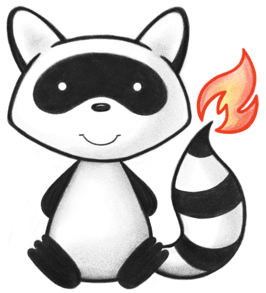
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.exceptions.FHIRFormatError; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042import org.hl7.fhir.utilities.Utilities; 043 044import ca.uhn.fhir.model.api.annotation.Block; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049/** 050 * Risk of harmful or undesirable, physiological response which is unique to an individual and associated with exposure to a substance. 051 */ 052@ResourceDef(name="AllergyIntolerance", profile="http://hl7.org/fhir/Profile/AllergyIntolerance") 053public class AllergyIntolerance extends DomainResource { 054 055 public enum AllergyIntoleranceClinicalStatus { 056 /** 057 * An active record of a risk of a reaction to the identified substance. 058 */ 059 ACTIVE, 060 /** 061 * An inactivated record of a risk of a reaction to the identified substance. 062 */ 063 INACTIVE, 064 /** 065 * A reaction to the identified substance has been clinically reassessed by testing or re-exposure and considered to be resolved. 066 */ 067 RESOLVED, 068 /** 069 * added to help the parsers with the generic types 070 */ 071 NULL; 072 public static AllergyIntoleranceClinicalStatus fromCode(String codeString) throws FHIRException { 073 if (codeString == null || "".equals(codeString)) 074 return null; 075 if ("active".equals(codeString)) 076 return ACTIVE; 077 if ("inactive".equals(codeString)) 078 return INACTIVE; 079 if ("resolved".equals(codeString)) 080 return RESOLVED; 081 if (Configuration.isAcceptInvalidEnums()) 082 return null; 083 else 084 throw new FHIRException("Unknown AllergyIntoleranceClinicalStatus code '"+codeString+"'"); 085 } 086 public String toCode() { 087 switch (this) { 088 case ACTIVE: return "active"; 089 case INACTIVE: return "inactive"; 090 case RESOLVED: return "resolved"; 091 case NULL: return null; 092 default: return "?"; 093 } 094 } 095 public String getSystem() { 096 switch (this) { 097 case ACTIVE: return "http://hl7.org/fhir/allergy-clinical-status"; 098 case INACTIVE: return "http://hl7.org/fhir/allergy-clinical-status"; 099 case RESOLVED: return "http://hl7.org/fhir/allergy-clinical-status"; 100 case NULL: return null; 101 default: return "?"; 102 } 103 } 104 public String getDefinition() { 105 switch (this) { 106 case ACTIVE: return "An active record of a risk of a reaction to the identified substance."; 107 case INACTIVE: return "An inactivated record of a risk of a reaction to the identified substance."; 108 case RESOLVED: return "A reaction to the identified substance has been clinically reassessed by testing or re-exposure and considered to be resolved."; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 public String getDisplay() { 114 switch (this) { 115 case ACTIVE: return "Active"; 116 case INACTIVE: return "Inactive"; 117 case RESOLVED: return "Resolved"; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 } 123 124 public static class AllergyIntoleranceClinicalStatusEnumFactory implements EnumFactory<AllergyIntoleranceClinicalStatus> { 125 public AllergyIntoleranceClinicalStatus fromCode(String codeString) throws IllegalArgumentException { 126 if (codeString == null || "".equals(codeString)) 127 if (codeString == null || "".equals(codeString)) 128 return null; 129 if ("active".equals(codeString)) 130 return AllergyIntoleranceClinicalStatus.ACTIVE; 131 if ("inactive".equals(codeString)) 132 return AllergyIntoleranceClinicalStatus.INACTIVE; 133 if ("resolved".equals(codeString)) 134 return AllergyIntoleranceClinicalStatus.RESOLVED; 135 throw new IllegalArgumentException("Unknown AllergyIntoleranceClinicalStatus code '"+codeString+"'"); 136 } 137 public Enumeration<AllergyIntoleranceClinicalStatus> fromType(PrimitiveType<?> code) throws FHIRException { 138 if (code == null) 139 return null; 140 if (code.isEmpty()) 141 return new Enumeration<AllergyIntoleranceClinicalStatus>(this); 142 String codeString = code.asStringValue(); 143 if (codeString == null || "".equals(codeString)) 144 return null; 145 if ("active".equals(codeString)) 146 return new Enumeration<AllergyIntoleranceClinicalStatus>(this, AllergyIntoleranceClinicalStatus.ACTIVE); 147 if ("inactive".equals(codeString)) 148 return new Enumeration<AllergyIntoleranceClinicalStatus>(this, AllergyIntoleranceClinicalStatus.INACTIVE); 149 if ("resolved".equals(codeString)) 150 return new Enumeration<AllergyIntoleranceClinicalStatus>(this, AllergyIntoleranceClinicalStatus.RESOLVED); 151 throw new FHIRException("Unknown AllergyIntoleranceClinicalStatus code '"+codeString+"'"); 152 } 153 public String toCode(AllergyIntoleranceClinicalStatus code) { 154 if (code == AllergyIntoleranceClinicalStatus.NULL) 155 return null; 156 if (code == AllergyIntoleranceClinicalStatus.ACTIVE) 157 return "active"; 158 if (code == AllergyIntoleranceClinicalStatus.INACTIVE) 159 return "inactive"; 160 if (code == AllergyIntoleranceClinicalStatus.RESOLVED) 161 return "resolved"; 162 return "?"; 163 } 164 public String toSystem(AllergyIntoleranceClinicalStatus code) { 165 return code.getSystem(); 166 } 167 } 168 169 public enum AllergyIntoleranceVerificationStatus { 170 /** 171 * A low level of certainty about the propensity for a reaction to the identified substance. 172 */ 173 UNCONFIRMED, 174 /** 175 * A high level of certainty about the propensity for a reaction to the identified substance, which may include clinical evidence by testing or rechallenge. 176 */ 177 CONFIRMED, 178 /** 179 * A propensity for a reaction to the identified substance has been disproven with a high level of clinical certainty, which may include testing or rechallenge, and is refuted. 180 */ 181 REFUTED, 182 /** 183 * The statement was entered in error and is not valid. 184 */ 185 ENTEREDINERROR, 186 /** 187 * added to help the parsers with the generic types 188 */ 189 NULL; 190 public static AllergyIntoleranceVerificationStatus fromCode(String codeString) throws FHIRException { 191 if (codeString == null || "".equals(codeString)) 192 return null; 193 if ("unconfirmed".equals(codeString)) 194 return UNCONFIRMED; 195 if ("confirmed".equals(codeString)) 196 return CONFIRMED; 197 if ("refuted".equals(codeString)) 198 return REFUTED; 199 if ("entered-in-error".equals(codeString)) 200 return ENTEREDINERROR; 201 if (Configuration.isAcceptInvalidEnums()) 202 return null; 203 else 204 throw new FHIRException("Unknown AllergyIntoleranceVerificationStatus code '"+codeString+"'"); 205 } 206 public String toCode() { 207 switch (this) { 208 case UNCONFIRMED: return "unconfirmed"; 209 case CONFIRMED: return "confirmed"; 210 case REFUTED: return "refuted"; 211 case ENTEREDINERROR: return "entered-in-error"; 212 case NULL: return null; 213 default: return "?"; 214 } 215 } 216 public String getSystem() { 217 switch (this) { 218 case UNCONFIRMED: return "http://hl7.org/fhir/allergy-verification-status"; 219 case CONFIRMED: return "http://hl7.org/fhir/allergy-verification-status"; 220 case REFUTED: return "http://hl7.org/fhir/allergy-verification-status"; 221 case ENTEREDINERROR: return "http://hl7.org/fhir/allergy-verification-status"; 222 case NULL: return null; 223 default: return "?"; 224 } 225 } 226 public String getDefinition() { 227 switch (this) { 228 case UNCONFIRMED: return "A low level of certainty about the propensity for a reaction to the identified substance."; 229 case CONFIRMED: return "A high level of certainty about the propensity for a reaction to the identified substance, which may include clinical evidence by testing or rechallenge."; 230 case REFUTED: return "A propensity for a reaction to the identified substance has been disproven with a high level of clinical certainty, which may include testing or rechallenge, and is refuted."; 231 case ENTEREDINERROR: return "The statement was entered in error and is not valid."; 232 case NULL: return null; 233 default: return "?"; 234 } 235 } 236 public String getDisplay() { 237 switch (this) { 238 case UNCONFIRMED: return "Unconfirmed"; 239 case CONFIRMED: return "Confirmed"; 240 case REFUTED: return "Refuted"; 241 case ENTEREDINERROR: return "Entered In Error"; 242 case NULL: return null; 243 default: return "?"; 244 } 245 } 246 } 247 248 public static class AllergyIntoleranceVerificationStatusEnumFactory implements EnumFactory<AllergyIntoleranceVerificationStatus> { 249 public AllergyIntoleranceVerificationStatus fromCode(String codeString) throws IllegalArgumentException { 250 if (codeString == null || "".equals(codeString)) 251 if (codeString == null || "".equals(codeString)) 252 return null; 253 if ("unconfirmed".equals(codeString)) 254 return AllergyIntoleranceVerificationStatus.UNCONFIRMED; 255 if ("confirmed".equals(codeString)) 256 return AllergyIntoleranceVerificationStatus.CONFIRMED; 257 if ("refuted".equals(codeString)) 258 return AllergyIntoleranceVerificationStatus.REFUTED; 259 if ("entered-in-error".equals(codeString)) 260 return AllergyIntoleranceVerificationStatus.ENTEREDINERROR; 261 throw new IllegalArgumentException("Unknown AllergyIntoleranceVerificationStatus code '"+codeString+"'"); 262 } 263 public Enumeration<AllergyIntoleranceVerificationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 264 if (code == null) 265 return null; 266 if (code.isEmpty()) 267 return new Enumeration<AllergyIntoleranceVerificationStatus>(this); 268 String codeString = code.asStringValue(); 269 if (codeString == null || "".equals(codeString)) 270 return null; 271 if ("unconfirmed".equals(codeString)) 272 return new Enumeration<AllergyIntoleranceVerificationStatus>(this, AllergyIntoleranceVerificationStatus.UNCONFIRMED); 273 if ("confirmed".equals(codeString)) 274 return new Enumeration<AllergyIntoleranceVerificationStatus>(this, AllergyIntoleranceVerificationStatus.CONFIRMED); 275 if ("refuted".equals(codeString)) 276 return new Enumeration<AllergyIntoleranceVerificationStatus>(this, AllergyIntoleranceVerificationStatus.REFUTED); 277 if ("entered-in-error".equals(codeString)) 278 return new Enumeration<AllergyIntoleranceVerificationStatus>(this, AllergyIntoleranceVerificationStatus.ENTEREDINERROR); 279 throw new FHIRException("Unknown AllergyIntoleranceVerificationStatus code '"+codeString+"'"); 280 } 281 public String toCode(AllergyIntoleranceVerificationStatus code) { 282 if (code == AllergyIntoleranceVerificationStatus.NULL) 283 return null; 284 if (code == AllergyIntoleranceVerificationStatus.UNCONFIRMED) 285 return "unconfirmed"; 286 if (code == AllergyIntoleranceVerificationStatus.CONFIRMED) 287 return "confirmed"; 288 if (code == AllergyIntoleranceVerificationStatus.REFUTED) 289 return "refuted"; 290 if (code == AllergyIntoleranceVerificationStatus.ENTEREDINERROR) 291 return "entered-in-error"; 292 return "?"; 293 } 294 public String toSystem(AllergyIntoleranceVerificationStatus code) { 295 return code.getSystem(); 296 } 297 } 298 299 public enum AllergyIntoleranceType { 300 /** 301 * A propensity for hypersensitivity reaction(s) to a substance. These reactions are most typically type I hypersensitivity, plus other "allergy-like" reactions, including pseudoallergy. 302 */ 303 ALLERGY, 304 /** 305 * A propensity for adverse reactions to a substance that is not judged to be allergic or "allergy-like". These reactions are typically (but not necessarily) non-immune. They are to some degree idiosyncratic and/or individually specific (i.e. are not a reaction that is expected to occur with most or all patients given similar circumstances). 306 */ 307 INTOLERANCE, 308 /** 309 * added to help the parsers with the generic types 310 */ 311 NULL; 312 public static AllergyIntoleranceType fromCode(String codeString) throws FHIRException { 313 if (codeString == null || "".equals(codeString)) 314 return null; 315 if ("allergy".equals(codeString)) 316 return ALLERGY; 317 if ("intolerance".equals(codeString)) 318 return INTOLERANCE; 319 if (Configuration.isAcceptInvalidEnums()) 320 return null; 321 else 322 throw new FHIRException("Unknown AllergyIntoleranceType code '"+codeString+"'"); 323 } 324 public String toCode() { 325 switch (this) { 326 case ALLERGY: return "allergy"; 327 case INTOLERANCE: return "intolerance"; 328 case NULL: return null; 329 default: return "?"; 330 } 331 } 332 public String getSystem() { 333 switch (this) { 334 case ALLERGY: return "http://hl7.org/fhir/allergy-intolerance-type"; 335 case INTOLERANCE: return "http://hl7.org/fhir/allergy-intolerance-type"; 336 case NULL: return null; 337 default: return "?"; 338 } 339 } 340 public String getDefinition() { 341 switch (this) { 342 case ALLERGY: return "A propensity for hypersensitivity reaction(s) to a substance. These reactions are most typically type I hypersensitivity, plus other \"allergy-like\" reactions, including pseudoallergy."; 343 case INTOLERANCE: return "A propensity for adverse reactions to a substance that is not judged to be allergic or \"allergy-like\". These reactions are typically (but not necessarily) non-immune. They are to some degree idiosyncratic and/or individually specific (i.e. are not a reaction that is expected to occur with most or all patients given similar circumstances)."; 344 case NULL: return null; 345 default: return "?"; 346 } 347 } 348 public String getDisplay() { 349 switch (this) { 350 case ALLERGY: return "Allergy"; 351 case INTOLERANCE: return "Intolerance"; 352 case NULL: return null; 353 default: return "?"; 354 } 355 } 356 } 357 358 public static class AllergyIntoleranceTypeEnumFactory implements EnumFactory<AllergyIntoleranceType> { 359 public AllergyIntoleranceType fromCode(String codeString) throws IllegalArgumentException { 360 if (codeString == null || "".equals(codeString)) 361 if (codeString == null || "".equals(codeString)) 362 return null; 363 if ("allergy".equals(codeString)) 364 return AllergyIntoleranceType.ALLERGY; 365 if ("intolerance".equals(codeString)) 366 return AllergyIntoleranceType.INTOLERANCE; 367 throw new IllegalArgumentException("Unknown AllergyIntoleranceType code '"+codeString+"'"); 368 } 369 public Enumeration<AllergyIntoleranceType> fromType(PrimitiveType<?> code) throws FHIRException { 370 if (code == null) 371 return null; 372 if (code.isEmpty()) 373 return new Enumeration<AllergyIntoleranceType>(this); 374 String codeString = code.asStringValue(); 375 if (codeString == null || "".equals(codeString)) 376 return null; 377 if ("allergy".equals(codeString)) 378 return new Enumeration<AllergyIntoleranceType>(this, AllergyIntoleranceType.ALLERGY); 379 if ("intolerance".equals(codeString)) 380 return new Enumeration<AllergyIntoleranceType>(this, AllergyIntoleranceType.INTOLERANCE); 381 throw new FHIRException("Unknown AllergyIntoleranceType code '"+codeString+"'"); 382 } 383 public String toCode(AllergyIntoleranceType code) { 384 if (code == AllergyIntoleranceType.NULL) 385 return null; 386 if (code == AllergyIntoleranceType.ALLERGY) 387 return "allergy"; 388 if (code == AllergyIntoleranceType.INTOLERANCE) 389 return "intolerance"; 390 return "?"; 391 } 392 public String toSystem(AllergyIntoleranceType code) { 393 return code.getSystem(); 394 } 395 } 396 397 public enum AllergyIntoleranceCategory { 398 /** 399 * Any substance consumed to provide nutritional support for the body. 400 */ 401 FOOD, 402 /** 403 * Substances administered to achieve a physiological effect. 404 */ 405 MEDICATION, 406 /** 407 * Any substances that are encountered in the environment, including any substance not already classified as food, medication, or biologic. 408 */ 409 ENVIRONMENT, 410 /** 411 * A preparation that is synthesized from living organisms or their products, especially a human or animal protein, such as a hormone or antitoxin, that is used as a diagnostic, preventive, or therapeutic agent. Examples of biologic medications include: vaccines; allergenic extracts, which are used for both diagnosis and treatment (for example, allergy shots); gene therapies; cellular therapies. There are other biologic products, such as tissues, that are not typically associated with allergies. 