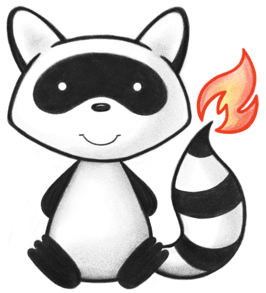
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.utilities.Utilities; 041 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046/** 047 * A reply to an appointment request for a patient and/or practitioner(s), such as a confirmation or rejection. 048 */ 049@ResourceDef(name="AppointmentResponse", profile="http://hl7.org/fhir/Profile/AppointmentResponse") 050public class AppointmentResponse extends DomainResource { 051 052 public enum ParticipantStatus { 053 /** 054 * The participant has accepted the appointment. 055 */ 056 ACCEPTED, 057 /** 058 * The participant has declined the appointment and will not participate in the appointment. 059 */ 060 DECLINED, 061 /** 062 * The participant has tentatively accepted the appointment. This could be automatically created by a system and requires further processing before it can be accepted. There is no commitment that attendance will occur. 063 */ 064 TENTATIVE, 065 /** 066 * The participant needs to indicate if they accept the appointment by changing this status to one of the other statuses. 067 */ 068 NEEDSACTION, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static ParticipantStatus fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("accepted".equals(codeString)) 077 return ACCEPTED; 078 if ("declined".equals(codeString)) 079 return DECLINED; 080 if ("tentative".equals(codeString)) 081 return TENTATIVE; 082 if ("needs-action".equals(codeString)) 083 return NEEDSACTION; 084 if (Configuration.isAcceptInvalidEnums()) 085 return null; 086 else 087 throw new FHIRException("Unknown ParticipantStatus code '"+codeString+"'"); 088 } 089 public String toCode() { 090 switch (this) { 091 case ACCEPTED: return "accepted"; 092 case DECLINED: return "declined"; 093 case TENTATIVE: return "tentative"; 094 case NEEDSACTION: return "needs-action"; 095 case NULL: return null; 096 default: return "?"; 097 } 098 } 099 public String getSystem() { 100 switch (this) { 101 case ACCEPTED: return "http://hl7.org/fhir/participationstatus"; 102 case DECLINED: return "http://hl7.org/fhir/participationstatus"; 103 case TENTATIVE: return "http://hl7.org/fhir/participationstatus"; 104 case NEEDSACTION: return "http://hl7.org/fhir/participationstatus"; 105 case NULL: return null; 106 default: return "?"; 107 } 108 } 109 public String getDefinition() { 110 switch (this) { 111 case ACCEPTED: return "The participant has accepted the appointment."; 112 case DECLINED: return "The participant has declined the appointment and will not participate in the appointment."; 113 case TENTATIVE: return "The participant has tentatively accepted the appointment. This could be automatically created by a system and requires further processing before it can be accepted. There is no commitment that attendance will occur."; 114 case NEEDSACTION: return "The participant needs to indicate if they accept the appointment by changing this status to one of the other statuses."; 115 case NULL: return null; 116 default: return "?"; 117 } 118 } 119 public String getDisplay() { 120 switch (this) { 121 case ACCEPTED: return "Accepted"; 122 case DECLINED: return "Declined"; 123 case TENTATIVE: return "Tentative"; 124 case NEEDSACTION: return "Needs Action"; 125 case NULL: return null; 126 default: return "?"; 127 } 128 } 129 } 130 131 public static class ParticipantStatusEnumFactory implements EnumFactory<ParticipantStatus> { 132 public ParticipantStatus fromCode(String codeString) throws IllegalArgumentException { 133 if (codeString == null || "".equals(codeString)) 134 if (codeString == null || "".equals(codeString)) 135 return null; 136 if ("accepted".equals(codeString)) 137 return ParticipantStatus.ACCEPTED; 138 if ("declined".equals(codeString)) 139 return ParticipantStatus.DECLINED; 140 if ("tentative".equals(codeString)) 141 return ParticipantStatus.TENTATIVE; 142 if ("needs-action".equals(codeString)) 143 return ParticipantStatus.NEEDSACTION; 144 throw new IllegalArgumentException("Unknown ParticipantStatus code '"+codeString+"'"); 145 } 146 public Enumeration<ParticipantStatus> fromType(PrimitiveType<?> code) throws FHIRException { 147 if (code == null) 148 return null; 149 if (code.isEmpty()) 150 return new Enumeration<ParticipantStatus>(this); 151 String codeString = code.asStringValue(); 152 if (codeString == null || "".equals(codeString)) 153 return null; 154 if ("accepted".equals(codeString)) 155 return new Enumeration<ParticipantStatus>(this, ParticipantStatus.ACCEPTED); 156 if ("declined".equals(codeString)) 157 return new Enumeration<ParticipantStatus>(this, ParticipantStatus.DECLINED); 158 if ("tentative".equals(codeString)) 159 return new Enumeration<ParticipantStatus>(this, ParticipantStatus.TENTATIVE); 160 if ("needs-action".equals(codeString)) 161 return new Enumeration<ParticipantStatus>(this, ParticipantStatus.NEEDSACTION); 162 throw new FHIRException("Unknown ParticipantStatus code '"+codeString+"'"); 163 } 164 public String toCode(ParticipantStatus code) { 165 if (code == ParticipantStatus.NULL) 166 return null; 167 if (code == ParticipantStatus.ACCEPTED) 168 return "accepted"; 169 if (code == ParticipantStatus.DECLINED) 170 return "declined"; 171 if (code == ParticipantStatus.TENTATIVE) 172 return "tentative"; 173 if (code == ParticipantStatus.NEEDSACTION) 174 return "needs-action"; 175 return "?"; 176 } 177 public String toSystem(ParticipantStatus code) { 178 return code.getSystem(); 179 } 180 } 181 182 /** 183 * This records identifiers associated with this appointment response concern that are defined by business processes and/ or used to refer to it when a direct URL reference to the resource itself is not appropriate. 