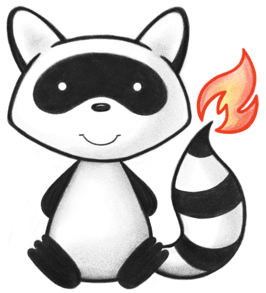
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048/** 049 * A booking of a healthcare event among patient(s), practitioner(s), related person(s) and/or device(s) for a specific date/time. This may result in one or more Encounter(s). 050 */ 051@ResourceDef(name="Appointment", profile="http://hl7.org/fhir/Profile/Appointment") 052public class Appointment extends DomainResource { 053 054 public enum AppointmentStatus { 055 /** 056 * None of the participant(s) have finalized their acceptance of the appointment request, and the start/end time may not be set yet. 057 */ 058 PROPOSED, 059 /** 060 * Some or all of the participant(s) have not finalized their acceptance of the appointment request. 061 */ 062 PENDING, 063 /** 064 * All participant(s) have been considered and the appointment is confirmed to go ahead at the date/times specified. 065 */ 066 BOOKED, 067 /** 068 * Some of the patients have arrived. 069 */ 070 ARRIVED, 071 /** 072 * This appointment has completed and may have resulted in an encounter. 073 */ 074 FULFILLED, 075 /** 076 * The appointment has been cancelled. 077 */ 078 CANCELLED, 079 /** 080 * Some or all of the participant(s) have not/did not appear for the appointment (usually the patient). 081 */ 082 NOSHOW, 083 /** 084 * This instance should not have been part of this patient's medical record. 085 */ 086 ENTEREDINERROR, 087 /** 088 * added to help the parsers with the generic types 089 */ 090 NULL; 091 public static AppointmentStatus fromCode(String codeString) throws FHIRException { 092 if (codeString == null || "".equals(codeString)) 093 return null; 094 if ("proposed".equals(codeString)) 095 return PROPOSED; 096 if ("pending".equals(codeString)) 097 return PENDING; 098 if ("booked".equals(codeString)) 099 return BOOKED; 100 if ("arrived".equals(codeString)) 101 return ARRIVED; 102 if ("fulfilled".equals(codeString)) 103 return FULFILLED; 104 if ("cancelled".equals(codeString)) 105 return CANCELLED; 106 if ("noshow".equals(codeString)) 107 return NOSHOW; 108 if ("entered-in-error".equals(codeString)) 109 return ENTEREDINERROR; 110 if (Configuration.isAcceptInvalidEnums()) 111 return null; 112 else 113 throw new FHIRException("Unknown AppointmentStatus code '"+codeString+"'"); 114 } 115 public String toCode() { 116 switch (this) { 117 case PROPOSED: return "proposed"; 118 case PENDING: return "pending"; 119 case BOOKED: return "booked"; 120 case ARRIVED: return "arrived"; 121 case FULFILLED: return "fulfilled"; 122 case CANCELLED: return "cancelled"; 123 case NOSHOW: return "noshow"; 124 case ENTEREDINERROR: return "entered-in-error"; 125 case NULL: return null; 126 default: return "?"; 127 } 128 } 129 public String getSystem() { 130 switch (this) { 131 case PROPOSED: return "http://hl7.org/fhir/appointmentstatus"; 132 case PENDING: return "http://hl7.org/fhir/appointmentstatus"; 133 case BOOKED: return "http://hl7.org/fhir/appointmentstatus"; 134 case ARRIVED: return "http://hl7.org/fhir/appointmentstatus"; 135 case FULFILLED: return "http://hl7.org/fhir/appointmentstatus"; 136 case CANCELLED: return "http://hl7.org/fhir/appointmentstatus"; 137 case NOSHOW: return "http://hl7.org/fhir/appointmentstatus"; 138 case ENTEREDINERROR: return "http://hl7.org/fhir/appointmentstatus"; 139 case NULL: return null; 140 default: return "?"; 141 } 142 } 143 public String getDefinition() { 144 switch (this) { 145 case PROPOSED: return "None of the participant(s) have finalized their acceptance of the appointment request, and the start/end time may not be set yet."; 146 case PENDING: return "Some or all of the participant(s) have not finalized their acceptance of the appointment request."; 147 case BOOKED: return "All participant(s) have been considered and the appointment is confirmed to go ahead at the date/times specified."; 148 case ARRIVED: return "Some of the patients have arrived."; 149 case FULFILLED: return "This appointment has completed and may have resulted in an encounter."; 150 case CANCELLED: return "The appointment has been cancelled."; 151 case NOSHOW: return "Some or all of the participant(s) have not/did not appear for the appointment (usually the patient)."; 152 case ENTEREDINERROR: return "This instance should not have been part of this patient's medical record."; 153 case NULL: return null; 154 default: return "?"; 155 } 156 } 157 public String getDisplay() { 158 switch (this) { 159 case PROPOSED: return "Proposed"; 160 case PENDING: return "Pending"; 161 case BOOKED: return "Booked"; 162 case ARRIVED: return "Arrived"; 163 case FULFILLED: return "Fulfilled"; 164 case CANCELLED: return "Cancelled"; 165 case NOSHOW: return "No Show"; 166 case ENTEREDINERROR: return "Entered in error"; 167 case NULL: return null; 168 default: return "?"; 169 } 170 } 171 } 172 173 public static class AppointmentStatusEnumFactory implements EnumFactory<AppointmentStatus> { 174 public AppointmentStatus fromCode(String codeString) throws IllegalArgumentException { 175 if (codeString == null || "".equals(codeString)) 176 if (codeString == null || "".equals(codeString)) 177 return null; 178 if ("proposed".equals(codeString)) 179 return AppointmentStatus.PROPOSED; 180 if ("pending".equals(codeString)) 181 return AppointmentStatus.PENDING; 182 if ("booked".equals(codeString)) 183 return AppointmentStatus.BOOKED; 184 if ("arrived".equals(codeString)) 185 return AppointmentStatus.ARRIVED; 186 if ("fulfilled".equals(codeString)) 187 return AppointmentStatus.FULFILLED; 188 if ("cancelled".equals(codeString)) 189 return AppointmentStatus.CANCELLED; 190 if ("noshow".equals(codeString)) 191 return AppointmentStatus.NOSHOW; 192 if ("entered-in-error".equals(codeString)) 193 return AppointmentStatus.ENTEREDINERROR; 194 throw new IllegalArgumentException("Unknown AppointmentStatus code '"+codeString+"'"); 195 } 196 public Enumeration<AppointmentStatus> fromType(PrimitiveType<?> code) throws FHIRException { 197 if (code == null) 198 return null; 199 if (code.isEmpty()) 200 return new Enumeration<AppointmentStatus>(this); 201 String codeString = code.asStringValue(); 202 if (codeString == null || "".equals(codeString)) 203 return null; 204 if ("proposed".equals(codeString)) 205 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.PROPOSED); 206 if ("pending".equals(codeString)) 207 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.PENDING); 208 if ("booked".equals(codeString)) 209 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.BOOKED); 210 if ("arrived".equals(codeString)) 211 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.ARRIVED); 212 if ("fulfilled".equals(codeString)) 213 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.FULFILLED); 214 if ("cancelled".equals(codeString)) 215 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.CANCELLED); 216 if ("noshow".equals(codeString)) 217 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.NOSHOW); 218 if ("entered-in-error".equals(codeString)) 219 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.ENTEREDINERROR); 220 throw new FHIRException("Unknown AppointmentStatus code '"+codeString+"'"); 221 } 222 public String toCode(AppointmentStatus code) { 223 if (code == AppointmentStatus.NULL) 224 return null; 225 if (code == AppointmentStatus.PROPOSED) 226 return "proposed"; 227 if (code == AppointmentStatus.PENDING) 228 return "pending"; 229 if (code == AppointmentStatus.BOOKED) 230 return "booked"; 231 if (code == AppointmentStatus.ARRIVED) 232 return "arrived"; 233 if (code == AppointmentStatus.FULFILLED) 234 return "fulfilled"; 235 if (code == AppointmentStatus.CANCELLED) 236 return "cancelled"; 237 if (code == AppointmentStatus.NOSHOW) 238 return "noshow"; 239 if (code == AppointmentStatus.ENTEREDINERROR) 240 return "entered-in-error"; 241 return "?"; 242 } 243 public String toSystem(AppointmentStatus code) { 244 return code.getSystem(); 245 } 246 } 247 248 public enum ParticipantRequired { 249 /** 250 * The participant is required to attend the appointment. 251 */ 252 REQUIRED, 253 /** 254 * The participant may optionally attend the appointment. 255 */ 256 OPTIONAL, 257 /** 258 * The participant is excluded from the appointment, and may not be informed of the appointment taking place. (Appointment is about them, not for them - such as 2 doctors discussing results about a patient's test). 259 */ 260 INFORMATIONONLY, 261 /** 262 * added to help the parsers with the generic types 263 */ 264 NULL; 265 public static ParticipantRequired fromCode(String codeString) throws FHIRException { 266 if (codeString == null || "".equals(codeString)) 267 return null; 268 if ("required".equals(codeString)) 269 return REQUIRED; 270 if ("optional".equals(codeString)) 271 return OPTIONAL; 272 if ("information-only".equals(codeString)) 273 return INFORMATIONONLY; 274 if (Configuration.isAcceptInvalidEnums()) 275 return null; 276 else 277 throw new FHIRException("Unknown ParticipantRequired code '"+codeString+"'"); 278 } 279 public String toCode() { 280 switch (this) { 281 case REQUIRED: return "required"; 282 case OPTIONAL: return "optional"; 283 case INFORMATIONONLY: return "information-only"; 284 case NULL: return null; 285 default: return "?"; 286 } 287 } 288 public String getSystem() { 289 switch (this) { 290 case REQUIRED: return "http://hl7.org/fhir/participantrequired"; 291 case OPTIONAL: return "http://hl7.org/fhir/participantrequired"; 292 case INFORMATIONONLY: return "http://hl7.org/fhir/participantrequired"; 293 case NULL: return null; 294 default: return "?"; 295 } 296 } 297 public String getDefinition() { 298 switch (this) { 299 case REQUIRED: return "The participant is required to attend the appointment."; 300 case OPTIONAL: return "The participant may optionally attend the appointment."; 301 case INFORMATIONONLY: return "The participant is excluded from the appointment, and may not be informed of the appointment taking place. (Appointment is about them, not for them - such as 2 doctors discussing results about a patient's test)."; 302 case NULL: return null; 303 default: return "?"; 304 } 305 } 306 public String getDisplay() { 307 switch (this) { 308 case REQUIRED: return "Required"; 309 case OPTIONAL: return "Optional"; 310 case INFORMATIONONLY: return "Information Only"; 311 case NULL: return null; 312 default: return "?"; 313 } 314 } 315 } 316 317 public static class ParticipantRequiredEnumFactory implements EnumFactory<ParticipantRequired> { 318 public ParticipantRequired fromCode(String codeString) throws IllegalArgumentException { 319 if (codeString == null || "".equals(codeString)) 320 if (codeString == null || "".equals(codeString)) 321 return null; 322 if ("required".equals(codeString)) 323 return ParticipantRequired.REQUIRED; 324 if ("optional".equals(codeString)) 325 return ParticipantRequired.OPTIONAL; 326 if ("information-only".equals(codeString)) 327 return ParticipantRequired.INFORMATIONONLY; 328 throw new IllegalArgumentException("Unknown ParticipantRequired code '"+codeString+"'"); 329 } 330 public Enumeration<ParticipantRequired> fromType(PrimitiveType<?> code) throws FHIRException { 331 if (code == null) 332 return null; 333 if (code.isEmpty()) 334 return new Enumeration<ParticipantRequired>(this); 335 String codeString = code.asStringValue(); 336 if (codeString == null || "".equals(codeString)) 337 return null; 338 if ("required".equals(codeString)) 339 return new Enumeration<ParticipantRequired>(this, ParticipantRequired.REQUIRED); 340 if ("optional".equals(codeString)) 341 return new Enumeration<ParticipantRequired>(this, ParticipantRequired.OPTIONAL); 342 if ("information-only".equals(codeString)) 343 return new Enumeration<ParticipantRequired>(this, ParticipantRequired.INFORMATIONONLY); 344 throw new FHIRException("Unknown ParticipantRequired code '"+codeString+"'"); 345 } 346 public String toCode(ParticipantRequired code) { 347 if (code == ParticipantRequired.NULL) 348 return null; 349 if (code == ParticipantRequired.REQUIRED) 350 return "required"; 351 if (code == ParticipantRequired.OPTIONAL) 352 return "optional"; 353 if (code == ParticipantRequired.INFORMATIONONLY) 354 return "information-only"; 355 return "?"; 356 } 357 public String toSystem(ParticipantRequired code) { 358 return code.getSystem(); 359 } 360 } 361 362 public enum ParticipationStatus { 363 /** 364 * The participant has accepted the appointment. 365 */ 366 ACCEPTED, 367 /** 368 * The participant has declined the appointment and will not participate in the appointment. 369 */ 370 DECLINED, 371 /** 372 * The participant has tentatively accepted the appointment. This could be automatically created by a system and requires further processing before it can be accepted. There is no commitment that attendance will occur. 373 */ 374 TENTATIVE, 375 /** 376 * The participant needs to indicate if they accept the appointment by changing this status to one of the other statuses. 377 */ 378 NEEDSACTION, 379 /** 380 * added to help the parsers with the generic types 381 */ 382 NULL; 383 public static ParticipationStatus fromCode(String codeString) throws FHIRException { 384 if (codeString == null || "".equals(codeString)) 385 return null; 386 if ("accepted".equals(codeString)) 387 return ACCEPTED; 388 if ("declined".equals(codeString)) 389 return DECLINED; 390 if ("tentative".equals(codeString)) 391 return TENTATIVE; 392 if ("needs-action".equals(codeString)) 393 return NEEDSACTION; 394 if (Configuration.isAcceptInvalidEnums()) 395 return null; 396 else 397 throw new FHIRException("Unknown ParticipationStatus code '"+codeString+"'"); 398 } 399 public String toCode() { 400 switch (this) { 401 case ACCEPTED: return "accepted"; 402 case DECLINED: return "declined"; 403 case TENTATIVE: return "tentative"; 404 case NEEDSACTION: return "needs-action"; 405 case NULL: return null; 406 default: return "?"; 407 } 408 } 409 public String getSystem() { 410 switch (this) { 411 case ACCEPTED: return "http://hl7.org/fhir/participationstatus"; 412 case DECLINED: return "http://hl7.org/fhir/participationstatus"; 413 case TENTATIVE: return "http://hl7.org/fhir/participationstatus"; 414 case NEEDSACTION: return "http://hl7.org/fhir/participationstatus"; 415 case NULL: return null; 416 default: return "?"; 417 } 418 } 419 public String getDefinition() { 420 switch (this) { 421 case ACCEPTED: return "The participant has accepted the appointment."; 422 case DECLINED: return "The participant has declined the appointment and will not participate in the appointment."; 423 case TENTATIVE: return "The participant has tentatively accepted the appointment. This could be automatically created by a system and requires further processing before it can be accepted. There is no commitment that attendance will occur."; 424 case NEEDSACTION: return "The participant needs to indicate if they accept the appointment by changing this status to one of the other statuses."; 425 case NULL: return null; 426 default: return "?"; 427 } 428 } 429 public String getDisplay() { 430 switch (this) { 431 case ACCEPTED: return "Accepted"; 432 case DECLINED: return "Declined"; 433 case TENTATIVE: return "Tentative"; 434 case NEEDSACTION: return "Needs Action"; 435 case NULL: return null; 436 default: return "?"; 437 } 438 } 439 } 440 441 public static class