412 */ 413 BIOLOGIC, 414 /** 415 * added to help the parsers with the generic types 416 */ 417 NULL; 418 public static AllergyIntoleranceCategory fromCode(String codeString) throws FHIRException { 419 if (codeString == null || "".equals(codeString)) 420 return null; 421 if ("food".equals(codeString)) 422 return FOOD; 423 if ("medication".equals(codeString)) 424 return MEDICATION; 425 if ("environment".equals(codeString)) 426 return ENVIRONMENT; 427 if ("biologic".equals(codeString)) 428 return BIOLOGIC; 429 if (Configuration.isAcceptInvalidEnums()) 430 return null; 431 else 432 throw new FHIRException("Unknown AllergyIntoleranceCategory code '"+codeString+"'"); 433 } 434 public String toCode() { 435 switch (this) { 436 case FOOD: return "food"; 437 case MEDICATION: return "medication"; 438 case ENVIRONMENT: return "environment"; 439 case BIOLOGIC: return "biologic"; 440 case NULL: return null; 441 default: return "?"; 442 } 443 } 444 public String getSystem() { 445 switch (this) { 446 case FOOD: return "http://hl7.org/fhir/allergy-intolerance-category"; 447 case MEDICATION: return "http://hl7.org/fhir/allergy-intolerance-category"; 448 case ENVIRONMENT: return "http://hl7.org/fhir/allergy-intolerance-category"; 449 case BIOLOGIC: return "http://hl7.org/fhir/allergy-intolerance-category"; 450 case NULL: return null; 451 default: return "?"; 452 } 453 } 454 public String getDefinition() { 455 switch (this) { 456 case FOOD: return "Any substance consumed to provide nutritional support for the body."; 457 case MEDICATION: return "Substances administered to achieve a physiological effect."; 458 case ENVIRONMENT: return "Any substances that are encountered in the environment, including any substance not already classified as food, medication, or biologic."; 459 case BIOLOGIC: return "A preparation that is synthesized from living organisms or their products, especially a human or animal protein, such as a hormone or antitoxin, that is used as a diagnostic, preventive, or therapeutic agent. Examples of biologic medications include: vaccines; allergenic extracts, which are used for both diagnosis and treatment (for example, allergy shots); gene therapies; cellular therapies. There are other biologic products, such as tissues, that are not typically associated with allergies."; 460 case NULL: return null; 461 default: return "?"; 462 } 463 } 464 public String getDisplay() { 465 switch (this) { 466 case FOOD: return "Food"; 467 case MEDICATION: return "Medication"; 468 case ENVIRONMENT: return "Environment"; 469 case BIOLOGIC: return "Biologic"; 470 case NULL: return null; 471 default: return "?"; 472 } 473 } 474 } 475 476 public static class AllergyIntoleranceCategoryEnumFactory implements EnumFactory<AllergyIntoleranceCategory> { 477 public AllergyIntoleranceCategory fromCode(String codeString) throws IllegalArgumentException { 478 if (codeString == null || "".equals(codeString)) 479 if (codeString == null || "".equals(codeString)) 480 return null; 481 if ("food".equals(codeString)) 482 return AllergyIntoleranceCategory.FOOD; 483 if ("medication".equals(codeString)) 484 return AllergyIntoleranceCategory.MEDICATION; 485 if ("environment".equals(codeString)) 486 return AllergyIntoleranceCategory.ENVIRONMENT; 487 if ("biologic".equals(codeString)) 488 return AllergyIntoleranceCategory.BIOLOGIC; 489 throw new IllegalArgumentException("Unknown AllergyIntoleranceCategory code '"+codeString+"'"); 490 } 491 public Enumeration<AllergyIntoleranceCategory> fromType(PrimitiveType<?> code) throws FHIRException { 492 if (code == null) 493 return null; 494 if (code.isEmpty()) 495 return new Enumeration<AllergyIntoleranceCategory>(this); 496 String codeString = code.asStringValue(); 497 if (codeString == null || "".equals(codeString)) 498 return null; 499 if ("food".equals(codeString)) 500 return new Enumeration<AllergyIntoleranceCategory>(this, AllergyIntoleranceCategory.FOOD); 501 if ("medication".equals(codeString)) 502 return new Enumeration<AllergyIntoleranceCategory>(this, AllergyIntoleranceCategory.MEDICATION); 503 if ("environment".equals(codeString)) 504 return new Enumeration<AllergyIntoleranceCategory>(this, AllergyIntoleranceCategory.ENVIRONMENT); 505 if ("biologic".equals(codeString)) 506 return new Enumeration<AllergyIntoleranceCategory>(this, AllergyIntoleranceCategory.BIOLOGIC); 507 throw new FHIRException("Unknown AllergyIntoleranceCategory code '"+codeString+"'"); 508 } 509 public String toCode(AllergyIntoleranceCategory code) { 510 if (code == AllergyIntoleranceCategory.NULL) 511 return null; 512 if (code == AllergyIntoleranceCategory.FOOD) 513 return "food"; 514 if (code == AllergyIntoleranceCategory.MEDICATION) 515 return "medication"; 516 if (code == AllergyIntoleranceCategory.ENVIRONMENT) 517 return "environment"; 518 if (code == AllergyIntoleranceCategory.BIOLOGIC) 519 return "biologic"; 520 return "?"; 521 } 522 public String toSystem(AllergyIntoleranceCategory code) { 523 return code.getSystem(); 524 } 525 } 526 527 public enum AllergyIntoleranceCriticality { 528 /** 529 * Worst case result of a future exposure is not assessed to be life-threatening or having high potential for organ system failure. 530 */ 531 LOW, 532 /** 533 * Worst case result of a future exposure is assessed to be life-threatening or having high potential for organ system failure. 534 */ 535 HIGH, 536 /** 537 * Unable to assess the worst case result of a future exposure. 538 */ 539 UNABLETOASSESS, 540 /** 541 * added to help the parsers with the generic types 542 */ 543 NULL; 544 public static AllergyIntoleranceCriticality fromCode(String codeString) throws FHIRException { 545 if (codeString == null || "".equals(codeString)) 546 return null; 547 if ("low".equals(codeString)) 548 return LOW; 549 if ("high".equals(codeString)) 550 return HIGH; 551 if ("unable-to-assess".equals(codeString)) 552 return UNABLETOASSESS; 553 if (Configuration.isAcceptInvalidEnums()) 554 return null; 555 else 556 throw new FHIRException("Unknown AllergyIntoleranceCriticality code '"+codeString+"'"); 557 } 558 public String toCode() { 559 switch (this) { 560 case LOW: return "low"; 561 case HIGH: return "high"; 562 case UNABLETOASSESS: return "unable-to-assess"; 563 case NULL: return null; 564 default: return "?"; 565 } 566 } 567 public String getSystem() { 568 switch (this) { 569 case LOW: return "http://hl7.org/fhir/allergy-intolerance-criticality"; 570 case HIGH: return "http://hl7.org/fhir/allergy-intolerance-criticality"; 571 case UNABLETOASSESS: return "http://hl7.org/fhir/allergy-intolerance-criticality"; 572 case NULL: return null; 573 default: return "?"; 574 } 575 } 576 public String getDefinition() { 577 switch (this) { 578 case LOW: return "Worst case result of a future exposure is not assessed to be life-threatening or having high potential for organ system failure."; 579 case HIGH: return "Worst case result of a future exposure is assessed to be life-threatening or having high potential for organ system failure."; 580 case UNABLETOASSESS: return "Unable to assess the worst case result of a future exposure."; 581 case NULL: return null; 582 default: return "?"; 583 } 584 } 585 public String getDisplay() { 586 switch (this) { 587 case LOW: return "Low Risk"; 588 case HIGH: return "High Risk"; 589 case UNABLETOASSESS: return "Unable to Assess Risk"; 590 case NULL: return null; 591 default: return "?"; 592 } 593 } 594 } 595 596 public static class AllergyIntoleranceCriticalityEnumFactory implements EnumFactory<AllergyIntoleranceCriticality> { 597 public AllergyIntoleranceCriticality fromCode(String codeString) throws IllegalArgumentException { 598 if (codeString == null || "".equals(codeString)) 599 if (codeString == null || "".equals(codeString)) 600 return null; 601 if ("low".equals(codeString)) 602 return AllergyIntoleranceCriticality.LOW; 603 if ("high".equals(codeString)) 604 return AllergyIntoleranceCriticality.HIGH; 605 if ("unable-to-assess".equals(codeString)) 606 return AllergyIntoleranceCriticality.UNABLETOASSESS; 607 throw new IllegalArgumentException("Unknown AllergyIntoleranceCriticality code '"+codeString+"'"); 608 } 609 public Enumeration<AllergyIntoleranceCriticality> fromType(PrimitiveType<?> code) throws FHIRException { 610 if (code == null) 611 return null; 612 if (code.isEmpty()) 613 return new Enumeration<AllergyIntoleranceCriticality>(this); 614 String codeString = code.asStringValue(); 615 if (codeString == null || "".equals(codeString)) 616 return null; 617 if ("low".equals(codeString)) 618 return new Enumeration<AllergyIntoleranceCriticality>(this, AllergyIntoleranceCriticality.LOW); 619 if ("high".equals(codeString)) 620 return new Enumeration<AllergyIntoleranceCriticality>(this, AllergyIntoleranceCriticality.HIGH); 621 if ("unable-to-assess".equals(codeString)) 622 return new Enumeration<AllergyIntoleranceCriticality>(this, AllergyIntoleranceCriticality.UNABLETOASSESS); 623 throw new FHIRException("Unknown AllergyIntoleranceCriticality code '"+codeString+"'"); 624 } 625 public String toCode(AllergyIntoleranceCriticality code) { 626 if (code == AllergyIntoleranceCriticality.NULL) 627 return null; 628 if (code == AllergyIntoleranceCriticality.LOW) 629 return "low"; 630 if (code == AllergyIntoleranceCriticality.HIGH) 631 return "high"; 632 if (code == AllergyIntoleranceCriticality.UNABLETOASSESS) 633 return "unable-to-assess"; 634 return "?"; 635 } 636 public String toSystem(AllergyIntoleranceCriticality code) { 637 return code.getSystem(); 638 } 639 } 640 641 public enum AllergyIntoleranceSeverity { 642 /** 643 * Causes mild physiological effects. 644 */ 645 MILD, 646 /** 647 * Causes moderate physiological effects. 648 */ 649 MODERATE, 650 /** 651 * Causes severe physiological effects. 652 */ 653 SEVERE, 654 /** 655 * added to help the parsers with the generic types 656 */ 657 NULL; 658 public static AllergyIntoleranceSeverity fromCode(String codeString) throws FHIRException { 659 if (codeString == null || "".equals(codeString)) 660 return null; 661 if ("mild".equals(codeString)) 662 return MILD; 663 if ("moderate".equals(codeString)) 664 return MODERATE; 665 if ("severe".equals(codeString)) 666 return SEVERE; 667 if (Configuration.isAcceptInvalidEnums()) 668 return null; 669 else 670 throw new FHIRException("Unknown AllergyIntoleranceSeverity code '"+codeString+"'"); 671 } 672 public String toCode() { 673 switch (this) { 674 case MILD: return "mild"; 675 case MODERATE: return "moderate"; 676 case SEVERE: return "severe"; 677 case NULL: return null; 678 default: return "?"; 679 } 680 } 681 public String getSystem() { 682 switch (this) { 683 case MILD: return "http://hl7.org/fhir/reaction-event-severity"; 684 case MODERATE: return "http://hl7.org/fhir/reaction-event-severity"; 685 case SEVERE: return "http://hl7.org/fhir/reaction-event-severity"; 686 case NULL: return null; 687 default: return "?"; 688 } 689 } 690 public String getDefinition() { 691 switch (this) { 692 case MILD: return "Causes mild physiological effects."; 693 case MODERATE: return "Causes moderate physiological effects."; 694 case SEVERE: return "Causes severe physiological effects."; 695 case NULL: return null; 696 default: return "?"; 697 } 698 } 699 public String getDisplay() { 700 switch (this) { 701 case MILD: return "Mild"; 702 case MODERATE: return "Moderate"; 703 case SEVERE: return "Severe"; 704 case NULL: return null; 705 default: return "?"; 706 } 707 } 708 } 709 710 public static class AllergyIntoleranceSeverityEnumFactory implements EnumFactory<AllergyIntoleranceSeverity> { 711 public AllergyIntoleranceSeverity fromCode(String codeString) throws IllegalArgumentException { 712 if (codeString == null || "".equals(codeString)) 713 if (codeString == null || "".equals(codeString)) 714 return null; 715 if ("mild".equals(codeString)) 716 return AllergyIntoleranceSeverity.MILD; 717 if ("moderate".equals(codeString)) 718 return AllergyIntoleranceSeverity.MODERATE; 719 if ("severe".equals(codeString)) 720 return AllergyIntoleranceSeverity.SEVERE; 721 throw new IllegalArgumentException("Unknown AllergyIntoleranceSeverity code '"+codeString+"'"); 722 } 723 public Enumeration<AllergyIntoleranceSeverity> fromType(PrimitiveType<?> code) throws FHIRException { 724 if (code == null) 725 return null; 726 if (code.isEmpty()) 727 return new Enumeration<AllergyIntoleranceSeverity>(this); 728 String codeString = code.asStringValue(); 729 if (codeString == null || "".equals(codeString)) 730 return null; 731 if ("mild".equals(codeString)) 732 return new Enumeration<AllergyIntoleranceSeverity>(this, AllergyIntoleranceSeverity.MILD); 733 if ("moderate".equals(codeString)) 734 return new Enumeration<AllergyIntoleranceSeverity>(this, AllergyIntoleranceSeverity.MODERATE); 735 if ("severe".equals(codeString)) 736 return new Enumeration<AllergyIntoleranceSeverity>(this, AllergyIntoleranceSeverity.SEVERE); 737 throw new FHIRException("Unknown AllergyIntoleranceSeverity code '"+codeString+"'"); 738 } 739 public String toCode(AllergyIntoleranceSeverity code) { 740 if (code == AllergyIntoleranceSeverity.NULL) 741 return null; 742 if (code == AllergyIntoleranceSeverity.MILD) 743 return "mild"; 744 if (code == AllergyIntoleranceSeverity.MODERATE) 745 return "moderate"; 746 if (code == AllergyIntoleranceSeverity.SEVERE) 747 return "severe"; 748 return "?"; 749 } 750 public String toSystem(AllergyIntoleranceSeverity code) { 751 return code.getSystem(); 752 } 753 } 754 755 @Block() 756 public static class AllergyIntoleranceReactionComponent extends BackboneElement implements IBaseBackboneElement { 757 /** 758 * Identification of the specific substance (or pharmaceutical product) considered to be responsible for the Adverse Reaction event. Note: the substance for a specific reaction may be different from the substance identified as the cause of the risk, but it must be consistent with it. For instance, it may be a more specific substance (e.g. a brand medication) or a composite product that includes the identified substance. It must be clinically safe to only process the 'code' and ignore the 'reaction.substance'. 759 */ 760 @Child(name = "substance", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 761 @Description(shortDefinition="Specific substance or pharmaceutical product considered to be responsible for event", formalDefinition="Identification of the specific substance (or pharmaceutical product) considered to be responsible for the Adverse Reaction event. Note: the substance for a specific reaction may be different from the substance identified as the cause of the risk, but it must be consistent with it. For instance, it may be a more specific substance (e.g. a brand medication) or a composite product that includes the identified substance. It must be clinically safe to only process the 'code' and ignore the 'reaction.substance'." ) 762 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-code") 763 protected CodeableConcept substance; 764 765 /** 766 * Clinical symptoms and/or signs that are observed or associated with the adverse reaction event. 767 */ 768 @Child(name = "manifestation", type = {CodeableConcept.class}, order=2, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 769 @Description(shortDefinition="Clinical symptoms/signs associated with the Event", formalDefinition="Clinical symptoms and/or signs that are observed or associated with the adverse reaction event." ) 770 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/clinical-findings") 771 protected List<CodeableConcept> manifestation; 772 773 /** 774 * Text description about the reaction as a whole, including details of the manifestation if required. 775 */ 776 @Child(name = "description", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 777 @Description(shortDefinition="Description of the event as a whole", formalDefinition="Text description about the reaction as a whole, including details of the manifestation if required." ) 778 protected StringType description; 779 780 /** 781 * Record of the date and/or time of the onset of the Reaction. 782 */ 783 @Child(name = "onset", type = {DateTimeType.class}, order=4, min=0, max=1, modifier=false, summary=false) 784 @Description(shortDefinition="Date(/time) when manifestations showed", formalDefinition="Record of the date and/or time of the onset of the Reaction." ) 785 protected DateTimeType onset; 786 787 /** 788 * Clinical assessment of the severity of the reaction event as a whole, potentially considering multiple different manifestations. 789 */ 790 @Child(name = "severity", type = {CodeType.class}, order=5, min=0, max=1, modifier=false, summary=false) 791 @Description(shortDefinition="mild | moderate | severe (of event as a whole)", formalDefinition="Clinical assessment of the severity of the reaction event as a whole, potentially considering multiple different manifestations." ) 792 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/reaction-event-severity") 793 protected Enumeration<AllergyIntoleranceSeverity> severity; 794 795 /** 796 * Identification of the route by which the subject was exposed to the substance. 