184 */ 185 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 186 @Description(shortDefinition="External Ids for this item", formalDefinition="This records identifiers associated with this appointment response concern that are defined by business processes and/ or used to refer to it when a direct URL reference to the resource itself is not appropriate." ) 187 protected List<Identifier> identifier; 188 189 /** 190 * Appointment that this response is replying to. 191 */ 192 @Child(name = "appointment", type = {Appointment.class}, order=1, min=1, max=1, modifier=false, summary=true) 193 @Description(shortDefinition="Appointment this response relates to", formalDefinition="Appointment that this response is replying to." ) 194 protected Reference appointment; 195 196 /** 197 * The actual object that is the target of the reference (Appointment that this response is replying to.) 198 */ 199 protected Appointment appointmentTarget; 200 201 /** 202 * Date/Time that the appointment is to take place, or requested new start time. 203 */ 204 @Child(name = "start", type = {InstantType.class}, order=2, min=0, max=1, modifier=false, summary=false) 205 @Description(shortDefinition="Time from appointment, or requested new start time", formalDefinition="Date/Time that the appointment is to take place, or requested new start time." ) 206 protected InstantType start; 207 208 /** 209 * This may be either the same as the appointment request to confirm the details of the appointment, or alternately a new time to request a re-negotiation of the end time. 210 */ 211 @Child(name = "end", type = {InstantType.class}, order=3, min=0, max=1, modifier=false, summary=false) 212 @Description(shortDefinition="Time from appointment, or requested new end time", formalDefinition="This may be either the same as the appointment request to confirm the details of the appointment, or alternately a new time to request a re-negotiation of the end time." ) 213 protected InstantType end; 214 215 /** 216 * Role of participant in the appointment. 217 */ 218 @Child(name = "participantType", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 219 @Description(shortDefinition="Role of participant in the appointment", formalDefinition="Role of participant in the appointment." ) 220 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-participant-type") 221 protected List<CodeableConcept> participantType; 222 223 /** 224 * A Person, Location/HealthcareService or Device that is participating in the appointment. 225 */ 226 @Child(name = "actor", type = {Patient.class, Practitioner.class, RelatedPerson.class, Device.class, HealthcareService.class, Location.class}, order=5, min=0, max=1, modifier=false, summary=true) 227 @Description(shortDefinition="Person, Location/HealthcareService or Device", formalDefinition="A Person, Location/HealthcareService or Device that is participating in the appointment." ) 228 protected Reference actor; 229 230 /** 231 * The actual object that is the target of the reference (A Person, Location/HealthcareService or Device that is participating in the appointment.) 232 */ 233 protected Resource actorTarget; 234 235 /** 236 * Participation status of the participant. When the status is declined or tentative if the start/end times are different to the appointment, then these times should be interpreted as a requested time change. When the status is accepted, the times can either be the time of the appointment (as a confirmation of the time) or can be empty. 237 */ 238 @Child(name = "participantStatus", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 239 @Description(shortDefinition="accepted | declined | tentative | in-process | completed | needs-action | entered-in-error", formalDefinition="Participation status of the participant. When the status is declined or tentative if the start/end times are different to the appointment, then these times should be interpreted as a requested time change. When the status is accepted, the times can either be the time of the appointment (as a confirmation of the time) or can be empty." ) 240 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/participationstatus") 241 protected Enumeration<ParticipantStatus> participantStatus; 242 243 /** 244 * Additional comments about the appointment. 245 */ 246 @Child(name = "comment", type = {StringType.class}, order=7, min=0, max=1, modifier=false, summary=false) 247 @Description(shortDefinition="Additional comments", formalDefinition="Additional comments about the appointment." ) 248 protected StringType comment; 249 250 private static final long serialVersionUID = 248548635L; 251 252 /** 253 * Constructor 254 */ 255 public AppointmentResponse() { 256 super(); 257 } 258 259 /** 260 * Constructor 261 */ 262 public AppointmentResponse(Reference appointment, Enumeration<ParticipantStatus> participantStatus) { 263 super(); 264 this.appointment = appointment; 265 this.participantStatus = participantStatus; 266 } 267 268 /** 269 * @return {@link #identifier} (This records identifiers associated with this appointment response concern that are defined by business processes and/ or used to refer to it when a direct URL reference to the resource itself is not appropriate.) 