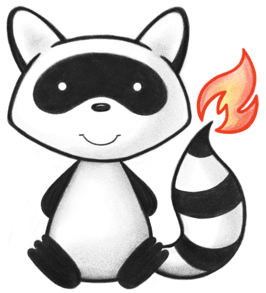
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.ArrayList; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.ICompositeType; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046/** 047 * An address expressed using postal conventions (as opposed to GPS or other location definition formats). This data type may be used to convey addresses for use in delivering mail as well as for visiting locations which might not be valid for mail delivery. There are a variety of postal address formats defined around the world. 048 */ 049@DatatypeDef(name="Address") 050public class Address extends Type implements ICompositeType { 051 052 public enum AddressUse { 053 /** 054 * A communication address at a home. 055 */ 056 HOME, 057 /** 058 * An office address. First choice for business related contacts during business hours. 059 */ 060 WORK, 061 /** 062 * A temporary address. The period can provide more detailed information. 063 */ 064 TEMP, 065 /** 066 * This address is no longer in use (or was never correct, but retained for records). 067 */ 068 OLD, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static AddressUse fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("home".equals(codeString)) 077 return HOME; 078 if ("work".equals(codeString)) 079 return WORK; 080 if ("temp".equals(codeString)) 081 return TEMP; 082 if ("old".equals(codeString)) 083 return OLD; 084 if (Configuration.isAcceptInvalidEnums()) 085 return null; 086 else 087 throw new FHIRException("Unknown AddressUse code '"+codeString+"'"); 088 } 089 public String toCode() { 090 switch (this) { 091 case HOME: return "home"; 092 case WORK: return "work"; 093 case TEMP: return "temp"; 094 case OLD: return "old"; 095 case NULL: return null; 096 default: return "?"; 097 } 098 } 099 public String getSystem() { 100 switch (this) { 101 case HOME: return "http://hl7.org/fhir/address-use"; 102 case WORK: return "http://hl7.org/fhir/address-use"; 103 case TEMP: return "http://hl7.org/fhir/address-use"; 104 case OLD: return "http://hl7.org/fhir/address-use"; 105 case NULL: return null; 106 default: return "?"; 107 } 108 } 109 public String getDefinition() { 110 switch (this) { 111 case HOME: return "A communication address at a home."; 112 case WORK: return "An office address. First choice for business related contacts during business hours."; 113 case TEMP: return "A temporary address. The period can provide more detailed information."; 114 case OLD: return "This address is no longer in use (or was never correct, but retained for records)."; 115 case NULL: return null; 116 default: return "?"; 117 } 118 } 119 public String getDisplay() { 120 switch (this) { 121 case HOME: return "Home"; 122 case WORK: return "Work"; 123 case TEMP: return "Temporary"; 124 case OLD: return "Old / Incorrect"; 125 case NULL: return null; 126 default: return "?"; 127 } 128 } 129 } 130 131 public static class AddressUseEnumFactory implements EnumFactory<AddressUse> { 132 public AddressUse fromCode(String codeString) throws IllegalArgumentException { 133 if (codeString == null || "".equals(codeString)) 134 if (codeString == null || "".equals(codeString)) 135 return null; 136 if ("home".equals(codeString)) 137 return AddressUse.HOME; 138 if ("work".equals(codeString)) 139 return AddressUse.WORK; 140 if ("temp".equals(codeString)) 141 return AddressUse.TEMP; 142 if ("old".equals(codeString)) 143 return AddressUse.OLD; 144 throw new IllegalArgumentException("Unknown AddressUse code '"+codeString+"'"); 145 } 146 public Enumeration<AddressUse> fromType(PrimitiveType<?> code) throws FHIRException { 147 if (code == null) 148 return null; 149 if (code.isEmpty()) 150 return new Enumeration<AddressUse>(this); 151 String codeString = code.asStringValue(); 152 if (codeString == null || "".equals(codeString)) 153 return null; 154 if ("home".equals(codeString)) 155 return new Enumeration<AddressUse>(this, AddressUse.HOME); 156 if ("work".equals(codeString)) 157 return new Enumeration<AddressUse>(this, AddressUse.WORK); 158 if ("temp".equals(codeString)) 159 return new Enumeration<AddressUse>(this, AddressUse.TEMP); 160 if ("old".equals(codeString)) 161 return new Enumeration<AddressUse>(this, AddressUse.OLD); 162 throw new FHIRException("Unknown AddressUse code '"+codeString+"'"); 163 } 164 public String toCode(AddressUse code) { 165 if (code == AddressUse.NULL) 166 return null; 167 if (code == AddressUse.HOME) 168 return "home"; 169 if (code == AddressUse.WORK) 170 return "work"; 171 if (code == AddressUse.TEMP) 172 return "temp"; 173 if (code == AddressUse.OLD) 174 return "old"; 175 return "?"; 176 } 177 public String toSystem(AddressUse code) { 178 return code.getSystem(); 179 } 180 } 181 182 public enum AddressType { 183 /** 184 * Mailing addresses - PO Boxes and care-of addresses. 185 */ 186 POSTAL, 187 /** 188 * A physical address that can be visited. 189 */ 190 PHYSICAL, 191 /** 192 * An address that is both physical and postal. 