797 */ 798 @Child(name = "exposureRoute", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 799 @Description(shortDefinition="How the subject was exposed to the substance", formalDefinition="Identification of the route by which the subject was exposed to the substance." ) 800 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/route-codes") 801 protected CodeableConcept exposureRoute; 802 803 /** 804 * Additional text about the adverse reaction event not captured in other fields. 805 */ 806 @Child(name = "note", type = {Annotation.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 807 @Description(shortDefinition="Text about event not captured in other fields", formalDefinition="Additional text about the adverse reaction event not captured in other fields." ) 808 protected List<Annotation> note; 809 810 private static final long serialVersionUID = -752118516L; 811 812 /** 813 * Constructor 814 */ 815 public AllergyIntoleranceReactionComponent() { 816 super(); 817 } 818 819 /** 820 * @return {@link #substance} (Identification of the specific substance (or pharmaceutical product) considered to be responsible for the Adverse Reaction event. Note: the substance for a specific reaction may be different from the substance identified as the cause of the risk, but it must be consistent with it. For instance, it may be a more specific substance (e.g. a brand medication) or a composite product that includes the identified substance. It must be clinically safe to only process the 'code' and ignore the 'reaction.substance'.) 821 */ 822 public CodeableConcept getSubstance() { 823 if (this.substance == null) 824 if (Configuration.errorOnAutoCreate()) 825 throw new Error("Attempt to auto-create AllergyIntoleranceReactionComponent.substance"); 826 else if (Configuration.doAutoCreate()) 827 this.substance = new CodeableConcept(); // cc 828 return this.substance; 829 } 830 831 public boolean hasSubstance() { 832 return this.substance != null && !this.substance.isEmpty(); 833 } 834 835 /** 836 * @param value {@link #substance} (Identification of the specific substance (or pharmaceutical product) considered to be responsible for the Adverse Reaction event. Note: the substance for a specific reaction may be different from the substance identified as the cause of the risk, but it must be consistent with it. For instance, it may be a more specific substance (e.g. a brand medication) or a composite product that includes the identified substance. It must be clinically safe to only process the 'code' and ignore the 'reaction.substance'.) 837 */ 838 public AllergyIntoleranceReactionComponent setSubstance(CodeableConcept value) { 839 this.substance = value; 840 return this; 841 } 842 843 /** 844 * @return {@link #manifestation} (Clinical symptoms and/or signs that are observed or associated with the adverse reaction event.) 845 */ 846 public List<CodeableConcept> getManifestation() { 847 if (this.manifestation == null) 848 this.manifestation = new ArrayList<CodeableConcept>(); 849 return this.manifestation; 850 } 851 852 /** 853 * @return Returns a reference to <code>this</code> for easy method chaining 854 */ 855 public AllergyIntoleranceReactionComponent setManifestation(List<CodeableConcept> theManifestation) { 856 this.manifestation = theManifestation; 857 return this; 858 } 859 860 public boolean hasManifestation() { 861 if (this.manifestation == null) 862 return false; 863 for (CodeableConcept item : this.manifestation) 864 if (!item.isEmpty()) 865 return true; 866 return false; 867 } 868 869 public CodeableConcept addManifestation() { //3 870 CodeableConcept t = new CodeableConcept(); 871 if (this.manifestation == null) 872 this.manifestation = new ArrayList<CodeableConcept>(); 873 this.manifestation.add(t); 874 return t; 875 } 876 877 public AllergyIntoleranceReactionComponent addManifestation(CodeableConcept t) { //3 878 if (t == null) 879 return this; 880 if (this.manifestation == null) 881 this.manifestation = new ArrayList<CodeableConcept>(); 882 this.manifestation.add(t); 883 return this; 884 } 885 886 /** 887 * @return The first repetition of repeating field {@link #manifestation}, creating it if it does not already exist 888 */ 889 public CodeableConcept getManifestationFirstRep() { 890 if (getManifestation().isEmpty()) { 891 addManifestation(); 892 } 893 return getManifestation().get(0); 894 } 895 896 /** 897 * @return {@link #description} (Text description about the reaction as a whole, including details of the manifestation if required.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 898 */ 899 public StringType getDescriptionElement() { 900 if (this.description == null) 901 if (Configuration.errorOnAutoCreate()) 902 throw new Error("Attempt to auto-create AllergyIntoleranceReactionComponent.description"); 903 else if (Configuration.doAutoCreate()) 904 this.description = new StringType(); // bb 905 return this.description; 906 } 907 908 public boolean hasDescriptionElement() { 909 return this.description != null && !this.description.isEmpty(); 910 } 911 912 public boolean hasDescription() { 913 return this.description != null && !this.description.isEmpty(); 914 } 915 916 /** 917 * @param value {@link #description} (Text description about the reaction as a whole, including details of the manifestation if required.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 918 */ 919 public AllergyIntoleranceReactionComponent setDescriptionElement(StringType value) { 920 this.description = value; 921 return this; 922 } 923 924 /** 925 * @return Text description about the reaction as a whole, including details of the manifestation if required. 926 */ 927 public String getDescription() { 928 return this.description == null ? null : this.description.getValue(); 929 } 930 931 /** 932 * @param value Text description about the reaction as a whole, including details of the manifestation if required. 933 */ 934 public AllergyIntoleranceReactionComponent setDescription(String value) { 935 if (Utilities.noString(value)) 936 this.description = null; 937 else { 938 if (this.description == null) 939 this.description = new StringType(); 940 this.description.setValue(value); 941 } 942 return this; 943 } 944 945 /** 946 * @return {@link #onset} (Record of the date and/or time of the onset of the Reaction.). This is the underlying object with id, value and extensions. The accessor "getOnset" gives direct access to the value 947 */ 948 public DateTimeType getOnsetElement() { 949 if (this.onset == null) 950 if (Configuration.errorOnAutoCreate()) 951 throw new Error("Attempt to auto-create AllergyIntoleranceReactionComponent.onset"); 952 else if (Configuration.doAutoCreate()) 953 this.onset = new DateTimeType(); // bb 954 return this.onset; 955 } 956 957 public boolean hasOnsetElement() { 958 return this.onset != null && !this.onset.isEmpty(); 959 } 960 961 public boolean hasOnset() { 962 return this.onset != null && !this.onset.isEmpty(); 963 } 964 965 /** 966 * @param value {@link #onset} (Record of the date and/or time of the onset of the Reaction.). This is the underlying object with id, value and extensions. The accessor "getOnset" gives direct access to the value 967 */ 968 public AllergyIntoleranceReactionComponent setOnsetElement(DateTimeType value) { 969 this.onset = value; 970 return this; 971 } 972 973 /** 974 * @return Record of the date and/or time of the onset of the Reaction. 975 */ 976 public Date getOnset() { 977 return this.onset == null ? null : this.onset.getValue(); 978 } 979 980 /** 981 * @param value Record of the date and/or time of the onset of the Reaction. 982 */ 983 public AllergyIntoleranceReactionComponent setOnset(Date value) { 984 if (value == null) 985 this.onset = null; 986 else { 987 if (this.onset == null) 988 this.onset = new DateTimeType(); 989 this.onset.setValue(value); 990 } 991 return this; 992 } 993 994 /** 995 * @return {@link #severity} (Clinical assessment of the severity of the reaction event as a whole, potentially considering multiple different manifestations.). This is the underlying object with id, value and extensions. The accessor "getSeverity" gives direct access to the value 996 */ 997 public Enumeration<AllergyIntoleranceSeverity> getSeverityElement() { 998 if (this.severity == null) 999 if (Configuration.errorOnAutoCreate()) 1000 throw new Error("Attempt to auto-create AllergyIntoleranceReactionComponent.severity"); 1001 else if (Configuration.doAutoCreate()) 1002 this.severity = new Enumeration<AllergyIntoleranceSeverity>(new AllergyIntoleranceSeverityEnumFactory()); // bb 1003 return this.severity; 1004 } 1005 1006 public boolean hasSeverityElement() { 1007 return this.severity != null && !this.severity.isEmpty(); 1008 } 1009 1010 public boolean hasSeverity() { 1011 return this.severity != null && !this.severity.isEmpty(); 1012 } 1013 1014 /** 1015 * @param value {@link #severity} (Clinical assessment of the severity of the reaction event as a whole, potentially considering multiple different manifestations.). This is the underlying object with id, value and extensions. The accessor "getSeverity" gives direct access to the value 1016 */ 1017 public AllergyIntoleranceReactionComponent setSeverityElement(Enumeration<AllergyIntoleranceSeverity> value) { 1018 this.severity = value; 1019 return this; 1020 } 1021 1022 /** 1023 * @return Clinical assessment of the severity of the reaction event as a whole, potentially considering multiple different manifestations. 1024 */ 1025 public AllergyIntoleranceSeverity getSeverity() { 1026 return this.severity == null ? null : this.severity.getValue(); 1027 } 1028 1029 /** 1030 * @param value Clinical assessment of the severity of the reaction event as a whole, potentially considering multiple different manifestations. 1031 */ 1032 public AllergyIntoleranceReactionComponent setSeverity(AllergyIntoleranceSeverity value) { 1033 if (value == null) 1034 this.severity = null; 1035 else { 1036 if (this.severity == null) 1037 this.severity = new Enumeration<AllergyIntoleranceSeverity>(new AllergyIntoleranceSeverityEnumFactory()); 1038 this.severity.setValue(value); 1039 } 1040 return this; 1041 } 1042 1043 /** 1044 * @return {@link #exposureRoute} (Identification of the route by which the subject was exposed to the substance.) 1045 */ 1046 public CodeableConcept getExposureRoute() { 1047 if (this.exposureRoute == null) 1048 if (Configuration.errorOnAutoCreate()) 1049 throw new Error("Attempt to auto-create AllergyIntoleranceReactionComponent.exposureRoute"); 1050 else if (Configuration.doAutoCreate()) 1051 this.exposureRoute = new CodeableConcept(); // cc 1052 return this.exposureRoute; 1053 } 1054 1055 public boolean hasExposureRoute() { 1056 return this.exposureRoute != null && !this.exposureRoute.isEmpty(); 1057 } 1058 1059 /** 1060 * @param value {@link #exposureRoute} (Identification of the route by which the subject was exposed to the substance.) 1061 */ 1062 public AllergyIntoleranceReactionComponent setExposureRoute(CodeableConcept value) { 1063 this.exposureRoute = value; 1064 return this; 1065 } 1066 1067 /** 1068 * @return {@link #note} (Additional text about the adverse reaction event not captured in other fields.) 1069 */ 1070 public List<Annotation> getNote() { 1071 if (this.note == null) 1072 this.note = new ArrayList<Annotation>(); 1073 return this.note; 1074 } 1075 1076 /** 1077 * @return Returns a reference to <code>this</code> for easy method chaining 1078 */ 1079 public AllergyIntoleranceReactionComponent setNote(List<Annotation> theNote) { 1080 this.note = theNote; 1081 return this; 1082 } 1083 1084 public boolean hasNote() { 1085 if (this.note == null) 1086 return false; 1087 for (Annotation item : this.note) 1088 if (!item.isEmpty()) 1089 return true; 1090 return false; 1091 } 1092 1093 public Annotation addNote() { //3 1094 Annotation t = new Annotation(); 1095 if (this.note == null) 1096 this.note = new ArrayList<Annotation>(); 1097 this.note.add(t); 1098 return t; 1099 } 1100 1101 public AllergyIntoleranceReactionComponent addNote(Annotation t) { //3 1102 if (t == null) 1103 return this; 1104 if (this.note == null) 1105 this.note = new ArrayList<Annotation>(); 1106 this.note.add(t); 1107 return this; 1108 } 1109 1110 /** 1111 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 1112 */ 1113 public Annotation getNoteFirstRep() { 1114 if (getNote().isEmpty()) { 1115 addNote(); 1116 } 1117 return getNote().get(0); 1118 } 1119 1120 protected void listChildren(List<Property> children) { 1121 super.listChildren(children); 1122 children.add(new Property("substance", "CodeableConcept", "Identification of the specific substance (or pharmaceutical product) considered to be responsible for the Adverse Reaction event. Note: the substance for a specific reaction may be different from the substance identified as the cause of the risk, but it must be consistent with it. For instance, it may be a more specific substance (e.g. a brand medication) or a composite product that includes the identified substance. It must be clinically safe to only process the 'code' and ignore the 'reaction.substance'.", 0, 1, substance)); 1123 children.add(new Property("manifestation", "CodeableConcept", "Clinical symptoms and/or signs that are observed or associated with the adverse reaction event.", 0, java.lang.Integer.MAX_VALUE, manifestation)); 1124 children.add(new Property("description", "string", "Text description about the reaction as a whole, including details of the manifestation if required.", 0, 1, description)); 1125 children.add(new Property("onset", "dateTime", "Record of the date and/or time of the onset of the Reaction.", 0, 1, onset)); 1126 children.add(new Property("severity", "code", "Clinical assessment of the severity of the reaction event as a whole, potentially considering multiple different manifestations.", 0, 1, severity)); 1127 children.add(new Property("exposureRoute", "CodeableConcept", "Identification of the route by which the subject was exposed to the substance.", 0, 1, exposureRoute)); 1128 children.add(new Property("note", "Annotation", "Additional text about the adverse reaction event not captured in other fields.", 0, java.lang.Integer.MAX_VALUE, note)); 1129 } 1130 1131 @Override 1132 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1133 switch (_hash) { 1134 case 530040176: /*substance*/ return new Property("substance", "CodeableConcept", "Identification of the specific substance (or pharmaceutical product) considered to be responsible for the Adverse Reaction event. Note: the substance for a specific reaction may be different from the substance identified as the cause of the risk, but it must be consistent with it. For instance, it may be a more specific substance (e.g. a brand medication) or a composite product that includes the identified substance. It must be clinically safe to only process the 'code' and ignore the 'reaction.substance'.", 0, 1, substance); 1135 case 1115984422: /*manifestation*/ return new Property("manifestation", "CodeableConcept", "Clinical symptoms and/or signs that are observed or associated with the adverse reaction event.", 0, java.lang.Integer.MAX_VALUE, manifestation); 1136 case -1724546052: /*description*/ return new Property("description", "string", "Text description about the reaction as a whole, including details of the manifestation if required.", 0, 1, description); 1137 case 105901603: /*onset*/ return new Property("onset", "dateTime", "Record of the date and/or time of the onset of the Reaction.", 0, 1, onset); 1138 case 1478300413: /*severity*/ return new Property("severity", "code", "Clinical assessment of the severity of the reaction event as a whole, potentially considering multiple different manifestations.", 0, 1, severity); 1139 case 421286274: /*exposureRoute*/ return new Property("exposureRoute", "CodeableConcept", "Identification of the route by which the subject was exposed to the substance.", 0, 1, exposureRoute); 1140 case 3387378: /*note*/ return new Property("note", "Annotation", "Additional text about the adverse reaction event not captured in other fields.", 0, java.lang.Integer.MAX_VALUE, note); 1141 default: return super.getNamedProperty(_hash, _name, _checkValid); 1142 } 1143 1144 } 1145 1146 @Override 1147 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1148 switch (hash) { 1149 case 530040176: /*substance*/ return this.substance == null ? new Base[0] : new Base[] {this.substance}; // CodeableConcept 1150 case 1115984422: /*manifestation*/ return this.manifestation == null ? new Base[0] : this.manifestation.toArray(new Base[this.manifestation.size()]); // CodeableConcept 