270 */ 271 public List<Identifier> getIdentifier() { 272 if (this.identifier == null) 273 this.identifier = new ArrayList<Identifier>(); 274 return this.identifier; 275 } 276 277 /** 278 * @return Returns a reference to <code>this</code> for easy method chaining 279 */ 280 public AppointmentResponse setIdentifier(List<Identifier> theIdentifier) { 281 this.identifier = theIdentifier; 282 return this; 283 } 284 285 public boolean hasIdentifier() { 286 if (this.identifier == null) 287 return false; 288 for (Identifier item : this.identifier) 289 if (!item.isEmpty()) 290 return true; 291 return false; 292 } 293 294 public Identifier addIdentifier() { //3 295 Identifier t = new Identifier(); 296 if (this.identifier == null) 297 this.identifier = new ArrayList<Identifier>(); 298 this.identifier.add(t); 299 return t; 300 } 301 302 public AppointmentResponse addIdentifier(Identifier t) { //3 303 if (t == null) 304 return this; 305 if (this.identifier == null) 306 this.identifier = new ArrayList<Identifier>(); 307 this.identifier.add(t); 308 return this; 309 } 310 311 /** 312 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 313 */ 314 public Identifier getIdentifierFirstRep() { 315 if (getIdentifier().isEmpty()) { 316 addIdentifier(); 317 } 318 return getIdentifier().get(0); 319 } 320 321 /** 322 * @return {@link #appointment} (Appointment that this response is replying to.) 323 */ 324 public Reference getAppointment() { 325 if (this.appointment == null) 326 if (Configuration.errorOnAutoCreate()) 327 throw new Error("Attempt to auto-create AppointmentResponse.appointment"); 328 else if (Configuration.doAutoCreate()) 329 this.appointment = new Reference(); // cc 330 return this.appointment; 331 } 332 333 public boolean hasAppointment() { 334 return this.appointment != null && !this.appointment.isEmpty(); 335 } 336 337 /** 338 * @param value {@link #appointment} (Appointment that this response is replying to.) 339 */ 340 public AppointmentResponse setAppointment(Reference value) { 341 this.appointment = value; 342 return this; 343 } 344 345 /** 346 * @return {@link #appointment} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Appointment that this response is replying to.) 347 */ 348 public Appointment getAppointmentTarget() { 349 if (this.appointmentTarget == null) 350 if (Configuration.errorOnAutoCreate()) 351 throw new Error("Attempt to auto-create AppointmentResponse.appointment"); 352 else if (Configuration.doAutoCreate()) 353 this.appointmentTarget = new Appointment(); // aa 354 return this.appointmentTarget; 355 } 356 357 /** 358 * @param value {@link #appointment} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Appointment that this response is replying to.) 359 */ 360 public AppointmentResponse setAppointmentTarget(Appointment value) { 361 this.appointmentTarget = value; 362 return this; 363 } 364 365 /** 366 * @return {@link #start} (Date/Time that the appointment is to take place, or requested new start time.). This is the underlying object with id, value and extensions. The accessor "getStart" gives direct access to the value 367 */ 368 public InstantType getStartElement() { 369 if (this.start == null) 370 if (Configuration.errorOnAutoCreate()) 371 throw new Error("Attempt to auto-create AppointmentResponse.start"); 372 else if (Configuration.doAutoCreate()) 373 this.start = new InstantType(); // bb 374 return this.start; 375 } 376 377 public boolean hasStartElement() { 378 return this.start != null && !this.start.isEmpty(); 379 } 380 381 public boolean hasStart() { 382 return this.start != null && !this.start.isEmpty(); 383 } 384 385 /** 386 * @param value {@link #start} (Date/Time that the appointment is to take place, or requested new start time.). This is the underlying object with id, value and extensions. The accessor "getStart" gives direct access to the value 387 */ 388 public AppointmentResponse setStartElement(InstantType value) { 389 this.start = value; 390 return this; 391 } 392 393 /** 394 * @return Date/Time that the appointment is to take place, or requested new start time. 395 */ 396 public Date getStart() { 397 return this.start == null ? null : this.start.getValue(); 398 } 399 400 /** 401 * @param value Date/Time that the appointment is to take place, or requested new start time. 402 */ 403 public AppointmentResponse setStart(Date value) { 404 if (value == null) 405 this.start = null; 406 else { 407 if (this.start == null) 408 this.start = new InstantType(); 409 this.start.setValue(value); 410 } 411 return this; 412 } 413 414 /** 415 * @return {@link #end} (This may be either the same as the appointment request to confirm the details of the appointment, or alternately a new time to request a re-negotiation of the end time.). This is the underlying object with id, value and extensions. The accessor "getEnd" gives direct access to the value 416 */ 417 public InstantType getEndElement() { 418 if (this.end == null) 419 if (Configuration.errorOnAutoCreate()) 420 throw new Error("Attempt to auto-create AppointmentResponse.end"); 421 else if (Configuration.doAutoCreate()) 422 this.end = new InstantType(); // bb 423 return this.end; 424 } 425 426 public boolean hasEndElement() { 427 return this.end != null && !this.end.isEmpty(); 428 } 429 430 public boolean hasEnd() { 431 return this.end != null && !this.end.isEmpty(); 432 } 433 434 /** 435 * @param value {@link #end} (This may be either the same as the appointment request to confirm the details of the appointment, or alternately a new time to request a re-negotiation of the end time.). This is the underlying object with id, value and extensions. The accessor "getEnd" gives direct access to the value 436 */ 437 public AppointmentResponse setEndElement(InstantType value) { 438 this.end = value; 439 return this; 440 } 441 442 /** 443 * @return This may be either the same as the appointment request to confirm the details of the appointment, or alternately a new time to request a re-negotiation of the end time. 444 */ 445 public Date getEnd() { 446 return this.end == null ? null : this.end.getValue(); 447 } 448 449 /** 450 * @param value This may be either the same as the appointment request to confirm the details of the appointment, or alternately a new time to request a re-negotiation of the end time. 451 */ 452 public AppointmentResponse setEnd(Date value) { 453 if (value == null) 454 this.end = null; 455 else { 456 if (this.end == null) 457 this.end = new InstantType(); 458 this.end.setValue(value); 459 } 460 return this; 461 } 462 463 /** 464 * @return {@link #participantType} (Role of participant in the appointment.) 