3361 3362 /** 3363 * @param value {@link #publisher} (The name of the individual or organization that published the activity definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 3364 */ 3365 public ActivityDefinition setPublisherElement(StringType value) { 3366 this.publisher = value; 3367 return this; 3368 } 3369 3370 /** 3371 * @return The name of the individual or organization that published the activity definition. 3372 */ 3373 public String getPublisher() { 3374 return this.publisher == null ? null : this.publisher.getValue(); 3375 } 3376 3377 /** 3378 * @param value The name of the individual or organization that published the activity definition. 3379 */ 3380 public ActivityDefinition setPublisher(String value) { 3381 if (Utilities.noString(value)) 3382 this.publisher = null; 3383 else { 3384 if (this.publisher == null) 3385 this.publisher = new StringType(); 3386 this.publisher.setValue(value); 3387 } 3388 return this; 3389 } 3390 3391 /** 3392 * @return {@link #description} (A free text natural language description of the activity definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3393 */ 3394 public MarkdownType getDescriptionElement() { 3395 if (this.description == null) 3396 if (Configuration.errorOnAutoCreate()) 3397 throw new Error("Attempt to auto-create ActivityDefinition.description"); 3398 else if (Configuration.doAutoCreate()) 3399 this.description = new MarkdownType(); // bb 3400 return this.description; 3401 } 3402 3403 public boolean hasDescriptionElement() { 3404 return this.description != null && !this.description.isEmpty(); 3405 } 3406 3407 public boolean hasDescription() { 3408 return this.description != null && !this.description.isEmpty(); 3409 } 3410 3411 /** 3412 * @param value {@link #description} (A free text natural language description of the activity definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3413 */ 3414 public ActivityDefinition setDescriptionElement(MarkdownType value) { 3415 this.description = value; 3416 return this; 3417 } 3418 3419 /** 3420 * @return A free text natural language description of the activity definition from a consumer's perspective. 3421 */ 3422 public String getDescription() { 3423 return this.description == null ? null : this.description.getValue(); 3424 } 3425 3426 /** 3427 * @param value A free text natural language description of the activity definition from a consumer's perspective. 3428 */ 3429 public ActivityDefinition setDescription(String value) { 3430 if (value == null) 3431 this.description = null; 3432 else { 3433 if (this.description == null) 3434 this.description = new MarkdownType(); 3435 this.description.setValue(value); 3436 } 3437 return this; 3438 } 3439 3440 /** 3441 * @return {@link #purpose} (Explaination of why this activity definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 3442 */ 3443 public MarkdownType getPurposeElement() { 3444 if (this.purpose == null) 3445 if (Configuration.errorOnAutoCreate()) 3446 throw new Error("Attempt to auto-create ActivityDefinition.purpose"); 3447 else if (Configuration.doAutoCreate()) 3448 this.purpose = new MarkdownType(); // bb 3449 return this.purpose; 3450 } 3451 3452 public boolean hasPurposeElement() { 3453 return this.purpose != null && !this.purpose.isEmpty(); 3454 } 3455 3456 public boolean hasPurpose() { 3457 return this.purpose != null && !this.purpose.isEmpty(); 3458 } 3459 3460 /** 3461 * @param value {@link #purpose} (Explaination of why this activity definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 3462 */ 3463 public ActivityDefinition setPurposeElement(MarkdownType value) { 3464 this.purpose = value; 3465 return this; 3466 } 3467 3468 /** 3469 * @return Explaination of why this activity definition is needed and why it has been designed as it has. 3470 */ 3471 public String getPurpose() { 3472 return this.purpose == null ? null : this.purpose.getValue(); 3473 } 3474 3475 /** 3476 * @param value Explaination of why this activity definition is needed and why it has been designed as it has. 3477 */ 3478 public ActivityDefinition setPurpose(String value) { 3479 if (value == null) 3480 this.purpose = null; 3481 else { 3482 if (this.purpose == null) 3483 this.purpose = new MarkdownType(); 3484 this.purpose.setValue(value); 3485 } 3486 return this; 3487 } 3488 3489 /** 3490 * @return {@link #usage} (A detailed description of how the asset is used from a clinical perspective.). This is the underlying object with id, value and extensions. The accessor "getUsage" gives direct access to the value 3491 */ 3492 public StringType getUsageElement() { 3493 if (this.usage == null) 3494 if (Configuration.errorOnAutoCreate()) 3495 throw new Error("Attempt to auto-create ActivityDefinition.usage"); 3496 else if (Configuration.doAutoCreate()) 3497 this.usage = new StringType(); // bb 3498 return this.usage; 3499 } 3500 3501 public boolean hasUsageElement() { 3502 return this.usage != null && !this.usage.isEmpty(); 3503 } 3504 3505 public boolean hasUsage() { 3506 return this.usage != null && !this.usage.isEmpty(); 3507 } 3508 3509 /** 3510 * @param value {@link #usage} (A detailed description of how the asset is used from a clinical perspective.). This is the underlying object with id, value and extensions. The accessor "getUsage" gives direct access to the value 3511 */ 3512 public ActivityDefinition setUsageElement(StringType value) { 3513 this.usage = value; 3514 return this; 3515 } 3516 3517 /** 3518 * @return A detailed description of how the asset is used from a clinical perspective. 3519 */ 3520 public String getUsage() { 3521 return this.usage == null ? null : this.usage.getValue(); 3522 } 3523 3524 /** 3525 * @param value A detailed description of how the asset is used from a clinical perspective. 3526 */ 3527 public ActivityDefinition setUsage(String value) { 3528 if (Utilities.noString(value)) 3529 this.usage = null; 3530 else { 3531 if (this.usage == null) 3532 this.usage = new StringType(); 3533 this.usage.setValue(value); 3534 } 3535 return this; 3536 } 3537 3538 /** 3539 * @return {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 3540 */ 3541 public DateType getApprovalDateElement() { 3542 if (this.approvalDate == null) 3543 if (Configuration.errorOnAutoCreate()) 3544 throw new Error("Attempt to auto-create ActivityDefinition.approvalDate"); 3545 else if (Configuration.doAutoCreate()) 3546 this.approvalDate = new DateType(); // bb 3547 return this.approvalDate; 3548 } 3549 3550 public boolean hasApprovalDateElement() { 3551 return this.approvalDate != null && !this.approvalDate.isEmpty(); 3552 } 3553 3554 public boolean hasApprovalDate() { 3555 return this.approvalDate != null && !this.approvalDate.isEmpty(); 3556 } 3557 3558 /** 3559 * @param value {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 3560 */ 3561 public ActivityDefinition setApprovalDateElement(DateType value) { 3562 this.approvalDate = value; 3563 return this; 3564 } 3565 3566 /** 3567 * @return The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 3568 */ 3569 public Date getApprovalDate() { 3570 return this.approvalDate == null ? null : this.approvalDate.getValue(); 3571 } 3572 3573 /** 3574 * @param value The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 3575 */ 3576 public ActivityDefinition setApprovalDate(Date value) { 3577 if (value == null) 3578 this.approvalDate = null; 3579 else { 3580 if (this.approvalDate == null) 3581 this.approvalDate = new DateType(); 3582 this.approvalDate.setValue(value); 3583 } 3584 return this; 3585 } 3586 3587 /** 3588 * @return {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 3589 */ 3590 public DateType getLastReviewDateElement() { 3591 if (this.lastReviewDate == null) 3592 if (Configuration.errorOnAutoCreate()) 3593 throw new Error("Attempt to auto-create ActivityDefinition.lastReviewDate"); 3594 else if (Configuration.doAutoCreate()) 3595 this.lastReviewDate = new DateType(); // bb 3596 return this.lastReviewDate; 3597 } 3598 3599 public boolean hasLastReviewDateElement() { 3600 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 3601 } 3602 3603 public boolean hasLastReviewDate() { 3604 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 3605 } 3606 3607 /** 3608 * @param value {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 3609 */ 3610 public ActivityDefinition setLastReviewDateElement(DateType value) { 3611 this.lastReviewDate = value; 3612 return this; 3613 } 3614 3615 /** 3616 * @return The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date. 3617 */ 3618 public Date getLastReviewDate() { 3619 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 3620 } 3621 3622 /** 3623 * @param value The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date. 3624 */ 3625 public ActivityDefinition setLastReviewDate(Date value) { 3626 if (value == null) 3627 this.lastReviewDate = null; 3628 else { 3629 if (this.lastReviewDate == null) 3630 this.lastReviewDate = new DateType(); 3631 this.lastReviewDate.setValue(value); 3632 } 3633 return this; 3634 } 3635 3636 /** 3637 * @return {@link #effectivePeriod} (The period during which the activity definition content was or is planned to be in active use.) 3638 */ 3639 public Period getEffectivePeriod() { 3640 if (this.effectivePeriod == null) 3641 if (Configuration.errorOnAutoCreate()) 3642 throw new Error("Attempt to auto-create ActivityDefinition.effectivePeriod"); 3643 else if (Configuration.doAutoCreate()) 3644 this.effectivePeriod = new Period(); // cc 3645 return this.effectivePeriod; 3646 } 3647 3648 public boolean hasEffectivePeriod() { 3649 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 3650 } 3651 3652 /** 3653 * @param value {@link #effectivePeriod} (The period during which the activity definition content was or is planned to be in active use.) 3654 */ 3655 public ActivityDefinition setEffectivePeriod(Period value) { 3656 this.effectivePeriod = value; 3657 return this; 3658 } 3659 3660 /** 3661 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate activity definition instances.) 3662 */ 3663 public List<UsageContext> getUseContext() { 3664 if (this.useContext == null) 3665 this.useContext = new ArrayList<UsageContext>(); 3666 return this.useContext; 3667 } 3668 3669 /** 3670 * @return Returns a reference to <code>this</code> for easy method chaining 3671 */ 3672 public ActivityDefinition setUseContext(List<UsageContext> theUseContext) { 3673 this.useContext = theUseContext; 3674 return this; 3675 } 3676 3677 public boolean hasUseContext() { 3678 if (this.useContext == null) 3679 return false; 3680 for (UsageContext item : this.useContext) 3681 if (!item.isEmpty()) 3682 return true; 3683 return false; 3684 } 3685 3686 public UsageContext addUseContext() { //3 3687 UsageContext t = new UsageContext(); 3688 if (this.useContext == null) 3689 this.useContext = new ArrayList<UsageContext>(); 3690 this.useContext.add(t); 3691 return t; 3692 } 3693 3694 public ActivityDefinition addUseContext(UsageContext t) { //3 3695 if (t == null) 3696 return this; 3697 if (this.useContext == null) 3698 this.useContext = new ArrayList<UsageContext>(); 3699 this.useContext.add(t); 3700 return this; 3701 } 3702 3703 /** 3704 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist 3705 */ 3706 public UsageContext getUseContextFirstRep() { 3707 if (getUseContext().isEmpty()) { 3708 addUseContext(); 3709 } 3710 return getUseContext().get(0); 3711 } 3712 3713 /** 3714 * @return {@link #jurisdiction} (A legal or geographic region in which the activity definition is intended to be used.) 3715 */ 3716 public List<CodeableConcept> getJurisdiction() { 3717 if (this.jurisdiction == null) 3718 this.jurisdiction = new ArrayList<CodeableConcept>(); 3719 return this.jurisdiction; 3720 } 3721 3722 /** 3723 * @return Returns a reference to <code>this</code> for easy method chaining 3724 */ 3725 public ActivityDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 3726 this.jurisdiction = theJurisdiction; 3727 return this; 3728 } 3729 3730 public boolean hasJurisdiction() { 3731 if (this.jurisdiction == null) 3732 return false; 3733 for (CodeableConcept item : this.jurisdiction) 3734 if (!item.isEmpty()) 3735 return true; 3736 return false; 3737 } 3738 3739 public CodeableConcept addJurisdiction() { //3 3740 CodeableConcept t = new CodeableConcept(); 3741 if (this.jurisdiction == null) 3742 this.jurisdiction = new ArrayList<CodeableConcept>(); 3743 this.jurisdiction.add(t); 3744 return t; 3745 } 3746 3747 public ActivityDefinition addJurisdiction(CodeableConcept t) { //3 3748 if (t == null) 3749 return this; 3750 if (this.jurisdiction == null) 3751 this.jurisdiction = new ArrayList<CodeableConcept>(); 3752 this.jurisdiction.add(t); 3753 return this; 3754 } 3755 3756 /** 3757 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist 3758 */ 3759 public CodeableConcept getJurisdictionFirstRep() { 3760 if (getJurisdiction().isEmpty()) { 3761 addJurisdiction(); 3762 } 3763 return getJurisdiction().get(0); 3764 } 3765 3766 /** 3767 * @return {@link #topic} (Descriptive topics related to the content of the activity. Topics provide a high-level categorization of the activity that can be useful for filtering and searching.) 