193 */ 194 BOTH, 195 /** 196 * added to help the parsers with the generic types 197 */ 198 NULL; 199 public static AddressType fromCode(String codeString) throws FHIRException { 200 if (codeString == null || "".equals(codeString)) 201 return null; 202 if ("postal".equals(codeString)) 203 return POSTAL; 204 if ("physical".equals(codeString)) 205 return PHYSICAL; 206 if ("both".equals(codeString)) 207 return BOTH; 208 if (Configuration.isAcceptInvalidEnums()) 209 return null; 210 else 211 throw new FHIRException("Unknown AddressType code '"+codeString+"'"); 212 } 213 public String toCode() { 214 switch (this) { 215 case POSTAL: return "postal"; 216 case PHYSICAL: return "physical"; 217 case BOTH: return "both"; 218 case NULL: return null; 219 default: return "?"; 220 } 221 } 222 public String getSystem() { 223 switch (this) { 224 case POSTAL: return "http://hl7.org/fhir/address-type"; 225 case PHYSICAL: return "http://hl7.org/fhir/address-type"; 226 case BOTH: return "http://hl7.org/fhir/address-type"; 227 case NULL: return null; 228 default: return "?"; 229 } 230 } 231 public String getDefinition() { 232 switch (this) { 233 case POSTAL: return "Mailing addresses - PO Boxes and care-of addresses."; 234 case PHYSICAL: return "A physical address that can be visited."; 235 case BOTH: return "An address that is both physical and postal."; 236 case NULL: return null; 237 default: return "?"; 238 } 239 } 240 public String getDisplay() { 241 switch (this) { 242 case POSTAL: return "Postal"; 243 case PHYSICAL: return "Physical"; 244 case BOTH: return "Postal & Physical"; 245 case NULL: return null; 246 default: return "?"; 247 } 248 } 249 } 250 251 public static class AddressTypeEnumFactory implements EnumFactory<AddressType> { 252 public AddressType fromCode(String codeString) throws IllegalArgumentException { 253 if (codeString == null || "".equals(codeString)) 254 if (codeString == null || "".equals(codeString)) 255 return null; 256 if ("postal".equals(codeString)) 257 return AddressType.POSTAL; 258 if ("physical".equals(codeString)) 259 return AddressType.PHYSICAL; 260 if ("both".equals(codeString)) 261 return AddressType.BOTH; 262 throw new IllegalArgumentException("Unknown AddressType code '"+codeString+"'"); 263 } 264 public Enumeration<AddressType> fromType(PrimitiveType<?> code) throws FHIRException { 265 if (code == null) 266 return null; 267 if (code.isEmpty()) 268 return new Enumeration<AddressType>(this); 269 String codeString = code.asStringValue(); 270 if (codeString == null || "".equals(codeString)) 271 return null; 272 if ("postal".equals(codeString)) 273 return new Enumeration<AddressType>(this, AddressType.POSTAL); 274 if ("physical".equals(codeString)) 275 return new Enumeration<AddressType>(this, AddressType.PHYSICAL); 276 if ("both".equals(codeString)) 277 return new Enumeration<AddressType>(this, AddressType.BOTH); 278 throw new FHIRException("Unknown AddressType code '"+codeString+"'"); 279 } 280 public String toCode(AddressType code) { 281 if (code == AddressType.NULL) 282 return null; 283 if (code == AddressType.POSTAL) 284 return "postal"; 285 if (code == AddressType.PHYSICAL) 286 return "physical"; 287 if (code == AddressType.BOTH) 288 return "both"; 289 return "?"; 290 } 291 public String toSystem(AddressType code) { 292 return code.getSystem(); 293 } 294 } 295 296 /** 297 * The purpose of this address. 298 */ 299 @Child(name = "use", type = {CodeType.class}, order=0, min=0, max=1, modifier=true, summary=true) 300 @Description(shortDefinition="home | work | temp | old - purpose of this address", formalDefinition="The purpose of this address." ) 301 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/address-use") 302 protected Enumeration<AddressUse> use; 303 304 /** 305 * Distinguishes between physical addresses (those you can visit) and mailing addresses (e.g. PO Boxes and care-of addresses). Most addresses are both. 306 */ 307 @Child(name = "type", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=true) 308 @Description(shortDefinition="postal | physical | both", formalDefinition="Distinguishes between physical addresses (those you can visit) and mailing addresses (e.g. PO Boxes and care-of addresses). Most addresses are both." ) 309 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/address-type") 310 protected Enumeration<AddressType> type; 311 312 /** 313 * A full text representation of the address. 314 */ 315 @Child(name = "text", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 316 @Description(shortDefinition="Text representation of the address", formalDefinition="A full text representation of the address." ) 317 protected StringType text; 318 319 /** 320 * This component contains the house number, apartment number, street name, street direction, P.O. Box number, delivery hints, and similar address information. 321 */ 322 @Child(name = "line", type = {StringType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 323 @Description(shortDefinition="Street name, number, direction & P.O. Box etc.", formalDefinition="This component contains the house number, apartment number, street name, street direction, P.O. Box number, delivery hints, and similar address information." ) 324 protected List<StringType> line; 325 326 /** 327 * The name of the city, town, village or other community or delivery center. 