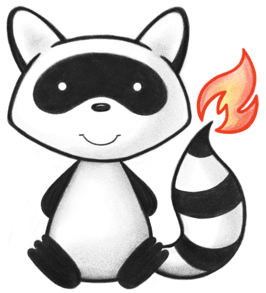
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048/** 049 * Actual or potential/avoided event causing unintended physical injury resulting from or contributed to by medical care, a research study or other healthcare setting factors that requires additional monitoring, treatment, or hospitalization, or that results in death. 050 */ 051@ResourceDef(name="AdverseEvent", profile="http://hl7.org/fhir/Profile/AdverseEvent") 052public class AdverseEvent extends DomainResource { 053 054 public enum AdverseEventCategory { 055 /** 056 * null 057 */ 058 AE, 059 /** 060 * null 061 */ 062 PAE, 063 /** 064 * added to help the parsers with the generic types 065 */ 066 NULL; 067 public static AdverseEventCategory fromCode(String codeString) throws FHIRException { 068 if (codeString == null || "".equals(codeString)) 069 return null; 070 if ("AE".equals(codeString)) 071 return AE; 072 if ("PAE".equals(codeString)) 073 return PAE; 074 if (Configuration.isAcceptInvalidEnums()) 075 return null; 076 else 077 throw new FHIRException("Unknown AdverseEventCategory code '"+codeString+"'"); 078 } 079 public String toCode() { 080 switch (this) { 081 case AE: return "AE"; 082 case PAE: return "PAE"; 083 case NULL: return null; 084 default: return "?"; 085 } 086 } 087 public String getSystem() { 088 switch (this) { 089 case AE: return "http://hl7.org/fhir/adverse-event-category"; 090 case PAE: return "http://hl7.org/fhir/adverse-event-category"; 091 case NULL: return null; 092 default: return "?"; 093 } 094 } 095 public String getDefinition() { 096 switch (this) { 097 case AE: return ""; 098 case PAE: return ""; 099 case NULL: return null; 100 default: return "?"; 101 } 102 } 103 public String getDisplay() { 104 switch (this) { 105 case AE: return "Adverse Event"; 106 case PAE: return "Potential Adverse Event"; 107 case NULL: return null; 108 default: return "?"; 109 } 110 } 111 } 112 113 public static class AdverseEventCategoryEnumFactory implements EnumFactory<AdverseEventCategory> { 114 public AdverseEventCategory fromCode(String codeString) throws IllegalArgumentException { 115 if (codeString == null || "".equals(codeString)) 116 if (codeString == null || "".equals(codeString)) 117 return null; 118 if ("AE".equals(codeString)) 119 return AdverseEventCategory.AE; 120 if ("PAE".equals(codeString)) 121 return AdverseEventCategory.PAE; 122 throw new IllegalArgumentException("Unknown AdverseEventCategory code '"+codeString+"'"); 123 } 124 public Enumeration<AdverseEventCategory> fromType(PrimitiveType<?> code) throws FHIRException { 125 if (code == null) 126 return null; 127 if (code.isEmpty()) 128 return new Enumeration<AdverseEventCategory>(this); 129 String codeString = code.asStringValue(); 130 if (codeString == null || "".equals(codeString)) 131 return null; 132 if ("AE".equals(codeString)) 133 return new Enumeration<AdverseEventCategory>(this, AdverseEventCategory.AE); 134 if ("PAE".equals(codeString)) 135 return new Enumeration<AdverseEventCategory>(this, AdverseEventCategory.PAE); 136 throw new FHIRException("Unknown AdverseEventCategory code '"+codeString+"'"); 137 } 138 public String toCode(AdverseEventCategory code) { 139 if (code == AdverseEventCategory.NULL) 140 return null; 141 if (code == AdverseEventCategory.AE) 142 return "AE"; 143 if (code == AdverseEventCategory.PAE) 144 return "PAE"; 145 return "?"; 146 } 147 public String toSystem(AdverseEventCategory code) { 148 return code.getSystem(); 149 } 150 } 151 152 public enum AdverseEventCausality { 153 /** 154 * null 155 */ 156 CAUSALITY1, 157 /** 158 * null 159 */ 160 CAUSALITY2, 161 /** 162 * added to help the parsers with the generic types 163 */ 164 NULL; 165 public static AdverseEventCausality fromCode(String codeString) throws FHIRException { 166 if (codeString == null || "".equals(codeString)) 167 return null; 168 if ("causality1".equals(codeString)) 169 return CAUSALITY1; 170 if ("causality2".equals(codeString)) 171 return CAUSALITY2; 172 if (Configuration.isAcceptInvalidEnums()) 173 return null; 174 else 175 throw new FHIRException("Unknown AdverseEventCausality code '"+codeString+"'"); 176 } 177 public String toCode() { 178 switch (this) { 179 case CAUSALITY1: return "causality1"; 180 case CAUSALITY2: return "causality2"; 181 case NULL: return null; 182 default: return "?"; 183 } 184 } 185 public String getSystem() { 186 switch (this) { 187 case CAUSALITY1: return "http://hl7.org/fhir/adverse-event-causality"; 188 case CAUSALITY2: return "http://hl7.org/fhir/adverse-event-causality"; 189 case NULL: return null; 190 default: return "?"; 191 } 192 } 193 public String getDefinition() { 194 switch (this) { 195 case CAUSALITY1: return ""; 196 case CAUSALITY2: return ""; 197 case NULL: return null; 198 default: return "?"; 199 } 200 } 201 public String getDisplay() { 202 switch (this) { 203 case CAUSALITY1: return "causality1 placeholder"; 204 case CAUSALITY2: return "causality2 placeholder"; 205 case NULL: return null; 206 default: return "?"; 207 } 208 } 209 } 210 211 public static class AdverseEventCausalityEnumFactory implements EnumFactory<AdverseEventCausality> { 212 public AdverseEventCausality fromCode(String codeString) throws IllegalArgumentException { 213 if (codeString == null || "".equals(codeString)) 214 if (codeString == null || "".equals(codeString)) 215 return null; 216 if ("causality1".equals(codeString)) 217 return AdverseEventCausality.CAUSALITY1; 218 if ("causality2".equals(codeString)) 219 return AdverseEventCausality.CAUSALITY2; 220 throw new IllegalArgumentException("Unknown AdverseEventCausality code '"+codeString+"'"); 221 } 222 public Enumeration<AdverseEventCausality> fromType(PrimitiveType<?> code) throws FHIRException { 223 if (code == null) 224 return null; 225 if (code.isEmpty()) 226 return new Enumeration<AdverseEventCausality>(this); 227 String codeString = code.asStringValue(); 228 if (codeString == null || "".equals(codeString)) 229 return null; 230 if ("causality1".equals(codeString)) 231 return new Enumeration<AdverseEventCausality>(this, AdverseEventCausality.CAUSALITY1); 232 if ("causality2".equals(codeString)) 233 return new Enumeration<AdverseEventCausality>(this, AdverseEventCausality.CAUSALITY2); 234 throw new FHIRException("Unknown AdverseEventCausality code '"+codeString+"'"); 235 } 236 public String toCode(AdverseEventCausality code) { 237 if (code == AdverseEventCausality.NULL) 238 return null; 239 if (code == AdverseEventCausality.CAUSALITY1) 240 return "causality1"; 241 if (code == AdverseEventCausality.CAUSALITY2) 242 return "causality2"; 243 return "?"; 244 } 245 public String toSystem(AdverseEventCausality code) { 246 return code.getSystem(); 247 } 248 } 249 250 @Block() 251 public static class AdverseEventSuspectEntityComponent extends BackboneElement implements IBaseBackboneElement { 252 /** 253 * Identifies the actual instance of what caused the adverse event. May be a substance, medication, medication administration, medication statement or a device. 254 */ 255 @Child(name = "instance", type = {Substance.class, Medication.class, MedicationAdministration.class, MedicationStatement.class, Device.class}, order=1, min=1, max=1, modifier=false, summary=true) 256 @Description(shortDefinition="Refers to the specific entity that caused the adverse event", formalDefinition="Identifies the actual instance of what caused the adverse event. May be a substance, medication, medication administration, medication statement or a device." ) 257 protected Reference instance; 258 259 /** 260 * The actual object that is the target of the reference (Identifies the actual instance of what caused the adverse event. May be a substance, medication, medication administration, medication statement or a device.) 261 */ 262 protected Resource instanceTarget; 263 264 /** 265 * causality1 | causality2. 266 */ 267 @Child(name = "causality", type = {CodeType.class}, order=2, min=0, max=1, modifier=false, summary=true) 268 @Description(shortDefinition="causality1 | causality2", formalDefinition="causality1 | causality2." ) 269 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adverse-event-causality") 270 protected Enumeration<AdverseEventCausality> causality; 271 272 /** 273 * assess1 | assess2. 274 */ 275 @Child(name = "causalityAssessment", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 276 @Description(shortDefinition="assess1 | assess2", formalDefinition="assess1 | assess2." ) 277 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adverse-event-causality-assess") 278 protected CodeableConcept causalityAssessment; 279 280 /** 281 * AdverseEvent.suspectEntity.causalityProductRelatedness. 282 */ 283 @Child(name = "causalityProductRelatedness", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 284 @Description(shortDefinition="AdverseEvent.suspectEntity.causalityProductRelatedness", formalDefinition="AdverseEvent.suspectEntity.causalityProductRelatedness." ) 285 protected StringType causalityProductRelatedness; 286 287 /** 288 * method1 | method2. 289 */ 290 @Child(name = "causalityMethod", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=true) 291 @Description(shortDefinition="method1 | method2", formalDefinition="method1 | method2." ) 292 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adverse-event-causality-method") 293 protected CodeableConcept causalityMethod; 294 295 /** 296 * AdverseEvent.suspectEntity.causalityAuthor. 297 */ 298 @Child(name = "causalityAuthor", type = {Practitioner.class, PractitionerRole.class}, order=6, min=0, max=1, modifier=false, summary=true) 299 @Description(shortDefinition="AdverseEvent.suspectEntity.causalityAuthor", formalDefinition="AdverseEvent.suspectEntity.causalityAuthor." ) 300 protected Reference causalityAuthor; 301 302 /** 303 * The actual object that is the target of the reference (AdverseEvent.suspectEntity.causalityAuthor.) 304 */ 305 protected Resource causalityAuthorTarget; 306 307 /** 308 * result1 | result2. 309 */ 310 @Child(name = "causalityResult", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=true) 311 @Description(shortDefinition="result1 | result2", formalDefinition="result1 | result2." ) 312 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adverse-event-causality-result") 313 protected CodeableConcept causalityResult; 314 315 private static final long serialVersionUID = -815429592L; 316 317 /** 318 * Constructor 319 */ 320 public AdverseEventSuspectEntityComponent() { 321 super(); 322 } 323 324 /** 325 * Constructor 326 */ 327 public AdverseEventSuspectEntityComponent(Reference instance) { 328 super(); 329 this.instance = instance; 330 } 331 332 /** 333 * @return {@link #instance} (Identifies the actual instance of what caused the adverse event. May be a substance, medication, medication administration, medication statement or a device.) 334 */ 335 public Reference getInstance() { 336 if (this.instance == null) 337 if (Configuration.errorOnAutoCreate()) 338 throw new Error("Attempt to auto-create AdverseEventSuspectEntityComponent.instance"); 339 else if (Configuration.doAutoCreate()) 340 this.instance = new Reference(); // cc 341 return this.instance; 342 } 343 344 public boolean hasInstance() { 345 return this.instance != null && !this.instance.isEmpty(); 346 } 347 348 /** 349 * @param value {@link #instance} (Identifies the actual instance of what caused the adverse event. May be a substance, medication, medication administration, medication statement or a device.) 350 */ 351 public AdverseEventSuspectEntityComponent setInstance(Reference value) { 352 this.instance = value; 353 return this; 354 } 355 356 /** 357 * @return {@link #instance} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Identifies the actual instance of what caused the adverse event. May be a substance, medication, medication administration, medication statement or a device.) 358 */ 359 public Resource getInstanceTarget() { 360 return this.instanceTarget; 361 } 362 363 /** 364 * @param value {@link #instance} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Identifies the actual instance of what caused the adverse event. May be a substance, medication, medication administration, medication statement or a device.) 