3768 */ 3769 public List<CodeableConcept> getTopic() { 3770 if (this.topic == null) 3771 this.topic = new ArrayList<CodeableConcept>(); 3772 return this.topic; 3773 } 3774 3775 /** 3776 * @return Returns a reference to <code>this</code> for easy method chaining 3777 */ 3778 public ActivityDefinition setTopic(List<CodeableConcept> theTopic) { 3779 this.topic = theTopic; 3780 return this; 3781 } 3782 3783 public boolean hasTopic() { 3784 if (this.topic == null) 3785 return false; 3786 for (CodeableConcept item : this.topic) 3787 if (!item.isEmpty()) 3788 return true; 3789 return false; 3790 } 3791 3792 public CodeableConcept addTopic() { //3 3793 CodeableConcept t = new CodeableConcept(); 3794 if (this.topic == null) 3795 this.topic = new ArrayList<CodeableConcept>(); 3796 this.topic.add(t); 3797 return t; 3798 } 3799 3800 public ActivityDefinition addTopic(CodeableConcept t) { //3 3801 if (t == null) 3802 return this; 3803 if (this.topic == null) 3804 this.topic = new ArrayList<CodeableConcept>(); 3805 this.topic.add(t); 3806 return this; 3807 } 3808 3809 /** 3810 * @return The first repetition of repeating field {@link #topic}, creating it if it does not already exist 3811 */ 3812 public CodeableConcept getTopicFirstRep() { 3813 if (getTopic().isEmpty()) { 3814 addTopic(); 3815 } 3816 return getTopic().get(0); 3817 } 3818 3819 /** 3820 * @return {@link #contributor} (A contributor to the content of the asset, including authors, editors, reviewers, and endorsers.) 3821 */ 3822 public List<Contributor> getContributor() { 3823 if (this.contributor == null) 3824 this.contributor = new ArrayList<Contributor>(); 3825 return this.contributor; 3826 } 3827 3828 /** 3829 * @return Returns a reference to <code>this</code> for easy method chaining 3830 */ 3831 public ActivityDefinition setContributor(List<Contributor> theContributor) { 3832 this.contributor = theContributor; 3833 return this; 3834 } 3835 3836 public boolean hasContributor() { 3837 if (this.contributor == null) 3838 return false; 3839 for (Contributor item : this.contributor) 3840 if (!item.isEmpty()) 3841 return true; 3842 return false; 3843 } 3844 3845 public Contributor addContributor() { //3 3846 Contributor t = new Contributor(); 3847 if (this.contributor == null) 3848 this.contributor = new ArrayList<Contributor>(); 3849 this.contributor.add(t); 3850 return t; 3851 } 3852 3853 public ActivityDefinition addContributor(Contributor t) { //3 3854 if (t == null) 3855 return this; 3856 if (this.contributor == null) 3857 this.contributor = new ArrayList<Contributor>(); 3858 this.contributor.add(t); 3859 return this; 3860 } 3861 3862 /** 3863 * @return The first repetition of repeating field {@link #contributor}, creating it if it does not already exist 3864 */ 3865 public Contributor getContributorFirstRep() { 3866 if (getContributor().isEmpty()) { 3867 addContributor(); 3868 } 3869 return getContributor().get(0); 3870 } 3871 3872 /** 3873 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 3874 */ 3875 public List<ContactDetail> getContact() { 3876 if (this.contact == null) 3877 this.contact = new ArrayList<ContactDetail>(); 3878 return this.contact; 3879 } 3880 3881 /** 3882 * @return Returns a reference to <code>this</code> for easy method chaining 3883 */ 3884 public ActivityDefinition setContact(List<ContactDetail> theContact) { 3885 this.contact = theContact; 3886 return this; 3887 } 3888 3889 public boolean hasContact() { 3890 if (this.contact == null) 3891 return false; 3892 for (ContactDetail item : this.contact) 3893 if (!item.isEmpty()) 3894 return true; 3895 return false; 3896 } 3897 3898 public ContactDetail addContact() { //3 3899 ContactDetail t = new ContactDetail(); 3900 if (this.contact == null) 3901 this.contact = new ArrayList<ContactDetail>(); 3902 this.contact.add(t); 3903 return t; 3904 } 3905 3906 public ActivityDefinition addContact(ContactDetail t) { //3 3907 if (t == null) 3908 return this; 3909 if (this.contact == null) 3910 this.contact = new ArrayList<ContactDetail>(); 3911 this.contact.add(t); 3912 return this; 3913 } 3914 3915 /** 3916 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist 3917 */ 3918 public ContactDetail getContactFirstRep() { 3919 if (getContact().isEmpty()) { 3920 addContact(); 3921 } 3922 return getContact().get(0); 3923 } 3924 3925 /** 3926 * @return {@link #copyright} (A copyright statement relating to the activity definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the activity definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 3927 */ 3928 public MarkdownType getCopyrightElement() { 3929 if (this.copyright == null) 3930 if (Configuration.errorOnAutoCreate()) 3931 throw new Error("Attempt to auto-create ActivityDefinition.copyright"); 3932 else if (Configuration.doAutoCreate()) 3933 this.copyright = new MarkdownType(); // bb 3934 return this.copyright; 3935 } 3936 3937 public boolean hasCopyrightElement() { 3938 return this.copyright != null && !this.copyright.isEmpty(); 3939 } 3940 3941 public boolean hasCopyright() { 3942 return this.copyright != null && !this.copyright.isEmpty(); 3943 } 3944 3945 /** 3946 * @param value {@link #copyright} (A copyright statement relating to the activity definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the activity definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 3947 */ 3948 public ActivityDefinition setCopyrightElement(MarkdownType value) { 3949 this.copyright = value; 3950 return this; 3951 } 3952 3953 /** 3954 * @return A copyright statement relating to the activity definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the activity definition. 3955 */ 3956 public String getCopyright() { 3957 return this.copyright == null ? null : this.copyright.getValue(); 3958 } 3959 3960 /** 3961 * @param value A copyright statement relating to the activity definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the activity definition. 3962 */ 3963 public ActivityDefinition setCopyright(String value) { 3964 if (value == null) 3965 this.copyright = null; 3966 else { 3967 if (this.copyright == null) 3968 this.copyright = new MarkdownType(); 3969 this.copyright.setValue(value); 3970 } 3971 return this; 3972 } 3973 3974 /** 3975 * @return {@link #relatedArtifact} (Related artifacts such as additional documentation, justification, or bibliographic references.) 3976 */ 3977 public List<RelatedArtifact> getRelatedArtifact() { 3978 if (this.relatedArtifact == null) 3979 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 3980 return this.relatedArtifact; 3981 } 3982 3983 /** 3984 * @return Returns a reference to <code>this</code> for easy method chaining 3985 */ 3986 public ActivityDefinition setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 3987 this.relatedArtifact = theRelatedArtifact; 3988 return this; 3989 } 3990 3991 public boolean hasRelatedArtifact() { 3992 if (this.relatedArtifact == null) 3993 return false; 3994 for (RelatedArtifact item : this.relatedArtifact) 3995 if (!item.isEmpty()) 3996 return true; 3997 return false; 3998 } 3999 4000 public RelatedArtifact addRelatedArtifact() { //3 4001 RelatedArtifact t = new RelatedArtifact(); 4002 if (this.relatedArtifact == null) 4003 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 4004 this.relatedArtifact.add(t); 4005 return t; 4006 } 4007 4008 public ActivityDefinition addRelatedArtifact(RelatedArtifact t) { //3 4009 if (t == null) 4010 return this; 4011 if (this.relatedArtifact == null) 4012 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 4013 this.relatedArtifact.add(t); 4014 return this; 4015 } 4016 4017 /** 4018 * @return The first repetition of repeating field {@link #relatedArtifact}, creating it if it does not already exist 4019 */ 4020 public RelatedArtifact getRelatedArtifactFirstRep() { 4021 if (getRelatedArtifact().isEmpty()) { 4022 addRelatedArtifact(); 4023 } 4024 return getRelatedArtifact().get(0); 4025 } 4026 4027 /** 4028 * @return {@link #library} (A reference to a Library resource containing any formal logic used by the asset.) 4029 */ 4030 public List<Reference> getLibrary() { 4031 if (this.library == null) 4032 this.library = new ArrayList<Reference>(); 4033 return this.library; 4034 } 4035 4036 /** 4037 * @return Returns a reference to <code>this</code> for easy method chaining 4038 */ 4039 public ActivityDefinition setLibrary(List<Reference> theLibrary) { 4040 this.library = theLibrary; 4041 return this; 4042 } 4043 4044 public boolean hasLibrary() { 4045 if (this.library == null) 4046 return false; 4047 for (Reference item : this.library) 4048 if (!item.isEmpty()) 4049 return true; 4050 return false; 4051 } 4052 4053 public Reference addLibrary() { //3 4054 Reference t = new Reference(); 4055 if (this.library == null) 4056 this.library = new ArrayList<Reference>(); 4057 this.library.add(t); 4058 return t; 4059 } 4060 4061 public ActivityDefinition addLibrary(Reference t) { //3 4062 if (t == null) 4063 return this; 4064 if (this.library == null) 4065 this.library = new ArrayList<Reference>(); 4066 this.library.add(t); 4067 return this; 4068 } 4069 4070 /** 4071 * @return The first repetition of repeating field {@link #library}, creating it if it does not already exist 4072 */ 4073 public Reference getLibraryFirstRep() { 4074 if (getLibrary().isEmpty()) { 4075 addLibrary(); 4076 } 4077 return getLibrary().get(0); 4078 } 4079 4080 /** 4081 * @deprecated Use Reference#setResource(IBaseResource) instead 4082 */ 4083 @Deprecated 4084 public List<Library> getLibraryTarget() { 4085 if (this.libraryTarget == null) 4086 this.libraryTarget = new ArrayList<Library>(); 4087 return this.libraryTarget; 4088 } 4089 4090 /** 4091 * @deprecated Use Reference#setResource(IBaseResource) instead 4092 */ 4093 @Deprecated 4094 public Library addLibraryTarget() { 4095 Library r = new Library(); 4096 if (this.libraryTarget == null) 4097 this.libraryTarget = new ArrayList<Library>(); 4098 this.libraryTarget.add(r); 4099 return r; 4100 } 4101 4102 /** 4103 * @return {@link #kind} (A description of the kind of resource the activity definition is representing. For example, a MedicationRequest, a ProcedureRequest, or a CommunicationRequest. Typically, but not always, this is a Request resource.). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 4104 */ 4105 public Enumeration<ActivityDefinitionKind> getKindElement() { 4106 if (this.kind == null) 4107 if (Configuration.errorOnAutoCreate()) 4108 throw new Error("Attempt to auto-create ActivityDefinition.kind"); 4109 else if (Configuration.doAutoCreate()) 4110 this.kind = new Enumeration<ActivityDefinitionKind>(new ActivityDefinitionKindEnumFactory()); // bb 4111 return this.kind; 4112 } 4113 4114 public boolean hasKindElement() { 4115 return this.kind != null && !this.kind.isEmpty(); 4116 } 4117 4118 public boolean hasKind() { 4119 return this.kind != null && !this.kind.isEmpty(); 4120 } 4121 4122 /** 4123 * @param value {@link #kind} (A description of the kind of resource the activity definition is representing. For example, a MedicationRequest, a ProcedureRequest, or a CommunicationRequest. Typically, but not always, this is a Request resource.). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 4124 */ 4125 public ActivityDefinition setKindElement(Enumeration<ActivityDefinitionKind> value) { 4126 this.kind = value; 4127 return this; 4128 } 4129 4130 /** 4131 * @return A description of the kind of resource the activity definition is representing. For example, a MedicationRequest, a ProcedureRequest, or a CommunicationRequest. Typically, but not always, this is a Request resource. 