ParticipationStatusEnumFactory implements EnumFactory<ParticipationStatus> { 442 public ParticipationStatus fromCode(String codeString) throws IllegalArgumentException { 443 if (codeString == null || "".equals(codeString)) 444 if (codeString == null || "".equals(codeString)) 445 return null; 446 if ("accepted".equals(codeString)) 447 return ParticipationStatus.ACCEPTED; 448 if ("declined".equals(codeString)) 449 return ParticipationStatus.DECLINED; 450 if ("tentative".equals(codeString)) 451 return ParticipationStatus.TENTATIVE; 452 if ("needs-action".equals(codeString)) 453 return ParticipationStatus.NEEDSACTION; 454 throw new IllegalArgumentException("Unknown ParticipationStatus code '"+codeString+"'"); 455 } 456 public Enumeration<ParticipationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 457 if (code == null) 458 return null; 459 if (code.isEmpty()) 460 return new Enumeration<ParticipationStatus>(this); 461 String codeString = code.asStringValue(); 462 if (codeString == null || "".equals(codeString)) 463 return null; 464 if ("accepted".equals(codeString)) 465 return new Enumeration<ParticipationStatus>(this, ParticipationStatus.ACCEPTED); 466 if ("declined".equals(codeString)) 467 return new Enumeration<ParticipationStatus>(this, ParticipationStatus.DECLINED); 468 if ("tentative".equals(codeString)) 469 return new Enumeration<ParticipationStatus>(this, ParticipationStatus.TENTATIVE); 470 if ("needs-action".equals(codeString)) 471 return new Enumeration<ParticipationStatus>(this, ParticipationStatus.NEEDSACTION); 472 throw new FHIRException("Unknown ParticipationStatus code '"+codeString+"'"); 473 } 474 public String toCode(ParticipationStatus code) { 475 if (code == ParticipationStatus.NULL) 476 return null; 477 if (code == ParticipationStatus.ACCEPTED) 478 return "accepted"; 479 if (code == ParticipationStatus.DECLINED) 480 return "declined"; 481 if (code == ParticipationStatus.TENTATIVE) 482 return "tentative"; 483 if (code == ParticipationStatus.NEEDSACTION) 484 return "needs-action"; 485 return "?"; 486 } 487 public String toSystem(ParticipationStatus code) { 488 return code.getSystem(); 489 } 490 } 491 492 @Block() 493 public static class AppointmentParticipantComponent extends BackboneElement implements IBaseBackboneElement { 494 /** 495 * Role of participant in the appointment. 496 */ 497 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 498 @Description(shortDefinition="Role of participant in the appointment", formalDefinition="Role of participant in the appointment." ) 499 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-participant-type") 500 protected List<CodeableConcept> type; 501 502 /** 503 * A Person, Location/HealthcareService or Device that is participating in the appointment. 504 */ 505 @Child(name = "actor", type = {Patient.class, Practitioner.class, RelatedPerson.class, Device.class, HealthcareService.class, Location.class}, order=2, min=0, max=1, modifier=false, summary=true) 506 @Description(shortDefinition="Person, Location/HealthcareService or Device", formalDefinition="A Person, Location/HealthcareService or Device that is participating in the appointment." ) 507 protected Reference actor; 508 509 /** 510 * The actual object that is the target of the reference (A Person, Location/HealthcareService or Device that is participating in the appointment.) 511 */ 512 protected Resource actorTarget; 513 514 /** 515 * Is this participant required to be present at the meeting. This covers a use-case where 2 doctors need to meet to discuss the results for a specific patient, and the patient is not required to be present. 516 */ 517 @Child(name = "required", type = {CodeType.class}, order=3, min=0, max=1, modifier=false, summary=true) 518 @Description(shortDefinition="required | optional | information-only", formalDefinition="Is this participant required to be present at the meeting. This covers a use-case where 2 doctors need to meet to discuss the results for a specific patient, and the patient is not required to be present." ) 519 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/participantrequired") 520 protected Enumeration<ParticipantRequired> required; 521 522 /** 523 * Participation status of the actor. 524 */ 525 @Child(name = "status", type = {CodeType.class}, order=4, min=1, max=1, modifier=false, summary=false) 526 @Description(shortDefinition="accepted | declined | tentative | needs-action", formalDefinition="Participation status of the actor." ) 527 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/participationstatus") 528 protected Enumeration<ParticipationStatus> status; 529 530 private static final long serialVersionUID = -1620552507L; 531 532 /** 533 * Constructor 534 */ 535 public AppointmentParticipantComponent() { 536 super(); 537 } 538 539 /** 540 * Constructor 541 */ 542 public AppointmentParticipantComponent(Enumeration<ParticipationStatus> status) { 543 super(); 544 this.status = status; 545 } 546 547 /** 548 * @return {@link #type} (Role of participant in the appointment.) 549 */ 550 public List<CodeableConcept> getType() { 551 if (this.type == null) 552 this.type = new ArrayList<CodeableConcept>(); 553 return this.type; 554 } 555 556 /** 557 * @return Returns a reference to <code>this</code> for easy method chaining 558 */ 559 public AppointmentParticipantComponent setType(List<CodeableConcept> theType) { 560 this.type = theType; 561 return this; 562 } 563 564 public boolean hasType() { 565 if (this.type == null) 566 return false; 567 for (CodeableConcept item : this.type) 568 if (!item.isEmpty()) 569 return true; 570 return false; 571 } 572 573 public CodeableConcept addType() { //3 574 CodeableConcept t = new CodeableConcept(); 575 if (this.type == null) 576 this.type = new ArrayList<CodeableConcept>(); 577 this.type.add(t); 578 return t; 579 } 580 581 public AppointmentParticipantComponent addType(CodeableConcept t) { //3 582 if (t == null) 583 return this; 584 if (this.type == null) 585 this.type = new ArrayList<CodeableConcept>(); 586 this.type.add(t); 587 return this; 588 } 589 590 /** 591 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist 592 */ 593 public CodeableConcept getTypeFirstRep() { 594 if (getType().isEmpty()) { 595 addType(); 596 } 597 return getType().get(0); 598 } 599 600 /** 601 * @return {@link #actor} (A Person, Location/HealthcareService or Device that is participating in the appointment.) 602 */ 603 public Reference getActor() { 604 if (this.actor == null) 605 if (Configuration.errorOnAutoCreate()) 606 throw new Error("Attempt to auto-create AppointmentParticipantComponent.actor"); 607 else if (Configuration.doAutoCreate()) 608 this.actor = new Reference(); // cc 609 return this.actor; 610 } 611 612 public boolean hasActor() { 613 return this.actor != null && !this.actor.isEmpty(); 614 } 615 616 /** 617 * @param value {@link #actor} (A Person, Location/HealthcareService or Device that is participating in the appointment.) 618 */ 619 public AppointmentParticipantComponent setActor(Reference value) { 620 this.actor = value; 621 return this; 622 } 623 624 /** 625 * @return {@link #actor} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A Person, Location/HealthcareService or Device that is participating in the appointment.) 626 */ 627 public Resource getActorTarget() { 628 return this.actorTarget; 629 } 630 631 /** 632 * @param value {@link #actor} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A Person, Location/HealthcareService or Device that is participating in the appointment.) 633 */ 634 public AppointmentParticipantComponent setActorTarget(Resource value) { 635 this.actorTarget = value; 636 return this; 637 } 638 639 /** 640 * @return {@link #required} (Is this participant required to be present at the meeting. This covers a use-case where 2 doctors need to meet to discuss the results for a specific patient, and the patient is not required to be present.). This is the underlying object with id, value and extensions. The accessor "getRequired" gives direct access to the value 641 */ 642 public Enumeration<ParticipantRequired> getRequiredElement() { 643 if (this.required == null) 644 if (Configuration.errorOnAutoCreate()) 645 throw new Error("Attempt to auto-create AppointmentParticipantComponent.required"); 646 else if (Configuration.doAutoCreate()) 647 this.required = new Enumeration<ParticipantRequired>(new ParticipantRequiredEnumFactory()); // bb 648 return this.required; 649 } 650 651 public boolean hasRequiredElement() { 652 return this.required != null && !this.required.isEmpty(); 653 } 654 655 public boolean hasRequired() { 656 return this.required != null && !this.required.isEmpty(); 657 } 658 659 /** 660 * @param value {@link #required} (Is this participant required to be present at the meeting. This covers a use-case where 2 doctors need to meet to discuss the results for a specific patient, and the patient is not required to be present.). This is the underlying object with id, value and extensions. The accessor "getRequired" gives direct access to the value 661 */ 662 public AppointmentParticipantComponent setRequiredElement(Enumeration<ParticipantRequired> value) { 663 this.required = value; 664 return this; 665 } 666 667 /** 668 * @return Is this participant required to be present at the meeting. This covers a use-case where 2 doctors need to meet to discuss the results for a specific patient, and the patient is not required to be present. 669 */ 670 public ParticipantRequired getRequired() { 671 return this.required == null ? null : this.required.getValue(); 672 } 673 674 /** 675 * @param value Is this participant required to be present at the meeting. This covers a use-case where 2 doctors need to meet to discuss the results for a specific patient, and the patient is not required to be present. 676 */ 677 public AppointmentParticipantComponent setRequired(ParticipantRequired value) { 678 if (value == null) 679 this.required = null; 680 else { 681 if (this.required == null) 682 this.required = new Enumeration<ParticipantRequired>(new ParticipantRequiredEnumFactory()); 683 this.required.setValue(value); 684 } 685 return this; 686 } 687 688 /** 689 * @return {@link #status} (Participation status of the actor.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 690 */ 691 public Enumeration<ParticipationStatus> getStatusElement() { 692 if (this.status == null) 693 if (Configuration.errorOnAutoCreate()) 694 throw new Error("Attempt to auto-create AppointmentParticipantComponent.status"); 695 else if (Configuration.doAutoCreate()) 696 this.status = new Enumeration<ParticipationStatus>(new ParticipationStatusEnumFactory()); // bb 697 return this.status; 698 } 699 700 public boolean hasStatusElement() { 701 return this.status != null && !this.status.isEmpty(); 702 } 703 704 public boolean hasStatus() { 705 return this.status != null && !this.status.isEmpty(); 706 } 707 708 /** 709 * @param value {@link #status} (Participation status of the actor.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 710 */ 711 public AppointmentParticipantComponent setStatusElement(Enumeration<ParticipationStatus> value) { 712 this.status = value; 713 return this; 714 } 715 716 /** 717 * @return Participation status of the actor. 718 */ 719 public ParticipationStatus getStatus() { 720 return this.status == null ? null : this.status.getValue(); 721 } 722 723 /** 724 * @param value Participation status of the actor. 725 */ 726 public AppointmentParticipantComponent setStatus(ParticipationStatus value) { 727 if (this.status == null) 728 this.status = new Enumeration<ParticipationStatus>(new ParticipationStatusEnumFactory()); 729 this.status.setValue(value); 730 return this; 731 } 732 733 protected void listChildren(List<Property> children) { 734 super.listChildren(children); 735 children.add(new Property("type", "CodeableConcept", "Role of participant in the appointment.", 0, java.lang.Integer.MAX_VALUE, type)); 736 children.add(new Property("actor", "Reference(Patient|Practitioner|RelatedPerson|Device|HealthcareService|Location)", "A Person, Location/HealthcareService or Device that is participating in the appointment.", 0, 1, actor)); 737 children.add(new Property("required", "code", "Is this participant required to be present at the meeting. This covers a use-case where 2 doctors need to meet to discuss the results for a specific patient, and the patient is not required to be present.", 0, 1, required)); 738 children.add(new Property("status", "code", "Participation status of the actor.", 0, 1, status)); 739 } 740 741 @Override 742 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 743 switch (_hash) { 744 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Role of participant in the appointment.", 0, java.lang.Integer.MAX_VALUE, type); 745 case 92645877: /*actor*/ return new Property("actor", "Reference(Patient|Practitioner|RelatedPerson|Device|HealthcareService|Location)", "A Person, Location/HealthcareService or Device that is participating in the appointment.", 0, 1, actor); 746 case -393139297: /*required*/ return new Property("required", "code", "Is this participant required to be present at the meeting. This covers a use-case where 2 doctors need to meet to discuss the results for a specific patient, and the patient is not required to be present.", 0, 1, required); 747 case -892481550: /*status*/ return new Property("status", "code", "Participation status of the actor.", 0, 1, status); 748 default: return super.getNamedProperty(_hash, _name, _checkValid); 749 } 750 751 } 752 753 @Override 754 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 755 switch (hash) { 756 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 757 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // Reference 758 case -393139297: /*required*/ return this.required == null ? new Base[0] : new Base[] {this.required}; // Enumeration<ParticipantRequired> 759 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ParticipationStatus> 760 default: return super.getProperty(hash, name, checkValid); 761 } 762 763 } 764 765 @Override 766 public Base setProperty(int hash, String name, Base value) throws FHIRException { 767 switch (hash) { 768 case 3575610: // type 769 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 770 return value; 771 case 92645877: // actor 772 this.actor = castToReference(value); // Reference 773 return value; 774 case -393139297: // required 775 value = new ParticipantRequiredEnumFactory().fromType(castToCode(value)); 776 this.required = (Enumeration) value; // Enumeration<ParticipantRequired> 777 return value; 778 case -892481550: // status 779 value = new ParticipationStatusEnumFactory().fromType(castToCode(value)); 780 this.status = (Enumeration) value; // Enumeration<ParticipationStatus> 781 return value; 782 default: return super.setProperty(hash, name, value); 783 } 784 785 } 786 787 @Override 788 public Base setProperty(String name, Base value) throws FHIRException { 789 if (name.equals("type")) { 790 this.getType().add(castToCodeableConcept(value)); 791 } else if (name.equals("actor")) { 792 this.actor = castToReference(value); // Reference 793 } else if (name.equals("required")) { 794 value = new ParticipantRequiredEnumFactory().fromType(castToCode(value)); 795 this.required = (Enumeration) value; // Enumeration<ParticipantRequired> 796 } else if (name.equals("status")) { 797 value = new ParticipationStatusEnumFactory().fromType(castToCode(value)); 798 this.status = (Enumeration) value; // Enumeration<ParticipationStatus> 799 } else 800 return super.setProperty(name, value); 801 return value; 802 } 803 804 @Override 805 public Base makeProperty(int hash, String name) throws FHIRException { 806 switch (hash) { 807 case 3575610: return addType(); 808 case 92645877: return getActor(); 809 case -393139297: return getRequiredElement(); 810 case -892481550: return getStatusElement(); 811 default: return super.makeProperty(hash, name); 812 } 813 814 } 815 816 @Override 817 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 818 switch (hash) { 819 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 820 case 92645877: /*actor*/ return new String[] {"Reference"}; 821 case -393139297: /*required*/ return new String[] {"code"}; 822 case -892481550: /*status*/ return new String[] {"code"}; 823 default: return super.getTypesForProperty(hash, name); 824 } 825 826 } 827 828 @Override 829 public Base addChild(String name) throws FHIRException { 830 if (name.equals("type")) { 831 return addType(); 832 } 833 else if (name.equals("actor")) { 834 this.actor = new Reference(); 835 return this.actor; 836 } 837 else if (name.equals("required")) { 838 throw new FHIRException("Cannot call addChild on a singleton property Appointment.required"); 839 } 840 else if (name.equals("status")) { 841 throw new FHIRException("Cannot call addChild on a singleton property Appointment.status"); 842 } 843 else 844 return super.addChild(name); 845 } 846 847 public AppointmentParticipantComponent copy() { 848 AppointmentParticipantComponent dst = new AppointmentParticipantComponent(); 849 copyValues(dst); 850 if (type != null) { 851 dst.type = new ArrayList<CodeableConcept>(); 852 for (CodeableConcept i : type) 853 dst.type.add(i.copy()); 854 }; 855 dst.actor = actor == null ? null : actor.copy(); 856 dst.required = required == null ? null : required.copy(); 857 dst.status = status == null ? null : status.copy(); 858 return dst; 859 } 860 861 @Override 862 public boolean equalsDeep(Base other_) { 863 if (!super.equalsDeep(other_)) 864 return false; 865 if (!