1151 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 1152 case 105901603: /*onset*/ return this.onset == null ? new Base[0] : new Base[] {this.onset}; // DateTimeType 1153 case 1478300413: /*severity*/ return this.severity == null ? new Base[0] : new Base[] {this.severity}; // Enumeration<AllergyIntoleranceSeverity> 1154 case 421286274: /*exposureRoute*/ return this.exposureRoute == null ? new Base[0] : new Base[] {this.exposureRoute}; // CodeableConcept 1155 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1156 default: return super.getProperty(hash, name, checkValid); 1157 } 1158 1159 } 1160 1161 @Override 1162 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1163 switch (hash) { 1164 case 530040176: // substance 1165 this.substance = castToCodeableConcept(value); // CodeableConcept 1166 return value; 1167 case 1115984422: // manifestation 1168 this.getManifestation().add(castToCodeableConcept(value)); // CodeableConcept 1169 return value; 1170 case -1724546052: // description 1171 this.description = castToString(value); // StringType 1172 return value; 1173 case 105901603: // onset 1174 this.onset = castToDateTime(value); // DateTimeType 1175 return value; 1176 case 1478300413: // severity 1177 value = new AllergyIntoleranceSeverityEnumFactory().fromType(castToCode(value)); 1178 this.severity = (Enumeration) value; // Enumeration<AllergyIntoleranceSeverity> 1179 return value; 1180 case 421286274: // exposureRoute 1181 this.exposureRoute = castToCodeableConcept(value); // CodeableConcept 1182 return value; 1183 case 3387378: // note 1184 this.getNote().add(castToAnnotation(value)); // Annotation 1185 return value; 1186 default: return super.setProperty(hash, name, value); 1187 } 1188 1189 } 1190 1191 @Override 1192 public Base setProperty(String name, Base value) throws FHIRException { 1193 if (name.equals("substance")) { 1194 this.substance = castToCodeableConcept(value); // CodeableConcept 1195 } else if (name.equals("manifestation")) { 1196 this.getManifestation().add(castToCodeableConcept(value)); 1197 } else if (name.equals("description")) { 1198 this.description = castToString(value); // StringType 1199 } else if (name.equals("onset")) { 1200 this.onset = castToDateTime(value); // DateTimeType 1201 } else if (name.equals("severity")) { 1202 value = new AllergyIntoleranceSeverityEnumFactory().fromType(castToCode(value)); 1203 this.severity = (Enumeration) value; // Enumeration<AllergyIntoleranceSeverity> 1204 } else if (name.equals("exposureRoute")) { 1205 this.exposureRoute = castToCodeableConcept(value); // CodeableConcept 1206 } else if (name.equals("note")) { 1207 this.getNote().add(castToAnnotation(value)); 1208 } else 1209 return super.setProperty(name, value); 1210 return value; 1211 } 1212 1213 @Override 1214 public Base makeProperty(int hash, String name) throws FHIRException { 1215 switch (hash) { 1216 case 530040176: return getSubstance(); 1217 case 1115984422: return addManifestation(); 1218 case -1724546052: return getDescriptionElement(); 1219 case 105901603: return getOnsetElement(); 1220 case 1478300413: return getSeverityElement(); 1221 case 421286274: return getExposureRoute(); 1222 case 3387378: return addNote(); 1223 default: return super.makeProperty(hash, name); 1224 } 1225 1226 } 1227 1228 @Override 1229 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1230 switch (hash) { 1231 case 530040176: /*substance*/ return new String[] {"CodeableConcept"}; 1232 case 1115984422: /*manifestation*/ return new String[] {"CodeableConcept"}; 1233 case -1724546052: /*description*/ return new String[] {"string"}; 1234 case 105901603: /*onset*/ return new String[] {"dateTime"}; 1235 case 1478300413: /*severity*/ return new String[] {"code"}; 1236 case 421286274: /*exposureRoute*/ return new String[] {"CodeableConcept"}; 1237 case 3387378: /*note*/ return new String[] {"Annotation"}; 1238 default: return super.getTypesForProperty(hash, name); 1239 } 1240 1241 } 1242 1243 @Override 1244 public Base addChild(String name) throws FHIRException { 1245 if (name.equals("substance")) { 1246 this.substance = new CodeableConcept(); 1247 return this.substance; 1248 } 1249 else if (name.equals("manifestation")) { 1250 return addManifestation(); 1251 } 1252 else if (name.equals("description")) { 1253 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.description"); 1254 } 1255 else if (name.equals("onset")) { 1256 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.onset"); 1257 } 1258 else if (name.equals("severity")) { 1259 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.severity"); 1260 } 1261 else if (name.equals("exposureRoute")) { 1262 this.exposureRoute = new CodeableConcept(); 1263 return this.exposureRoute; 1264 } 1265 else if (name.equals("note")) { 1266 return addNote(); 1267 } 1268 else 1269 return super.addChild(name); 1270 } 1271 1272 public AllergyIntoleranceReactionComponent copy() { 1273 AllergyIntoleranceReactionComponent dst = new AllergyIntoleranceReactionComponent(); 1274 copyValues(dst); 1275 dst.substance = substance == null ? null : substance.copy(); 1276 if (manifestation != null) { 1277 dst.manifestation = new ArrayList<CodeableConcept>(); 1278 for (CodeableConcept i : manifestation) 1279 dst.manifestation.add(i.copy()); 1280 }; 1281 dst.description = description == null ? null : description.copy(); 1282 dst.onset = onset == null ? null : onset.copy(); 1283 dst.severity = severity == null ? null : severity.copy(); 1284 dst.exposureRoute = exposureRoute == null ? null : exposureRoute.copy(); 1285 if (note != null) { 1286 dst.note = new ArrayList<Annotation>(); 1287 for (Annotation i : note) 1288 dst.note.add(i.copy()); 1289 }; 1290 return dst; 1291 } 1292 1293 @Override 1294 public boolean equalsDeep(Base other_) { 1295 if (!super.equalsDeep(other_)) 1296 return false; 1297 if (!(other_ instanceof AllergyIntoleranceReactionComponent)) 1298 return false; 1299 AllergyIntoleranceReactionComponent o = (AllergyIntoleranceReactionComponent) other_; 1300 return compareDeep(substance, o.substance, true) && compareDeep(manifestation, o.manifestation, true) 1301 && compareDeep(description, o.description, true) && compareDeep(onset, o.onset, true) && compareDeep(severity, o.severity, true) 1302 && compareDeep(exposureRoute, o.exposureRoute, true) && compareDeep(note, o.note, true); 1303 } 1304 1305 @Override 1306 public boolean equalsShallow(Base other_) { 1307 if (!super.equalsShallow(other_)) 1308 return false; 1309 if (!(other_ instanceof AllergyIntoleranceReactionComponent)) 1310 return false; 1311 AllergyIntoleranceReactionComponent o = (AllergyIntoleranceReactionComponent) other_; 1312 return compareValues(description, o.description, true) && compareValues(onset, o.onset, true) && compareValues(severity, o.severity, true) 1313 ; 1314 } 1315 1316 public boolean isEmpty() { 1317 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(substance, manifestation, description 1318 , onset, severity, exposureRoute, note); 1319 } 1320 1321 public String fhirType() { 1322 return "AllergyIntolerance.reaction"; 1323 1324 } 1325 1326 } 1327 1328 /** 1329 * This records identifiers associated with this allergy/intolerance concern that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation). 1330 */ 1331 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1332 @Description(shortDefinition="External ids for this item", formalDefinition="This records identifiers associated with this allergy/intolerance concern that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation)." ) 1333 protected List<Identifier> identifier; 1334 1335 /** 1336 * The clinical status of the allergy or intolerance. 1337 */ 1338 @Child(name = "clinicalStatus", type = {CodeType.class}, order=1, min=0, max=1, modifier=true, summary=true) 1339 @Description(shortDefinition="active | inactive | resolved", formalDefinition="The clinical status of the allergy or intolerance." ) 1340 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/allergy-clinical-status") 1341 protected Enumeration<AllergyIntoleranceClinicalStatus> clinicalStatus; 1342 1343 /** 1344 * Assertion about certainty associated with the propensity, or potential risk, of a reaction to the identified substance (including pharmaceutical product). 1345 */ 1346 @Child(name = "verificationStatus", type = {CodeType.class}, order=2, min=1, max=1, modifier=true, summary=true) 1347 @Description(shortDefinition="unconfirmed | confirmed | refuted | entered-in-error", formalDefinition="Assertion about certainty associated with the propensity, or potential risk, of a reaction to the identified substance (including pharmaceutical product)." ) 1348 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/allergy-verification-status") 1349 protected Enumeration<AllergyIntoleranceVerificationStatus> verificationStatus; 1350 1351 /** 1352 * Identification of the underlying physiological mechanism for the reaction risk. 1353 */ 1354 @Child(name = "type", type = {CodeType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1355 @Description(shortDefinition="allergy | intolerance - Underlying mechanism (if known)", formalDefinition="Identification of the underlying physiological mechanism for the reaction risk." ) 1356 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/allergy-intolerance-type") 1357 protected Enumeration<AllergyIntoleranceType> type; 1358 1359 /** 1360 * Category of the identified substance. 1361 */ 1362 @Child(name = "category", type = {CodeType.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1363 @Description(shortDefinition="food | medication | environment | biologic", formalDefinition="Category of the identified substance." ) 1364 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/allergy-intolerance-category") 1365 protected List<Enumeration<AllergyIntoleranceCategory>> category; 1366 1367 /** 1368 * Estimate of the potential clinical harm, or seriousness, of the reaction to the identified substance. 1369 */ 1370 @Child(name = "criticality", type = {CodeType.class}, order=5, min=0, max=1, modifier=false, summary=true) 1371 @Description(shortDefinition="low | high | unable-to-assess", formalDefinition="Estimate of the potential clinical harm, or seriousness, of the reaction to the identified substance." ) 1372 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/allergy-intolerance-criticality") 1373 protected Enumeration<AllergyIntoleranceCriticality> criticality; 1374 1375 /** 1376 * Code for an allergy or intolerance statement (either a positive or a negated/excluded statement). This may be a code for a substance or pharmaceutical product that is considered to be responsible for the adverse reaction risk (e.g., "Latex"), an allergy or intolerance condition (e.g., "Latex allergy"), or a negated/excluded code for a specific substance or class (e.g., "No latex allergy") or a general or categorical negated statement (e.g., "No known allergy", "No known drug allergies"). 1377 */ 1378 @Child(name = "code", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=true) 1379 @Description(shortDefinition="Code that identifies the allergy or intolerance", formalDefinition="Code for an allergy or intolerance statement (either a positive or a negated/excluded statement). This may be a code for a substance or pharmaceutical product that is considered to be responsible for the adverse reaction risk (e.g., \"Latex\"), an allergy or intolerance condition (e.g., \"Latex allergy\"), or a negated/excluded code for a specific substance or class (e.g., \"No latex allergy\") or a general or categorical negated statement (e.g., \"No known allergy\", \"No known drug allergies\")." ) 1380 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/allergyintolerance-code") 1381 protected CodeableConcept code; 1382 1383 /** 1384 * The patient who has the allergy or intolerance. 1385 */ 1386 @Child(name = "patient", type = {Patient.class}, order=7, min=1, max=1, modifier=false, summary=true) 1387 @Description(shortDefinition="Who the sensitivity is for", formalDefinition="The patient who has the allergy or intolerance." ) 1388 protected Reference patient; 1389 1390 /** 1391 * The actual object that is the target of the reference (The patient who has the allergy or intolerance.) 1392 */ 1393 protected Patient patientTarget; 1394 1395 /** 1396 * Estimated or actual date, date-time, or age when allergy or intolerance was identified. 1397 */ 1398 @Child(name = "onset", type = {DateTimeType.class, Age.class, Period.class, Range.class, StringType.class}, order=8, min=0, max=1, modifier=false, summary=false) 1399 @Description(shortDefinition="When allergy or intolerance was identified", formalDefinition="Estimated or actual date, date-time, or age when allergy or intolerance was identified." ) 1400 protected Type onset; 1401 1402 /** 1403 * The date on which the existance of the AllergyIntolerance was first asserted or acknowledged. 1404 */ 1405 @Child(name = "assertedDate", type = {DateTimeType.class}, order=9, min=0, max=1, modifier=false, summary=false) 1406 @Description(shortDefinition="Date record was believed accurate", formalDefinition="The date on which the existance of the AllergyIntolerance was first asserted or acknowledged." ) 1407 protected DateTimeType assertedDate; 1408 1409 /** 1410 * Individual who recorded the record and takes responsibility for its content. 1411 */ 1412 @Child(name = "recorder", type = {Practitioner.class, Patient.class}, order=10, min=0, max=1, modifier=false, summary=false) 1413 @Description(shortDefinition="Who recorded the sensitivity", formalDefinition="Individual who recorded the record and takes responsibility for its content." ) 1414 protected Reference recorder; 1415 1416 /** 1417 * The actual object that is the target of the reference (Individual who recorded the record and takes responsibility for its content.) 1418 */ 1419 protected Resource recorderTarget; 1420 1421 /** 1422 * The source of the information about the allergy that is recorded. 1423 */ 1424 @Child(name = "asserter", type = {Patient.class, RelatedPerson.class, Practitioner.class}, order=11, min=0, max=1, modifier=false, summary=true) 1425 @Description(shortDefinition="Source of the information about the allergy", formalDefinition="The source of the information about the allergy that is recorded." ) 1426 protected Reference asserter; 1427 1428 /** 1429 * The actual object that is the target of the reference (The source of the information about the allergy that is recorded.) 1430 */ 1431 protected Resource asserterTarget; 1432 1433 /** 1434 * Represents the date and/or time of the last known occurrence of a reaction event. 1435 */ 1436 @Child(name = "lastOccurrence", type = {DateTimeType.class}, order=12, min=0, max=1, modifier=false, summary=false) 1437 @Description(shortDefinition="Date(/time) of last known occurrence of a reaction", formalDefinition="Represents the date and/or time of the last known occurrence of a reaction event." ) 1438 protected DateTimeType lastOccurrence; 1439 1440 /** 1441 * Additional narrative about the propensity for the Adverse Reaction, not captured in other fields. 1442 */ 1443 @Child(name = "note", type = {Annotation.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1444 @Description(shortDefinition="Additional text not captured in other fields", formalDefinition="Additional narrative about the propensity for the Adverse Reaction, not captured in other fields." ) 1445 protected List<Annotation> note; 1446 1447 /** 1448 * Details about each adverse reaction event linked to exposure to the identified substance. 1449 */ 1450 @Child(name = "reaction", type = {}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1451 @Description(shortDefinition="Adverse Reaction Events linked to exposure to substance", formalDefinition="Details about each adverse reaction event linked to exposure to the identified substance." ) 1452 protected List<AllergyIntoleranceReactionComponent> reaction; 1453 1454 private static final long serialVersionUID = 948924623L; 1455 1456 /** 1457 * Constructor 1458 */ 1459 public AllergyIntolerance() { 1460 super(); 1461 } 1462 1463 /** 1464 * Constructor 1465 */ 1466 public AllergyIntolerance(Enumeration<AllergyIntoleranceVerificationStatus> verificationStatus, Reference patient) { 1467 super(); 1468 this.verificationStatus = verificationStatus; 1469 this.patient = patient; 1470 } 1471 1472 /** 1473 * @return {@link #identifier} (This records identifiers associated with this allergy/intolerance concern that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).) 