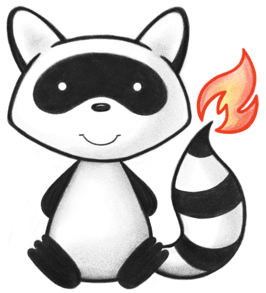
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.Date; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.exceptions.FHIRFormatError; 040import org.hl7.fhir.instance.model.api.ICompositeType; 041 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.DatatypeDef; 044import ca.uhn.fhir.model.api.annotation.Description; 045/** 046 * A text note which also contains information about who made the statement and when. 047 */ 048@DatatypeDef(name="Annotation") 049public class Annotation extends Type implements ICompositeType { 050 051 /** 052 * The individual responsible for making the annotation. 053 */ 054 @Child(name = "author", type = {Practitioner.class, Patient.class, RelatedPerson.class, StringType.class}, order=0, min=0, max=1, modifier=false, summary=true) 055 @Description(shortDefinition="Individual responsible for the annotation", formalDefinition="The individual responsible for making the annotation." ) 056 protected Type author; 057 058 /** 059 * Indicates when this particular annotation was made. 060 */ 061 @Child(name = "time", type = {DateTimeType.class}, order=1, min=0, max=1, modifier=false, summary=true) 062 @Description(shortDefinition="When the annotation was made", formalDefinition="Indicates when this particular annotation was made." ) 063 protected DateTimeType time; 064 065 /** 066 * The text of the annotation. 067 */ 068 @Child(name = "text", type = {StringType.class}, order=2, min=1, max=1, modifier=false, summary=false) 069 @Description(shortDefinition="The annotation - text content", formalDefinition="The text of the annotation." ) 070 protected StringType text; 071 072 private static final long serialVersionUID = -575590381L; 073 074 /** 075 * Constructor 076 */ 077 public Annotation() { 078 super(); 079 } 080 081 /** 082 * Constructor 083 */ 084 public Annotation(StringType text) { 085 super(); 086 this.text = text; 087 } 088 089 /** 090 * @return {@link #author} (The individual responsible for making the annotation.) 091 */ 092 public Type getAuthor() { 093 return this.author; 094 } 095 096 /** 097 * @return {@link #author} (The individual responsible for making the annotation.) 098 */ 099 public Reference getAuthorReference() throws FHIRException { 100 if (this.author == null) 101 return null; 102 if (!(this.author instanceof Reference)) 103 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.author.getClass().getName()+" was encountered"); 104 return (Reference) this.author; 105 } 106 107 public boolean hasAuthorReference() { 108 return this != null && this.author instanceof Reference; 109 } 110 111 /** 112 * @return {@link #author} (The individual responsible for making the annotation.) 113 */ 114 public StringType getAuthorStringType() throws FHIRException { 115 if (this.author == null) 116 return null; 117 if (!(this.author instanceof StringType)) 118 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.author.getClass().getName()+" was encountered"); 119 return (StringType) this.author; 120 } 121 122 public boolean hasAuthorStringType() { 123 return this != null && this.author instanceof StringType; 124 } 125 126 public boolean hasAuthor() { 127 return this.author != null && !this.author.isEmpty(); 128 } 129 130 /** 131 * @param value {@link #author} (The individual responsible for making the annotation.) 132 */ 133 public Annotation setAuthor(Type value) throws FHIRFormatError { 134 if (value != null && !