(other_ instanceof AppointmentParticipantComponent)) 866 return false; 867 AppointmentParticipantComponent o = (AppointmentParticipantComponent) other_; 868 return compareDeep(type, o.type, true) && compareDeep(actor, o.actor, true) && compareDeep(required, o.required, true) 869 && compareDeep(status, o.status, true); 870 } 871 872 @Override 873 public boolean equalsShallow(Base other_) { 874 if (!super.equalsShallow(other_)) 875 return false; 876 if (!(other_ instanceof AppointmentParticipantComponent)) 877 return false; 878 AppointmentParticipantComponent o = (AppointmentParticipantComponent) other_; 879 return compareValues(required, o.required, true) && compareValues(status, o.status, true); 880 } 881 882 public boolean isEmpty() { 883 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, actor, required, status 884 ); 885 } 886 887 public String fhirType() { 888 return "Appointment.participant"; 889 890 } 891 892 } 893 894 /** 895 * This records identifiers associated with this appointment concern that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation). 896 */ 897 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 898 @Description(shortDefinition="External Ids for this item", formalDefinition="This records identifiers associated with this appointment concern that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation)." ) 899 protected List<Identifier> identifier; 900 901 /** 902 * The overall status of the Appointment. Each of the participants has their own participation status which indicates their involvement in the process, however this status indicates the shared status. 903 */ 904 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 905 @Description(shortDefinition="proposed | pending | booked | arrived | fulfilled | cancelled | noshow | entered-in-error", formalDefinition="The overall status of the Appointment. Each of the participants has their own participation status which indicates their involvement in the process, however this status indicates the shared status." ) 906 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/appointmentstatus") 907 protected Enumeration<AppointmentStatus> status; 908 909 /** 910 * A broad categorisation of the service that is to be performed during this appointment. 911 */ 912 @Child(name = "serviceCategory", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 913 @Description(shortDefinition="A broad categorisation of the service that is to be performed during this appointment", formalDefinition="A broad categorisation of the service that is to be performed during this appointment." ) 914 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-category") 915 protected CodeableConcept serviceCategory; 916 917 /** 918 * The specific service that is to be performed during this appointment. 919 */ 920 @Child(name = "serviceType", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 921 @Description(shortDefinition="The specific service that is to be performed during this appointment", formalDefinition="The specific service that is to be performed during this appointment." ) 922 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-type") 923 protected List<CodeableConcept> serviceType; 924 925 /** 926 * The specialty of a practitioner that would be required to perform the service requested in this appointment. 927 */ 928 @Child(name = "specialty", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 929 @Description(shortDefinition="The specialty of a practitioner that would be required to perform the service requested in this appointment", formalDefinition="The specialty of a practitioner that would be required to perform the service requested in this appointment." ) 930 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/c80-practice-codes") 931 protected List<CodeableConcept> specialty; 932 933 /** 934 * The style of appointment or patient that has been booked in the slot (not service type). 935 */ 936 @Child(name = "appointmentType", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=true) 937 @Description(shortDefinition="The style of appointment or patient that has been booked in the slot (not service type)", formalDefinition="The style of appointment or patient that has been booked in the slot (not service type)." ) 938 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v2-0276") 939 protected CodeableConcept appointmentType; 940 941 /** 942 * The reason that this appointment is being scheduled. This is more clinical than administrative. 943 */ 944 @Child(name = "reason", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 945 @Description(shortDefinition="Reason this appointment is scheduled", formalDefinition="The reason that this appointment is being scheduled. This is more clinical than administrative." ) 946 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-reason") 947 protected List<CodeableConcept> reason; 948 949 /** 950 * Reason the appointment has been scheduled to take place, as specified using information from another resource. When the patient arrives and the encounter begins it may be used as the admission diagnosis. The indication will typically be a Condition (with other resources referenced in the evidence.detail), or a Procedure. 951 */ 952 @Child(name = "indication", type = {Condition.class, Procedure.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 953 @Description(shortDefinition="Reason the appointment is to takes place (resource)", formalDefinition="Reason the appointment has been scheduled to take place, as specified using information from another resource. When the patient arrives and the encounter begins it may be used as the admission diagnosis. The indication will typically be a Condition (with other resources referenced in the evidence.detail), or a Procedure." ) 954 protected List<Reference> indication; 955 /** 956 * The actual objects that are the target of the reference (Reason the appointment has been scheduled to take place, as specified using information from another resource. When the patient arrives and the encounter begins it may be used as the admission diagnosis. The indication will typically be a Condition (with other resources referenced in the evidence.detail), or a Procedure.) 957 */ 958 protected List<Resource> indicationTarget; 959 960 961 /** 962 * The priority of the appointment. Can be used to make informed decisions if needing to re-prioritize appointments. (The iCal Standard specifies 0 as undefined, 1 as highest, 9 as lowest priority). 963 */ 964 @Child(name = "priority", type = {UnsignedIntType.class}, order=8, min=0, max=1, modifier=false, summary=false) 965 @Description(shortDefinition="Used to make informed decisions if needing to re-prioritize", formalDefinition="The priority of the appointment. Can be used to make informed decisions if needing to re-prioritize appointments. (The iCal Standard specifies 0 as undefined, 1 as highest, 9 as lowest priority)." ) 966 protected UnsignedIntType priority; 967 968 /** 969 * The brief description of the appointment as would be shown on a subject line in a meeting request, or appointment list. Detailed or expanded information should be put in the comment field. 970 */ 971 @Child(name = "description", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=false) 972 @Description(shortDefinition="Shown on a subject line in a meeting request, or appointment list", formalDefinition="The brief description of the appointment as would be shown on a subject line in a meeting request, or appointment list. Detailed or expanded information should be put in the comment field." ) 973 protected StringType description; 974 975 /** 976 * Additional information to support the appointment provided when making the appointment. 977 */ 978 @Child(name = "supportingInformation", type = {Reference.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 979 @Description(shortDefinition="Additional information to support the appointment", formalDefinition="Additional information to support the appointment provided when making the appointment." ) 980 protected List<Reference> supportingInformation; 981 /** 982 * The actual objects that are the target of the reference (Additional information to support the appointment provided when making the appointment.) 983 */ 984 protected List<Resource> supportingInformationTarget; 985 986 987 /** 988 * Date/Time that the appointment is to take place. 989 */ 990 @Child(name = "start", type = {InstantType.class}, order=11, min=0, max=1, modifier=false, summary=true) 991 @Description(shortDefinition="When appointment is to take place", formalDefinition="Date/Time that the appointment is to take place." ) 992 protected InstantType start; 993 994 /** 995 * Date/Time that the appointment is to conclude. 996 */ 997 @Child(name = "end", type = {InstantType.class}, order=12, min=0, max=1, modifier=false, summary=true) 998 @Description(shortDefinition="When appointment is to conclude", formalDefinition="Date/Time that the appointment is to conclude." ) 999 protected InstantType end; 1000 1001 /** 1002 * Number of minutes that the appointment is to take. This can be less than the duration between the start and end times (where actual time of appointment is only an estimate or is a planned appointment request). 1003 */ 1004 @Child(name = "minutesDuration", type = {PositiveIntType.class}, order=13, min=0, max=1, modifier=false, summary=false) 1005 @Description(shortDefinition="Can be less than start/end (e.g. estimate)", formalDefinition="Number of minutes that the appointment is to take. This can be less than the duration between the start and end times (where actual time of appointment is only an estimate or is a planned appointment request)." ) 1006 protected PositiveIntType minutesDuration; 1007 1008 /** 1009 * The slots from the participants' schedules that will be filled by the appointment. 1010 */ 1011 @Child(name = "slot", type = {Slot.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1012 @Description(shortDefinition="The slots that this appointment is filling", formalDefinition="The slots from the participants' schedules that will be filled by the appointment." ) 1013 protected List<Reference> slot; 1014 /** 1015 * The actual objects that are the target of the reference (The slots from the participants' schedules that will be filled by the appointment.) 1016 */ 1017 protected List<Slot> slotTarget; 1018 1019 1020 /** 1021 * The date that this appointment was initially created. This could be different to the meta.lastModified value on the initial entry, as this could have been before the resource was created on the FHIR server, and should remain unchanged over the lifespan of the appointment. 1022 */ 1023 @Child(name = "created", type = {DateTimeType.class}, order=15, min=0, max=1, modifier=false, summary=false) 1024 @Description(shortDefinition="The date that this appointment was initially created", formalDefinition="The date that this appointment was initially created. This could be different to the meta.lastModified value on the initial entry, as this could have been before the resource was created on the FHIR server, and should remain unchanged over the lifespan of the appointment." ) 1025 protected DateTimeType created; 1026 1027 /** 1028 * Additional comments about the appointment. 1029 */ 1030 @Child(name = "comment", type = {StringType.class}, order=16, min=0, max=1, modifier=false, summary=false) 1031 @Description(shortDefinition="Additional comments", formalDefinition="Additional comments about the appointment." ) 1032 protected StringType comment; 1033 1034 /** 1035 * The referral request this appointment is allocated to assess (incoming referral). 1036 */ 1037 @Child(name = "incomingReferral", type = {ReferralRequest.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1038 @Description(shortDefinition="The ReferralRequest provided as information to allocate to the Encounter", formalDefinition="The referral request this appointment is allocated to assess (incoming referral)." ) 1039 protected List<Reference> incomingReferral; 1040 /** 1041 * The actual objects that are the target of the reference (The referral request this appointment is allocated to assess (incoming referral).) 1042 */ 1043 protected List<ReferralRequest> incomingReferralTarget; 1044 1045 1046 /** 1047 * List of participants involved in the appointment. 1048 */ 1049 @Child(name = "participant", type = {}, order=18, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1050 @Description(shortDefinition="Participants involved in appointment", formalDefinition="List of participants involved in the appointment." ) 1051 protected List<AppointmentParticipantComponent> participant; 1052 1053 /** 1054 * A set of date ranges (potentially including times) that the appointment is preferred to be scheduled within. When using these values, the minutes duration should be provided to indicate the length of the appointment to fill and populate the start/end times for the actual allocated time. 1055 */ 1056 @Child(name = "requestedPeriod", type = {Period.