465 */ 466 public List<CodeableConcept> getParticipantType() { 467 if (this.participantType == null) 468 this.participantType = new ArrayList<CodeableConcept>(); 469 return this.participantType; 470 } 471 472 /** 473 * @return Returns a reference to <code>this</code> for easy method chaining 474 */ 475 public AppointmentResponse setParticipantType(List<CodeableConcept> theParticipantType) { 476 this.participantType = theParticipantType; 477 return this; 478 } 479 480 public boolean hasParticipantType() { 481 if (this.participantType == null) 482 return false; 483 for (CodeableConcept item : this.participantType) 484 if (!item.isEmpty()) 485 return true; 486 return false; 487 } 488 489 public CodeableConcept addParticipantType() { //3 490 CodeableConcept t = new CodeableConcept(); 491 if (this.participantType == null) 492 this.participantType = new ArrayList<CodeableConcept>(); 493 this.participantType.add(t); 494 return t; 495 } 496 497 public AppointmentResponse addParticipantType(CodeableConcept t) { //3 498 if (t == null) 499 return this; 500 if (this.participantType == null) 501 this.participantType = new ArrayList<CodeableConcept>(); 502 this.participantType.add(t); 503 return this; 504 } 505 506 /** 507 * @return The first repetition of repeating field {@link #participantType}, creating it if it does not already exist 508 */ 509 public CodeableConcept getParticipantTypeFirstRep() { 510 if (getParticipantType().isEmpty()) { 511 addParticipantType(); 512 } 513 return getParticipantType().get(0); 514 } 515 516 /** 517 * @return {@link #actor} (A Person, Location/HealthcareService or Device that is participating in the appointment.) 518 */ 519 public Reference getActor() { 520 if (this.actor == null) 521 if (Configuration.errorOnAutoCreate()) 522 throw new Error("Attempt to auto-create AppointmentResponse.actor"); 523 else if (Configuration.doAutoCreate()) 524 this.actor = new Reference(); // cc 525 return this.actor; 526 } 527 528 public boolean hasActor() { 529 return this.actor != null && !this.actor.isEmpty(); 530 } 531 532 /** 533 * @param value {@link #actor} (A Person, Location/HealthcareService or Device that is participating in the appointment.) 534 */ 535 public AppointmentResponse setActor(Reference value) { 536 this.actor = value; 537 return this; 538 } 539 540 /** 541 * @return {@link #actor} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A Person, Location/HealthcareService or Device that is participating in the appointment.) 542 */ 543 public Resource getActorTarget() { 544 return this.actorTarget; 545 } 546 547 /** 548 * @param value {@link #actor} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A Person, Location/HealthcareService or Device that is participating in the appointment.) 549 */ 550 public AppointmentResponse setActorTarget(Resource value) { 551 this.actorTarget = value; 552 return this; 553 } 554 555 /** 556 * @return {@link #participantStatus} (Participation status of the participant. When the status is declined or tentative if the start/end times are different to the appointment, then these times should be interpreted as a requested time change. When the status is accepted, the times can either be the time of the appointment (as a confirmation of the time) or can be empty.). This is the underlying object with id, value and extensions. The accessor "getParticipantStatus" gives direct access to the value 557 */ 558 public Enumeration<ParticipantStatus> getParticipantStatusElement() { 559 if (this.participantStatus == null) 560 if (Configuration.errorOnAutoCreate()) 561 throw new Error("Attempt to auto-create AppointmentResponse.participantStatus"); 562 else if (Configuration.doAutoCreate()) 563 this.participantStatus = new Enumeration<ParticipantStatus>(new ParticipantStatusEnumFactory()); // bb 564 return this.participantStatus; 565 } 566 567 public boolean hasParticipantStatusElement() { 568 return this.participantStatus != null && !this.participantStatus.isEmpty(); 569 } 570 571 public boolean hasParticipantStatus() { 572 return this.participantStatus != null && !this.participantStatus.isEmpty(); 573 } 574 575 /** 576 * @param value {@link #participantStatus} (Participation status of the participant. When the status is declined or tentative if the start/end times are different to the appointment, then these times should be interpreted as a requested time change. When the status is accepted, the times can either be the time of the appointment (as a confirmation of the time) or can be empty.). This is the underlying object with id, value and extensions. The accessor "getParticipantStatus" gives direct access to the value 577 */ 578 public AppointmentResponse setParticipantStatusElement(Enumeration<ParticipantStatus> value) { 579 this.participantStatus = value; 580 return this; 581 } 582 583 /** 584 * @return Participation status of the participant. When the status is declined or tentative if the start/end times are different to the appointment, then these times should be interpreted as a requested time change. When the status is accepted, the times can either be the time of the appointment (as a confirmation of the time) or can be empty. 