328 */ 329 @Child(name = "city", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 330 @Description(shortDefinition="Name of city, town etc.", formalDefinition="The name of the city, town, village or other community or delivery center." ) 331 protected StringType city; 332 333 /** 334 * The name of the administrative area (county). 335 */ 336 @Child(name = "district", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 337 @Description(shortDefinition="District name (aka county)", formalDefinition="The name of the administrative area (county)." ) 338 protected StringType district; 339 340 /** 341 * Sub-unit of a country with limited sovereignty in a federally organized country. A code may be used if codes are in common use (i.e. US 2 letter state codes). 342 */ 343 @Child(name = "state", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=true) 344 @Description(shortDefinition="Sub-unit of country (abbreviations ok)", formalDefinition="Sub-unit of a country with limited sovereignty in a federally organized country. A code may be used if codes are in common use (i.e. US 2 letter state codes)." ) 345 protected StringType state; 346 347 /** 348 * A postal code designating a region defined by the postal service. 349 */ 350 @Child(name = "postalCode", type = {StringType.class}, order=7, min=0, max=1, modifier=false, summary=true) 351 @Description(shortDefinition="Postal code for area", formalDefinition="A postal code designating a region defined by the postal service." ) 352 protected StringType postalCode; 353 354 /** 355 * Country - a nation as commonly understood or generally accepted. 356 */ 357 @Child(name = "country", type = {StringType.class}, order=8, min=0, max=1, modifier=false, summary=true) 358 @Description(shortDefinition="Country (e.g. can be ISO 3166 2 or 3 letter code)", formalDefinition="Country - a nation as commonly understood or generally accepted." ) 359 protected StringType country; 360 361 /** 362 * Time period when address was/is in use. 363 */ 364 @Child(name = "period", type = {Period.class}, order=9, min=0, max=1, modifier=false, summary=true) 365 @Description(shortDefinition="Time period when address was/is in use", formalDefinition="Time period when address was/is in use." ) 366 protected Period period; 367 368 private static final long serialVersionUID = 561490318L; 369 370 /** 371 * Constructor 372 */ 373 public Address() { 374 super(); 375 } 376 377 /** 378 * @return {@link #use} (The purpose of this address.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 379 */ 380 public Enumeration<AddressUse> getUseElement() { 381 if (this.use == null) 382 if (Configuration.errorOnAutoCreate()) 383 throw new Error("Attempt to auto-create Address.use"); 384 else if (Configuration.doAutoCreate()) 385 this.use = new Enumeration<AddressUse>(new AddressUseEnumFactory()); // bb 386 return this.use; 387 } 388 389 public boolean hasUseElement() { 390 return this.use != null && !this.use.isEmpty(); 391 } 392 393 public boolean hasUse() { 394 return this.use != null && !this.use.isEmpty(); 395 } 396 397 /** 398 * @param value {@link #use} (The purpose of this address.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 399 */ 400 public Address setUseElement(Enumeration<AddressUse> value) { 401 this.use = value; 402 return this; 403 } 404 405 /** 406 * @return The purpose of this address. 407 */ 408 public AddressUse getUse() { 409 return this.use == null ? null : this.use.getValue(); 410 } 411 412 /** 413 * @param value The purpose of this address. 414 */ 415 public Address setUse(AddressUse value) { 416 if (value == null) 417 this.use = null; 418 else { 419 if (this.use == null) 420 this.use = new Enumeration<AddressUse>(new AddressUseEnumFactory()); 421 this.use.setValue(value); 422 } 423 return this; 424 } 425 426 /** 427 * @return {@link #type} (Distinguishes between physical addresses (those you can visit) and mailing addresses (e.g. PO Boxes and care-of addresses). Most addresses are both.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 428 */ 429 public Enumeration<AddressType> getTypeElement() { 430 if (this.type == null) 431 if (Configuration.errorOnAutoCreate()) 432 throw new Error("Attempt to auto-create Address.type"); 433 else if (Configuration.doAutoCreate()) 434 this.type = new Enumeration<AddressType>(new AddressTypeEnumFactory()); // bb 435 return this.type; 436 } 437 438 public boolean hasTypeElement() { 439 return this.type != null && !this.type.isEmpty(); 440 } 441 442 public boolean hasType() { 443 return this.type != null && !this.type.isEmpty(); 444 } 445 446 /** 447 * @param value {@link #type} (Distinguishes between physical addresses (those you can visit) and mailing addresses (e.g. PO Boxes and care-of addresses). Most addresses are both.