365 */ 366 public AdverseEventSuspectEntityComponent setInstanceTarget(Resource value) { 367 this.instanceTarget = value; 368 return this; 369 } 370 371 /** 372 * @return {@link #causality} (causality1 | causality2.). This is the underlying object with id, value and extensions. The accessor "getCausality" gives direct access to the value 373 */ 374 public Enumeration<AdverseEventCausality> getCausalityElement() { 375 if (this.causality == null) 376 if (Configuration.errorOnAutoCreate()) 377 throw new Error("Attempt to auto-create AdverseEventSuspectEntityComponent.causality"); 378 else if (Configuration.doAutoCreate()) 379 this.causality = new Enumeration<AdverseEventCausality>(new AdverseEventCausalityEnumFactory()); // bb 380 return this.causality; 381 } 382 383 public boolean hasCausalityElement() { 384 return this.causality != null && !this.causality.isEmpty(); 385 } 386 387 public boolean hasCausality() { 388 return this.causality != null && !this.causality.isEmpty(); 389 } 390 391 /** 392 * @param value {@link #causality} (causality1 | causality2.). This is the underlying object with id, value and extensions. The accessor "getCausality" gives direct access to the value 393 */ 394 public AdverseEventSuspectEntityComponent setCausalityElement(Enumeration<AdverseEventCausality> value) { 395 this.causality = value; 396 return this; 397 } 398 399 /** 400 * @return causality1 | causality2. 401 */ 402 public AdverseEventCausality getCausality() { 403 return this.causality == null ? null : this.causality.getValue(); 404 } 405 406 /** 407 * @param value causality1 | causality2. 408 */ 409 public AdverseEventSuspectEntityComponent setCausality(AdverseEventCausality value) { 410 if (value == null) 411 this.causality = null; 412 else { 413 if (this.causality == null) 414 this.causality = new Enumeration<AdverseEventCausality>(new AdverseEventCausalityEnumFactory()); 415 this.causality.setValue(value); 416 } 417 return this; 418 } 419 420 /** 421 * @return {@link #causalityAssessment} (assess1 | assess2.) 422 */ 423 public CodeableConcept getCausalityAssessment() { 424 if (this.causalityAssessment == null) 425 if (Configuration.errorOnAutoCreate()) 426 throw new Error("Attempt to auto-create AdverseEventSuspectEntityComponent.causalityAssessment"); 427 else if (Configuration.doAutoCreate()) 428 this.causalityAssessment = new CodeableConcept(); // cc 429 return this.causalityAssessment; 430 } 431 432 public boolean hasCausalityAssessment() { 433 return this.causalityAssessment != null && !this.causalityAssessment.isEmpty(); 434 } 435 436 /** 437 * @param value {@link #causalityAssessment} (assess1 | assess2.) 438 */ 439 public AdverseEventSuspectEntityComponent setCausalityAssessment(CodeableConcept value) { 440 this.causalityAssessment = value; 441 return this; 442 } 443 444 /** 445 * @return {@link #causalityProductRelatedness} (AdverseEvent.suspectEntity.causalityProductRelatedness.). This is the underlying object with id, value and extensions. The accessor "getCausalityProductRelatedness" gives direct access to the value 446 */ 447 public StringType getCausalityProductRelatednessElement() { 448 if (this.causalityProductRelatedness == null) 449 if (Configuration.errorOnAutoCreate()) 450 throw new Error("Attempt to auto-create AdverseEventSuspectEntityComponent.causalityProductRelatedness"); 451 else if (Configuration.doAutoCreate()) 452 this.causalityProductRelatedness = new StringType(); // bb 453 return this.causalityProductRelatedness; 454 } 455 456 public boolean hasCausalityProductRelatednessElement() { 457 return this.causalityProductRelatedness != null && !this.causalityProductRelatedness.isEmpty(); 458 } 459 460 public boolean hasCausalityProductRelatedness() { 461 return this.causalityProductRelatedness != null && !this.causalityProductRelatedness.isEmpty(); 462 } 463 464 /** 465 * @param value {@link #causalityProductRelatedness} (AdverseEvent.suspectEntity.causalityProductRelatedness.). This is the underlying object with id, value and extensions. The accessor "getCausalityProductRelatedness" gives direct access to the value 466 */ 467 public AdverseEventSuspectEntityComponent setCausalityProductRelatednessElement(StringType value) { 468 this.causalityProductRelatedness = value; 469 return this; 470 } 471 472 /** 473 * @return AdverseEvent.suspectEntity.causalityProductRelatedness. 474 */ 475 public String getCausalityProductRelatedness() { 476 return this.causalityProductRelatedness == null ? null : this.causalityProductRelatedness.getValue(); 477 } 478 479 /** 480 * @param value AdverseEvent.suspectEntity.causalityProductRelatedness. 481 */ 482 public AdverseEventSuspectEntityComponent setCausalityProductRelatedness(String value) { 483 if (Utilities.noString(value)) 484 this.causalityProductRelatedness = null; 485 else { 486 if (this.causalityProductRelatedness == null) 487 this.causalityProductRelatedness = new StringType(); 488 this.causalityProductRelatedness.setValue(value); 489 } 490 return this; 491 } 492 493 /** 494 * @return {@link #causalityMethod} (method1 | method2.) 495 */ 496 public CodeableConcept getCausalityMethod() { 497 if (this.causalityMethod == null) 498 if (Configuration.errorOnAutoCreate()) 499 throw new Error("Attempt to auto-create AdverseEventSuspectEntityComponent.causalityMethod"); 500 else if (Configuration.doAutoCreate()) 501 this.causalityMethod = new CodeableConcept(); // cc 502 return this.causalityMethod; 503 } 504 505 public boolean hasCausalityMethod() { 506 return this.causalityMethod != null && !this.causalityMethod.isEmpty(); 507 } 508 509 /** 510 * @param value {@link #causalityMethod} (method1 | method2.) 511 */ 512 public AdverseEventSuspectEntityComponent setCausalityMethod(CodeableConcept value) { 513 this.causalityMethod = value; 514 return this; 515 } 516 517 /** 518 * @return {@link #causalityAuthor} (AdverseEvent.suspectEntity.causalityAuthor.) 519 */ 520 public Reference getCausalityAuthor() { 521 if (this.causalityAuthor == null) 522 if (Configuration.errorOnAutoCreate()) 523 throw new Error("Attempt to auto-create AdverseEventSuspectEntityComponent.causalityAuthor"); 524 else if (Configuration.doAutoCreate()) 525 this.causalityAuthor = new Reference(); // cc 526 return this.causalityAuthor; 527 } 528 529 public boolean hasCausalityAuthor() { 530 return this.causalityAuthor != null && !this.causalityAuthor.isEmpty(); 531 } 532 533 /** 534 * @param value {@link #causalityAuthor} (AdverseEvent.suspectEntity.causalityAuthor.) 535 */ 536 public AdverseEventSuspectEntityComponent setCausalityAuthor(Reference value) { 537 this.causalityAuthor = value; 538 return this; 539 } 540 541 /** 542 * @return {@link #causalityAuthor} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (AdverseEvent.suspectEntity.causalityAuthor.) 543 */ 544 public Resource getCausalityAuthorTarget() { 545 return this.causalityAuthorTarget; 546 } 547 548 /** 549 * @param value {@link #causalityAuthor} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (AdverseEvent.suspectEntity.causalityAuthor.) 550 */ 551 public AdverseEventSuspectEntityComponent setCausalityAuthorTarget(Resource value) { 552 this.causalityAuthorTarget = value; 553 return this; 554 } 555 556 /** 557 * @return {@link #causalityResult} (result1 | result2.) 558 */ 559 public CodeableConcept getCausalityResult() { 560 if (this.causalityResult == null) 561 if (Configuration.errorOnAutoCreate()) 562 throw new Error("Attempt to auto-create AdverseEventSuspectEntityComponent.causalityResult"); 563 else if (Configuration.doAutoCreate()) 564 this.causalityResult = new CodeableConcept(); // cc 565 return this.causalityResult; 566 } 567 568 public boolean hasCausalityResult() { 569 return this.causalityResult != null && !this.causalityResult.isEmpty(); 570 } 571 572 /** 573 * @param value {@link #causalityResult} (result1 | result2.) 574 */ 575 public AdverseEventSuspectEntityComponent setCausalityResult(CodeableConcept value) { 576 this.causalityResult = value; 577 return this; 578 } 579 580 protected void listChildren(List<Property> children) { 581 super.listChildren(children); 582 children.add(new Property("instance", "Reference(Substance|Medication|MedicationAdministration|MedicationStatement|Device)", "Identifies the actual instance of what caused the adverse event. May be a substance, medication, medication administration, medication statement or a device.", 0, 1, instance)); 583 children.add(new Property("causality", "code", "causality1 | causality2.", 0, 1, causality)); 584 children.add(new Property("causalityAssessment", "CodeableConcept", "assess1 | assess2.", 0, 1, causalityAssessment)); 585 children.add(new Property("causalityProductRelatedness", "string", "AdverseEvent.suspectEntity.causalityProductRelatedness.", 0, 1, causalityProductRelatedness)); 586 children.add(new Property("causalityMethod", "CodeableConcept", "method1 | method2.", 0, 1, causalityMethod)); 587 children.add(new Property("causalityAuthor", "Reference(Practitioner|PractitionerRole)", "AdverseEvent.suspectEntity.causalityAuthor.", 0, 1, causalityAuthor)); 588 children.add(new Property("causalityResult", "CodeableConcept", "result1 | result2.", 0, 1, causalityResult)); 589 } 590 591 @Override 592 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 593 switch (_hash) { 594 case 555127957: /*instance*/ return new Property("instance", "Reference(Substance|Medication|MedicationAdministration|MedicationStatement|Device)", "Identifies the actual instance of what caused the adverse event. May be a substance, medication, medication administration, medication statement or a device.", 0, 1, instance); 595 case -1446450521: /*causality*/ return new Property("causality", "code", "causality1 | causality2.", 0, 1, causality); 596 case 830609609: /*causalityAssessment*/ return new Property("causalityAssessment", "CodeableConcept", "assess1 | assess2.", 0, 1, causalityAssessment); 597 case -1983069286: /*causalityProductRelatedness*/ return new Property("causalityProductRelatedness", "string", "AdverseEvent.suspectEntity.causalityProductRelatedness.", 0, 1, causalityProductRelatedness); 598 case -1320366488: /*causalityMethod*/ return new Property("causalityMethod", "CodeableConcept", "method1 | method2.", 0, 1, causalityMethod); 599 case -1649139950: /*causalityAuthor*/ return new Property("causalityAuthor", "Reference(Practitioner|PractitionerRole)", "AdverseEvent.suspectEntity.causalityAuthor.", 0, 1, causalityAuthor); 600 case -1177238108: /*causalityResult*/ return new Property("causalityResult", "CodeableConcept", "result1 | result2.", 0, 1, causalityResult); 601 default: return super.getNamedProperty(_hash, _name, _checkValid); 602 } 603 604 } 605 606 @Override 607 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 608 switch (hash) { 609 case 555127957: /*instance*/ return this.instance == null ? new Base[0] : new Base[] {this.instance}; // Reference 610 case -1446450521: /*causality*/ return this.causality == null ? new Base[0] : new Base[] {this.causality}; // Enumeration<AdverseEventCausality> 611 case 830609609: /*causalityAssessment*/ return this.causalityAssessment == null ? new Base[0] : new Base[] {this.causalityAssessment}; // CodeableConcept 612 case -1983069286: /*causalityProductRelatedness*/ return this.causalityProductRelatedness == null ? new Base[0] : new Base[] {this.causalityProductRelatedness}; // StringType 613 case -1320366488: /*causalityMethod*/ return this.causalityMethod == null ? new Base[0] : new Base[] {this.causalityMethod}; // CodeableConcept 614 case -1649139950: /*causalityAuthor*/ return this.causalityAuthor == null ? new Base[0] : new Base[] {this.causalityAuthor}; // Reference 615 case -1177238108: /*causalityResult*/ return this.causalityResult == null ? new Base[0] : new Base[] {this.causalityResult}; // CodeableConcept 616 default: return super.getProperty(hash, name, checkValid); 617 } 618 619 } 620 621 @Override 622 public Base setProperty(int hash, String name, Base value) throws FHIRException { 623 switch (hash) { 624 case 555127957: // instance 625 this.instance = castToReference(value); // Reference 626 return value; 627 case -1446450521: // causality 628 value = new AdverseEventCausalityEnumFactory().fromType(castToCode(value)); 629 this.causality = (Enumeration) value; // Enumeration<AdverseEventCausality> 630 return value; 631 case 830609609: // causalityAssessment 632 this.causalityAssessment = castToCodeableConcept(value); // CodeableConcept 633 return value; 634 case -1983069286: // causalityProductRelatedness 635 this.causalityProductRelatedness = castToString(value); // StringType 636 return value; 637 case -1320366488: // causalityMethod 638 this.causalityMethod = castToCodeableConcept(value); // CodeableConcept 639 return value; 640 case -1649139950: // causalityAuthor 641 this.causalityAuthor = castToReference(value); // Reference 642 return value; 643 case -1177238108: // causalityResult 644 this.causalityResult = castToCodeableConcept(value); // CodeableConcept 645 return value; 646 default: return super.setProperty(hash, name, value); 647 } 648 649 } 650 651 @Override 652 public Base setProperty(String name, Base value) throws FHIRException { 653 if (name.equals("instance")) { 654 this.instance = castToReference(value); // Reference 655 } else if (name.equals("causality")) { 656 value = new AdverseEventCausalityEnumFactory().fromType(castToCode(value)); 657 this.causality = (Enumeration) value; // Enumeration<AdverseEventCausality> 658 } else if (name.equals("causalityAssessment")) { 659 this.causalityAssessment = castToCodeableConcept(value); // CodeableConcept 660 } else if (name.equals("causalityProductRelatedness")) { 661 this.causalityProductRelatedness = castToString(value); // StringType 662 } else if (name.equals("causalityMethod")) { 663 this.causalityMethod = castToCodeableConcept(value); // CodeableConcept 664 } else if (name.equals("causalityAuthor")) { 665 this.causalityAuthor = castToReference(value); // Reference 666 } else if (name.equals("causalityResult")) { 667 this.causalityResult = castToCodeableConcept(value); // CodeableConcept 668 } else 669 return super.setProperty(name, value); 670 return value; 671 } 672 673 @Override 674 public Base makeProperty(int hash, String name) throws FHIRException { 675 switch (hash) { 676 case 555127957: return getInstance(); 677 case -1446450521: return getCausalityElement(); 678 case 830609609: return getCausalityAssessment(); 679 case -1983069286: return getCausalityProductRelatednessElement(); 680 case -1320366488: return getCausalityMethod(); 681 case -1649139950: return getCausalityAuthor(); 682 case -1177238108: return getCausalityResult(); 683 default: return super.makeProperty(hash, name); 684 } 685 686 } 687 688 @Override 689 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 690 switch (hash) { 691 case 555127957: /*instance*/ return new String[] {"Reference"}; 692 case -1446450521: /*causality*/ return new String[] {"code"}; 693 case 830609609: /*causalityAssessment*/ return new String[] {"CodeableConcept"}; 694 case -1983069286: /*causalityProductRelatedness*/ return new String[] {"string"}; 695 case -1320366488: /*causalityMethod*/ return new String[] {"CodeableConcept"}; 696 case -1649139950: /*causalityAuthor*/ return new String[] {"Reference"}; 697 case -1177238108: /*causalityResult*/ return new String[] {"CodeableConcept"}; 698 default: return super.getTypesForProperty(hash, name); 699 } 700 701 } 702 703 @Override 704 public Base addChild(String name) throws FHIRException { 705 if (name.equals("instance")) { 706 this.instance = new Reference(); 707 return this.instance; 708 } 709 else if (name.equals("causality")) { 710 throw new FHIRException("Cannot call addChild on a singleton property AdverseEvent.causality"); 711 } 712 else if (name.equals("causalityAssessment")) { 713 this.causalityAssessment = new CodeableConcept(); 714 return this.causalityAssessment; 715 } 716 else if (name.equals("causalityProductRelatedness")) { 717 throw new FHIRException("Cannot call addChild on a singleton property AdverseEvent.causalityProductRelatedness"); 718 } 719 else if (name.equals("causalityMethod")) { 720 this.causalityMethod = new CodeableConcept(); 721 return this.causalityMethod; 722 } 723 else if (name.equals("causalityAuthor")) { 724 this.causalityAuthor = new Reference(); 725 return this.causalityAuthor; 726 } 727 else if (name.equals("causalityResult")) { 728 this.causalityResult = new CodeableConcept(); 729 return this.causalityResult; 730 } 731 else 732 return super.addChild(name); 733 } 734 735 public AdverseEventSuspectEntityComponent copy() { 736 AdverseEventSuspectEntityComponent dst = new AdverseEventSuspectEntityComponent(); 737 copyValues(dst); 738 dst.instance = instance == null ? null : instance.copy(); 739 dst.causality = causality == null ? null : causality.copy(); 740 dst.causalityAssessment = causalityAssessment == null ? null : causalityAssessment.copy(); 741 dst.causalityProductRelatedness = causalityProductRelatedness == null ? null : causalityProductRelatedness.copy(); 742 dst.causalityMethod = causalityMethod == null ? null : causalityMethod.copy(); 743 dst.causalityAuthor = causalityAuthor == null ? null : causalityAuthor.copy(); 744 dst.causalityResult = causalityResult == null ? null : causalityResult.copy(); 745 return dst; 746 } 747 748 @Override 749 public boolean equalsDeep(Base other_) { 750 if (!super.equalsDeep(other_)) 751 return false; 752 if (!(other_ instanceof AdverseEventSuspectEntityComponent)) 753 return false; 754 AdverseEventSuspectEntityComponent o = (AdverseEventSuspectEntityComponent) other_; 755 return compareDeep(instance, o.instance, true) && compareDeep(causality, o.causality, true) && compareDeep(causalityAssessment, o.causalityAssessment, true) 756 && compareDeep(causalityProductRelatedness, o.causalityProductRelatedness, true) && compareDeep(causalityMethod, o.causalityMethod, true) 757 && compareDeep(causalityAuthor, o.causalityAuthor, true) && compareDeep(causalityResult, o.causalityResult, true) 758 ; 759 } 760 761 @Override 762 public boolean equalsShallow(Base other_) { 763 if (!super.equalsShallow(other_)) 764 return false; 765 if (!(other_ instanceof AdverseEventSuspectEntityComponent)) 766 return false; 767 AdverseEventSuspectEntityComponent o = (AdverseEventSuspectEntityComponent) other_; 768 return compareValues(causality, o.causality, true) && compareValues(causalityProductRelatedness, o.causalityProductRelatedness, true) 769 ; 770 } 771 772 public boolean isEmpty() { 773 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(instance, causality, causalityAssessment 774 , causalityProductRelatedness, causalityMethod, causalityAuthor, causalityResult); 775 } 776 777 public String fhirType() { 778 return "AdverseEvent.suspectEntity"; 779 780 } 781 782 } 783 784 /** 785 * The identifier(s) of this adverse event that are assigned by business processes and/or used to refer to it when a direct URL reference to the resource itsefl is not appropriate. 786 */ 787 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=1, modifier=false, summary=true) 788 @Description(shortDefinition="Business identifier for the event", formalDefinition="The identifier(s) of this adverse event that are assigned by business processes and/or used to refer to it when a direct URL reference to the resource itsefl is not appropriate." ) 789 protected Identifier identifier; 790 791 /** 792 * The type of event which is important to characterize what occurred and caused harm to the subject, or had the potential to cause harm to the subject. 793 */ 794 @Child(name = "category", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=true) 795 @Description(shortDefinition="AE | PAE \rAn adverse event is an event that caused harm to a patient, an adverse reaction is a something that is a subject-specific event that is a result of an exposure to a medication, food, device or environmental substance, a potential adverse event is something that occurred and that could have caused harm to a patient but did not", formalDefinition="The type of event which is important to characterize what occurred and caused harm to the subject, or had the potential to cause harm to the subject." ) 796 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adverse-event-category") 797 protected Enumeration<AdverseEventCategory> category; 798 799 /** 800 * This element defines the specific type of event that occurred or that was prevented from occurring. 801 */ 802 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 803 @Description(shortDefinition="actual | potential", formalDefinition="This element defines the specific type of event that occurred or that was prevented from occurring." ) 804 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adverse-event-type") 805 protected CodeableConcept type; 806 807 /** 808 * This subject or group impacted by the event. With a prospective adverse event, there will be no subject as the adverse event was prevented. 809 */ 810 @Child(name = "subject", type = {Patient.class, ResearchSubject.class, Medication.class, Device.class}, order=3, min=0, max=1, modifier=false, summary=true) 811 @Description(shortDefinition="Subject or group impacted by event", formalDefinition="This subject or group impacted by the event. With a prospective adverse event, there will be no subject as the adverse event was prevented." ) 812 protected Reference subject; 813 814 /** 815 * The actual object that is the target of the reference (This subject or group impacted by the event. With a prospective adverse event, there will be no subject as the adverse event was prevented.) 816 */ 817 protected Resource subjectTarget; 818 819 /** 820 * The date (and perhaps time) when the adverse event occurred. 821 */ 822 @Child(name = "date", type = {DateTimeType.class}, order=4, min=0, max=1, modifier=false, summary=true) 823 @Description(shortDefinition="When the event occurred", formalDefinition="The date (and perhaps time) when the adverse event occurred." ) 824 protected DateTimeType date; 825 826 /** 827 * Includes information about the reaction that occurred as a result of exposure to a substance (for example, a drug or a chemical). 828 */ 829 @Child(name = "reaction", type = {Condition.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 830 @Description(shortDefinition="Adverse Reaction Events linked to exposure to substance", formalDefinition="Includes information about the reaction that occurred as a result of exposure to a substance (for example, a drug or a chemical)." ) 831 protected List<Reference> reaction; 832 /** 833 * The actual objects that are the target of the reference (Includes information about the reaction that occurred as a result of exposure to a substance (for example, a drug or a chemical).) 834 */ 835 protected List<Condition> reactionTarget; 836 837 838 /** 839 * The information about where the adverse event occurred. 840 */ 841 @Child(name = "location", type = {Location.class}, order=6, min=0, max=1, modifier=false, summary=true) 842 @Description(shortDefinition="Location where adverse event occurred", formalDefinition="The information about where the adverse event occurred." ) 843 protected Reference location; 844 845 /** 846 * The actual object that is the target of the reference (The information about where the adverse event occurred.) 847 */ 848 protected Location locationTarget; 849 850 /** 851 * Describes the seriousness or severity of the adverse event. 852 */ 853 @Child(name = "seriousness", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=true) 854 @Description(shortDefinition="Mild | Moderate | Severe", formalDefinition="Describes the seriousness or severity of the adverse event." ) 855 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adverse-event-seriousness") 856 protected CodeableConcept seriousness; 857 858 /** 859 * Describes the type of outcome from the adverse event. 860 */ 861 @Child(name = "outcome", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=true) 862 @Description(shortDefinition="resolved | recovering | ongoing | resolvedWithSequelae | fatal | unknown", formalDefinition="Describes the type of outcome from the adverse event." ) 863 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adverse-event-outcome") 864 protected CodeableConcept outcome; 865 866 /** 867 * Information on who recorded the adverse event. May be the patient or a practitioner. 868 */ 869 @Child(name = "recorder", type = {Patient.class, Practitioner.class, RelatedPerson.class}, order=9, min=0, max=1, modifier=false, summary=true) 870 @Description(shortDefinition="Who recorded the adverse event", formalDefinition="Information on who recorded the adverse event. May be the patient or a practitioner." ) 871 protected Reference recorder; 872 873 /** 874 * The actual object that is the target of the reference (Information on who recorded the adverse event. May be the patient or a practitioner.) 