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1057 @Description(shortDefinition="Potential date/time interval(s) requested to allocate the appointment within", formalDefinition="A set of date ranges (potentially including times) that the appointment is preferred to be scheduled within. When using these values, the minutes duration should be provided to indicate the length of the appointment to fill and populate the start/end times for the actual allocated time." ) 1058 protected List<Period> requestedPeriod; 1059 1060 private static final long serialVersionUID = -1589372810L; 1061 1062 /** 1063 * Constructor 1064 */ 1065 public Appointment() { 1066 super(); 1067 } 1068 1069 /** 1070 * Constructor 1071 */ 1072 public Appointment(Enumeration<AppointmentStatus> status) { 1073 super(); 1074 this.status = status; 1075 } 1076 1077 /** 1078 * @return {@link #identifier} (This records identifiers associated with this appointment concern that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).) 1079 */ 1080 public List<Identifier> getIdentifier() { 1081 if (this.identifier == null) 1082 this.identifier = new ArrayList<Identifier>(); 1083 return this.identifier; 1084 } 1085 1086 /** 1087 * @return Returns a reference to <code>this</code> for easy method chaining 1088 */ 1089 public Appointment setIdentifier(List<Identifier> theIdentifier) { 1090 this.identifier = theIdentifier; 1091 return this; 1092 } 1093 1094 public boolean hasIdentifier() { 1095 if (this.identifier == null) 1096 return false; 1097 for (Identifier item : this.identifier) 1098 if (!item.isEmpty()) 1099 return true; 1100 return false; 1101 } 1102 1103 public Identifier addIdentifier() { //3 1104 Identifier t = new Identifier(); 1105 if (this.identifier == null) 1106 this.identifier = new ArrayList<Identifier>(); 1107 this.identifier.add(t); 1108 return t; 1109 } 1110 1111 public Appointment addIdentifier(Identifier t) { //3 1112 if (t == null) 1113 return this; 1114 if (this.identifier == null) 1115 this.identifier = new ArrayList<Identifier>(); 1116 this.identifier.add(t); 1117 return this; 1118 } 1119 1120 /** 1121 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 1122 */ 1123 public Identifier getIdentifierFirstRep() { 1124 if (getIdentifier().isEmpty()) { 1125 addIdentifier(); 1126 } 1127 return getIdentifier().get(0); 1128 } 1129 1130 /** 1131 * @return {@link #status} (The overall status of the Appointment. Each of the participants has their own participation status which indicates their involvement in the process, however this status indicates the shared status.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1132 */ 1133 public Enumeration<AppointmentStatus> getStatusElement() { 1134 if (this.status == null) 1135 if (Configuration.errorOnAutoCreate()) 1136 throw new Error("Attempt to auto-create Appointment.status"); 1137 else if (Configuration.doAutoCreate()) 1138 this.status = new Enumeration<AppointmentStatus>(new AppointmentStatusEnumFactory()); // bb 1139 return this.status; 1140 } 1141 1142 public boolean hasStatusElement() { 1143 return this.status != null && !this.status.isEmpty(); 1144 } 1145 1146 public boolean hasStatus() { 1147 return this.status != null && !this.status.isEmpty(); 1148 } 1149 1150 /** 1151 * @param value {@link #status} (The overall status of the Appointment. Each of the participants has their own participation status which indicates their involvement in the process, however this status indicates the shared status.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1152 */ 1153 public Appointment setStatusElement(Enumeration<AppointmentStatus> value) { 1154 this.status = value; 1155 return this; 1156 } 1157 1158 /** 1159 * @return The overall status of the Appointment. Each of the participants has their own participation status which indicates their involvement in the process, however this status indicates the shared status. 1160 */ 1161 public AppointmentStatus getStatus() { 1162 return this.status == null ? null : this.status.getValue(); 1163 } 1164 1165 /** 1166 * @param value The overall status of the Appointment. Each of the participants has their own participation status which indicates their involvement in the process, however this status indicates the shared status. 1167 */ 1168 public Appointment setStatus(AppointmentStatus value) { 1169 if (this.status == null) 1170 this.status = new Enumeration<AppointmentStatus>(new AppointmentStatusEnumFactory()); 1171 this.status.setValue(value); 1172 return this; 1173 } 1174 1175 /** 1176 * @return {@link #serviceCategory} (A broad categorisation of the service that is to be performed during this appointment.) 1177 */ 1178 public CodeableConcept getServiceCategory() { 1179 if (this.serviceCategory == null) 1180 if (Configuration.errorOnAutoCreate()) 1181 throw new Error("Attempt to auto-create Appointment.serviceCategory"); 1182 else if (Configuration.doAutoCreate()) 1183 this.serviceCategory = new CodeableConcept(); // cc 1184 return this.serviceCategory; 1185 } 1186 1187 public boolean hasServiceCategory() { 1188 return this.serviceCategory != null && !this.serviceCategory.isEmpty(); 1189 } 1190 1191 /** 1192 * @param value {@link #serviceCategory} (A broad categorisation of the service that is to be performed during this appointment.) 1193 */ 1194 public Appointment setServiceCategory(CodeableConcept value) { 1195 this.serviceCategory = value; 1196 return this; 1197 } 1198 1199 /** 1200 * @return {@link #serviceType} (The specific service that is to be performed during this appointment.) 1201 */ 1202 public List<CodeableConcept> getServiceType() { 1203 if (this.serviceType == null) 1204 this.serviceType = new ArrayList<CodeableConcept>(); 1205 return this.serviceType; 1206 } 1207 1208 /** 1209 * @return Returns a reference to <code>this</code> for easy method chaining 1210 */ 1211 public Appointment setServiceType(List<CodeableConcept> theServiceType) { 1212 this.serviceType = theServiceType; 1213 return this; 1214 } 1215 1216 public boolean hasServiceType() { 1217 if (this.serviceType == null) 1218 return false; 1219 for (CodeableConcept item : this.serviceType) 1220 if (!item.isEmpty()) 1221 return true; 1222 return false; 1223 } 1224 1225 public CodeableConcept addServiceType() { //3 1226 CodeableConcept t = new CodeableConcept(); 1227 if (this.serviceType == null) 1228 this.serviceType = new ArrayList<CodeableConcept>(); 1229 this.serviceType.add(t); 1230 return t; 1231 } 1232 1233 public Appointment addServiceType(CodeableConcept t) { //3 1234 if (t == null) 1235 return this; 1236 if (this.serviceType == null) 1237 this.serviceType = new ArrayList<CodeableConcept>(); 1238 this.serviceType.add(t); 1239 return this; 1240 } 1241 1242 /** 1243 * @return The first repetition of repeating field {@link #serviceType}, creating it if it does not already exist 1244 */ 1245 public CodeableConcept getServiceTypeFirstRep() { 1246 if (getServiceType().isEmpty()) { 1247 addServiceType(); 1248 } 1249 return getServiceType().get(0); 1250 } 1251 1252 /** 1253 * @return {@link #specialty} (The specialty of a practitioner that would be required to perform the service requested in this appointment.) 1254 */ 1255 public List<CodeableConcept> getSpecialty() { 1256 if (this.specialty == null) 1257 this.specialty = new ArrayList<CodeableConcept>(); 1258 return this.specialty; 1259 } 1260 1261 /** 1262 * @return Returns a reference to <code>this</code> for easy method chaining 1263 */ 1264 public Appointment setSpecialty(List<CodeableConcept> theSpecialty) { 1265 this.specialty = theSpecialty; 1266 return this; 1267 } 1268 1269 public boolean hasSpecialty() { 1270 if (this.specialty == null) 1271 return false; 1272 for (CodeableConcept item : this.specialty) 1273 if (!item.isEmpty()) 1274 return true; 1275 return false; 1276 } 1277 1278 public CodeableConcept addSpecialty() { //3 1279 CodeableConcept t = new CodeableConcept(); 1280 if (this.specialty == null) 1281 this.specialty = new ArrayList<CodeableConcept>(); 1282 this.specialty.add(t); 1283 return t; 1284 } 1285 1286 public Appointment addSpecialty(CodeableConcept t) { //3 1287 if (t == null) 1288 return this; 1289 if (this.specialty == null) 1290 this.specialty = new ArrayList<CodeableConcept>(); 1291 this.specialty.add(t); 1292 return this; 1293 } 1294 1295 /** 1296 * @return The first repetition of repeating field {@link #specialty}, creating it if it does not already exist 1297 */ 1298 public CodeableConcept getSpecialtyFirstRep() { 1299 if (getSpecialty().isEmpty()) { 1300 addSpecialty(); 1301 } 1302 return getSpecialty().get(0); 1303 } 1304 1305 /** 1306 * @return {@link #appointmentType} (The style of appointment or patient that has been booked in the slot (not service type).) 1307 */ 1308 public CodeableConcept getAppointmentType() { 1309 if (this.appointmentType == null) 1310 if (Configuration.errorOnAutoCreate()) 1311 throw new Error("Attempt to auto-create Appointment.appointmentType"); 1312 else if (Configuration.doAutoCreate()) 1313 this.appointmentType = new CodeableConcept(); // cc 1314 return this.appointmentType; 1315 } 1316 1317 public boolean hasAppointmentType() { 1318 return this.appointmentType != null && !this.appointmentType.isEmpty(); 1319 } 1320 1321 /** 1322 * @param value {@link #appointmentType} (The style of appointment or patient that has been booked in the slot (not service type).) 1323 */ 1324 public Appointment setAppointmentType(CodeableConcept value) { 1325 this.appointmentType = value; 1326 return this; 1327 } 1328 1329 /** 1330 * @return {@link #reason} (The reason that this appointment is being scheduled. This is more clinical than administrative.) 1331 */ 1332 public List<CodeableConcept> getReason() { 1333 if (this.reason == null) 1334 this.reason = new ArrayList<CodeableConcept>(); 1335 return this.reason; 1336 } 1337 1338 /** 1339 * @return Returns a reference to <code>this</code> for easy method chaining 1340 */ 1341 public Appointment setReason(List<CodeableConcept> theReason) { 1342 this.reason = theReason; 1343 return this; 1344 } 1345 1346 public boolean hasReason() { 1347 if (this.reason == null) 1348 return false; 1349 for (CodeableConcept item : this.reason) 1350 if (!item.isEmpty()) 1351 return true; 1352 return false; 1353 } 1354 1355 public CodeableConcept addReason() { //3 1356 CodeableConcept t = new CodeableConcept(); 1357 if (this.reason == null) 1358 this.reason = new ArrayList<CodeableConcept>(); 1359 this.reason.add(t); 1360 return t; 1361 } 1362 1363 public Appointment addReason(CodeableConcept t) { //3 1364 if (t == null) 1365 return this; 1366 if (this.reason == null) 1367 this.reason = new ArrayList<CodeableConcept>(); 1368 this.reason.add(t); 1369 return this; 1370 } 1371 1372 /** 1373 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist 1374 */ 1375 public CodeableConcept getReasonFirstRep() { 1376 if (getReason().isEmpty()) { 1377 addReason(); 1378 } 1379 return getReason().get(0); 1380 } 1381 1382 /** 1383 * @return {@link #indication} (Reason the appointment has been scheduled to take place, as specified using information from another resource. When the patient arrives and the encounter begins it may be used as the admission diagnosis. The indication will typically be a Condition (with other resources referenced in the evidence.detail), or a Procedure.) 1384 */ 1385 public List<Reference> getIndication() { 1386 if (this.indication == null) 1387 this.indication = new ArrayList<Reference>(); 1388 return this.indication; 1389 } 1390 1391 /** 1392 * @return Returns a reference to <code>this</code> for easy method chaining 1393 */ 1394 public Appointment setIndication(List<Reference> theIndication) { 1395 this.indication = theIndication; 1396 return this; 1397 } 1398 1399 public boolean hasIndication() { 1400 if (this.indication == null) 1401 return false; 1402 for (Reference item : this.indication) 1403 if (!item.isEmpty()) 1404 return true; 1405 return false; 1406 } 1407 1408 public Reference addIndication() { //3 1409 Reference t = new Reference(); 1410 if (this.indication == null) 1411 this.indication = new ArrayList<Reference>(); 1412 this.indication.add(t); 1413 return t; 1414 } 1415 1416 public Appointment addIndication(Reference t) { //3 1417 if (t == null) 1418 return this; 1419 if (this.indication == null) 1420 this.indication = new ArrayList<Reference>(); 1421 this.indication.add(t); 1422 return this; 1423 } 1424 1425 /** 1426 * @return The first repetition of repeating field {@link #indication}, creating it if it does not already exist 1427 */ 1428 public Reference getIndicationFirstRep() { 1429 if (getIndication().isEmpty()) { 1430 addIndication(); 1431 } 1432 return getIndication().get(0); 1433 } 1434 1435 /** 1436 * @deprecated Use Reference#setResource(IBaseResource) instead 1437 */ 1438 @Deprecated 1439 public List<Resource> getIndicationTarget() { 1440 if (this.indicationTarget == null) 1441 this.indicationTarget = new ArrayList<Resource>(); 1442 return this.indicationTarget; 1443 } 1444 1445 /** 1446 * @return {@link #priority} (The priority of the appointment. Can be used to make informed decisions if needing to re-prioritize appointments. (The iCal Standard specifies 0 as undefined, 1 as highest, 9 as lowest priority).). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 1447 */ 1448 public UnsignedIntType getPriorityElement() { 1449 if (this.priority == null) 1450 if (Configuration.errorOnAutoCreate()) 1451 throw new Error("Attempt to auto-create Appointment.priority"); 1452 else if (Configuration.doAutoCreate()) 1453 this.priority = new UnsignedIntType(); // bb 1454 return this.priority; 1455 } 1456 1457 public boolean hasPriorityElement() { 1458 return this.priority != null && !this.priority.isEmpty(); 1459 } 1460 1461 public boolean hasPriority() { 1462 return this.priority != null && !this.priority.isEmpty(); 1463 } 1464 1465 /** 1466 * @param value {@link #priority} (The priority of the appointment. Can be used to make informed decisions if needing to re-prioritize appointments. (The iCal Standard specifies 0 as undefined, 1 as highest, 9 as lowest priority).). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 1467 */ 1468 public Appointment setPriorityElement(UnsignedIntType value) { 1469 this.priority = value; 1470 return this; 1471 } 1472 1473 /** 1474 * @return The priority of the appointment. Can be used to make informed decisions if needing to re-prioritize appointments. (The iCal Standard specifies 0 as undefined, 1 as highest, 9 as lowest priority). 1475 */ 1476 public int getPriority() { 1477 return this.priority == null || this.priority.isEmpty() ? 0 : this.priority.getValue(); 1478 } 1479 1480 /** 1481 * @param value The priority of the appointment. Can be used to make informed decisions if needing to re-prioritize appointments. (The iCal Standard specifies 0 as undefined, 1 as highest, 9 as lowest priority). 1482 */ 1483 public Appointment setPriority(int value) { 1484 if (this.priority == null) 1485 this.priority = new UnsignedIntType(); 1486 this.priority.setValue(value); 1487 return this; 1488 } 1489 1490 /** 1491 * @return {@link #description} (The brief description of the appointment as would be shown on a subject line in a meeting request, or appointment list. Detailed or expanded information should be put in the comment field.