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 448 */ 449 public Address setTypeElement(Enumeration<AddressType> value) { 450 this.type = value; 451 return this; 452 } 453 454 /** 455 * @return Distinguishes between physical addresses (those you can visit) and mailing addresses (e.g. PO Boxes and care-of addresses). Most addresses are both. 456 */ 457 public AddressType getType() { 458 return this.type == null ? null : this.type.getValue(); 459 } 460 461 /** 462 * @param value Distinguishes between physical addresses (those you can visit) and mailing addresses (e.g. PO Boxes and care-of addresses). Most addresses are both. 463 */ 464 public Address setType(AddressType value) { 465 if (value == null) 466 this.type = null; 467 else { 468 if (this.type == null) 469 this.type = new Enumeration<AddressType>(new AddressTypeEnumFactory()); 470 this.type.setValue(value); 471 } 472 return this; 473 } 474 475 /** 476 * @return {@link #text} (A full text representation of the address.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 477 */ 478 public StringType getTextElement() { 479 if (this.text == null) 480 if (Configuration.errorOnAutoCreate()) 481 throw new Error("Attempt to auto-create Address.text"); 482 else if (Configuration.doAutoCreate()) 483 this.text = new StringType(); // bb 484 return this.text; 485 } 486 487 public boolean hasTextElement() { 488 return this.text != null && !this.text.isEmpty(); 489 } 490 491 public boolean hasText() { 492 return this.text != null && !this.text.isEmpty(); 493 } 494 495 /** 496 * @param value {@link #text} (A full text representation of the address.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 497 */ 498 public Address setTextElement(StringType value) { 499 this.text = value; 500 return this; 501 } 502 503 /** 504 * @return A full text representation of the address. 505 */ 506 public String getText() { 507 return this.text == null ? null : this.text.getValue(); 508 } 509 510 /** 511 * @param value A full text representation of the address. 512 */ 513 public Address setText(String value) { 514 if (Utilities.noString(value)) 515 this.text = null; 516 else { 517 if (this.text == null) 518 this.text = new StringType(); 519 this.text.setValue(value); 520 } 521 return this; 522 } 523 524 /** 525 * @return {@link #line} (This component contains the house number, apartment number, street name, street direction, P.O. Box number, delivery hints, and similar address information.) 526 */ 527 public List<StringType> getLine() { 528 if (this.line == null) 529 this.line = new ArrayList<StringType>(); 530 return this.line; 531 } 532 533 /** 534 * @return Returns a reference to <code>this</code> for easy method chaining 535 */ 536 public Address setLine(List<StringType> theLine) { 537 this.line = theLine; 538 return this; 539 } 540 541 public boolean hasLine() { 542 if (this.line == null) 543 return false; 544 for (StringType item : this.line) 545 if (!item.isEmpty()) 546 return true; 547 return false; 548 } 549 550 /** 551 * @return {@link #line} (This component contains the house number, apartment number, street name, street direction, P.O. Box number, delivery hints, and similar address information.) 552 */ 553 public StringType addLineElement() {//2 554 StringType t = new StringType(); 555 if (this.line == null) 556 this.line = new ArrayList<StringType>(); 557 this.line.add(t); 558 return t; 559 } 560 561 /** 562 * @param value {@link #line} (This component contains the house number, apartment number, street name, street direction, P.O. Box number, delivery hints, and similar address information.) 563 */ 564 public Address addLine(String value) { //1 565 StringType t = new StringType(); 566 t.setValue(value); 567 if (this.line == null) 568 this.line = new ArrayList<StringType>(); 569 this.line.add(t); 570 return this; 571 } 572 573 /** 574 * @param value {@link #line} (This component contains the house number, apartment number, street name, street direction, P.O. Box number, delivery hints, and similar address information.) 575 */ 576 public boolean hasLine(String value) { 577 if (this.line == null) 578 return false; 579 for (StringType v : this.line) 580 if (v.getValue().equals(value)) // string 581 return true; 582 return false; 583 } 584 585 /** 586 * @return {@link #city} (The name of the city, town, village or other community or delivery center.). This is the underlying object with id, value and extensions. The accessor "getCity" gives direct access to the value 587 */ 588 public StringType getCityElement() { 589 if (this.city == null) 590 if (Configuration.errorOnAutoCreate()) 591 throw new Error("Attempt to auto-create Address.city"); 592 else if (Configuration.doAutoCreate()) 593 this.city = new StringType(); // bb 594 return this.city; 595 } 596 597 public boolean hasCityElement() { 598 return this.city != null && !this.city.isEmpty(); 599 } 600 601 public boolean hasCity() { 602 return this.city != null && !this.city.isEmpty(); 603 } 604 605 /** 606 * @param value {@link #city} (The name of the city, town, village or other community or delivery center.). This is the underlying object with id, value and extensions. The accessor "getCity" gives direct access to the value 607 */ 608 public Address setCityElement(StringType value) { 609 this.city = value; 610 return this; 611 } 612 613 /** 614 * @return The name of the city, town, village or other community or delivery center. 