875 */ 876 protected Resource recorderTarget; 877 878 /** 879 * Parties that may or should contribute or have contributed information to the Act. Such information includes information leading to the decision to perform the Act and how to perform the Act (e.g. consultant), information that the Act itself seeks to reveal (e.g. informant of clinical history), or information about what Act was performed (e.g. informant witness). 880 */ 881 @Child(name = "eventParticipant", type = {Practitioner.class, Device.class}, order=10, min=0, max=1, modifier=false, summary=true) 882 @Description(shortDefinition="Who was involved in the adverse event or the potential adverse event", formalDefinition="Parties that may or should contribute or have contributed information to the Act. Such information includes information leading to the decision to perform the Act and how to perform the Act (e.g. consultant), information that the Act itself seeks to reveal (e.g. informant of clinical history), or information about what Act was performed (e.g. informant witness)." ) 883 protected Reference eventParticipant; 884 885 /** 886 * The actual object that is the target of the reference (Parties that may or should contribute or have contributed information to the Act. Such information includes information leading to the decision to perform the Act and how to perform the Act (e.g. consultant), information that the Act itself seeks to reveal (e.g. informant of clinical history), or information about what Act was performed (e.g. informant witness).) 887 */ 888 protected Resource eventParticipantTarget; 889 890 /** 891 * Describes the adverse event in text. 892 */ 893 @Child(name = "description", type = {StringType.class}, order=11, min=0, max=1, modifier=false, summary=true) 894 @Description(shortDefinition="Description of the adverse event", formalDefinition="Describes the adverse event in text." ) 895 protected StringType description; 896 897 /** 898 * Describes the entity that is suspected to have caused the adverse event. 899 */ 900 @Child(name = "suspectEntity", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 901 @Description(shortDefinition="The suspected agent causing the adverse event", formalDefinition="Describes the entity that is suspected to have caused the adverse event." ) 902 protected List<AdverseEventSuspectEntityComponent> suspectEntity; 903 904 /** 905 * AdverseEvent.subjectMedicalHistory. 906 */ 907 @Child(name = "subjectMedicalHistory", type = {Condition.class, Observation.class, AllergyIntolerance.class, FamilyMemberHistory.class, Immunization.class, Procedure.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 908 @Description(shortDefinition="AdverseEvent.subjectMedicalHistory", formalDefinition="AdverseEvent.subjectMedicalHistory." ) 909 protected List<Reference> subjectMedicalHistory; 910 /** 911 * The actual objects that are the target of the reference (AdverseEvent.subjectMedicalHistory.) 912 */ 913 protected List<Resource> subjectMedicalHistoryTarget; 914 915 916 /** 917 * AdverseEvent.referenceDocument. 918 */ 919 @Child(name = "referenceDocument", type = {DocumentReference.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 920 @Description(shortDefinition="AdverseEvent.referenceDocument", formalDefinition="AdverseEvent.referenceDocument." ) 921 protected List<Reference> referenceDocument; 922 /** 923 * The actual objects that are the target of the reference (AdverseEvent.referenceDocument.) 924 */ 925 protected List<DocumentReference> referenceDocumentTarget; 926 927 928 /** 929 * AdverseEvent.study. 930 */ 931 @Child(name = "study", type = {ResearchStudy.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 932 @Description(shortDefinition="AdverseEvent.study", formalDefinition="AdverseEvent.study." ) 933 protected List<Reference> study; 934 /** 935 * The actual objects that are the target of the reference (AdverseEvent.study.) 936 */ 937 protected List<ResearchStudy> studyTarget; 938 939 940 private static final long serialVersionUID = 156251238L; 941 942 /** 943 * Constructor 944 */ 945 public AdverseEvent() { 946 super(); 947 } 948 949 /** 950 * @return {@link #identifier} (The identifier(s) of this adverse event that are assigned by business processes and/or used to refer to it when a direct URL reference to the resource itsefl is not appropriate.) 951 */ 952 public Identifier getIdentifier() { 953 if (this.identifier == null) 954 if (Configuration.errorOnAutoCreate()) 955 throw new Error("Attempt to auto-create AdverseEvent.identifier"); 956 else if (Configuration.doAutoCreate()) 957 this.identifier = new Identifier(); // cc 958 return this.identifier; 959 } 960 961 public boolean hasIdentifier() { 962 return this.identifier != null && !this.identifier.isEmpty(); 963 } 964 965 /** 966 * @param value {@link #identifier} (The identifier(s) of this adverse event that are assigned by business processes and/or used to refer to it when a direct URL reference to the resource itsefl is not appropriate.) 967 */ 968 public AdverseEvent setIdentifier(Identifier value) { 969 this.identifier = value; 970 return this; 971 } 972 973 /** 974 * @return {@link #category} (The type of event which is important to characterize what occurred and caused harm to the subject, or had the potential to cause harm to the subject.). This is the underlying object with id, value and extensions. The accessor "getCategory" gives direct access to the value 975 */ 976 public Enumeration<AdverseEventCategory> getCategoryElement() { 977 if (this.category == null) 978 if (Configuration.errorOnAutoCreate()) 979 throw new Error("Attempt to auto-create AdverseEvent.category"); 980 else if (Configuration.doAutoCreate()) 981 this.category = new Enumeration<AdverseEventCategory>(new AdverseEventCategoryEnumFactory()); // bb 982 return this.category; 983 } 984 985 public boolean hasCategoryElement() { 986 return this.category != null && !this.category.isEmpty(); 987 } 988 989 public boolean hasCategory() { 990 return this.category != null && !this.category.isEmpty(); 991 } 992 993 /** 994 * @param value {@link #category} (The type of event which is important to characterize what occurred and caused harm to the subject, or had the potential to cause harm to the subject.). This is the underlying object with id, value and extensions. The accessor "getCategory" gives direct access to the value 995 */ 996 public AdverseEvent setCategoryElement(Enumeration<AdverseEventCategory> value) { 997 this.category = value; 998 return this; 999 } 1000 1001 /** 1002 * @return The type of event which is important to characterize what occurred and caused harm to the subject, or had the potential to cause harm to the subject. 1003 */ 1004 public AdverseEventCategory getCategory() { 1005 return this.category == null ? null : this.category.getValue(); 1006 } 1007 1008 /** 1009 * @param value The type of event which is important to characterize what occurred and caused harm to the subject, or had the potential to cause harm to the subject. 1010 */ 1011 public AdverseEvent setCategory(AdverseEventCategory value) { 1012 if (value == null) 1013 this.category = null; 1014 else { 1015 if (this.category == null) 1016 this.category = new Enumeration<AdverseEventCategory>(new AdverseEventCategoryEnumFactory()); 1017 this.category.setValue(value); 1018 } 1019 return this; 1020 } 1021 1022 /** 1023 * @return {@link #type} (This element defines the specific type of event that occurred or that was prevented from occurring.) 1024 */ 1025 public CodeableConcept getType() { 1026 if (this.type == null) 1027 if (Configuration.errorOnAutoCreate()) 1028 throw new Error("Attempt to auto-create AdverseEvent.type"); 1029 else if (Configuration.doAutoCreate()) 1030 this.type = new CodeableConcept(); // cc 1031 return this.type; 1032 } 1033 1034 public boolean hasType() { 1035 return this.type != null && !this.type.isEmpty(); 1036 } 1037 1038 /** 1039 * @param value {@link #type} (This element defines the specific type of event that occurred or that was prevented from occurring.) 1040 */ 1041 public AdverseEvent setType(CodeableConcept value) { 1042 this.type = value; 1043 return this; 1044 } 1045 1046 /** 1047 * @return {@link #subject} (This subject or group impacted by the event. With a prospective adverse event, there will be no subject as the adverse event was prevented.) 1048 */ 1049 public Reference getSubject() { 1050 if (this.subject == null) 1051 if (Configuration.errorOnAutoCreate()) 1052 throw new Error("Attempt to auto-create AdverseEvent.subject"); 1053 else if (Configuration.doAutoCreate()) 1054 this.subject = new Reference(); // cc 1055 return this.subject; 1056 } 1057 1058 public boolean hasSubject() { 1059 return this.subject != null && !this.subject.isEmpty(); 1060 } 1061 1062 /** 1063 * @param value {@link #subject} (This subject or group impacted by the event. With a prospective adverse event, there will be no subject as the adverse event was prevented.) 1064 */ 1065 public AdverseEvent setSubject(Reference value) { 1066 this.subject = value; 1067 return this; 1068 } 1069 1070 /** 1071 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (This subject or group impacted by the event. With a prospective adverse event, there will be no subject as the adverse event was prevented.) 1072 */ 1073 public Resource getSubjectTarget() { 1074 return this.subjectTarget; 1075 } 1076 1077 /** 1078 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (This subject or group impacted by the event. With a prospective adverse event, there will be no subject as the adverse event was prevented.) 1079 */ 1080 public AdverseEvent setSubjectTarget(Resource value) { 1081 this.subjectTarget = value; 1082 return this; 1083 } 1084 1085 /** 1086 * @return {@link #date} (The date (and perhaps time) when the adverse event occurred.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1087 */ 1088 public DateTimeType getDateElement() { 1089 if (this.date == null) 1090 if (Configuration.errorOnAutoCreate()) 1091 throw new Error("Attempt to auto-create AdverseEvent.date"); 1092 else if (Configuration.doAutoCreate()) 1093 this.date = new DateTimeType(); // bb 1094 return this.date; 1095 } 1096 1097 public boolean hasDateElement() { 1098 return this.date != null && !this.date.isEmpty(); 1099 } 1100 1101 public boolean hasDate() { 1102 return this.date != null && !this.date.isEmpty(); 1103 } 1104 1105 /** 1106 * @param value {@link #date} (The date (and perhaps time) when the adverse event occurred.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1107 */ 1108 public AdverseEvent setDateElement(DateTimeType value) { 1109 this.date = value; 1110 return this; 1111 } 1112 1113 /** 1114 * @return The date (and perhaps time) when the adverse event occurred. 1115 */ 1116 public Date getDate() { 1117 return this.date == null ? null : this.date.getValue(); 1118 } 1119 1120 /** 1121 * @param value The date (and perhaps time) when the adverse event occurred. 1122 */ 1123 public AdverseEvent setDate(Date value) { 1124 if (value == null) 1125 this.date = null; 1126 else { 1127 if (this.date == null) 1128 this.date = new DateTimeType(); 1129 this.date.setValue(value); 1130 } 1131 return this; 1132 } 1133 1134 /** 1135 * @return {@link #reaction} (Includes information about the reaction that occurred as a result of exposure to a substance (for example, a drug or a chemical).) 1136 */ 1137 public List<Reference> getReaction() { 1138 if (this.reaction == null) 1139 this.reaction = new ArrayList<Reference>(); 1140 return this.reaction; 1141 } 1142 1143 /** 1144 * @return Returns a reference to <code>this</code> for easy method chaining 1145 */ 1146 public AdverseEvent setReaction(List<Reference> theReaction) { 1147 this.reaction = theReaction; 1148 return this; 1149 } 1150 1151 public boolean hasReaction() { 1152 if (this.reaction == null) 1153 return false; 1154 for (Reference item : this.reaction) 1155 if (!item.isEmpty()) 1156 return true; 1157 return false; 1158 } 1159 1160 public Reference addReaction() { //3 1161 Reference t = new Reference(); 1162 if (this.reaction == null) 1163 this.reaction = new ArrayList<Reference>(); 1164 this.reaction.add(t); 1165 return t; 1166 } 1167 1168 public AdverseEvent addReaction(Reference t) { //3 1169 if (t == null) 1170 return this; 1171 if (this.reaction == null) 1172 this.reaction = new ArrayList<Reference>(); 1173 this.reaction.add(t); 1174 return this; 1175 } 1176 1177 /** 1178 * @return The first repetition of repeating field {@link #reaction}, creating it if it does not already exist 1179 */ 1180 public Reference getReactionFirstRep() { 1181 if (getReaction().isEmpty()) { 1182 addReaction(); 1183 } 1184 return getReaction().get(0); 1185 } 1186 1187 /** 1188 * @deprecated Use Reference#setResource(IBaseResource) instead 1189 */ 1190 @Deprecated 1191 public List<Condition> getReactionTarget() { 1192 if (this.reactionTarget == null) 1193 this.reactionTarget = new ArrayList<Condition>(); 1194 return this.reactionTarget; 1195 } 1196 1197 /** 1198 * @deprecated Use Reference#setResource(IBaseResource) instead 1199 */ 1200 @Deprecated 1201 public Condition addReactionTarget() { 1202 Condition r = new Condition(); 1203 if (this.reactionTarget == null) 1204 this.reactionTarget = new ArrayList<Condition>(); 1205 this.reactionTarget.add(r); 1206 return r; 1207 } 1208 1209 /** 1210 * @return {@link #location} (The information about where the adverse event occurred.) 