1474 */ 1475 public List<Identifier> getIdentifier() { 1476 if (this.identifier == null) 1477 this.identifier = new ArrayList<Identifier>(); 1478 return this.identifier; 1479 } 1480 1481 /** 1482 * @return Returns a reference to <code>this</code> for easy method chaining 1483 */ 1484 public AllergyIntolerance setIdentifier(List<Identifier> theIdentifier) { 1485 this.identifier = theIdentifier; 1486 return this; 1487 } 1488 1489 public boolean hasIdentifier() { 1490 if (this.identifier == null) 1491 return false; 1492 for (Identifier item : this.identifier) 1493 if (!item.isEmpty()) 1494 return true; 1495 return false; 1496 } 1497 1498 public Identifier addIdentifier() { //3 1499 Identifier t = new Identifier(); 1500 if (this.identifier == null) 1501 this.identifier = new ArrayList<Identifier>(); 1502 this.identifier.add(t); 1503 return t; 1504 } 1505 1506 public AllergyIntolerance addIdentifier(Identifier t) { //3 1507 if (t == null) 1508 return this; 1509 if (this.identifier == null) 1510 this.identifier = new ArrayList<Identifier>(); 1511 this.identifier.add(t); 1512 return this; 1513 } 1514 1515 /** 1516 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 1517 */ 1518 public Identifier getIdentifierFirstRep() { 1519 if (getIdentifier().isEmpty()) { 1520 addIdentifier(); 1521 } 1522 return getIdentifier().get(0); 1523 } 1524 1525 /** 1526 * @return {@link #clinicalStatus} (The clinical status of the allergy or intolerance.). This is the underlying object with id, value and extensions. The accessor "getClinicalStatus" gives direct access to the value 1527 */ 1528 public Enumeration<AllergyIntoleranceClinicalStatus> getClinicalStatusElement() { 1529 if (this.clinicalStatus == null) 1530 if (Configuration.errorOnAutoCreate()) 1531 throw new Error("Attempt to auto-create AllergyIntolerance.clinicalStatus"); 1532 else if (Configuration.doAutoCreate()) 1533 this.clinicalStatus = new Enumeration<AllergyIntoleranceClinicalStatus>(new AllergyIntoleranceClinicalStatusEnumFactory()); // bb 1534 return this.clinicalStatus; 1535 } 1536 1537 public boolean hasClinicalStatusElement() { 1538 return this.clinicalStatus != null && !this.clinicalStatus.isEmpty(); 1539 } 1540 1541 public boolean hasClinicalStatus() { 1542 return this.clinicalStatus != null && !this.clinicalStatus.isEmpty(); 1543 } 1544 1545 /** 1546 * @param value {@link #clinicalStatus} (The clinical status of the allergy or intolerance.). This is the underlying object with id, value and extensions. The accessor "getClinicalStatus" gives direct access to the value 1547 */ 1548 public AllergyIntolerance setClinicalStatusElement(Enumeration<AllergyIntoleranceClinicalStatus> value) { 1549 this.clinicalStatus = value; 1550 return this; 1551 } 1552 1553 /** 1554 * @return The clinical status of the allergy or intolerance. 1555 */ 1556 public AllergyIntoleranceClinicalStatus getClinicalStatus() { 1557 return this.clinicalStatus == null ? null : this.clinicalStatus.getValue(); 1558 } 1559 1560 /** 1561 * @param value The clinical status of the allergy or intolerance. 1562 */ 1563 public AllergyIntolerance setClinicalStatus(AllergyIntoleranceClinicalStatus value) { 1564 if (value == null) 1565 this.clinicalStatus = null; 1566 else { 1567 if (this.clinicalStatus == null) 1568 this.clinicalStatus = new Enumeration<AllergyIntoleranceClinicalStatus>(new AllergyIntoleranceClinicalStatusEnumFactory()); 1569 this.clinicalStatus.setValue(value); 1570 } 1571 return this; 1572 } 1573 1574 /** 1575 * @return {@link #verificationStatus} (Assertion about certainty associated with the propensity, or potential risk, of a reaction to the identified substance (including pharmaceutical product).). This is the underlying object with id, value and extensions. The accessor "getVerificationStatus" gives direct access to the value 1576 */ 1577 public Enumeration<AllergyIntoleranceVerificationStatus> getVerificationStatusElement() { 1578 if (this.verificationStatus == null) 1579 if (Configuration.errorOnAutoCreate()) 1580 throw new Error("Attempt to auto-create AllergyIntolerance.verificationStatus"); 1581 else if (Configuration.doAutoCreate()) 1582 this.verificationStatus = new Enumeration<AllergyIntoleranceVerificationStatus>(new AllergyIntoleranceVerificationStatusEnumFactory()); // bb 1583 return this.verificationStatus; 1584 } 1585 1586 public boolean hasVerificationStatusElement() { 1587 return this.verificationStatus != null && !this.verificationStatus.isEmpty(); 1588 } 1589 1590 public boolean hasVerificationStatus() { 1591 return this.verificationStatus != null && !this.verificationStatus.isEmpty(); 1592 } 1593 1594 /** 1595 * @param value {@link #verificationStatus} (Assertion about certainty associated with the propensity, or potential risk, of a reaction to the identified substance (including pharmaceutical product).). This is the underlying object with id, value and extensions. The accessor "getVerificationStatus" gives direct access to the value 1596 */ 1597 public AllergyIntolerance setVerificationStatusElement(Enumeration<AllergyIntoleranceVerificationStatus> value) { 1598 this.verificationStatus = value; 1599 return this; 1600 } 1601 1602 /** 1603 * @return Assertion about certainty associated with the propensity, or potential risk, of a reaction to the identified substance (including pharmaceutical product). 1604 */ 1605 public AllergyIntoleranceVerificationStatus getVerificationStatus() { 1606 return this.verificationStatus == null ? null : this.verificationStatus.getValue(); 1607 } 1608 1609 /** 1610 * @param value Assertion about certainty associated with the propensity, or potential risk, of a reaction to the identified substance (including pharmaceutical product). 1611 */ 1612 public AllergyIntolerance setVerificationStatus(AllergyIntoleranceVerificationStatus value) { 1613 if (this.verificationStatus == null) 1614 this.verificationStatus = new Enumeration<AllergyIntoleranceVerificationStatus>(new AllergyIntoleranceVerificationStatusEnumFactory()); 1615 this.verificationStatus.setValue(value); 1616 return this; 1617 } 1618 1619 /** 1620 * @return {@link #type} (Identification of the underlying physiological mechanism for the reaction risk.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1621 */ 1622 public Enumeration<AllergyIntoleranceType> getTypeElement() { 1623 if (this.type == null) 1624 if (Configuration.errorOnAutoCreate()) 1625 throw new Error("Attempt to auto-create AllergyIntolerance.type"); 1626 else if (Configuration.doAutoCreate()) 1627 this.type = new Enumeration<AllergyIntoleranceType>(new AllergyIntoleranceTypeEnumFactory()); // bb 1628 return this.type; 1629 } 1630 1631 public boolean hasTypeElement() { 1632 return this.type != null && !this.type.isEmpty(); 1633 } 1634 1635 public boolean hasType() { 1636 return this.type != null && !this.type.isEmpty(); 1637 } 1638 1639 /** 1640 * @param value {@link #type} (Identification of the underlying physiological mechanism for the reaction risk.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1641 */ 1642 public AllergyIntolerance setTypeElement(Enumeration<AllergyIntoleranceType> value) { 1643 this.type = value; 1644 return this; 1645 } 1646 1647 /** 1648 * @return Identification of the underlying physiological mechanism for the reaction risk. 1649 */ 1650 public AllergyIntoleranceType getType() { 1651 return this.type == null ? null : this.type.getValue(); 1652 } 1653 1654 /** 1655 * @param value Identification of the underlying physiological mechanism for the reaction risk. 1656 */ 1657 public AllergyIntolerance setType(AllergyIntoleranceType value) { 1658 if (value == null) 1659 this.type = null; 1660 else { 1661 if (this.type == null) 1662 this.type = new Enumeration<AllergyIntoleranceType>(new AllergyIntoleranceTypeEnumFactory()); 1663 this.type.setValue(value); 1664 } 1665 return this; 1666 } 1667 1668 /** 1669 * @return {@link #category} (Category of the identified substance.) 1670 */ 1671 public List<Enumeration<AllergyIntoleranceCategory>> getCategory() { 1672 if (this.category == null) 1673 this.category = new ArrayList<Enumeration<AllergyIntoleranceCategory>>(); 1674 return this.category; 1675 } 1676 1677 /** 1678 * @return Returns a reference to <code>this</code> for easy method chaining 1679 */ 1680 public AllergyIntolerance setCategory(List<Enumeration<AllergyIntoleranceCategory>> theCategory) { 1681 this.category = theCategory; 1682 return this; 1683 } 1684 1685 public boolean hasCategory() { 1686 if (this.category == null) 1687 return false; 1688 for (Enumeration<AllergyIntoleranceCategory> item : this.category) 1689 if (!item.isEmpty()) 1690 return true; 1691 return false; 1692 } 1693 1694 /** 1695 * @return {@link #category} (Category of the identified substance.) 1696 */ 1697 public Enumeration<AllergyIntoleranceCategory> addCategoryElement() {//2 1698 Enumeration<AllergyIntoleranceCategory> t = new Enumeration<AllergyIntoleranceCategory>(new AllergyIntoleranceCategoryEnumFactory()); 1699 if (this.category == null) 1700 this.category = new ArrayList<Enumeration<AllergyIntoleranceCategory>>(); 1701 this.category.add(t); 1702 return t; 1703 } 1704 1705 /** 1706 * @param value {@link #category} (Category of the identified substance.) 1707 */ 1708 public AllergyIntolerance addCategory(AllergyIntoleranceCategory value) { //1 1709 Enumeration<AllergyIntoleranceCategory> t = new Enumeration<AllergyIntoleranceCategory>(new AllergyIntoleranceCategoryEnumFactory()); 1710 t.setValue(value); 1711 if (this.category == null) 1712 this.category = new ArrayList<Enumeration<AllergyIntoleranceCategory>>(); 1713 this.category.add(t); 1714 return this; 1715 } 1716 1717 /** 1718 * @param value {@link #category} (Category of the identified substance.) 1719 */ 1720 public boolean hasCategory(AllergyIntoleranceCategory value) { 1721 if (this.category == null) 1722 return false; 1723 for (Enumeration<AllergyIntoleranceCategory> v : this.category) 1724 if (v.getValue().equals(value)) // code 1725 return true; 1726 return false; 1727 } 1728 1729 /** 1730 * @return {@link #criticality} (Estimate of the potential clinical harm, or seriousness, of the reaction to the identified substance.). This is the underlying object with id, value and extensions. The accessor "getCriticality" gives direct access to the value 1731 */ 1732 public Enumeration<AllergyIntoleranceCriticality> getCriticalityElement() { 1733 if (this.criticality == null) 1734 if (Configuration.errorOnAutoCreate()) 1735 throw new Error("Attempt to auto-create AllergyIntolerance.criticality"); 1736 else if (Configuration.doAutoCreate()) 1737 this.criticality = new Enumeration<AllergyIntoleranceCriticality>(new AllergyIntoleranceCriticalityEnumFactory()); // bb 1738 return this.criticality; 1739 } 1740 1741 public boolean hasCriticalityElement() { 1742 return this.criticality != null && !this.criticality.isEmpty(); 1743 } 1744 1745 public boolean hasCriticality() { 1746 return this.criticality != null && !this.criticality.isEmpty(); 1747 } 1748 1749 /** 1750 * @param value {@link #criticality} (Estimate of the potential clinical harm, or seriousness, of the reaction to the identified substance.). This is the underlying object with id, value and extensions. The accessor "getCriticality" gives direct access to the value 1751 */ 1752 public AllergyIntolerance setCriticalityElement(Enumeration<AllergyIntoleranceCriticality> value) { 1753 this.criticality = value; 1754 return this; 1755 } 1756 1757 /** 1758 * @return Estimate of the potential clinical harm, or seriousness, of the reaction to the identified substance. 1759 */ 1760 public AllergyIntoleranceCriticality getCriticality() { 1761 return this.criticality == null ? null : this.criticality.getValue(); 1762 } 1763 1764 /** 1765 * @param value Estimate of the potential clinical harm, or seriousness, of the reaction to the identified substance. 1766 */ 1767 public AllergyIntolerance setCriticality(AllergyIntoleranceCriticality value) { 1768 if (value == null) 1769 this.criticality = null; 1770 else { 1771 if (this.criticality == null) 1772 this.criticality = new Enumeration<AllergyIntoleranceCriticality>(new AllergyIntoleranceCriticalityEnumFactory()); 1773 this.criticality.setValue(value); 1774 } 1775 return this; 1776 } 1777 1778 /** 1779 * @return {@link #code} (Code for an allergy or intolerance statement (either a positive or a negated/excluded statement). This may be a code for a substance or pharmaceutical product that is considered to be responsible for the adverse reaction risk (e.g., "Latex"), an allergy or intolerance condition (e.g., "Latex allergy"), or a negated/excluded code for a specific substance or class (e.g., "No latex allergy") or a general or categorical negated statement (e.g., "No known allergy", "No known drug allergies").) 1780 */ 1781 public CodeableConcept getCode() { 1782 if (this.code == null) 1783 if (Configuration.errorOnAutoCreate()) 1784 throw new Error("Attempt to auto-create AllergyIntolerance.code"); 1785 else if (Configuration.doAutoCreate()) 1786 this.code = new CodeableConcept(); // cc 1787 return this.code; 1788 } 1789 1790 public boolean hasCode() { 1791 return this.code != null && !this.code.isEmpty(); 1792 } 1793 1794 /** 1795 * @param value {@link #code} (Code for an allergy or intolerance statement (either a positive or a negated/excluded statement). This may be a code for a substance or pharmaceutical product that is considered to be responsible for the adverse reaction risk (e.g., "Latex"), an allergy or intolerance condition (e.g., "Latex allergy"), or a negated/excluded code for a specific substance or class (e.g., "No latex allergy") or a general or categorical negated statement (e.g., "No known allergy", "No known drug allergies").) 1796 */ 1797 public AllergyIntolerance setCode(CodeableConcept value) { 1798 this.code = value; 1799 return this; 1800 } 1801 1802 /** 1803 * @return {@link #patient} (The patient who has the allergy or intolerance.) 1804 */ 1805 public Reference getPatient() { 1806 if (this.patient == null) 1807 if (Configuration.errorOnAutoCreate()) 1808 throw new Error("Attempt to auto-create AllergyIntolerance.patient"); 1809 else if (Configuration.doAutoCreate()) 1810 this.patient = new Reference(); // cc 1811 return this.patient; 1812 } 1813 1814 public boolean hasPatient() { 1815 return this.patient != null && !this.patient.isEmpty(); 1816 } 1817 1818 /** 1819 * @param value {@link #patient} (The patient who has the allergy or intolerance.) 1820 */ 1821 public AllergyIntolerance setPatient(Reference value) { 1822 this.patient = value; 1823 return this; 1824 } 1825 1826 /** 1827 * @return {@link #patient} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The patient who has the allergy or intolerance.) 1828 */ 1829 public Patient getPatientTarget() { 1830 if (this.patientTarget == null) 1831 if (Configuration.errorOnAutoCreate()) 1832 throw new Error("Attempt to auto-create AllergyIntolerance.patient"); 1833 else if (Configuration.doAutoCreate()) 1834 this.patientTarget = new Patient(); // aa 1835 return this.patientTarget; 1836 } 1837 1838 /** 1839 * @param value {@link #patient} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The patient who has the allergy or intolerance.) 1840 */ 1841 public AllergyIntolerance setPatientTarget(Patient value) { 1842 this.patientTarget = value; 1843 return this; 1844 } 1845 1846 /** 1847 * @return {@link #onset} (Estimated or actual date, date-time, or age when allergy or intolerance was identified.) 1848 */ 1849 public Type getOnset() { 1850 return this.onset; 1851 } 1852 1853 /** 1854 * @return {@link #onset} (Estimated or actual date, date-time, or age when allergy or intolerance was identified.) 1855 */ 1856 public DateTimeType getOnsetDateTimeType() throws FHIRException { 1857 if (this.onset == null) 1858 return null; 1859 if (!(this.onset instanceof DateTimeType)) 1860 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.onset.getClass().getName()+" was encountered"); 1861 return (DateTimeType) this.onset; 1862 } 1863 1864 public boolean hasOnsetDateTimeType() { 1865 return this != null && this.onset instanceof DateTimeType; 1866 } 1867 1868 /** 1869 * @return {@link #onset} (Estimated or actual date, date-time, or age when allergy or intolerance was identified.) 1870 */ 1871 public Age getOnsetAge() throws FHIRException { 1872 if (this.onset == null) 1873 return null; 1874 if (!