615 */ 616 public String getCity() { 617 return this.city == null ? null : this.city.getValue(); 618 } 619 620 /** 621 * @param value The name of the city, town, village or other community or delivery center. 622 */ 623 public Address setCity(String value) { 624 if (Utilities.noString(value)) 625 this.city = null; 626 else { 627 if (this.city == null) 628 this.city = new StringType(); 629 this.city.setValue(value); 630 } 631 return this; 632 } 633 634 /** 635 * @return {@link #district} (The name of the administrative area (county).). This is the underlying object with id, value and extensions. The accessor "getDistrict" gives direct access to the value 636 */ 637 public StringType getDistrictElement() { 638 if (this.district == null) 639 if (Configuration.errorOnAutoCreate()) 640 throw new Error("Attempt to auto-create Address.district"); 641 else if (Configuration.doAutoCreate()) 642 this.district = new StringType(); // bb 643 return this.district; 644 } 645 646 public boolean hasDistrictElement() { 647 return this.district != null && !this.district.isEmpty(); 648 } 649 650 public boolean hasDistrict() { 651 return this.district != null && !this.district.isEmpty(); 652 } 653 654 /** 655 * @param value {@link #district} (The name of the administrative area (county).). This is the underlying object with id, value and extensions. The accessor "getDistrict" gives direct access to the value 656 */ 657 public Address setDistrictElement(StringType value) { 658 this.district = value; 659 return this; 660 } 661 662 /** 663 * @return The name of the administrative area (county). 664 */ 665 public String getDistrict() { 666 return this.district == null ? null : this.district.getValue(); 667 } 668 669 /** 670 * @param value The name of the administrative area (county). 671 */ 672 public Address setDistrict(String value) { 673 if (Utilities.noString(value)) 674 this.district = null; 675 else { 676 if (this.district == null) 677 this.district = new StringType(); 678 this.district.setValue(value); 679 } 680 return this; 681 } 682 683 /** 684 * @return {@link #state} (Sub-unit of a country with limited sovereignty in a federally organized country. A code may be used if codes are in common use (i.e. US 2 letter state codes).). This is the underlying object with id, value and extensions. The accessor "getState" gives direct access to the value 685 */ 686 public StringType getStateElement() { 687 if (this.state == null) 688 if (Configuration.errorOnAutoCreate()) 689 throw new Error("Attempt to auto-create Address.state"); 690 else if (Configuration.doAutoCreate()) 691 this.state = new StringType(); // bb 692 return this.state; 693 } 694 695 public boolean hasStateElement() { 696 return this.state != null && !this.state.isEmpty(); 697 } 698 699 public boolean hasState() { 700 return this.state != null && !this.state.isEmpty(); 701 } 702 703 /** 704 * @param value {@link #state} (Sub-unit of a country with limited sovereignty in a federally organized country. A code may be used if codes are in common use (i.e. US 2 letter state codes).). This is the underlying object with id, value and extensions. The accessor "getState" gives direct access to the value 705 */ 706 public Address setStateElement(StringType value) { 707 this.state = value; 708 return this; 709 } 710 711 /** 712 * @return Sub-unit of a country with limited sovereignty in a federally organized country. A code may be used if codes are in common use (i.e. US 2 letter state codes). 713 */ 714 public String getState() { 715 return this.state == null ? null : this.state.getValue(); 716 } 717 718 /** 719 * @param value Sub-unit of a country with limited sovereignty in a federally organized country. A code may be used if codes are in common use (i.e. US 2 letter state codes). 720 */ 721 public Address setState(String value) { 722 if (Utilities.noString(value)) 723 this.state = null; 724 else { 725 if (this.state == null) 726 this.state = new StringType(); 727 this.state.setValue(value); 728 } 729 return this; 730 } 731 732 /** 733 * @return {@link #postalCode} (A postal code designating a region defined by the postal service.). This is the underlying object with id, value and extensions. The accessor "getPostalCode" gives direct access to the value 734 */ 735 public StringType getPostalCodeElement() { 736 if (this.postalCode == null) 737 if (Configuration.errorOnAutoCreate()) 738 throw new Error("Attempt to auto-create Address.postalCode"); 739 else if (Configuration.doAutoCreate()) 740 this.postalCode = new StringType(); // bb 741 return this.postalCode; 742 } 743 744 public boolean hasPostalCodeElement() { 745 return this.postalCode != null && !this.postalCode.isEmpty(); 746 } 747 748 public boolean hasPostalCode() { 749 return this.postalCode != null && !this.postalCode.isEmpty(); 750 } 751 752 /** 753 * @param value {@link #postalCode} (A postal code designating a region defined by the postal service.). This is the underlying object with id, value and extensions. The accessor "getPostalCode" gives direct access to the value 754 */ 755 public Address setPostalCodeElement(StringType value) { 756 this.postalCode = value; 757 return this; 758 } 759 760 /** 761 * @return A postal code designating a region defined by the postal service. 