1211 */ 1212 public Reference getLocation() { 1213 if (this.location == null) 1214 if (Configuration.errorOnAutoCreate()) 1215 throw new Error("Attempt to auto-create AdverseEvent.location"); 1216 else if (Configuration.doAutoCreate()) 1217 this.location = new Reference(); // cc 1218 return this.location; 1219 } 1220 1221 public boolean hasLocation() { 1222 return this.location != null && !this.location.isEmpty(); 1223 } 1224 1225 /** 1226 * @param value {@link #location} (The information about where the adverse event occurred.) 1227 */ 1228 public AdverseEvent setLocation(Reference value) { 1229 this.location = value; 1230 return this; 1231 } 1232 1233 /** 1234 * @return {@link #location} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The information about where the adverse event occurred.) 1235 */ 1236 public Location getLocationTarget() { 1237 if (this.locationTarget == null) 1238 if (Configuration.errorOnAutoCreate()) 1239 throw new Error("Attempt to auto-create AdverseEvent.location"); 1240 else if (Configuration.doAutoCreate()) 1241 this.locationTarget = new Location(); // aa 1242 return this.locationTarget; 1243 } 1244 1245 /** 1246 * @param value {@link #location} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The information about where the adverse event occurred.) 1247 */ 1248 public AdverseEvent setLocationTarget(Location value) { 1249 this.locationTarget = value; 1250 return this; 1251 } 1252 1253 /** 1254 * @return {@link #seriousness} (Describes the seriousness or severity of the adverse event.) 1255 */ 1256 public CodeableConcept getSeriousness() { 1257 if (this.seriousness == null) 1258 if (Configuration.errorOnAutoCreate()) 1259 throw new Error("Attempt to auto-create AdverseEvent.seriousness"); 1260 else if (Configuration.doAutoCreate()) 1261 this.seriousness = new CodeableConcept(); // cc 1262 return this.seriousness; 1263 } 1264 1265 public boolean hasSeriousness() { 1266 return this.seriousness != null && !this.seriousness.isEmpty(); 1267 } 1268 1269 /** 1270 * @param value {@link #seriousness} (Describes the seriousness or severity of the adverse event.) 1271 */ 1272 public AdverseEvent setSeriousness(CodeableConcept value) { 1273 this.seriousness = value; 1274 return this; 1275 } 1276 1277 /** 1278 * @return {@link #outcome} (Describes the type of outcome from the adverse event.) 1279 */ 1280 public CodeableConcept getOutcome() { 1281 if (this.outcome == null) 1282 if (Configuration.errorOnAutoCreate()) 1283 throw new Error("Attempt to auto-create AdverseEvent.outcome"); 1284 else if (Configuration.doAutoCreate()) 1285 this.outcome = new CodeableConcept(); // cc 1286 return this.outcome; 1287 } 1288 1289 public boolean hasOutcome() { 1290 return this.outcome != null && !this.outcome.isEmpty(); 1291 } 1292 1293 /** 1294 * @param value {@link #outcome} (Describes the type of outcome from the adverse event.) 1295 */ 1296 public AdverseEvent setOutcome(CodeableConcept value) { 1297 this.outcome = value; 1298 return this; 1299 } 1300 1301 /** 1302 * @return {@link #recorder} (Information on who recorded the adverse event. May be the patient or a practitioner.) 1303 */ 1304 public Reference getRecorder() { 1305 if (this.recorder == null) 1306 if (Configuration.errorOnAutoCreate()) 1307 throw new Error("Attempt to auto-create AdverseEvent.recorder"); 1308 else if (Configuration.doAutoCreate()) 1309 this.recorder = new Reference(); // cc 1310 return this.recorder; 1311 } 1312 1313 public boolean hasRecorder() { 1314 return this.recorder != null && !this.recorder.isEmpty(); 1315 } 1316 1317 /** 1318 * @param value {@link #recorder} (Information on who recorded the adverse event. May be the patient or a practitioner.) 1319 */ 1320 public AdverseEvent setRecorder(Reference value) { 1321 this.recorder = value; 1322 return this; 1323 } 1324 1325 /** 1326 * @return {@link #recorder} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Information on who recorded the adverse event. May be the patient or a practitioner.) 1327 */ 1328 public Resource getRecorderTarget() { 1329 return this.recorderTarget; 1330 } 1331 1332 /** 1333 * @param value {@link #recorder} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Information on who recorded the adverse event. May be the patient or a practitioner.) 1334 */ 1335 public AdverseEvent setRecorderTarget(Resource value) { 1336 this.recorderTarget = value; 1337 return this; 1338 } 1339 1340 /** 1341 * @return {@link #eventParticipant} (Parties that may or should contribute or have contributed information to the Act. Such information includes information leading to the decision to perform the Act and how to perform the Act (e.g. consultant), information that the Act itself seeks to reveal (e.g. informant of clinical history), or information about what Act was performed (e.g. informant witness).) 1342 */ 1343 public Reference getEventParticipant() { 1344 if (this.eventParticipant == null) 1345 if (Configuration.errorOnAutoCreate()) 1346 throw new Error("Attempt to auto-create AdverseEvent.eventParticipant"); 1347 else if (Configuration.doAutoCreate()) 1348 this.eventParticipant = new Reference(); // cc 1349 return this.eventParticipant; 1350 } 1351 1352 public boolean hasEventParticipant() { 1353 return this.eventParticipant != null && !this.eventParticipant.isEmpty(); 1354 } 1355 1356 /** 1357 * @param value {@link #eventParticipant} (Parties that may or should contribute or have contributed information to the Act. Such information includes information leading to the decision to perform the Act and how to perform the Act (e.g. consultant), information that the Act itself seeks to reveal (e.g. informant of clinical history), or information about what Act was performed (e.g. informant witness).) 1358 */ 1359 public AdverseEvent setEventParticipant(Reference value) { 1360 this.eventParticipant = value; 1361 return this; 1362 } 1363 1364 /** 1365 * @return {@link #eventParticipant} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Parties that may or should contribute or have contributed information to the Act. Such information includes information leading to the decision to perform the Act and how to perform the Act (e.g. consultant), information that the Act itself seeks to reveal (e.g. informant of clinical history), or information about what Act was performed (e.g. informant witness).) 1366 */ 1367 public Resource getEventParticipantTarget() { 1368 return this.eventParticipantTarget; 1369 } 1370 1371 /** 1372 * @param value {@link #eventParticipant} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Parties that may or should contribute or have contributed information to the Act. Such information includes information leading to the decision to perform the Act and how to perform the Act (e.g. consultant), information that the Act itself seeks to reveal (e.g. informant of clinical history), or information about what Act was performed (e.g. informant witness).) 1373 */ 1374 public AdverseEvent setEventParticipantTarget(Resource value) { 1375 this.eventParticipantTarget = value; 1376 return this; 1377 } 1378 1379 /** 1380 * @return {@link #description} (Describes the adverse event in text.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1381 */ 1382 public StringType getDescriptionElement() { 1383 if (this.description == null) 1384 if (Configuration.errorOnAutoCreate()) 1385 throw new Error("Attempt to auto-create AdverseEvent.description"); 1386 else if (Configuration.doAutoCreate()) 1387 this.description = new StringType(); // bb 1388 return this.description; 1389 } 1390 1391 public boolean hasDescriptionElement() { 1392 return this.description != null && !this.description.isEmpty(); 1393 } 1394 1395 public boolean hasDescription() { 1396 return this.description != null && !this.description.isEmpty(); 1397 } 1398 1399 /** 1400 * @param value {@link #description} (Describes the adverse event in text.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1401 */ 1402 public AdverseEvent setDescriptionElement(StringType value) { 1403 this.description = value; 1404 return this; 1405 } 1406 1407 /** 1408 * @return Describes the adverse event in text. 1409 */ 1410 public String getDescription() { 1411 return this.description == null ? null : this.description.getValue(); 1412 } 1413 1414 /** 1415 * @param value Describes the adverse event in text. 1416 */ 1417 public AdverseEvent setDescription(String value) { 1418 if (Utilities.noString(value)) 1419 this.description = null; 1420 else { 1421 if (this.description == null) 1422 this.description = new StringType(); 1423 this.description.setValue(value); 1424 } 1425 return this; 1426 } 1427 1428 /** 1429 * @return {@link #suspectEntity} (Describes the entity that is suspected to have caused the adverse event.) 1430 */ 1431 public List<AdverseEventSuspectEntityComponent> getSuspectEntity() { 1432 if (this.suspectEntity == null) 1433 this.suspectEntity = new ArrayList<AdverseEventSuspectEntityComponent>(); 1434 return this.suspectEntity; 1435 } 1436 1437 /** 1438 * @return Returns a reference to <code>this</code> for easy method chaining 1439 */ 1440 public AdverseEvent setSuspectEntity(List<AdverseEventSuspectEntityComponent> theSuspectEntity) { 1441 this.suspectEntity = theSuspectEntity; 1442 return this; 1443 } 1444 1445 public boolean hasSuspectEntity() { 1446 if (this.suspectEntity == null) 1447 return false; 1448 for (AdverseEventSuspectEntityComponent item : this.suspectEntity) 1449 if (!item.isEmpty()) 1450 return true; 1451 return false; 1452 } 1453 1454 public AdverseEventSuspectEntityComponent addSuspectEntity() { //3 1455 AdverseEventSuspectEntityComponent t = new AdverseEventSuspectEntityComponent(); 1456 if (this.suspectEntity == null) 1457 this.suspectEntity = new ArrayList<AdverseEventSuspectEntityComponent>(); 1458 this.suspectEntity.add(t); 1459 return t; 1460 } 1461 1462 public AdverseEvent addSuspectEntity(AdverseEventSuspectEntityComponent t) { //3 1463 if (t == null) 1464 return this; 1465 if (this.suspectEntity == null) 1466 this.suspectEntity = new ArrayList<AdverseEventSuspectEntityComponent>(); 1467 this.suspectEntity.add(t); 1468 return this; 1469 } 1470 1471 /** 1472 * @return The first repetition of repeating field {@link #suspectEntity}, creating it if it does not already exist 1473 */ 1474 public AdverseEventSuspectEntityComponent getSuspectEntityFirstRep() { 1475 if (getSuspectEntity().isEmpty()) { 1476 addSuspectEntity(); 1477 } 1478 return getSuspectEntity().get(0); 1479 } 1480 1481 /** 1482 * @return {@link #subjectMedicalHistory} (AdverseEvent.subjectMedicalHistory.) 