4132 */ 4133 public ActivityDefinitionKind getKind() { 4134 return this.kind == null ? null : this.kind.getValue(); 4135 } 4136 4137 /** 4138 * @param value A description of the kind of resource the activity definition is representing. For example, a MedicationRequest, a ProcedureRequest, or a CommunicationRequest. Typically, but not always, this is a Request resource. 4139 */ 4140 public ActivityDefinition setKind(ActivityDefinitionKind value) { 4141 if (value == null) 4142 this.kind = null; 4143 else { 4144 if (this.kind == null) 4145 this.kind = new Enumeration<ActivityDefinitionKind>(new ActivityDefinitionKindEnumFactory()); 4146 this.kind.setValue(value); 4147 } 4148 return this; 4149 } 4150 4151 /** 4152 * @return {@link #code} (Detailed description of the type of activity; e.g. What lab test, what procedure, what kind of encounter.) 4153 */ 4154 public CodeableConcept getCode() { 4155 if (this.code == null) 4156 if (Configuration.errorOnAutoCreate()) 4157 throw new Error("Attempt to auto-create ActivityDefinition.code"); 4158 else if (Configuration.doAutoCreate()) 4159 this.code = new CodeableConcept(); // cc 4160 return this.code; 4161 } 4162 4163 public boolean hasCode() { 4164 return this.code != null && !this.code.isEmpty(); 4165 } 4166 4167 /** 4168 * @param value {@link #code} (Detailed description of the type of activity; e.g. What lab test, what procedure, what kind of encounter.) 4169 */ 4170 public ActivityDefinition setCode(CodeableConcept value) { 4171 this.code = value; 4172 return this; 4173 } 4174 4175 /** 4176 * @return {@link #timing} (The period, timing or frequency upon which the described activity is to occur.) 4177 */ 4178 public Type getTiming() { 4179 return this.timing; 4180 } 4181 4182 /** 4183 * @return {@link #timing} (The period, timing or frequency upon which the described activity is to occur.) 4184 */ 4185 public Timing getTimingTiming() throws FHIRException { 4186 if (this.timing == null) 4187 return null; 4188 if (!(this.timing instanceof Timing)) 4189 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.timing.getClass().getName()+" was encountered"); 4190 return (Timing) this.timing; 4191 } 4192 4193 public boolean hasTimingTiming() { 4194 return this != null && this.timing instanceof Timing; 4195 } 4196 4197 /** 4198 * @return {@link #timing} (The period, timing or frequency upon which the described activity is to occur.) 4199 */ 4200 public DateTimeType getTimingDateTimeType() throws FHIRException { 4201 if (this.timing == null) 4202 return null; 4203 if (!(this.timing instanceof DateTimeType)) 4204 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.timing.getClass().getName()+" was encountered"); 4205 return (DateTimeType) this.timing; 4206 } 4207 4208 public boolean hasTimingDateTimeType() { 4209 return this != null && this.timing instanceof DateTimeType; 4210 } 4211 4212 /** 4213 * @return {@link #timing} (The period, timing or frequency upon which the described activity is to occur.) 4214 */ 4215 public Period getTimingPeriod() throws FHIRException { 4216 if (this.timing == null) 4217 return null; 4218 if (!(this.timing instanceof Period)) 4219 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.timing.getClass().getName()+" was encountered"); 4220 return (Period) this.timing; 4221 } 4222 4223 public boolean hasTimingPeriod() { 4224 return this != null && this.timing instanceof Period; 4225 } 4226 4227 /** 4228 * @return {@link #timing} (The period, timing or frequency upon which the described activity is to occur.) 4229 */ 4230 public Range getTimingRange() throws FHIRException { 4231 if (this.timing == null) 4232 return null; 4233 if (!(this.timing instanceof Range)) 4234 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.timing.getClass().getName()+" was encountered"); 4235 return (Range) this.timing; 4236 } 4237 4238 public boolean hasTimingRange() { 4239 return this != null && this.timing instanceof Range; 4240 } 4241 4242 public boolean hasTiming() { 4243 return this.timing != null && !this.timing.isEmpty(); 4244 } 4245 4246 /** 4247 * @param value {@link #timing} (The period, timing or frequency upon which the described activity is to occur.) 4248 */ 4249 public ActivityDefinition setTiming(Type value) throws FHIRFormatError { 4250 if (value != null && !(value instanceof Timing || value instanceof DateTimeType || value instanceof Period || value instanceof Range)) 4251 throw new FHIRFormatError("Not the right type for ActivityDefinition.timing[x]: "+value.fhirType()); 4252 this.timing = value; 4253 return this; 4254 } 4255 4256 /** 4257 * @return {@link #location} (Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc.) 4258 */ 4259 public Reference getLocation() { 4260 if (this.location == null) 4261 if (Configuration.errorOnAutoCreate()) 4262 throw new Error("Attempt to auto-create ActivityDefinition.location"); 4263 else if (Configuration.doAutoCreate()) 4264 this.location = new Reference(); // cc 4265 return this.location; 4266 } 4267 4268 public boolean hasLocation() { 4269 return this.location != null && !this.location.isEmpty(); 4270 } 4271 4272 /** 4273 * @param value {@link #location} (Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc.) 4274 */ 4275 public ActivityDefinition setLocation(Reference value) { 4276 this.location = value; 4277 return this; 4278 } 4279 4280 /** 4281 * @return {@link #location} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc.) 4282 */ 4283 public Location getLocationTarget() { 4284 if (this.locationTarget == null) 4285 if (Configuration.errorOnAutoCreate()) 4286 throw new Error("Attempt to auto-create ActivityDefinition.location"); 4287 else if (Configuration.doAutoCreate()) 4288 this.locationTarget = new Location(); // aa 4289 return this.locationTarget; 4290 } 4291 4292 /** 4293 * @param value {@link #location} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc.) 4294 */ 4295 public ActivityDefinition setLocationTarget(Location value) { 4296 this.locationTarget = value; 4297 return this; 4298 } 4299 4300 /** 4301 * @return {@link #participant} (Indicates who should participate in performing the action described.) 4302 */ 4303 public List<ActivityDefinitionParticipantComponent> getParticipant() { 4304 if (this.participant == null) 4305 this.participant = new ArrayList<ActivityDefinitionParticipantComponent>(); 4306 return this.participant; 4307 } 4308 4309 /** 4310 * @return Returns a reference to <code>this</code> for easy method chaining 4311 */ 4312 public ActivityDefinition setParticipant(List<ActivityDefinitionParticipantComponent> theParticipant) { 4313 this.participant = theParticipant; 4314 return this; 4315 } 4316 4317 public boolean hasParticipant() { 4318 if (this.participant == null) 4319 return false; 4320 for (ActivityDefinitionParticipantComponent item : this.participant) 4321 if (!item.isEmpty()) 4322 return true; 4323 return false; 4324 } 4325 4326 public ActivityDefinitionParticipantComponent addParticipant() { //3 4327 ActivityDefinitionParticipantComponent t = new ActivityDefinitionParticipantComponent(); 4328 if (this.participant == null) 4329 this.participant = new ArrayList<ActivityDefinitionParticipantComponent>(); 4330 this.participant.add(t); 4331 return t; 4332 } 4333 4334 public ActivityDefinition addParticipant(ActivityDefinitionParticipantComponent t) { //3 4335 if (t == null) 4336 return this; 4337 if (this.participant == null) 4338 this.participant = new ArrayList<ActivityDefinitionParticipantComponent>(); 4339 this.participant.add(t); 4340 return this; 4341 } 4342 4343 /** 4344 * @return The first repetition of repeating field {@link #participant}, creating it if it does not already exist 4345 */ 4346 public ActivityDefinitionParticipantComponent getParticipantFirstRep() { 4347 if (getParticipant().isEmpty()) { 4348 addParticipant(); 4349 } 4350 return getParticipant().get(0); 4351 } 4352 4353 /** 4354 * @return {@link #product} (Identifies the food, drug or other product being consumed or supplied in the activity.) 4355 */ 4356 public Type getProduct() { 4357 return this.product; 4358 } 4359 4360 /** 4361 * @return {@link #product} (Identifies the food, drug or other product being consumed or supplied in the activity.) 4362 */ 4363 public Reference getProductReference() throws FHIRException { 4364 if (this.product == null) 4365 return null; 4366 if (!(this.product instanceof Reference)) 4367 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.product.getClass().getName()+" was encountered"); 4368 return (Reference) this.product; 4369 } 4370 4371 public boolean hasProductReference() { 4372 return this != null && this.product instanceof Reference; 4373 } 4374 4375 /** 4376 * @return {@link #product} (Identifies the food, drug or other product being consumed or supplied in the activity.) 4377 */ 4378 public CodeableConcept getProductCodeableConcept() throws FHIRException { 4379 if (this.product == null) 4380 return null; 4381 if (!(this.product instanceof CodeableConcept)) 4382 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.product.getClass().getName()+" was encountered"); 4383 return (CodeableConcept) this.product; 4384 } 4385 4386 public boolean hasProductCodeableConcept() { 4387 return this != null && this.product instanceof CodeableConcept; 4388 } 4389 4390 public boolean hasProduct() { 4391 return this.product != null && !this.product.isEmpty(); 4392 } 4393 4394 /** 4395 * @param value {@link #product} (Identifies the food, drug or other product being consumed or supplied in the activity.) 4396 */ 4397 public ActivityDefinition setProduct(Type value) throws FHIRFormatError { 4398 if (value != null && !(value instanceof Reference || value instanceof CodeableConcept)) 4399 throw new FHIRFormatError("Not the right type for ActivityDefinition.product[x]: "+value.fhirType()); 4400 this.product = value; 4401 return this; 4402 } 4403 4404 /** 4405 * @return {@link #quantity} (Identifies the quantity expected to be consumed at once (per dose, per meal, etc.).) 4406 */ 4407 public SimpleQuantity getQuantity() { 4408 if (this.quantity == null) 4409 if (Configuration.errorOnAutoCreate()) 4410 throw new Error("Attempt to auto-create ActivityDefinition.quantity"); 4411 else if (Configuration.doAutoCreate()) 4412 this.quantity = new SimpleQuantity(); // cc 4413 return this.quantity; 4414 } 4415 4416 public boolean hasQuantity() { 4417 return this.quantity != null && !this.quantity.isEmpty(); 4418 } 4419 4420 /** 4421 * @param value {@link #quantity} (Identifies the quantity expected to be consumed at once (per dose, per meal, etc.).) 4422 */ 4423 public ActivityDefinition setQuantity(SimpleQuantity value) { 4424 this.quantity = value; 4425 return this; 4426 } 4427 4428 /** 4429 * @return {@link #dosage} (Provides detailed dosage instructions in the same way that they are described for MedicationRequest resources.) 4430 */ 4431 public List<Dosage> getDosage() { 4432 if (this.dosage == null) 4433 this.dosage = new ArrayList<Dosage>(); 4434 return this.dosage; 4435 } 4436 4437 /** 4438 * @return Returns a reference to <code>this</code> for easy method chaining 4439 */ 4440 public ActivityDefinition setDosage(List<Dosage> theDosage) { 4441 this.dosage = theDosage; 4442 return this; 4443 } 4444 4445 public boolean hasDosage() { 4446 if (this.dosage == null) 4447 return false; 4448 for (Dosage item : this.dosage) 4449 if (!item.isEmpty()) 4450 return true; 4451 return false; 4452 } 4453 4454 public Dosage addDosage() { //3 4455 Dosage t = new Dosage(); 4456 if (this.dosage == null) 4457 this.dosage = new ArrayList<Dosage>(); 4458 this.dosage.add(t); 4459 return t; 4460 } 4461 4462 public ActivityDefinition addDosage(Dosage t) { //3 4463 if (t == null) 4464 return this; 4465 if (this.dosage == null) 4466 this.dosage = new ArrayList<Dosage>(); 4467 this.dosage.add(t); 4468 return this; 4469 } 4470 4471 /** 4472 * @return The first repetition of repeating field {@link #dosage}, creating it if it does not already exist 4473 */ 4474 public Dosage getDosageFirstRep() { 4475 if (getDosage().isEmpty()) { 4476 addDosage(); 4477 } 4478 return getDosage().get(0); 4479 } 4480 4481 /** 4482 * @return {@link #bodySite} (Indicates the sites on the subject's body where the procedure should be performed (I.e. the target sites).) 