(value instanceof Reference || value instanceof StringType)) 135 throw new FHIRFormatError("Not the right type for Annotation.author[x]: "+value.fhirType()); 136 this.author = value; 137 return this; 138 } 139 140 /** 141 * @return {@link #time} (Indicates when this particular annotation was made.). This is the underlying object with id, value and extensions. The accessor "getTime" gives direct access to the value 142 */ 143 public DateTimeType getTimeElement() { 144 if (this.time == null) 145 if (Configuration.errorOnAutoCreate()) 146 throw new Error("Attempt to auto-create Annotation.time"); 147 else if (Configuration.doAutoCreate()) 148 this.time = new DateTimeType(); // bb 149 return this.time; 150 } 151 152 public boolean hasTimeElement() { 153 return this.time != null && !this.time.isEmpty(); 154 } 155 156 public boolean hasTime() { 157 return this.time != null && !this.time.isEmpty(); 158 } 159 160 /** 161 * @param value {@link #time} (Indicates when this particular annotation was made.). This is the underlying object with id, value and extensions. The accessor "getTime" gives direct access to the value 162 */ 163 public Annotation setTimeElement(DateTimeType value) { 164 this.time = value; 165 return this; 166 } 167 168 /** 169 * @return Indicates when this particular annotation was made. 170 */ 171 public Date getTime() { 172 return this.time == null ? null : this.time.getValue(); 173 } 174 175 /** 176 * @param value Indicates when this particular annotation was made. 177 */ 178 public Annotation setTime(Date value) { 179 if (value == null) 180 this.time = null; 181 else { 182 if (this.time == null) 183 this.time = new DateTimeType(); 184 this.time.setValue(value); 185 } 186 return this; 187 } 188 189 /** 190 * @return {@link #text} (The text of the annotation.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 191 */ 192 public StringType getTextElement() { 193 if (this.text == null) 194 if (Configuration.errorOnAutoCreate()) 195 throw new Error("Attempt to auto-create Annotation.text"); 196 else if (Configuration.doAutoCreate()) 197 this.text = new StringType(); // bb 198 return this.text; 199 } 200 201 public boolean hasTextElement() { 202 return this.text != null && !this.text.isEmpty(); 203 } 204 205 public boolean hasText() { 206 return this.text != null && !this.text.isEmpty(); 207 } 208 209 /** 210 * @param value {@link #text} (The text of the annotation.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 211 */ 212 public Annotation setTextElement(StringType value) { 213 this.text = value; 214 return this; 215 } 216 217 /** 218 * @return The text of the annotation. 219 */ 220 public String getText() { 221 return this.text == null ? null : this.text.getValue(); 222 } 223 224 /** 225 * @param value The text of the annotation. 226 */ 227 public Annotation setText(String value) { 228 if (this.text == null) 229 this.text = new StringType(); 230 this.text.setValue(value); 231 return this; 232 } 233 234 protected void listChildren(List<Property> children) { 235 super.listChildren(children); 236 children.add(new Property("author[x]", "Reference(Practitioner|Patient|RelatedPerson)|string", "The individual responsible for making the annotation.", 0, 1, author)); 237 children.add(new Property("time", "dateTime", "Indicates when this particular annotation was made.", 0, 1, time)); 238 children.add(new Property("text", "string", "The text of the annotation.", 0, 1, text)); 239 } 240 241 @Override 242 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 243 switch (_hash) { 244 case 1475597077: /*author[x]*/ return new Property("author[x]", "Reference(Practitioner|Patient|RelatedPerson)|string", "The individual responsible for making the annotation.", 0, 1, author); 245 case -1406328437: /*author*/ return new Property("author[x]", "Reference(Practitioner|Patient|RelatedPerson)|string", "The individual responsible for making the annotation.", 0, 1, author); 246 case 305515008: /*authorReference*/ return new Property("author[x]", "Reference(Practitioner|Patient|RelatedPerson)|string", "The individual responsible for making the annotation.", 0, 1, author); 247 case 290249084: /*authorString*/ return new Property("author[x]", "Reference(Practitioner|Patient|RelatedPerson)|string", "The individual responsible for making the annotation.", 0, 1, author); 248 case 3560141: /*time*/ return new Property("time", "dateTime", "Indicates when this particular annotation was made.", 0, 1, time); 249 case 3556653: /*text*/ return new Property("text", "string", "The text of the annotation.", 0, 