1483 */ 1484 public List<Reference> getSubjectMedicalHistory() { 1485 if (this.subjectMedicalHistory == null) 1486 this.subjectMedicalHistory = new ArrayList<Reference>(); 1487 return this.subjectMedicalHistory; 1488 } 1489 1490 /** 1491 * @return Returns a reference to <code>this</code> for easy method chaining 1492 */ 1493 public AdverseEvent setSubjectMedicalHistory(List<Reference> theSubjectMedicalHistory) { 1494 this.subjectMedicalHistory = theSubjectMedicalHistory; 1495 return this; 1496 } 1497 1498 public boolean hasSubjectMedicalHistory() { 1499 if (this.subjectMedicalHistory == null) 1500 return false; 1501 for (Reference item : this.subjectMedicalHistory) 1502 if (!item.isEmpty()) 1503 return true; 1504 return false; 1505 } 1506 1507 public Reference addSubjectMedicalHistory() { //3 1508 Reference t = new Reference(); 1509 if (this.subjectMedicalHistory == null) 1510 this.subjectMedicalHistory = new ArrayList<Reference>(); 1511 this.subjectMedicalHistory.add(t); 1512 return t; 1513 } 1514 1515 public AdverseEvent addSubjectMedicalHistory(Reference t) { //3 1516 if (t == null) 1517 return this; 1518 if (this.subjectMedicalHistory == null) 1519 this.subjectMedicalHistory = new ArrayList<Reference>(); 1520 this.subjectMedicalHistory.add(t); 1521 return this; 1522 } 1523 1524 /** 1525 * @return The first repetition of repeating field {@link #subjectMedicalHistory}, creating it if it does not already exist 1526 */ 1527 public Reference getSubjectMedicalHistoryFirstRep() { 1528 if (getSubjectMedicalHistory().isEmpty()) { 1529 addSubjectMedicalHistory(); 1530 } 1531 return getSubjectMedicalHistory().get(0); 1532 } 1533 1534 /** 1535 * @deprecated Use Reference#setResource(IBaseResource) instead 1536 */ 1537 @Deprecated 1538 public List<Resource> getSubjectMedicalHistoryTarget() { 1539 if (this.subjectMedicalHistoryTarget == null) 1540 this.subjectMedicalHistoryTarget = new ArrayList<Resource>(); 1541 return this.subjectMedicalHistoryTarget; 1542 } 1543 1544 /** 1545 * @return {@link #referenceDocument} (AdverseEvent.referenceDocument.) 1546 */ 1547 public List<Reference> getReferenceDocument() { 1548 if (this.referenceDocument == null) 1549 this.referenceDocument = new ArrayList<Reference>(); 1550 return this.referenceDocument; 1551 } 1552 1553 /** 1554 * @return Returns a reference to <code>this</code> for easy method chaining 1555 */ 1556 public AdverseEvent setReferenceDocument(List<Reference> theReferenceDocument) { 1557 this.referenceDocument = theReferenceDocument; 1558 return this; 1559 } 1560 1561 public boolean hasReferenceDocument() { 1562 if (this.referenceDocument == null) 1563 return false; 1564 for (Reference item : this.referenceDocument) 1565 if (!item.isEmpty()) 1566 return true; 1567 return false; 1568 } 1569 1570 public Reference addReferenceDocument() { //3 1571 Reference t = new Reference(); 1572 if (this.referenceDocument == null) 1573 this.referenceDocument = new ArrayList<Reference>(); 1574 this.referenceDocument.add(t); 1575 return t; 1576 } 1577 1578 public AdverseEvent addReferenceDocument(Reference t) { //3 1579 if (t == null) 1580 return this; 1581 if (this.referenceDocument == null) 1582 this.referenceDocument = new ArrayList<Reference>(); 1583 this.referenceDocument.add(t); 1584 return this; 1585 } 1586 1587 /** 1588 * @return The first repetition of repeating field {@link #referenceDocument}, creating it if it does not already exist 1589 */ 1590 public Reference getReferenceDocumentFirstRep() { 1591 if (getReferenceDocument().isEmpty()) { 1592 addReferenceDocument(); 1593 } 1594 return getReferenceDocument().get(0); 1595 } 1596 1597 /** 1598 * @deprecated Use Reference#setResource(IBaseResource) instead 1599 */ 1600 @Deprecated 1601 public List<DocumentReference> getReferenceDocumentTarget() { 1602 if (this.referenceDocumentTarget == null) 1603 this.referenceDocumentTarget = new ArrayList<DocumentReference>(); 1604 return this.referenceDocumentTarget; 1605 } 1606 1607 /** 1608 * @deprecated Use Reference#setResource(IBaseResource) instead 1609 */ 1610 @Deprecated 1611 public DocumentReference addReferenceDocumentTarget() { 1612 DocumentReference r = new DocumentReference(); 1613 if (this.referenceDocumentTarget == null) 1614 this.referenceDocumentTarget = new ArrayList<DocumentReference>(); 1615 this.referenceDocumentTarget.add(r); 1616 return r; 1617 } 1618 1619 /** 1620 * @return {@link #study} (AdverseEvent.study.) 1621 */ 1622 public List<Reference> getStudy() { 1623 if (this.study == null) 1624 this.study = new ArrayList<Reference>(); 1625 return this.study; 1626 } 1627 1628 /** 1629 * @return Returns a reference to <code>this</code> for easy method chaining 1630 */ 1631 public AdverseEvent setStudy(List<Reference> theStudy) { 1632 this.study = theStudy; 1633 return this; 1634 } 1635 1636 public boolean hasStudy() { 1637 if (this.study == null) 1638 return false; 1639 for (Reference item : this.study) 1640 if (!item.isEmpty()) 1641 return true; 1642 return false; 1643 } 1644 1645 public Reference addStudy() { //3 1646 Reference t = new Reference(); 1647 if (this.study == null) 1648 this.study = new ArrayList<Reference>(); 1649 this.study.add(t); 1650 return t; 1651 } 1652 1653 public AdverseEvent addStudy(Reference t) { //3 1654 if (t == null) 1655 return this; 1656 if (this.study == null) 1657 this.study = new ArrayList<Reference>(); 1658 this.study.add(t); 1659 return this; 1660 } 1661 1662 /** 1663 * @return The first repetition of repeating field {@link #study}, creating it if it does not already exist 1664 */ 1665 public Reference getStudyFirstRep() { 1666 if (getStudy().isEmpty()) { 1667 addStudy(); 1668 } 1669 return getStudy().get(0); 1670 } 1671 1672 /** 1673 * @deprecated Use Reference#setResource(IBaseResource) instead 1674 */ 1675 @Deprecated 1676 public List<ResearchStudy> getStudyTarget() { 1677 if (this.studyTarget == null) 1678 this.studyTarget = new ArrayList<ResearchStudy>(); 1679 return this.studyTarget; 1680 } 1681 1682 /** 1683 * @deprecated Use Reference#setResource(IBaseResource) instead 1684 */ 1685 @Deprecated 1686 public ResearchStudy addStudyTarget() { 1687 ResearchStudy r = new ResearchStudy(); 1688 if (this.studyTarget == null) 1689 this.studyTarget = new ArrayList<ResearchStudy>(); 1690 this.studyTarget.add(r); 1691 return r; 1692 } 1693 1694 protected void listChildren(List<Property> children) { 1695 super.listChildren(children); 1696 children.add(new Property("identifier", "Identifier", "The identifier(s) of this adverse event that are assigned by business processes and/or used to refer to it when a direct URL reference to the resource itsefl is not appropriate.", 0, 1, identifier)); 1697 children.add(new Property("category", "code", "The type of event which is important to characterize what occurred and caused harm to the subject, or had the potential to cause harm to the subject.", 0, 1, category)); 1698 children.add(new Property("type", "CodeableConcept", "This element defines the specific type of event that occurred or that was prevented from occurring.", 0, 1, type)); 1699 children.add(new Property("subject", "Reference(Patient|ResearchSubject|Medication|Device)", "This subject or group impacted by the event. With a prospective adverse event, there will be no subject as the adverse event was prevented.", 0, 1, subject)); 1700 children.add(new Property("date", "dateTime", "The date (and perhaps time) when the adverse event occurred.", 0, 1, date)); 1701 children.add(new Property("reaction", "Reference(Condition)", "Includes information about the reaction that occurred as a result of exposure to a substance (for example, a drug or a chemical).", 0, java.lang.Integer.MAX_VALUE, reaction)); 1702 children.add(new Property("location", "Reference(Location)", "The information about where the adverse event occurred.", 0, 1, location)); 1703 children.add(new Property("seriousness", "CodeableConcept", "Describes the seriousness or severity of the adverse event.", 0, 1, seriousness)); 1704 children.add(new Property("outcome", "CodeableConcept", "Describes the type of outcome from the adverse event.", 0, 1, outcome)); 1705 children.add(new Property("recorder", "Reference(Patient|Practitioner|RelatedPerson)", "Information on who recorded the adverse event. May be the patient or a practitioner.", 0, 1, recorder)); 1706 children.add(new Property("eventParticipant", "Reference(Practitioner|Device)", "Parties that may or should contribute or have contributed information to the Act. Such information includes information leading to the decision to perform the Act and how to perform the Act (e.g. consultant), information that the Act itself seeks to reveal (e.g. informant of clinical history), or information about what Act was performed (e.g. informant witness).", 0, 1, eventParticipant)); 1707 children.add(new Property("description", "string", "Describes the adverse event in text.", 0, 1, description)); 1708 children.add(new Property("suspectEntity", "", "Describes the entity that is suspected to have caused the adverse event.", 0, java.lang.Integer.MAX_VALUE, suspectEntity)); 1709 children.add(new Property("subjectMedicalHistory", "Reference(Condition|Observation|AllergyIntolerance|FamilyMemberHistory|Immunization|Procedure)", "AdverseEvent.subjectMedicalHistory.", 0, java.lang.Integer.MAX_VALUE, subjectMedicalHistory)); 1710 children.add(new Property("referenceDocument", "Reference(DocumentReference)", "AdverseEvent.referenceDocument.", 0, java.lang.Integer.MAX_VALUE, referenceDocument)); 1711 children.add(new Property("study", "Reference(ResearchStudy)", "AdverseEvent.study.", 0, java.lang.Integer.MAX_VALUE, study)); 1712 } 1713 1714 @Override 1715 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1716 switch (_hash) { 1717 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The identifier(s) of this adverse event that are assigned by business processes and/or used to refer to it when a direct URL reference to the resource itsefl is not appropriate.", 0, 1, identifier); 1718 case 50511102: /*category*/ return new Property("category", "code", "The type of event which is important to characterize what occurred and caused harm to the subject, or had the potential to cause harm to the subject.", 0, 1, category); 1719 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "This element defines the specific type of event that occurred or that was prevented from occurring.", 0, 1, type); 1720 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|ResearchSubject|Medication|Device)", "This subject or group impacted by the event. With a prospective adverse event, there will be no subject as the adverse event was prevented.", 0, 1, subject); 1721 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and perhaps time) when the adverse event occurred.", 0, 1, date); 1722 case -867509719: /*reaction*/ return new Property("reaction", "Reference(Condition)", "Includes information about the reaction that occurred as a result of exposure to a substance (for example, a drug or a chemical).", 0, java.lang.Integer.MAX_VALUE, reaction); 1723 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "The information about where the adverse event occurred.", 0, 1, location); 1724 case -1551003909: /*seriousness*/ return new Property("seriousness", "CodeableConcept", "Describes the seriousness or severity of the adverse event.", 0, 1, seriousness); 1725 case -1106507950: /*outcome*/ return new Property("outcome", "CodeableConcept", "Describes the type of outcome from the adverse event.", 0, 1, outcome); 1726 case -799233858: /*recorder*/ return new Property("recorder", "Reference(Patient|Practitioner|RelatedPerson)", "Information on who recorded the adverse event. May be the patient or a practitioner.", 0, 1, recorder); 1727 case 270753849: /*eventParticipant*/ return new Property("eventParticipant", "Reference(Practitioner|Device)", "Parties that may or should contribute or have contributed information to the Act. Such information includes information leading to the decision to perform the Act and how to perform the Act (e.g. consultant), information that the Act itself seeks to reveal (e.g. informant of clinical history), or information about what Act was performed (e.g. informant witness).", 0, 1, eventParticipant); 1728 case -1724546052: /*description*/ return new Property("description", "string", "Describes the adverse event in text.", 0, 1, description); 1729 case -1957422662: /*suspectEntity*/ return new Property("suspectEntity", "", "Describes the entity that is suspected to have caused the adverse event.", 0, java.lang.Integer.MAX_VALUE, suspectEntity); 1730 case -1685245681: /*subjectMedicalHistory*/ return new Property("subjectMedicalHistory", "Reference(Condition|Observation|AllergyIntolerance|FamilyMemberHistory|Immunization|Procedure)", "AdverseEvent.subjectMedicalHistory.", 0, java.lang.Integer.MAX_VALUE, subjectMedicalHistory); 1731 case 1013971334: /*referenceDocument*/ return new Property("referenceDocument", "Reference(DocumentReference)", "AdverseEvent.referenceDocument.", 0, java.lang.Integer.MAX_VALUE, referenceDocument); 1732 case 109776329: /*study*/ return new Property("study", "Reference(ResearchStudy)", "AdverseEvent.study.", 0, java.lang.Integer.MAX_VALUE, study); 1733 default: return super.getNamedProperty(_hash, _name, _checkValid); 1734 } 1735 1736 } 1737 1738 @Override 1739 