585 */ 586 public ParticipantStatus getParticipantStatus() { 587 return this.participantStatus == null ? null : this.participantStatus.getValue(); 588 } 589 590 /** 591 * @param value Participation status of the participant. When the status is declined or tentative if the start/end times are different to the appointment, then these times should be interpreted as a requested time change. When the status is accepted, the times can either be the time of the appointment (as a confirmation of the time) or can be empty. 592 */ 593 public AppointmentResponse setParticipantStatus(ParticipantStatus value) { 594 if (this.participantStatus == null) 595 this.participantStatus = new Enumeration<ParticipantStatus>(new ParticipantStatusEnumFactory()); 596 this.participantStatus.setValue(value); 597 return this; 598 } 599 600 /** 601 * @return {@link #comment} (Additional comments about the appointment.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 602 */ 603 public StringType getCommentElement() { 604 if (this.comment == null) 605 if (Configuration.errorOnAutoCreate()) 606 throw new Error("Attempt to auto-create AppointmentResponse.comment"); 607 else if (Configuration.doAutoCreate()) 608 this.comment = new StringType(); // bb 609 return this.comment; 610 } 611 612 public boolean hasCommentElement() { 613 return this.comment != null && !this.comment.isEmpty(); 614 } 615 616 public boolean hasComment() { 617 return this.comment != null && !this.comment.isEmpty(); 618 } 619 620 /** 621 * @param value {@link #comment} (Additional comments about the appointment.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 622 */ 623 public AppointmentResponse setCommentElement(StringType value) { 624 this.comment = value; 625 return this; 626 } 627 628 /** 629 * @return Additional comments about the appointment. 630 */ 631 public String getComment() { 632 return this.comment == null ? null : this.comment.getValue(); 633 } 634 635 /** 636 * @param value Additional comments about the appointment. 637 */ 638 public AppointmentResponse setComment(String value) { 639 if (Utilities.noString(value)) 640 this.comment = null; 641 else { 642 if (this.comment == null) 643 this.comment = new StringType(); 644 this.comment.setValue(value); 645 } 646 return this; 647 } 648 649 protected void listChildren(List<Property> children) { 650 super.listChildren(children); 651 children.add(new Property("identifier", "Identifier", "This records identifiers associated with this appointment response concern that are defined by business processes and/ or used to refer to it when a direct URL reference to the resource itself is not appropriate.", 0, java.lang.Integer.MAX_VALUE, identifier)); 652 children.add(new Property("appointment", "Reference(Appointment)", "Appointment that this response is replying to.", 0, 1, appointment)); 653 children.add(new Property("start", "instant", "Date/Time that the appointment is to take place, or requested new start time.", 0, 1, start)); 654 children.add(new Property("end", "instant", "This may be either the same as the appointment request to confirm the details of the appointment, or alternately a new time to request a re-negotiation of the end time.", 0, 1, end)); 655 children.add(new Property("participantType", "CodeableConcept", "Role of participant in the appointment.", 0, java.lang.Integer.MAX_VALUE, participantType)); 656 children.add(new Property("actor", "Reference(Patient|Practitioner|RelatedPerson|Device|HealthcareService|Location)", "A Person, Location/HealthcareService or Device that is participating in the appointment.", 0, 1, actor)); 657 children.add(new Property("participantStatus", "code", "Participation status of the participant. When the status is declined or tentative if the start/end times are different to the appointment, then these times should be interpreted as a requested time change. When the status is accepted, the times can either be the time of the appointment (as a confirmation of the time) or can be empty.", 0, 1, participantStatus)); 658 children.add(new Property("comment", "string", "Additional comments about the appointment.", 0, 1, comment)); 659 } 660 661 @Override 662 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 663 switch (_hash) { 664 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "This records identifiers associated with this appointment response concern that are defined by business processes and/ or used to refer to it when a direct URL reference to the resource itself is not appropriate.", 0, java.lang.Integer.MAX_VALUE, identifier); 665 case -1474995297: /*appointment*/ return new Property("appointment", "Reference(Appointment)", "Appointment that this response is replying to.", 0, 1, appointment); 666 case 109757538: /*start*/ return new Property("start", "instant", "Date/Time that the appointment is to take place, or requested new start time.", 0, 1, start); 667 case 100571: /*end*/ return new Property("end", "instant", "This may be either the same as the appointment request to confirm the details of the appointment, or alternately a new time to request a re-negotiation of the end time.", 0, 1, end); 668 case 841294093: /*participantType*/ return new Property("participantType", "CodeableConcept", "Role