4483 */ 4484 public List<CodeableConcept> getBodySite() { 4485 if (this.bodySite == null) 4486 this.bodySite = new ArrayList<CodeableConcept>(); 4487 return this.bodySite; 4488 } 4489 4490 /** 4491 * @return Returns a reference to <code>this</code> for easy method chaining 4492 */ 4493 public ActivityDefinition setBodySite(List<CodeableConcept> theBodySite) { 4494 this.bodySite = theBodySite; 4495 return this; 4496 } 4497 4498 public boolean hasBodySite() { 4499 if (this.bodySite == null) 4500 return false; 4501 for (CodeableConcept item : this.bodySite) 4502 if (!item.isEmpty()) 4503 return true; 4504 return false; 4505 } 4506 4507 public CodeableConcept addBodySite() { //3 4508 CodeableConcept t = new CodeableConcept(); 4509 if (this.bodySite == null) 4510 this.bodySite = new ArrayList<CodeableConcept>(); 4511 this.bodySite.add(t); 4512 return t; 4513 } 4514 4515 public ActivityDefinition addBodySite(CodeableConcept t) { //3 4516 if (t == null) 4517 return this; 4518 if (this.bodySite == null) 4519 this.bodySite = new ArrayList<CodeableConcept>(); 4520 this.bodySite.add(t); 4521 return this; 4522 } 4523 4524 /** 4525 * @return The first repetition of repeating field {@link #bodySite}, creating it if it does not already exist 4526 */ 4527 public CodeableConcept getBodySiteFirstRep() { 4528 if (getBodySite().isEmpty()) { 4529 addBodySite(); 4530 } 4531 return getBodySite().get(0); 4532 } 4533 4534 /** 4535 * @return {@link #transform} (A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.) 4536 */ 4537 public Reference getTransform() { 4538 if (this.transform == null) 4539 if (Configuration.errorOnAutoCreate()) 4540 throw new Error("Attempt to auto-create ActivityDefinition.transform"); 4541 else if (Configuration.doAutoCreate()) 4542 this.transform = new Reference(); // cc 4543 return this.transform; 4544 } 4545 4546 public boolean hasTransform() { 4547 return this.transform != null && !this.transform.isEmpty(); 4548 } 4549 4550 /** 4551 * @param value {@link #transform} (A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.) 4552 */ 4553 public ActivityDefinition setTransform(Reference value) { 4554 this.transform = value; 4555 return this; 4556 } 4557 4558 /** 4559 * @return {@link #transform} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.) 4560 */ 4561 public StructureMap getTransformTarget() { 4562 if (this.transformTarget == null) 4563 if (Configuration.errorOnAutoCreate()) 4564 throw new Error("Attempt to auto-create ActivityDefinition.transform"); 4565 else if (Configuration.doAutoCreate()) 4566 this.transformTarget = new StructureMap(); // aa 4567 return this.transformTarget; 4568 } 4569 4570 /** 4571 * @param value {@link #transform} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.) 4572 */ 4573 public ActivityDefinition setTransformTarget(StructureMap value) { 4574 this.transformTarget = value; 4575 return this; 4576 } 4577 4578 /** 4579 * @return {@link #dynamicValue} (Dynamic values that will be evaluated to produce values for elements of the resulting resource. For example, if the dosage of a medication must be computed based on the patient's weight, a dynamic value would be used to specify an expression that calculated the weight, and the path on the intent resource that would contain the result.) 4580 */ 4581 public List<ActivityDefinitionDynamicValueComponent> getDynamicValue() { 4582 if (this.dynamicValue == null) 4583 this.dynamicValue = new ArrayList<ActivityDefinitionDynamicValueComponent>(); 4584 return this.dynamicValue; 4585 } 4586 4587 /** 4588 * @return Returns a reference to <code>this</code> for easy method chaining 4589 */ 4590 public ActivityDefinition setDynamicValue(List<ActivityDefinitionDynamicValueComponent> theDynamicValue) { 4591 this.dynamicValue = theDynamicValue; 4592 return this; 4593 } 4594 4595 public boolean hasDynamicValue() { 4596 if (this.dynamicValue == null) 4597 return false; 4598 for (ActivityDefinitionDynamicValueComponent item : this.dynamicValue) 4599 if (!item.isEmpty()) 4600 return true; 4601 return false; 4602 } 4603 4604 public ActivityDefinitionDynamicValueComponent addDynamicValue() { //3 4605 ActivityDefinitionDynamicValueComponent t = new ActivityDefinitionDynamicValueComponent(); 4606 if (this.dynamicValue == null) 4607 this.dynamicValue = new ArrayList<ActivityDefinitionDynamicValueComponent>(); 4608 this.dynamicValue.add(t); 4609 return t; 4610 } 4611 4612 public ActivityDefinition addDynamicValue(ActivityDefinitionDynamicValueComponent t) { //3 4613 if (t == null) 4614 return this; 4615 if (this.dynamicValue == null) 4616 this.dynamicValue = new ArrayList<ActivityDefinitionDynamicValueComponent>(); 4617 this.dynamicValue.add(t); 4618 return this; 4619 } 4620 4621 /** 4622 * @return The first repetition of repeating field {@link #dynamicValue}, creating it if it does not already exist 4623 */ 4624 public ActivityDefinitionDynamicValueComponent getDynamicValueFirstRep() { 4625 if (getDynamicValue().isEmpty()) { 4626 addDynamicValue(); 4627 } 4628 return getDynamicValue().get(0); 4629 } 4630 4631 protected void listChildren(List<Property> children) { 4632 super.listChildren(children); 4633 children.add(new Property("url", "uri", "An absolute URI that is used to identify this activity definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this activity definition is (or will be) published. The URL SHOULD include the major version of the activity definition. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url)); 4634 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this activity definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 4635 children.add(new Property("version", "string", "The identifier that is used to identify this version of the activity definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the activity definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets.", 0, 1, version)); 4636 children.add(new Property("name", "string", "A natural language name identifying the activity definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 4637 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the activity definition.", 0, 1, title)); 4638 children.add(new Property("status", "code", "The status of this activity definition. Enables tracking the life-cycle of the content.", 0, 1, status)); 4639 children.add(new Property("experimental", "boolean", "A boolean value to indicate that this activity definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental)); 4640 children.add(new Property("date", "dateTime", "The date (and optionally time) when the activity definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the activity definition changes.", 0, 1, date)); 4641 children.add(new Property("publisher", "string", "The name of the individual or organization that published the activity definition.", 0, 1, publisher)); 4642 children.add(new Property("description", "markdown", "A free text natural language description of the activity definition from a consumer's perspective.", 0, 1, description)); 4643 children.add(new Property("purpose", "markdown", "Explaination of why this activity definition is needed and why it has been designed as it has.", 0, 1, purpose)); 4644 children.add(new Property("usage", "string", "A detailed description of how the asset is used from a clinical perspective.", 0, 1, usage)); 4645 children.add(new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate)); 4646 children.add(new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date.", 0, 1, lastReviewDate)); 4647 children.add(new Property("effectivePeriod", "Period", "The period during which the activity definition content was or is planned to be in active use.", 0, 1, effectivePeriod)); 4648 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate activity definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 4649 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the activity definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 4650 children.add(new Property("topic", "CodeableConcept", "Descriptive topics related to the content of the activity. Topics provide a high-level categorization of the activity that can be useful for filtering and searching.", 0, java.lang.Integer.MAX_VALUE, topic)); 4651 children.add(new Property("contributor", "Contributor", "A contributor to the content of the asset, including authors, editors, reviewers, and endorsers.", 0, java.lang.Integer.MAX_VALUE, contributor)); 4652 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 4653 children.add(new Property("copyright", "markdown", "A copyright statement relating to the activity definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the activity definition.", 0, 1, copyright)); 4654 children.add(new Property("relatedArtifact", "RelatedArtifact", "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact)); 4655 children.add(new Property("library", "Reference(Library)", "A reference to a Library resource containing any formal logic used by the asset.", 0, java.lang.Integer.MAX_VALUE, library)); 4656 children.add(new Property("kind", "code", "A description of the kind of resource the activity definition is representing. For example, a MedicationRequest, a ProcedureRequest, or a CommunicationRequest. Typically, but not always, this is a Request resource.", 0, 1, kind)); 4657 children.add(new Property("code", "CodeableConcept", "Detailed description of the type of activity; e.g. What lab test, what procedure, what kind of encounter.", 0, 1, code)); 4658 children.add(new Property("timing[x]", "Timing|dateTime|Period|Range", "The period, timing or frequency upon which the described activity is to occur.", 0, 1, timing)); 4659 children.add(new Property("location", "Reference(Location)", "Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc.", 0, 1, location)); 4660 children.add(new Property("participant", "", "Indicates who should participate in performing the action described.", 0, java.lang.Integer.MAX_VALUE, participant)); 4661 children.add(new Property("product[x]", "Reference(Medication|Substance)|CodeableConcept", "Identifies the food, drug or other product being consumed or supplied in the activity.", 0, 1, product)); 4662 children.add(new Property("quantity", "SimpleQuantity", "Identifies the quantity expected to be consumed at once (per dose, per meal, etc.).", 0, 1, quantity)); 4663 children.add(new Property("dosage", "Dosage", "Provides detailed dosage instructions in the same way that they are described for MedicationRequest resources.", 0, java.lang.Integer.MAX_VALUE, dosage)); 4664 children.add(new Property("bodySite", "CodeableConcept", "Indicates the sites on the subject's body where the procedure should be performed (I.e. the target sites).", 0, java.lang.Integer.MAX_VALUE, bodySite)); 4665 children.add(new Property("transform", "Reference(StructureMap)", "A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.", 0, 1, transform)); 4666 children.add(new Property("dynamicValue", "", "Dynamic values that will be evaluated to produce values for elements of the resulting resource. For example, if the dosage of a medication must be computed based on the patient's weight, a dynamic value would be used to specify an expression that calculated the weight, and the path on the intent resource that would contain the result.", 0, java.lang.Integer.MAX_VALUE, dynamicValue)); 4667 } 4668 4669 @Override 4670 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4671 switch (_hash) { 4672 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this activity definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this activity definition is (or will be) published. The URL SHOULD include the major version of the activity definition. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url); 4673 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this activity definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 4674 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the activity definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the activity definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets.", 0, 1, version); 4675 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the activity definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 4676 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the activity definition.", 0, 1, title); 4677 case -892481550: /*status*/ return new Property("status", "code", "The status of this activity definition. Enables tracking the life-cycle of the content.", 0, 1, status); 4678 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A boolean value to indicate that this activity definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental); 4679 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the activity definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the activity definition changes.", 0, 1, date); 4680 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the individual or organization that published the activity definition.", 0, 1, publisher); 4681 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the activity definition from a consumer's perspective.", 0, 1, description); 4682 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explaination of why this activity definition is needed and why it has been designed as it has.", 0, 1, purpose); 4683 case 111574433: /*usage*/ return new Property("usage", "string", "A detailed description of how the asset is used from a clinical perspective.", 0, 1, usage); 4684 case 223539345: /*approvalDate*/ return new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate); 4685 case -1687512484: /*lastReviewDate*/ return new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date.", 0, 1, lastReviewDate); 4686 case -403934648: /*effectivePeriod*/ return new Property("effectivePeriod", "Period", "The period during which the activity definition content was or is planned to be in active use.", 0, 1, effectivePeriod); 4687 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate activity definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 4688 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the activity definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 4689 case 110546223: /*topic*/ return new Property("topic", "CodeableConcept", "Descriptive topics related to the content of the activity. Topics provide a high-level categorization of the activity that can be useful for filtering and searching.", 0, java.lang.Integer.MAX_VALUE, topic); 4690 case -1895276325: /*contributor*/ return new Property("contributor", "Contributor", "A contributor to the content of the asset, including authors, editors, reviewers, and endorsers.", 0, java.lang.Integer.MAX_VALUE, contributor); 4691 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 4692 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the activity definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the activity definition.", 0, 1, copyright); 4693 case 666807069: /*relatedArtifact*/ return new Property("relatedArtifact", "RelatedArtifact", "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact); 4694 case 166208699: /*library*/ return new Property("library", "Reference(Library)", "A reference to a Library resource containing any formal logic used by the asset.", 0, java.lang.Integer.MAX_VALUE, library); 4695 case 3292052: /*kind*/ return new Property("kind", "code", "A description of the kind of resource the activity definition is representing. For example, a MedicationRequest, a ProcedureRequest, or a CommunicationRequest. Typically, but not always, this is a Request resource.", 0, 1, kind); 4696 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Detailed description of the type of activity; e.g. What lab test, what procedure, what kind of encounter.", 0, 1, code); 4697 case 164632566: /*timing[x]*/ return new Property("timing[x]", "Timing|dateTime|Period|Range", "The period, timing or frequency upon which the described activity is to occur.", 0, 1, timing); 4698 case -873664438: /*timing*/ return new Property("timing[x]", "Timing|dateTime|Period|Range", "The period, timing or frequency upon which the described activity is to occur.", 0, 1, timing); 4699 case -497554124: /*timingTiming*/ return new Property("timing[x]", "Timing|dateTime|Period|Range", "The period, timing or frequency upon which the described activity is to occur.", 0, 1, timing); 4700 case -1837458939: /*timingDateTime*/ return new Property("timing[x]", "Timing|dateTime|Period|Range", "The period, timing or frequency upon which the described activity is to occur.", 0, 1, timing); 4701 case -615615829: /*timingPeriod*/ return new Property("timing[x]", "Timing|dateTime|Period|Range", "The period, timing or frequency upon which the described activity is to occur.", 0, 1, timing); 4702 case -710871277: /*timingRange*/ return new Property("timing[x]", "Timing|dateTime|Period|Range", "The period, timing or frequency upon which the described activity is to occur.", 0, 1, timing); 4703 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc.", 0, 1, location); 4704 case 767422259: /*participant*/ return new Property("participant", "", "Indicates who should participate in performing the action described.", 0, java.lang.Integer.MAX_VALUE, participant); 4705 case 1753005361: /*product[x]*/ return new Property("product[x]", "Reference(Medication|Substance)|CodeableConcept", "Identifies the food, drug or other product being consumed or supplied in the activity.", 0, 1, product); 4706 case -309474065: /*product*/ return new Property("product[x]", "Reference(Medication|Substance)|CodeableConcept", "Identifies the food, drug or other product being consumed or supplied in the activity.", 0, 1, product); 4707 case -669667556: /*productReference*/ return new Property("product[x]", "Reference(Medication|Substance)|CodeableConcept", "Identifies the food, drug or other product being consumed or supplied in the activity.", 0, 1, product); 4708 case 906854066: /*productCodeableConcept*/ return new Property("product[x]", "Reference(Medication|Substance)|CodeableConcept", "Identifies the food, drug or other product being consumed or supplied in the activity.", 0, 1, product); 4709 case -1285004149: /*quantity*/ return new Property("quantity", "SimpleQuantity", "Identifies the quantity expected to be consumed at once (per dose, per meal, etc.).", 0, 1, quantity); 4710 case -1326018889: /*dosage*/ return new Property("dosage", "Dosage", "Provides detailed dosage instructions in the same way that they are described for MedicationRequest resources.", 0, java.lang.Integer.MAX_VALUE, dosage); 4711 case 1702620169: /*bodySite*/ return new Property("bodySite", "CodeableConcept", "Indicates the sites on the subject's body where the procedure should be performed (I.e. the target sites).", 0, java.lang.Integer.MAX_VALUE, bodySite); 4712 case 1052666732: /*transform*/ return new Property("transform", "Reference(StructureMap)", "A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.", 0, 1, transform); 4713 case 572625010: /*dynamicValue*/ return new Property("dynamicValue", "", "Dynamic values that will be evaluated to produce values for elements of the resulting resource. For example, if the dosage of a medication must be computed based on the patient's weight, a dynamic value would be used to specify an expression that calculated the weight, and the path on the intent resource that would contain the result.", 0, java.lang.Integer.MAX_VALUE, dynamicValue); 4714 default: return super.getNamedProperty(_hash, _name, _checkValid); 4715 } 4716 4717 } 4718 4719 @Override 4720 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4721 switch (hash) { 4722 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 4723 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 4724 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 4725 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 4726 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 4727 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 4728 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 4729 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 4730 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 4731 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 4732 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 4733 case 111574433: /*usage*/ return this.usage == null ? new Base[0] : new Base[] {this.usage}; // StringType 4734 case 223539345: /*approvalDate*/ return this.approvalDate == null ? new Base[0] : new Base[] {this.approvalDate}; // DateType 4735 case -1687512484: /*lastReviewDate*/ return this.lastReviewDate == null ? new Base[0] : new Base[] {this.lastReviewDate}; // DateType 4736 case -403934648: /*effectivePeriod*/ return this.effectivePeriod == null ? new Base[0] : new Base[] {this.effectivePeriod}; // Period 4737 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 4738 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 4739 case 110546223: /*topic*/ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // CodeableConcept 4740 case -1895276325: /*contributor*/ return this.contributor == null ? new Base[0] : this.contributor.toArray(new Base[this.contributor.size()]); // Contributor 4741 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 4742 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 4743 case 666807069: /*relatedArtifact*/ return this.relatedArtifact == null ? new Base[0] : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 4744 case 166208699: /*library*/ return this.library == null ? new Base[0] : this.library.toArray(new Base[this.library.size()]); // Reference 4745 case 3292052: /*kind*/ return this.kind == null ? new Base[0] : new Base[] {this.kind}; // Enumeration<ActivityDefinitionKind> 4746 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 4747 case -873664438: /*timing*/ return this.timing == null ? new Base[0] : new Base[] {this.timing}; // Type 4748 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Reference 4749 case 767422259: /*participant*/ return this.participant == null ? new Base[0] : this.participant.toArray(new Base[this.participant.size()]); // ActivityDefinitionParticipantComponent 4750 case -309474065: /*product*/ return this.product == null ? new Base[0] : new Base[] {this.product}; // Type 4751 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // SimpleQuantity 4752 case -1326018889: /*dosage*/ return this.dosage == null ? new Base[0] : this.dosage.toArray(new Base[this.dosage.size()]); // Dosage 4753 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : this.bodySite.toArray(new Base[this.bodySite.size()]); // CodeableConcept 4754 case 1052666732: /*transform*/ return this.transform == null ? new Base[0] : new Base[] {this.transform}; // Reference 4755 case 572625010: /*dynamicValue*/ return this.dynamicValue == null ? new Base[0] : this.dynamicValue.toArray(new Base[this.dynamicValue.size()]); // ActivityDefinitionDynamicValueComponent 4756 default: return super.getProperty(hash, name, checkValid); 4757 } 4758 4759 } 4760 4761 @Override 4762 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4763 switch (hash) { 4764 case 116079: // url 4765 this.url = castToUri(value); // UriType 4766 return value; 4767 case -1618432855: // identifier 4768 this.getIdentifier().add(castToIdentifier(value)); // Identifier 4769 return value; 4770 case 351608024: // version 4771 this.version = castToString(value); // StringType 4772 return value; 4773 case 3373707: // name 4774 this.name = castToString(value); // StringType 4775 return value; 4776 case 110371416: // title 4777 this.title = castToString(value); // StringType 4778 return value; 4779 case -892481550: // status 4780 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 4781 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4782 return value; 4783 case -404562712: // experimental 4784 this.experimental = castToBoolean(value); // BooleanType 4785 return value; 4786 case 3076014: // date 4787 this.date = castToDateTime(value); // DateTimeType 4788 return value; 4789 case 1447404028: // publisher 4790 this.publisher = castToString(value); // StringType 4791 return value; 4792 case -1724546052: // description 4793 this.description = castToMarkdown(value); // MarkdownType 4794 return value; 4795 case -220463842: // purpose 4796 this.purpose = castToMarkdown(value); // MarkdownType 4797 return value; 4798 case 