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1740 switch (hash) { 1741 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 1742 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // Enumeration<AdverseEventCategory> 1743 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1744 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1745 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 1746 case -867509719: /*reaction*/ return this.reaction == null ? new Base[0] : this.reaction.toArray(new Base[this.reaction.size()]); // Reference 1747 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Reference 1748 case -1551003909: /*seriousness*/ return this.seriousness == null ? new Base[0] : new Base[] {this.seriousness}; // CodeableConcept 1749 case -1106507950: /*outcome*/ return this.outcome == null ? new Base[0] : new Base[] {this.outcome}; // CodeableConcept 1750 case -799233858: /*recorder*/ return this.recorder == null ? new Base[0] : new Base[] {this.recorder}; // Reference 1751 case 270753849: /*eventParticipant*/ return this.eventParticipant == null ? new Base[0] : new Base[] {this.eventParticipant}; // Reference 1752 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 1753 case -1957422662: /*suspectEntity*/ return this.suspectEntity == null ? new Base[0] : this.suspectEntity.toArray(new Base[this.suspectEntity.size()]); // AdverseEventSuspectEntityComponent 1754 case -1685245681: /*subjectMedicalHistory*/ return this.subjectMedicalHistory == null ? new Base[0] : this.subjectMedicalHistory.toArray(new Base[this.subjectMedicalHistory.size()]); // Reference 1755 case 1013971334: /*referenceDocument*/ return this.referenceDocument == null ? new Base[0] : this.referenceDocument.toArray(new Base[this.referenceDocument.size()]); // Reference 1756 case 109776329: /*study*/ return this.study == null ? new Base[0] : this.study.toArray(new Base[this.study.size()]); // Reference 1757 default: return super.getProperty(hash, name, checkValid); 1758 } 1759 1760 } 1761 1762 @Override 1763 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1764 switch (hash) { 1765 case -1618432855: // identifier 1766 this.identifier = castToIdentifier(value); // Identifier 1767 return value; 1768 case 50511102: // category 1769 value = new AdverseEventCategoryEnumFactory().fromType(castToCode(value)); 1770 this.category = (Enumeration) value; // Enumeration<AdverseEventCategory> 1771 return value; 1772 case 3575610: // type 1773 this.type = castToCodeableConcept(value); // CodeableConcept 1774 return value; 1775 case -1867885268: // subject 1776 this.subject = castToReference(value); // Reference 1777 return value; 1778 case 3076014: // date 1779 this.date = castToDateTime(value); // DateTimeType 1780 return value; 1781 case -867509719: // reaction 1782 this.getReaction().add(castToReference(value)); // Reference 1783 return value; 1784 case 1901043637: // location 1785 this.location = castToReference(value); // Reference 1786 return value; 1787 case -1551003909: // seriousness 1788 this.seriousness = castToCodeableConcept(value); // CodeableConcept 1789 return value; 1790 case -1106507950: // outcome 1791 this.outcome = castToCodeableConcept(value); // CodeableConcept 1792 return value; 1793 case -799233858: // recorder 1794 this.recorder = castToReference(value); // Reference 1795 return value; 1796 case 270753849: // eventParticipant 1797 this.eventParticipant = castToReference(value); // Reference 1798 return value; 1799 case -1724546052: // description 1800 this.description = castToString(value); // StringType 1801 return value; 1802 case -1957422662: // suspectEntity 1803 this.getSuspectEntity().add((AdverseEventSuspectEntityComponent) value); // AdverseEventSuspectEntityComponent 1804 return value; 1805 case -1685245681: // subjectMedicalHistory 1806 this.getSubjectMedicalHistory().add(castToReference(value)); // Reference 1807 return value; 1808 case 1013971334: // referenceDocument 1809 this.getReferenceDocument().add(castToReference(value)); // Reference 1810 return value; 1811 case 109776329: // study 1812 this.getStudy().add(castToReference(value)); // Reference 1813 return value; 1814 default: return super.setProperty(hash, name, value); 1815 } 1816 1817 } 1818 1819 @Override 1820 public Base setProperty(String name, Base value) throws FHIRException { 1821 if (name.equals("identifier")) { 1822 this.identifier = castToIdentifier(value); // Identifier 1823 } else if (name.equals("category")) { 1824 value = new AdverseEventCategoryEnumFactory().fromType(castToCode(value)); 1825 this.category = (Enumeration) value; // Enumeration<AdverseEventCategory> 1826 } else if (name.equals("type")) { 1827 this.type = castToCodeableConcept(value); // CodeableConcept 1828 } else if (name.equals("subject")) { 1829 this.subject = castToReference(value); // Reference 1830 } else if (name.equals("date")) { 1831 this.date = castToDateTime(value); // DateTimeType 1832 } else if (name.equals("reaction")) { 1833 this.getReaction().add(castToReference(value)); 1834 } else if (name.equals("location")) { 1835 this.location = castToReference(value); // Reference 1836 } else if (name.equals("seriousness")) { 1837 this.seriousness = castToCodeableConcept(value); // CodeableConcept 1838 } else if (name.equals("outcome")) { 1839 this.outcome = castToCodeableConcept(value); // CodeableConcept 1840 } else if (name.equals("recorder")) { 1841 this.recorder = castToReference(value); // Reference 1842 } else if (name.equals("eventParticipant")) { 1843 this.eventParticipant = castToReference(value); // Reference 1844 } else if (name.equals("description")) { 1845 this.description = castToString(value); // StringType 1846 } else if (name.equals("suspectEntity")) { 1847 this.getSuspectEntity().add((AdverseEventSuspectEntityComponent) value); 1848 } else if (name.equals("subjectMedicalHistory")) { 1849 this.getSubjectMedicalHistory().add(castToReference(value)); 1850 } else if (name.equals("referenceDocument")) { 1851 this.getReferenceDocument().add(castToReference(value)); 1852 } else if (name.equals("study")) { 1853 this.getStudy().add(castToReference(value)); 1854 } else 1855 return super.setProperty(name, value); 1856 return value; 1857 } 1858 1859 @Override 1860 public Base makeProperty(int hash, String name) throws FHIRException { 1861 switch (hash) { 1862 case -1618432855: return getIdentifier(); 1863 case 50511102: return getCategoryElement(); 1864 case 3575610: return getType(); 1865 case -1867885268: return getSubject(); 1866 case 3076014: return getDateElement(); 1867 case -867509719: return addReaction(); 1868 case 1901043637: return getLocation(); 1869 case -1551003909: return getSeriousness(); 1870 case -1106507950: return getOutcome(); 1871 case -799233858: return getRecorder(); 1872 case 270753849: return getEventParticipant(); 1873 case -1724546052: return getDescriptionElement(); 1874 case -1957422662: return addSuspectEntity(); 1875 case -1685245681: return addSubjectMedicalHistory(); 1876 case 1013971334: return addReferenceDocument(); 1877 case 109776329: return addStudy(); 1878 default: return super.makeProperty(hash, name); 1879 } 1880 1881 } 1882 1883 @Override 1884 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1885 switch (hash) { 1886 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1887 case 50511102: /*category*/ return new String[] {"code"}; 1888 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1889 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1890 case 3076014: /*date*/ return new String[] {"dateTime"}; 1891 case -867509719: /*reaction*/ return new String[] {"Reference"}; 1892 case 1901043637: /*location*/ return new String[] {"Reference"}; 1893 case -1551003909: /*seriousness*/ return new String[] {"CodeableConcept"}; 1894 case -1106507950: /*outcome*/ return new String[] {"CodeableConcept"}; 1895 case -799233858: /*recorder*/ return new String[] {"Reference"}; 1896 case 270753849: /*eventParticipant*/ return new String[] {"Reference"}; 1897 case -1724546052: /*description*/ return new String[] {"string"}; 1898 case -1957422662: /*suspectEntity*/ return new String[] {}; 1899 case -1685245681: /*subjectMedicalHistory*/ return new String[] {"Reference"}; 1900 case 1013971334: /*referenceDocument*/ return new String[] {"Reference"}; 1901 case 109776329: /*study*/ return new String[] {"Reference"}; 1902 default: return super.getTypesForProperty(hash, name); 1903 } 1904 1905 } 1906 1907 @Override 1908 public Base addChild(String name) throws FHIRException { 1909 if (name.equals("identifier")) { 1910 this.identifier = new Identifier(); 1911 return this.identifier; 1912 } 1913 else if (name.equals("category")) { 1914 throw new FHIRException("Cannot call addChild on a singleton property AdverseEvent.category"); 1915 } 1916 else if (name.equals("type")) { 1917 this.type = new CodeableConcept(); 1918 return this.type; 1919 } 1920 else if (name.equals("subject")) { 1921 this.subject = new Reference(); 1922 return this.subject; 1923 } 1924 else if (name.equals("date")) { 1925 throw new FHIRException("Cannot call addChild on a singleton property AdverseEvent.date"); 1926 } 1927 else if (name.equals("reaction")) { 1928 return addReaction(); 1929 } 1930 else if (name.equals("location")) { 1931 this.location = new Reference(); 1932 return this.location; 1933 } 1934 else if (name.equals("seriousness")) { 1935 this.seriousness = new CodeableConcept(); 1936 return this.seriousness; 1937 } 1938 else if (name.equals("outcome")) { 1939 this.outcome = new CodeableConcept(); 1940 return this.outcome; 1941 } 1942 else if (name.equals("recorder")) { 1943 this.recorder = new Reference(); 1944 return this.recorder; 1945 } 1946 else if (name.equals("eventParticipant")) { 1947 this.eventParticipant = new Reference(); 1948 return this.eventParticipant; 1949 } 1950 else if (name.equals("description")) { 1951 throw new FHIRException("Cannot call addChild on a singleton property AdverseEvent.description"); 1952 } 1953 else if (name.equals("suspectEntity")) { 1954 return addSuspectEntity(); 1955 } 1956 else if (name.equals("subjectMedicalHistory")) { 1957 return addSubjectMedicalHistory(); 1958 } 1959 else if (name.equals("referenceDocument")) { 1960 return addReferenceDocument(); 1961 } 1962 else if (name.equals("study")) { 1963 return addStudy(); 1964 } 1965 else 1966 return super.addChild(name); 1967 } 1968 1969 public String fhirType() { 1970 return "AdverseEvent"; 1971 1972 } 1973 1974 public AdverseEvent copy() { 1975 AdverseEvent dst = new AdverseEvent(); 1976 copyValues(dst); 1977 dst.identifier = identifier == null ? null : identifier.copy(); 1978 dst.category = category == null ? null : category.copy(); 1979 dst.type = type == null ? null : type.copy(); 1980 dst.subject = subject == null ? null : subject.copy(); 1981 dst.date = date == null ? null : date.copy(); 1982 if (reaction != null) { 1983 dst.reaction = new ArrayList<Reference>(); 1984 for (Reference i : reaction) 1985 dst.reaction.add(i.copy()); 1986 }; 1987 dst.location = location == null ? null : location.copy(); 1988 dst.seriousness = seriousness == null ? null : seriousness.copy(); 1989 dst.outcome = outcome == null ? null : outcome.copy(); 1990 dst.recorder = recorder == null ? null : recorder.copy(); 1991 dst.eventParticipant = eventParticipant == null ? null : eventParticipant.copy(); 1992 dst.description = description == null ? null : description.copy(); 1993 if (suspectEntity != null) { 1994 dst.suspectEntity = new ArrayList<AdverseEventSuspectEntityComponent>(); 1995 for (AdverseEventSuspectEntityComponent i : suspectEntity) 1996 dst.suspectEntity.add(i.copy()); 1997 }; 1998 if (subjectMedicalHistory != null) { 1999 dst.subjectMedicalHistory = new ArrayList<Reference>(); 2000 for (Reference i : subjectMedicalHistory) 2001 dst.subjectMedicalHistory.add(i.copy()); 2002 }; 2003 if (referenceDocument != null) { 2004 dst.referenceDocument = new ArrayList<Reference>(); 2005 for (Reference i : referenceDocument) 2006 dst.referenceDocument.add(i.copy()); 2007 }; 2008 if (study != null) { 2009 dst.study = new ArrayList<Reference>(); 2010 for (Reference i : study) 2011 dst.study.add(i.copy()); 2012 }; 2013 return dst; 2014 } 2015 2016 protected AdverseEvent typedCopy() { 2017 return copy(); 2018 } 2019 2020 @Override 2021 public boolean equalsDeep(Base other_) { 2022 if (!super.equalsDeep(other_)) 2023 return false; 2024 if (!