of participant in the appointment.", 0, java.lang.Integer.MAX_VALUE, participantType); 669 case 92645877: /*actor*/ return new Property("actor", "Reference(Patient|Practitioner|RelatedPerson|Device|HealthcareService|Location)", "A Person, Location/HealthcareService or Device that is participating in the appointment.", 0, 1, actor); 670 case 996096261: /*participantStatus*/ return new Property("participantStatus", "code", "Participation status of the participant. When the status is declined or tentative if the start/end times are different to the appointment, then these times should be interpreted as a requested time change. When the status is accepted, the times can either be the time of the appointment (as a confirmation of the time) or can be empty.", 0, 1, participantStatus); 671 case 950398559: /*comment*/ return new Property("comment", "string", "Additional comments about the appointment.", 0, 1, comment); 672 default: return super.getNamedProperty(_hash, _name, _checkValid); 673 } 674 675 } 676 677 @Override 678 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 679 switch (hash) { 680 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 681 case -1474995297: /*appointment*/ return this.appointment == null ? new Base[0] : new Base[] {this.appointment}; // Reference 682 case 109757538: /*start*/ return this.start == null ? new Base[0] : new Base[] {this.start}; // InstantType 683 case 100571: /*end*/ return this.end == null ? new Base[0] : new Base[] {this.end}; // InstantType 684 case 841294093: /*participantType*/ return this.participantType == null ? new Base[0] : this.participantType.toArray(new Base[this.participantType.size()]); // CodeableConcept 685 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // Reference 686 case 996096261: /*participantStatus*/ return this.participantStatus == null ? new Base[0] : new Base[] {this.participantStatus}; // Enumeration<ParticipantStatus> 687 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // StringType 688 default: return super.getProperty(hash, name, checkValid); 689 } 690 691 } 692 693 @Override 694 public Base setProperty(int hash, String name, Base value) throws FHIRException { 695 switch (hash) { 696 case -1618432855: // identifier 697 this.getIdentifier().add(castToIdentifier(value)); // Identifier 698 return value; 699 case -1474995297: // appointment 700 this.appointment = castToReference(value); // Reference 701 return value; 702 case 109757538: // start 703 this.start = castToInstant(value); // InstantType 704 return value; 705 case 100571: // end 706 this.end = castToInstant(value); // InstantType 707 return value; 708 case 841294093: // participantType 709 this.getParticipantType().add(castToCodeableConcept(value)); // CodeableConcept 710 return value; 711 case 92645877: // actor 712 this.actor = castToReference(value); // Reference 713 return value; 714 case 996096261: // participantStatus 715 value = new ParticipantStatusEnumFactory().fromType(castToCode(value)); 716 this.participantStatus = (Enumeration) value; // Enumeration<ParticipantStatus> 717 return value; 718 case 950398559: // comment 719 this.comment = castToString(value); // StringType 720 return value; 721 default: return super.setProperty(hash, name, value); 722 } 723 724 } 725 726 @Override 727 public Base setProperty(String name, Base value) throws FHIRException { 728 if (name.equals("identifier")) { 729 this.getIdentifier().add(castToIdentifier(value)); 730 } else if (name.equals("appointment")) { 731 this.appointment = castToReference(value); // Reference 732 } else if (name.equals("start")) { 733 this.start = castToInstant(value); // InstantType 734 } else if (name.equals("end")) { 735 this.end = castToInstant(value); // InstantType 736 } else if (name.equals("participantType")) { 737 this.getParticipantType().add(castToCodeableConcept(value)); 738 } else if (name.equals("actor")) { 739 this.actor = castToReference(value); // Reference 740 } else if (name.equals("participantStatus")) { 741 value = new ParticipantStatusEnumFactory().fromType(castToCode(value)); 742 this.participantStatus = (Enumeration) value; // Enumeration<ParticipantStatus> 743 } else if (name.equals("comment")) { 744 this.comment = castToString(value); // StringType 745 } else 746 return super.setProperty(name, value); 747 return value; 748 } 749 750 @Override 751 public Base makeProperty(int hash, String name) throws FHIRException { 752 switch (hash) { 753 case -1618432855: return addIdentifier(); 754 case -1474995297: return getAppointment(); 755 case 109757538: return getStartElement(); 756 case 100571: return getEndElement(); 757 case 841294093: return addParticipantType(); 758 case 92645877: return getActor(); 759 case 996096261: return getParticipantStatusElement(); 760 case 950398559: return getCommentElement(); 761 default: return super.makeProperty(hash, name); 762 } 763 764 } 765 766 @Override 767 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 768 switch (hash) { 769 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 770 case -1474995297: /*appointment*/ return new String[] {"Reference"}; 771 case 109757538: /*start*/ return new String[] {"instant"}; 772 case 100571: /*end*/ return new String[] {"instant"}; 773 case 841294093: /*participantType*/ return new String[] {"CodeableConcept"}; 774 case 92645877: /*actor*/ return new String[] {"Reference"}; 775 case 996096261: /*participantStatus*/ return new String[] {"code"}; 776 case 950398559: /*comment*/ return new String[] {"string"}; 777 default: return super.getTypesForProperty(hash, name); 778 } 779 