(this.onset instanceof Age)) 1875 throw new FHIRException("Type mismatch: the type Age was expected, but "+this.onset.getClass().getName()+" was encountered"); 1876 return (Age) this.onset; 1877 } 1878 1879 public boolean hasOnsetAge() { 1880 return this != null && this.onset instanceof Age; 1881 } 1882 1883 /** 1884 * @return {@link #onset} (Estimated or actual date, date-time, or age when allergy or intolerance was identified.) 1885 */ 1886 public Period getOnsetPeriod() throws FHIRException { 1887 if (this.onset == null) 1888 return null; 1889 if (!(this.onset instanceof Period)) 1890 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.onset.getClass().getName()+" was encountered"); 1891 return (Period) this.onset; 1892 } 1893 1894 public boolean hasOnsetPeriod() { 1895 return this != null && this.onset instanceof Period; 1896 } 1897 1898 /** 1899 * @return {@link #onset} (Estimated or actual date, date-time, or age when allergy or intolerance was identified.) 1900 */ 1901 public Range getOnsetRange() throws FHIRException { 1902 if (this.onset == null) 1903 return null; 1904 if (!(this.onset instanceof Range)) 1905 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.onset.getClass().getName()+" was encountered"); 1906 return (Range) this.onset; 1907 } 1908 1909 public boolean hasOnsetRange() { 1910 return this != null && this.onset instanceof Range; 1911 } 1912 1913 /** 1914 * @return {@link #onset} (Estimated or actual date, date-time, or age when allergy or intolerance was identified.) 1915 */ 1916 public StringType getOnsetStringType() throws FHIRException { 1917 if (this.onset == null) 1918 return null; 1919 if (!(this.onset instanceof StringType)) 1920 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.onset.getClass().getName()+" was encountered"); 1921 return (StringType) this.onset; 1922 } 1923 1924 public boolean hasOnsetStringType() { 1925 return this != null && this.onset instanceof StringType; 1926 } 1927 1928 public boolean hasOnset() { 1929 return this.onset != null && !this.onset.isEmpty(); 1930 } 1931 1932 /** 1933 * @param value {@link #onset} (Estimated or actual date, date-time, or age when allergy or intolerance was identified.) 1934 */ 1935 public AllergyIntolerance setOnset(Type value) throws FHIRFormatError { 1936 if (value != null && !(value instanceof DateTimeType || value instanceof Age || value instanceof Period || value instanceof Range || value instanceof StringType)) 1937 throw new FHIRFormatError("Not the right type for AllergyIntolerance.onset[x]: "+value.fhirType()); 1938 this.onset = value; 1939 return this; 1940 } 1941 1942 /** 1943 * @return {@link #assertedDate} (The date on which the existance of the AllergyIntolerance was first asserted or acknowledged.). This is the underlying object with id, value and extensions. The accessor "getAssertedDate" gives direct access to the value 1944 */ 1945 public DateTimeType getAssertedDateElement() { 1946 if (this.assertedDate == null) 1947 if (Configuration.errorOnAutoCreate()) 1948 throw new Error("Attempt to auto-create AllergyIntolerance.assertedDate"); 1949 else if (Configuration.doAutoCreate()) 1950 this.assertedDate = new DateTimeType(); // bb 1951 return this.assertedDate; 1952 } 1953 1954 public boolean hasAssertedDateElement() { 1955 return this.assertedDate != null && !this.assertedDate.isEmpty(); 1956 } 1957 1958 public boolean hasAssertedDate() { 1959 return this.assertedDate != null && !this.assertedDate.isEmpty(); 1960 } 1961 1962 /** 1963 * @param value {@link #assertedDate} (The date on which the existance of the AllergyIntolerance was first asserted or acknowledged.). This is the underlying object with id, value and extensions. The accessor "getAssertedDate" gives direct access to the value 1964 */ 1965 public AllergyIntolerance setAssertedDateElement(DateTimeType value) { 1966 this.assertedDate = value; 1967 return this; 1968 } 1969 1970 /** 1971 * @return The date on which the existance of the AllergyIntolerance was first asserted or acknowledged. 1972 */ 1973 public Date getAssertedDate() { 1974 return this.assertedDate == null ? null : this.assertedDate.getValue(); 1975 } 1976 1977 /** 1978 * @param value The date on which the existance of the AllergyIntolerance was first asserted or acknowledged. 1979 */ 1980 public AllergyIntolerance setAssertedDate(Date value) { 1981 if (value == null) 1982 this.assertedDate = null; 1983 else { 1984 if (this.assertedDate == null) 1985 this.assertedDate = new DateTimeType(); 1986 this.assertedDate.setValue(value); 1987 } 1988 return this; 1989 } 1990 1991 /** 1992 * @return {@link #recorder} (Individual who recorded the record and takes responsibility for its content.) 1993 */ 1994 public Reference getRecorder() { 1995 if (this.recorder == null) 1996 if (Configuration.errorOnAutoCreate()) 1997 throw new Error("Attempt to auto-create AllergyIntolerance.recorder"); 1998 else if (Configuration.doAutoCreate()) 1999 this.recorder = new Reference(); // cc 2000 return this.recorder; 2001 } 2002 2003 public boolean hasRecorder() { 2004 return this.recorder != null && !this.recorder.isEmpty(); 2005 } 2006 2007 /** 2008 * @param value {@link #recorder} (Individual who recorded the record and takes responsibility for its content.) 2009 */ 2010 public AllergyIntolerance setRecorder(Reference value) { 2011 this.recorder = value; 2012 return this; 2013 } 2014 2015 /** 2016 * @return {@link #recorder} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Individual who recorded the record and takes responsibility for its content.) 2017 */ 2018 public Resource getRecorderTarget() { 2019 return this.recorderTarget; 2020 } 2021 2022 /** 2023 * @param value {@link #recorder} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Individual who recorded the record and takes responsibility for its content.) 2024 */ 2025 public AllergyIntolerance setRecorderTarget(Resource value) { 2026 this.recorderTarget = value; 2027 return this; 2028 } 2029 2030 /** 2031 * @return {@link #asserter} (The source of the information about the allergy that is recorded.) 2032 */ 2033 public Reference getAsserter() { 2034 if (this.asserter == null) 2035 if (Configuration.errorOnAutoCreate()) 2036 throw new Error("Attempt to auto-create AllergyIntolerance.asserter"); 2037 else if (Configuration.doAutoCreate()) 2038 this.asserter = new Reference(); // cc 2039 return this.asserter; 2040 } 2041 2042 public boolean hasAsserter() { 2043 return this.asserter != null && !this.asserter.isEmpty(); 2044 } 2045 2046 /** 2047 * @param value {@link #asserter} (The source of the information about the allergy that is recorded.) 2048 */ 2049 public AllergyIntolerance setAsserter(Reference value) { 2050 this.asserter = value; 2051 return this; 2052 } 2053 2054 /** 2055 * @return {@link #asserter} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The source of the information about the allergy that is recorded.) 2056 */ 2057 public Resource getAsserterTarget() { 2058 return this.asserterTarget; 2059 } 2060 2061 /** 2062 * @param value {@link #asserter} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The source of the information about the allergy that is recorded.) 2063 */ 2064 public AllergyIntolerance setAsserterTarget(Resource value) { 2065 this.asserterTarget = value; 2066 return this; 2067 } 2068 2069 /** 2070 * @return {@link #lastOccurrence} (Represents the date and/or time of the last known occurrence of a reaction event.). This is the underlying object with id, value and extensions. The accessor "getLastOccurrence" gives direct access to the value 2071 */ 2072 public DateTimeType getLastOccurrenceElement() { 2073 if (this.lastOccurrence == null) 2074 if (Configuration.errorOnAutoCreate()) 2075 throw new Error("Attempt to auto-create AllergyIntolerance.lastOccurrence"); 2076 else if (Configuration.doAutoCreate()) 2077 this.lastOccurrence = new DateTimeType(); // bb 2078 return this.lastOccurrence; 2079 } 2080 2081 public boolean hasLastOccurrenceElement() { 2082 return this.lastOccurrence != null && !this.lastOccurrence.isEmpty(); 2083 } 2084 2085 public boolean hasLastOccurrence() { 2086 return this.lastOccurrence != null && !this.lastOccurrence.isEmpty(); 2087 } 2088 2089 /** 2090 * @param value {@link #lastOccurrence} (Represents the date and/or time of the last known occurrence of a reaction event.). This is the underlying object with id, value and extensions. The accessor "getLastOccurrence" gives direct access to the value 2091 */ 2092 public AllergyIntolerance setLastOccurrenceElement(DateTimeType value) { 2093 this.lastOccurrence = value; 2094 return this; 2095 } 2096 2097 /** 2098 * @return Represents the date and/or time of the last known occurrence of a reaction event. 2099 */ 2100 public Date getLastOccurrence() { 2101 return this.lastOccurrence == null ? null : this.lastOccurrence.getValue(); 2102 } 2103 2104 /** 2105 * @param value Represents the date and/or time of the last known occurrence of a reaction event. 2106 */ 2107 public AllergyIntolerance setLastOccurrence(Date value) { 2108 if (value == null) 2109 this.lastOccurrence = null; 2110 else { 2111 if (this.lastOccurrence == null) 2112 this.lastOccurrence = new DateTimeType(); 2113 this.lastOccurrence.setValue(value); 2114 } 2115 return this; 2116 } 2117 2118 /** 2119 * @return {@link #note} (Additional narrative about the propensity for the Adverse Reaction, not captured in other fields.) 2120 */ 2121 public List<Annotation> getNote() { 2122 if (this.note == null) 2123 this.note = new ArrayList<Annotation>(); 2124 return this.note; 2125 } 2126 2127 /** 2128 * @return Returns a reference to <code>this</code> for easy method chaining 2129 */ 2130 public AllergyIntolerance setNote(List<Annotation> theNote) { 2131 this.note = theNote; 2132 return this; 2133 } 2134 2135 public boolean hasNote() { 2136 if (this.note == null) 2137 return false; 2138 for (Annotation item : this.note) 2139 if (!item.isEmpty()) 2140 return true; 2141 return false; 2142 } 2143 2144 public Annotation addNote() { //3 2145 Annotation t = new Annotation(); 2146 if (this.note == null) 2147 this.note = new ArrayList<Annotation>(); 2148 this.note.add(t); 2149 return t; 2150 } 2151 2152 public AllergyIntolerance addNote(Annotation t) { //3 2153 if (t == null) 2154 return this; 2155 if (this.note == null) 2156 this.note = new ArrayList<Annotation>(); 2157 this.note.add(t); 2158 return this; 2159 } 2160 2161 /** 2162 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 2163 */ 2164 public Annotation getNoteFirstRep() { 2165 if (getNote().isEmpty()) { 2166 addNote(); 2167 } 2168 return getNote().get(0); 2169 } 2170 2171 /** 2172 * @return {@link #reaction} (Details about each adverse reaction event linked to exposure to the identified substance.) 2173 */ 2174 public List<AllergyIntoleranceReactionComponent> getReaction() { 2175 if (this.reaction == null) 2176 this.reaction = new ArrayList<AllergyIntoleranceReactionComponent>(); 2177 return this.reaction; 2178 } 2179 2180 /** 2181 * @return Returns a reference to <code>this</code> for easy method chaining 2182 */ 2183 public AllergyIntolerance setReaction(List<AllergyIntoleranceReactionComponent> theReaction) { 2184 this.reaction = theReaction; 2185 return this; 2186 } 2187 2188 public boolean hasReaction() { 2189 if (this.reaction == null) 2190 return false; 2191 for (AllergyIntoleranceReactionComponent item : this.reaction) 2192 if (!item.isEmpty()) 2193 return true; 2194 return false; 2195 } 2196 2197 public AllergyIntoleranceReactionComponent addReaction() { //3 2198 AllergyIntoleranceReactionComponent t = new AllergyIntoleranceReactionComponent(); 2199 if (this.reaction == null) 2200 this.reaction = new ArrayList<AllergyIntoleranceReactionComponent>(); 2201 this.reaction.add(t); 2202 return t; 2203 } 2204 2205 public AllergyIntolerance addReaction(AllergyIntoleranceReactionComponent t) { //3 2206 if (t == null) 2207 return this; 2208 if (this.reaction == null) 2209 this.reaction = new ArrayList<AllergyIntoleranceReactionComponent>(); 2210 this.reaction.add(t); 2211 return this; 2212 } 2213 2214 /** 2215 * @return The first repetition of repeating field {@link #reaction}, creating it if it does not already exist 2216 */ 2217 public AllergyIntoleranceReactionComponent getReactionFirstRep() { 2218 if (getReaction().isEmpty()) { 2219 addReaction(); 2220 } 2221 return getReaction().get(0); 2222 } 2223 2224 protected void listChildren(List<Property> children) { 2225 super.listChildren(children); 2226 children.add(new Property("identifier", "Identifier", "This records identifiers associated with this allergy/intolerance concern that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 0, java.lang.Integer.MAX_VALUE, identifier)); 2227 children.add(new Property("clinicalStatus", "code", "The clinical status of the allergy or intolerance.", 0, 1, clinicalStatus)); 2228 children.add(new Property("verificationStatus", "code", "Assertion about certainty associated with the propensity, or potential risk, of a reaction to the identified substance (including pharmaceutical product).", 0, 1, verificationStatus)); 2229 children.add(new Property("type", "code", "Identification of the underlying physiological mechanism for the reaction risk.", 0, 1, type)); 2230 children.add(new Property("category", "code", "Category of the identified substance.", 0, java.lang.Integer.MAX_VALUE, category)); 2231 children.add(new Property("criticality", "code", "Estimate of the potential clinical harm, or seriousness, of the reaction to the identified substance.", 0, 1, criticality)); 2232 children.add(new Property("code", "CodeableConcept", "Code for an allergy or intolerance statement (either a positive or a negated/excluded statement). This may be a code for a substance or pharmaceutical product that is considered to be responsible for the adverse reaction risk (e.g., \"Latex\"), an allergy or intolerance condition (e.g., \"Latex allergy\"), or a negated/excluded code for a specific substance or class (e.g., \"No latex allergy\") or a general or categorical negated statement (e.g., \"No known allergy\", \"No known drug allergies\").", 0, 1, code)); 2233 children.add(new Property("patient", "Reference(Patient)", "The patient who has the allergy or intolerance.", 0, 1, patient)); 2234 children.add(new Property("onset[x]", "dateTime|Age|Period|Range|string", "Estimated or actual date, date-time, or age when allergy or intolerance was identified.", 0, 1, onset)); 2235 children.add(new Property("assertedDate", "dateTime", "The date on which the existance of the AllergyIntolerance was first asserted or acknowledged.", 0, 1, assertedDate)); 2236 children.add(new Property("recorder", "Reference(Practitioner|Patient)", "Individual who recorded the record and takes responsibility for its content.", 0, 1, recorder)); 2237 children.add(new Property("asserter", "Reference(Patient|RelatedPerson|Practitioner)", "The source of the information about the allergy that is recorded.", 0, 1, asserter)); 2238 children.add(new Property("lastOccurrence", "dateTime", "Represents the date and/or time of the last known occurrence of a reaction event.", 0, 1, lastOccurrence)); 2239 children.add(new Property("note", "Annotation", "Additional narrative about the propensity for the Adverse Reaction, not captured in other fields.", 0, java.lang.Integer.MAX_VALUE, note)); 2240 children.add(new Property("reaction", "", "Details about each adverse reaction event linked to exposure to the identified substance.", 0, java.lang.Integer.MAX_VALUE, reaction)); 2241 } 2242 2243 @Override 2244 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2245 switch (_hash) { 2246 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "This records identifiers associated with this allergy/intolerance concern that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 0, java.lang.Integer.MAX_VALUE, identifier); 2247 case -462853915: /*clinicalStatus*/ return new Property("clinicalStatus", "code", "The clinical status of the allergy or intolerance.", 0, 1, clinicalStatus); 2248 case -842509843: /*verificationStatus*/ return new Property("verificationStatus", "code", "Assertion about certainty associated with the propensity, or potential risk, of a reaction to the identified substance (including pharmaceutical product).", 0, 1, verificationStatus); 2249 case 3575610: /*type*/ return new Property("type", "code", "Identification of the underlying physiological mechanism for the reaction risk.", 0, 1, type); 2250 case 50511102: /*category*/ return new Property("category", "code", "Category of the identified substance.", 0, java.lang.Integer.MAX_VALUE, category); 2251 case -1608054609: /*criticality*/ return new