(other_ instanceof AdverseEvent)) 2025 return false; 2026 AdverseEvent o = (AdverseEvent) other_; 2027 return compareDeep(identifier, o.identifier, true) && compareDeep(category, o.category, true) && compareDeep(type, o.type, true) 2028 && compareDeep(subject, o.subject, true) && compareDeep(date, o.date, true) && compareDeep(reaction, o.reaction, true) 2029 && compareDeep(location, o.location, true) && compareDeep(seriousness, o.seriousness, true) && compareDeep(outcome, o.outcome, true) 2030 && compareDeep(recorder, o.recorder, true) && compareDeep(eventParticipant, o.eventParticipant, true) 2031 && compareDeep(description, o.description, true) && compareDeep(suspectEntity, o.suspectEntity, true) 2032 && compareDeep(subjectMedicalHistory, o.subjectMedicalHistory, true) && compareDeep(referenceDocument, o.referenceDocument, true) 2033 && compareDeep(study, o.study, true); 2034 } 2035 2036 @Override 2037 public boolean equalsShallow(Base other_) { 2038 if (!super.equalsShallow(other_)) 2039 return false; 2040 if (!(other_ instanceof AdverseEvent)) 2041 return false; 2042 AdverseEvent o = (AdverseEvent) other_; 2043 return compareValues(category, o.category, true) && compareValues(date, o.date, true) && compareValues(description, o.description, true) 2044 ; 2045 } 2046 2047 public boolean isEmpty() { 2048 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, category, type 2049 , subject, date, reaction, location, seriousness, outcome, recorder, eventParticipant 2050 , description, suspectEntity, subjectMedicalHistory, referenceDocument, study); 2051 } 2052 2053 @Override 2054 public ResourceType getResourceType() { 2055 return ResourceType.AdverseEvent; 2056 } 2057 2058 /** 2059 * Search parameter: <b>date</b> 2060 * <p> 2061 * Description: <b>When the event occurred</b><br> 2062 * Type: <b>date</b><br> 2063 * Path: <b>AdverseEvent.date</b><br> 2064 * </p> 2065 */ 2066 @SearchParamDefinition(name="date", path="AdverseEvent.date", description="When the event occurred", type="date" ) 2067 public static final String SP_DATE = "date"; 2068 /** 2069 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2070 * <p> 2071 * Description: <b>When the event occurred</b><br> 2072 * Type: <b>date</b><br> 2073 * Path: <b>AdverseEvent.date</b><br> 2074 * </p> 2075 */ 2076 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 2077 2078 /** 2079 * Search parameter: <b>recorder</b> 2080 * <p> 2081 * Description: <b>Who recorded the adverse event</b><br> 2082 * Type: <b>reference</b><br> 2083 * Path: <b>AdverseEvent.recorder</b><br> 2084 * </p> 2085 */ 2086 @SearchParamDefinition(name="recorder", path="AdverseEvent.recorder", description="Who recorded the adverse event", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Patient.class, Practitioner.class, RelatedPerson.class } ) 2087 public static final String SP_RECORDER = "recorder"; 2088 /** 2089 * <b>Fluent Client</b> search parameter constant for <b>recorder</b> 2090 * <p> 2091 * Description: <b>Who recorded the adverse event</b><br> 2092 * Type: <b>reference</b><br> 2093 * Path: <b>AdverseEvent.recorder</b><br> 2094 * </p> 2095 */ 2096 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RECORDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RECORDER); 2097 2098/** 2099 * Constant for fluent queries to be used to add include statements. Specifies 2100 * the path value of "<b>AdverseEvent:recorder</b>". 2101 */ 2102 public static final ca.uhn.fhir.model.api.Include INCLUDE_RECORDER = new ca.uhn.fhir.model.api.Include("AdverseEvent:recorder").toLocked(); 2103 2104 /** 2105 * Search parameter: <b>study</b> 2106 * <p> 2107 * Description: <b>AdverseEvent.study</b><br> 2108 * Type: <b>reference</b><br> 2109 * Path: <b>AdverseEvent.study</b><br> 2110 * </p> 2111 */ 2112 @SearchParamDefinition(name="study", path="AdverseEvent.study", description="AdverseEvent.study", type="reference", target={ResearchStudy.class } ) 2113 public static final String SP_STUDY = "study"; 2114 /** 2115 * <b>Fluent Client</b> search parameter constant for <b>study</b> 2116 * <p> 2117 * Description: <b>AdverseEvent.study</b><br> 2118 * Type: <b>reference</b><br> 2119 * Path: <b>AdverseEvent.study</b><br> 2120 * </p> 2121 */ 2122 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam STUDY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_STUDY); 2123 2124/** 2125 * Constant for fluent queries to be used to add include statements. Specifies 2126 * the path value of "<b>AdverseEvent:study</b>". 2127 */ 2128 public static final ca.uhn.fhir.model.api.Include INCLUDE_STUDY = new ca.uhn.fhir.model.api.Include("AdverseEvent:study").toLocked(); 2129 2130 /** 2131 * Search parameter: <b>reaction</b> 2132 * <p> 2133 * Description: <b>Adverse Reaction Events linked to exposure to substance</b><br> 2134 * Type: <b>reference</b><br> 2135 * Path: <b>AdverseEvent.reaction</b><br> 2136 * </p> 2137 */ 2138 @SearchParamDefinition(name="reaction", path="AdverseEvent.reaction", description="Adverse Reaction Events linked to exposure to substance", type="reference", target={Condition.class } ) 2139 public static final String SP_REACTION = "reaction"; 2140 /** 2141 * <b>Fluent Client</b> search parameter constant for <b>reaction</b> 2142 * <p> 2143 * Description: <b>Adverse Reaction Events linked to exposure to substance</b><br> 2144 * Type: <b>reference</b><br> 2145 * Path: <b>AdverseEvent.reaction</b><br> 2146 * </p> 2147 */ 2148 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REACTION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REACTION); 2149 2150/** 2151 * Constant for fluent queries to be used to add include statements. Specifies 2152 * the path value of "<b>AdverseEvent:reaction</b>". 2153 */ 2154 public static final ca.uhn.fhir.model.api.Include INCLUDE_REACTION = new ca.uhn.fhir.model.api.Include("AdverseEvent:reaction").toLocked(); 2155 2156 /** 2157 * Search parameter: <b>seriousness</b> 2158 * <p> 2159 * Description: <b>Mild | Moderate | Severe</b><br> 2160 * Type: <b>token</b><br> 2161 * Path: <b>AdverseEvent.seriousness</b><br> 2162 * </p> 2163 */ 2164 @SearchParamDefinition(name="seriousness", path="AdverseEvent.seriousness", description="Mild | Moderate | Severe", type="token" ) 2165 public static final String SP_SERIOUSNESS = "seriousness"; 2166 /** 2167 * <b>Fluent Client</b> search parameter constant for <b>seriousness</b> 2168 * <p> 2169 * Description: <b>Mild | Moderate | Severe</b><br> 2170 * Type: <b>token</b><br> 2171 * Path: <b>AdverseEvent.seriousness</b><br> 2172 * </p> 2173 */ 2174 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SERIOUSNESS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SERIOUSNESS); 2175 2176 /** 2177 * Search parameter: <b>subject</b> 2178 * <p> 2179 * Description: <b>Subject or group impacted by event</b><br> 2180 * Type: <b>reference</b><br> 2181 * Path: <b>AdverseEvent.subject</b><br> 2182 * </p> 2183 */ 2184 @SearchParamDefinition(name="subject", path="AdverseEvent.subject", description="Subject or group impacted by event", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Device.class, Medication.class, Patient.class, ResearchSubject.class } ) 2185 public static final String SP_SUBJECT = "subject"; 2186 /** 2187 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2188 * <p> 2189 * Description: <b>Subject or group impacted by event</b><br> 2190 * Type: <b>reference</b><br> 2191 * Path: <b>AdverseEvent.subject</b><br> 2192 * </p> 2193 */ 2194 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2195 2196/** 2197 * Constant for fluent queries to be used to add include statements. Specifies 2198 * the path value of "<b>AdverseEvent:subject</b>". 2199 */ 2200 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("AdverseEvent:subject").toLocked(); 2201 2202 /** 2203 * Search parameter: <b>substance</b> 2204 * <p> 2205 * Description: <b>Refers to the specific entity that caused the adverse event</b><br> 2206 * Type: <b>reference</b><br> 2207 * Path: <b>AdverseEvent.suspectEntity.instance</b><br> 2208 * </p> 2209 */ 2210 @SearchParamDefinition(name="substance", path="AdverseEvent.suspectEntity.instance", description="Refers to the specific entity that caused the adverse event", type="reference", target={Device.class, Medication.class, MedicationAdministration.class, MedicationStatement.class, Substance.class } ) 2211 public static final String SP_SUBSTANCE = "substance"; 2212 /** 2213 * <b>Fluent Client</b> search parameter constant for <b>substance</b> 2214 * <p> 2215 * Description: <b>Refers to the specific entity that caused the adverse event</b><br> 2216 * Type: <b>reference</b><br> 2217 * Path: <b>AdverseEvent.suspectEntity.instance</b><br> 2218 * </p> 2219 */ 2220 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBSTANCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBSTANCE); 2221 2222/** 2223 * Constant for fluent queries to be used to add include statements. Specifies 2224 * the path value of "<b>AdverseEvent:substance</b>". 2225 */ 2226 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBSTANCE = new ca.uhn.fhir.model.api.Include("AdverseEvent:substance").toLocked(); 2227 2228 /** 2229 * Search parameter: <b>location</b> 2230 * <p> 2231 * Description: <b>Location where adverse event occurred</b><br> 2232 * Type: <b>reference</b><br> 2233 * Path: <b>AdverseEvent.location</b><br> 2234 * </p> 2235 */ 2236 @SearchParamDefinition(name="location", path="AdverseEvent.location", description="Location where adverse event occurred", type="reference", target={Location.class } ) 2237 public static final String SP_LOCATION = "location"; 2238 /** 2239 * <b>Fluent Client</b> search parameter constant for <b>location</b> 2240 * <p> 2241 * Description: <b>Location where adverse event occurred</b><br> 2242 * Type: <b>reference</b><br> 2243 * Path: <b>AdverseEvent.location</b><br> 2244 * </p> 2245 */ 2246 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_LOCATION); 2247 2248/** 2249 * Constant for fluent queries to be used to add include statements. Specifies 2250 * the path value of "<b>AdverseEvent:location</b>". 2251 */ 2252 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include("AdverseEvent:location").toLocked(); 2253 2254 /** 2255 * Search parameter: <b>category</b> 2256 * <p> 2257 * Description: <b>AE | PAE 2258An adverse event is an event that caused harm to a patient, an adverse reaction is a something that is a subject-specific event that is a result of an exposure to a medication, food, device or environmental substance, a potential adverse event is something that occurred and that could have caused harm to a patient but did not</b><br> 2259 * Type: <b>token</b><br> 2260 * Path: <b>AdverseEvent.category</b><br> 2261 * </p> 2262 */ 2263 @SearchParamDefinition(name="category", path="AdverseEvent.category", description="AE | PAE \rAn adverse event is an event that caused harm to a patient, an adverse reaction is a something that is a subject-specific event that is a result of an exposure to a medication, food, device or environmental substance, a potential adverse event is something that occurred and that could have caused harm to a patient but did not", type="token" ) 2264 public static final String SP_CATEGORY = "category"; 2265 /** 2266 * <b>Fluent Client</b> search parameter constant for <b>category</b> 2267 * <p> 2268 * Description: <b>AE | PAE 2269An adverse event is an event that caused harm to a patient, an adverse reaction is a something that is a subject-specific event that is a result of an exposure to a medication, food, device or environmental substance, a potential adverse event is something that occurred and that could have caused harm to a patient but did not</b><br> 2270 * Type: <b>token</b><br> 2271 * Path: <b>AdverseEvent.category</b><br> 2272 * </p> 2273 */ 2274 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 2275 2276 /** 2277 * Search parameter: <b>type</b> 2278 * <p> 2279 * Description: <b>actual | potential</b><br> 2280 * Type: <b>token</b><br> 2281 * Path: <b>AdverseEvent.type</b><br> 2282 * </p> 2283 */ 2284 @SearchParamDefinition(name="type", path="AdverseEvent.type", description="actual | potential", type="token" ) 2285 public static final String SP_TYPE = "type"; 2286 /** 2287 * <b>Fluent Client</b> search parameter constant for <b>type</b> 2288 * <p> 2289 * Description: <b>actual | potential</b><br> 2290 * Type: <b>token</b><br> 2291 * Path: <b>AdverseEvent.type</b><br> 2292 * </p> 2293 */ 2294 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 2295 2296 2297}