111574433: // usage 4799 this.usage = castToString(value); // StringType 4800 return value; 4801 case 223539345: // approvalDate 4802 this.approvalDate = castToDate(value); // DateType 4803 return value; 4804 case -1687512484: // lastReviewDate 4805 this.lastReviewDate = castToDate(value); // DateType 4806 return value; 4807 case -403934648: // effectivePeriod 4808 this.effectivePeriod = castToPeriod(value); // Period 4809 return value; 4810 case -669707736: // useContext 4811 this.getUseContext().add(castToUsageContext(value)); // UsageContext 4812 return value; 4813 case -507075711: // jurisdiction 4814 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 4815 return value; 4816 case 110546223: // topic 4817 this.getTopic().add(castToCodeableConcept(value)); // CodeableConcept 4818 return value; 4819 case -1895276325: // contributor 4820 this.getContributor().add(castToContributor(value)); // Contributor 4821 return value; 4822 case 951526432: // contact 4823 this.getContact().add(castToContactDetail(value)); // ContactDetail 4824 return value; 4825 case 1522889671: // copyright 4826 this.copyright = castToMarkdown(value); // MarkdownType 4827 return value; 4828 case 666807069: // relatedArtifact 4829 this.getRelatedArtifact().add(castToRelatedArtifact(value)); // RelatedArtifact 4830 return value; 4831 case 166208699: // library 4832 this.getLibrary().add(castToReference(value)); // Reference 4833 return value; 4834 case 3292052: // kind 4835 value = new ActivityDefinitionKindEnumFactory().fromType(castToCode(value)); 4836 this.kind = (Enumeration) value; // Enumeration<ActivityDefinitionKind> 4837 return value; 4838 case 3059181: // code 4839 this.code = castToCodeableConcept(value); // CodeableConcept 4840 return value; 4841 case -873664438: // timing 4842 this.timing = castToType(value); // Type 4843 return value; 4844 case 1901043637: // location 4845 this.location = castToReference(value); // Reference 4846 return value; 4847 case 767422259: // participant 4848 this.getParticipant().add((ActivityDefinitionParticipantComponent) value); // ActivityDefinitionParticipantComponent 4849 return value; 4850 case -309474065: // product 4851 this.product = castToType(value); // Type 4852 return value; 4853 case -1285004149: // quantity 4854 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 4855 return value; 4856 case -1326018889: // dosage 4857 this.getDosage().add(castToDosage(value)); // Dosage 4858 return value; 4859 case 1702620169: // bodySite 4860 this.getBodySite().add(castToCodeableConcept(value)); // CodeableConcept 4861 return value; 4862 case 1052666732: // transform 4863 this.transform = castToReference(value); // Reference 4864 return value; 4865 case 572625010: // dynamicValue 4866 this.getDynamicValue().add((ActivityDefinitionDynamicValueComponent) value); // ActivityDefinitionDynamicValueComponent 4867 return value; 4868 default: return super.setProperty(hash, name, value); 4869 } 4870 4871 } 4872 4873 @Override 4874 public Base setProperty(String name, Base value) throws FHIRException { 4875 if (name.equals("url")) { 4876 this.url = castToUri(value); // UriType 4877 } else if (name.equals("identifier")) { 4878 this.getIdentifier().add(castToIdentifier(value)); 4879 } else if (name.equals("version")) { 4880 this.version = castToString(value); // StringType 4881 } else if (name.equals("name")) { 4882 this.name = castToString(value); // StringType 4883 } else if (name.equals("title")) { 4884 this.title = castToString(value); // StringType 4885 } else if (name.equals("status")) { 4886 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 4887 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4888 } else if (name.equals("experimental")) { 4889 this.experimental = castToBoolean(value); // BooleanType 4890 } else if (name.equals("date")) { 4891 this.date = castToDateTime(value); // DateTimeType 4892 } else if (name.equals("publisher")) { 4893 this.publisher = castToString(value); // StringType 4894 } else if (name.equals("description")) { 4895 this.description = castToMarkdown(value); // MarkdownType 4896 } else if (name.equals("purpose")) { 4897 this.purpose = castToMarkdown(value); // MarkdownType 4898 } else if (name.equals("usage")) { 4899 this.usage = castToString(value); // StringType 4900 } else if (name.equals("approvalDate")) { 4901 this.approvalDate = castToDate(value); // DateType 4902 } else if (name.equals("lastReviewDate")) { 4903 this.lastReviewDate = castToDate(value); // DateType 4904 } else if (name.equals("effectivePeriod")) { 4905 this.effectivePeriod = castToPeriod(value); // Period 4906 } else if (name.equals("useContext")) { 4907 this.getUseContext().add(castToUsageContext(value)); 4908 } else if (name.equals("jurisdiction")) { 4909 this.getJurisdiction().add(castToCodeableConcept(value)); 4910 } else if (name.equals("topic")) { 4911 this.getTopic().add(castToCodeableConcept(value)); 4912 } else if (name.equals("contributor")) { 4913 this.getContributor().add(castToContributor(value)); 4914 } else if (name.equals("contact")) { 4915 this.getContact().add(castToContactDetail(value)); 4916 } else if (name.equals("copyright")) { 4917 this.copyright = castToMarkdown(value); // MarkdownType 4918 } else if (name.equals("relatedArtifact")) { 4919 this.getRelatedArtifact().add(castToRelatedArtifact(value)); 4920 } else if (name.equals("library")) { 4921 this.getLibrary().add(castToReference(value)); 4922 } else if (name.equals("kind")) { 4923 value = new ActivityDefinitionKindEnumFactory().fromType(castToCode(value)); 4924 this.kind = (Enumeration) value; // Enumeration<ActivityDefinitionKind> 4925 } else if (name.equals("code")) { 4926 this.code = castToCodeableConcept(value); // CodeableConcept 4927 } else if (name.equals("timing[x]")) { 4928 this.timing = castToType(value); // Type 4929 } else if (name.equals("location")) { 4930 this.location = castToReference(value); // Reference 4931 } else if (name.equals("participant")) { 4932 this.getParticipant().add((ActivityDefinitionParticipantComponent) value); 4933 } else if (name.equals("product[x]")) { 4934 this.product = castToType(value); // Type 4935 } else if (name.equals("quantity")) { 4936 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 4937 } else if (name.equals("dosage")) { 4938 this.getDosage().add(castToDosage(value)); 4939 } else if (name.equals("bodySite")) { 4940 this.getBodySite().add(castToCodeableConcept(value)); 4941 } else if (name.equals("transform")) { 4942 this.transform = castToReference(value); // Reference 4943 } else if (name.equals("dynamicValue")) { 4944 this.getDynamicValue().add((ActivityDefinitionDynamicValueComponent) value); 4945 } else 4946 return super.setProperty(name, value); 4947 return value; 4948 } 4949 4950 @Override 4951 public Base makeProperty(int hash, String name) throws FHIRException { 4952 switch (hash) { 4953 case 116079: return getUrlElement(); 4954 case -1618432855: return addIdentifier(); 4955 case 351608024: return getVersionElement(); 4956 case 3373707: return getNameElement(); 4957 case 110371416: return getTitleElement(); 4958 case -892481550: return getStatusElement(); 4959 case -404562712: return getExperimentalElement(); 4960 case 3076014: return getDateElement(); 4961 case 1447404028: return getPublisherElement(); 4962 case -1724546052: return getDescriptionElement(); 4963 case -220463842: return getPurposeElement(); 4964 case 111574433: return getUsageElement(); 4965 case 223539345: return getApprovalDateElement(); 4966 case -1687512484: return getLastReviewDateElement(); 4967 case -403934648: return getEffectivePeriod(); 4968 case -669707736: return addUseContext(); 4969 case -507075711: return addJurisdiction(); 4970 case 110546223: return addTopic(); 4971 case -1895276325: return addContributor(); 4972 case 951526432: return addContact(); 4973 case 1522889671: return getCopyrightElement(); 4974 case 666807069: return addRelatedArtifact(); 4975 case 166208699: return addLibrary(); 4976 case 3292052: return getKindElement(); 4977 case 3059181: return getCode(); 4978 case 164632566: return getTiming(); 4979 case -873664438: return getTiming(); 4980 case 1901043637: return getLocation(); 4981 case 767422259: return addParticipant(); 4982 case 1753005361: return getProduct(); 4983 case -309474065: return getProduct(); 4984 case -1285004149: return getQuantity(); 4985 case -1326018889: return addDosage(); 4986 case 1702620169: return addBodySite(); 4987 case 1052666732: return getTransform(); 4988 case 572625010: return addDynamicValue(); 4989 default: return super.makeProperty(hash, name); 4990 } 4991 4992 } 4993 4994 @Override 4995 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4996 switch (hash) { 4997 case 116079: /*url*/ return new String[] {"uri"}; 4998 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 4999 case 351608024: /*version*/ return new String[] {"string"}; 5000 case 3373707: /*name*/ return new String[] {"string"}; 5001 case 110371416: /*title*/ return new String[] {"string"}; 5002 case -892481550: /*status*/ return new String[] {"code"}; 5003 case -404562712: /*experimental*/ return new String[] {"boolean"}; 5004 case 3076014: /*date*/ return new String[] {"dateTime"}; 5005 case 1447404028: /*publisher*/ return new String[] {"string"}; 5006 case -1724546052: /*description*/ return new String[] {"markdown"}; 5007 case -220463842: /*purpose*/ return new String[] {"markdown"}; 5008 case 111574433: /*usage*/ return new String[] {"string"}; 5009 case 223539345: /*approvalDate*/ return new String[] {"date"}; 5010 case -1687512484: /*lastReviewDate*/ return new String[] {"date"}; 5011 case -403934648: /*effectivePeriod*/ return new String[] {"Period"}; 5012 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 5013 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 5014 case 110546223: /*topic*/ return new String[] {"CodeableConcept"}; 5015 case -1895276325: /*contributor*/ return new String[] {"Contributor"}; 5016 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 5017 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 5018 case 666807069: /*relatedArtifact*/ return new String[] {"RelatedArtifact"}; 5019 case 166208699: /*library*/ return new String[] {"Reference"}; 5020 case 3292052: /*kind*/ return new String[] {"code"}; 5021 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 5022 case -873664438: /*timing*/ return new String[] {"Timing", "dateTime", "Period", "Range"}; 5023 case 1901043637: /*location*/ return new String[] {"Reference"}; 5024 case 767422259: /*participant*/ return new String[] {}; 5025 case -309474065: /*product*/ return new String[] {"Reference", "CodeableConcept"}; 5026 case -1285004149: /*quantity*/ return new String[] {"SimpleQuantity"}; 5027 case -1326018889: /*dosage*/ return new String[] {"Dosage"}; 5028 case 1702620169: /*bodySite*/ return new String[] {"CodeableConcept"}; 5029 case 1052666732: /*transform*/ return new String[] {"Reference"}; 5030 case 572625010: /*dynamicValue*/ return new String[] {}; 5031 default: return super.getTypesForProperty(hash, name); 5032 } 5033 5034 } 5035 5036 @Override 5037 public Base addChild(String name) throws FHIRException { 5038 if (name.equals("url")) { 5039 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.url"); 5040 } 5041 else if (name.equals("identifier")) { 5042 return addIdentifier(); 5043 } 5044 else if (name.equals("version")) { 5045 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.version"); 5046 } 5047 else if (name.equals("name")) { 5048 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.name"); 5049 } 5050 else if (name.equals("title")) { 5051 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.title"); 5052 } 5053 else if (name.equals("status")) { 5054 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.status"); 5055 } 5056 else if (name.equals("experimental")) { 5057 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.experimental"); 5058 } 5059 else if (name.equals("date")) { 5060 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.date"); 5061 } 5062 else if (name.equals("publisher")) { 5063 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.publisher"); 5064 } 5065 else if (name.equals("description")) { 5066 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.description"); 5067 } 5068 