Property("criticality", "code", "Estimate of the potential clinical harm, or seriousness, of the reaction to the identified substance.", 0, 1, criticality); 2252 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Code for an allergy or intolerance statement (either a positive or a negated/excluded statement). This may be a code for a substance or pharmaceutical product that is considered to be responsible for the adverse reaction risk (e.g., \"Latex\"), an allergy or intolerance condition (e.g., \"Latex allergy\"), or a negated/excluded code for a specific substance or class (e.g., \"No latex allergy\") or a general or categorical negated statement (e.g., \"No known allergy\", \"No known drug allergies\").", 0, 1, code); 2253 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "The patient who has the allergy or intolerance.", 0, 1, patient); 2254 case -1886216323: /*onset[x]*/ return new Property("onset[x]", "dateTime|Age|Period|Range|string", "Estimated or actual date, date-time, or age when allergy or intolerance was identified.", 0, 1, onset); 2255 case 105901603: /*onset*/ return new Property("onset[x]", "dateTime|Age|Period|Range|string", "Estimated or actual date, date-time, or age when allergy or intolerance was identified.", 0, 1, onset); 2256 case -1701663010: /*onsetDateTime*/ return new Property("onset[x]", "dateTime|Age|Period|Range|string", "Estimated or actual date, date-time, or age when allergy or intolerance was identified.", 0, 1, onset); 2257 case -1886241828: /*onsetAge*/ return new Property("onset[x]", "dateTime|Age|Period|Range|string", "Estimated or actual date, date-time, or age when allergy or intolerance was identified.", 0, 1, onset); 2258 case -1545082428: /*onsetPeriod*/ return new Property("onset[x]", "dateTime|Age|Period|Range|string", "Estimated or actual date, date-time, or age when allergy or intolerance was identified.", 0, 1, onset); 2259 case -186664742: /*onsetRange*/ return new Property("onset[x]", "dateTime|Age|Period|Range|string", "Estimated or actual date, date-time, or age when allergy or intolerance was identified.", 0, 1, onset); 2260 case -1445342188: /*onsetString*/ return new Property("onset[x]", "dateTime|Age|Period|Range|string", "Estimated or actual date, date-time, or age when allergy or intolerance was identified.", 0, 1, onset); 2261 case -174231629: /*assertedDate*/ return new Property("assertedDate", "dateTime", "The date on which the existance of the AllergyIntolerance was first asserted or acknowledged.", 0, 1, assertedDate); 2262 case -799233858: /*recorder*/ return new Property("recorder", "Reference(Practitioner|Patient)", "Individual who recorded the record and takes responsibility for its content.", 0, 1, recorder); 2263 case -373242253: /*asserter*/ return new Property("asserter", "Reference(Patient|RelatedPerson|Practitioner)", "The source of the information about the allergy that is recorded.", 0, 1, asserter); 2264 case 1896977671: /*lastOccurrence*/ return new Property("lastOccurrence", "dateTime", "Represents the date and/or time of the last known occurrence of a reaction event.", 0, 1, lastOccurrence); 2265 case 3387378: /*note*/ return new Property("note", "Annotation", "Additional narrative about the propensity for the Adverse Reaction, not captured in other fields.", 0, java.lang.Integer.MAX_VALUE, note); 2266 case -867509719: /*reaction*/ return new Property("reaction", "", "Details about each adverse reaction event linked to exposure to the identified substance.", 0, java.lang.Integer.MAX_VALUE, reaction); 2267 default: return super.getNamedProperty(_hash, _name, _checkValid); 2268 } 2269 2270 } 2271 2272 @Override 2273 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2274 switch (hash) { 2275 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2276 case -462853915: /*clinicalStatus*/ return this.clinicalStatus == null ? new Base[0] : new Base[] {this.clinicalStatus}; // Enumeration<AllergyIntoleranceClinicalStatus> 2277 case -842509843: /*verificationStatus*/ return this.verificationStatus == null ? new Base[0] : new Base[] {this.verificationStatus}; // Enumeration<AllergyIntoleranceVerificationStatus> 2278 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<AllergyIntoleranceType> 2279 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // Enumeration<AllergyIntoleranceCategory> 2280 case -1608054609: /*criticality*/ return this.criticality == null ? new Base[0] : new Base[] {this.criticality}; // Enumeration<AllergyIntoleranceCriticality> 2281 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 2282 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 2283 case 105901603: /*onset*/ return this.onset == null ? new Base[0] : new Base[] {this.onset}; // Type 2284 case -174231629: /*assertedDate*/ return this.assertedDate == null ? new Base[0] : new Base[] {this.assertedDate}; // DateTimeType 2285 case -799233858: /*recorder*/ return this.recorder == null ? new Base[0] : new Base[] {this.recorder}; // Reference 2286 case -373242253: /*asserter*/ return this.asserter == null ? new Base[0] : new Base[] {this.asserter}; // Reference 2287 case 1896977671: /*lastOccurrence*/ return this.lastOccurrence == null ? new Base[0] : new Base[] {this.lastOccurrence}; // DateTimeType 2288 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2289 case -867509719: /*reaction*/ return this.reaction == null ? new Base[0] : this.reaction.toArray(new Base[this.reaction.size()]); // AllergyIntoleranceReactionComponent 2290 default: return super.getProperty(hash, name, checkValid); 2291 } 2292 2293 } 2294 2295 @Override 2296 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2297 switch (hash) { 2298 case -1618432855: // identifier 2299 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2300 return value; 2301 case -462853915: // clinicalStatus 2302 value = new AllergyIntoleranceClinicalStatusEnumFactory().fromType(castToCode(value)); 2303 this.clinicalStatus = (Enumeration) value; // Enumeration<AllergyIntoleranceClinicalStatus> 2304 return value; 2305 case -842509843: // verificationStatus 2306 value = new AllergyIntoleranceVerificationStatusEnumFactory().fromType(castToCode(value)); 2307 this.verificationStatus = (Enumeration) value; // Enumeration<AllergyIntoleranceVerificationStatus> 2308 return value; 2309 case 3575610: // type 2310 value = new AllergyIntoleranceTypeEnumFactory().fromType(castToCode(value)); 2311 this.type = (Enumeration) value; // Enumeration<AllergyIntoleranceType> 2312 return value; 2313 case 50511102: // category 2314 value = new AllergyIntoleranceCategoryEnumFactory().fromType(castToCode(value)); 2315 this.getCategory().add((Enumeration) value); // Enumeration<AllergyIntoleranceCategory> 2316 return value; 2317 case -1608054609: // criticality 2318 value = new AllergyIntoleranceCriticalityEnumFactory().fromType(castToCode(value)); 2319 this.criticality = (Enumeration) value; // Enumeration<AllergyIntoleranceCriticality> 2320 return value; 2321 case 3059181: // code 2322 this.code = castToCodeableConcept(value); // CodeableConcept 2323 return value; 2324 case -791418107: // patient 2325 this.patient = castToReference(value); // Reference 2326 return value; 2327 case 105901603: // onset 2328 this.onset = castToType(value); // Type 2329 return value; 2330 case -174231629: // assertedDate 2331 this.assertedDate = castToDateTime(value); // DateTimeType 2332 return value; 2333 case -799233858: // recorder 2334 this.recorder = castToReference(value); // Reference 2335 return value; 2336 case -373242253: // asserter 2337 this.asserter = castToReference(value); // Reference 2338 return value; 2339 case 1896977671: // lastOccurrence 2340 this.lastOccurrence = castToDateTime(value); // DateTimeType 2341 return value; 2342 case 3387378: // note 2343 this.getNote().add(castToAnnotation(value)); // Annotation 2344 return value; 2345 case -867509719: // reaction 2346 this.getReaction().add((AllergyIntoleranceReactionComponent) value); // AllergyIntoleranceReactionComponent 2347 return value; 2348 default: return super.setProperty(hash, name, value); 2349 } 2350 2351 } 2352 2353 @Override 2354 public Base setProperty(String name, Base value) throws FHIRException { 2355 if (name.equals("identifier")) { 2356 this.getIdentifier().add(castToIdentifier(value)); 2357 } else if (name.equals("clinicalStatus")) { 2358 value = new AllergyIntoleranceClinicalStatusEnumFactory().fromType(castToCode(value)); 2359 this.clinicalStatus = (Enumeration) value; // Enumeration<AllergyIntoleranceClinicalStatus> 2360 } else if (name.equals("verificationStatus")) { 2361 value = new AllergyIntoleranceVerificationStatusEnumFactory().fromType(castToCode(value)); 2362 this.verificationStatus = (Enumeration) value; // Enumeration<AllergyIntoleranceVerificationStatus> 2363 } else if (name.equals("type")) { 2364 value = new AllergyIntoleranceTypeEnumFactory().fromType(castToCode(value)); 2365 this.type = (Enumeration) value; // Enumeration<AllergyIntoleranceType> 2366 } else if (name.equals("category")) { 2367 value = new AllergyIntoleranceCategoryEnumFactory().fromType(castToCode(value)); 2368 this.getCategory().add((Enumeration) value); 2369 } else if (name.equals("criticality")) { 2370 value = new AllergyIntoleranceCriticalityEnumFactory().fromType(castToCode(value)); 2371 this.criticality = (Enumeration) value; // Enumeration<AllergyIntoleranceCriticality> 2372 } else if (name.equals("code")) { 2373 this.code = castToCodeableConcept(value); // CodeableConcept 2374 } else if (name.equals("patient")) { 2375 this.patient = castToReference(value); // Reference 2376 } else if (name.equals("onset[x]")) { 2377 this.onset = castToType(value); // Type 2378 } else if (name.equals("assertedDate")) { 2379 this.assertedDate = castToDateTime(value); // DateTimeType 2380 } else if (name.equals("recorder")) { 2381 this.recorder = castToReference(value); // Reference 2382 } else if (name.equals("asserter")) { 2383 this.asserter = castToReference(value); // Reference 2384 } else if (name.equals("lastOccurrence")) { 2385 this.lastOccurrence = castToDateTime(value); // DateTimeType 2386 } else if (name.equals("note")) { 2387 this.getNote().add(castToAnnotation(value)); 2388 } else if (name.equals("reaction")) { 2389 this.getReaction().add((AllergyIntoleranceReactionComponent) value); 2390 } else 2391 return super.setProperty(name, value); 2392 return value; 2393 } 2394 2395 @Override 2396 public Base makeProperty(int hash, String name) throws FHIRException { 2397 switch (hash) { 2398 case -1618432855: return addIdentifier(); 2399 case -462853915: return getClinicalStatusElement(); 2400 case -842509843: return getVerificationStatusElement(); 2401 case 3575610: return getTypeElement(); 2402 case 50511102: return addCategoryElement(); 2403 case -1608054609: return getCriticalityElement(); 2404 case 3059181: return getCode(); 2405 case -791418107: return getPatient(); 2406 case -1886216323: return getOnset(); 2407 case 105901603: return getOnset(); 2408 case -174231629: return getAssertedDateElement(); 2409 case -799233858: return getRecorder(); 2410 case -373242253: return getAsserter(); 2411 case 1896977671: return getLastOccurrenceElement(); 2412 case 3387378: return addNote(); 2413 case -867509719: return addReaction(); 2414 default: return super.makeProperty(hash, name); 2415 } 2416 2417 } 2418 2419 @Override 2420 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2421 switch (hash) { 2422 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2423 case -462853915: /*clinicalStatus*/ return new String[] {"code"}; 2424 case -842509843: /*verificationStatus*/ return new String[] {"code"}; 2425 case 3575610: /*type*/ return new String[] {"code"}; 2426 case 50511102: /*category*/ return new String[] {"code"}; 2427 case -1608054609: /*criticality*/ return new String[] {"code"}; 2428 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 2429 case -791418107: /*patient*/ return new String[] {"Reference"}; 2430 case 105901603: /*onset*/ return new String[] {"dateTime", "Age", "Period", "Range", "string"}; 2431 case -174231629: /*assertedDate*/ return new String[] {"dateTime"}; 2432 case -799233858: /*recorder*/ return new String[] {"Reference"}; 2433 case -373242253: /*asserter*/ return new String[] {"Reference"}; 2434 case 1896977671: /*lastOccurrence*/ return new String[] {"dateTime"}; 2435 case 3387378: /*note*/ return new String[] {"Annotation"}; 2436 case -867509719: /*reaction*/ return new String[] {}; 2437 default: return super.getTypesForProperty(hash, name); 2438 } 2439 2440 } 2441 2442 @Override 2443 public Base addChild(String name) throws FHIRException { 2444 if (name.equals("identifier")) { 2445 return addIdentifier(); 2446 } 2447 else if (name.equals("clinicalStatus")) { 2448 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.clinicalStatus"); 2449 } 2450 else if (name.equals("verificationStatus")) { 2451 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.verificationStatus"); 2452 } 2453 else if (name.equals("type")) { 2454 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.type"); 2455 } 2456 else if (name.equals("category")) { 2457 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.category"); 2458 } 2459 else if (name.equals("criticality")) { 2460 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.criticality"); 2461 } 2462 else if (name.equals("code")) { 2463 this.code = new CodeableConcept(); 2464 return this.code; 2465 } 2466 else if (name.equals("patient")) { 2467 this.patient = new Reference(); 2468 return this.patient; 2469 } 2470 else if (name.equals("onsetDateTime")) { 2471 this.onset = new DateTimeType(); 2472 return this.onset; 2473 } 2474 else if (name.equals("onsetAge")) { 2475 this.onset = new Age(); 2476 return this.onset; 2477 } 2478 else if (name.equals("onsetPeriod")) { 2479 this.onset = new Period(); 2480 return this.onset; 2481 } 2482 else if (name.equals("onsetRange")) { 2483 this.onset = new Range(); 2484 return this.onset; 2485 } 2486 else if (name.equals("onsetString")) { 2487 this.onset = new StringType(); 2488 return this.onset; 2489 } 2490 else if (name.equals("assertedDate")) { 2491 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.assertedDate"); 2492 } 2493 else if (name.equals("recorder")) { 2494 this.recorder = new Reference(); 2495 return this.recorder; 2496 } 2497 else if (name.equals("asserter")) { 2498 this.asserter = new Reference(); 2499 return this.asserter; 2500 } 2501 else if (name.equals("lastOccurrence")) { 2502 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.lastOccurrence"); 2503 } 2504 else if (name.equals("note")) { 2505 return addNote(); 2506 } 2507 else if (name.equals("reaction")) { 2508 return addReaction(); 2509 } 2510 else 2511 return super.addChild(name); 2512 } 2513 2514 public String fhirType() { 2515 return "AllergyIntolerance"; 2516 2517 } 2518 2519 public AllergyIntolerance copy() { 2520 AllergyIntolerance dst = new AllergyIntolerance(); 2521 copyValues(dst); 2522 if (identifier != null) { 2523 dst.identifier = new ArrayList<Identifier>(); 2524 for (Identifier i : identifier) 2525 dst.identifier.add(i.copy()); 2526 }; 2527 dst.clinicalStatus = clinicalStatus == null ? null : clinicalStatus.copy(); 2528 dst.verificationStatus = verificationStatus == null ? null : verificationStatus.copy(); 2529 dst.type = type == null ? null : type.copy(); 2530 if (category != null) { 2531 dst.category = new ArrayList<Enumeration<AllergyIntoleranceCategory>>(); 2532 for (Enumeration<AllergyIntoleranceCategory> i : category) 2533 dst.category.add(i.copy()); 2534 }; 2535 dst.criticality = criticality == null ? null : criticality.copy(); 2536 dst.code = code == null ? null : code.copy(); 2537 dst.patient = patient == null ? null : patient.copy(); 2538 dst.onset = onset == null ? null : onset.copy(); 2539 dst.assertedDate = assertedDate == null ? null : assertedDate.copy(); 2540 dst.recorder = recorder == null ? null : recorder.copy(); 2541 dst.asserter = asserter == null ? null : asserter.copy(); 2542 dst.lastOccurrence = lastOccurrence == null ? null : lastOccurrence.copy(); 2543 if (note != null) { 2544 dst.note = new ArrayList<Annotation>(); 2545 for (Annotation i : note) 2546 dst.note.add(i.copy()); 2547 }; 2548 if (reaction != null) { 2549 dst.reaction = new ArrayList<AllergyIntoleranceReactionComponent>(); 2550 for (AllergyIntoleranceReactionComponent i : reaction) 2551 dst.reaction.add(i.copy()); 2552 }; 2553 return dst; 2554 } 2555 2556 protected AllergyIntolerance typedCopy() { 2557 return copy(); 2558 } 2559 2560 @Override 2561 public boolean equalsDeep(Base other_) { 2562 if (!super.equalsDeep(other_)) 2563 return false; 2564 if (!