762 */ 763 public String getPostalCode() { 764 return this.postalCode == null ? null : this.postalCode.getValue(); 765 } 766 767 /** 768 * @param value A postal code designating a region defined by the postal service. 769 */ 770 public Address setPostalCode(String value) { 771 if (Utilities.noString(value)) 772 this.postalCode = null; 773 else { 774 if (this.postalCode == null) 775 this.postalCode = new StringType(); 776 this.postalCode.setValue(value); 777 } 778 return this; 779 } 780 781 /** 782 * @return {@link #country} (Country - a nation as commonly understood or generally accepted.). This is the underlying object with id, value and extensions. The accessor "getCountry" gives direct access to the value 783 */ 784 public StringType getCountryElement() { 785 if (this.country == null) 786 if (Configuration.errorOnAutoCreate()) 787 throw new Error("Attempt to auto-create Address.country"); 788 else if (Configuration.doAutoCreate()) 789 this.country = new StringType(); // bb 790 return this.country; 791 } 792 793 public boolean hasCountryElement() { 794 return this.country != null && !this.country.isEmpty(); 795 } 796 797 public boolean hasCountry() { 798 return this.country != null && !this.country.isEmpty(); 799 } 800 801 /** 802 * @param value {@link #country} (Country - a nation as commonly understood or generally accepted.). This is the underlying object with id, value and extensions. The accessor "getCountry" gives direct access to the value 803 */ 804 public Address setCountryElement(StringType value) { 805 this.country = value; 806 return this; 807 } 808 809 /** 810 * @return Country - a nation as commonly understood or generally accepted. 811 */ 812 public String getCountry() { 813 return this.country == null ? null : this.country.getValue(); 814 } 815 816 /** 817 * @param value Country - a nation as commonly understood or generally accepted. 818 */ 819 public Address setCountry(String value) { 820 if (Utilities.noString(value)) 821 this.country = null; 822 else { 823 if (this.country == null) 824 this.country = new StringType(); 825 this.country.setValue(value); 826 } 827 return this; 828 } 829 830 /** 831 * @return {@link #period} (Time period when address was/is in use.) 832 */ 833 public Period getPeriod() { 834 if (this.period == null) 835 if (Configuration.errorOnAutoCreate()) 836 throw new Error("Attempt to auto-create Address.period"); 837 else if (Configuration.doAutoCreate()) 838 this.period = new Period(); // cc 839 return this.period; 840 } 841 842 public boolean hasPeriod() { 843 return this.period != null && !this.period.isEmpty(); 844 } 845 846 /** 847 * @param value {@link #period} (Time period when address was/is in use.) 848 */ 849 public Address setPeriod(Period value) { 850 this.period = value; 851 return this; 852 } 853 854 protected void listChildren(List<Property> children) { 855 super.listChildren(children); 856 children.add(new Property("use", "code", "The purpose of this address.", 0, 1, use)); 857 children.add(new Property("type", "code", "Distinguishes between physical addresses (those you can visit) and mailing addresses (e.g. PO Boxes and care-of addresses). Most addresses are both.", 0, 1, type)); 858 children.add(new Property("text", "string", "A full text representation of the address.", 0, 1, text)); 859 children.add(new Property("line", "string", "This component contains the house number, apartment number, street name, street direction, P.O. Box number, delivery hints, and similar address information.", 0, java.lang.Integer.MAX_VALUE, line)); 860 children.add(new Property("city", "string", "The name of the city, town, village or other community or delivery center.", 0, 1, city)); 861 children.add(new Property("district", "string", "The name of the administrative area (county).", 0, 1, district)); 862 children.add(new Property("state", "string", "Sub-unit of a country with limited sovereignty in a federally organized country. A code may be used if codes are in common use (i.e. US 2 letter state codes).", 0, 1, state)); 863 children.add(new Property("postalCode", "string", "A postal code designating a region defined by the postal service.", 0, 1, postalCode)); 864 children.add(new Property("country", "string", "Country - a nation as commonly understood or generally accepted.", 0, 1, country)); 865 children.add(new Property("period", "Period", "Time period when address was/is in use.", 0, 1, period)); 866 } 867 868 @Override 869 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 870 switch (_hash) { 871 case 116103: /*use*/ return new Property("use", "code", "The purpose of this address.", 0, 1, use); 872 case 3575610: /*type*/ return new Property("type", "code", "Distinguishes between physical addresses (those you can visit) and mailing addresses (e.g. PO Boxes and care-of addresses). Most addresses are both.", 0, 1, type); 873 case 3556653: /*text*/ return new Property("text", "string", "A full text representation of the address.", 0, 1, text); 874 case 3321844: /*line*/ return new Property("line", "string", "This component contains