else if (name.equals("purpose")) { 5069 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.purpose"); 5070 } 5071 else if (name.equals("usage")) { 5072 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.usage"); 5073 } 5074 else if (name.equals("approvalDate")) { 5075 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.approvalDate"); 5076 } 5077 else if (name.equals("lastReviewDate")) { 5078 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.lastReviewDate"); 5079 } 5080 else if (name.equals("effectivePeriod")) { 5081 this.effectivePeriod = new Period(); 5082 return this.effectivePeriod; 5083 } 5084 else if (name.equals("useContext")) { 5085 return addUseContext(); 5086 } 5087 else if (name.equals("jurisdiction")) { 5088 return addJurisdiction(); 5089 } 5090 else if (name.equals("topic")) { 5091 return addTopic(); 5092 } 5093 else if (name.equals("contributor")) { 5094 return addContributor(); 5095 } 5096 else if (name.equals("contact")) { 5097 return addContact(); 5098 } 5099 else if (name.equals("copyright")) { 5100 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.copyright"); 5101 } 5102 else if (name.equals("relatedArtifact")) { 5103 return addRelatedArtifact(); 5104 } 5105 else if (name.equals("library")) { 5106 return addLibrary(); 5107 } 5108 else if (name.equals("kind")) { 5109 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.kind"); 5110 } 5111 else if (name.equals("code")) { 5112 this.code = new CodeableConcept(); 5113 return this.code; 5114 } 5115 else if (name.equals("timingTiming")) { 5116 this.timing = new Timing(); 5117 return this.timing; 5118 } 5119 else if (name.equals("timingDateTime")) { 5120 this.timing = new DateTimeType(); 5121 return this.timing; 5122 } 5123 else if (name.equals("timingPeriod")) { 5124 this.timing = new Period(); 5125 return this.timing; 5126 } 5127 else if (name.equals("timingRange")) { 5128 this.timing = new Range(); 5129 return this.timing; 5130 } 5131 else if (name.equals("location")) { 5132 this.location = new Reference(); 5133 return this.location; 5134 } 5135 else if (name.equals("participant")) { 5136 return addParticipant(); 5137 } 5138 else if (name.equals("productReference")) { 5139 this.product = new Reference(); 5140 return this.product; 5141 } 5142 else if (name.equals("productCodeableConcept")) { 5143 this.product = new CodeableConcept(); 5144 return this.product; 5145 } 5146 else if (name.equals("quantity")) { 5147 this.quantity = new SimpleQuantity(); 5148 return this.quantity; 5149 } 5150 else if (name.equals("dosage")) { 5151 return addDosage(); 5152 } 5153 else if (name.equals("bodySite")) { 5154 return addBodySite(); 5155 } 5156 else if (name.equals("transform")) { 5157 this.transform = new Reference(); 5158 return this.transform; 5159 } 5160 else if (name.equals("dynamicValue")) { 5161 return addDynamicValue(); 5162 } 5163 else 5164 return super.addChild(name); 5165 } 5166 5167 public String fhirType() { 5168 return "ActivityDefinition"; 5169 5170 } 5171 5172 public ActivityDefinition copy() { 5173 ActivityDefinition dst = new ActivityDefinition(); 5174 copyValues(dst); 5175 dst.url = url == null ? null : url.copy(); 5176 if (identifier != null) { 5177 dst.identifier = new ArrayList<Identifier>(); 5178 for (Identifier i : identifier) 5179 dst.identifier.add(i.copy()); 5180 }; 5181 dst.version = version == null ? null : version.copy(); 5182 dst.name = name == null ? null : name.copy(); 5183 dst.title = title == null ? null : title.copy(); 5184 dst.status = status == null ? null : status.copy(); 5185 dst.experimental = experimental == null ? null : experimental.copy(); 5186 dst.date = date == null ? null : date.copy(); 5187 dst.publisher = publisher == null ? null : publisher.copy(); 5188 dst.description = description == null ? null : description.copy(); 5189 dst.purpose = purpose == null ? null : purpose.copy(); 5190 dst.usage = usage == null ? null : usage.copy(); 5191 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 5192 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 5193 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 5194 if (useContext != null) { 5195 dst.useContext = new ArrayList<UsageContext>(); 5196 for (UsageContext i : useContext) 5197 dst.useContext.add(i.copy()); 5198 }; 5199 if (jurisdiction != null) { 5200 dst.jurisdiction = new ArrayList<CodeableConcept>(); 5201 for (CodeableConcept i : jurisdiction) 5202 dst.jurisdiction.add(i.copy()); 5203 }; 5204 if (topic != null) { 5205 dst.topic = new ArrayList<CodeableConcept>(); 5206 for (CodeableConcept i : topic) 5207 dst.topic.add(i.copy()); 5208 }; 5209 if (contributor != null) { 5210 dst.contributor = new ArrayList<Contributor>(); 5211 for (Contributor i : contributor) 5212 dst.contributor.add(i.copy()); 5213 }; 5214 if (contact != null) { 5215 dst.contact = new ArrayList<ContactDetail>(); 5216 for (ContactDetail i : contact) 5217 dst.contact.add(i.copy()); 5218 }; 5219 dst.copyright = copyright == null ? null : copyright.copy(); 5220 if (relatedArtifact != null) { 5221 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 5222 for (RelatedArtifact i : relatedArtifact) 5223 dst.relatedArtifact.add(i.copy()); 5224 }; 5225 if (library != null) { 5226 dst.library = new ArrayList<Reference>(); 5227 for (Reference i : library) 5228 dst.library.add(i.copy()); 5229 }; 5230 dst.kind = kind == null ? null : kind.copy(); 5231 dst.code = code == null ? null : code.copy(); 5232 dst.timing = timing == null ? null : timing.copy(); 5233 dst.location = location == null ? null : location.copy(); 5234 if (participant != null) { 5235 dst.participant = new ArrayList<ActivityDefinitionParticipantComponent>(); 5236 for (ActivityDefinitionParticipantComponent i : participant) 5237 dst.participant.add(i.copy()); 5238 }; 5239 dst.product = product == null ? null : product.copy(); 5240 dst.quantity = quantity == null ? null : quantity.copy(); 5241 if (dosage != null) { 5242 dst.dosage = new ArrayList<Dosage>(); 5243 for (Dosage i : dosage) 5244 dst.dosage.add(i.copy()); 5245 }; 5246 if (bodySite != null) { 5247 dst.bodySite = new ArrayList<CodeableConcept>(); 5248 for (CodeableConcept i : bodySite) 5249 dst.bodySite.add(i.copy()); 5250 }; 5251 dst.transform = transform == null ? null : transform.copy(); 5252 if (dynamicValue != null) { 5253 dst.dynamicValue = new ArrayList<ActivityDefinitionDynamicValueComponent>(); 5254 for (ActivityDefinitionDynamicValueComponent i : dynamicValue) 5255 dst.dynamicValue.add(i.copy()); 5256 }; 5257 return dst; 5258 } 5259 5260 protected ActivityDefinition typedCopy() { 5261 return copy(); 5262 } 5263 5264 @Override 5265 public boolean equalsDeep(Base other_) { 5266 if (!super.equalsDeep(other_)) 5267 return false; 5268 if (!(other_ instanceof ActivityDefinition)) 5269 return false; 5270 ActivityDefinition o = (ActivityDefinition) other_; 5271 return compareDeep(identifier, o.identifier, true) && compareDeep(purpose, o.purpose, true) && compareDeep(usage, o.usage, true) 5272 && compareDeep(approvalDate, o.approvalDate, true) && compareDeep(lastReviewDate, o.lastReviewDate, true) 5273 && compareDeep(effectivePeriod, o.effectivePeriod, true) && compareDeep(topic, o.topic, true) && compareDeep(contributor, o.contributor, true) 5274 && compareDeep(copyright, o.copyright, true) && compareDeep(relatedArtifact, o.relatedArtifact, true) 5275 && compareDeep(library, o.library, true) && compareDeep(kind, o.kind, true) && compareDeep(code, o.code, true) 5276 && compareDeep(timing, o.timing, true) && compareDeep(location, o.location, true) && compareDeep(participant, o.participant, true) 5277 && compareDeep(product, o.product, true) && compareDeep(quantity, o.quantity, true) && compareDeep(dosage, o.dosage, true) 5278 && compareDeep(bodySite, o.bodySite, true) && compareDeep(transform, o.transform, true) && compareDeep(dynamicValue, o.dynamicValue, true) 5279 ; 5280 } 5281 5282 @Override 5283 public boolean equalsShallow(Base other_) { 5284 if (!super.equalsShallow(other_)) 5285 return false; 5286 if (!(other_ instanceof ActivityDefinition)) 5287 return false; 5288 ActivityDefinition o = (ActivityDefinition) other_; 5289 return compareValues(purpose, o.purpose, true) && compareValues(usage, o.usage, true) && compareValues(approvalDate, o.approvalDate, true) 5290 && compareValues(lastReviewDate, o.lastReviewDate, true) && compareValues(copyright, o.copyright, true) 5291 && compareValues(kind, o.kind, true); 5292 } 5293 5294 public boolean isEmpty() { 5295 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, purpose, usage 5296 , approvalDate, lastReviewDate, effectivePeriod, topic, contributor, copyright, relatedArtifact 5297 , library, kind, code, timing, location, participant, product, quantity, dosage 5298 , bodySite, transform, dynamicValue); 5299 } 5300 5301 @Override 5302 public ResourceType getResourceType() { 5303 return ResourceType.ActivityDefinition; 5304 } 5305 5306 /** 5307 * Search parameter: <b>date</b> 5308 * <p> 5309 * Description: <b>The activity definition publication date</b><br> 5310 * Type: <b>date</b><br> 5311 * Path: <b>ActivityDefinition.date</b><br> 5312 * </p> 5313 */ 5314 @SearchParamDefinition(name="date", path="ActivityDefinition.date", description="The activity definition publication date", type="date" ) 5315 public static final String SP_DATE = "date"; 5316 /** 5317 * <b>Fluent Client</b> search parameter constant for <b>date</b> 5318 * <p> 5319 * Description: <b>The activity definition publication date</b><br> 5320 * Type: <b>date</b><br> 5321 * Path: <b>ActivityDefinition.date</b><br> 5322 * </p> 5323 */ 5324 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 5325 5326 /** 5327 * Search parameter: <b>identifier</b> 5328 * <p> 5329 * Description: <b>External identifier for the activity definition</b><br> 5330 * Type: <b>token</b><br> 5331 * Path: <b>ActivityDefinition.identifier</b><br> 5332 * </p> 5333 */ 5334 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier", description="External identifier for the activity definition", type="token" ) 5335 public static final String SP_IDENTIFIER = "identifier"; 5336 /** 5337 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 5338 * <p> 5339 * Description: <b>External identifier for the activity definition</b><br> 5340 * Type: <b>token</b><br> 5341 * Path: <b>ActivityDefinition.identifier</b><br> 5342 * </p> 5343 */ 5344 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 5345 5346 /** 5347 * Search parameter: <b>successor</b> 5348 * <p> 5349 * Description: <b>What resource is being referenced</b><br> 5350 * Type: <b>reference</b><br> 5351 * Path: <b>ActivityDefinition.relatedArtifact.resource</b><br> 5352 * </p> 5353 */ 5354 @SearchParamDefinition(name="successor", path="ActivityDefinition.relatedArtifact.where(type='successor').resource", description="What resource is being referenced", type="reference" ) 5355 public static final String SP_SUCCESSOR = "successor"; 5356 /** 5357 * <b>Fluent Client</b> search parameter constant for <b>successor</b> 5358 * <p> 5359 * Description: <b>What resource is being referenced</b><br> 5360 * Type: <b>reference</b><br> 5361 * Path: <b>ActivityDefinition.relatedArtifact.resource</b><br> 5362 * </p> 5363 */ 5364 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUCCESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUCCESSOR); 5365 5366/** 5367 * Constant for fluent queries to be used to add include statements. Specifies 5368 * the path value of "<b>ActivityDefinition:successor</b>". 