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1492 */ 1493 public StringType getDescriptionElement() { 1494 if (this.description == null) 1495 if (Configuration.errorOnAutoCreate()) 1496 throw new Error("Attempt to auto-create Appointment.description"); 1497 else if (Configuration.doAutoCreate()) 1498 this.description = new StringType(); // bb 1499 return this.description; 1500 } 1501 1502 public boolean hasDescriptionElement() { 1503 return this.description != null && !this.description.isEmpty(); 1504 } 1505 1506 public boolean hasDescription() { 1507 return this.description != null && !this.description.isEmpty(); 1508 } 1509 1510 /** 1511 * @param value {@link #description} (The brief description of the appointment as would be shown on a subject line in a meeting request, or appointment list. Detailed or expanded information should be put in the comment field.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1512 */ 1513 public Appointment setDescriptionElement(StringType value) { 1514 this.description = value; 1515 return this; 1516 } 1517 1518 /** 1519 * @return The brief description of the appointment as would be shown on a subject line in a meeting request, or appointment list. Detailed or expanded information should be put in the comment field. 1520 */ 1521 public String getDescription() { 1522 return this.description == null ? null : this.description.getValue(); 1523 } 1524 1525 /** 1526 * @param value The brief description of the appointment as would be shown on a subject line in a meeting request, or appointment list. Detailed or expanded information should be put in the comment field. 1527 */ 1528 public Appointment setDescription(String value) { 1529 if (Utilities.noString(value)) 1530 this.description = null; 1531 else { 1532 if (this.description == null) 1533 this.description = new StringType(); 1534 this.description.setValue(value); 1535 } 1536 return this; 1537 } 1538 1539 /** 1540 * @return {@link #supportingInformation} (Additional information to support the appointment provided when making the appointment.) 1541 */ 1542 public List<Reference> getSupportingInformation() { 1543 if (this.supportingInformation == null) 1544 this.supportingInformation = new ArrayList<Reference>(); 1545 return this.supportingInformation; 1546 } 1547 1548 /** 1549 * @return Returns a reference to <code>this</code> for easy method chaining 1550 */ 1551 public Appointment setSupportingInformation(List<Reference> theSupportingInformation) { 1552 this.supportingInformation = theSupportingInformation; 1553 return this; 1554 } 1555 1556 public boolean hasSupportingInformation() { 1557 if (this.supportingInformation == null) 1558 return false; 1559 for (Reference item : this.supportingInformation) 1560 if (!item.isEmpty()) 1561 return true; 1562 return false; 1563 } 1564 1565 public Reference addSupportingInformation() { //3 1566 Reference t = new Reference(); 1567 if (this.supportingInformation == null) 1568 this.supportingInformation = new ArrayList<Reference>(); 1569 this.supportingInformation.add(t); 1570 return t; 1571 } 1572 1573 public Appointment addSupportingInformation(Reference t) { //3 1574 if (t == null) 1575 return this; 1576 if (this.supportingInformation == null) 1577 this.supportingInformation = new ArrayList<Reference>(); 1578 this.supportingInformation.add(t); 1579 return this; 1580 } 1581 1582 /** 1583 * @return The first repetition of repeating field {@link #supportingInformation}, creating it if it does not already exist 1584 */ 1585 public Reference getSupportingInformationFirstRep() { 1586 if (getSupportingInformation().isEmpty()) { 1587 addSupportingInformation(); 1588 } 1589 return getSupportingInformation().get(0); 1590 } 1591 1592 /** 1593 * @deprecated Use Reference#setResource(IBaseResource) instead 1594 */ 1595 @Deprecated 1596 public List<Resource> getSupportingInformationTarget() { 1597 if (this.supportingInformationTarget == null) 1598 this.supportingInformationTarget = new ArrayList<Resource>(); 1599 return this.supportingInformationTarget; 1600 } 1601 1602 /** 1603 * @return {@link #start} (Date/Time that the appointment is to take place.). This is the underlying object with id, value and extensions. The accessor "getStart" gives direct access to the value 1604 */ 1605 public InstantType getStartElement() { 1606 if (this.start == null) 1607 if (Configuration.errorOnAutoCreate()) 1608 throw new Error("Attempt to auto-create Appointment.start"); 1609 else if (Configuration.doAutoCreate()) 1610 this.start = new InstantType(); // bb 1611 return this.start; 1612 } 1613 1614 public boolean hasStartElement() { 1615 return this.start != null && !this.start.isEmpty(); 1616 } 1617 1618 public boolean hasStart() { 1619 return this.start != null && !this.start.isEmpty(); 1620 } 1621 1622 /** 1623 * @param value {@link #start} (Date/Time that the appointment is to take place.). This is the underlying object with id, value and extensions. The accessor "getStart" gives direct access to the value 1624 */ 1625 public Appointment setStartElement(InstantType value) { 1626 this.start = value; 1627 return this; 1628 } 1629 1630 /** 1631 * @return Date/Time that the appointment is to take place. 1632 */ 1633 public Date getStart() { 1634 return this.start == null ? null : this.start.getValue(); 1635 } 1636 1637 /** 1638 * @param value Date/Time that the appointment is to take place. 1639 */ 1640 public Appointment setStart(Date value) { 1641 if (value == null) 1642 this.start = null; 1643 else { 1644 if (this.start == null) 1645 this.start = new InstantType(); 1646 this.start.setValue(value); 1647 } 1648 return this; 1649 } 1650 1651 /** 1652 * @return {@link #end} (Date/Time that the appointment is to conclude.). This is the underlying object with id, value and extensions. The accessor "getEnd" gives direct access to the value 1653 */ 1654 public InstantType getEndElement() { 1655 if (this.end == null) 1656 if (Configuration.errorOnAutoCreate()) 1657 throw new Error("Attempt to auto-create Appointment.end"); 1658 else if (Configuration.doAutoCreate()) 1659 this.end = new InstantType(); // bb 1660 return this.end; 1661 } 1662 1663 public boolean hasEndElement() { 1664 return this.end != null && !this.end.isEmpty(); 1665 } 1666 1667 public boolean hasEnd() { 1668 return this.end != null && !this.end.isEmpty(); 1669 } 1670 1671 /** 1672 * @param value {@link #end} (Date/Time that the appointment is to conclude.). This is the underlying object with id, value and extensions. The accessor "getEnd" gives direct access to the value 1673 */ 1674 public Appointment setEndElement(InstantType value) { 1675 this.end = value; 1676 return this; 1677 } 1678 1679 /** 1680 * @return Date/Time that the appointment is to conclude. 1681 */ 1682 public Date getEnd() { 1683 return this.end == null ? null : this.end.getValue(); 1684 } 1685 1686 /** 1687 * @param value Date/Time that the appointment is to conclude. 1688 */ 1689 public Appointment setEnd(Date value) { 1690 if (value == null) 1691 this.end = null; 1692 else { 1693 if (this.end == null) 1694 this.end = new InstantType(); 1695 this.end.setValue(value); 1696 } 1697 return this; 1698 } 1699 1700 /** 1701 * @return {@link #minutesDuration} (Number of minutes that the appointment is to take. This can be less than the duration between the start and end times (where actual time of appointment is only an estimate or is a planned appointment request).). This is the underlying object with id, value and extensions. The accessor "getMinutesDuration" gives direct access to the value 1702 */ 1703 public PositiveIntType getMinutesDurationElement() { 1704 if (this.minutesDuration == null) 1705 if (Configuration.errorOnAutoCreate()) 1706 throw new Error("Attempt to auto-create Appointment.minutesDuration"); 1707 else if (Configuration.doAutoCreate()) 1708 this.minutesDuration = new PositiveIntType(); // bb 1709 return this.minutesDuration; 1710 } 1711 1712 public boolean hasMinutesDurationElement() { 1713 return this.minutesDuration != null && !this.minutesDuration.isEmpty(); 1714 } 1715 1716 public boolean hasMinutesDuration() { 1717 return this.minutesDuration != null && !this.minutesDuration.isEmpty(); 1718 } 1719 1720 /** 1721 * @param value {@link #minutesDuration} (Number of minutes that the appointment is to take. This can be less than the duration between the start and end times (where actual time of appointment is only an estimate or is a planned appointment request).). This is the underlying object with id, value and extensions. The accessor "getMinutesDuration" gives direct access to the value 1722 */ 1723 public Appointment setMinutesDurationElement(PositiveIntType value) { 1724 this.minutesDuration = value; 1725 return this; 1726 } 1727 1728 /** 1729 * @return Number of minutes that the appointment is to take. This can be less than the duration between the start and end times (where actual time of appointment is only an estimate or is a planned appointment request). 1730 */ 1731 public int getMinutesDuration() { 1732 return this.minutesDuration == null || this.minutesDuration.isEmpty() ? 0 : this.minutesDuration.getValue(); 1733 } 1734 1735 /** 1736 * @param value Number of minutes that the appointment is to take. This can be less than the duration between the start and end times (where actual time of appointment is only an estimate or is a planned appointment request). 1737 */ 1738 public Appointment setMinutesDuration(int value) { 1739 if (this.minutesDuration == null) 1740 this.minutesDuration = new PositiveIntType(); 1741 this.minutesDuration.setValue(value); 1742 return this; 1743 } 1744 1745 /** 1746 * @return {@link #slot} (The slots from the participants' schedules that will be filled by the appointment.) 1747 */ 1748 public List<Reference> getSlot() { 1749 if (this.slot == null) 1750 this.slot = new ArrayList<Reference>(); 1751 return this.slot; 1752 } 1753 1754 /** 1755 * @return Returns a reference to <code>this</code> for easy method chaining 1756 */ 1757 public Appointment setSlot(List<Reference> theSlot) { 1758 this.slot = theSlot; 1759 return this; 1760 } 1761 1762 public boolean hasSlot() { 1763 if (this.slot == null) 1764 return false; 1765 for (Reference item : this.slot) 1766 if (!item.isEmpty()) 1767 return true; 1768 return false; 1769 } 1770 1771 public Reference addSlot() { //3 1772 Reference t = new Reference(); 1773 if (this.slot == null) 1774 this.slot = new ArrayList<Reference>(); 1775 this.slot.add(t); 1776 return t; 1777 } 1778 1779 public Appointment addSlot(Reference t) { //3 1780 if (t == null) 1781 return this; 1782 if (this.slot == null) 1783 this.slot = new ArrayList<Reference>(); 1784 this.slot.add(t); 1785 return this; 1786 } 1787 1788 /** 1789 * @return The first repetition of repeating field {@link #slot}, creating it if it does not already exist 1790 */ 1791 public Reference getSlotFirstRep() { 1792 if (getSlot().isEmpty()) { 1793 addSlot(); 1794 } 1795 return getSlot().get(0); 1796 } 1797 1798 /** 1799 * @deprecated Use Reference#setResource(IBaseResource) instead 1800 */ 1801 @Deprecated 1802 public List<Slot> getSlotTarget() { 1803 if (this.slotTarget == null) 1804 this.slotTarget = new ArrayList<Slot>(); 1805 return this.slotTarget; 1806 } 1807 1808 /** 1809 * @deprecated Use Reference#setResource(IBaseResource) instead 1810 */ 1811 @Deprecated 1812 public Slot addSlotTarget() { 1813 Slot r = new Slot(); 1814 if (this.slotTarget == null) 1815 this.slotTarget = new ArrayList<Slot>(); 1816 this.slotTarget.add(r); 1817 return r; 1818 } 1819 1820 /** 1821 * @return {@link #created} (The date that this appointment was initially created. This could be different to the meta.lastModified value on the initial entry, as this could have been before the resource was created on the FHIR server, and should remain unchanged over the lifespan of the appointment.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 1822 */ 1823 public DateTimeType getCreatedElement() { 1824 if (this.created == null) 1825 if (Configuration.errorOnAutoCreate()) 1826 throw new Error("Attempt to auto-create Appointment.created"); 1827 else if (Configuration.doAutoCreate()) 1828 this.created = new DateTimeType(); // bb 1829 return this.created; 1830 } 1831 1832 public boolean hasCreatedElement() { 1833 return this.created != null && !this.created.isEmpty(); 1834 } 1835 1836 public boolean hasCreated() { 1837 return this.created != null && !this.created.isEmpty(); 1838 } 1839 1840 /** 1841 * @param value {@link #created} (The date that this appointment was initially created. This could be different to the meta.lastModified value on the initial entry, as this could have been before the resource was created on the FHIR server, and should remain unchanged over the lifespan of the appointment.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 1842 */ 1843 public Appointment setCreatedElement(DateTimeType value) { 1844 this.created = value; 1845 return this; 1846 } 1847 1848 /** 1849 * @return The date that this appointment was initially created. This could be different to the meta.lastModified value on the initial entry, as this could have been before the resource was created on the FHIR server, and should remain unchanged over the lifespan of the appointment. 1850 */ 1851 public Date getCreated() { 1852 return this.created == null ? null : this.created.getValue(); 1853 } 1854 1855 /** 1856 * @param value The date that this appointment was initially created. This could be different to the meta.lastModified value on the initial entry, as this could have been before the resource was created on the FHIR server, and should remain unchanged over the lifespan of the appointment. 1857 */ 1858 public Appointment setCreated(Date value) { 1859 if (value == null) 1860 this.created = null; 1861 else { 1862 if (this.created == null) 1863 this.created = new DateTimeType(); 1864 this.created.setValue(value); 1865 } 1866 return this; 1867 } 1868 1869 /** 1870 * @return {@link #comment} (Additional comments about the appointment.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 1871 */ 1872 public StringType getCommentElement() { 1873 if (this.comment == null) 1874 if (Configuration.errorOnAutoCreate()) 1875 throw new Error("Attempt to auto-create Appointment.comment"); 1876 else if (Configuration.doAutoCreate()) 1877 this.comment = new StringType(); // bb 1878 return this.comment; 1879 } 1880 1881 public boolean hasCommentElement() { 1882 return this.comment != null && !this.comment.isEmpty(); 1883 } 1884 1885 public boolean hasComment() { 1886 return this.comment != null && !this.comment.isEmpty(); 1887 } 1888 1889 /** 1890 * @param value {@link #comment} (Additional comments about the appointment.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 1891 */ 1892 public Appointment setCommentElement(StringType value) { 1893 this.comment = value; 1894 return this; 1895 } 1896 1897 /** 1898 * @return Additional comments about the appointment. 1899 */ 1900 public String getComment() { 1901 return this.comment == null ? null : this.comment.getValue(); 1902 } 1903 1904 /** 1905 * @param value Additional comments about the appointment. 