the house number, apartment number, street name, street direction, P.O. Box number, delivery hints, and similar address information.", 0, java.lang.Integer.MAX_VALUE, line); 875 case 3053931: /*city*/ return new Property("city", "string", "The name of the city, town, village or other community or delivery center.", 0, 1, city); 876 case 288961422: /*district*/ return new Property("district", "string", "The name of the administrative area (county).", 0, 1, district); 877 case 109757585: /*state*/ return new Property("state", "string", "Sub-unit of a country with limited sovereignty in a federally organized country. A code may be used if codes are in common use (i.e. US 2 letter state codes).", 0, 1, state); 878 case 2011152728: /*postalCode*/ return new Property("postalCode", "string", "A postal code designating a region defined by the postal service.", 0, 1, postalCode); 879 case 957831062: /*country*/ return new Property("country", "string", "Country - a nation as commonly understood or generally accepted.", 0, 1, country); 880 case -991726143: /*period*/ return new Property("period", "Period", "Time period when address was/is in use.", 0, 1, period); 881 default: return super.getNamedProperty(_hash, _name, _checkValid); 882 } 883 884 } 885 886 @Override 887 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 888 switch (hash) { 889 case 116103: /*use*/ return this.use == null ? new Base[0] : new Base[] {this.use}; // Enumeration<AddressUse> 890 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<AddressType> 891 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // StringType 892 case 3321844: /*line*/ return this.line == null ? new Base[0] : this.line.toArray(new Base[this.line.size()]); // StringType 893 case 3053931: /*city*/ return this.city == null ? new Base[0] : new Base[] {this.city}; // StringType 894 case 288961422: /*district*/ return this.district == null ? new Base[0] : new Base[] {this.district}; // StringType 895 case 109757585: /*state*/ return this.state == null ? new Base[0] : new Base[] {this.state}; // StringType 896 case 2011152728: /*postalCode*/ return this.postalCode == null ? new Base[0] : new Base[] {this.postalCode}; // StringType 897 case 957831062: /*country*/ return this.country == null ? new Base[0] : new Base[] {this.country}; // StringType 898 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 899 default: return super.getProperty(hash, name, checkValid); 900 } 901 902 } 903 904 @Override 905 public Base setProperty(int hash, String name, Base value) throws FHIRException { 906 switch (hash) { 907 case 116103: // use 908 value = new AddressUseEnumFactory().fromType(castToCode(value)); 909 this.use = (Enumeration) value; // Enumeration<AddressUse> 910 return value; 911 case 3575610: // type 912 value = new AddressTypeEnumFactory().fromType(castToCode(value)); 913 this.type = (Enumeration) value; // Enumeration<AddressType> 914 return value; 915 case 3556653: // text 916 this.text = castToString(value); // StringType 917 return value; 918 case 3321844: // line 919 this.getLine().add(castToString(value)); // StringType 920 return value; 921 case 3053931: // city 922 this.city = castToString(value); // StringType 923 return value; 924 case 288961422: // district 925 this.district = castToString(value); // StringType 926 return value; 927 case 109757585: // state 928 this.state = castToString(value); // StringType 929 return value; 930 case 2011152728: // postalCode 931 this.postalCode = castToString(value); // StringType 932 return value; 933 case 957831062: // country 934 this.country = castToString(value); // StringType 935 return value; 936 case -991726143: // period 937 this.period = castToPeriod(value); // Period 938 return value; 939 default: return super.setProperty(hash, name, value); 940 } 941 942 } 943 944 @Override 945 public Base setProperty(String name, Base value) throws FHIRException { 946 if (name.equals("use")) { 947 value = new AddressUseEnumFactory().fromType(castToCode(value)); 948 this.use = (Enumeration) value; // Enumeration<AddressUse> 949 } else if (name.equals("type")) { 950 value = new AddressTypeEnumFactory().fromType(castToCode(value)); 951 this.type = (Enumeration) value; // Enumeration<AddressType> 952 } else if (name.equals("text")) { 953 this.text = castToString(value); // StringType 954 } else if (name.equals("line")) { 955 this.getLine().add(castToString(value)); 956 } else if (name.equals("city")) { 957 this.city = castToString(value); // StringType 958 } else if (name.equals("district")) { 959 this.district = castToString(value); // StringType 960 } else if (name.equals("state")) { 961 this.state = castToString(value); // StringType 962 } else if (name.equals("postalCode")) { 963 this.postalCode = castToString(value); // StringType 964 } else if (name.equals("country")) { 965 this.country = castToString(value); // StringType 966 } else if (name.equals("period")) { 967 this.period = castToPeriod(value); // Period 968 } else 969 return super.setProperty(name, value); 970 return value; 971 } 972 973 @Override 974 public Base makeProperty(int hash, String name) throws FHIRException { 975 switch (hash) { 976 case 116103: return getUseElement(); 977 case 3575610: return getTypeElement(); 978 case 3556653: return getTextElement(); 979 case 3321844: return addLineElement(); 980 case 3053931: return getCityElement(); 981 case 288961422: return getDistrictElement(); 982 case 109757585: return getStateElement(); 983 case 2011152728: return getPostalCodeElement(); 984 case 957831062: return getCountryElement(); 985 case -991726143: return getPeriod(); 986 default: return super.makeProperty(hash, name); 987 } 988 989 } 990 991 @Override 992 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 993 switch (hash) { 994 case 116103: /*use*/ return new String[] {"code"}; 995 case 3575610: /*type*/ return new String[] {"code"}; 996 case 3556653: /*text*/ return new String[] {"string"}; 997 case 3321844: /*line*/ return new String[] {"string"}; 998 case 3053931: /*city*/ return new String[] {"string"}; 999 case 288961422: /*district*/ return new String[] {"string"}; 1000 case 109757585: /*state*/ return new String[] {"string"}; 1001 case 2011152728: /*postalCode*/ return new String[] {"string"}; 1002 case 957831062: /*country*/ return new String[] {"string"}; 1003 case -991726143: /*period*/ return new String[] {"Period"}; 1004 default: return super.getTypesForProperty(hash, name); 1005 } 1006 1007 } 1008 1009 @Override 1010 public Base addChild(String name) throws FHIRException { 1011 if (name.equals("use")) { 1012 throw new FHIRException("Cannot call addChild on a singleton property Address.use"); 1013 } 1014 else if (name.equals("type")) { 1015 throw new FHIRException("Cannot call addChild on a singleton property Address.type"); 1016 } 1017 else if (name.equals("text")) { 1018 throw new FHIRException("Cannot call addChild on a singleton property Address.text"); 1019 } 1020 else if (name.equals("line")) { 1021 throw new FHIRException("Cannot call addChild on a singleton property Address.line"); 1022 } 1023 else if (name.equals("city")) { 1024 throw new FHIRException("Cannot call addChild on a singleton property Address.city"); 1025 } 1026 else if (name.equals("district")) { 1027 throw new FHIRException("Cannot call addChild on a singleton property Address.district"); 1028 } 1029 else if (name.equals("state")) { 1030 throw new FHIRException("Cannot call addChild on a singleton property Address.state"); 1031 } 1032 else if (name.equals("postalCode")) { 1033 throw new FHIRException("Cannot call addChild on a singleton property Address.postalCode"); 1034 } 1035 else if (name.equals("country")) { 1036 throw new FHIRException("Cannot call addChild on a singleton property Address.country"); 1037 } 1038 else if (name.equals("period")) { 1039 this.period = new Period(); 1040 return this.period; 1041 } 1042 else 1043 return super.addChild(name); 1044 } 1045 1046 public String fhirType() { 1047 return "Address"; 1048 1049 } 1050 1051 public Address copy() { 1052 Address dst = new Address(); 1053 copyValues(dst); 1054 dst.use = use == null ? null : use.copy(); 1055 dst.type = type == null ? null : type.copy(); 1056 dst.text = text == null ? null : text.copy(); 1057 if (line != null) { 1058 dst.line = new ArrayList<StringType>(); 1059 for (StringType i : line) 1060 dst.line.add(i.copy()); 1061 }; 1062 dst.city = city == null ? null : city.copy(); 1063 dst.district = district == null ? null : district.copy(); 1064 dst.state = state == null ? null : state.copy(); 1065 dst.postalCode = postalCode == null ? null : postalCode.copy(); 1066 dst.country = country == null ? null : country.copy(); 1067 dst.period = period == null ? null : period.copy(); 1068 return dst; 1069 } 1070 1071 protected Address typedCopy() { 1072 return copy(); 1073 } 1074 1075 @Override 1076 public boolean equalsDeep(Base other_) { 1077 if (!super.equalsDeep(other_)) 1078 return false; 1079 if (!(other_ instanceof Address)) 1080 return false; 1081 Address o = (Address) other_; 1082 return compareDeep(use, o.use, true) && compareDeep(type, o.type, true) && compareDeep(text, o.text, true) 1083 && compareDeep(line, o.line, true) && compareDeep(city, o.city, true) && compareDeep(district, o.district, true) 1084 && compareDeep(state, o.state, true) && compareDeep(postalCode, o.postalCode, true) && compareDeep(country, o.country, true) 1085 && compareDeep(period, o.period, true); 1086 } 1087 1088 @Override 1089 public boolean equalsShallow(Base other_) { 1090 if (!super.equalsShallow(other_)) 1091 return false; 1092 if (!(other_ instanceof Address)) 1093 return false; 1094 Address o = (Address) other_; 1095 return compareValues(use, o.use, true) && compareValues(type, o.type, true) && compareValues(text, o.text, true) 1096 && compareValues(line, o.line, true) && compareValues(city, o.city, true) && compareValues(district, o.district, true) 1097 && compareValues(state, o.state, true) && compareValues(postalCode, o.postalCode, true) && compareValues(country, o.country, true) 1098 ; 1099 } 1100 1101 public boolean isEmpty() { 1102 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(use, type, text, line 1103 , city, district, state, postalCode, country, period); 1104 } 1105 1106 1107}