(other_ instanceof AllergyIntolerance)) 2565 return false; 2566 AllergyIntolerance o = (AllergyIntolerance) other_; 2567 return compareDeep(identifier, o.identifier, true) && compareDeep(clinicalStatus, o.clinicalStatus, true) 2568 && compareDeep(verificationStatus, o.verificationStatus, true) && compareDeep(type, o.type, true) 2569 && compareDeep(category, o.category, true) && compareDeep(criticality, o.criticality, true) && compareDeep(code, o.code, true) 2570 && compareDeep(patient, o.patient, true) && compareDeep(onset, o.onset, true) && compareDeep(assertedDate, o.assertedDate, true) 2571 && compareDeep(recorder, o.recorder, true) && compareDeep(asserter, o.asserter, true) && compareDeep(lastOccurrence, o.lastOccurrence, true) 2572 && compareDeep(note, o.note, true) && compareDeep(reaction, o.reaction, true); 2573 } 2574 2575 @Override 2576 public boolean equalsShallow(Base other_) { 2577 if (!super.equalsShallow(other_)) 2578 return false; 2579 if (!(other_ instanceof AllergyIntolerance)) 2580 return false; 2581 AllergyIntolerance o = (AllergyIntolerance) other_; 2582 return compareValues(clinicalStatus, o.clinicalStatus, true) && compareValues(verificationStatus, o.verificationStatus, true) 2583 && compareValues(type, o.type, true) && compareValues(category, o.category, true) && compareValues(criticality, o.criticality, true) 2584 && compareValues(assertedDate, o.assertedDate, true) && compareValues(lastOccurrence, o.lastOccurrence, true) 2585 ; 2586 } 2587 2588 public boolean isEmpty() { 2589 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, clinicalStatus 2590 , verificationStatus, type, category, criticality, code, patient, onset, assertedDate 2591 , recorder, asserter, lastOccurrence, note, reaction); 2592 } 2593 2594 @Override 2595 public ResourceType getResourceType() { 2596 return ResourceType.AllergyIntolerance; 2597 } 2598 2599 /** 2600 * Search parameter: <b>severity</b> 2601 * <p> 2602 * Description: <b>mild | moderate | severe (of event as a whole)</b><br> 2603 * Type: <b>token</b><br> 2604 * Path: <b>AllergyIntolerance.reaction.severity</b><br> 2605 * </p> 2606 */ 2607 @SearchParamDefinition(name="severity", path="AllergyIntolerance.reaction.severity", description="mild | moderate | severe (of event as a whole)", type="token" ) 2608 public static final String SP_SEVERITY = "severity"; 2609 /** 2610 * <b>Fluent Client</b> search parameter constant for <b>severity</b> 2611 * <p> 2612 * Description: <b>mild | moderate | severe (of event as a whole)</b><br> 2613 * Type: <b>token</b><br> 2614 * Path: <b>AllergyIntolerance.reaction.severity</b><br> 2615 * </p> 2616 */ 2617 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SEVERITY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SEVERITY); 2618 2619 /** 2620 * Search parameter: <b>date</b> 2621 * <p> 2622 * Description: <b>Date record was believed accurate</b><br> 2623 * Type: <b>date</b><br> 2624 * Path: <b>AllergyIntolerance.assertedDate</b><br> 2625 * </p> 2626 */ 2627 @SearchParamDefinition(name="date", path="AllergyIntolerance.assertedDate", description="Date record was believed accurate", type="date" ) 2628 public static final String SP_DATE = "date"; 2629 /** 2630 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2631 * <p> 2632 * Description: <b>Date record was believed accurate</b><br> 2633 * Type: <b>date</b><br> 2634 * Path: <b>AllergyIntolerance.assertedDate</b><br> 2635 * </p> 2636 */ 2637 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 2638 2639 /** 2640 * Search parameter: <b>identifier</b> 2641 * <p> 2642 * Description: <b>External ids for this item</b><br> 2643 * Type: <b>token</b><br> 2644 * Path: <b>AllergyIntolerance.identifier</b><br> 2645 * </p> 2646 */ 2647 @SearchParamDefinition(name="identifier", path="AllergyIntolerance.identifier", description="External ids for this item", type="token" ) 2648 public static final String SP_IDENTIFIER = "identifier"; 2649 /** 2650 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2651 * <p> 2652 * Description: <b>External ids for this item</b><br> 2653 * Type: <b>token</b><br> 2654 * Path: <b>AllergyIntolerance.identifier</b><br> 2655 * </p> 2656 */ 2657 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2658 2659 /** 2660 * Search parameter: <b>manifestation</b> 2661 * <p> 2662 * Description: <b>Clinical symptoms/signs associated with the Event</b><br> 2663 * Type: <b>token</b><br> 2664 * Path: <b>AllergyIntolerance.reaction.manifestation</b><br> 2665 * </p> 2666 */ 2667 @SearchParamDefinition(name="manifestation", path="AllergyIntolerance.reaction.manifestation", description="Clinical symptoms/signs associated with the Event", type="token" ) 2668 public static final String SP_MANIFESTATION = "manifestation"; 2669 /** 2670 * <b>Fluent Client</b> search parameter constant for <b>manifestation</b> 2671 * <p> 2672 * Description: <b>Clinical symptoms/signs associated with the Event</b><br> 2673 * Type: <b>token</b><br> 2674 * Path: <b>AllergyIntolerance.reaction.manifestation</b><br> 2675 * </p> 2676 */ 2677 public static final ca.uhn.fhir.rest.gclient.TokenClientParam MANIFESTATION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_MANIFESTATION); 2678 2679 /** 2680 * Search parameter: <b>recorder</b> 2681 * <p> 2682 * Description: <b>Who recorded the sensitivity</b><br> 2683 * Type: <b>reference</b><br> 2684 * Path: <b>AllergyIntolerance.recorder</b><br> 2685 * </p> 2686 */ 2687 @SearchParamDefinition(name="recorder", path="AllergyIntolerance.recorder", description="Who recorded the sensitivity", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Patient.class, Practitioner.class } ) 2688 public static final String SP_RECORDER = "recorder"; 2689 /** 2690 * <b>Fluent Client</b> search parameter constant for <b>recorder</b> 2691 * <p> 2692 * Description: <b>Who recorded the sensitivity</b><br> 2693 * Type: <b>reference</b><br> 2694 * Path: <b>AllergyIntolerance.recorder</b><br> 2695 * </p> 2696 */ 2697 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RECORDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RECORDER); 2698 2699/** 2700 * Constant for fluent queries to be used to add include statements. Specifies 2701 * the path value of "<b>AllergyIntolerance:recorder</b>". 2702 */ 2703 public static final ca.uhn.fhir.model.api.Include INCLUDE_RECORDER = new ca.uhn.fhir.model.api.Include("AllergyIntolerance:recorder").toLocked(); 2704 2705 /** 2706 * Search parameter: <b>code</b> 2707 * <p> 2708 * Description: <b>Code that identifies the allergy or intolerance</b><br> 2709 * Type: <b>token</b><br> 2710 * Path: <b>AllergyIntolerance.code, AllergyIntolerance.reaction.substance</b><br> 2711 * </p> 2712 */ 2713 @SearchParamDefinition(name="code", path="AllergyIntolerance.code | AllergyIntolerance.reaction.substance", description="Code that identifies the allergy or intolerance", type="token" ) 2714 public static final String SP_CODE = "code"; 2715 /** 2716 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2717 * <p> 2718 * Description: <b>Code that identifies the allergy or intolerance</b><br> 2719 * Type: <b>token</b><br> 2720 * Path: <b>AllergyIntolerance.code, AllergyIntolerance.reaction.substance</b><br> 2721 * </p> 2722 */ 2723 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 2724 2725 /** 2726 * Search parameter: <b>verification-status</b> 2727 * <p> 2728 * Description: <b>unconfirmed | confirmed | refuted | entered-in-error</b><br> 2729 * Type: <b>token</b><br> 2730 * Path: <b>AllergyIntolerance.verificationStatus</b><br> 2731 * </p> 2732 */ 2733 @SearchParamDefinition(name="verification-status", path="AllergyIntolerance.verificationStatus", description="unconfirmed | confirmed | refuted | entered-in-error", type="token" ) 2734 public static final String SP_VERIFICATION_STATUS = "verification-status"; 2735 /** 2736 * <b>Fluent Client</b> search parameter constant for <b>verification-status</b> 2737 * <p> 2738 * Description: <b>unconfirmed | confirmed | refuted | entered-in-error</b><br> 2739 * Type: <b>token</b><br> 2740 * Path: <b>AllergyIntolerance.verificationStatus</b><br> 2741 * </p> 2742 */ 2743 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERIFICATION_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERIFICATION_STATUS); 2744 2745 /** 2746 * Search parameter: <b>criticality</b> 2747 * <p> 2748 * Description: <b>low | high | unable-to-assess</b><br> 2749 * Type: <b>token</b><br> 2750 * Path: <b>AllergyIntolerance.criticality</b><br> 2751 * </p> 2752 */ 2753 @SearchParamDefinition(name="criticality", path="AllergyIntolerance.criticality", description="low | high | unable-to-assess", type="token" ) 2754 public static final String SP_CRITICALITY = "criticality"; 2755 /** 2756 * <b>Fluent Client</b> search parameter constant for <b>criticality</b> 2757 * <p> 2758 * Description: <b>low | high | unable-to-assess</b><br> 2759 * Type: <b>token</b><br> 2760 * Path: <b>AllergyIntolerance.criticality</b><br> 2761 * </p> 2762 */ 2763 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CRITICALITY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CRITICALITY); 2764 2765 /** 2766 * Search parameter: <b>clinical-status</b> 2767 * <p> 2768 * Description: <b>active | inactive | resolved</b><br> 2769 * Type: <b>token</b><br> 2770 * Path: <b>AllergyIntolerance.clinicalStatus</b><br> 2771 * </p> 2772 */ 2773 @SearchParamDefinition(name="clinical-status", path="AllergyIntolerance.clinicalStatus", description="active | inactive | resolved", type="token" ) 2774 public static final String SP_CLINICAL_STATUS = "clinical-status"; 2775 /** 2776 * <b>Fluent Client</b> search parameter constant for <b>clinical-status</b> 2777 * <p> 2778 * Description: <b>active | inactive | resolved</b><br> 2779 * Type: <b>token</b><br> 2780 * Path: <b>AllergyIntolerance.clinicalStatus</b><br> 2781 * </p> 2782 */ 2783 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CLINICAL_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CLINICAL_STATUS); 2784 2785 /** 2786 * Search parameter: <b>type</b> 2787 * <p> 2788 * Description: <b>allergy | intolerance - Underlying mechanism (if known)</b><br> 2789 * Type: <b>token</b><br> 2790 * Path: <b>AllergyIntolerance.type</b><br> 2791 * </p> 2792 */ 2793 @SearchParamDefinition(name="type", path="AllergyIntolerance.type", description="allergy | intolerance - Underlying mechanism (if known)", type="token" ) 2794 public static final String SP_TYPE = "type"; 2795 /** 2796 * <b>Fluent Client</b> search parameter constant for <b>type</b> 2797 * <p> 2798 * Description: <b>allergy | intolerance - Underlying mechanism (if known)</b><br> 2799 * Type: <b>token</b><br> 2800 * Path: <b>AllergyIntolerance.type</b><br> 2801 * </p> 2802 */ 2803 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 2804 2805 /** 2806 * Search parameter: <b>onset</b> 2807 * <p> 2808 * Description: <b>Date(/time) when manifestations showed</b><br> 2809 * Type: <b>date</b><br> 2810 * Path: <b>AllergyIntolerance.reaction.onset</b><br> 2811 * </p> 2812 */ 2813 @SearchParamDefinition(name="onset", path="AllergyIntolerance.reaction.onset", description="Date(/time) when manifestations showed", type="date" ) 2814 public static final String SP_ONSET = "onset"; 2815 /** 2816 * <b>Fluent Client</b> search parameter constant for <b>onset</b> 2817 * <p> 2818 * Description: <b>Date(/time) when manifestations showed</b><br> 2819 * Type: <b>date</b><br> 2820 * Path: <b>AllergyIntolerance.reaction.onset</b><br> 2821 * </p> 2822 */ 2823 public static final ca.uhn.fhir.rest.gclient.DateClientParam ONSET = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_ONSET); 2824 2825 /** 2826 * Search parameter: <b>route</b> 2827 * <p> 2828 * Description: <b>How the subject was exposed to the substance</b><br> 2829 * Type: <b>token</b><br> 2830 * Path: <b>AllergyIntolerance.reaction.exposureRoute</b><br> 2831 * </p> 2832 */ 2833 @SearchParamDefinition(name="route", path="AllergyIntolerance.reaction.exposureRoute", description="How the subject was exposed to the substance", type="token" ) 2834 public static final String SP_ROUTE = "route"; 2835 /** 2836 * <b>Fluent Client</b> search parameter constant for <b>route</b> 2837 * <p> 2838 * Description: <b>How the subject was exposed to the substance</b><br> 2839 * Type: <b>token</b><br> 2840 * Path: <b>AllergyIntolerance.reaction.exposureRoute</b><br> 2841 * </p> 2842 */ 2843 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ROUTE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ROUTE); 2844 2845 /** 2846 * Search parameter: <b>asserter</b> 2847 * <p> 2848 * Description: <b>Source of the information about the allergy</b><br> 2849 * Type: <b>reference</b><br> 2850 * Path: <b>AllergyIntolerance.asserter</b><br> 2851 * </p> 2852 */ 2853 @SearchParamDefinition(name="asserter", path="AllergyIntolerance.asserter", description="Source of the information about the allergy", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Patient.class, Practitioner.class, RelatedPerson.class } ) 2854 public static final String SP_ASSERTER = "asserter"; 2855 /** 2856 * <b>Fluent Client</b> search parameter constant for <b>asserter</b> 2857 * <p> 2858 * Description: <b>Source of the information about the allergy</b><br> 2859 * Type: <b>reference</b><br> 2860 * Path: <b>AllergyIntolerance.asserter</b><br> 2861 * </p> 2862 */ 2863 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ASSERTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ASSERTER); 2864 2865/** 2866 * Constant for fluent queries to be used to add include statements. Specifies 2867 * the path value of "<b>AllergyIntolerance:asserter</b>". 2868 */ 2869 public static final ca.uhn.fhir.model.api.Include INCLUDE_ASSERTER = new ca.uhn.fhir.model.api.Include("AllergyIntolerance:asserter").toLocked(); 2870 2871 /** 2872 * Search parameter: <b>patient</b> 2873 * <p> 2874 * Description: <b>Who the sensitivity is for</b><br> 2875 * Type: <b>reference</b><br> 2876 * Path: <b>AllergyIntolerance.patient</b><br> 2877 * </p> 2878 */ 2879 @SearchParamDefinition(name="patient", path="AllergyIntolerance.patient", description="Who the sensitivity is for", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 2880 public static final String SP_PATIENT = "patient"; 2881 /** 2882 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2883 * <p> 2884 * Description: <b>Who the sensitivity is for</b><br> 2885 * Type: <b>reference</b><br> 2886 * Path: <b>AllergyIntolerance.patient</b><br> 2887 * </p> 2888 */ 2889 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2890 2891/** 2892 * Constant for fluent queries to be used to add include statements. Specifies 2893 * the path value of "<b>AllergyIntolerance:patient</b>". 2894 */ 2895 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("AllergyIntolerance:patient").toLocked(); 2896 2897 /** 2898 * Search parameter: <b>category</b> 2899 * <p> 2900 * Description: <b>food | medication | environment | biologic</b><br> 2901 * Type: <b>token</b><br> 2902 * Path: <b>AllergyIntolerance.category</b><br> 2903 * </p> 2904 */ 2905 @SearchParamDefinition(name="category", path="AllergyIntolerance.category", description="food | medication | environment | biologic", type="token" ) 2906 public static final String SP_CATEGORY = "category"; 2907 /** 2908 * <b>Fluent Client</b> search parameter constant for <b>category</b> 2909 * <p> 2910 * Description: <b>food | medication | environment | biologic</b><br> 2911 * Type: <b>token</b><br> 2912 * Path: <b>AllergyIntolerance.category</b><br> 2913 * </p> 2914 */ 2915 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 2916 2917 /** 2918 * Search parameter: <b>last-date</b> 2919 * <p> 2920 * Description: <b>Date(/time) of last known occurrence of a reaction</b><br> 2921 * Type: <b>date</b><br> 2922 * Path: <b>AllergyIntolerance.lastOccurrence</b><br> 2923 * </p> 2924 */ 2925 @SearchParamDefinition(name="last-date", path="AllergyIntolerance.lastOccurrence", description="Date(/time) of last known occurrence of a reaction", type="date" ) 2926 public static final String SP_LAST_DATE = "last-date"; 2927 /** 2928 * <b>Fluent Client</b> search parameter constant for <b>last-date</b> 2929 * <p> 2930 * Description: <b>Date(/time) of last known occurrence of a reaction</b><br> 2931 * Type: <b>date</b><br> 2932 * Path: <b>AllergyIntolerance.lastOccurrence</b><br> 2933 * </p> 2934 */ 2935 public static final ca.uhn.fhir.rest.gclient.DateClientParam LAST_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_LAST_DATE); 2936 2937 2938}