1, text); 250 default: return super.getNamedProperty(_hash, _name, _checkValid); 251 } 252 253 } 254 255 @Override 256 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 257 switch (hash) { 258 case -1406328437: /*author*/ return this.author == null ? new Base[0] : new Base[] {this.author}; // Type 259 case 3560141: /*time*/ return this.time == null ? new Base[0] : new Base[] {this.time}; // DateTimeType 260 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // StringType 261 default: return super.getProperty(hash, name, checkValid); 262 } 263 264 } 265 266 @Override 267 public Base setProperty(int hash, String name, Base value) throws FHIRException { 268 switch (hash) { 269 case -1406328437: // author 270 this.author = castToType(value); // Type 271 return value; 272 case 3560141: // time 273 this.time = castToDateTime(value); // DateTimeType 274 return value; 275 case 3556653: // text 276 this.text = castToString(value); // StringType 277 return value; 278 default: return super.setProperty(hash, name, value); 279 } 280 281 } 282 283 @Override 284 public Base setProperty(String name, Base value) throws FHIRException { 285 if (name.equals("author[x]")) { 286 this.author = castToType(value); // Type 287 } else if (name.equals("time")) { 288 this.time = castToDateTime(value); // DateTimeType 289 } else if (name.equals("text")) { 290 this.text = castToString(value); // StringType 291 } else 292 return super.setProperty(name, value); 293 return value; 294 } 295 296 @Override 297 public Base makeProperty(int hash, String name) throws FHIRException { 298 switch (hash) { 299 case 1475597077: return getAuthor(); 300 case -1406328437: return getAuthor(); 301 case 3560141: return getTimeElement(); 302 case 3556653: return getTextElement(); 303 default: return super.makeProperty(hash, name); 304 } 305 306 } 307 308 @Override 309 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 310 switch (hash) { 311 case -1406328437: /*author*/ return new String[] {"Reference", "string"}; 312 case 3560141: /*time*/ return new String[] {"dateTime"}; 313 case 3556653: /*text*/ return new String[] {"string"}; 314 default: return super.getTypesForProperty(hash, name); 315 } 316 317 } 318 319 @Override 320 public Base addChild(String name) throws FHIRException { 321 if (name.equals("authorReference")) { 322 this.author = new Reference(); 323 return this.author; 324 } 325 else if (name.equals("authorString")) { 326 this.author = new StringType(); 327 return this.author; 328 } 329 else if (name.equals("time")) { 330 throw new FHIRException("Cannot call addChild on a singleton property Annotation.time"); 331 } 332 else if (name.equals("text")) { 333 throw new FHIRException("Cannot call addChild on a singleton property Annotation.text"); 334 } 335 else 336 return super.addChild(name); 337 } 338 339 public String fhirType() { 340 return "Annotation"; 341 342 } 343 344 public Annotation copy() { 345 Annotation dst = new Annotation(); 346 copyValues(dst); 347 dst.author = author == null ? null : author.copy(); 348 dst.time = time == null ? null : time.copy(); 349 dst.text = text == null ? null : text.copy(); 350 return dst; 351 } 352 353 protected Annotation typedCopy() { 354 return copy(); 355 } 356 357 @Override 358 public boolean equalsDeep(Base other_) { 359 if (!super.equalsDeep(other_)) 360 return false; 361 if (!(other_ instanceof Annotation)) 362 return false; 363 Annotation o = (Annotation) other_; 364 return compareDeep(author, o.author, true) && compareDeep(time, o.time, true) && compareDeep(text, o.text, true) 365 ; 366 } 367 368 @Override 369 public boolean equalsShallow(Base other_) { 370 if (!super.equalsShallow(other_)) 371 return false; 372 if (!(other_ instanceof Annotation)) 373 return false; 374 Annotation o = (Annotation) other_; 375 return compareValues(time, o.time, true) && compareValues(text, o.text, true); 376 } 377 378 public boolean isEmpty() { 379 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(author, time, text); 380 } 381 382 383}