780 } 781 782 @Override 783 public Base addChild(String name) throws FHIRException { 784 if (name.equals("identifier")) { 785 return addIdentifier(); 786 } 787 else if (name.equals("appointment")) { 788 this.appointment = new Reference(); 789 return this.appointment; 790 } 791 else if (name.equals("start")) { 792 throw new FHIRException("Cannot call addChild on a singleton property AppointmentResponse.start"); 793 } 794 else if (name.equals("end")) { 795 throw new FHIRException("Cannot call addChild on a singleton property AppointmentResponse.end"); 796 } 797 else if (name.equals("participantType")) { 798 return addParticipantType(); 799 } 800 else if (name.equals("actor")) { 801 this.actor = new Reference(); 802 return this.actor; 803 } 804 else if (name.equals("participantStatus")) { 805 throw new FHIRException("Cannot call addChild on a singleton property AppointmentResponse.participantStatus"); 806 } 807 else if (name.equals("comment")) { 808 throw new FHIRException("Cannot call addChild on a singleton property AppointmentResponse.comment"); 809 } 810 else 811 return super.addChild(name); 812 } 813 814 public String fhirType() { 815 return "AppointmentResponse"; 816 817 } 818 819 public AppointmentResponse copy() { 820 AppointmentResponse dst = new AppointmentResponse(); 821 copyValues(dst); 822 if (identifier != null) { 823 dst.identifier = new ArrayList<Identifier>(); 824 for (Identifier i : identifier) 825 dst.identifier.add(i.copy()); 826 }; 827 dst.appointment = appointment == null ? null : appointment.copy(); 828 dst.start = start == null ? null : start.copy(); 829 dst.end = end == null ? null : end.copy(); 830 if (participantType != null) { 831 dst.participantType = new ArrayList<CodeableConcept>(); 832 for (CodeableConcept i : participantType) 833 dst.participantType.add(i.copy()); 834 }; 835 dst.actor = actor == null ? null : actor.copy(); 836 dst.participantStatus = participantStatus == null ? null : participantStatus.copy(); 837 dst.comment = comment == null ? null : comment.copy(); 838 return dst; 839 } 840 841 protected AppointmentResponse typedCopy() { 842 return copy(); 843 } 844 845 @Override 846 public boolean equalsDeep(Base other_) { 847 if (!super.equalsDeep(other_)) 848 return false; 849 if (!(other_ instanceof AppointmentResponse)) 850 return false; 851 AppointmentResponse o = (AppointmentResponse) other_; 852 return compareDeep(identifier, o.identifier, true) && compareDeep(appointment, o.appointment, true) 853 && compareDeep(start, o.start, true) && compareDeep(end, o.end, true) && compareDeep(participantType, o.participantType, true) 854 && compareDeep(actor, o.actor, true) && compareDeep(participantStatus, o.participantStatus, true) 855 && compareDeep(comment, o.comment, true); 856 } 857 858 @Override 859 public boolean equalsShallow(Base other_) { 860 if (!super.equalsShallow(other_)) 861 return false; 862 if (!(other_ instanceof AppointmentResponse)) 863 return false; 864 AppointmentResponse o = (AppointmentResponse) other_; 865 return compareValues(start, o.start, true) && compareValues(end, o.end, true) && compareValues(participantStatus, o.participantStatus, true) 866 && compareValues(comment, o.comment, true); 867 } 868 869 public boolean isEmpty() { 870 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, appointment, start 871 , end, participantType, actor, participantStatus, comment); 872 } 873 874 @Override 875 public ResourceType getResourceType() { 876 return ResourceType.AppointmentResponse; 877 } 878 879 /** 880 * Search parameter: <b>actor</b> 881 * <p> 882 * Description: <b>The Person, Location/HealthcareService or Device that this appointment response replies for</b><br> 883 * Type: <b>reference</b><br> 884 * Path: <b>AppointmentResponse.actor</b><br> 885 * </p> 886 */ 887 @SearchParamDefinition(name="actor", path="AppointmentResponse.actor", description="The Person, Location/HealthcareService or Device that this appointment response replies for", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Device.class, HealthcareService.class, Location.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 888 public static final String SP_ACTOR = "actor"; 889 /** 890 * <b>Fluent Client</b> search parameter constant for <b>actor</b> 891 * <p> 892 * Description: <b>The Person, Location/HealthcareService or Device that this appointment response replies for</b><br> 893 * Type: <b>reference</b><br> 894 * Path: <b>AppointmentResponse.actor</b><br> 895 * </p> 896 */ 897 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ACTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ACTOR); 898 899/** 900 * Constant for fluent queries to be used to add include statements. Specifies 901 * the path value of "<b>AppointmentResponse:actor</b>". 