1906 */ 1907 public Appointment setComment(String value) { 1908 if (Utilities.noString(value)) 1909 this.comment = null; 1910 else { 1911 if (this.comment == null) 1912 this.comment = new StringType(); 1913 this.comment.setValue(value); 1914 } 1915 return this; 1916 } 1917 1918 /** 1919 * @return {@link #incomingReferral} (The referral request this appointment is allocated to assess (incoming referral).) 1920 */ 1921 public List<Reference> getIncomingReferral() { 1922 if (this.incomingReferral == null) 1923 this.incomingReferral = new ArrayList<Reference>(); 1924 return this.incomingReferral; 1925 } 1926 1927 /** 1928 * @return Returns a reference to <code>this</code> for easy method chaining 1929 */ 1930 public Appointment setIncomingReferral(List<Reference> theIncomingReferral) { 1931 this.incomingReferral = theIncomingReferral; 1932 return this; 1933 } 1934 1935 public boolean hasIncomingReferral() { 1936 if (this.incomingReferral == null) 1937 return false; 1938 for (Reference item : this.incomingReferral) 1939 if (!item.isEmpty()) 1940 return true; 1941 return false; 1942 } 1943 1944 public Reference addIncomingReferral() { //3 1945 Reference t = new Reference(); 1946 if (this.incomingReferral == null) 1947 this.incomingReferral = new ArrayList<Reference>(); 1948 this.incomingReferral.add(t); 1949 return t; 1950 } 1951 1952 public Appointment addIncomingReferral(Reference t) { //3 1953 if (t == null) 1954 return this; 1955 if (this.incomingReferral == null) 1956 this.incomingReferral = new ArrayList<Reference>(); 1957 this.incomingReferral.add(t); 1958 return this; 1959 } 1960 1961 /** 1962 * @return The first repetition of repeating field {@link #incomingReferral}, creating it if it does not already exist 1963 */ 1964 public Reference getIncomingReferralFirstRep() { 1965 if (getIncomingReferral().isEmpty()) { 1966 addIncomingReferral(); 1967 } 1968 return getIncomingReferral().get(0); 1969 } 1970 1971 /** 1972 * @deprecated Use Reference#setResource(IBaseResource) instead 1973 */ 1974 @Deprecated 1975 public List<ReferralRequest> getIncomingReferralTarget() { 1976 if (this.incomingReferralTarget == null) 1977 this.incomingReferralTarget = new ArrayList<ReferralRequest>(); 1978 return this.incomingReferralTarget; 1979 } 1980 1981 /** 1982 * @deprecated Use Reference#setResource(IBaseResource) instead 1983 */ 1984 @Deprecated 1985 public ReferralRequest addIncomingReferralTarget() { 1986 ReferralRequest r = new ReferralRequest(); 1987 if (this.incomingReferralTarget == null) 1988 this.incomingReferralTarget = new ArrayList<ReferralRequest>(); 1989 this.incomingReferralTarget.add(r); 1990 return r; 1991 } 1992 1993 /** 1994 * @return {@link #participant} (List of participants involved in the appointment.) 1995 */ 1996 public List<AppointmentParticipantComponent> getParticipant() { 1997 if (this.participant == null) 1998 this.participant = new ArrayList<AppointmentParticipantComponent>(); 1999 return this.participant; 2000 } 2001 2002 /** 2003 * @return Returns a reference to <code>this</code> for easy method chaining 2004 */ 2005 public Appointment setParticipant(List<AppointmentParticipantComponent> theParticipant) { 2006 this.participant = theParticipant; 2007 return this; 2008 } 2009 2010 public boolean hasParticipant() { 2011 if (this.participant == null) 2012 return false; 2013 for (AppointmentParticipantComponent item : this.participant) 2014 if (!item.isEmpty()) 2015 return true; 2016 return false; 2017 } 2018 2019 public AppointmentParticipantComponent addParticipant() { //3 2020 AppointmentParticipantComponent t = new AppointmentParticipantComponent(); 2021 if (this.participant == null) 2022 this.participant = new ArrayList<AppointmentParticipantComponent>(); 2023 this.participant.add(t); 2024 return t; 2025 } 2026 2027 public Appointment addParticipant(AppointmentParticipantComponent t) { //3 2028 if (t == null) 2029 return this; 2030 if (this.participant == null) 2031 this.participant = new ArrayList<AppointmentParticipantComponent>(); 2032 this.participant.add(t); 2033 return this; 2034 } 2035 2036 /** 2037 * @return The first repetition of repeating field {@link #participant}, creating it if it does not already exist 2038 */ 2039 public AppointmentParticipantComponent getParticipantFirstRep() { 2040 if (getParticipant().isEmpty()) { 2041 addParticipant(); 2042 } 2043 return getParticipant().get(0); 2044 } 2045 2046 /** 2047 * @return {@link #requestedPeriod} (A set of date ranges (potentially including times) that the appointment is preferred to be scheduled within. When using these values, the minutes duration should be provided to indicate the length of the appointment to fill and populate the start/end times for the actual allocated time.) 2048 */ 2049 public List<Period> getRequestedPeriod() { 2050 if (this.requestedPeriod == null) 2051 this.requestedPeriod = new ArrayList<Period>(); 2052 return this.requestedPeriod; 2053 } 2054 2055 /** 2056 * @return Returns a reference to <code>this</code> for easy method chaining 2057 */ 2058 public Appointment setRequestedPeriod(List<Period> theRequestedPeriod) { 2059 this.requestedPeriod = theRequestedPeriod; 2060 return this; 2061 } 2062 2063 public boolean hasRequestedPeriod() { 2064 if (this.requestedPeriod == null) 2065 return false; 2066 for (Period item : this.requestedPeriod) 2067 if (!item.isEmpty()) 2068 return true; 2069 return false; 2070 } 2071 2072 public Period addRequestedPeriod() { //3 2073 Period t = new Period(); 2074 if (this.requestedPeriod == null) 2075 this.requestedPeriod = new ArrayList<Period>(); 2076 this.requestedPeriod.add(t); 2077 return t; 2078 } 2079 2080 public Appointment addRequestedPeriod(Period t) { //3 2081 if (t == null) 2082 return this; 2083 if (this.requestedPeriod == null) 2084 this.requestedPeriod = new ArrayList<Period>(); 2085 this.requestedPeriod.add(t); 2086 return this; 2087 } 2088 2089 /** 2090 * @return The first repetition of repeating field {@link #requestedPeriod}, creating it if it does not already exist 2091 */ 2092 public Period getRequestedPeriodFirstRep() { 2093 if (getRequestedPeriod().isEmpty()) { 2094 addRequestedPeriod(); 2095 } 2096 return getRequestedPeriod().get(0); 2097 } 2098 2099 protected void listChildren(List<Property> children) { 2100 super.listChildren(children); 2101 children.add(new Property("identifier", "Identifier", "This records identifiers associated with this appointment concern that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 0, java.lang.Integer.MAX_VALUE, identifier)); 2102 children.add(new Property("status", "code", "The overall status of the Appointment. Each of the participants has their own participation status which indicates their involvement in the process, however this status indicates the shared status.", 0, 1, status)); 2103 children.add(new Property("serviceCategory", "CodeableConcept", "A broad categorisation of the service that is to be performed during this appointment.", 0, 1, serviceCategory)); 2104 children.add(new Property("serviceType", "CodeableConcept", "The specific service that is to be performed during this appointment.", 0, java.lang.Integer.MAX_VALUE, serviceType)); 2105 children.add(new Property("specialty", "CodeableConcept", "The specialty of a practitioner that would be required to perform the service requested in this appointment.", 0, java.lang.Integer.MAX_VALUE, specialty)); 2106 children.add(new Property("appointmentType", "CodeableConcept", "The style of appointment or patient that has been booked in the slot (not service type).", 0, 1, appointmentType)); 2107 children.add(new Property("reason", "CodeableConcept", "The reason that this appointment is being scheduled. This is more clinical than administrative.", 0, java.lang.Integer.MAX_VALUE, reason)); 2108 children.add(new Property("indication", "Reference(Condition|Procedure)", "Reason the appointment has been scheduled to take place, as specified using information from another resource. When the patient arrives and the encounter begins it may be used as the admission diagnosis. The indication will typically be a Condition (with other resources referenced in the evidence.detail), or a Procedure.", 0, java.lang.Integer.MAX_VALUE, indication)); 2109 children.add(new Property("priority", "unsignedInt", "The priority of the appointment. Can be used to make informed decisions if needing to re-prioritize appointments. (The iCal Standard specifies 0 as undefined, 1 as highest, 9 as lowest priority).", 0, 1, priority)); 2110 children.add(new Property("description", "string", "The brief description of the appointment as would be shown on a subject line in a meeting request, or appointment list. Detailed or expanded information should be put in the comment field.", 0, 1, description)); 2111 children.add(new Property("supportingInformation", "Reference(Any)", "Additional information to support the appointment provided when making the appointment.", 0, java.lang.Integer.MAX_VALUE, supportingInformation)); 2112 children.add(new Property("start", "instant", "Date/Time that the appointment is to take place.", 0, 1, start)); 2113 children.add(new Property("end", "instant", "Date/Time that the appointment is to conclude.", 0, 1, end)); 2114 children.add(new Property("minutesDuration", "positiveInt", "Number of minutes that the appointment is to take. This can be less than the duration between the start and end times (where actual time of appointment is only an estimate or is a planned appointment request).", 0, 1, minutesDuration)); 2115 children.add(new Property("slot", "Reference(Slot)", "The slots from the participants' schedules that will be filled by the appointment.", 0, java.lang.Integer.MAX_VALUE, slot)); 2116 children.add(new Property("created", "dateTime", "The date that this appointment was initially created. This could be different to the meta.lastModified value on the initial entry, as this could have been before the resource was created on the FHIR server, and should remain unchanged over the lifespan of the appointment.", 0, 1, created)); 2117 children.add(new Property("comment", "string", "Additional comments about the appointment.", 0, 1, comment)); 2118 children.add(new Property("incomingReferral", "Reference(ReferralRequest)", "The referral request this appointment is allocated to assess (incoming referral).", 0, java.lang.Integer.MAX_VALUE, incomingReferral)); 2119 children.add(new Property("participant", "", "List of participants involved in the appointment.", 0, java.lang.Integer.MAX_VALUE, participant)); 2120 children.add(new Property("requestedPeriod", "Period", "A set of date ranges (potentially including times) that the appointment is preferred to be scheduled within. When using these values, the minutes duration should be provided to indicate the length of the appointment to fill and populate the start/end times for the actual allocated time.", 0, java.lang.Integer.MAX_VALUE, requestedPeriod)); 2121 } 2122 2123 @Override 2124 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2125 switch (_hash) { 2126 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "This records identifiers associated with this appointment concern that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 0, java.lang.Integer.MAX_VALUE, identifier); 2127 case -892481550: /*status*/ return new Property("status", "code", "The overall status of the Appointment. Each of the participants has their own participation status which indicates their involvement in the process, however this status indicates the shared status.", 0, 1, status); 2128 case 1281188563: /*serviceCategory*/ return new Property("serviceCategory", "CodeableConcept", "A broad categorisation of the service that is to be performed during this appointment.", 0, 1, serviceCategory); 2129 case -1928370289: /*serviceType*/ return new Property("serviceType", "CodeableConcept", "The specific service that is to be performed during this appointment.", 0, java.lang.Integer.MAX_VALUE, serviceType); 2130 case -1694759682: /*specialty*/ return new Property("specialty", "CodeableConcept", "The specialty of a practitioner that would be required to perform the service requested in this appointment.", 0, java.lang.Integer.MAX_VALUE, specialty); 2131 case -1596426375: /*appointmentType*/ return new Property("appointmentType", "CodeableConcept", "The style of appointment or patient that has been booked in the slot (not service type).", 0, 1, appointmentType); 2132 case -934964668: /*reason*/ return new Property("reason", "CodeableConcept", "The reason that this appointment is being scheduled. This is more clinical than administrative.", 0, java.lang.Integer.MAX_VALUE, reason); 2133 case -597168804: /*indication*/ return new Property("indication", "Reference(Condition|Procedure)", "Reason the appointment has been scheduled to take place, as specified using information from another resource. When the patient arrives and the encounter begins it may be used as the admission diagnosis. The indication will typically be a Condition (with other resources referenced in the evidence.detail), or a Procedure.", 0, java.lang.Integer.MAX_VALUE, indication); 2134 case -1165461084: /*priority*/ return new Property("priority", "unsignedInt", "The priority of the appointment. Can be used to make informed decisions if needing to re-prioritize appointments. (The iCal Standard specifies 0 as undefined, 1 as highest, 9 as lowest priority).", 0, 1, priority); 2135 case -1724546052: /*description*/ return new Property("description", "string", "The brief description of the appointment as would be shown on a subject line in a meeting request, or appointment list. Detailed or expanded information should be put in the comment field.", 0, 1, description); 2136 case -1248768647: /*supportingInformation*/ return new Property("supportingInformation", "Reference(Any)", "Additional information to support the appointment provided when making the appointment.", 0, java.lang.Integer.MAX_VALUE, supportingInformation); 2137 case 109757538: /*start*/ return new Property("start", "instant", "Date/Time that the appointment is to take place.", 0, 1, start); 2138 case 100571: /*end*/ return new Property("end", "instant", "Date/Time that the appointment is to conclude.", 0, 1, end); 2139 case -413630573: /*minutesDuration*/ return new Property("minutesDuration", "positiveInt", "Number of minutes that the appointment is to take. This can be less than the duration between the start and end times (where actual time of appointment is only an estimate or is a planned appointment request).", 0, 1, minutesDuration); 2140 case 3533310: /*slot*/ return new Property("slot", "Reference(Slot)", "The slots from the participants' schedules that will be filled by the appointment.", 0, java.lang.Integer.MAX_VALUE, slot); 2141 case 1028554472: /*created*/ return new Property("created", "dateTime", "The date that this appointment was initially created. This could be different to the meta.lastModified value on the initial entry, as this could have been before the resource was created on the FHIR server, and should remain unchanged over the lifespan of the appointment.", 0, 1, created); 2142 case 950398559: /*comment*/ return new Property("comment", "string", "Additional comments about the