5369 */ 5370 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUCCESSOR = new ca.uhn.fhir.model.api.Include("ActivityDefinition:successor").toLocked(); 5371 5372 /** 5373 * Search parameter: <b>jurisdiction</b> 5374 * <p> 5375 * Description: <b>Intended jurisdiction for the activity definition</b><br> 5376 * Type: <b>token</b><br> 5377 * Path: <b>ActivityDefinition.jurisdiction</b><br> 5378 * </p> 5379 */ 5380 @SearchParamDefinition(name="jurisdiction", path="ActivityDefinition.jurisdiction", description="Intended jurisdiction for the activity definition", type="token" ) 5381 public static final String SP_JURISDICTION = "jurisdiction"; 5382 /** 5383 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 5384 * <p> 5385 * Description: <b>Intended jurisdiction for the activity definition</b><br> 5386 * Type: <b>token</b><br> 5387 * Path: <b>ActivityDefinition.jurisdiction</b><br> 5388 * </p> 5389 */ 5390 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 5391 5392 /** 5393 * Search parameter: <b>description</b> 5394 * <p> 5395 * Description: <b>The description of the activity definition</b><br> 5396 * Type: <b>string</b><br> 5397 * Path: <b>ActivityDefinition.description</b><br> 5398 * </p> 5399 */ 5400 @SearchParamDefinition(name="description", path="ActivityDefinition.description", description="The description of the activity definition", type="string" ) 5401 public static final String SP_DESCRIPTION = "description"; 5402 /** 5403 * <b>Fluent Client</b> search parameter constant for <b>description</b> 5404 * <p> 5405 * Description: <b>The description of the activity definition</b><br> 5406 * Type: <b>string</b><br> 5407 * Path: <b>ActivityDefinition.description</b><br> 5408 * </p> 5409 */ 5410 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 5411 5412 /** 5413 * Search parameter: <b>derived-from</b> 5414 * <p> 5415 * Description: <b>What resource is being referenced</b><br> 5416 * Type: <b>reference</b><br> 5417 * Path: <b>ActivityDefinition.relatedArtifact.resource</b><br> 5418 * </p> 5419 */ 5420 @SearchParamDefinition(name="derived-from", path="ActivityDefinition.relatedArtifact.where(type='derived-from').resource", description="What resource is being referenced", type="reference" ) 5421 public static final String SP_DERIVED_FROM = "derived-from"; 5422 /** 5423 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 5424 * <p> 5425 * Description: <b>What resource is being referenced</b><br> 5426 * Type: <b>reference</b><br> 5427 * Path: <b>ActivityDefinition.relatedArtifact.resource</b><br> 5428 * </p> 5429 */ 5430 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DERIVED_FROM); 5431 5432/** 5433 * Constant for fluent queries to be used to add include statements. Specifies 5434 * the path value of "<b>ActivityDefinition:derived-from</b>". 5435 */ 5436 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include("ActivityDefinition:derived-from").toLocked(); 5437 5438 /** 5439 * Search parameter: <b>predecessor</b> 5440 * <p> 5441 * Description: <b>What resource is being referenced</b><br> 5442 * Type: <b>reference</b><br> 5443 * Path: <b>ActivityDefinition.relatedArtifact.resource</b><br> 5444 * </p> 5445 */ 5446 @SearchParamDefinition(name="predecessor", path="ActivityDefinition.relatedArtifact.where(type='predecessor').resource", description="What resource is being referenced", type="reference" ) 5447 public static final String SP_PREDECESSOR = "predecessor"; 5448 /** 5449 * <b>Fluent Client</b> search parameter constant for <b>predecessor</b> 5450 * <p> 5451 * Description: <b>What resource is being referenced</b><br> 5452 * Type: <b>reference</b><br> 5453 * Path: <b>ActivityDefinition.relatedArtifact.resource</b><br> 5454 * </p> 5455 */ 5456 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PREDECESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PREDECESSOR); 5457 5458/** 5459 * Constant for fluent queries to be used to add include statements. Specifies 5460 * the path value of "<b>ActivityDefinition:predecessor</b>". 5461 */ 5462 public static final ca.uhn.fhir.model.api.Include INCLUDE_PREDECESSOR = new ca.uhn.fhir.model.api.Include("ActivityDefinition:predecessor").toLocked(); 5463 5464 /** 5465 * Search parameter: <b>title</b> 5466 * <p> 5467 * Description: <b>The human-friendly name of the activity definition</b><br> 5468 * Type: <b>string</b><br> 5469 * Path: <b>ActivityDefinition.title</b><br> 5470 * </p> 5471 */ 5472 @SearchParamDefinition(name="title", path="ActivityDefinition.title", description="The human-friendly name of the activity definition", type="string" ) 5473 public static final String SP_TITLE = "title"; 5474 /** 5475 * <b>Fluent Client</b> search parameter constant for <b>title</b> 5476 * <p> 5477 * Description: <b>The human-friendly name of the activity definition</b><br> 5478 * Type: <b>string</b><br> 5479 * Path: <b>ActivityDefinition.title</b><br> 5480 * </p> 5481 */ 5482 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 5483 5484 /** 5485 * Search parameter: <b>composed-of</b> 5486 * <p> 5487 * Description: <b>What resource is being referenced</b><br> 5488 * Type: <b>reference</b><br> 5489 * Path: <b>ActivityDefinition.relatedArtifact.resource</b><br> 5490 * </p> 5491 */ 5492 @SearchParamDefinition(name="composed-of", path="ActivityDefinition.relatedArtifact.where(type='composed-of').resource", description="What resource is being referenced", type="reference" ) 5493 public static final String SP_COMPOSED_OF = "composed-of"; 5494 /** 5495 * <b>Fluent Client</b> search parameter constant for <b>composed-of</b> 5496 * <p> 5497 * Description: <b>What resource is being referenced</b><br> 5498 * Type: <b>reference</b><br> 5499 * Path: <b>ActivityDefinition.relatedArtifact.resource</b><br> 5500 * </p> 5501 */ 5502 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPOSED_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_COMPOSED_OF); 5503 5504/** 5505 * Constant for fluent queries to be used to add include statements. Specifies 5506 * the path value of "<b>ActivityDefinition:composed-of</b>". 5507 */ 5508 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPOSED_OF = new ca.uhn.fhir.model.api.Include("ActivityDefinition:composed-of").toLocked(); 5509 5510 /** 5511 * Search parameter: <b>version</b> 5512 * <p> 5513 * Description: <b>The business version of the activity definition</b><br> 5514 * Type: <b>token</b><br> 5515 * Path: <b>ActivityDefinition.version</b><br> 5516 * </p> 5517 */ 5518 @SearchParamDefinition(name="version", path="ActivityDefinition.version", description="The business version of the activity definition", type="token" ) 5519 public static final String SP_VERSION = "version"; 5520 /** 5521 * <b>Fluent Client</b> search parameter constant for <b>version</b> 5522 * <p> 5523 * Description: <b>The business version of the activity definition</b><br> 5524 * Type: <b>token</b><br> 5525 * Path: <b>ActivityDefinition.version</b><br> 5526 * </p> 5527 */ 5528 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 5529 5530 /** 5531 * Search parameter: <b>url</b> 5532 * <p> 5533 * Description: <b>The uri that identifies the activity definition</b><br> 5534 * Type: <b>uri</b><br> 5535 * Path: <b>ActivityDefinition.url</b><br> 5536 * </p> 5537 */ 5538 @SearchParamDefinition(name="url", path="ActivityDefinition.url", description="The uri that identifies the activity definition", type="uri" ) 5539 public static final String SP_URL = "url"; 5540 /** 5541 * <b>Fluent Client</b> search parameter constant for <b>url</b> 5542 * <p> 5543 * Description: <b>The uri that identifies the activity definition</b><br> 5544 * Type: <b>uri</b><br> 5545 * Path: <b>ActivityDefinition.url</b><br> 5546 * </p> 5547 */ 5548 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 5549 5550 /** 5551 * Search parameter: <b>effective</b> 5552 * <p> 5553 * Description: <b>The time during which the activity definition is intended to be in use</b><br> 5554 * Type: <b>date</b><br> 5555 * Path: <b>ActivityDefinition.effectivePeriod</b><br> 5556 * </p> 5557 */ 5558 @SearchParamDefinition(name="effective", path="ActivityDefinition.effectivePeriod", description="The time during which the activity definition is intended to be in use", type="date" ) 5559 public static final String SP_EFFECTIVE = "effective"; 5560 /** 5561 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 5562 * <p> 5563 * Description: <b>The time during which the activity definition is intended to be in use</b><br> 5564 * Type: <b>date</b><br> 5565 * Path: <b>ActivityDefinition.effectivePeriod</b><br> 5566 * </p> 5567 */ 5568 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EFFECTIVE); 5569 5570 /** 5571 * Search parameter: <b>depends-on</b> 5572 * <p> 5573 * Description: <b>What resource is being referenced</b><br> 5574 * Type: <b>reference</b><br> 5575 * Path: <b>ActivityDefinition.relatedArtifact.resource, ActivityDefinition.library</b><br> 5576 * </p> 5577 */ 5578 @SearchParamDefinition(name="depends-on", path="ActivityDefinition.relatedArtifact.where(type='depends-on').resource | ActivityDefinition.library", description="What resource is being referenced", type="reference" ) 5579 public static final String SP_DEPENDS_ON = "depends-on"; 5580 /** 5581 * <b>Fluent Client</b> search parameter constant for <b>depends-on</b> 5582 * <p> 5583 * Description: <b>What resource is being referenced</b><br> 5584 * Type: <b>reference</b><br> 5585 * Path: <b>ActivityDefinition.relatedArtifact.resource, ActivityDefinition.library</b><br> 5586 * </p> 5587 */ 5588 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEPENDS_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEPENDS_ON); 5589 5590/** 5591 * Constant for fluent queries to be used to add include statements. Specifies 5592 * the path value of "<b>ActivityDefinition:depends-on</b>". 5593 */ 5594 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEPENDS_ON = new ca.uhn.fhir.model.api.Include("ActivityDefinition:depends-on").toLocked(); 5595 5596 /** 5597 * Search parameter: <b>name</b> 5598 * <p> 5599 * Description: <b>Computationally friendly name of the activity definition</b><br> 5600 * Type: <b>string</b><br> 5601 * Path: <b>ActivityDefinition.name</b><br> 5602 * </p> 5603 */ 5604 @SearchParamDefinition(name="name", path="ActivityDefinition.name", description="Computationally friendly name of the activity definition", type="string" ) 5605 public static final String SP_NAME = "name"; 5606 /** 5607 * <b>Fluent Client</b> search parameter constant for <b>name</b> 5608 * <p> 5609 * Description: <b>Computationally friendly name of the activity definition</b><br> 5610 * Type: <b>string</b><br> 5611 * Path: <b>ActivityDefinition.name</b><br> 5612 * </p> 5613 */ 5614 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 5615 5616 /** 5617 * Search parameter: <b>publisher</b> 5618 * <p> 5619 * Description: <b>Name of the publisher of the activity definition</b><br> 5620 * Type: <b>string</b><br> 5621 * Path: <b>ActivityDefinition.publisher</b><br> 5622 * </p> 5623 */ 5624 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher", description="Name of the publisher of the activity definition", type="string" ) 5625 public static final String SP_PUBLISHER = "publisher"; 5626 /** 5627 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 5628 * <p> 5629 * Description: <b>Name of the publisher of the activity definition</b><br> 5630 * Type: <b>string</b><br> 5631 * Path: <b>ActivityDefinition.publisher</b><br> 5632 * </p> 5633 */ 5634 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 5635 5636 /** 5637 * Search parameter: <b>topic</b> 5638 * <p> 5639 * Description: <b>Topics associated with the module</b><br> 5640 * Type: <b>token</b><br> 5641 * Path: <b>ActivityDefinition.topic</b><br> 5642 * </p> 5643 */ 5644 @SearchParamDefinition(name="topic", path="ActivityDefinition.topic", description="Topics associated with the module", type="token" ) 5645 public static final String SP_TOPIC = "topic"; 5646 /** 5647 * <b>Fluent Client</b> search parameter constant for <b>topic</b> 5648 * <p> 5649 * Description: <b>Topics associated with the module</b><br> 5650 * Type: <b>token</b><br> 5651 * Path: <b>ActivityDefinition.topic</b><br> 5652 * </p> 5653 */ 5654 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TOPIC = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TOPIC); 5655 5656 /** 5657 * Search parameter: <b>status</b> 5658 * <p> 5659 * Description: <b>The current status of the activity definition</b><br> 5660 * Type: <b>token</b><br> 5661 * Path: <b>ActivityDefinition.status</b><br> 5662 * </p> 5663 */ 5664 @SearchParamDefinition(name="status", path="ActivityDefinition.status", description="The current status of the activity definition", type="token" ) 5665 public static final String SP_STATUS = "status"; 5666 /** 5667 * <b>Fluent Client</b> search parameter constant for <b>status</b> 5668 * <p> 5669 * Description: <b>The current status of the activity definition</b><br> 5670 * Type: <b>token</b><br> 5671 * Path: <b>ActivityDefinition.status</b><br> 5672 * </p> 5673 */ 5674 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 5675 5676 5677}