902 */ 903 public static final ca.uhn.fhir.model.api.Include INCLUDE_ACTOR = new ca.uhn.fhir.model.api.Include("AppointmentResponse:actor").toLocked(); 904 905 /** 906 * Search parameter: <b>identifier</b> 907 * <p> 908 * Description: <b>An Identifier in this appointment response</b><br> 909 * Type: <b>token</b><br> 910 * Path: <b>AppointmentResponse.identifier</b><br> 911 * </p> 912 */ 913 @SearchParamDefinition(name="identifier", path="AppointmentResponse.identifier", description="An Identifier in this appointment response", type="token" ) 914 public static final String SP_IDENTIFIER = "identifier"; 915 /** 916 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 917 * <p> 918 * Description: <b>An Identifier in this appointment response</b><br> 919 * Type: <b>token</b><br> 920 * Path: <b>AppointmentResponse.identifier</b><br> 921 * </p> 922 */ 923 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 924 925 /** 926 * Search parameter: <b>practitioner</b> 927 * <p> 928 * Description: <b>This Response is for this Practitioner</b><br> 929 * Type: <b>reference</b><br> 930 * Path: <b>AppointmentResponse.actor</b><br> 931 * </p> 932 */ 933 @SearchParamDefinition(name="practitioner", path="AppointmentResponse.actor", description="This Response is for this Practitioner", type="reference", target={Practitioner.class } ) 934 public static final String SP_PRACTITIONER = "practitioner"; 935 /** 936 * <b>Fluent Client</b> search parameter constant for <b>practitioner</b> 937 * <p> 938 * Description: <b>This Response is for this Practitioner</b><br> 939 * Type: <b>reference</b><br> 940 * Path: <b>AppointmentResponse.actor</b><br> 941 * </p> 942 */ 943 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRACTITIONER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PRACTITIONER); 944 945/** 946 * Constant for fluent queries to be used to add include statements. Specifies 947 * the path value of "<b>AppointmentResponse:practitioner</b>". 948 */ 949 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRACTITIONER = new ca.uhn.fhir.model.api.Include("AppointmentResponse:practitioner").toLocked(); 950 951 /** 952 * Search parameter: <b>part-status</b> 953 * <p> 954 * Description: <b>The participants acceptance status for this appointment</b><br> 955 * Type: <b>token</b><br> 956 * Path: <b>AppointmentResponse.participantStatus</b><br> 957 * </p> 958 */ 959 @SearchParamDefinition(name="part-status", path="AppointmentResponse.participantStatus", description="The participants acceptance status for this appointment", type="token" ) 960 public static final String SP_PART_STATUS = "part-status"; 961 /** 962 * <b>Fluent Client</b> search parameter constant for <b>part-status</b> 963 * <p> 964 * Description: <b>The participants acceptance status for this appointment</b><br> 965 * Type: <b>token</b><br> 966 * Path: <b>AppointmentResponse.participantStatus</b><br> 967 * </p> 968 */ 969 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PART_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PART_STATUS); 970 971 /** 972 * Search parameter: <b>patient</b> 973 * <p> 974 * Description: <b>This Response is for this Patient</b><br> 975 * Type: <b>reference</b><br> 976 * Path: <b>AppointmentResponse.actor</b><br> 977 * </p> 978 */ 979 @SearchParamDefinition(name="patient", path="AppointmentResponse.actor", description="This Response is for this Patient", type="reference", target={Patient.class } ) 980 public static final String SP_PATIENT = "patient"; 981 /** 982 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 983 * <p> 984 * Description: <b>This Response is for this Patient</b><br> 985 * Type: <b>reference</b><br> 986 * Path: <b>AppointmentResponse.actor</b><br> 987 * </p> 988 */ 989 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 990 991/** 992 * Constant for fluent queries to be used to add include statements. Specifies 993 * the path value of "<b>AppointmentResponse:patient</b>". 994 */ 995 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("AppointmentResponse:patient").toLocked(); 996 997 /** 998 * Search parameter: <b>appointment</b> 999 * <p> 1000 * Description: <b>The appointment that the response is attached to</b><br> 1001 * Type: <b>reference</b><br> 1002 * Path: <b>AppointmentResponse.appointment</b><br> 1003 * </p> 1004 */ 1005 @SearchParamDefinition(name="appointment", path="AppointmentResponse.appointment", description="The appointment that the response is attached to", type="reference", target={Appointment.class } ) 1006 public static final String SP_APPOINTMENT = "appointment"; 1007 /** 1008 * <b>Fluent Client</b> search parameter constant for <b>appointment</b> 1009 * <p> 1010 * Description: <b>The appointment that the response is attached to</b><br> 1011 * Type: <b>reference</b><br> 1012 * Path: <b>AppointmentResponse.appointment</b><br> 1013 * </p> 1014 */ 1015 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam APPOINTMENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_APPOINTMENT); 1016 1017/** 1018 * Constant for fluent queries to be used to add include statements. Specifies 1019 * the path value of "<b>AppointmentResponse:appointment</b>". 1020 */ 1021 public static final ca.uhn.fhir.model.api.Include INCLUDE_APPOINTMENT = new ca.uhn.fhir.model.api.Include("AppointmentResponse:appointment").toLocked(); 1022 1023 /** 1024 * Search parameter: <b>location</b> 1025 * <p> 1026 * Description: <b>This Response is for this Location</b><br> 1027 * Type: <b>reference</b><br> 1028 * Path: <b>AppointmentResponse.actor</b><br> 1029 * </p> 1030 */ 1031 @SearchParamDefinition(name="location", path="AppointmentResponse.actor", description="This Response is for this Location", type="reference", target={Location.class } ) 1032 public static final String SP_LOCATION = "location"; 1033 /** 1034 * <b>Fluent Client</b> search parameter constant for <b>location</b> 1035 * <p> 1036 * Description: <b>This Response is for this Location</b><br> 1037 * Type: <b>reference</b><br> 1038 * Path: <b>AppointmentResponse.actor</b><br> 1039 * </p> 1040 */ 1041 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_LOCATION); 1042 1043/** 1044 * Constant for fluent queries to be used to add include statements. Specifies 1045 * the path value of "<b>AppointmentResponse:location</b>". 1046 */ 1047 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include("AppointmentResponse:location").toLocked(); 1048 1049 1050}