appointment.", 0, 1, comment); 2143 case -1258204701: /*incomingReferral*/ return new Property("incomingReferral", "Reference(ReferralRequest)", "The referral request this appointment is allocated to assess (incoming referral).", 0, java.lang.Integer.MAX_VALUE, incomingReferral); 2144 case 767422259: /*participant*/ return new Property("participant", "", "List of participants involved in the appointment.", 0, java.lang.Integer.MAX_VALUE, participant); 2145 case -897241393: /*requestedPeriod*/ return new Property("requestedPeriod", "Period", "A set of date ranges (potentially including times) that the appointment is preferred to be scheduled within. When using these values, the minutes duration should be provided to indicate the length of the appointment to fill and populate the start/end times for the actual allocated time.", 0, java.lang.Integer.MAX_VALUE, requestedPeriod); 2146 default: return super.getNamedProperty(_hash, _name, _checkValid); 2147 } 2148 2149 } 2150 2151 @Override 2152 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2153 switch (hash) { 2154 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2155 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<AppointmentStatus> 2156 case 1281188563: /*serviceCategory*/ return this.serviceCategory == null ? new Base[0] : new Base[] {this.serviceCategory}; // CodeableConcept 2157 case -1928370289: /*serviceType*/ return this.serviceType == null ? new Base[0] : this.serviceType.toArray(new Base[this.serviceType.size()]); // CodeableConcept 2158 case -1694759682: /*specialty*/ return this.specialty == null ? new Base[0] : this.specialty.toArray(new Base[this.specialty.size()]); // CodeableConcept 2159 case -1596426375: /*appointmentType*/ return this.appointmentType == null ? new Base[0] : new Base[] {this.appointmentType}; // CodeableConcept 2160 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableConcept 2161 case -597168804: /*indication*/ return this.indication == null ? new Base[0] : this.indication.toArray(new Base[this.indication.size()]); // Reference 2162 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // UnsignedIntType 2163 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 2164 case -1248768647: /*supportingInformation*/ return this.supportingInformation == null ? new Base[0] : this.supportingInformation.toArray(new Base[this.supportingInformation.size()]); // Reference 2165 case 109757538: /*start*/ return this.start == null ? new Base[0] : new Base[] {this.start}; // InstantType 2166 case 100571: /*end*/ return this.end == null ? new Base[0] : new Base[] {this.end}; // InstantType 2167 case -413630573: /*minutesDuration*/ return this.minutesDuration == null ? new Base[0] : new Base[] {this.minutesDuration}; // PositiveIntType 2168 case 3533310: /*slot*/ return this.slot == null ? new Base[0] : this.slot.toArray(new Base[this.slot.size()]); // Reference 2169 case 1028554472: /*created*/ return this.created == null ? new Base[0] : new Base[] {this.created}; // DateTimeType 2170 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // StringType 2171 case -1258204701: /*incomingReferral*/ return this.incomingReferral == null ? new Base[0] : this.incomingReferral.toArray(new Base[this.incomingReferral.size()]); // Reference 2172 case 767422259: /*participant*/ return this.participant == null ? new Base[0] : this.participant.toArray(new Base[this.participant.size()]); // AppointmentParticipantComponent 2173 case -897241393: /*requestedPeriod*/ return this.requestedPeriod == null ? new Base[0] : this.requestedPeriod.toArray(new Base[this.requestedPeriod.size()]); // Period 2174 default: return super.getProperty(hash, name, checkValid); 2175 } 2176 2177 } 2178 2179 @Override 2180 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2181 switch (hash) { 2182 case -1618432855: // identifier 2183 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2184 return value; 2185 case -892481550: // status 2186 value = new AppointmentStatusEnumFactory().fromType(castToCode(value)); 2187 this.status = (Enumeration) value; // Enumeration<AppointmentStatus> 2188 return value; 2189 case 1281188563: // serviceCategory 2190 this.serviceCategory = castToCodeableConcept(value); // CodeableConcept 2191 return value; 2192 case -1928370289: // serviceType 2193 this.getServiceType().add(castToCodeableConcept(value)); // CodeableConcept 2194 return value; 2195 case -1694759682: // specialty 2196 this.getSpecialty().add(castToCodeableConcept(value)); // CodeableConcept 2197 return value; 2198 case -1596426375: // appointmentType 2199 this.appointmentType = castToCodeableConcept(value); // CodeableConcept 2200 return value; 2201 case -934964668: // reason 2202 this.getReason().add(castToCodeableConcept(value)); // CodeableConcept 2203 return value; 2204 case -597168804: // indication 2205 this.getIndication().add(castToReference(value)); // Reference 2206 return value; 2207 case -1165461084: // priority 2208 this.priority = castToUnsignedInt(value); // UnsignedIntType 2209 return value; 2210 case -1724546052: // description 2211 this.description = castToString(value); // StringType 2212 return value; 2213 case -1248768647: // supportingInformation 2214 this.getSupportingInformation().add(castToReference(value)); // Reference 2215 return value; 2216 case 109757538: // start 2217 this.start = castToInstant(value); // InstantType 2218 return value; 2219 case 100571: // end 2220 this.end = castToInstant(value); // InstantType 2221 return value; 2222 case -413630573: // minutesDuration 2223 this.minutesDuration = castToPositiveInt(value); // PositiveIntType 2224 return value; 2225 case 3533310: // slot 2226 this.getSlot().add(castToReference(value)); // Reference 2227 return value; 2228 case 1028554472: // created 2229 this.created = castToDateTime(value); // DateTimeType 2230 return value; 2231 case 950398559: // comment 2232 this.comment = castToString(value); // StringType 2233 return value; 2234 case -1258204701: // incomingReferral 2235 this.getIncomingReferral().add(castToReference(value)); // Reference 2236 return value; 2237 case 767422259: // participant 2238 this.getParticipant().add((AppointmentParticipantComponent) value); // AppointmentParticipantComponent 2239 return value; 2240 case -897241393: // requestedPeriod 2241 this.getRequestedPeriod().add(castToPeriod(value)); // Period 2242 return value; 2243 default: return super.setProperty(hash, name, value); 2244 } 2245 2246 } 2247 2248 @Override 2249 public Base setProperty(String name, Base value) throws FHIRException { 2250 if (name.equals("identifier")) { 2251 this.getIdentifier().add(castToIdentifier(value)); 2252 } else if (name.equals("status")) { 2253 value = new AppointmentStatusEnumFactory().fromType(castToCode(value)); 2254 this.status = (Enumeration) value; // Enumeration<AppointmentStatus> 2255 } else if (name.equals("serviceCategory")) { 2256 this.serviceCategory = castToCodeableConcept(value); // CodeableConcept 2257 } else if (name.equals("serviceType")) { 2258 this.getServiceType().add(castToCodeableConcept(value)); 2259 } else if (name.equals("specialty")) { 2260 this.getSpecialty().add(castToCodeableConcept(value)); 2261 } else if (name.equals("appointmentType")) { 2262 this.appointmentType = castToCodeableConcept(value); // CodeableConcept 2263 } else if (name.equals("reason")) { 2264 this.getReason().add(castToCodeableConcept(value)); 2265 } else if (name.equals("indication")) { 2266 this.getIndication().add(castToReference(value)); 2267 } else if (name.equals("priority")) { 2268 this.priority = castToUnsignedInt(value); // UnsignedIntType 2269 } else if (name.equals("description")) { 2270 this.description = castToString(value); // StringType 2271 } else if (name.equals("supportingInformation")) { 2272 this.getSupportingInformation().add(castToReference(value)); 2273 } else if (name.equals("start")) { 2274 this.start = castToInstant(value); // InstantType 2275 } else if (name.equals("end")) { 2276 this.end = castToInstant(value); // InstantType 2277 } else if (name.equals("minutesDuration")) { 2278 this.minutesDuration = castToPositiveInt(value); // PositiveIntType 2279 } else if (name.equals("slot")) { 2280 this.getSlot().add(castToReference(value)); 2281 } else if (name.equals("created")) { 2282 this.created = castToDateTime(value); // DateTimeType 2283 } else if (name.equals("comment")) { 2284 this.comment = castToString(value); // StringType 2285 } else if (name.equals("incomingReferral")) { 2286 this.getIncomingReferral().add(castToReference(value)); 2287 } else if (name.equals("participant")) { 2288 this.getParticipant().add((AppointmentParticipantComponent) value); 2289 } else if (name.equals("requestedPeriod")) { 2290 this.getRequestedPeriod().add(castToPeriod(value)); 2291 } else 2292 return super.setProperty(name, value); 2293 return value; 2294 } 2295 2296 @Override 2297 public Base makeProperty(int hash, String name) throws FHIRException { 2298 switch (hash) { 2299 case -1618432855: return addIdentifier(); 2300 case -892481550: return getStatusElement(); 2301 case 1281188563: return getServiceCategory(); 2302 case -1928370289: return addServiceType(); 2303 case -1694759682: return addSpecialty(); 2304 case -1596426375: return getAppointmentType(); 2305 case -934964668: return addReason(); 2306 case -597168804: return addIndication(); 2307 case -1165461084: return getPriorityElement(); 2308 case -1724546052: return getDescriptionElement(); 2309 case -1248768647: return addSupportingInformation(); 2310 case 109757538: return getStartElement(); 2311 case 100571: return getEndElement(); 2312 case -413630573: return getMinutesDurationElement(); 2313 case 3533310: return addSlot(); 2314 case 1028554472: return getCreatedElement(); 2315 case 950398559: return getCommentElement(); 2316 case -1258204701: return addIncomingReferral(); 2317 case 767422259: return addParticipant(); 2318 case -897241393: return addRequestedPeriod(); 2319 default: return super.makeProperty(hash, name); 2320 } 2321 2322 } 2323 2324 @Override 2325 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2326 switch (hash) { 2327 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2328 case -892481550: /*status*/ return new String[] {"code"}; 2329 case 1281188563: /*serviceCategory*/ return new String[] {"CodeableConcept"}; 2330 case -1928370289: /*serviceType*/ return new String[] {"CodeableConcept"}; 2331 case -1694759682: /*specialty*/ return new String[] {"CodeableConcept"}; 2332 case -1596426375: /*appointmentType*/ return new String[] {"CodeableConcept"}; 2333 case -934964668: /*reason*/ return new String[] {"CodeableConcept"}; 2334 case -597168804: /*indication*/ return new String[] {"Reference"}; 2335 case -1165461084: /*priority*/ return new String[] {"unsignedInt"}; 2336 case -1724546052: /*description*/ return new String[] {"string"}; 2337 case -1248768647: /*supportingInformation*/ return new String[] {"Reference"}; 2338 case 109757538: /*start*/ return new String[] {"instant"}; 2339 case 100571: /*end*/ return new String[] {"instant"}; 2340 case -413630573: /*minutesDuration*/ return new String[] {"positiveInt"}; 2341 case 3533310: /*slot*/ return new String[] {"Reference"}; 2342 case 1028554472: /*created*/ return new String[] {"dateTime"}; 2343 case 950398559: /*comment*/ return new String[] {"string"}; 2344 case -1258204701: /*incomingReferral*/ return new String[] {"Reference"}; 2345 case 767422259: /*participant*/ return new String[] {}; 2346 case -897241393: /*requestedPeriod*/ return new String[] {"Period"}; 2347 default: return super.getTypesForProperty(hash, name); 2348 } 2349 2350 } 2351 2352 @Override 2353 public Base addChild(String name) throws FHIRException { 2354 if (name.equals("identifier")) { 2355 return addIdentifier(); 2356 } 2357 else if (name.equals("status")) { 2358 throw new FHIRException("Cannot call addChild on a singleton property Appointment.status"); 2359 } 2360 else if (name.equals("serviceCategory")) { 2361 this.serviceCategory = new CodeableConcept(); 2362 return this.serviceCategory; 2363 } 2364 else if (name.equals("serviceType")) { 2365 return addServiceType(); 2366 } 2367 else if (name.equals("specialty")) { 2368 return addSpecialty(); 2369 } 2370 else if (name.equals("appointmentType")) { 2371 this.appointmentType = new CodeableConcept(); 2372 return this.appointmentType; 2373 } 2374 else if (name.equals("reason")) { 2375 return addReason(); 2376 } 2377 else if (name.equals("indication")) { 2378 return addIndication(); 2379 } 2380 else if (name.equals("priority")) { 2381 throw new FHIRException("Cannot call addChild on a singleton property Appointment.priority"); 2382 } 2383 else if (name.equals("description")) { 2384 throw new FHIRException("Cannot call addChild on a singleton property Appointment.description"); 2385 } 2386 else if (name.equals("supportingInformation")) { 2387 return addSupportingInformation(); 2388 } 2389 else if (name.equals("start")) { 2390 throw new FHIRException("Cannot call addChild on a singleton property Appointment.start"); 2391 } 2392 else if (name.equals("end")) { 2393 throw new FHIRException("Cannot call addChild on a singleton property Appointment.end"); 2394 } 2395 else if (name.equals("minutesDuration")) { 2396 throw new FHIRException("Cannot call addChild on a singleton property Appointment.minutesDuration"); 2397 } 2398 else if (name.equals("slot")) { 2399 return addSlot(); 2400 } 2401 else if (name.equals("created")) { 2402 throw new FHIRException("Cannot call addChild on a singleton property Appointment.created"); 2403 } 2404 else if (name.equals("comment")) { 2405 throw new FHIRException("Cannot call addChild on a singleton property Appointment.comment"); 2406 } 2407 else if (name.equals("incomingReferral")) { 2408 return addIncomingReferral(); 2409 } 2410 else if (name.equals("participant")) { 2411 return addParticipant(); 2412 } 2413 else if (name.equals("requestedPeriod")) { 2414 return addRequestedPeriod(); 2415 } 2416 else 2417 return super.addChild(name); 2418 } 2419 2420 public String fhirType() { 2421 return "Appointment"; 2422 2423 } 2424 2425 public Appointment copy() { 2426 Appointment dst = new Appointment(); 2427 copyValues(dst); 2428 if (identifier != null) { 2429 dst.identifier = new ArrayList<Identifier>(); 2430 for (Identifier i : identifier) 2431 dst.identifier.add(i.copy()); 2432 }; 2433 dst.status = status == null ? null : status.copy(); 2434 dst.serviceCategory = serviceCategory == null ? null : serviceCategory.copy(); 2435 if (serviceType != null) { 2436 dst.serviceType = new ArrayList<CodeableConcept>(); 2437 for (CodeableConcept i : serviceType) 2438 dst.serviceType.add(i.copy()); 2439 }; 2440 if (specialty != null) { 2441 dst.specialty = new ArrayList<CodeableConcept>(); 2442 for (CodeableConcept i : specialty) 2443 dst.specialty.add(i.copy()); 2444 }; 2445 dst.appointmentType = appointmentType == null ? null : appointmentType.copy(); 2446 if (reason != null) { 2447 dst.reason = new ArrayList<CodeableConcept>(); 2448 for (CodeableConcept i : reason) 2449 dst.reason.add(i.copy()); 2450 }; 2451 if (indication != null) { 2452 dst.indication = new ArrayList<Reference>(); 2453 for (Reference i : indication) 2454 dst.indication.add(i.copy()); 2455 }; 2456 dst.priority = priority == null ? null : priority.copy(); 2457 dst.description = description == null ? null : description.copy(); 2458 if (supportingInformation != null) { 2459 dst.supportingInformation = new ArrayList<Reference>(); 2460 for (Reference i : supportingInformation) 2461 dst.supportingInformation.add(i.copy()); 2462 }; 2463 dst.start = start == null ? null : start.copy(); 2464 dst.end = end == null ? null : end.copy(); 2465 dst.minutesDuration = minutesDuration == null ? null : minutesDuration.copy(); 2466 if (slot != null) { 2467 dst.slot = new ArrayList<Reference>(); 2468 for (Reference i : slot) 2469 dst.slot.add(i.copy()); 2470 }; 2471 dst.created = created == null ? null : created.copy(); 2472 dst.comment = comment == null ? null : comment.copy(); 2473 if (incomingReferral != null) { 2474 dst.incomingReferral = new ArrayList<Reference>(); 2475 for (Reference i : incomingReferral) 2476 dst.incomingReferral.add(i.copy()); 2477 }; 2478 if (participant != null) { 2479 dst.participant = new ArrayList<AppointmentParticipantComponent>(); 2480 for (AppointmentParticipantComponent i : participant) 2481 dst.participant.add(i.copy()); 2482 }; 2483 if (requestedPeriod != null) { 2484 dst.requestedPeriod = new ArrayList<Period>(); 2485 for (Period i : requestedPeriod) 2486 dst.requestedPeriod.add(i.copy()); 2487 }; 2488 return dst; 2489 } 2490 2491 protected Appointment typedCopy() { 2492 return copy(); 2493 } 2494 2495 @Override 2496 public boolean equalsDeep(Base other_) { 2497 if (!super.equalsDeep(other_)) 2498 return false; 2499 if (!(other_ instanceof Appointment)) 2500 return false; 2501 Appointment o = (Appointment) other_; 2502 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(serviceCategory, o.serviceCategory, true) 2503 && compareDeep(serviceType, o.serviceType, true) && compareDeep(specialty, o.specialty, true) && compareDeep(appointmentType, o.appointmentType, true) 2504 && compareDeep(reason, o.reason, true) && compareDeep(indication, o.indication, true) && compareDeep(priority, o.priority, true) 2505 && compareDeep(description, o.description, true) && compareDeep(supportingInformation, o.supportingInformation, true) 2506 && compareDeep(start, o.start, true) && compareDeep(end, o.end, true) && compareDeep(minutesDuration, o.minutesDuration, true) 2507 && compareDeep(slot, o.slot, true) && compareDeep(created, o.created, true) && compareDeep(comment, o.comment, true) 2508 && compareDeep(incomingReferral, o.incomingReferral, true) && compareDeep(participant, o.participant, true) 2509 && compareDeep(requestedPeriod, o.requestedPeriod, true); 2510 } 2511 2512 @Override 2513 public boolean equalsShallow(Base other_) { 2514 if (!super.equalsShallow(other_)) 2515 return false; 2516 if (!(other_ instanceof Appointment)) 2517 return false; 2518 Appointment o = (Appointment) other_; 2519 return compareValues(status, o.status, true) && compareValues(priority, o.priority, true) && compareValues(description, o.description, true) 2520 && compareValues(start, o.start, true) && compareValues(end, o.end, true) && compareValues(minutesDuration, o.minutesDuration, true) 2521 && compareValues(created, o.created, true) && compareValues(comment, o.comment, true); 2522 } 2523 2524 public boolean isEmpty() { 2525 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, serviceCategory 2526 , serviceType, specialty, appointmentType, reason, indication, priority, description 2527 , supportingInformation, start, end, minutesDuration, slot, created, comment, incomingReferral 2528 , participant, requestedPeriod); 2529 } 2530 2531 @Override 2532 public ResourceType getResourceType() { 2533 return ResourceType.Appointment; 2534 } 2535 2536 /** 2537 * Search parameter: <b>date</b> 2538 * <p> 2539 * Description: <b>Appointment date/time.</b><br> 2540 * Type: <b>date</b><br> 2541 * Path: <b>Appointment.start</b><br> 2542 * </p> 2543 */ 2544 @SearchParamDefinition(name="date", path="Appointment.start", description="Appointment date/time.", type="date" ) 2545 public static final String SP_DATE = "date"; 2546 /** 2547 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2548 * <p> 2549 * Description: <b>Appointment date/time.</b><br> 2550 * Type: <b>date</b><br> 2551 * Path: <b>Appointment.start</b><br> 2552 * </p> 2553 */ 2554 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 2555 2556 /** 2557 * Search parameter: <b>actor</b> 2558 * <p> 2559 * Description: <b>Any one of the individuals participating in the appointment</b><br> 2560 * Type: <b>reference</b><br> 2561 * Path: <b>Appointment.participant.actor</b><br> 2562 * </p> 2563 */ 2564 @SearchParamDefinition(name="actor", path="Appointment.participant.actor", description="Any one of the individuals participating in the appointment", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Device.class, HealthcareService.class, Location.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 2565 public static final String SP_ACTOR = "actor"; 2566 /** 2567 * <b>Fluent Client</b> search parameter constant for <b>actor</b> 2568 * <p> 2569 * Description: <b>Any one of the individuals participating in the appointment</b><br> 2570 * Type: <b>reference</b><br> 2571 * Path: <b>Appointment.participant.actor</b><br> 2572 * </p> 2573 */ 2574 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ACTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ACTOR); 2575 2576/** 2577 * Constant for fluent queries to be used to add include statements. Specifies 2578 * the path value of "<b>Appointment:actor</b>". 2579 */ 2580 public static final ca.uhn.fhir.model.api.Include INCLUDE_ACTOR = new ca.uhn.fhir.model.api.Include("Appointment:actor").toLocked(); 2581 2582 /** 2583 * Search parameter: <b>identifier</b> 2584 * <p> 2585 * Description: <b>An Identifier of the Appointment</b><br> 2586 * Type: <b>token</b><br> 2587 * Path: <b>Appointment.identifier</b><br> 2588 * </p> 2589 */ 2590 @SearchParamDefinition(name="identifier", path="Appointment.identifier", description="An Identifier of the Appointment", type="token" ) 2591 public static final String SP_IDENTIFIER = "identifier"; 2592 /** 2593 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2594 * <p> 2595 * Description: <b>An Identifier of the Appointment</b><br> 2596 * Type: <b>token</b><br> 2597 * Path: <b>Appointment.identifier</b><br> 2598 * </p> 2599 */ 2600 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2601 2602 /** 2603 * Search parameter: <b>practitioner</b> 2604 * <p> 2605 * Description: <b>One of the individuals of the appointment is this practitioner</b><br> 2606 * Type: <b>reference</b><br> 2607 * Path: <b>Appointment.participant.actor</b><br> 2608 * </p> 2609 */ 2610 @SearchParamDefinition(name="practitioner", path="Appointment.participant.actor", description="One of the individuals of the appointment is this practitioner", type="reference", target={Practitioner.class } ) 2611 public static final String SP_PRACTITIONER = "practitioner"; 2612 /** 2613 * <b>Fluent Client</b> search parameter constant for <b>practitioner</b> 2614 * <p> 2615 * Description: <b>One of the individuals of the appointment is this practitioner</b><br> 2616 * Type: <b>reference</b><br> 2617 * Path: <b>Appointment.participant.actor</b><br> 2618 * </p> 2619 */ 2620 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRACTITIONER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PRACTITIONER); 2621 2622/** 2623 * Constant for fluent queries to be used to add include statements. Specifies 2624 * the path value of "<b>Appointment:practitioner</b>". 2625 */ 2626 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRACTITIONER = new ca.uhn.fhir.model.api.Include("Appointment:practitioner").toLocked(); 2627 2628 /** 2629 * Search parameter: <b>incomingreferral</b> 2630 * <p> 2631 * Description: <b>The ReferralRequest provided as information to allocate to the Encounter</b><br> 2632 * Type: <b>reference</b><br> 2633 * Path: <b>Appointment.incomingReferral</b><br> 2634 * </p> 2635 */ 2636 @SearchParamDefinition(name="incomingreferral", path="Appointment.incomingReferral", description="The ReferralRequest provided as information to allocate to the Encounter", type="reference", target={ReferralRequest.class } ) 2637 public static final String SP_INCOMINGREFERRAL = "incomingreferral"; 2638 /** 2639 * <b>Fluent Client</b> search parameter constant for <b>incomingreferral</b> 2640 * <p> 2641 * Description: <b>The ReferralRequest provided as information to allocate to the Encounter</b><br> 2642 * Type: <b>reference</b><br> 2643 * Path: <b>Appointment.incomingReferral</b><br> 2644 * </p> 2645 */ 2646 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INCOMINGREFERRAL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INCOMINGREFERRAL); 2647 2648/** 2649 * Constant for fluent queries to be used to add include statements. Specifies 2650 * the path value of "<b>Appointment:incomingreferral</b>". 2651 */ 2652 public static final ca.uhn.fhir.model.api.Include INCLUDE_INCOMINGREFERRAL = new ca.uhn.fhir.model.api.Include("Appointment:incomingreferral").toLocked(); 2653 2654 /** 2655 * Search parameter: <b>part-status</b> 2656 * <p> 2657 * Description: <b>The Participation status of the subject, or other participant on the appointment. Can be used to locate participants that have not responded to meeting requests.</b><br> 2658 * Type: <b>token</b><br> 2659 * Path: <b>Appointment.participant.status</b><br> 2660 * </p> 2661 */ 2662 @SearchParamDefinition(name="part-status", path="Appointment.participant.status", description="The Participation status of the subject, or other participant on the appointment. Can be used to locate participants that have not responded to meeting requests.", type="token" ) 2663 public static final String SP_PART_STATUS = "part-status"; 2664 /** 2665 * <b>Fluent Client</b> search parameter constant for <b>part-status</b> 2666 * <p> 2667 * Description: <b>The Participation status of the subject, or other participant on the appointment. Can be used to locate participants that have not responded to meeting requests.</b><br> 2668 * Type: <b>token</b><br> 2669 * Path: <b>Appointment.participant.status</b><br> 2670 * </p> 2671 */ 2672 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PART_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PART_STATUS); 2673 2674 /** 2675 * Search parameter: <b>patient</b> 2676 * <p> 2677 * Description: <b>One of the individuals of the appointment is this patient</b><br> 2678 * Type: <b>reference</b><br> 2679 * Path: <b>Appointment.participant.actor</b><br> 2680 * </p> 2681 */ 2682 @SearchParamDefinition(name="patient", path="Appointment.participant.actor", description="One of the individuals of the appointment is this patient", type="reference", target={Patient.class } ) 2683 public static final String SP_PATIENT = "patient"; 2684 /** 2685 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2686 * <p> 2687 * Description: <b>One of the individuals of the appointment is this patient</b><br> 2688 * Type: <b>reference</b><br> 2689 * Path: <b>Appointment.participant.actor</b><br> 2690 * </p> 2691 */ 2692 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2693 2694/** 2695 * Constant for fluent queries to be used to add include statements. Specifies 2696 * the path value of "<b>Appointment:patient</b>". 2697 */ 2698 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Appointment:patient").toLocked(); 2699 2700 /** 2701 * Search parameter: <b>appointment-type</b> 2702 * <p> 2703 * Description: <b>The style of appointment or patient that has been booked in the slot (not service type)</b><br> 2704 * Type: <b>token</b><br> 2705 * Path: <b>Appointment.appointmentType</b><br> 2706 * </p> 2707 */ 2708 @SearchParamDefinition(name="appointment-type", path="Appointment.appointmentType", description="The style of appointment or patient that has been booked in the slot (not service type)", type="token" ) 2709 public static final String SP_APPOINTMENT_TYPE = "appointment-type"; 2710 /** 2711 * <b>Fluent Client</b> search parameter constant for <b>appointment-type</b> 2712 * <p> 2713 * Description: <b>The style of appointment or patient that has been booked in the slot (not service type)</b><br> 2714 * Type: <b>token</b><br> 2715 * Path: <b>Appointment.appointmentType</b><br> 2716 * </p> 2717 */ 2718 public static final ca.uhn.fhir.rest.gclient.TokenClientParam APPOINTMENT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_APPOINTMENT_TYPE); 2719 2720 /** 2721 * Search parameter: <b>service-type</b> 2722 * <p> 2723 * Description: <b>The specific service that is to be performed during this appointment</b><br> 2724 * Type: <b>token</b><br> 2725 * Path: <b>Appointment.serviceType</b><br> 2726 * </p> 2727 */ 2728 @SearchParamDefinition(name="service-type", path="Appointment.serviceType", description="The specific service that is to be performed during this appointment", type="token" ) 2729 public static final String SP_SERVICE_TYPE = "service-type"; 2730 /** 2731 * <b>Fluent Client</b> search parameter constant for <b>service-type</b> 2732 * <p> 2733 * Description: <b>The specific service that is to be performed during this appointment</b><br> 2734 * Type: <b>token</b><br> 2735 * Path: <b>Appointment.serviceType</b><br> 2736 * </p> 2737 */ 2738 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SERVICE_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SERVICE_TYPE); 2739 2740 /** 2741 * Search parameter: <b>location</b> 2742 * <p> 2743 * Description: <b>This location is listed in the participants of the appointment</b><br> 2744 * Type: <b>reference</b><br> 2745 * Path: <b>Appointment.participant.actor</b><br> 2746 * </p> 2747 */ 2748 @SearchParamDefinition(name="location", path="Appointment.participant.actor", description="This location is listed in the participants of the appointment", type="reference", target={Location.class } ) 2749 public static final String SP_LOCATION = "location"; 2750 /** 2751 * <b>Fluent Client</b> search parameter constant for <b>location</b> 2752 * <p> 2753 * Description: <b>This location is listed in the participants of the appointment</b><br> 2754 * Type: <b>reference</b><br> 2755 * Path: <b>Appointment.participant.actor</b><br> 2756 * </p> 2757 */ 2758 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_LOCATION); 2759 2760/** 2761 * Constant for fluent queries to be used to add include statements. Specifies 2762 * the path value of "<b>Appointment:location</b>". 2763 */ 2764 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include("Appointment:location").toLocked(); 2765 2766 /** 2767 * Search parameter: <b>status</b> 2768 * <p> 2769 * Description: <b>The overall status of the appointment</b><br> 2770 * Type: <b>token</b><br> 2771 * Path: <b>Appointment.status</b><br> 2772 * </p> 2773 */ 2774 @SearchParamDefinition(name="status", path="Appointment.status", description="The overall status of the appointment", type="token" ) 2775 public static final String SP_STATUS = "status"; 2776 /** 2777 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2778 * <p> 2779 * Description: <b>The overall status of the appointment</b><br> 2780 * Type: <b>token</b><br> 2781 * Path: <b>Appointment.status</b><br> 2782 * </p> 2783 */ 2784 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2785 2786 2787}